-
Notifications
You must be signed in to change notification settings - Fork 0
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
[2020-04-04] Immutable.js #92
Labels
Comments
const state = {
name: 'Vivi',
age: 18,
}
// 使用相同的 age : 18 去 Shallow-cloning
const a = {...state, age:18}
state.name === a.name // true
state.age === a.age // true |
沒有 Immutable 的概念前 const props = {
id: 1,
list: [1, 2, 3]
}
const list = props.list;
list.push(4)
const nextProps = {
...props,
list // 放入舊的 list
}
props.list === nextProps.list // true 有了 Immutable 的概念 const props = {
id: 1,
list: [1, 2, 3]
}
const nextProps = {
...props,
list:[...props.list, 4] // 做一次 spread operator
}
props.list === nextProps.list // false |
改 points 的值,也是必須從最上層一直複製下去到 points 才能做更改 const state = {
school: {
name: "school",
house: {
name: "room1",
points: {
vip:225
}
},
open:'am7:00'
}
} const newState = {
...state,
school:{
...state.school,
house:{
...state.school.house, points: {
vip:450
}
}
}
} state.school.house.points // {vip: 225}
newState.school.house.points // {vip: 450} |
const state = {
school: {
name: "school",
house: {id:'room1'}
},
open:{am:'7:00'}
} const newState = {
...state, school:{
...state.school, house:{
id:333
}
}
} newState.open === state.open // true
// 第一層的 open ,修改後還是共用記憶體位置
// 對應 spread operator 複製第一層
// 此結果合理
state.school === newState.school // false
// 第一層的 school 在一開始就被給予了一個新的 object 所以記憶體位置就換了 |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Immutable
immutable 是指在創建變數、賦值後便不可改變,若對其有任何變更(例如:新增、修改、刪除),就要回傳一個新值。
對 arr1 進行修改產生 arr2, arr3
why use Immutable
當我們在 redux 或是 react 中使用 immutable 的寫法
其實最主要的目的,是要在資料有異動之後
產生新的記憶體位置
讓 react 判別需要 re-render
重點是 state
redux store 裡的數據,是不可改變的,不能直接對它做編輯
你能做的就只有把整個 state 都換掉
如果 newState === oldState 就不會 re-render
所以才要用 Object.assign({}, state, {age: 25}) 這種方法
而不是直接 state.age = 25; return state
因為後者 newState === oldState 會是 true
重點
沒有變動的地方,就直接使用;有變動的地方就需要 new 一份新的去更改值
--> 實做的時候,有幾種方法可以選擇
Immutable.js
利用結構共享(structural sharing)的方式實作 persistent(持久的)data structure
此篇文章解釋了效能優化與 persistent
Good Morning, Functional JS (Day 24, 使用 Immutable.js)
Immutable.js api
Immutable 資料結構與原生 JS
fromJS()
主要功能就是將你丟給他的 JavaScript 物件或陣列轉換成對應的 Immutable.js 結構
List.of()
listA 並沒有因為 push() 的關係改變了自已原本的結構
List 修改(只有一層)
set(index: number, value)
List 取值(只有一層)
List 內部有包其他 List 時,則需要使用 setIn() 與 getIn()
Map
深度取值與改值也是使用
setIn()
與getIn()
:總結:
更多
Immutable.is();
Value equality check
你需要 immutable.js 嗎?
你需要 immutable.js 嗎?
寫 React 的時候常常聽到 immutable,什麼是 immutable ?
同場加映 Immer
Immutability is Changing: From Immutable.js to Immer - ForwardJS 2019
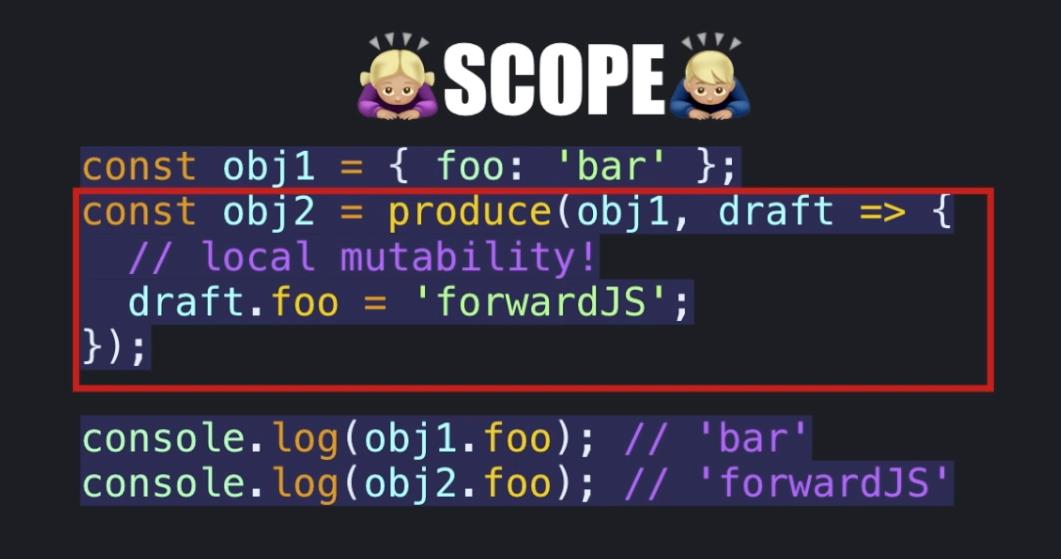
參考文章
Immutable 详解及 React 中实践
Immutable.js 簡介
Immutable.js, persistent data structures and structural sharing
React.js Conf 2015 - Immutable Data and React
Immutability 為何重要?淺談 immutable.js 和 seamless-immutable
深入探究Immutable.js的实现机制(一) 這篇在解釋原理
The text was updated successfully, but these errors were encountered: