-
Notifications
You must be signed in to change notification settings - Fork 270
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Is there an option to add encoding
field to the requestBody
?
#714
Comments
Hi @marcintabaka, so the short answer is no. It is currently not possible as we do not expose the encoding object and give only limited access to the media type object. When I read the spec correctly, it can occur in both request and response. The problem is that we kind of compress down these objects to make OpenAPI more accessible. The added convenience limits the flexibility though. We do have something called Your use-case is absolutely valid, but at this point the question is whether this functionality warrants making the interface more complicated. I'm undecided because spectacular has been used a lot by a lot of people and no one ever asked about the encoding object. |
@tfranzel thank you for your quick reply. What do you think about adding some global setting flag, to change Swagger UI behavior in this regard? Simply if someone set this flag to True, then for every array type field, drf-spectacular will add this part to schema:
|
I don't think a global setting is the right way. It is too coarse and also since it's bound to the field name, unwanted collisions might occur. It would be impossible to use this only selectively. |
I'm also interested in getting access to the encoding property. My use is case is being able to specify particular image types
|
It will be easier to explain the problem on the example.
I have models with Many To Many relationship:
And simple serializer with
PrimaryKeyRelatedField
(withoutread_only
, so we can read and write m2m relation) :Then simple ViewSet:
And Router:
Let's assume that I have an Article with two tags:
Response body:
Now I would like to add third tag to the article, so I would use PATCH. I use Swagger UI with Content-Type set to
application/json
, and get something like thisResponse Body:
Everything works fine so far.
There is a problem, when I would like to use
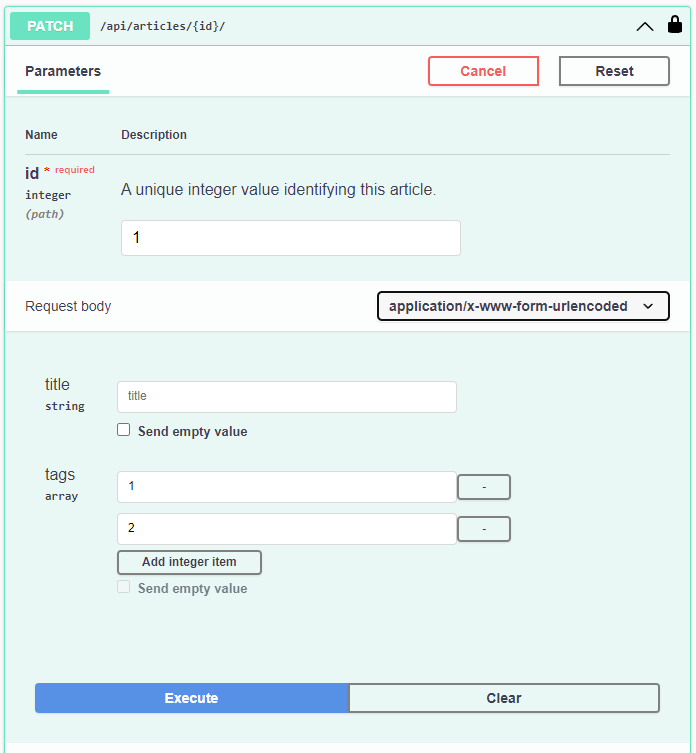
application/x-www-form-urlencoded
ormultipart/form-data
content type in Swagger UI:The form looks ok, but generated Curl Request looks like this:
Django Rest Framework doesn't accept parameters in that form
tags=1,2
, so the Response is:Swagger UI should generate request like this:
Then it will be accepted by the API.
To achieve this (generating such format of request by Swagger UI), we need to add extra field
encoding
to OpenAPI schema: https://github.com/OAI/OpenAPI-Specification/blob/main/versions/3.0.3.md#encoding-objectExample of currently generated OpenAPI file:
Example of expected OpenAPI file:
The part:
Is the key.
Do you know if it is possible to add this part using
drf-spectacular
?The text was updated successfully, but these errors were encountered: