-
Notifications
You must be signed in to change notification settings - Fork 4
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Spring boot 入门之Hello World #193
Comments
Another way按照官方的例子,先创建 导入依赖点击 pom.xml 输入如下信息: <parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.3.7.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies> 刷新即可加载 创建 package 和 HelloWorldService.class在 |
Cannot resolve symbol 'java'按照 使用 sdkman 安装并维护多个版本的 JDK 方法,安装JDK8时,提示错误 其实图中已经提示 “JDK corretto-1.8....” 解决方法: |
一、环境搭建
1.1、安装 JDK8
使用
sdkman
安装JDK8
详情请阅读 使用 sdkman 安装并维护多个版本的 JDK
1.2、安装 IDEA
详情请阅读 IntelliJ IDEA 2020.3激活破解教程(亲测激活至 2089 年,长期更新)
二、New Project
File
->New Project
->Spring Initializr
(图2.1)->Java Version 8
(图2.2)->选择Dependencies
(图2.3)图2.1
图2.2
图2.3
删除
pom.xml
里的下面, 否则加载很慢此时,已经有了
Spring
项目的骨架。SpringBoot
提供的这些“开箱即用”的依赖模块都约定以spring-boot-starter-
作为命名的前缀,并且皆位于org.springframework.boot
包或者命名空间下工程目录结构
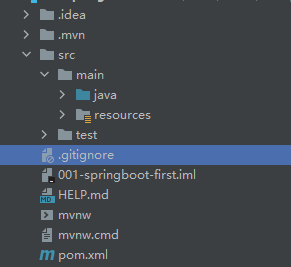
三、Hello World
1.1 新建
HelloController.java
输入如下代码
1.2 创建 AppConfig
创建
AppConfig.java
右键
Run AppConfig
127.0.0.0:8080/hello, 跑一跑看看
SpringBootApplication
,这个Annotation
是以下三个注解的合体:所以,如果我们使用如下的 SpringBoot 启动类:
四、总结
4.1 Sprint boot skeleton
IDEA
的Spring initializr
来创建Sprint boot
项目skeleton
骨架,Skeleton
来实现减少代码量,提高代码质量,加快项目开发速度。4.2 重要的文件结构
src/main/java
路径下的AppConfig.java
类 :程序入口src/main/resources
路径下的application.properties
:项目配置文件src/test
:单元测试文件路径SpringBoot工程代码存放的位置:程序入口文件同级目录及其子目录
4.3 pom.xml
pom
中指定parent
为以下内容,表示此项目继承了spring-boot-starter-parent
的maven
配置(主要是指定了常用依赖、插件的版本)。此外,
pom
中默认引入两个依赖包,和一个插件。spring-boot-starter-web
:核心模块,包括自动配置支持、日志和YAML
。spring-boot-starter-test
:测试模块,包括JUnit
、Hamcrest
、Mockito
。spring-boot-maven-plugin
:spring boot
插件, 提供了一系列spring boot
相关的maven
操作。spring-boot:build-info
,生成Actuator
使用的构建信息文件build-info.properties
spring-boot:repackage
,默认goal
。在mvn package
之后,再次打包可执行的jar/war
,同时保留mvn package
生成的jar/war
为.origin
spring-boot:run
,运行Spring Boot
应用spring-boot:start
,在mvn integration-test
阶段,进行Spring Boot
应用生命周期的管理spring-boot:stop
,在mvn integration-test
阶段,进行Spring Boot
应用生命周期的管理The text was updated successfully, but these errors were encountered: