You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
constperson={}Object.defineProperty(person,'name',{get: function(){console.log('getting name')return'Tink'}})console.log('this person is ',person.name)// [console]: getting name// this person is Tink
defineComputed(person,// 计算属性所属的对象'status',// 需要做计算的属性function(){// 执行计算的具体方法console.log('status getter')if(person.age>18){return'man'}else{return'boy'}},function(newVal){// 计算属性的值更新后执行console.log('status is now: '+newVal)})// 还可以像普通属性一样访问计算属性console.log('The person is a '+person.status)
person.age=20defineComputed({
person,'stauts',function(){if(person.age>18){console.log('this is man')return'man'}else{console.log('this is boy')return'boy'}},function(newVal){console.log('person.status now is '+newVal)}})console.log('person.status is '+person.status)
理解 Vue.js 的计算属性
通过模拟一个简单的例子来理解 Vue.js 的计算属性
Object.defineProperty
通过 Object.defineProperty 的
getter
setter
来实现对象的数据劫持,例如:Observable 对象
Vue.js 最基本的架构,就是可以把一个普通对象转成可被观察(observable)的对象。
对上面的
person
对象实现一个简单的 Observer 如下:定义一个计算属性
首先创建一个名为
defineComputed
的函数来定义计算属性defineComputed
函数应该可以这样用然后,具体的实现一个简单的
defineComputed
函数现在有两个问题
computedFn
updateCallBack
)最后,计算属性的预期应是如下的方式
增加依赖 Dependency
首先,添加一个全局的
Dep
对象这就是用来跟踪的 Dependency tracker
然后,给
defineComputed
函数添加依赖我们再来改进最初实现 Observer 的
defineReactive
函数同时我们改进下
defineComputed
方法中的onDependencyUpdate
来执行回调图解
将
person.status
设置为计算属性Step 1
person.status 的
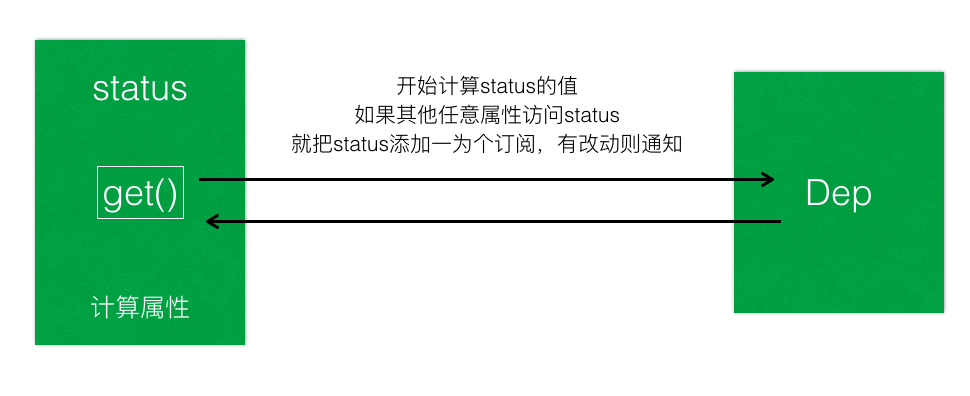
get()
被调用,并把Dep.target
设为它的回调Step 2
执行 person.status 的
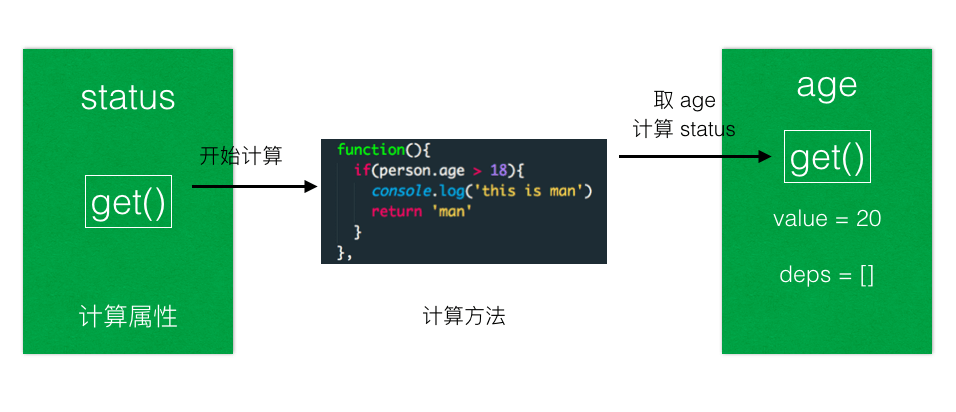
get()
里的计算函数Step 3
person.age 的
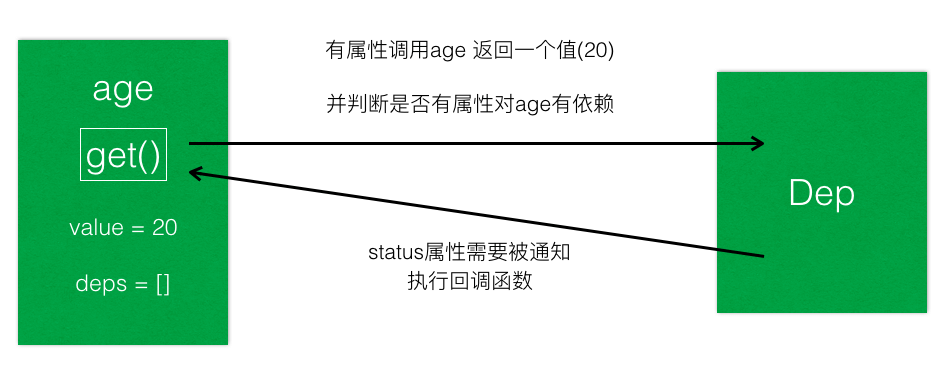
get()
检查 Dep 是否有可用的 targetStep 4
计算函数获取到新的值并返回。而且现在 person.age 的值只要变化就会通知给 person.status
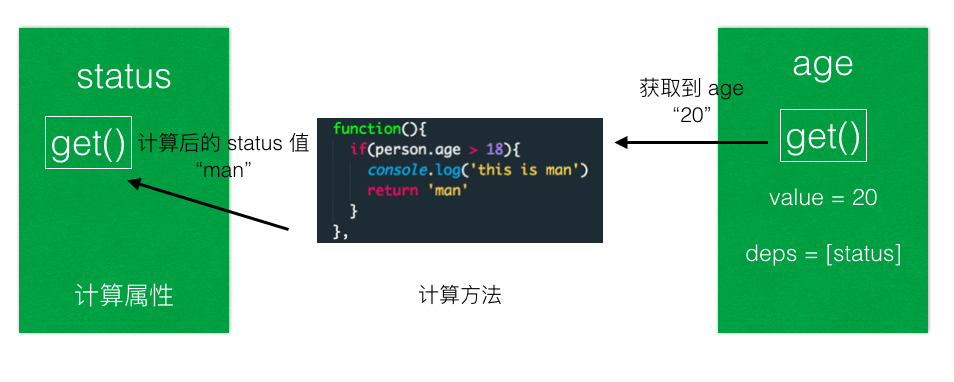
The text was updated successfully, but these errors were encountered: