Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
this PR handle #153, #154 and also propose a tool/workaround for #161
API Changes
Defining + Using fonts
The example above miss to define a variant!
Defining variants (required)
Compatibility
Previous ways of using msdf fonts are still available as being not recommended.
This will automatically; create new FontFamily, retrieve existing FontFamily, create new FontVariant(MSDF ONLY), retrieve existing variant according to context
Preloading fonts
1 Recommended way : Project side loading
If you are building a production application, chances are high that you'll already have some kind of asset loader, loader queue, loader sequences, etc... USE IT then add variants with your loaded assets.
2 FontLibrary.load() Async
Rely FontLibrary.load() which return a Promise
3 FontLibrary.load() Sync
Rely FontLibrary.load() which return a Promise that can be await
Under-the-hood
This FontLibrary FontClass proposal highly rely on classes, interfaces and abstract (typescript should be the way).
Note that this is under-the-hood, and users won't have to deal with it
FontFamily
Reference a fontFamily(string) that can be used to set
.fontFamily
of anyMeshUIComponent
.A FontFamily holds variants
Array.<FontVariant>
which really are the fonts.FontVariant
FontVariant
class is abstract.TextTypes are now handled via
FontVariant
class extension.MSDFFontVariant
is the default text-type implementation implemented.FontFamily::addFontVariant()
currently always goes for msdf implementation.FontFamily::addFontVariant(variant:FontVariant)
is the way to create a variant that is not msdf.FontVariant
holds.font
asTypographyFont
which is the static informations of the font : lineHeight, lineBase, sizeFontVariant
holds._chars
asArray.<TypographyCharacter>
which are the static information of each character in that font variant.ThreeMeshUI.Text
Text
holds.content
asstring
which is the string representationText
holds._textContent
asstring
which is.content
after being whitespaced (according to .whiteSpace property)Text
holds._textContentGlyphs
asArray.<TypographyCharacter>
which is the mapping of._textContent
in TypographyCharacter. This should be only rebuilt when content, font (fontWeight/fontStyle) or whitespace change.Text
holds._textContentInlines
asArray.<InlineCharacter>
which is the contextual mapping of._textContentGlyphs
in InlineCharacter. Contextual is : String Sequence(kerning), fontSize,Character Adjustement
As described in under-the-hood, font are highly OOP and lots of pointers are shared. It was therefore not hard to provide a way to tweak / fine-tune glyph alignment issue #161
Development example is already prepared
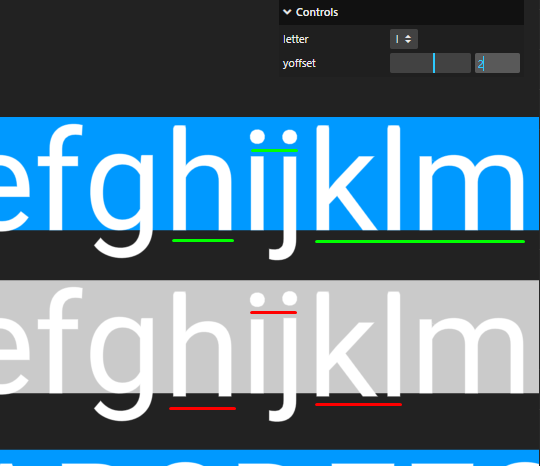
And a codesandbox with ability to load custom fonts might also be on the way
Next