diff --git a/README.md b/README.md
index 44b42b9..1edc764 100644
--- a/README.md
+++ b/README.md
@@ -12,21 +12,21 @@ Ballerina AWS Redshift Connector
The `ballerinax/aws.redshift` connector facilitates seamless integration with Amazon Redshift, offering Ballerina users a convenient and expressive way to connect, query, and interact with Redshift clusters, utilizing the AWS Redshift JDBC driver available through [`ballerinax/aws.redshift.driver`](https://central.ballerina.io/ballerinax/aws.redshift.driver/latest).
-## Set up guide
+## Setup guide
To effectively utilize the Ballerina AWS Redshift connector, you must have an Amazon Redshift cluster. Follow these steps to create an AWS Redshift cluster.
-### Step 1: Login to AWS Console
+### Step 1: Login to AWS console
1. Begin by logging into the [AWS Management Console](https://aws.amazon.com/).
-### Step 2: Navigate to Amazon Redshift and Create a Cluster
+### Step 2: Navigate to Amazon Redshift and create a cluster
1. In the AWS Console, navigate to the Amazon Redshift service. Click on the "Create cluster" button to initiate the process of creating a new Amazon Redshift cluster.
-### Step 3: Configure Cluster Settings
+### Step 3: Configure cluster settings
1. Follow the on-screen instructions to configure your Redshift cluster settings, including cluster identifier, database name, credentials, and other relevant parameters.
@@ -41,7 +41,7 @@ To effectively utilize the Ballerina AWS Redshift connector, you must have an Am
4. Finally, review your configuration settings, and once satisfied, click "Create cluster" to launch your Amazon Redshift cluster.
-### Step 4: Wait for Cluster Availability
+### Step 4: Wait for cluster availability
1. It may take some time for your Redshift cluster to be available. Monitor the cluster status in the AWS Console until it shows as "Available".
@@ -56,11 +56,11 @@ To use the `aws.redshift` connector in your Ballerina application, modify the `.
### Step 1: Import the connector
-Import the `ballerinax/aws.redshift` package and the `ballerinax/aws.redshift.driver` into your Ballerina project.
+Import `ballerinax/aws.redshift` and `ballerinax/aws.redshift.driver` modules.
```ballerina
-import ballerinax/aws.redshift; // Get the AWS Redshift connector
-import ballerinax/aws.redshift.driver as _; // Get the AWS Redshift driver
+import ballerinax/aws.redshift;
+import ballerinax/aws.redshift.driver as _;
```
### Step 2: Instantiate a new client
@@ -68,13 +68,11 @@ import ballerinax/aws.redshift.driver as _; // Get the AWS Redshift driver
Create a `redshift:Client` with the values obtained in the previous steps.
```ballerina
-// Connection Configurations
configurable string jdbcUrl = ?;
configurable string user = ?;
configurable string password = ?;
-// Initialize the Redshift client
-redshift:Client dbClient = check new (jdbcUrl, user, password);
+redshift:Client redshift = check new (jdbcUrl, user, password);
```
### Step 3: Invoke the connector operation
@@ -84,7 +82,6 @@ Now, utilize the available connector operations.
#### Read data from the database
```ballerina
-// Create the result record to match the columns of the table being queried.
type User record {|
string name;
string email;
@@ -92,7 +89,7 @@ type User record {|
|};
sql:ParameterizedQuery sqlQuery = `SELECT * FROM Users limit 10`;
-stream resultStream = dbClient->query(sqlQuery);
+stream resultStream = redshift->query(sqlQuery);
check from User user in resultStream
do {
io:println("Full details of users: ", user);
@@ -103,7 +100,7 @@ check from User user in resultStream
```ballerina
sql:ParameterizedQuery sqlQuery = `INSERT INTO your_table_name (firstname, lastname, state, email, username)
VALUES ('Cody', 'Moss', 'ON', 'dolor.nonummy@ipsumdolorsit.ca', 'WWZ18EOX');`;
-_ = check dbClient->execute(sqlQuery);
+_ = check redshift->execute(sqlQuery);
```
## Examples
diff --git a/ballerina/Module.md b/ballerina/Module.md
index 57f8e0d..754a00b 100644
--- a/ballerina/Module.md
+++ b/ballerina/Module.md
@@ -3,15 +3,15 @@
The `ballerinax/aws.redshift` connector facilitates seamless integration with Amazon Redshift, offering Ballerina users a convenient and expressive way to connect, query, and interact with Redshift clusters.
-## Set up guide
+## Setup guide
To effectively utilize the Ballerina AWS Redshift connector, you must have an Amazon Redshift cluster. Follow these steps to create an AWS Redshift cluster.
-### Step 1: Login to AWS Console
+### Step 1: Login to AWS console
1. Begin by logging into the [AWS Management Console](https://aws.amazon.com/).
-### Step 2: Navigate to Amazon Redshift and Create a Cluster
+### Step 2: Navigate to Amazon Redshift and create a cluster
1. In the AWS Console, navigate to the Amazon Redshift service. Click on the "Create cluster" button to initiate the process of creating a new Amazon Redshift cluster.
@@ -19,7 +19,7 @@ To effectively utilize the Ballerina AWS Redshift connector, you must have an Am
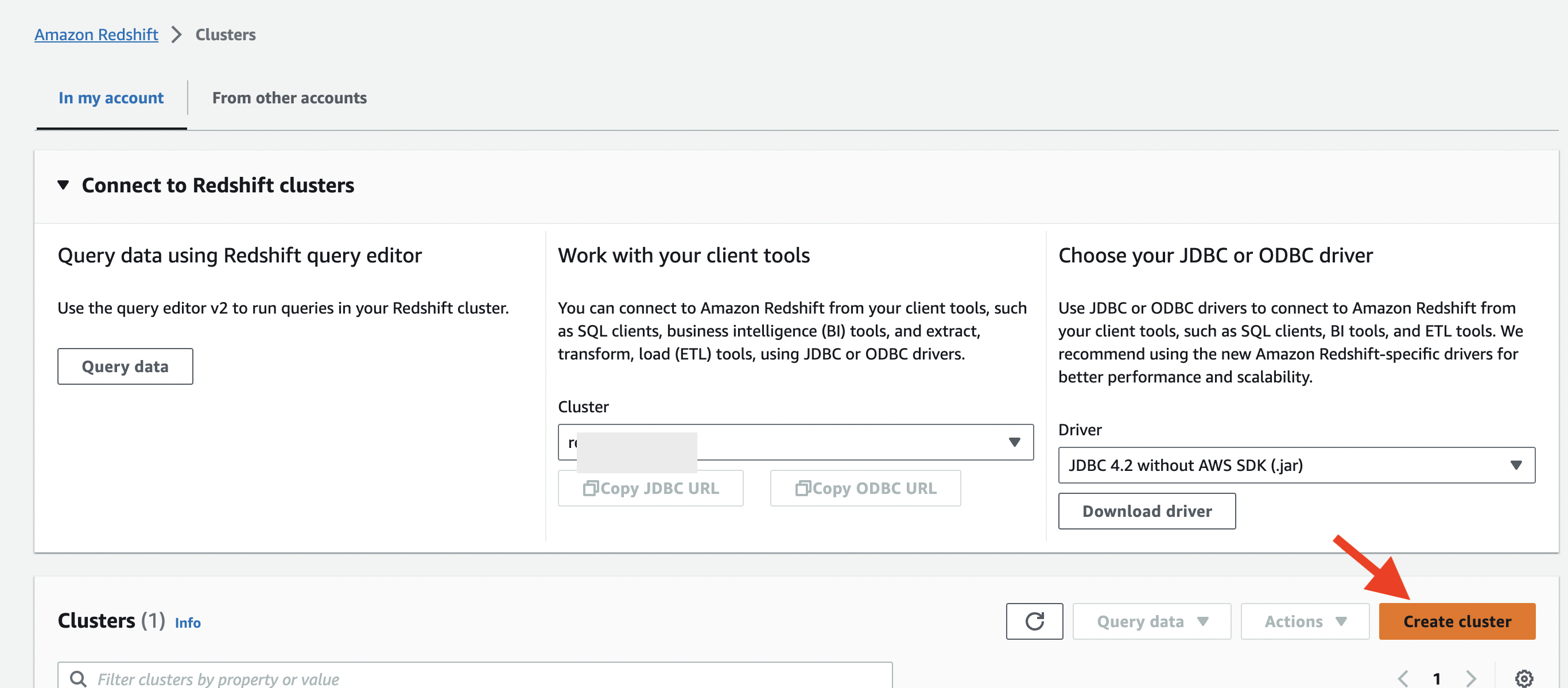
-### Step 3: Configure Cluster Settings
+### Step 3: Configure cluster settings
1. Follow the on-screen instructions to configure your Redshift cluster settings, including cluster identifier, database name, credentials, and other relevant parameters.
@@ -35,7 +35,7 @@ To effectively utilize the Ballerina AWS Redshift connector, you must have an Am
4. Finally, review your configuration settings, and once satisfied, click "Create cluster" to launch your Amazon Redshift cluster.
-### Step 4: Wait for Cluster Availability
+### Step 4: Wait for cluster availability
1. It may take some time for your Redshift cluster to be available. Monitor the cluster status in the AWS Console until it shows as "Available".
@@ -51,11 +51,11 @@ To use the `aws.redshift` connector in your Ballerina application, modify the `.
### Step 1: Import the connector
-Import the `ballerinax/aws.redshift` package and the `ballerinax/aws.redshift.driver` into your Ballerina project.
+Import `ballerinax/aws.redshift` and `ballerinax/aws.redshift.driver` modules.
```ballerina
-import ballerinax/aws.redshift; // Get the AWS Redshift connector
-import ballerinax/aws.redshift.driver as _; // Get the AWS Redshift driver
+import ballerinax/aws.redshift;
+import ballerinax/aws.redshift.driver as _;
```
### Step 2: Instantiate a new client
@@ -63,13 +63,11 @@ import ballerinax/aws.redshift.driver as _; // Get the AWS Redshift driver
Create a `redshift:Client` with the values obtained in the previous steps.
```ballerina
-// Connection Configurations
configurable string jdbcUrl = ?;
configurable string user = ?;
configurable string password = ?;
-// Initialize the Redshift client
-redshift:Client dbClient = check new (jdbcUrl, user, password);
+redshift:Client redshift = check new (jdbcUrl, user, password);
```
### Step 3: Invoke the connector operation
@@ -79,7 +77,6 @@ Now, utilize the available connector operations.
#### Read data from the database
```ballerina
-// Create the result record to match the columns of the table being queried.
type User record {|
string name;
string email;
@@ -87,7 +84,7 @@ type User record {|
|};
sql:ParameterizedQuery sqlQuery = `SELECT * FROM Users limit 10`;
-stream resultStream = dbClient->query(sqlQuery);
+stream resultStream = redshift->query(sqlQuery);
check from User user in resultStream
do {
io:println("Full details of users: ", user);
@@ -99,7 +96,7 @@ check from User user in resultStream
```ballerina
sql:ParameterizedQuery sqlQuery = `INSERT INTO your_table_name (firstname, lastname, state, email, username)
VALUES ('Cody', 'Moss', 'ON', 'dolor.nonummy@ipsumdolorsit.ca', 'WWZ18EOX');`;
-_ = check dbClient->execute(sqlQuery);
+_ = check redshift->execute(sqlQuery);
```
## Examples
diff --git a/ballerina/Package.md b/ballerina/Package.md
index c0e6032..0d62686 100644
--- a/ballerina/Package.md
+++ b/ballerina/Package.md
@@ -4,15 +4,15 @@
The `ballerinax/aws.redshift` connector facilitates seamless integration with Amazon Redshift, offering Ballerina users a convenient and expressive way to connect, query, and interact with Redshift clusters.
-## Set up guide
+## Setup guide
To effectively utilize the Ballerina AWS Redshift connector, you must have an Amazon Redshift cluster. Follow these steps to create an AWS Redshift cluster.
-### Step 1: Login to AWS Console
+### Step 1: Login to AWS console
1. Begin by logging into the [AWS Management Console](https://aws.amazon.com/).
-### Step 2: Navigate to Amazon Redshift and Create a Cluster
+### Step 2: Navigate to Amazon Redshift and create a cluster
1. In the AWS Console, navigate to the Amazon Redshift service. Click on the "Create cluster" button to initiate the process of creating a new Amazon Redshift cluster.
@@ -20,7 +20,7 @@ To effectively utilize the Ballerina AWS Redshift connector, you must have an Am
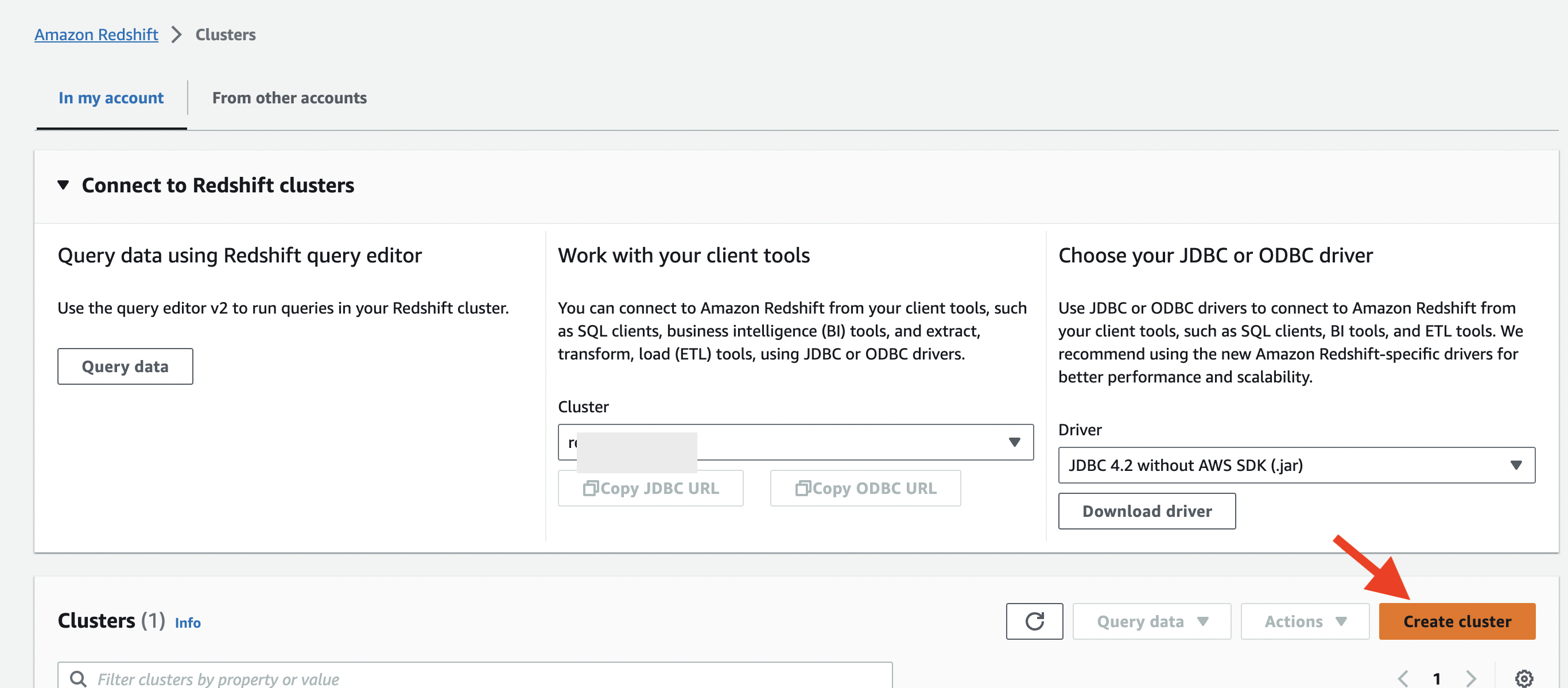
-### Step 3: Configure Cluster Settings
+### Step 3: Configure cluster settings
1. Follow the on-screen instructions to configure your Redshift cluster settings, including cluster identifier, database name, credentials, and other relevant parameters.
