diff --git a/CHANGELOG.md b/CHANGELOG.md
index 2696a72..1fb7471 100644
--- a/CHANGELOG.md
+++ b/CHANGELOG.md
@@ -19,6 +19,8 @@
- `OutlineOpen` without `!`, (and `outline.open`) will now focus in the outline if it is
already open when invoked.
([#71](https://github.com/hedyhli/outline.nvim/issues/71))
+- The `symbols.icon_fetcher` function now supports a second parameter, the code
+ buffer number. Use it to fetch icons based on buffer options such as filetype.
## v1.0.0
diff --git a/README.md b/README.md
index ebf1e00..36d9981 100644
--- a/README.md
+++ b/README.md
@@ -391,6 +391,10 @@ Pass a table to the setup call with your configuration options.
-- You can use a custom function that returns the icon for each symbol kind.
-- This function takes a kind (string) as parameter and should return an
-- icon as string.
+ ---@param kind string
+ ---@param bufnr integer Code buffer
+ ---@returns string|boolean The icon string (key of `icons` table), or `false`
+ --- to fallback to `icon_source`.
icon_fetcher = nil,
-- 3rd party source for fetching icons. Fallback if icon_fetcher returned
-- empty string. Currently supported values: 'lspkind'
@@ -890,6 +894,21 @@ symbols = {
}
```
+ The `icon_fetcher` function may also accept a second parameter, the buffer
+ number of the code buffer. For example, you can use it to determine the icon
+ to use based on the filetype.
+
+```lua
+symbols = {
+ icon_fetcher = function(kind, bufnr)
+ local ft = vim.api.nvim_buf_get_option(bufnr, 'ft')
+ -- ...
+ end,
+}
+```
+
+ See [this section](#custom-icons) for other examples of this function.
+
- You can customize the split command used for creating the outline window split
using `outline_window.split_command`, such as `"topleft vsp"`. See `:h windows`
@@ -1046,7 +1065,7 @@ that simply returns in plain text, the first letter of the given kind.
```lua
symbols = {
- icon_fetcher = function(kind) return kind:sub(1,1) end
+ icon_fetcher = function(kind, bufnr) return kind:sub(1,1) end,
}
```
@@ -1055,13 +1074,24 @@ and `icons` as fallback.
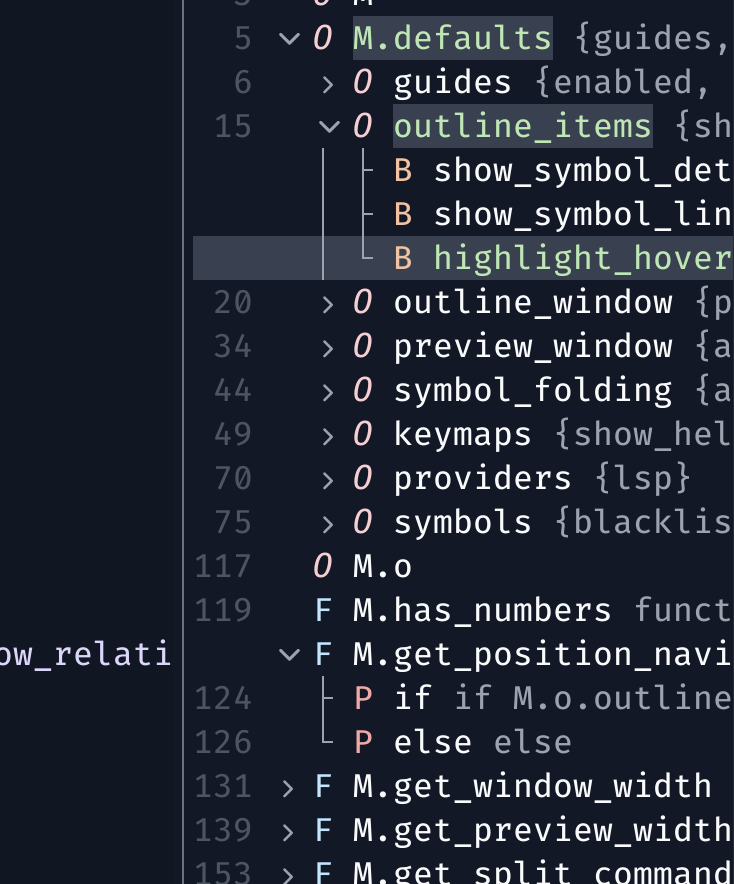
+### Different icons based on filetype
+
+```lua
+symbols = {
+ icon_fetcher = function(kind, bufnr)
+ local ft = vim.api.nvim_buf_get_option(bufnr, 'ft')
+ -- ...
+ end,
+}
+```
+
### Disable icons
Disable all icons:
```lua
symbols = {
- icon_fetcher = function(_) return "" end,
+ icon_fetcher = function() return "" end,
}
```
@@ -1069,7 +1099,7 @@ Disable icons for specific kinds, and for others use lspkind:
```lua
symbols = {
- icon_fetcher = function(k)
+ icon_fetcher = function(k, buf)
if k == 'String' then
return ""
end
@@ -1081,6 +1111,24 @@ symbols = {

+### Disable icons for a specific filetype
+
+In this example, icons are disabled for markdown, and `lspkind` is used for
+other filetypes.
+
+```lua
+symbols = {
+ icon_fetcher = function(k, buf)
+ local ft = vim.api.nvim_buf_get_option(buf, "ft")
+ if ft == 'markdown' then
+ return ""
+ end
+ return false
+ end,
+ icon_source = "lspkind",
+}
+```
+
### Live, editable previews
Press `K` to open the preview, press `K` again to focus on the preview window
diff --git a/lua/outline/parser.lua b/lua/outline/parser.lua
index 6eafad7..3f3415d 100644
--- a/lua/outline/parser.lua
+++ b/lua/outline/parser.lua
@@ -37,7 +37,7 @@ local function parse_result(result, depth, hierarchy, parent, bufnr)
local node = {
deprecated = value.deprecated,
kind = value.kind,
- icon = symbols.icon_from_kind(value.kind),
+ icon = symbols.icon_from_kind(value.kind, bufnr),
name = value.name or value.text,
detail = value.detail,
line = selectionRange.start.line,
diff --git a/lua/outline/symbols.lua b/lua/outline/symbols.lua
index 9547d5d..3f17959 100644
--- a/lua/outline/symbols.lua
+++ b/lua/outline/symbols.lua
@@ -46,7 +46,8 @@ for k, v in pairs(M.kinds) do
end
---@param kind string|integer
-function M.icon_from_kind(kind)
+---@param bufnr integer
+function M.icon_from_kind(kind, bufnr)
local kindstr = kind
if type(kind) ~= 'string' then
kindstr = M.kinds[kind]
@@ -56,7 +57,7 @@ function M.icon_from_kind(kind)
end
if type(cfg.o.symbols.icon_fetcher) == 'function' then
- local icon = cfg.o.symbols.icon_fetcher(kindstr)
+ local icon = cfg.o.symbols.icon_fetcher(kindstr, bufnr)
-- Allow returning empty string
if icon then
return icon