' );
- * false === $processor->next_tag();
- * WP_HTML_Processor::ERROR_UNSUPPORTED === $processor->get_last_error();
- *
- * @since 6.4.0
- *
- * @see self::ERROR_UNSUPPORTED
- * @see self::ERROR_EXCEEDED_MAX_BOOKMARKS
- *
- * @return string|null The last error, if one exists, otherwise null.
- */
- public function get_last_error() {
- return $this->last_error;
- }
-
- /**
- * Finds the next tag matching the $query.
- *
- * @todo Support matching the class name and tag name.
- *
- * @since 6.4.0
- * @since 6.6.0 Visits all tokens, including virtual ones.
- *
- * @throws Exception When unable to allocate a bookmark for the next token in the input HTML document.
- *
- * @param array|string|null $query {
- * Optional. Which tag name to find, having which class, etc. Default is to find any tag.
- *
- * @type string|null $tag_name Which tag to find, or `null` for "any tag."
- * @type string $tag_closers 'visit' to pause at tag closers, 'skip' or unset to only visit openers.
- * @type int|null $match_offset Find the Nth tag matching all search criteria.
- * 1 for "first" tag, 3 for "third," etc.
- * Defaults to first tag.
- * @type string|null $class_name Tag must contain this whole class name to match.
- * @type string[] $breadcrumbs DOM sub-path at which element is found, e.g. `array( 'FIGURE', 'IMG' )`.
- * May also contain the wildcard `*` which matches a single element, e.g. `array( 'SECTION', '*' )`.
- * }
- * @return bool Whether a tag was matched.
- */
- public function next_tag( $query = null ) {
- $visit_closers = isset( $query['tag_closers'] ) && 'visit' === $query['tag_closers'];
-
- if ( null === $query ) {
- while ( $this->next_token() ) {
- if ( '#tag' !== $this->get_token_type() ) {
- continue;
- }
-
- if ( ! $this->is_tag_closer() || $visit_closers ) {
- return true;
- }
- }
-
- return false;
- }
-
- if ( is_string( $query ) ) {
- $query = array( 'breadcrumbs' => array( $query ) );
- }
-
- if ( ! is_array( $query ) ) {
- _doing_it_wrong(
- __METHOD__,
- __( 'Please pass a query array to this function.' ),
- '6.4.0'
- );
- return false;
- }
-
- $needs_class = ( isset( $query['class_name'] ) && is_string( $query['class_name'] ) )
- ? $query['class_name']
- : null;
-
- if ( ! ( array_key_exists( 'breadcrumbs', $query ) && is_array( $query['breadcrumbs'] ) ) ) {
- while ( $this->next_token() ) {
- if ( '#tag' !== $this->get_token_type() ) {
- continue;
- }
-
- if ( isset( $needs_class ) && ! $this->has_class( $needs_class ) ) {
- continue;
- }
-
- if ( ! $this->is_tag_closer() || $visit_closers ) {
- return true;
- }
- }
-
- return false;
- }
-
- $breadcrumbs = $query['breadcrumbs'];
- $match_offset = isset( $query['match_offset'] ) ? (int) $query['match_offset'] : 1;
-
- while ( $match_offset > 0 && $this->next_token() ) {
- if ( '#tag' !== $this->get_token_type() || $this->is_tag_closer() ) {
- continue;
- }
-
- if ( isset( $needs_class ) && ! $this->has_class( $needs_class ) ) {
- continue;
- }
-
- if ( $this->matches_breadcrumbs( $breadcrumbs ) && 0 === --$match_offset ) {
- return true;
- }
- }
-
- return false;
- }
-
- /**
- * Ensures internal accounting is maintained for HTML semantic rules while
- * the underlying Tag Processor class is seeking to a bookmark.
- *
- * This doesn't currently have a way to represent non-tags and doesn't process
- * semantic rules for text nodes. For access to the raw tokens consider using
- * WP_HTML_Tag_Processor instead.
- *
- * @since 6.5.0 Added for internal support; do not use.
- *
- * @access private
- *
- * @return bool
- */
- public function next_token() {
- $this->current_element = null;
-
- if ( isset( $this->last_error ) ) {
- return false;
- }
-
- if ( 'done' !== $this->has_seen_context_node && 0 === count( $this->element_queue ) && ! $this->step() ) {
- while ( 'context-node' !== $this->state->stack_of_open_elements->current_node()->bookmark_name && $this->state->stack_of_open_elements->pop() ) {
- continue;
- }
- $this->has_seen_context_node = 'done';
- return $this->next_token();
- }
-
- $this->current_element = array_shift( $this->element_queue );
- while ( isset( $this->context_node ) && ! $this->has_seen_context_node ) {
- if ( isset( $this->current_element ) ) {
- if ( $this->context_node === $this->current_element->token && Gutenberg_HTML_Stack_Event_6_6::PUSH === $this->current_element->operation ) {
- $this->has_seen_context_node = true;
- return $this->next_token();
- }
- }
- $this->current_element = array_shift( $this->element_queue );
- }
-
- if ( ! isset( $this->current_element ) ) {
- if ( 'done' === $this->has_seen_context_node ) {
- return false;
- } else {
- return $this->next_token();
- }
- }
-
- if ( isset( $this->context_node ) && Gutenberg_HTML_Stack_Event_6_6::POP === $this->current_element->operation && $this->context_node === $this->current_element->token ) {
- $this->element_queue = array();
- $this->current_element = null;
- return false;
- }
-
- // Avoid sending close events for elements which don't expect a closing.
- if (
- Gutenberg_HTML_Stack_Event_6_6::POP === $this->current_element->operation &&
- ! static::expects_closer( $this->current_element->token )
- ) {
- return $this->next_token();
- }
-
- return true;
- }
-
-
- /**
- * Indicates if the current tag token is a tag closer.
- *
- * Example:
- *
- * $p = WP_HTML_Processor::create_fragment( '
' );
- * $p->next_tag( array( 'tag_name' => 'div', 'tag_closers' => 'visit' ) );
- * $p->is_tag_closer() === false;
- *
- * $p->next_tag( array( 'tag_name' => 'div', 'tag_closers' => 'visit' ) );
- * $p->is_tag_closer() === true;
- *
- * @since 6.6.0 Subclassed for HTML Processor.
- *
- * @return bool Whether the current tag is a tag closer.
- */
- public function is_tag_closer() {
- return $this->is_virtual()
- ? ( Gutenberg_HTML_Stack_Event_6_6::POP === $this->current_element->operation && '#tag' === $this->get_token_type() )
- : parent::is_tag_closer();
- }
-
- /**
- * Indicates if the currently-matched token is virtual, created by a stack operation
- * while processing HTML, rather than a token found in the HTML text itself.
- *
- * @since 6.6.0
- *
- * @return bool Whether the current token is virtual.
- */
- private function is_virtual() {
- return (
- isset( $this->current_element->provenance ) &&
- 'virtual' === $this->current_element->provenance
- );
- }
-
- /**
- * Indicates if the currently-matched tag matches the given breadcrumbs.
- *
- * A "*" represents a single tag wildcard, where any tag matches, but not no tags.
- *
- * At some point this function _may_ support a `**` syntax for matching any number
- * of unspecified tags in the breadcrumb stack. This has been intentionally left
- * out, however, to keep this function simple and to avoid introducing backtracking,
- * which could open up surprising performance breakdowns.
- *
- * Example:
- *
- * $processor = WP_HTML_Processor::create_fragment( '' );
- * $processor->next_tag( 'img' );
- * true === $processor->matches_breadcrumbs( array( 'figure', 'img' ) );
- * true === $processor->matches_breadcrumbs( array( 'span', 'figure', 'img' ) );
- * false === $processor->matches_breadcrumbs( array( 'span', 'img' ) );
- * true === $processor->matches_breadcrumbs( array( 'span', '*', 'img' ) );
- *
- * @since 6.4.0
- *
- * @param string[] $breadcrumbs DOM sub-path at which element is found, e.g. `array( 'FIGURE', 'IMG' )`.
- * May also contain the wildcard `*` which matches a single element, e.g. `array( 'SECTION', '*' )`.
- * @return bool Whether the currently-matched tag is found at the given nested structure.
- */
- public function matches_breadcrumbs( $breadcrumbs ) {
- // Everything matches when there are zero constraints.
- if ( 0 === count( $breadcrumbs ) ) {
- return true;
- }
-
- // Start at the last crumb.
- $crumb = end( $breadcrumbs );
-
- if ( '*' !== $crumb && $this->get_tag() !== strtoupper( $crumb ) ) {
- return false;
- }
-
- foreach ( $this->state->stack_of_open_elements->walk_up() as $node ) {
- $crumb = strtoupper( current( $breadcrumbs ) );
-
- if ( '*' !== $crumb && $node->node_name !== $crumb ) {
- return false;
- }
-
- if ( false === prev( $breadcrumbs ) ) {
- return true;
- }
- }
-
- return false;
- }
-
- /**
- * Indicates if the currently-matched node expects a closing
- * token, or if it will self-close on the next step.
- *
- * Most HTML elements expect a closer, such as a P element or
- * a DIV element. Others, like an IMG element are void and don't
- * have a closing tag. Special elements, such as SCRIPT and STYLE,
- * are treated just like void tags. Text nodes and self-closing
- * foreign content will also act just like a void tag, immediately
- * closing as soon as the processor advances to the next token.
- *
- * @since 6.6.0
- *
- * @todo When adding support for foreign content, ensure that
- * this returns false for self-closing elements in the
- * SVG and MathML namespace.
- *
- * @param ?WP_HTML_Token $node Node to examine instead of current node, if provided.
- * @return bool Whether to expect a closer for the currently-matched node,
- * or `null` if not matched on any token.
- */
- public function expects_closer( $node = null ) {
- $token_name = $node->node_name ?? $this->get_token_name();
- if ( ! isset( $token_name ) ) {
- return null;
- }
-
- return ! (
- // Comments, text nodes, and other atomic tokens.
- '#' === $token_name[0] ||
- // Doctype declarations.
- 'html' === $token_name ||
- // Void elements.
- self::is_void( $token_name ) ||
- // Special atomic elements.
- in_array( $token_name, array( 'IFRAME', 'NOEMBED', 'NOFRAMES', 'SCRIPT', 'STYLE', 'TEXTAREA', 'TITLE', 'XMP' ), true )
- );
- }
-
- /**
- * Steps through the HTML document and stop at the next tag, if any.
- *
- * @since 6.4.0
- *
- * @throws Exception When unable to allocate a bookmark for the next token in the input HTML document.
- *
- * @see self::PROCESS_NEXT_NODE
- * @see self::REPROCESS_CURRENT_NODE
- *
- * @param string $node_to_process Whether to parse the next node or reprocess the current node.
- * @return bool Whether a tag was matched.
- */
- public function step( $node_to_process = self::PROCESS_NEXT_NODE ) {
- // Refuse to proceed if there was a previous error.
- if ( null !== $this->last_error ) {
- return false;
- }
-
- if ( self::REPROCESS_CURRENT_NODE !== $node_to_process ) {
- /*
- * Void elements still hop onto the stack of open elements even though
- * there's no corresponding closing tag. This is important for managing
- * stack-based operations such as "navigate to parent node" or checking
- * on an element's breadcrumbs.
- *
- * When moving on to the next node, therefore, if the bottom-most element
- * on the stack is a void element, it must be closed.
- *
- * @todo Once self-closing foreign elements and BGSOUND are supported,
- * they must also be implicitly closed here too. BGSOUND is
- * special since it's only self-closing if the self-closing flag
- * is provided in the opening tag, otherwise it expects a tag closer.
- */
- $top_node = $this->state->stack_of_open_elements->current_node();
- if ( isset( $top_node ) && ! static::expects_closer( $top_node ) ) {
- $this->state->stack_of_open_elements->pop();
- }
- }
-
- if ( self::PROCESS_NEXT_NODE === $node_to_process ) {
- parent::next_token();
- }
-
- // Finish stepping when there are no more tokens in the document.
- if (
- Gutenberg_HTML_Tag_Processor_6_6::STATE_INCOMPLETE_INPUT === $this->parser_state ||
- Gutenberg_HTML_Tag_Processor_6_6::STATE_COMPLETE === $this->parser_state
- ) {
- return false;
- }
-
- $this->state->current_token = new WP_HTML_Token(
- $this->bookmark_token(),
- $this->get_token_name(),
- $this->has_self_closing_flag(),
- $this->release_internal_bookmark_on_destruct
- );
-
- try {
- switch ( $this->state->insertion_mode ) {
- case Gutenberg_HTML_Processor_State_6_6::INSERTION_MODE_IN_BODY:
- return $this->step_in_body();
-
- default:
- $this->last_error = self::ERROR_UNSUPPORTED;
- throw new WP_HTML_Unsupported_Exception( "No support for parsing in the '{$this->state->insertion_mode}' state." );
- }
- } catch ( WP_HTML_Unsupported_Exception $e ) {
- /*
- * Exceptions are used in this class to escape deep call stacks that
- * otherwise might involve messier calling and return conventions.
- */
- return false;
- }
- }
-
- /**
- * Computes the HTML breadcrumbs for the currently-matched node, if matched.
- *
- * Breadcrumbs start at the outermost parent and descend toward the matched element.
- * They always include the entire path from the root HTML node to the matched element.
- *
- * @todo It could be more efficient to expose a generator-based version of this function
- * to avoid creating the array copy on tag iteration. If this is done, it would likely
- * be more useful to walk up the stack when yielding instead of starting at the top.
- *
- * Example
- *
- * $processor = WP_HTML_Processor::create_fragment( '
' );
- * $processor->next_tag( 'IMG' );
- * $processor->get_breadcrumbs() === array( 'HTML', 'BODY', 'P', 'STRONG', 'EM', 'IMG' );
- *
- * @since 6.4.0
- *
- * @return string[]|null Array of tag names representing path to matched node, if matched, otherwise NULL.
- */
- public function get_breadcrumbs() {
- $breadcrumbs = array();
-
- foreach ( $this->state->stack_of_open_elements->walk_down() as $stack_item ) {
- $breadcrumbs[] = $stack_item->node_name;
- }
-
- if ( ! $this->is_virtual() ) {
- return $breadcrumbs;
- }
-
- foreach ( $this->element_queue as $queue_item ) {
- if ( $this->current_element->token->bookmark_name === $queue_item->token->bookmark_name ) {
- break;
- }
-
- if ( 'context-node' === $queue_item->token->bookmark_name ) {
- break;
- }
-
- if ( 'real' === $queue_item->provenance ) {
- break;
- }
-
- if ( Gutenberg_HTML_Stack_Event_6_6::PUSH === $queue_item->operation ) {
- $breadcrumbs[] = $queue_item->token->node_name;
- } else {
- array_pop( $breadcrumbs );
- }
- }
-
- if ( null !== parent::get_token_name() && ! parent::is_tag_closer() ) {
- array_pop( $breadcrumbs );
- }
-
- // Add the virtual node we're at.
- if ( Gutenberg_HTML_Stack_Event_6_6::PUSH === $this->current_element->operation ) {
- $breadcrumbs[] = $this->current_element->token->node_name;
- }
-
- return $breadcrumbs;
- }
-
- /**
- * Returns the nesting depth of the current location in the document.
- *
- * Example:
- *
- * $processor = WP_HTML_Processor::create_fragment( '' );
- * // The processor starts in the BODY context, meaning it has depth from the start: HTML > BODY.
- * 2 === $processor->get_current_depth();
- *
- * // Opening the DIV element increases the depth.
- * $processor->next_token();
- * 3 === $processor->get_current_depth();
- *
- * // Opening the P element increases the depth.
- * $processor->next_token();
- * 4 === $processor->get_current_depth();
- *
- * // The P element is closed during `next_token()` so the depth is decreased to reflect that.
- * $processor->next_token();
- * 3 === $processor->get_current_depth();
- *
- * @since 6.6.0
- *
- * @return int Nesting-depth of current location in the document.
- */
- public function get_current_depth() {
- return $this->is_virtual()
- ? count( $this->get_breadcrumbs() )
- : $this->state->stack_of_open_elements->count();
- }
-
- /**
- * Parses next element in the 'in body' insertion mode.
- *
- * This internal function performs the 'in body' insertion mode
- * logic for the generalized WP_HTML_Processor::step() function.
- *
- * @since 6.4.0
- *
- * @throws WP_HTML_Unsupported_Exception When encountering unsupported HTML input.
- *
- * @see https://html.spec.whatwg.org/#parsing-main-inbody
- * @see WP_HTML_Processor::step
- *
- * @return bool Whether an element was found.
- */
- private function step_in_body() {
- $token_name = $this->get_token_name();
- $token_type = $this->get_token_type();
- $op_sigil = '#tag' === $token_type ? ( parent::is_tag_closer() ? '-' : '+' ) : '';
- $op = "{$op_sigil}{$token_name}";
-
- switch ( $op ) {
- case '#comment':
- case '#funky-comment':
- case '#presumptuous-tag':
- $this->insert_html_element( $this->state->current_token );
- return true;
-
- case '#text':
- $this->reconstruct_active_formatting_elements();
-
- $current_token = $this->bookmarks[ $this->state->current_token->bookmark_name ];
-
- /*
- * > A character token that is U+0000 NULL
- *
- * Any successive sequence of NULL bytes is ignored and won't
- * trigger active format reconstruction. Therefore, if the text
- * only comprises NULL bytes then the token should be ignored
- * here, but if there are any other characters in the stream
- * the active formats should be reconstructed.
- */
- if (
- 1 <= $current_token->length &&
- "\x00" === $this->html[ $current_token->start ] &&
- strspn( $this->html, "\x00", $current_token->start, $current_token->length ) === $current_token->length
- ) {
- // Parse error: ignore the token.
- return $this->step();
- }
-
- /*
- * Whitespace-only text does not affect the frameset-ok flag.
- * It is probably inter-element whitespace, but it may also
- * contain character references which decode only to whitespace.
- */
- $text = $this->get_modifiable_text();
- if ( strlen( $text ) !== strspn( $text, " \t\n\f\r" ) ) {
- $this->state->frameset_ok = false;
- }
-
- $this->insert_html_element( $this->state->current_token );
- return true;
-
- case 'html':
- /*
- * > A DOCTYPE token
- * > Parse error. Ignore the token.
- */
- return $this->step();
-
- /*
- * > A start tag whose tag name is "button"
- */
- case '+BUTTON':
- if ( $this->state->stack_of_open_elements->has_element_in_scope( 'BUTTON' ) ) {
- // @todo Indicate a parse error once it's possible. This error does not impact the logic here.
- $this->generate_implied_end_tags();
- $this->state->stack_of_open_elements->pop_until( 'BUTTON' );
- }
-
- $this->reconstruct_active_formatting_elements();
- $this->insert_html_element( $this->state->current_token );
- $this->state->frameset_ok = false;
-
- return true;
-
- /*
- * > A start tag whose tag name is one of: "address", "article", "aside",
- * > "blockquote", "center", "details", "dialog", "dir", "div", "dl",
- * > "fieldset", "figcaption", "figure", "footer", "header", "hgroup",
- * > "main", "menu", "nav", "ol", "p", "search", "section", "summary", "ul"
- */
- case '+ADDRESS':
- case '+ARTICLE':
- case '+ASIDE':
- case '+BLOCKQUOTE':
- case '+CENTER':
- case '+DETAILS':
- case '+DIALOG':
- case '+DIR':
- case '+DIV':
- case '+DL':
- case '+FIELDSET':
- case '+FIGCAPTION':
- case '+FIGURE':
- case '+FOOTER':
- case '+HEADER':
- case '+HGROUP':
- case '+MAIN':
- case '+MENU':
- case '+NAV':
- case '+OL':
- case '+P':
- case '+SEARCH':
- case '+SECTION':
- case '+SUMMARY':
- case '+UL':
- if ( $this->state->stack_of_open_elements->has_p_in_button_scope() ) {
- $this->close_a_p_element();
- }
-
- $this->insert_html_element( $this->state->current_token );
- return true;
-
- /*
- * > An end tag whose tag name is one of: "address", "article", "aside", "blockquote",
- * > "button", "center", "details", "dialog", "dir", "div", "dl", "fieldset",
- * > "figcaption", "figure", "footer", "header", "hgroup", "listing", "main",
- * > "menu", "nav", "ol", "pre", "search", "section", "summary", "ul"
- */
- case '-ADDRESS':
- case '-ARTICLE':
- case '-ASIDE':
- case '-BLOCKQUOTE':
- case '-BUTTON':
- case '-CENTER':
- case '-DETAILS':
- case '-DIALOG':
- case '-DIR':
- case '-DIV':
- case '-DL':
- case '-FIELDSET':
- case '-FIGCAPTION':
- case '-FIGURE':
- case '-FOOTER':
- case '-HEADER':
- case '-HGROUP':
- case '-LISTING':
- case '-MAIN':
- case '-MENU':
- case '-NAV':
- case '-OL':
- case '-PRE':
- case '-SEARCH':
- case '-SECTION':
- case '-SUMMARY':
- case '-UL':
- if ( ! $this->state->stack_of_open_elements->has_element_in_scope( $token_name ) ) {
- // @todo Report parse error.
- // Ignore the token.
- return $this->step();
- }
-
- $this->generate_implied_end_tags();
- if ( $this->state->stack_of_open_elements->current_node()->node_name !== $token_name ) {
- // @todo Record parse error: this error doesn't impact parsing.
- }
- $this->state->stack_of_open_elements->pop_until( $token_name );
- return true;
-
- /*
- * > A start tag whose tag name is one of: "h1", "h2", "h3", "h4", "h5", "h6"
- */
- case '+H1':
- case '+H2':
- case '+H3':
- case '+H4':
- case '+H5':
- case '+H6':
- if ( $this->state->stack_of_open_elements->has_p_in_button_scope() ) {
- $this->close_a_p_element();
- }
-
- if (
- in_array(
- $this->state->stack_of_open_elements->current_node()->node_name,
- array( 'H1', 'H2', 'H3', 'H4', 'H5', 'H6' ),
- true
- )
- ) {
- // @todo Indicate a parse error once it's possible.
- $this->state->stack_of_open_elements->pop();
- }
-
- $this->insert_html_element( $this->state->current_token );
- return true;
-
- /*
- * > A start tag whose tag name is one of: "pre", "listing"
- */
- case '+PRE':
- case '+LISTING':
- if ( $this->state->stack_of_open_elements->has_p_in_button_scope() ) {
- $this->close_a_p_element();
- }
- $this->insert_html_element( $this->state->current_token );
- $this->state->frameset_ok = false;
- return true;
-
- /*
- * > An end tag whose tag name is one of: "h1", "h2", "h3", "h4", "h5", "h6"
- */
- case '-H1':
- case '-H2':
- case '-H3':
- case '-H4':
- case '-H5':
- case '-H6':
- if ( ! $this->state->stack_of_open_elements->has_element_in_scope( '(internal: H1 through H6 - do not use)' ) ) {
- /*
- * This is a parse error; ignore the token.
- *
- * @todo Indicate a parse error once it's possible.
- */
- return $this->step();
- }
-
- $this->generate_implied_end_tags();
-
- if ( $this->state->stack_of_open_elements->current_node()->node_name !== $token_name ) {
- // @todo Record parse error: this error doesn't impact parsing.
- }
-
- $this->state->stack_of_open_elements->pop_until( '(internal: H1 through H6 - do not use)' );
- return true;
-
- /*
- * > A start tag whose tag name is "li"
- * > A start tag whose tag name is one of: "dd", "dt"
- */
- case '+DD':
- case '+DT':
- case '+LI':
- $this->state->frameset_ok = false;
- $node = $this->state->stack_of_open_elements->current_node();
- $is_li = 'LI' === $token_name;
-
- in_body_list_loop:
- /*
- * The logic for LI and DT/DD is the same except for one point: LI elements _only_
- * close other LI elements, but a DT or DD element closes _any_ open DT or DD element.
- */
- if ( $is_li ? 'LI' === $node->node_name : ( 'DD' === $node->node_name || 'DT' === $node->node_name ) ) {
- $node_name = $is_li ? 'LI' : $node->node_name;
- $this->generate_implied_end_tags( $node_name );
- if ( $node_name !== $this->state->stack_of_open_elements->current_node()->node_name ) {
- // @todo Indicate a parse error once it's possible. This error does not impact the logic here.
- }
-
- $this->state->stack_of_open_elements->pop_until( $node_name );
- goto in_body_list_done;
- }
-
- if (
- 'ADDRESS' !== $node->node_name &&
- 'DIV' !== $node->node_name &&
- 'P' !== $node->node_name &&
- $this->is_special( $node->node_name )
- ) {
- /*
- * > If node is in the special category, but is not an address, div,
- * > or p element, then jump to the step labeled done below.
- */
- goto in_body_list_done;
- } else {
- /*
- * > Otherwise, set node to the previous entry in the stack of open elements
- * > and return to the step labeled loop.
- */
- foreach ( $this->state->stack_of_open_elements->walk_up( $node ) as $item ) {
- $node = $item;
- break;
- }
- goto in_body_list_loop;
- }
-
- in_body_list_done:
- if ( $this->state->stack_of_open_elements->has_p_in_button_scope() ) {
- $this->close_a_p_element();
- }
-
- $this->insert_html_element( $this->state->current_token );
- return true;
-
- /*
- * > An end tag whose tag name is "li"
- * > An end tag whose tag name is one of: "dd", "dt"
- */
- case '-DD':
- case '-DT':
- case '-LI':
- if (
- /*
- * An end tag whose tag name is "li":
- * If the stack of open elements does not have an li element in list item scope,
- * then this is a parse error; ignore the token.
- */
- (
- 'LI' === $token_name &&
- ! $this->state->stack_of_open_elements->has_element_in_list_item_scope( 'LI' )
- ) ||
- /*
- * An end tag whose tag name is one of: "dd", "dt":
- * If the stack of open elements does not have an element in scope that is an
- * HTML element with the same tag name as that of the token, then this is a
- * parse error; ignore the token.
- */
- (
- 'LI' !== $token_name &&
- ! $this->state->stack_of_open_elements->has_element_in_scope( $token_name )
- )
- ) {
- /*
- * This is a parse error, ignore the token.
- *
- * @todo Indicate a parse error once it's possible.
- */
- return $this->step();
- }
-
- $this->generate_implied_end_tags( $token_name );
-
- if ( $token_name !== $this->state->stack_of_open_elements->current_node()->node_name ) {
- // @todo Indicate a parse error once it's possible. This error does not impact the logic here.
- }
-
- $this->state->stack_of_open_elements->pop_until( $token_name );
- return true;
-
- /*
- * > An end tag whose tag name is "p"
- */
- case '-P':
- if ( ! $this->state->stack_of_open_elements->has_p_in_button_scope() ) {
- $this->insert_html_element( $this->state->current_token );
- }
-
- $this->close_a_p_element();
- return true;
-
- // > A start tag whose tag name is "a"
- case '+A':
- foreach ( $this->state->active_formatting_elements->walk_up() as $item ) {
- switch ( $item->node_name ) {
- case 'marker':
- break;
-
- case 'A':
- $this->run_adoption_agency_algorithm();
- $this->state->active_formatting_elements->remove_node( $item );
- $this->state->stack_of_open_elements->remove_node( $item );
- break;
- }
- }
-
- $this->reconstruct_active_formatting_elements();
- $this->insert_html_element( $this->state->current_token );
- $this->state->active_formatting_elements->push( $this->state->current_token );
- return true;
-
- /*
- * > A start tag whose tag name is one of: "b", "big", "code", "em", "font", "i",
- * > "s", "small", "strike", "strong", "tt", "u"
- */
- case '+B':
- case '+BIG':
- case '+CODE':
- case '+EM':
- case '+FONT':
- case '+I':
- case '+S':
- case '+SMALL':
- case '+STRIKE':
- case '+STRONG':
- case '+TT':
- case '+U':
- $this->reconstruct_active_formatting_elements();
- $this->insert_html_element( $this->state->current_token );
- $this->state->active_formatting_elements->push( $this->state->current_token );
- return true;
-
- /*
- * > An end tag whose tag name is one of: "a", "b", "big", "code", "em", "font", "i",
- * > "nobr", "s", "small", "strike", "strong", "tt", "u"
- */
- case '-A':
- case '-B':
- case '-BIG':
- case '-CODE':
- case '-EM':
- case '-FONT':
- case '-I':
- case '-S':
- case '-SMALL':
- case '-STRIKE':
- case '-STRONG':
- case '-TT':
- case '-U':
- $this->run_adoption_agency_algorithm();
- return true;
-
- /*
- * > An end tag whose tag name is "br"
- * > Parse error. Drop the attributes from the token, and act as described in the next
- * > entry; i.e. act as if this was a "br" start tag token with no attributes, rather
- * > than the end tag token that it actually is.
- */
- case '-BR':
- $this->last_error = self::ERROR_UNSUPPORTED;
- throw new WP_HTML_Unsupported_Exception( 'Closing BR tags require unimplemented special handling.' );
-
- /*
- * > A start tag whose tag name is one of: "area", "br", "embed", "img", "keygen", "wbr"
- */
- case '+AREA':
- case '+BR':
- case '+EMBED':
- case '+IMG':
- case '+KEYGEN':
- case '+WBR':
- $this->reconstruct_active_formatting_elements();
- $this->insert_html_element( $this->state->current_token );
- $this->state->frameset_ok = false;
- return true;
-
- /*
- * > A start tag whose tag name is "input"
- */
- case '+INPUT':
- $this->reconstruct_active_formatting_elements();
- $this->insert_html_element( $this->state->current_token );
- $type_attribute = $this->get_attribute( 'type' );
- /*
- * > If the token does not have an attribute with the name "type", or if it does,
- * > but that attribute's value is not an ASCII case-insensitive match for the
- * > string "hidden", then: set the frameset-ok flag to "not ok".
- */
- if ( ! is_string( $type_attribute ) || 'hidden' !== strtolower( $type_attribute ) ) {
- $this->state->frameset_ok = false;
- }
- return true;
-
- /*
- * > A start tag whose tag name is "hr"
- */
- case '+HR':
- if ( $this->state->stack_of_open_elements->has_p_in_button_scope() ) {
- $this->close_a_p_element();
- }
- $this->insert_html_element( $this->state->current_token );
- $this->state->frameset_ok = false;
- return true;
-
- /*
- * > A start tag whose tag name is one of: "param", "source", "track"
- */
- case '+PARAM':
- case '+SOURCE':
- case '+TRACK':
- $this->insert_html_element( $this->state->current_token );
- return true;
- }
-
- /*
- * These tags require special handling in the 'in body' insertion mode
- * but that handling hasn't yet been implemented.
- *
- * As the rules for each tag are implemented, the corresponding tag
- * name should be removed from this list. An accompanying test should
- * help ensure this list is maintained.
- *
- * @see Tests_HtmlApi_WpHtmlProcessor::test_step_in_body_fails_on_unsupported_tags
- *
- * Since this switch structure throws a WP_HTML_Unsupported_Exception, it's
- * possible to handle "any other start tag" and "any other end tag" below,
- * as that guarantees execution doesn't proceed for the unimplemented tags.
- *
- * @see https://html.spec.whatwg.org/multipage/parsing.html#parsing-main-inbody
- */
- switch ( $token_name ) {
- case 'APPLET':
- case 'BASE':
- case 'BASEFONT':
- case 'BGSOUND':
- case 'BODY':
- case 'CAPTION':
- case 'COL':
- case 'COLGROUP':
- case 'FORM':
- case 'FRAME':
- case 'FRAMESET':
- case 'HEAD':
- case 'HTML':
- case 'IFRAME':
- case 'LINK':
- case 'MARQUEE':
- case 'MATH':
- case 'META':
- case 'NOBR':
- case 'NOEMBED':
- case 'NOFRAMES':
- case 'NOSCRIPT':
- case 'OBJECT':
- case 'OPTGROUP':
- case 'OPTION':
- case 'PLAINTEXT':
- case 'RB':
- case 'RP':
- case 'RT':
- case 'RTC':
- case 'SARCASM':
- case 'SCRIPT':
- case 'SELECT':
- case 'STYLE':
- case 'SVG':
- case 'TABLE':
- case 'TBODY':
- case 'TD':
- case 'TEMPLATE':
- case 'TEXTAREA':
- case 'TFOOT':
- case 'TH':
- case 'THEAD':
- case 'TITLE':
- case 'TR':
- case 'XMP':
- $this->last_error = self::ERROR_UNSUPPORTED;
- throw new WP_HTML_Unsupported_Exception( "Cannot process {$token_name} element." );
- }
-
- if ( ! parent::is_tag_closer() ) {
- /*
- * > Any other start tag
- */
- $this->reconstruct_active_formatting_elements();
- $this->insert_html_element( $this->state->current_token );
- return true;
- } else {
- /*
- * > Any other end tag
- */
-
- /*
- * Find the corresponding tag opener in the stack of open elements, if
- * it exists before reaching a special element, which provides a kind
- * of boundary in the stack. For example, a `` should not
- * close anything beyond its containing `P` or `DIV` element.
- */
- foreach ( $this->state->stack_of_open_elements->walk_up() as $node ) {
- if ( $token_name === $node->node_name ) {
- break;
- }
-
- if ( self::is_special( $node->node_name ) ) {
- // This is a parse error, ignore the token.
- return $this->step();
- }
- }
-
- $this->generate_implied_end_tags( $token_name );
- if ( $node !== $this->state->stack_of_open_elements->current_node() ) {
- // @todo Record parse error: this error doesn't impact parsing.
- }
-
- foreach ( $this->state->stack_of_open_elements->walk_up() as $item ) {
- $this->state->stack_of_open_elements->pop();
- if ( $node === $item ) {
- return true;
- }
- }
- }
- }
-
- /*
- * Internal helpers
- */
-
- /**
- * Creates a new bookmark for the currently-matched token and returns the generated name.
- *
- * @since 6.4.0
- * @since 6.5.0 Renamed from bookmark_tag() to bookmark_token().
- *
- * @throws Exception When unable to allocate requested bookmark.
- *
- * @return string|false Name of created bookmark, or false if unable to create.
- */
- private function bookmark_token() {
- if ( ! parent::set_bookmark( ++$this->bookmark_counter ) ) {
- $this->last_error = self::ERROR_EXCEEDED_MAX_BOOKMARKS;
- throw new Exception( 'could not allocate bookmark' );
- }
-
- return "{$this->bookmark_counter}";
- }
-
- /*
- * HTML semantic overrides for Tag Processor
- */
-
- /**
- * Returns the uppercase name of the matched tag.
- *
- * The semantic rules for HTML specify that certain tags be reprocessed
- * with a different tag name. Because of this, the tag name presented
- * by the HTML Processor may differ from the one reported by the HTML
- * Tag Processor, which doesn't apply these semantic rules.
- *
- * Example:
- *
- * $processor = new WP_HTML_Tag_Processor( 'Test
' );
- * $processor->next_tag() === true;
- * $processor->get_tag() === 'DIV';
- *
- * $processor->next_tag() === false;
- * $processor->get_tag() === null;
- *
- * @since 6.4.0
- *
- * @return string|null Name of currently matched tag in input HTML, or `null` if none found.
- */
- public function get_tag() {
- if ( null !== $this->last_error ) {
- return null;
- }
-
- if ( $this->is_virtual() ) {
- return $this->current_element->token->node_name;
- }
-
- $tag_name = parent::get_tag();
-
- switch ( $tag_name ) {
- case 'IMAGE':
- /*
- * > A start tag whose tag name is "image"
- * > Change the token's tag name to "img" and reprocess it. (Don't ask.)
- */
- return 'IMG';
-
- default:
- return $tag_name;
- }
- }
-
- /**
- * Indicates if the currently matched tag contains the self-closing flag.
- *
- * No HTML elements ought to have the self-closing flag and for those, the self-closing
- * flag will be ignored. For void elements this is benign because they "self close"
- * automatically. For non-void HTML elements though problems will appear if someone
- * intends to use a self-closing element in place of that element with an empty body.
- * For HTML foreign elements and custom elements the self-closing flag determines if
- * they self-close or not.
- *
- * This function does not determine if a tag is self-closing,
- * but only if the self-closing flag is present in the syntax.
- *
- * @since 6.6.0 Subclassed for the HTML Processor.
- *
- * @return bool Whether the currently matched tag contains the self-closing flag.
- */
- public function has_self_closing_flag() {
- return $this->is_virtual() ? false : parent::has_self_closing_flag();
- }
-
- /**
- * Returns the node name represented by the token.
- *
- * This matches the DOM API value `nodeName`. Some values
- * are static, such as `#text` for a text node, while others
- * are dynamically generated from the token itself.
- *
- * Dynamic names:
- * - Uppercase tag name for tag matches.
- * - `html` for DOCTYPE declarations.
- *
- * Note that if the Tag Processor is not matched on a token
- * then this function will return `null`, either because it
- * hasn't yet found a token or because it reached the end
- * of the document without matching a token.
- *
- * @since 6.6.0 Subclassed for the HTML Processor.
- *
- * @return string|null Name of the matched token.
- */
- public function get_token_name() {
- return $this->is_virtual()
- ? $this->current_element->token->node_name
- : parent::get_token_name();
- }
-
- /**
- * Indicates the kind of matched token, if any.
- *
- * This differs from `get_token_name()` in that it always
- * returns a static string indicating the type, whereas
- * `get_token_name()` may return values derived from the
- * token itself, such as a tag name or processing
- * instruction tag.
- *
- * Possible values:
- * - `#tag` when matched on a tag.
- * - `#text` when matched on a text node.
- * - `#cdata-section` when matched on a CDATA node.
- * - `#comment` when matched on a comment.
- * - `#doctype` when matched on a DOCTYPE declaration.
- * - `#presumptuous-tag` when matched on an empty tag closer.
- * - `#funky-comment` when matched on a funky comment.
- *
- * @since 6.6.0 Subclassed for the HTML Processor.
- *
- * @return string|null What kind of token is matched, or null.
- */
- public function get_token_type() {
- if ( $this->is_virtual() ) {
- /*
- * This logic comes from the Tag Processor.
- *
- * @todo It would be ideal not to repeat this here, but it's not clearly
- * better to allow passing a token name to `get_token_type()`.
- */
- $node_name = $this->current_element->token->node_name;
- $starting_char = $node_name[0];
- if ( 'A' <= $starting_char && 'Z' >= $starting_char ) {
- return '#tag';
- }
-
- if ( 'html' === $node_name ) {
- return '#doctype';
- }
-
- return $node_name;
- }
-
- return parent::get_token_type();
- }
-
- /**
- * Returns the value of a requested attribute from a matched tag opener if that attribute exists.
- *
- * Example:
- *
- * $p = WP_HTML_Processor::create_fragment( 'Test
' );
- * $p->next_token() === true;
- * $p->get_attribute( 'data-test-id' ) === '14';
- * $p->get_attribute( 'enabled' ) === true;
- * $p->get_attribute( 'aria-label' ) === null;
- *
- * $p->next_tag() === false;
- * $p->get_attribute( 'class' ) === null;
- *
- * @since 6.6.0 Subclassed for HTML Processor.
- *
- * @param string $name Name of attribute whose value is requested.
- * @return string|true|null Value of attribute or `null` if not available. Boolean attributes return `true`.
- */
- public function get_attribute( $name ) {
- return $this->is_virtual() ? null : parent::get_attribute( $name );
- }
-
- /**
- * Updates or creates a new attribute on the currently matched tag with the passed value.
- *
- * For boolean attributes special handling is provided:
- * - When `true` is passed as the value, then only the attribute name is added to the tag.
- * - When `false` is passed, the attribute gets removed if it existed before.
- *
- * For string attributes, the value is escaped using the `esc_attr` function.
- *
- * @since 6.6.0 Subclassed for the HTML Processor.
- *
- * @param string $name The attribute name to target.
- * @param string|bool $value The new attribute value.
- * @return bool Whether an attribute value was set.
- */
- public function set_attribute( $name, $value ) {
- return $this->is_virtual() ? false : parent::set_attribute( $name, $value );
- }
-
- /**
- * Remove an attribute from the currently-matched tag.
- *
- * @since 6.6.0 Subclassed for HTML Processor.
- *
- * @param string $name The attribute name to remove.
- * @return bool Whether an attribute was removed.
- */
- public function remove_attribute( $name ) {
- return $this->is_virtual() ? false : parent::remove_attribute( $name );
- }
-
- /**
- * Gets lowercase names of all attributes matching a given prefix in the current tag.
- *
- * Note that matching is case-insensitive. This is in accordance with the spec:
- *
- * > There must never be two or more attributes on
- * > the same start tag whose names are an ASCII
- * > case-insensitive match for each other.
- * - HTML 5 spec
- *
- * Example:
- *
- * $p = new WP_HTML_Tag_Processor( 'Test
' );
- * $p->next_tag( array( 'class_name' => 'test' ) ) === true;
- * $p->get_attribute_names_with_prefix( 'data-' ) === array( 'data-enabled', 'data-test-id' );
- *
- * $p->next_tag() === false;
- * $p->get_attribute_names_with_prefix( 'data-' ) === null;
- *
- * @since 6.6.0 Subclassed for the HTML Processor.
- *
- * @see https://html.spec.whatwg.org/multipage/syntax.html#attributes-2:ascii-case-insensitive
- *
- * @param string $prefix Prefix of requested attribute names.
- * @return array|null List of attribute names, or `null` when no tag opener is matched.
- */
- public function get_attribute_names_with_prefix( $prefix ) {
- return $this->is_virtual() ? null : parent::get_attribute_names_with_prefix( $prefix );
- }
-
- /**
- * Adds a new class name to the currently matched tag.
- *
- * @since 6.6.0 Subclassed for the HTML Processor.
- *
- * @param string $class_name The class name to add.
- * @return bool Whether the class was set to be added.
- */
- public function add_class( $class_name ) {
- return $this->is_virtual() ? false : parent::add_class( $class_name );
- }
-
- /**
- * Removes a class name from the currently matched tag.
- *
- * @since 6.6.0 Subclassed for the HTML Processor.
- *
- * @param string $class_name The class name to remove.
- * @return bool Whether the class was set to be removed.
- */
- public function remove_class( $class_name ) {
- return $this->is_virtual() ? false : parent::remove_class( $class_name );
- }
-
- /**
- * Returns if a matched tag contains the given ASCII case-insensitive class name.
- *
- * @since 6.6.0 Subclassed for the HTML Processor.
- *
- * @param string $wanted_class Look for this CSS class name, ASCII case-insensitive.
- * @return bool|null Whether the matched tag contains the given class name, or null if not matched.
- */
- public function has_class( $wanted_class ) {
- return $this->is_virtual() ? null : parent::has_class( $wanted_class );
- }
-
- /**
- * Generator for a foreach loop to step through each class name for the matched tag.
- *
- * This generator function is designed to be used inside a "foreach" loop.
- *
- * Example:
- *
- * $p = WP_HTML_Processor::create_fragment( "" );
- * $p->next_tag();
- * foreach ( $p->class_list() as $class_name ) {
- * echo "{$class_name} ";
- * }
- * // Outputs: "free
lang-en "
- *
- * @since 6.6.0 Subclassed for the HTML Processor.
- */
- public function class_list() {
- return $this->is_virtual() ? null : parent::class_list();
- }
-
- /**
- * Returns the modifiable text for a matched token, or an empty string.
- *
- * Modifiable text is text content that may be read and changed without
- * changing the HTML structure of the document around it. This includes
- * the contents of `#text` nodes in the HTML as well as the inner
- * contents of HTML comments, Processing Instructions, and others, even
- * though these nodes aren't part of a parsed DOM tree. They also contain
- * the contents of SCRIPT and STYLE tags, of TEXTAREA tags, and of any
- * other section in an HTML document which cannot contain HTML markup (DATA).
- *
- * If a token has no modifiable text then an empty string is returned to
- * avoid needless crashing or type errors. An empty string does not mean
- * that a token has modifiable text, and a token with modifiable text may
- * have an empty string (e.g. a comment with no contents).
- *
- * @since 6.6.0 Subclassed for the HTML Processor.
- *
- * @return string
- */
- public function get_modifiable_text() {
- return $this->is_virtual() ? '' : parent::get_modifiable_text();
- }
-
- /**
- * Indicates what kind of comment produced the comment node.
- *
- * Because there are different kinds of HTML syntax which produce
- * comments, the Tag Processor tracks and exposes this as a type
- * for the comment. Nominally only regular HTML comments exist as
- * they are commonly known, but a number of unrelated syntax errors
- * also produce comments.
- *
- * @see self::COMMENT_AS_ABRUPTLY_CLOSED_COMMENT
- * @see self::COMMENT_AS_CDATA_LOOKALIKE
- * @see self::COMMENT_AS_INVALID_HTML
- * @see self::COMMENT_AS_HTML_COMMENT
- * @see self::COMMENT_AS_PI_NODE_LOOKALIKE
- *
- * @since 6.6.0 Subclassed for the HTML Processor.
- *
- * @return string|null
- */
- public function get_comment_type() {
- return $this->is_virtual() ? null : parent::get_comment_type();
- }
-
- /**
- * Removes a bookmark that is no longer needed.
- *
- * Releasing a bookmark frees up the small
- * performance overhead it requires.
- *
- * @since 6.4.0
- *
- * @param string $bookmark_name Name of the bookmark to remove.
- * @return bool Whether the bookmark already existed before removal.
- */
- public function release_bookmark( $bookmark_name ) {
- return parent::release_bookmark( "_{$bookmark_name}" );
- }
-
- /**
- * Moves the internal cursor in the HTML Processor to a given bookmark's location.
- *
- * Be careful! Seeking backwards to a previous location resets the parser to the
- * start of the document and reparses the entire contents up until it finds the
- * sought-after bookmarked location.
- *
- * In order to prevent accidental infinite loops, there's a
- * maximum limit on the number of times seek() can be called.
- *
- * @throws Exception When unable to allocate a bookmark for the next token in the input HTML document.
- *
- * @since 6.4.0
- *
- * @param string $bookmark_name Jump to the place in the document identified by this bookmark name.
- * @return bool Whether the internal cursor was successfully moved to the bookmark's location.
- */
- public function seek( $bookmark_name ) {
- // Flush any pending updates to the document before beginning.
- $this->get_updated_html();
-
- $actual_bookmark_name = "_{$bookmark_name}";
- $processor_started_at = $this->state->current_token
- ? $this->bookmarks[ $this->state->current_token->bookmark_name ]->start
- : 0;
- $bookmark_starts_at = $this->bookmarks[ $actual_bookmark_name ]->start;
- $bookmark_length = $this->bookmarks[ $actual_bookmark_name ]->length;
- $direction = $bookmark_starts_at > $processor_started_at ? 'forward' : 'backward';
-
- /*
- * If seeking backwards, it's possible that the sought-after bookmark exists within an element
- * which has been closed before the current cursor; in other words, it has already been removed
- * from the stack of open elements. This means that it's insufficient to simply pop off elements
- * from the stack of open elements which appear after the bookmarked location and then jump to
- * that location, as the elements which were open before won't be re-opened.
- *
- * In order to maintain consistency, the HTML Processor rewinds to the start of the document
- * and reparses everything until it finds the sought-after bookmark.
- *
- * There are potentially better ways to do this: cache the parser state for each bookmark and
- * restore it when seeking; store an immutable and idempotent register of where elements open
- * and close.
- *
- * If caching the parser state it will be essential to properly maintain the cached stack of
- * open elements and active formatting elements when modifying the document. This could be a
- * tedious and time-consuming process as well, and so for now will not be performed.
- *
- * It may be possible to track bookmarks for where elements open and close, and in doing so
- * be able to quickly recalculate breadcrumbs for any element in the document. It may even
- * be possible to remove the stack of open elements and compute it on the fly this way.
- * If doing this, the parser would need to track the opening and closing locations for all
- * tokens in the breadcrumb path for any and all bookmarks. By utilizing bookmarks themselves
- * this list could be automatically maintained while modifying the document. Finding the
- * breadcrumbs would then amount to traversing that list from the start until the token
- * being inspected. Once an element closes, if there are no bookmarks pointing to locations
- * within that element, then all of these locations may be forgotten to save on memory use
- * and computation time.
- */
- if ( 'backward' === $direction ) {
- /*
- * Instead of clearing the parser state and starting fresh, calling the stack methods
- * maintains the proper flags in the parser.
- */
- foreach ( $this->state->stack_of_open_elements->walk_up() as $item ) {
- if ( 'context-node' === $item->bookmark_name ) {
- break;
- }
-
- $this->state->stack_of_open_elements->remove_node( $item );
- }
-
- foreach ( $this->state->active_formatting_elements->walk_up() as $item ) {
- if ( 'context-node' === $item->bookmark_name ) {
- break;
- }
-
- $this->state->active_formatting_elements->remove_node( $item );
- }
-
- parent::seek( 'context-node' );
- $this->state->insertion_mode = Gutenberg_HTML_Processor_State_6_6::INSERTION_MODE_IN_BODY;
- $this->state->frameset_ok = true;
- $this->element_queue = array();
- $this->current_element = null;
- }
-
- // When moving forwards, reparse the document until reaching the same location as the original bookmark.
- if ( $bookmark_starts_at === $this->bookmarks[ $this->state->current_token->bookmark_name ]->start ) {
- return true;
- }
-
- while ( $this->next_token() ) {
- if ( $bookmark_starts_at === $this->bookmarks[ $this->state->current_token->bookmark_name ]->start ) {
- while ( isset( $this->current_element ) && Gutenberg_HTML_Stack_Event_6_6::POP === $this->current_element->operation ) {
- $this->current_element = array_shift( $this->element_queue );
- }
- return true;
- }
- }
-
- return false;
- }
-
- /**
- * Sets a bookmark in the HTML document.
- *
- * Bookmarks represent specific places or tokens in the HTML
- * document, such as a tag opener or closer. When applying
- * edits to a document, such as setting an attribute, the
- * text offsets of that token may shift; the bookmark is
- * kept updated with those shifts and remains stable unless
- * the entire span of text in which the token sits is removed.
- *
- * Release bookmarks when they are no longer needed.
- *
- * Example:
- *
- * Surprising fact you may not know!
- * ^ ^
- * \-|-- this `H2` opener bookmark tracks the token
- *
- * Surprising fact you may no…
- * ^ ^
- * \-|-- it shifts with edits
- *
- * Bookmarks provide the ability to seek to a previously-scanned
- * place in the HTML document. This avoids the need to re-scan
- * the entire document.
- *
- * Example:
- *
- *
- * ^^^^
- * want to note this last item
- *
- * $p = new WP_HTML_Tag_Processor( $html );
- * $in_list = false;
- * while ( $p->next_tag( array( 'tag_closers' => $in_list ? 'visit' : 'skip' ) ) ) {
- * if ( 'UL' === $p->get_tag() ) {
- * if ( $p->is_tag_closer() ) {
- * $in_list = false;
- * $p->set_bookmark( 'resume' );
- * if ( $p->seek( 'last-li' ) ) {
- * $p->add_class( 'last-li' );
- * }
- * $p->seek( 'resume' );
- * $p->release_bookmark( 'last-li' );
- * $p->release_bookmark( 'resume' );
- * } else {
- * $in_list = true;
- * }
- * }
- *
- * if ( 'LI' === $p->get_tag() ) {
- * $p->set_bookmark( 'last-li' );
- * }
- * }
- *
- * Bookmarks intentionally hide the internal string offsets
- * to which they refer. They are maintained internally as
- * updates are applied to the HTML document and therefore
- * retain their "position" - the location to which they
- * originally pointed. The inability to use bookmarks with
- * functions like `substr` is therefore intentional to guard
- * against accidentally breaking the HTML.
- *
- * Because bookmarks allocate memory and require processing
- * for every applied update, they are limited and require
- * a name. They should not be created with programmatically-made
- * names, such as "li_{$index}" with some loop. As a general
- * rule they should only be created with string-literal names
- * like "start-of-section" or "last-paragraph".
- *
- * Bookmarks are a powerful tool to enable complicated behavior.
- * Consider double-checking that you need this tool if you are
- * reaching for it, as inappropriate use could lead to broken
- * HTML structure or unwanted processing overhead.
- *
- * @since 6.4.0
- *
- * @param string $bookmark_name Identifies this particular bookmark.
- * @return bool Whether the bookmark was successfully created.
- */
- public function set_bookmark( $bookmark_name ) {
- return parent::set_bookmark( "_{$bookmark_name}" );
- }
-
- /**
- * Checks whether a bookmark with the given name exists.
- *
- * @since 6.5.0
- *
- * @param string $bookmark_name Name to identify a bookmark that potentially exists.
- * @return bool Whether that bookmark exists.
- */
- public function has_bookmark( $bookmark_name ) {
- return parent::has_bookmark( "_{$bookmark_name}" );
- }
-
- /*
- * HTML Parsing Algorithms
- */
-
- /**
- * Closes a P element.
- *
- * @since 6.4.0
- *
- * @throws WP_HTML_Unsupported_Exception When encountering unsupported HTML input.
- *
- * @see https://html.spec.whatwg.org/#close-a-p-element
- */
- private function close_a_p_element() {
- $this->generate_implied_end_tags( 'P' );
- $this->state->stack_of_open_elements->pop_until( 'P' );
- }
-
- /**
- * Closes elements that have implied end tags.
- *
- * @since 6.4.0
- *
- * @see https://html.spec.whatwg.org/#generate-implied-end-tags
- *
- * @param string|null $except_for_this_element Perform as if this element doesn't exist in the stack of open elements.
- */
- private function generate_implied_end_tags( $except_for_this_element = null ) {
- $elements_with_implied_end_tags = array(
- 'DD',
- 'DT',
- 'LI',
- 'P',
- );
-
- $current_node = $this->state->stack_of_open_elements->current_node();
- while (
- $current_node && $current_node->node_name !== $except_for_this_element &&
- in_array( $this->state->stack_of_open_elements->current_node(), $elements_with_implied_end_tags, true )
- ) {
- $this->state->stack_of_open_elements->pop();
- }
- }
-
- /**
- * Closes elements that have implied end tags, thoroughly.
- *
- * See the HTML specification for an explanation why this is
- * different from generating end tags in the normal sense.
- *
- * @since 6.4.0
- *
- * @see WP_HTML_Processor::generate_implied_end_tags
- * @see https://html.spec.whatwg.org/#generate-implied-end-tags
- */
- private function generate_implied_end_tags_thoroughly() {
- $elements_with_implied_end_tags = array(
- 'DD',
- 'DT',
- 'LI',
- 'P',
- );
-
- while ( in_array( $this->state->stack_of_open_elements->current_node(), $elements_with_implied_end_tags, true ) ) {
- $this->state->stack_of_open_elements->pop();
- }
- }
-
- /**
- * Reconstructs the active formatting elements.
- *
- * > This has the effect of reopening all the formatting elements that were opened
- * > in the current body, cell, or caption (whichever is youngest) that haven't
- * > been explicitly closed.
- *
- * @since 6.4.0
- *
- * @throws WP_HTML_Unsupported_Exception When encountering unsupported HTML input.
- *
- * @see https://html.spec.whatwg.org/#reconstruct-the-active-formatting-elements
- *
- * @return bool Whether any formatting elements needed to be reconstructed.
- */
- private function reconstruct_active_formatting_elements() {
- /*
- * > If there are no entries in the list of active formatting elements, then there is nothing
- * > to reconstruct; stop this algorithm.
- */
- if ( 0 === $this->state->active_formatting_elements->count() ) {
- return false;
- }
-
- $last_entry = $this->state->active_formatting_elements->current_node();
- if (
-
- /*
- * > If the last (most recently added) entry in the list of active formatting elements is a marker;
- * > stop this algorithm.
- */
- 'marker' === $last_entry->node_name ||
-
- /*
- * > If the last (most recently added) entry in the list of active formatting elements is an
- * > element that is in the stack of open elements, then there is nothing to reconstruct;
- * > stop this algorithm.
- */
- $this->state->stack_of_open_elements->contains_node( $last_entry )
- ) {
- return false;
- }
-
- $this->last_error = self::ERROR_UNSUPPORTED;
- throw new WP_HTML_Unsupported_Exception( 'Cannot reconstruct active formatting elements when advancing and rewinding is required.' );
- }
-
- /**
- * Runs the adoption agency algorithm.
- *
- * @since 6.4.0
- *
- * @throws WP_HTML_Unsupported_Exception When encountering unsupported HTML input.
- *
- * @see https://html.spec.whatwg.org/#adoption-agency-algorithm
- */
- private function run_adoption_agency_algorithm() {
- $budget = 1000;
- $subject = $this->get_tag();
- $current_node = $this->state->stack_of_open_elements->current_node();
-
- if (
- // > If the current node is an HTML element whose tag name is subject
- $current_node && $subject === $current_node->node_name &&
- // > the current node is not in the list of active formatting elements
- ! $this->state->active_formatting_elements->contains_node( $current_node )
- ) {
- $this->state->stack_of_open_elements->pop();
- return;
- }
-
- $outer_loop_counter = 0;
- while ( $budget-- > 0 ) {
- if ( $outer_loop_counter++ >= 8 ) {
- return;
- }
-
- /*
- * > Let formatting element be the last element in the list of active formatting elements that:
- * > - is between the end of the list and the last marker in the list,
- * > if any, or the start of the list otherwise,
- * > - and has the tag name subject.
- */
- $formatting_element = null;
- foreach ( $this->state->active_formatting_elements->walk_up() as $item ) {
- if ( 'marker' === $item->node_name ) {
- break;
- }
-
- if ( $subject === $item->node_name ) {
- $formatting_element = $item;
- break;
- }
- }
-
- // > If there is no such element, then return and instead act as described in the "any other end tag" entry above.
- if ( null === $formatting_element ) {
- $this->last_error = self::ERROR_UNSUPPORTED;
- throw new WP_HTML_Unsupported_Exception( 'Cannot run adoption agency when "any other end tag" is required.' );
- }
-
- // > If formatting element is not in the stack of open elements, then this is a parse error; remove the element from the list, and return.
- if ( ! $this->state->stack_of_open_elements->contains_node( $formatting_element ) ) {
- $this->state->active_formatting_elements->remove_node( $formatting_element );
- return;
- }
-
- // > If formatting element is in the stack of open elements, but the element is not in scope, then this is a parse error; return.
- if ( ! $this->state->stack_of_open_elements->has_element_in_scope( $formatting_element->node_name ) ) {
- return;
- }
-
- /*
- * > Let furthest block be the topmost node in the stack of open elements that is lower in the stack
- * > than formatting element, and is an element in the special category. There might not be one.
- */
- $is_above_formatting_element = true;
- $furthest_block = null;
- foreach ( $this->state->stack_of_open_elements->walk_down() as $item ) {
- if ( $is_above_formatting_element && $formatting_element->bookmark_name !== $item->bookmark_name ) {
- continue;
- }
-
- if ( $is_above_formatting_element ) {
- $is_above_formatting_element = false;
- continue;
- }
-
- if ( self::is_special( $item->node_name ) ) {
- $furthest_block = $item;
- break;
- }
- }
-
- /*
- * > If there is no furthest block, then the UA must first pop all the nodes from the bottom of the
- * > stack of open elements, from the current node up to and including formatting element, then
- * > remove formatting element from the list of active formatting elements, and finally return.
- */
- if ( null === $furthest_block ) {
- foreach ( $this->state->stack_of_open_elements->walk_up() as $item ) {
- $this->state->stack_of_open_elements->pop();
-
- if ( $formatting_element->bookmark_name === $item->bookmark_name ) {
- $this->state->active_formatting_elements->remove_node( $formatting_element );
- return;
- }
- }
- }
-
- $this->last_error = self::ERROR_UNSUPPORTED;
- throw new WP_HTML_Unsupported_Exception( 'Cannot extract common ancestor in adoption agency algorithm.' );
- }
-
- $this->last_error = self::ERROR_UNSUPPORTED;
- throw new WP_HTML_Unsupported_Exception( 'Cannot run adoption agency when looping required.' );
- }
-
- /**
- * Inserts an HTML element on the stack of open elements.
- *
- * @since 6.4.0
- *
- * @see https://html.spec.whatwg.org/#insert-a-foreign-element
- *
- * @param WP_HTML_Token $token Name of bookmark pointing to element in original input HTML.
- */
- private function insert_html_element( $token ) {
- $this->state->stack_of_open_elements->push( $token );
- }
-
- /*
- * HTML Specification Helpers
- */
-
- /**
- * Returns whether an element of a given name is in the HTML special category.
- *
- * @since 6.4.0
- *
- * @see https://html.spec.whatwg.org/#special
- *
- * @param string $tag_name Name of element to check.
- * @return bool Whether the element of the given name is in the special category.
- */
- public static function is_special( $tag_name ) {
- $tag_name = strtoupper( $tag_name );
-
- return (
- 'ADDRESS' === $tag_name ||
- 'APPLET' === $tag_name ||
- 'AREA' === $tag_name ||
- 'ARTICLE' === $tag_name ||
- 'ASIDE' === $tag_name ||
- 'BASE' === $tag_name ||
- 'BASEFONT' === $tag_name ||
- 'BGSOUND' === $tag_name ||
- 'BLOCKQUOTE' === $tag_name ||
- 'BODY' === $tag_name ||
- 'BR' === $tag_name ||
- 'BUTTON' === $tag_name ||
- 'CAPTION' === $tag_name ||
- 'CENTER' === $tag_name ||
- 'COL' === $tag_name ||
- 'COLGROUP' === $tag_name ||
- 'DD' === $tag_name ||
- 'DETAILS' === $tag_name ||
- 'DIR' === $tag_name ||
- 'DIV' === $tag_name ||
- 'DL' === $tag_name ||
- 'DT' === $tag_name ||
- 'EMBED' === $tag_name ||
- 'FIELDSET' === $tag_name ||
- 'FIGCAPTION' === $tag_name ||
- 'FIGURE' === $tag_name ||
- 'FOOTER' === $tag_name ||
- 'FORM' === $tag_name ||
- 'FRAME' === $tag_name ||
- 'FRAMESET' === $tag_name ||
- 'H1' === $tag_name ||
- 'H2' === $tag_name ||
- 'H3' === $tag_name ||
- 'H4' === $tag_name ||
- 'H5' === $tag_name ||
- 'H6' === $tag_name ||
- 'HEAD' === $tag_name ||
- 'HEADER' === $tag_name ||
- 'HGROUP' === $tag_name ||
- 'HR' === $tag_name ||
- 'HTML' === $tag_name ||
- 'IFRAME' === $tag_name ||
- 'IMG' === $tag_name ||
- 'INPUT' === $tag_name ||
- 'KEYGEN' === $tag_name ||
- 'LI' === $tag_name ||
- 'LINK' === $tag_name ||
- 'LISTING' === $tag_name ||
- 'MAIN' === $tag_name ||
- 'MARQUEE' === $tag_name ||
- 'MENU' === $tag_name ||
- 'META' === $tag_name ||
- 'NAV' === $tag_name ||
- 'NOEMBED' === $tag_name ||
- 'NOFRAMES' === $tag_name ||
- 'NOSCRIPT' === $tag_name ||
- 'OBJECT' === $tag_name ||
- 'OL' === $tag_name ||
- 'P' === $tag_name ||
- 'PARAM' === $tag_name ||
- 'PLAINTEXT' === $tag_name ||
- 'PRE' === $tag_name ||
- 'SCRIPT' === $tag_name ||
- 'SEARCH' === $tag_name ||
- 'SECTION' === $tag_name ||
- 'SELECT' === $tag_name ||
- 'SOURCE' === $tag_name ||
- 'STYLE' === $tag_name ||
- 'SUMMARY' === $tag_name ||
- 'TABLE' === $tag_name ||
- 'TBODY' === $tag_name ||
- 'TD' === $tag_name ||
- 'TEMPLATE' === $tag_name ||
- 'TEXTAREA' === $tag_name ||
- 'TFOOT' === $tag_name ||
- 'TH' === $tag_name ||
- 'THEAD' === $tag_name ||
- 'TITLE' === $tag_name ||
- 'TR' === $tag_name ||
- 'TRACK' === $tag_name ||
- 'UL' === $tag_name ||
- 'WBR' === $tag_name ||
- 'XMP' === $tag_name ||
-
- // MathML.
- 'MI' === $tag_name ||
- 'MO' === $tag_name ||
- 'MN' === $tag_name ||
- 'MS' === $tag_name ||
- 'MTEXT' === $tag_name ||
- 'ANNOTATION-XML' === $tag_name ||
-
- // SVG.
- 'FOREIGNOBJECT' === $tag_name ||
- 'DESC' === $tag_name ||
- 'TITLE' === $tag_name
- );
- }
-
- /**
- * Returns whether a given element is an HTML Void Element
- *
- * > area, base, br, col, embed, hr, img, input, link, meta, source, track, wbr
- *
- * @since 6.4.0
- *
- * @see https://html.spec.whatwg.org/#void-elements
- *
- * @param string $tag_name Name of HTML tag to check.
- * @return bool Whether the given tag is an HTML Void Element.
- */
- public static function is_void( $tag_name ) {
- $tag_name = strtoupper( $tag_name );
-
- return (
- 'AREA' === $tag_name ||
- 'BASE' === $tag_name ||
- 'BASEFONT' === $tag_name || // Obsolete but still treated as void.
- 'BGSOUND' === $tag_name || // Obsolete but still treated as void.
- 'BR' === $tag_name ||
- 'COL' === $tag_name ||
- 'EMBED' === $tag_name ||
- 'FRAME' === $tag_name ||
- 'HR' === $tag_name ||
- 'IMG' === $tag_name ||
- 'INPUT' === $tag_name ||
- 'KEYGEN' === $tag_name || // Obsolete but still treated as void.
- 'LINK' === $tag_name ||
- 'META' === $tag_name ||
- 'PARAM' === $tag_name || // Obsolete but still treated as void.
- 'SOURCE' === $tag_name ||
- 'TRACK' === $tag_name ||
- 'WBR' === $tag_name
- );
- }
-
- /*
- * Constants that would pollute the top of the class if they were found there.
- */
-
- /**
- * Indicates that the next HTML token should be parsed and processed.
- *
- * @since 6.4.0
- *
- * @var string
- */
- const PROCESS_NEXT_NODE = 'process-next-node';
-
- /**
- * Indicates that the current HTML token should be reprocessed in the newly-selected insertion mode.
- *
- * @since 6.4.0
- *
- * @var string
- */
- const REPROCESS_CURRENT_NODE = 'reprocess-current-node';
-
- /**
- * Indicates that the current HTML token should be processed without advancing the parser.
- *
- * @since 6.5.0
- *
- * @var string
- */
- const PROCESS_CURRENT_NODE = 'process-current-node';
-
- /**
- * Indicates that the parser encountered unsupported markup and has bailed.
- *
- * @since 6.4.0
- *
- * @var string
- */
- const ERROR_UNSUPPORTED = 'unsupported';
-
- /**
- * Indicates that the parser encountered more HTML tokens than it
- * was able to process and has bailed.
- *
- * @since 6.4.0
- *
- * @var string
- */
- const ERROR_EXCEEDED_MAX_BOOKMARKS = 'exceeded-max-bookmarks';
-
- /**
- * Unlock code that must be passed into the constructor to create this class.
- *
- * This class extends the WP_HTML_Tag_Processor, which has a public class
- * constructor. Therefore, it's not possible to have a private constructor here.
- *
- * This unlock code is used to ensure that anyone calling the constructor is
- * doing so with a full understanding that it's intended to be a private API.
- *
- * @access private
- */
- const CONSTRUCTOR_UNLOCK_CODE = 'Use WP_HTML_Processor::create_fragment() instead of calling the class constructor directly.';
-}
diff --git a/lib/compat/wordpress-6.6/html-api/class-gutenberg-html-processor-state-6-6.php b/lib/compat/wordpress-6.6/html-api/class-gutenberg-html-processor-state-6-6.php
deleted file mode 100644
index 69896986d53f0..0000000000000
--- a/lib/compat/wordpress-6.6/html-api/class-gutenberg-html-processor-state-6-6.php
+++ /dev/null
@@ -1,143 +0,0 @@
- The frameset-ok flag is set to "ok" when the parser is created. It is set to "not ok" after certain tokens are seen.
- *
- * @since 6.4.0
- *
- * @see https://html.spec.whatwg.org/#frameset-ok-flag
- *
- * @var bool
- */
- public $frameset_ok = true;
-
- /**
- * Constructor - creates a new and empty state value.
- *
- * @since 6.4.0
- *
- * @see WP_HTML_Processor
- */
- public function __construct() {
- $this->stack_of_open_elements = new Gutenberg_HTML_Open_Elements_6_6();
- $this->active_formatting_elements = new WP_HTML_Active_Formatting_Elements();
- }
-}
diff --git a/lib/compat/wordpress-6.6/html-api/class-gutenberg-html-stack-event-6-6.php b/lib/compat/wordpress-6.6/html-api/class-gutenberg-html-stack-event-6-6.php
deleted file mode 100644
index 0be6e709363bb..0000000000000
--- a/lib/compat/wordpress-6.6/html-api/class-gutenberg-html-stack-event-6-6.php
+++ /dev/null
@@ -1,82 +0,0 @@
-token = $token;
- $this->operation = $operation;
- $this->provenance = $provenance;
- }
-}
diff --git a/lib/compat/wordpress-6.6/html-api/class-gutenberg-html-tag-processor-6-6.php b/lib/compat/wordpress-6.6/html-api/class-gutenberg-html-tag-processor-6-6.php
deleted file mode 100644
index ecbc6d0d7b6af..0000000000000
--- a/lib/compat/wordpress-6.6/html-api/class-gutenberg-html-tag-processor-6-6.php
+++ /dev/null
@@ -1,3559 +0,0 @@
- "c" not " c".
- * This would increase the size of the changes for some operations but leave more
- * natural-looking output HTML.
- *
- * @package WordPress
- * @subpackage HTML-API
- * @since 6.2.0
- */
-
-/**
- * Core class used to modify attributes in an HTML document for tags matching a query.
- *
- * ## Usage
- *
- * Use of this class requires three steps:
- *
- * 1. Create a new class instance with your input HTML document.
- * 2. Find the tag(s) you are looking for.
- * 3. Request changes to the attributes in those tag(s).
- *
- * Example:
- *
- * $tags = new WP_HTML_Tag_Processor( $html );
- * if ( $tags->next_tag( 'option' ) ) {
- * $tags->set_attribute( 'selected', true );
- * }
- *
- * ### Finding tags
- *
- * The `next_tag()` function moves the internal cursor through
- * your input HTML document until it finds a tag meeting any of
- * the supplied restrictions in the optional query argument. If
- * no argument is provided then it will find the next HTML tag,
- * regardless of what kind it is.
- *
- * If you want to _find whatever the next tag is_:
- *
- * $tags->next_tag();
- *
- * | Goal | Query |
- * |-----------------------------------------------------------|---------------------------------------------------------------------------------|
- * | Find any tag. | `$tags->next_tag();` |
- * | Find next image tag. | `$tags->next_tag( array( 'tag_name' => 'img' ) );` |
- * | Find next image tag (without passing the array). | `$tags->next_tag( 'img' );` |
- * | Find next tag containing the `fullwidth` CSS class. | `$tags->next_tag( array( 'class_name' => 'fullwidth' ) );` |
- * | Find next image tag containing the `fullwidth` CSS class. | `$tags->next_tag( array( 'tag_name' => 'img', 'class_name' => 'fullwidth' ) );` |
- *
- * If a tag was found meeting your criteria then `next_tag()`
- * will return `true` and you can proceed to modify it. If it
- * returns `false`, however, it failed to find the tag and
- * moved the cursor to the end of the file.
- *
- * Once the cursor reaches the end of the file the processor
- * is done and if you want to reach an earlier tag you will
- * need to recreate the processor and start over, as it's
- * unable to back up or move in reverse.
- *
- * See the section on bookmarks for an exception to this
- * no-backing-up rule.
- *
- * #### Custom queries
- *
- * Sometimes it's necessary to further inspect an HTML tag than
- * the query syntax here permits. In these cases one may further
- * inspect the search results using the read-only functions
- * provided by the processor or external state or variables.
- *
- * Example:
- *
- * // Paint up to the first five DIV or SPAN tags marked with the "jazzy" style.
- * $remaining_count = 5;
- * while ( $remaining_count > 0 && $tags->next_tag() ) {
- * if (
- * ( 'DIV' === $tags->get_tag() || 'SPAN' === $tags->get_tag() ) &&
- * 'jazzy' === $tags->get_attribute( 'data-style' )
- * ) {
- * $tags->add_class( 'theme-style-everest-jazz' );
- * $remaining_count--;
- * }
- * }
- *
- * `get_attribute()` will return `null` if the attribute wasn't present
- * on the tag when it was called. It may return `""` (the empty string)
- * in cases where the attribute was present but its value was empty.
- * For boolean attributes, those whose name is present but no value is
- * given, it will return `true` (the only way to set `false` for an
- * attribute is to remove it).
- *
- * #### When matching fails
- *
- * When `next_tag()` returns `false` it could mean different things:
- *
- * - The requested tag wasn't found in the input document.
- * - The input document ended in the middle of an HTML syntax element.
- *
- * When a document ends in the middle of a syntax element it will pause
- * the processor. This is to make it possible in the future to extend the
- * input document and proceed - an important requirement for chunked
- * streaming parsing of a document.
- *
- * Example:
- *
- * $processor = new WP_HTML_Tag_Processor( 'This ` inside an HTML comment.
- * - STYLE content is raw text.
- * - TITLE content is plain text but character references are decoded.
- * - TEXTAREA content is plain text but character references are decoded.
- * - XMP (deprecated) content is raw text.
- *
- * ### Modifying HTML attributes for a found tag
- *
- * Once you've found the start of an opening tag you can modify
- * any number of the attributes on that tag. You can set a new
- * value for an attribute, remove the entire attribute, or do
- * nothing and move on to the next opening tag.
- *
- * Example:
- *
- * if ( $tags->next_tag( array( 'class_name' => 'wp-group-block' ) ) ) {
- * $tags->set_attribute( 'title', 'This groups the contained content.' );
- * $tags->remove_attribute( 'data-test-id' );
- * }
- *
- * If `set_attribute()` is called for an existing attribute it will
- * overwrite the existing value. Similarly, calling `remove_attribute()`
- * for a non-existing attribute has no effect on the document. Both
- * of these methods are safe to call without knowing if a given attribute
- * exists beforehand.
- *
- * ### Modifying CSS classes for a found tag
- *
- * The tag processor treats the `class` attribute as a special case.
- * Because it's a common operation to add or remove CSS classes, this
- * interface adds helper methods to make that easier.
- *
- * As with attribute values, adding or removing CSS classes is a safe
- * operation that doesn't require checking if the attribute or class
- * exists before making changes. If removing the only class then the
- * entire `class` attribute will be removed.
- *
- * Example:
- *
- * // from `
Yippee! `
- * // to `
Yippee! `
- * $tags->add_class( 'is-active' );
- *
- * // from `
Yippee! `
- * // to `
Yippee! `
- * $tags->add_class( 'is-active' );
- *
- * // from `
Yippee! `
- * // to `
Yippee! `
- * $tags->add_class( 'is-active' );
- *
- * // from `
`
- * // to `
- * $tags->remove_class( 'rugby' );
- *
- * // from `
`
- * // to `
- * $tags->remove_class( 'rugby' );
- *
- * // from `
`
- * // to `
- * $tags->remove_class( 'rugby' );
- *
- * When class changes are enqueued but a direct change to `class` is made via
- * `set_attribute` then the changes to `set_attribute` (or `remove_attribute`)
- * will take precedence over those made through `add_class` and `remove_class`.
- *
- * ### Bookmarks
- *
- * While scanning through the input HTMl document it's possible to set
- * a named bookmark when a particular tag is found. Later on, after
- * continuing to scan other tags, it's possible to `seek` to one of
- * the set bookmarks and then proceed again from that point forward.
- *
- * Because bookmarks create processing overhead one should avoid
- * creating too many of them. As a rule, create only bookmarks
- * of known string literal names; avoid creating "mark_{$index}"
- * and so on. It's fine from a performance standpoint to create a
- * bookmark and update it frequently, such as within a loop.
- *
- * $total_todos = 0;
- * while ( $p->next_tag( array( 'tag_name' => 'UL', 'class_name' => 'todo' ) ) ) {
- * $p->set_bookmark( 'list-start' );
- * while ( $p->next_tag( array( 'tag_closers' => 'visit' ) ) ) {
- * if ( 'UL' === $p->get_tag() && $p->is_tag_closer() ) {
- * $p->set_bookmark( 'list-end' );
- * $p->seek( 'list-start' );
- * $p->set_attribute( 'data-contained-todos', (string) $total_todos );
- * $total_todos = 0;
- * $p->seek( 'list-end' );
- * break;
- * }
- *
- * if ( 'LI' === $p->get_tag() && ! $p->is_tag_closer() ) {
- * $total_todos++;
- * }
- * }
- * }
- *
- * ## Tokens and finer-grained processing.
- *
- * It's possible to scan through every lexical token in the
- * HTML document using the `next_token()` function. This
- * alternative form takes no argument and provides no built-in
- * query syntax.
- *
- * Example:
- *
- * $title = '(untitled)';
- * $text = '';
- * while ( $processor->next_token() ) {
- * switch ( $processor->get_token_name() ) {
- * case '#text':
- * $text .= $processor->get_modifiable_text();
- * break;
- *
- * case 'BR':
- * $text .= "\n";
- * break;
- *
- * case 'TITLE':
- * $title = $processor->get_modifiable_text();
- * break;
- * }
- * }
- * return trim( "# {$title}\n\n{$text}" );
- *
- * ### Tokens and _modifiable text_.
- *
- * #### Special "atomic" HTML elements.
- *
- * Not all HTML elements are able to contain other elements inside of them.
- * For instance, the contents inside a TITLE element are plaintext (except
- * that character references like & will be decoded). This means that
- * if the string `
` appears inside a TITLE element, then it's not an
- * image tag, but rather it's text describing an image tag. Likewise, the
- * contents of a SCRIPT or STYLE element are handled entirely separately in
- * a browser than the contents of other elements because they represent a
- * different language than HTML.
- *
- * For these elements the Tag Processor treats the entire sequence as one,
- * from the opening tag, including its contents, through its closing tag.
- * This means that the it's not possible to match the closing tag for a
- * SCRIPT element unless it's unexpected; the Tag Processor already matched
- * it when it found the opening tag.
- *
- * The inner contents of these elements are that element's _modifiable text_.
- *
- * The special elements are:
- * - `SCRIPT` whose contents are treated as raw plaintext but supports a legacy
- * style of including JavaScript inside of HTML comments to avoid accidentally
- * closing the SCRIPT from inside a JavaScript string. E.g. `console.log( '' )`.
- * - `TITLE` and `TEXTAREA` whose contents are treated as plaintext and then any
- * character references are decoded. E.g. `1 < 2 < 3` becomes `1 < 2 < 3`.
- * - `IFRAME`, `NOSCRIPT`, `NOEMBED`, `NOFRAME`, `STYLE` whose contents are treated as
- * raw plaintext and left as-is. E.g. `1 < 2 < 3` remains `1 < 2 < 3`.
- *
- * #### Other tokens with modifiable text.
- *
- * There are also non-elements which are void/self-closing in nature and contain
- * modifiable text that is part of that individual syntax token itself.
- *
- * - `#text` nodes, whose entire token _is_ the modifiable text.
- * - HTML comments and tokens that become comments due to some syntax error. The
- * text for these tokens is the portion of the comment inside of the syntax.
- * E.g. for `` the text is `" comment "` (note the spaces are included).
- * - `CDATA` sections, whose text is the content inside of the section itself. E.g. for
- * `` the text is `"some content"` (with restrictions [1]).
- * - "Funky comments," which are a special case of invalid closing tags whose name is
- * invalid. The text for these nodes is the text that a browser would transform into
- * an HTML comment when parsing. E.g. for `%post_author>` the text is `%post_author`.
- * - `DOCTYPE` declarations like `
` which have no closing tag.
- * - XML Processing instruction nodes like `` (with restrictions [2]).
- * - The empty end tag `>` which is ignored in the browser and DOM.
- *
- * [1]: There are no CDATA sections in HTML. When encountering `` becomes a bogus HTML comment, meaning there can be no CDATA
- * section in an HTML document containing `>`. The Tag Processor will first find
- * all valid and bogus HTML comments, and then if the comment _would_ have been a
- * CDATA section _were they to exist_, it will indicate this as the type of comment.
- *
- * [2]: XML allows a broader range of characters in a processing instruction's target name
- * and disallows "xml" as a name, since it's special. The Tag Processor only recognizes
- * target names with an ASCII-representable subset of characters. It also exhibits the
- * same constraint as with CDATA sections, in that `>` cannot exist within the token
- * since Processing Instructions do no exist within HTML and their syntax transforms
- * into a bogus comment in the DOM.
- *
- * ## Design and limitations
- *
- * The Tag Processor is designed to linearly scan HTML documents and tokenize
- * HTML tags and their attributes. It's designed to do this as efficiently as
- * possible without compromising parsing integrity. Therefore it will be
- * slower than some methods of modifying HTML, such as those incorporating
- * over-simplified PCRE patterns, but will not introduce the defects and
- * failures that those methods bring in, which lead to broken page renders
- * and often to security vulnerabilities. On the other hand, it will be faster
- * than full-blown HTML parsers such as DOMDocument and use considerably
- * less memory. It requires a negligible memory overhead, enough to consider
- * it a zero-overhead system.
- *
- * The performance characteristics are maintained by avoiding tree construction
- * and semantic cleanups which are specified in HTML5. Because of this, for
- * example, it's not possible for the Tag Processor to associate any given
- * opening tag with its corresponding closing tag, or to return the inner markup
- * inside an element. Systems may be built on top of the Tag Processor to do
- * this, but the Tag Processor is and should be constrained so it can remain an
- * efficient, low-level, and reliable HTML scanner.
- *
- * The Tag Processor's design incorporates a "garbage-in-garbage-out" philosophy.
- * HTML5 specifies that certain invalid content be transformed into different forms
- * for display, such as removing null bytes from an input document and replacing
- * invalid characters with the Unicode replacement character `U+FFFD` (visually "�").
- * Where errors or transformations exist within the HTML5 specification, the Tag Processor
- * leaves those invalid inputs untouched, passing them through to the final browser
- * to handle. While this implies that certain operations will be non-spec-compliant,
- * such as reading the value of an attribute with invalid content, it also preserves a
- * simplicity and efficiency for handling those error cases.
- *
- * Most operations within the Tag Processor are designed to minimize the difference
- * between an input and output document for any given change. For example, the
- * `add_class` and `remove_class` methods preserve whitespace and the class ordering
- * within the `class` attribute; and when encountering tags with duplicated attributes,
- * the Tag Processor will leave those invalid duplicate attributes where they are but
- * update the proper attribute which the browser will read for parsing its value. An
- * exception to this rule is that all attribute updates store their values as
- * double-quoted strings, meaning that attributes on input with single-quoted or
- * unquoted values will appear in the output with double-quotes.
- *
- * ### Scripting Flag
- *
- * The Tag Processor parses HTML with the "scripting flag" disabled. This means
- * that it doesn't run any scripts while parsing the page. In a browser with
- * JavaScript enabled, for example, the script can change the parse of the
- * document as it loads. On the server, however, evaluating JavaScript is not
- * only impractical, but also unwanted.
- *
- * Practically this means that the Tag Processor will descend into NOSCRIPT
- * elements and process its child tags. Were the scripting flag enabled, such
- * as in a typical browser, the contents of NOSCRIPT are skipped entirely.
- *
- * This allows the HTML API to process the content that will be presented in
- * a browser when scripting is disabled, but it offers a different view of a
- * page than most browser sessions will experience. E.g. the tags inside the
- * NOSCRIPT disappear.
- *
- * ### Text Encoding
- *
- * The Tag Processor assumes that the input HTML document is encoded with a
- * text encoding compatible with 7-bit ASCII's '<', '>', '&', ';', '/', '=',
- * "'", '"', 'a' - 'z', 'A' - 'Z', and the whitespace characters ' ', tab,
- * carriage-return, newline, and form-feed.
- *
- * In practice, this includes almost every single-byte encoding as well as
- * UTF-8. Notably, however, it does not include UTF-16. If providing input
- * that's incompatible, then convert the encoding beforehand.
- *
- * @since 6.2.0
- * @since 6.2.1 Fix: Support for various invalid comments; attribute updates are case-insensitive.
- * @since 6.3.2 Fix: Skip HTML-like content inside rawtext elements such as STYLE.
- * @since 6.5.0 Pauses processor when input ends in an incomplete syntax token.
- * Introduces "special" elements which act like void elements, e.g. TITLE, STYLE.
- * Allows scanning through all tokens and processing modifiable text, where applicable.
- */
-class Gutenberg_HTML_Tag_Processor_6_6 {
- /**
- * The maximum number of bookmarks allowed to exist at
- * any given time.
- *
- * @since 6.2.0
- * @var int
- *
- * @see WP_HTML_Tag_Processor::set_bookmark()
- */
- const MAX_BOOKMARKS = 10;
-
- /**
- * Maximum number of times seek() can be called.
- * Prevents accidental infinite loops.
- *
- * @since 6.2.0
- * @var int
- *
- * @see WP_HTML_Tag_Processor::seek()
- */
- const MAX_SEEK_OPS = 1000;
-
- /**
- * The HTML document to parse.
- *
- * @since 6.2.0
- * @var string
- */
- protected $html;
-
- /**
- * The last query passed to next_tag().
- *
- * @since 6.2.0
- * @var array|null
- */
- private $last_query;
-
- /**
- * The tag name this processor currently scans for.
- *
- * @since 6.2.0
- * @var string|null
- */
- private $sought_tag_name;
-
- /**
- * The CSS class name this processor currently scans for.
- *
- * @since 6.2.0
- * @var string|null
- */
- private $sought_class_name;
-
- /**
- * The match offset this processor currently scans for.
- *
- * @since 6.2.0
- * @var int|null
- */
- private $sought_match_offset;
-
- /**
- * Whether to visit tag closers, e.g. , when walking an input document.
- *
- * @since 6.2.0
- * @var bool
- */
- private $stop_on_tag_closers;
-
- /**
- * Specifies mode of operation of the parser at any given time.
- *
- * | State | Meaning |
- * | ----------------|----------------------------------------------------------------------|
- * | *Ready* | The parser is ready to run. |
- * | *Complete* | There is nothing left to parse. |
- * | *Incomplete* | The HTML ended in the middle of a token; nothing more can be parsed. |
- * | *Matched tag* | Found an HTML tag; it's possible to modify its attributes. |
- * | *Text node* | Found a #text node; this is plaintext and modifiable. |
- * | *CDATA node* | Found a CDATA section; this is modifiable. |
- * | *Comment* | Found a comment or bogus comment; this is modifiable. |
- * | *Presumptuous* | Found an empty tag closer: `>`. |
- * | *Funky comment* | Found a tag closer with an invalid tag name; this is modifiable. |
- *
- * @since 6.5.0
- *
- * @see WP_HTML_Tag_Processor::STATE_READY
- * @see WP_HTML_Tag_Processor::STATE_COMPLETE
- * @see WP_HTML_Tag_Processor::STATE_INCOMPLETE_INPUT
- * @see WP_HTML_Tag_Processor::STATE_MATCHED_TAG
- * @see WP_HTML_Tag_Processor::STATE_TEXT_NODE
- * @see WP_HTML_Tag_Processor::STATE_CDATA_NODE
- * @see WP_HTML_Tag_Processor::STATE_COMMENT
- * @see WP_HTML_Tag_Processor::STATE_DOCTYPE
- * @see WP_HTML_Tag_Processor::STATE_PRESUMPTUOUS_TAG
- * @see WP_HTML_Tag_Processor::STATE_FUNKY_COMMENT
- *
- * @var string
- */
- protected $parser_state = self::STATE_READY;
-
- /**
- * What kind of syntax token became an HTML comment.
- *
- * Since there are many ways in which HTML syntax can create an HTML comment,
- * this indicates which of those caused it. This allows the Tag Processor to
- * represent more from the original input document than would appear in the DOM.
- *
- * @since 6.5.0
- *
- * @var string|null
- */
- protected $comment_type = null;
-
- /**
- * How many bytes from the original HTML document have been read and parsed.
- *
- * This value points to the latest byte offset in the input document which
- * has been already parsed. It is the internal cursor for the Tag Processor
- * and updates while scanning through the HTML tokens.
- *
- * @since 6.2.0
- * @var int
- */
- private $bytes_already_parsed = 0;
-
- /**
- * Byte offset in input document where current token starts.
- *
- * Example:
- *
- * ...
- * 01234
- * - token starts at 0
- *
- * @since 6.5.0
- *
- * @var int|null
- */
- private $token_starts_at;
-
- /**
- * Byte length of current token.
- *
- * Example:
- *
- *
...
- * 012345678901234
- * - token length is 14 - 0 = 14
- *
- * a is a token.
- * 0123456789 123456789 123456789
- * - token length is 17 - 2 = 15
- *
- * @since 6.5.0
- *
- * @var int|null
- */
- private $token_length;
-
- /**
- * Byte offset in input document where current tag name starts.
- *
- * Example:
- *
- *
...
- * 01234
- * - tag name starts at 1
- *
- * @since 6.2.0
- *
- * @var int|null
- */
- private $tag_name_starts_at;
-
- /**
- * Byte length of current tag name.
- *
- * Example:
- *
- *
...
- * 01234
- * --- tag name length is 3
- *
- * @since 6.2.0
- *
- * @var int|null
- */
- private $tag_name_length;
-
- /**
- * Byte offset into input document where current modifiable text starts.
- *
- * @since 6.5.0
- *
- * @var int
- */
- private $text_starts_at;
-
- /**
- * Byte length of modifiable text.
- *
- * @since 6.5.0
- *
- * @var string
- */
- private $text_length;
-
- /**
- * Whether the current tag is an opening tag, e.g.
, or a closing tag, e.g.
.
- *
- * @var bool
- */
- private $is_closing_tag;
-
- /**
- * Lazily-built index of attributes found within an HTML tag, keyed by the attribute name.
- *
- * Example:
- *
- * // Supposing the parser is working through this content
- * // and stops after recognizing the `id` attribute.
- * //
- * // ^ parsing will continue from this point.
- * $this->attributes = array(
- * 'id' => new WP_HTML_Attribute_Token( 'id', 9, 6, 5, 11, false )
- * );
- *
- * // When picking up parsing again, or when asking to find the
- * // `class` attribute we will continue and add to this array.
- * $this->attributes = array(
- * 'id' => new WP_HTML_Attribute_Token( 'id', 9, 6, 5, 11, false ),
- * 'class' => new WP_HTML_Attribute_Token( 'class', 23, 7, 17, 13, false )
- * );
- *
- * // Note that only the `class` attribute value is stored in the index.
- * // That's because it is the only value used by this class at the moment.
- *
- * @since 6.2.0
- * @var WP_HTML_Attribute_Token[]
- */
- private $attributes = array();
-
- /**
- * Tracks spans of duplicate attributes on a given tag, used for removing
- * all copies of an attribute when calling `remove_attribute()`.
- *
- * @since 6.3.2
- *
- * @var (WP_HTML_Span[])[]|null
- */
- private $duplicate_attributes = null;
-
- /**
- * Which class names to add or remove from a tag.
- *
- * These are tracked separately from attribute updates because they are
- * semantically distinct, whereas this interface exists for the common
- * case of adding and removing class names while other attributes are
- * generally modified as with DOM `setAttribute` calls.
- *
- * When modifying an HTML document these will eventually be collapsed
- * into a single `set_attribute( 'class', $changes )` call.
- *
- * Example:
- *
- * // Add the `wp-block-group` class, remove the `wp-group` class.
- * $classname_updates = array(
- * // Indexed by a comparable class name.
- * 'wp-block-group' => WP_HTML_Tag_Processor::ADD_CLASS,
- * 'wp-group' => WP_HTML_Tag_Processor::REMOVE_CLASS
- * );
- *
- * @since 6.2.0
- * @var bool[]
- */
- private $classname_updates = array();
-
- /**
- * Tracks a semantic location in the original HTML which
- * shifts with updates as they are applied to the document.
- *
- * @since 6.2.0
- * @var WP_HTML_Span[]
- */
- protected $bookmarks = array();
-
- const ADD_CLASS = true;
- const REMOVE_CLASS = false;
- const SKIP_CLASS = null;
-
- /**
- * Lexical replacements to apply to input HTML document.
- *
- * "Lexical" in this class refers to the part of this class which
- * operates on pure text _as text_ and not as HTML. There's a line
- * between the public interface, with HTML-semantic methods like
- * `set_attribute` and `add_class`, and an internal state that tracks
- * text offsets in the input document.
- *
- * When higher-level HTML methods are called, those have to transform their
- * operations (such as setting an attribute's value) into text diffing
- * operations (such as replacing the sub-string from indices A to B with
- * some given new string). These text-diffing operations are the lexical
- * updates.
- *
- * As new higher-level methods are added they need to collapse their
- * operations into these lower-level lexical updates since that's the
- * Tag Processor's internal language of change. Any code which creates
- * these lexical updates must ensure that they do not cross HTML syntax
- * boundaries, however, so these should never be exposed outside of this
- * class or any classes which intentionally expand its functionality.
- *
- * These are enqueued while editing the document instead of being immediately
- * applied to avoid processing overhead, string allocations, and string
- * copies when applying many updates to a single document.
- *
- * Example:
- *
- * // Replace an attribute stored with a new value, indices
- * // sourced from the lazily-parsed HTML recognizer.
- * $start = $attributes['src']->start;
- * $length = $attributes['src']->length;
- * $modifications[] = new WP_HTML_Text_Replacement( $start, $length, $new_value );
- *
- * // Correspondingly, something like this will appear in this array.
- * $lexical_updates = array(
- * WP_HTML_Text_Replacement( 14, 28, 'https://my-site.my-domain/wp-content/uploads/2014/08/kittens.jpg' )
- * );
- *
- * @since 6.2.0
- * @var WP_HTML_Text_Replacement[]
- */
- protected $lexical_updates = array();
-
- /**
- * Tracks and limits `seek()` calls to prevent accidental infinite loops.
- *
- * @since 6.2.0
- * @var int
- *
- * @see WP_HTML_Tag_Processor::seek()
- */
- protected $seek_count = 0;
-
- /**
- * Constructor.
- *
- * @since 6.2.0
- *
- * @param string $html HTML to process.
- */
- public function __construct( $html ) {
- $this->html = $html;
- }
-
- /**
- * Finds the next tag matching the $query.
- *
- * @since 6.2.0
- * @since 6.5.0 No longer processes incomplete tokens at end of document; pauses the processor at start of token.
- *
- * @param array|string|null $query {
- * Optional. Which tag name to find, having which class, etc. Default is to find any tag.
- *
- * @type string|null $tag_name Which tag to find, or `null` for "any tag."
- * @type int|null $match_offset Find the Nth tag matching all search criteria.
- * 1 for "first" tag, 3 for "third," etc.
- * Defaults to first tag.
- * @type string|null $class_name Tag must contain this whole class name to match.
- * @type string|null $tag_closers "visit" or "skip": whether to stop on tag closers, e.g.
.
- * }
- * @return bool Whether a tag was matched.
- */
- public function next_tag( $query = null ) {
- $this->parse_query( $query );
- $already_found = 0;
-
- do {
- if ( false === $this->next_token() ) {
- return false;
- }
-
- if ( self::STATE_MATCHED_TAG !== $this->parser_state ) {
- continue;
- }
-
- if ( $this->matches() ) {
- ++$already_found;
- }
- } while ( $already_found < $this->sought_match_offset );
-
- return true;
- }
-
- /**
- * Finds the next token in the HTML document.
- *
- * An HTML document can be viewed as a stream of tokens,
- * where tokens are things like HTML tags, HTML comments,
- * text nodes, etc. This method finds the next token in
- * the HTML document and returns whether it found one.
- *
- * If it starts parsing a token and reaches the end of the
- * document then it will seek to the start of the last
- * token and pause, returning `false` to indicate that it
- * failed to find a complete token.
- *
- * Possible token types, based on the HTML specification:
- *
- * - an HTML tag, whether opening, closing, or void.
- * - a text node - the plaintext inside tags.
- * - an HTML comment.
- * - a DOCTYPE declaration.
- * - a processing instruction, e.g. ``.
- *
- * The Tag Processor currently only supports the tag token.
- *
- * @since 6.5.0
- *
- * @return bool Whether a token was parsed.
- */
- public function next_token() {
- return $this->base_class_next_token();
- }
-
- /**
- * Internal method which finds the next token in the HTML document.
- *
- * This method is a protected internal function which implements the logic for
- * finding the next token in a document. It exists so that the parser can update
- * its state without affecting the location of the cursor in the document and
- * without triggering subclass methods for things like `next_token()`, e.g. when
- * applying patches before searching for the next token.
- *
- * @since 6.5.0
- *
- * @access private
- *
- * @return bool Whether a token was parsed.
- */
- private function base_class_next_token() {
- $was_at = $this->bytes_already_parsed;
- $this->after_tag();
-
- // Don't proceed if there's nothing more to scan.
- if (
- self::STATE_COMPLETE === $this->parser_state ||
- self::STATE_INCOMPLETE_INPUT === $this->parser_state
- ) {
- return false;
- }
-
- /*
- * The next step in the parsing loop determines the parsing state;
- * clear it so that state doesn't linger from the previous step.
- */
- $this->parser_state = self::STATE_READY;
-
- if ( $this->bytes_already_parsed >= strlen( $this->html ) ) {
- $this->parser_state = self::STATE_COMPLETE;
- return false;
- }
-
- // Find the next tag if it exists.
- if ( false === $this->parse_next_tag() ) {
- if ( self::STATE_INCOMPLETE_INPUT === $this->parser_state ) {
- $this->bytes_already_parsed = $was_at;
- }
-
- return false;
- }
-
- /*
- * For legacy reasons the rest of this function handles tags and their
- * attributes. If the processor has reached the end of the document
- * or if it matched any other token then it should return here to avoid
- * attempting to process tag-specific syntax.
- */
- if (
- self::STATE_INCOMPLETE_INPUT !== $this->parser_state &&
- self::STATE_COMPLETE !== $this->parser_state &&
- self::STATE_MATCHED_TAG !== $this->parser_state
- ) {
- return true;
- }
-
- // Parse all of its attributes.
- while ( $this->parse_next_attribute() ) {
- continue;
- }
-
- // Ensure that the tag closes before the end of the document.
- if (
- self::STATE_INCOMPLETE_INPUT === $this->parser_state ||
- $this->bytes_already_parsed >= strlen( $this->html )
- ) {
- // Does this appropriately clear state (parsed attributes)?
- $this->parser_state = self::STATE_INCOMPLETE_INPUT;
- $this->bytes_already_parsed = $was_at;
-
- return false;
- }
-
- $tag_ends_at = strpos( $this->html, '>', $this->bytes_already_parsed );
- if ( false === $tag_ends_at ) {
- $this->parser_state = self::STATE_INCOMPLETE_INPUT;
- $this->bytes_already_parsed = $was_at;
-
- return false;
- }
- $this->parser_state = self::STATE_MATCHED_TAG;
- $this->bytes_already_parsed = $tag_ends_at + 1;
- $this->token_length = $this->bytes_already_parsed - $this->token_starts_at;
-
- /*
- * For non-DATA sections which might contain text that looks like HTML tags but
- * isn't, scan with the appropriate alternative mode. Looking at the first letter
- * of the tag name as a pre-check avoids a string allocation when it's not needed.
- */
- $t = $this->html[ $this->tag_name_starts_at ];
- if (
- $this->is_closing_tag ||
- ! (
- 'i' === $t || 'I' === $t ||
- 'n' === $t || 'N' === $t ||
- 's' === $t || 'S' === $t ||
- 't' === $t || 'T' === $t ||
- 'x' === $t || 'X' === $t
- )
- ) {
- return true;
- }
-
- $tag_name = $this->get_tag();
-
- /*
- * Preserve the opening tag pointers, as these will be overwritten
- * when finding the closing tag. They will be reset after finding
- * the closing to tag to point to the opening of the special atomic
- * tag sequence.
- */
- $tag_name_starts_at = $this->tag_name_starts_at;
- $tag_name_length = $this->tag_name_length;
- $tag_ends_at = $this->token_starts_at + $this->token_length;
- $attributes = $this->attributes;
- $duplicate_attributes = $this->duplicate_attributes;
-
- // Find the closing tag if necessary.
- $found_closer = false;
- switch ( $tag_name ) {
- case 'SCRIPT':
- $found_closer = $this->skip_script_data();
- break;
-
- case 'TEXTAREA':
- case 'TITLE':
- $found_closer = $this->skip_rcdata( $tag_name );
- break;
-
- /*
- * In the browser this list would include the NOSCRIPT element,
- * but the Tag Processor is an environment with the scripting
- * flag disabled, meaning that it needs to descend into the
- * NOSCRIPT element to be able to properly process what will be
- * sent to a browser.
- *
- * Note that this rule makes HTML5 syntax incompatible with XML,
- * because the parsing of this token depends on client application.
- * The NOSCRIPT element cannot be represented in the XHTML syntax.
- */
- case 'IFRAME':
- case 'NOEMBED':
- case 'NOFRAMES':
- case 'STYLE':
- case 'XMP':
- $found_closer = $this->skip_rawtext( $tag_name );
- break;
-
- // No other tags should be treated in their entirety here.
- default:
- return true;
- }
-
- if ( ! $found_closer ) {
- $this->parser_state = self::STATE_INCOMPLETE_INPUT;
- $this->bytes_already_parsed = $was_at;
- return false;
- }
-
- /*
- * The values here look like they reference the opening tag but they reference
- * the closing tag instead. This is why the opening tag values were stored
- * above in a variable. It reads confusingly here, but that's because the
- * functions that skip the contents have moved all the internal cursors past
- * the inner content of the tag.
- */
- $this->token_starts_at = $was_at;
- $this->token_length = $this->bytes_already_parsed - $this->token_starts_at;
- $this->text_starts_at = $tag_ends_at;
- $this->text_length = $this->tag_name_starts_at - $this->text_starts_at;
- $this->tag_name_starts_at = $tag_name_starts_at;
- $this->tag_name_length = $tag_name_length;
- $this->attributes = $attributes;
- $this->duplicate_attributes = $duplicate_attributes;
-
- return true;
- }
-
- /**
- * Whether the processor paused because the input HTML document ended
- * in the middle of a syntax element, such as in the middle of a tag.
- *
- * Example:
- *
- * $processor = new WP_HTML_Tag_Processor( '
" );
- * $p->next_tag();
- * foreach ( $p->class_list() as $class_name ) {
- * echo "{$class_name} ";
- * }
- * // Outputs: "free
lang-en "
- *
- * @since 6.4.0
- */
- public function class_list() {
- if ( self::STATE_MATCHED_TAG !== $this->parser_state ) {
- return;
- }
-
- /** @var string $class contains the string value of the class attribute, with character references decoded. */
- $class = $this->get_attribute( 'class' );
-
- if ( ! is_string( $class ) ) {
- return;
- }
-
- $seen = array();
-
- $at = 0;
- while ( $at < strlen( $class ) ) {
- // Skip past any initial boundary characters.
- $at += strspn( $class, " \t\f\r\n", $at );
- if ( $at >= strlen( $class ) ) {
- return;
- }
-
- // Find the byte length until the next boundary.
- $length = strcspn( $class, " \t\f\r\n", $at );
- if ( 0 === $length ) {
- return;
- }
-
- /*
- * CSS class names are case-insensitive in the ASCII range.
- *
- * @see https://www.w3.org/TR/CSS2/syndata.html#x1
- */
- $name = strtolower( substr( $class, $at, $length ) );
- $at += $length;
-
- /*
- * It's expected that the number of class names for a given tag is relatively small.
- * Given this, it is probably faster overall to scan an array for a value rather
- * than to use the class name as a key and check if it's a key of $seen.
- */
- if ( in_array( $name, $seen, true ) ) {
- continue;
- }
-
- $seen[] = $name;
- yield $name;
- }
- }
-
-
- /**
- * Returns if a matched tag contains the given ASCII case-insensitive class name.
- *
- * @since 6.4.0
- *
- * @param string $wanted_class Look for this CSS class name, ASCII case-insensitive.
- * @return bool|null Whether the matched tag contains the given class name, or null if not matched.
- */
- public function has_class( $wanted_class ) {
- if ( self::STATE_MATCHED_TAG !== $this->parser_state ) {
- return null;
- }
-
- $wanted_class = strtolower( $wanted_class );
-
- foreach ( $this->class_list() as $class_name ) {
- if ( $class_name === $wanted_class ) {
- return true;
- }
- }
-
- return false;
- }
-
-
- /**
- * Sets a bookmark in the HTML document.
- *
- * Bookmarks represent specific places or tokens in the HTML
- * document, such as a tag opener or closer. When applying
- * edits to a document, such as setting an attribute, the
- * text offsets of that token may shift; the bookmark is
- * kept updated with those shifts and remains stable unless
- * the entire span of text in which the token sits is removed.
- *
- * Release bookmarks when they are no longer needed.
- *
- * Example:
- *
- * Surprising fact you may not know!
- * ^ ^
- * \-|-- this `H2` opener bookmark tracks the token
- *
- * Surprising fact you may no…
- * ^ ^
- * \-|-- it shifts with edits
- *
- * Bookmarks provide the ability to seek to a previously-scanned
- * place in the HTML document. This avoids the need to re-scan
- * the entire document.
- *
- * Example:
- *
- *
- * ^^^^
- * want to note this last item
- *
- * $p = new WP_HTML_Tag_Processor( $html );
- * $in_list = false;
- * while ( $p->next_tag( array( 'tag_closers' => $in_list ? 'visit' : 'skip' ) ) ) {
- * if ( 'UL' === $p->get_tag() ) {
- * if ( $p->is_tag_closer() ) {
- * $in_list = false;
- * $p->set_bookmark( 'resume' );
- * if ( $p->seek( 'last-li' ) ) {
- * $p->add_class( 'last-li' );
- * }
- * $p->seek( 'resume' );
- * $p->release_bookmark( 'last-li' );
- * $p->release_bookmark( 'resume' );
- * } else {
- * $in_list = true;
- * }
- * }
- *
- * if ( 'LI' === $p->get_tag() ) {
- * $p->set_bookmark( 'last-li' );
- * }
- * }
- *
- * Bookmarks intentionally hide the internal string offsets
- * to which they refer. They are maintained internally as
- * updates are applied to the HTML document and therefore
- * retain their "position" - the location to which they
- * originally pointed. The inability to use bookmarks with
- * functions like `substr` is therefore intentional to guard
- * against accidentally breaking the HTML.
- *
- * Because bookmarks allocate memory and require processing
- * for every applied update, they are limited and require
- * a name. They should not be created with programmatically-made
- * names, such as "li_{$index}" with some loop. As a general
- * rule they should only be created with string-literal names
- * like "start-of-section" or "last-paragraph".
- *
- * Bookmarks are a powerful tool to enable complicated behavior.
- * Consider double-checking that you need this tool if you are
- * reaching for it, as inappropriate use could lead to broken
- * HTML structure or unwanted processing overhead.
- *
- * @since 6.2.0
- *
- * @param string $name Identifies this particular bookmark.
- * @return bool Whether the bookmark was successfully created.
- */
- public function set_bookmark( $name ) {
- // It only makes sense to set a bookmark if the parser has paused on a concrete token.
- if (
- self::STATE_COMPLETE === $this->parser_state ||
- self::STATE_INCOMPLETE_INPUT === $this->parser_state
- ) {
- return false;
- }
-
- if ( ! array_key_exists( $name, $this->bookmarks ) && count( $this->bookmarks ) >= static::MAX_BOOKMARKS ) {
- _doing_it_wrong(
- __METHOD__,
- __( 'Too many bookmarks: cannot create any more.' ),
- '6.2.0'
- );
- return false;
- }
-
- $this->bookmarks[ $name ] = new WP_HTML_Span( $this->token_starts_at, $this->token_length );
-
- return true;
- }
-
-
- /**
- * Removes a bookmark that is no longer needed.
- *
- * Releasing a bookmark frees up the small
- * performance overhead it requires.
- *
- * @param string $name Name of the bookmark to remove.
- * @return bool Whether the bookmark already existed before removal.
- */
- public function release_bookmark( $name ) {
- if ( ! array_key_exists( $name, $this->bookmarks ) ) {
- return false;
- }
-
- unset( $this->bookmarks[ $name ] );
-
- return true;
- }
-
- /**
- * Skips contents of generic rawtext elements.
- *
- * @since 6.3.2
- *
- * @see https://html.spec.whatwg.org/#generic-raw-text-element-parsing-algorithm
- *
- * @param string $tag_name The uppercase tag name which will close the RAWTEXT region.
- * @return bool Whether an end to the RAWTEXT region was found before the end of the document.
- */
- private function skip_rawtext( $tag_name ) {
- /*
- * These two functions distinguish themselves on whether character references are
- * decoded, and since functionality to read the inner markup isn't supported, it's
- * not necessary to implement these two functions separately.
- */
- return $this->skip_rcdata( $tag_name );
- }
-
- /**
- * Skips contents of RCDATA elements, namely title and textarea tags.
- *
- * @since 6.2.0
- *
- * @see https://html.spec.whatwg.org/multipage/parsing.html#rcdata-state
- *
- * @param string $tag_name The uppercase tag name which will close the RCDATA region.
- * @return bool Whether an end to the RCDATA region was found before the end of the document.
- */
- private function skip_rcdata( $tag_name ) {
- $html = $this->html;
- $doc_length = strlen( $html );
- $tag_length = strlen( $tag_name );
-
- $at = $this->bytes_already_parsed;
-
- while ( false !== $at && $at < $doc_length ) {
- $at = strpos( $this->html, '', $at );
- $this->tag_name_starts_at = $at;
-
- // Fail if there is no possible tag closer.
- if ( false === $at || ( $at + $tag_length ) >= $doc_length ) {
- return false;
- }
-
- $at += 2;
-
- /*
- * Find a case-insensitive match to the tag name.
- *
- * Because tag names are limited to US-ASCII there is no
- * need to perform any kind of Unicode normalization when
- * comparing; any character which could be impacted by such
- * normalization could not be part of a tag name.
- */
- for ( $i = 0; $i < $tag_length; $i++ ) {
- $tag_char = $tag_name[ $i ];
- $html_char = $html[ $at + $i ];
-
- if ( $html_char !== $tag_char && strtoupper( $html_char ) !== $tag_char ) {
- $at += $i;
- continue 2;
- }
- }
-
- $at += $tag_length;
- $this->bytes_already_parsed = $at;
-
- if ( $at >= strlen( $html ) ) {
- return false;
- }
-
- /*
- * Ensure that the tag name terminates to avoid matching on
- * substrings of a longer tag name. For example, the sequence
- * "' !== $c ) {
- continue;
- }
-
- while ( $this->parse_next_attribute() ) {
- continue;
- }
-
- $at = $this->bytes_already_parsed;
- if ( $at >= strlen( $this->html ) ) {
- return false;
- }
-
- if ( '>' === $html[ $at ] ) {
- $this->bytes_already_parsed = $at + 1;
- return true;
- }
-
- if ( $at + 1 >= strlen( $this->html ) ) {
- return false;
- }
-
- if ( '/' === $html[ $at ] && '>' === $html[ $at + 1 ] ) {
- $this->bytes_already_parsed = $at + 2;
- return true;
- }
- }
-
- return false;
- }
-
- /**
- * Skips contents of script tags.
- *
- * @since 6.2.0
- *
- * @return bool Whether the script tag was closed before the end of the document.
- */
- private function skip_script_data() {
- $state = 'unescaped';
- $html = $this->html;
- $doc_length = strlen( $html );
- $at = $this->bytes_already_parsed;
-
- while ( false !== $at && $at < $doc_length ) {
- $at += strcspn( $html, '-<', $at );
-
- /*
- * For all script states a "-->" transitions
- * back into the normal unescaped script mode,
- * even if that's the current state.
- */
- if (
- $at + 2 < $doc_length &&
- '-' === $html[ $at ] &&
- '-' === $html[ $at + 1 ] &&
- '>' === $html[ $at + 2 ]
- ) {
- $at += 3;
- $state = 'unescaped';
- continue;
- }
-
- // Everything of interest past here starts with "<".
- if ( $at + 1 >= $doc_length || '<' !== $html[ $at++ ] ) {
- continue;
- }
-
- /*
- * Unlike with "-->", the "`. Unlike other comment
- * and bogus comment syntax, these leave no clear insertion point for text and
- * they need to be modified specially in order to contain text. E.g. to store
- * `?` as the modifiable text, the `` needs to become ``, which
- * involves inserting an additional `-` into the token after the modifiable text.
- */
- $this->parser_state = self::STATE_COMMENT;
- $this->comment_type = self::COMMENT_AS_ABRUPTLY_CLOSED_COMMENT;
- $this->token_length = $closer_at + $span_of_dashes + 1 - $this->token_starts_at;
-
- // Only provide modifiable text if the token is long enough to contain it.
- if ( $span_of_dashes >= 2 ) {
- $this->comment_type = self::COMMENT_AS_HTML_COMMENT;
- $this->text_starts_at = $this->token_starts_at + 4;
- $this->text_length = $span_of_dashes - 2;
- }
-
- $this->bytes_already_parsed = $closer_at + $span_of_dashes + 1;
- return true;
- }
-
- /*
- * Comments may be closed by either a --> or an invalid --!>.
- * The first occurrence closes the comment.
- *
- * See https://html.spec.whatwg.org/#parse-error-incorrectly-closed-comment
- */
- --$closer_at; // Pre-increment inside condition below reduces risk of accidental infinite looping.
- while ( ++$closer_at < $doc_length ) {
- $closer_at = strpos( $html, '--', $closer_at );
- if ( false === $closer_at ) {
- $this->parser_state = self::STATE_INCOMPLETE_INPUT;
-
- return false;
- }
-
- if ( $closer_at + 2 < $doc_length && '>' === $html[ $closer_at + 2 ] ) {
- $this->parser_state = self::STATE_COMMENT;
- $this->comment_type = self::COMMENT_AS_HTML_COMMENT;
- $this->token_length = $closer_at + 3 - $this->token_starts_at;
- $this->text_starts_at = $this->token_starts_at + 4;
- $this->text_length = $closer_at - $this->text_starts_at;
- $this->bytes_already_parsed = $closer_at + 3;
- return true;
- }
-
- if (
- $closer_at + 3 < $doc_length &&
- '!' === $html[ $closer_at + 2 ] &&
- '>' === $html[ $closer_at + 3 ]
- ) {
- $this->parser_state = self::STATE_COMMENT;
- $this->comment_type = self::COMMENT_AS_HTML_COMMENT;
- $this->token_length = $closer_at + 4 - $this->token_starts_at;
- $this->text_starts_at = $this->token_starts_at + 4;
- $this->text_length = $closer_at - $this->text_starts_at;
- $this->bytes_already_parsed = $closer_at + 4;
- return true;
- }
- }
- }
-
- /*
- * `
- * These are ASCII-case-insensitive.
- * https://html.spec.whatwg.org/multipage/parsing.html#tag-open-state
- */
- if (
- $doc_length > $at + 8 &&
- ( 'D' === $html[ $at + 2 ] || 'd' === $html[ $at + 2 ] ) &&
- ( 'O' === $html[ $at + 3 ] || 'o' === $html[ $at + 3 ] ) &&
- ( 'C' === $html[ $at + 4 ] || 'c' === $html[ $at + 4 ] ) &&
- ( 'T' === $html[ $at + 5 ] || 't' === $html[ $at + 5 ] ) &&
- ( 'Y' === $html[ $at + 6 ] || 'y' === $html[ $at + 6 ] ) &&
- ( 'P' === $html[ $at + 7 ] || 'p' === $html[ $at + 7 ] ) &&
- ( 'E' === $html[ $at + 8 ] || 'e' === $html[ $at + 8 ] )
- ) {
- $closer_at = strpos( $html, '>', $at + 9 );
- if ( false === $closer_at ) {
- $this->parser_state = self::STATE_INCOMPLETE_INPUT;
-
- return false;
- }
-
- $this->parser_state = self::STATE_DOCTYPE;
- $this->token_length = $closer_at + 1 - $this->token_starts_at;
- $this->text_starts_at = $this->token_starts_at + 9;
- $this->text_length = $closer_at - $this->text_starts_at;
- $this->bytes_already_parsed = $closer_at + 1;
- return true;
- }
-
- /*
- * Anything else here is an incorrectly-opened comment and transitions
- * to the bogus comment state - skip to the nearest >. If no closer is
- * found then the HTML was truncated inside the markup declaration.
- */
- $closer_at = strpos( $html, '>', $at + 1 );
- if ( false === $closer_at ) {
- $this->parser_state = self::STATE_INCOMPLETE_INPUT;
-
- return false;
- }
-
- $this->parser_state = self::STATE_COMMENT;
- $this->comment_type = self::COMMENT_AS_INVALID_HTML;
- $this->token_length = $closer_at + 1 - $this->token_starts_at;
- $this->text_starts_at = $this->token_starts_at + 2;
- $this->text_length = $closer_at - $this->text_starts_at;
- $this->bytes_already_parsed = $closer_at + 1;
-
- /*
- * Identify nodes that would be CDATA if HTML had CDATA sections.
- *
- * This section must occur after identifying the bogus comment end
- * because in an HTML parser it will span to the nearest `>`, even
- * if there's no `]]>` as would be required in an XML document. It
- * is therefore not possible to parse a CDATA section containing
- * a `>` in the HTML syntax.
- *
- * Inside foreign elements there is a discrepancy between browsers
- * and the specification on this.
- *
- * @todo Track whether the Tag Processor is inside a foreign element
- * and require the proper closing `]]>` in those cases.
- */
- if (
- $this->token_length >= 10 &&
- '[' === $html[ $this->token_starts_at + 2 ] &&
- 'C' === $html[ $this->token_starts_at + 3 ] &&
- 'D' === $html[ $this->token_starts_at + 4 ] &&
- 'A' === $html[ $this->token_starts_at + 5 ] &&
- 'T' === $html[ $this->token_starts_at + 6 ] &&
- 'A' === $html[ $this->token_starts_at + 7 ] &&
- '[' === $html[ $this->token_starts_at + 8 ] &&
- ']' === $html[ $closer_at - 1 ] &&
- ']' === $html[ $closer_at - 2 ]
- ) {
- $this->parser_state = self::STATE_COMMENT;
- $this->comment_type = self::COMMENT_AS_CDATA_LOOKALIKE;
- $this->text_starts_at += 7;
- $this->text_length -= 9;
- }
-
- return true;
- }
-
- /*
- * > is a missing end tag name, which is ignored.
- *
- * This was also known as the "presumptuous empty tag"
- * in early discussions as it was proposed to close
- * the nearest previous opening tag.
- *
- * See https://html.spec.whatwg.org/#parse-error-missing-end-tag-name
- */
- if ( '>' === $html[ $at + 1 ] ) {
- // `<>` is interpreted as plaintext.
- if ( ! $this->is_closing_tag ) {
- ++$at;
- continue;
- }
-
- $this->parser_state = self::STATE_PRESUMPTUOUS_TAG;
- $this->token_length = $at + 2 - $this->token_starts_at;
- $this->bytes_already_parsed = $at + 2;
- return true;
- }
-
- /*
- * `` transitions to a bogus comment state – skip to the nearest >
- * See https://html.spec.whatwg.org/multipage/parsing.html#tag-open-state
- */
- if ( ! $this->is_closing_tag && '?' === $html[ $at + 1 ] ) {
- $closer_at = strpos( $html, '>', $at + 2 );
- if ( false === $closer_at ) {
- $this->parser_state = self::STATE_INCOMPLETE_INPUT;
-
- return false;
- }
-
- $this->parser_state = self::STATE_COMMENT;
- $this->comment_type = self::COMMENT_AS_INVALID_HTML;
- $this->token_length = $closer_at + 1 - $this->token_starts_at;
- $this->text_starts_at = $this->token_starts_at + 2;
- $this->text_length = $closer_at - $this->text_starts_at;
- $this->bytes_already_parsed = $closer_at + 1;
-
- /*
- * Identify a Processing Instruction node were HTML to have them.
- *
- * This section must occur after identifying the bogus comment end
- * because in an HTML parser it will span to the nearest `>`, even
- * if there's no `?>` as would be required in an XML document. It
- * is therefore not possible to parse a Processing Instruction node
- * containing a `>` in the HTML syntax.
- *
- * XML allows for more target names, but this code only identifies
- * those with ASCII-representable target names. This means that it
- * may identify some Processing Instruction nodes as bogus comments,
- * but it will not misinterpret the HTML structure. By limiting the
- * identification to these target names the Tag Processor can avoid
- * the need to start parsing UTF-8 sequences.
- *
- * > NameStartChar ::= ":" | [A-Z] | "_" | [a-z] | [#xC0-#xD6] | [#xD8-#xF6] | [#xF8-#x2FF] |
- * [#x370-#x37D] | [#x37F-#x1FFF] | [#x200C-#x200D] | [#x2070-#x218F] |
- * [#x2C00-#x2FEF] | [#x3001-#xD7FF] | [#xF900-#xFDCF] | [#xFDF0-#xFFFD] |
- * [#x10000-#xEFFFF]
- * > NameChar ::= NameStartChar | "-" | "." | [0-9] | #xB7 | [#x0300-#x036F] | [#x203F-#x2040]
- *
- * @see https://www.w3.org/TR/2006/REC-xml11-20060816/#NT-PITarget
- */
- if ( $this->token_length >= 5 && '?' === $html[ $closer_at - 1 ] ) {
- $comment_text = substr( $html, $this->token_starts_at + 2, $this->token_length - 4 );
- $pi_target_length = strspn( $comment_text, 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ:_' );
-
- if ( 0 < $pi_target_length ) {
- $pi_target_length += strspn( $comment_text, 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789:_-.', $pi_target_length );
-
- $this->comment_type = self::COMMENT_AS_PI_NODE_LOOKALIKE;
- $this->tag_name_starts_at = $this->token_starts_at + 2;
- $this->tag_name_length = $pi_target_length;
- $this->text_starts_at += $pi_target_length;
- $this->text_length -= $pi_target_length + 1;
- }
- }
-
- return true;
- }
-
- /*
- * If a non-alpha starts the tag name in a tag closer it's a comment.
- * Find the first `>`, which closes the comment.
- *
- * This parser classifies these particular comments as special "funky comments"
- * which are made available for further processing.
- *
- * See https://html.spec.whatwg.org/#parse-error-invalid-first-character-of-tag-name
- */
- if ( $this->is_closing_tag ) {
- // No chance of finding a closer.
- if ( $at + 3 > $doc_length ) {
- return false;
- }
-
- $closer_at = strpos( $html, '>', $at + 2 );
- if ( false === $closer_at ) {
- $this->parser_state = self::STATE_INCOMPLETE_INPUT;
-
- return false;
- }
-
- $this->parser_state = self::STATE_FUNKY_COMMENT;
- $this->token_length = $closer_at + 1 - $this->token_starts_at;
- $this->text_starts_at = $this->token_starts_at + 2;
- $this->text_length = $closer_at - $this->text_starts_at;
- $this->bytes_already_parsed = $closer_at + 1;
- return true;
- }
-
- ++$at;
- }
-
- return false;
- }
-
- /**
- * Parses the next attribute.
- *
- * @since 6.2.0
- *
- * @return bool Whether an attribute was found before the end of the document.
- */
- private function parse_next_attribute() {
- // Skip whitespace and slashes.
- $this->bytes_already_parsed += strspn( $this->html, " \t\f\r\n/", $this->bytes_already_parsed );
- if ( $this->bytes_already_parsed >= strlen( $this->html ) ) {
- $this->parser_state = self::STATE_INCOMPLETE_INPUT;
-
- return false;
- }
-
- /*
- * Treat the equal sign as a part of the attribute
- * name if it is the first encountered byte.
- *
- * @see https://html.spec.whatwg.org/multipage/parsing.html#before-attribute-name-state
- */
- $name_length = '=' === $this->html[ $this->bytes_already_parsed ]
- ? 1 + strcspn( $this->html, "=/> \t\f\r\n", $this->bytes_already_parsed + 1 )
- : strcspn( $this->html, "=/> \t\f\r\n", $this->bytes_already_parsed );
-
- // No attribute, just tag closer.
- if ( 0 === $name_length || $this->bytes_already_parsed + $name_length >= strlen( $this->html ) ) {
- return false;
- }
-
- $attribute_start = $this->bytes_already_parsed;
- $attribute_name = substr( $this->html, $attribute_start, $name_length );
- $this->bytes_already_parsed += $name_length;
- if ( $this->bytes_already_parsed >= strlen( $this->html ) ) {
- $this->parser_state = self::STATE_INCOMPLETE_INPUT;
-
- return false;
- }
-
- $this->skip_whitespace();
- if ( $this->bytes_already_parsed >= strlen( $this->html ) ) {
- $this->parser_state = self::STATE_INCOMPLETE_INPUT;
-
- return false;
- }
-
- $has_value = '=' === $this->html[ $this->bytes_already_parsed ];
- if ( $has_value ) {
- ++$this->bytes_already_parsed;
- $this->skip_whitespace();
- if ( $this->bytes_already_parsed >= strlen( $this->html ) ) {
- $this->parser_state = self::STATE_INCOMPLETE_INPUT;
-
- return false;
- }
-
- switch ( $this->html[ $this->bytes_already_parsed ] ) {
- case "'":
- case '"':
- $quote = $this->html[ $this->bytes_already_parsed ];
- $value_start = $this->bytes_already_parsed + 1;
- $value_length = strcspn( $this->html, $quote, $value_start );
- $attribute_end = $value_start + $value_length + 1;
- $this->bytes_already_parsed = $attribute_end;
- break;
-
- default:
- $value_start = $this->bytes_already_parsed;
- $value_length = strcspn( $this->html, "> \t\f\r\n", $value_start );
- $attribute_end = $value_start + $value_length;
- $this->bytes_already_parsed = $attribute_end;
- }
- } else {
- $value_start = $this->bytes_already_parsed;
- $value_length = 0;
- $attribute_end = $attribute_start + $name_length;
- }
-
- if ( $attribute_end >= strlen( $this->html ) ) {
- $this->parser_state = self::STATE_INCOMPLETE_INPUT;
-
- return false;
- }
-
- if ( $this->is_closing_tag ) {
- return true;
- }
-
- /*
- * > There must never be two or more attributes on
- * > the same start tag whose names are an ASCII
- * > case-insensitive match for each other.
- * - HTML 5 spec
- *
- * @see https://html.spec.whatwg.org/multipage/syntax.html#attributes-2:ascii-case-insensitive
- */
- $comparable_name = strtolower( $attribute_name );
-
- // If an attribute is listed many times, only use the first declaration and ignore the rest.
- if ( ! array_key_exists( $comparable_name, $this->attributes ) ) {
- $this->attributes[ $comparable_name ] = new WP_HTML_Attribute_Token(
- $attribute_name,
- $value_start,
- $value_length,
- $attribute_start,
- $attribute_end - $attribute_start,
- ! $has_value
- );
-
- return true;
- }
-
- /*
- * Track the duplicate attributes so if we remove it, all disappear together.
- *
- * While `$this->duplicated_attributes` could always be stored as an `array()`,
- * which would simplify the logic here, storing a `null` and only allocating
- * an array when encountering duplicates avoids needless allocations in the
- * normative case of parsing tags with no duplicate attributes.
- */
- $duplicate_span = new WP_HTML_Span( $attribute_start, $attribute_end - $attribute_start );
- if ( null === $this->duplicate_attributes ) {
- $this->duplicate_attributes = array( $comparable_name => array( $duplicate_span ) );
- } elseif ( ! array_key_exists( $comparable_name, $this->duplicate_attributes ) ) {
- $this->duplicate_attributes[ $comparable_name ] = array( $duplicate_span );
- } else {
- $this->duplicate_attributes[ $comparable_name ][] = $duplicate_span;
- }
-
- return true;
- }
-
- /**
- * Move the internal cursor past any immediate successive whitespace.
- *
- * @since 6.2.0
- */
- private function skip_whitespace() {
- $this->bytes_already_parsed += strspn( $this->html, " \t\f\r\n", $this->bytes_already_parsed );
- }
-
- /**
- * Applies attribute updates and cleans up once a tag is fully parsed.
- *
- * @since 6.2.0
- */
- private function after_tag() {
- /*
- * There could be lexical updates enqueued for an attribute that
- * also exists on the next tag. In order to avoid conflating the
- * attributes across the two tags, lexical updates with names
- * need to be flushed to raw lexical updates.
- */
- $this->class_name_updates_to_attributes_updates();
-
- /*
- * Purge updates if there are too many. The actual count isn't
- * scientific, but a few values from 100 to a few thousand were
- * tests to find a practically-useful limit.
- *
- * If the update queue grows too big, then the Tag Processor
- * will spend more time iterating through them and lose the
- * efficiency gains of deferring applying them.
- */
- if ( 1000 < count( $this->lexical_updates ) ) {
- $this->get_updated_html();
- }
-
- foreach ( $this->lexical_updates as $name => $update ) {
- /*
- * Any updates appearing after the cursor should be applied
- * before proceeding, otherwise they may be overlooked.
- */
- if ( $update->start >= $this->bytes_already_parsed ) {
- $this->get_updated_html();
- break;
- }
-
- if ( is_int( $name ) ) {
- continue;
- }
-
- $this->lexical_updates[] = $update;
- unset( $this->lexical_updates[ $name ] );
- }
-
- $this->token_starts_at = null;
- $this->token_length = null;
- $this->tag_name_starts_at = null;
- $this->tag_name_length = null;
- $this->text_starts_at = 0;
- $this->text_length = 0;
- $this->is_closing_tag = null;
- $this->attributes = array();
- $this->comment_type = null;
- $this->duplicate_attributes = null;
- }
-
- /**
- * Converts class name updates into tag attributes updates
- * (they are accumulated in different data formats for performance).
- *
- * @since 6.2.0
- *
- * @see WP_HTML_Tag_Processor::$lexical_updates
- * @see WP_HTML_Tag_Processor::$classname_updates
- */
- private function class_name_updates_to_attributes_updates() {
- if ( count( $this->classname_updates ) === 0 ) {
- return;
- }
-
- $existing_class = $this->get_enqueued_attribute_value( 'class' );
- if ( null === $existing_class || true === $existing_class ) {
- $existing_class = '';
- }
-
- if ( false === $existing_class && isset( $this->attributes['class'] ) ) {
- $existing_class = substr(
- $this->html,
- $this->attributes['class']->value_starts_at,
- $this->attributes['class']->value_length
- );
- }
-
- if ( false === $existing_class ) {
- $existing_class = '';
- }
-
- /**
- * Updated "class" attribute value.
- *
- * This is incrementally built while scanning through the existing class
- * attribute, skipping removed classes on the way, and then appending
- * added classes at the end. Only when finished processing will the
- * value contain the final new value.
-
- * @var string $class
- */
- $class = '';
-
- /**
- * Tracks the cursor position in the existing
- * class attribute value while parsing.
- *
- * @var int $at
- */
- $at = 0;
-
- /**
- * Indicates if there's any need to modify the existing class attribute.
- *
- * If a call to `add_class()` and `remove_class()` wouldn't impact
- * the `class` attribute value then there's no need to rebuild it.
- * For example, when adding a class that's already present or
- * removing one that isn't.
- *
- * This flag enables a performance optimization when none of the enqueued
- * class updates would impact the `class` attribute; namely, that the
- * processor can continue without modifying the input document, as if
- * none of the `add_class()` or `remove_class()` calls had been made.
- *
- * This flag is set upon the first change that requires a string update.
- *
- * @var bool $modified
- */
- $modified = false;
-
- // Remove unwanted classes by only copying the new ones.
- $existing_class_length = strlen( $existing_class );
- while ( $at < $existing_class_length ) {
- // Skip to the first non-whitespace character.
- $ws_at = $at;
- $ws_length = strspn( $existing_class, " \t\f\r\n", $ws_at );
- $at += $ws_length;
-
- // Capture the class name – it's everything until the next whitespace.
- $name_length = strcspn( $existing_class, " \t\f\r\n", $at );
- if ( 0 === $name_length ) {
- // If no more class names are found then that's the end.
- break;
- }
-
- $name = substr( $existing_class, $at, $name_length );
- $at += $name_length;
-
- // If this class is marked for removal, start processing the next one.
- $remove_class = (
- isset( $this->classname_updates[ $name ] ) &&
- self::REMOVE_CLASS === $this->classname_updates[ $name ]
- );
-
- // If a class has already been seen then skip it; it should not be added twice.
- if ( ! $remove_class ) {
- $this->classname_updates[ $name ] = self::SKIP_CLASS;
- }
-
- if ( $remove_class ) {
- $modified = true;
- continue;
- }
-
- /*
- * Otherwise, append it to the new "class" attribute value.
- *
- * There are options for handling whitespace between tags.
- * Preserving the existing whitespace produces fewer changes
- * to the HTML content and should clarify the before/after
- * content when debugging the modified output.
- *
- * This approach contrasts normalizing the inter-class
- * whitespace to a single space, which might appear cleaner
- * in the output HTML but produce a noisier change.
- */
- $class .= substr( $existing_class, $ws_at, $ws_length );
- $class .= $name;
- }
-
- // Add new classes by appending those which haven't already been seen.
- foreach ( $this->classname_updates as $name => $operation ) {
- if ( self::ADD_CLASS === $operation ) {
- $modified = true;
-
- $class .= strlen( $class ) > 0 ? ' ' : '';
- $class .= $name;
- }
- }
-
- $this->classname_updates = array();
- if ( ! $modified ) {
- return;
- }
-
- if ( strlen( $class ) > 0 ) {
- $this->set_attribute( 'class', $class );
- } else {
- $this->remove_attribute( 'class' );
- }
- }
-
- /**
- * Applies attribute updates to HTML document.
- *
- * @since 6.2.0
- * @since 6.2.1 Accumulates shift for internal cursor and passed pointer.
- * @since 6.3.0 Invalidate any bookmarks whose targets are overwritten.
- *
- * @param int $shift_this_point Accumulate and return shift for this position.
- * @return int How many bytes the given pointer moved in response to the updates.
- */
- private function apply_attributes_updates( $shift_this_point ) {
- if ( ! count( $this->lexical_updates ) ) {
- return 0;
- }
-
- $accumulated_shift_for_given_point = 0;
-
- /*
- * Attribute updates can be enqueued in any order but updates
- * to the document must occur in lexical order; that is, each
- * replacement must be made before all others which follow it
- * at later string indices in the input document.
- *
- * Sorting avoid making out-of-order replacements which
- * can lead to mangled output, partially-duplicated
- * attributes, and overwritten attributes.
- */
- usort( $this->lexical_updates, array( self::class, 'sort_start_ascending' ) );
-
- $bytes_already_copied = 0;
- $output_buffer = '';
- foreach ( $this->lexical_updates as $diff ) {
- $shift = strlen( $diff->text ) - $diff->length;
-
- // Adjust the cursor position by however much an update affects it.
- if ( $diff->start < $this->bytes_already_parsed ) {
- $this->bytes_already_parsed += $shift;
- }
-
- // Accumulate shift of the given pointer within this function call.
- if ( $diff->start <= $shift_this_point ) {
- $accumulated_shift_for_given_point += $shift;
- }
-
- $output_buffer .= substr( $this->html, $bytes_already_copied, $diff->start - $bytes_already_copied );
- $output_buffer .= $diff->text;
- $bytes_already_copied = $diff->start + $diff->length;
- }
-
- $this->html = $output_buffer . substr( $this->html, $bytes_already_copied );
-
- /*
- * Adjust bookmark locations to account for how the text
- * replacements adjust offsets in the input document.
- */
- foreach ( $this->bookmarks as $bookmark_name => $bookmark ) {
- $bookmark_end = $bookmark->start + $bookmark->length;
-
- /*
- * Each lexical update which appears before the bookmark's endpoints
- * might shift the offsets for those endpoints. Loop through each change
- * and accumulate the total shift for each bookmark, then apply that
- * shift after tallying the full delta.
- */
- $head_delta = 0;
- $tail_delta = 0;
-
- foreach ( $this->lexical_updates as $diff ) {
- $diff_end = $diff->start + $diff->length;
-
- if ( $bookmark->start < $diff->start && $bookmark_end < $diff->start ) {
- break;
- }
-
- if ( $bookmark->start >= $diff->start && $bookmark_end < $diff_end ) {
- $this->release_bookmark( $bookmark_name );
- continue 2;
- }
-
- $delta = strlen( $diff->text ) - $diff->length;
-
- if ( $bookmark->start >= $diff->start ) {
- $head_delta += $delta;
- }
-
- if ( $bookmark_end >= $diff_end ) {
- $tail_delta += $delta;
- }
- }
-
- $bookmark->start += $head_delta;
- $bookmark->length += $tail_delta - $head_delta;
- }
-
- $this->lexical_updates = array();
-
- return $accumulated_shift_for_given_point;
- }
-
- /**
- * Checks whether a bookmark with the given name exists.
- *
- * @since 6.3.0
- *
- * @param string $bookmark_name Name to identify a bookmark that potentially exists.
- * @return bool Whether that bookmark exists.
- */
- public function has_bookmark( $bookmark_name ) {
- return array_key_exists( $bookmark_name, $this->bookmarks );
- }
-
- /**
- * Move the internal cursor in the Tag Processor to a given bookmark's location.
- *
- * In order to prevent accidental infinite loops, there's a
- * maximum limit on the number of times seek() can be called.
- *
- * @since 6.2.0
- *
- * @param string $bookmark_name Jump to the place in the document identified by this bookmark name.
- * @return bool Whether the internal cursor was successfully moved to the bookmark's location.
- */
- public function seek( $bookmark_name ) {
- if ( ! array_key_exists( $bookmark_name, $this->bookmarks ) ) {
- _doing_it_wrong(
- __METHOD__,
- __( 'Unknown bookmark name.' ),
- '6.2.0'
- );
- return false;
- }
-
- if ( ++$this->seek_count > static::MAX_SEEK_OPS ) {
- _doing_it_wrong(
- __METHOD__,
- __( 'Too many calls to seek() - this can lead to performance issues.' ),
- '6.2.0'
- );
- return false;
- }
-
- // Flush out any pending updates to the document.
- $this->get_updated_html();
-
- // Point this tag processor before the sought tag opener and consume it.
- $this->bytes_already_parsed = $this->bookmarks[ $bookmark_name ]->start;
- $this->parser_state = self::STATE_READY;
- return $this->next_token();
- }
-
- /**
- * Compare two WP_HTML_Text_Replacement objects.
- *
- * @since 6.2.0
- *
- * @param WP_HTML_Text_Replacement $a First attribute update.
- * @param WP_HTML_Text_Replacement $b Second attribute update.
- * @return int Comparison value for string order.
- */
- private static function sort_start_ascending( $a, $b ) {
- $by_start = $a->start - $b->start;
- if ( 0 !== $by_start ) {
- return $by_start;
- }
-
- $by_text = isset( $a->text, $b->text ) ? strcmp( $a->text, $b->text ) : 0;
- if ( 0 !== $by_text ) {
- return $by_text;
- }
-
- /*
- * This code should be unreachable, because it implies the two replacements
- * start at the same location and contain the same text.
- */
- return $a->length - $b->length;
- }
-
- /**
- * Return the enqueued value for a given attribute, if one exists.
- *
- * Enqueued updates can take different data types:
- * - If an update is enqueued and is boolean, the return will be `true`
- * - If an update is otherwise enqueued, the return will be the string value of that update.
- * - If an attribute is enqueued to be removed, the return will be `null` to indicate that.
- * - If no updates are enqueued, the return will be `false` to differentiate from "removed."
- *
- * @since 6.2.0
- *
- * @param string $comparable_name The attribute name in its comparable form.
- * @return string|boolean|null Value of enqueued update if present, otherwise false.
- */
- private function get_enqueued_attribute_value( $comparable_name ) {
- if ( self::STATE_MATCHED_TAG !== $this->parser_state ) {
- return false;
- }
-
- if ( ! isset( $this->lexical_updates[ $comparable_name ] ) ) {
- return false;
- }
-
- $enqueued_text = $this->lexical_updates[ $comparable_name ]->text;
-
- // Removed attributes erase the entire span.
- if ( '' === $enqueued_text ) {
- return null;
- }
-
- /*
- * Boolean attribute updates are just the attribute name without a corresponding value.
- *
- * This value might differ from the given comparable name in that there could be leading
- * or trailing whitespace, and that the casing follows the name given in `set_attribute`.
- *
- * Example:
- *
- * $p->set_attribute( 'data-TEST-id', 'update' );
- * 'update' === $p->get_enqueued_attribute_value( 'data-test-id' );
- *
- * Detect this difference based on the absence of the `=`, which _must_ exist in any
- * attribute containing a value, e.g. ` `.
- * ¹ ²
- * 1. Attribute with a string value.
- * 2. Boolean attribute whose value is `true`.
- */
- $equals_at = strpos( $enqueued_text, '=' );
- if ( false === $equals_at ) {
- return true;
- }
-
- /*
- * Finally, a normal update's value will appear after the `=` and
- * be double-quoted, as performed incidentally by `set_attribute`.
- *
- * e.g. `type="text"`
- * ¹² ³
- * 1. Equals is here.
- * 2. Double-quoting starts one after the equals sign.
- * 3. Double-quoting ends at the last character in the update.
- */
- $enqueued_value = substr( $enqueued_text, $equals_at + 2, -1 );
- return Gutenberg_HTML_Decoder_6_6::decode_attribute( $enqueued_value );
- }
-
- /**
- * Returns the value of a requested attribute from a matched tag opener if that attribute exists.
- *
- * Example:
- *
- * $p = new WP_HTML_Tag_Processor( ' Test
' );
- * $p->next_tag( array( 'class_name' => 'test' ) ) === true;
- * $p->get_attribute( 'data-test-id' ) === '14';
- * $p->get_attribute( 'enabled' ) === true;
- * $p->get_attribute( 'aria-label' ) === null;
- *
- * $p->next_tag() === false;
- * $p->get_attribute( 'class' ) === null;
- *
- * @since 6.2.0
- *
- * @param string $name Name of attribute whose value is requested.
- * @return string|true|null Value of attribute or `null` if not available. Boolean attributes return `true`.
- */
- public function get_attribute( $name ) {
- if ( self::STATE_MATCHED_TAG !== $this->parser_state ) {
- return null;
- }
-
- $comparable = strtolower( $name );
-
- /*
- * For every attribute other than `class` it's possible to perform a quick check if
- * there's an enqueued lexical update whose value takes priority over what's found in
- * the input document.
- *
- * The `class` attribute is special though because of the exposed helpers `add_class`
- * and `remove_class`. These form a builder for the `class` attribute, so an additional
- * check for enqueued class changes is required in addition to the check for any enqueued
- * attribute values. If any exist, those enqueued class changes must first be flushed out
- * into an attribute value update.
- */
- if ( 'class' === $name ) {
- $this->class_name_updates_to_attributes_updates();
- }
-
- // Return any enqueued attribute value updates if they exist.
- $enqueued_value = $this->get_enqueued_attribute_value( $comparable );
- if ( false !== $enqueued_value ) {
- return $enqueued_value;
- }
-
- if ( ! isset( $this->attributes[ $comparable ] ) ) {
- return null;
- }
-
- $attribute = $this->attributes[ $comparable ];
-
- /*
- * This flag distinguishes an attribute with no value
- * from an attribute with an empty string value. For
- * unquoted attributes this could look very similar.
- * It refers to whether an `=` follows the name.
- *
- * e.g.
- * ¹ ²
- * 1. Attribute `boolean-attribute` is `true`.
- * 2. Attribute `empty-attribute` is `""`.
- */
- if ( true === $attribute->is_true ) {
- return true;
- }
-
- $raw_value = substr( $this->html, $attribute->value_starts_at, $attribute->value_length );
-
- return Gutenberg_HTML_Decoder_6_6::decode_attribute( $raw_value );
- }
-
- /**
- * Gets lowercase names of all attributes matching a given prefix in the current tag.
- *
- * Note that matching is case-insensitive. This is in accordance with the spec:
- *
- * > There must never be two or more attributes on
- * > the same start tag whose names are an ASCII
- * > case-insensitive match for each other.
- * - HTML 5 spec
- *
- * Example:
- *
- * $p = new WP_HTML_Tag_Processor( 'Test
' );
- * $p->next_tag( array( 'class_name' => 'test' ) ) === true;
- * $p->get_attribute_names_with_prefix( 'data-' ) === array( 'data-enabled', 'data-test-id' );
- *
- * $p->next_tag() === false;
- * $p->get_attribute_names_with_prefix( 'data-' ) === null;
- *
- * @since 6.2.0
- *
- * @see https://html.spec.whatwg.org/multipage/syntax.html#attributes-2:ascii-case-insensitive
- *
- * @param string $prefix Prefix of requested attribute names.
- * @return array|null List of attribute names, or `null` when no tag opener is matched.
- */
- public function get_attribute_names_with_prefix( $prefix ) {
- if (
- self::STATE_MATCHED_TAG !== $this->parser_state ||
- $this->is_closing_tag
- ) {
- return null;
- }
-
- $comparable = strtolower( $prefix );
-
- $matches = array();
- foreach ( array_keys( $this->attributes ) as $attr_name ) {
- if ( str_starts_with( $attr_name, $comparable ) ) {
- $matches[] = $attr_name;
- }
- }
- return $matches;
- }
-
- /**
- * Returns the uppercase name of the matched tag.
- *
- * Example:
- *
- * $p = new WP_HTML_Tag_Processor( 'Test
' );
- * $p->next_tag() === true;
- * $p->get_tag() === 'DIV';
- *
- * $p->next_tag() === false;
- * $p->get_tag() === null;
- *
- * @since 6.2.0
- *
- * @return string|null Name of currently matched tag in input HTML, or `null` if none found.
- */
- public function get_tag() {
- if ( null === $this->tag_name_starts_at ) {
- return null;
- }
-
- $tag_name = substr( $this->html, $this->tag_name_starts_at, $this->tag_name_length );
-
- if ( self::STATE_MATCHED_TAG === $this->parser_state ) {
- return strtoupper( $tag_name );
- }
-
- if (
- self::STATE_COMMENT === $this->parser_state &&
- self::COMMENT_AS_PI_NODE_LOOKALIKE === $this->get_comment_type()
- ) {
- return $tag_name;
- }
-
- return null;
- }
-
- /**
- * Indicates if the currently matched tag contains the self-closing flag.
- *
- * No HTML elements ought to have the self-closing flag and for those, the self-closing
- * flag will be ignored. For void elements this is benign because they "self close"
- * automatically. For non-void HTML elements though problems will appear if someone
- * intends to use a self-closing element in place of that element with an empty body.
- * For HTML foreign elements and custom elements the self-closing flag determines if
- * they self-close or not.
- *
- * This function does not determine if a tag is self-closing,
- * but only if the self-closing flag is present in the syntax.
- *
- * @since 6.3.0
- *
- * @return bool Whether the currently matched tag contains the self-closing flag.
- */
- public function has_self_closing_flag() {
- if ( self::STATE_MATCHED_TAG !== $this->parser_state ) {
- return false;
- }
-
- /*
- * The self-closing flag is the solidus at the _end_ of the tag, not the beginning.
- *
- * Example:
- *
- *
- * ^ this appears one character before the end of the closing ">".
- */
- return '/' === $this->html[ $this->token_starts_at + $this->token_length - 2 ];
- }
-
- /**
- * Indicates if the current tag token is a tag closer.
- *
- * Example:
- *
- * $p = new WP_HTML_Tag_Processor( '
' );
- * $p->next_tag( array( 'tag_name' => 'div', 'tag_closers' => 'visit' ) );
- * $p->is_tag_closer() === false;
- *
- * $p->next_tag( array( 'tag_name' => 'div', 'tag_closers' => 'visit' ) );
- * $p->is_tag_closer() === true;
- *
- * @since 6.2.0
- *
- * @return bool Whether the current tag is a tag closer.
- */
- public function is_tag_closer() {
- return (
- self::STATE_MATCHED_TAG === $this->parser_state &&
- $this->is_closing_tag
- );
- }
-
- /**
- * Indicates the kind of matched token, if any.
- *
- * This differs from `get_token_name()` in that it always
- * returns a static string indicating the type, whereas
- * `get_token_name()` may return values derived from the
- * token itself, such as a tag name or processing
- * instruction tag.
- *
- * Possible values:
- * - `#tag` when matched on a tag.
- * - `#text` when matched on a text node.
- * - `#cdata-section` when matched on a CDATA node.
- * - `#comment` when matched on a comment.
- * - `#doctype` when matched on a DOCTYPE declaration.
- * - `#presumptuous-tag` when matched on an empty tag closer.
- * - `#funky-comment` when matched on a funky comment.
- *
- * @since 6.5.0
- *
- * @return string|null What kind of token is matched, or null.
- */
- public function get_token_type() {
- switch ( $this->parser_state ) {
- case self::STATE_MATCHED_TAG:
- return '#tag';
-
- case self::STATE_DOCTYPE:
- return '#doctype';
-
- default:
- return $this->get_token_name();
- }
- }
-
- /**
- * Returns the node name represented by the token.
- *
- * This matches the DOM API value `nodeName`. Some values
- * are static, such as `#text` for a text node, while others
- * are dynamically generated from the token itself.
- *
- * Dynamic names:
- * - Uppercase tag name for tag matches.
- * - `html` for DOCTYPE declarations.
- *
- * Note that if the Tag Processor is not matched on a token
- * then this function will return `null`, either because it
- * hasn't yet found a token or because it reached the end
- * of the document without matching a token.
- *
- * @since 6.5.0
- *
- * @return string|null Name of the matched token.
- */
- public function get_token_name() {
- switch ( $this->parser_state ) {
- case self::STATE_MATCHED_TAG:
- return $this->get_tag();
-
- case self::STATE_TEXT_NODE:
- return '#text';
-
- case self::STATE_CDATA_NODE:
- return '#cdata-section';
-
- case self::STATE_COMMENT:
- return '#comment';
-
- case self::STATE_DOCTYPE:
- return 'html';
-
- case self::STATE_PRESUMPTUOUS_TAG:
- return '#presumptuous-tag';
-
- case self::STATE_FUNKY_COMMENT:
- return '#funky-comment';
- }
-
- return null;
- }
-
- /**
- * Indicates what kind of comment produced the comment node.
- *
- * Because there are different kinds of HTML syntax which produce
- * comments, the Tag Processor tracks and exposes this as a type
- * for the comment. Nominally only regular HTML comments exist as
- * they are commonly known, but a number of unrelated syntax errors
- * also produce comments.
- *
- * @see self::COMMENT_AS_ABRUPTLY_CLOSED_COMMENT
- * @see self::COMMENT_AS_CDATA_LOOKALIKE
- * @see self::COMMENT_AS_INVALID_HTML
- * @see self::COMMENT_AS_HTML_COMMENT
- * @see self::COMMENT_AS_PI_NODE_LOOKALIKE
- *
- * @since 6.5.0
- *
- * @return string|null
- */
- public function get_comment_type() {
- if ( self::STATE_COMMENT !== $this->parser_state ) {
- return null;
- }
-
- return $this->comment_type;
- }
-
- /**
- * Returns the modifiable text for a matched token, or an empty string.
- *
- * Modifiable text is text content that may be read and changed without
- * changing the HTML structure of the document around it. This includes
- * the contents of `#text` nodes in the HTML as well as the inner
- * contents of HTML comments, Processing Instructions, and others, even
- * though these nodes aren't part of a parsed DOM tree. They also contain
- * the contents of SCRIPT and STYLE tags, of TEXTAREA tags, and of any
- * other section in an HTML document which cannot contain HTML markup (DATA).
- *
- * If a token has no modifiable text then an empty string is returned to
- * avoid needless crashing or type errors. An empty string does not mean
- * that a token has modifiable text, and a token with modifiable text may
- * have an empty string (e.g. a comment with no contents).
- *
- * @since 6.5.0
- *
- * @return string
- */
- public function get_modifiable_text() {
- if ( null === $this->text_starts_at ) {
- return '';
- }
-
- $text = substr( $this->html, $this->text_starts_at, $this->text_length );
-
- // Comment data is not decoded.
- if (
- self::STATE_CDATA_NODE === $this->parser_state ||
- self::STATE_COMMENT === $this->parser_state ||
- self::STATE_DOCTYPE === $this->parser_state ||
- self::STATE_FUNKY_COMMENT === $this->parser_state
- ) {
- return $text;
- }
-
- $tag_name = $this->get_tag();
- if (
- // Script data is not decoded.
- 'SCRIPT' === $tag_name ||
-
- // RAWTEXT data is not decoded.
- 'IFRAME' === $tag_name ||
- 'NOEMBED' === $tag_name ||
- 'NOFRAMES' === $tag_name ||
- 'STYLE' === $tag_name ||
- 'XMP' === $tag_name
- ) {
- return $text;
- }
-
- $decoded = Gutenberg_HTML_Decoder_6_6::decode_text_node( $text );
-
- /*
- * TEXTAREA skips a leading newline, but this newline may appear not only as the
- * literal character `\n`, but also as a character reference, such as in the
- * following markup: ``.
- *
- * For these cases it's important to first decode the text content before checking
- * for a leading newline and removing it.
- */
- if (
- self::STATE_MATCHED_TAG === $this->parser_state &&
- 'TEXTAREA' === $tag_name &&
- strlen( $decoded ) > 0 &&
- "\n" === $decoded[0]
- ) {
- return substr( $decoded, 1 );
- }
-
- return $decoded;
- }
-
- /**
- * Updates or creates a new attribute on the currently matched tag with the passed value.
- *
- * For boolean attributes special handling is provided:
- * - When `true` is passed as the value, then only the attribute name is added to the tag.
- * - When `false` is passed, the attribute gets removed if it existed before.
- *
- * For string attributes, the value is escaped using the `esc_attr` function.
- *
- * @since 6.2.0
- * @since 6.2.1 Fix: Only create a single update for multiple calls with case-variant attribute names.
- *
- * @param string $name The attribute name to target.
- * @param string|bool $value The new attribute value.
- * @return bool Whether an attribute value was set.
- */
- public function set_attribute( $name, $value ) {
- if (
- self::STATE_MATCHED_TAG !== $this->parser_state ||
- $this->is_closing_tag
- ) {
- return false;
- }
-
- /*
- * WordPress rejects more characters than are strictly forbidden
- * in HTML5. This is to prevent additional security risks deeper
- * in the WordPress and plugin stack. Specifically the
- * less-than (<) greater-than (>) and ampersand (&) aren't allowed.
- *
- * The use of a PCRE match enables looking for specific Unicode
- * code points without writing a UTF-8 decoder. Whereas scanning
- * for one-byte characters is trivial (with `strcspn`), scanning
- * for the longer byte sequences would be more complicated. Given
- * that this shouldn't be in the hot path for execution, it's a
- * reasonable compromise in efficiency without introducing a
- * noticeable impact on the overall system.
- *
- * @see https://html.spec.whatwg.org/#attributes-2
- *
- * @todo As the only regex pattern maybe we should take it out?
- * Are Unicode patterns available broadly in Core?
- */
- if ( preg_match(
- '~[' .
- // Syntax-like characters.
- '"\'>& =' .
- // Control characters.
- '\x{00}-\x{1F}' .
- // HTML noncharacters.
- '\x{FDD0}-\x{FDEF}' .
- '\x{FFFE}\x{FFFF}\x{1FFFE}\x{1FFFF}\x{2FFFE}\x{2FFFF}\x{3FFFE}\x{3FFFF}' .
- '\x{4FFFE}\x{4FFFF}\x{5FFFE}\x{5FFFF}\x{6FFFE}\x{6FFFF}\x{7FFFE}\x{7FFFF}' .
- '\x{8FFFE}\x{8FFFF}\x{9FFFE}\x{9FFFF}\x{AFFFE}\x{AFFFF}\x{BFFFE}\x{BFFFF}' .
- '\x{CFFFE}\x{CFFFF}\x{DFFFE}\x{DFFFF}\x{EFFFE}\x{EFFFF}\x{FFFFE}\x{FFFFF}' .
- '\x{10FFFE}\x{10FFFF}' .
- ']~Ssu',
- $name
- ) ) {
- _doing_it_wrong(
- __METHOD__,
- __( 'Invalid attribute name.' ),
- '6.2.0'
- );
-
- return false;
- }
-
- /*
- * > The values "true" and "false" are not allowed on boolean attributes.
- * > To represent a false value, the attribute has to be omitted altogether.
- * - HTML5 spec, https://html.spec.whatwg.org/#boolean-attributes
- */
- if ( false === $value ) {
- return $this->remove_attribute( $name );
- }
-
- if ( true === $value ) {
- $updated_attribute = $name;
- } else {
- $comparable_name = strtolower( $name );
-
- /*
- * Escape URL attributes.
- *
- * @see https://html.spec.whatwg.org/#attributes-3
- */
- $escaped_new_value = in_array( $comparable_name, wp_kses_uri_attributes() ) ? esc_url( $value ) : esc_attr( $value );
- $updated_attribute = "{$name}=\"{$escaped_new_value}\"";
- }
-
- /*
- * > There must never be two or more attributes on
- * > the same start tag whose names are an ASCII
- * > case-insensitive match for each other.
- * - HTML 5 spec
- *
- * @see https://html.spec.whatwg.org/multipage/syntax.html#attributes-2:ascii-case-insensitive
- */
- $comparable_name = strtolower( $name );
-
- if ( isset( $this->attributes[ $comparable_name ] ) ) {
- /*
- * Update an existing attribute.
- *
- * Example – set attribute id to "new" in
:
- *
- *
- * ^-------------^
- * start end
- * replacement: `id="new"`
- *
- * Result:
- */
- $existing_attribute = $this->attributes[ $comparable_name ];
- $this->lexical_updates[ $comparable_name ] = new WP_HTML_Text_Replacement(
- $existing_attribute->start,
- $existing_attribute->length,
- $updated_attribute
- );
- } else {
- /*
- * Create a new attribute at the tag's name end.
- *
- * Example – add attribute id="new" to
:
- *
- *
- * ^
- * start and end
- * replacement: ` id="new"`
- *
- * Result:
- */
- $this->lexical_updates[ $comparable_name ] = new WP_HTML_Text_Replacement(
- $this->tag_name_starts_at + $this->tag_name_length,
- 0,
- ' ' . $updated_attribute
- );
- }
-
- /*
- * Any calls to update the `class` attribute directly should wipe out any
- * enqueued class changes from `add_class` and `remove_class`.
- */
- if ( 'class' === $comparable_name && ! empty( $this->classname_updates ) ) {
- $this->classname_updates = array();
- }
-
- return true;
- }
-
- /**
- * Remove an attribute from the currently-matched tag.
- *
- * @since 6.2.0
- *
- * @param string $name The attribute name to remove.
- * @return bool Whether an attribute was removed.
- */
- public function remove_attribute( $name ) {
- if (
- self::STATE_MATCHED_TAG !== $this->parser_state ||
- $this->is_closing_tag
- ) {
- return false;
- }
-
- /*
- * > There must never be two or more attributes on
- * > the same start tag whose names are an ASCII
- * > case-insensitive match for each other.
- * - HTML 5 spec
- *
- * @see https://html.spec.whatwg.org/multipage/syntax.html#attributes-2:ascii-case-insensitive
- */
- $name = strtolower( $name );
-
- /*
- * Any calls to update the `class` attribute directly should wipe out any
- * enqueued class changes from `add_class` and `remove_class`.
- */
- if ( 'class' === $name && count( $this->classname_updates ) !== 0 ) {
- $this->classname_updates = array();
- }
-
- /*
- * If updating an attribute that didn't exist in the input
- * document, then remove the enqueued update and move on.
- *
- * For example, this might occur when calling `remove_attribute()`
- * after calling `set_attribute()` for the same attribute
- * and when that attribute wasn't originally present.
- */
- if ( ! isset( $this->attributes[ $name ] ) ) {
- if ( isset( $this->lexical_updates[ $name ] ) ) {
- unset( $this->lexical_updates[ $name ] );
- }
- return false;
- }
-
- /*
- * Removes an existing tag attribute.
- *
- * Example – remove the attribute id from
:
- *
- * ^-------------^
- * start end
- * replacement: ``
- *
- * Result:
- */
- $this->lexical_updates[ $name ] = new WP_HTML_Text_Replacement(
- $this->attributes[ $name ]->start,
- $this->attributes[ $name ]->length,
- ''
- );
-
- // Removes any duplicated attributes if they were also present.
- if ( null !== $this->duplicate_attributes && array_key_exists( $name, $this->duplicate_attributes ) ) {
- foreach ( $this->duplicate_attributes[ $name ] as $attribute_token ) {
- $this->lexical_updates[] = new WP_HTML_Text_Replacement(
- $attribute_token->start,
- $attribute_token->length,
- ''
- );
- }
- }
-
- return true;
- }
-
- /**
- * Adds a new class name to the currently matched tag.
- *
- * @since 6.2.0
- *
- * @param string $class_name The class name to add.
- * @return bool Whether the class was set to be added.
- */
- public function add_class( $class_name ) {
- if (
- self::STATE_MATCHED_TAG !== $this->parser_state ||
- $this->is_closing_tag
- ) {
- return false;
- }
-
- $this->classname_updates[ $class_name ] = self::ADD_CLASS;
-
- return true;
- }
-
- /**
- * Removes a class name from the currently matched tag.
- *
- * @since 6.2.0
- *
- * @param string $class_name The class name to remove.
- * @return bool Whether the class was set to be removed.
- */
- public function remove_class( $class_name ) {
- if (
- self::STATE_MATCHED_TAG !== $this->parser_state ||
- $this->is_closing_tag
- ) {
- return false;
- }
-
- if ( null !== $this->tag_name_starts_at ) {
- $this->classname_updates[ $class_name ] = self::REMOVE_CLASS;
- }
-
- return true;
- }
-
- /**
- * Returns the string representation of the HTML Tag Processor.
- *
- * @since 6.2.0
- *
- * @see WP_HTML_Tag_Processor::get_updated_html()
- *
- * @return string The processed HTML.
- */
- public function __toString() {
- return $this->get_updated_html();
- }
-
- /**
- * Returns the string representation of the HTML Tag Processor.
- *
- * @since 6.2.0
- * @since 6.2.1 Shifts the internal cursor corresponding to the applied updates.
- * @since 6.4.0 No longer calls subclass method `next_tag()` after updating HTML.
- *
- * @return string The processed HTML.
- */
- public function get_updated_html() {
- $requires_no_updating = 0 === count( $this->classname_updates ) && 0 === count( $this->lexical_updates );
-
- /*
- * When there is nothing more to update and nothing has already been
- * updated, return the original document and avoid a string copy.
- */
- if ( $requires_no_updating ) {
- return $this->html;
- }
-
- /*
- * Keep track of the position right before the current tag. This will
- * be necessary for reparsing the current tag after updating the HTML.
- */
- $before_current_tag = $this->token_starts_at ?? 0;
-
- /*
- * 1. Apply the enqueued edits and update all the pointers to reflect those changes.
- */
- $this->class_name_updates_to_attributes_updates();
- $before_current_tag += $this->apply_attributes_updates( $before_current_tag );
-
- /*
- * 2. Rewind to before the current tag and reparse to get updated attributes.
- *
- * At this point the internal cursor points to the end of the tag name.
- * Rewind before the tag name starts so that it's as if the cursor didn't
- * move; a call to `next_tag()` will reparse the recently-updated attributes
- * and additional calls to modify the attributes will apply at this same
- * location, but in order to avoid issues with subclasses that might add
- * behaviors to `next_tag()`, the internal methods should be called here
- * instead.
- *
- * It's important to note that in this specific place there will be no change
- * because the processor was already at a tag when this was called and it's
- * rewinding only to the beginning of this very tag before reprocessing it
- * and its attributes.
- *
- * Previous HTMLMore HTML
- * ↑ │ back up by the length of the tag name plus the opening <
- * └←─┘ back up by strlen("em") + 1 ==> 3
- */
- $this->bytes_already_parsed = $before_current_tag;
- $this->base_class_next_token();
-
- return $this->html;
- }
-
- /**
- * Parses tag query input into internal search criteria.
- *
- * @since 6.2.0
- *
- * @param array|string|null $query {
- * Optional. Which tag name to find, having which class, etc. Default is to find any tag.
- *
- * @type string|null $tag_name Which tag to find, or `null` for "any tag."
- * @type int|null $match_offset Find the Nth tag matching all search criteria.
- * 1 for "first" tag, 3 for "third," etc.
- * Defaults to first tag.
- * @type string|null $class_name Tag must contain this class name to match.
- * @type string $tag_closers "visit" or "skip": whether to stop on tag closers, e.g. .
- * }
- */
- private function parse_query( $query ) {
- if ( null !== $query && $query === $this->last_query ) {
- return;
- }
-
- $this->last_query = $query;
- $this->sought_tag_name = null;
- $this->sought_class_name = null;
- $this->sought_match_offset = 1;
- $this->stop_on_tag_closers = false;
-
- // A single string value means "find the tag of this name".
- if ( is_string( $query ) ) {
- $this->sought_tag_name = $query;
- return;
- }
-
- // An empty query parameter applies no restrictions on the search.
- if ( null === $query ) {
- return;
- }
-
- // If not using the string interface, an associative array is required.
- if ( ! is_array( $query ) ) {
- _doing_it_wrong(
- __METHOD__,
- __( 'The query argument must be an array or a tag name.' ),
- '6.2.0'
- );
- return;
- }
-
- if ( isset( $query['tag_name'] ) && is_string( $query['tag_name'] ) ) {
- $this->sought_tag_name = $query['tag_name'];
- }
-
- if ( isset( $query['class_name'] ) && is_string( $query['class_name'] ) ) {
- $this->sought_class_name = $query['class_name'];
- }
-
- if ( isset( $query['match_offset'] ) && is_int( $query['match_offset'] ) && 0 < $query['match_offset'] ) {
- $this->sought_match_offset = $query['match_offset'];
- }
-
- if ( isset( $query['tag_closers'] ) ) {
- $this->stop_on_tag_closers = 'visit' === $query['tag_closers'];
- }
- }
-
-
- /**
- * Checks whether a given tag and its attributes match the search criteria.
- *
- * @since 6.2.0
- *
- * @return bool Whether the given tag and its attribute match the search criteria.
- */
- private function matches() {
- if ( $this->is_closing_tag && ! $this->stop_on_tag_closers ) {
- return false;
- }
-
- // Does the tag name match the requested tag name in a case-insensitive manner?
- if ( null !== $this->sought_tag_name ) {
- /*
- * String (byte) length lookup is fast. If they aren't the
- * same length then they can't be the same string values.
- */
- if ( strlen( $this->sought_tag_name ) !== $this->tag_name_length ) {
- return false;
- }
-
- /*
- * Check each character to determine if they are the same.
- * Defer calls to `strtoupper()` to avoid them when possible.
- * Calling `strcasecmp()` here tested slowed than comparing each
- * character, so unless benchmarks show otherwise, it should
- * not be used.
- *
- * It's expected that most of the time that this runs, a
- * lower-case tag name will be supplied and the input will
- * contain lower-case tag names, thus normally bypassing
- * the case comparison code.
- */
- for ( $i = 0; $i < $this->tag_name_length; $i++ ) {
- $html_char = $this->html[ $this->tag_name_starts_at + $i ];
- $tag_char = $this->sought_tag_name[ $i ];
-
- if ( $html_char !== $tag_char && strtoupper( $html_char ) !== $tag_char ) {
- return false;
- }
- }
- }
-
- if ( null !== $this->sought_class_name && ! $this->has_class( $this->sought_class_name ) ) {
- return false;
- }
-
- return true;
- }
-
- /**
- * Parser Ready State.
- *
- * Indicates that the parser is ready to run and waiting for a state transition.
- * It may not have started yet, or it may have just finished parsing a token and
- * is ready to find the next one.
- *
- * @since 6.5.0
- *
- * @access private
- */
- const STATE_READY = 'STATE_READY';
-
- /**
- * Parser Complete State.
- *
- * Indicates that the parser has reached the end of the document and there is
- * nothing left to scan. It finished parsing the last token completely.
- *
- * @since 6.5.0
- *
- * @access private
- */
- const STATE_COMPLETE = 'STATE_COMPLETE';
-
- /**
- * Parser Incomplete Input State.
- *
- * Indicates that the parser has reached the end of the document before finishing
- * a token. It started parsing a token but there is a possibility that the input
- * HTML document was truncated in the middle of a token.
- *
- * The parser is reset at the start of the incomplete token and has paused. There
- * is nothing more than can be scanned unless provided a more complete document.
- *
- * @since 6.5.0
- *
- * @access private
- */
- const STATE_INCOMPLETE_INPUT = 'STATE_INCOMPLETE_INPUT';
-
- /**
- * Parser Matched Tag State.
- *
- * Indicates that the parser has found an HTML tag and it's possible to get
- * the tag name and read or modify its attributes (if it's not a closing tag).
- *
- * @since 6.5.0
- *
- * @access private
- */
- const STATE_MATCHED_TAG = 'STATE_MATCHED_TAG';
-
- /**
- * Parser Text Node State.
- *
- * Indicates that the parser has found a text node and it's possible
- * to read and modify that text.
- *
- * @since 6.5.0
- *
- * @access private
- */
- const STATE_TEXT_NODE = 'STATE_TEXT_NODE';
-
- /**
- * Parser CDATA Node State.
- *
- * Indicates that the parser has found a CDATA node and it's possible
- * to read and modify its modifiable text. Note that in HTML there are
- * no CDATA nodes outside of foreign content (SVG and MathML). Outside
- * of foreign content, they are treated as HTML comments.
- *
- * @since 6.5.0
- *
- * @access private
- */
- const STATE_CDATA_NODE = 'STATE_CDATA_NODE';
-
- /**
- * Indicates that the parser has found an HTML comment and it's
- * possible to read and modify its modifiable text.
- *
- * @since 6.5.0
- *
- * @access private
- */
- const STATE_COMMENT = 'STATE_COMMENT';
-
- /**
- * Indicates that the parser has found a DOCTYPE node and it's
- * possible to read and modify its modifiable text.
- *
- * @since 6.5.0
- *
- * @access private
- */
- const STATE_DOCTYPE = 'STATE_DOCTYPE';
-
- /**
- * Indicates that the parser has found an empty tag closer `>`.
- *
- * Note that in HTML there are no empty tag closers, and they
- * are ignored. Nonetheless, the Tag Processor still
- * recognizes them as they appear in the HTML stream.
- *
- * These were historically discussed as a "presumptuous tag
- * closer," which would close the nearest open tag, but were
- * dismissed in favor of explicitly-closing tags.
- *
- * @since 6.5.0
- *
- * @access private
- */
- const STATE_PRESUMPTUOUS_TAG = 'STATE_PRESUMPTUOUS_TAG';
-
- /**
- * Indicates that the parser has found a "funky comment"
- * and it's possible to read and modify its modifiable text.
- *
- * Example:
- *
- * %url>
- * {"wp-bit":"query/post-author"}>
- * 2>
- *
- * Funky comments are tag closers with invalid tag names. Note
- * that in HTML these are turn into bogus comments. Nonetheless,
- * the Tag Processor recognizes them in a stream of HTML and
- * exposes them for inspection and modification.
- *
- * @since 6.5.0
- *
- * @access private
- */
- const STATE_FUNKY_COMMENT = 'STATE_WP_FUNKY';
-
- /**
- * Indicates that a comment was created when encountering abruptly-closed HTML comment.
- *
- * Example:
- *
- *
- *
- *
- * @since 6.5.0
- */
- const COMMENT_AS_ABRUPTLY_CLOSED_COMMENT = 'COMMENT_AS_ABRUPTLY_CLOSED_COMMENT';
-
- /**
- * Indicates that a comment would be parsed as a CDATA node,
- * were HTML to allow CDATA nodes outside of foreign content.
- *
- * Example:
- *
- *
- *
- * This is an HTML comment, but it looks like a CDATA node.
- *
- * @since 6.5.0
- */
- const COMMENT_AS_CDATA_LOOKALIKE = 'COMMENT_AS_CDATA_LOOKALIKE';
-
- /**
- * Indicates that a comment was created when encountering
- * normative HTML comment syntax.
- *
- * Example:
- *
- *
- *
- * @since 6.5.0
- */
- const COMMENT_AS_HTML_COMMENT = 'COMMENT_AS_HTML_COMMENT';
-
- /**
- * Indicates that a comment would be parsed as a Processing
- * Instruction node, were they to exist within HTML.
- *
- * Example:
- *
- *
- *
- * This is an HTML comment, but it looks like a CDATA node.
- *
- * @since 6.5.0
- */
- const COMMENT_AS_PI_NODE_LOOKALIKE = 'COMMENT_AS_PI_NODE_LOOKALIKE';
-
- /**
- * Indicates that a comment was created when encountering invalid
- * HTML input, a so-called "bogus comment."
- *
- * Example:
- *
- *
- *
- *
- * @since 6.5.0
- */
- const COMMENT_AS_INVALID_HTML = 'COMMENT_AS_INVALID_HTML';
-}
diff --git a/lib/compat/wordpress-6.6/html-api/gutenberg-html5-named-character-references-6-6.php b/lib/compat/wordpress-6.6/html-api/gutenberg-html5-named-character-references-6-6.php
deleted file mode 100644
index c09deb3c9e664..0000000000000
--- a/lib/compat/wordpress-6.6/html-api/gutenberg-html5-named-character-references-6-6.php
+++ /dev/null
@@ -1,1315 +0,0 @@
- "6.6.0-trunk",
- "key_length" => 2,
- "groups" => "AE\x00AM\x00Aa\x00Ab\x00Ac\x00Af\x00Ag\x00Al\x00Am\x00An\x00Ao\x00Ap\x00Ar\x00As\x00At\x00Au\x00Ba\x00Bc\x00Be\x00Bf\x00Bo\x00Br\x00Bs\x00Bu\x00CH\x00CO\x00Ca\x00Cc\x00Cd\x00Ce\x00Cf\x00Ch\x00Ci\x00Cl\x00Co\x00Cr\x00Cs\x00Cu\x00DD\x00DJ\x00DS\x00DZ\x00Da\x00Dc\x00De\x00Df\x00Di\x00Do\x00Ds\x00EN\x00ET\x00Ea\x00Ec\x00Ed\x00Ef\x00Eg\x00El\x00Em\x00Eo\x00Ep\x00Eq\x00Es\x00Et\x00Eu\x00Ex\x00Fc\x00Ff\x00Fi\x00Fo\x00Fs\x00GJ\x00GT\x00Ga\x00Gb\x00Gc\x00Gd\x00Gf\x00Gg\x00Go\x00Gr\x00Gs\x00Gt\x00HA\x00Ha\x00Hc\x00Hf\x00Hi\x00Ho\x00Hs\x00Hu\x00IE\x00IJ\x00IO\x00Ia\x00Ic\x00Id\x00If\x00Ig\x00Im\x00In\x00Io\x00Is\x00It\x00Iu\x00Jc\x00Jf\x00Jo\x00Js\x00Ju\x00KH\x00KJ\x00Ka\x00Kc\x00Kf\x00Ko\x00Ks\x00LJ\x00LT\x00La\x00Lc\x00Le\x00Lf\x00Ll\x00Lm\x00Lo\x00Ls\x00Lt\x00Ma\x00Mc\x00Me\x00Mf\x00Mi\x00Mo\x00Ms\x00Mu\x00NJ\x00Na\x00Nc\x00Ne\x00Nf\x00No\x00Ns\x00Nt\x00Nu\x00OE\x00Oa\x00Oc\x00Od\x00Of\x00Og\x00Om\x00Oo\x00Op\x00Or\x00Os\x00Ot\x00Ou\x00Ov\x00Pa\x00Pc\x00Pf\x00Ph\x00Pi\x00Pl\x00Po\x00Pr\x00Ps\x00QU\x00Qf\x00Qo\x00Qs\x00RB\x00RE\x00Ra\x00Rc\x00Re\x00Rf\x00Rh\x00Ri\x00Ro\x00Rr\x00Rs\x00Ru\x00SH\x00SO\x00Sa\x00Sc\x00Sf\x00Sh\x00Si\x00Sm\x00So\x00Sq\x00Ss\x00St\x00Su\x00TH\x00TR\x00TS\x00Ta\x00Tc\x00Tf\x00Th\x00Ti\x00To\x00Tr\x00Ts\x00Ua\x00Ub\x00Uc\x00Ud\x00Uf\x00Ug\x00Um\x00Un\x00Uo\x00Up\x00Ur\x00Us\x00Ut\x00Uu\x00VD\x00Vb\x00Vc\x00Vd\x00Ve\x00Vf\x00Vo\x00Vs\x00Vv\x00Wc\x00We\x00Wf\x00Wo\x00Ws\x00Xf\x00Xi\x00Xo\x00Xs\x00YA\x00YI\x00YU\x00Ya\x00Yc\x00Yf\x00Yo\x00Ys\x00Yu\x00ZH\x00Za\x00Zc\x00Zd\x00Ze\x00Zf\x00Zo\x00Zs\x00aa\x00ab\x00ac\x00ae\x00af\x00ag\x00al\x00am\x00an\x00ao\x00ap\x00ar\x00as\x00at\x00au\x00aw\x00bN\x00ba\x00bb\x00bc\x00bd\x00be\x00bf\x00bi\x00bk\x00bl\x00bn\x00bo\x00bp\x00br\x00bs\x00bu\x00ca\x00cc\x00cd\x00ce\x00cf\x00ch\x00ci\x00cl\x00co\x00cr\x00cs\x00ct\x00cu\x00cw\x00cy\x00dA\x00dH\x00da\x00db\x00dc\x00dd\x00de\x00df\x00dh\x00di\x00dj\x00dl\x00do\x00dr\x00ds\x00dt\x00du\x00dw\x00dz\x00eD\x00ea\x00ec\x00ed\x00ee\x00ef\x00eg\x00el\x00em\x00en\x00eo\x00ep\x00eq\x00er\x00es\x00et\x00eu\x00ex\x00fa\x00fc\x00fe\x00ff\x00fi\x00fj\x00fl\x00fn\x00fo\x00fp\x00fr\x00fs\x00gE\x00ga\x00gb\x00gc\x00gd\x00ge\x00gf\x00gg\x00gi\x00gj\x00gl\x00gn\x00go\x00gr\x00gs\x00gt\x00gv\x00hA\x00ha\x00hb\x00hc\x00he\x00hf\x00hk\x00ho\x00hs\x00hy\x00ia\x00ic\x00ie\x00if\x00ig\x00ii\x00ij\x00im\x00in\x00io\x00ip\x00iq\x00is\x00it\x00iu\x00jc\x00jf\x00jm\x00jo\x00js\x00ju\x00ka\x00kc\x00kf\x00kg\x00kh\x00kj\x00ko\x00ks\x00lA\x00lB\x00lE\x00lH\x00la\x00lb\x00lc\x00ld\x00le\x00lf\x00lg\x00lh\x00lj\x00ll\x00lm\x00ln\x00lo\x00lp\x00lr\x00ls\x00lt\x00lu\x00lv\x00mD\x00ma\x00mc\x00md\x00me\x00mf\x00mh\x00mi\x00ml\x00mn\x00mo\x00mp\x00ms\x00mu\x00nG\x00nL\x00nR\x00nV\x00na\x00nb\x00nc\x00nd\x00ne\x00nf\x00ng\x00nh\x00ni\x00nj\x00nl\x00nm\x00no\x00np\x00nr\x00ns\x00nt\x00nu\x00nv\x00nw\x00oS\x00oa\x00oc\x00od\x00oe\x00of\x00og\x00oh\x00oi\x00ol\x00om\x00oo\x00op\x00or\x00os\x00ot\x00ou\x00ov\x00pa\x00pc\x00pe\x00pf\x00ph\x00pi\x00pl\x00pm\x00po\x00pr\x00ps\x00pu\x00qf\x00qi\x00qo\x00qp\x00qs\x00qu\x00rA\x00rB\x00rH\x00ra\x00rb\x00rc\x00rd\x00re\x00rf\x00rh\x00ri\x00rl\x00rm\x00rn\x00ro\x00rp\x00rr\x00rs\x00rt\x00ru\x00rx\x00sa\x00sb\x00sc\x00sd\x00se\x00sf\x00sh\x00si\x00sl\x00sm\x00so\x00sp\x00sq\x00sr\x00ss\x00st\x00su\x00sw\x00sz\x00ta\x00tb\x00tc\x00td\x00te\x00tf\x00th\x00ti\x00to\x00tp\x00tr\x00ts\x00tw\x00uA\x00uH\x00ua\x00ub\x00uc\x00ud\x00uf\x00ug\x00uh\x00ul\x00um\x00uo\x00up\x00ur\x00us\x00ut\x00uu\x00uw\x00vA\x00vB\x00vD\x00va\x00vc\x00vd\x00ve\x00vf\x00vl\x00vn\x00vo\x00vp\x00vr\x00vs\x00vz\x00wc\x00we\x00wf\x00wo\x00wp\x00wr\x00ws\x00xc\x00xd\x00xf\x00xh\x00xi\x00xl\x00xm\x00xn\x00xo\x00xr\x00xs\x00xu\x00xv\x00xw\x00ya\x00yc\x00ye\x00yf\x00yi\x00yo\x00ys\x00yu\x00za\x00zc\x00zd\x00ze\x00zf\x00zh\x00zi\x00zo\x00zs\x00zw\x00",
- "large_words" => array(
- // AElig;[Æ] AElig[Æ].
- "\x04lig;\x02Æ\x03lig\x02Æ",
- // AMP;[&] AMP[&].
- "\x02P;\x01&\x01P\x01&",
- // Aacute;[Á] Aacute[Á].
- "\x05cute;\x02Á\x04cute\x02Á",
- // Abreve;[Ă].
- "\x05reve;\x02Ă",
- // Acirc;[Â] Acirc[Â] Acy;[А].
- "\x04irc;\x02Â\x03irc\x02Â\x02y;\x02А",
- // Afr;[𝔄].
- "\x02r;\x04𝔄",
- // Agrave;[À] Agrave[À].
- "\x05rave;\x02À\x04rave\x02À",
- // Alpha;[Α].
- "\x04pha;\x02Α",
- // Amacr;[Ā].
- "\x04acr;\x02Ā",
- // And;[⩓].
- "\x02d;\x03⩓",
- // Aogon;[Ą] Aopf;[𝔸].
- "\x04gon;\x02Ą\x03pf;\x04𝔸",
- // ApplyFunction;[].
- "\x0cplyFunction;\x03",
- // Aring;[Å] Aring[Å].
- "\x04ing;\x02Å\x03ing\x02Å",
- // Assign;[≔] Ascr;[𝒜].
- "\x05sign;\x03≔\x03cr;\x04𝒜",
- // Atilde;[Ã] Atilde[Ã].
- "\x05ilde;\x02Ã\x04ilde\x02Ã",
- // Auml;[Ä] Auml[Ä].
- "\x03ml;\x02Ä\x02ml\x02Ä",
- // Backslash;[∖] Barwed;[⌆] Barv;[⫧].
- "\x08ckslash;\x03∖\x05rwed;\x03⌆\x03rv;\x03⫧",
- // Bcy;[Б].
- "\x02y;\x02Б",
- // Bernoullis;[ℬ] Because;[∵] Beta;[Β].
- "\x09rnoullis;\x03ℬ\x06cause;\x03∵\x03ta;\x02Β",
- // Bfr;[𝔅].
- "\x02r;\x04𝔅",
- // Bopf;[𝔹].
- "\x03pf;\x04𝔹",
- // Breve;[˘].
- "\x04eve;\x02˘",
- // Bscr;[ℬ].
- "\x03cr;\x03ℬ",
- // Bumpeq;[≎].
- "\x05mpeq;\x03≎",
- // CHcy;[Ч].
- "\x03cy;\x02Ч",
- // COPY;[©] COPY[©].
- "\x03PY;\x02©\x02PY\x02©",
- // CapitalDifferentialD;[ⅅ] Cayleys;[ℭ] Cacute;[Ć] Cap;[⋒].
- "\x13pitalDifferentialD;\x03ⅅ\x06yleys;\x03ℭ\x05cute;\x02Ć\x02p;\x03⋒",
- // Cconint;[∰] Ccaron;[Č] Ccedil;[Ç] Ccedil[Ç] Ccirc;[Ĉ].
- "\x06onint;\x03∰\x05aron;\x02Č\x05edil;\x02Ç\x04edil\x02Ç\x04irc;\x02Ĉ",
- // Cdot;[Ċ].
- "\x03ot;\x02Ċ",
- // CenterDot;[·] Cedilla;[¸].
- "\x08nterDot;\x02·\x06dilla;\x02¸",
- // Cfr;[ℭ].
- "\x02r;\x03ℭ",
- // Chi;[Χ].
- "\x02i;\x02Χ",
- // CircleMinus;[⊖] CircleTimes;[⊗] CirclePlus;[⊕] CircleDot;[⊙].
- "\x0arcleMinus;\x03⊖\x0arcleTimes;\x03⊗\x09rclePlus;\x03⊕\x08rcleDot;\x03⊙",
- // ClockwiseContourIntegral;[∲] CloseCurlyDoubleQuote;[”] CloseCurlyQuote;[’].
- "\x17ockwiseContourIntegral;\x03∲\x14oseCurlyDoubleQuote;\x03”\x0eoseCurlyQuote;\x03’",
- // CounterClockwiseContourIntegral;[∳] ContourIntegral;[∮] Congruent;[≡] Coproduct;[∐] Colone;[⩴] Conint;[∯] Colon;[∷] Copf;[ℂ].
- "\x1eunterClockwiseContourIntegral;\x03∳\x0entourIntegral;\x03∮\x08ngruent;\x03≡\x08product;\x03∐\x05lone;\x03⩴\x05nint;\x03∯\x04lon;\x03∷\x03pf;\x03ℂ",
- // Cross;[⨯].
- "\x04oss;\x03⨯",
- // Cscr;[𝒞].
- "\x03cr;\x04𝒞",
- // CupCap;[≍] Cup;[⋓].
- "\x05pCap;\x03≍\x02p;\x03⋓",
- // DDotrahd;[⤑] DD;[ⅅ].
- "\x07otrahd;\x03⤑\x01;\x03ⅅ",
- // DJcy;[Ђ].
- "\x03cy;\x02Ђ",
- // DScy;[Ѕ].
- "\x03cy;\x02Ѕ",
- // DZcy;[Џ].
- "\x03cy;\x02Џ",
- // Dagger;[‡] Dashv;[⫤] Darr;[↡].
- "\x05gger;\x03‡\x04shv;\x03⫤\x03rr;\x03↡",
- // Dcaron;[Ď] Dcy;[Д].
- "\x05aron;\x02Ď\x02y;\x02Д",
- // Delta;[Δ] Del;[∇].
- "\x04lta;\x02Δ\x02l;\x03∇",
- // Dfr;[𝔇].
- "\x02r;\x04𝔇",
- // DiacriticalDoubleAcute;[˝] DiacriticalAcute;[´] DiacriticalGrave;[`] DiacriticalTilde;[˜] DiacriticalDot;[˙] DifferentialD;[ⅆ] Diamond;[⋄].
- "\x15acriticalDoubleAcute;\x02˝\x0facriticalAcute;\x02´\x0facriticalGrave;\x01`\x0facriticalTilde;\x02˜\x0dacriticalDot;\x02˙\x0cfferentialD;\x03ⅆ\x06amond;\x03⋄",
- // DoubleLongLeftRightArrow;[⟺] DoubleContourIntegral;[∯] DoubleLeftRightArrow;[⇔] DoubleLongRightArrow;[⟹] DoubleLongLeftArrow;[⟸] DownLeftRightVector;[⥐] DownRightTeeVector;[⥟] DownRightVectorBar;[⥗] DoubleUpDownArrow;[⇕] DoubleVerticalBar;[∥] DownLeftTeeVector;[⥞] DownLeftVectorBar;[⥖] DoubleRightArrow;[⇒] DownArrowUpArrow;[⇵] DoubleDownArrow;[⇓] DoubleLeftArrow;[⇐] DownRightVector;[⇁] DoubleRightTee;[⊨] DownLeftVector;[↽] DoubleLeftTee;[⫤] DoubleUpArrow;[⇑] DownArrowBar;[⤓] DownTeeArrow;[↧] DoubleDot;[¨] DownArrow;[↓] DownBreve;[̑] Downarrow;[⇓] DotEqual;[≐] DownTee;[⊤] DotDot;[⃜] Dopf;[𝔻] Dot;[¨].
- "\x17ubleLongLeftRightArrow;\x03⟺\x14ubleContourIntegral;\x03∯\x13ubleLeftRightArrow;\x03⇔\x13ubleLongRightArrow;\x03⟹\x12ubleLongLeftArrow;\x03⟸\x12wnLeftRightVector;\x03⥐\x11wnRightTeeVector;\x03⥟\x11wnRightVectorBar;\x03⥗\x10ubleUpDownArrow;\x03⇕\x10ubleVerticalBar;\x03∥\x10wnLeftTeeVector;\x03⥞\x10wnLeftVectorBar;\x03⥖\x0fubleRightArrow;\x03⇒\x0fwnArrowUpArrow;\x03⇵\x0eubleDownArrow;\x03⇓\x0eubleLeftArrow;\x03⇐\x0ewnRightVector;\x03⇁\x0dubleRightTee;\x03⊨\x0dwnLeftVector;\x03↽\x0cubleLeftTee;\x03⫤\x0cubleUpArrow;\x03⇑\x0bwnArrowBar;\x03⤓\x0bwnTeeArrow;\x03↧\x08ubleDot;\x02¨\x08wnArrow;\x03↓\x08wnBreve;\x02̑\x08wnarrow;\x03⇓\x07tEqual;\x03≐\x06wnTee;\x03⊤\x05tDot;\x03⃜\x03pf;\x04𝔻\x02t;\x02¨",
- // Dstrok;[Đ] Dscr;[𝒟].
- "\x05trok;\x02Đ\x03cr;\x04𝒟",
- // ENG;[Ŋ].
- "\x02G;\x02Ŋ",
- // ETH;[Ð] ETH[Ð].
- "\x02H;\x02Ð\x01H\x02Ð",
- // Eacute;[É] Eacute[É].
- "\x05cute;\x02É\x04cute\x02É",
- // Ecaron;[Ě] Ecirc;[Ê] Ecirc[Ê] Ecy;[Э].
- "\x05aron;\x02Ě\x04irc;\x02Ê\x03irc\x02Ê\x02y;\x02Э",
- // Edot;[Ė].
- "\x03ot;\x02Ė",
- // Efr;[𝔈].
- "\x02r;\x04𝔈",
- // Egrave;[È] Egrave[È].
- "\x05rave;\x02È\x04rave\x02È",
- // Element;[∈].
- "\x06ement;\x03∈",
- // EmptyVerySmallSquare;[▫] EmptySmallSquare;[◻] Emacr;[Ē].