@@ -36,7 +36,7 @@ To effectively utilize the Ballerina AWS Redshift connector, you must have an Am
4. Finally, review your configuration settings, and once satisfied, click "Create cluster" to launch your Amazon Redshift cluster.
-### Step 4: Wait for Cluster Availability
+### Step 4: Wait for cluster availability
1. It may take some time for your Redshift cluster to be available. Monitor the cluster status in the AWS Console until it shows as "Available".
@@ -52,11 +52,11 @@ To use the `aws.redshift` connector in your Ballerina application, modify the `.
### Step 1: Import the connector
-Import the `ballerinax/aws.redshift` package and the `ballerinax/aws.redshift.driver` into your Ballerina project.
+Import `ballerinax/aws.redshift` and `ballerinax/aws.redshift.driver` modules.
```ballerina
-import ballerinax/aws.redshift; // Get the AWS Redshift connector
-import ballerinax/aws.redshift.driver as _; // Get the AWS Redshift driver
+import ballerinax/aws.redshift;
+import ballerinax/aws.redshift.driver as _;
```
### Step 2: Instantiate a new client
@@ -64,13 +64,11 @@ import ballerinax/aws.redshift.driver as _; // Get the AWS Redshift driver
Create a `redshift:Client` with the values obtained in the previous steps.
```ballerina
-// Connection Configurations
configurable string jdbcUrl = ?;
configurable string user = ?;
configurable string password = ?;
-// Initialize the Redshift client
-redshift:Client dbClient = check new (jdbcUrl, user, password);
+redshift:Client redshift = check new (jdbcUrl, user, password);
```
### Step 3: Invoke the connector operation
@@ -80,7 +78,6 @@ Now, utilize the available connector operations.
#### Read data from the database
```ballerina
-// Create the result record to match the columns of the table being queried.
type User record {|
string name;
string email;
@@ -88,7 +85,7 @@ type User record {|
|};
sql:ParameterizedQuery sqlQuery = `SELECT * FROM Users limit 10`;
-stream resultStream = dbClient->query(sqlQuery);
+stream resultStream = redshift->query(sqlQuery);
check from User user in resultStream
do {
io:println("Full details of users: ", user);
@@ -99,7 +96,7 @@ check from User user in resultStream
```ballerina
sql:ParameterizedQuery sqlQuery = `INSERT INTO your_table_name (firstname, lastname, state, email, username)
VALUES ('Cody', 'Moss', 'ON', 'dolor.nonummy@ipsumdolorsit.ca', 'WWZ18EOX');`;
-_ = check dbClient->execute(sqlQuery);
+_ = check redshift->execute(sqlQuery);
```
## Examples
diff --git a/examples/README.md b/examples/README.md
index ac0c745..7fe05fa 100644
--- a/examples/README.md
+++ b/examples/README.md
@@ -10,7 +10,7 @@ The `ballerinax/aws.redshift` connector facilitates seamless integration with Am
## Prerequisites
-1. Follow the [instructions](https://github.com/ballerina-platform/module-ballerinax-aws.redshift#set-up-guide) to set up the AWS Redshift cluster.
+1. Follow the [instructions](https://github.com/ballerina-platform/module-ballerinax-aws.redshift#setup-guide) to set up the AWS Redshift cluster.
2. For each example, create a new Ballerina project. Copy the provided example code into the project, and include a `config.toml` file with the necessary JDBC URL, username, and password. Below is an example illustrating how your `config.toml` file should be structured:
```toml