diff --git a/README.md b/README.md
index e69de29..a69dfe5 100644
--- a/README.md
+++ b/README.md
@@ -0,0 +1,1190 @@
+> This is designed to be an all-encompassing guide to essential principles of ReactJS. We will walk through the basics first then scaffold to more complex examples, such as isomorphic ReactJS components. If you following this guide closely and try out every example, this will be the last tutorial you will ever need for ReactJS.
+
+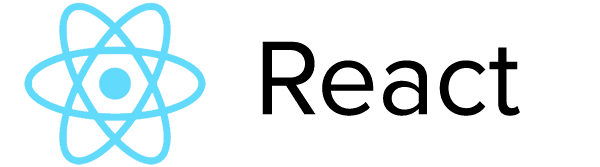
+
+Bookmark this page for the entirety of your ReactJS career: [GitHub Repository](https://github.com/stanleycyang/react-bible-boilerplate).
+
+Want to make this guide better? View the [issues](https://github.com/stanleycyang/react-bible-boilerplate/issues)! [Pull requests](https://github.com/stanleycyang/react-bible-boilerplate/pulls) are welcomed!
+
+### ReactJS Intro
+
+[](http://www.youtube.com/watch?v= lbmurKlQ3Ac)
+
+Completion time: **3 hours**
+
+In this guide, you will learn:
+
+- Fundamental ReactJS concepts
+- How to build ES2015 components
+- The component lifecycle
+- Difference between state vs. props
+- Building a client-side React app
+- Build an isomorphic React app
+
+I recommend you break this read into bite-sized sections to better digest the content. Now let's get into it.
+
+## What is ReactJS?
+
+Simply put, it is a JavaScript library for building User Interfaces **(UI)** created and maintained by Facebook.
+
+ReactJS makes no assumptions about your technology stack, so you can use ReactJS to:
+
+- Build a widget
+- Add a reusable component (header, footer, etc.)
+- Build the entire front-end experience (like Facebook)
+
+Just to reiterate, you can incorporate ReactJS into different types of front-end tech stacks (AngularJS, Backbone, etc.) or you can choose to build entire applications out of ReactJS!
+
+## The Virtual DOM
+
+You are going to hear this term all the time. Simply put, ReactJS builds an abstract version of the DOM. ReactJS observes when the data changes, then it will find the difference and re-render what needs to be changed. What makes it fast is:
+
+- Efficient diff algorithms
+- Efficient update of sub-tree only
+
+Instead of traditional HTML:
+
+```html
+...
+
+
+
This is a header
+
+...
+
+```
+
+You will see Component-based programming:
+
+```js
+// Import essentials
+import React, { Component } from 'react'
+import { render } from 'react-dom'
+
+// A basic React Component
+class Header extends Component {
+ render() {
+ return (
+ {/* JSX code */}
+
+
This is a reusable header
+
+ )
+ }
+}
+
+// Render the Component onto the page
+render(, mountNode)
+```
+
+In the above example, you will notice that it uses an XML-like syntax to simulate the DOM. This is called **JSX**, a preprocessor step that allows an elegant way to write ReactJS component. Just like XML, JSX tags will have tag names, attributes, and children.
+
+Using the Virtual DOM provides:
+
+- A simpler programming model
+- Better performance
+- Server-side rendering
+- Modular, reusable components
+
+For more information, check out [Virtual Dom](https://github.com/Matt-Esch/virtual-dom).
+
+## Unidirectional Data Flow
+
+ReactJS implements a one-way data flow. This means that data is passed from the top-down, through **props**. Data is transferred from the top component to its children, so on and so forth.
+
+```js
+...
+// A simple component flowing down the props
+
+...
+```
+
+Most times, the properties will be explicitly passed down.
+
+```js
+import React, { Component } from 'react'
+import { render } from 'react-dom'
+
+class FancyForm extends Component {
+ // When component is initialized
+ constructor(props) {
+ // Bring in the props. We can now reference `this.props`
+ super(props)
+ }
+ render() {
+ // Use spread operators to break apart the props
+ const { hidden, ...others } = this.props
+ // Determine whether to hide to show div
+ let formClass = hidden ? 'FormHidden' : 'FormShow'
+ return (
+ {/* Put in the class, pass all the other props down
+
+ ...
+ )
+ }
+}
+
+```
+
+We will see this in action in the future examples.
+
+## Getting started
+
+Getting started is ReactJS is easy. I have provided a boilerplate [here](https://github.com/stanleycyang/react-bible-boilerplate).
+
+This [repository](https://github.com/stanleycyang/react-bible-boilerplate) contains:
+
+- The boilerplate
+- The walkthrough
+- All the completed examples
+
+I recommend you **star**, **fork**, or **clone** this repo for your own reference. If you want to follow along, you will need to follow the instructions below:
+
+**Installation:**
+
+```bash
+$ git clone git@github.com:stanleycyang/react-bible-boilerplate.git book-inventory
+$ cd book-inventory
+$ npm install
+```
+
+The boilerplate includes:
+
+- Babel
+- Webpack
+- Express
+- ReactJS
+
+Once you have the boilerplate installed, we can get started building some ReactJS components!
+
+Our **roadmap**:
+
+- Client-side ReactJS
+- Isomorphic ReactJS
+
+## Book Inventory (client-side React)
+
+This is our current directory structure:
+
+ ├── LICENSE
+ ├── README.md
+ ├── bin
+ │ └── www
+ ├── client
+ │ ├── container
+ │ │ └── index.js
+ │ ├── index.js
+ │ ├── polyfills.js
+ │ └── views
+ │ ├── index.jade
+ │ └── layout.jade
+ ├── config
+ │ └── index.js
+ ├── nodemon.json
+ ├── package.json
+ ├── server
+ │ ├── index.js
+ │ └── routes
+ │ └── index.js
+ └── webpack
+ ├── dev.config.js
+ ├── index.js
+ └── prod.config.js
+
+- **client**: contains all client-side code
+- **server**: contains all server-side code
+- **webpack**: our production and development configurations live here
+- **config**: we place all [ENV](http://www.computerhope.com/jargon/e/envivari.htm) variables here
+- **bin**: We place any scripts (`www`, `seed`, etc.) in here
+
+### Starting the Express Server
+
+To start the **Express** server, run in the root of the app directory:
+
+```bash
+$ npm run dev
+$ open http://localhost:3000
+```
+
+This will start a web server at `http://localhost:3000` with **hot reloading** and **nodemon**. This means you can see all the changes in real-time when the files are saved!
+
+### Writing Your First React Component
+
+Let's take a look inside of `client/container/index.js`. Here we will find a very basic React component:
+
+```js
+// client/container/index.js
+
+// Load in React
+import React, { Component, PropTypes } from 'react'
+
+// Create a basic component called App and export the App to use
+export default class App extends Component {
+ render() {
+ return (
+
Hello world! Love, Stanley
+ )
+ }
+}
+```
+
+**Import & Export**
+
+In ReactJS, it is best practice to build apps in a modular fashion. That means you should split up components into different files to better organize it.
+
+You will see `import` and `export` all throughout our examples. This helps us break up code into different pages.
+
+You can see the **destructuring assignment syntax** here:
+
+```js
+import React, { Component, PropTypes } from 'react'
+```
+This lets us extract multiple object properties in a single line. Now we no longer have to prefix them with React (ie. React.PropTypes or React.Component).
+
+For more info, here are the MDN docs:
+
+- [Import](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/import)
+- [Export](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/export)
+
+**Classes**
+
+In **ES2015+**, you can build React Components incredibly easy with [`classes`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Classes).
+
+JavaScripts classes are syntactical sugar over the prototypical inheritance. It allows us to build templates for objects as such:
+
+```js
+class Square {
+ // Constructor gets called when an object is intialized
+ constructor(height, width) {
+ this.height = height;
+ this.width = width;
+ }
+}
+
+// Initialize a new Square
+let Shape = new Square(10, 10)
+```
+
+In React, we use the **extends** keyword to create a class as a child to the React Component.
+
+```js
+class App extends React.Component {
+ render() {
+ return (
+
Hello world! Love, Stanley
+ )
+ }
+}
+```
+
+If you're not familiar with Object-oriented programming with JavaScript, I recommend taking a look at [this](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Introduction_to_Object-Oriented_JavaScript).
+
+Notice how we have a **render()** method? For every React component, the render method is **absolutely required**.
+
+You can **return null or false** to indicate that you don't want to render anything. Behind the scenes, React renders a `