- "\x13ptyVerySmallSquare;\x03▫\x0fptySmallSquare;\x03◻\x04acr;\x02Ē",
- // Eogon;[Ę] Eopf;[𝔼].
- "\x04gon;\x02Ę\x03pf;\x04𝔼",
- // Epsilon;[Ε].
- "\x06silon;\x02Ε",
- // Equilibrium;[⇌] EqualTilde;[≂] Equal;[⩵].
- "\x0auilibrium;\x03⇌\x09ualTilde;\x03≂\x04ual;\x03⩵",
- // Escr;[ℰ] Esim;[⩳].
- "\x03cr;\x03ℰ\x03im;\x03⩳",
- // Eta;[Η].
- "\x02a;\x02Η",
- // Euml;[Ë] Euml[Ë].
- "\x03ml;\x02Ë\x02ml\x02Ë",
- // ExponentialE;[ⅇ] Exists;[∃].
- "\x0bponentialE;\x03ⅇ\x05ists;\x03∃",
- // Fcy;[Ф].
- "\x02y;\x02Ф",
- // Ffr;[𝔉].
- "\x02r;\x04𝔉",
- // FilledVerySmallSquare;[▪] FilledSmallSquare;[◼].
- "\x14lledVerySmallSquare;\x03▪\x10lledSmallSquare;\x03◼",
- // Fouriertrf;[ℱ] ForAll;[∀] Fopf;[𝔽].
- "\x09uriertrf;\x03ℱ\x05rAll;\x03∀\x03pf;\x04𝔽",
- // Fscr;[ℱ].
- "\x03cr;\x03ℱ",
- // GJcy;[Ѓ].
- "\x03cy;\x02Ѓ",
- // GT;[>].
- "\x01;\x01>",
- // Gammad;[Ϝ] Gamma;[Γ].
- "\x05mmad;\x02Ϝ\x04mma;\x02Γ",
- // Gbreve;[Ğ].
- "\x05reve;\x02Ğ",
- // Gcedil;[Ģ] Gcirc;[Ĝ] Gcy;[Г].
- "\x05edil;\x02Ģ\x04irc;\x02Ĝ\x02y;\x02Г",
- // Gdot;[Ġ].
- "\x03ot;\x02Ġ",
- // Gfr;[𝔊].
- "\x02r;\x04𝔊",
- // Gg;[⋙].
- "\x01;\x03⋙",
- // Gopf;[𝔾].
- "\x03pf;\x04𝔾",
- // GreaterSlantEqual;[⩾] GreaterEqualLess;[⋛] GreaterFullEqual;[≧] GreaterGreater;[⪢] GreaterEqual;[≥] GreaterTilde;[≳] GreaterLess;[≷].
- "\x10eaterSlantEqual;\x03⩾\x0featerEqualLess;\x03⋛\x0featerFullEqual;\x03≧\x0deaterGreater;\x03⪢\x0beaterEqual;\x03≥\x0beaterTilde;\x03≳\x0aeaterLess;\x03≷",
- // Gscr;[𝒢].
- "\x03cr;\x04𝒢",
- // Gt;[≫].
- "\x01;\x03≫",
- // HARDcy;[Ъ].
- "\x05RDcy;\x02Ъ",
- // Hacek;[ˇ] Hat;[^].
- "\x04cek;\x02ˇ\x02t;\x01^",
- // Hcirc;[Ĥ].
- "\x04irc;\x02Ĥ",
- // Hfr;[ℌ].
- "\x02r;\x03ℌ",
- // HilbertSpace;[ℋ].
- "\x0blbertSpace;\x03ℋ",
- // HorizontalLine;[─] Hopf;[ℍ].
- "\x0drizontalLine;\x03─\x03pf;\x03ℍ",
- // Hstrok;[Ħ] Hscr;[ℋ].
- "\x05trok;\x02Ħ\x03cr;\x03ℋ",
- // HumpDownHump;[≎] HumpEqual;[≏].
- "\x0bmpDownHump;\x03≎\x08mpEqual;\x03≏",
- // IEcy;[Е].
- "\x03cy;\x02Е",
- // IJlig;[IJ].
- "\x04lig;\x02IJ",
- // IOcy;[Ё].
- "\x03cy;\x02Ё",
- // Iacute;[Í] Iacute[Í].
- "\x05cute;\x02Í\x04cute\x02Í",
- // Icirc;[Î] Icirc[Î] Icy;[И].
- "\x04irc;\x02Î\x03irc\x02Î\x02y;\x02И",
- // Idot;[İ].
- "\x03ot;\x02İ",
- // Ifr;[ℑ].
- "\x02r;\x03ℑ",
- // Igrave;[Ì] Igrave[Ì].
- "\x05rave;\x02Ì\x04rave\x02Ì",
- // ImaginaryI;[ⅈ] Implies;[⇒] Imacr;[Ī] Im;[ℑ].
- "\x09aginaryI;\x03ⅈ\x06plies;\x03⇒\x04acr;\x02Ī\x01;\x03ℑ",
- // InvisibleComma;[] InvisibleTimes;[] Intersection;[⋂] Integral;[∫] Int;[∬].
- "\x0dvisibleComma;\x03\x0dvisibleTimes;\x03\x0btersection;\x03⋂\x07tegral;\x03∫\x02t;\x03∬",
- // Iogon;[Į] Iopf;[𝕀] Iota;[Ι].
- "\x04gon;\x02Į\x03pf;\x04𝕀\x03ta;\x02Ι",
- // Iscr;[ℐ].
- "\x03cr;\x03ℐ",
- // Itilde;[Ĩ].
- "\x05ilde;\x02Ĩ",
- // Iukcy;[І] Iuml;[Ï] Iuml[Ï].
- "\x04kcy;\x02І\x03ml;\x02Ï\x02ml\x02Ï",
- // Jcirc;[Ĵ] Jcy;[Й].
- "\x04irc;\x02Ĵ\x02y;\x02Й",
- // Jfr;[𝔍].
- "\x02r;\x04𝔍",
- // Jopf;[𝕁].
- "\x03pf;\x04𝕁",
- // Jsercy;[Ј] Jscr;[𝒥].
- "\x05ercy;\x02Ј\x03cr;\x04𝒥",
- // Jukcy;[Є].
- "\x04kcy;\x02Є",
- // KHcy;[Х].
- "\x03cy;\x02Х",
- // KJcy;[Ќ].
- "\x03cy;\x02Ќ",
- // Kappa;[Κ].
- "\x04ppa;\x02Κ",
- // Kcedil;[Ķ] Kcy;[К].
- "\x05edil;\x02Ķ\x02y;\x02К",
- // Kfr;[𝔎].
- "\x02r;\x04𝔎",
- // Kopf;[𝕂].
- "\x03pf;\x04𝕂",
- // Kscr;[𝒦].
- "\x03cr;\x04𝒦",
- // LJcy;[Љ].
- "\x03cy;\x02Љ",
- // LT;[<].
- "\x01;\x01<",
- // Laplacetrf;[ℒ] Lacute;[Ĺ] Lambda;[Λ] Lang;[⟪] Larr;[↞].
- "\x09placetrf;\x03ℒ\x05cute;\x02Ĺ\x05mbda;\x02Λ\x03ng;\x03⟪\x03rr;\x03↞",
- // Lcaron;[Ľ] Lcedil;[Ļ] Lcy;[Л].
- "\x05aron;\x02Ľ\x05edil;\x02Ļ\x02y;\x02Л",
- // LeftArrowRightArrow;[⇆] LeftDoubleBracket;[⟦] LeftDownTeeVector;[⥡] LeftDownVectorBar;[⥙] LeftTriangleEqual;[⊴] LeftAngleBracket;[⟨] LeftUpDownVector;[⥑] LessEqualGreater;[⋚] LeftRightVector;[⥎] LeftTriangleBar;[⧏] LeftUpTeeVector;[⥠] LeftUpVectorBar;[⥘] LeftDownVector;[⇃] LeftRightArrow;[↔] Leftrightarrow;[⇔] LessSlantEqual;[⩽] LeftTeeVector;[⥚] LeftVectorBar;[⥒] LessFullEqual;[≦] LeftArrowBar;[⇤] LeftTeeArrow;[↤] LeftTriangle;[⊲] LeftUpVector;[↿] LeftCeiling;[⌈] LessGreater;[≶] LeftVector;[↼] LeftArrow;[←] LeftFloor;[⌊] Leftarrow;[⇐] LessTilde;[≲] LessLess;[⪡] LeftTee;[⊣].
- "\x12ftArrowRightArrow;\x03⇆\x10ftDoubleBracket;\x03⟦\x10ftDownTeeVector;\x03⥡\x10ftDownVectorBar;\x03⥙\x10ftTriangleEqual;\x03⊴\x0fftAngleBracket;\x03⟨\x0fftUpDownVector;\x03⥑\x0fssEqualGreater;\x03⋚\x0eftRightVector;\x03⥎\x0eftTriangleBar;\x03⧏\x0eftUpTeeVector;\x03⥠\x0eftUpVectorBar;\x03⥘\x0dftDownVector;\x03⇃\x0dftRightArrow;\x03↔\x0dftrightarrow;\x03⇔\x0dssSlantEqual;\x03⩽\x0cftTeeVector;\x03⥚\x0cftVectorBar;\x03⥒\x0cssFullEqual;\x03≦\x0bftArrowBar;\x03⇤\x0bftTeeArrow;\x03↤\x0bftTriangle;\x03⊲\x0bftUpVector;\x03↿\x0aftCeiling;\x03⌈\x0assGreater;\x03≶\x09ftVector;\x03↼\x08ftArrow;\x03←\x08ftFloor;\x03⌊\x08ftarrow;\x03⇐\x08ssTilde;\x03≲\x07ssLess;\x03⪡\x06ftTee;\x03⊣",
- // Lfr;[𝔏].
- "\x02r;\x04𝔏",
- // Lleftarrow;[⇚] Ll;[⋘].
- "\x09eftarrow;\x03⇚\x01;\x03⋘",
- // Lmidot;[Ŀ].
- "\x05idot;\x02Ŀ",
- // LongLeftRightArrow;[⟷] Longleftrightarrow;[⟺] LowerRightArrow;[↘] LongRightArrow;[⟶] Longrightarrow;[⟹] LowerLeftArrow;[↙] LongLeftArrow;[⟵] Longleftarrow;[⟸] Lopf;[𝕃].
- "\x11ngLeftRightArrow;\x03⟷\x11ngleftrightarrow;\x03⟺\x0ewerRightArrow;\x03↘\x0dngRightArrow;\x03⟶\x0dngrightarrow;\x03⟹\x0dwerLeftArrow;\x03↙\x0cngLeftArrow;\x03⟵\x0cngleftarrow;\x03⟸\x03pf;\x04𝕃",
- // Lstrok;[Ł] Lscr;[ℒ] Lsh;[↰].
- "\x05trok;\x02Ł\x03cr;\x03ℒ\x02h;\x03↰",
- // Lt;[≪].
- "\x01;\x03≪",
- // Map;[⤅].
- "\x02p;\x03⤅",
- // Mcy;[М].
- "\x02y;\x02М",
- // MediumSpace;[ ] Mellintrf;[ℳ].
- "\x0adiumSpace;\x03 \x08llintrf;\x03ℳ",
- // Mfr;[𝔐].
- "\x02r;\x04𝔐",
- // MinusPlus;[∓].
- "\x08nusPlus;\x03∓",
- // Mopf;[𝕄].
- "\x03pf;\x04𝕄",
- // Mscr;[ℳ].
- "\x03cr;\x03ℳ",
- // Mu;[Μ].
- "\x01;\x02Μ",
- // NJcy;[Њ].
- "\x03cy;\x02Њ",
- // Nacute;[Ń].
- "\x05cute;\x02Ń",
- // Ncaron;[Ň] Ncedil;[Ņ] Ncy;[Н].
- "\x05aron;\x02Ň\x05edil;\x02Ņ\x02y;\x02Н",
- // NegativeVeryThinSpace;[] NestedGreaterGreater;[≫] NegativeMediumSpace;[] NegativeThickSpace;[] NegativeThinSpace;[] NestedLessLess;[≪] NewLine;[\xa].
- "\x14gativeVeryThinSpace;\x03\x13stedGreaterGreater;\x03≫\x12gativeMediumSpace;\x03\x11gativeThickSpace;\x03\x10gativeThinSpace;\x03\x0dstedLessLess;\x03≪\x06wLine;\x01\xa",
- // Nfr;[𝔑].
- "\x02r;\x04𝔑",
- // NotNestedGreaterGreater;[⪢̸] NotSquareSupersetEqual;[⋣] NotPrecedesSlantEqual;[⋠] NotRightTriangleEqual;[⋭] NotSucceedsSlantEqual;[⋡] NotDoubleVerticalBar;[∦] NotGreaterSlantEqual;[⩾̸] NotLeftTriangleEqual;[⋬] NotSquareSubsetEqual;[⋢] NotGreaterFullEqual;[≧̸] NotRightTriangleBar;[⧐̸] NotLeftTriangleBar;[⧏̸] NotGreaterGreater;[≫̸] NotLessSlantEqual;[⩽̸] NotNestedLessLess;[⪡̸] NotReverseElement;[∌] NotSquareSuperset;[⊐̸] NotTildeFullEqual;[≇] NonBreakingSpace;[ ] NotPrecedesEqual;[⪯̸] NotRightTriangle;[⋫] NotSucceedsEqual;[⪰̸] NotSucceedsTilde;[≿̸] NotSupersetEqual;[⊉] NotGreaterEqual;[≱] NotGreaterTilde;[≵] NotHumpDownHump;[≎̸] NotLeftTriangle;[⋪] NotSquareSubset;[⊏̸] NotGreaterLess;[≹] NotLessGreater;[≸] NotSubsetEqual;[⊈] NotVerticalBar;[∤] NotEqualTilde;[≂̸] NotTildeEqual;[≄] NotTildeTilde;[≉] NotCongruent;[≢] NotHumpEqual;[≏̸] NotLessEqual;[≰] NotLessTilde;[≴] NotLessLess;[≪̸] NotPrecedes;[⊀] NotSucceeds;[⊁] NotSuperset;[⊃⃒] NotElement;[∉] NotGreater;[≯] NotCupCap;[≭] NotExists;[∄] NotSubset;[⊂⃒] NotEqual;[≠] NotTilde;[≁] NoBreak;[] NotLess;[≮] Nopf;[ℕ] Not;[⫬].
- "\x16tNestedGreaterGreater;\x05⪢̸\x15tSquareSupersetEqual;\x03⋣\x14tPrecedesSlantEqual;\x03⋠\x14tRightTriangleEqual;\x03⋭\x14tSucceedsSlantEqual;\x03⋡\x13tDoubleVerticalBar;\x03∦\x13tGreaterSlantEqual;\x05⩾̸\x13tLeftTriangleEqual;\x03⋬\x13tSquareSubsetEqual;\x03⋢\x12tGreaterFullEqual;\x05≧̸\x12tRightTriangleBar;\x05⧐̸\x11tLeftTriangleBar;\x05⧏̸\x10tGreaterGreater;\x05≫̸\x10tLessSlantEqual;\x05⩽̸\x10tNestedLessLess;\x05⪡̸\x10tReverseElement;\x03∌\x10tSquareSuperset;\x05⊐̸\x10tTildeFullEqual;\x03≇\x0fnBreakingSpace;\x02 \x0ftPrecedesEqual;\x05⪯̸\x0ftRightTriangle;\x03⋫\x0ftSucceedsEqual;\x05⪰̸\x0ftSucceedsTilde;\x05≿̸\x0ftSupersetEqual;\x03⊉\x0etGreaterEqual;\x03≱\x0etGreaterTilde;\x03≵\x0etHumpDownHump;\x05≎̸\x0etLeftTriangle;\x03⋪\x0etSquareSubset;\x05⊏̸\x0dtGreaterLess;\x03≹\x0dtLessGreater;\x03≸\x0dtSubsetEqual;\x03⊈\x0dtVerticalBar;\x03∤\x0ctEqualTilde;\x05≂̸\x0ctTildeEqual;\x03≄\x0ctTildeTilde;\x03≉\x0btCongruent;\x03≢\x0btHumpEqual;\x05≏̸\x0btLessEqual;\x03≰\x0btLessTilde;\x03≴\x0atLessLess;\x05≪̸\x0atPrecedes;\x03⊀\x0atSucceeds;\x03⊁\x0atSuperset;\x06⊃⃒\x09tElement;\x03∉\x09tGreater;\x03≯\x08tCupCap;\x03≭\x08tExists;\x03∄\x08tSubset;\x06⊂⃒\x07tEqual;\x03≠\x07tTilde;\x03≁\x06Break;\x03\x06tLess;\x03≮\x03pf;\x03ℕ\x02t;\x03⫬",
- // Nscr;[𝒩].
- "\x03cr;\x04𝒩",
- // Ntilde;[Ñ] Ntilde[Ñ].
- "\x05ilde;\x02Ñ\x04ilde\x02Ñ",
- // Nu;[Ν].
- "\x01;\x02Ν",
- // OElig;[Œ].
- "\x04lig;\x02Œ",
- // Oacute;[Ó] Oacute[Ó].
- "\x05cute;\x02Ó\x04cute\x02Ó",
- // Ocirc;[Ô] Ocirc[Ô] Ocy;[О].
- "\x04irc;\x02Ô\x03irc\x02Ô\x02y;\x02О",
- // Odblac;[Ő].
- "\x05blac;\x02Ő",
- // Ofr;[𝔒].
- "\x02r;\x04𝔒",
- // Ograve;[Ò] Ograve[Ò].
- "\x05rave;\x02Ò\x04rave\x02Ò",
- // Omicron;[Ο] Omacr;[Ō] Omega;[Ω].
- "\x06icron;\x02Ο\x04acr;\x02Ō\x04ega;\x02Ω",
- // Oopf;[𝕆].
- "\x03pf;\x04𝕆",
- // OpenCurlyDoubleQuote;[“] OpenCurlyQuote;[‘].
- "\x13enCurlyDoubleQuote;\x03“\x0denCurlyQuote;\x03‘",
- // Or;[⩔].
- "\x01;\x03⩔",
- // Oslash;[Ø] Oslash[Ø] Oscr;[𝒪].
- "\x05lash;\x02Ø\x04lash\x02Ø\x03cr;\x04𝒪",
- // Otilde;[Õ] Otimes;[⨷] Otilde[Õ].
- "\x05ilde;\x02Õ\x05imes;\x03⨷\x04ilde\x02Õ",
- // Ouml;[Ö] Ouml[Ö].
- "\x03ml;\x02Ö\x02ml\x02Ö",
- // OverParenthesis;[⏜] OverBracket;[⎴] OverBrace;[⏞] OverBar;[‾].
- "\x0eerParenthesis;\x03⏜\x0aerBracket;\x03⎴\x08erBrace;\x03⏞\x06erBar;\x03‾",
- // PartialD;[∂].
- "\x07rtialD;\x03∂",
- // Pcy;[П].
- "\x02y;\x02П",
- // Pfr;[𝔓].
- "\x02r;\x04𝔓",
- // Phi;[Φ].
- "\x02i;\x02Φ",
- // Pi;[Π].
- "\x01;\x02Π",
- // PlusMinus;[±].
- "\x08usMinus;\x02±",
- // Poincareplane;[ℌ] Popf;[ℙ].
- "\x0cincareplane;\x03ℌ\x03pf;\x03ℙ",
- // PrecedesSlantEqual;[≼] PrecedesEqual;[⪯] PrecedesTilde;[≾] Proportional;[∝] Proportion;[∷] Precedes;[≺] Product;[∏] Prime;[″] Pr;[⪻].
- "\x11ecedesSlantEqual;\x03≼\x0cecedesEqual;\x03⪯\x0cecedesTilde;\x03≾\x0boportional;\x03∝\x09oportion;\x03∷\x07ecedes;\x03≺\x06oduct;\x03∏\x04ime;\x03″\x01;\x03⪻",
- // Pscr;[𝒫] Psi;[Ψ].
- "\x03cr;\x04𝒫\x02i;\x02Ψ",
- // QUOT;[\"] QUOT[\"].
- "\x03OT;\x01\"\x02OT\x01\"",
- // Qfr;[𝔔].
- "\x02r;\x04𝔔",
- // Qopf;[ℚ].
- "\x03pf;\x03ℚ",
- // Qscr;[𝒬].
- "\x03cr;\x04𝒬",
- // RBarr;[⤐].
- "\x04arr;\x03⤐",
- // REG;[®] REG[®].
- "\x02G;\x02®\x01G\x02®",
- // Racute;[Ŕ] Rarrtl;[⤖] Rang;[⟫] Rarr;[↠].
- "\x05cute;\x02Ŕ\x05rrtl;\x03⤖\x03ng;\x03⟫\x03rr;\x03↠",
- // Rcaron;[Ř] Rcedil;[Ŗ] Rcy;[Р].
- "\x05aron;\x02Ř\x05edil;\x02Ŗ\x02y;\x02Р",
- // ReverseUpEquilibrium;[⥯] ReverseEquilibrium;[⇋] ReverseElement;[∋] Re;[ℜ].
- "\x13verseUpEquilibrium;\x03⥯\x11verseEquilibrium;\x03⇋\x0dverseElement;\x03∋\x01;\x03ℜ",
- // Rfr;[ℜ].
- "\x02r;\x03ℜ",
- // Rho;[Ρ].
- "\x02o;\x02Ρ",
- // RightArrowLeftArrow;[⇄] RightDoubleBracket;[⟧] RightDownTeeVector;[⥝] RightDownVectorBar;[⥕] RightTriangleEqual;[⊵] RightAngleBracket;[⟩] RightUpDownVector;[⥏] RightTriangleBar;[⧐] RightUpTeeVector;[⥜] RightUpVectorBar;[⥔] RightDownVector;[⇂] RightTeeVector;[⥛] RightVectorBar;[⥓] RightArrowBar;[⇥] RightTeeArrow;[↦] RightTriangle;[⊳] RightUpVector;[↾] RightCeiling;[⌉] RightVector;[⇀] RightArrow;[→] RightFloor;[⌋] Rightarrow;[⇒] RightTee;[⊢].
- "\x12ghtArrowLeftArrow;\x03⇄\x11ghtDoubleBracket;\x03⟧\x11ghtDownTeeVector;\x03⥝\x11ghtDownVectorBar;\x03⥕\x11ghtTriangleEqual;\x03⊵\x10ghtAngleBracket;\x03⟩\x10ghtUpDownVector;\x03⥏\x0fghtTriangleBar;\x03⧐\x0fghtUpTeeVector;\x03⥜\x0fghtUpVectorBar;\x03⥔\x0eghtDownVector;\x03⇂\x0dghtTeeVector;\x03⥛\x0dghtVectorBar;\x03⥓\x0cghtArrowBar;\x03⇥\x0cghtTeeArrow;\x03↦\x0cghtTriangle;\x03⊳\x0cghtUpVector;\x03↾\x0bghtCeiling;\x03⌉\x0aghtVector;\x03⇀\x09ghtArrow;\x03→\x09ghtFloor;\x03⌋\x09ghtarrow;\x03⇒\x07ghtTee;\x03⊢",
- // RoundImplies;[⥰] Ropf;[ℝ].
- "\x0bundImplies;\x03⥰\x03pf;\x03ℝ",
- // Rrightarrow;[⇛].
- "\x0aightarrow;\x03⇛",
- // Rscr;[ℛ] Rsh;[↱].
- "\x03cr;\x03ℛ\x02h;\x03↱",
- // RuleDelayed;[⧴].
- "\x0aleDelayed;\x03⧴",
- // SHCHcy;[Щ] SHcy;[Ш].
- "\x05CHcy;\x02Щ\x03cy;\x02Ш",
- // SOFTcy;[Ь].
- "\x05FTcy;\x02Ь",
- // Sacute;[Ś].
- "\x05cute;\x02Ś",
- // Scaron;[Š] Scedil;[Ş] Scirc;[Ŝ] Scy;[С] Sc;[⪼].
- "\x05aron;\x02Š\x05edil;\x02Ş\x04irc;\x02Ŝ\x02y;\x02С\x01;\x03⪼",
- // Sfr;[𝔖].
- "\x02r;\x04𝔖",
- // ShortRightArrow;[→] ShortDownArrow;[↓] ShortLeftArrow;[←] ShortUpArrow;[↑].
- "\x0eortRightArrow;\x03→\x0dortDownArrow;\x03↓\x0dortLeftArrow;\x03←\x0bortUpArrow;\x03↑",
- // Sigma;[Σ].
- "\x04gma;\x02Σ",
- // SmallCircle;[∘].
- "\x0aallCircle;\x03∘",
- // Sopf;[𝕊].
- "\x03pf;\x04𝕊",
- // SquareSupersetEqual;[⊒] SquareIntersection;[⊓] SquareSubsetEqual;[⊑] SquareSuperset;[⊐] SquareSubset;[⊏] SquareUnion;[⊔] Square;[□] Sqrt;[√].
- "\x12uareSupersetEqual;\x03⊒\x11uareIntersection;\x03⊓\x10uareSubsetEqual;\x03⊑\x0duareSuperset;\x03⊐\x0buareSubset;\x03⊏\x0auareUnion;\x03⊔\x05uare;\x03□\x03rt;\x03√",
- // Sscr;[𝒮].
- "\x03cr;\x04𝒮",
- // Star;[⋆].
- "\x03ar;\x03⋆",
- // SucceedsSlantEqual;[≽] SucceedsEqual;[⪰] SucceedsTilde;[≿] SupersetEqual;[⊇] SubsetEqual;[⊆] Succeeds;[≻] SuchThat;[∋] Superset;[⊃] Subset;[⋐] Supset;[⋑] Sub;[⋐] Sum;[∑] Sup;[⋑].
- "\x11cceedsSlantEqual;\x03≽\x0ccceedsEqual;\x03⪰\x0ccceedsTilde;\x03≿\x0cpersetEqual;\x03⊇\x0absetEqual;\x03⊆\x07cceeds;\x03≻\x07chThat;\x03∋\x07perset;\x03⊃\x05bset;\x03⋐\x05pset;\x03⋑\x02b;\x03⋐\x02m;\x03∑\x02p;\x03⋑",
- // THORN;[Þ] THORN[Þ].
- "\x04ORN;\x02Þ\x03ORN\x02Þ",
- // TRADE;[™].
- "\x04ADE;\x03™",
- // TSHcy;[Ћ] TScy;[Ц].
- "\x04Hcy;\x02Ћ\x03cy;\x02Ц",
- // Tab;[\x9] Tau;[Τ].
- "\x02b;\x01\x9\x02u;\x02Τ",
- // Tcaron;[Ť] Tcedil;[Ţ] Tcy;[Т].
- "\x05aron;\x02Ť\x05edil;\x02Ţ\x02y;\x02Т",
- // Tfr;[𝔗].
- "\x02r;\x04𝔗",
- // ThickSpace;[ ] Therefore;[∴] ThinSpace;[ ] Theta;[Θ].
- "\x09ickSpace;\x06 \x08erefore;\x03∴\x08inSpace;\x03 \x04eta;\x02Θ",
- // TildeFullEqual;[≅] TildeEqual;[≃] TildeTilde;[≈] Tilde;[∼].
- "\x0dldeFullEqual;\x03≅\x09ldeEqual;\x03≃\x09ldeTilde;\x03≈\x04lde;\x03∼",
- // Topf;[𝕋].
- "\x03pf;\x04𝕋",
- // TripleDot;[⃛].
- "\x08ipleDot;\x03⃛",
- // Tstrok;[Ŧ] Tscr;[𝒯].
- "\x05trok;\x02Ŧ\x03cr;\x04𝒯",
- // Uarrocir;[⥉] Uacute;[Ú] Uacute[Ú] Uarr;[↟].
- "\x07rrocir;\x03⥉\x05cute;\x02Ú\x04cute\x02Ú\x03rr;\x03↟",
- // Ubreve;[Ŭ] Ubrcy;[Ў].
- "\x05reve;\x02Ŭ\x04rcy;\x02Ў",
- // Ucirc;[Û] Ucirc[Û] Ucy;[У].
- "\x04irc;\x02Û\x03irc\x02Û\x02y;\x02У",
- // Udblac;[Ű].
- "\x05blac;\x02Ű",
- // Ufr;[𝔘].
- "\x02r;\x04𝔘",
- // Ugrave;[Ù] Ugrave[Ù].
- "\x05rave;\x02Ù\x04rave\x02Ù",
- // Umacr;[Ū].
- "\x04acr;\x02Ū",
- // UnderParenthesis;[⏝] UnderBracket;[⎵] UnderBrace;[⏟] UnionPlus;[⊎] UnderBar;[_] Union;[⋃].
- "\x0fderParenthesis;\x03⏝\x0bderBracket;\x03⎵\x09derBrace;\x03⏟\x08ionPlus;\x03⊎\x07derBar;\x01_\x04ion;\x03⋃",
- // Uogon;[Ų] Uopf;[𝕌].
- "\x04gon;\x02Ų\x03pf;\x04𝕌",
- // UpArrowDownArrow;[⇅] UpperRightArrow;[↗] UpperLeftArrow;[↖] UpEquilibrium;[⥮] UpDownArrow;[↕] Updownarrow;[⇕] UpArrowBar;[⤒] UpTeeArrow;[↥] UpArrow;[↑] Uparrow;[⇑] Upsilon;[Υ] UpTee;[⊥] Upsi;[ϒ].
- "\x0fArrowDownArrow;\x03⇅\x0eperRightArrow;\x03↗\x0dperLeftArrow;\x03↖\x0cEquilibrium;\x03⥮\x0aDownArrow;\x03↕\x0adownarrow;\x03⇕\x09ArrowBar;\x03⤒\x09TeeArrow;\x03↥\x06Arrow;\x03↑\x06arrow;\x03⇑\x06silon;\x02Υ\x04Tee;\x03⊥\x03si;\x02ϒ",
- // Uring;[Ů].
- "\x04ing;\x02Ů",
- // Uscr;[𝒰].
- "\x03cr;\x04𝒰",
- // Utilde;[Ũ].
- "\x05ilde;\x02Ũ",
- // Uuml;[Ü] Uuml[Ü].
- "\x03ml;\x02Ü\x02ml\x02Ü",
- // VDash;[⊫].
- "\x04ash;\x03⊫",
- // Vbar;[⫫].
- "\x03ar;\x03⫫",
- // Vcy;[В].
- "\x02y;\x02В",
- // Vdashl;[⫦] Vdash;[⊩].
- "\x05ashl;\x03⫦\x04ash;\x03⊩",
- // VerticalSeparator;[❘] VerticalTilde;[≀] VeryThinSpace;[ ] VerticalLine;[|] VerticalBar;[∣] Verbar;[‖] Vert;[‖] Vee;[⋁].
- "\x10rticalSeparator;\x03❘\x0crticalTilde;\x03≀\x0cryThinSpace;\x03 \x0brticalLine;\x01|\x0articalBar;\x03∣\x05rbar;\x03‖\x03rt;\x03‖\x02e;\x03⋁",
- // Vfr;[𝔙].
- "\x02r;\x04𝔙",
- // Vopf;[𝕍].
- "\x03pf;\x04𝕍",
- // Vscr;[𝒱].
- "\x03cr;\x04𝒱",
- // Vvdash;[⊪].
- "\x05dash;\x03⊪",
- // Wcirc;[Ŵ].
- "\x04irc;\x02Ŵ",
- // Wedge;[⋀].
- "\x04dge;\x03⋀",
- // Wfr;[𝔚].
- "\x02r;\x04𝔚",
- // Wopf;[𝕎].
- "\x03pf;\x04𝕎",
- // Wscr;[𝒲].
- "\x03cr;\x04𝒲",
- // Xfr;[𝔛].
- "\x02r;\x04𝔛",
- // Xi;[Ξ].
- "\x01;\x02Ξ",
- // Xopf;[𝕏].
- "\x03pf;\x04𝕏",
- // Xscr;[𝒳].
- "\x03cr;\x04𝒳",
- // YAcy;[Я].
- "\x03cy;\x02Я",
- // YIcy;[Ї].
- "\x03cy;\x02Ї",
- // YUcy;[Ю].
- "\x03cy;\x02Ю",
- // Yacute;[Ý] Yacute[Ý].
- "\x05cute;\x02Ý\x04cute\x02Ý",
- // Ycirc;[Ŷ] Ycy;[Ы].
- "\x04irc;\x02Ŷ\x02y;\x02Ы",
- // Yfr;[𝔜].
- "\x02r;\x04𝔜",
- // Yopf;[𝕐].
- "\x03pf;\x04𝕐",
- // Yscr;[𝒴].
- "\x03cr;\x04𝒴",
- // Yuml;[Ÿ].
- "\x03ml;\x02Ÿ",
- // ZHcy;[Ж].
- "\x03cy;\x02Ж",
- // Zacute;[Ź].
- "\x05cute;\x02Ź",
- // Zcaron;[Ž] Zcy;[З].
- "\x05aron;\x02Ž\x02y;\x02З",
- // Zdot;[Ż].
- "\x03ot;\x02Ż",
- // ZeroWidthSpace;[] Zeta;[Ζ].
- "\x0droWidthSpace;\x03\x03ta;\x02Ζ",
- // Zfr;[ℨ].
- "\x02r;\x03ℨ",
- // Zopf;[ℤ].
- "\x03pf;\x03ℤ",
- // Zscr;[𝒵].
- "\x03cr;\x04𝒵",
- // aacute;[á] aacute[á].
- "\x05cute;\x02á\x04cute\x02á",
- // abreve;[ă].
- "\x05reve;\x02ă",
- // acirc;[â] acute;[´] acirc[â] acute[´] acE;[∾̳] acd;[∿] acy;[а] ac;[∾].
- "\x04irc;\x02â\x04ute;\x02´\x03irc\x02â\x03ute\x02´\x02E;\x05∾̳\x02d;\x03∿\x02y;\x02а\x01;\x03∾",
- // aelig;[æ] aelig[æ].
- "\x04lig;\x02æ\x03lig\x02æ",
- // afr;[𝔞] af;[].
- "\x02r;\x04𝔞\x01;\x03",
- // agrave;[à] agrave[à].
- "\x05rave;\x02à\x04rave\x02à",
- // alefsym;[ℵ] aleph;[ℵ] alpha;[α].
- "\x06efsym;\x03ℵ\x04eph;\x03ℵ\x04pha;\x02α",
- // amacr;[ā] amalg;[⨿] amp;[&] amp[&].
- "\x04acr;\x02ā\x04alg;\x03⨿\x02p;\x01&\x01p\x01&",
- // andslope;[⩘] angmsdaa;[⦨] angmsdab;[⦩] angmsdac;[⦪] angmsdad;[⦫] angmsdae;[⦬] angmsdaf;[⦭] angmsdag;[⦮] angmsdah;[⦯] angrtvbd;[⦝] angrtvb;[⊾] angzarr;[⍼] andand;[⩕] angmsd;[∡] angsph;[∢] angle;[∠] angrt;[∟] angst;[Å] andd;[⩜] andv;[⩚] ange;[⦤] and;[∧] ang;[∠].
- "\x07dslope;\x03⩘\x07gmsdaa;\x03⦨\x07gmsdab;\x03⦩\x07gmsdac;\x03⦪\x07gmsdad;\x03⦫\x07gmsdae;\x03⦬\x07gmsdaf;\x03⦭\x07gmsdag;\x03⦮\x07gmsdah;\x03⦯\x07grtvbd;\x03⦝\x06grtvb;\x03⊾\x06gzarr;\x03⍼\x05dand;\x03⩕\x05gmsd;\x03∡\x05gsph;\x03∢\x04gle;\x03∠\x04grt;\x03∟\x04gst;\x02Å\x03dd;\x03⩜\x03dv;\x03⩚\x03ge;\x03⦤\x02d;\x03∧\x02g;\x03∠",
- // aogon;[ą] aopf;[𝕒].
- "\x04gon;\x02ą\x03pf;\x04𝕒",
- // approxeq;[≊] apacir;[⩯] approx;[≈] apid;[≋] apos;['] apE;[⩰] ape;[≊] ap;[≈].
- "\x07proxeq;\x03≊\x05acir;\x03⩯\x05prox;\x03≈\x03id;\x03≋\x03os;\x01'\x02E;\x03⩰\x02e;\x03≊\x01;\x03≈",
- // aring;[å] aring[å].
- "\x04ing;\x02å\x03ing\x02å",
- // asympeq;[≍] asymp;[≈] ascr;[𝒶] ast;[*].
- "\x06ympeq;\x03≍\x04ymp;\x03≈\x03cr;\x04𝒶\x02t;\x01*",
- // atilde;[ã] atilde[ã].
- "\x05ilde;\x02ã\x04ilde\x02ã",
- // auml;[ä] auml[ä].
- "\x03ml;\x02ä\x02ml\x02ä",
- // awconint;[∳] awint;[⨑].
- "\x07conint;\x03∳\x04int;\x03⨑",
- // bNot;[⫭].
- "\x03ot;\x03⫭",
- // backepsilon;[϶] backprime;[‵] backsimeq;[⋍] backcong;[≌] barwedge;[⌅] backsim;[∽] barvee;[⊽] barwed;[⌅].
- "\x0ackepsilon;\x02϶\x08ckprime;\x03‵\x08cksimeq;\x03⋍\x07ckcong;\x03≌\x07rwedge;\x03⌅\x06cksim;\x03∽\x05rvee;\x03⊽\x05rwed;\x03⌅",
- // bbrktbrk;[⎶] bbrk;[⎵].
- "\x07rktbrk;\x03⎶\x03rk;\x03⎵",
- // bcong;[≌] bcy;[б].
- "\x04ong;\x03≌\x02y;\x02б",
- // bdquo;[„].
- "\x04quo;\x03„",
- // because;[∵] bemptyv;[⦰] between;[≬] becaus;[∵] bernou;[ℬ] bepsi;[϶] beta;[β] beth;[ℶ].
- "\x06cause;\x03∵\x06mptyv;\x03⦰\x06tween;\x03≬\x05caus;\x03∵\x05rnou;\x03ℬ\x04psi;\x02϶\x03ta;\x02β\x03th;\x03ℶ",
- // bfr;[𝔟].
- "\x02r;\x04𝔟",
- // bigtriangledown;[▽] bigtriangleup;[△] bigotimes;[⨂] bigoplus;[⨁] bigsqcup;[⨆] biguplus;[⨄] bigwedge;[⋀] bigcirc;[◯] bigodot;[⨀] bigstar;[★] bigcap;[⋂] bigcup;[⋃] bigvee;[⋁].
- "\x0egtriangledown;\x03▽\x0cgtriangleup;\x03△\x08gotimes;\x03⨂\x07goplus;\x03⨁\x07gsqcup;\x03⨆\x07guplus;\x03⨄\x07gwedge;\x03⋀\x06gcirc;\x03◯\x06godot;\x03⨀\x06gstar;\x03★\x05gcap;\x03⋂\x05gcup;\x03⋃\x05gvee;\x03⋁",
- // bkarow;[⤍].
- "\x05arow;\x03⤍",
- // blacktriangleright;[▸] blacktriangledown;[▾] blacktriangleleft;[◂] blacktriangle;[▴] blacklozenge;[⧫] blacksquare;[▪] blank;[␣] blk12;[▒] blk14;[░] blk34;[▓] block;[█].
- "\x11acktriangleright;\x03▸\x10acktriangledown;\x03▾\x10acktriangleleft;\x03◂\x0cacktriangle;\x03▴\x0backlozenge;\x03⧫\x0aacksquare;\x03▪\x04ank;\x03␣\x04k12;\x03▒\x04k14;\x03░\x04k34;\x03▓\x04ock;\x03█",
- // bnequiv;[≡⃥] bnot;[⌐] bne;[=⃥].
- "\x06equiv;\x06≡⃥\x03ot;\x03⌐\x02e;\x04=⃥",
- // boxminus;[⊟] boxtimes;[⊠] boxplus;[⊞] bottom;[⊥] bowtie;[⋈] boxbox;[⧉] boxDL;[╗] boxDR;[╔] boxDl;[╖] boxDr;[╓] boxHD;[╦] boxHU;[╩] boxHd;[╤] boxHu;[╧] boxUL;[╝] boxUR;[╚] boxUl;[╜] boxUr;[╙] boxVH;[╬] boxVL;[╣] boxVR;[╠] boxVh;[╫] boxVl;[╢] boxVr;[╟] boxdL;[╕] boxdR;[╒] boxdl;[┐] boxdr;[┌] boxhD;[╥] boxhU;[╨] boxhd;[┬] boxhu;[┴] boxuL;[╛] boxuR;[╘] boxul;[┘] boxur;[└] boxvH;[╪] boxvL;[╡] boxvR;[╞] boxvh;[┼] boxvl;[┤] boxvr;[├] bopf;[𝕓] boxH;[═] boxV;[║] boxh;[─] boxv;[│] bot;[⊥].
- "\x07xminus;\x03⊟\x07xtimes;\x03⊠\x06xplus;\x03⊞\x05ttom;\x03⊥\x05wtie;\x03⋈\x05xbox;\x03⧉\x04xDL;\x03╗\x04xDR;\x03╔\x04xDl;\x03╖\x04xDr;\x03╓\x04xHD;\x03╦\x04xHU;\x03╩\x04xHd;\x03╤\x04xHu;\x03╧\x04xUL;\x03╝\x04xUR;\x03╚\x04xUl;\x03╜\x04xUr;\x03╙\x04xVH;\x03╬\x04xVL;\x03╣\x04xVR;\x03╠\x04xVh;\x03╫\x04xVl;\x03╢\x04xVr;\x03╟\x04xdL;\x03╕\x04xdR;\x03╒\x04xdl;\x03┐\x04xdr;\x03┌\x04xhD;\x03╥\x04xhU;\x03╨\x04xhd;\x03┬\x04xhu;\x03┴\x04xuL;\x03╛\x04xuR;\x03╘\x04xul;\x03┘\x04xur;\x03└\x04xvH;\x03╪\x04xvL;\x03╡\x04xvR;\x03╞\x04xvh;\x03┼\x04xvl;\x03┤\x04xvr;\x03├\x03pf;\x04𝕓\x03xH;\x03═\x03xV;\x03║\x03xh;\x03─\x03xv;\x03│\x02t;\x03⊥",
- // bprime;[‵].
- "\x05rime;\x03‵",
- // brvbar;[¦] breve;[˘] brvbar[¦].
- "\x05vbar;\x02¦\x04eve;\x02˘\x04vbar\x02¦",
- // bsolhsub;[⟈] bsemi;[⁏] bsime;[⋍] bsolb;[⧅] bscr;[𝒷] bsim;[∽] bsol;[\\].
- "\x07olhsub;\x03⟈\x04emi;\x03⁏\x04ime;\x03⋍\x04olb;\x03⧅\x03cr;\x04𝒷\x03im;\x03∽\x03ol;\x01\\",
- // bullet;[•] bumpeq;[≏] bumpE;[⪮] bumpe;[≏] bull;[•] bump;[≎].
- "\x05llet;\x03•\x05mpeq;\x03≏\x04mpE;\x03⪮\x04mpe;\x03≏\x03ll;\x03•\x03mp;\x03≎",
- // capbrcup;[⩉] cacute;[ć] capand;[⩄] capcap;[⩋] capcup;[⩇] capdot;[⩀] caret;[⁁] caron;[ˇ] caps;[∩︀] cap;[∩].
- "\x07pbrcup;\x03⩉\x05cute;\x02ć\x05pand;\x03⩄\x05pcap;\x03⩋\x05pcup;\x03⩇\x05pdot;\x03⩀\x04ret;\x03⁁\x04ron;\x02ˇ\x03ps;\x06∩︀\x02p;\x03∩",
- // ccupssm;[⩐] ccaron;[č] ccedil;[ç] ccaps;[⩍] ccedil[ç] ccirc;[ĉ] ccups;[⩌].
- "\x06upssm;\x03⩐\x05aron;\x02č\x05edil;\x02ç\x04aps;\x03⩍\x04edil\x02ç\x04irc;\x02ĉ\x04ups;\x03⩌",
- // cdot;[ċ].
- "\x03ot;\x02ċ",
- // centerdot;[·] cemptyv;[⦲] cedil;[¸] cedil[¸] cent;[¢] cent[¢].
- "\x08nterdot;\x02·\x06mptyv;\x03⦲\x04dil;\x02¸\x03dil\x02¸\x03nt;\x02¢\x02nt\x02¢",
- // cfr;[𝔠].
- "\x02r;\x04𝔠",
- // checkmark;[✓] check;[✓] chcy;[ч] chi;[χ].
- "\x08eckmark;\x03✓\x04eck;\x03✓\x03cy;\x02ч\x02i;\x02χ",
- // circlearrowright;[↻] circlearrowleft;[↺] circledcirc;[⊚] circleddash;[⊝] circledast;[⊛] circledR;[®] circledS;[Ⓢ] cirfnint;[⨐] cirscir;[⧂] circeq;[≗] cirmid;[⫯] cirE;[⧃] circ;[ˆ] cire;[≗] cir;[○].
- "\x0frclearrowright;\x03↻\x0erclearrowleft;\x03↺\x0arcledcirc;\x03⊚\x0arcleddash;\x03⊝\x09rcledast;\x03⊛\x07rcledR;\x02®\x07rcledS;\x03Ⓢ\x07rfnint;\x03⨐\x06rscir;\x03⧂\x05rceq;\x03≗\x05rmid;\x03⫯\x03rE;\x03⧃\x03rc;\x02ˆ\x03re;\x03≗\x02r;\x03○",
- // clubsuit;[♣] clubs;[♣].
- "\x07ubsuit;\x03♣\x04ubs;\x03♣",
- // complement;[∁] complexes;[ℂ] coloneq;[≔] congdot;[⩭] colone;[≔] commat;[@] compfn;[∘] conint;[∮] coprod;[∐] copysr;[℗] colon;[:] comma;[,] comp;[∁] cong;[≅] copf;[𝕔] copy;[©] copy[©].
- "\x09mplement;\x03∁\x08mplexes;\x03ℂ\x06loneq;\x03≔\x06ngdot;\x03⩭\x05lone;\x03≔\x05mmat;\x01@\x05mpfn;\x03∘\x05nint;\x03∮\x05prod;\x03∐\x05pysr;\x03℗\x04lon;\x01:\x04mma;\x01,\x03mp;\x03∁\x03ng;\x03≅\x03pf;\x04𝕔\x03py;\x02©\x02py\x02©",
- // crarr;[↵] cross;[✗].
- "\x04arr;\x03↵\x04oss;\x03✗",
- // csube;[⫑] csupe;[⫒] cscr;[𝒸] csub;[⫏] csup;[⫐].
- "\x04ube;\x03⫑\x04upe;\x03⫒\x03cr;\x04𝒸\x03ub;\x03⫏\x03up;\x03⫐",
- // ctdot;[⋯].
- "\x04dot;\x03⋯",
- // curvearrowright;[↷] curvearrowleft;[↶] curlyeqprec;[⋞] curlyeqsucc;[⋟] curlywedge;[⋏] cupbrcap;[⩈] curlyvee;[⋎] cudarrl;[⤸] cudarrr;[⤵] cularrp;[⤽] curarrm;[⤼] cularr;[↶] cupcap;[⩆] cupcup;[⩊] cupdot;[⊍] curarr;[↷] curren;[¤] cuepr;[⋞] cuesc;[⋟] cupor;[⩅] curren[¤] cuvee;[⋎] cuwed;[⋏] cups;[∪︀] cup;[∪].
- "\x0ervearrowright;\x03↷\x0drvearrowleft;\x03↶\x0arlyeqprec;\x03⋞\x0arlyeqsucc;\x03⋟\x09rlywedge;\x03⋏\x07pbrcap;\x03⩈\x07rlyvee;\x03⋎\x06darrl;\x03⤸\x06darrr;\x03⤵\x06larrp;\x03⤽\x06rarrm;\x03⤼\x05larr;\x03↶\x05pcap;\x03⩆\x05pcup;\x03⩊\x05pdot;\x03⊍\x05rarr;\x03↷\x05rren;\x02¤\x04epr;\x03⋞\x04esc;\x03⋟\x04por;\x03⩅\x04rren\x02¤\x04vee;\x03⋎\x04wed;\x03⋏\x03ps;\x06∪︀\x02p;\x03∪",
- // cwconint;[∲] cwint;[∱].
- "\x07conint;\x03∲\x04int;\x03∱",
- // cylcty;[⌭].
- "\x05lcty;\x03⌭",
- // dArr;[⇓].
- "\x03rr;\x03⇓",
- // dHar;[⥥].
- "\x03ar;\x03⥥",
- // dagger;[†] daleth;[ℸ] dashv;[⊣] darr;[↓] dash;[‐].
- "\x05gger;\x03†\x05leth;\x03ℸ\x04shv;\x03⊣\x03rr;\x03↓\x03sh;\x03‐",
- // dbkarow;[⤏] dblac;[˝].
- "\x06karow;\x03⤏\x04lac;\x02˝",
- // dcaron;[ď] dcy;[д].
- "\x05aron;\x02ď\x02y;\x02д",
- // ddagger;[‡] ddotseq;[⩷] ddarr;[⇊] dd;[ⅆ].
- "\x06agger;\x03‡\x06otseq;\x03⩷\x04arr;\x03⇊\x01;\x03ⅆ",
- // demptyv;[⦱] delta;[δ] deg;[°] deg[°].
- "\x06mptyv;\x03⦱\x04lta;\x02δ\x02g;\x02°\x01g\x02°",
- // dfisht;[⥿] dfr;[𝔡].
- "\x05isht;\x03⥿\x02r;\x04𝔡",
- // dharl;[⇃] dharr;[⇂].
- "\x04arl;\x03⇃\x04arr;\x03⇂",
- // divideontimes;[⋇] diamondsuit;[♦] diamond;[⋄] digamma;[ϝ] divide;[÷] divonx;[⋇] diams;[♦] disin;[⋲] divide[÷] diam;[⋄] die;[¨] div;[÷].
- "\x0cvideontimes;\x03⋇\x0aamondsuit;\x03♦\x06amond;\x03⋄\x06gamma;\x02ϝ\x05vide;\x02÷\x05vonx;\x03⋇\x04ams;\x03♦\x04sin;\x03⋲\x04vide\x02÷\x03am;\x03⋄\x02e;\x02¨\x02v;\x02÷",
- // djcy;[ђ].
- "\x03cy;\x02ђ",
- // dlcorn;[⌞] dlcrop;[⌍].
- "\x05corn;\x03⌞\x05crop;\x03⌍",
- // downharpoonright;[⇂] downharpoonleft;[⇃] doublebarwedge;[⌆] downdownarrows;[⇊] dotsquare;[⊡] downarrow;[↓] doteqdot;[≑] dotminus;[∸] dotplus;[∔] dollar;[$] doteq;[≐] dopf;[𝕕] dot;[˙].
- "\x0fwnharpoonright;\x03⇂\x0ewnharpoonleft;\x03⇃\x0dublebarwedge;\x03⌆\x0dwndownarrows;\x03⇊\x08tsquare;\x03⊡\x08wnarrow;\x03↓\x07teqdot;\x03≑\x07tminus;\x03∸\x06tplus;\x03∔\x05llar;\x01$\x04teq;\x03≐\x03pf;\x04𝕕\x02t;\x02˙",
- // drbkarow;[⤐] drcorn;[⌟] drcrop;[⌌].
- "\x07bkarow;\x03⤐\x05corn;\x03⌟\x05crop;\x03⌌",
- // dstrok;[đ] dscr;[𝒹] dscy;[ѕ] dsol;[⧶].
- "\x05trok;\x02đ\x03cr;\x04𝒹\x03cy;\x02ѕ\x03ol;\x03⧶",
- // dtdot;[⋱] dtrif;[▾] dtri;[▿].
- "\x04dot;\x03⋱\x04rif;\x03▾\x03ri;\x03▿",
- // duarr;[⇵] duhar;[⥯].
- "\x04arr;\x03⇵\x04har;\x03⥯",
- // dwangle;[⦦].
- "\x06angle;\x03⦦",
- // dzigrarr;[⟿] dzcy;[џ].
- "\x07igrarr;\x03⟿\x03cy;\x02џ",
- // eDDot;[⩷] eDot;[≑].
- "\x04Dot;\x03⩷\x03ot;\x03≑",
- // eacute;[é] easter;[⩮] eacute[é].
- "\x05cute;\x02é\x05ster;\x03⩮\x04cute\x02é",
- // ecaron;[ě] ecolon;[≕] ecirc;[ê] ecir;[≖] ecirc[ê] ecy;[э].
- "\x05aron;\x02ě\x05olon;\x03≕\x04irc;\x02ê\x03ir;\x03≖\x03irc\x02ê\x02y;\x02э",
- // edot;[ė].
- "\x03ot;\x02ė",
- // ee;[ⅇ].
- "\x01;\x03ⅇ",
- // efDot;[≒] efr;[𝔢].
- "\x04Dot;\x03≒\x02r;\x04𝔢",
- // egrave;[è] egsdot;[⪘] egrave[è] egs;[⪖] eg;[⪚].
- "\x05rave;\x02è\x05sdot;\x03⪘\x04rave\x02è\x02s;\x03⪖\x01;\x03⪚",
- // elinters;[⏧] elsdot;[⪗] ell;[ℓ] els;[⪕] el;[⪙].
- "\x07inters;\x03⏧\x05sdot;\x03⪗\x02l;\x03ℓ\x02s;\x03⪕\x01;\x03⪙",
- // emptyset;[∅] emptyv;[∅] emsp13;[ ] emsp14;[ ] emacr;[ē] empty;[∅] emsp;[ ].
- "\x07ptyset;\x03∅\x05ptyv;\x03∅\x05sp13;\x03 \x05sp14;\x03 \x04acr;\x02ē\x04pty;\x03∅\x03sp;\x03 ",
- // ensp;[ ] eng;[ŋ].
- "\x03sp;\x03 \x02g;\x02ŋ",
- // eogon;[ę] eopf;[𝕖].
- "\x04gon;\x02ę\x03pf;\x04𝕖",
- // epsilon;[ε] eparsl;[⧣] eplus;[⩱] epsiv;[ϵ] epar;[⋕] epsi;[ε].
- "\x06silon;\x02ε\x05arsl;\x03⧣\x04lus;\x03⩱\x04siv;\x02ϵ\x03ar;\x03⋕\x03si;\x02ε",
- // eqslantless;[⪕] eqslantgtr;[⪖] eqvparsl;[⧥] eqcolon;[≕] equivDD;[⩸] eqcirc;[≖] equals;[=] equest;[≟] eqsim;[≂] equiv;[≡].
- "\x0aslantless;\x03⪕\x09slantgtr;\x03⪖\x07vparsl;\x03⧥\x06colon;\x03≕\x06uivDD;\x03⩸\x05circ;\x03≖\x05uals;\x01=\x05uest;\x03≟\x04sim;\x03≂\x04uiv;\x03≡",
- // erDot;[≓] erarr;[⥱].
- "\x04Dot;\x03≓\x04arr;\x03⥱",
- // esdot;[≐] escr;[ℯ] esim;[≂].
- "\x04dot;\x03≐\x03cr;\x03ℯ\x03im;\x03≂",
- // eta;[η] eth;[ð] eth[ð].
- "\x02a;\x02η\x02h;\x02ð\x01h\x02ð",
- // euml;[ë] euro;[€] euml[ë].
- "\x03ml;\x02ë\x03ro;\x03€\x02ml\x02ë",
- // exponentiale;[ⅇ] expectation;[ℰ] exist;[∃] excl;[!].
- "\x0bponentiale;\x03ⅇ\x0apectation;\x03ℰ\x04ist;\x03∃\x03cl;\x01!",
- // fallingdotseq;[≒].
- "\x0cllingdotseq;\x03≒",
- // fcy;[ф].
- "\x02y;\x02ф",
- // female;[♀].
- "\x05male;\x03♀",
- // ffilig;[ffi] ffllig;[ffl] fflig;[ff] ffr;[𝔣].
- "\x05ilig;\x03ffi\x05llig;\x03ffl\x04lig;\x03ff\x02r;\x04𝔣",
- // filig;[fi].
- "\x04lig;\x03fi",
- // fjlig;[fj].
- "\x04lig;\x02fj",
- // fllig;[fl] fltns;[▱] flat;[♭].
- "\x04lig;\x03fl\x04tns;\x03▱\x03at;\x03♭",
- // fnof;[ƒ].
- "\x03of;\x02ƒ",
- // forall;[∀] forkv;[⫙] fopf;[𝕗] fork;[⋔].
- "\x05rall;\x03∀\x04rkv;\x03⫙\x03pf;\x04𝕗\x03rk;\x03⋔",
- // fpartint;[⨍].
- "\x07artint;\x03⨍",
- // frac12;[½] frac13;[⅓] frac14;[¼] frac15;[⅕] frac16;[⅙] frac18;[⅛] frac23;[⅔] frac25;[⅖] frac34;[¾] frac35;[⅗] frac38;[⅜] frac45;[⅘] frac56;[⅚] frac58;[⅝] frac78;[⅞] frac12[½] frac14[¼] frac34[¾] frasl;[⁄] frown;[⌢].
- "\x05ac12;\x02½\x05ac13;\x03⅓\x05ac14;\x02¼\x05ac15;\x03⅕\x05ac16;\x03⅙\x05ac18;\x03⅛\x05ac23;\x03⅔\x05ac25;\x03⅖\x05ac34;\x02¾\x05ac35;\x03⅗\x05ac38;\x03⅜\x05ac45;\x03⅘\x05ac56;\x03⅚\x05ac58;\x03⅝\x05ac78;\x03⅞\x04ac12\x02½\x04ac14\x02¼\x04ac34\x02¾\x04asl;\x03⁄\x04own;\x03⌢",
- // fscr;[𝒻].
- "\x03cr;\x04𝒻",
- // gEl;[⪌] gE;[≧].
- "\x02l;\x03⪌\x01;\x03≧",
- // gacute;[ǵ] gammad;[ϝ] gamma;[γ] gap;[⪆].
- "\x05cute;\x02ǵ\x05mmad;\x02ϝ\x04mma;\x02γ\x02p;\x03⪆",
- // gbreve;[ğ].
- "\x05reve;\x02ğ",
- // gcirc;[ĝ] gcy;[г].
- "\x04irc;\x02ĝ\x02y;\x02г",
- // gdot;[ġ].
- "\x03ot;\x02ġ",
- // geqslant;[⩾] gesdotol;[⪄] gesdoto;[⪂] gesdot;[⪀] gesles;[⪔] gescc;[⪩] geqq;[≧] gesl;[⋛︀] gel;[⋛] geq;[≥] ges;[⩾] ge;[≥].
- "\x07qslant;\x03⩾\x07sdotol;\x03⪄\x06sdoto;\x03⪂\x05sdot;\x03⪀\x05sles;\x03⪔\x04scc;\x03⪩\x03qq;\x03≧\x03sl;\x06⋛︀\x02l;\x03⋛\x02q;\x03≥\x02s;\x03⩾\x01;\x03≥",
- // gfr;[𝔤].
- "\x02r;\x04𝔤",
- // ggg;[⋙] gg;[≫].
- "\x02g;\x03⋙\x01;\x03≫",
- // gimel;[ℷ].
- "\x04mel;\x03ℷ",
- // gjcy;[ѓ].
- "\x03cy;\x02ѓ",
- // glE;[⪒] gla;[⪥] glj;[⪤] gl;[≷].
- "\x02E;\x03⪒\x02a;\x03⪥\x02j;\x03⪤\x01;\x03≷",
- // gnapprox;[⪊] gneqq;[≩] gnsim;[⋧] gnap;[⪊] gneq;[⪈] gnE;[≩] gne;[⪈].
- "\x07approx;\x03⪊\x04eqq;\x03≩\x04sim;\x03⋧\x03ap;\x03⪊\x03eq;\x03⪈\x02E;\x03≩\x02e;\x03⪈",
- // gopf;[𝕘].
- "\x03pf;\x04𝕘",
- // grave;[`].
- "\x04ave;\x01`",
- // gsime;[⪎] gsiml;[⪐] gscr;[ℊ] gsim;[≳].
- "\x04ime;\x03⪎\x04iml;\x03⪐\x03cr;\x03ℊ\x03im;\x03≳",
- // gtreqqless;[⪌] gtrapprox;[⪆] gtreqless;[⋛] gtquest;[⩼] gtrless;[≷] gtlPar;[⦕] gtrarr;[⥸] gtrdot;[⋗] gtrsim;[≳] gtcir;[⩺] gtdot;[⋗] gtcc;[⪧] gt;[>].
- "\x09reqqless;\x03⪌\x08rapprox;\x03⪆\x08reqless;\x03⋛\x06quest;\x03⩼\x06rless;\x03≷\x05lPar;\x03⦕\x05rarr;\x03⥸\x05rdot;\x03⋗\x05rsim;\x03≳\x04cir;\x03⩺\x04dot;\x03⋗\x03cc;\x03⪧\x01;\x01>",
- // gvertneqq;[≩︀] gvnE;[≩︀].
- "\x08ertneqq;\x06≩︀\x03nE;\x06≩︀",
- // hArr;[⇔].
- "\x03rr;\x03⇔",
- // harrcir;[⥈] hairsp;[ ] hamilt;[ℋ] hardcy;[ъ] harrw;[↭] half;[½] harr;[↔].
- "\x06rrcir;\x03⥈\x05irsp;\x03 \x05milt;\x03ℋ\x05rdcy;\x02ъ\x04rrw;\x03↭\x03lf;\x02½\x03rr;\x03↔",
- // hbar;[ℏ].
- "\x03ar;\x03ℏ",
- // hcirc;[ĥ].
- "\x04irc;\x02ĥ",
- // heartsuit;[♥] hearts;[♥] hellip;[…] hercon;[⊹].
- "\x08artsuit;\x03♥\x05arts;\x03♥\x05llip;\x03…\x05rcon;\x03⊹",
- // hfr;[𝔥].
- "\x02r;\x04𝔥",
- // hksearow;[⤥] hkswarow;[⤦].
- "\x07searow;\x03⤥\x07swarow;\x03⤦",
- // hookrightarrow;[↪] hookleftarrow;[↩] homtht;[∻] horbar;[―] hoarr;[⇿] hopf;[𝕙].
- "\x0dokrightarrow;\x03↪\x0cokleftarrow;\x03↩\x05mtht;\x03∻\x05rbar;\x03―\x04arr;\x03⇿\x03pf;\x04𝕙",
- // hslash;[ℏ] hstrok;[ħ] hscr;[𝒽].
- "\x05lash;\x03ℏ\x05trok;\x02ħ\x03cr;\x04𝒽",
- // hybull;[⁃] hyphen;[‐].
- "\x05bull;\x03⁃\x05phen;\x03‐",
- // iacute;[í] iacute[í].
- "\x05cute;\x02í\x04cute\x02í",
- // icirc;[î] icirc[î] icy;[и] ic;[].
- "\x04irc;\x02î\x03irc\x02î\x02y;\x02и\x01;\x03",
- // iexcl;[¡] iecy;[е] iexcl[¡].
- "\x04xcl;\x02¡\x03cy;\x02е\x03xcl\x02¡",
- // iff;[⇔] ifr;[𝔦].
- "\x02f;\x03⇔\x02r;\x04𝔦",
- // igrave;[ì] igrave[ì].
- "\x05rave;\x02ì\x04rave\x02ì",
- // iiiint;[⨌] iinfin;[⧜] iiint;[∭] iiota;[℩] ii;[ⅈ].
- "\x05iint;\x03⨌\x05nfin;\x03⧜\x04int;\x03∭\x04ota;\x03℩\x01;\x03ⅈ",
- // ijlig;[ij].
- "\x04lig;\x02ij",
- // imagline;[ℐ] imagpart;[ℑ] imacr;[ī] image;[ℑ] imath;[ı] imped;[Ƶ] imof;[⊷].
- "\x07agline;\x03ℐ\x07agpart;\x03ℑ\x04acr;\x02ī\x04age;\x03ℑ\x04ath;\x02ı\x04ped;\x02Ƶ\x03of;\x03⊷",
- // infintie;[⧝] integers;[ℤ] intercal;[⊺] intlarhk;[⨗] intprod;[⨼] incare;[℅] inodot;[ı] intcal;[⊺] infin;[∞] int;[∫] in;[∈].
- "\x07fintie;\x03⧝\x07tegers;\x03ℤ\x07tercal;\x03⊺\x07tlarhk;\x03⨗\x06tprod;\x03⨼\x05care;\x03℅\x05odot;\x02ı\x05tcal;\x03⊺\x04fin;\x03∞\x02t;\x03∫\x01;\x03∈",
- // iogon;[į] iocy;[ё] iopf;[𝕚] iota;[ι].
- "\x04gon;\x02į\x03cy;\x02ё\x03pf;\x04𝕚\x03ta;\x02ι",
- // iprod;[⨼].
- "\x04rod;\x03⨼",
- // iquest;[¿] iquest[¿].
- "\x05uest;\x02¿\x04uest\x02¿",
- // isindot;[⋵] isinsv;[⋳] isinE;[⋹] isins;[⋴] isinv;[∈] iscr;[𝒾] isin;[∈].
- "\x06indot;\x03⋵\x05insv;\x03⋳\x04inE;\x03⋹\x04ins;\x03⋴\x04inv;\x03∈\x03cr;\x04𝒾\x03in;\x03∈",
- // itilde;[ĩ] it;[].
- "\x05ilde;\x02ĩ\x01;\x03",
- // iukcy;[і] iuml;[ï] iuml[ï].
- "\x04kcy;\x02і\x03ml;\x02ï\x02ml\x02ï",
- // jcirc;[ĵ] jcy;[й].
- "\x04irc;\x02ĵ\x02y;\x02й",
- // jfr;[𝔧].
- "\x02r;\x04𝔧",
- // jmath;[ȷ].
- "\x04ath;\x02ȷ",
- // jopf;[𝕛].
- "\x03pf;\x04𝕛",
- // jsercy;[ј] jscr;[𝒿].
- "\x05ercy;\x02ј\x03cr;\x04𝒿",
- // jukcy;[є].
- "\x04kcy;\x02є",
- // kappav;[ϰ] kappa;[κ].
- "\x05ppav;\x02ϰ\x04ppa;\x02κ",
- // kcedil;[ķ] kcy;[к].
- "\x05edil;\x02ķ\x02y;\x02к",
- // kfr;[𝔨].
- "\x02r;\x04𝔨",
- // kgreen;[ĸ].
- "\x05reen;\x02ĸ",
- // khcy;[х].
- "\x03cy;\x02х",
- // kjcy;[ќ].
- "\x03cy;\x02ќ",
- // kopf;[𝕜].
- "\x03pf;\x04𝕜",
- // kscr;[𝓀].
- "\x03cr;\x04𝓀",
- // lAtail;[⤛] lAarr;[⇚] lArr;[⇐].
- "\x05tail;\x03⤛\x04arr;\x03⇚\x03rr;\x03⇐",
- // lBarr;[⤎].
- "\x04arr;\x03⤎",
- // lEg;[⪋] lE;[≦].
- "\x02g;\x03⪋\x01;\x03≦",
- // lHar;[⥢].
- "\x03ar;\x03⥢",
- // laemptyv;[⦴] larrbfs;[⤟] larrsim;[⥳] lacute;[ĺ] lagran;[ℒ] lambda;[λ] langle;[⟨] larrfs;[⤝] larrhk;[↩] larrlp;[↫] larrpl;[⤹] larrtl;[↢] latail;[⤙] langd;[⦑] laquo;[«] larrb;[⇤] lates;[⪭︀] lang;[⟨] laquo[«] larr;[←] late;[⪭] lap;[⪅] lat;[⪫].
- "\x07emptyv;\x03⦴\x06rrbfs;\x03⤟\x06rrsim;\x03⥳\x05cute;\x02ĺ\x05gran;\x03ℒ\x05mbda;\x02λ\x05ngle;\x03⟨\x05rrfs;\x03⤝\x05rrhk;\x03↩\x05rrlp;\x03↫\x05rrpl;\x03⤹\x05rrtl;\x03↢\x05tail;\x03⤙\x04ngd;\x03⦑\x04quo;\x02«\x04rrb;\x03⇤\x04tes;\x06⪭︀\x03ng;\x03⟨\x03quo\x02«\x03rr;\x03←\x03te;\x03⪭\x02p;\x03⪅\x02t;\x03⪫",
- // lbrksld;[⦏] lbrkslu;[⦍] lbrace;[{] lbrack;[[] lbarr;[⤌] lbbrk;[❲] lbrke;[⦋].
- "\x06rksld;\x03⦏\x06rkslu;\x03⦍\x05race;\x01{\x05rack;\x01[\x04arr;\x03⤌\x04brk;\x03❲\x04rke;\x03⦋",
- // lcaron;[ľ] lcedil;[ļ] lceil;[⌈] lcub;[{] lcy;[л].
- "\x05aron;\x02ľ\x05edil;\x02ļ\x04eil;\x03⌈\x03ub;\x01{\x02y;\x02л",
- // ldrushar;[⥋] ldrdhar;[⥧] ldquor;[„] ldquo;[“] ldca;[⤶] ldsh;[↲].
- "\x07rushar;\x03⥋\x06rdhar;\x03⥧\x05quor;\x03„\x04quo;\x03“\x03ca;\x03⤶\x03sh;\x03↲",
- // leftrightsquigarrow;[↭] leftrightharpoons;[⇋] leftharpoondown;[↽] leftrightarrows;[⇆] leftleftarrows;[⇇] leftrightarrow;[↔] leftthreetimes;[⋋] leftarrowtail;[↢] leftharpoonup;[↼] lessapprox;[⪅] lesseqqgtr;[⪋] leftarrow;[←] lesseqgtr;[⋚] leqslant;[⩽] lesdotor;[⪃] lesdoto;[⪁] lessdot;[⋖] lessgtr;[≶] lesssim;[≲] lesdot;[⩿] lesges;[⪓] lescc;[⪨] leqq;[≦] lesg;[⋚︀] leg;[⋚] leq;[≤] les;[⩽] le;[≤].
- "\x12ftrightsquigarrow;\x03↭\x10ftrightharpoons;\x03⇋\x0eftharpoondown;\x03↽\x0eftrightarrows;\x03⇆\x0dftleftarrows;\x03⇇\x0dftrightarrow;\x03↔\x0dftthreetimes;\x03⋋\x0cftarrowtail;\x03↢\x0cftharpoonup;\x03↼\x09ssapprox;\x03⪅\x09sseqqgtr;\x03⪋\x08ftarrow;\x03←\x08sseqgtr;\x03⋚\x07qslant;\x03⩽\x07sdotor;\x03⪃\x06sdoto;\x03⪁\x06ssdot;\x03⋖\x06ssgtr;\x03≶\x06sssim;\x03≲\x05sdot;\x03⩿\x05sges;\x03⪓\x04scc;\x03⪨\x03qq;\x03≦\x03sg;\x06⋚︀\x02g;\x03⋚\x02q;\x03≤\x02s;\x03⩽\x01;\x03≤",
- // lfisht;[⥼] lfloor;[⌊] lfr;[𝔩].
- "\x05isht;\x03⥼\x05loor;\x03⌊\x02r;\x04𝔩",
- // lgE;[⪑] lg;[≶].
- "\x02E;\x03⪑\x01;\x03≶",
- // lharul;[⥪] lhard;[↽] lharu;[↼] lhblk;[▄].
- "\x05arul;\x03⥪\x04ard;\x03↽\x04aru;\x03↼\x04blk;\x03▄",
- // ljcy;[љ].
- "\x03cy;\x02љ",
- // llcorner;[⌞] llhard;[⥫] llarr;[⇇] lltri;[◺] ll;[≪].
- "\x07corner;\x03⌞\x05hard;\x03⥫\x04arr;\x03⇇\x04tri;\x03◺\x01;\x03≪",
- // lmoustache;[⎰] lmidot;[ŀ] lmoust;[⎰].
- "\x09oustache;\x03⎰\x05idot;\x02ŀ\x05oust;\x03⎰",
- // lnapprox;[⪉] lneqq;[≨] lnsim;[⋦] lnap;[⪉] lneq;[⪇] lnE;[≨] lne;[⪇].
- "\x07approx;\x03⪉\x04eqq;\x03≨\x04sim;\x03⋦\x03ap;\x03⪉\x03eq;\x03⪇\x02E;\x03≨\x02e;\x03⪇",
- // longleftrightarrow;[⟷] longrightarrow;[⟶] looparrowright;[↬] longleftarrow;[⟵] looparrowleft;[↫] longmapsto;[⟼] lotimes;[⨴] lozenge;[◊] loplus;[⨭] lowast;[∗] lowbar;[_] loang;[⟬] loarr;[⇽] lobrk;[⟦] lopar;[⦅] lopf;[𝕝] lozf;[⧫] loz;[◊].
- "\x11ngleftrightarrow;\x03⟷\x0dngrightarrow;\x03⟶\x0doparrowright;\x03↬\x0cngleftarrow;\x03⟵\x0coparrowleft;\x03↫\x09ngmapsto;\x03⟼\x06times;\x03⨴\x06zenge;\x03◊\x05plus;\x03⨭\x05wast;\x03∗\x05wbar;\x01_\x04ang;\x03⟬\x04arr;\x03⇽\x04brk;\x03⟦\x04par;\x03⦅\x03pf;\x04𝕝\x03zf;\x03⧫\x02z;\x03◊",
- // lparlt;[⦓] lpar;[(].
- "\x05arlt;\x03⦓\x03ar;\x01(",
- // lrcorner;[⌟] lrhard;[⥭] lrarr;[⇆] lrhar;[⇋] lrtri;[⊿] lrm;[].
- "\x07corner;\x03⌟\x05hard;\x03⥭\x04arr;\x03⇆\x04har;\x03⇋\x04tri;\x03⊿\x02m;\x03",
- // lsaquo;[‹] lsquor;[‚] lstrok;[ł] lsime;[⪍] lsimg;[⪏] lsquo;[‘] lscr;[𝓁] lsim;[≲] lsqb;[[] lsh;[↰].
- "\x05aquo;\x03‹\x05quor;\x03‚\x05trok;\x02ł\x04ime;\x03⪍\x04img;\x03⪏\x04quo;\x03‘\x03cr;\x04𝓁\x03im;\x03≲\x03qb;\x01[\x02h;\x03↰",
- // ltquest;[⩻] lthree;[⋋] ltimes;[⋉] ltlarr;[⥶] ltrPar;[⦖] ltcir;[⩹] ltdot;[⋖] ltrie;[⊴] ltrif;[◂] ltcc;[⪦] ltri;[◃] lt;[<].
- "\x06quest;\x03⩻\x05hree;\x03⋋\x05imes;\x03⋉\x05larr;\x03⥶\x05rPar;\x03⦖\x04cir;\x03⩹\x04dot;\x03⋖\x04rie;\x03⊴\x04rif;\x03◂\x03cc;\x03⪦\x03ri;\x03◃\x01;\x01<",
- // lurdshar;[⥊] luruhar;[⥦].
- "\x07rdshar;\x03⥊\x06ruhar;\x03⥦",
- // lvertneqq;[≨︀] lvnE;[≨︀].
- "\x08ertneqq;\x06≨︀\x03nE;\x06≨︀",
- // mDDot;[∺].
- "\x04Dot;\x03∺",
- // mapstodown;[↧] mapstoleft;[↤] mapstoup;[↥] maltese;[✠] mapsto;[↦] marker;[▮] macr;[¯] male;[♂] malt;[✠] macr[¯] map;[↦].
- "\x09pstodown;\x03↧\x09pstoleft;\x03↤\x07pstoup;\x03↥\x06ltese;\x03✠\x05psto;\x03↦\x05rker;\x03▮\x03cr;\x02¯\x03le;\x03♂\x03lt;\x03✠\x02cr\x02¯\x02p;\x03↦",
- // mcomma;[⨩] mcy;[м].
- "\x05omma;\x03⨩\x02y;\x02м",
- // mdash;[—].
- "\x04ash;\x03—",
- // measuredangle;[∡].
- "\x0casuredangle;\x03∡",
- // mfr;[𝔪].
- "\x02r;\x04𝔪",
- // mho;[℧].
- "\x02o;\x03℧",
- // minusdu;[⨪] midast;[*] midcir;[⫰] middot;[·] minusb;[⊟] minusd;[∸] micro;[µ] middot[·] minus;[−] micro[µ] mid;[∣].
- "\x06nusdu;\x03⨪\x05dast;\x01*\x05dcir;\x03⫰\x05ddot;\x02·\x05nusb;\x03⊟\x05nusd;\x03∸\x04cro;\x02µ\x04ddot\x02·\x04nus;\x03−\x03cro\x02µ\x02d;\x03∣",
- // mlcp;[⫛] mldr;[…].
- "\x03cp;\x03⫛\x03dr;\x03…",
- // mnplus;[∓].
- "\x05plus;\x03∓",
- // models;[⊧] mopf;[𝕞].
- "\x05dels;\x03⊧\x03pf;\x04𝕞",
- // mp;[∓].
- "\x01;\x03∓",
- // mstpos;[∾] mscr;[𝓂].
- "\x05tpos;\x03∾\x03cr;\x04𝓂",
- // multimap;[⊸] mumap;[⊸] mu;[μ].
- "\x07ltimap;\x03⊸\x04map;\x03⊸\x01;\x02μ",
- // nGtv;[≫̸] nGg;[⋙̸] nGt;[≫⃒].
- "\x03tv;\x05≫̸\x02g;\x05⋙̸\x02t;\x06≫⃒",
- // nLeftrightarrow;[⇎] nLeftarrow;[⇍] nLtv;[≪̸] nLl;[⋘̸] nLt;[≪⃒].
- "\x0eeftrightarrow;\x03⇎\x09eftarrow;\x03⇍\x03tv;\x05≪̸\x02l;\x05⋘̸\x02t;\x06≪⃒",
- // nRightarrow;[⇏].
- "\x0aightarrow;\x03⇏",
- // nVDash;[⊯] nVdash;[⊮].
- "\x05Dash;\x03⊯\x05dash;\x03⊮",
- // naturals;[ℕ] napprox;[≉] natural;[♮] nacute;[ń] nabla;[∇] napid;[≋̸] napos;[ʼn] natur;[♮] nang;[∠⃒] napE;[⩰̸] nap;[≉].
- "\x07turals;\x03ℕ\x06pprox;\x03≉\x06tural;\x03♮\x05cute;\x02ń\x04bla;\x03∇\x04pid;\x05≋̸\x04pos;\x02ʼn\x04tur;\x03♮\x03ng;\x06∠⃒\x03pE;\x05⩰̸\x02p;\x03≉",
- // nbumpe;[≏̸] nbump;[≎̸] nbsp;[ ] nbsp[ ].
- "\x05umpe;\x05≏̸\x04ump;\x05≎̸\x03sp;\x02 \x02sp\x02 ",
- // ncongdot;[⩭̸] ncaron;[ň] ncedil;[ņ] ncong;[≇] ncap;[⩃] ncup;[⩂] ncy;[н].
- "\x07ongdot;\x05⩭̸\x05aron;\x02ň\x05edil;\x02ņ\x04ong;\x03≇\x03ap;\x03⩃\x03up;\x03⩂\x02y;\x02н",
- // ndash;[–].
- "\x04ash;\x03–",
- // nearrow;[↗] nexists;[∄] nearhk;[⤤] nequiv;[≢] nesear;[⤨] nexist;[∄] neArr;[⇗] nearr;[↗] nedot;[≐̸] nesim;[≂̸] ne;[≠].
- "\x06arrow;\x03↗\x06xists;\x03∄\x05arhk;\x03⤤\x05quiv;\x03≢\x05sear;\x03⤨\x05xist;\x03∄\x04Arr;\x03⇗\x04arr;\x03↗\x04dot;\x05≐̸\x04sim;\x05≂̸\x01;\x03≠",
- // nfr;[𝔫].
- "\x02r;\x04𝔫",
- // ngeqslant;[⩾̸] ngeqq;[≧̸] ngsim;[≵] ngeq;[≱] nges;[⩾̸] ngtr;[≯] ngE;[≧̸] nge;[≱] ngt;[≯].
- "\x08eqslant;\x05⩾̸\x04eqq;\x05≧̸\x04sim;\x03≵\x03eq;\x03≱\x03es;\x05⩾̸\x03tr;\x03≯\x02E;\x05≧̸\x02e;\x03≱\x02t;\x03≯",
- // nhArr;[⇎] nharr;[↮] nhpar;[⫲].
- "\x04Arr;\x03⇎\x04arr;\x03↮\x04par;\x03⫲",
- // nisd;[⋺] nis;[⋼] niv;[∋] ni;[∋].
- "\x03sd;\x03⋺\x02s;\x03⋼\x02v;\x03∋\x01;\x03∋",
- // njcy;[њ].
- "\x03cy;\x02њ",
- // nleftrightarrow;[↮] nleftarrow;[↚] nleqslant;[⩽̸] nltrie;[⋬] nlArr;[⇍] nlarr;[↚] nleqq;[≦̸] nless;[≮] nlsim;[≴] nltri;[⋪] nldr;[‥] nleq;[≰] nles;[⩽̸] nlE;[≦̸] nle;[≰] nlt;[≮].
- "\x0eeftrightarrow;\x03↮\x09eftarrow;\x03↚\x08eqslant;\x05⩽̸\x05trie;\x03⋬\x04Arr;\x03⇍\x04arr;\x03↚\x04eqq;\x05≦̸\x04ess;\x03≮\x04sim;\x03≴\x04tri;\x03⋪\x03dr;\x03‥\x03eq;\x03≰\x03es;\x05⩽̸\x02E;\x05≦̸\x02e;\x03≰\x02t;\x03≮",
- // nmid;[∤].
- "\x03id;\x03∤",
- // notindot;[⋵̸] notinva;[∉] notinvb;[⋷] notinvc;[⋶] notniva;[∌] notnivb;[⋾] notnivc;[⋽] notinE;[⋹̸] notin;[∉] notni;[∌] nopf;[𝕟] not;[¬] not[¬].
- "\x07tindot;\x05⋵̸\x06tinva;\x03∉\x06tinvb;\x03⋷\x06tinvc;\x03⋶\x06tniva;\x03∌\x06tnivb;\x03⋾\x06tnivc;\x03⋽\x05tinE;\x05⋹̸\x04tin;\x03∉\x04tni;\x03∌\x03pf;\x04𝕟\x02t;\x02¬\x01t\x02¬",
- // nparallel;[∦] npolint;[⨔] npreceq;[⪯̸] nparsl;[⫽⃥] nprcue;[⋠] npart;[∂̸] nprec;[⊀] npar;[∦] npre;[⪯̸] npr;[⊀].
- "\x08arallel;\x03∦\x06olint;\x03⨔\x06receq;\x05⪯̸\x05arsl;\x06⫽⃥\x05rcue;\x03⋠\x04art;\x05∂̸\x04rec;\x03⊀\x03ar;\x03∦\x03re;\x05⪯̸\x02r;\x03⊀",
- // nrightarrow;[↛] nrarrc;[⤳̸] nrarrw;[↝̸] nrtrie;[⋭] nrArr;[⇏] nrarr;[↛] nrtri;[⋫].
- "\x0aightarrow;\x03↛\x05arrc;\x05⤳̸\x05arrw;\x05↝̸\x05trie;\x03⋭\x04Arr;\x03⇏\x04arr;\x03↛\x04tri;\x03⋫",
- // nshortparallel;[∦] nsubseteqq;[⫅̸] nsupseteqq;[⫆̸] nshortmid;[∤] nsubseteq;[⊈] nsupseteq;[⊉] nsqsube;[⋢] nsqsupe;[⋣] nsubset;[⊂⃒] nsucceq;[⪰̸] nsupset;[⊃⃒] nsccue;[⋡] nsimeq;[≄] nsime;[≄] nsmid;[∤] nspar;[∦] nsubE;[⫅̸] nsube;[⊈] nsucc;[⊁] nsupE;[⫆̸] nsupe;[⊉] nsce;[⪰̸] nscr;[𝓃] nsim;[≁] nsub;[⊄] nsup;[⊅] nsc;[⊁].
- "\x0dhortparallel;\x03∦\x09ubseteqq;\x05⫅̸\x09upseteqq;\x05⫆̸\x08hortmid;\x03∤\x08ubseteq;\x03⊈\x08upseteq;\x03⊉\x06qsube;\x03⋢\x06qsupe;\x03⋣\x06ubset;\x06⊂⃒\x06ucceq;\x05⪰̸\x06upset;\x06⊃⃒\x05ccue;\x03⋡\x05imeq;\x03≄\x04ime;\x03≄\x04mid;\x03∤\x04par;\x03∦\x04ubE;\x05⫅̸\x04ube;\x03⊈\x04ucc;\x03⊁\x04upE;\x05⫆̸\x04upe;\x03⊉\x03ce;\x05⪰̸\x03cr;\x04𝓃\x03im;\x03≁\x03ub;\x03⊄\x03up;\x03⊅\x02c;\x03⊁",
- // ntrianglerighteq;[⋭] ntrianglelefteq;[⋬] ntriangleright;[⋫] ntriangleleft;[⋪] ntilde;[ñ] ntilde[ñ] ntgl;[≹] ntlg;[≸].
- "\x0frianglerighteq;\x03⋭\x0erianglelefteq;\x03⋬\x0driangleright;\x03⋫\x0criangleleft;\x03⋪\x05ilde;\x02ñ\x04ilde\x02ñ\x03gl;\x03≹\x03lg;\x03≸",
- // numero;[№] numsp;[ ] num;[#] nu;[ν].
- "\x05mero;\x03№\x04msp;\x03 \x02m;\x01#\x01;\x02ν",
- // nvinfin;[⧞] nvltrie;[⊴⃒] nvrtrie;[⊵⃒] nvDash;[⊭] nvHarr;[⤄] nvdash;[⊬] nvlArr;[⤂] nvrArr;[⤃] nvsim;[∼⃒] nvap;[≍⃒] nvge;[≥⃒] nvgt;[>⃒] nvle;[≤⃒] nvlt;[<⃒].
- "\x06infin;\x03⧞\x06ltrie;\x06⊴⃒\x06rtrie;\x06⊵⃒\x05Dash;\x03⊭\x05Harr;\x03⤄\x05dash;\x03⊬\x05lArr;\x03⤂\x05rArr;\x03⤃\x04sim;\x06∼⃒\x03ap;\x06≍⃒\x03ge;\x06≥⃒\x03gt;\x04>⃒\x03le;\x06≤⃒\x03lt;\x04<⃒",
- // nwarrow;[↖] nwarhk;[⤣] nwnear;[⤧] nwArr;[⇖] nwarr;[↖].
- "\x06arrow;\x03↖\x05arhk;\x03⤣\x05near;\x03⤧\x04Arr;\x03⇖\x04arr;\x03↖",
- // oS;[Ⓢ].
- "\x01;\x03Ⓢ",
- // oacute;[ó] oacute[ó] oast;[⊛].
- "\x05cute;\x02ó\x04cute\x02ó\x03st;\x03⊛",
- // ocirc;[ô] ocir;[⊚] ocirc[ô] ocy;[о].
- "\x04irc;\x02ô\x03ir;\x03⊚\x03irc\x02ô\x02y;\x02о",
- // odblac;[ő] odsold;[⦼] odash;[⊝] odiv;[⨸] odot;[⊙].
- "\x05blac;\x02ő\x05sold;\x03⦼\x04ash;\x03⊝\x03iv;\x03⨸\x03ot;\x03⊙",
- // oelig;[œ].
- "\x04lig;\x02œ",
- // ofcir;[⦿] ofr;[𝔬].
- "\x04cir;\x03⦿\x02r;\x04𝔬",
- // ograve;[ò] ograve[ò] ogon;[˛] ogt;[⧁].
- "\x05rave;\x02ò\x04rave\x02ò\x03on;\x02˛\x02t;\x03⧁",
- // ohbar;[⦵] ohm;[Ω].
- "\x04bar;\x03⦵\x02m;\x02Ω",
- // oint;[∮].
- "\x03nt;\x03∮",
- // olcross;[⦻] olarr;[↺] olcir;[⦾] oline;[‾] olt;[⧀].
- "\x06cross;\x03⦻\x04arr;\x03↺\x04cir;\x03⦾\x04ine;\x03‾\x02t;\x03⧀",
- // omicron;[ο] ominus;[⊖] omacr;[ō] omega;[ω] omid;[⦶].
- "\x06icron;\x02ο\x05inus;\x03⊖\x04acr;\x02ō\x04ega;\x02ω\x03id;\x03⦶",
- // oopf;[𝕠].
- "\x03pf;\x04𝕠",
- // operp;[⦹] oplus;[⊕] opar;[⦷].
- "\x04erp;\x03⦹\x04lus;\x03⊕\x03ar;\x03⦷",
- // orderof;[ℴ] orslope;[⩗] origof;[⊶] orarr;[↻] order;[ℴ] ordf;[ª] ordm;[º] oror;[⩖] ord;[⩝] ordf[ª] ordm[º] orv;[⩛] or;[∨].
- "\x06derof;\x03ℴ\x06slope;\x03⩗\x05igof;\x03⊶\x04arr;\x03↻\x04der;\x03ℴ\x03df;\x02ª\x03dm;\x02º\x03or;\x03⩖\x02d;\x03⩝\x02df\x02ª\x02dm\x02º\x02v;\x03⩛\x01;\x03∨",
- // oslash;[ø] oslash[ø] oscr;[ℴ] osol;[⊘].
- "\x05lash;\x02ø\x04lash\x02ø\x03cr;\x03ℴ\x03ol;\x03⊘",
- // otimesas;[⨶] otilde;[õ] otimes;[⊗] otilde[õ].
- "\x07imesas;\x03⨶\x05ilde;\x02õ\x05imes;\x03⊗\x04ilde\x02õ",
- // ouml;[ö] ouml[ö].
- "\x03ml;\x02ö\x02ml\x02ö",
- // ovbar;[⌽].
- "\x04bar;\x03⌽",
- // parallel;[∥] parsim;[⫳] parsl;[⫽] para;[¶] part;[∂] par;[∥] para[¶].
- "\x07rallel;\x03∥\x05rsim;\x03⫳\x04rsl;\x03⫽\x03ra;\x02¶\x03rt;\x03∂\x02r;\x03∥\x02ra\x02¶",
- // pcy;[п].
- "\x02y;\x02п",
- // pertenk;[‱] percnt;[%] period;[.] permil;[‰] perp;[⊥].
- "\x06rtenk;\x03‱\x05rcnt;\x01%\x05riod;\x01.\x05rmil;\x03‰\x03rp;\x03⊥",
- // pfr;[𝔭].
- "\x02r;\x04𝔭",
- // phmmat;[ℳ] phone;[☎] phiv;[ϕ] phi;[φ].
- "\x05mmat;\x03ℳ\x04one;\x03☎\x03iv;\x02ϕ\x02i;\x02φ",
- // pitchfork;[⋔] piv;[ϖ] pi;[π].
- "\x08tchfork;\x03⋔\x02v;\x02ϖ\x01;\x02π",
- // plusacir;[⨣] planckh;[ℎ] pluscir;[⨢] plussim;[⨦] plustwo;[⨧] planck;[ℏ] plankv;[ℏ] plusdo;[∔] plusdu;[⨥] plusmn;[±] plusb;[⊞] pluse;[⩲] plusmn[±] plus;[+].
- "\x07usacir;\x03⨣\x06anckh;\x03ℎ\x06uscir;\x03⨢\x06ussim;\x03⨦\x06ustwo;\x03⨧\x05anck;\x03ℏ\x05ankv;\x03ℏ\x05usdo;\x03∔\x05usdu;\x03⨥\x05usmn;\x02±\x04usb;\x03⊞\x04use;\x03⩲\x04usmn\x02±\x03us;\x01+",
- // pm;[±].
- "\x01;\x02±",
- // pointint;[⨕] pound;[£] popf;[𝕡] pound[£].
- "\x07intint;\x03⨕\x04und;\x02£\x03pf;\x04𝕡\x03und\x02£",
- // preccurlyeq;[≼] precnapprox;[⪹] precapprox;[⪷] precneqq;[⪵] precnsim;[⋨] profalar;[⌮] profline;[⌒] profsurf;[⌓] precsim;[≾] preceq;[⪯] primes;[ℙ] prnsim;[⋨] propto;[∝] prurel;[⊰] prcue;[≼] prime;[′] prnap;[⪹] prsim;[≾] prap;[⪷] prec;[≺] prnE;[⪵] prod;[∏] prop;[∝] prE;[⪳] pre;[⪯] pr;[≺].
- "\x0aeccurlyeq;\x03≼\x0aecnapprox;\x03⪹\x09ecapprox;\x03⪷\x07ecneqq;\x03⪵\x07ecnsim;\x03⋨\x07ofalar;\x03⌮\x07ofline;\x03⌒\x07ofsurf;\x03⌓\x06ecsim;\x03≾\x05eceq;\x03⪯\x05imes;\x03ℙ\x05nsim;\x03⋨\x05opto;\x03∝\x05urel;\x03⊰\x04cue;\x03≼\x04ime;\x03′\x04nap;\x03⪹\x04sim;\x03≾\x03ap;\x03⪷\x03ec;\x03≺\x03nE;\x03⪵\x03od;\x03∏\x03op;\x03∝\x02E;\x03⪳\x02e;\x03⪯\x01;\x03≺",
- // pscr;[𝓅] psi;[ψ].
- "\x03cr;\x04𝓅\x02i;\x02ψ",
- // puncsp;[ ].
- "\x05ncsp;\x03 ",
- // qfr;[𝔮].
- "\x02r;\x04𝔮",
- // qint;[⨌].
- "\x03nt;\x03⨌",
- // qopf;[𝕢].
- "\x03pf;\x04𝕢",
- // qprime;[⁗].
- "\x05rime;\x03⁗",
- // qscr;[𝓆].
- "\x03cr;\x04𝓆",
- // quaternions;[ℍ] quatint;[⨖] questeq;[≟] quest;[?] quot;[\"] quot[\"].
- "\x0aaternions;\x03ℍ\x06atint;\x03⨖\x06esteq;\x03≟\x04est;\x01?\x03ot;\x01\"\x02ot\x01\"",
- // rAtail;[⤜] rAarr;[⇛] rArr;[⇒].
- "\x05tail;\x03⤜\x04arr;\x03⇛\x03rr;\x03⇒",
- // rBarr;[⤏].
- "\x04arr;\x03⤏",
- // rHar;[⥤].
- "\x03ar;\x03⥤",
- // rationals;[ℚ] raemptyv;[⦳] rarrbfs;[⤠] rarrsim;[⥴] racute;[ŕ] rangle;[⟩] rarrap;[⥵] rarrfs;[⤞] rarrhk;[↪] rarrlp;[↬] rarrpl;[⥅] rarrtl;[↣] ratail;[⤚] radic;[√] rangd;[⦒] range;[⦥] raquo;[»] rarrb;[⇥] rarrc;[⤳] rarrw;[↝] ratio;[∶] race;[∽̱] rang;[⟩] raquo[»] rarr;[→].
- "\x08tionals;\x03ℚ\x07emptyv;\x03⦳\x06rrbfs;\x03⤠\x06rrsim;\x03⥴\x05cute;\x02ŕ\x05ngle;\x03⟩\x05rrap;\x03⥵\x05rrfs;\x03⤞\x05rrhk;\x03↪\x05rrlp;\x03↬\x05rrpl;\x03⥅\x05rrtl;\x03↣\x05tail;\x03⤚\x04dic;\x03√\x04ngd;\x03⦒\x04nge;\x03⦥\x04quo;\x02»\x04rrb;\x03⇥\x04rrc;\x03⤳\x04rrw;\x03↝\x04tio;\x03∶\x03ce;\x05∽̱\x03ng;\x03⟩\x03quo\x02»\x03rr;\x03→",
- // rbrksld;[⦎] rbrkslu;[⦐] rbrace;[}] rbrack;[]] rbarr;[⤍] rbbrk;[❳] rbrke;[⦌].
- "\x06rksld;\x03⦎\x06rkslu;\x03⦐\x05race;\x01}\x05rack;\x01]\x04arr;\x03⤍\x04brk;\x03❳\x04rke;\x03⦌",
- // rcaron;[ř] rcedil;[ŗ] rceil;[⌉] rcub;[}] rcy;[р].
- "\x05aron;\x02ř\x05edil;\x02ŗ\x04eil;\x03⌉\x03ub;\x01}\x02y;\x02р",
- // rdldhar;[⥩] rdquor;[”] rdquo;[”] rdca;[⤷] rdsh;[↳].
- "\x06ldhar;\x03⥩\x05quor;\x03”\x04quo;\x03”\x03ca;\x03⤷\x03sh;\x03↳",
- // realpart;[ℜ] realine;[ℛ] reals;[ℝ] real;[ℜ] rect;[▭] reg;[®] reg[®].
- "\x07alpart;\x03ℜ\x06aline;\x03ℛ\x04als;\x03ℝ\x03al;\x03ℜ\x03ct;\x03▭\x02g;\x02®\x01g\x02®",
- // rfisht;[⥽] rfloor;[⌋] rfr;[𝔯].
- "\x05isht;\x03⥽\x05loor;\x03⌋\x02r;\x04𝔯",
- // rharul;[⥬] rhard;[⇁] rharu;[⇀] rhov;[ϱ] rho;[ρ].
- "\x05arul;\x03⥬\x04ard;\x03⇁\x04aru;\x03⇀\x03ov;\x02ϱ\x02o;\x02ρ",
- // rightleftharpoons;[⇌] rightharpoondown;[⇁] rightrightarrows;[⇉] rightleftarrows;[⇄] rightsquigarrow;[↝] rightthreetimes;[⋌] rightarrowtail;[↣] rightharpoonup;[⇀] risingdotseq;[≓] rightarrow;[→] ring;[˚].
- "\x10ghtleftharpoons;\x03⇌\x0fghtharpoondown;\x03⇁\x0fghtrightarrows;\x03⇉\x0eghtleftarrows;\x03⇄\x0eghtsquigarrow;\x03↝\x0eghtthreetimes;\x03⋌\x0dghtarrowtail;\x03↣\x0dghtharpoonup;\x03⇀\x0bsingdotseq;\x03≓\x09ghtarrow;\x03→\x03ng;\x02˚",
- // rlarr;[⇄] rlhar;[⇌] rlm;[].
- "\x04arr;\x03⇄\x04har;\x03⇌\x02m;\x03",
- // rmoustache;[⎱] rmoust;[⎱].
- "\x09oustache;\x03⎱\x05oust;\x03⎱",
- // rnmid;[⫮].
- "\x04mid;\x03⫮",
- // rotimes;[⨵] roplus;[⨮] roang;[⟭] roarr;[⇾] robrk;[⟧] ropar;[⦆] ropf;[𝕣].
- "\x06times;\x03⨵\x05plus;\x03⨮\x04ang;\x03⟭\x04arr;\x03⇾\x04brk;\x03⟧\x04par;\x03⦆\x03pf;\x04𝕣",
- // rppolint;[⨒] rpargt;[⦔] rpar;[)].
- "\x07polint;\x03⨒\x05argt;\x03⦔\x03ar;\x01)",
- // rrarr;[⇉].
- "\x04arr;\x03⇉",
- // rsaquo;[›] rsquor;[’] rsquo;[’] rscr;[𝓇] rsqb;[]] rsh;[↱].
- "\x05aquo;\x03›\x05quor;\x03’\x04quo;\x03’\x03cr;\x04𝓇\x03qb;\x01]\x02h;\x03↱",
- // rtriltri;[⧎] rthree;[⋌] rtimes;[⋊] rtrie;[⊵] rtrif;[▸] rtri;[▹].
- "\x07riltri;\x03⧎\x05hree;\x03⋌\x05imes;\x03⋊\x04rie;\x03⊵\x04rif;\x03▸\x03ri;\x03▹",
- // ruluhar;[⥨].
- "\x06luhar;\x03⥨",
- // rx;[℞].
- "\x01;\x03℞",
- // sacute;[ś].
- "\x05cute;\x02ś",
- // sbquo;[‚].
- "\x04quo;\x03‚",
- // scpolint;[⨓] scaron;[š] scedil;[ş] scnsim;[⋩] sccue;[≽] scirc;[ŝ] scnap;[⪺] scsim;[≿] scap;[⪸] scnE;[⪶] scE;[⪴] sce;[⪰] scy;[с] sc;[≻].
- "\x07polint;\x03⨓\x05aron;\x02š\x05edil;\x02ş\x05nsim;\x03⋩\x04cue;\x03≽\x04irc;\x02ŝ\x04nap;\x03⪺\x04sim;\x03≿\x03ap;\x03⪸\x03nE;\x03⪶\x02E;\x03⪴\x02e;\x03⪰\x02y;\x02с\x01;\x03≻",
- // sdotb;[⊡] sdote;[⩦] sdot;[⋅].
- "\x04otb;\x03⊡\x04ote;\x03⩦\x03ot;\x03⋅",
- // setminus;[∖] searrow;[↘] searhk;[⤥] seswar;[⤩] seArr;[⇘] searr;[↘] setmn;[∖] sect;[§] semi;[;] sext;[✶] sect[§].
- "\x07tminus;\x03∖\x06arrow;\x03↘\x05arhk;\x03⤥\x05swar;\x03⤩\x04Arr;\x03⇘\x04arr;\x03↘\x04tmn;\x03∖\x03ct;\x02§\x03mi;\x01;\x03xt;\x03✶\x02ct\x02§",
- // sfrown;[⌢] sfr;[𝔰].
- "\x05rown;\x03⌢\x02r;\x04𝔰",
- // shortparallel;[∥] shortmid;[∣] shchcy;[щ] sharp;[♯] shcy;[ш] shy;[] shy[].
- "\x0cortparallel;\x03∥\x07ortmid;\x03∣\x05chcy;\x02щ\x04arp;\x03♯\x03cy;\x02ш\x02y;\x02\x01y\x02",
- // simplus;[⨤] simrarr;[⥲] sigmaf;[ς] sigmav;[ς] simdot;[⩪] sigma;[σ] simeq;[≃] simgE;[⪠] simlE;[⪟] simne;[≆] sime;[≃] simg;[⪞] siml;[⪝] sim;[∼].
- "\x06mplus;\x03⨤\x06mrarr;\x03⥲\x05gmaf;\x02ς\x05gmav;\x02ς\x05mdot;\x03⩪\x04gma;\x02σ\x04meq;\x03≃\x04mgE;\x03⪠\x04mlE;\x03⪟\x04mne;\x03≆\x03me;\x03≃\x03mg;\x03⪞\x03ml;\x03⪝\x02m;\x03∼",
- // slarr;[←].
- "\x04arr;\x03←",
- // smallsetminus;[∖] smeparsl;[⧤] smashp;[⨳] smile;[⌣] smtes;[⪬︀] smid;[∣] smte;[⪬] smt;[⪪].
- "\x0callsetminus;\x03∖\x07eparsl;\x03⧤\x05ashp;\x03⨳\x04ile;\x03⌣\x04tes;\x06⪬︀\x03id;\x03∣\x03te;\x03⪬\x02t;\x03⪪",
- // softcy;[ь] solbar;[⌿] solb;[⧄] sopf;[𝕤] sol;[/].
- "\x05ftcy;\x02ь\x05lbar;\x03⌿\x03lb;\x03⧄\x03pf;\x04𝕤\x02l;\x01/",
- // spadesuit;[♠] spades;[♠] spar;[∥].
- "\x08adesuit;\x03♠\x05ades;\x03♠\x03ar;\x03∥",
- // sqsubseteq;[⊑] sqsupseteq;[⊒] sqsubset;[⊏] sqsupset;[⊐] sqcaps;[⊓︀] sqcups;[⊔︀] sqsube;[⊑] sqsupe;[⊒] square;[□] squarf;[▪] sqcap;[⊓] sqcup;[⊔] sqsub;[⊏] sqsup;[⊐] squf;[▪] squ;[□].
- "\x09subseteq;\x03⊑\x09supseteq;\x03⊒\x07subset;\x03⊏\x07supset;\x03⊐\x05caps;\x06⊓︀\x05cups;\x06⊔︀\x05sube;\x03⊑\x05supe;\x03⊒\x05uare;\x03□\x05uarf;\x03▪\x04cap;\x03⊓\x04cup;\x03⊔\x04sub;\x03⊏\x04sup;\x03⊐\x03uf;\x03▪\x02u;\x03□",
- // srarr;[→].
- "\x04arr;\x03→",
- // ssetmn;[∖] ssmile;[⌣] sstarf;[⋆] sscr;[𝓈].
- "\x05etmn;\x03∖\x05mile;\x03⌣\x05tarf;\x03⋆\x03cr;\x04𝓈",
- // straightepsilon;[ϵ] straightphi;[ϕ] starf;[★] strns;[¯] star;[☆].
- "\x0eraightepsilon;\x02ϵ\x0araightphi;\x02ϕ\x04arf;\x03★\x04rns;\x02¯\x03ar;\x03☆",
- // succcurlyeq;[≽] succnapprox;[⪺] subsetneqq;[⫋] succapprox;[⪸] supsetneqq;[⫌] subseteqq;[⫅] subsetneq;[⊊] supseteqq;[⫆] supsetneq;[⊋] subseteq;[⊆] succneqq;[⪶] succnsim;[⋩] supseteq;[⊇] subedot;[⫃] submult;[⫁] subplus;[⪿] subrarr;[⥹] succsim;[≿] supdsub;[⫘] supedot;[⫄] suphsol;[⟉] suphsub;[⫗] suplarr;[⥻] supmult;[⫂] supplus;[⫀] subdot;[⪽] subset;[⊂] subsim;[⫇] subsub;[⫕] subsup;[⫓] succeq;[⪰] supdot;[⪾] supset;[⊃] supsim;[⫈] supsub;[⫔] supsup;[⫖] subnE;[⫋] subne;[⊊] supnE;[⫌] supne;[⊋] subE;[⫅] sube;[⊆] succ;[≻] sung;[♪] sup1;[¹] sup2;[²] sup3;[³] supE;[⫆] supe;[⊇] sub;[⊂] sum;[∑] sup1[¹] sup2[²] sup3[³] sup;[⊃].
- "\x0acccurlyeq;\x03≽\x0accnapprox;\x03⪺\x09bsetneqq;\x03⫋\x09ccapprox;\x03⪸\x09psetneqq;\x03⫌\x08bseteqq;\x03⫅\x08bsetneq;\x03⊊\x08pseteqq;\x03⫆\x08psetneq;\x03⊋\x07bseteq;\x03⊆\x07ccneqq;\x03⪶\x07ccnsim;\x03⋩\x07pseteq;\x03⊇\x06bedot;\x03⫃\x06bmult;\x03⫁\x06bplus;\x03⪿\x06brarr;\x03⥹\x06ccsim;\x03≿\x06pdsub;\x03⫘\x06pedot;\x03⫄\x06phsol;\x03⟉\x06phsub;\x03⫗\x06plarr;\x03⥻\x06pmult;\x03⫂\x06pplus;\x03⫀\x05bdot;\x03⪽\x05bset;\x03⊂\x05bsim;\x03⫇\x05bsub;\x03⫕\x05bsup;\x03⫓\x05cceq;\x03⪰\x05pdot;\x03⪾\x05pset;\x03⊃\x05psim;\x03⫈\x05psub;\x03⫔\x05psup;\x03⫖\x04bnE;\x03⫋\x04bne;\x03⊊\x04pnE;\x03⫌\x04pne;\x03⊋\x03bE;\x03⫅\x03be;\x03⊆\x03cc;\x03≻\x03ng;\x03♪\x03p1;\x02¹\x03p2;\x02²\x03p3;\x02³\x03pE;\x03⫆\x03pe;\x03⊇\x02b;\x03⊂\x02m;\x03∑\x02p1\x02¹\x02p2\x02²\x02p3\x02³\x02p;\x03⊃",
- // swarrow;[↙] swarhk;[⤦] swnwar;[⤪] swArr;[⇙] swarr;[↙].
- "\x06arrow;\x03↙\x05arhk;\x03⤦\x05nwar;\x03⤪\x04Arr;\x03⇙\x04arr;\x03↙",
- // szlig;[ß] szlig[ß].
- "\x04lig;\x02ß\x03lig\x02ß",
- // target;[⌖] tau;[τ].
- "\x05rget;\x03⌖\x02u;\x02τ",
- // tbrk;[⎴].
- "\x03rk;\x03⎴",
- // tcaron;[ť] tcedil;[ţ] tcy;[т].
- "\x05aron;\x02ť\x05edil;\x02ţ\x02y;\x02т",
- // tdot;[⃛].
- "\x03ot;\x03⃛",
- // telrec;[⌕].
- "\x05lrec;\x03⌕",
- // tfr;[𝔱].
- "\x02r;\x04𝔱",
- // thickapprox;[≈] therefore;[∴] thetasym;[ϑ] thicksim;[∼] there4;[∴] thetav;[ϑ] thinsp;[ ] thksim;[∼] theta;[θ] thkap;[≈] thorn;[þ] thorn[þ].
- "\x0aickapprox;\x03≈\x08erefore;\x03∴\x07etasym;\x02ϑ\x07icksim;\x03∼\x05ere4;\x03∴\x05etav;\x02ϑ\x05insp;\x03 \x05ksim;\x03∼\x04eta;\x02θ\x04kap;\x03≈\x04orn;\x02þ\x03orn\x02þ",
- // timesbar;[⨱] timesb;[⊠] timesd;[⨰] tilde;[˜] times;[×] times[×] tint;[∭].
- "\x07mesbar;\x03⨱\x05mesb;\x03⊠\x05mesd;\x03⨰\x04lde;\x02˜\x04mes;\x02×\x03mes\x02×\x03nt;\x03∭",
- // topfork;[⫚] topbot;[⌶] topcir;[⫱] toea;[⤨] topf;[𝕥] tosa;[⤩] top;[⊤].
- "\x06pfork;\x03⫚\x05pbot;\x03⌶\x05pcir;\x03⫱\x03ea;\x03⤨\x03pf;\x04𝕥\x03sa;\x03⤩\x02p;\x03⊤",
- // tprime;[‴].
- "\x05rime;\x03‴",
- // trianglerighteq;[⊵] trianglelefteq;[⊴] triangleright;[▹] triangledown;[▿] triangleleft;[◃] triangleq;[≜] triangle;[▵] triminus;[⨺] trpezium;[⏢] triplus;[⨹] tritime;[⨻] tridot;[◬] trade;[™] trisb;[⧍] trie;[≜].
- "\x0eianglerighteq;\x03⊵\x0dianglelefteq;\x03⊴\x0ciangleright;\x03▹\x0biangledown;\x03▿\x0biangleleft;\x03◃\x08iangleq;\x03≜\x07iangle;\x03▵\x07iminus;\x03⨺\x07pezium;\x03⏢\x06iplus;\x03⨹\x06itime;\x03⨻\x05idot;\x03◬\x04ade;\x03™\x04isb;\x03⧍\x03ie;\x03≜",
- // tstrok;[ŧ] tshcy;[ћ] tscr;[𝓉] tscy;[ц].
- "\x05trok;\x02ŧ\x04hcy;\x02ћ\x03cr;\x04𝓉\x03cy;\x02ц",
- // twoheadrightarrow;[↠] twoheadleftarrow;[↞] twixt;[≬].
- "\x10oheadrightarrow;\x03↠\x0foheadleftarrow;\x03↞\x04ixt;\x03≬",
- // uArr;[⇑].
- "\x03rr;\x03⇑",
- // uHar;[⥣].
- "\x03ar;\x03⥣",
- // uacute;[ú] uacute[ú] uarr;[↑].
- "\x05cute;\x02ú\x04cute\x02ú\x03rr;\x03↑",
- // ubreve;[ŭ] ubrcy;[ў].
- "\x05reve;\x02ŭ\x04rcy;\x02ў",
- // ucirc;[û] ucirc[û] ucy;[у].
- "\x04irc;\x02û\x03irc\x02û\x02y;\x02у",
- // udblac;[ű] udarr;[⇅] udhar;[⥮].
- "\x05blac;\x02ű\x04arr;\x03⇅\x04har;\x03⥮",
- // ufisht;[⥾] ufr;[𝔲].
- "\x05isht;\x03⥾\x02r;\x04𝔲",
- // ugrave;[ù] ugrave[ù].
- "\x05rave;\x02ù\x04rave\x02ù",
- // uharl;[↿] uharr;[↾] uhblk;[▀].
- "\x04arl;\x03↿\x04arr;\x03↾\x04blk;\x03▀",
- // ulcorner;[⌜] ulcorn;[⌜] ulcrop;[⌏] ultri;[◸].
- "\x07corner;\x03⌜\x05corn;\x03⌜\x05crop;\x03⌏\x04tri;\x03◸",
- // umacr;[ū] uml;[¨] uml[¨].
- "\x04acr;\x02ū\x02l;\x02¨\x01l\x02¨",
- // uogon;[ų] uopf;[𝕦].
- "\x04gon;\x02ų\x03pf;\x04𝕦",
- // upharpoonright;[↾] upharpoonleft;[↿] updownarrow;[↕] upuparrows;[⇈] uparrow;[↑] upsilon;[υ] uplus;[⊎] upsih;[ϒ] upsi;[υ].
- "\x0dharpoonright;\x03↾\x0charpoonleft;\x03↿\x0adownarrow;\x03↕\x09uparrows;\x03⇈\x06arrow;\x03↑\x06silon;\x02υ\x04lus;\x03⊎\x04sih;\x02ϒ\x03si;\x02υ",
- // urcorner;[⌝] urcorn;[⌝] urcrop;[⌎] uring;[ů] urtri;[◹].
- "\x07corner;\x03⌝\x05corn;\x03⌝\x05crop;\x03⌎\x04ing;\x02ů\x04tri;\x03◹",
- // uscr;[𝓊].
- "\x03cr;\x04𝓊",
- // utilde;[ũ] utdot;[⋰] utrif;[▴] utri;[▵].
- "\x05ilde;\x02ũ\x04dot;\x03⋰\x04rif;\x03▴\x03ri;\x03▵",
- // uuarr;[⇈] uuml;[ü] uuml[ü].
- "\x04arr;\x03⇈\x03ml;\x02ü\x02ml\x02ü",
- // uwangle;[⦧].
- "\x06angle;\x03⦧",
- // vArr;[⇕].
- "\x03rr;\x03⇕",
- // vBarv;[⫩] vBar;[⫨].
- "\x04arv;\x03⫩\x03ar;\x03⫨",
- // vDash;[⊨].
- "\x04ash;\x03⊨",
- // vartriangleright;[⊳] vartriangleleft;[⊲] varsubsetneqq;[⫋︀] varsupsetneqq;[⫌︀] varsubsetneq;[⊊︀] varsupsetneq;[⊋︀] varepsilon;[ϵ] varnothing;[∅] varpropto;[∝] varkappa;[ϰ] varsigma;[ς] vartheta;[ϑ] vangrt;[⦜] varphi;[ϕ] varrho;[ϱ] varpi;[ϖ] varr;[↕].
- "\x0frtriangleright;\x03⊳\x0ertriangleleft;\x03⊲\x0crsubsetneqq;\x06⫋︀\x0crsupsetneqq;\x06⫌︀\x0brsubsetneq;\x06⊊︀\x0brsupsetneq;\x06⊋︀\x09repsilon;\x02ϵ\x09rnothing;\x03∅\x08rpropto;\x03∝\x07rkappa;\x02ϰ\x07rsigma;\x02ς\x07rtheta;\x02ϑ\x05ngrt;\x03⦜\x05rphi;\x02ϕ\x05rrho;\x02ϱ\x04rpi;\x02ϖ\x03rr;\x03↕",
- // vcy;[в].
- "\x02y;\x02в",
- // vdash;[⊢].
- "\x04ash;\x03⊢",
- // veebar;[⊻] vellip;[⋮] verbar;[|] veeeq;[≚] vert;[|] vee;[∨].
- "\x05ebar;\x03⊻\x05llip;\x03⋮\x05rbar;\x01|\x04eeq;\x03≚\x03rt;\x01|\x02e;\x03∨",
- // vfr;[𝔳].
- "\x02r;\x04𝔳",
- // vltri;[⊲].
- "\x04tri;\x03⊲",
- // vnsub;[⊂⃒] vnsup;[⊃⃒].
- "\x04sub;\x06⊂⃒\x04sup;\x06⊃⃒",
- // vopf;[𝕧].
- "\x03pf;\x04𝕧",
- // vprop;[∝].
- "\x04rop;\x03∝",
- // vrtri;[⊳].
- "\x04tri;\x03⊳",
- // vsubnE;[⫋︀] vsubne;[⊊︀] vsupnE;[⫌︀] vsupne;[⊋︀] vscr;[𝓋].
- "\x05ubnE;\x06⫋︀\x05ubne;\x06⊊︀\x05upnE;\x06⫌︀\x05upne;\x06⊋︀\x03cr;\x04𝓋",
- // vzigzag;[⦚].
- "\x06igzag;\x03⦚",
- // wcirc;[ŵ].
- "\x04irc;\x02ŵ",
- // wedbar;[⩟] wedgeq;[≙] weierp;[℘] wedge;[∧].
- "\x05dbar;\x03⩟\x05dgeq;\x03≙\x05ierp;\x03℘\x04dge;\x03∧",
- // wfr;[𝔴].
- "\x02r;\x04𝔴",
- // wopf;[𝕨].
- "\x03pf;\x04𝕨",
- // wp;[℘].
- "\x01;\x03℘",
- // wreath;[≀] wr;[≀].
- "\x05eath;\x03≀\x01;\x03≀",
- // wscr;[𝓌].
- "\x03cr;\x04𝓌",
- // xcirc;[◯] xcap;[⋂] xcup;[⋃].
- "\x04irc;\x03◯\x03ap;\x03⋂\x03up;\x03⋃",
- // xdtri;[▽].
- "\x04tri;\x03▽",
- // xfr;[𝔵].
- "\x02r;\x04𝔵",
- // xhArr;[⟺] xharr;[⟷].
- "\x04Arr;\x03⟺\x04arr;\x03⟷",
- // xi;[ξ].
- "\x01;\x02ξ",
- // xlArr;[⟸] xlarr;[⟵].
- "\x04Arr;\x03⟸\x04arr;\x03⟵",
- // xmap;[⟼].
- "\x03ap;\x03⟼",
- // xnis;[⋻].
- "\x03is;\x03⋻",
- // xoplus;[⨁] xotime;[⨂] xodot;[⨀] xopf;[𝕩].
- "\x05plus;\x03⨁\x05time;\x03⨂\x04dot;\x03⨀\x03pf;\x04𝕩",
- // xrArr;[⟹] xrarr;[⟶].
- "\x04Arr;\x03⟹\x04arr;\x03⟶",
- // xsqcup;[⨆] xscr;[𝓍].
- "\x05qcup;\x03⨆\x03cr;\x04𝓍",
- // xuplus;[⨄] xutri;[△].
- "\x05plus;\x03⨄\x04tri;\x03△",
- // xvee;[⋁].
- "\x03ee;\x03⋁",
- // xwedge;[⋀].
- "\x05edge;\x03⋀",
- // yacute;[ý] yacute[ý] yacy;[я].
- "\x05cute;\x02ý\x04cute\x02ý\x03cy;\x02я",
- // ycirc;[ŷ] ycy;[ы].
- "\x04irc;\x02ŷ\x02y;\x02ы",
- // yen;[¥] yen[¥].
- "\x02n;\x02¥\x01n\x02¥",
- // yfr;[𝔶].
- "\x02r;\x04𝔶",
- // yicy;[ї].
- "\x03cy;\x02ї",
- // yopf;[𝕪].
- "\x03pf;\x04𝕪",
- // yscr;[𝓎].
- "\x03cr;\x04𝓎",
- // yucy;[ю] yuml;[ÿ] yuml[ÿ].
- "\x03cy;\x02ю\x03ml;\x02ÿ\x02ml\x02ÿ",
- // zacute;[ź].
- "\x05cute;\x02ź",
- // zcaron;[ž] zcy;[з].
- "\x05aron;\x02ž\x02y;\x02з",
- // zdot;[ż].
- "\x03ot;\x02ż",
- // zeetrf;[ℨ] zeta;[ζ].
- "\x05etrf;\x03ℨ\x03ta;\x02ζ",
- // zfr;[𝔷].
- "\x02r;\x04𝔷",
- // zhcy;[ж].
- "\x03cy;\x02ж",
- // zigrarr;[⇝].
- "\x06grarr;\x03⇝",
- // zopf;[𝕫].
- "\x03pf;\x04𝕫",
- // zscr;[𝓏].
- "\x03cr;\x04𝓏",
- // zwnj;[] zwj;[].
- "\x03nj;\x03\x02j;\x03",
- ),
- "small_words" => "GT\x00LT\x00gt\x00lt\x00",
- "small_mappings" => array(
- ">",
- "<",
- ">",
- "<",
- )
- )
- );
-}
diff --git a/lib/compat/wordpress-6.6/option.php b/lib/compat/wordpress-6.6/option.php
deleted file mode 100644
index 9ec81467c261d..0000000000000
--- a/lib/compat/wordpress-6.6/option.php
+++ /dev/null
@@ -1,53 +0,0 @@
- __( 'Title' ),
- 'blogdescription' => __( 'Tagline' ),
- 'show_on_front' => __( 'Show on front' ),
- 'page_on_front' => __( 'Page on front' ),
- 'posts_per_page' => __( 'Maximum posts per page' ),
- 'default_comment_status' => __( 'Allow comments on new posts' ),
- );
-
- if ( isset( $settings_label_map[ $option_name ] ) ) {
- $args['label'] = $settings_label_map[ $option_name ];
- }
-
- // Don't update schema when a setting isn't exposed via REST API.
- if ( ! isset( $args['show_in_rest'] ) ) {
- return $args;
- }
-
- // Don't update schema when label isn't provided.
- if ( ! isset( $args['label'] ) ) {
- return $args;
- }
-
- $schema = array( 'title' => $args['label'] );
- if ( ! is_array( $args['show_in_rest'] ) ) {
- $args['show_in_rest'] = array(
- 'schema' => $schema,
- );
- return $args;
- }
-
- if ( ! empty( $args['show_in_rest']['schema'] ) ) {
- $args['show_in_rest']['schema'] = array_merge( $args['show_in_rest']['schema'], $schema );
- } else {
- $args['show_in_rest']['schema'] = $schema;
- }
-
- return $args;
-}
-add_filter( 'register_setting_args', 'gutenberg_update_initial_settings', 10, 4 );
diff --git a/lib/compat/wordpress-6.6/post.php b/lib/compat/wordpress-6.6/post.php
deleted file mode 100644
index 8415f3bf42f18..0000000000000
--- a/lib/compat/wordpress-6.6/post.php
+++ /dev/null
@@ -1,39 +0,0 @@
-item_updated = __( 'Template updated.', 'gutenberg' );
- return $labels;
-}
-add_filter( 'post_type_labels_wp_template', 'gutenberg_update_wp_template_labels', 10, 1 );
-
-/**
- * Updates the labels for the template parts post type.
- *
- * @param object $labels Object with labels for the post type as member variables.
- * @return object Object with all the labels as member variables.
- */
-function gutenberg_update_wp_template__part_labels( $labels ) {
- $labels->item_updated = __( 'Template part updated.', 'gutenberg' );
- return $labels;
-}
-add_filter( 'post_type_labels_wp_template_part', 'gutenberg_update_wp_template__part_labels', 10, 1 );
diff --git a/lib/compat/wordpress-6.6/resolve-patterns.php b/lib/compat/wordpress-6.6/resolve-patterns.php
deleted file mode 100644
index 5105619c42613..0000000000000
--- a/lib/compat/wordpress-6.6/resolve-patterns.php
+++ /dev/null
@@ -1,84 +0,0 @@
-get_registered( $slug );
-
- // Skip unknown patterns.
- if ( ! $pattern ) {
- ++$i;
- continue;
- }
-
- $blocks_to_insert = parse_blocks( $pattern['content'] );
- $seen_refs[ $slug ] = true;
- $blocks_to_insert = gutenberg_replace_pattern_blocks( $blocks_to_insert );
- unset( $seen_refs[ $slug ] );
- array_splice( $blocks, $i, 1, $blocks_to_insert );
-
- // If we have inner content, we need to insert nulls in the
- // inner content array, otherwise serialize_blocks will skip
- // blocks.
- if ( $inner_content ) {
- $null_indices = array_keys( $inner_content, null, true );
- $content_index = $null_indices[ $i ];
- $nulls = array_fill( 0, count( $blocks_to_insert ), null );
- array_splice( $inner_content, $content_index, 1, $nulls );
- }
-
- // Skip inserted blocks.
- $i += count( $blocks_to_insert );
- } else {
- if ( ! empty( $blocks[ $i ]['innerBlocks'] ) ) {
- $blocks[ $i ]['innerBlocks'] = gutenberg_replace_pattern_blocks(
- $blocks[ $i ]['innerBlocks'],
- $blocks[ $i ]['innerContent']
- );
- }
- ++$i;
- }
- }
- return $blocks;
-}
-
-function gutenberg_replace_pattern_blocks_patterns_endpoint( $result, $server, $request ) {
- if ( $request->get_route() !== '/wp/v2/block-patterns/patterns' ) {
- return $result;
- }
-
- $data = $result->get_data();
-
- foreach ( $data as $index => $pattern ) {
- $blocks = parse_blocks( $pattern['content'] );
- $blocks = gutenberg_replace_pattern_blocks( $blocks );
- $data[ $index ]['content'] = serialize_blocks( $blocks );
- }
-
- $result->set_data( $data );
-
- return $result;
-}
-
-// Similarly, for patterns, we can avoid the double parse here:
-// https://github.com/WordPress/wordpress-develop/blob/02fb53498f1ce7e63d807b9bafc47a7dba19d169/src/wp-includes/class-wp-block-patterns-registry.php#L175
-add_filter( 'rest_post_dispatch', 'gutenberg_replace_pattern_blocks_patterns_endpoint', 10, 3 );
diff --git a/lib/compat/wordpress-6.6/rest-api.php b/lib/compat/wordpress-6.6/rest-api.php
deleted file mode 100644
index eadd3b1d376a7..0000000000000
--- a/lib/compat/wordpress-6.6/rest-api.php
+++ /dev/null
@@ -1,216 +0,0 @@
- true,
- 'show_in_rest' => true,
- ),
- 'names'
- );
-
- if ( ! empty( $post_types ) ) {
- register_rest_field(
- $post_types,
- 'class_list',
- array(
- 'get_callback' => 'gutenberg_add_class_list_to_api_response',
- 'schema' => array(
- 'description' => __( 'An array of the class names for the post container element.', 'gutenberg' ),
- 'type' => 'array',
- 'items' => array(
- 'type' => 'string',
- ),
- ),
- )
- );
- }
-}
-add_action( 'rest_api_init', 'gutenberg_add_class_list_to_public_post_types' );
-
-
-/**
- * Registers the Global Styles Revisions REST API routes.
- */
-function gutenberg_register_global_styles_revisions_endpoints() {
- $global_styles_revisions_controller = new Gutenberg_REST_Global_Styles_Revisions_Controller_6_6();
- $global_styles_revisions_controller->register_routes();
-}
-
-add_action( 'rest_api_init', 'gutenberg_register_global_styles_revisions_endpoints' );
-
-/**
- * Adds `stylesheet_uri` fields to WP_REST_Themes_Controller class.
- */
-function gutenberg_register_wp_rest_themes_stylesheet_directory_uri_field() {
- register_rest_field(
- 'theme',
- 'stylesheet_uri',
- array(
- 'get_callback' => function ( $item ) {
- if ( ! empty( $item['stylesheet'] ) ) {
- $theme = wp_get_theme( $item['stylesheet'] );
- $current_theme = wp_get_theme();
- if ( $theme->get_stylesheet() === $current_theme->get_stylesheet() ) {
- return get_stylesheet_directory_uri();
- } else {
- return $theme->get_stylesheet_directory_uri();
- }
- }
-
- return null;
- },
- 'schema' => array(
- 'type' => 'string',
- 'description' => __( 'The uri for the theme\'s stylesheet directory.', 'gutenberg' ),
- 'format' => 'uri',
- 'readonly' => true,
- 'context' => array( 'view', 'edit', 'embed' ),
- ),
- )
- );
-}
-add_action( 'rest_api_init', 'gutenberg_register_wp_rest_themes_stylesheet_directory_uri_field' );
-
-/**
- * Adds `template_uri` fields to WP_REST_Themes_Controller class.
- */
-function gutenberg_register_wp_rest_themes_template_directory_uri_field() {
- register_rest_field(
- 'theme',
- 'template_uri',
- array(
- 'get_callback' => function ( $item ) {
- if ( ! empty( $item['stylesheet'] ) ) {
- $theme = wp_get_theme( $item['stylesheet'] );
- $current_theme = wp_get_theme();
- if ( $theme->get_stylesheet() === $current_theme->get_stylesheet() ) {
- return get_template_directory_uri();
- } else {
- return $theme->get_template_directory_uri();
- }
- }
-
- return null;
- },
- 'schema' => array(
- 'type' => 'string',
- 'description' => __( 'The uri for the theme\'s template directory. If this is a child theme, this refers to the parent theme, otherwise this is the same as the theme\'s stylesheet directory.', 'gutenberg' ),
- 'format' => 'uri',
- 'readonly' => true,
- 'context' => array( 'view', 'edit', 'embed' ),
- ),
- )
- );
-}
-add_action( 'rest_api_init', 'gutenberg_register_wp_rest_themes_template_directory_uri_field' );
-
-/**
- * Adds `template` and `template_lock` fields to WP_REST_Post_Types_Controller class.
- */
-function gutenberg_register_wp_rest_post_types_controller_fields() {
- register_rest_field(
- 'type',
- 'template',
- array(
- 'get_callback' => function ( $item ) {
- $post_type = get_post_type_object( $item['slug'] );
- if ( ! empty( $post_type ) ) {
- return $post_type->template ?? array();
- }
- },
- 'schema' => array(
- 'type' => 'array',
- 'description' => __( 'The block template associated with the post type.', 'gutenberg' ),
- 'readonly' => true,
- 'context' => array( 'view', 'edit', 'embed' ),
- ),
- )
- );
- register_rest_field(
- 'type',
- 'template_lock',
- array(
- 'get_callback' => function ( $item ) {
- $post_type = get_post_type_object( $item['slug'] );
- if ( ! empty( $post_type ) ) {
- return ! empty( $post_type->template_lock ) ? $post_type->template_lock : false;
- }
- },
- 'schema' => array(
- 'type' => array( 'string', 'boolean' ),
- 'enum' => array( 'all', 'insert', 'contentOnly', false ),
- 'description' => __( 'The template_lock associated with the post type, or false if none.', 'gutenberg' ),
- 'readonly' => true,
- 'context' => array( 'view', 'edit', 'embed' ),
- ),
- )
- );
-}
-add_action( 'rest_api_init', 'gutenberg_register_wp_rest_post_types_controller_fields' );
-
-/**
- * Preload theme and global styles paths to avoid flash of variation styles in post editor.
- *
- * @param array $paths REST API paths to preload.
- * @param WP_Block_Editor_Context $context Current block editor context.
- * @return array Filtered preload paths.
- */
-function gutenberg_block_editor_preload_paths_6_6( $paths, $context ) {
- if ( 'core/edit-post' === $context->name ) {
- $paths[] = '/wp/v2/global-styles/themes/' . get_stylesheet();
- $paths[] = '/wp/v2/themes?context=edit&status=active';
- $paths[] = '/wp/v2/global-styles/' . WP_Theme_JSON_Resolver_Gutenberg::get_user_global_styles_post_id() . '?context=edit';
- }
- return $paths;
-}
-add_filter( 'block_editor_rest_api_preload_paths', 'gutenberg_block_editor_preload_paths_6_6', 10, 2 );
diff --git a/lib/compat/wordpress-6.7/class-gutenberg-rest-templates-controller-6-7.php b/lib/compat/wordpress-6.7/class-gutenberg-rest-templates-controller-6-7.php
index ed67dded75ecb..e5f6eb126f2a6 100644
--- a/lib/compat/wordpress-6.7/class-gutenberg-rest-templates-controller-6-7.php
+++ b/lib/compat/wordpress-6.7/class-gutenberg-rest-templates-controller-6-7.php
@@ -9,7 +9,7 @@
* Gutenberg_REST_Templates_Controller_6_7 class
*
*/
-class Gutenberg_REST_Templates_Controller_6_7 extends Gutenberg_REST_Templates_Controller_6_6 {
+class Gutenberg_REST_Templates_Controller_6_7 extends WP_REST_Templates_Controller {
/**
* Returns the given template
*
diff --git a/lib/compat/wordpress-6.7/compat.php b/lib/compat/wordpress-6.7/compat.php
index e58eca56ef71f..33c123a14860d 100644
--- a/lib/compat/wordpress-6.7/compat.php
+++ b/lib/compat/wordpress-6.7/compat.php
@@ -46,7 +46,7 @@ function _gutenberg_add_block_templates_from_registry( $query_result, $query, $t
// See: https://github.com/WordPress/gutenberg/issues/65584
$template_files_query = $query;
unset( $template_files_query['post_type'] );
- $template_files = _gutenberg_get_block_templates_files( $template_type, $template_files_query );
+ $template_files = _get_block_templates_files( $template_type, $template_files_query );
/*
* Add templates registered in the template registry. Filtering out the ones which have a theme file.
diff --git a/lib/compat/wordpress-6.7/rest-api.php b/lib/compat/wordpress-6.7/rest-api.php
index 741d0ad1805e0..2b53e6865dfa9 100644
--- a/lib/compat/wordpress-6.7/rest-api.php
+++ b/lib/compat/wordpress-6.7/rest-api.php
@@ -124,15 +124,18 @@ function gutenberg_register_wp_rest_templates_controller_plugin_field() {
}
add_action( 'rest_api_init', 'gutenberg_register_wp_rest_templates_controller_plugin_field' );
-/**
- * Overrides the default 'WP_REST_Server' class.
- *
- * @return string The name of the custom server class.
- */
-function gutenberg_override_default_rest_server() {
- return 'Gutenberg_REST_Server';
+// The `get_user` function was introduced in WP 6.7.
+if ( ! function_exists( 'get_user' ) ) {
+ /**
+ * Overrides the default 'WP_REST_Server' class.
+ *
+ * @return string The name of the custom server class.
+ */
+ function gutenberg_override_default_rest_server() {
+ return 'Gutenberg_REST_Server';
+ }
+ add_filter( 'wp_rest_server_class', 'gutenberg_override_default_rest_server', 1 );
}
-add_filter( 'wp_rest_server_class', 'gutenberg_override_default_rest_server', 1 );
/**
diff --git a/lib/compat/wordpress-6.8/blocks.php b/lib/compat/wordpress-6.8/blocks.php
index 3124dd2a12a61..8e176e58c8d7f 100644
--- a/lib/compat/wordpress-6.8/blocks.php
+++ b/lib/compat/wordpress-6.8/blocks.php
@@ -6,42 +6,308 @@
*/
/**
- * Filters the block type arguments during registration to stabilize experimental block supports.
+ * Filters the block type arguments during registration to stabilize
+ * experimental block supports.
*
- * This is a temporary compatibility shim as the approach in core is for this to be handled
- * within the WP_Block_Type class rather than requiring a filter.
+ * This is a temporary compatibility shim as the approach in core is for this
+ * to be handled within the WP_Block_Type class rather than requiring a filter.
*
* @param array $args Array of arguments for registering a block type.
* @return array Array of arguments for registering a block type.
*/
function gutenberg_stabilize_experimental_block_supports( $args ) {
- if ( empty( $args['supports']['typography'] ) ) {
+ if ( empty( $args['supports'] ) ) {
return $args;
}
- $experimental_typography_supports_to_stable = array(
- '__experimentalFontFamily' => 'fontFamily',
- '__experimentalFontStyle' => 'fontStyle',
- '__experimentalFontWeight' => 'fontWeight',
- '__experimentalLetterSpacing' => 'letterSpacing',
- '__experimentalTextDecoration' => 'textDecoration',
- '__experimentalTextTransform' => 'textTransform',
+ $experimental_supports_map = array( '__experimentalBorder' => 'border' );
+ $common_experimental_properties = array(
+ '__experimentalDefaultControls' => 'defaultControls',
+ '__experimentalSkipSerialization' => 'skipSerialization',
);
+ $experimental_support_properties = array(
+ 'typography' => array(
+ '__experimentalFontFamily' => 'fontFamily',
+ '__experimentalFontStyle' => 'fontStyle',
+ '__experimentalFontWeight' => 'fontWeight',
+ '__experimentalLetterSpacing' => 'letterSpacing',
+ '__experimentalTextDecoration' => 'textDecoration',
+ '__experimentalTextTransform' => 'textTransform',
+ ),
+ );
+ $done = array();
+
+ $updated_supports = array();
+ foreach ( $args['supports'] as $support => $config ) {
+ /*
+ * If this support config has already been stabilized, skip it.
+ * A stable support key occurring after an experimental key, gets
+ * stabilized then so that the two configs can be merged effectively.
+ */
+ if ( isset( $done[ $support ] ) ) {
+ continue;
+ }
+
+ $stable_support_key = $experimental_supports_map[ $support ] ?? $support;
+
+ /*
+ * Use the support's config as is when it's not in need of stabilization.
+ *
+ * A support does not need stabilization if:
+ * - The support key doesn't need stabilization AND
+ * - Either:
+ * - The config isn't an array, so can't have experimental properties OR
+ * - The config is an array but has no experimental properties to stabilize.
+ */
+ if ( $support === $stable_support_key &&
+ ( ! is_array( $config ) ||
+ ( ! isset( $experimental_support_properties[ $stable_support_key ] ) &&
+ empty( array_intersect_key( $common_experimental_properties, $config ) )
+ )
+ )
+ ) {
+ $updated_supports[ $support ] = $config;
+ continue;
+ }
+
+ $stabilize_config = function ( $unstable_config, $stable_support_key ) use ( $experimental_support_properties, $common_experimental_properties ) {
+ if ( ! is_array( $unstable_config ) ) {
+ return $unstable_config;
+ }
+
+ $stable_config = array();
+ foreach ( $unstable_config as $key => $value ) {
+ // Get stable key from support-specific map, common properties map, or keep original.
+ $stable_key = $experimental_support_properties[ $stable_support_key ][ $key ] ??
+ $common_experimental_properties[ $key ] ??
+ $key;
- $current_typography_supports = $args['supports']['typography'];
- $stable_typography_supports = array();
+ $stable_config[ $stable_key ] = $value;
- foreach ( $current_typography_supports as $key => $value ) {
- if ( array_key_exists( $key, $experimental_typography_supports_to_stable ) ) {
- $stable_typography_supports[ $experimental_typography_supports_to_stable[ $key ] ] = $value;
- } else {
- $stable_typography_supports[ $key ] = $value;
+ /*
+ * The `__experimentalSkipSerialization` key needs to be kept until
+ * WP 6.8 becomes the minimum supported version. This is due to the
+ * core `wp_should_skip_block_supports_serialization` function only
+ * checking for `__experimentalSkipSerialization` in earlier versions.
+ */
+ if ( '__experimentalSkipSerialization' === $key || 'skipSerialization' === $key ) {
+ $stable_config['__experimentalSkipSerialization'] = $value;
+ }
+ }
+ return $stable_config;
+ };
+
+ // Stabilize the config value.
+ $stable_config = is_array( $config ) ? $stabilize_config( $config, $stable_support_key ) : $config;
+
+ /*
+ * If a plugin overrides the support config with the `register_block_type_args`
+ * filter, both experimental and stable configs may be present. In that case,
+ * use the order keys are defined in to determine the final value.
+ * - If config is an array, merge the arrays in their order of definition.
+ * - If config is not an array, use the value defined last.
+ *
+ * The reason for preferring the last defined key is that after filters
+ * are applied, the last inserted key is likely the most up-to-date value.
+ * We cannot determine with certainty which value was "last modified" so
+ * the insertion order is the best guess. The extreme edge case of multiple
+ * filters tweaking the same support property will become less over time as
+ * extenders migrate existing blocks and plugins to stable keys.
+ */
+ if ( $support !== $stable_support_key && isset( $args['supports'][ $stable_support_key ] ) ) {
+ $key_positions = array_flip( array_keys( $args['supports'] ) );
+ $experimental_first =
+ ( $key_positions[ $support ] ?? PHP_INT_MAX ) <
+ ( $key_positions[ $stable_support_key ] ?? PHP_INT_MAX );
+
+ /*
+ * To merge the alternative support config effectively, it also needs to be
+ * stabilized before merging to keep stabilized and experimental flags in
+ * sync.
+ */
+ $args['supports'][ $stable_support_key ] = $stabilize_config( $args['supports'][ $stable_support_key ], $stable_support_key );
+ // Prevents reprocessing this support as it was stabilized above.
+ $done[ $stable_support_key ] = true;
+
+ if ( is_array( $stable_config ) && is_array( $args['supports'][ $stable_support_key ] ) ) {
+ $stable_config = $experimental_first
+ ? array_merge( $stable_config, $args['supports'][ $stable_support_key ] )
+ : array_merge( $args['supports'][ $stable_support_key ], $stable_config );
+ } else {
+ $stable_config = $experimental_first
+ ? $args['supports'][ $stable_support_key ]
+ : $stable_config;
+ }
}
+
+ $updated_supports[ $stable_support_key ] = $stable_config;
}
- $args['supports']['typography'] = $stable_typography_supports;
+ $args['supports'] = $updated_supports;
return $args;
}
add_filter( 'register_block_type_args', 'gutenberg_stabilize_experimental_block_supports', PHP_INT_MAX, 1 );
+
+function gutenberg_apply_block_hooks_to_post_content( $content ) {
+ // The `the_content` filter does not provide the post that the content is coming from.
+ // However, we can infer it by calling `get_post()`, which will return the current post
+ // if no post ID is provided.
+ return apply_block_hooks_to_content( $content, get_post(), 'insert_hooked_blocks' );
+}
+// We need to apply this filter before `do_blocks` (which is hooked to `the_content` at priority 9).
+add_filter( 'the_content', 'gutenberg_apply_block_hooks_to_post_content', 8 );
+
+/**
+ * Hooks into the REST API response for the Posts endpoint and adds the first and last inner blocks.
+ *
+ * @since 6.6.0
+ * @since 6.8.0 Support non-`wp_navigation` post types.
+ *
+ * @param WP_REST_Response $response The response object.
+ * @param WP_Post $post Post object.
+ * @return WP_REST_Response The response object.
+ */
+function gutenberg_insert_hooked_blocks_into_rest_response( $response, $post ) {
+ if ( empty( $response->data['content']['raw'] ) || empty( $response->data['content']['rendered'] ) ) {
+ return $response;
+ }
+
+ $attributes = array();
+ $ignored_hooked_blocks = get_post_meta( $post->ID, '_wp_ignored_hooked_blocks', true );
+ if ( ! empty( $ignored_hooked_blocks ) ) {
+ $ignored_hooked_blocks = json_decode( $ignored_hooked_blocks, true );
+ $attributes['metadata'] = array(
+ 'ignoredHookedBlocks' => $ignored_hooked_blocks,
+ );
+ }
+
+ if ( 'wp_navigation' === $post->post_type ) {
+ $wrapper_block_type = 'core/navigation';
+ } else {
+ $wrapper_block_type = 'core/post-content';
+ }
+
+ $content = get_comment_delimited_block_content(
+ $wrapper_block_type,
+ $attributes,
+ $response->data['content']['raw']
+ );
+
+ $content = apply_block_hooks_to_content(
+ $content,
+ $post,
+ 'insert_hooked_blocks_and_set_ignored_hooked_blocks_metadata'
+ );
+
+ // Remove mock block wrapper.
+ $content = remove_serialized_parent_block( $content );
+
+ $response->data['content']['raw'] = $content;
+
+ // No need to inject hooked blocks twice.
+ $priority = has_filter( 'the_content', 'apply_block_hooks_to_content' );
+ if ( false !== $priority ) {
+ remove_filter( 'the_content', 'apply_block_hooks_to_content', $priority );
+ }
+
+ /** This filter is documented in wp-includes/post-template.php */
+ $response->data['content']['rendered'] = apply_filters( 'the_content', $content );
+
+ // Add back the filter.
+ if ( false !== $priority ) {
+ add_filter( 'the_content', 'apply_block_hooks_to_content', $priority );
+ }
+
+ return $response;
+}
+add_filter( 'rest_prepare_page', 'gutenberg_insert_hooked_blocks_into_rest_response', 10, 2 );
+add_filter( 'rest_prepare_post', 'gutenberg_insert_hooked_blocks_into_rest_response', 10, 2 );
+
+/**
+ * Updates the wp_postmeta with the list of ignored hooked blocks
+ * where the inner blocks are stored as post content.
+ *
+ * @since 6.6.0
+ * @since 6.8.0 Support other post types. (Previously, it was limited to `wp_navigation` only.)
+ * @access private
+ *
+ * @param stdClass $post Post object.
+ * @return stdClass The updated post object.
+ */
+function gutenberg_update_ignored_hooked_blocks_postmeta( $post ) {
+ /*
+ * In this scenario the user has likely tried to create a new post object via the REST API.
+ * In which case we won't have a post ID to work with and store meta against.
+ */
+ if ( empty( $post->ID ) ) {
+ return $post;
+ }
+
+ /*
+ * Skip meta generation when consumers intentionally update specific fields
+ * and omit the content update.
+ */
+ if ( ! isset( $post->post_content ) ) {
+ return $post;
+ }
+
+ /*
+ * Skip meta generation if post type is not set.
+ */
+ if ( ! isset( $post->post_type ) ) {
+ return $post;
+ }
+
+ $attributes = array();
+
+ $ignored_hooked_blocks = get_post_meta( $post->ID, '_wp_ignored_hooked_blocks', true );
+ if ( ! empty( $ignored_hooked_blocks ) ) {
+ $ignored_hooked_blocks = json_decode( $ignored_hooked_blocks, true );
+ $attributes['metadata'] = array(
+ 'ignoredHookedBlocks' => $ignored_hooked_blocks,
+ );
+ }
+
+ if ( 'wp_navigation' === $post->post_type ) {
+ $wrapper_block_type = 'core/navigation';
+ } else {
+ $wrapper_block_type = 'core/post-content';
+ }
+
+ $markup = get_comment_delimited_block_content(
+ $wrapper_block_type,
+ $attributes,
+ $post->post_content
+ );
+
+ $existing_post = get_post( $post->ID );
+ // Merge the existing post object with the updated post object to pass to the block hooks algorithm for context.
+ $context = (object) array_merge( (array) $existing_post, (array) $post );
+ $context = new WP_Post( $context ); // Convert to WP_Post object.
+ $serialized_block = apply_block_hooks_to_content( $markup, $context, 'set_ignored_hooked_blocks_metadata' );
+ $root_block = parse_blocks( $serialized_block )[0];
+
+ $ignored_hooked_blocks = isset( $root_block['attrs']['metadata']['ignoredHookedBlocks'] )
+ ? $root_block['attrs']['metadata']['ignoredHookedBlocks']
+ : array();
+
+ if ( ! empty( $ignored_hooked_blocks ) ) {
+ $existing_ignored_hooked_blocks = get_post_meta( $post->ID, '_wp_ignored_hooked_blocks', true );
+ if ( ! empty( $existing_ignored_hooked_blocks ) ) {
+ $existing_ignored_hooked_blocks = json_decode( $existing_ignored_hooked_blocks, true );
+ $ignored_hooked_blocks = array_unique( array_merge( $ignored_hooked_blocks, $existing_ignored_hooked_blocks ) );
+ }
+
+ if ( ! isset( $post->meta_input ) ) {
+ $post->meta_input = array();
+ }
+ $post->meta_input['_wp_ignored_hooked_blocks'] = json_encode( $ignored_hooked_blocks );
+ }
+
+ $post->post_content = remove_serialized_parent_block( $serialized_block );
+ return $post;
+}
+add_filter( 'rest_pre_insert_page', 'gutenberg_update_ignored_hooked_blocks_postmeta' );
+add_filter( 'rest_pre_insert_post', 'gutenberg_update_ignored_hooked_blocks_postmeta' );
diff --git a/lib/compat/wordpress-6.8/class-gutenberg-rest-post-types-controller-6-8.php b/lib/compat/wordpress-6.8/class-gutenberg-rest-post-types-controller-6-8.php
new file mode 100644
index 0000000000000..da0489210e21f
--- /dev/null
+++ b/lib/compat/wordpress-6.8/class-gutenberg-rest-post-types-controller-6-8.php
@@ -0,0 +1,61 @@
+default_rendering_mode, $item );
+
+ /**
+ * Filters the block editor rendering mode for a specific post type.
+ * Applied after the generic `post_type_default_rendering_mode` filter.
+ *
+ * The dynamic portion of the hook name, `$item->name`, refers to the post type slug.
+ *
+ * @since 6.8.0
+ * @param string $default_rendering_mode Default rendering mode for the post type.
+ * @param WP_Post_Type $post_type Post type object.
+ * @return string Default rendering mode for the post type.
+ */
+ $rendering_mode = apply_filters( "post_type_{$item->name}_default_rendering_mode", $rendering_mode, $item );
+
+ // Validate the filtered rendering mode.
+ if ( ! in_array( $rendering_mode, gutenberg_post_type_rendering_modes(), true ) ) {
+ $rendering_mode = 'post-only';
+ }
+
+ $response->data['default_rendering_mode'] = $rendering_mode;
+ }
+
+ return rest_ensure_response( $response );
+ }
+}
diff --git a/lib/compat/wordpress-6.8/class-gutenberg-rest-user-controller.php b/lib/compat/wordpress-6.8/class-gutenberg-rest-user-controller.php
new file mode 100644
index 0000000000000..c1ecb8c86660c
--- /dev/null
+++ b/lib/compat/wordpress-6.8/class-gutenberg-rest-user-controller.php
@@ -0,0 +1,62 @@
+ array(),
+ 'description' => __( 'Array of column names to be searched.' ),
+ 'type' => 'array',
+ 'items' => array(
+ 'enum' => array( 'email', 'name', 'id', 'username', 'slug' ),
+ 'type' => 'string',
+ ),
+ );
+
+ return $query_params;
+}
+
+add_filter( 'rest_user_collection_params', 'gutenberg_add_search_columns_param', 10, 1 );
+
+/**
+ * Modify user query based on search_columns parameter
+ *
+ * @param array $prepared_args Array of arguments for WP_User_Query.
+ * @param WP_REST_Request $request The REST API request.
+ * @return array Modified arguments
+ */
+function gutenberg_modify_user_query_args( $prepared_args, $request ) {
+ if ( $request->get_param( 'search' ) && $request->get_param( 'search_columns' ) ) {
+ $search_columns = $request->get_param( 'search_columns' );
+
+ // Validate search columns
+ $valid_columns = isset( $prepared_args['search_columns'] )
+ ? $prepared_args['search_columns']
+ : array( 'ID', 'user_login', 'user_nicename', 'user_email', 'user_url', 'display_name' );
+ $search_columns_mapping = array(
+ 'id' => 'ID',
+ 'username' => 'user_login',
+ 'slug' => 'user_nicename',
+ 'email' => 'user_email',
+ 'name' => 'display_name',
+ );
+ $search_columns = array_map(
+ static function ( $column ) use ( $search_columns_mapping ) {
+ return $search_columns_mapping[ $column ];
+ },
+ $search_columns
+ );
+ $search_columns = array_intersect( $search_columns, $valid_columns );
+
+ if ( ! empty( $search_columns ) ) {
+ $prepared_args['search_columns'] = $search_columns;
+ }
+ }
+
+ return $prepared_args;
+}
+add_filter( 'rest_user_query', 'gutenberg_modify_user_query_args', 10, 2 );
diff --git a/lib/compat/wordpress-6.8/post.php b/lib/compat/wordpress-6.8/post.php
new file mode 100644
index 0000000000000..be842d89b5151
--- /dev/null
+++ b/lib/compat/wordpress-6.8/post.php
@@ -0,0 +1,60 @@
+name ) {
- if ( ! empty( $_GET['postId'] ) ) {
- $route_for_post = rest_get_route_for_post( $_GET['postId'] );
+ $post = null;
+ if ( isset( $_GET['postId'] ) && is_numeric( $_GET['postId'] ) ) {
+ $post = get_post( (int) $_GET['postId'] );
+ }
+ if ( isset( $_GET['p'] ) && preg_match( '/^\/page\/(\d+)$/', $_GET['p'], $matches ) ) {
+ $post = get_post( (int) $matches[1] );
+ }
+
+ if ( $post ) {
+ $route_for_post = rest_get_route_for_post( $post );
if ( $route_for_post ) {
$paths[] = add_query_arg( 'context', 'edit', $route_for_post );
+ $paths[] = add_query_arg( 'context', 'edit', '/wp/v2/types/' . $post->post_type );
+ if ( 'page' === $post->post_type ) {
+ $paths[] = add_query_arg(
+ 'slug',
+ // @see https://github.com/WordPress/gutenberg/blob/489f6067c623926bce7151a76755bb68d8e22ea7/packages/edit-site/src/components/sync-state-with-url/use-init-edited-entity-from-url.js#L139-L140
+ 'page-' . $post->post_name,
+ '/wp/v2/templates/lookup'
+ );
+ }
}
}
@@ -31,6 +48,8 @@ function gutenberg_block_editor_preload_paths_6_8( $paths, $context ) {
'site_icon_url',
'site_logo',
'timezone_string',
+ 'default_template_part_areas',
+ 'default_template_types',
'url',
)
);
@@ -68,6 +87,9 @@ function gutenberg_block_editor_preload_paths_6_8( $paths, $context ) {
*/
$context = current_user_can( 'edit_theme_options' ) ? 'edit' : 'view';
$paths[] = "/wp/v2/global-styles/$global_styles_id?context=$context";
+
+ // Used by getBlockPatternCategories in useBlockEditorSettings.
+ $paths[] = '/wp/v2/block-patterns/categories';
}
return $paths;
}
diff --git a/lib/compat/wordpress-6.8/rest-api.php b/lib/compat/wordpress-6.8/rest-api.php
new file mode 100644
index 0000000000000..b94e42d5f2ccd
--- /dev/null
+++ b/lib/compat/wordpress-6.8/rest-api.php
@@ -0,0 +1,89 @@
+register_routes();
+ }
+}
+add_action( 'rest_api_init', 'gutenberg_add_post_type_rendering_mode' );
+
+// When querying terms for a given taxonomy in the REST API, respect the default
+// query arguments set for that taxonomy upon registration.
+function gutenberg_respect_taxonomy_default_args_in_rest_api( $args ) {
+ // If a `post` argument is provided, the Terms controller will use
+ // `wp_get_object_terms`, which respects the default query arguments,
+ // so we don't need to do anything.
+ if ( ! empty( $args['post'] ) ) {
+ return $args;
+ }
+
+ $t = get_taxonomy( $args['taxonomy'] );
+ if ( isset( $t->args ) && is_array( $t->args ) ) {
+ $args = array_merge( $args, $t->args );
+ }
+ return $args;
+}
+add_action(
+ 'registered_taxonomy',
+ function ( $taxonomy ) {
+ add_filter( "rest_{$taxonomy}_query", 'gutenberg_respect_taxonomy_default_args_in_rest_api' );
+ }
+);
+add_action(
+ 'unregistered_taxonomy',
+ function ( $taxonomy ) {
+ remove_filter( "rest_{$taxonomy}_query", 'gutenberg_respect_taxonomy_default_args_in_rest_api' );
+ }
+);
+
+/**
+ * Adds the default template part areas to the REST API index.
+ *
+ * This function exposes the default template part areas through the WordPress REST API.
+ * Note: This function backports into the wp-includes/rest-api/class-wp-rest-server.php file.
+ *
+ * @param WP_REST_Response $response REST API response.
+ * @return WP_REST_Response Modified REST API response with default template part areas.
+ */
+function gutenberg_add_default_template_part_areas_to_index( WP_REST_Response $response ) {
+ $response->data['default_template_part_areas'] = get_allowed_block_template_part_areas();
+ return $response;
+}
+
+add_filter( 'rest_index', 'gutenberg_add_default_template_part_areas_to_index' );
+
+/**
+ * Adds the default template types to the REST API index.
+ *
+ * This function exposes the default template types through the WordPress REST API.
+ * Note: This function backports into the wp-includes/rest-api/class-wp-rest-server.php file.
+ *
+ * @param WP_REST_Response $response REST API response.
+ * @return WP_REST_Response Modified REST API response with default template part areas.
+ */
+function gutenberg_add_default_template_types_to_index( WP_REST_Response $response ) {
+ $indexed_template_types = array();
+ foreach ( get_default_block_template_types() as $slug => $template_type ) {
+ $template_type['slug'] = (string) $slug;
+ $indexed_template_types[] = $template_type;
+ }
+
+ $response->data['default_template_types'] = $indexed_template_types;
+ return $response;
+}
+
+add_filter( 'rest_index', 'gutenberg_add_default_template_types_to_index' );
diff --git a/lib/compat/wordpress-6.8/site-editor.php b/lib/compat/wordpress-6.8/site-editor.php
new file mode 100644
index 0000000000000..53d04c2e543f4
--- /dev/null
+++ b/lib/compat/wordpress-6.8/site-editor.php
@@ -0,0 +1,148 @@
+ '/wp_navigation/' . $_REQUEST['postId'] ), remove_query_arg( array( 'postType', 'postId' ) ) );
+ }
+
+ if ( isset( $_REQUEST['postType'] ) && 'wp_navigation' === $_REQUEST['postType'] && empty( $_REQUEST['postId'] ) ) {
+ return add_query_arg( array( 'p' => '/navigation' ), remove_query_arg( 'postType' ) );
+ }
+
+ if ( isset( $_REQUEST['path'] ) && '/wp_global_styles' === $_REQUEST['path'] ) {
+ return add_query_arg( array( 'p' => '/styles' ), remove_query_arg( 'path' ) );
+ }
+
+ if ( isset( $_REQUEST['postType'] ) && 'page' === $_REQUEST['postType'] && ( empty( $_REQUEST['canvas'] ) || empty( $_REQUEST['postId'] ) ) ) {
+ return add_query_arg( array( 'p' => '/page' ), remove_query_arg( 'postType' ) );
+ }
+
+ if ( isset( $_REQUEST['postType'] ) && 'page' === $_REQUEST['postType'] && ! empty( $_REQUEST['postId'] ) ) {
+ return add_query_arg( array( 'p' => '/page/' . $_REQUEST['postId'] ), remove_query_arg( array( 'postType', 'postId' ) ) );
+ }
+
+ if ( isset( $_REQUEST['postType'] ) && 'wp_template' === $_REQUEST['postType'] && ( empty( $_REQUEST['canvas'] ) || empty( $_REQUEST['postId'] ) ) ) {
+ return add_query_arg( array( 'p' => '/template' ), remove_query_arg( 'postType' ) );
+ }
+
+ if ( isset( $_REQUEST['postType'] ) && 'wp_template' === $_REQUEST['postType'] && ! empty( $_REQUEST['postId'] ) ) {
+ return add_query_arg( array( 'p' => '/wp_template/' . $_REQUEST['postId'] ), remove_query_arg( array( 'postType', 'postId' ) ) );
+ }
+
+ if ( isset( $_REQUEST['postType'] ) && 'wp_block' === $_REQUEST['postType'] && ( empty( $_REQUEST['canvas'] ) || empty( $_REQUEST['postId'] ) ) ) {
+ return add_query_arg( array( 'p' => '/pattern' ), remove_query_arg( 'postType' ) );
+ }
+
+ if ( isset( $_REQUEST['postType'] ) && 'wp_block' === $_REQUEST['postType'] && ! empty( $_REQUEST['postId'] ) ) {
+ return add_query_arg( array( 'p' => '/wp_block/' . $_REQUEST['postId'] ), remove_query_arg( array( 'postType', 'postId' ) ) );
+ }
+
+ if ( isset( $_REQUEST['postType'] ) && 'wp_template_part' === $_REQUEST['postType'] && ( empty( $_REQUEST['canvas'] ) || empty( $_REQUEST['postId'] ) ) ) {
+ return add_query_arg( array( 'p' => '/pattern' ) );
+ }
+
+ if ( isset( $_REQUEST['postType'] ) && 'wp_template_part' === $_REQUEST['postType'] && ! empty( $_REQUEST['postId'] ) ) {
+ return add_query_arg( array( 'p' => '/wp_template_part/' . $_REQUEST['postId'] ), remove_query_arg( array( 'postType', 'postId' ) ) );
+ }
+
+ // The following redirects are for backward compatibility with the old site editor URLs.
+ if ( isset( $_REQUEST['path'] ) && '/wp_template_part/all' === $_REQUEST['path'] ) {
+ return add_query_arg(
+ array(
+ 'p' => '/pattern',
+ 'postType' => 'wp_template_part',
+ ),
+ remove_query_arg( 'path' )
+ );
+ }
+
+ if ( isset( $_REQUEST['path'] ) && '/page' === $_REQUEST['path'] ) {
+ return add_query_arg( array( 'p' => '/page' ), remove_query_arg( 'path' ) );
+ }
+
+ if ( isset( $_REQUEST['path'] ) && '/wp_template' === $_REQUEST['path'] ) {
+ return add_query_arg( array( 'p' => '/template' ), remove_query_arg( 'path' ) );
+ }
+
+ if ( isset( $_REQUEST['path'] ) && '/patterns' === $_REQUEST['path'] ) {
+ return add_query_arg( array( 'p' => '/pattern' ), remove_query_arg( 'path' ) );
+ }
+
+ if ( isset( $_REQUEST['path'] ) && '/navigation' === $_REQUEST['path'] ) {
+ return add_query_arg( array( 'p' => '/navigation' ), remove_query_arg( 'path' ) );
+ }
+
+ return add_query_arg( array( 'p' => '/' ) );
+}
+
+function gutenberg_redirect_site_editor_deprecated_urls() {
+ $redirection = gutenberg_get_site_editor_redirection();
+ if ( false !== $redirection ) {
+ wp_redirect( $redirection, 301 );
+ exit;
+ }
+}
+add_action( 'admin_init', 'gutenberg_redirect_site_editor_deprecated_urls' );
+
+/**
+ * Filter the `wp_die_handler` to allow access to the Site Editor's new pages page
+ * for Classic themes.
+ *
+ * site-editor.php's access is forbidden for hybrid/classic themes and only allowed with some very special query args (some very special pages like template parts...).
+ * The only way to disable this protection since we're changing the urls in Gutenberg is to override the wp_die_handler.
+ *
+ * @param callable $default_handler The default handler.
+ * @return callable The default handler or a custom handler.
+ */
+function gutenberg_styles_wp_die_handler( $default_handler ) {
+ if ( ! wp_is_block_theme() && str_contains( $_SERVER['REQUEST_URI'], 'site-editor.php' ) && current_user_can( 'edit_theme_options' ) ) {
+ return '__return_false';
+ }
+ return $default_handler;
+}
+add_filter( 'wp_die_handler', 'gutenberg_styles_wp_die_handler' );
+
+/**
+ * Add a Styles submenu under the Appearance menu
+ * for Classic themes.
+ *
+ * @global array $submenu
+ */
+function gutenberg_add_styles_submenu_item() {
+ if ( ! wp_is_block_theme() && ( current_theme_supports( 'editor-styles' ) || wp_theme_has_theme_json() ) ) {
+ global $submenu;
+
+ $styles_menu_item = array(
+ __( 'Design', 'gutenberg' ),
+ 'edit_theme_options',
+ 'site-editor.php',
+ );
+ // If $submenu exists, insert the Styles submenu item at position 2.
+ if ( $submenu && isset( $submenu['themes.php'] ) ) {
+ // This might not work as expected if the submenu has already been modified.
+ array_splice( $submenu['themes.php'], 1, 1, array( $styles_menu_item ) );
+ }
+ }
+}
+add_action( 'admin_init', 'gutenberg_add_styles_submenu_item' );
diff --git a/lib/experimental/blocks.php b/lib/experimental/blocks.php
index 68113276ec1c0..b4bf0d409b159 100644
--- a/lib/experimental/blocks.php
+++ b/lib/experimental/blocks.php
@@ -117,3 +117,22 @@ function gutenberg_register_block_style( $block_name, $style_properties ) {
return $result;
}
+
+/**
+ * Additional data to expose to the view script module in the Form block.
+ */
+function gutenberg_block_core_form_view_script_module( $data ) {
+ if ( ! gutenberg_is_experiment_enabled( 'gutenberg-form-blocks' ) ) {
+ return $data;
+ }
+
+ $data['nonce'] = wp_create_nonce( 'wp-block-form' );
+ $data['ajaxUrl'] = admin_url( 'admin-ajax.php' );
+ $data['action'] = 'wp_block_form_email_submit';
+
+ return $data;
+}
+add_filter(
+ 'script_module_data_@wordpress/block-library/form/view',
+ 'gutenberg_block_core_form_view_script_module'
+);
diff --git a/lib/experimental/editor-settings.php b/lib/experimental/editor-settings.php
index afc6d7e220f67..5b36c32b3c829 100644
--- a/lib/experimental/editor-settings.php
+++ b/lib/experimental/editor-settings.php
@@ -37,6 +37,9 @@ function gutenberg_enable_experiments() {
if ( $gutenberg_experiments && array_key_exists( 'gutenberg-media-processing', $gutenberg_experiments ) ) {
wp_add_inline_script( 'wp-block-editor', 'window.__experimentalMediaProcessing = true', 'before' );
}
+ if ( $gutenberg_experiments && array_key_exists( 'gutenberg-editor-write-mode', $gutenberg_experiments ) ) {
+ wp_add_inline_script( 'wp-block-editor', 'window.__experimentalEditorWriteMode = true', 'before' );
+ }
}
add_action( 'admin_init', 'gutenberg_enable_experiments' );
diff --git a/lib/experimental/posts/load.php b/lib/experimental/posts/load.php
index 7321392b11a25..699534f1886f5 100644
--- a/lib/experimental/posts/load.php
+++ b/lib/experimental/posts/load.php
@@ -69,18 +69,6 @@ function gutenberg_posts_dashboard() {
echo '
';
}
-/**
- * Redirects to the new posts dashboard page and adds the postType query arg.
- */
-function gutenberg_add_post_type_arg() {
- global $pagenow;
- if ( 'admin.php' === $pagenow && isset( $_GET['page'] ) && 'gutenberg-posts-dashboard' === $_GET['page'] && empty( $_GET['postType'] ) ) {
- wp_redirect( admin_url( '/admin.php?page=gutenberg-posts-dashboard&postType=post' ) );
- exit;
- }
-}
-add_action( 'admin_init', 'gutenberg_add_post_type_arg' );
-
/**
* Replaces the default posts menu item with the new posts dashboard.
*/
diff --git a/lib/experiments-page.php b/lib/experiments-page.php
index 946b68283a3e0..c308b7cb5d403 100644
--- a/lib/experiments-page.php
+++ b/lib/experiments-page.php
@@ -44,146 +44,158 @@ function gutenberg_initialize_experiments_settings() {
);
add_settings_field(
- 'gutenberg-sync-collaboration',
- __( 'Live Collaboration and offline persistence', 'gutenberg' ),
+ 'gutenberg-block-experiments',
+ __( 'Blocks: add experimental blocks', 'gutenberg' ),
'gutenberg_display_experiment_field',
'gutenberg-experiments',
'gutenberg_experiments_section',
array(
- 'label' => __( 'Enable the live collaboration and offline persistence between peers', 'gutenberg' ),
- 'id' => 'gutenberg-sync-collaboration',
+ 'label' => __( 'Enables experimental blocks on a rolling basis as they are developed.
(Warning: these blocks may have significant changes during development that cause validation errors and display issues.)
', 'gutenberg' ),
+ 'id' => 'gutenberg-block-experiments',
)
);
add_settings_field(
- 'gutenberg-custom-dataviews',
- __( 'Custom dataviews', 'gutenberg' ),
+ 'gutenberg-form-blocks',
+ __( 'Blocks: add Form and input blocks', 'gutenberg' ),
'gutenberg_display_experiment_field',
'gutenberg-experiments',
'gutenberg_experiments_section',
array(
- 'label' => __( 'Test the custom dataviews in the pages page.', 'gutenberg' ),
- 'id' => 'gutenberg-custom-dataviews',
+ 'label' => __( 'Enables new blocks to allow building forms. You are likely to experience UX issues that are being addressed.', 'gutenberg' ),
+ 'id' => 'gutenberg-form-blocks',
)
);
add_settings_field(
- 'gutenberg-color-randomizer',
- __( 'Color randomizer ', 'gutenberg' ),
+ 'gutenberg-grid-interactivity',
+ __( 'Blocks: add Grid interactivity', 'gutenberg' ),
'gutenberg_display_experiment_field',
'gutenberg-experiments',
'gutenberg_experiments_section',
array(
- 'label' => __( 'Test the Global Styles color randomizer; a utility that lets you mix the current color palette pseudo-randomly.', 'gutenberg' ),
- 'id' => 'gutenberg-color-randomizer',
+ 'label' => __( 'Enables enhancements to the Grid block that let you move and resize items in the editor canvas.', 'gutenberg' ),
+ 'id' => 'gutenberg-grid-interactivity',
)
);
add_settings_field(
- 'gutenberg-block-experiments',
- __( 'Experimental blocks', 'gutenberg' ),
+ 'gutenberg-no-tinymce',
+ __( 'Blocks: disable TinyMCE and Classic block', 'gutenberg' ),
'gutenberg_display_experiment_field',
'gutenberg-experiments',
'gutenberg_experiments_section',
array(
- 'label' => __( 'Enable experimental blocks.
(Warning: these blocks may have significant changes during development that cause validation errors and display issues.)
', 'gutenberg' ),
- 'id' => 'gutenberg-block-experiments',
+ 'label' => __( 'Disables the TinyMCE and Classic block', 'gutenberg' ),
+ 'id' => 'gutenberg-no-tinymce',
)
);
add_settings_field(
- 'gutenberg-form-blocks',
- __( 'Form and input blocks', 'gutenberg' ),
+ 'gutenberg-media-processing',
+ __( 'Client-side media processing', 'gutenberg' ),
'gutenberg_display_experiment_field',
'gutenberg-experiments',
'gutenberg_experiments_section',
array(
- 'label' => __( 'Test new blocks to allow building forms (Warning: The new feature is not ready. You may experience UX issues that are being addressed)', 'gutenberg' ),
- 'id' => 'gutenberg-form-blocks',
+ 'label' => __( 'Enables client-side media processing to leverage the browser\'s capabilities to handle tasks like image resizing and compression.', 'gutenberg' ),
+ 'id' => 'gutenberg-media-processing',
)
);
add_settings_field(
- 'gutenberg-grid-interactivity',
- __( 'Grid interactivity', 'gutenberg' ),
+ 'gutenberg-block-comment',
+ __( 'Collaboration: add block level comments', 'gutenberg' ),
'gutenberg_display_experiment_field',
'gutenberg-experiments',
'gutenberg_experiments_section',
array(
- 'label' => __( 'Test enhancements to the Grid block that let you move and resize items in the editor canvas.', 'gutenberg' ),
- 'id' => 'gutenberg-grid-interactivity',
+ 'label' => __( 'Enables multi-user block level commenting.', 'gutenberg' ),
+ 'id' => 'gutenberg-block-comment',
)
);
add_settings_field(
- 'gutenberg-no-tinymce',
- __( 'Disable TinyMCE and Classic block', 'gutenberg' ),
+ 'gutenberg-sync-collaboration',
+ __( 'Collaboration: add real time editing', 'gutenberg' ),
'gutenberg_display_experiment_field',
'gutenberg-experiments',
'gutenberg_experiments_section',
array(
- 'label' => __( 'Disable TinyMCE and Classic block', 'gutenberg' ),
- 'id' => 'gutenberg-no-tinymce',
+ 'label' => __( 'Enables live collaboration and offline persistence between peers.', 'gutenberg' ),
+ 'id' => 'gutenberg-sync-collaboration',
)
);
add_settings_field(
- 'gutenberg-full-page-client-side-navigation',
- __( 'Enable full page client-side navigation', 'gutenberg' ),
+ 'gutenberg-color-randomizer',
+ __( 'Color randomizer', 'gutenberg' ),
'gutenberg_display_experiment_field',
'gutenberg-experiments',
'gutenberg_experiments_section',
array(
- 'label' => __( 'Enable full page client-side navigation using the Interactivity API', 'gutenberg' ),
- 'id' => 'gutenberg-full-page-client-side-navigation',
+ 'label' => __( 'Enables the Global Styles color randomizer in the Site Editor; a utility that lets you mix the current color palette pseudo-randomly.', 'gutenberg' ),
+ 'id' => 'gutenberg-color-randomizer',
+ )
+ );
+
+ add_settings_field(
+ 'gutenberg-custom-dataviews',
+ __( 'Data Views: add Custom Views', 'gutenberg' ),
+ 'gutenberg_display_experiment_field',
+ 'gutenberg-experiments',
+ 'gutenberg_experiments_section',
+ array(
+ 'label' => __( 'Enables the ability to add, edit, and save custom views when in the Site Editor.', 'gutenberg' ),
+ 'id' => 'gutenberg-custom-dataviews',
)
);
add_settings_field(
'gutenberg-new-posts-dashboard',
- __( 'Redesigned posts dashboard', 'gutenberg' ),
+ __( 'Data Views: enable for Posts', 'gutenberg' ),
'gutenberg_display_experiment_field',
'gutenberg-experiments',
'gutenberg_experiments_section',
array(
- 'label' => __( 'Enable a redesigned posts dashboard.', 'gutenberg' ),
+ 'label' => __( 'Enables a redesigned posts dashboard accessible through a submenu item in the Gutenberg plugin.', 'gutenberg' ),
'id' => 'gutenberg-new-posts-dashboard',
)
);
add_settings_field(
'gutenberg-quick-edit-dataviews',
- __( 'Quick Edit in DataViews', 'gutenberg' ),
+ __( 'Data Views: add Quick Edit', 'gutenberg' ),
'gutenberg_display_experiment_field',
'gutenberg-experiments',
'gutenberg_experiments_section',
array(
- 'label' => __( 'Allow access to a quick edit panel in the pages data views.', 'gutenberg' ),
+ 'label' => __( 'Enables access to a Quick Edit panel in the Site Editor Pages experience.', 'gutenberg' ),
'id' => 'gutenberg-quick-edit-dataviews',
)
);
add_settings_field(
- 'gutenberg-block-comment',
- __( 'Block Comments', 'gutenberg' ),
+ 'gutenberg-full-page-client-side-navigation',
+ __( 'iAPI: full page client side navigation', 'gutenberg' ),
'gutenberg_display_experiment_field',
'gutenberg-experiments',
'gutenberg_experiments_section',
array(
- 'label' => __( 'Enable multi-user commenting on blocks', 'gutenberg' ),
- 'id' => 'gutenberg-block-comment',
+ 'label' => __( 'Enables full-page client-side navigation with the Interactivity API, updating HTML while preserving application state.', 'gutenberg' ),
+ 'id' => 'gutenberg-full-page-client-side-navigation',
)
);
add_settings_field(
- 'gutenberg-media-processing',
- __( 'Client-side media processing', 'gutenberg' ),
+ 'gutenberg-editor-write-mode',
+ __( 'Simplified site editing', 'gutenberg' ),
'gutenberg_display_experiment_field',
'gutenberg-experiments',
'gutenberg_experiments_section',
array(
- 'label' => __( 'Enable client-side media processing.', 'gutenberg' ),
- 'id' => 'gutenberg-media-processing',
+ 'label' => __( 'Enables Write mode in the Site Editor for a simplified editing experience.', 'gutenberg' ),
+ 'id' => 'gutenberg-editor-write-mode',
)
);
diff --git a/lib/global-styles-and-settings.php b/lib/global-styles-and-settings.php
index c4446cf29cf01..3ff5e6cb135e1 100644
--- a/lib/global-styles-and-settings.php
+++ b/lib/global-styles-and-settings.php
@@ -9,7 +9,7 @@
* Returns the stylesheet resulting of merging core, theme, and user data.
*
* @param array $types Types of styles to load. Optional.
- * It accepts as values: 'variables', 'presets', 'styles', 'base-layout-styles.
+ * See {@see 'WP_Theme_JSON::get_stylesheet'} for all valid types.
* If empty, it'll load the following:
* - for themes without theme.json: 'variables', 'presets', 'base-layout-styles'.
* - for themes with theme.json: 'variables', 'presets', 'styles'.
@@ -142,6 +142,8 @@ function gutenberg_get_global_settings( $path = array(), $context = array() ) {
/**
* Gets the global styles custom css from theme.json.
*
+ * @deprecated Gutenberg 18.6.0 Use {@see 'gutenberg_get_global_stylesheet'} instead for top-level custom CSS, or {@see 'WP_Theme_JSON_Gutenberg::get_styles_for_block'} for block-level custom CSS.
+ *
* @return string
*/
function gutenberg_get_global_styles_custom_css() {
diff --git a/lib/load.php b/lib/load.php
index d7e4a33cd02c9..26af78f3173c5 100644
--- a/lib/load.php
+++ b/lib/load.php
@@ -35,11 +35,6 @@ function gutenberg_is_experiment_enabled( $name ) {
require_once __DIR__ . '/experimental/class-wp-rest-block-editor-settings-controller.php';
}
- // WordPress 6.6 compat.
- require __DIR__ . '/compat/wordpress-6.6/class-gutenberg-rest-global-styles-revisions-controller-6-6.php';
- require __DIR__ . '/compat/wordpress-6.6/class-gutenberg-rest-templates-controller-6-6.php';
- require __DIR__ . '/compat/wordpress-6.6/rest-api.php';
-
// WordPress 6.7 compat.
require __DIR__ . '/compat/wordpress-6.7/class-gutenberg-rest-posts-controller-6-7.php';
require __DIR__ . '/compat/wordpress-6.7/class-gutenberg-rest-templates-controller-6-7.php';
@@ -49,6 +44,8 @@ function gutenberg_is_experiment_enabled( $name ) {
// WordPress 6.8 compat.
require __DIR__ . '/compat/wordpress-6.8/block-comments.php';
require __DIR__ . '/compat/wordpress-6.8/class-gutenberg-rest-comment-controller-6-8.php';
+ require __DIR__ . '/compat/wordpress-6.8/class-gutenberg-rest-post-types-controller-6-8.php';
+ require __DIR__ . '/compat/wordpress-6.8/rest-api.php';
// Plugin specific code.
require_once __DIR__ . '/class-wp-rest-global-styles-controller-gutenberg.php';
@@ -70,18 +67,9 @@ function gutenberg_is_experiment_enabled( $name ) {
require __DIR__ . '/compat/plugin/edit-site-routes-backwards-compat.php';
require __DIR__ . '/compat/plugin/fonts.php';
-// The Token Map was created during 6.6 in order to support the HTML API. It must be loaded before it.
-require __DIR__ . '/compat/wordpress-6.6/class-gutenberg-token-map-6-6.php';
+// The Token Map was created to support the HTML API. It must be loaded before it.
require __DIR__ . '/compat/wordpress-6.7/class-gutenberg-token-map-6-7.php';
-require __DIR__ . '/compat/wordpress-6.6/html-api/gutenberg-html5-named-character-references-6-6.php';
-require __DIR__ . '/compat/wordpress-6.6/html-api/class-gutenberg-html-decoder-6-6.php';
-require __DIR__ . '/compat/wordpress-6.6/html-api/class-gutenberg-html-tag-processor-6-6.php';
-require __DIR__ . '/compat/wordpress-6.6/html-api/class-gutenberg-html-open-elements-6-6.php';
-require __DIR__ . '/compat/wordpress-6.6/html-api/class-gutenberg-html-stack-event-6-6.php';
-require __DIR__ . '/compat/wordpress-6.6/html-api/class-gutenberg-html-processor-state-6-6.php';
-require __DIR__ . '/compat/wordpress-6.6/html-api/class-gutenberg-html-processor-6-6.php';
-
// Type annotations were added in 6.7 so every file is updated.
require __DIR__ . '/compat/wordpress-6.7/html-api/class-gutenberg-html-active-formatting-elements-6-7.php';
require __DIR__ . '/compat/wordpress-6.7/html-api/class-gutenberg-html-attribute-token-6-7.php';
@@ -96,17 +84,6 @@ function gutenberg_is_experiment_enabled( $name ) {
require __DIR__ . '/compat/wordpress-6.7/html-api/class-gutenberg-html-processor-state-6-7.php';
require __DIR__ . '/compat/wordpress-6.7/html-api/class-gutenberg-html-processor-6-7.php';
-// WordPress 6.6 compat.
-require __DIR__ . '/compat/wordpress-6.6/admin-bar.php';
-require __DIR__ . '/compat/wordpress-6.6/blocks.php';
-require __DIR__ . '/compat/wordpress-6.6/block-editor.php';
-require __DIR__ . '/compat/wordpress-6.6/compat.php';
-require __DIR__ . '/compat/wordpress-6.6/resolve-patterns.php';
-require __DIR__ . '/compat/wordpress-6.6/block-bindings/pattern-overrides.php';
-require __DIR__ . '/compat/wordpress-6.6/block-template-utils.php';
-require __DIR__ . '/compat/wordpress-6.6/option.php';
-require __DIR__ . '/compat/wordpress-6.6/post.php';
-
// WordPress 6.7 compat.
require __DIR__ . '/compat/wordpress-6.7/block-templates.php';
require __DIR__ . '/compat/wordpress-6.7/blocks.php';
@@ -120,6 +97,9 @@ function gutenberg_is_experiment_enabled( $name ) {
require __DIR__ . '/compat/wordpress-6.8/preload.php';
require __DIR__ . '/compat/wordpress-6.8/blocks.php';
require __DIR__ . '/compat/wordpress-6.8/functions.php';
+require __DIR__ . '/compat/wordpress-6.8/post.php';
+require __DIR__ . '/compat/wordpress-6.8/site-editor.php';
+require __DIR__ . '/compat/wordpress-6.8/class-gutenberg-rest-user-controller.php';
// Experimental features.
require __DIR__ . '/experimental/block-editor-settings-mobile.php';
diff --git a/lib/rest-api.php b/lib/rest-api.php
index 7570bb1973723..424927acf1f4a 100644
--- a/lib/rest-api.php
+++ b/lib/rest-api.php
@@ -19,11 +19,10 @@
* @return array Array of arguments for registering a post type.
*/
function gutenberg_override_global_styles_endpoint( array $args ): array {
- $args['rest_controller_class'] = 'WP_REST_Global_Styles_Controller_Gutenberg';
- $args['revisions_rest_controller_class'] = 'Gutenberg_REST_Global_Styles_Revisions_Controller_6_6';
- $args['late_route_registration'] = true;
- $args['show_in_rest'] = true;
- $args['rest_base'] = 'global-styles';
+ $args['rest_controller_class'] = 'WP_REST_Global_Styles_Controller_Gutenberg';
+ $args['late_route_registration'] = true;
+ $args['show_in_rest'] = true;
+ $args['rest_base'] = 'global-styles';
return $args;
}
diff --git a/package-lock.json b/package-lock.json
index 0bcf8aee842fd..e2063a35c7d0a 100644
--- a/package-lock.json
+++ b/package-lock.json
@@ -1,12 +1,12 @@
{
"name": "gutenberg",
- "version": "19.7.0-rc.1",
+ "version": "19.9.0-rc.1",
"lockfileVersion": 3,
"requires": true,
"packages": {
"": {
"name": "gutenberg",
- "version": "19.7.0-rc.1",
+ "version": "19.9.0-rc.1",
"hasInstallScript": true,
"license": "GPL-2.0-or-later",
"workspaces": [
@@ -16,7 +16,7 @@
"@actions/core": "1.9.1",
"@actions/github": "5.0.0",
"@apidevtools/json-schema-ref-parser": "11.6.4",
- "@ariakit/test": "^0.4.2",
+ "@ariakit/test": "^0.4.7",
"@babel/core": "7.25.7",
"@babel/plugin-syntax-jsx": "7.25.7",
"@babel/runtime-corejs3": "7.25.7",
@@ -24,7 +24,7 @@
"@emotion/babel-plugin": "11.11.0",
"@emotion/jest": "11.7.1",
"@emotion/native": "11.0.0",
- "@geometricpanda/storybook-addon-badges": "2.0.1",
+ "@geometricpanda/storybook-addon-badges": "2.0.5",
"@octokit/rest": "16.26.0",
"@octokit/types": "6.34.0",
"@octokit/webhooks-types": "5.8.0",
@@ -33,16 +33,19 @@
"@react-native/babel-preset": "0.73.10",
"@react-native/metro-babel-transformer": "0.73.10",
"@react-native/metro-config": "0.73.4",
- "@storybook/addon-a11y": "7.6.15",
- "@storybook/addon-actions": "7.6.15",
- "@storybook/addon-controls": "7.6.15",
- "@storybook/addon-docs": "7.6.15",
- "@storybook/addon-toolbars": "7.6.15",
- "@storybook/addon-viewport": "7.6.15",
- "@storybook/react": "7.6.15",
- "@storybook/react-webpack5": "7.6.15",
- "@storybook/source-loader": "7.6.15",
- "@storybook/theming": "7.6.15",
+ "@storybook/addon-a11y": "8.4.7",
+ "@storybook/addon-actions": "8.4.7",
+ "@storybook/addon-controls": "8.4.7",
+ "@storybook/addon-docs": "8.4.7",
+ "@storybook/addon-toolbars": "8.4.7",
+ "@storybook/addon-viewport": "8.4.7",
+ "@storybook/addon-webpack5-compiler-babel": "3.0.3",
+ "@storybook/react": "8.4.7",
+ "@storybook/react-webpack5": "8.4.7",
+ "@storybook/source-loader": "8.4.7",
+ "@storybook/test": "8.4.7",
+ "@storybook/theming": "8.4.7",
+ "@storybook/types": "8.4.7",
"@testing-library/jest-dom": "5.16.5",
"@testing-library/react": "14.3.0",
"@testing-library/react-native": "12.4.3",
@@ -78,19 +81,21 @@
"commander": "9.2.0",
"concurrently": "3.5.0",
"copy-webpack-plugin": "10.2.0",
- "core-js-builder": "3.38.1",
- "cross-env": "3.2.4",
+ "core-js-builder": "3.39.0",
+ "cross-env": "7.0.3",
"css-loader": "6.2.0",
"cssnano": "6.0.1",
"deep-freeze": "0.0.1",
"equivalent-key-map": "0.2.2",
+ "esbuild": "0.18.20",
"escape-html": "1.0.3",
"eslint-import-resolver-node": "0.3.4",
"eslint-plugin-eslint-comments": "3.1.2",
"eslint-plugin-import": "2.25.2",
- "eslint-plugin-jest": "27.2.3",
+ "eslint-plugin-jest": "27.4.3",
"eslint-plugin-jest-dom": "5.0.2",
"eslint-plugin-prettier": "5.0.0",
+ "eslint-plugin-react-compiler": "19.0.0-beta-0dec889-20241115",
"eslint-plugin-ssr-friendly": "1.0.6",
"eslint-plugin-storybook": "0.6.13",
"eslint-plugin-testing-library": "6.0.2",
@@ -107,7 +112,7 @@
"jest-message-util": "29.6.2",
"jest-watch-typeahead": "2.2.2",
"json2md": "2.0.1",
- "lerna": "7.1.4",
+ "lerna": "8.1.9",
"lint-staged": "10.0.2",
"make-dir": "3.0.0",
"mkdirp": "3.0.1",
@@ -119,12 +124,11 @@
"npm-run-all": "4.1.5",
"patch-package": "8.0.0",
"postcss": "8.4.38",
- "postcss-import": "16.1.0",
"postcss-loader": "6.2.1",
"postcss-local-keyframes": "^0.0.2",
"prettier": "npm:wp-prettier@3.0.3",
"progress": "2.0.3",
- "puppeteer-core": "23.1.0",
+ "puppeteer-core": "23.10.1",
"raw-loader": "4.0.2",
"react": "18.3.1",
"react-docgen-typescript": "2.2.2",
@@ -137,25 +141,25 @@
"reassure": "0.7.1",
"redux": "5.0.1",
"resize-observer-polyfill": "1.5.1",
- "rimraf": "3.0.2",
+ "rimraf": "5.0.10",
"rtlcss": "4.0.0",
- "sass": "1.35.2",
- "sass-loader": "12.1.0",
+ "sass": "1.50.1",
+ "sass-loader": "16.0.3",
"semver": "7.5.4",
"simple-git": "3.24.0",
"snapshot-diff": "0.10.0",
"source-map-loader": "3.0.0",
"sprintf-js": "1.1.1",
- "storybook": "7.6.15",
+ "storybook": "8.4.7",
"storybook-source-link": "2.0.9",
"strip-json-comments": "5.0.0",
"style-loader": "3.2.1",
"terser": "5.32.0",
"terser-webpack-plugin": "5.3.10",
- "typescript": "5.5.3",
+ "typescript": "5.7.2",
"uuid": "9.0.1",
"webdriverio": "8.16.20",
- "webpack": "5.95.0",
+ "webpack": "5.97.0",
"webpack-bundle-analyzer": "4.9.1",
"worker-farm": "1.7.0"
},
@@ -497,6 +501,22 @@
"integrity": "sha512-JLyh7xT1kizaEvcaXOQwOc2/Yhw6KZOvPf1S8401UyLk86CU79LN3vl7ztXGm/pZ+YjoyAJ4rxmHwbkBXJX+yw==",
"dev": true
},
+ "node_modules/@appium/base-driver/node_modules/qs": {
+ "version": "6.11.0",
+ "resolved": "https://registry.npmjs.org/qs/-/qs-6.11.0.tgz",
+ "integrity": "sha512-MvjoMCJwEarSbUYk5O+nmoSzSutSsTwF85zcHPQ9OrlFoZOYIjaqBAJIqIXjptyD5vThxGq52Xu/MaJzRkIk4Q==",
+ "dev": true,
+ "license": "BSD-3-Clause",
+ "dependencies": {
+ "side-channel": "^1.0.4"
+ },
+ "engines": {
+ "node": ">=0.6"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/ljharb"
+ }
+ },
"node_modules/@appium/base-driver/node_modules/raw-body": {
"version": "2.5.2",
"resolved": "https://registry.npmjs.org/raw-body/-/raw-body-2.5.2.tgz",
@@ -596,20 +616,6 @@
"url": "https://github.com/chalk/chalk?sponsor=1"
}
},
- "node_modules/@appium/docutils/node_modules/cliui": {
- "version": "8.0.1",
- "resolved": "https://registry.npmjs.org/cliui/-/cliui-8.0.1.tgz",
- "integrity": "sha512-BSeNnyus75C4//NQ9gQt1/csTXyo/8Sb+afLAkzAptFuMsod9HFokGNudZpi/oQV73hnVK+sR+5PVRMd+Dr7YQ==",
- "dev": true,
- "dependencies": {
- "string-width": "^4.2.0",
- "strip-ansi": "^6.0.1",
- "wrap-ansi": "^7.0.0"
- },
- "engines": {
- "node": ">=12"
- }
- },
"node_modules/@appium/docutils/node_modules/diff": {
"version": "5.1.0",
"resolved": "https://registry.npmjs.org/diff/-/diff-5.1.0.tgz",
@@ -619,12 +625,6 @@
"node": ">=0.3.1"
}
},
- "node_modules/@appium/docutils/node_modules/emoji-regex": {
- "version": "8.0.0",
- "resolved": "https://registry.npmjs.org/emoji-regex/-/emoji-regex-8.0.0.tgz",
- "integrity": "sha512-MSjYzcWNOA0ewAHpz0MxpYFvwg6yjy1NG3xteoqz644VCo/RPgnr1/GGt+ic3iJTzQ8Eu3TdM14SawnVUmGE6A==",
- "dev": true
- },
"node_modules/@appium/docutils/node_modules/figures": {
"version": "3.2.0",
"resolved": "https://registry.npmjs.org/figures/-/figures-3.2.0.tgz",
@@ -665,15 +665,6 @@
"node": ">=8"
}
},
- "node_modules/@appium/docutils/node_modules/is-fullwidth-code-point": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/is-fullwidth-code-point/-/is-fullwidth-code-point-3.0.0.tgz",
- "integrity": "sha512-zymm5+u+sCsSWyD9qNaejV3DFvhCKclKdizYaJUuHA83RLjb7nSuGnddCHGv0hk+KY7BMAlsWeK4Ueg6EV6XQg==",
- "dev": true,
- "engines": {
- "node": ">=8"
- }
- },
"node_modules/@appium/docutils/node_modules/locate-path": {
"version": "6.0.0",
"resolved": "https://registry.npmjs.org/locate-path/-/locate-path-6.0.0.tgz",
@@ -725,20 +716,6 @@
"node": ">=10"
}
},
- "node_modules/@appium/docutils/node_modules/string-width": {
- "version": "4.2.3",
- "resolved": "https://registry.npmjs.org/string-width/-/string-width-4.2.3.tgz",
- "integrity": "sha512-wKyQRQpjJ0sIp62ErSZdGsjMJWsap5oRNihHhu6G7JVO/9jIB6UyevL+tXuOqrng8j/cxKTWyWUwvSTriiZz/g==",
- "dev": true,
- "dependencies": {
- "emoji-regex": "^8.0.0",
- "is-fullwidth-code-point": "^3.0.0",
- "strip-ansi": "^6.0.1"
- },
- "engines": {
- "node": ">=8"
- }
- },
"node_modules/@appium/docutils/node_modules/supports-color": {
"version": "7.2.0",
"resolved": "https://registry.npmjs.org/supports-color/-/supports-color-7.2.0.tgz",
@@ -792,32 +769,6 @@
"node": ">=12.20"
}
},
- "node_modules/@appium/docutils/node_modules/wrap-ansi": {
- "version": "7.0.0",
- "resolved": "https://registry.npmjs.org/wrap-ansi/-/wrap-ansi-7.0.0.tgz",
- "integrity": "sha512-YVGIj2kamLSTxw6NsZjoBxfSwsn0ycdesmc4p+Q21c5zPuZ1pl+NfxVdxPtdHvmNVOQ6XSYG4AUtyt/Fi7D16Q==",
- "dev": true,
- "dependencies": {
- "ansi-styles": "^4.0.0",
- "string-width": "^4.1.0",
- "strip-ansi": "^6.0.0"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/chalk/wrap-ansi?sponsor=1"
- }
- },
- "node_modules/@appium/docutils/node_modules/y18n": {
- "version": "5.0.8",
- "resolved": "https://registry.npmjs.org/y18n/-/y18n-5.0.8.tgz",
- "integrity": "sha512-0pfFzegeDWJHJIAmTLRP2DwHjdF5s7jo9tuztdQxAhINCdvS+3nGINqPd00AphqJR/0LhANUS6/+7SCb98YOfA==",
- "dev": true,
- "engines": {
- "node": ">=10"
- }
- },
"node_modules/@appium/docutils/node_modules/yaml": {
"version": "2.3.3",
"resolved": "https://registry.npmjs.org/yaml/-/yaml-2.3.3.tgz",
@@ -827,24 +778,6 @@
"node": ">= 14"
}
},
- "node_modules/@appium/docutils/node_modules/yargs": {
- "version": "17.7.2",
- "resolved": "https://registry.npmjs.org/yargs/-/yargs-17.7.2.tgz",
- "integrity": "sha512-7dSzzRQ++CKnNI/krKnYRV7JKKPUXMEh61soaHKg9mrWEhzFWhFnxPxGl+69cD1Ou63C13NUPCnmIcrvqCuM6w==",
- "dev": true,
- "dependencies": {
- "cliui": "^8.0.1",
- "escalade": "^3.1.1",
- "get-caller-file": "^2.0.5",
- "require-directory": "^2.1.1",
- "string-width": "^4.2.3",
- "y18n": "^5.0.5",
- "yargs-parser": "^21.1.1"
- },
- "engines": {
- "node": ">=12"
- }
- },
"node_modules/@appium/docutils/node_modules/yargs-parser": {
"version": "21.1.1",
"resolved": "https://registry.npmjs.org/yargs-parser/-/yargs-parser-21.1.1.tgz",
@@ -1168,6 +1101,25 @@
"node": ">=16"
}
},
+ "node_modules/@appium/support/node_modules/jackspeak": {
+ "version": "2.3.6",
+ "resolved": "https://registry.npmjs.org/jackspeak/-/jackspeak-2.3.6.tgz",
+ "integrity": "sha512-N3yCS/NegsOBokc8GAdM8UcmfsKiSS8cipheD/nivzr700H+nsMOxJjQnvwOcRYVuFkdH0wGUvW2WbXGmrZGbQ==",
+ "dev": true,
+ "license": "BlueOak-1.0.0",
+ "dependencies": {
+ "@isaacs/cliui": "^8.0.2"
+ },
+ "engines": {
+ "node": ">=14"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
+ },
+ "optionalDependencies": {
+ "@pkgjs/parseargs": "^0.11.0"
+ }
+ },
"node_modules/@appium/support/node_modules/locate-path": {
"version": "6.0.0",
"resolved": "https://registry.npmjs.org/locate-path/-/locate-path-6.0.0.tgz",
@@ -1432,17 +1384,51 @@
}
},
"node_modules/@ariakit/core": {
- "version": "0.4.9",
- "resolved": "https://registry.npmjs.org/@ariakit/core/-/core-0.4.9.tgz",
- "integrity": "sha512-nV0B/OTK/0iB+P9RC7fudznYZ8eR6rR1F912Zc54e3+wSW5RrRvNOiRxyMrgENidd4R7cCMDw77XJLSBLKgEPQ=="
+ "version": "0.4.14",
+ "resolved": "https://registry.npmjs.org/@ariakit/core/-/core-0.4.14.tgz",
+ "integrity": "sha512-hpzZvyYzGhP09S9jW1XGsU/FD5K3BKsH1eG/QJ8rfgEeUdPS7BvHPt5lHbOeJ2cMrRzBEvsEzLi1ivfDifHsVA==",
+ "license": "MIT"
+ },
+ "node_modules/@ariakit/react": {
+ "version": "0.4.15",
+ "resolved": "https://registry.npmjs.org/@ariakit/react/-/react-0.4.15.tgz",
+ "integrity": "sha512-0V2LkNPFrGRT+SEIiObx/LQjR6v3rR+mKEDUu/3tq7jfCZ+7+6Q6EMR1rFaK+XMkaRY1RWUcj/rRDWAUWnsDww==",
+ "license": "MIT",
+ "dependencies": {
+ "@ariakit/react-core": "0.4.15"
+ },
+ "funding": {
+ "type": "opencollective",
+ "url": "https://opencollective.com/ariakit"
+ },
+ "peerDependencies": {
+ "react": "^17.0.0 || ^18.0.0 || ^19.0.0",
+ "react-dom": "^17.0.0 || ^18.0.0 || ^19.0.0"
+ }
+ },
+ "node_modules/@ariakit/react-core": {
+ "version": "0.4.15",
+ "resolved": "https://registry.npmjs.org/@ariakit/react-core/-/react-core-0.4.15.tgz",
+ "integrity": "sha512-Up8+U97nAPJdyUh9E8BCEhJYTA+eVztWpHoo1R9zZfHd4cnBWAg5RHxEmMH+MamlvuRxBQA71hFKY/735fDg+A==",
+ "license": "MIT",
+ "dependencies": {
+ "@ariakit/core": "0.4.14",
+ "@floating-ui/dom": "^1.0.0",
+ "use-sync-external-store": "^1.2.0"
+ },
+ "peerDependencies": {
+ "react": "^17.0.0 || ^18.0.0 || ^19.0.0",
+ "react-dom": "^17.0.0 || ^18.0.0 || ^19.0.0"
+ }
},
"node_modules/@ariakit/test": {
- "version": "0.4.2",
- "resolved": "https://registry.npmjs.org/@ariakit/test/-/test-0.4.2.tgz",
- "integrity": "sha512-WXAAiAyTaHV9klntOB81Y+YHyA5iGxy9wXCmjQOfYK5InsuIour+7TVXICUxn2NF0XD6j6OoEJbCVDJ2Y46xEA==",
+ "version": "0.4.7",
+ "resolved": "https://registry.npmjs.org/@ariakit/test/-/test-0.4.7.tgz",
+ "integrity": "sha512-Zb5bnulzYGjr6sDubxOeOhk5Es6BYQq5lbcIe8xNrWUlpRiHsje/FlXNFpHnI92/7ESxH6X4pHhbb+qFAho1lw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "@ariakit/core": "0.4.9",
+ "@ariakit/core": "0.4.14",
"@testing-library/dom": "^8.0.0 || ^9.0.0 || ^10.0.0"
},
"peerDependencies": {
@@ -1462,18 +1448,6 @@
}
}
},
- "node_modules/@aw-web-design/x-default-browser": {
- "version": "1.4.126",
- "resolved": "https://registry.npmjs.org/@aw-web-design/x-default-browser/-/x-default-browser-1.4.126.tgz",
- "integrity": "sha512-Xk1sIhyNC/esHGGVjL/niHLowM0csl/kFO5uawBy4IrWwy0o1G8LGt3jP6nmWGz+USxeeqbihAmp/oVZju6wug==",
- "dev": true,
- "dependencies": {
- "default-browser-id": "3.0.0"
- },
- "bin": {
- "x-default-browser": "bin/x-default-browser.js"
- }
- },
"node_modules/@axe-core/puppeteer": {
"version": "4.0.0",
"resolved": "https://registry.npmjs.org/@axe-core/puppeteer/-/puppeteer-4.0.0.tgz",
@@ -2479,6 +2453,23 @@
"@babel/core": "^7.0.0-0"
}
},
+ "node_modules/@babel/plugin-proposal-private-methods": {
+ "version": "7.18.6",
+ "resolved": "https://registry.npmjs.org/@babel/plugin-proposal-private-methods/-/plugin-proposal-private-methods-7.18.6.tgz",
+ "integrity": "sha512-nutsvktDItsNn4rpGItSNV2sz1XwS+nfU0Rg8aCx3W3NOKVzdMjJRu0O5OkgDp3ZGICSTbgRpxZoWsxoKRvbeA==",
+ "deprecated": "This proposal has been merged to the ECMAScript standard and thus this plugin is no longer maintained. Please use @babel/plugin-transform-private-methods instead.",
+ "dev": true,
+ "dependencies": {
+ "@babel/helper-create-class-features-plugin": "^7.18.6",
+ "@babel/helper-plugin-utils": "^7.18.6"
+ },
+ "engines": {
+ "node": ">=6.9.0"
+ },
+ "peerDependencies": {
+ "@babel/core": "^7.0.0-0"
+ }
+ },
"node_modules/@babel/plugin-proposal-private-property-in-object": {
"version": "7.21.0-placeholder-for-preset-env.2",
"resolved": "https://registry.npmjs.org/@babel/plugin-proposal-private-property-in-object/-/plugin-proposal-private-property-in-object-7.21.0-placeholder-for-preset-env.2.tgz",
@@ -4160,12 +4151,6 @@
"node": ">=6.9.0"
}
},
- "node_modules/@base2/pretty-print-object": {
- "version": "1.0.1",
- "resolved": "https://registry.npmjs.org/@base2/pretty-print-object/-/pretty-print-object-1.0.1.tgz",
- "integrity": "sha512-4iri8i1AqYHJE2DstZYkyEprg6Pq6sKx3xn5FpySk9sNhH7qN2LLlHJCfDTZRILNwQNPD7mATWM0TBui7uC1pA==",
- "dev": true
- },
"node_modules/@bcoe/v8-coverage": {
"version": "0.2.3",
"resolved": "https://registry.npmjs.org/@bcoe/v8-coverage/-/v8-coverage-0.2.3.tgz",
@@ -4185,102 +4170,6 @@
"reassure": "lib/commonjs/bin.js"
}
},
- "node_modules/@callstack/reassure-cli/node_modules/cliui": {
- "version": "8.0.1",
- "resolved": "https://registry.npmjs.org/cliui/-/cliui-8.0.1.tgz",
- "integrity": "sha512-BSeNnyus75C4//NQ9gQt1/csTXyo/8Sb+afLAkzAptFuMsod9HFokGNudZpi/oQV73hnVK+sR+5PVRMd+Dr7YQ==",
- "dev": true,
- "dependencies": {
- "string-width": "^4.2.0",
- "strip-ansi": "^6.0.1",
- "wrap-ansi": "^7.0.0"
- },
- "engines": {
- "node": ">=12"
- }
- },
- "node_modules/@callstack/reassure-cli/node_modules/emoji-regex": {
- "version": "8.0.0",
- "resolved": "https://registry.npmjs.org/emoji-regex/-/emoji-regex-8.0.0.tgz",
- "integrity": "sha512-MSjYzcWNOA0ewAHpz0MxpYFvwg6yjy1NG3xteoqz644VCo/RPgnr1/GGt+ic3iJTzQ8Eu3TdM14SawnVUmGE6A==",
- "dev": true
- },
- "node_modules/@callstack/reassure-cli/node_modules/is-fullwidth-code-point": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/is-fullwidth-code-point/-/is-fullwidth-code-point-3.0.0.tgz",
- "integrity": "sha512-zymm5+u+sCsSWyD9qNaejV3DFvhCKclKdizYaJUuHA83RLjb7nSuGnddCHGv0hk+KY7BMAlsWeK4Ueg6EV6XQg==",
- "dev": true,
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@callstack/reassure-cli/node_modules/string-width": {
- "version": "4.2.3",
- "resolved": "https://registry.npmjs.org/string-width/-/string-width-4.2.3.tgz",
- "integrity": "sha512-wKyQRQpjJ0sIp62ErSZdGsjMJWsap5oRNihHhu6G7JVO/9jIB6UyevL+tXuOqrng8j/cxKTWyWUwvSTriiZz/g==",
- "dev": true,
- "dependencies": {
- "emoji-regex": "^8.0.0",
- "is-fullwidth-code-point": "^3.0.0",
- "strip-ansi": "^6.0.1"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@callstack/reassure-cli/node_modules/wrap-ansi": {
- "version": "7.0.0",
- "resolved": "https://registry.npmjs.org/wrap-ansi/-/wrap-ansi-7.0.0.tgz",
- "integrity": "sha512-YVGIj2kamLSTxw6NsZjoBxfSwsn0ycdesmc4p+Q21c5zPuZ1pl+NfxVdxPtdHvmNVOQ6XSYG4AUtyt/Fi7D16Q==",
- "dev": true,
- "dependencies": {
- "ansi-styles": "^4.0.0",
- "string-width": "^4.1.0",
- "strip-ansi": "^6.0.0"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/chalk/wrap-ansi?sponsor=1"
- }
- },
- "node_modules/@callstack/reassure-cli/node_modules/y18n": {
- "version": "5.0.8",
- "resolved": "https://registry.npmjs.org/y18n/-/y18n-5.0.8.tgz",
- "integrity": "sha512-0pfFzegeDWJHJIAmTLRP2DwHjdF5s7jo9tuztdQxAhINCdvS+3nGINqPd00AphqJR/0LhANUS6/+7SCb98YOfA==",
- "dev": true,
- "engines": {
- "node": ">=10"
- }
- },
- "node_modules/@callstack/reassure-cli/node_modules/yargs": {
- "version": "17.7.2",
- "resolved": "https://registry.npmjs.org/yargs/-/yargs-17.7.2.tgz",
- "integrity": "sha512-7dSzzRQ++CKnNI/krKnYRV7JKKPUXMEh61soaHKg9mrWEhzFWhFnxPxGl+69cD1Ou63C13NUPCnmIcrvqCuM6w==",
- "dev": true,
- "dependencies": {
- "cliui": "^8.0.1",
- "escalade": "^3.1.1",
- "get-caller-file": "^2.0.5",
- "require-directory": "^2.1.1",
- "string-width": "^4.2.3",
- "y18n": "^5.0.5",
- "yargs-parser": "^21.1.1"
- },
- "engines": {
- "node": ">=12"
- }
- },
- "node_modules/@callstack/reassure-cli/node_modules/yargs-parser": {
- "version": "21.1.1",
- "resolved": "https://registry.npmjs.org/yargs-parser/-/yargs-parser-21.1.1.tgz",
- "integrity": "sha512-tVpsJW7DdjecAiFpbIB1e3qxIQsE6NoPc5/eTdrbbIC4h0LVsWhnoa3g+m2HclBIujHzsxZ4VJVA+GUuc2/LBw==",
- "dev": true,
- "engines": {
- "node": ">=12"
- }
- },
"node_modules/@callstack/reassure-compare": {
"version": "0.3.0",
"resolved": "https://registry.npmjs.org/@callstack/reassure-compare/-/reassure-compare-0.3.0.tgz",
@@ -4453,6 +4342,37 @@
"node": ">=0.8.0"
}
},
+ "node_modules/@emnapi/core": {
+ "version": "1.3.1",
+ "resolved": "https://registry.npmjs.org/@emnapi/core/-/core-1.3.1.tgz",
+ "integrity": "sha512-pVGjBIt1Y6gg3EJN8jTcfpP/+uuRksIo055oE/OBkDNcjZqVbfkWCksG1Jp4yZnj3iKWyWX8fdG/j6UDYPbFog==",
+ "dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "@emnapi/wasi-threads": "1.0.1",
+ "tslib": "^2.4.0"
+ }
+ },
+ "node_modules/@emnapi/runtime": {
+ "version": "1.3.1",
+ "resolved": "https://registry.npmjs.org/@emnapi/runtime/-/runtime-1.3.1.tgz",
+ "integrity": "sha512-kEBmG8KyqtxJZv+ygbEim+KCGtIq1fC22Ms3S4ziXmYKm8uyoLX0MHONVKwp+9opg390VaKRNt4a7A9NwmpNhw==",
+ "dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "tslib": "^2.4.0"
+ }
+ },
+ "node_modules/@emnapi/wasi-threads": {
+ "version": "1.0.1",
+ "resolved": "https://registry.npmjs.org/@emnapi/wasi-threads/-/wasi-threads-1.0.1.tgz",
+ "integrity": "sha512-iIBu7mwkq4UQGeMEM8bLwNK962nXdhodeScX4slfQnRhEMMzvYivHhutCIk8uojvmASXXPC2WNEjwxFWk72Oqw==",
+ "dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "tslib": "^2.4.0"
+ }
+ },
"node_modules/@emotion/babel-plugin": {
"version": "11.11.0",
"resolved": "https://registry.npmjs.org/@emotion/babel-plugin/-/babel-plugin-11.11.0.tgz",
@@ -4716,15 +4636,6 @@
"resolved": "https://registry.npmjs.org/@emotion/unitless/-/unitless-0.8.1.tgz",
"integrity": "sha512-KOEGMu6dmJZtpadb476IsZBclKvILjopjUii3V+7MnXIQCYh8W3NgNcgwo21n9LXZX6EDIKvqfjYxXebDwxKmQ=="
},
- "node_modules/@emotion/use-insertion-effect-with-fallbacks": {
- "version": "1.0.1",
- "resolved": "https://registry.npmjs.org/@emotion/use-insertion-effect-with-fallbacks/-/use-insertion-effect-with-fallbacks-1.0.1.tgz",
- "integrity": "sha512-jT/qyKZ9rzLErtrjGgdkMBn2OP8wl0G3sQlBb3YPryvKHsjvINUhVaPFfP+fpBcOkmrVOVEEHQFJ7nbj2TH2gw==",
- "dev": true,
- "peerDependencies": {
- "react": ">=16.8.0"
- }
- },
"node_modules/@emotion/utils": {
"version": "1.2.1",
"resolved": "https://registry.npmjs.org/@emotion/utils/-/utils-1.2.1.tgz",
@@ -5202,12 +5113,6 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@fal-works/esbuild-plugin-global-externals": {
- "version": "2.1.2",
- "resolved": "https://registry.npmjs.org/@fal-works/esbuild-plugin-global-externals/-/esbuild-plugin-global-externals-2.1.2.tgz",
- "integrity": "sha512-cEee/Z+I12mZcFJshKcCqC8tuX5hG3s+d+9nZ3LabqKF1vKdF41B92pJVCBggjAGORAeOzyyDDKrZwIkLffeOQ==",
- "dev": true
- },
"node_modules/@fastify/busboy": {
"version": "2.1.1",
"resolved": "https://registry.npmjs.org/@fastify/busboy/-/busboy-2.1.1.tgz",
@@ -5239,38 +5144,74 @@
"integrity": "sha512-kym7SodPp8/wloecOpcmSnWJsK7M0E5Wg8UcFA+uO4B9s5d0ywXOEro/8HM9x0rW+TljRzul/14UYz3TleT3ig==",
"license": "MIT"
},
- "node_modules/@floating-ui/react-dom": {
- "version": "2.1.2",
- "resolved": "https://registry.npmjs.org/@floating-ui/react-dom/-/react-dom-2.1.2.tgz",
- "integrity": "sha512-06okr5cgPzMNBy+Ycse2A6udMi4bqwW/zgBF/rwjcNqWkyr82Mcg8b0vjX8OJpZFy/FKjJmw6wV7t44kK6kW7A==",
- "dev": true,
- "license": "MIT",
- "dependencies": {
- "@floating-ui/dom": "^1.0.0"
- },
- "peerDependencies": {
- "react": ">=16.8.0",
- "react-dom": ">=16.8.0"
- }
- },
"node_modules/@floating-ui/utils": {
"version": "0.1.6",
"resolved": "https://registry.npmjs.org/@floating-ui/utils/-/utils-0.1.6.tgz",
"integrity": "sha512-OfX7E2oUDYxtBvsuS4e/jSn4Q9Qb6DzgeYtsAdkPZ47znpoNsMgZw0+tVijiv3uGNR6dgNlty6r9rzIzHjtd/A=="
},
+ "node_modules/@formatjs/ecma402-abstract": {
+ "version": "2.2.4",
+ "resolved": "https://registry.npmjs.org/@formatjs/ecma402-abstract/-/ecma402-abstract-2.2.4.tgz",
+ "integrity": "sha512-lFyiQDVvSbQOpU+WFd//ILolGj4UgA/qXrKeZxdV14uKiAUiPAtX6XAn7WBCRi7Mx6I7EybM9E5yYn4BIpZWYg==",
+ "license": "MIT",
+ "dependencies": {
+ "@formatjs/fast-memoize": "2.2.3",
+ "@formatjs/intl-localematcher": "0.5.8",
+ "tslib": "2"
+ }
+ },
+ "node_modules/@formatjs/fast-memoize": {
+ "version": "2.2.3",
+ "resolved": "https://registry.npmjs.org/@formatjs/fast-memoize/-/fast-memoize-2.2.3.tgz",
+ "integrity": "sha512-3jeJ+HyOfu8osl3GNSL4vVHUuWFXR03Iz9jjgI7RwjG6ysu/Ymdr0JRCPHfF5yGbTE6JCrd63EpvX1/WybYRbA==",
+ "license": "MIT",
+ "dependencies": {
+ "tslib": "2"
+ }
+ },
+ "node_modules/@formatjs/icu-messageformat-parser": {
+ "version": "2.9.4",
+ "resolved": "https://registry.npmjs.org/@formatjs/icu-messageformat-parser/-/icu-messageformat-parser-2.9.4.tgz",
+ "integrity": "sha512-Tbvp5a9IWuxUcpWNIW6GlMQYEc4rwNHR259uUFoKWNN1jM9obf9Ul0e+7r7MvFOBNcN+13K7NuKCKqQiAn1QEg==",
+ "license": "MIT",
+ "dependencies": {
+ "@formatjs/ecma402-abstract": "2.2.4",
+ "@formatjs/icu-skeleton-parser": "1.8.8",
+ "tslib": "2"
+ }
+ },
+ "node_modules/@formatjs/icu-skeleton-parser": {
+ "version": "1.8.8",
+ "resolved": "https://registry.npmjs.org/@formatjs/icu-skeleton-parser/-/icu-skeleton-parser-1.8.8.tgz",
+ "integrity": "sha512-vHwK3piXwamFcx5YQdCdJxUQ1WdTl6ANclt5xba5zLGDv5Bsur7qz8AD7BevaKxITwpgDeU0u8My3AIibW9ywA==",
+ "license": "MIT",
+ "dependencies": {
+ "@formatjs/ecma402-abstract": "2.2.4",
+ "tslib": "2"
+ }
+ },
+ "node_modules/@formatjs/intl-localematcher": {
+ "version": "0.5.8",
+ "resolved": "https://registry.npmjs.org/@formatjs/intl-localematcher/-/intl-localematcher-0.5.8.tgz",
+ "integrity": "sha512-I+WDNWWJFZie+jkfkiK5Mp4hEDyRSEvmyfYadflOno/mmKJKcB17fEpEH0oJu/OWhhCJ8kJBDz2YMd/6cDl7Mg==",
+ "license": "MIT",
+ "dependencies": {
+ "tslib": "2"
+ }
+ },
"node_modules/@geometricpanda/storybook-addon-badges": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/@geometricpanda/storybook-addon-badges/-/storybook-addon-badges-2.0.1.tgz",
- "integrity": "sha512-dCEK/xJewuFe1d+ndF0hQIAJRnUsV9q5kuDmp7zvO7fTd7cDz0X9Bjz0lNRn6n4Z9bL9/iFHKzJESDHFfs4ihQ==",
+ "version": "2.0.5",
+ "resolved": "https://registry.npmjs.org/@geometricpanda/storybook-addon-badges/-/storybook-addon-badges-2.0.5.tgz",
+ "integrity": "sha512-FH56ly6ZhltjyKQWxUKORP67BxhL9FMJRByS5lqKZpeP8J2MMsMXG7eQmFXKcZGQORfVQye+1uYYWXweDOiFTQ==",
"dev": true,
"peerDependencies": {
- "@storybook/blocks": "^7.0.0",
- "@storybook/components": "^7.0.0",
- "@storybook/core-events": "^7.0.0",
- "@storybook/manager-api": "^7.0.0",
- "@storybook/preview-api": "^7.0.0",
- "@storybook/theming": "^7.0.0",
- "@storybook/types": "^7.0.0",
+ "@storybook/blocks": "^8.3.0",
+ "@storybook/components": "^8.3.0",
+ "@storybook/core-events": "^8.3.0",
+ "@storybook/manager-api": "^8.3.0",
+ "@storybook/preview-api": "^8.3.0",
+ "@storybook/theming": "^8.3.0",
+ "@storybook/types": "^8.3.0",
"react": "^16.8.0 || ^17.0.0 || ^18.0.0",
"react-dom": "^16.8.0 || ^17.0.0 || ^18.0.0"
},
@@ -5319,6 +5260,7 @@
"resolved": "https://registry.npmjs.org/@hutson/parse-repository-url/-/parse-repository-url-3.0.2.tgz",
"integrity": "sha512-H9XAx3hc0BQHY6l+IFSWHDySypcXsvsuLhgYLUGywmJ5pswRVQJUHpOsobnLYp2ZUaUlKiKDrgWWhosOwAEM8Q==",
"dev": true,
+ "license": "Apache-2.0",
"engines": {
"node": ">=6.9.0"
}
@@ -5327,7 +5269,6 @@
"version": "8.0.2",
"resolved": "https://registry.npmjs.org/@isaacs/cliui/-/cliui-8.0.2.tgz",
"integrity": "sha512-O8jcjabXaleOG9DQ0+ARXWZBTfnP4WNAqzuiJK7ll44AmxGKv/J2M4TPjxjY3znBCfvBXFzucm1twdyFybFqEA==",
- "dev": true,
"dependencies": {
"string-width": "^5.1.2",
"string-width-cjs": "npm:string-width@^4.2.0",
@@ -5344,7 +5285,6 @@
"version": "6.1.0",
"resolved": "https://registry.npmjs.org/ansi-regex/-/ansi-regex-6.1.0.tgz",
"integrity": "sha512-7HSX4QQb4CspciLpVFwyRe79O3xsIZDDLER21kERQ71oaPodF8jL725AgJMFAYbooIqolJoRLuM81SpeUkpkvA==",
- "dev": true,
"license": "MIT",
"engines": {
"node": ">=12"
@@ -5357,7 +5297,6 @@
"version": "6.2.1",
"resolved": "https://registry.npmjs.org/ansi-styles/-/ansi-styles-6.2.1.tgz",
"integrity": "sha512-bN798gFfQX+viw3R7yrGWRqnrN2oRkEkUjjl4JNn4E8GxxbjtG3FbrEIIY3l8/hrwUwIeCZvi4QuOTP4MErVug==",
- "dev": true,
"engines": {
"node": ">=12"
},
@@ -5368,14 +5307,12 @@
"node_modules/@isaacs/cliui/node_modules/emoji-regex": {
"version": "9.2.2",
"resolved": "https://registry.npmjs.org/emoji-regex/-/emoji-regex-9.2.2.tgz",
- "integrity": "sha512-L18DaJsXSUk2+42pv8mLs5jJT2hqFkFE4j21wOmgbUqsZ2hL72NsUU785g9RXgo3s0ZNgVl42TiHp3ZtOv/Vyg==",
- "dev": true
+ "integrity": "sha512-L18DaJsXSUk2+42pv8mLs5jJT2hqFkFE4j21wOmgbUqsZ2hL72NsUU785g9RXgo3s0ZNgVl42TiHp3ZtOv/Vyg=="
},
"node_modules/@isaacs/cliui/node_modules/string-width": {
"version": "5.1.2",
"resolved": "https://registry.npmjs.org/string-width/-/string-width-5.1.2.tgz",
"integrity": "sha512-HnLOCR3vjcY8beoNLtcjZ5/nxn2afmME6lhrDrebokqMap+XbeW8n9TXpPDOqdGK5qcI3oT0GKTW6wC7EMiVqA==",
- "dev": true,
"dependencies": {
"eastasianwidth": "^0.2.0",
"emoji-regex": "^9.2.2",
@@ -5392,7 +5329,6 @@
"version": "7.1.0",
"resolved": "https://registry.npmjs.org/strip-ansi/-/strip-ansi-7.1.0.tgz",
"integrity": "sha512-iq6eVVI64nQQTRYq2KtEg2d2uU7LElhTJwsH4YzIHZshxlgZms/wIc4VoDQTlG/IvVIrBKG06CrZnp0qv7hkcQ==",
- "dev": true,
"dependencies": {
"ansi-regex": "^6.0.1"
},
@@ -5407,7 +5343,6 @@
"version": "8.1.0",
"resolved": "https://registry.npmjs.org/wrap-ansi/-/wrap-ansi-8.1.0.tgz",
"integrity": "sha512-si7QWI6zUMq56bESFvagtmzMdGOtoxfR+Sez11Mobfc7tm+VkUckk9bW2UeffTGVUbOksxmSw0AA2gs8g71NCQ==",
- "dev": true,
"dependencies": {
"ansi-styles": "^6.1.0",
"string-width": "^5.0.1",
@@ -5420,6 +5355,13 @@
"url": "https://github.com/chalk/wrap-ansi?sponsor=1"
}
},
+ "node_modules/@isaacs/string-locale-compare": {
+ "version": "1.1.0",
+ "resolved": "https://registry.npmjs.org/@isaacs/string-locale-compare/-/string-locale-compare-1.1.0.tgz",
+ "integrity": "sha512-SQ7Kzhh9+D+ZW9MA0zkYv3VXhIDNx+LzM6EJ+/65I3QY+enU6Itte7E5XX7EWrqLW2FN4n06GWzBnPoC3th2aQ==",
+ "dev": true,
+ "license": "ISC"
+ },
"node_modules/@isaacs/ttlcache": {
"version": "1.4.1",
"resolved": "https://registry.npmjs.org/@isaacs/ttlcache/-/ttlcache-1.4.1.tgz",
@@ -5891,9 +5833,9 @@
}
},
"node_modules/@jridgewell/sourcemap-codec": {
- "version": "1.4.15",
- "resolved": "https://registry.npmjs.org/@jridgewell/sourcemap-codec/-/sourcemap-codec-1.4.15.tgz",
- "integrity": "sha512-eF2rxCRulEKXHTRiDrDy6erMYWqNw4LPdQ8UQA4huuxaQsVeRPFl2oM8oDGxMFhJUWZf9McpLtJasDDZb/Bpeg=="
+ "version": "1.5.0",
+ "resolved": "https://registry.npmjs.org/@jridgewell/sourcemap-codec/-/sourcemap-codec-1.5.0.tgz",
+ "integrity": "sha512-gv3ZRaISU3fjPAgNsriBRqGWQL6quFx04YMPW/zD8XMLsU32mhCCbfbO6KZFLjvYpCZ8zyDEgqsgf+PwPaM7GQ=="
},
"node_modules/@jridgewell/trace-mapping": {
"version": "0.3.25",
@@ -5910,12 +5852,6 @@
"integrity": "sha512-4JQNk+3mVzK3xh2rqd6RB4J46qUR19azEHBneZyTZM+c456qOrbbM/5xcR8huNCCcbVt7+UmizG6GuUvPvKUYg==",
"dev": true
},
- "node_modules/@juggle/resize-observer": {
- "version": "3.4.0",
- "resolved": "https://registry.npmjs.org/@juggle/resize-observer/-/resize-observer-3.4.0.tgz",
- "integrity": "sha512-dfLbk+PwWvFzSxwk3n5ySL0hfBog779o8h68wK/7/APo/7cgyWp5jcXockbxdk5kFRkbeXWm4Fbi9FrdN381sA==",
- "dev": true
- },
"node_modules/@kwsites/file-exists": {
"version": "1.1.1",
"resolved": "https://registry.npmjs.org/@kwsites/file-exists/-/file-exists-1.1.1.tgz",
@@ -5934,273 +5870,428 @@
"resolved": "https://registry.npmjs.org/@leichtgewicht/ip-codec/-/ip-codec-2.0.4.tgz",
"integrity": "sha512-Hcv+nVC0kZnQ3tD9GVu5xSMR4VVYOteQIr/hwFPVEvPdlXqgGEuRjiheChHgdM+JyqdgNcmzZOX/tnl0JOiI7A=="
},
- "node_modules/@lerna/child-process": {
- "version": "7.1.4",
- "resolved": "https://registry.npmjs.org/@lerna/child-process/-/child-process-7.1.4.tgz",
- "integrity": "sha512-cSiMDx9oI9vvVT+V/WHcbqrksNoc9PIPFiks1lPS7zrVWkEbgA6REQyYmRd2H71kihzqhX5TJ20f2dWv6oEPdA==",
+ "node_modules/@lerna/create": {
+ "version": "8.1.9",
+ "resolved": "https://registry.npmjs.org/@lerna/create/-/create-8.1.9.tgz",
+ "integrity": "sha512-DPnl5lPX4v49eVxEbJnAizrpMdMTBz1qykZrAbBul9rfgk531v8oAt+Pm6O/rpAleRombNM7FJb5rYGzBJatOQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "chalk": "^4.1.0",
- "execa": "^5.0.0",
- "strong-log-transformer": "^2.1.0"
+ "@npmcli/arborist": "7.5.4",
+ "@npmcli/package-json": "5.2.0",
+ "@npmcli/run-script": "8.1.0",
+ "@nx/devkit": ">=17.1.2 < 21",
+ "@octokit/plugin-enterprise-rest": "6.0.1",
+ "@octokit/rest": "19.0.11",
+ "aproba": "2.0.0",
+ "byte-size": "8.1.1",
+ "chalk": "4.1.0",
+ "clone-deep": "4.0.1",
+ "cmd-shim": "6.0.3",
+ "color-support": "1.1.3",
+ "columnify": "1.6.0",
+ "console-control-strings": "^1.1.0",
+ "conventional-changelog-core": "5.0.1",
+ "conventional-recommended-bump": "7.0.1",
+ "cosmiconfig": "9.0.0",
+ "dedent": "1.5.3",
+ "execa": "5.0.0",
+ "fs-extra": "^11.2.0",
+ "get-stream": "6.0.0",
+ "git-url-parse": "14.0.0",
+ "glob-parent": "6.0.2",
+ "globby": "11.1.0",
+ "graceful-fs": "4.2.11",
+ "has-unicode": "2.0.1",
+ "ini": "^1.3.8",
+ "init-package-json": "6.0.3",
+ "inquirer": "^8.2.4",
+ "is-ci": "3.0.1",
+ "is-stream": "2.0.0",
+ "js-yaml": "4.1.0",
+ "libnpmpublish": "9.0.9",
+ "load-json-file": "6.2.0",
+ "lodash": "^4.17.21",
+ "make-dir": "4.0.0",
+ "minimatch": "3.0.5",
+ "multimatch": "5.0.0",
+ "node-fetch": "2.6.7",
+ "npm-package-arg": "11.0.2",
+ "npm-packlist": "8.0.2",
+ "npm-registry-fetch": "^17.1.0",
+ "nx": ">=17.1.2 < 21",
+ "p-map": "4.0.0",
+ "p-map-series": "2.1.0",
+ "p-queue": "6.6.2",
+ "p-reduce": "^2.1.0",
+ "pacote": "^18.0.6",
+ "pify": "5.0.0",
+ "read-cmd-shim": "4.0.0",
+ "resolve-from": "5.0.0",
+ "rimraf": "^4.4.1",
+ "semver": "^7.3.4",
+ "set-blocking": "^2.0.0",
+ "signal-exit": "3.0.7",
+ "slash": "^3.0.0",
+ "ssri": "^10.0.6",
+ "string-width": "^4.2.3",
+ "strip-ansi": "^6.0.1",
+ "strong-log-transformer": "2.1.0",
+ "tar": "6.2.1",
+ "temp-dir": "1.0.0",
+ "upath": "2.0.1",
+ "uuid": "^10.0.0",
+ "validate-npm-package-license": "^3.0.4",
+ "validate-npm-package-name": "5.0.1",
+ "wide-align": "1.1.5",
+ "write-file-atomic": "5.0.1",
+ "write-pkg": "4.0.0",
+ "yargs": "17.7.2",
+ "yargs-parser": "21.1.1"
},
"engines": {
- "node": "^14.17.0 || >=16.0.0"
+ "node": ">=18.0.0"
}
},
- "node_modules/@lerna/child-process/node_modules/cross-spawn": {
- "version": "7.0.3",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-7.0.3.tgz",
- "integrity": "sha512-iRDPJKUPVEND7dHPO8rkbOnPpyDygcDFtWjpeWNCgy8WP2rXcxXL8TskReQl6OrB2G7+UJrags1q15Fudc7G6w==",
+ "node_modules/@lerna/create/node_modules/@octokit/auth-token": {
+ "version": "3.0.4",
+ "resolved": "https://registry.npmjs.org/@octokit/auth-token/-/auth-token-3.0.4.tgz",
+ "integrity": "sha512-TWFX7cZF2LXoCvdmJWY7XVPi74aSY0+FfBZNSXEXFkMpjcqsQwDSYVv5FhRFaI0V1ECnwbz4j59T/G+rXNWaIQ==",
"dev": true,
- "dependencies": {
- "path-key": "^3.1.0",
- "shebang-command": "^2.0.0",
- "which": "^2.0.1"
- },
+ "license": "MIT",
"engines": {
- "node": ">= 8"
+ "node": ">= 14"
}
},
- "node_modules/@lerna/child-process/node_modules/execa": {
- "version": "5.1.1",
- "resolved": "https://registry.npmjs.org/execa/-/execa-5.1.1.tgz",
- "integrity": "sha512-8uSpZZocAZRBAPIEINJj3Lo9HyGitllczc27Eh5YYojjMFMn8yHMDMaUHE2Jqfq05D/wucwI4JGURyXt1vchyg==",
+ "node_modules/@lerna/create/node_modules/@octokit/core": {
+ "version": "4.2.4",
+ "resolved": "https://registry.npmjs.org/@octokit/core/-/core-4.2.4.tgz",
+ "integrity": "sha512-rYKilwgzQ7/imScn3M9/pFfUf4I1AZEH3KhyJmtPdE2zfaXAn2mFfUy4FbKewzc2We5y/LlKLj36fWJLKC2SIQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "cross-spawn": "^7.0.3",
- "get-stream": "^6.0.0",
- "human-signals": "^2.1.0",
- "is-stream": "^2.0.0",
- "merge-stream": "^2.0.0",
- "npm-run-path": "^4.0.1",
- "onetime": "^5.1.2",
- "signal-exit": "^3.0.3",
- "strip-final-newline": "^2.0.0"
+ "@octokit/auth-token": "^3.0.0",
+ "@octokit/graphql": "^5.0.0",
+ "@octokit/request": "^6.0.0",
+ "@octokit/request-error": "^3.0.0",
+ "@octokit/types": "^9.0.0",
+ "before-after-hook": "^2.2.0",
+ "universal-user-agent": "^6.0.0"
},
"engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/sindresorhus/execa?sponsor=1"
+ "node": ">= 14"
}
},
- "node_modules/@lerna/child-process/node_modules/get-stream": {
- "version": "6.0.1",
- "resolved": "https://registry.npmjs.org/get-stream/-/get-stream-6.0.1.tgz",
- "integrity": "sha512-ts6Wi+2j3jQjqi70w5AlN8DFnkSwC+MqmxEzdEALB2qXZYV3X/b1CTfgPLGJNMeAWxdPfU8FO1ms3NUfaHCPYg==",
+ "node_modules/@lerna/create/node_modules/@octokit/endpoint": {
+ "version": "7.0.6",
+ "resolved": "https://registry.npmjs.org/@octokit/endpoint/-/endpoint-7.0.6.tgz",
+ "integrity": "sha512-5L4fseVRUsDFGR00tMWD/Trdeeihn999rTMGRMC1G/Ldi1uWlWJzI98H4Iak5DB/RVvQuyMYKqSK/R6mbSOQyg==",
"dev": true,
- "engines": {
- "node": ">=10"
+ "license": "MIT",
+ "dependencies": {
+ "@octokit/types": "^9.0.0",
+ "is-plain-object": "^5.0.0",
+ "universal-user-agent": "^6.0.0"
},
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "engines": {
+ "node": ">= 14"
}
},
- "node_modules/@lerna/child-process/node_modules/human-signals": {
- "version": "2.1.0",
- "resolved": "https://registry.npmjs.org/human-signals/-/human-signals-2.1.0.tgz",
- "integrity": "sha512-B4FFZ6q/T2jhhksgkbEW3HBvWIfDW85snkQgawt07S7J5QXTk6BkNV+0yAeZrM5QpMAdYlocGoljn0sJ/WQkFw==",
+ "node_modules/@lerna/create/node_modules/@octokit/graphql": {
+ "version": "5.0.6",
+ "resolved": "https://registry.npmjs.org/@octokit/graphql/-/graphql-5.0.6.tgz",
+ "integrity": "sha512-Fxyxdy/JH0MnIB5h+UQ3yCoh1FG4kWXfFKkpWqjZHw/p+Kc8Y44Hu/kCgNBT6nU1shNumEchmW/sUO1JuQnPcw==",
"dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "@octokit/request": "^6.0.0",
+ "@octokit/types": "^9.0.0",
+ "universal-user-agent": "^6.0.0"
+ },
"engines": {
- "node": ">=10.17.0"
+ "node": ">= 14"
}
},
- "node_modules/@lerna/child-process/node_modules/is-stream": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/is-stream/-/is-stream-2.0.1.tgz",
- "integrity": "sha512-hFoiJiTl63nn+kstHGBtewWSKnQLpyb155KHheA1l39uvtO9nWIop1p3udqPcUd/xbF1VLMO4n7OI6p7RbngDg==",
+ "node_modules/@lerna/create/node_modules/@octokit/openapi-types": {
+ "version": "18.1.1",
+ "resolved": "https://registry.npmjs.org/@octokit/openapi-types/-/openapi-types-18.1.1.tgz",
+ "integrity": "sha512-VRaeH8nCDtF5aXWnjPuEMIYf1itK/s3JYyJcWFJT8X9pSNnBtriDf7wlEWsGuhPLl4QIH4xM8fqTXDwJ3Mu6sw==",
+ "dev": true,
+ "license": "MIT"
+ },
+ "node_modules/@lerna/create/node_modules/@octokit/plugin-paginate-rest": {
+ "version": "6.1.2",
+ "resolved": "https://registry.npmjs.org/@octokit/plugin-paginate-rest/-/plugin-paginate-rest-6.1.2.tgz",
+ "integrity": "sha512-qhrmtQeHU/IivxucOV1bbI/xZyC/iOBhclokv7Sut5vnejAIAEXVcGQeRpQlU39E0WwK9lNvJHphHri/DB6lbQ==",
"dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "@octokit/tsconfig": "^1.0.2",
+ "@octokit/types": "^9.2.3"
+ },
"engines": {
- "node": ">=8"
+ "node": ">= 14"
},
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "peerDependencies": {
+ "@octokit/core": ">=4"
}
},
- "node_modules/@lerna/child-process/node_modules/mimic-fn": {
- "version": "2.1.0",
- "resolved": "https://registry.npmjs.org/mimic-fn/-/mimic-fn-2.1.0.tgz",
- "integrity": "sha512-OqbOk5oEQeAZ8WXWydlu9HJjz9WVdEIvamMCcXmuqUYjTknH/sqsWvhQ3vgwKFRR1HpjvNBKQ37nbJgYzGqGcg==",
+ "node_modules/@lerna/create/node_modules/@octokit/plugin-rest-endpoint-methods": {
+ "version": "7.2.3",
+ "resolved": "https://registry.npmjs.org/@octokit/plugin-rest-endpoint-methods/-/plugin-rest-endpoint-methods-7.2.3.tgz",
+ "integrity": "sha512-I5Gml6kTAkzVlN7KCtjOM+Ruwe/rQppp0QU372K1GP7kNOYEKe8Xn5BW4sE62JAHdwpq95OQK/qGNyKQMUzVgA==",
"dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "@octokit/types": "^10.0.0"
+ },
"engines": {
- "node": ">=6"
+ "node": ">= 14"
+ },
+ "peerDependencies": {
+ "@octokit/core": ">=3"
}
},
- "node_modules/@lerna/child-process/node_modules/npm-run-path": {
- "version": "4.0.1",
- "resolved": "https://registry.npmjs.org/npm-run-path/-/npm-run-path-4.0.1.tgz",
- "integrity": "sha512-S48WzZW777zhNIrn7gxOlISNAqi9ZC/uQFnRdbeIHhZhCA6UqpkOT8T1G7BvfdgP4Er8gF4sUbaS0i7QvIfCWw==",
+ "node_modules/@lerna/create/node_modules/@octokit/plugin-rest-endpoint-methods/node_modules/@octokit/types": {
+ "version": "10.0.0",
+ "resolved": "https://registry.npmjs.org/@octokit/types/-/types-10.0.0.tgz",
+ "integrity": "sha512-Vm8IddVmhCgU1fxC1eyinpwqzXPEYu0NrYzD3YZjlGjyftdLBTeqNblRC0jmJmgxbJIsQlyogVeGnrNaaMVzIg==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "path-key": "^3.0.0"
- },
- "engines": {
- "node": ">=8"
+ "@octokit/openapi-types": "^18.0.0"
}
},
- "node_modules/@lerna/child-process/node_modules/onetime": {
- "version": "5.1.2",
- "resolved": "https://registry.npmjs.org/onetime/-/onetime-5.1.2.tgz",
- "integrity": "sha512-kbpaSSGJTWdAY5KPVeMOKXSrPtr8C8C7wodJbcsd51jRnmD+GZu8Y0VoU6Dm5Z4vWr0Ig/1NKuWRKf7j5aaYSg==",
+ "node_modules/@lerna/create/node_modules/@octokit/request": {
+ "version": "6.2.8",
+ "resolved": "https://registry.npmjs.org/@octokit/request/-/request-6.2.8.tgz",
+ "integrity": "sha512-ow4+pkVQ+6XVVsekSYBzJC0VTVvh/FCTUUgTsboGq+DTeWdyIFV8WSCdo0RIxk6wSkBTHqIK1mYuY7nOBXOchw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "mimic-fn": "^2.1.0"
+ "@octokit/endpoint": "^7.0.0",
+ "@octokit/request-error": "^3.0.0",
+ "@octokit/types": "^9.0.0",
+ "is-plain-object": "^5.0.0",
+ "node-fetch": "^2.6.7",
+ "universal-user-agent": "^6.0.0"
},
"engines": {
- "node": ">=6"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "node": ">= 14"
}
},
- "node_modules/@lerna/child-process/node_modules/path-key": {
- "version": "3.1.1",
- "resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
- "integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
+ "node_modules/@lerna/create/node_modules/@octokit/request-error": {
+ "version": "3.0.3",
+ "resolved": "https://registry.npmjs.org/@octokit/request-error/-/request-error-3.0.3.tgz",
+ "integrity": "sha512-crqw3V5Iy2uOU5Np+8M/YexTlT8zxCfI+qu+LxUB7SZpje4Qmx3mub5DfEKSO8Ylyk0aogi6TYdf6kxzh2BguQ==",
"dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "@octokit/types": "^9.0.0",
+ "deprecation": "^2.0.0",
+ "once": "^1.4.0"
+ },
"engines": {
- "node": ">=8"
+ "node": ">= 14"
}
},
- "node_modules/@lerna/child-process/node_modules/shebang-command": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/shebang-command/-/shebang-command-2.0.0.tgz",
- "integrity": "sha512-kHxr2zZpYtdmrN1qDjrrX/Z1rR1kG8Dx+gkpK1G4eXmvXswmcE1hTWBWYUzlraYw1/yZp6YuDY77YtvbN0dmDA==",
+ "node_modules/@lerna/create/node_modules/@octokit/rest": {
+ "version": "19.0.11",
+ "resolved": "https://registry.npmjs.org/@octokit/rest/-/rest-19.0.11.tgz",
+ "integrity": "sha512-m2a9VhaP5/tUw8FwfnW2ICXlXpLPIqxtg3XcAiGMLj/Xhw3RSBfZ8le/466ktO1Gcjr8oXudGnHhxV1TXJgFxw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "shebang-regex": "^3.0.0"
+ "@octokit/core": "^4.2.1",
+ "@octokit/plugin-paginate-rest": "^6.1.2",
+ "@octokit/plugin-request-log": "^1.0.4",
+ "@octokit/plugin-rest-endpoint-methods": "^7.1.2"
},
"engines": {
- "node": ">=8"
+ "node": ">= 14"
}
},
- "node_modules/@lerna/child-process/node_modules/shebang-regex": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/shebang-regex/-/shebang-regex-3.0.0.tgz",
- "integrity": "sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==",
+ "node_modules/@lerna/create/node_modules/@octokit/types": {
+ "version": "9.3.2",
+ "resolved": "https://registry.npmjs.org/@octokit/types/-/types-9.3.2.tgz",
+ "integrity": "sha512-D4iHGTdAnEEVsB8fl95m1hiz7D5YiRdQ9b/OEb3BYRVwbLsGHcRVPz+u+BgRLNk0Q0/4iZCBqDN96j2XNxfXrA==",
"dev": true,
- "engines": {
- "node": ">=8"
+ "license": "MIT",
+ "dependencies": {
+ "@octokit/openapi-types": "^18.0.0"
}
},
- "node_modules/@lerna/child-process/node_modules/which": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
- "integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
+ "node_modules/@lerna/create/node_modules/aproba": {
+ "version": "2.0.0",
+ "resolved": "https://registry.npmjs.org/aproba/-/aproba-2.0.0.tgz",
+ "integrity": "sha512-lYe4Gx7QT+MKGbDsA+Z+he/Wtef0BiwDOlK/XkBrdfsh9J/jPPXbX0tE9x9cl27Tmu5gg3QUbUrQYa/y+KOHPQ==",
+ "dev": true,
+ "license": "ISC"
+ },
+ "node_modules/@lerna/create/node_modules/argparse": {
+ "version": "2.0.1",
+ "resolved": "https://registry.npmjs.org/argparse/-/argparse-2.0.1.tgz",
+ "integrity": "sha512-8+9WqebbFzpX9OR+Wa6O29asIogeRMzcGtAINdpMHHyAg10f05aSFVBbcEqGf/PXw1EjAZ+q2/bEBg3DvurK3Q==",
+ "dev": true,
+ "license": "Python-2.0"
+ },
+ "node_modules/@lerna/create/node_modules/before-after-hook": {
+ "version": "2.2.3",
+ "resolved": "https://registry.npmjs.org/before-after-hook/-/before-after-hook-2.2.3.tgz",
+ "integrity": "sha512-NzUnlZexiaH/46WDhANlyR2bXRopNg4F/zuSA3OpZnllCUgRaOF2znDioDWrmbNVsuZk6l9pMquQB38cfBZwkQ==",
+ "dev": true,
+ "license": "Apache-2.0"
+ },
+ "node_modules/@lerna/create/node_modules/chalk": {
+ "version": "4.1.0",
+ "resolved": "https://registry.npmjs.org/chalk/-/chalk-4.1.0.tgz",
+ "integrity": "sha512-qwx12AxXe2Q5xQ43Ac//I6v5aXTipYrSESdOgzrN+9XjgEpyjpKuvSGaN4qE93f7TQTlerQQ8S+EQ0EyDoVL1A==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "isexe": "^2.0.0"
- },
- "bin": {
- "node-which": "bin/node-which"
+ "ansi-styles": "^4.1.0",
+ "supports-color": "^7.1.0"
},
"engines": {
- "node": ">= 8"
+ "node": ">=10"
+ },
+ "funding": {
+ "url": "https://github.com/chalk/chalk?sponsor=1"
}
},
- "node_modules/@lerna/create": {
- "version": "7.1.4",
- "resolved": "https://registry.npmjs.org/@lerna/create/-/create-7.1.4.tgz",
- "integrity": "sha512-D5YWXsXIxWb1aGqcbtttczg86zMzkNhcs00/BleFNxdNYlTRdjLIReELOGBGrq3Hij05UN+7Dv9EKnPFJVbqAw==",
+ "node_modules/@lerna/create/node_modules/cli-cursor": {
+ "version": "3.1.0",
+ "resolved": "https://registry.npmjs.org/cli-cursor/-/cli-cursor-3.1.0.tgz",
+ "integrity": "sha512-I/zHAwsKf9FqGoXM4WWRACob9+SNukZTd94DWF57E4toouRulbCxcUh6RKUEOQlYTHJnzkPMySvPNaaSLNfLZw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "@lerna/child-process": "7.1.4",
- "dedent": "0.7.0",
- "fs-extra": "^11.1.1",
- "init-package-json": "5.0.0",
- "npm-package-arg": "8.1.1",
- "p-reduce": "^2.1.0",
- "pacote": "^15.2.0",
- "pify": "5.0.0",
- "semver": "^7.3.4",
- "slash": "^3.0.0",
- "validate-npm-package-license": "^3.0.4",
- "validate-npm-package-name": "5.0.0",
- "yargs-parser": "20.2.4"
+ "restore-cursor": "^3.1.0"
},
"engines": {
- "node": "^14.17.0 || >=16.0.0"
+ "node": ">=8"
}
},
- "node_modules/@lerna/create/node_modules/fs-extra": {
- "version": "11.2.0",
- "resolved": "https://registry.npmjs.org/fs-extra/-/fs-extra-11.2.0.tgz",
- "integrity": "sha512-PmDi3uwK5nFuXh7XDTlVnS17xJS7vW36is2+w3xcv8SVxiB4NyATf4ctkVY5bkSjX0Y4nbvZCq1/EjtEyr9ktw==",
+ "node_modules/@lerna/create/node_modules/cli-width": {
+ "version": "3.0.0",
+ "resolved": "https://registry.npmjs.org/cli-width/-/cli-width-3.0.0.tgz",
+ "integrity": "sha512-FxqpkPPwu1HjuN93Omfm4h8uIanXofW0RxVEW3k5RKx+mJJYSthzNhp32Kzxxy3YAEZ/Dc/EWN1vZRY0+kOhbw==",
"dev": true,
- "dependencies": {
- "graceful-fs": "^4.2.0",
- "jsonfile": "^6.0.1",
- "universalify": "^2.0.0"
- },
+ "license": "ISC",
"engines": {
- "node": ">=14.14"
+ "node": ">= 10"
}
},
- "node_modules/@lerna/create/node_modules/hosted-git-info": {
- "version": "3.0.8",
- "resolved": "https://registry.npmjs.org/hosted-git-info/-/hosted-git-info-3.0.8.tgz",
- "integrity": "sha512-aXpmwoOhRBrw6X3j0h5RloK4x1OzsxMPyxqIHyNfSe2pypkVTZFpEiRoSipPEPlMrh0HW/XsjkJ5WgnCirpNUw==",
+ "node_modules/@lerna/create/node_modules/cosmiconfig": {
+ "version": "9.0.0",
+ "resolved": "https://registry.npmjs.org/cosmiconfig/-/cosmiconfig-9.0.0.tgz",
+ "integrity": "sha512-itvL5h8RETACmOTFc4UfIyB2RfEHi71Ax6E/PivVxq9NseKbOWpeyHEOIbmAw1rs8Ak0VursQNww7lf7YtUwzg==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "lru-cache": "^6.0.0"
+ "env-paths": "^2.2.1",
+ "import-fresh": "^3.3.0",
+ "js-yaml": "^4.1.0",
+ "parse-json": "^5.2.0"
},
"engines": {
- "node": ">=10"
+ "node": ">=14"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/d-fischer"
+ },
+ "peerDependencies": {
+ "typescript": ">=4.9.5"
+ },
+ "peerDependenciesMeta": {
+ "typescript": {
+ "optional": true
+ }
}
},
- "node_modules/@lerna/create/node_modules/jsonfile": {
- "version": "6.1.0",
- "resolved": "https://registry.npmjs.org/jsonfile/-/jsonfile-6.1.0.tgz",
- "integrity": "sha512-5dgndWOriYSm5cnYaJNhalLNDKOqFwyDB/rr1E9ZsGciGvKPs8R2xYGCacuf3z6K1YKDz182fd+fY3cn3pMqXQ==",
+ "node_modules/@lerna/create/node_modules/dedent": {
+ "version": "1.5.3",
+ "resolved": "https://registry.npmjs.org/dedent/-/dedent-1.5.3.tgz",
+ "integrity": "sha512-NHQtfOOW68WD8lgypbLA5oT+Bt0xXJhiYvoR6SmmNXZfpzOGXwdKWmcwG8N7PwVVWV3eF/68nmD9BaJSsTBhyQ==",
"dev": true,
- "dependencies": {
- "universalify": "^2.0.0"
+ "license": "MIT",
+ "peerDependencies": {
+ "babel-plugin-macros": "^3.1.0"
},
- "optionalDependencies": {
- "graceful-fs": "^4.1.6"
+ "peerDependenciesMeta": {
+ "babel-plugin-macros": {
+ "optional": true
+ }
}
},
- "node_modules/@lerna/create/node_modules/lru-cache": {
- "version": "6.0.0",
- "resolved": "https://registry.npmjs.org/lru-cache/-/lru-cache-6.0.0.tgz",
- "integrity": "sha512-Jo6dJ04CmSjuznwJSS3pUeWmd/H0ffTlkXXgwZi+eq1UCmqQwCh+eLsYOYCwY991i2Fah4h1BEMCx4qThGbsiA==",
+ "node_modules/@lerna/create/node_modules/emoji-regex": {
+ "version": "8.0.0",
+ "resolved": "https://registry.npmjs.org/emoji-regex/-/emoji-regex-8.0.0.tgz",
+ "integrity": "sha512-MSjYzcWNOA0ewAHpz0MxpYFvwg6yjy1NG3xteoqz644VCo/RPgnr1/GGt+ic3iJTzQ8Eu3TdM14SawnVUmGE6A==",
+ "dev": true,
+ "license": "MIT"
+ },
+ "node_modules/@lerna/create/node_modules/execa": {
+ "version": "5.0.0",
+ "resolved": "https://registry.npmjs.org/execa/-/execa-5.0.0.tgz",
+ "integrity": "sha512-ov6w/2LCiuyO4RLYGdpFGjkcs0wMTgGE8PrkTHikeUy5iJekXyPIKUjifk5CsE0pt7sMCrMZ3YNqoCj6idQOnQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "yallist": "^4.0.0"
+ "cross-spawn": "^7.0.3",
+ "get-stream": "^6.0.0",
+ "human-signals": "^2.1.0",
+ "is-stream": "^2.0.0",
+ "merge-stream": "^2.0.0",
+ "npm-run-path": "^4.0.1",
+ "onetime": "^5.1.2",
+ "signal-exit": "^3.0.3",
+ "strip-final-newline": "^2.0.0"
},
"engines": {
"node": ">=10"
+ },
+ "funding": {
+ "url": "https://github.com/sindresorhus/execa?sponsor=1"
}
},
- "node_modules/@lerna/create/node_modules/npm-package-arg": {
- "version": "8.1.1",
- "resolved": "https://registry.npmjs.org/npm-package-arg/-/npm-package-arg-8.1.1.tgz",
- "integrity": "sha512-CsP95FhWQDwNqiYS+Q0mZ7FAEDytDZAkNxQqea6IaAFJTAY9Lhhqyl0irU/6PMc7BGfUmnsbHcqxJD7XuVM/rg==",
+ "node_modules/@lerna/create/node_modules/figures": {
+ "version": "3.2.0",
+ "resolved": "https://registry.npmjs.org/figures/-/figures-3.2.0.tgz",
+ "integrity": "sha512-yaduQFRKLXYOGgEn6AZau90j3ggSOyiqXU0F9JZfeXYhNa+Jk4X+s45A2zg5jns87GAFa34BBm2kXw4XpNcbdg==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "hosted-git-info": "^3.0.6",
- "semver": "^7.0.0",
- "validate-npm-package-name": "^3.0.0"
+ "escape-string-regexp": "^1.0.5"
},
"engines": {
- "node": ">=10"
+ "node": ">=8"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@lerna/create/node_modules/npm-package-arg/node_modules/validate-npm-package-name": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/validate-npm-package-name/-/validate-npm-package-name-3.0.0.tgz",
- "integrity": "sha512-M6w37eVCMMouJ9V/sdPGnC5H4uDr73/+xdq0FBLO3TFFX1+7wiUY6Es328NN+y43tmY+doUdN9g9J21vqB7iLw==",
+ "node_modules/@lerna/create/node_modules/fs-extra": {
+ "version": "11.2.0",
+ "resolved": "https://registry.npmjs.org/fs-extra/-/fs-extra-11.2.0.tgz",
+ "integrity": "sha512-PmDi3uwK5nFuXh7XDTlVnS17xJS7vW36is2+w3xcv8SVxiB4NyATf4ctkVY5bkSjX0Y4nbvZCq1/EjtEyr9ktw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "builtins": "^1.0.3"
+ "graceful-fs": "^4.2.0",
+ "jsonfile": "^6.0.1",
+ "universalify": "^2.0.0"
+ },
+ "engines": {
+ "node": ">=14.14"
}
},
- "node_modules/@lerna/create/node_modules/pify": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/pify/-/pify-5.0.0.tgz",
- "integrity": "sha512-eW/gHNMlxdSP6dmG6uJip6FXN0EQBwm2clYYd8Wul42Cwu/DK8HEftzsapcNdYe2MfLiIwZqsDk2RDEsTE79hA==",
+ "node_modules/@lerna/create/node_modules/get-stream": {
+ "version": "6.0.0",
+ "resolved": "https://registry.npmjs.org/get-stream/-/get-stream-6.0.0.tgz",
+ "integrity": "sha512-A1B3Bh1UmL0bidM/YX2NsCOTnGJePL9rO/M+Mw3m9f2gUpfokS0hi5Eah0WSUEWZdZhIZtMjkIYS7mDfOqNHbg==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=10"
},
@@ -6208,3275 +6299,2246 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@lerna/create/node_modules/universalify": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/universalify/-/universalify-2.0.1.tgz",
- "integrity": "sha512-gptHNQghINnc/vTGIk0SOFGFNXw7JVrlRUtConJRlvaw6DuX0wO5Jeko9sWrMBhh+PsYAZ7oXAiOnf/UKogyiw==",
+ "node_modules/@lerna/create/node_modules/glob": {
+ "version": "9.3.5",
+ "resolved": "https://registry.npmjs.org/glob/-/glob-9.3.5.tgz",
+ "integrity": "sha512-e1LleDykUz2Iu+MTYdkSsuWX8lvAjAcs0Xef0lNIu0S2wOAzuTxCJtcd9S3cijlwYF18EsU3rzb8jPVobxDh9Q==",
"dev": true,
+ "license": "ISC",
+ "dependencies": {
+ "fs.realpath": "^1.0.0",
+ "minimatch": "^8.0.2",
+ "minipass": "^4.2.4",
+ "path-scurry": "^1.6.1"
+ },
"engines": {
- "node": ">= 10.0.0"
+ "node": ">=16 || 14 >=14.17"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
}
},
- "node_modules/@lerna/create/node_modules/validate-npm-package-name": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/validate-npm-package-name/-/validate-npm-package-name-5.0.0.tgz",
- "integrity": "sha512-YuKoXDAhBYxY7SfOKxHBDoSyENFeW5VvIIQp2TGQuit8gpK6MnWaQelBKxso72DoxTZfZdcP3W90LqpSkgPzLQ==",
+ "node_modules/@lerna/create/node_modules/glob-parent": {
+ "version": "6.0.2",
+ "resolved": "https://registry.npmjs.org/glob-parent/-/glob-parent-6.0.2.tgz",
+ "integrity": "sha512-XxwI8EOhVQgWp6iDL+3b0r86f4d6AX6zSU55HfB4ydCEuXLXc5FcYeOu+nnGftS4TEju/11rt4KJPTMgbfmv4A==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "builtins": "^5.0.0"
+ "is-glob": "^4.0.3"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": ">=10.13.0"
}
},
- "node_modules/@lerna/create/node_modules/validate-npm-package-name/node_modules/builtins": {
- "version": "5.1.0",
- "resolved": "https://registry.npmjs.org/builtins/-/builtins-5.1.0.tgz",
- "integrity": "sha512-SW9lzGTLvWTP1AY8xeAMZimqDrIaSdLQUcVr9DMef51niJ022Ri87SwRRKYm4A6iHfkPaiVUu/Duw2Wc4J7kKg==",
+ "node_modules/@lerna/create/node_modules/glob/node_modules/brace-expansion": {
+ "version": "2.0.1",
+ "resolved": "https://registry.npmjs.org/brace-expansion/-/brace-expansion-2.0.1.tgz",
+ "integrity": "sha512-XnAIvQ8eM+kC6aULx6wuQiwVsnzsi9d3WxzV3FpWTGA19F621kwdbsAcFKXgKUHZWsy+mY6iL1sHTxWEFCytDA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "semver": "^7.0.0"
+ "balanced-match": "^1.0.0"
}
},
- "node_modules/@lerna/create/node_modules/yallist": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/yallist/-/yallist-4.0.0.tgz",
- "integrity": "sha512-3wdGidZyq5PB084XLES5TpOSRA3wjXAlIWMhum2kRcv/41Sn2emQ0dycQW4uZXLejwKvg6EsvbdlVL+FYEct7A==",
- "dev": true
- },
- "node_modules/@mdx-js/react": {
- "version": "2.3.0",
- "resolved": "https://registry.npmjs.org/@mdx-js/react/-/react-2.3.0.tgz",
- "integrity": "sha512-zQH//gdOmuu7nt2oJR29vFhDv88oGPmVw6BggmrHeMI+xgEkp1B2dX9/bMBSYtK0dyLX/aOmesKS09g222K1/g==",
+ "node_modules/@lerna/create/node_modules/glob/node_modules/minimatch": {
+ "version": "8.0.4",
+ "resolved": "https://registry.npmjs.org/minimatch/-/minimatch-8.0.4.tgz",
+ "integrity": "sha512-W0Wvr9HyFXZRGIDgCicunpQ299OKXs9RgZfaukz4qAW/pJhcpUfupc9c+OObPOFueNy8VSrZgEmDtk6Kh4WzDA==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "@types/mdx": "^2.0.0",
- "@types/react": ">=16"
+ "brace-expansion": "^2.0.1"
},
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/unified"
+ "engines": {
+ "node": ">=16 || 14 >=14.17"
},
- "peerDependencies": {
- "react": ">=16"
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
}
},
- "node_modules/@ndelangen/get-tarball": {
- "version": "3.0.9",
- "resolved": "https://registry.npmjs.org/@ndelangen/get-tarball/-/get-tarball-3.0.9.tgz",
- "integrity": "sha512-9JKTEik4vq+yGosHYhZ1tiH/3WpUS0Nh0kej4Agndhox8pAdWhEx5knFVRcb/ya9knCRCs1rPxNrSXTDdfVqpA==",
- "dev": true,
- "dependencies": {
- "gunzip-maybe": "^1.4.2",
- "pump": "^3.0.0",
- "tar-fs": "^2.1.1"
+ "node_modules/@lerna/create/node_modules/glob/node_modules/minipass": {
+ "version": "4.2.8",
+ "resolved": "https://registry.npmjs.org/minipass/-/minipass-4.2.8.tgz",
+ "integrity": "sha512-fNzuVyifolSLFL4NzpF+wEF4qrgqaaKX0haXPQEdQ7NKAN+WecoKMHV09YcuL/DHxrUsYQOK3MiuDf7Ip2OXfQ==",
+ "dev": true,
+ "license": "ISC",
+ "engines": {
+ "node": ">=8"
}
},
- "node_modules/@ndelangen/get-tarball/node_modules/pump": {
- "version": "3.0.2",
- "resolved": "https://registry.npmjs.org/pump/-/pump-3.0.2.tgz",
- "integrity": "sha512-tUPXtzlGM8FE3P0ZL6DVs/3P58k9nk8/jZeQCurTJylQA8qFYzHFfhBJkuqyE0FifOsQ0uKWekiZ5g8wtr28cw==",
+ "node_modules/@lerna/create/node_modules/has-flag": {
+ "version": "4.0.0",
+ "resolved": "https://registry.npmjs.org/has-flag/-/has-flag-4.0.0.tgz",
+ "integrity": "sha512-EykJT/Q1KjTWctppgIAgfSO0tKVuZUjhgMr17kqTumMl6Afv3EISleU7qZUzoXDFTAHTDC4NOoG/ZxU3EvlMPQ==",
"dev": true,
"license": "MIT",
- "dependencies": {
- "end-of-stream": "^1.1.0",
- "once": "^1.3.1"
+ "engines": {
+ "node": ">=8"
}
},
- "node_modules/@nicolo-ribaudo/eslint-scope-5-internals": {
- "version": "5.1.1-v1",
- "resolved": "https://registry.npmjs.org/@nicolo-ribaudo/eslint-scope-5-internals/-/eslint-scope-5-internals-5.1.1-v1.tgz",
- "integrity": "sha512-54/JRvkLIzzDWshCWfuhadfrfZVPiElY8Fcgmg1HroEly/EDSszzhBAsarCux+D/kOslTRquNzuyGSmUSTTHGg==",
- "license": "MIT",
+ "node_modules/@lerna/create/node_modules/hosted-git-info": {
+ "version": "7.0.2",
+ "resolved": "https://registry.npmjs.org/hosted-git-info/-/hosted-git-info-7.0.2.tgz",
+ "integrity": "sha512-puUZAUKT5m8Zzvs72XWy3HtvVbTWljRE66cP60bxJzAqf2DgICo7lYTY2IHUmLnNpjYvw5bvmoHvPc0QO2a62w==",
+ "dev": true,
+ "license": "ISC",
"dependencies": {
- "eslint-scope": "5.1.1"
+ "lru-cache": "^10.0.1"
+ },
+ "engines": {
+ "node": "^16.14.0 || >=18.0.0"
}
},
- "node_modules/@nicolo-ribaudo/eslint-scope-5-internals/node_modules/eslint-scope": {
- "version": "5.1.1",
- "resolved": "https://registry.npmjs.org/eslint-scope/-/eslint-scope-5.1.1.tgz",
- "integrity": "sha512-2NxwbF/hZ0KpepYN0cNbo+FN6XoK7GaHlQhgx/hIZl6Va0bF45RQOOwhLIy8lQDbuCiadSLCBnH2CFYquit5bw==",
- "license": "BSD-2-Clause",
- "dependencies": {
- "esrecurse": "^4.3.0",
- "estraverse": "^4.1.1"
- },
+ "node_modules/@lerna/create/node_modules/human-signals": {
+ "version": "2.1.0",
+ "resolved": "https://registry.npmjs.org/human-signals/-/human-signals-2.1.0.tgz",
+ "integrity": "sha512-B4FFZ6q/T2jhhksgkbEW3HBvWIfDW85snkQgawt07S7J5QXTk6BkNV+0yAeZrM5QpMAdYlocGoljn0sJ/WQkFw==",
+ "dev": true,
+ "license": "Apache-2.0",
"engines": {
- "node": ">=8.0.0"
+ "node": ">=10.17.0"
}
},
- "node_modules/@nodelib/fs.scandir": {
- "version": "2.1.2",
- "resolved": "https://registry.npmjs.org/@nodelib/fs.scandir/-/fs.scandir-2.1.2.tgz",
- "integrity": "sha512-wrIBsjA5pl13f0RN4Zx4FNWmU71lv03meGKnqRUoCyan17s4V3WL92f3w3AIuWbNnpcrQyFBU5qMavJoB8d27w==",
+ "node_modules/@lerna/create/node_modules/ignore-walk": {
+ "version": "6.0.5",
+ "resolved": "https://registry.npmjs.org/ignore-walk/-/ignore-walk-6.0.5.tgz",
+ "integrity": "sha512-VuuG0wCnjhnylG1ABXT3dAuIpTNDs/G8jlpmwXY03fXoXy/8ZK8/T+hMzt8L4WnrLCJgdybqgPagnF/f97cg3A==",
+ "dev": true,
+ "license": "ISC",
"dependencies": {
- "@nodelib/fs.stat": "2.0.2",
- "run-parallel": "^1.1.9"
+ "minimatch": "^9.0.0"
},
"engines": {
- "node": ">= 8"
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/@nodelib/fs.stat": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/@nodelib/fs.stat/-/fs.stat-2.0.2.tgz",
- "integrity": "sha512-z8+wGWV2dgUhLqrtRYa03yDx4HWMvXKi1z8g3m2JyxAx8F7xk74asqPk5LAETjqDSGLFML/6CDl0+yFunSYicw==",
+ "node_modules/@lerna/create/node_modules/ignore-walk/node_modules/brace-expansion": {
+ "version": "2.0.1",
+ "resolved": "https://registry.npmjs.org/brace-expansion/-/brace-expansion-2.0.1.tgz",
+ "integrity": "sha512-XnAIvQ8eM+kC6aULx6wuQiwVsnzsi9d3WxzV3FpWTGA19F621kwdbsAcFKXgKUHZWsy+mY6iL1sHTxWEFCytDA==",
+ "dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "balanced-match": "^1.0.0"
+ }
+ },
+ "node_modules/@lerna/create/node_modules/ignore-walk/node_modules/minimatch": {
+ "version": "9.0.5",
+ "resolved": "https://registry.npmjs.org/minimatch/-/minimatch-9.0.5.tgz",
+ "integrity": "sha512-G6T0ZX48xgozx7587koeX9Ys2NYy6Gmv//P89sEte9V9whIapMNF4idKxnW2QtCcLiTWlb/wfCabAtAFWhhBow==",
+ "dev": true,
+ "license": "ISC",
+ "dependencies": {
+ "brace-expansion": "^2.0.1"
+ },
"engines": {
- "node": ">= 8"
+ "node": ">=16 || 14 >=14.17"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
}
},
- "node_modules/@nodelib/fs.walk": {
- "version": "1.2.3",
- "resolved": "https://registry.npmjs.org/@nodelib/fs.walk/-/fs.walk-1.2.3.tgz",
- "integrity": "sha512-l6t8xEhfK9Sa4YO5mIRdau7XSOADfmh3jCr0evNHdY+HNkW6xuQhgMH7D73VV6WpZOagrW0UludvMTiifiwTfA==",
+ "node_modules/@lerna/create/node_modules/inquirer": {
+ "version": "8.2.6",
+ "resolved": "https://registry.npmjs.org/inquirer/-/inquirer-8.2.6.tgz",
+ "integrity": "sha512-M1WuAmb7pn9zdFRtQYk26ZBoY043Sse0wVDdk4Bppr+JOXyQYybdtvK+l9wUibhtjdjvtoiNy8tk+EgsYIUqKg==",
+ "dev": true,
+ "license": "MIT",
"dependencies": {
- "@nodelib/fs.scandir": "2.1.2",
- "fastq": "^1.6.0"
+ "ansi-escapes": "^4.2.1",
+ "chalk": "^4.1.1",
+ "cli-cursor": "^3.1.0",
+ "cli-width": "^3.0.0",
+ "external-editor": "^3.0.3",
+ "figures": "^3.0.0",
+ "lodash": "^4.17.21",
+ "mute-stream": "0.0.8",
+ "ora": "^5.4.1",
+ "run-async": "^2.4.0",
+ "rxjs": "^7.5.5",
+ "string-width": "^4.1.0",
+ "strip-ansi": "^6.0.0",
+ "through": "^2.3.6",
+ "wrap-ansi": "^6.0.1"
},
"engines": {
- "node": ">= 8"
+ "node": ">=12.0.0"
}
},
- "node_modules/@npmcli/fs": {
- "version": "3.1.0",
- "resolved": "https://registry.npmjs.org/@npmcli/fs/-/fs-3.1.0.tgz",
- "integrity": "sha512-7kZUAaLscfgbwBQRbvdMYaZOWyMEcPTH/tJjnyAWJ/dvvs9Ef+CERx/qJb9GExJpl1qipaDGn7KqHnFGGixd0w==",
+ "node_modules/@lerna/create/node_modules/inquirer/node_modules/chalk": {
+ "version": "4.1.2",
+ "resolved": "https://registry.npmjs.org/chalk/-/chalk-4.1.2.tgz",
+ "integrity": "sha512-oKnbhFyRIXpUuez8iBMmyEa4nbj4IOQyuhc/wy9kY7/WVPcwIO9VA668Pu8RkO7+0G76SLROeyw9CpQ061i4mA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "semver": "^7.3.5"
+ "ansi-styles": "^4.1.0",
+ "supports-color": "^7.1.0"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": ">=10"
+ },
+ "funding": {
+ "url": "https://github.com/chalk/chalk?sponsor=1"
}
},
- "node_modules/@npmcli/git": {
- "version": "4.1.0",
- "resolved": "https://registry.npmjs.org/@npmcli/git/-/git-4.1.0.tgz",
- "integrity": "sha512-9hwoB3gStVfa0N31ymBmrX+GuDGdVA/QWShZVqE0HK2Af+7QGGrCTbZia/SW0ImUTjTne7SP91qxDmtXvDHRPQ==",
+ "node_modules/@lerna/create/node_modules/is-ci": {
+ "version": "3.0.1",
+ "resolved": "https://registry.npmjs.org/is-ci/-/is-ci-3.0.1.tgz",
+ "integrity": "sha512-ZYvCgrefwqoQ6yTyYUbQu64HsITZ3NfKX1lzaEYdkTDcfKzzCI/wthRRYKkdjHKFVgNiXKAKm65Zo1pk2as/QQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "@npmcli/promise-spawn": "^6.0.0",
- "lru-cache": "^7.4.4",
- "npm-pick-manifest": "^8.0.0",
- "proc-log": "^3.0.0",
- "promise-inflight": "^1.0.1",
- "promise-retry": "^2.0.1",
- "semver": "^7.3.5",
- "which": "^3.0.0"
+ "ci-info": "^3.2.0"
},
+ "bin": {
+ "is-ci": "bin.js"
+ }
+ },
+ "node_modules/@lerna/create/node_modules/is-fullwidth-code-point": {
+ "version": "3.0.0",
+ "resolved": "https://registry.npmjs.org/is-fullwidth-code-point/-/is-fullwidth-code-point-3.0.0.tgz",
+ "integrity": "sha512-zymm5+u+sCsSWyD9qNaejV3DFvhCKclKdizYaJUuHA83RLjb7nSuGnddCHGv0hk+KY7BMAlsWeK4Ueg6EV6XQg==",
+ "dev": true,
+ "license": "MIT",
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": ">=8"
}
},
- "node_modules/@npmcli/git/node_modules/lru-cache": {
- "version": "7.18.3",
- "resolved": "https://registry.npmjs.org/lru-cache/-/lru-cache-7.18.3.tgz",
- "integrity": "sha512-jumlc0BIUrS3qJGgIkWZsyfAM7NCWiBcCDhnd+3NNM5KbBmLTgHVfWBcg6W+rLUsIpzpERPsvwUP7CckAQSOoA==",
+ "node_modules/@lerna/create/node_modules/is-stream": {
+ "version": "2.0.0",
+ "resolved": "https://registry.npmjs.org/is-stream/-/is-stream-2.0.0.tgz",
+ "integrity": "sha512-XCoy+WlUr7d1+Z8GgSuXmpuUFC9fOhRXglJMx+dwLKTkL44Cjd4W1Z5P+BQZpr+cR93aGP4S/s7Ftw6Nd/kiEw==",
"dev": true,
+ "license": "MIT",
"engines": {
- "node": ">=12"
+ "node": ">=8"
}
},
- "node_modules/@npmcli/git/node_modules/which": {
- "version": "3.0.1",
- "resolved": "https://registry.npmjs.org/which/-/which-3.0.1.tgz",
- "integrity": "sha512-XA1b62dzQzLfaEOSQFTCOd5KFf/1VSzZo7/7TUjnya6u0vGGKzU96UQBZTAThCb2j4/xjBAyii1OhRLJEivHvg==",
+ "node_modules/@lerna/create/node_modules/js-yaml": {
+ "version": "4.1.0",
+ "resolved": "https://registry.npmjs.org/js-yaml/-/js-yaml-4.1.0.tgz",
+ "integrity": "sha512-wpxZs9NoxZaJESJGIZTyDEaYpl0FKSA+FB9aJiyemKhMwkxQg63h4T1KJgUGHpTqPDNRcmmYLugrRjJlBtWvRA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "isexe": "^2.0.0"
+ "argparse": "^2.0.1"
},
"bin": {
- "node-which": "bin/which.js"
+ "js-yaml": "bin/js-yaml.js"
+ }
+ },
+ "node_modules/@lerna/create/node_modules/jsonfile": {
+ "version": "6.1.0",
+ "resolved": "https://registry.npmjs.org/jsonfile/-/jsonfile-6.1.0.tgz",
+ "integrity": "sha512-5dgndWOriYSm5cnYaJNhalLNDKOqFwyDB/rr1E9ZsGciGvKPs8R2xYGCacuf3z6K1YKDz182fd+fY3cn3pMqXQ==",
+ "dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "universalify": "^2.0.0"
},
- "engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "optionalDependencies": {
+ "graceful-fs": "^4.1.6"
}
},
- "node_modules/@npmcli/installed-package-contents": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/@npmcli/installed-package-contents/-/installed-package-contents-2.0.2.tgz",
- "integrity": "sha512-xACzLPhnfD51GKvTOOuNX2/V4G4mz9/1I2MfDoye9kBM3RYe5g2YbscsaGoTlaWqkxeiapBWyseULVKpSVHtKQ==",
+ "node_modules/@lerna/create/node_modules/make-dir": {
+ "version": "4.0.0",
+ "resolved": "https://registry.npmjs.org/make-dir/-/make-dir-4.0.0.tgz",
+ "integrity": "sha512-hXdUTZYIVOt1Ex//jAQi+wTZZpUpwBj/0QsOzqegb3rGMMeJiSEu5xLHnYfBrRV4RH2+OCSOO95Is/7x1WJ4bw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "npm-bundled": "^3.0.0",
- "npm-normalize-package-bin": "^3.0.0"
+ "semver": "^7.5.3"
},
- "bin": {
- "installed-package-contents": "lib/index.js"
+ "engines": {
+ "node": ">=10"
},
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
+ }
+ },
+ "node_modules/@lerna/create/node_modules/mimic-fn": {
+ "version": "2.1.0",
+ "resolved": "https://registry.npmjs.org/mimic-fn/-/mimic-fn-2.1.0.tgz",
+ "integrity": "sha512-OqbOk5oEQeAZ8WXWydlu9HJjz9WVdEIvamMCcXmuqUYjTknH/sqsWvhQ3vgwKFRR1HpjvNBKQ37nbJgYzGqGcg==",
+ "dev": true,
+ "license": "MIT",
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": ">=6"
}
},
- "node_modules/@npmcli/installed-package-contents/node_modules/npm-bundled": {
- "version": "3.0.1",
- "resolved": "https://registry.npmjs.org/npm-bundled/-/npm-bundled-3.0.1.tgz",
- "integrity": "sha512-+AvaheE/ww1JEwRHOrn4WHNzOxGtVp+adrg2AeZS/7KuxGUYFuBta98wYpfHBbJp6Tg6j1NKSEVHNcfZzJHQwQ==",
+ "node_modules/@lerna/create/node_modules/minimatch": {
+ "version": "3.0.5",
+ "resolved": "https://registry.npmjs.org/minimatch/-/minimatch-3.0.5.tgz",
+ "integrity": "sha512-tUpxzX0VAzJHjLu0xUfFv1gwVp9ba3IOuRAVH2EGuRW8a5emA2FlACLqiT/lDVtS1W+TGNwqz3sWaNyLgDJWuw==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "npm-normalize-package-bin": "^3.0.0"
+ "brace-expansion": "^1.1.7"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": "*"
}
},
- "node_modules/@npmcli/installed-package-contents/node_modules/npm-normalize-package-bin": {
- "version": "3.0.1",
- "resolved": "https://registry.npmjs.org/npm-normalize-package-bin/-/npm-normalize-package-bin-3.0.1.tgz",
- "integrity": "sha512-dMxCf+zZ+3zeQZXKxmyuCKlIDPGuv8EF940xbkC4kQVDTtqoh6rJFO+JTKSA6/Rwi0getWmtuy4Itup0AMcaDQ==",
+ "node_modules/@lerna/create/node_modules/minipass": {
+ "version": "7.1.2",
+ "resolved": "https://registry.npmjs.org/minipass/-/minipass-7.1.2.tgz",
+ "integrity": "sha512-qOOzS1cBTWYF4BH8fVePDBOO9iptMnGUEZwNc/cMWnTV2nVLZ7VoNWEPHkYczZA0pdoA7dl6e7FL659nX9S2aw==",
"dev": true,
+ "license": "ISC",
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": ">=16 || 14 >=14.17"
}
},
- "node_modules/@npmcli/node-gyp": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/@npmcli/node-gyp/-/node-gyp-3.0.0.tgz",
- "integrity": "sha512-gp8pRXC2oOxu0DUE1/M3bYtb1b3/DbJ5aM113+XJBgfXdussRAsX0YOrOhdd8WvnAR6auDBvJomGAkLKA5ydxA==",
+ "node_modules/@lerna/create/node_modules/node-fetch": {
+ "version": "2.6.7",
+ "resolved": "https://registry.npmjs.org/node-fetch/-/node-fetch-2.6.7.tgz",
+ "integrity": "sha512-ZjMPFEfVx5j+y2yF35Kzx5sF7kDzxuDj6ziH4FFbOp87zKDZNx8yExJIb05OGF4Nlt9IHFIMBkRl41VdvcNdbQ==",
"dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "whatwg-url": "^5.0.0"
+ },
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": "4.x || >=6.0.0"
+ },
+ "peerDependencies": {
+ "encoding": "^0.1.0"
+ },
+ "peerDependenciesMeta": {
+ "encoding": {
+ "optional": true
+ }
}
},
- "node_modules/@npmcli/promise-spawn": {
- "version": "6.0.2",
- "resolved": "https://registry.npmjs.org/@npmcli/promise-spawn/-/promise-spawn-6.0.2.tgz",
- "integrity": "sha512-gGq0NJkIGSwdbUt4yhdF8ZrmkGKVz9vAdVzpOfnom+V8PLSmSOVhZwbNvZZS1EYcJN5hzzKBxmmVVAInM6HQLg==",
+ "node_modules/@lerna/create/node_modules/npm-package-arg": {
+ "version": "11.0.2",
+ "resolved": "https://registry.npmjs.org/npm-package-arg/-/npm-package-arg-11.0.2.tgz",
+ "integrity": "sha512-IGN0IAwmhDJwy13Wc8k+4PEbTPhpJnMtfR53ZbOyjkvmEcLS4nCwp6mvMWjS5sUjeiW3mpx6cHmuhKEu9XmcQw==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "which": "^3.0.0"
+ "hosted-git-info": "^7.0.0",
+ "proc-log": "^4.0.0",
+ "semver": "^7.3.5",
+ "validate-npm-package-name": "^5.0.0"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": "^16.14.0 || >=18.0.0"
}
},
- "node_modules/@npmcli/promise-spawn/node_modules/which": {
- "version": "3.0.1",
- "resolved": "https://registry.npmjs.org/which/-/which-3.0.1.tgz",
- "integrity": "sha512-XA1b62dzQzLfaEOSQFTCOd5KFf/1VSzZo7/7TUjnya6u0vGGKzU96UQBZTAThCb2j4/xjBAyii1OhRLJEivHvg==",
+ "node_modules/@lerna/create/node_modules/npm-packlist": {
+ "version": "8.0.2",
+ "resolved": "https://registry.npmjs.org/npm-packlist/-/npm-packlist-8.0.2.tgz",
+ "integrity": "sha512-shYrPFIS/JLP4oQmAwDyk5HcyysKW8/JLTEA32S0Z5TzvpaeeX2yMFfoK1fjEBnCBvVyIB/Jj/GBFdm0wsgzbA==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "isexe": "^2.0.0"
- },
- "bin": {
- "node-which": "bin/which.js"
