-
Notifications
You must be signed in to change notification settings - Fork 1.8k
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
fix( radio-tile, selectable-tile): deprecates iconDescription and adds handleOnChange function #4966
Merged
Merged
fix( radio-tile, selectable-tile): deprecates iconDescription and adds handleOnChange function #4966
Changes from all commits
Commits
Show all changes
5 commits
Select commit
Hold shift + click to select a range
d4a9bf0
fix(radio-tile,tile): refactor radio tile to fn and add onChange to tile
abbeyhrt 0ba84bc
chore(tile): removes default prop for deprecated icon description
abbeyhrt b83b64e
Merge branch 'master' into fix-tiles-VO
joshblack 1f5e5e2
Merge branch 'master' into fix-tiles-VO
joshblack c91f70b
Merge branch 'master' into fix-tiles-VO
joshblack File filter
Filter by extension
Conversations
Failed to load comments.
Loading
Jump to
Jump to file
Failed to load files.
Loading
Diff view
Diff view
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Instead of ignoring
no-unused-vars
here, we can safely removeiconDescription
here?There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
I tried that but it seemed to cause this warning to pop up if iconDescription is defined:
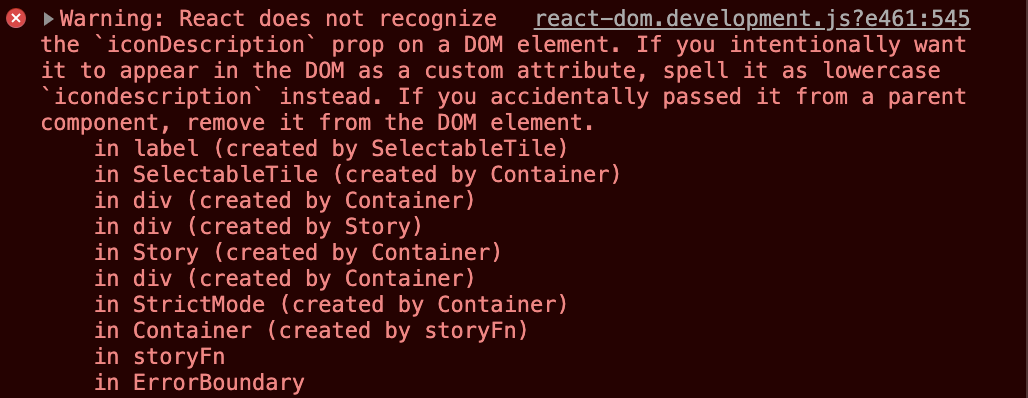
Seems like if folks are still using
iconDescription
the prop will be spread onto...other
and cause the warning?There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Though this has been merged already - You are right. I don't see it's a problem, though, given it's a React warning that doesn't prevent user applications from working, and they can simply remove
iconDescription
to get rid of the warning.