+ "ignore-walk": "^6.0.4"
},
"engines": {
"node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/@npmcli/run-script": {
- "version": "6.0.2",
- "resolved": "https://registry.npmjs.org/@npmcli/run-script/-/run-script-6.0.2.tgz",
- "integrity": "sha512-NCcr1uQo1k5U+SYlnIrbAh3cxy+OQT1VtqiAbxdymSlptbzBb62AjH2xXgjNCoP073hoa1CfCAcwoZ8k96C4nA==",
+ "node_modules/@lerna/create/node_modules/npm-run-path": {
+ "version": "4.0.1",
+ "resolved": "https://registry.npmjs.org/npm-run-path/-/npm-run-path-4.0.1.tgz",
+ "integrity": "sha512-S48WzZW777zhNIrn7gxOlISNAqi9ZC/uQFnRdbeIHhZhCA6UqpkOT8T1G7BvfdgP4Er8gF4sUbaS0i7QvIfCWw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "@npmcli/node-gyp": "^3.0.0",
- "@npmcli/promise-spawn": "^6.0.0",
- "node-gyp": "^9.0.0",
- "read-package-json-fast": "^3.0.0",
- "which": "^3.0.0"
+ "path-key": "^3.0.0"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": ">=8"
}
},
- "node_modules/@npmcli/run-script/node_modules/which": {
- "version": "3.0.1",
- "resolved": "https://registry.npmjs.org/which/-/which-3.0.1.tgz",
- "integrity": "sha512-XA1b62dzQzLfaEOSQFTCOd5KFf/1VSzZo7/7TUjnya6u0vGGKzU96UQBZTAThCb2j4/xjBAyii1OhRLJEivHvg==",
+ "node_modules/@lerna/create/node_modules/onetime": {
+ "version": "5.1.2",
+ "resolved": "https://registry.npmjs.org/onetime/-/onetime-5.1.2.tgz",
+ "integrity": "sha512-kbpaSSGJTWdAY5KPVeMOKXSrPtr8C8C7wodJbcsd51jRnmD+GZu8Y0VoU6Dm5Z4vWr0Ig/1NKuWRKf7j5aaYSg==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "isexe": "^2.0.0"
- },
- "bin": {
- "node-which": "bin/which.js"
+ "mimic-fn": "^2.1.0"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": ">=6"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@nrwl/devkit": {
- "version": "16.6.0",
- "resolved": "https://registry.npmjs.org/@nrwl/devkit/-/devkit-16.6.0.tgz",
- "integrity": "sha512-xZEN6wfA1uJwv+FVRQFOHsCcpvGvIYGx2zutbzungDodWkfzlJ3tzIGqYjIpPCBVT83erM6Gscnka2W46AuKfA==",
+ "node_modules/@lerna/create/node_modules/ora": {
+ "version": "5.4.1",
+ "resolved": "https://registry.npmjs.org/ora/-/ora-5.4.1.tgz",
+ "integrity": "sha512-5b6Y85tPxZZ7QytO+BQzysW31HJku27cRIlkbAXaNx+BdcVi+LlRFmVXzeF6a7JCwJpyw5c4b+YSVImQIrBpuQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "@nx/devkit": "16.6.0"
+ "bl": "^4.1.0",
+ "chalk": "^4.1.0",
+ "cli-cursor": "^3.1.0",
+ "cli-spinners": "^2.5.0",
+ "is-interactive": "^1.0.0",
+ "is-unicode-supported": "^0.1.0",
+ "log-symbols": "^4.1.0",
+ "strip-ansi": "^6.0.0",
+ "wcwidth": "^1.0.1"
+ },
+ "engines": {
+ "node": ">=10"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@nrwl/tao": {
- "version": "16.6.0",
- "resolved": "https://registry.npmjs.org/@nrwl/tao/-/tao-16.6.0.tgz",
- "integrity": "sha512-NQkDhmzlR1wMuYzzpl4XrKTYgyIzELdJ+dVrNKf4+p4z5WwKGucgRBj60xMQ3kdV25IX95/fmMDB8qVp/pNQ0Q==",
+ "node_modules/@lerna/create/node_modules/path-key": {
+ "version": "3.1.1",
+ "resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
+ "integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
"dev": true,
- "dependencies": {
- "nx": "16.6.0",
- "tslib": "^2.3.0"
- },
- "bin": {
- "tao": "index.js"
+ "license": "MIT",
+ "engines": {
+ "node": ">=8"
}
},
- "node_modules/@nx/devkit": {
- "version": "16.6.0",
- "resolved": "https://registry.npmjs.org/@nx/devkit/-/devkit-16.6.0.tgz",
- "integrity": "sha512-rhJ0y+MSPHDuoZPxsOYdj/n5ks+gK74TIMgTb8eZgPT/uR86a4oxf62wUQXgECedR5HzLE2HunbnoLhhJXmpJw==",
+ "node_modules/@lerna/create/node_modules/resolve-from": {
+ "version": "5.0.0",
+ "resolved": "https://registry.npmjs.org/resolve-from/-/resolve-from-5.0.0.tgz",
+ "integrity": "sha512-qYg9KP24dD5qka9J47d0aVky0N+b4fTU89LN9iDnjB5waksiC49rvMB0PrUJQGoTmH50XPiqOvAjDfaijGxYZw==",
"dev": true,
- "dependencies": {
- "@nrwl/devkit": "16.6.0",
- "ejs": "^3.1.7",
- "ignore": "^5.0.4",
- "semver": "7.5.3",
- "tmp": "~0.2.1",
- "tslib": "^2.3.0"
- },
- "peerDependencies": {
- "nx": ">= 15 <= 17"
+ "license": "MIT",
+ "engines": {
+ "node": ">=8"
}
},
- "node_modules/@nx/devkit/node_modules/lru-cache": {
- "version": "6.0.0",
- "resolved": "https://registry.npmjs.org/lru-cache/-/lru-cache-6.0.0.tgz",
- "integrity": "sha512-Jo6dJ04CmSjuznwJSS3pUeWmd/H0ffTlkXXgwZi+eq1UCmqQwCh+eLsYOYCwY991i2Fah4h1BEMCx4qThGbsiA==",
+ "node_modules/@lerna/create/node_modules/restore-cursor": {
+ "version": "3.1.0",
+ "resolved": "https://registry.npmjs.org/restore-cursor/-/restore-cursor-3.1.0.tgz",
+ "integrity": "sha512-l+sSefzHpj5qimhFSE5a8nufZYAM3sBSVMAPtYkmC+4EH2anSGaEMXSD0izRQbu9nfyQ9y5JrVmp7E8oZrUjvA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "yallist": "^4.0.0"
+ "onetime": "^5.1.0",
+ "signal-exit": "^3.0.2"
},
"engines": {
- "node": ">=10"
+ "node": ">=8"
}
},
- "node_modules/@nx/devkit/node_modules/semver": {
- "version": "7.5.3",
- "resolved": "https://registry.npmjs.org/semver/-/semver-7.5.3.tgz",
- "integrity": "sha512-QBlUtyVk/5EeHbi7X0fw6liDZc7BBmEaSYn01fMU1OUYbf6GPsbTtd8WmnqbI20SeycoHSeiybkE/q1Q+qlThQ==",
+ "node_modules/@lerna/create/node_modules/rimraf": {
+ "version": "4.4.1",
+ "resolved": "https://registry.npmjs.org/rimraf/-/rimraf-4.4.1.tgz",
+ "integrity": "sha512-Gk8NlF062+T9CqNGn6h4tls3k6T1+/nXdOcSZVikNVtlRdYpA7wRJJMoXmuvOnLW844rPjdQ7JgXCYM6PPC/og==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "lru-cache": "^6.0.0"
+ "glob": "^9.2.0"
},
"bin": {
- "semver": "bin/semver.js"
+ "rimraf": "dist/cjs/src/bin.js"
},
"engines": {
- "node": ">=10"
+ "node": ">=14"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
}
},
- "node_modules/@nx/devkit/node_modules/tmp": {
- "version": "0.2.3",
- "resolved": "https://registry.npmjs.org/tmp/-/tmp-0.2.3.tgz",
- "integrity": "sha512-nZD7m9iCPC5g0pYmcaxogYKggSfLsdxl8of3Q/oIbqCqLLIO9IAF0GWjX1z9NZRHPiXv8Wex4yDCaZsgEw0Y8w==",
+ "node_modules/@lerna/create/node_modules/rxjs": {
+ "version": "7.8.1",
+ "resolved": "https://registry.npmjs.org/rxjs/-/rxjs-7.8.1.tgz",
+ "integrity": "sha512-AA3TVj+0A2iuIoQkWEK/tqFjBq2j+6PO6Y0zJcvzLAFhEFIO3HL0vls9hWLncZbAAbK0mar7oZ4V079I/qPMxg==",
"dev": true,
- "engines": {
- "node": ">=14.14"
+ "license": "Apache-2.0",
+ "dependencies": {
+ "tslib": "^2.1.0"
}
},
- "node_modules/@nx/devkit/node_modules/yallist": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/yallist/-/yallist-4.0.0.tgz",
- "integrity": "sha512-3wdGidZyq5PB084XLES5TpOSRA3wjXAlIWMhum2kRcv/41Sn2emQ0dycQW4uZXLejwKvg6EsvbdlVL+FYEct7A==",
- "dev": true
- },
- "node_modules/@nx/nx-darwin-arm64": {
- "version": "16.6.0",
- "resolved": "https://registry.npmjs.org/@nx/nx-darwin-arm64/-/nx-darwin-arm64-16.6.0.tgz",
- "integrity": "sha512-8nJuqcWG/Ob39rebgPLpv2h/V46b9Rqqm/AGH+bYV9fNJpxgMXclyincbMIWvfYN2tW+Vb9DusiTxV6RPrLapA==",
- "cpu": [
- "arm64"
- ],
+ "node_modules/@lerna/create/node_modules/ssri": {
+ "version": "10.0.6",
+ "resolved": "https://registry.npmjs.org/ssri/-/ssri-10.0.6.tgz",
+ "integrity": "sha512-MGrFH9Z4NP9Iyhqn16sDtBpRRNJ0Y2hNa6D65h736fVSaPCHr4DM4sWUNvVaSuC+0OBGhwsrydQwmgfg5LncqQ==",
"dev": true,
- "optional": true,
- "os": [
- "darwin"
- ],
+ "license": "ISC",
+ "dependencies": {
+ "minipass": "^7.0.3"
+ },
"engines": {
- "node": ">= 10"
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/@nx/nx-darwin-x64": {
- "version": "16.6.0",
- "resolved": "https://registry.npmjs.org/@nx/nx-darwin-x64/-/nx-darwin-x64-16.6.0.tgz",
- "integrity": "sha512-T4DV0/2PkPZjzjmsmQEyjPDNBEKc4Rhf7mbIZlsHXj27BPoeNjEcbjtXKuOZHZDIpGFYECGT/sAF6C2NVYgmxw==",
- "cpu": [
- "x64"
- ],
+ "node_modules/@lerna/create/node_modules/string-width": {
+ "version": "4.2.3",
+ "resolved": "https://registry.npmjs.org/string-width/-/string-width-4.2.3.tgz",
+ "integrity": "sha512-wKyQRQpjJ0sIp62ErSZdGsjMJWsap5oRNihHhu6G7JVO/9jIB6UyevL+tXuOqrng8j/cxKTWyWUwvSTriiZz/g==",
"dev": true,
- "optional": true,
- "os": [
- "darwin"
- ],
+ "license": "MIT",
+ "dependencies": {
+ "emoji-regex": "^8.0.0",
+ "is-fullwidth-code-point": "^3.0.0",
+ "strip-ansi": "^6.0.1"
+ },
"engines": {
- "node": ">= 10"
+ "node": ">=8"
}
},
- "node_modules/@nx/nx-freebsd-x64": {
- "version": "16.6.0",
- "resolved": "https://registry.npmjs.org/@nx/nx-freebsd-x64/-/nx-freebsd-x64-16.6.0.tgz",
- "integrity": "sha512-Ck/yejYgp65dH9pbExKN/X0m22+xS3rWF1DBr2LkP6j1zJaweRc3dT83BWgt5mCjmcmZVk3J8N01AxULAzUAqA==",
- "cpu": [
- "x64"
- ],
+ "node_modules/@lerna/create/node_modules/supports-color": {
+ "version": "7.2.0",
+ "resolved": "https://registry.npmjs.org/supports-color/-/supports-color-7.2.0.tgz",
+ "integrity": "sha512-qpCAvRl9stuOHveKsn7HncJRvv501qIacKzQlO/+Lwxc9+0q2wLyv4Dfvt80/DPn2pqOBsJdDiogXGR9+OvwRw==",
"dev": true,
- "optional": true,
- "os": [
- "freebsd"
- ],
+ "license": "MIT",
+ "dependencies": {
+ "has-flag": "^4.0.0"
+ },
"engines": {
- "node": ">= 10"
+ "node": ">=8"
}
},
- "node_modules/@nx/nx-linux-arm-gnueabihf": {
- "version": "16.6.0",
- "resolved": "https://registry.npmjs.org/@nx/nx-linux-arm-gnueabihf/-/nx-linux-arm-gnueabihf-16.6.0.tgz",
- "integrity": "sha512-eyk/R1mBQ3X0PCSS+Cck3onvr3wmZVmM/+x0x9Ai02Vm6q9Eq6oZ1YtZGQsklNIyw1vk2WV9rJCStfu9mLecEw==",
- "cpu": [
- "arm"
- ],
+ "node_modules/@lerna/create/node_modules/universal-user-agent": {
+ "version": "6.0.1",
+ "resolved": "https://registry.npmjs.org/universal-user-agent/-/universal-user-agent-6.0.1.tgz",
+ "integrity": "sha512-yCzhz6FN2wU1NiiQRogkTQszlQSlpWaw8SvVegAc+bDxbzHgh1vX8uIe8OYyMH6DwH+sdTJsgMl36+mSMdRJIQ==",
"dev": true,
- "optional": true,
- "os": [
- "linux"
- ],
- "engines": {
- "node": ">= 10"
- }
+ "license": "ISC"
},
- "node_modules/@nx/nx-linux-arm64-gnu": {
- "version": "16.6.0",
- "resolved": "https://registry.npmjs.org/@nx/nx-linux-arm64-gnu/-/nx-linux-arm64-gnu-16.6.0.tgz",
- "integrity": "sha512-S0qFFdQFDmBIEZqBAJl4K47V3YuMvDvthbYE0enXrXApWgDApmhtxINXSOjSus7DNq9kMrgtSDGkBmoBot61iw==",
- "cpu": [
- "arm64"
- ],
+ "node_modules/@lerna/create/node_modules/universalify": {
+ "version": "2.0.1",
+ "resolved": "https://registry.npmjs.org/universalify/-/universalify-2.0.1.tgz",
+ "integrity": "sha512-gptHNQghINnc/vTGIk0SOFGFNXw7JVrlRUtConJRlvaw6DuX0wO5Jeko9sWrMBhh+PsYAZ7oXAiOnf/UKogyiw==",
"dev": true,
- "optional": true,
- "os": [
- "linux"
- ],
+ "license": "MIT",
"engines": {
- "node": ">= 10"
+ "node": ">= 10.0.0"
}
},
- "node_modules/@nx/nx-linux-arm64-musl": {
- "version": "16.6.0",
- "resolved": "https://registry.npmjs.org/@nx/nx-linux-arm64-musl/-/nx-linux-arm64-musl-16.6.0.tgz",
- "integrity": "sha512-TXWY5VYtg2wX/LWxyrUkDVpqCyJHF7fWoVMUSlFe+XQnk9wp/yIbq2s0k3h8I4biYb6AgtcVqbR4ID86lSNuMA==",
- "cpu": [
- "arm64"
- ],
+ "node_modules/@lerna/create/node_modules/upath": {
+ "version": "2.0.1",
+ "resolved": "https://registry.npmjs.org/upath/-/upath-2.0.1.tgz",
+ "integrity": "sha512-1uEe95xksV1O0CYKXo8vQvN1JEbtJp7lb7C5U9HMsIp6IVwntkH/oNUzyVNQSd4S1sYk2FpSSW44FqMc8qee5w==",
"dev": true,
- "optional": true,
- "os": [
- "linux"
- ],
+ "license": "MIT",
"engines": {
- "node": ">= 10"
+ "node": ">=4",
+ "yarn": "*"
}
},
- "node_modules/@nx/nx-linux-x64-gnu": {
- "version": "16.6.0",
- "resolved": "https://registry.npmjs.org/@nx/nx-linux-x64-gnu/-/nx-linux-x64-gnu-16.6.0.tgz",
- "integrity": "sha512-qQIpSVN8Ij4oOJ5v+U+YztWJ3YQkeCIevr4RdCE9rDilfq9RmBD94L4VDm7NRzYBuQL8uQxqWzGqb7ZW4mfHpw==",
- "cpu": [
- "x64"
- ],
+ "node_modules/@lerna/create/node_modules/uuid": {
+ "version": "10.0.0",
+ "resolved": "https://registry.npmjs.org/uuid/-/uuid-10.0.0.tgz",
+ "integrity": "sha512-8XkAphELsDnEGrDxUOHB3RGvXz6TeuYSGEZBOjtTtPm2lwhGBjLgOzLHB63IUWfBpNucQjND6d3AOudO+H3RWQ==",
"dev": true,
- "optional": true,
- "os": [
- "linux"
+ "funding": [
+ "https://github.com/sponsors/broofa",
+ "https://github.com/sponsors/ctavan"
],
- "engines": {
- "node": ">= 10"
+ "license": "MIT",
+ "bin": {
+ "uuid": "dist/bin/uuid"
}
},
- "node_modules/@nx/nx-linux-x64-musl": {
- "version": "16.6.0",
- "resolved": "https://registry.npmjs.org/@nx/nx-linux-x64-musl/-/nx-linux-x64-musl-16.6.0.tgz",
- "integrity": "sha512-EYOHe11lfVfEfZqSAIa1c39mx2Obr4mqd36dBZx+0UKhjrcmWiOdsIVYMQSb3n0TqB33BprjI4p9ZcFSDuoNbA==",
- "cpu": [
- "x64"
- ],
+ "node_modules/@lerna/create/node_modules/validate-npm-package-name": {
+ "version": "5.0.1",
+ "resolved": "https://registry.npmjs.org/validate-npm-package-name/-/validate-npm-package-name-5.0.1.tgz",
+ "integrity": "sha512-OljLrQ9SQdOUqTaQxqL5dEfZWrXExyyWsozYlAWFawPVNuD83igl7uJD2RTkNMbniIYgt8l81eCJGIdQF7avLQ==",
"dev": true,
- "optional": true,
- "os": [
- "linux"
- ],
+ "license": "ISC",
"engines": {
- "node": ">= 10"
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/@nx/nx-win32-arm64-msvc": {
- "version": "16.6.0",
- "resolved": "https://registry.npmjs.org/@nx/nx-win32-arm64-msvc/-/nx-win32-arm64-msvc-16.6.0.tgz",
- "integrity": "sha512-f1BmuirOrsAGh5+h/utkAWNuqgohvBoekQgMxYcyJxSkFN+pxNG1U68P59Cidn0h9mkyonxGVCBvWwJa3svVFA==",
- "cpu": [
- "arm64"
- ],
+ "node_modules/@lerna/create/node_modules/write-file-atomic": {
+ "version": "5.0.1",
+ "resolved": "https://registry.npmjs.org/write-file-atomic/-/write-file-atomic-5.0.1.tgz",
+ "integrity": "sha512-+QU2zd6OTD8XWIJCbffaiQeH9U73qIqafo1x6V1snCWYGJf6cVE0cDR4D8xRzcEnfI21IFrUPzPGtcPf8AC+Rw==",
"dev": true,
- "optional": true,
- "os": [
- "win32"
- ],
+ "license": "ISC",
+ "dependencies": {
+ "imurmurhash": "^0.1.4",
+ "signal-exit": "^4.0.1"
+ },
"engines": {
- "node": ">= 10"
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/@nx/nx-win32-x64-msvc": {
- "version": "16.6.0",
- "resolved": "https://registry.npmjs.org/@nx/nx-win32-x64-msvc/-/nx-win32-x64-msvc-16.6.0.tgz",
- "integrity": "sha512-UmTTjFLpv4poVZE3RdUHianU8/O9zZYBiAnTRq5spwSDwxJHnLTZBUxFFf3ztCxeHOUIfSyW9utpGfCMCptzvQ==",
- "cpu": [
- "x64"
- ],
+ "node_modules/@lerna/create/node_modules/write-file-atomic/node_modules/signal-exit": {
+ "version": "4.1.0",
+ "resolved": "https://registry.npmjs.org/signal-exit/-/signal-exit-4.1.0.tgz",
+ "integrity": "sha512-bzyZ1e88w9O1iNJbKnOlvYTrWPDl46O1bG0D3XInv+9tkPrxrN8jUUTiFlDkkmKWgn1M6CfIA13SuGqOa9Korw==",
"dev": true,
- "optional": true,
- "os": [
- "win32"
- ],
+ "license": "ISC",
"engines": {
- "node": ">= 10"
+ "node": ">=14"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
}
},
- "node_modules/@octokit/auth-token": {
- "version": "2.5.0",
- "resolved": "https://registry.npmjs.org/@octokit/auth-token/-/auth-token-2.5.0.tgz",
- "integrity": "sha512-r5FVUJCOLl19AxiuZD2VRZ/ORjp/4IN98Of6YJoJOkY75CIBuYfmiNHGrDwXr+aLGG55igl9QrxX3hbiXlLb+g==",
- "dependencies": {
- "@octokit/types": "^6.0.3"
+ "node_modules/@lerna/create/node_modules/yargs-parser": {
+ "version": "21.1.1",
+ "resolved": "https://registry.npmjs.org/yargs-parser/-/yargs-parser-21.1.1.tgz",
+ "integrity": "sha512-tVpsJW7DdjecAiFpbIB1e3qxIQsE6NoPc5/eTdrbbIC4h0LVsWhnoa3g+m2HclBIujHzsxZ4VJVA+GUuc2/LBw==",
+ "dev": true,
+ "license": "ISC",
+ "engines": {
+ "node": ">=12"
}
},
- "node_modules/@octokit/core": {
- "version": "3.6.0",
- "resolved": "https://registry.npmjs.org/@octokit/core/-/core-3.6.0.tgz",
- "integrity": "sha512-7RKRKuA4xTjMhY+eG3jthb3hlZCsOwg3rztWh75Xc+ShDWOfDDATWbeZpAHBNRpm4Tv9WgBMOy1zEJYXG6NJ7Q==",
+ "node_modules/@mdx-js/react": {
+ "version": "3.1.0",
+ "resolved": "https://registry.npmjs.org/@mdx-js/react/-/react-3.1.0.tgz",
+ "integrity": "sha512-QjHtSaoameoalGnKDT3FoIl4+9RwyTmo9ZJGBdLOks/YOiWHoRDI3PUwEzOE7kEmGcV3AFcp9K6dYu9rEuKLAQ==",
+ "dev": true,
"dependencies": {
- "@octokit/auth-token": "^2.4.4",
- "@octokit/graphql": "^4.5.8",
- "@octokit/request": "^5.6.3",
- "@octokit/request-error": "^2.0.5",
- "@octokit/types": "^6.0.3",
- "before-after-hook": "^2.2.0",
- "universal-user-agent": "^6.0.0"
+ "@types/mdx": "^2.0.0"
+ },
+ "funding": {
+ "type": "opencollective",
+ "url": "https://opencollective.com/unified"
+ },
+ "peerDependencies": {
+ "@types/react": ">=16",
+ "react": ">=16"
}
},
- "node_modules/@octokit/core/node_modules/@octokit/endpoint": {
- "version": "6.0.12",
- "resolved": "https://registry.npmjs.org/@octokit/endpoint/-/endpoint-6.0.12.tgz",
- "integrity": "sha512-lF3puPwkQWGfkMClXb4k/eUT/nZKQfxinRWJrdZaJO85Dqwo/G0yOC434Jr2ojwafWJMYqFGFa5ms4jJUgujdA==",
+ "node_modules/@napi-rs/wasm-runtime": {
+ "version": "0.2.4",
+ "resolved": "https://registry.npmjs.org/@napi-rs/wasm-runtime/-/wasm-runtime-0.2.4.tgz",
+ "integrity": "sha512-9zESzOO5aDByvhIAsOy9TbpZ0Ur2AJbUI7UT73kcUTS2mxAMHOBaa1st/jAymNoCtvrit99kkzT1FZuXVcgfIQ==",
+ "dev": true,
+ "license": "MIT",
"dependencies": {
- "@octokit/types": "^6.0.3",
- "is-plain-object": "^5.0.0",
- "universal-user-agent": "^6.0.0"
+ "@emnapi/core": "^1.1.0",
+ "@emnapi/runtime": "^1.1.0",
+ "@tybys/wasm-util": "^0.9.0"
}
},
- "node_modules/@octokit/core/node_modules/@octokit/request": {
- "version": "5.6.3",
- "resolved": "https://registry.npmjs.org/@octokit/request/-/request-5.6.3.tgz",
- "integrity": "sha512-bFJl0I1KVc9jYTe9tdGGpAMPy32dLBXXo1dS/YwSCTL/2nd9XeHsY616RE3HPXDVk+a+dBuzyz5YdlXwcDTr2A==",
+ "node_modules/@nicolo-ribaudo/eslint-scope-5-internals": {
+ "version": "5.1.1-v1",
+ "resolved": "https://registry.npmjs.org/@nicolo-ribaudo/eslint-scope-5-internals/-/eslint-scope-5-internals-5.1.1-v1.tgz",
+ "integrity": "sha512-54/JRvkLIzzDWshCWfuhadfrfZVPiElY8Fcgmg1HroEly/EDSszzhBAsarCux+D/kOslTRquNzuyGSmUSTTHGg==",
+ "license": "MIT",
"dependencies": {
- "@octokit/endpoint": "^6.0.1",
- "@octokit/request-error": "^2.1.0",
- "@octokit/types": "^6.16.1",
- "is-plain-object": "^5.0.0",
- "node-fetch": "^2.6.7",
- "universal-user-agent": "^6.0.0"
+ "eslint-scope": "5.1.1"
}
},
- "node_modules/@octokit/core/node_modules/before-after-hook": {
- "version": "2.2.3",
- "resolved": "https://registry.npmjs.org/before-after-hook/-/before-after-hook-2.2.3.tgz",
- "integrity": "sha512-NzUnlZexiaH/46WDhANlyR2bXRopNg4F/zuSA3OpZnllCUgRaOF2znDioDWrmbNVsuZk6l9pMquQB38cfBZwkQ=="
- },
- "node_modules/@octokit/core/node_modules/universal-user-agent": {
- "version": "6.0.1",
- "resolved": "https://registry.npmjs.org/universal-user-agent/-/universal-user-agent-6.0.1.tgz",
- "integrity": "sha512-yCzhz6FN2wU1NiiQRogkTQszlQSlpWaw8SvVegAc+bDxbzHgh1vX8uIe8OYyMH6DwH+sdTJsgMl36+mSMdRJIQ=="
- },
- "node_modules/@octokit/endpoint": {
- "version": "5.5.3",
- "resolved": "https://registry.npmjs.org/@octokit/endpoint/-/endpoint-5.5.3.tgz",
- "integrity": "sha512-EzKwkwcxeegYYah5ukEeAI/gYRLv2Y9U5PpIsseGSFDk+G3RbipQGBs8GuYS1TLCtQaqoO66+aQGtITPalxsNQ==",
- "dev": true,
+ "node_modules/@nicolo-ribaudo/eslint-scope-5-internals/node_modules/eslint-scope": {
+ "version": "5.1.1",
+ "resolved": "https://registry.npmjs.org/eslint-scope/-/eslint-scope-5.1.1.tgz",
+ "integrity": "sha512-2NxwbF/hZ0KpepYN0cNbo+FN6XoK7GaHlQhgx/hIZl6Va0bF45RQOOwhLIy8lQDbuCiadSLCBnH2CFYquit5bw==",
+ "license": "BSD-2-Clause",
"dependencies": {
- "@octokit/types": "^2.0.0",
- "is-plain-object": "^3.0.0",
- "universal-user-agent": "^5.0.0"
+ "esrecurse": "^4.3.0",
+ "estraverse": "^4.1.1"
+ },
+ "engines": {
+ "node": ">=8.0.0"
}
},
- "node_modules/@octokit/endpoint/node_modules/@octokit/types": {
- "version": "2.16.2",
- "resolved": "https://registry.npmjs.org/@octokit/types/-/types-2.16.2.tgz",
- "integrity": "sha512-O75k56TYvJ8WpAakWwYRN8Bgu60KrmX0z1KqFp1kNiFNkgW+JW+9EBKZ+S33PU6SLvbihqd+3drvPxKK68Ee8Q==",
- "dev": true,
+ "node_modules/@nodelib/fs.scandir": {
+ "version": "2.1.2",
+ "resolved": "https://registry.npmjs.org/@nodelib/fs.scandir/-/fs.scandir-2.1.2.tgz",
+ "integrity": "sha512-wrIBsjA5pl13f0RN4Zx4FNWmU71lv03meGKnqRUoCyan17s4V3WL92f3w3AIuWbNnpcrQyFBU5qMavJoB8d27w==",
"dependencies": {
- "@types/node": ">= 8"
+ "@nodelib/fs.stat": "2.0.2",
+ "run-parallel": "^1.1.9"
+ },
+ "engines": {
+ "node": ">= 8"
}
},
- "node_modules/@octokit/endpoint/node_modules/is-plain-object": {
- "version": "3.0.1",
- "resolved": "https://registry.npmjs.org/is-plain-object/-/is-plain-object-3.0.1.tgz",
- "integrity": "sha512-Xnpx182SBMrr/aBik8y+GuR4U1L9FqMSojwDQwPMmxyC6bvEqly9UBCxhauBF5vNh2gwWJNX6oDV7O+OM4z34g==",
- "dev": true,
+ "node_modules/@nodelib/fs.stat": {
+ "version": "2.0.2",
+ "resolved": "https://registry.npmjs.org/@nodelib/fs.stat/-/fs.stat-2.0.2.tgz",
+ "integrity": "sha512-z8+wGWV2dgUhLqrtRYa03yDx4HWMvXKi1z8g3m2JyxAx8F7xk74asqPk5LAETjqDSGLFML/6CDl0+yFunSYicw==",
"engines": {
- "node": ">=0.10.0"
+ "node": ">= 8"
}
},
- "node_modules/@octokit/endpoint/node_modules/universal-user-agent": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/universal-user-agent/-/universal-user-agent-5.0.0.tgz",
- "integrity": "sha512-B5TPtzZleXyPrUMKCpEHFmVhMN6EhmJYjG5PQna9s7mXeSqGTLap4OpqLl5FCEFUI3UBmllkETwKf/db66Y54Q==",
- "dev": true,
+ "node_modules/@nodelib/fs.walk": {
+ "version": "1.2.3",
+ "resolved": "https://registry.npmjs.org/@nodelib/fs.walk/-/fs.walk-1.2.3.tgz",
+ "integrity": "sha512-l6t8xEhfK9Sa4YO5mIRdau7XSOADfmh3jCr0evNHdY+HNkW6xuQhgMH7D73VV6WpZOagrW0UludvMTiifiwTfA==",
"dependencies": {
- "os-name": "^3.1.0"
+ "@nodelib/fs.scandir": "2.1.2",
+ "fastq": "^1.6.0"
+ },
+ "engines": {
+ "node": ">= 8"
}
},
- "node_modules/@octokit/graphql": {
- "version": "4.8.0",
- "resolved": "https://registry.npmjs.org/@octokit/graphql/-/graphql-4.8.0.tgz",
- "integrity": "sha512-0gv+qLSBLKF0z8TKaSKTsS39scVKF9dbMxJpj3U0vC7wjNWFuIpL/z76Qe2fiuCbDRcJSavkXsVtMS6/dtQQsg==",
+ "node_modules/@npmcli/agent": {
+ "version": "2.2.2",
+ "resolved": "https://registry.npmjs.org/@npmcli/agent/-/agent-2.2.2.tgz",
+ "integrity": "sha512-OrcNPXdpSl9UX7qPVRWbmWMCSXrcDa2M9DvrbOTj7ao1S4PlqVFYv9/yLKMkrJKZ/V5A/kDBC690or307i26Og==",
+ "dev": true,
+ "license": "ISC",
"dependencies": {
- "@octokit/request": "^5.6.0",
- "@octokit/types": "^6.0.3",
- "universal-user-agent": "^6.0.0"
+ "agent-base": "^7.1.0",
+ "http-proxy-agent": "^7.0.0",
+ "https-proxy-agent": "^7.0.1",
+ "lru-cache": "^10.0.1",
+ "socks-proxy-agent": "^8.0.3"
+ },
+ "engines": {
+ "node": "^16.14.0 || >=18.0.0"
}
},
- "node_modules/@octokit/graphql/node_modules/@octokit/endpoint": {
- "version": "6.0.12",
- "resolved": "https://registry.npmjs.org/@octokit/endpoint/-/endpoint-6.0.12.tgz",
- "integrity": "sha512-lF3puPwkQWGfkMClXb4k/eUT/nZKQfxinRWJrdZaJO85Dqwo/G0yOC434Jr2ojwafWJMYqFGFa5ms4jJUgujdA==",
+ "node_modules/@npmcli/arborist": {
+ "version": "7.5.4",
+ "resolved": "https://registry.npmjs.org/@npmcli/arborist/-/arborist-7.5.4.tgz",
+ "integrity": "sha512-nWtIc6QwwoUORCRNzKx4ypHqCk3drI+5aeYdMTQQiRCcn4lOOgfQh7WyZobGYTxXPSq1VwV53lkpN/BRlRk08g==",
+ "dev": true,
+ "license": "ISC",
"dependencies": {
- "@octokit/types": "^6.0.3",
- "is-plain-object": "^5.0.0",
- "universal-user-agent": "^6.0.0"
+ "@isaacs/string-locale-compare": "^1.1.0",
+ "@npmcli/fs": "^3.1.1",
+ "@npmcli/installed-package-contents": "^2.1.0",
+ "@npmcli/map-workspaces": "^3.0.2",
+ "@npmcli/metavuln-calculator": "^7.1.1",
+ "@npmcli/name-from-folder": "^2.0.0",
+ "@npmcli/node-gyp": "^3.0.0",
+ "@npmcli/package-json": "^5.1.0",
+ "@npmcli/query": "^3.1.0",
+ "@npmcli/redact": "^2.0.0",
+ "@npmcli/run-script": "^8.1.0",
+ "bin-links": "^4.0.4",
+ "cacache": "^18.0.3",
+ "common-ancestor-path": "^1.0.1",
+ "hosted-git-info": "^7.0.2",
+ "json-parse-even-better-errors": "^3.0.2",
+ "json-stringify-nice": "^1.1.4",
+ "lru-cache": "^10.2.2",
+ "minimatch": "^9.0.4",
+ "nopt": "^7.2.1",
+ "npm-install-checks": "^6.2.0",
+ "npm-package-arg": "^11.0.2",
+ "npm-pick-manifest": "^9.0.1",
+ "npm-registry-fetch": "^17.0.1",
+ "pacote": "^18.0.6",
+ "parse-conflict-json": "^3.0.0",
+ "proc-log": "^4.2.0",
+ "proggy": "^2.0.0",
+ "promise-all-reject-late": "^1.0.0",
+ "promise-call-limit": "^3.0.1",
+ "read-package-json-fast": "^3.0.2",
+ "semver": "^7.3.7",
+ "ssri": "^10.0.6",
+ "treeverse": "^3.0.0",
+ "walk-up-path": "^3.0.1"
+ },
+ "bin": {
+ "arborist": "bin/index.js"
+ },
+ "engines": {
+ "node": "^16.14.0 || >=18.0.0"
}
},
- "node_modules/@octokit/graphql/node_modules/@octokit/request": {
- "version": "5.6.3",
- "resolved": "https://registry.npmjs.org/@octokit/request/-/request-5.6.3.tgz",
- "integrity": "sha512-bFJl0I1KVc9jYTe9tdGGpAMPy32dLBXXo1dS/YwSCTL/2nd9XeHsY616RE3HPXDVk+a+dBuzyz5YdlXwcDTr2A==",
+ "node_modules/@npmcli/arborist/node_modules/brace-expansion": {
+ "version": "2.0.1",
+ "resolved": "https://registry.npmjs.org/brace-expansion/-/brace-expansion-2.0.1.tgz",
+ "integrity": "sha512-XnAIvQ8eM+kC6aULx6wuQiwVsnzsi9d3WxzV3FpWTGA19F621kwdbsAcFKXgKUHZWsy+mY6iL1sHTxWEFCytDA==",
+ "dev": true,
+ "license": "MIT",
"dependencies": {
- "@octokit/endpoint": "^6.0.1",
- "@octokit/request-error": "^2.1.0",
- "@octokit/types": "^6.16.1",
- "is-plain-object": "^5.0.0",
- "node-fetch": "^2.6.7",
- "universal-user-agent": "^6.0.0"
+ "balanced-match": "^1.0.0"
}
},
- "node_modules/@octokit/graphql/node_modules/universal-user-agent": {
- "version": "6.0.1",
- "resolved": "https://registry.npmjs.org/universal-user-agent/-/universal-user-agent-6.0.1.tgz",
- "integrity": "sha512-yCzhz6FN2wU1NiiQRogkTQszlQSlpWaw8SvVegAc+bDxbzHgh1vX8uIe8OYyMH6DwH+sdTJsgMl36+mSMdRJIQ=="
- },
- "node_modules/@octokit/openapi-types": {
- "version": "11.2.0",
- "resolved": "https://registry.npmjs.org/@octokit/openapi-types/-/openapi-types-11.2.0.tgz",
- "integrity": "sha512-PBsVO+15KSlGmiI8QAzaqvsNlZlrDlyAJYcrXBCvVUxCp7VnXjkwPoFHgjEJXx3WF9BAwkA6nfCUA7i9sODzKA=="
- },
- "node_modules/@octokit/plugin-enterprise-rest": {
- "version": "6.0.1",
- "resolved": "https://registry.npmjs.org/@octokit/plugin-enterprise-rest/-/plugin-enterprise-rest-6.0.1.tgz",
- "integrity": "sha512-93uGjlhUD+iNg1iWhUENAtJata6w5nE+V4urXOAlIXdco6xNZtUSfYY8dzp3Udy74aqO/B5UZL80x/YMa5PKRw==",
- "dev": true
- },
- "node_modules/@octokit/plugin-paginate-rest": {
- "version": "2.21.3",
- "resolved": "https://registry.npmjs.org/@octokit/plugin-paginate-rest/-/plugin-paginate-rest-2.21.3.tgz",
- "integrity": "sha512-aCZTEf0y2h3OLbrgKkrfFdjRL6eSOo8komneVQJnYecAxIej7Bafor2xhuDJOIFau4pk0i/P28/XgtbyPF0ZHw==",
+ "node_modules/@npmcli/arborist/node_modules/cacache": {
+ "version": "18.0.4",
+ "resolved": "https://registry.npmjs.org/cacache/-/cacache-18.0.4.tgz",
+ "integrity": "sha512-B+L5iIa9mgcjLbliir2th36yEwPftrzteHYujzsx3dFP/31GCHcIeS8f5MGd80odLOjaOvSpU3EEAmRQptkxLQ==",
+ "dev": true,
+ "license": "ISC",
"dependencies": {
- "@octokit/types": "^6.40.0"
+ "@npmcli/fs": "^3.1.0",
+ "fs-minipass": "^3.0.0",
+ "glob": "^10.2.2",
+ "lru-cache": "^10.0.1",
+ "minipass": "^7.0.3",
+ "minipass-collect": "^2.0.1",
+ "minipass-flush": "^1.0.5",
+ "minipass-pipeline": "^1.2.4",
+ "p-map": "^4.0.0",
+ "ssri": "^10.0.0",
+ "tar": "^6.1.11",
+ "unique-filename": "^3.0.0"
},
- "peerDependencies": {
- "@octokit/core": ">=2"
+ "engines": {
+ "node": "^16.14.0 || >=18.0.0"
}
},
- "node_modules/@octokit/plugin-paginate-rest/node_modules/@octokit/openapi-types": {
- "version": "12.11.0",
- "resolved": "https://registry.npmjs.org/@octokit/openapi-types/-/openapi-types-12.11.0.tgz",
- "integrity": "sha512-VsXyi8peyRq9PqIz/tpqiL2w3w80OgVMwBHltTml3LmVvXiphgeqmY9mvBw9Wu7e0QWk/fqD37ux8yP5uVekyQ=="
- },
- "node_modules/@octokit/plugin-paginate-rest/node_modules/@octokit/types": {
- "version": "6.41.0",
- "resolved": "https://registry.npmjs.org/@octokit/types/-/types-6.41.0.tgz",
- "integrity": "sha512-eJ2jbzjdijiL3B4PrSQaSjuF2sPEQPVCPzBvTHJD9Nz+9dw2SGH4K4xeQJ77YfTq5bRQ+bD8wT11JbeDPmxmGg==",
+ "node_modules/@npmcli/arborist/node_modules/fs-minipass": {
+ "version": "3.0.3",
+ "resolved": "https://registry.npmjs.org/fs-minipass/-/fs-minipass-3.0.3.tgz",
+ "integrity": "sha512-XUBA9XClHbnJWSfBzjkm6RvPsyg3sryZt06BEQoXcF7EK/xpGaQYJgQKDJSUH5SGZ76Y7pFx1QBnXz09rU5Fbw==",
+ "dev": true,
+ "license": "ISC",
"dependencies": {
- "@octokit/openapi-types": "^12.11.0"
+ "minipass": "^7.0.3"
+ },
+ "engines": {
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/@octokit/plugin-request-log": {
- "version": "1.0.4",
- "resolved": "https://registry.npmjs.org/@octokit/plugin-request-log/-/plugin-request-log-1.0.4.tgz",
- "integrity": "sha512-mLUsMkgP7K/cnFEw07kWqXGF5LKrOkD+lhCrKvPHXWDywAwuDUeDwWBpc69XK3pNX0uKiVt8g5z96PJ6z9xCFA==",
+ "node_modules/@npmcli/arborist/node_modules/glob": {
+ "version": "10.4.5",
+ "resolved": "https://registry.npmjs.org/glob/-/glob-10.4.5.tgz",
+ "integrity": "sha512-7Bv8RF0k6xjo7d4A/PxYLbUCfb6c+Vpd2/mB2yRDlew7Jb5hEXiCD9ibfO7wpk8i4sevK6DFny9h7EYbM3/sHg==",
"dev": true,
- "peerDependencies": {
- "@octokit/core": ">=3"
- }
- },
- "node_modules/@octokit/plugin-rest-endpoint-methods": {
- "version": "5.16.2",
- "resolved": "https://registry.npmjs.org/@octokit/plugin-rest-endpoint-methods/-/plugin-rest-endpoint-methods-5.16.2.tgz",
- "integrity": "sha512-8QFz29Fg5jDuTPXVtey05BLm7OB+M8fnvE64RNegzX7U+5NUXcOcnpTIK0YfSHBg8gYd0oxIq3IZTe9SfPZiRw==",
+ "license": "ISC",
"dependencies": {
- "@octokit/types": "^6.39.0",
- "deprecation": "^2.3.1"
+ "foreground-child": "^3.1.0",
+ "jackspeak": "^3.1.2",
+ "minimatch": "^9.0.4",
+ "minipass": "^7.1.2",
+ "package-json-from-dist": "^1.0.0",
+ "path-scurry": "^1.11.1"
},
- "peerDependencies": {
- "@octokit/core": ">=3"
+ "bin": {
+ "glob": "dist/esm/bin.mjs"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
}
},
- "node_modules/@octokit/plugin-rest-endpoint-methods/node_modules/@octokit/openapi-types": {
- "version": "12.11.0",
- "resolved": "https://registry.npmjs.org/@octokit/openapi-types/-/openapi-types-12.11.0.tgz",
- "integrity": "sha512-VsXyi8peyRq9PqIz/tpqiL2w3w80OgVMwBHltTml3LmVvXiphgeqmY9mvBw9Wu7e0QWk/fqD37ux8yP5uVekyQ=="
- },
- "node_modules/@octokit/plugin-rest-endpoint-methods/node_modules/@octokit/types": {
- "version": "6.41.0",
- "resolved": "https://registry.npmjs.org/@octokit/types/-/types-6.41.0.tgz",
- "integrity": "sha512-eJ2jbzjdijiL3B4PrSQaSjuF2sPEQPVCPzBvTHJD9Nz+9dw2SGH4K4xeQJ77YfTq5bRQ+bD8wT11JbeDPmxmGg==",
+ "node_modules/@npmcli/arborist/node_modules/hosted-git-info": {
+ "version": "7.0.2",
+ "resolved": "https://registry.npmjs.org/hosted-git-info/-/hosted-git-info-7.0.2.tgz",
+ "integrity": "sha512-puUZAUKT5m8Zzvs72XWy3HtvVbTWljRE66cP60bxJzAqf2DgICo7lYTY2IHUmLnNpjYvw5bvmoHvPc0QO2a62w==",
+ "dev": true,
+ "license": "ISC",
"dependencies": {
- "@octokit/openapi-types": "^12.11.0"
+ "lru-cache": "^10.0.1"
+ },
+ "engines": {
+ "node": "^16.14.0 || >=18.0.0"
}
},
- "node_modules/@octokit/request": {
- "version": "4.1.1",
- "resolved": "https://registry.npmjs.org/@octokit/request/-/request-4.1.1.tgz",
- "integrity": "sha512-LOyL0i3oxRo418EXRSJNk/3Q4I0/NKawTn6H/CQp+wnrG1UFLGu080gSsgnWobhPo5BpUNgSQ5BRk5FOOJhD1Q==",
+ "node_modules/@npmcli/arborist/node_modules/json-parse-even-better-errors": {
+ "version": "3.0.2",
+ "resolved": "https://registry.npmjs.org/json-parse-even-better-errors/-/json-parse-even-better-errors-3.0.2.tgz",
+ "integrity": "sha512-fi0NG4bPjCHunUJffmLd0gxssIgkNmArMvis4iNah6Owg1MCJjWhEcDLmsK6iGkJq3tHwbDkTlce70/tmXN4cQ==",
"dev": true,
- "dependencies": {
- "@octokit/endpoint": "^5.1.0",
- "@octokit/request-error": "^1.0.1",
- "deprecation": "^2.0.0",
- "is-plain-object": "^3.0.0",
- "node-fetch": "^2.3.0",
- "once": "^1.4.0",
- "universal-user-agent": "^2.1.0"
+ "license": "MIT",
+ "engines": {
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/@octokit/request-error": {
- "version": "2.1.0",
- "resolved": "https://registry.npmjs.org/@octokit/request-error/-/request-error-2.1.0.tgz",
- "integrity": "sha512-1VIvgXxs9WHSjicsRwq8PlR2LR2x6DwsJAaFgzdi0JfJoGSO8mYI/cHJQ+9FbN21aa+DrgNLnwObmyeSC8Rmpg==",
+ "node_modules/@npmcli/arborist/node_modules/minimatch": {
+ "version": "9.0.5",
+ "resolved": "https://registry.npmjs.org/minimatch/-/minimatch-9.0.5.tgz",
+ "integrity": "sha512-G6T0ZX48xgozx7587koeX9Ys2NYy6Gmv//P89sEte9V9whIapMNF4idKxnW2QtCcLiTWlb/wfCabAtAFWhhBow==",
+ "dev": true,
+ "license": "ISC",
"dependencies": {
- "@octokit/types": "^6.0.3",
- "deprecation": "^2.0.0",
- "once": "^1.4.0"
+ "brace-expansion": "^2.0.1"
+ },
+ "engines": {
+ "node": ">=16 || 14 >=14.17"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
}
},
- "node_modules/@octokit/request/node_modules/@octokit/request-error": {
- "version": "1.2.1",
- "resolved": "https://registry.npmjs.org/@octokit/request-error/-/request-error-1.2.1.tgz",
- "integrity": "sha512-+6yDyk1EES6WK+l3viRDElw96MvwfJxCt45GvmjDUKWjYIb3PJZQkq3i46TwGwoPD4h8NmTrENmtyA1FwbmhRA==",
+ "node_modules/@npmcli/arborist/node_modules/minipass": {
+ "version": "7.1.2",
+ "resolved": "https://registry.npmjs.org/minipass/-/minipass-7.1.2.tgz",
+ "integrity": "sha512-qOOzS1cBTWYF4BH8fVePDBOO9iptMnGUEZwNc/cMWnTV2nVLZ7VoNWEPHkYczZA0pdoA7dl6e7FL659nX9S2aw==",
"dev": true,
- "dependencies": {
- "@octokit/types": "^2.0.0",
- "deprecation": "^2.0.0",
- "once": "^1.4.0"
+ "license": "ISC",
+ "engines": {
+ "node": ">=16 || 14 >=14.17"
}
},
- "node_modules/@octokit/request/node_modules/@octokit/types": {
- "version": "2.16.2",
- "resolved": "https://registry.npmjs.org/@octokit/types/-/types-2.16.2.tgz",
- "integrity": "sha512-O75k56TYvJ8WpAakWwYRN8Bgu60KrmX0z1KqFp1kNiFNkgW+JW+9EBKZ+S33PU6SLvbihqd+3drvPxKK68Ee8Q==",
+ "node_modules/@npmcli/arborist/node_modules/npm-package-arg": {
+ "version": "11.0.3",
+ "resolved": "https://registry.npmjs.org/npm-package-arg/-/npm-package-arg-11.0.3.tgz",
+ "integrity": "sha512-sHGJy8sOC1YraBywpzQlIKBE4pBbGbiF95U6Auspzyem956E0+FtDtsx1ZxlOJkQCZ1AFXAY/yuvtFYrOxF+Bw==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "@types/node": ">= 8"
+ "hosted-git-info": "^7.0.0",
+ "proc-log": "^4.0.0",
+ "semver": "^7.3.5",
+ "validate-npm-package-name": "^5.0.0"
+ },
+ "engines": {
+ "node": "^16.14.0 || >=18.0.0"
}
},
- "node_modules/@octokit/request/node_modules/is-plain-object": {
- "version": "3.0.1",
- "resolved": "https://registry.npmjs.org/is-plain-object/-/is-plain-object-3.0.1.tgz",
- "integrity": "sha512-Xnpx182SBMrr/aBik8y+GuR4U1L9FqMSojwDQwPMmxyC6bvEqly9UBCxhauBF5vNh2gwWJNX6oDV7O+OM4z34g==",
+ "node_modules/@npmcli/arborist/node_modules/ssri": {
+ "version": "10.0.6",
+ "resolved": "https://registry.npmjs.org/ssri/-/ssri-10.0.6.tgz",
+ "integrity": "sha512-MGrFH9Z4NP9Iyhqn16sDtBpRRNJ0Y2hNa6D65h736fVSaPCHr4DM4sWUNvVaSuC+0OBGhwsrydQwmgfg5LncqQ==",
"dev": true,
+ "license": "ISC",
+ "dependencies": {
+ "minipass": "^7.0.3"
+ },
"engines": {
- "node": ">=0.10.0"
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/@octokit/rest": {
- "version": "16.26.0",
- "resolved": "https://registry.npmjs.org/@octokit/rest/-/rest-16.26.0.tgz",
- "integrity": "sha512-NBpzre44ZAQWZhlH+zUYTgqI0pHN+c9rNj4d+pCydGEiKTGc1HKmoTghEUyr9GxazDyoAvmpx9nL0I7QS1Olvg==",
+ "node_modules/@npmcli/arborist/node_modules/unique-filename": {
+ "version": "3.0.0",
+ "resolved": "https://registry.npmjs.org/unique-filename/-/unique-filename-3.0.0.tgz",
+ "integrity": "sha512-afXhuC55wkAmZ0P18QsVE6kp8JaxrEokN2HGIoIVv2ijHQd419H0+6EigAFcIzXeMIkcIkNBpB3L/DXB3cTS/g==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "@octokit/request": "^4.0.1",
- "@octokit/request-error": "^1.0.2",
- "atob-lite": "^2.0.0",
- "before-after-hook": "^1.4.0",
- "btoa-lite": "^1.0.0",
- "deprecation": "^2.0.0",
- "lodash.get": "^4.4.2",
- "lodash.set": "^4.3.2",
- "lodash.uniq": "^4.5.0",
- "octokit-pagination-methods": "^1.1.0",
- "once": "^1.4.0",
- "universal-user-agent": "^2.0.0",
- "url-template": "^2.0.8"
+ "unique-slug": "^4.0.0"
+ },
+ "engines": {
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/@octokit/rest/node_modules/@octokit/request-error": {
- "version": "1.2.1",
- "resolved": "https://registry.npmjs.org/@octokit/request-error/-/request-error-1.2.1.tgz",
- "integrity": "sha512-+6yDyk1EES6WK+l3viRDElw96MvwfJxCt45GvmjDUKWjYIb3PJZQkq3i46TwGwoPD4h8NmTrENmtyA1FwbmhRA==",
+ "node_modules/@npmcli/arborist/node_modules/unique-slug": {
+ "version": "4.0.0",
+ "resolved": "https://registry.npmjs.org/unique-slug/-/unique-slug-4.0.0.tgz",
+ "integrity": "sha512-WrcA6AyEfqDX5bWige/4NQfPZMtASNVxdmWR76WESYQVAACSgWcR6e9i0mofqqBxYFtL4oAxPIptY73/0YE1DQ==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "@octokit/types": "^2.0.0",
- "deprecation": "^2.0.0",
- "once": "^1.4.0"
+ "imurmurhash": "^0.1.4"
+ },
+ "engines": {
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/@octokit/rest/node_modules/@octokit/types": {
- "version": "2.16.2",
- "resolved": "https://registry.npmjs.org/@octokit/types/-/types-2.16.2.tgz",
- "integrity": "sha512-O75k56TYvJ8WpAakWwYRN8Bgu60KrmX0z1KqFp1kNiFNkgW+JW+9EBKZ+S33PU6SLvbihqd+3drvPxKK68Ee8Q==",
+ "node_modules/@npmcli/arborist/node_modules/validate-npm-package-name": {
+ "version": "5.0.1",
+ "resolved": "https://registry.npmjs.org/validate-npm-package-name/-/validate-npm-package-name-5.0.1.tgz",
+ "integrity": "sha512-OljLrQ9SQdOUqTaQxqL5dEfZWrXExyyWsozYlAWFawPVNuD83igl7uJD2RTkNMbniIYgt8l81eCJGIdQF7avLQ==",
"dev": true,
- "dependencies": {
- "@types/node": ">= 8"
+ "license": "ISC",
+ "engines": {
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/@octokit/tsconfig": {
- "version": "1.0.2",
- "resolved": "https://registry.npmjs.org/@octokit/tsconfig/-/tsconfig-1.0.2.tgz",
- "integrity": "sha512-I0vDR0rdtP8p2lGMzvsJzbhdOWy405HcGovrspJ8RRibHnyRgggUSNO5AIox5LmqiwmatHKYsvj6VGFHkqS7lA==",
- "dev": true
- },
- "node_modules/@octokit/types": {
- "version": "6.34.0",
- "resolved": "https://registry.npmjs.org/@octokit/types/-/types-6.34.0.tgz",
- "integrity": "sha512-s1zLBjWhdEI2zwaoSgyOFoKSl109CUcVBCc7biPJ3aAf6LGLU6szDvi31JPU7bxfla2lqfhjbbg/5DdFNxOwHw==",
+ "node_modules/@npmcli/fs": {
+ "version": "3.1.1",
+ "resolved": "https://registry.npmjs.org/@npmcli/fs/-/fs-3.1.1.tgz",
+ "integrity": "sha512-q9CRWjpHCMIh5sVyefoD1cA7PkvILqCZsnSOEUUivORLjxCO/Irmue2DprETiNgEqktDBZaM1Bi+jrarx1XdCg==",
+ "dev": true,
+ "license": "ISC",
"dependencies": {
- "@octokit/openapi-types": "^11.2.0"
+ "semver": "^7.3.5"
+ },
+ "engines": {
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/@octokit/webhooks-methods": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/@octokit/webhooks-methods/-/webhooks-methods-2.0.0.tgz",
- "integrity": "sha512-35cfQ4YWlnZnmZKmIxlGPUPLtbkF8lr/A/1Sk1eC0ddLMwQN06dOuLc+dI3YLQS+T+MoNt3DIQ0NynwgKPilig==",
- "license": "MIT"
- },
- "node_modules/@octokit/webhooks-types": {
- "version": "5.8.0",
- "resolved": "https://registry.npmjs.org/@octokit/webhooks-types/-/webhooks-types-5.8.0.tgz",
- "integrity": "sha512-8adktjIb76A7viIdayQSFuBEwOzwhDC+9yxZpKNHjfzrlostHCw0/N7JWpWMObfElwvJMk2fY2l1noENCk9wmw==",
- "license": "MIT"
- },
- "node_modules/@parcel/watcher": {
- "version": "2.0.4",
- "resolved": "https://registry.npmjs.org/@parcel/watcher/-/watcher-2.0.4.tgz",
- "integrity": "sha512-cTDi+FUDBIUOBKEtj+nhiJ71AZVlkAsQFuGQTun5tV9mwQBQgZvhCzG+URPQc8myeN32yRVZEfVAPCs1RW+Jvg==",
+ "node_modules/@npmcli/git": {
+ "version": "5.0.8",
+ "resolved": "https://registry.npmjs.org/@npmcli/git/-/git-5.0.8.tgz",
+ "integrity": "sha512-liASfw5cqhjNW9UFd+ruwwdEf/lbOAQjLL2XY2dFW/bkJheXDYZgOyul/4gVvEV4BWkTXjYGmDqMw9uegdbJNQ==",
"dev": true,
- "hasInstallScript": true,
+ "license": "ISC",
"dependencies": {
- "node-addon-api": "^3.2.1",
- "node-gyp-build": "^4.3.0"
+ "@npmcli/promise-spawn": "^7.0.0",
+ "ini": "^4.1.3",
+ "lru-cache": "^10.0.1",
+ "npm-pick-manifest": "^9.0.0",
+ "proc-log": "^4.0.0",
+ "promise-inflight": "^1.0.1",
+ "promise-retry": "^2.0.1",
+ "semver": "^7.3.5",
+ "which": "^4.0.0"
},
"engines": {
- "node": ">= 10.0.0"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/parcel"
+ "node": "^16.14.0 || >=18.0.0"
}
},
- "node_modules/@pkgjs/parseargs": {
- "version": "0.11.0",
- "resolved": "https://registry.npmjs.org/@pkgjs/parseargs/-/parseargs-0.11.0.tgz",
- "integrity": "sha512-+1VkjdD0QBLPodGrJUeqarH8VAIvQODIbwh9XpP5Syisf7YoQgsJKPNFoqqLQlu+VQ/tVSshMR6loPMn8U+dPg==",
+ "node_modules/@npmcli/git/node_modules/ini": {
+ "version": "4.1.3",
+ "resolved": "https://registry.npmjs.org/ini/-/ini-4.1.3.tgz",
+ "integrity": "sha512-X7rqawQBvfdjS10YU1y1YVreA3SsLrW9dX2CewP2EbBJM4ypVNLDkO5y04gejPwKIY9lR+7r9gn3rFPt/kmWFg==",
"dev": true,
- "optional": true,
+ "license": "ISC",
"engines": {
- "node": ">=14"
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/@pkgr/utils": {
- "version": "2.4.2",
- "resolved": "https://registry.npmjs.org/@pkgr/utils/-/utils-2.4.2.tgz",
- "integrity": "sha512-POgTXhjrTfbTV63DiFXav4lBHiICLKKwDeaKn9Nphwj7WH6m0hMMCaJkMyRWjgtPFyRKRVoMXXjczsTQRDEhYw==",
- "dependencies": {
- "cross-spawn": "^7.0.3",
- "fast-glob": "^3.3.0",
- "is-glob": "^4.0.3",
- "open": "^9.1.0",
- "picocolors": "^1.0.0",
- "tslib": "^2.6.0"
- },
+ "node_modules/@npmcli/git/node_modules/isexe": {
+ "version": "3.1.1",
+ "resolved": "https://registry.npmjs.org/isexe/-/isexe-3.1.1.tgz",
+ "integrity": "sha512-LpB/54B+/2J5hqQ7imZHfdU31OlgQqx7ZicVlkm9kzg9/w8GKLEcFfJl/t7DCEDueOyBAD6zCCwTO6Fzs0NoEQ==",
+ "dev": true,
+ "license": "ISC",
"engines": {
- "node": "^12.20.0 || ^14.18.0 || >=16.0.0"
- },
- "funding": {
- "url": "https://opencollective.com/unts"
+ "node": ">=16"
}
},
- "node_modules/@pkgr/utils/node_modules/cross-spawn": {
- "version": "7.0.3",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-7.0.3.tgz",
- "integrity": "sha512-iRDPJKUPVEND7dHPO8rkbOnPpyDygcDFtWjpeWNCgy8WP2rXcxXL8TskReQl6OrB2G7+UJrags1q15Fudc7G6w==",
+ "node_modules/@npmcli/git/node_modules/which": {
+ "version": "4.0.0",
+ "resolved": "https://registry.npmjs.org/which/-/which-4.0.0.tgz",
+ "integrity": "sha512-GlaYyEb07DPxYCKhKzplCWBJtvxZcZMrL+4UkrTSJHHPyZU4mYYTv3qaOe77H7EODLSSopAUFAc6W8U4yqvscg==",
+ "dev": true,
+ "license": "ISC",
"dependencies": {
- "path-key": "^3.1.0",
- "shebang-command": "^2.0.0",
- "which": "^2.0.1"
+ "isexe": "^3.1.1"
+ },
+ "bin": {
+ "node-which": "bin/which.js"
},
"engines": {
- "node": ">= 8"
+ "node": "^16.13.0 || >=18.0.0"
}
},
- "node_modules/@pkgr/utils/node_modules/define-lazy-prop": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/define-lazy-prop/-/define-lazy-prop-3.0.0.tgz",
- "integrity": "sha512-N+MeXYoqr3pOgn8xfyRPREN7gHakLYjhsHhWGT3fWAiL4IkAt0iDw14QiiEm2bE30c5XX5q0FtAA3CK5f9/BUg==",
- "engines": {
- "node": ">=12"
+ "node_modules/@npmcli/installed-package-contents": {
+ "version": "2.1.0",
+ "resolved": "https://registry.npmjs.org/@npmcli/installed-package-contents/-/installed-package-contents-2.1.0.tgz",
+ "integrity": "sha512-c8UuGLeZpm69BryRykLuKRyKFZYJsZSCT4aVY5ds4omyZqJ172ApzgfKJ5eV/r3HgLdUYgFVe54KSFVjKoe27w==",
+ "dev": true,
+ "license": "ISC",
+ "dependencies": {
+ "npm-bundled": "^3.0.0",
+ "npm-normalize-package-bin": "^3.0.0"
},
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "bin": {
+ "installed-package-contents": "bin/index.js"
+ },
+ "engines": {
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/@pkgr/utils/node_modules/fast-glob": {
- "version": "3.3.2",
- "resolved": "https://registry.npmjs.org/fast-glob/-/fast-glob-3.3.2.tgz",
- "integrity": "sha512-oX2ruAFQwf/Orj8m737Y5adxDQO0LAB7/S5MnxCdTNDd4p6BsyIVsv9JQsATbTSq8KHRpLwIHbVlUNatxd+1Ow==",
+ "node_modules/@npmcli/installed-package-contents/node_modules/npm-bundled": {
+ "version": "3.0.1",
+ "resolved": "https://registry.npmjs.org/npm-bundled/-/npm-bundled-3.0.1.tgz",
+ "integrity": "sha512-+AvaheE/ww1JEwRHOrn4WHNzOxGtVp+adrg2AeZS/7KuxGUYFuBta98wYpfHBbJp6Tg6j1NKSEVHNcfZzJHQwQ==",
+ "dev": true,
+ "license": "ISC",
"dependencies": {
- "@nodelib/fs.stat": "^2.0.2",
- "@nodelib/fs.walk": "^1.2.3",
- "glob-parent": "^5.1.2",
- "merge2": "^1.3.0",
- "micromatch": "^4.0.4"
+ "npm-normalize-package-bin": "^3.0.0"
},
"engines": {
- "node": ">=8.6.0"
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/@pkgr/utils/node_modules/glob-parent": {
- "version": "5.1.2",
- "resolved": "https://registry.npmjs.org/glob-parent/-/glob-parent-5.1.2.tgz",
- "integrity": "sha512-AOIgSQCepiJYwP3ARnGx+5VnTu2HBYdzbGP45eLw1vr3zB3vZLeyed1sC9hnbcOc9/SrMyM5RPQrkGz4aS9Zow==",
+ "node_modules/@npmcli/installed-package-contents/node_modules/npm-normalize-package-bin": {
+ "version": "3.0.1",
+ "resolved": "https://registry.npmjs.org/npm-normalize-package-bin/-/npm-normalize-package-bin-3.0.1.tgz",
+ "integrity": "sha512-dMxCf+zZ+3zeQZXKxmyuCKlIDPGuv8EF940xbkC4kQVDTtqoh6rJFO+JTKSA6/Rwi0getWmtuy4Itup0AMcaDQ==",
+ "dev": true,
+ "license": "ISC",
+ "engines": {
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ }
+ },
+ "node_modules/@npmcli/map-workspaces": {
+ "version": "3.0.6",
+ "resolved": "https://registry.npmjs.org/@npmcli/map-workspaces/-/map-workspaces-3.0.6.tgz",
+ "integrity": "sha512-tkYs0OYnzQm6iIRdfy+LcLBjcKuQCeE5YLb8KnrIlutJfheNaPvPpgoFEyEFgbjzl5PLZ3IA/BWAwRU0eHuQDA==",
+ "dev": true,
+ "license": "ISC",
"dependencies": {
- "is-glob": "^4.0.1"
+ "@npmcli/name-from-folder": "^2.0.0",
+ "glob": "^10.2.2",
+ "minimatch": "^9.0.0",
+ "read-package-json-fast": "^3.0.0"
},
"engines": {
- "node": ">= 6"
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/@pkgr/utils/node_modules/is-wsl": {
- "version": "2.2.0",
- "resolved": "https://registry.npmjs.org/is-wsl/-/is-wsl-2.2.0.tgz",
- "integrity": "sha512-fKzAra0rGJUUBwGBgNkHZuToZcn+TtXHpeCgmkMJMMYx1sQDYaCSyjJBSCa2nH1DGm7s3n1oBnohoVTBaN7Lww==",
+ "node_modules/@npmcli/map-workspaces/node_modules/brace-expansion": {
+ "version": "2.0.1",
+ "resolved": "https://registry.npmjs.org/brace-expansion/-/brace-expansion-2.0.1.tgz",
+ "integrity": "sha512-XnAIvQ8eM+kC6aULx6wuQiwVsnzsi9d3WxzV3FpWTGA19F621kwdbsAcFKXgKUHZWsy+mY6iL1sHTxWEFCytDA==",
+ "dev": true,
+ "license": "MIT",
"dependencies": {
- "is-docker": "^2.0.0"
+ "balanced-match": "^1.0.0"
+ }
+ },
+ "node_modules/@npmcli/map-workspaces/node_modules/glob": {
+ "version": "10.4.5",
+ "resolved": "https://registry.npmjs.org/glob/-/glob-10.4.5.tgz",
+ "integrity": "sha512-7Bv8RF0k6xjo7d4A/PxYLbUCfb6c+Vpd2/mB2yRDlew7Jb5hEXiCD9ibfO7wpk8i4sevK6DFny9h7EYbM3/sHg==",
+ "dev": true,
+ "license": "ISC",
+ "dependencies": {
+ "foreground-child": "^3.1.0",
+ "jackspeak": "^3.1.2",
+ "minimatch": "^9.0.4",
+ "minipass": "^7.1.2",
+ "package-json-from-dist": "^1.0.0",
+ "path-scurry": "^1.11.1"
},
- "engines": {
- "node": ">=8"
+ "bin": {
+ "glob": "dist/esm/bin.mjs"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
}
},
- "node_modules/@pkgr/utils/node_modules/open": {
- "version": "9.1.0",
- "resolved": "https://registry.npmjs.org/open/-/open-9.1.0.tgz",
- "integrity": "sha512-OS+QTnw1/4vrf+9hh1jc1jnYjzSG4ttTBB8UxOwAnInG3Uo4ssetzC1ihqaIHjLJnA5GGlRl6QlZXOTQhRBUvg==",
+ "node_modules/@npmcli/map-workspaces/node_modules/minimatch": {
+ "version": "9.0.5",
+ "resolved": "https://registry.npmjs.org/minimatch/-/minimatch-9.0.5.tgz",
+ "integrity": "sha512-G6T0ZX48xgozx7587koeX9Ys2NYy6Gmv//P89sEte9V9whIapMNF4idKxnW2QtCcLiTWlb/wfCabAtAFWhhBow==",
+ "dev": true,
+ "license": "ISC",
"dependencies": {
- "default-browser": "^4.0.0",
- "define-lazy-prop": "^3.0.0",
- "is-inside-container": "^1.0.0",
- "is-wsl": "^2.2.0"
+ "brace-expansion": "^2.0.1"
},
"engines": {
- "node": ">=14.16"
+ "node": ">=16 || 14 >=14.17"
},
"funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "url": "https://github.com/sponsors/isaacs"
}
},
- "node_modules/@pkgr/utils/node_modules/path-key": {
- "version": "3.1.1",
- "resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
- "integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
+ "node_modules/@npmcli/map-workspaces/node_modules/minipass": {
+ "version": "7.1.2",
+ "resolved": "https://registry.npmjs.org/minipass/-/minipass-7.1.2.tgz",
+ "integrity": "sha512-qOOzS1cBTWYF4BH8fVePDBOO9iptMnGUEZwNc/cMWnTV2nVLZ7VoNWEPHkYczZA0pdoA7dl6e7FL659nX9S2aw==",
+ "dev": true,
+ "license": "ISC",
"engines": {
- "node": ">=8"
+ "node": ">=16 || 14 >=14.17"
}
},
- "node_modules/@pkgr/utils/node_modules/shebang-command": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/shebang-command/-/shebang-command-2.0.0.tgz",
- "integrity": "sha512-kHxr2zZpYtdmrN1qDjrrX/Z1rR1kG8Dx+gkpK1G4eXmvXswmcE1hTWBWYUzlraYw1/yZp6YuDY77YtvbN0dmDA==",
+ "node_modules/@npmcli/metavuln-calculator": {
+ "version": "7.1.1",
+ "resolved": "https://registry.npmjs.org/@npmcli/metavuln-calculator/-/metavuln-calculator-7.1.1.tgz",
+ "integrity": "sha512-Nkxf96V0lAx3HCpVda7Vw4P23RILgdi/5K1fmj2tZkWIYLpXAN8k2UVVOsW16TsS5F8Ws2I7Cm+PU1/rsVF47g==",
+ "dev": true,
+ "license": "ISC",
"dependencies": {
- "shebang-regex": "^3.0.0"
+ "cacache": "^18.0.0",
+ "json-parse-even-better-errors": "^3.0.0",
+ "pacote": "^18.0.0",
+ "proc-log": "^4.1.0",
+ "semver": "^7.3.5"
},
"engines": {
- "node": ">=8"
+ "node": "^16.14.0 || >=18.0.0"
}
},
- "node_modules/@pkgr/utils/node_modules/shebang-regex": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/shebang-regex/-/shebang-regex-3.0.0.tgz",
- "integrity": "sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==",
- "engines": {
- "node": ">=8"
+ "node_modules/@npmcli/metavuln-calculator/node_modules/brace-expansion": {
+ "version": "2.0.1",
+ "resolved": "https://registry.npmjs.org/brace-expansion/-/brace-expansion-2.0.1.tgz",
+ "integrity": "sha512-XnAIvQ8eM+kC6aULx6wuQiwVsnzsi9d3WxzV3FpWTGA19F621kwdbsAcFKXgKUHZWsy+mY6iL1sHTxWEFCytDA==",
+ "dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "balanced-match": "^1.0.0"
}
},
- "node_modules/@pkgr/utils/node_modules/which": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
- "integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
+ "node_modules/@npmcli/metavuln-calculator/node_modules/cacache": {
+ "version": "18.0.4",
+ "resolved": "https://registry.npmjs.org/cacache/-/cacache-18.0.4.tgz",
+ "integrity": "sha512-B+L5iIa9mgcjLbliir2th36yEwPftrzteHYujzsx3dFP/31GCHcIeS8f5MGd80odLOjaOvSpU3EEAmRQptkxLQ==",
+ "dev": true,
+ "license": "ISC",
"dependencies": {
- "isexe": "^2.0.0"
- },
- "bin": {
- "node-which": "bin/node-which"
+ "@npmcli/fs": "^3.1.0",
+ "fs-minipass": "^3.0.0",
+ "glob": "^10.2.2",
+ "lru-cache": "^10.0.1",
+ "minipass": "^7.0.3",
+ "minipass-collect": "^2.0.1",
+ "minipass-flush": "^1.0.5",
+ "minipass-pipeline": "^1.2.4",
+ "p-map": "^4.0.0",
+ "ssri": "^10.0.0",
+ "tar": "^6.1.11",
+ "unique-filename": "^3.0.0"
},
"engines": {
- "node": ">= 8"
+ "node": "^16.14.0 || >=18.0.0"
}
},
- "node_modules/@playwright/test": {
- "version": "1.48.1",
- "resolved": "https://registry.npmjs.org/@playwright/test/-/test-1.48.1.tgz",
- "integrity": "sha512-s9RtWoxkOLmRJdw3oFvhFbs9OJS0BzrLUc8Hf6l2UdCNd1rqeEyD4BhCJkvzeEoD1FsK4mirsWwGerhVmYKtZg==",
+ "node_modules/@npmcli/metavuln-calculator/node_modules/fs-minipass": {
+ "version": "3.0.3",
+ "resolved": "https://registry.npmjs.org/fs-minipass/-/fs-minipass-3.0.3.tgz",
+ "integrity": "sha512-XUBA9XClHbnJWSfBzjkm6RvPsyg3sryZt06BEQoXcF7EK/xpGaQYJgQKDJSUH5SGZ76Y7pFx1QBnXz09rU5Fbw==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "playwright": "1.48.1"
- },
- "bin": {
- "playwright": "cli.js"
+ "minipass": "^7.0.3"
},
"engines": {
- "node": ">=18"
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/@pmmmwh/react-refresh-webpack-plugin": {
- "version": "0.5.11",
- "resolved": "https://registry.npmjs.org/@pmmmwh/react-refresh-webpack-plugin/-/react-refresh-webpack-plugin-0.5.11.tgz",
- "integrity": "sha512-7j/6vdTym0+qZ6u4XbSAxrWBGYSdCfTzySkj7WAFgDLmSyWlOrWvpyzxlFh5jtw9dn0oL/jtW+06XfFiisN3JQ==",
+ "node_modules/@npmcli/metavuln-calculator/node_modules/glob": {
+ "version": "10.4.5",
+ "resolved": "https://registry.npmjs.org/glob/-/glob-10.4.5.tgz",
+ "integrity": "sha512-7Bv8RF0k6xjo7d4A/PxYLbUCfb6c+Vpd2/mB2yRDlew7Jb5hEXiCD9ibfO7wpk8i4sevK6DFny9h7EYbM3/sHg==",
+ "dev": true,
+ "license": "ISC",
"dependencies": {
- "ansi-html-community": "^0.0.8",
- "common-path-prefix": "^3.0.0",
- "core-js-pure": "^3.23.3",
- "error-stack-parser": "^2.0.6",
- "find-up": "^5.0.0",
- "html-entities": "^2.1.0",
- "loader-utils": "^2.0.4",
- "schema-utils": "^3.0.0",
- "source-map": "^0.7.3"
- },
- "engines": {
- "node": ">= 10.13"
+ "foreground-child": "^3.1.0",
+ "jackspeak": "^3.1.2",
+ "minimatch": "^9.0.4",
+ "minipass": "^7.1.2",
+ "package-json-from-dist": "^1.0.0",
+ "path-scurry": "^1.11.1"
},
- "peerDependencies": {
- "@types/webpack": "4.x || 5.x",
- "react-refresh": ">=0.10.0 <1.0.0",
- "sockjs-client": "^1.4.0",
- "type-fest": ">=0.17.0 <5.0.0",
- "webpack": ">=4.43.0 <6.0.0",
- "webpack-dev-server": "3.x || 4.x",
- "webpack-hot-middleware": "2.x",
- "webpack-plugin-serve": "0.x || 1.x"
+ "bin": {
+ "glob": "dist/esm/bin.mjs"
},
- "peerDependenciesMeta": {
- "@types/webpack": {
- "optional": true
- },
- "sockjs-client": {
- "optional": true
- },
- "type-fest": {
- "optional": true
- },
- "webpack-dev-server": {
- "optional": true
- },
- "webpack-hot-middleware": {
- "optional": true
- },
- "webpack-plugin-serve": {
- "optional": true
- }
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
}
},
- "node_modules/@pmmmwh/react-refresh-webpack-plugin/node_modules/ajv": {
- "version": "6.12.6",
- "resolved": "https://registry.npmjs.org/ajv/-/ajv-6.12.6.tgz",
- "integrity": "sha512-j3fVLgvTo527anyYyJOGTYJbG+vnnQYvE0m5mmkc1TK+nxAppkCLMIL0aZ4dblVCNoGShhm+kzE4ZUykBoMg4g==",
- "dependencies": {
- "fast-deep-equal": "^3.1.1",
- "fast-json-stable-stringify": "^2.0.0",
- "json-schema-traverse": "^0.4.1",
- "uri-js": "^4.2.2"
- },
- "funding": {
- "type": "github",
- "url": "https://github.com/sponsors/epoberezkin"
+ "node_modules/@npmcli/metavuln-calculator/node_modules/json-parse-even-better-errors": {
+ "version": "3.0.2",
+ "resolved": "https://registry.npmjs.org/json-parse-even-better-errors/-/json-parse-even-better-errors-3.0.2.tgz",
+ "integrity": "sha512-fi0NG4bPjCHunUJffmLd0gxssIgkNmArMvis4iNah6Owg1MCJjWhEcDLmsK6iGkJq3tHwbDkTlce70/tmXN4cQ==",
+ "dev": true,
+ "license": "MIT",
+ "engines": {
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/@pmmmwh/react-refresh-webpack-plugin/node_modules/find-up": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/find-up/-/find-up-5.0.0.tgz",
- "integrity": "sha512-78/PXT1wlLLDgTzDs7sjq9hzz0vXD+zn+7wypEe4fXQxCmdmqfGsEPQxmiCSQI3ajFV91bVSsvNtrJRiW6nGng==",
+ "node_modules/@npmcli/metavuln-calculator/node_modules/minimatch": {
+ "version": "9.0.5",
+ "resolved": "https://registry.npmjs.org/minimatch/-/minimatch-9.0.5.tgz",
+ "integrity": "sha512-G6T0ZX48xgozx7587koeX9Ys2NYy6Gmv//P89sEte9V9whIapMNF4idKxnW2QtCcLiTWlb/wfCabAtAFWhhBow==",
+ "dev": true,
+ "license": "ISC",
"dependencies": {
- "locate-path": "^6.0.0",
- "path-exists": "^4.0.0"
+ "brace-expansion": "^2.0.1"
},
"engines": {
- "node": ">=10"
+ "node": ">=16 || 14 >=14.17"
},
"funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "url": "https://github.com/sponsors/isaacs"
}
},
- "node_modules/@pmmmwh/react-refresh-webpack-plugin/node_modules/locate-path": {
- "version": "6.0.0",
- "resolved": "https://registry.npmjs.org/locate-path/-/locate-path-6.0.0.tgz",
- "integrity": "sha512-iPZK6eYjbxRu3uB4/WZ3EsEIMJFMqAoopl3R+zuq0UjcAm/MO6KCweDgPfP3elTztoKP3KtnVHxTn2NHBSDVUw==",
- "dependencies": {
- "p-locate": "^5.0.0"
- },
+ "node_modules/@npmcli/metavuln-calculator/node_modules/minipass": {
+ "version": "7.1.2",
+ "resolved": "https://registry.npmjs.org/minipass/-/minipass-7.1.2.tgz",
+ "integrity": "sha512-qOOzS1cBTWYF4BH8fVePDBOO9iptMnGUEZwNc/cMWnTV2nVLZ7VoNWEPHkYczZA0pdoA7dl6e7FL659nX9S2aw==",
+ "dev": true,
+ "license": "ISC",
"engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "node": ">=16 || 14 >=14.17"
}
},
- "node_modules/@pmmmwh/react-refresh-webpack-plugin/node_modules/p-locate": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/p-locate/-/p-locate-5.0.0.tgz",
- "integrity": "sha512-LaNjtRWUBY++zB5nE/NwcaoMylSPk+S+ZHNB1TzdbMJMny6dynpAGt7X/tl/QYq3TIeE6nxHppbo2LGymrG5Pw==",
+ "node_modules/@npmcli/metavuln-calculator/node_modules/ssri": {
+ "version": "10.0.6",
+ "resolved": "https://registry.npmjs.org/ssri/-/ssri-10.0.6.tgz",
+ "integrity": "sha512-MGrFH9Z4NP9Iyhqn16sDtBpRRNJ0Y2hNa6D65h736fVSaPCHr4DM4sWUNvVaSuC+0OBGhwsrydQwmgfg5LncqQ==",
+ "dev": true,
+ "license": "ISC",
"dependencies": {
- "p-limit": "^3.0.2"
+ "minipass": "^7.0.3"
},
"engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/@pmmmwh/react-refresh-webpack-plugin/node_modules/path-exists": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/path-exists/-/path-exists-4.0.0.tgz",
- "integrity": "sha512-ak9Qy5Q7jYb2Wwcey5Fpvg2KoAc/ZIhLSLOSBmRmygPsGwkVVt0fZa0qrtMz+m6tJTAHfZQ8FnmB4MG4LWy7/w==",
+ "node_modules/@npmcli/metavuln-calculator/node_modules/unique-filename": {
+ "version": "3.0.0",
+ "resolved": "https://registry.npmjs.org/unique-filename/-/unique-filename-3.0.0.tgz",
+ "integrity": "sha512-afXhuC55wkAmZ0P18QsVE6kp8JaxrEokN2HGIoIVv2ijHQd419H0+6EigAFcIzXeMIkcIkNBpB3L/DXB3cTS/g==",
+ "dev": true,
+ "license": "ISC",
+ "dependencies": {
+ "unique-slug": "^4.0.0"
+ },
"engines": {
- "node": ">=8"
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/@pmmmwh/react-refresh-webpack-plugin/node_modules/schema-utils": {
- "version": "3.3.0",
- "resolved": "https://registry.npmjs.org/schema-utils/-/schema-utils-3.3.0.tgz",
- "integrity": "sha512-pN/yOAvcC+5rQ5nERGuwrjLlYvLTbCibnZ1I7B1LaiAz9BRBlE9GMgE/eqV30P7aJQUf7Ddimy/RsbYO/GrVGg==",
+ "node_modules/@npmcli/metavuln-calculator/node_modules/unique-slug": {
+ "version": "4.0.0",
+ "resolved": "https://registry.npmjs.org/unique-slug/-/unique-slug-4.0.0.tgz",
+ "integrity": "sha512-WrcA6AyEfqDX5bWige/4NQfPZMtASNVxdmWR76WESYQVAACSgWcR6e9i0mofqqBxYFtL4oAxPIptY73/0YE1DQ==",
+ "dev": true,
+ "license": "ISC",
"dependencies": {
- "@types/json-schema": "^7.0.8",
- "ajv": "^6.12.5",
- "ajv-keywords": "^3.5.2"
+ "imurmurhash": "^0.1.4"
},
"engines": {
- "node": ">= 10.13.0"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/webpack"
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/@pmmmwh/react-refresh-webpack-plugin/node_modules/source-map": {
- "version": "0.7.4",
- "resolved": "https://registry.npmjs.org/source-map/-/source-map-0.7.4.tgz",
- "integrity": "sha512-l3BikUxvPOcn5E74dZiq5BGsTb5yEwhaTSzccU6t4sDOH8NWJCstKO5QT2CvtFoK6F0saL7p9xHAqHOlCPJygA==",
+ "node_modules/@npmcli/name-from-folder": {
+ "version": "2.0.0",
+ "resolved": "https://registry.npmjs.org/@npmcli/name-from-folder/-/name-from-folder-2.0.0.tgz",
+ "integrity": "sha512-pwK+BfEBZJbKdNYpHHRTNBwBoqrN/iIMO0AiGvYsp3Hoaq0WbgGSWQR6SCldZovoDpY3yje5lkFUe6gsDgJ2vg==",
+ "dev": true,
+ "license": "ISC",
"engines": {
- "node": ">= 8"
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/@polka/url": {
- "version": "1.0.0-next.23",
- "resolved": "https://registry.npmjs.org/@polka/url/-/url-1.0.0-next.23.tgz",
- "integrity": "sha512-C16M+IYz0rgRhWZdCmK+h58JMv8vijAA61gmz2rspCSwKwzBebpdcsiUmwrtJRdphuY30i6BSLEOP8ppbNLyLg=="
+ "node_modules/@npmcli/node-gyp": {
+ "version": "3.0.0",
+ "resolved": "https://registry.npmjs.org/@npmcli/node-gyp/-/node-gyp-3.0.0.tgz",
+ "integrity": "sha512-gp8pRXC2oOxu0DUE1/M3bYtb1b3/DbJ5aM113+XJBgfXdussRAsX0YOrOhdd8WvnAR6auDBvJomGAkLKA5ydxA==",
+ "dev": true,
+ "license": "ISC",
+ "engines": {
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ }
},
- "node_modules/@preact/signals": {
- "version": "1.3.0",
- "resolved": "https://registry.npmjs.org/@preact/signals/-/signals-1.3.0.tgz",
- "integrity": "sha512-EOMeg42SlLS72dhoq6Vjq08havnLseWmPQ8A0YsgIAqMgWgx7V1a39+Pxo6i7SY5NwJtH4849JogFq3M67AzWg==",
- "license": "MIT",
+ "node_modules/@npmcli/package-json": {
+ "version": "5.2.0",
+ "resolved": "https://registry.npmjs.org/@npmcli/package-json/-/package-json-5.2.0.tgz",
+ "integrity": "sha512-qe/kiqqkW0AGtvBjL8TJKZk/eBBSpnJkUWvHdQ9jM2lKHXRYYJuyNpJPlJw3c8QjC2ow6NZYiLExhUaeJelbxQ==",
+ "dev": true,
+ "license": "ISC",
"dependencies": {
- "@preact/signals-core": "^1.7.0"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/preact"
+ "@npmcli/git": "^5.0.0",
+ "glob": "^10.2.2",
+ "hosted-git-info": "^7.0.0",
+ "json-parse-even-better-errors": "^3.0.0",
+ "normalize-package-data": "^6.0.0",
+ "proc-log": "^4.0.0",
+ "semver": "^7.5.3"
},
- "peerDependencies": {
- "preact": "10.x"
+ "engines": {
+ "node": "^16.14.0 || >=18.0.0"
}
},
- "node_modules/@preact/signals-core": {
- "version": "1.8.0",
- "resolved": "https://registry.npmjs.org/@preact/signals-core/-/signals-core-1.8.0.tgz",
- "integrity": "sha512-OBvUsRZqNmjzCZXWLxkZfhcgT+Fk8DDcT/8vD6a1xhDemodyy87UJRJfASMuSD8FaAIeGgGm85ydXhm7lr4fyA==",
+ "node_modules/@npmcli/package-json/node_modules/brace-expansion": {
+ "version": "2.0.1",
+ "resolved": "https://registry.npmjs.org/brace-expansion/-/brace-expansion-2.0.1.tgz",
+ "integrity": "sha512-XnAIvQ8eM+kC6aULx6wuQiwVsnzsi9d3WxzV3FpWTGA19F621kwdbsAcFKXgKUHZWsy+mY6iL1sHTxWEFCytDA==",
+ "dev": true,
"license": "MIT",
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/preact"
+ "dependencies": {
+ "balanced-match": "^1.0.0"
}
},
- "node_modules/@puppeteer/browsers": {
- "version": "1.4.6",
- "resolved": "https://registry.npmjs.org/@puppeteer/browsers/-/browsers-1.4.6.tgz",
- "integrity": "sha512-x4BEjr2SjOPowNeiguzjozQbsc6h437ovD/wu+JpaenxVLm3jkgzHY2xOslMTp50HoTvQreMjiexiGQw1sqZlQ==",
+ "node_modules/@npmcli/package-json/node_modules/glob": {
+ "version": "10.4.5",
+ "resolved": "https://registry.npmjs.org/glob/-/glob-10.4.5.tgz",
+ "integrity": "sha512-7Bv8RF0k6xjo7d4A/PxYLbUCfb6c+Vpd2/mB2yRDlew7Jb5hEXiCD9ibfO7wpk8i4sevK6DFny9h7EYbM3/sHg==",
+ "dev": true,
+ "license": "ISC",
"dependencies": {
- "debug": "4.3.4",
- "extract-zip": "2.0.1",
- "progress": "2.0.3",
- "proxy-agent": "6.3.0",
- "tar-fs": "3.0.4",
- "unbzip2-stream": "1.4.3",
- "yargs": "17.7.1"
+ "foreground-child": "^3.1.0",
+ "jackspeak": "^3.1.2",
+ "minimatch": "^9.0.4",
+ "minipass": "^7.1.2",
+ "package-json-from-dist": "^1.0.0",
+ "path-scurry": "^1.11.1"
},
"bin": {
- "browsers": "lib/cjs/main-cli.js"
- },
- "engines": {
- "node": ">=16.3.0"
- },
- "peerDependencies": {
- "typescript": ">= 4.7.4"
+ "glob": "dist/esm/bin.mjs"
},
- "peerDependenciesMeta": {
- "typescript": {
- "optional": true
- }
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
}
},
- "node_modules/@puppeteer/browsers/node_modules/cliui": {
- "version": "8.0.1",
- "resolved": "https://registry.npmjs.org/cliui/-/cliui-8.0.1.tgz",
- "integrity": "sha512-BSeNnyus75C4//NQ9gQt1/csTXyo/8Sb+afLAkzAptFuMsod9HFokGNudZpi/oQV73hnVK+sR+5PVRMd+Dr7YQ==",
+ "node_modules/@npmcli/package-json/node_modules/hosted-git-info": {
+ "version": "7.0.2",
+ "resolved": "https://registry.npmjs.org/hosted-git-info/-/hosted-git-info-7.0.2.tgz",
+ "integrity": "sha512-puUZAUKT5m8Zzvs72XWy3HtvVbTWljRE66cP60bxJzAqf2DgICo7lYTY2IHUmLnNpjYvw5bvmoHvPc0QO2a62w==",
+ "dev": true,
+ "license": "ISC",
"dependencies": {
- "string-width": "^4.2.0",
- "strip-ansi": "^6.0.1",
- "wrap-ansi": "^7.0.0"
+ "lru-cache": "^10.0.1"
},
"engines": {
- "node": ">=12"
+ "node": "^16.14.0 || >=18.0.0"
}
},
- "node_modules/@puppeteer/browsers/node_modules/emoji-regex": {
- "version": "8.0.0",
- "resolved": "https://registry.npmjs.org/emoji-regex/-/emoji-regex-8.0.0.tgz",
- "integrity": "sha512-MSjYzcWNOA0ewAHpz0MxpYFvwg6yjy1NG3xteoqz644VCo/RPgnr1/GGt+ic3iJTzQ8Eu3TdM14SawnVUmGE6A=="
+ "node_modules/@npmcli/package-json/node_modules/json-parse-even-better-errors": {
+ "version": "3.0.2",
+ "resolved": "https://registry.npmjs.org/json-parse-even-better-errors/-/json-parse-even-better-errors-3.0.2.tgz",
+ "integrity": "sha512-fi0NG4bPjCHunUJffmLd0gxssIgkNmArMvis4iNah6Owg1MCJjWhEcDLmsK6iGkJq3tHwbDkTlce70/tmXN4cQ==",
+ "dev": true,
+ "license": "MIT",
+ "engines": {
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ }
},
- "node_modules/@puppeteer/browsers/node_modules/extract-zip": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/extract-zip/-/extract-zip-2.0.1.tgz",
- "integrity": "sha512-GDhU9ntwuKyGXdZBUgTIe+vXnWj0fppUEtMDL0+idd5Sta8TGpHssn/eusA9mrPr9qNDym6SxAYZjNvCn/9RBg==",
+ "node_modules/@npmcli/package-json/node_modules/minimatch": {
+ "version": "9.0.5",
+ "resolved": "https://registry.npmjs.org/minimatch/-/minimatch-9.0.5.tgz",
+ "integrity": "sha512-G6T0ZX48xgozx7587koeX9Ys2NYy6Gmv//P89sEte9V9whIapMNF4idKxnW2QtCcLiTWlb/wfCabAtAFWhhBow==",
+ "dev": true,
+ "license": "ISC",
"dependencies": {
- "debug": "^4.1.1",
- "get-stream": "^5.1.0",
- "yauzl": "^2.10.0"
- },
- "bin": {
- "extract-zip": "cli.js"
+ "brace-expansion": "^2.0.1"
},
"engines": {
- "node": ">= 10.17.0"
+ "node": ">=16 || 14 >=14.17"
},
- "optionalDependencies": {
- "@types/yauzl": "^2.9.1"
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
}
},
- "node_modules/@puppeteer/browsers/node_modules/is-fullwidth-code-point": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/is-fullwidth-code-point/-/is-fullwidth-code-point-3.0.0.tgz",
- "integrity": "sha512-zymm5+u+sCsSWyD9qNaejV3DFvhCKclKdizYaJUuHA83RLjb7nSuGnddCHGv0hk+KY7BMAlsWeK4Ueg6EV6XQg==",
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@puppeteer/browsers/node_modules/pump": {
- "version": "3.0.2",
- "resolved": "https://registry.npmjs.org/pump/-/pump-3.0.2.tgz",
- "integrity": "sha512-tUPXtzlGM8FE3P0ZL6DVs/3P58k9nk8/jZeQCurTJylQA8qFYzHFfhBJkuqyE0FifOsQ0uKWekiZ5g8wtr28cw==",
- "license": "MIT",
- "dependencies": {
- "end-of-stream": "^1.1.0",
- "once": "^1.3.1"
+ "node_modules/@npmcli/package-json/node_modules/minipass": {
+ "version": "7.1.2",
+ "resolved": "https://registry.npmjs.org/minipass/-/minipass-7.1.2.tgz",
+ "integrity": "sha512-qOOzS1cBTWYF4BH8fVePDBOO9iptMnGUEZwNc/cMWnTV2nVLZ7VoNWEPHkYczZA0pdoA7dl6e7FL659nX9S2aw==",
+ "dev": true,
+ "license": "ISC",
+ "engines": {
+ "node": ">=16 || 14 >=14.17"
}
},
- "node_modules/@puppeteer/browsers/node_modules/string-width": {
- "version": "4.2.3",
- "resolved": "https://registry.npmjs.org/string-width/-/string-width-4.2.3.tgz",
- "integrity": "sha512-wKyQRQpjJ0sIp62ErSZdGsjMJWsap5oRNihHhu6G7JVO/9jIB6UyevL+tXuOqrng8j/cxKTWyWUwvSTriiZz/g==",
+ "node_modules/@npmcli/package-json/node_modules/normalize-package-data": {
+ "version": "6.0.2",
+ "resolved": "https://registry.npmjs.org/normalize-package-data/-/normalize-package-data-6.0.2.tgz",
+ "integrity": "sha512-V6gygoYb/5EmNI+MEGrWkC+e6+Rr7mTmfHrxDbLzxQogBkgzo76rkok0Am6thgSF7Mv2nLOajAJj5vDJZEFn7g==",
+ "dev": true,
+ "license": "BSD-2-Clause",
"dependencies": {
- "emoji-regex": "^8.0.0",
- "is-fullwidth-code-point": "^3.0.0",
- "strip-ansi": "^6.0.1"
+ "hosted-git-info": "^7.0.0",
+ "semver": "^7.3.5",
+ "validate-npm-package-license": "^3.0.4"
},
"engines": {
- "node": ">=8"
+ "node": "^16.14.0 || >=18.0.0"
}
},
- "node_modules/@puppeteer/browsers/node_modules/tar-fs": {
- "version": "3.0.4",
- "resolved": "https://registry.npmjs.org/tar-fs/-/tar-fs-3.0.4.tgz",
- "integrity": "sha512-5AFQU8b9qLfZCX9zp2duONhPmZv0hGYiBPJsyUdqMjzq/mqVpy/rEUSeHk1+YitmxugaptgBh5oDGU3VsAJq4w==",
+ "node_modules/@npmcli/promise-spawn": {
+ "version": "7.0.2",
+ "resolved": "https://registry.npmjs.org/@npmcli/promise-spawn/-/promise-spawn-7.0.2.tgz",
+ "integrity": "sha512-xhfYPXoV5Dy4UkY0D+v2KkwvnDfiA/8Mt3sWCGI/hM03NsYIH8ZaG6QzS9x7pje5vHZBZJ2v6VRFVTWACnqcmQ==",
+ "dev": true,
+ "license": "ISC",
"dependencies": {
- "mkdirp-classic": "^0.5.2",
- "pump": "^3.0.0",
- "tar-stream": "^3.1.5"
+ "which": "^4.0.0"
+ },
+ "engines": {
+ "node": "^16.14.0 || >=18.0.0"
}
},
- "node_modules/@puppeteer/browsers/node_modules/tar-stream": {
- "version": "3.1.7",
- "resolved": "https://registry.npmjs.org/tar-stream/-/tar-stream-3.1.7.tgz",
- "integrity": "sha512-qJj60CXt7IU1Ffyc3NJMjh6EkuCFej46zUqJ4J7pqYlThyd9bO0XBTmcOIhSzZJVWfsLks0+nle/j538YAW9RQ==",
- "dependencies": {
- "b4a": "^1.6.4",
- "fast-fifo": "^1.2.0",
- "streamx": "^2.15.0"
+ "node_modules/@npmcli/promise-spawn/node_modules/isexe": {
+ "version": "3.1.1",
+ "resolved": "https://registry.npmjs.org/isexe/-/isexe-3.1.1.tgz",
+ "integrity": "sha512-LpB/54B+/2J5hqQ7imZHfdU31OlgQqx7ZicVlkm9kzg9/w8GKLEcFfJl/t7DCEDueOyBAD6zCCwTO6Fzs0NoEQ==",
+ "dev": true,
+ "license": "ISC",
+ "engines": {
+ "node": ">=16"
}
},
- "node_modules/@puppeteer/browsers/node_modules/wrap-ansi": {
- "version": "7.0.0",
- "resolved": "https://registry.npmjs.org/wrap-ansi/-/wrap-ansi-7.0.0.tgz",
- "integrity": "sha512-YVGIj2kamLSTxw6NsZjoBxfSwsn0ycdesmc4p+Q21c5zPuZ1pl+NfxVdxPtdHvmNVOQ6XSYG4AUtyt/Fi7D16Q==",
+ "node_modules/@npmcli/promise-spawn/node_modules/which": {
+ "version": "4.0.0",
+ "resolved": "https://registry.npmjs.org/which/-/which-4.0.0.tgz",
+ "integrity": "sha512-GlaYyEb07DPxYCKhKzplCWBJtvxZcZMrL+4UkrTSJHHPyZU4mYYTv3qaOe77H7EODLSSopAUFAc6W8U4yqvscg==",
+ "dev": true,
+ "license": "ISC",
"dependencies": {
- "ansi-styles": "^4.0.0",
- "string-width": "^4.1.0",
- "strip-ansi": "^6.0.0"
+ "isexe": "^3.1.1"
},
- "engines": {
- "node": ">=10"
+ "bin": {
+ "node-which": "bin/which.js"
},
- "funding": {
- "url": "https://github.com/chalk/wrap-ansi?sponsor=1"
- }
- },
- "node_modules/@puppeteer/browsers/node_modules/y18n": {
- "version": "5.0.8",
- "resolved": "https://registry.npmjs.org/y18n/-/y18n-5.0.8.tgz",
- "integrity": "sha512-0pfFzegeDWJHJIAmTLRP2DwHjdF5s7jo9tuztdQxAhINCdvS+3nGINqPd00AphqJR/0LhANUS6/+7SCb98YOfA==",
"engines": {
- "node": ">=10"
+ "node": "^16.13.0 || >=18.0.0"
}
},
- "node_modules/@puppeteer/browsers/node_modules/yargs": {
- "version": "17.7.1",
- "resolved": "https://registry.npmjs.org/yargs/-/yargs-17.7.1.tgz",
- "integrity": "sha512-cwiTb08Xuv5fqF4AovYacTFNxk62th7LKJ6BL9IGUpTJrWoU7/7WdQGTP2SjKf1dUNBGzDd28p/Yfs/GI6JrLw==",
+ "node_modules/@npmcli/query": {
+ "version": "3.1.0",
+ "resolved": "https://registry.npmjs.org/@npmcli/query/-/query-3.1.0.tgz",
+ "integrity": "sha512-C/iR0tk7KSKGldibYIB9x8GtO/0Bd0I2mhOaDb8ucQL/bQVTmGoeREaFj64Z5+iCBRf3dQfed0CjJL7I8iTkiQ==",
+ "dev": true,
+ "license": "ISC",
"dependencies": {
- "cliui": "^8.0.1",
- "escalade": "^3.1.1",
- "get-caller-file": "^2.0.5",
- "require-directory": "^2.1.1",
- "string-width": "^4.2.3",
- "y18n": "^5.0.5",
- "yargs-parser": "^21.1.1"
+ "postcss-selector-parser": "^6.0.10"
},
"engines": {
- "node": ">=12"
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/@puppeteer/browsers/node_modules/yargs-parser": {
- "version": "21.1.1",
- "resolved": "https://registry.npmjs.org/yargs-parser/-/yargs-parser-21.1.1.tgz",
- "integrity": "sha512-tVpsJW7DdjecAiFpbIB1e3qxIQsE6NoPc5/eTdrbbIC4h0LVsWhnoa3g+m2HclBIujHzsxZ4VJVA+GUuc2/LBw==",
+ "node_modules/@npmcli/redact": {
+ "version": "2.0.1",
+ "resolved": "https://registry.npmjs.org/@npmcli/redact/-/redact-2.0.1.tgz",
+ "integrity": "sha512-YgsR5jCQZhVmTJvjduTOIHph0L73pK8xwMVaDY0PatySqVM9AZj93jpoXYSJqfHFxFkN9dmqTw6OiqExsS3LPw==",
+ "dev": true,
+ "license": "ISC",
"engines": {
- "node": ">=12"
+ "node": "^16.14.0 || >=18.0.0"
}
},
- "node_modules/@radix-ui/number": {
- "version": "1.0.1",
- "resolved": "https://registry.npmjs.org/@radix-ui/number/-/number-1.0.1.tgz",
- "integrity": "sha512-T5gIdVO2mmPW3NNhjNgEP3cqMXjXL9UbO0BzWcXfvdBs+BohbQxvd/K5hSVKmn9/lbTdsQVKbUcP5WLCwvUbBg==",
+ "node_modules/@npmcli/run-script": {
+ "version": "8.1.0",
+ "resolved": "https://registry.npmjs.org/@npmcli/run-script/-/run-script-8.1.0.tgz",
+ "integrity": "sha512-y7efHHwghQfk28G2z3tlZ67pLG0XdfYbcVG26r7YIXALRsrVQcTq4/tdenSmdOrEsNahIYA/eh8aEVROWGFUDg==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "@babel/runtime": "^7.13.10"
- }
- },
- "node_modules/@radix-ui/primitive": {
- "version": "1.0.1",
- "resolved": "https://registry.npmjs.org/@radix-ui/primitive/-/primitive-1.0.1.tgz",
- "integrity": "sha512-yQ8oGX2GVsEYMWGxcovu1uGWPCxV5BFfeeYxqPmuAzUyLT9qmaMXSAhXpb0WrspIeqYzdJpkh2vHModJPgRIaw==",
- "license": "MIT",
- "dependencies": {
- "@babel/runtime": "^7.13.10"
+ "@npmcli/node-gyp": "^3.0.0",
+ "@npmcli/package-json": "^5.0.0",
+ "@npmcli/promise-spawn": "^7.0.0",
+ "node-gyp": "^10.0.0",
+ "proc-log": "^4.0.0",
+ "which": "^4.0.0"
+ },
+ "engines": {
+ "node": "^16.14.0 || >=18.0.0"
}
},
- "node_modules/@radix-ui/react-arrow": {
- "version": "1.0.3",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-arrow/-/react-arrow-1.0.3.tgz",
- "integrity": "sha512-wSP+pHsB/jQRaL6voubsQ/ZlrGBHHrOjmBnr19hxYgtS0WvAFwZhK2WP/YY5yF9uKECCEEDGxuLxq1NBK51wFA==",
+ "node_modules/@npmcli/run-script/node_modules/isexe": {
+ "version": "3.1.1",
+ "resolved": "https://registry.npmjs.org/isexe/-/isexe-3.1.1.tgz",
+ "integrity": "sha512-LpB/54B+/2J5hqQ7imZHfdU31OlgQqx7ZicVlkm9kzg9/w8GKLEcFfJl/t7DCEDueOyBAD6zCCwTO6Fzs0NoEQ==",
"dev": true,
- "dependencies": {
- "@babel/runtime": "^7.13.10",
- "@radix-ui/react-primitive": "1.0.3"
- },
- "peerDependencies": {
- "@types/react": "*",
- "@types/react-dom": "*",
- "react": "^16.8 || ^17.0 || ^18.0",
- "react-dom": "^16.8 || ^17.0 || ^18.0"
- },
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- },
- "@types/react-dom": {
- "optional": true
- }
+ "license": "ISC",
+ "engines": {
+ "node": ">=16"
}
},
- "node_modules/@radix-ui/react-collection": {
- "version": "1.0.3",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-collection/-/react-collection-1.0.3.tgz",
- "integrity": "sha512-3SzW+0PW7yBBoQlT8wNcGtaxaD0XSu0uLUFgrtHY08Acx05TaHaOmVLR73c0j/cqpDy53KBMO7s0dx2wmOIDIA==",
+ "node_modules/@npmcli/run-script/node_modules/which": {
+ "version": "4.0.0",
+ "resolved": "https://registry.npmjs.org/which/-/which-4.0.0.tgz",
+ "integrity": "sha512-GlaYyEb07DPxYCKhKzplCWBJtvxZcZMrL+4UkrTSJHHPyZU4mYYTv3qaOe77H7EODLSSopAUFAc6W8U4yqvscg==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "@babel/runtime": "^7.13.10",
- "@radix-ui/react-compose-refs": "1.0.1",
- "@radix-ui/react-context": "1.0.1",
- "@radix-ui/react-primitive": "1.0.3",
- "@radix-ui/react-slot": "1.0.2"
+ "isexe": "^3.1.1"
},
- "peerDependencies": {
- "@types/react": "*",
- "@types/react-dom": "*",
- "react": "^16.8 || ^17.0 || ^18.0",
- "react-dom": "^16.8 || ^17.0 || ^18.0"
+ "bin": {
+ "node-which": "bin/which.js"
},
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- },
- "@types/react-dom": {
- "optional": true
- }
+ "engines": {
+ "node": "^16.13.0 || >=18.0.0"
}
},
- "node_modules/@radix-ui/react-compose-refs": {
- "version": "1.0.1",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-compose-refs/-/react-compose-refs-1.0.1.tgz",
- "integrity": "sha512-fDSBgd44FKHa1FRMU59qBMPFcl2PZE+2nmqunj+BWFyYYjnhIDWL2ItDs3rrbJDQOtzt5nIebLCQc4QRfz6LJw==",
+ "node_modules/@nx/devkit": {
+ "version": "20.2.1",
+ "resolved": "https://registry.npmjs.org/@nx/devkit/-/devkit-20.2.1.tgz",
+ "integrity": "sha512-boNTu7Z7oHkYjrYg5Wzg+cQfbEJ2nntRj1eI99w8mp4qz2B4PEEjJOB0BZafR54ZcKpGEbyp/QBB945GsjTUbw==",
+ "dev": true,
"license": "MIT",
"dependencies": {
- "@babel/runtime": "^7.13.10"
+ "ejs": "^3.1.7",
+ "enquirer": "~2.3.6",
+ "ignore": "^5.0.4",
+ "minimatch": "9.0.3",
+ "semver": "^7.5.3",
+ "tmp": "~0.2.1",
+ "tslib": "^2.3.0",
+ "yargs-parser": "21.1.1"
},
"peerDependencies": {
- "@types/react": "*",
- "react": "^16.8 || ^17.0 || ^18.0"
- },
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- }
+ "nx": ">= 19 <= 21"
}
},
- "node_modules/@radix-ui/react-context": {
- "version": "1.0.1",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-context/-/react-context-1.0.1.tgz",
- "integrity": "sha512-ebbrdFoYTcuZ0v4wG5tedGnp9tzcV8awzsxYph7gXUyvnNLuTIcCk1q17JEbnVhXAKG9oX3KtchwiMIAYp9NLg==",
+ "node_modules/@nx/devkit/node_modules/brace-expansion": {
+ "version": "2.0.1",
+ "resolved": "https://registry.npmjs.org/brace-expansion/-/brace-expansion-2.0.1.tgz",
+ "integrity": "sha512-XnAIvQ8eM+kC6aULx6wuQiwVsnzsi9d3WxzV3FpWTGA19F621kwdbsAcFKXgKUHZWsy+mY6iL1sHTxWEFCytDA==",
+ "dev": true,
"license": "MIT",
"dependencies": {
- "@babel/runtime": "^7.13.10"
- },
- "peerDependencies": {
- "@types/react": "*",
- "react": "^16.8 || ^17.0 || ^18.0"
- },
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- }
+ "balanced-match": "^1.0.0"
}
},
- "node_modules/@radix-ui/react-direction": {
- "version": "1.0.1",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-direction/-/react-direction-1.0.1.tgz",
- "integrity": "sha512-RXcvnXgyvYvBEOhCBuddKecVkoMiI10Jcm5cTI7abJRAHYfFxeu+FBQs/DvdxSYucxR5mna0dNsL6QFlds5TMA==",
+ "node_modules/@nx/devkit/node_modules/minimatch": {
+ "version": "9.0.3",
+ "resolved": "https://registry.npmjs.org/minimatch/-/minimatch-9.0.3.tgz",
+ "integrity": "sha512-RHiac9mvaRw0x3AYRgDC1CxAP7HTcNrrECeA8YYJeWnpo+2Q5CegtZjaotWTWxDG3UeGA1coE05iH1mPjT/2mg==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "@babel/runtime": "^7.13.10"
+ "brace-expansion": "^2.0.1"
},
- "peerDependencies": {
- "@types/react": "*",
- "react": "^16.8 || ^17.0 || ^18.0"
+ "engines": {
+ "node": ">=16 || 14 >=14.17"
},
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- }
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
}
},
- "node_modules/@radix-ui/react-dismissable-layer": {
- "version": "1.0.4",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-dismissable-layer/-/react-dismissable-layer-1.0.4.tgz",
- "integrity": "sha512-7UpBa/RKMoHJYjie1gkF1DlK8l1fdU/VKDpoS3rCCo8YBJR294GwcEHyxHw72yvphJ7ld0AXEcSLAzY2F/WyCg==",
+ "node_modules/@nx/devkit/node_modules/tmp": {
+ "version": "0.2.3",
+ "resolved": "https://registry.npmjs.org/tmp/-/tmp-0.2.3.tgz",
+ "integrity": "sha512-nZD7m9iCPC5g0pYmcaxogYKggSfLsdxl8of3Q/oIbqCqLLIO9IAF0GWjX1z9NZRHPiXv8Wex4yDCaZsgEw0Y8w==",
"dev": true,
"license": "MIT",
- "dependencies": {
- "@babel/runtime": "^7.13.10",
- "@radix-ui/primitive": "1.0.1",
- "@radix-ui/react-compose-refs": "1.0.1",
- "@radix-ui/react-primitive": "1.0.3",
- "@radix-ui/react-use-callback-ref": "1.0.1",
- "@radix-ui/react-use-escape-keydown": "1.0.3"
- },
- "peerDependencies": {
- "@types/react": "*",
- "@types/react-dom": "*",
- "react": "^16.8 || ^17.0 || ^18.0",
- "react-dom": "^16.8 || ^17.0 || ^18.0"
- },
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- },
- "@types/react-dom": {
- "optional": true
- }
+ "engines": {
+ "node": ">=14.14"
}
},
- "node_modules/@radix-ui/react-focus-guards": {
- "version": "1.0.1",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-focus-guards/-/react-focus-guards-1.0.1.tgz",
- "integrity": "sha512-Rect2dWbQ8waGzhMavsIbmSVCgYxkXLxxR3ZvCX79JOglzdEy4JXMb98lq4hPxUbLr77nP0UOGf4rcMU+s1pUA==",
- "license": "MIT",
- "dependencies": {
- "@babel/runtime": "^7.13.10"
- },
- "peerDependencies": {
- "@types/react": "*",
- "react": "^16.8 || ^17.0 || ^18.0"
- },
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- }
+ "node_modules/@nx/devkit/node_modules/yargs-parser": {
+ "version": "21.1.1",
+ "resolved": "https://registry.npmjs.org/yargs-parser/-/yargs-parser-21.1.1.tgz",
+ "integrity": "sha512-tVpsJW7DdjecAiFpbIB1e3qxIQsE6NoPc5/eTdrbbIC4h0LVsWhnoa3g+m2HclBIujHzsxZ4VJVA+GUuc2/LBw==",
+ "dev": true,
+ "license": "ISC",
+ "engines": {
+ "node": ">=12"
}
},
- "node_modules/@radix-ui/react-focus-scope": {
- "version": "1.0.3",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-focus-scope/-/react-focus-scope-1.0.3.tgz",
- "integrity": "sha512-upXdPfqI4islj2CslyfUBNlaJCPybbqRHAi1KER7Isel9Q2AtSJ0zRBZv8mWQiFXD2nyAJ4BhC3yXgZ6kMBSrQ==",
+ "node_modules/@nx/nx-darwin-arm64": {
+ "version": "20.2.1",
+ "resolved": "https://registry.npmjs.org/@nx/nx-darwin-arm64/-/nx-darwin-arm64-20.2.1.tgz",
+ "integrity": "sha512-nJcyPZfH6Vq4cG6gRnQ8PcnVOLePeT3exzLnQu0I4I2EtCTPyCSRA3gxoGzZ3qZFMQTsCbwv4HYfdx42AXOTAQ==",
+ "cpu": [
+ "arm64"
+ ],
"dev": true,
"license": "MIT",
- "dependencies": {
- "@babel/runtime": "^7.13.10",
- "@radix-ui/react-compose-refs": "1.0.1",
- "@radix-ui/react-primitive": "1.0.3",
- "@radix-ui/react-use-callback-ref": "1.0.1"
- },
- "peerDependencies": {
- "@types/react": "*",
- "@types/react-dom": "*",
- "react": "^16.8 || ^17.0 || ^18.0",
- "react-dom": "^16.8 || ^17.0 || ^18.0"
- },
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- },
- "@types/react-dom": {
- "optional": true
- }
+ "optional": true,
+ "os": [
+ "darwin"
+ ],
+ "engines": {
+ "node": ">= 10"
}
},
- "node_modules/@radix-ui/react-id": {
- "version": "1.0.1",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-id/-/react-id-1.0.1.tgz",
- "integrity": "sha512-tI7sT/kqYp8p96yGWY1OAnLHrqDgzHefRBKQ2YAkBS5ja7QLcZ9Z/uY7bEjPUatf8RomoXM8/1sMj1IJaE5UzQ==",
+ "node_modules/@nx/nx-darwin-x64": {
+ "version": "20.2.1",
+ "resolved": "https://registry.npmjs.org/@nx/nx-darwin-x64/-/nx-darwin-x64-20.2.1.tgz",
+ "integrity": "sha512-SEiN8fjEs010ME4PRP8O9f8qG8AMZBGz8hOkF6ZrdlC+iEi4iyAGpgWFq8PKBlpVW4G5gxR91Y7eVaTKAsgH5w==",
+ "cpu": [
+ "x64"
+ ],
+ "dev": true,
"license": "MIT",
- "dependencies": {
- "@babel/runtime": "^7.13.10",
- "@radix-ui/react-use-layout-effect": "1.0.1"
- },
- "peerDependencies": {
- "@types/react": "*",
- "react": "^16.8 || ^17.0 || ^18.0"
- },
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- }
+ "optional": true,
+ "os": [
+ "darwin"
+ ],
+ "engines": {
+ "node": ">= 10"
}
},
- "node_modules/@radix-ui/react-popper": {
- "version": "1.1.2",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-popper/-/react-popper-1.1.2.tgz",
- "integrity": "sha512-1CnGGfFi/bbqtJZZ0P/NQY20xdG3E0LALJaLUEoKwPLwl6PPPfbeiCqMVQnhoFRAxjJj4RpBRJzDmUgsex2tSg==",
+ "node_modules/@nx/nx-freebsd-x64": {
+ "version": "20.2.1",
+ "resolved": "https://registry.npmjs.org/@nx/nx-freebsd-x64/-/nx-freebsd-x64-20.2.1.tgz",
+ "integrity": "sha512-/yEKS9q17EG2Ci130McvpZM5YUghH1ql9UXWbVmitufn+RQD90hoblkG/B+cxJeZonrdKAjdpLQ+hfVz+FBd/g==",
+ "cpu": [
+ "x64"
+ ],
"dev": true,
- "dependencies": {
- "@babel/runtime": "^7.13.10",
- "@floating-ui/react-dom": "^2.0.0",
- "@radix-ui/react-arrow": "1.0.3",
- "@radix-ui/react-compose-refs": "1.0.1",
- "@radix-ui/react-context": "1.0.1",
- "@radix-ui/react-primitive": "1.0.3",
- "@radix-ui/react-use-callback-ref": "1.0.1",
- "@radix-ui/react-use-layout-effect": "1.0.1",
- "@radix-ui/react-use-rect": "1.0.1",
- "@radix-ui/react-use-size": "1.0.1",
- "@radix-ui/rect": "1.0.1"
- },
- "peerDependencies": {
- "@types/react": "*",
- "@types/react-dom": "*",
- "react": "^16.8 || ^17.0 || ^18.0",
- "react-dom": "^16.8 || ^17.0 || ^18.0"
- },
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- },
- "@types/react-dom": {
- "optional": true
- }
+ "license": "MIT",
+ "optional": true,
+ "os": [
+ "freebsd"
+ ],
+ "engines": {
+ "node": ">= 10"
}
},
- "node_modules/@radix-ui/react-portal": {
- "version": "1.0.3",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-portal/-/react-portal-1.0.3.tgz",
- "integrity": "sha512-xLYZeHrWoPmA5mEKEfZZevoVRK/Q43GfzRXkWV6qawIWWK8t6ifIiLQdd7rmQ4Vk1bmI21XhqF9BN3jWf+phpA==",
+ "node_modules/@nx/nx-linux-arm-gnueabihf": {
+ "version": "20.2.1",
+ "resolved": "https://registry.npmjs.org/@nx/nx-linux-arm-gnueabihf/-/nx-linux-arm-gnueabihf-20.2.1.tgz",
+ "integrity": "sha512-DPtRjTCJ5++stTGtjqYftCb2c0CNed2s2EZZLQuDP+tikTsLm0d3S3ZaU5eHhqZW35tQuMOVweOfC1nJ3/DTSA==",
+ "cpu": [
+ "arm"
+ ],
"dev": true,
"license": "MIT",
- "dependencies": {
- "@babel/runtime": "^7.13.10",
- "@radix-ui/react-primitive": "1.0.3"
- },
- "peerDependencies": {
- "@types/react": "*",
- "@types/react-dom": "*",
- "react": "^16.8 || ^17.0 || ^18.0",
- "react-dom": "^16.8 || ^17.0 || ^18.0"
- },
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- },
- "@types/react-dom": {
- "optional": true
- }
+ "optional": true,
+ "os": [
+ "linux"
+ ],
+ "engines": {
+ "node": ">= 10"
}
},
- "node_modules/@radix-ui/react-primitive": {
- "version": "1.0.3",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-primitive/-/react-primitive-1.0.3.tgz",
- "integrity": "sha512-yi58uVyoAcK/Nq1inRY56ZSjKypBNKTa/1mcL8qdl6oJeEaDbOldlzrGn7P6Q3Id5d+SYNGc5AJgc4vGhjs5+g==",
+ "node_modules/@nx/nx-linux-arm64-gnu": {
+ "version": "20.2.1",
+ "resolved": "https://registry.npmjs.org/@nx/nx-linux-arm64-gnu/-/nx-linux-arm64-gnu-20.2.1.tgz",
+ "integrity": "sha512-ggGwHOEP6UjXeqv6DtRxizeBnX/zRZi8BRJbEJBwAt1cAUnLlklk8d+Hmjs+j/FlFXBV9f+ylpAqoYkplFR8jg==",
+ "cpu": [
+ "arm64"
+ ],
+ "dev": true,
"license": "MIT",
- "dependencies": {
- "@babel/runtime": "^7.13.10",
- "@radix-ui/react-slot": "1.0.2"
- },
- "peerDependencies": {
- "@types/react": "*",
- "@types/react-dom": "*",
- "react": "^16.8 || ^17.0 || ^18.0",
- "react-dom": "^16.8 || ^17.0 || ^18.0"
- },
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- },
- "@types/react-dom": {
- "optional": true
- }
+ "optional": true,
+ "os": [
+ "linux"
+ ],
+ "engines": {
+ "node": ">= 10"
}
},
- "node_modules/@radix-ui/react-roving-focus": {
- "version": "1.0.4",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-roving-focus/-/react-roving-focus-1.0.4.tgz",
- "integrity": "sha512-2mUg5Mgcu001VkGy+FfzZyzbmuUWzgWkj3rvv4yu+mLw03+mTzbxZHvfcGyFp2b8EkQeMkpRQ5FiA2Vr2O6TeQ==",
+ "node_modules/@nx/nx-linux-arm64-musl": {
+ "version": "20.2.1",
+ "resolved": "https://registry.npmjs.org/@nx/nx-linux-arm64-musl/-/nx-linux-arm64-musl-20.2.1.tgz",
+ "integrity": "sha512-HZBGxsBJUFbWVTiyJxqt0tS8tlvp+Tp0D533mGKW75cU0rv9dnmbtTwkkkx+LXqerjSRvNS3Qtj0Uh2w92Vtig==",
+ "cpu": [
+ "arm64"
+ ],
"dev": true,
- "dependencies": {
- "@babel/runtime": "^7.13.10",
- "@radix-ui/primitive": "1.0.1",
- "@radix-ui/react-collection": "1.0.3",
- "@radix-ui/react-compose-refs": "1.0.1",
- "@radix-ui/react-context": "1.0.1",
- "@radix-ui/react-direction": "1.0.1",
- "@radix-ui/react-id": "1.0.1",
- "@radix-ui/react-primitive": "1.0.3",
- "@radix-ui/react-use-callback-ref": "1.0.1",
- "@radix-ui/react-use-controllable-state": "1.0.1"
- },
- "peerDependencies": {
- "@types/react": "*",
- "@types/react-dom": "*",
- "react": "^16.8 || ^17.0 || ^18.0",
- "react-dom": "^16.8 || ^17.0 || ^18.0"
- },
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- },
- "@types/react-dom": {
- "optional": true
- }
+ "license": "MIT",
+ "optional": true,
+ "os": [
+ "linux"
+ ],
+ "engines": {
+ "node": ">= 10"
}
},
- "node_modules/@radix-ui/react-select": {
- "version": "1.2.2",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-select/-/react-select-1.2.2.tgz",
- "integrity": "sha512-zI7McXr8fNaSrUY9mZe4x/HC0jTLY9fWNhO1oLWYMQGDXuV4UCivIGTxwioSzO0ZCYX9iSLyWmAh/1TOmX3Cnw==",
+ "node_modules/@nx/nx-linux-x64-gnu": {
+ "version": "20.2.1",
+ "resolved": "https://registry.npmjs.org/@nx/nx-linux-x64-gnu/-/nx-linux-x64-gnu-20.2.1.tgz",
+ "integrity": "sha512-pTytPwGiPRakqz2PKiWTSRNm9taE1U9n0+kRAAFzbOtzeW+eIoebe5xY5QMoZ+XtIZ6pJM2BUOyMD+/TX57r8Q==",
+ "cpu": [
+ "x64"
+ ],
"dev": true,
- "dependencies": {
- "@babel/runtime": "^7.13.10",
- "@radix-ui/number": "1.0.1",
- "@radix-ui/primitive": "1.0.1",
- "@radix-ui/react-collection": "1.0.3",
- "@radix-ui/react-compose-refs": "1.0.1",
- "@radix-ui/react-context": "1.0.1",
- "@radix-ui/react-direction": "1.0.1",
- "@radix-ui/react-dismissable-layer": "1.0.4",
- "@radix-ui/react-focus-guards": "1.0.1",
- "@radix-ui/react-focus-scope": "1.0.3",
- "@radix-ui/react-id": "1.0.1",
- "@radix-ui/react-popper": "1.1.2",
- "@radix-ui/react-portal": "1.0.3",
- "@radix-ui/react-primitive": "1.0.3",
- "@radix-ui/react-slot": "1.0.2",
- "@radix-ui/react-use-callback-ref": "1.0.1",
- "@radix-ui/react-use-controllable-state": "1.0.1",
- "@radix-ui/react-use-layout-effect": "1.0.1",
- "@radix-ui/react-use-previous": "1.0.1",
- "@radix-ui/react-visually-hidden": "1.0.3",
- "aria-hidden": "^1.1.1",
- "react-remove-scroll": "2.5.5"
- },
- "peerDependencies": {
- "@types/react": "*",
- "@types/react-dom": "*",
- "react": "^16.8 || ^17.0 || ^18.0",
- "react-dom": "^16.8 || ^17.0 || ^18.0"
- },
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- },
- "@types/react-dom": {
- "optional": true
- }
+ "license": "MIT",
+ "optional": true,
+ "os": [
+ "linux"
+ ],
+ "engines": {
+ "node": ">= 10"
}
},
- "node_modules/@radix-ui/react-separator": {
- "version": "1.0.3",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-separator/-/react-separator-1.0.3.tgz",
- "integrity": "sha512-itYmTy/kokS21aiV5+Z56MZB54KrhPgn6eHDKkFeOLR34HMN2s8PaN47qZZAGnvupcjxHaFZnW4pQEh0BvvVuw==",
+ "node_modules/@nx/nx-linux-x64-musl": {
+ "version": "20.2.1",
+ "resolved": "https://registry.npmjs.org/@nx/nx-linux-x64-musl/-/nx-linux-x64-musl-20.2.1.tgz",
+ "integrity": "sha512-p3egqe5zmwiDl6xSwHi2K9UZWiKbZ/s/j4qV+pZttzMyNPfhohTeP+VwQqjTeQ1hPBl2YhwmmktEPsIPYJG7YA==",
+ "cpu": [
+ "x64"
+ ],
"dev": true,
- "dependencies": {
- "@babel/runtime": "^7.13.10",
- "@radix-ui/react-primitive": "1.0.3"
- },
- "peerDependencies": {
- "@types/react": "*",
- "@types/react-dom": "*",
- "react": "^16.8 || ^17.0 || ^18.0",
- "react-dom": "^16.8 || ^17.0 || ^18.0"
- },
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- },
- "@types/react-dom": {
- "optional": true
- }
- }
- },
- "node_modules/@radix-ui/react-slot": {
- "version": "1.0.2",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-slot/-/react-slot-1.0.2.tgz",
- "integrity": "sha512-YeTpuq4deV+6DusvVUW4ivBgnkHwECUu0BiN43L5UCDFgdhsRUWAghhTF5MbvNTPzmiFOx90asDSUjWuCNapwg==",
"license": "MIT",
- "dependencies": {
- "@babel/runtime": "^7.13.10",
- "@radix-ui/react-compose-refs": "1.0.1"
- },
- "peerDependencies": {
- "@types/react": "*",
- "react": "^16.8 || ^17.0 || ^18.0"
- },
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- }
- }
- },
- "node_modules/@radix-ui/react-toggle": {
- "version": "1.0.3",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-toggle/-/react-toggle-1.0.3.tgz",
- "integrity": "sha512-Pkqg3+Bc98ftZGsl60CLANXQBBQ4W3mTFS9EJvNxKMZ7magklKV69/id1mlAlOFDDfHvlCms0fx8fA4CMKDJHg==",
- "dev": true,
- "dependencies": {
- "@babel/runtime": "^7.13.10",
- "@radix-ui/primitive": "1.0.1",
- "@radix-ui/react-primitive": "1.0.3",
- "@radix-ui/react-use-controllable-state": "1.0.1"
- },
- "peerDependencies": {
- "@types/react": "*",
- "@types/react-dom": "*",
- "react": "^16.8 || ^17.0 || ^18.0",
- "react-dom": "^16.8 || ^17.0 || ^18.0"
- },
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- },
- "@types/react-dom": {
- "optional": true
- }
- }
- },
- "node_modules/@radix-ui/react-toggle-group": {
- "version": "1.0.4",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-toggle-group/-/react-toggle-group-1.0.4.tgz",
- "integrity": "sha512-Uaj/M/cMyiyT9Bx6fOZO0SAG4Cls0GptBWiBmBxofmDbNVnYYoyRWj/2M/6VCi/7qcXFWnHhRUfdfZFvvkuu8A==",
- "dev": true,
- "dependencies": {
- "@babel/runtime": "^7.13.10",
- "@radix-ui/primitive": "1.0.1",
- "@radix-ui/react-context": "1.0.1",
- "@radix-ui/react-direction": "1.0.1",
- "@radix-ui/react-primitive": "1.0.3",
- "@radix-ui/react-roving-focus": "1.0.4",
- "@radix-ui/react-toggle": "1.0.3",
- "@radix-ui/react-use-controllable-state": "1.0.1"
- },
- "peerDependencies": {
- "@types/react": "*",
- "@types/react-dom": "*",
- "react": "^16.8 || ^17.0 || ^18.0",
- "react-dom": "^16.8 || ^17.0 || ^18.0"
- },
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- },
- "@types/react-dom": {
- "optional": true
- }
+ "optional": true,
+ "os": [
+ "linux"
+ ],
+ "engines": {
+ "node": ">= 10"
}
},
- "node_modules/@radix-ui/react-toolbar": {
- "version": "1.0.4",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-toolbar/-/react-toolbar-1.0.4.tgz",
- "integrity": "sha512-tBgmM/O7a07xbaEkYJWYTXkIdU/1pW4/KZORR43toC/4XWyBCURK0ei9kMUdp+gTPPKBgYLxXmRSH1EVcIDp8Q==",
+ "node_modules/@nx/nx-win32-arm64-msvc": {
+ "version": "20.2.1",
+ "resolved": "https://registry.npmjs.org/@nx/nx-win32-arm64-msvc/-/nx-win32-arm64-msvc-20.2.1.tgz",
+ "integrity": "sha512-Wujist6k08pjgWWQ1pjXrCArmMgnyIXNVmDP14cWo1KHecBuxNWa9i62PrxQ0K8MLYMcAzLHJxN9t54GzBbd+g==",
+ "cpu": [
+ "arm64"
+ ],
"dev": true,
- "dependencies": {
- "@babel/runtime": "^7.13.10",
- "@radix-ui/primitive": "1.0.1",
- "@radix-ui/react-context": "1.0.1",
- "@radix-ui/react-direction": "1.0.1",
- "@radix-ui/react-primitive": "1.0.3",
- "@radix-ui/react-roving-focus": "1.0.4",
- "@radix-ui/react-separator": "1.0.3",
- "@radix-ui/react-toggle-group": "1.0.4"
- },
- "peerDependencies": {
- "@types/react": "*",
- "@types/react-dom": "*",
- "react": "^16.8 || ^17.0 || ^18.0",
- "react-dom": "^16.8 || ^17.0 || ^18.0"
- },
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- },
- "@types/react-dom": {
- "optional": true
- }
- }
- },
- "node_modules/@radix-ui/react-use-callback-ref": {
- "version": "1.0.1",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-use-callback-ref/-/react-use-callback-ref-1.0.1.tgz",
- "integrity": "sha512-D94LjX4Sp0xJFVaoQOd3OO9k7tpBYNOXdVhkltUbGv2Qb9OXdrg/CpsjlZv7ia14Sylv398LswWBVVu5nqKzAQ==",
"license": "MIT",
- "dependencies": {
- "@babel/runtime": "^7.13.10"
- },
- "peerDependencies": {
- "@types/react": "*",
- "react": "^16.8 || ^17.0 || ^18.0"
- },
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- }
- }
- },
- "node_modules/@radix-ui/react-use-controllable-state": {
- "version": "1.0.1",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-use-controllable-state/-/react-use-controllable-state-1.0.1.tgz",
- "integrity": "sha512-Svl5GY5FQeN758fWKrjM6Qb7asvXeiZltlT4U2gVfl8Gx5UAv2sMR0LWo8yhsIZh2oQ0eFdZ59aoOOMV7b47VA==",
- "license": "MIT",
- "dependencies": {
- "@babel/runtime": "^7.13.10",
- "@radix-ui/react-use-callback-ref": "1.0.1"
- },
- "peerDependencies": {
- "@types/react": "*",
- "react": "^16.8 || ^17.0 || ^18.0"
- },
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- }
- }
- },
- "node_modules/@radix-ui/react-use-escape-keydown": {
- "version": "1.0.3",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-use-escape-keydown/-/react-use-escape-keydown-1.0.3.tgz",
- "integrity": "sha512-vyL82j40hcFicA+M4Ex7hVkB9vHgSse1ZWomAqV2Je3RleKGO5iM8KMOEtfoSB0PnIelMd2lATjTGMYqN5ylTg==",
- "license": "MIT",
- "dependencies": {
- "@babel/runtime": "^7.13.10",
- "@radix-ui/react-use-callback-ref": "1.0.1"
- },
- "peerDependencies": {
- "@types/react": "*",
- "react": "^16.8 || ^17.0 || ^18.0"
- },
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- }
- }
- },
- "node_modules/@radix-ui/react-use-layout-effect": {
- "version": "1.0.1",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-use-layout-effect/-/react-use-layout-effect-1.0.1.tgz",
- "integrity": "sha512-v/5RegiJWYdoCvMnITBkNNx6bCj20fiaJnWtRkU18yITptraXjffz5Qbn05uOiQnOvi+dbkznkoaMltz1GnszQ==",
- "license": "MIT",
- "dependencies": {
- "@babel/runtime": "^7.13.10"
- },
- "peerDependencies": {
- "@types/react": "*",
- "react": "^16.8 || ^17.0 || ^18.0"
- },
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- }
- }
- },
- "node_modules/@radix-ui/react-use-previous": {
- "version": "1.0.1",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-use-previous/-/react-use-previous-1.0.1.tgz",
- "integrity": "sha512-cV5La9DPwiQ7S0gf/0qiD6YgNqM5Fk97Kdrlc5yBcrF3jyEZQwm7vYFqMo4IfeHgJXsRaMvLABFtd0OVEmZhDw==",
- "dev": true,
- "dependencies": {
- "@babel/runtime": "^7.13.10"
- },
- "peerDependencies": {
- "@types/react": "*",
- "react": "^16.8 || ^17.0 || ^18.0"
- },
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- }
- }
- },
- "node_modules/@radix-ui/react-use-rect": {
- "version": "1.0.1",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-use-rect/-/react-use-rect-1.0.1.tgz",
- "integrity": "sha512-Cq5DLuSiuYVKNU8orzJMbl15TXilTnJKUCltMVQg53BQOF1/C5toAaGrowkgksdBQ9H+SRL23g0HDmg9tvmxXw==",
- "dev": true,
- "dependencies": {
- "@babel/runtime": "^7.13.10",
- "@radix-ui/rect": "1.0.1"
- },
- "peerDependencies": {
- "@types/react": "*",
- "react": "^16.8 || ^17.0 || ^18.0"
- },
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- }
- }
- },
- "node_modules/@radix-ui/react-use-size": {
- "version": "1.0.1",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-use-size/-/react-use-size-1.0.1.tgz",
- "integrity": "sha512-ibay+VqrgcaI6veAojjofPATwledXiSmX+C0KrBk/xgpX9rBzPV3OsfwlhQdUOFbh+LKQorLYT+xTXW9V8yd0g==",
- "dev": true,
- "dependencies": {
- "@babel/runtime": "^7.13.10",
- "@radix-ui/react-use-layout-effect": "1.0.1"
- },
- "peerDependencies": {
- "@types/react": "*",
- "react": "^16.8 || ^17.0 || ^18.0"
- },
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- }
- }
- },
- "node_modules/@radix-ui/react-visually-hidden": {
- "version": "1.0.3",
- "resolved": "https://registry.npmjs.org/@radix-ui/react-visually-hidden/-/react-visually-hidden-1.0.3.tgz",
- "integrity": "sha512-D4w41yN5YRKtu464TLnByKzMDG/JlMPHtfZgQAu9v6mNakUqGUI9vUrfQKz8NK41VMm/xbZbh76NUTVtIYqOMA==",
- "dev": true,
- "dependencies": {
- "@babel/runtime": "^7.13.10",
- "@radix-ui/react-primitive": "1.0.3"
- },
- "peerDependencies": {
- "@types/react": "*",
- "@types/react-dom": "*",
- "react": "^16.8 || ^17.0 || ^18.0",
- "react-dom": "^16.8 || ^17.0 || ^18.0"
- },
- "peerDependenciesMeta": {
- "@types/react": {
- "optional": true
- },
- "@types/react-dom": {
- "optional": true
- }
- }
- },
- "node_modules/@radix-ui/rect": {
- "version": "1.0.1",
- "resolved": "https://registry.npmjs.org/@radix-ui/rect/-/rect-1.0.1.tgz",
- "integrity": "sha512-fyrgCaedtvMg9NK3en0pnOYJdtfwxUcNolezkNPUsoX57X8oQk+NkqcvzHXD2uKNij6GXmWU9NDru2IWjrO4BQ==",
- "dev": true,
- "dependencies": {
- "@babel/runtime": "^7.13.10"
- }
- },
- "node_modules/@react-native-clipboard/clipboard": {
- "version": "1.11.2",
- "resolved": "https://registry.npmjs.org/@react-native-clipboard/clipboard/-/clipboard-1.11.2.tgz",
- "integrity": "sha512-bHyZVW62TuleiZsXNHS1Pv16fWc0fh8O9WvBzl4h2fykqZRW9a+Pv/RGTH56E3X2PqzHP38K5go8zmCZUoIsoQ==",
- "peerDependencies": {
- "react": ">=16.0",
- "react-native": ">=0.57.0"
- }
- },
- "node_modules/@react-native-community/blur": {
- "version": "4.2.0",
- "resolved": "https://registry.npmjs.org/@react-native-community/blur/-/blur-4.2.0.tgz",
- "integrity": "sha512-StgP5zQJOCHqDRjmcKnzVkJ920S6DYBKRJfigSUnlkNQp+HzZtVtyKq0j5a7x84NtHcV7j8Uy5mz1Lx9ZKRKfA==",
- "peerDependencies": {
- "react": "*",
- "react-native": "*"
- }
- },
- "node_modules/@react-native-community/cli": {
- "version": "12.3.2",
- "resolved": "https://registry.npmjs.org/@react-native-community/cli/-/cli-12.3.2.tgz",
- "integrity": "sha512-WgoUWwLDcf/G1Su2COUUVs3RzAwnV/vUTdISSpAUGgSc57mPabaAoUctKTnfYEhCnE3j02k3VtaVPwCAFRO3TQ==",
- "dependencies": {
- "@react-native-community/cli-clean": "12.3.2",
- "@react-native-community/cli-config": "12.3.2",
- "@react-native-community/cli-debugger-ui": "12.3.2",
- "@react-native-community/cli-doctor": "12.3.2",
- "@react-native-community/cli-hermes": "12.3.2",
- "@react-native-community/cli-plugin-metro": "12.3.2",
- "@react-native-community/cli-server-api": "12.3.2",
- "@react-native-community/cli-tools": "12.3.2",
- "@react-native-community/cli-types": "12.3.2",
- "chalk": "^4.1.2",
- "commander": "^9.4.1",
- "deepmerge": "^4.3.0",
- "execa": "^5.0.0",
- "find-up": "^4.1.0",
- "fs-extra": "^8.1.0",
- "graceful-fs": "^4.1.3",
- "prompts": "^2.4.2",
- "semver": "^7.5.2"
- },
- "bin": {
- "react-native": "build/bin.js"
- },
- "engines": {
- "node": ">=18"
- }
- },
- "node_modules/@react-native-community/cli-clean": {
- "version": "12.3.2",
- "resolved": "https://registry.npmjs.org/@react-native-community/cli-clean/-/cli-clean-12.3.2.tgz",
- "integrity": "sha512-90k2hCX0ddSFPT7EN7h5SZj0XZPXP0+y/++v262hssoey3nhurwF57NGWN0XAR0o9BSW7+mBfeInfabzDraO6A==",
- "dependencies": {
- "@react-native-community/cli-tools": "12.3.2",
- "chalk": "^4.1.2",
- "execa": "^5.0.0"
- }
- },
- "node_modules/@react-native-community/cli-clean/node_modules/chalk": {
- "version": "4.1.2",
- "resolved": "https://registry.npmjs.org/chalk/-/chalk-4.1.2.tgz",
- "integrity": "sha512-oKnbhFyRIXpUuez8iBMmyEa4nbj4IOQyuhc/wy9kY7/WVPcwIO9VA668Pu8RkO7+0G76SLROeyw9CpQ061i4mA==",
- "dependencies": {
- "ansi-styles": "^4.1.0",
- "supports-color": "^7.1.0"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/chalk/chalk?sponsor=1"
- }
- },
- "node_modules/@react-native-community/cli-clean/node_modules/cross-spawn": {
- "version": "7.0.3",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-7.0.3.tgz",
- "integrity": "sha512-iRDPJKUPVEND7dHPO8rkbOnPpyDygcDFtWjpeWNCgy8WP2rXcxXL8TskReQl6OrB2G7+UJrags1q15Fudc7G6w==",
- "dependencies": {
- "path-key": "^3.1.0",
- "shebang-command": "^2.0.0",
- "which": "^2.0.1"
- },
- "engines": {
- "node": ">= 8"
- }
- },
- "node_modules/@react-native-community/cli-clean/node_modules/execa": {
- "version": "5.1.1",
- "resolved": "https://registry.npmjs.org/execa/-/execa-5.1.1.tgz",
- "integrity": "sha512-8uSpZZocAZRBAPIEINJj3Lo9HyGitllczc27Eh5YYojjMFMn8yHMDMaUHE2Jqfq05D/wucwI4JGURyXt1vchyg==",
- "dependencies": {
- "cross-spawn": "^7.0.3",
- "get-stream": "^6.0.0",
- "human-signals": "^2.1.0",
- "is-stream": "^2.0.0",
- "merge-stream": "^2.0.0",
- "npm-run-path": "^4.0.1",
- "onetime": "^5.1.2",
- "signal-exit": "^3.0.3",
- "strip-final-newline": "^2.0.0"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/sindresorhus/execa?sponsor=1"
- }
- },
- "node_modules/@react-native-community/cli-clean/node_modules/get-stream": {
- "version": "6.0.1",
- "resolved": "https://registry.npmjs.org/get-stream/-/get-stream-6.0.1.tgz",
- "integrity": "sha512-ts6Wi+2j3jQjqi70w5AlN8DFnkSwC+MqmxEzdEALB2qXZYV3X/b1CTfgPLGJNMeAWxdPfU8FO1ms3NUfaHCPYg==",
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/@react-native-community/cli-clean/node_modules/has-flag": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/has-flag/-/has-flag-4.0.0.tgz",
- "integrity": "sha512-EykJT/Q1KjTWctppgIAgfSO0tKVuZUjhgMr17kqTumMl6Afv3EISleU7qZUzoXDFTAHTDC4NOoG/ZxU3EvlMPQ==",
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@react-native-community/cli-clean/node_modules/human-signals": {
- "version": "2.1.0",
- "resolved": "https://registry.npmjs.org/human-signals/-/human-signals-2.1.0.tgz",
- "integrity": "sha512-B4FFZ6q/T2jhhksgkbEW3HBvWIfDW85snkQgawt07S7J5QXTk6BkNV+0yAeZrM5QpMAdYlocGoljn0sJ/WQkFw==",
- "engines": {
- "node": ">=10.17.0"
- }
- },
- "node_modules/@react-native-community/cli-clean/node_modules/is-stream": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/is-stream/-/is-stream-2.0.1.tgz",
- "integrity": "sha512-hFoiJiTl63nn+kstHGBtewWSKnQLpyb155KHheA1l39uvtO9nWIop1p3udqPcUd/xbF1VLMO4n7OI6p7RbngDg==",
- "engines": {
- "node": ">=8"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/@react-native-community/cli-clean/node_modules/mimic-fn": {
- "version": "2.1.0",
- "resolved": "https://registry.npmjs.org/mimic-fn/-/mimic-fn-2.1.0.tgz",
- "integrity": "sha512-OqbOk5oEQeAZ8WXWydlu9HJjz9WVdEIvamMCcXmuqUYjTknH/sqsWvhQ3vgwKFRR1HpjvNBKQ37nbJgYzGqGcg==",
- "engines": {
- "node": ">=6"
- }
- },
- "node_modules/@react-native-community/cli-clean/node_modules/npm-run-path": {
- "version": "4.0.1",
- "resolved": "https://registry.npmjs.org/npm-run-path/-/npm-run-path-4.0.1.tgz",
- "integrity": "sha512-S48WzZW777zhNIrn7gxOlISNAqi9ZC/uQFnRdbeIHhZhCA6UqpkOT8T1G7BvfdgP4Er8gF4sUbaS0i7QvIfCWw==",
- "dependencies": {
- "path-key": "^3.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@react-native-community/cli-clean/node_modules/onetime": {
- "version": "5.1.2",
- "resolved": "https://registry.npmjs.org/onetime/-/onetime-5.1.2.tgz",
- "integrity": "sha512-kbpaSSGJTWdAY5KPVeMOKXSrPtr8C8C7wodJbcsd51jRnmD+GZu8Y0VoU6Dm5Z4vWr0Ig/1NKuWRKf7j5aaYSg==",
- "dependencies": {
- "mimic-fn": "^2.1.0"
- },
- "engines": {
- "node": ">=6"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/@react-native-community/cli-clean/node_modules/path-key": {
- "version": "3.1.1",
- "resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
- "integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@react-native-community/cli-clean/node_modules/shebang-command": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/shebang-command/-/shebang-command-2.0.0.tgz",
- "integrity": "sha512-kHxr2zZpYtdmrN1qDjrrX/Z1rR1kG8Dx+gkpK1G4eXmvXswmcE1hTWBWYUzlraYw1/yZp6YuDY77YtvbN0dmDA==",
- "dependencies": {
- "shebang-regex": "^3.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@react-native-community/cli-clean/node_modules/shebang-regex": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/shebang-regex/-/shebang-regex-3.0.0.tgz",
- "integrity": "sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==",
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@react-native-community/cli-clean/node_modules/supports-color": {
- "version": "7.2.0",
- "resolved": "https://registry.npmjs.org/supports-color/-/supports-color-7.2.0.tgz",
- "integrity": "sha512-qpCAvRl9stuOHveKsn7HncJRvv501qIacKzQlO/+Lwxc9+0q2wLyv4Dfvt80/DPn2pqOBsJdDiogXGR9+OvwRw==",
- "dependencies": {
- "has-flag": "^4.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@react-native-community/cli-clean/node_modules/which": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
- "integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
- "dependencies": {
- "isexe": "^2.0.0"
- },
- "bin": {
- "node-which": "bin/node-which"
- },
- "engines": {
- "node": ">= 8"
- }
- },
- "node_modules/@react-native-community/cli-config": {
- "version": "12.3.2",
- "resolved": "https://registry.npmjs.org/@react-native-community/cli-config/-/cli-config-12.3.2.tgz",
- "integrity": "sha512-UUCzDjQgvAVL/57rL7eOuFUhd+d+6qfM7V8uOegQFeFEmSmvUUDLYoXpBa5vAK9JgQtSqMBJ1Shmwao+/oElxQ==",
- "dependencies": {
- "@react-native-community/cli-tools": "12.3.2",
- "chalk": "^4.1.2",
- "cosmiconfig": "^5.1.0",
- "deepmerge": "^4.3.0",
- "glob": "^7.1.3",
- "joi": "^17.2.1"
- }
- },
- "node_modules/@react-native-community/cli-config/node_modules/chalk": {
- "version": "4.1.2",
- "resolved": "https://registry.npmjs.org/chalk/-/chalk-4.1.2.tgz",
- "integrity": "sha512-oKnbhFyRIXpUuez8iBMmyEa4nbj4IOQyuhc/wy9kY7/WVPcwIO9VA668Pu8RkO7+0G76SLROeyw9CpQ061i4mA==",
- "dependencies": {
- "ansi-styles": "^4.1.0",
- "supports-color": "^7.1.0"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/chalk/chalk?sponsor=1"
- }
- },
- "node_modules/@react-native-community/cli-config/node_modules/cosmiconfig": {
- "version": "5.2.1",
- "resolved": "https://registry.npmjs.org/cosmiconfig/-/cosmiconfig-5.2.1.tgz",
- "integrity": "sha512-H65gsXo1SKjf8zmrJ67eJk8aIRKV5ff2D4uKZIBZShbhGSpEmsQOPW/SKMKYhSTrqR7ufy6RP69rPogdaPh/kA==",
- "dependencies": {
- "import-fresh": "^2.0.0",
- "is-directory": "^0.3.1",
- "js-yaml": "^3.13.1",
- "parse-json": "^4.0.0"
- },
- "engines": {
- "node": ">=4"
- }
- },
- "node_modules/@react-native-community/cli-config/node_modules/glob": {
- "version": "7.2.3",
- "resolved": "https://registry.npmjs.org/glob/-/glob-7.2.3.tgz",
- "integrity": "sha512-nFR0zLpU2YCaRxwoCJvL6UvCH2JFyFVIvwTLsIf21AuHlMskA1hhTdk+LlYJtOlYt9v6dvszD2BGRqBL+iQK9Q==",
- "dependencies": {
- "fs.realpath": "^1.0.0",
- "inflight": "^1.0.4",
- "inherits": "2",
- "minimatch": "^3.1.1",
- "once": "^1.3.0",
- "path-is-absolute": "^1.0.0"
- },
- "engines": {
- "node": "*"
- },
- "funding": {
- "url": "https://github.com/sponsors/isaacs"
- }
- },
- "node_modules/@react-native-community/cli-config/node_modules/has-flag": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/has-flag/-/has-flag-4.0.0.tgz",
- "integrity": "sha512-EykJT/Q1KjTWctppgIAgfSO0tKVuZUjhgMr17kqTumMl6Afv3EISleU7qZUzoXDFTAHTDC4NOoG/ZxU3EvlMPQ==",
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@react-native-community/cli-config/node_modules/import-fresh": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/import-fresh/-/import-fresh-2.0.0.tgz",
- "integrity": "sha512-eZ5H8rcgYazHbKC3PG4ClHNykCSxtAhxSSEM+2mb+7evD2CKF5V7c0dNum7AdpDh0ZdICwZY9sRSn8f+KH96sg==",
- "dependencies": {
- "caller-path": "^2.0.0",
- "resolve-from": "^3.0.0"
- },
- "engines": {
- "node": ">=4"
- }
- },
- "node_modules/@react-native-community/cli-config/node_modules/parse-json": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/parse-json/-/parse-json-4.0.0.tgz",
- "integrity": "sha512-aOIos8bujGN93/8Ox/jPLh7RwVnPEysynVFE+fQZyg6jKELEHwzgKdLRFHUgXJL6kylijVSBC4BvN9OmsB48Rw==",
- "dependencies": {
- "error-ex": "^1.3.1",
- "json-parse-better-errors": "^1.0.1"
- },
- "engines": {
- "node": ">=4"
- }
- },
- "node_modules/@react-native-community/cli-config/node_modules/supports-color": {
- "version": "7.2.0",
- "resolved": "https://registry.npmjs.org/supports-color/-/supports-color-7.2.0.tgz",
- "integrity": "sha512-qpCAvRl9stuOHveKsn7HncJRvv501qIacKzQlO/+Lwxc9+0q2wLyv4Dfvt80/DPn2pqOBsJdDiogXGR9+OvwRw==",
- "dependencies": {
- "has-flag": "^4.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@react-native-community/cli-debugger-ui": {
- "version": "12.3.2",
- "resolved": "https://registry.npmjs.org/@react-native-community/cli-debugger-ui/-/cli-debugger-ui-12.3.2.tgz",
- "integrity": "sha512-nSWQUL+51J682DlfcC1bjkUbQbGvHCC25jpqTwHIjmmVjYCX1uHuhPSqQKgPNdvtfOkrkACxczd7kVMmetxY2Q==",
- "dependencies": {
- "serve-static": "^1.13.1"
- }
- },
- "node_modules/@react-native-community/cli-doctor": {
- "version": "12.3.2",
- "resolved": "https://registry.npmjs.org/@react-native-community/cli-doctor/-/cli-doctor-12.3.2.tgz",
- "integrity": "sha512-GrAabdY4qtBX49knHFvEAdLtCjkmndjTeqhYO6BhsbAeKOtspcLT/0WRgdLIaKODRa61ADNB3K5Zm4dU0QrZOg==",
- "dependencies": {
- "@react-native-community/cli-config": "12.3.2",
- "@react-native-community/cli-platform-android": "12.3.2",
- "@react-native-community/cli-platform-ios": "12.3.2",
- "@react-native-community/cli-tools": "12.3.2",
- "chalk": "^4.1.2",
- "command-exists": "^1.2.8",
- "deepmerge": "^4.3.0",
- "envinfo": "^7.10.0",
- "execa": "^5.0.0",
- "hermes-profile-transformer": "^0.0.6",
- "ip": "^1.1.5",
- "node-stream-zip": "^1.9.1",
- "ora": "^5.4.1",
- "semver": "^7.5.2",
- "strip-ansi": "^5.2.0",
- "wcwidth": "^1.0.1",
- "yaml": "^2.2.1"
- }
- },
- "node_modules/@react-native-community/cli-doctor/node_modules/chalk": {
- "version": "4.1.2",
- "resolved": "https://registry.npmjs.org/chalk/-/chalk-4.1.2.tgz",
- "integrity": "sha512-oKnbhFyRIXpUuez8iBMmyEa4nbj4IOQyuhc/wy9kY7/WVPcwIO9VA668Pu8RkO7+0G76SLROeyw9CpQ061i4mA==",
- "dependencies": {
- "ansi-styles": "^4.1.0",
- "supports-color": "^7.1.0"
- },
+ "optional": true,
+ "os": [
+ "win32"
+ ],
"engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/chalk/chalk?sponsor=1"
+ "node": ">= 10"
}
},
- "node_modules/@react-native-community/cli-doctor/node_modules/cli-cursor": {
- "version": "3.1.0",
- "resolved": "https://registry.npmjs.org/cli-cursor/-/cli-cursor-3.1.0.tgz",
- "integrity": "sha512-I/zHAwsKf9FqGoXM4WWRACob9+SNukZTd94DWF57E4toouRulbCxcUh6RKUEOQlYTHJnzkPMySvPNaaSLNfLZw==",
- "dependencies": {
- "restore-cursor": "^3.1.0"
- },
+ "node_modules/@nx/nx-win32-x64-msvc": {
+ "version": "20.2.1",
+ "resolved": "https://registry.npmjs.org/@nx/nx-win32-x64-msvc/-/nx-win32-x64-msvc-20.2.1.tgz",
+ "integrity": "sha512-tsEYfNV2+CWSQmbh9TM8cX5wk6F2QAH0tfvt4topyOOaR40eszW8qc/eDM/kkJ5nj87BbNEqPBQAYFE0AP1OMA==",
+ "cpu": [
+ "x64"
+ ],
+ "dev": true,
+ "license": "MIT",
+ "optional": true,
+ "os": [
+ "win32"
+ ],
"engines": {
- "node": ">=8"
+ "node": ">= 10"
}
},
- "node_modules/@react-native-community/cli-doctor/node_modules/cross-spawn": {
- "version": "7.0.3",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-7.0.3.tgz",
- "integrity": "sha512-iRDPJKUPVEND7dHPO8rkbOnPpyDygcDFtWjpeWNCgy8WP2rXcxXL8TskReQl6OrB2G7+UJrags1q15Fudc7G6w==",
+ "node_modules/@octokit/auth-token": {
+ "version": "2.5.0",
+ "resolved": "https://registry.npmjs.org/@octokit/auth-token/-/auth-token-2.5.0.tgz",
+ "integrity": "sha512-r5FVUJCOLl19AxiuZD2VRZ/ORjp/4IN98Of6YJoJOkY75CIBuYfmiNHGrDwXr+aLGG55igl9QrxX3hbiXlLb+g==",
"dependencies": {
- "path-key": "^3.1.0",
- "shebang-command": "^2.0.0",
- "which": "^2.0.1"
- },
- "engines": {
- "node": ">= 8"
+ "@octokit/types": "^6.0.3"
}
},
- "node_modules/@react-native-community/cli-doctor/node_modules/execa": {
- "version": "5.1.1",
- "resolved": "https://registry.npmjs.org/execa/-/execa-5.1.1.tgz",
- "integrity": "sha512-8uSpZZocAZRBAPIEINJj3Lo9HyGitllczc27Eh5YYojjMFMn8yHMDMaUHE2Jqfq05D/wucwI4JGURyXt1vchyg==",
+ "node_modules/@octokit/core": {
+ "version": "3.6.0",
+ "resolved": "https://registry.npmjs.org/@octokit/core/-/core-3.6.0.tgz",
+ "integrity": "sha512-7RKRKuA4xTjMhY+eG3jthb3hlZCsOwg3rztWh75Xc+ShDWOfDDATWbeZpAHBNRpm4Tv9WgBMOy1zEJYXG6NJ7Q==",
"dependencies": {
- "cross-spawn": "^7.0.3",
- "get-stream": "^6.0.0",
- "human-signals": "^2.1.0",
- "is-stream": "^2.0.0",
- "merge-stream": "^2.0.0",
- "npm-run-path": "^4.0.1",
- "onetime": "^5.1.2",
- "signal-exit": "^3.0.3",
- "strip-final-newline": "^2.0.0"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/sindresorhus/execa?sponsor=1"
- }
- },
- "node_modules/@react-native-community/cli-doctor/node_modules/get-stream": {
- "version": "6.0.1",
- "resolved": "https://registry.npmjs.org/get-stream/-/get-stream-6.0.1.tgz",
- "integrity": "sha512-ts6Wi+2j3jQjqi70w5AlN8DFnkSwC+MqmxEzdEALB2qXZYV3X/b1CTfgPLGJNMeAWxdPfU8FO1ms3NUfaHCPYg==",
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/@react-native-community/cli-doctor/node_modules/has-flag": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/has-flag/-/has-flag-4.0.0.tgz",
- "integrity": "sha512-EykJT/Q1KjTWctppgIAgfSO0tKVuZUjhgMr17kqTumMl6Afv3EISleU7qZUzoXDFTAHTDC4NOoG/ZxU3EvlMPQ==",
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@react-native-community/cli-doctor/node_modules/human-signals": {
- "version": "2.1.0",
- "resolved": "https://registry.npmjs.org/human-signals/-/human-signals-2.1.0.tgz",
- "integrity": "sha512-B4FFZ6q/T2jhhksgkbEW3HBvWIfDW85snkQgawt07S7J5QXTk6BkNV+0yAeZrM5QpMAdYlocGoljn0sJ/WQkFw==",
- "engines": {
- "node": ">=10.17.0"
- }
- },
- "node_modules/@react-native-community/cli-doctor/node_modules/is-stream": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/is-stream/-/is-stream-2.0.1.tgz",
- "integrity": "sha512-hFoiJiTl63nn+kstHGBtewWSKnQLpyb155KHheA1l39uvtO9nWIop1p3udqPcUd/xbF1VLMO4n7OI6p7RbngDg==",
- "engines": {
- "node": ">=8"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/@react-native-community/cli-doctor/node_modules/mimic-fn": {
- "version": "2.1.0",
- "resolved": "https://registry.npmjs.org/mimic-fn/-/mimic-fn-2.1.0.tgz",
- "integrity": "sha512-OqbOk5oEQeAZ8WXWydlu9HJjz9WVdEIvamMCcXmuqUYjTknH/sqsWvhQ3vgwKFRR1HpjvNBKQ37nbJgYzGqGcg==",
- "engines": {
- "node": ">=6"
+ "@octokit/auth-token": "^2.4.4",
+ "@octokit/graphql": "^4.5.8",
+ "@octokit/request": "^5.6.3",
+ "@octokit/request-error": "^2.0.5",
+ "@octokit/types": "^6.0.3",
+ "before-after-hook": "^2.2.0",
+ "universal-user-agent": "^6.0.0"
}
},
- "node_modules/@react-native-community/cli-doctor/node_modules/npm-run-path": {
- "version": "4.0.1",
- "resolved": "https://registry.npmjs.org/npm-run-path/-/npm-run-path-4.0.1.tgz",
- "integrity": "sha512-S48WzZW777zhNIrn7gxOlISNAqi9ZC/uQFnRdbeIHhZhCA6UqpkOT8T1G7BvfdgP4Er8gF4sUbaS0i7QvIfCWw==",
+ "node_modules/@octokit/core/node_modules/@octokit/endpoint": {
+ "version": "6.0.12",
+ "resolved": "https://registry.npmjs.org/@octokit/endpoint/-/endpoint-6.0.12.tgz",
+ "integrity": "sha512-lF3puPwkQWGfkMClXb4k/eUT/nZKQfxinRWJrdZaJO85Dqwo/G0yOC434Jr2ojwafWJMYqFGFa5ms4jJUgujdA==",
"dependencies": {
- "path-key": "^3.0.0"
- },
- "engines": {
- "node": ">=8"
+ "@octokit/types": "^6.0.3",
+ "is-plain-object": "^5.0.0",
+ "universal-user-agent": "^6.0.0"
}
},
- "node_modules/@react-native-community/cli-doctor/node_modules/onetime": {
- "version": "5.1.2",
- "resolved": "https://registry.npmjs.org/onetime/-/onetime-5.1.2.tgz",
- "integrity": "sha512-kbpaSSGJTWdAY5KPVeMOKXSrPtr8C8C7wodJbcsd51jRnmD+GZu8Y0VoU6Dm5Z4vWr0Ig/1NKuWRKf7j5aaYSg==",
+ "node_modules/@octokit/core/node_modules/@octokit/request": {
+ "version": "5.6.3",
+ "resolved": "https://registry.npmjs.org/@octokit/request/-/request-5.6.3.tgz",
+ "integrity": "sha512-bFJl0I1KVc9jYTe9tdGGpAMPy32dLBXXo1dS/YwSCTL/2nd9XeHsY616RE3HPXDVk+a+dBuzyz5YdlXwcDTr2A==",
"dependencies": {
- "mimic-fn": "^2.1.0"
- },
- "engines": {
- "node": ">=6"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "@octokit/endpoint": "^6.0.1",
+ "@octokit/request-error": "^2.1.0",
+ "@octokit/types": "^6.16.1",
+ "is-plain-object": "^5.0.0",
+ "node-fetch": "^2.6.7",
+ "universal-user-agent": "^6.0.0"
}
},
- "node_modules/@react-native-community/cli-doctor/node_modules/ora": {
- "version": "5.4.1",
- "resolved": "https://registry.npmjs.org/ora/-/ora-5.4.1.tgz",
- "integrity": "sha512-5b6Y85tPxZZ7QytO+BQzysW31HJku27cRIlkbAXaNx+BdcVi+LlRFmVXzeF6a7JCwJpyw5c4b+YSVImQIrBpuQ==",
- "dependencies": {
- "bl": "^4.1.0",
- "chalk": "^4.1.0",
- "cli-cursor": "^3.1.0",
- "cli-spinners": "^2.5.0",
- "is-interactive": "^1.0.0",
- "is-unicode-supported": "^0.1.0",
- "log-symbols": "^4.1.0",
- "strip-ansi": "^6.0.0",
- "wcwidth": "^1.0.1"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
+ "node_modules/@octokit/core/node_modules/before-after-hook": {
+ "version": "2.2.3",
+ "resolved": "https://registry.npmjs.org/before-after-hook/-/before-after-hook-2.2.3.tgz",
+ "integrity": "sha512-NzUnlZexiaH/46WDhANlyR2bXRopNg4F/zuSA3OpZnllCUgRaOF2znDioDWrmbNVsuZk6l9pMquQB38cfBZwkQ=="
},
- "node_modules/@react-native-community/cli-doctor/node_modules/ora/node_modules/strip-ansi": {
+ "node_modules/@octokit/core/node_modules/universal-user-agent": {
"version": "6.0.1",
- "resolved": "https://registry.npmjs.org/strip-ansi/-/strip-ansi-6.0.1.tgz",
- "integrity": "sha512-Y38VPSHcqkFrCpFnQ9vuSXmquuv5oXOKpGeT6aGrr3o3Gc9AlVa6JBfUSOCnbxGGZF+/0ooI7KrPuUSztUdU5A==",
- "dependencies": {
- "ansi-regex": "^5.0.1"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@react-native-community/cli-doctor/node_modules/path-key": {
- "version": "3.1.1",
- "resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
- "integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@react-native-community/cli-doctor/node_modules/restore-cursor": {
- "version": "3.1.0",
- "resolved": "https://registry.npmjs.org/restore-cursor/-/restore-cursor-3.1.0.tgz",
- "integrity": "sha512-l+sSefzHpj5qimhFSE5a8nufZYAM3sBSVMAPtYkmC+4EH2anSGaEMXSD0izRQbu9nfyQ9y5JrVmp7E8oZrUjvA==",
- "dependencies": {
- "onetime": "^5.1.0",
- "signal-exit": "^3.0.2"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@react-native-community/cli-doctor/node_modules/shebang-command": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/shebang-command/-/shebang-command-2.0.0.tgz",
- "integrity": "sha512-kHxr2zZpYtdmrN1qDjrrX/Z1rR1kG8Dx+gkpK1G4eXmvXswmcE1hTWBWYUzlraYw1/yZp6YuDY77YtvbN0dmDA==",
- "dependencies": {
- "shebang-regex": "^3.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@react-native-community/cli-doctor/node_modules/shebang-regex": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/shebang-regex/-/shebang-regex-3.0.0.tgz",
- "integrity": "sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==",
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@react-native-community/cli-doctor/node_modules/strip-ansi": {
- "version": "5.2.0",
- "resolved": "https://registry.npmjs.org/strip-ansi/-/strip-ansi-5.2.0.tgz",
- "integrity": "sha512-DuRs1gKbBqsMKIZlrffwlug8MHkcnpjs5VPmL1PAh+mA30U0DTotfDZ0d2UUsXpPmPmMMJ6W773MaA3J+lbiWA==",
- "dependencies": {
- "ansi-regex": "^4.1.0"
- },
- "engines": {
- "node": ">=6"
- }
- },
- "node_modules/@react-native-community/cli-doctor/node_modules/strip-ansi/node_modules/ansi-regex": {
- "version": "4.1.1",
- "resolved": "https://registry.npmjs.org/ansi-regex/-/ansi-regex-4.1.1.tgz",
- "integrity": "sha512-ILlv4k/3f6vfQ4OoP2AGvirOktlQ98ZEL1k9FaQjxa3L1abBgbuTDAdPOpvbGncC0BTVQrl+OM8xZGK6tWXt7g==",
- "engines": {
- "node": ">=6"
- }
- },
- "node_modules/@react-native-community/cli-doctor/node_modules/supports-color": {
- "version": "7.2.0",
- "resolved": "https://registry.npmjs.org/supports-color/-/supports-color-7.2.0.tgz",
- "integrity": "sha512-qpCAvRl9stuOHveKsn7HncJRvv501qIacKzQlO/+Lwxc9+0q2wLyv4Dfvt80/DPn2pqOBsJdDiogXGR9+OvwRw==",
- "dependencies": {
- "has-flag": "^4.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@react-native-community/cli-doctor/node_modules/which": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
- "integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
- "dependencies": {
- "isexe": "^2.0.0"
- },
- "bin": {
- "node-which": "bin/node-which"
- },
- "engines": {
- "node": ">= 8"
- }
- },
- "node_modules/@react-native-community/cli-doctor/node_modules/yaml": {
- "version": "2.6.0",
- "resolved": "https://registry.npmjs.org/yaml/-/yaml-2.6.0.tgz",
- "integrity": "sha512-a6ae//JvKDEra2kdi1qzCyrJW/WZCgFi8ydDV+eXExl95t+5R+ijnqHJbz9tmMh8FUjx3iv2fCQ4dclAQlO2UQ==",
- "license": "ISC",
- "bin": {
- "yaml": "bin.mjs"
- },
- "engines": {
- "node": ">= 14"
- }
- },
- "node_modules/@react-native-community/cli-hermes": {
- "version": "12.3.2",
- "resolved": "https://registry.npmjs.org/@react-native-community/cli-hermes/-/cli-hermes-12.3.2.tgz",
- "integrity": "sha512-SL6F9O8ghp4ESBFH2YAPLtIN39jdnvGBKnK4FGKpDCjtB3DnUmDsGFlH46S+GGt5M6VzfG2eeKEOKf3pZ6jUzA==",
- "dependencies": {
- "@react-native-community/cli-platform-android": "12.3.2",
- "@react-native-community/cli-tools": "12.3.2",
- "chalk": "^4.1.2",
- "hermes-profile-transformer": "^0.0.6",
- "ip": "^1.1.5"
- }
- },
- "node_modules/@react-native-community/cli-hermes/node_modules/chalk": {
- "version": "4.1.2",
- "resolved": "https://registry.npmjs.org/chalk/-/chalk-4.1.2.tgz",
- "integrity": "sha512-oKnbhFyRIXpUuez8iBMmyEa4nbj4IOQyuhc/wy9kY7/WVPcwIO9VA668Pu8RkO7+0G76SLROeyw9CpQ061i4mA==",
- "dependencies": {
- "ansi-styles": "^4.1.0",
- "supports-color": "^7.1.0"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/chalk/chalk?sponsor=1"
- }
- },
- "node_modules/@react-native-community/cli-hermes/node_modules/has-flag": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/has-flag/-/has-flag-4.0.0.tgz",
- "integrity": "sha512-EykJT/Q1KjTWctppgIAgfSO0tKVuZUjhgMr17kqTumMl6Afv3EISleU7qZUzoXDFTAHTDC4NOoG/ZxU3EvlMPQ==",
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@react-native-community/cli-hermes/node_modules/supports-color": {
- "version": "7.2.0",
- "resolved": "https://registry.npmjs.org/supports-color/-/supports-color-7.2.0.tgz",
- "integrity": "sha512-qpCAvRl9stuOHveKsn7HncJRvv501qIacKzQlO/+Lwxc9+0q2wLyv4Dfvt80/DPn2pqOBsJdDiogXGR9+OvwRw==",
- "dependencies": {
- "has-flag": "^4.0.0"
- },
- "engines": {
- "node": ">=8"
- }
+ "resolved": "https://registry.npmjs.org/universal-user-agent/-/universal-user-agent-6.0.1.tgz",
+ "integrity": "sha512-yCzhz6FN2wU1NiiQRogkTQszlQSlpWaw8SvVegAc+bDxbzHgh1vX8uIe8OYyMH6DwH+sdTJsgMl36+mSMdRJIQ=="
},
- "node_modules/@react-native-community/cli-platform-android": {
- "version": "12.3.2",
- "resolved": "https://registry.npmjs.org/@react-native-community/cli-platform-android/-/cli-platform-android-12.3.2.tgz",
- "integrity": "sha512-MZ5nO8yi/N+Fj2i9BJcJ9C/ez+9/Ir7lQt49DWRo9YDmzye66mYLr/P2l/qxsixllbbDi7BXrlLpxaEhMrDopg==",
+ "node_modules/@octokit/endpoint": {
+ "version": "5.5.3",
+ "resolved": "https://registry.npmjs.org/@octokit/endpoint/-/endpoint-5.5.3.tgz",
+ "integrity": "sha512-EzKwkwcxeegYYah5ukEeAI/gYRLv2Y9U5PpIsseGSFDk+G3RbipQGBs8GuYS1TLCtQaqoO66+aQGtITPalxsNQ==",
+ "dev": true,
"dependencies": {
- "@react-native-community/cli-tools": "12.3.2",
- "chalk": "^4.1.2",
- "execa": "^5.0.0",
- "fast-xml-parser": "^4.2.4",
- "glob": "^7.1.3",
- "logkitty": "^0.7.1"
+ "@octokit/types": "^2.0.0",
+ "is-plain-object": "^3.0.0",
+ "universal-user-agent": "^5.0.0"
}
},
- "node_modules/@react-native-community/cli-platform-android/node_modules/chalk": {
- "version": "4.1.2",
- "resolved": "https://registry.npmjs.org/chalk/-/chalk-4.1.2.tgz",
- "integrity": "sha512-oKnbhFyRIXpUuez8iBMmyEa4nbj4IOQyuhc/wy9kY7/WVPcwIO9VA668Pu8RkO7+0G76SLROeyw9CpQ061i4mA==",
+ "node_modules/@octokit/endpoint/node_modules/@octokit/types": {
+ "version": "2.16.2",
+ "resolved": "https://registry.npmjs.org/@octokit/types/-/types-2.16.2.tgz",
+ "integrity": "sha512-O75k56TYvJ8WpAakWwYRN8Bgu60KrmX0z1KqFp1kNiFNkgW+JW+9EBKZ+S33PU6SLvbihqd+3drvPxKK68Ee8Q==",
+ "dev": true,
"dependencies": {
- "ansi-styles": "^4.1.0",
- "supports-color": "^7.1.0"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/chalk/chalk?sponsor=1"
+ "@types/node": ">= 8"
}
},
- "node_modules/@react-native-community/cli-platform-android/node_modules/cross-spawn": {
- "version": "7.0.3",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-7.0.3.tgz",
- "integrity": "sha512-iRDPJKUPVEND7dHPO8rkbOnPpyDygcDFtWjpeWNCgy8WP2rXcxXL8TskReQl6OrB2G7+UJrags1q15Fudc7G6w==",
- "dependencies": {
- "path-key": "^3.1.0",
- "shebang-command": "^2.0.0",
- "which": "^2.0.1"
- },
+ "node_modules/@octokit/endpoint/node_modules/is-plain-object": {
+ "version": "3.0.1",
+ "resolved": "https://registry.npmjs.org/is-plain-object/-/is-plain-object-3.0.1.tgz",
+ "integrity": "sha512-Xnpx182SBMrr/aBik8y+GuR4U1L9FqMSojwDQwPMmxyC6bvEqly9UBCxhauBF5vNh2gwWJNX6oDV7O+OM4z34g==",
+ "dev": true,
"engines": {
- "node": ">= 8"
+ "node": ">=0.10.0"
}
},
- "node_modules/@react-native-community/cli-platform-android/node_modules/execa": {
- "version": "5.1.1",
- "resolved": "https://registry.npmjs.org/execa/-/execa-5.1.1.tgz",
- "integrity": "sha512-8uSpZZocAZRBAPIEINJj3Lo9HyGitllczc27Eh5YYojjMFMn8yHMDMaUHE2Jqfq05D/wucwI4JGURyXt1vchyg==",
+ "node_modules/@octokit/endpoint/node_modules/universal-user-agent": {
+ "version": "5.0.0",
+ "resolved": "https://registry.npmjs.org/universal-user-agent/-/universal-user-agent-5.0.0.tgz",
+ "integrity": "sha512-B5TPtzZleXyPrUMKCpEHFmVhMN6EhmJYjG5PQna9s7mXeSqGTLap4OpqLl5FCEFUI3UBmllkETwKf/db66Y54Q==",
+ "dev": true,
"dependencies": {
- "cross-spawn": "^7.0.3",
- "get-stream": "^6.0.0",
- "human-signals": "^2.1.0",
- "is-stream": "^2.0.0",
- "merge-stream": "^2.0.0",
- "npm-run-path": "^4.0.1",
- "onetime": "^5.1.2",
- "signal-exit": "^3.0.3",
- "strip-final-newline": "^2.0.0"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/sindresorhus/execa?sponsor=1"
+ "os-name": "^3.1.0"
}
},
- "node_modules/@react-native-community/cli-platform-android/node_modules/get-stream": {
- "version": "6.0.1",
- "resolved": "https://registry.npmjs.org/get-stream/-/get-stream-6.0.1.tgz",
- "integrity": "sha512-ts6Wi+2j3jQjqi70w5AlN8DFnkSwC+MqmxEzdEALB2qXZYV3X/b1CTfgPLGJNMeAWxdPfU8FO1ms3NUfaHCPYg==",
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "node_modules/@octokit/graphql": {
+ "version": "4.8.0",
+ "resolved": "https://registry.npmjs.org/@octokit/graphql/-/graphql-4.8.0.tgz",
+ "integrity": "sha512-0gv+qLSBLKF0z8TKaSKTsS39scVKF9dbMxJpj3U0vC7wjNWFuIpL/z76Qe2fiuCbDRcJSavkXsVtMS6/dtQQsg==",
+ "dependencies": {
+ "@octokit/request": "^5.6.0",
+ "@octokit/types": "^6.0.3",
+ "universal-user-agent": "^6.0.0"
}
},
- "node_modules/@react-native-community/cli-platform-android/node_modules/glob": {
- "version": "7.2.3",
- "resolved": "https://registry.npmjs.org/glob/-/glob-7.2.3.tgz",
- "integrity": "sha512-nFR0zLpU2YCaRxwoCJvL6UvCH2JFyFVIvwTLsIf21AuHlMskA1hhTdk+LlYJtOlYt9v6dvszD2BGRqBL+iQK9Q==",
+ "node_modules/@octokit/graphql/node_modules/@octokit/endpoint": {
+ "version": "6.0.12",
+ "resolved": "https://registry.npmjs.org/@octokit/endpoint/-/endpoint-6.0.12.tgz",
+ "integrity": "sha512-lF3puPwkQWGfkMClXb4k/eUT/nZKQfxinRWJrdZaJO85Dqwo/G0yOC434Jr2ojwafWJMYqFGFa5ms4jJUgujdA==",
"dependencies": {
- "fs.realpath": "^1.0.0",
- "inflight": "^1.0.4",
- "inherits": "2",
- "minimatch": "^3.1.1",
- "once": "^1.3.0",
- "path-is-absolute": "^1.0.0"
- },
- "engines": {
- "node": "*"
- },
- "funding": {
- "url": "https://github.com/sponsors/isaacs"
+ "@octokit/types": "^6.0.3",
+ "is-plain-object": "^5.0.0",
+ "universal-user-agent": "^6.0.0"
}
},
- "node_modules/@react-native-community/cli-platform-android/node_modules/has-flag": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/has-flag/-/has-flag-4.0.0.tgz",
- "integrity": "sha512-EykJT/Q1KjTWctppgIAgfSO0tKVuZUjhgMr17kqTumMl6Afv3EISleU7qZUzoXDFTAHTDC4NOoG/ZxU3EvlMPQ==",
- "engines": {
- "node": ">=8"
+ "node_modules/@octokit/graphql/node_modules/@octokit/request": {
+ "version": "5.6.3",
+ "resolved": "https://registry.npmjs.org/@octokit/request/-/request-5.6.3.tgz",
+ "integrity": "sha512-bFJl0I1KVc9jYTe9tdGGpAMPy32dLBXXo1dS/YwSCTL/2nd9XeHsY616RE3HPXDVk+a+dBuzyz5YdlXwcDTr2A==",
+ "dependencies": {
+ "@octokit/endpoint": "^6.0.1",
+ "@octokit/request-error": "^2.1.0",
+ "@octokit/types": "^6.16.1",
+ "is-plain-object": "^5.0.0",
+ "node-fetch": "^2.6.7",
+ "universal-user-agent": "^6.0.0"
}
},
- "node_modules/@react-native-community/cli-platform-android/node_modules/human-signals": {
- "version": "2.1.0",
- "resolved": "https://registry.npmjs.org/human-signals/-/human-signals-2.1.0.tgz",
- "integrity": "sha512-B4FFZ6q/T2jhhksgkbEW3HBvWIfDW85snkQgawt07S7J5QXTk6BkNV+0yAeZrM5QpMAdYlocGoljn0sJ/WQkFw==",
- "engines": {
- "node": ">=10.17.0"
- }
+ "node_modules/@octokit/graphql/node_modules/universal-user-agent": {
+ "version": "6.0.1",
+ "resolved": "https://registry.npmjs.org/universal-user-agent/-/universal-user-agent-6.0.1.tgz",
+ "integrity": "sha512-yCzhz6FN2wU1NiiQRogkTQszlQSlpWaw8SvVegAc+bDxbzHgh1vX8uIe8OYyMH6DwH+sdTJsgMl36+mSMdRJIQ=="
},
- "node_modules/@react-native-community/cli-platform-android/node_modules/is-stream": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/is-stream/-/is-stream-2.0.1.tgz",
- "integrity": "sha512-hFoiJiTl63nn+kstHGBtewWSKnQLpyb155KHheA1l39uvtO9nWIop1p3udqPcUd/xbF1VLMO4n7OI6p7RbngDg==",
- "engines": {
- "node": ">=8"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
+ "node_modules/@octokit/openapi-types": {
+ "version": "11.2.0",
+ "resolved": "https://registry.npmjs.org/@octokit/openapi-types/-/openapi-types-11.2.0.tgz",
+ "integrity": "sha512-PBsVO+15KSlGmiI8QAzaqvsNlZlrDlyAJYcrXBCvVUxCp7VnXjkwPoFHgjEJXx3WF9BAwkA6nfCUA7i9sODzKA=="
},
- "node_modules/@react-native-community/cli-platform-android/node_modules/mimic-fn": {
- "version": "2.1.0",
- "resolved": "https://registry.npmjs.org/mimic-fn/-/mimic-fn-2.1.0.tgz",
- "integrity": "sha512-OqbOk5oEQeAZ8WXWydlu9HJjz9WVdEIvamMCcXmuqUYjTknH/sqsWvhQ3vgwKFRR1HpjvNBKQ37nbJgYzGqGcg==",
- "engines": {
- "node": ">=6"
- }
+ "node_modules/@octokit/plugin-enterprise-rest": {
+ "version": "6.0.1",
+ "resolved": "https://registry.npmjs.org/@octokit/plugin-enterprise-rest/-/plugin-enterprise-rest-6.0.1.tgz",
+ "integrity": "sha512-93uGjlhUD+iNg1iWhUENAtJata6w5nE+V4urXOAlIXdco6xNZtUSfYY8dzp3Udy74aqO/B5UZL80x/YMa5PKRw==",
+ "dev": true,
+ "license": "MIT"
},
- "node_modules/@react-native-community/cli-platform-android/node_modules/npm-run-path": {
- "version": "4.0.1",
- "resolved": "https://registry.npmjs.org/npm-run-path/-/npm-run-path-4.0.1.tgz",
- "integrity": "sha512-S48WzZW777zhNIrn7gxOlISNAqi9ZC/uQFnRdbeIHhZhCA6UqpkOT8T1G7BvfdgP4Er8gF4sUbaS0i7QvIfCWw==",
+ "node_modules/@octokit/plugin-paginate-rest": {
+ "version": "2.21.3",
+ "resolved": "https://registry.npmjs.org/@octokit/plugin-paginate-rest/-/plugin-paginate-rest-2.21.3.tgz",
+ "integrity": "sha512-aCZTEf0y2h3OLbrgKkrfFdjRL6eSOo8komneVQJnYecAxIej7Bafor2xhuDJOIFau4pk0i/P28/XgtbyPF0ZHw==",
"dependencies": {
- "path-key": "^3.0.0"
+ "@octokit/types": "^6.40.0"
},
- "engines": {
- "node": ">=8"
+ "peerDependencies": {
+ "@octokit/core": ">=2"
}
},
- "node_modules/@react-native-community/cli-platform-android/node_modules/onetime": {
- "version": "5.1.2",
- "resolved": "https://registry.npmjs.org/onetime/-/onetime-5.1.2.tgz",
- "integrity": "sha512-kbpaSSGJTWdAY5KPVeMOKXSrPtr8C8C7wodJbcsd51jRnmD+GZu8Y0VoU6Dm5Z4vWr0Ig/1NKuWRKf7j5aaYSg==",
+ "node_modules/@octokit/plugin-paginate-rest/node_modules/@octokit/openapi-types": {
+ "version": "12.11.0",
+ "resolved": "https://registry.npmjs.org/@octokit/openapi-types/-/openapi-types-12.11.0.tgz",
+ "integrity": "sha512-VsXyi8peyRq9PqIz/tpqiL2w3w80OgVMwBHltTml3LmVvXiphgeqmY9mvBw9Wu7e0QWk/fqD37ux8yP5uVekyQ=="
+ },
+ "node_modules/@octokit/plugin-paginate-rest/node_modules/@octokit/types": {
+ "version": "6.41.0",
+ "resolved": "https://registry.npmjs.org/@octokit/types/-/types-6.41.0.tgz",
+ "integrity": "sha512-eJ2jbzjdijiL3B4PrSQaSjuF2sPEQPVCPzBvTHJD9Nz+9dw2SGH4K4xeQJ77YfTq5bRQ+bD8wT11JbeDPmxmGg==",
"dependencies": {
- "mimic-fn": "^2.1.0"
- },
- "engines": {
- "node": ">=6"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "@octokit/openapi-types": "^12.11.0"
}
},
- "node_modules/@react-native-community/cli-platform-android/node_modules/path-key": {
- "version": "3.1.1",
- "resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
- "integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
- "engines": {
- "node": ">=8"
+ "node_modules/@octokit/plugin-request-log": {
+ "version": "1.0.4",
+ "resolved": "https://registry.npmjs.org/@octokit/plugin-request-log/-/plugin-request-log-1.0.4.tgz",
+ "integrity": "sha512-mLUsMkgP7K/cnFEw07kWqXGF5LKrOkD+lhCrKvPHXWDywAwuDUeDwWBpc69XK3pNX0uKiVt8g5z96PJ6z9xCFA==",
+ "dev": true,
+ "peerDependencies": {
+ "@octokit/core": ">=3"
}
},
- "node_modules/@react-native-community/cli-platform-android/node_modules/shebang-command": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/shebang-command/-/shebang-command-2.0.0.tgz",
- "integrity": "sha512-kHxr2zZpYtdmrN1qDjrrX/Z1rR1kG8Dx+gkpK1G4eXmvXswmcE1hTWBWYUzlraYw1/yZp6YuDY77YtvbN0dmDA==",
+ "node_modules/@octokit/plugin-rest-endpoint-methods": {
+ "version": "5.16.2",
+ "resolved": "https://registry.npmjs.org/@octokit/plugin-rest-endpoint-methods/-/plugin-rest-endpoint-methods-5.16.2.tgz",
+ "integrity": "sha512-8QFz29Fg5jDuTPXVtey05BLm7OB+M8fnvE64RNegzX7U+5NUXcOcnpTIK0YfSHBg8gYd0oxIq3IZTe9SfPZiRw==",
"dependencies": {
- "shebang-regex": "^3.0.0"
+ "@octokit/types": "^6.39.0",
+ "deprecation": "^2.3.1"
},
- "engines": {
- "node": ">=8"
+ "peerDependencies": {
+ "@octokit/core": ">=3"
}
},
- "node_modules/@react-native-community/cli-platform-android/node_modules/shebang-regex": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/shebang-regex/-/shebang-regex-3.0.0.tgz",
- "integrity": "sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==",
- "engines": {
- "node": ">=8"
- }
+ "node_modules/@octokit/plugin-rest-endpoint-methods/node_modules/@octokit/openapi-types": {
+ "version": "12.11.0",
+ "resolved": "https://registry.npmjs.org/@octokit/openapi-types/-/openapi-types-12.11.0.tgz",
+ "integrity": "sha512-VsXyi8peyRq9PqIz/tpqiL2w3w80OgVMwBHltTml3LmVvXiphgeqmY9mvBw9Wu7e0QWk/fqD37ux8yP5uVekyQ=="
},
- "node_modules/@react-native-community/cli-platform-android/node_modules/supports-color": {
- "version": "7.2.0",
- "resolved": "https://registry.npmjs.org/supports-color/-/supports-color-7.2.0.tgz",
- "integrity": "sha512-qpCAvRl9stuOHveKsn7HncJRvv501qIacKzQlO/+Lwxc9+0q2wLyv4Dfvt80/DPn2pqOBsJdDiogXGR9+OvwRw==",
+ "node_modules/@octokit/plugin-rest-endpoint-methods/node_modules/@octokit/types": {
+ "version": "6.41.0",
+ "resolved": "https://registry.npmjs.org/@octokit/types/-/types-6.41.0.tgz",
+ "integrity": "sha512-eJ2jbzjdijiL3B4PrSQaSjuF2sPEQPVCPzBvTHJD9Nz+9dw2SGH4K4xeQJ77YfTq5bRQ+bD8wT11JbeDPmxmGg==",
"dependencies": {
- "has-flag": "^4.0.0"
- },
- "engines": {
- "node": ">=8"
+ "@octokit/openapi-types": "^12.11.0"
}
},
- "node_modules/@react-native-community/cli-platform-android/node_modules/which": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
- "integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
+ "node_modules/@octokit/request": {
+ "version": "4.1.1",
+ "resolved": "https://registry.npmjs.org/@octokit/request/-/request-4.1.1.tgz",
+ "integrity": "sha512-LOyL0i3oxRo418EXRSJNk/3Q4I0/NKawTn6H/CQp+wnrG1UFLGu080gSsgnWobhPo5BpUNgSQ5BRk5FOOJhD1Q==",
+ "dev": true,
"dependencies": {
- "isexe": "^2.0.0"
- },
- "bin": {
- "node-which": "bin/node-which"
- },
- "engines": {
- "node": ">= 8"
+ "@octokit/endpoint": "^5.1.0",
+ "@octokit/request-error": "^1.0.1",
+ "deprecation": "^2.0.0",
+ "is-plain-object": "^3.0.0",
+ "node-fetch": "^2.3.0",
+ "once": "^1.4.0",
+ "universal-user-agent": "^2.1.0"
}
},
- "node_modules/@react-native-community/cli-platform-ios": {
- "version": "12.3.2",
- "resolved": "https://registry.npmjs.org/@react-native-community/cli-platform-ios/-/cli-platform-ios-12.3.2.tgz",
- "integrity": "sha512-OcWEAbkev1IL6SUiQnM6DQdsvfsKZhRZtoBNSj9MfdmwotVZSOEZJ+IjZ1FR9ChvMWayO9ns/o8LgoQxr1ZXeg==",
+ "node_modules/@octokit/request-error": {
+ "version": "2.1.0",
+ "resolved": "https://registry.npmjs.org/@octokit/request-error/-/request-error-2.1.0.tgz",
+ "integrity": "sha512-1VIvgXxs9WHSjicsRwq8PlR2LR2x6DwsJAaFgzdi0JfJoGSO8mYI/cHJQ+9FbN21aa+DrgNLnwObmyeSC8Rmpg==",
"dependencies": {
- "@react-native-community/cli-tools": "12.3.2",
- "chalk": "^4.1.2",
- "execa": "^5.0.0",
- "fast-xml-parser": "^4.0.12",
- "glob": "^7.1.3",
- "ora": "^5.4.1"
+ "@octokit/types": "^6.0.3",
+ "deprecation": "^2.0.0",
+ "once": "^1.4.0"
}
},
- "node_modules/@react-native-community/cli-platform-ios/node_modules/chalk": {
- "version": "4.1.2",
- "resolved": "https://registry.npmjs.org/chalk/-/chalk-4.1.2.tgz",
- "integrity": "sha512-oKnbhFyRIXpUuez8iBMmyEa4nbj4IOQyuhc/wy9kY7/WVPcwIO9VA668Pu8RkO7+0G76SLROeyw9CpQ061i4mA==",
+ "node_modules/@octokit/request/node_modules/@octokit/request-error": {
+ "version": "1.2.1",
+ "resolved": "https://registry.npmjs.org/@octokit/request-error/-/request-error-1.2.1.tgz",
+ "integrity": "sha512-+6yDyk1EES6WK+l3viRDElw96MvwfJxCt45GvmjDUKWjYIb3PJZQkq3i46TwGwoPD4h8NmTrENmtyA1FwbmhRA==",
+ "dev": true,
"dependencies": {
- "ansi-styles": "^4.1.0",
- "supports-color": "^7.1.0"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/chalk/chalk?sponsor=1"
+ "@octokit/types": "^2.0.0",
+ "deprecation": "^2.0.0",
+ "once": "^1.4.0"
}
},
- "node_modules/@react-native-community/cli-platform-ios/node_modules/cli-cursor": {
- "version": "3.1.0",
- "resolved": "https://registry.npmjs.org/cli-cursor/-/cli-cursor-3.1.0.tgz",
- "integrity": "sha512-I/zHAwsKf9FqGoXM4WWRACob9+SNukZTd94DWF57E4toouRulbCxcUh6RKUEOQlYTHJnzkPMySvPNaaSLNfLZw==",
+ "node_modules/@octokit/request/node_modules/@octokit/types": {
+ "version": "2.16.2",
+ "resolved": "https://registry.npmjs.org/@octokit/types/-/types-2.16.2.tgz",
+ "integrity": "sha512-O75k56TYvJ8WpAakWwYRN8Bgu60KrmX0z1KqFp1kNiFNkgW+JW+9EBKZ+S33PU6SLvbihqd+3drvPxKK68Ee8Q==",
+ "dev": true,
"dependencies": {
- "restore-cursor": "^3.1.0"
- },
- "engines": {
- "node": ">=8"
+ "@types/node": ">= 8"
}
},
- "node_modules/@react-native-community/cli-platform-ios/node_modules/cross-spawn": {
- "version": "7.0.3",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-7.0.3.tgz",
- "integrity": "sha512-iRDPJKUPVEND7dHPO8rkbOnPpyDygcDFtWjpeWNCgy8WP2rXcxXL8TskReQl6OrB2G7+UJrags1q15Fudc7G6w==",
- "dependencies": {
- "path-key": "^3.1.0",
- "shebang-command": "^2.0.0",
- "which": "^2.0.1"
- },
+ "node_modules/@octokit/request/node_modules/is-plain-object": {
+ "version": "3.0.1",
+ "resolved": "https://registry.npmjs.org/is-plain-object/-/is-plain-object-3.0.1.tgz",
+ "integrity": "sha512-Xnpx182SBMrr/aBik8y+GuR4U1L9FqMSojwDQwPMmxyC6bvEqly9UBCxhauBF5vNh2gwWJNX6oDV7O+OM4z34g==",
+ "dev": true,
"engines": {
- "node": ">= 8"
+ "node": ">=0.10.0"
}
},
- "node_modules/@react-native-community/cli-platform-ios/node_modules/execa": {
- "version": "5.1.1",
- "resolved": "https://registry.npmjs.org/execa/-/execa-5.1.1.tgz",
- "integrity": "sha512-8uSpZZocAZRBAPIEINJj3Lo9HyGitllczc27Eh5YYojjMFMn8yHMDMaUHE2Jqfq05D/wucwI4JGURyXt1vchyg==",
+ "node_modules/@octokit/rest": {
+ "version": "16.26.0",
+ "resolved": "https://registry.npmjs.org/@octokit/rest/-/rest-16.26.0.tgz",
+ "integrity": "sha512-NBpzre44ZAQWZhlH+zUYTgqI0pHN+c9rNj4d+pCydGEiKTGc1HKmoTghEUyr9GxazDyoAvmpx9nL0I7QS1Olvg==",
+ "dev": true,
"dependencies": {
- "cross-spawn": "^7.0.3",
- "get-stream": "^6.0.0",
- "human-signals": "^2.1.0",
- "is-stream": "^2.0.0",
- "merge-stream": "^2.0.0",
- "npm-run-path": "^4.0.1",
- "onetime": "^5.1.2",
- "signal-exit": "^3.0.3",
- "strip-final-newline": "^2.0.0"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/sindresorhus/execa?sponsor=1"
+ "@octokit/request": "^4.0.1",
+ "@octokit/request-error": "^1.0.2",
+ "atob-lite": "^2.0.0",
+ "before-after-hook": "^1.4.0",
+ "btoa-lite": "^1.0.0",
+ "deprecation": "^2.0.0",
+ "lodash.get": "^4.4.2",
+ "lodash.set": "^4.3.2",
+ "lodash.uniq": "^4.5.0",
+ "octokit-pagination-methods": "^1.1.0",
+ "once": "^1.4.0",
+ "universal-user-agent": "^2.0.0",
+ "url-template": "^2.0.8"
}
},
- "node_modules/@react-native-community/cli-platform-ios/node_modules/get-stream": {
- "version": "6.0.1",
- "resolved": "https://registry.npmjs.org/get-stream/-/get-stream-6.0.1.tgz",
- "integrity": "sha512-ts6Wi+2j3jQjqi70w5AlN8DFnkSwC+MqmxEzdEALB2qXZYV3X/b1CTfgPLGJNMeAWxdPfU8FO1ms3NUfaHCPYg==",
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "node_modules/@octokit/rest/node_modules/@octokit/request-error": {
+ "version": "1.2.1",
+ "resolved": "https://registry.npmjs.org/@octokit/request-error/-/request-error-1.2.1.tgz",
+ "integrity": "sha512-+6yDyk1EES6WK+l3viRDElw96MvwfJxCt45GvmjDUKWjYIb3PJZQkq3i46TwGwoPD4h8NmTrENmtyA1FwbmhRA==",
+ "dev": true,
+ "dependencies": {
+ "@octokit/types": "^2.0.0",
+ "deprecation": "^2.0.0",
+ "once": "^1.4.0"
}
},
- "node_modules/@react-native-community/cli-platform-ios/node_modules/glob": {
- "version": "7.2.3",
- "resolved": "https://registry.npmjs.org/glob/-/glob-7.2.3.tgz",
- "integrity": "sha512-nFR0zLpU2YCaRxwoCJvL6UvCH2JFyFVIvwTLsIf21AuHlMskA1hhTdk+LlYJtOlYt9v6dvszD2BGRqBL+iQK9Q==",
+ "node_modules/@octokit/rest/node_modules/@octokit/types": {
+ "version": "2.16.2",
+ "resolved": "https://registry.npmjs.org/@octokit/types/-/types-2.16.2.tgz",
+ "integrity": "sha512-O75k56TYvJ8WpAakWwYRN8Bgu60KrmX0z1KqFp1kNiFNkgW+JW+9EBKZ+S33PU6SLvbihqd+3drvPxKK68Ee8Q==",
+ "dev": true,
"dependencies": {
- "fs.realpath": "^1.0.0",
- "inflight": "^1.0.4",
- "inherits": "2",
- "minimatch": "^3.1.1",
- "once": "^1.3.0",
- "path-is-absolute": "^1.0.0"
- },
- "engines": {
- "node": "*"
- },
- "funding": {
- "url": "https://github.com/sponsors/isaacs"
+ "@types/node": ">= 8"
}
},
- "node_modules/@react-native-community/cli-platform-ios/node_modules/has-flag": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/has-flag/-/has-flag-4.0.0.tgz",
- "integrity": "sha512-EykJT/Q1KjTWctppgIAgfSO0tKVuZUjhgMr17kqTumMl6Afv3EISleU7qZUzoXDFTAHTDC4NOoG/ZxU3EvlMPQ==",
- "engines": {
- "node": ">=8"
- }
+ "node_modules/@octokit/tsconfig": {
+ "version": "1.0.2",
+ "resolved": "https://registry.npmjs.org/@octokit/tsconfig/-/tsconfig-1.0.2.tgz",
+ "integrity": "sha512-I0vDR0rdtP8p2lGMzvsJzbhdOWy405HcGovrspJ8RRibHnyRgggUSNO5AIox5LmqiwmatHKYsvj6VGFHkqS7lA==",
+ "dev": true
},
- "node_modules/@react-native-community/cli-platform-ios/node_modules/human-signals": {
- "version": "2.1.0",
- "resolved": "https://registry.npmjs.org/human-signals/-/human-signals-2.1.0.tgz",
- "integrity": "sha512-B4FFZ6q/T2jhhksgkbEW3HBvWIfDW85snkQgawt07S7J5QXTk6BkNV+0yAeZrM5QpMAdYlocGoljn0sJ/WQkFw==",
- "engines": {
- "node": ">=10.17.0"
+ "node_modules/@octokit/types": {
+ "version": "6.34.0",
+ "resolved": "https://registry.npmjs.org/@octokit/types/-/types-6.34.0.tgz",
+ "integrity": "sha512-s1zLBjWhdEI2zwaoSgyOFoKSl109CUcVBCc7biPJ3aAf6LGLU6szDvi31JPU7bxfla2lqfhjbbg/5DdFNxOwHw==",
+ "dependencies": {
+ "@octokit/openapi-types": "^11.2.0"
}
},
- "node_modules/@react-native-community/cli-platform-ios/node_modules/is-stream": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/is-stream/-/is-stream-2.0.1.tgz",
- "integrity": "sha512-hFoiJiTl63nn+kstHGBtewWSKnQLpyb155KHheA1l39uvtO9nWIop1p3udqPcUd/xbF1VLMO4n7OI6p7RbngDg==",
- "engines": {
- "node": ">=8"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
+ "node_modules/@octokit/webhooks-methods": {
+ "version": "2.0.0",
+ "resolved": "https://registry.npmjs.org/@octokit/webhooks-methods/-/webhooks-methods-2.0.0.tgz",
+ "integrity": "sha512-35cfQ4YWlnZnmZKmIxlGPUPLtbkF8lr/A/1Sk1eC0ddLMwQN06dOuLc+dI3YLQS+T+MoNt3DIQ0NynwgKPilig==",
+ "license": "MIT"
},
- "node_modules/@react-native-community/cli-platform-ios/node_modules/mimic-fn": {
- "version": "2.1.0",
- "resolved": "https://registry.npmjs.org/mimic-fn/-/mimic-fn-2.1.0.tgz",
- "integrity": "sha512-OqbOk5oEQeAZ8WXWydlu9HJjz9WVdEIvamMCcXmuqUYjTknH/sqsWvhQ3vgwKFRR1HpjvNBKQ37nbJgYzGqGcg==",
- "engines": {
- "node": ">=6"
- }
+ "node_modules/@octokit/webhooks-types": {
+ "version": "5.8.0",
+ "resolved": "https://registry.npmjs.org/@octokit/webhooks-types/-/webhooks-types-5.8.0.tgz",
+ "integrity": "sha512-8adktjIb76A7viIdayQSFuBEwOzwhDC+9yxZpKNHjfzrlostHCw0/N7JWpWMObfElwvJMk2fY2l1noENCk9wmw==",
+ "license": "MIT"
},
- "node_modules/@react-native-community/cli-platform-ios/node_modules/npm-run-path": {
- "version": "4.0.1",
- "resolved": "https://registry.npmjs.org/npm-run-path/-/npm-run-path-4.0.1.tgz",
- "integrity": "sha512-S48WzZW777zhNIrn7gxOlISNAqi9ZC/uQFnRdbeIHhZhCA6UqpkOT8T1G7BvfdgP4Er8gF4sUbaS0i7QvIfCWw==",
- "dependencies": {
- "path-key": "^3.0.0"
- },
- "engines": {
- "node": ">=8"
- }
+ "node_modules/@paulirish/trace_engine": {
+ "version": "0.0.32",
+ "resolved": "https://registry.npmjs.org/@paulirish/trace_engine/-/trace_engine-0.0.32.tgz",
+ "integrity": "sha512-KxWFdRNbv13U8bhYaQvH6gLG9CVEt2jKeosyOOYILVntWEVWhovbgDrbOiZ12pJO3vjZs0Zgbd3/Zgde98woEA==",
+ "license": "BSD-3-Clause"
},
- "node_modules/@react-native-community/cli-platform-ios/node_modules/onetime": {
- "version": "5.1.2",
- "resolved": "https://registry.npmjs.org/onetime/-/onetime-5.1.2.tgz",
- "integrity": "sha512-kbpaSSGJTWdAY5KPVeMOKXSrPtr8C8C7wodJbcsd51jRnmD+GZu8Y0VoU6Dm5Z4vWr0Ig/1NKuWRKf7j5aaYSg==",
- "dependencies": {
- "mimic-fn": "^2.1.0"
- },
+ "node_modules/@pkgjs/parseargs": {
+ "version": "0.11.0",
+ "resolved": "https://registry.npmjs.org/@pkgjs/parseargs/-/parseargs-0.11.0.tgz",
+ "integrity": "sha512-+1VkjdD0QBLPodGrJUeqarH8VAIvQODIbwh9XpP5Syisf7YoQgsJKPNFoqqLQlu+VQ/tVSshMR6loPMn8U+dPg==",
+ "optional": true,
"engines": {
- "node": ">=6"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "node": ">=14"
}
},
- "node_modules/@react-native-community/cli-platform-ios/node_modules/ora": {
- "version": "5.4.1",
- "resolved": "https://registry.npmjs.org/ora/-/ora-5.4.1.tgz",
- "integrity": "sha512-5b6Y85tPxZZ7QytO+BQzysW31HJku27cRIlkbAXaNx+BdcVi+LlRFmVXzeF6a7JCwJpyw5c4b+YSVImQIrBpuQ==",
+ "node_modules/@pkgr/utils": {
+ "version": "2.4.2",
+ "resolved": "https://registry.npmjs.org/@pkgr/utils/-/utils-2.4.2.tgz",
+ "integrity": "sha512-POgTXhjrTfbTV63DiFXav4lBHiICLKKwDeaKn9Nphwj7WH6m0hMMCaJkMyRWjgtPFyRKRVoMXXjczsTQRDEhYw==",
"dependencies": {
- "bl": "^4.1.0",
- "chalk": "^4.1.0",
- "cli-cursor": "^3.1.0",
- "cli-spinners": "^2.5.0",
- "is-interactive": "^1.0.0",
- "is-unicode-supported": "^0.1.0",
- "log-symbols": "^4.1.0",
- "strip-ansi": "^6.0.0",
- "wcwidth": "^1.0.1"
+ "cross-spawn": "^7.0.3",
+ "fast-glob": "^3.3.0",
+ "is-glob": "^4.0.3",
+ "open": "^9.1.0",
+ "picocolors": "^1.0.0",
+ "tslib": "^2.6.0"
},
"engines": {
- "node": ">=10"
+ "node": "^12.20.0 || ^14.18.0 || >=16.0.0"
},
"funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "url": "https://opencollective.com/unts"
}
},
- "node_modules/@react-native-community/cli-platform-ios/node_modules/path-key": {
- "version": "3.1.1",
- "resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
- "integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
+ "node_modules/@pkgr/utils/node_modules/define-lazy-prop": {
+ "version": "3.0.0",
+ "resolved": "https://registry.npmjs.org/define-lazy-prop/-/define-lazy-prop-3.0.0.tgz",
+ "integrity": "sha512-N+MeXYoqr3pOgn8xfyRPREN7gHakLYjhsHhWGT3fWAiL4IkAt0iDw14QiiEm2bE30c5XX5q0FtAA3CK5f9/BUg==",
"engines": {
- "node": ">=8"
+ "node": ">=12"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@react-native-community/cli-platform-ios/node_modules/restore-cursor": {
- "version": "3.1.0",
- "resolved": "https://registry.npmjs.org/restore-cursor/-/restore-cursor-3.1.0.tgz",
- "integrity": "sha512-l+sSefzHpj5qimhFSE5a8nufZYAM3sBSVMAPtYkmC+4EH2anSGaEMXSD0izRQbu9nfyQ9y5JrVmp7E8oZrUjvA==",
+ "node_modules/@pkgr/utils/node_modules/fast-glob": {
+ "version": "3.3.2",
+ "resolved": "https://registry.npmjs.org/fast-glob/-/fast-glob-3.3.2.tgz",
+ "integrity": "sha512-oX2ruAFQwf/Orj8m737Y5adxDQO0LAB7/S5MnxCdTNDd4p6BsyIVsv9JQsATbTSq8KHRpLwIHbVlUNatxd+1Ow==",
"dependencies": {
- "onetime": "^5.1.0",
- "signal-exit": "^3.0.2"
+ "@nodelib/fs.stat": "^2.0.2",
+ "@nodelib/fs.walk": "^1.2.3",
+ "glob-parent": "^5.1.2",
+ "merge2": "^1.3.0",
+ "micromatch": "^4.0.4"
},
"engines": {
- "node": ">=8"
+ "node": ">=8.6.0"
}
},
- "node_modules/@react-native-community/cli-platform-ios/node_modules/shebang-command": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/shebang-command/-/shebang-command-2.0.0.tgz",
- "integrity": "sha512-kHxr2zZpYtdmrN1qDjrrX/Z1rR1kG8Dx+gkpK1G4eXmvXswmcE1hTWBWYUzlraYw1/yZp6YuDY77YtvbN0dmDA==",
+ "node_modules/@pkgr/utils/node_modules/glob-parent": {
+ "version": "5.1.2",
+ "resolved": "https://registry.npmjs.org/glob-parent/-/glob-parent-5.1.2.tgz",
+ "integrity": "sha512-AOIgSQCepiJYwP3ARnGx+5VnTu2HBYdzbGP45eLw1vr3zB3vZLeyed1sC9hnbcOc9/SrMyM5RPQrkGz4aS9Zow==",
"dependencies": {
- "shebang-regex": "^3.0.0"
+ "is-glob": "^4.0.1"
},
"engines": {
- "node": ">=8"
- }
- },
- "node_modules/@react-native-community/cli-platform-ios/node_modules/shebang-regex": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/shebang-regex/-/shebang-regex-3.0.0.tgz",
- "integrity": "sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==",
- "engines": {
- "node": ">=8"
+ "node": ">= 6"
}
},
- "node_modules/@react-native-community/cli-platform-ios/node_modules/supports-color": {
- "version": "7.2.0",
- "resolved": "https://registry.npmjs.org/supports-color/-/supports-color-7.2.0.tgz",
- "integrity": "sha512-qpCAvRl9stuOHveKsn7HncJRvv501qIacKzQlO/+Lwxc9+0q2wLyv4Dfvt80/DPn2pqOBsJdDiogXGR9+OvwRw==",
+ "node_modules/@pkgr/utils/node_modules/is-wsl": {
+ "version": "2.2.0",
+ "resolved": "https://registry.npmjs.org/is-wsl/-/is-wsl-2.2.0.tgz",
+ "integrity": "sha512-fKzAra0rGJUUBwGBgNkHZuToZcn+TtXHpeCgmkMJMMYx1sQDYaCSyjJBSCa2nH1DGm7s3n1oBnohoVTBaN7Lww==",
"dependencies": {
- "has-flag": "^4.0.0"
+ "is-docker": "^2.0.0"
},
"engines": {
"node": ">=8"
}
},
- "node_modules/@react-native-community/cli-platform-ios/node_modules/which": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
- "integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
+ "node_modules/@pkgr/utils/node_modules/open": {
+ "version": "9.1.0",
+ "resolved": "https://registry.npmjs.org/open/-/open-9.1.0.tgz",
+ "integrity": "sha512-OS+QTnw1/4vrf+9hh1jc1jnYjzSG4ttTBB8UxOwAnInG3Uo4ssetzC1ihqaIHjLJnA5GGlRl6QlZXOTQhRBUvg==",
"dependencies": {
- "isexe": "^2.0.0"
- },
- "bin": {
- "node-which": "bin/node-which"
+ "default-browser": "^4.0.0",
+ "define-lazy-prop": "^3.0.0",
+ "is-inside-container": "^1.0.0",
+ "is-wsl": "^2.2.0"
},
"engines": {
- "node": ">= 8"
- }
- },
- "node_modules/@react-native-community/cli-plugin-metro": {
- "version": "12.3.2",
- "resolved": "https://registry.npmjs.org/@react-native-community/cli-plugin-metro/-/cli-plugin-metro-12.3.2.tgz",
- "integrity": "sha512-FpFBwu+d2E7KRhYPTkKvQsWb2/JKsJv+t1tcqgQkn+oByhp+qGyXBobFB8/R3yYvRRDCSDhS+atWTJzk9TjM8g=="
- },
- "node_modules/@react-native-community/cli-server-api": {
- "version": "12.3.2",
- "resolved": "https://registry.npmjs.org/@react-native-community/cli-server-api/-/cli-server-api-12.3.2.tgz",
- "integrity": "sha512-iwa7EO9XFA/OjI5pPLLpI/6mFVqv8L73kNck3CNOJIUCCveGXBKK0VMyOkXaf/BYnihgQrXh+x5cxbDbggr7+Q==",
- "dependencies": {
- "@react-native-community/cli-debugger-ui": "12.3.2",
- "@react-native-community/cli-tools": "12.3.2",
- "compression": "^1.7.1",
- "connect": "^3.6.5",
- "errorhandler": "^1.5.1",
- "nocache": "^3.0.1",
- "pretty-format": "^26.6.2",
- "serve-static": "^1.13.1",
- "ws": "^7.5.1"
- }
- },
- "node_modules/@react-native-community/cli-server-api/node_modules/@jest/types": {
- "version": "26.6.2",
- "resolved": "https://registry.npmjs.org/@jest/types/-/types-26.6.2.tgz",
- "integrity": "sha512-fC6QCp7Sc5sX6g8Tvbmj4XUTbyrik0akgRy03yjXbQaBWWNWGE7SGtJk98m0N8nzegD/7SggrUlivxo5ax4KWQ==",
- "dependencies": {
- "@types/istanbul-lib-coverage": "^2.0.0",
- "@types/istanbul-reports": "^3.0.0",
- "@types/node": "*",
- "@types/yargs": "^15.0.0",
- "chalk": "^4.0.0"
+ "node": ">=14.16"
},
- "engines": {
- "node": ">= 10.14.2"
- }
- },
- "node_modules/@react-native-community/cli-server-api/node_modules/@types/yargs": {
- "version": "15.0.19",
- "resolved": "https://registry.npmjs.org/@types/yargs/-/yargs-15.0.19.tgz",
- "integrity": "sha512-2XUaGVmyQjgyAZldf0D0c14vvo/yv0MhQBSTJcejMMaitsn3nxCB6TmH4G0ZQf+uxROOa9mpanoSm8h6SG/1ZA==",
- "dependencies": {
- "@types/yargs-parser": "*"
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@react-native-community/cli-server-api/node_modules/pretty-format": {
- "version": "26.6.2",
- "resolved": "https://registry.npmjs.org/pretty-format/-/pretty-format-26.6.2.tgz",
- "integrity": "sha512-7AeGuCYNGmycyQbCqd/3PWH4eOoX/OiCa0uphp57NVTeAGdJGaAliecxwBDHYQCIvrW7aDBZCYeNTP/WX69mkg==",
+ "node_modules/@playwright/test": {
+ "version": "1.48.1",
+ "resolved": "https://registry.npmjs.org/@playwright/test/-/test-1.48.1.tgz",
+ "integrity": "sha512-s9RtWoxkOLmRJdw3oFvhFbs9OJS0BzrLUc8Hf6l2UdCNd1rqeEyD4BhCJkvzeEoD1FsK4mirsWwGerhVmYKtZg==",
+ "dev": true,
"dependencies": {
- "@jest/types": "^26.6.2",
- "ansi-regex": "^5.0.0",
- "ansi-styles": "^4.0.0",
- "react-is": "^17.0.1"
+ "playwright": "1.48.1"
+ },
+ "bin": {
+ "playwright": "cli.js"
},
"engines": {
- "node": ">= 10"
+ "node": ">=18"
}
},
- "node_modules/@react-native-community/cli-server-api/node_modules/react-is": {
- "version": "17.0.2",
- "resolved": "https://registry.npmjs.org/react-is/-/react-is-17.0.2.tgz",
- "integrity": "sha512-w2GsyukL62IJnlaff/nRegPQR94C/XXamvMWmSHRJ4y7Ts/4ocGRmTHvOs8PSE6pB3dWOrD/nueuU5sduBsQ4w=="
- },
- "node_modules/@react-native-community/cli-tools": {
- "version": "12.3.2",
- "resolved": "https://registry.npmjs.org/@react-native-community/cli-tools/-/cli-tools-12.3.2.tgz",
- "integrity": "sha512-nDH7vuEicHI2TI0jac/DjT3fr977iWXRdgVAqPZFFczlbs7A8GQvEdGnZ1G8dqRUmg+kptw0e4hwczAOG89JzQ==",
+ "node_modules/@pmmmwh/react-refresh-webpack-plugin": {
+ "version": "0.5.11",
+ "resolved": "https://registry.npmjs.org/@pmmmwh/react-refresh-webpack-plugin/-/react-refresh-webpack-plugin-0.5.11.tgz",
+ "integrity": "sha512-7j/6vdTym0+qZ6u4XbSAxrWBGYSdCfTzySkj7WAFgDLmSyWlOrWvpyzxlFh5jtw9dn0oL/jtW+06XfFiisN3JQ==",
"dependencies": {
- "appdirsjs": "^1.2.4",
- "chalk": "^4.1.2",
+ "ansi-html-community": "^0.0.8",
+ "common-path-prefix": "^3.0.0",
+ "core-js-pure": "^3.23.3",
+ "error-stack-parser": "^2.0.6",
"find-up": "^5.0.0",
- "mime": "^2.4.1",
- "node-fetch": "^2.6.0",
- "open": "^6.2.0",
- "ora": "^5.4.1",
- "semver": "^7.5.2",
- "shell-quote": "^1.7.3",
- "sudo-prompt": "^9.0.0"
- }
- },
- "node_modules/@react-native-community/cli-tools/node_modules/chalk": {
- "version": "4.1.2",
- "resolved": "https://registry.npmjs.org/chalk/-/chalk-4.1.2.tgz",
- "integrity": "sha512-oKnbhFyRIXpUuez8iBMmyEa4nbj4IOQyuhc/wy9kY7/WVPcwIO9VA668Pu8RkO7+0G76SLROeyw9CpQ061i4mA==",
- "dependencies": {
- "ansi-styles": "^4.1.0",
- "supports-color": "^7.1.0"
+ "html-entities": "^2.1.0",
+ "loader-utils": "^2.0.4",
+ "schema-utils": "^3.0.0",
+ "source-map": "^0.7.3"
},
"engines": {
- "node": ">=10"
+ "node": ">= 10.13"
},
- "funding": {
- "url": "https://github.com/chalk/chalk?sponsor=1"
+ "peerDependencies": {
+ "@types/webpack": "4.x || 5.x",
+ "react-refresh": ">=0.10.0 <1.0.0",
+ "sockjs-client": "^1.4.0",
+ "type-fest": ">=0.17.0 <5.0.0",
+ "webpack": ">=4.43.0 <6.0.0",
+ "webpack-dev-server": "3.x || 4.x",
+ "webpack-hot-middleware": "2.x",
+ "webpack-plugin-serve": "0.x || 1.x"
+ },
+ "peerDependenciesMeta": {
+ "@types/webpack": {
+ "optional": true
+ },
+ "sockjs-client": {
+ "optional": true
+ },
+ "type-fest": {
+ "optional": true
+ },
+ "webpack-dev-server": {
+ "optional": true
+ },
+ "webpack-hot-middleware": {
+ "optional": true
+ },
+ "webpack-plugin-serve": {
+ "optional": true
+ }
}
},
- "node_modules/@react-native-community/cli-tools/node_modules/cli-cursor": {
- "version": "3.1.0",
- "resolved": "https://registry.npmjs.org/cli-cursor/-/cli-cursor-3.1.0.tgz",
- "integrity": "sha512-I/zHAwsKf9FqGoXM4WWRACob9+SNukZTd94DWF57E4toouRulbCxcUh6RKUEOQlYTHJnzkPMySvPNaaSLNfLZw==",
+ "node_modules/@pmmmwh/react-refresh-webpack-plugin/node_modules/ajv": {
+ "version": "6.12.6",
+ "resolved": "https://registry.npmjs.org/ajv/-/ajv-6.12.6.tgz",
+ "integrity": "sha512-j3fVLgvTo527anyYyJOGTYJbG+vnnQYvE0m5mmkc1TK+nxAppkCLMIL0aZ4dblVCNoGShhm+kzE4ZUykBoMg4g==",
"dependencies": {
- "restore-cursor": "^3.1.0"
+ "fast-deep-equal": "^3.1.1",
+ "fast-json-stable-stringify": "^2.0.0",
+ "json-schema-traverse": "^0.4.1",
+ "uri-js": "^4.2.2"
},
- "engines": {
- "node": ">=8"
+ "funding": {
+ "type": "github",
+ "url": "https://github.com/sponsors/epoberezkin"
}
},
- "node_modules/@react-native-community/cli-tools/node_modules/find-up": {
+ "node_modules/@pmmmwh/react-refresh-webpack-plugin/node_modules/find-up": {
"version": "5.0.0",
"resolved": "https://registry.npmjs.org/find-up/-/find-up-5.0.0.tgz",
"integrity": "sha512-78/PXT1wlLLDgTzDs7sjq9hzz0vXD+zn+7wypEe4fXQxCmdmqfGsEPQxmiCSQI3ajFV91bVSsvNtrJRiW6nGng==",
@@ -9491,15 +8553,7 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@react-native-community/cli-tools/node_modules/has-flag": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/has-flag/-/has-flag-4.0.0.tgz",
- "integrity": "sha512-EykJT/Q1KjTWctppgIAgfSO0tKVuZUjhgMr17kqTumMl6Afv3EISleU7qZUzoXDFTAHTDC4NOoG/ZxU3EvlMPQ==",
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@react-native-community/cli-tools/node_modules/locate-path": {
+ "node_modules/@pmmmwh/react-refresh-webpack-plugin/node_modules/locate-path": {
"version": "6.0.0",
"resolved": "https://registry.npmjs.org/locate-path/-/locate-path-6.0.0.tgz",
"integrity": "sha512-iPZK6eYjbxRu3uB4/WZ3EsEIMJFMqAoopl3R+zuq0UjcAm/MO6KCweDgPfP3elTztoKP3KtnVHxTn2NHBSDVUw==",
@@ -9513,62 +8567,7 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@react-native-community/cli-tools/node_modules/mime": {
- "version": "2.6.0",
- "resolved": "https://registry.npmjs.org/mime/-/mime-2.6.0.tgz",
- "integrity": "sha512-USPkMeET31rOMiarsBNIHZKLGgvKc/LrjofAnBlOttf5ajRvqiRA8QsenbcooctK6d6Ts6aqZXBA+XbkKthiQg==",
- "bin": {
- "mime": "cli.js"
- },
- "engines": {
- "node": ">=4.0.0"
- }
- },
- "node_modules/@react-native-community/cli-tools/node_modules/mimic-fn": {
- "version": "2.1.0",
- "resolved": "https://registry.npmjs.org/mimic-fn/-/mimic-fn-2.1.0.tgz",
- "integrity": "sha512-OqbOk5oEQeAZ8WXWydlu9HJjz9WVdEIvamMCcXmuqUYjTknH/sqsWvhQ3vgwKFRR1HpjvNBKQ37nbJgYzGqGcg==",
- "engines": {
- "node": ">=6"
- }
- },
- "node_modules/@react-native-community/cli-tools/node_modules/onetime": {
- "version": "5.1.2",
- "resolved": "https://registry.npmjs.org/onetime/-/onetime-5.1.2.tgz",
- "integrity": "sha512-kbpaSSGJTWdAY5KPVeMOKXSrPtr8C8C7wodJbcsd51jRnmD+GZu8Y0VoU6Dm5Z4vWr0Ig/1NKuWRKf7j5aaYSg==",
- "dependencies": {
- "mimic-fn": "^2.1.0"
- },
- "engines": {
- "node": ">=6"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/@react-native-community/cli-tools/node_modules/ora": {
- "version": "5.4.1",
- "resolved": "https://registry.npmjs.org/ora/-/ora-5.4.1.tgz",
- "integrity": "sha512-5b6Y85tPxZZ7QytO+BQzysW31HJku27cRIlkbAXaNx+BdcVi+LlRFmVXzeF6a7JCwJpyw5c4b+YSVImQIrBpuQ==",
- "dependencies": {
- "bl": "^4.1.0",
- "chalk": "^4.1.0",
- "cli-cursor": "^3.1.0",
- "cli-spinners": "^2.5.0",
- "is-interactive": "^1.0.0",
- "is-unicode-supported": "^0.1.0",
- "log-symbols": "^4.1.0",
- "strip-ansi": "^6.0.0",
- "wcwidth": "^1.0.1"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/@react-native-community/cli-tools/node_modules/p-locate": {
+ "node_modules/@pmmmwh/react-refresh-webpack-plugin/node_modules/p-locate": {
"version": "5.0.0",
"resolved": "https://registry.npmjs.org/p-locate/-/p-locate-5.0.0.tgz",
"integrity": "sha512-LaNjtRWUBY++zB5nE/NwcaoMylSPk+S+ZHNB1TzdbMJMny6dynpAGt7X/tl/QYq3TIeE6nxHppbo2LGymrG5Pw==",
@@ -9582,7 +8581,7 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@react-native-community/cli-tools/node_modules/path-exists": {
+ "node_modules/@pmmmwh/react-refresh-webpack-plugin/node_modules/path-exists": {
"version": "4.0.0",
"resolved": "https://registry.npmjs.org/path-exists/-/path-exists-4.0.0.tgz",
"integrity": "sha512-ak9Qy5Q7jYb2Wwcey5Fpvg2KoAc/ZIhLSLOSBmRmygPsGwkVVt0fZa0qrtMz+m6tJTAHfZQ8FnmB4MG4LWy7/w==",
@@ -9590,519 +8589,434 @@
"node": ">=8"
}
},
- "node_modules/@react-native-community/cli-tools/node_modules/restore-cursor": {
- "version": "3.1.0",
- "resolved": "https://registry.npmjs.org/restore-cursor/-/restore-cursor-3.1.0.tgz",
- "integrity": "sha512-l+sSefzHpj5qimhFSE5a8nufZYAM3sBSVMAPtYkmC+4EH2anSGaEMXSD0izRQbu9nfyQ9y5JrVmp7E8oZrUjvA==",
- "dependencies": {
- "onetime": "^5.1.0",
- "signal-exit": "^3.0.2"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@react-native-community/cli-tools/node_modules/supports-color": {
- "version": "7.2.0",
- "resolved": "https://registry.npmjs.org/supports-color/-/supports-color-7.2.0.tgz",
- "integrity": "sha512-qpCAvRl9stuOHveKsn7HncJRvv501qIacKzQlO/+Lwxc9+0q2wLyv4Dfvt80/DPn2pqOBsJdDiogXGR9+OvwRw==",
- "dependencies": {
- "has-flag": "^4.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@react-native-community/cli-types": {
- "version": "12.3.2",
- "resolved": "https://registry.npmjs.org/@react-native-community/cli-types/-/cli-types-12.3.2.tgz",
- "integrity": "sha512-9D0UEFqLW8JmS16mjHJxUJWX8E+zJddrHILSH8AJHZ0NNHv4u2DXKdb0wFLMobFxGNxPT+VSOjc60fGvXzWHog==",
- "dependencies": {
- "joi": "^17.2.1"
- }
- },
- "node_modules/@react-native-community/cli/node_modules/chalk": {
- "version": "4.1.2",
- "resolved": "https://registry.npmjs.org/chalk/-/chalk-4.1.2.tgz",
- "integrity": "sha512-oKnbhFyRIXpUuez8iBMmyEa4nbj4IOQyuhc/wy9kY7/WVPcwIO9VA668Pu8RkO7+0G76SLROeyw9CpQ061i4mA==",
+ "node_modules/@pmmmwh/react-refresh-webpack-plugin/node_modules/schema-utils": {
+ "version": "3.3.0",
+ "resolved": "https://registry.npmjs.org/schema-utils/-/schema-utils-3.3.0.tgz",
+ "integrity": "sha512-pN/yOAvcC+5rQ5nERGuwrjLlYvLTbCibnZ1I7B1LaiAz9BRBlE9GMgE/eqV30P7aJQUf7Ddimy/RsbYO/GrVGg==",
"dependencies": {
- "ansi-styles": "^4.1.0",
- "supports-color": "^7.1.0"
+ "@types/json-schema": "^7.0.8",
+ "ajv": "^6.12.5",
+ "ajv-keywords": "^3.5.2"
},
"engines": {
- "node": ">=10"
+ "node": ">= 10.13.0"
},
"funding": {
- "url": "https://github.com/chalk/chalk?sponsor=1"
- }
- },
- "node_modules/@react-native-community/cli/node_modules/commander": {
- "version": "9.5.0",
- "resolved": "https://registry.npmjs.org/commander/-/commander-9.5.0.tgz",
- "integrity": "sha512-KRs7WVDKg86PWiuAqhDrAQnTXZKraVcCc6vFdL14qrZ/DcWwuRo7VoiYXalXO7S5GKpqYiVEwCbgFDfxNHKJBQ==",
- "engines": {
- "node": "^12.20.0 || >=14"
+ "type": "opencollective",
+ "url": "https://opencollective.com/webpack"
}
},
- "node_modules/@react-native-community/cli/node_modules/cross-spawn": {
- "version": "7.0.3",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-7.0.3.tgz",
- "integrity": "sha512-iRDPJKUPVEND7dHPO8rkbOnPpyDygcDFtWjpeWNCgy8WP2rXcxXL8TskReQl6OrB2G7+UJrags1q15Fudc7G6w==",
- "dependencies": {
- "path-key": "^3.1.0",
- "shebang-command": "^2.0.0",
- "which": "^2.0.1"
- },
+ "node_modules/@pmmmwh/react-refresh-webpack-plugin/node_modules/source-map": {
+ "version": "0.7.4",
+ "resolved": "https://registry.npmjs.org/source-map/-/source-map-0.7.4.tgz",
+ "integrity": "sha512-l3BikUxvPOcn5E74dZiq5BGsTb5yEwhaTSzccU6t4sDOH8NWJCstKO5QT2CvtFoK6F0saL7p9xHAqHOlCPJygA==",
"engines": {
"node": ">= 8"
}
},
- "node_modules/@react-native-community/cli/node_modules/execa": {
- "version": "5.1.1",
- "resolved": "https://registry.npmjs.org/execa/-/execa-5.1.1.tgz",
- "integrity": "sha512-8uSpZZocAZRBAPIEINJj3Lo9HyGitllczc27Eh5YYojjMFMn8yHMDMaUHE2Jqfq05D/wucwI4JGURyXt1vchyg==",
- "dependencies": {
- "cross-spawn": "^7.0.3",
- "get-stream": "^6.0.0",
- "human-signals": "^2.1.0",
- "is-stream": "^2.0.0",
- "merge-stream": "^2.0.0",
- "npm-run-path": "^4.0.1",
- "onetime": "^5.1.2",
- "signal-exit": "^3.0.3",
- "strip-final-newline": "^2.0.0"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/sindresorhus/execa?sponsor=1"
- }
+ "node_modules/@polka/url": {
+ "version": "1.0.0-next.23",
+ "resolved": "https://registry.npmjs.org/@polka/url/-/url-1.0.0-next.23.tgz",
+ "integrity": "sha512-C16M+IYz0rgRhWZdCmK+h58JMv8vijAA61gmz2rspCSwKwzBebpdcsiUmwrtJRdphuY30i6BSLEOP8ppbNLyLg=="
},
- "node_modules/@react-native-community/cli/node_modules/find-up": {
- "version": "4.1.0",
- "resolved": "https://registry.npmjs.org/find-up/-/find-up-4.1.0.tgz",
- "integrity": "sha512-PpOwAdQ/YlXQ2vj8a3h8IipDuYRi3wceVQQGYWxNINccq40Anw7BlsEXCMbt1Zt+OLA6Fq9suIpIWD0OsnISlw==",
+ "node_modules/@preact/signals": {
+ "version": "1.3.0",
+ "resolved": "https://registry.npmjs.org/@preact/signals/-/signals-1.3.0.tgz",
+ "integrity": "sha512-EOMeg42SlLS72dhoq6Vjq08havnLseWmPQ8A0YsgIAqMgWgx7V1a39+Pxo6i7SY5NwJtH4849JogFq3M67AzWg==",
+ "license": "MIT",
"dependencies": {
- "locate-path": "^5.0.0",
- "path-exists": "^4.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@react-native-community/cli/node_modules/get-stream": {
- "version": "6.0.1",
- "resolved": "https://registry.npmjs.org/get-stream/-/get-stream-6.0.1.tgz",
- "integrity": "sha512-ts6Wi+2j3jQjqi70w5AlN8DFnkSwC+MqmxEzdEALB2qXZYV3X/b1CTfgPLGJNMeAWxdPfU8FO1ms3NUfaHCPYg==",
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/@react-native-community/cli/node_modules/has-flag": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/has-flag/-/has-flag-4.0.0.tgz",
- "integrity": "sha512-EykJT/Q1KjTWctppgIAgfSO0tKVuZUjhgMr17kqTumMl6Afv3EISleU7qZUzoXDFTAHTDC4NOoG/ZxU3EvlMPQ==",
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@react-native-community/cli/node_modules/human-signals": {
- "version": "2.1.0",
- "resolved": "https://registry.npmjs.org/human-signals/-/human-signals-2.1.0.tgz",
- "integrity": "sha512-B4FFZ6q/T2jhhksgkbEW3HBvWIfDW85snkQgawt07S7J5QXTk6BkNV+0yAeZrM5QpMAdYlocGoljn0sJ/WQkFw==",
- "engines": {
- "node": ">=10.17.0"
- }
- },
- "node_modules/@react-native-community/cli/node_modules/is-stream": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/is-stream/-/is-stream-2.0.1.tgz",
- "integrity": "sha512-hFoiJiTl63nn+kstHGBtewWSKnQLpyb155KHheA1l39uvtO9nWIop1p3udqPcUd/xbF1VLMO4n7OI6p7RbngDg==",
- "engines": {
- "node": ">=8"
+ "@preact/signals-core": "^1.7.0"
},
"funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/@react-native-community/cli/node_modules/locate-path": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/locate-path/-/locate-path-5.0.0.tgz",
- "integrity": "sha512-t7hw9pI+WvuwNJXwk5zVHpyhIqzg2qTlklJOf0mVxGSbe3Fp2VieZcduNYjaLDoy6p9uGpQEGWG87WpMKlNq8g==",
- "dependencies": {
- "p-locate": "^4.1.0"
+ "type": "opencollective",
+ "url": "https://opencollective.com/preact"
},
- "engines": {
- "node": ">=8"
+ "peerDependencies": {
+ "preact": "10.x"
}
},
- "node_modules/@react-native-community/cli/node_modules/mimic-fn": {
- "version": "2.1.0",
- "resolved": "https://registry.npmjs.org/mimic-fn/-/mimic-fn-2.1.0.tgz",
- "integrity": "sha512-OqbOk5oEQeAZ8WXWydlu9HJjz9WVdEIvamMCcXmuqUYjTknH/sqsWvhQ3vgwKFRR1HpjvNBKQ37nbJgYzGqGcg==",
- "engines": {
- "node": ">=6"
+ "node_modules/@preact/signals-core": {
+ "version": "1.8.0",
+ "resolved": "https://registry.npmjs.org/@preact/signals-core/-/signals-core-1.8.0.tgz",
+ "integrity": "sha512-OBvUsRZqNmjzCZXWLxkZfhcgT+Fk8DDcT/8vD6a1xhDemodyy87UJRJfASMuSD8FaAIeGgGm85ydXhm7lr4fyA==",
+ "license": "MIT",
+ "funding": {
+ "type": "opencollective",
+ "url": "https://opencollective.com/preact"
}
},
- "node_modules/@react-native-community/cli/node_modules/npm-run-path": {
- "version": "4.0.1",
- "resolved": "https://registry.npmjs.org/npm-run-path/-/npm-run-path-4.0.1.tgz",
- "integrity": "sha512-S48WzZW777zhNIrn7gxOlISNAqi9ZC/uQFnRdbeIHhZhCA6UqpkOT8T1G7BvfdgP4Er8gF4sUbaS0i7QvIfCWw==",
+ "node_modules/@promptbook/utils": {
+ "version": "0.69.5",
+ "resolved": "https://registry.npmjs.org/@promptbook/utils/-/utils-0.69.5.tgz",
+ "integrity": "sha512-xm5Ti/Hp3o4xHrsK9Yy3MS6KbDxYbq485hDsFvxqaNA7equHLPdo8H8faTitTeb14QCDfLW4iwCxdVYu5sn6YQ==",
+ "dev": true,
+ "funding": [
+ {
+ "type": "individual",
+ "url": "https://buymeacoffee.com/hejny"
+ },
+ {
+ "type": "github",
+ "url": "https://github.com/webgptorg/promptbook/blob/main/README.md#%EF%B8%8F-contributing"
+ }
+ ],
+ "license": "CC-BY-4.0",
"dependencies": {
- "path-key": "^3.0.0"
- },
- "engines": {
- "node": ">=8"
+ "spacetrim": "0.11.59"
}
},
- "node_modules/@react-native-community/cli/node_modules/onetime": {
- "version": "5.1.2",
- "resolved": "https://registry.npmjs.org/onetime/-/onetime-5.1.2.tgz",
- "integrity": "sha512-kbpaSSGJTWdAY5KPVeMOKXSrPtr8C8C7wodJbcsd51jRnmD+GZu8Y0VoU6Dm5Z4vWr0Ig/1NKuWRKf7j5aaYSg==",
+ "node_modules/@puppeteer/browsers": {
+ "version": "1.9.1",
+ "resolved": "https://registry.npmjs.org/@puppeteer/browsers/-/browsers-1.9.1.tgz",
+ "integrity": "sha512-PuvK6xZzGhKPvlx3fpfdM2kYY3P/hB1URtK8wA7XUJ6prn6pp22zvJHu48th0SGcHL9SutbPHrFuQgfXTFobWA==",
+ "dev": true,
+ "license": "Apache-2.0",
"dependencies": {
- "mimic-fn": "^2.1.0"
+ "debug": "4.3.4",
+ "extract-zip": "2.0.1",
+ "progress": "2.0.3",
+ "proxy-agent": "6.3.1",
+ "tar-fs": "3.0.4",
+ "unbzip2-stream": "1.4.3",
+ "yargs": "17.7.2"
},
- "engines": {
- "node": ">=6"
+ "bin": {
+ "browsers": "lib/cjs/main-cli.js"
},
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "engines": {
+ "node": ">=16.3.0"
}
},
- "node_modules/@react-native-community/cli/node_modules/p-limit": {
- "version": "2.3.0",
- "resolved": "https://registry.npmjs.org/p-limit/-/p-limit-2.3.0.tgz",
- "integrity": "sha512-//88mFWSJx8lxCzwdAABTJL2MyWB12+eIY7MDL2SqLmAkeKU9qxRvWuSyTjm3FUmpBEMuFfckAIqEaVGUDxb6w==",
+ "node_modules/@puppeteer/browsers/node_modules/extract-zip": {
+ "version": "2.0.1",
+ "resolved": "https://registry.npmjs.org/extract-zip/-/extract-zip-2.0.1.tgz",
+ "integrity": "sha512-GDhU9ntwuKyGXdZBUgTIe+vXnWj0fppUEtMDL0+idd5Sta8TGpHssn/eusA9mrPr9qNDym6SxAYZjNvCn/9RBg==",
+ "dev": true,
+ "license": "BSD-2-Clause",
"dependencies": {
- "p-try": "^2.0.0"
+ "debug": "^4.1.1",
+ "get-stream": "^5.1.0",
+ "yauzl": "^2.10.0"
+ },
+ "bin": {
+ "extract-zip": "cli.js"
},
"engines": {
- "node": ">=6"
+ "node": ">= 10.17.0"
},
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "optionalDependencies": {
+ "@types/yauzl": "^2.9.1"
}
},
- "node_modules/@react-native-community/cli/node_modules/p-locate": {
- "version": "4.1.0",
- "resolved": "https://registry.npmjs.org/p-locate/-/p-locate-4.1.0.tgz",
- "integrity": "sha512-R79ZZ/0wAxKGu3oYMlz8jy/kbhsNrS7SKZ7PxEHBgJ5+F2mtFW2fK2cOtBh1cHYkQsbzFV7I+EoRKe6Yt0oK7A==",
+ "node_modules/@puppeteer/browsers/node_modules/pump": {
+ "version": "3.0.2",
+ "resolved": "https://registry.npmjs.org/pump/-/pump-3.0.2.tgz",
+ "integrity": "sha512-tUPXtzlGM8FE3P0ZL6DVs/3P58k9nk8/jZeQCurTJylQA8qFYzHFfhBJkuqyE0FifOsQ0uKWekiZ5g8wtr28cw==",
+ "dev": true,
+ "license": "MIT",
"dependencies": {
- "p-limit": "^2.2.0"
- },
- "engines": {
- "node": ">=8"
+ "end-of-stream": "^1.1.0",
+ "once": "^1.3.1"
}
},
- "node_modules/@react-native-community/cli/node_modules/p-try": {
- "version": "2.2.0",
- "resolved": "https://registry.npmjs.org/p-try/-/p-try-2.2.0.tgz",
- "integrity": "sha512-R4nPAVTAU0B9D35/Gk3uJf/7XYbQcyohSKdvAxIRSNghFl4e71hVoGnBNQz9cWaXxO2I10KTC+3jMdvvoKw6dQ==",
- "engines": {
- "node": ">=6"
+ "node_modules/@puppeteer/browsers/node_modules/tar-fs": {
+ "version": "3.0.4",
+ "resolved": "https://registry.npmjs.org/tar-fs/-/tar-fs-3.0.4.tgz",
+ "integrity": "sha512-5AFQU8b9qLfZCX9zp2duONhPmZv0hGYiBPJsyUdqMjzq/mqVpy/rEUSeHk1+YitmxugaptgBh5oDGU3VsAJq4w==",
+ "dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "mkdirp-classic": "^0.5.2",
+ "pump": "^3.0.0",
+ "tar-stream": "^3.1.5"
}
},
- "node_modules/@react-native-community/cli/node_modules/path-exists": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/path-exists/-/path-exists-4.0.0.tgz",
- "integrity": "sha512-ak9Qy5Q7jYb2Wwcey5Fpvg2KoAc/ZIhLSLOSBmRmygPsGwkVVt0fZa0qrtMz+m6tJTAHfZQ8FnmB4MG4LWy7/w==",
- "engines": {
- "node": ">=8"
+ "node_modules/@puppeteer/browsers/node_modules/tar-stream": {
+ "version": "3.1.7",
+ "resolved": "https://registry.npmjs.org/tar-stream/-/tar-stream-3.1.7.tgz",
+ "integrity": "sha512-qJj60CXt7IU1Ffyc3NJMjh6EkuCFej46zUqJ4J7pqYlThyd9bO0XBTmcOIhSzZJVWfsLks0+nle/j538YAW9RQ==",
+ "dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "b4a": "^1.6.4",
+ "fast-fifo": "^1.2.0",
+ "streamx": "^2.15.0"
}
},
- "node_modules/@react-native-community/cli/node_modules/path-key": {
- "version": "3.1.1",
- "resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
- "integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
- "engines": {
- "node": ">=8"
+ "node_modules/@radix-ui/primitive": {
+ "version": "1.0.1",
+ "resolved": "https://registry.npmjs.org/@radix-ui/primitive/-/primitive-1.0.1.tgz",
+ "integrity": "sha512-yQ8oGX2GVsEYMWGxcovu1uGWPCxV5BFfeeYxqPmuAzUyLT9qmaMXSAhXpb0WrspIeqYzdJpkh2vHModJPgRIaw==",
+ "license": "MIT",
+ "dependencies": {
+ "@babel/runtime": "^7.13.10"
}
},
- "node_modules/@react-native-community/cli/node_modules/shebang-command": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/shebang-command/-/shebang-command-2.0.0.tgz",
- "integrity": "sha512-kHxr2zZpYtdmrN1qDjrrX/Z1rR1kG8Dx+gkpK1G4eXmvXswmcE1hTWBWYUzlraYw1/yZp6YuDY77YtvbN0dmDA==",
+ "node_modules/@radix-ui/react-compose-refs": {
+ "version": "1.0.1",
+ "resolved": "https://registry.npmjs.org/@radix-ui/react-compose-refs/-/react-compose-refs-1.0.1.tgz",
+ "integrity": "sha512-fDSBgd44FKHa1FRMU59qBMPFcl2PZE+2nmqunj+BWFyYYjnhIDWL2ItDs3rrbJDQOtzt5nIebLCQc4QRfz6LJw==",
+ "license": "MIT",
"dependencies": {
- "shebang-regex": "^3.0.0"
+ "@babel/runtime": "^7.13.10"
},
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@react-native-community/cli/node_modules/shebang-regex": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/shebang-regex/-/shebang-regex-3.0.0.tgz",
- "integrity": "sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==",
- "engines": {
- "node": ">=8"
+ "peerDependencies": {
+ "@types/react": "*",
+ "react": "^16.8 || ^17.0 || ^18.0"
+ },
+ "peerDependenciesMeta": {
+ "@types/react": {
+ "optional": true
+ }
}
},
- "node_modules/@react-native-community/cli/node_modules/supports-color": {
- "version": "7.2.0",
- "resolved": "https://registry.npmjs.org/supports-color/-/supports-color-7.2.0.tgz",
- "integrity": "sha512-qpCAvRl9stuOHveKsn7HncJRvv501qIacKzQlO/+Lwxc9+0q2wLyv4Dfvt80/DPn2pqOBsJdDiogXGR9+OvwRw==",
+ "node_modules/@radix-ui/react-context": {
+ "version": "1.0.1",
+ "resolved": "https://registry.npmjs.org/@radix-ui/react-context/-/react-context-1.0.1.tgz",
+ "integrity": "sha512-ebbrdFoYTcuZ0v4wG5tedGnp9tzcV8awzsxYph7gXUyvnNLuTIcCk1q17JEbnVhXAKG9oX3KtchwiMIAYp9NLg==",
+ "license": "MIT",
"dependencies": {
- "has-flag": "^4.0.0"
+ "@babel/runtime": "^7.13.10"
},
- "engines": {
- "node": ">=8"
+ "peerDependencies": {
+ "@types/react": "*",
+ "react": "^16.8 || ^17.0 || ^18.0"
+ },
+ "peerDependenciesMeta": {
+ "@types/react": {
+ "optional": true
+ }
}
},
- "node_modules/@react-native-community/cli/node_modules/which": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
- "integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
+ "node_modules/@radix-ui/react-focus-guards": {
+ "version": "1.0.1",
+ "resolved": "https://registry.npmjs.org/@radix-ui/react-focus-guards/-/react-focus-guards-1.0.1.tgz",
+ "integrity": "sha512-Rect2dWbQ8waGzhMavsIbmSVCgYxkXLxxR3ZvCX79JOglzdEy4JXMb98lq4hPxUbLr77nP0UOGf4rcMU+s1pUA==",
+ "license": "MIT",
"dependencies": {
- "isexe": "^2.0.0"
+ "@babel/runtime": "^7.13.10"
},
- "bin": {
- "node-which": "bin/node-which"
+ "peerDependencies": {
+ "@types/react": "*",
+ "react": "^16.8 || ^17.0 || ^18.0"
},
- "engines": {
- "node": ">= 8"
+ "peerDependenciesMeta": {
+ "@types/react": {
+ "optional": true
+ }
}
},
- "node_modules/@react-native-community/slider": {
- "version": "3.0.2-wp-5",
- "resolved": "https://raw.githubusercontent.com/wordpress-mobile/react-native-slider/v3.0.2-wp-5/react-native-community-slider-3.0.2-wp-5.tgz",
- "integrity": "sha512-19yl9Em1mFKFLB9o3IO3VpBFw+U7cswjRk6cZ7kkhDCpDD3u0cpzkaIZK6NuFIPCLqOwU0MPtP+gonanN1JQKw==",
- "workspaces": [
- "src",
- "example"
- ]
- },
- "node_modules/@react-native-masked-view/masked-view": {
- "version": "0.3.0",
- "resolved": "https://registry.npmjs.org/@react-native-masked-view/masked-view/-/masked-view-0.3.0.tgz",
- "integrity": "sha512-qLyoObcjzrkpNcoJjXquUePXfL1dXjHtuv+yX0zZ0Q4kG5yvVqd620+tSh7WbRoHkjpXhFBfLwvGhcWB2I0Lpw==",
+ "node_modules/@radix-ui/react-id": {
+ "version": "1.0.1",
+ "resolved": "https://registry.npmjs.org/@radix-ui/react-id/-/react-id-1.0.1.tgz",
+ "integrity": "sha512-tI7sT/kqYp8p96yGWY1OAnLHrqDgzHefRBKQ2YAkBS5ja7QLcZ9Z/uY7bEjPUatf8RomoXM8/1sMj1IJaE5UzQ==",
+ "license": "MIT",
+ "dependencies": {
+ "@babel/runtime": "^7.13.10",
+ "@radix-ui/react-use-layout-effect": "1.0.1"
+ },
"peerDependencies": {
- "react": ">=16",
- "react-native": ">=0.57"
- }
- },
- "node_modules/@react-native/assets-registry": {
- "version": "0.73.1",
- "resolved": "https://registry.npmjs.org/@react-native/assets-registry/-/assets-registry-0.73.1.tgz",
- "integrity": "sha512-2FgAbU7uKM5SbbW9QptPPZx8N9Ke2L7bsHb+EhAanZjFZunA9PaYtyjUQ1s7HD+zDVqOQIvjkpXSv7Kejd2tqg==",
- "engines": {
- "node": ">=18"
+ "@types/react": "*",
+ "react": "^16.8 || ^17.0 || ^18.0"
+ },
+ "peerDependenciesMeta": {
+ "@types/react": {
+ "optional": true
+ }
}
},
- "node_modules/@react-native/babel-plugin-codegen": {
- "version": "0.73.3",
- "resolved": "https://registry.npmjs.org/@react-native/babel-plugin-codegen/-/babel-plugin-codegen-0.73.3.tgz",
- "integrity": "sha512-+zQrDDbz6lB48LyzFHxNCgXDCBHH+oTRdXAjikRcBUdeG9St9ABbYFLtb799zSxLOrCqFVyXqhJR2vlgLLEbcg==",
+ "node_modules/@radix-ui/react-primitive": {
+ "version": "1.0.3",
+ "resolved": "https://registry.npmjs.org/@radix-ui/react-primitive/-/react-primitive-1.0.3.tgz",
+ "integrity": "sha512-yi58uVyoAcK/Nq1inRY56ZSjKypBNKTa/1mcL8qdl6oJeEaDbOldlzrGn7P6Q3Id5d+SYNGc5AJgc4vGhjs5+g==",
+ "license": "MIT",
"dependencies": {
- "@react-native/codegen": "0.73.2"
+ "@babel/runtime": "^7.13.10",
+ "@radix-ui/react-slot": "1.0.2"
},
- "engines": {
- "node": ">=18"
+ "peerDependencies": {
+ "@types/react": "*",
+ "@types/react-dom": "*",
+ "react": "^16.8 || ^17.0 || ^18.0",
+ "react-dom": "^16.8 || ^17.0 || ^18.0"
+ },
+ "peerDependenciesMeta": {
+ "@types/react": {
+ "optional": true
+ },
+ "@types/react-dom": {
+ "optional": true
+ }
}
},
- "node_modules/@react-native/babel-preset": {
- "version": "0.73.10",
- "resolved": "https://registry.npmjs.org/@react-native/babel-preset/-/babel-preset-0.73.10.tgz",
- "integrity": "sha512-LPxR9DZK1EtVSEm2+fpei/AWwzgyHfaY7fuX8wDiy2THRYc/56+v28IihN8g0IAbQH+gdjXVcshJRYfV6bh2gg==",
- "dev": true,
+ "node_modules/@radix-ui/react-slot": {
+ "version": "1.0.2",
+ "resolved": "https://registry.npmjs.org/@radix-ui/react-slot/-/react-slot-1.0.2.tgz",
+ "integrity": "sha512-YeTpuq4deV+6DusvVUW4ivBgnkHwECUu0BiN43L5UCDFgdhsRUWAghhTF5MbvNTPzmiFOx90asDSUjWuCNapwg==",
+ "license": "MIT",
"dependencies": {
- "@babel/core": "^7.20.0",
- "@babel/plugin-proposal-async-generator-functions": "^7.0.0",
- "@babel/plugin-proposal-class-properties": "^7.18.0",
- "@babel/plugin-proposal-export-default-from": "^7.0.0",
- "@babel/plugin-proposal-nullish-coalescing-operator": "^7.18.0",
- "@babel/plugin-proposal-numeric-separator": "^7.0.0",
- "@babel/plugin-proposal-object-rest-spread": "^7.20.0",
- "@babel/plugin-proposal-optional-catch-binding": "^7.0.0",
- "@babel/plugin-proposal-optional-chaining": "^7.20.0",
- "@babel/plugin-syntax-dynamic-import": "^7.8.0",
- "@babel/plugin-syntax-export-default-from": "^7.0.0",
- "@babel/plugin-syntax-flow": "^7.18.0",
- "@babel/plugin-syntax-nullish-coalescing-operator": "^7.0.0",
- "@babel/plugin-syntax-optional-chaining": "^7.0.0",
- "@babel/plugin-transform-arrow-functions": "^7.0.0",
- "@babel/plugin-transform-async-to-generator": "^7.20.0",
- "@babel/plugin-transform-block-scoping": "^7.0.0",
- "@babel/plugin-transform-classes": "^7.0.0",
- "@babel/plugin-transform-computed-properties": "^7.0.0",
- "@babel/plugin-transform-destructuring": "^7.20.0",
- "@babel/plugin-transform-flow-strip-types": "^7.20.0",
- "@babel/plugin-transform-function-name": "^7.0.0",
- "@babel/plugin-transform-literals": "^7.0.0",
- "@babel/plugin-transform-modules-commonjs": "^7.0.0",
- "@babel/plugin-transform-named-capturing-groups-regex": "^7.0.0",
- "@babel/plugin-transform-parameters": "^7.0.0",
- "@babel/plugin-transform-react-display-name": "^7.0.0",
- "@babel/plugin-transform-react-jsx": "^7.0.0",
- "@babel/plugin-transform-react-jsx-self": "^7.0.0",
- "@babel/plugin-transform-react-jsx-source": "^7.0.0",
- "@babel/plugin-transform-runtime": "^7.0.0",
- "@babel/plugin-transform-shorthand-properties": "^7.0.0",
- "@babel/plugin-transform-spread": "^7.0.0",
- "@babel/plugin-transform-sticky-regex": "^7.0.0",
- "@babel/plugin-transform-typescript": "^7.5.0",
- "@babel/plugin-transform-unicode-regex": "^7.0.0",
- "@babel/template": "^7.0.0",
- "babel-plugin-transform-flow-enums": "^0.0.2",
- "react-refresh": "^0.4.0"
- },
- "engines": {
- "node": ">=18"
+ "@babel/runtime": "^7.13.10",
+ "@radix-ui/react-compose-refs": "1.0.1"
},
"peerDependencies": {
- "@babel/core": "*"
- }
- },
- "node_modules/@react-native/babel-preset/node_modules/react-refresh": {
- "version": "0.4.3",
- "resolved": "https://registry.npmjs.org/react-refresh/-/react-refresh-0.4.3.tgz",
- "integrity": "sha512-Hwln1VNuGl/6bVwnd0Xdn1e84gT/8T9aYNL+HAKDArLCS7LWjwr7StE30IEYbIkx0Vi3vs+coQxe+SQDbGbbpA==",
- "dev": true,
- "engines": {
- "node": ">=0.10.0"
+ "@types/react": "*",
+ "react": "^16.8 || ^17.0 || ^18.0"
+ },
+ "peerDependenciesMeta": {
+ "@types/react": {
+ "optional": true
+ }
}
},
- "node_modules/@react-native/codegen": {
- "version": "0.73.2",
- "resolved": "https://registry.npmjs.org/@react-native/codegen/-/codegen-0.73.2.tgz",
- "integrity": "sha512-lfy8S7umhE3QLQG5ViC4wg5N1Z+E6RnaeIw8w1voroQsXXGPB72IBozh8dAHR3+ceTxIU0KX3A8OpJI8e1+HpQ==",
+ "node_modules/@radix-ui/react-use-callback-ref": {
+ "version": "1.0.1",
+ "resolved": "https://registry.npmjs.org/@radix-ui/react-use-callback-ref/-/react-use-callback-ref-1.0.1.tgz",
+ "integrity": "sha512-D94LjX4Sp0xJFVaoQOd3OO9k7tpBYNOXdVhkltUbGv2Qb9OXdrg/CpsjlZv7ia14Sylv398LswWBVVu5nqKzAQ==",
+ "license": "MIT",
"dependencies": {
- "@babel/parser": "^7.20.0",
- "flow-parser": "^0.206.0",
- "glob": "^7.1.1",
- "invariant": "^2.2.4",
- "jscodeshift": "^0.14.0",
- "mkdirp": "^0.5.1",
- "nullthrows": "^1.1.1"
- },
- "engines": {
- "node": ">=18"
+ "@babel/runtime": "^7.13.10"
},
"peerDependencies": {
- "@babel/preset-env": "^7.1.6"
+ "@types/react": "*",
+ "react": "^16.8 || ^17.0 || ^18.0"
+ },
+ "peerDependenciesMeta": {
+ "@types/react": {
+ "optional": true
+ }
}
},
- "node_modules/@react-native/codegen/node_modules/mkdirp": {
- "version": "0.5.6",
- "resolved": "https://registry.npmjs.org/mkdirp/-/mkdirp-0.5.6.tgz",
- "integrity": "sha512-FP+p8RB8OWpF3YZBCrP5gtADmtXApB5AMLn+vdyA+PyxCjrCs00mjyUozssO33cwDeT3wNGdLxJ5M//YqtHAJw==",
+ "node_modules/@radix-ui/react-use-controllable-state": {
+ "version": "1.0.1",
+ "resolved": "https://registry.npmjs.org/@radix-ui/react-use-controllable-state/-/react-use-controllable-state-1.0.1.tgz",
+ "integrity": "sha512-Svl5GY5FQeN758fWKrjM6Qb7asvXeiZltlT4U2gVfl8Gx5UAv2sMR0LWo8yhsIZh2oQ0eFdZ59aoOOMV7b47VA==",
+ "license": "MIT",
"dependencies": {
- "minimist": "^1.2.6"
+ "@babel/runtime": "^7.13.10",
+ "@radix-ui/react-use-callback-ref": "1.0.1"
},
- "bin": {
- "mkdirp": "bin/cmd.js"
+ "peerDependencies": {
+ "@types/react": "*",
+ "react": "^16.8 || ^17.0 || ^18.0"
+ },
+ "peerDependenciesMeta": {
+ "@types/react": {
+ "optional": true
+ }
}
},
- "node_modules/@react-native/community-cli-plugin": {
- "version": "0.73.14",
- "resolved": "https://registry.npmjs.org/@react-native/community-cli-plugin/-/community-cli-plugin-0.73.14.tgz",
- "integrity": "sha512-KzIwsTvAJrXPtwhGOSm+OcJH1B8TpY8cS4xxzu/e2qv3a2n4VLePHTPAfco1tmvekV8OHWvvD9JSIX7i2fB1gg==",
+ "node_modules/@radix-ui/react-use-escape-keydown": {
+ "version": "1.0.3",
+ "resolved": "https://registry.npmjs.org/@radix-ui/react-use-escape-keydown/-/react-use-escape-keydown-1.0.3.tgz",
+ "integrity": "sha512-vyL82j40hcFicA+M4Ex7hVkB9vHgSse1ZWomAqV2Je3RleKGO5iM8KMOEtfoSB0PnIelMd2lATjTGMYqN5ylTg==",
+ "license": "MIT",
"dependencies": {
- "@react-native-community/cli-server-api": "12.3.2",
- "@react-native-community/cli-tools": "12.3.2",
- "@react-native/dev-middleware": "0.73.7",
- "@react-native/metro-babel-transformer": "0.73.14",
- "chalk": "^4.0.0",
- "execa": "^5.1.1",
- "metro": "^0.80.3",
- "metro-config": "^0.80.3",
- "metro-core": "^0.80.3",
- "node-fetch": "^2.2.0",
- "readline": "^1.3.0"
+ "@babel/runtime": "^7.13.10",
+ "@radix-ui/react-use-callback-ref": "1.0.1"
},
- "engines": {
- "node": ">=18"
+ "peerDependencies": {
+ "@types/react": "*",
+ "react": "^16.8 || ^17.0 || ^18.0"
+ },
+ "peerDependenciesMeta": {
+ "@types/react": {
+ "optional": true
+ }
}
},
- "node_modules/@react-native/community-cli-plugin/node_modules/@react-native/babel-preset": {
- "version": "0.73.20",
- "resolved": "https://registry.npmjs.org/@react-native/babel-preset/-/babel-preset-0.73.20.tgz",
- "integrity": "sha512-fU9NqkusbfFq71l4BWQfqqD/lLcLC0MZ++UYgieA3j8lIEppJTLVauv2RwtD2yltBkjebgYEC5Rwvt1l0MUBXw==",
+ "node_modules/@radix-ui/react-use-layout-effect": {
+ "version": "1.0.1",
+ "resolved": "https://registry.npmjs.org/@radix-ui/react-use-layout-effect/-/react-use-layout-effect-1.0.1.tgz",
+ "integrity": "sha512-v/5RegiJWYdoCvMnITBkNNx6bCj20fiaJnWtRkU18yITptraXjffz5Qbn05uOiQnOvi+dbkznkoaMltz1GnszQ==",
+ "license": "MIT",
"dependencies": {
- "@babel/core": "^7.20.0",
- "@babel/plugin-proposal-async-generator-functions": "^7.0.0",
- "@babel/plugin-proposal-class-properties": "^7.18.0",
- "@babel/plugin-proposal-export-default-from": "^7.0.0",
- "@babel/plugin-proposal-nullish-coalescing-operator": "^7.18.0",
- "@babel/plugin-proposal-numeric-separator": "^7.0.0",
- "@babel/plugin-proposal-object-rest-spread": "^7.20.0",
- "@babel/plugin-proposal-optional-catch-binding": "^7.0.0",
- "@babel/plugin-proposal-optional-chaining": "^7.20.0",
- "@babel/plugin-syntax-dynamic-import": "^7.8.0",
- "@babel/plugin-syntax-export-default-from": "^7.0.0",
- "@babel/plugin-syntax-flow": "^7.18.0",
- "@babel/plugin-syntax-nullish-coalescing-operator": "^7.0.0",
- "@babel/plugin-syntax-optional-chaining": "^7.0.0",
- "@babel/plugin-transform-arrow-functions": "^7.0.0",
- "@babel/plugin-transform-async-to-generator": "^7.20.0",
- "@babel/plugin-transform-block-scoping": "^7.0.0",
- "@babel/plugin-transform-classes": "^7.0.0",
- "@babel/plugin-transform-computed-properties": "^7.0.0",
- "@babel/plugin-transform-destructuring": "^7.20.0",
- "@babel/plugin-transform-flow-strip-types": "^7.20.0",
- "@babel/plugin-transform-function-name": "^7.0.0",
- "@babel/plugin-transform-literals": "^7.0.0",
- "@babel/plugin-transform-modules-commonjs": "^7.0.0",
- "@babel/plugin-transform-named-capturing-groups-regex": "^7.0.0",
- "@babel/plugin-transform-parameters": "^7.0.0",
- "@babel/plugin-transform-private-methods": "^7.22.5",
- "@babel/plugin-transform-private-property-in-object": "^7.22.11",
- "@babel/plugin-transform-react-display-name": "^7.0.0",
- "@babel/plugin-transform-react-jsx": "^7.0.0",
- "@babel/plugin-transform-react-jsx-self": "^7.0.0",
- "@babel/plugin-transform-react-jsx-source": "^7.0.0",
- "@babel/plugin-transform-runtime": "^7.0.0",
- "@babel/plugin-transform-shorthand-properties": "^7.0.0",
- "@babel/plugin-transform-spread": "^7.0.0",
- "@babel/plugin-transform-sticky-regex": "^7.0.0",
- "@babel/plugin-transform-typescript": "^7.5.0",
- "@babel/plugin-transform-unicode-regex": "^7.0.0",
- "@babel/template": "^7.0.0",
- "@react-native/babel-plugin-codegen": "0.73.3",
- "babel-plugin-transform-flow-enums": "^0.0.2",
- "react-refresh": "^0.14.0"
+ "@babel/runtime": "^7.13.10"
},
- "engines": {
- "node": ">=18"
+ "peerDependencies": {
+ "@types/react": "*",
+ "react": "^16.8 || ^17.0 || ^18.0"
},
+ "peerDependenciesMeta": {
+ "@types/react": {
+ "optional": true
+ }
+ }
+ },
+ "node_modules/@react-native-clipboard/clipboard": {
+ "version": "1.11.2",
+ "resolved": "https://registry.npmjs.org/@react-native-clipboard/clipboard/-/clipboard-1.11.2.tgz",
+ "integrity": "sha512-bHyZVW62TuleiZsXNHS1Pv16fWc0fh8O9WvBzl4h2fykqZRW9a+Pv/RGTH56E3X2PqzHP38K5go8zmCZUoIsoQ==",
"peerDependencies": {
- "@babel/core": "*"
+ "react": ">=16.0",
+ "react-native": ">=0.57.0"
}
},
- "node_modules/@react-native/community-cli-plugin/node_modules/@react-native/metro-babel-transformer": {
- "version": "0.73.14",
- "resolved": "https://registry.npmjs.org/@react-native/metro-babel-transformer/-/metro-babel-transformer-0.73.14.tgz",
- "integrity": "sha512-5wLeYw/lormpSqYfI9H/geZ/EtPmi+x5qLkEit15Q/70hkzYo/M+aWztUtbOITfgTEOP8d6ybROzoGsqgyZLcw==",
+ "node_modules/@react-native-community/blur": {
+ "version": "4.2.0",
+ "resolved": "https://registry.npmjs.org/@react-native-community/blur/-/blur-4.2.0.tgz",
+ "integrity": "sha512-StgP5zQJOCHqDRjmcKnzVkJ920S6DYBKRJfigSUnlkNQp+HzZtVtyKq0j5a7x84NtHcV7j8Uy5mz1Lx9ZKRKfA==",
+ "peerDependencies": {
+ "react": "*",
+ "react-native": "*"
+ }
+ },
+ "node_modules/@react-native-community/cli": {
+ "version": "12.3.2",
+ "resolved": "https://registry.npmjs.org/@react-native-community/cli/-/cli-12.3.2.tgz",
+ "integrity": "sha512-WgoUWwLDcf/G1Su2COUUVs3RzAwnV/vUTdISSpAUGgSc57mPabaAoUctKTnfYEhCnE3j02k3VtaVPwCAFRO3TQ==",
"dependencies": {
- "@babel/core": "^7.20.0",
- "@react-native/babel-preset": "0.73.20",
- "hermes-parser": "0.15.0",
- "nullthrows": "^1.1.1"
+ "@react-native-community/cli-clean": "12.3.2",
+ "@react-native-community/cli-config": "12.3.2",
+ "@react-native-community/cli-debugger-ui": "12.3.2",
+ "@react-native-community/cli-doctor": "12.3.2",
+ "@react-native-community/cli-hermes": "12.3.2",
+ "@react-native-community/cli-plugin-metro": "12.3.2",
+ "@react-native-community/cli-server-api": "12.3.2",
+ "@react-native-community/cli-tools": "12.3.2",
+ "@react-native-community/cli-types": "12.3.2",
+ "chalk": "^4.1.2",
+ "commander": "^9.4.1",
+ "deepmerge": "^4.3.0",
+ "execa": "^5.0.0",
+ "find-up": "^4.1.0",
+ "fs-extra": "^8.1.0",
+ "graceful-fs": "^4.1.3",
+ "prompts": "^2.4.2",
+ "semver": "^7.5.2"
+ },
+ "bin": {
+ "react-native": "build/bin.js"
},
"engines": {
"node": ">=18"
- },
- "peerDependencies": {
- "@babel/core": "*"
}
},
- "node_modules/@react-native/community-cli-plugin/node_modules/cross-spawn": {
- "version": "7.0.3",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-7.0.3.tgz",
- "integrity": "sha512-iRDPJKUPVEND7dHPO8rkbOnPpyDygcDFtWjpeWNCgy8WP2rXcxXL8TskReQl6OrB2G7+UJrags1q15Fudc7G6w==",
+ "node_modules/@react-native-community/cli-clean": {
+ "version": "12.3.2",
+ "resolved": "https://registry.npmjs.org/@react-native-community/cli-clean/-/cli-clean-12.3.2.tgz",
+ "integrity": "sha512-90k2hCX0ddSFPT7EN7h5SZj0XZPXP0+y/++v262hssoey3nhurwF57NGWN0XAR0o9BSW7+mBfeInfabzDraO6A==",
"dependencies": {
- "path-key": "^3.1.0",
- "shebang-command": "^2.0.0",
- "which": "^2.0.1"
+ "@react-native-community/cli-tools": "12.3.2",
+ "chalk": "^4.1.2",
+ "execa": "^5.0.0"
+ }
+ },
+ "node_modules/@react-native-community/cli-clean/node_modules/chalk": {
+ "version": "4.1.2",
+ "resolved": "https://registry.npmjs.org/chalk/-/chalk-4.1.2.tgz",
+ "integrity": "sha512-oKnbhFyRIXpUuez8iBMmyEa4nbj4IOQyuhc/wy9kY7/WVPcwIO9VA668Pu8RkO7+0G76SLROeyw9CpQ061i4mA==",
+ "dependencies": {
+ "ansi-styles": "^4.1.0",
+ "supports-color": "^7.1.0"
},
"engines": {
- "node": ">= 8"
+ "node": ">=10"
+ },
+ "funding": {
+ "url": "https://github.com/chalk/chalk?sponsor=1"
}
},
- "node_modules/@react-native/community-cli-plugin/node_modules/execa": {
+ "node_modules/@react-native-community/cli-clean/node_modules/execa": {
"version": "5.1.1",
"resolved": "https://registry.npmjs.org/execa/-/execa-5.1.1.tgz",
"integrity": "sha512-8uSpZZocAZRBAPIEINJj3Lo9HyGitllczc27Eh5YYojjMFMn8yHMDMaUHE2Jqfq05D/wucwI4JGURyXt1vchyg==",
@@ -10124,7 +9038,7 @@
"url": "https://github.com/sindresorhus/execa?sponsor=1"
}
},
- "node_modules/@react-native/community-cli-plugin/node_modules/get-stream": {
+ "node_modules/@react-native-community/cli-clean/node_modules/get-stream": {
"version": "6.0.1",
"resolved": "https://registry.npmjs.org/get-stream/-/get-stream-6.0.1.tgz",
"integrity": "sha512-ts6Wi+2j3jQjqi70w5AlN8DFnkSwC+MqmxEzdEALB2qXZYV3X/b1CTfgPLGJNMeAWxdPfU8FO1ms3NUfaHCPYg==",
@@ -10135,7 +9049,15 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@react-native/community-cli-plugin/node_modules/human-signals": {
+ "node_modules/@react-native-community/cli-clean/node_modules/has-flag": {
+ "version": "4.0.0",
+ "resolved": "https://registry.npmjs.org/has-flag/-/has-flag-4.0.0.tgz",
+ "integrity": "sha512-EykJT/Q1KjTWctppgIAgfSO0tKVuZUjhgMr17kqTumMl6Afv3EISleU7qZUzoXDFTAHTDC4NOoG/ZxU3EvlMPQ==",
+ "engines": {
+ "node": ">=8"
+ }
+ },
+ "node_modules/@react-native-community/cli-clean/node_modules/human-signals": {
"version": "2.1.0",
"resolved": "https://registry.npmjs.org/human-signals/-/human-signals-2.1.0.tgz",
"integrity": "sha512-B4FFZ6q/T2jhhksgkbEW3HBvWIfDW85snkQgawt07S7J5QXTk6BkNV+0yAeZrM5QpMAdYlocGoljn0sJ/WQkFw==",
@@ -10143,7 +9065,7 @@
"node": ">=10.17.0"
}
},
- "node_modules/@react-native/community-cli-plugin/node_modules/is-stream": {
+ "node_modules/@react-native-community/cli-clean/node_modules/is-stream": {
"version": "2.0.1",
"resolved": "https://registry.npmjs.org/is-stream/-/is-stream-2.0.1.tgz",
"integrity": "sha512-hFoiJiTl63nn+kstHGBtewWSKnQLpyb155KHheA1l39uvtO9nWIop1p3udqPcUd/xbF1VLMO4n7OI6p7RbngDg==",
@@ -10154,7 +9076,7 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@react-native/community-cli-plugin/node_modules/mimic-fn": {
+ "node_modules/@react-native-community/cli-clean/node_modules/mimic-fn": {
"version": "2.1.0",
"resolved": "https://registry.npmjs.org/mimic-fn/-/mimic-fn-2.1.0.tgz",
"integrity": "sha512-OqbOk5oEQeAZ8WXWydlu9HJjz9WVdEIvamMCcXmuqUYjTknH/sqsWvhQ3vgwKFRR1HpjvNBKQ37nbJgYzGqGcg==",
@@ -10162,7 +9084,7 @@
"node": ">=6"
}
},
- "node_modules/@react-native/community-cli-plugin/node_modules/npm-run-path": {
+ "node_modules/@react-native-community/cli-clean/node_modules/npm-run-path": {
"version": "4.0.1",
"resolved": "https://registry.npmjs.org/npm-run-path/-/npm-run-path-4.0.1.tgz",
"integrity": "sha512-S48WzZW777zhNIrn7gxOlISNAqi9ZC/uQFnRdbeIHhZhCA6UqpkOT8T1G7BvfdgP4Er8gF4sUbaS0i7QvIfCWw==",
@@ -10173,7 +9095,7 @@
"node": ">=8"
}
},
- "node_modules/@react-native/community-cli-plugin/node_modules/onetime": {
+ "node_modules/@react-native-community/cli-clean/node_modules/onetime": {
"version": "5.1.2",
"resolved": "https://registry.npmjs.org/onetime/-/onetime-5.1.2.tgz",
"integrity": "sha512-kbpaSSGJTWdAY5KPVeMOKXSrPtr8C8C7wodJbcsd51jRnmD+GZu8Y0VoU6Dm5Z4vWr0Ig/1NKuWRKf7j5aaYSg==",
@@ -10187,7 +9109,7 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@react-native/community-cli-plugin/node_modules/path-key": {
+ "node_modules/@react-native-community/cli-clean/node_modules/path-key": {
"version": "3.1.1",
"resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
"integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
@@ -10195,340 +9117,467 @@
"node": ">=8"
}
},
- "node_modules/@react-native/community-cli-plugin/node_modules/shebang-command": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/shebang-command/-/shebang-command-2.0.0.tgz",
- "integrity": "sha512-kHxr2zZpYtdmrN1qDjrrX/Z1rR1kG8Dx+gkpK1G4eXmvXswmcE1hTWBWYUzlraYw1/yZp6YuDY77YtvbN0dmDA==",
+ "node_modules/@react-native-community/cli-clean/node_modules/supports-color": {
+ "version": "7.2.0",
+ "resolved": "https://registry.npmjs.org/supports-color/-/supports-color-7.2.0.tgz",
+ "integrity": "sha512-qpCAvRl9stuOHveKsn7HncJRvv501qIacKzQlO/+Lwxc9+0q2wLyv4Dfvt80/DPn2pqOBsJdDiogXGR9+OvwRw==",
"dependencies": {
- "shebang-regex": "^3.0.0"
+ "has-flag": "^4.0.0"
},
"engines": {
"node": ">=8"
}
},
- "node_modules/@react-native/community-cli-plugin/node_modules/shebang-regex": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/shebang-regex/-/shebang-regex-3.0.0.tgz",
- "integrity": "sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==",
+ "node_modules/@react-native-community/cli-config": {
+ "version": "12.3.2",
+ "resolved": "https://registry.npmjs.org/@react-native-community/cli-config/-/cli-config-12.3.2.tgz",
+ "integrity": "sha512-UUCzDjQgvAVL/57rL7eOuFUhd+d+6qfM7V8uOegQFeFEmSmvUUDLYoXpBa5vAK9JgQtSqMBJ1Shmwao+/oElxQ==",
+ "dependencies": {
+ "@react-native-community/cli-tools": "12.3.2",
+ "chalk": "^4.1.2",
+ "cosmiconfig": "^5.1.0",
+ "deepmerge": "^4.3.0",
+ "glob": "^7.1.3",
+ "joi": "^17.2.1"
+ }
+ },
+ "node_modules/@react-native-community/cli-config/node_modules/chalk": {
+ "version": "4.1.2",
+ "resolved": "https://registry.npmjs.org/chalk/-/chalk-4.1.2.tgz",
+ "integrity": "sha512-oKnbhFyRIXpUuez8iBMmyEa4nbj4IOQyuhc/wy9kY7/WVPcwIO9VA668Pu8RkO7+0G76SLROeyw9CpQ061i4mA==",
+ "dependencies": {
+ "ansi-styles": "^4.1.0",
+ "supports-color": "^7.1.0"
+ },
"engines": {
- "node": ">=8"
+ "node": ">=10"
+ },
+ "funding": {
+ "url": "https://github.com/chalk/chalk?sponsor=1"
}
},
- "node_modules/@react-native/community-cli-plugin/node_modules/which": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
- "integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
+ "node_modules/@react-native-community/cli-config/node_modules/cosmiconfig": {
+ "version": "5.2.1",
+ "resolved": "https://registry.npmjs.org/cosmiconfig/-/cosmiconfig-5.2.1.tgz",
+ "integrity": "sha512-H65gsXo1SKjf8zmrJ67eJk8aIRKV5ff2D4uKZIBZShbhGSpEmsQOPW/SKMKYhSTrqR7ufy6RP69rPogdaPh/kA==",
"dependencies": {
- "isexe": "^2.0.0"
+ "import-fresh": "^2.0.0",
+ "is-directory": "^0.3.1",
+ "js-yaml": "^3.13.1",
+ "parse-json": "^4.0.0"
},
- "bin": {
- "node-which": "bin/node-which"
+ "engines": {
+ "node": ">=4"
+ }
+ },
+ "node_modules/@react-native-community/cli-config/node_modules/glob": {
+ "version": "7.2.3",
+ "resolved": "https://registry.npmjs.org/glob/-/glob-7.2.3.tgz",
+ "integrity": "sha512-nFR0zLpU2YCaRxwoCJvL6UvCH2JFyFVIvwTLsIf21AuHlMskA1hhTdk+LlYJtOlYt9v6dvszD2BGRqBL+iQK9Q==",
+ "dependencies": {
+ "fs.realpath": "^1.0.0",
+ "inflight": "^1.0.4",
+ "inherits": "2",
+ "minimatch": "^3.1.1",
+ "once": "^1.3.0",
+ "path-is-absolute": "^1.0.0"
},
"engines": {
- "node": ">= 8"
+ "node": "*"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
}
},
- "node_modules/@react-native/debugger-frontend": {
- "version": "0.73.3",
- "resolved": "https://registry.npmjs.org/@react-native/debugger-frontend/-/debugger-frontend-0.73.3.tgz",
- "integrity": "sha512-RgEKnWuoo54dh7gQhV7kvzKhXZEhpF9LlMdZolyhGxHsBqZ2gXdibfDlfcARFFifPIiaZ3lXuOVVa4ei+uPgTw==",
+ "node_modules/@react-native-community/cli-config/node_modules/has-flag": {
+ "version": "4.0.0",
+ "resolved": "https://registry.npmjs.org/has-flag/-/has-flag-4.0.0.tgz",
+ "integrity": "sha512-EykJT/Q1KjTWctppgIAgfSO0tKVuZUjhgMr17kqTumMl6Afv3EISleU7qZUzoXDFTAHTDC4NOoG/ZxU3EvlMPQ==",
"engines": {
- "node": ">=18"
+ "node": ">=8"
}
},
- "node_modules/@react-native/dev-middleware": {
- "version": "0.73.7",
- "resolved": "https://registry.npmjs.org/@react-native/dev-middleware/-/dev-middleware-0.73.7.tgz",
- "integrity": "sha512-BZXpn+qKp/dNdr4+TkZxXDttfx8YobDh8MFHsMk9usouLm22pKgFIPkGBV0X8Do4LBkFNPGtrnsKkWk/yuUXKg==",
+ "node_modules/@react-native-community/cli-config/node_modules/import-fresh": {
+ "version": "2.0.0",
+ "resolved": "https://registry.npmjs.org/import-fresh/-/import-fresh-2.0.0.tgz",
+ "integrity": "sha512-eZ5H8rcgYazHbKC3PG4ClHNykCSxtAhxSSEM+2mb+7evD2CKF5V7c0dNum7AdpDh0ZdICwZY9sRSn8f+KH96sg==",
"dependencies": {
- "@isaacs/ttlcache": "^1.4.1",
- "@react-native/debugger-frontend": "0.73.3",
- "chrome-launcher": "^0.15.2",
- "chromium-edge-launcher": "^1.0.0",
- "connect": "^3.6.5",
- "debug": "^2.2.0",
- "node-fetch": "^2.2.0",
- "open": "^7.0.3",
- "serve-static": "^1.13.1",
- "temp-dir": "^2.0.0"
+ "caller-path": "^2.0.0",
+ "resolve-from": "^3.0.0"
},
"engines": {
- "node": ">=18"
+ "node": ">=4"
}
},
- "node_modules/@react-native/dev-middleware/node_modules/debug": {
- "version": "2.6.9",
- "resolved": "https://registry.npmjs.org/debug/-/debug-2.6.9.tgz",
- "integrity": "sha512-bC7ElrdJaJnPbAP+1EotYvqZsb3ecl5wi6Bfi6BJTUcNowp6cvspg0jXznRTKDjm/E7AdgFBVeAPVMNcKGsHMA==",
+ "node_modules/@react-native-community/cli-config/node_modules/parse-json": {
+ "version": "4.0.0",
+ "resolved": "https://registry.npmjs.org/parse-json/-/parse-json-4.0.0.tgz",
+ "integrity": "sha512-aOIos8bujGN93/8Ox/jPLh7RwVnPEysynVFE+fQZyg6jKELEHwzgKdLRFHUgXJL6kylijVSBC4BvN9OmsB48Rw==",
"dependencies": {
- "ms": "2.0.0"
+ "error-ex": "^1.3.1",
+ "json-parse-better-errors": "^1.0.1"
+ },
+ "engines": {
+ "node": ">=4"
}
},
- "node_modules/@react-native/dev-middleware/node_modules/is-wsl": {
- "version": "2.2.0",
- "resolved": "https://registry.npmjs.org/is-wsl/-/is-wsl-2.2.0.tgz",
- "integrity": "sha512-fKzAra0rGJUUBwGBgNkHZuToZcn+TtXHpeCgmkMJMMYx1sQDYaCSyjJBSCa2nH1DGm7s3n1oBnohoVTBaN7Lww==",
+ "node_modules/@react-native-community/cli-config/node_modules/supports-color": {
+ "version": "7.2.0",
+ "resolved": "https://registry.npmjs.org/supports-color/-/supports-color-7.2.0.tgz",
+ "integrity": "sha512-qpCAvRl9stuOHveKsn7HncJRvv501qIacKzQlO/+Lwxc9+0q2wLyv4Dfvt80/DPn2pqOBsJdDiogXGR9+OvwRw==",
"dependencies": {
- "is-docker": "^2.0.0"
+ "has-flag": "^4.0.0"
},
"engines": {
"node": ">=8"
}
},
- "node_modules/@react-native/dev-middleware/node_modules/open": {
- "version": "7.4.2",
- "resolved": "https://registry.npmjs.org/open/-/open-7.4.2.tgz",
- "integrity": "sha512-MVHddDVweXZF3awtlAS+6pgKLlm/JgxZ90+/NBurBoQctVOOB/zDdVjcyPzQ+0laDGbsWgrRkflI65sQeOgT9Q==",
+ "node_modules/@react-native-community/cli-debugger-ui": {
+ "version": "12.3.2",
+ "resolved": "https://registry.npmjs.org/@react-native-community/cli-debugger-ui/-/cli-debugger-ui-12.3.2.tgz",
+ "integrity": "sha512-nSWQUL+51J682DlfcC1bjkUbQbGvHCC25jpqTwHIjmmVjYCX1uHuhPSqQKgPNdvtfOkrkACxczd7kVMmetxY2Q==",
"dependencies": {
- "is-docker": "^2.0.0",
- "is-wsl": "^2.1.1"
+ "serve-static": "^1.13.1"
+ }
+ },
+ "node_modules/@react-native-community/cli-doctor": {
+ "version": "12.3.2",
+ "resolved": "https://registry.npmjs.org/@react-native-community/cli-doctor/-/cli-doctor-12.3.2.tgz",
+ "integrity": "sha512-GrAabdY4qtBX49knHFvEAdLtCjkmndjTeqhYO6BhsbAeKOtspcLT/0WRgdLIaKODRa61ADNB3K5Zm4dU0QrZOg==",
+ "dependencies": {
+ "@react-native-community/cli-config": "12.3.2",
+ "@react-native-community/cli-platform-android": "12.3.2",
+ "@react-native-community/cli-platform-ios": "12.3.2",
+ "@react-native-community/cli-tools": "12.3.2",
+ "chalk": "^4.1.2",
+ "command-exists": "^1.2.8",
+ "deepmerge": "^4.3.0",
+ "envinfo": "^7.10.0",
+ "execa": "^5.0.0",
+ "hermes-profile-transformer": "^0.0.6",
+ "ip": "^1.1.5",
+ "node-stream-zip": "^1.9.1",
+ "ora": "^5.4.1",
+ "semver": "^7.5.2",
+ "strip-ansi": "^5.2.0",
+ "wcwidth": "^1.0.1",
+ "yaml": "^2.2.1"
+ }
+ },
+ "node_modules/@react-native-community/cli-doctor/node_modules/chalk": {
+ "version": "4.1.2",
+ "resolved": "https://registry.npmjs.org/chalk/-/chalk-4.1.2.tgz",
+ "integrity": "sha512-oKnbhFyRIXpUuez8iBMmyEa4nbj4IOQyuhc/wy9kY7/WVPcwIO9VA668Pu8RkO7+0G76SLROeyw9CpQ061i4mA==",
+ "dependencies": {
+ "ansi-styles": "^4.1.0",
+ "supports-color": "^7.1.0"
+ },
+ "engines": {
+ "node": ">=10"
+ },
+ "funding": {
+ "url": "https://github.com/chalk/chalk?sponsor=1"
+ }
+ },
+ "node_modules/@react-native-community/cli-doctor/node_modules/cli-cursor": {
+ "version": "3.1.0",
+ "resolved": "https://registry.npmjs.org/cli-cursor/-/cli-cursor-3.1.0.tgz",
+ "integrity": "sha512-I/zHAwsKf9FqGoXM4WWRACob9+SNukZTd94DWF57E4toouRulbCxcUh6RKUEOQlYTHJnzkPMySvPNaaSLNfLZw==",
+ "dependencies": {
+ "restore-cursor": "^3.1.0"
},
"engines": {
"node": ">=8"
+ }
+ },
+ "node_modules/@react-native-community/cli-doctor/node_modules/execa": {
+ "version": "5.1.1",
+ "resolved": "https://registry.npmjs.org/execa/-/execa-5.1.1.tgz",
+ "integrity": "sha512-8uSpZZocAZRBAPIEINJj3Lo9HyGitllczc27Eh5YYojjMFMn8yHMDMaUHE2Jqfq05D/wucwI4JGURyXt1vchyg==",
+ "dependencies": {
+ "cross-spawn": "^7.0.3",
+ "get-stream": "^6.0.0",
+ "human-signals": "^2.1.0",
+ "is-stream": "^2.0.0",
+ "merge-stream": "^2.0.0",
+ "npm-run-path": "^4.0.1",
+ "onetime": "^5.1.2",
+ "signal-exit": "^3.0.3",
+ "strip-final-newline": "^2.0.0"
+ },
+ "engines": {
+ "node": ">=10"
+ },
+ "funding": {
+ "url": "https://github.com/sindresorhus/execa?sponsor=1"
+ }
+ },
+ "node_modules/@react-native-community/cli-doctor/node_modules/get-stream": {
+ "version": "6.0.1",
+ "resolved": "https://registry.npmjs.org/get-stream/-/get-stream-6.0.1.tgz",
+ "integrity": "sha512-ts6Wi+2j3jQjqi70w5AlN8DFnkSwC+MqmxEzdEALB2qXZYV3X/b1CTfgPLGJNMeAWxdPfU8FO1ms3NUfaHCPYg==",
+ "engines": {
+ "node": ">=10"
},
"funding": {
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@react-native/dev-middleware/node_modules/temp-dir": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/temp-dir/-/temp-dir-2.0.0.tgz",
- "integrity": "sha512-aoBAniQmmwtcKp/7BzsH8Cxzv8OL736p7v1ihGb5e9DJ9kTwGWHrQrVB5+lfVDzfGrdRzXch+ig7LHaY1JTOrg==",
+ "node_modules/@react-native-community/cli-doctor/node_modules/has-flag": {
+ "version": "4.0.0",
+ "resolved": "https://registry.npmjs.org/has-flag/-/has-flag-4.0.0.tgz",
+ "integrity": "sha512-EykJT/Q1KjTWctppgIAgfSO0tKVuZUjhgMr17kqTumMl6Afv3EISleU7qZUzoXDFTAHTDC4NOoG/ZxU3EvlMPQ==",
"engines": {
"node": ">=8"
}
},
- "node_modules/@react-native/gradle-plugin": {
- "version": "0.73.4",
- "resolved": "https://registry.npmjs.org/@react-native/gradle-plugin/-/gradle-plugin-0.73.4.tgz",
- "integrity": "sha512-PMDnbsZa+tD55Ug+W8CfqXiGoGneSSyrBZCMb5JfiB3AFST3Uj5e6lw8SgI/B6SKZF7lG0BhZ6YHZsRZ5MlXmg==",
+ "node_modules/@react-native-community/cli-doctor/node_modules/human-signals": {
+ "version": "2.1.0",
+ "resolved": "https://registry.npmjs.org/human-signals/-/human-signals-2.1.0.tgz",
+ "integrity": "sha512-B4FFZ6q/T2jhhksgkbEW3HBvWIfDW85snkQgawt07S7J5QXTk6BkNV+0yAeZrM5QpMAdYlocGoljn0sJ/WQkFw==",
"engines": {
- "node": ">=18"
+ "node": ">=10.17.0"
}
},
- "node_modules/@react-native/js-polyfills": {
- "version": "0.73.1",
- "resolved": "https://registry.npmjs.org/@react-native/js-polyfills/-/js-polyfills-0.73.1.tgz",
- "integrity": "sha512-ewMwGcumrilnF87H4jjrnvGZEaPFCAC4ebraEK+CurDDmwST/bIicI4hrOAv+0Z0F7DEK4O4H7r8q9vH7IbN4g==",
+ "node_modules/@react-native-community/cli-doctor/node_modules/is-stream": {
+ "version": "2.0.1",
+ "resolved": "https://registry.npmjs.org/is-stream/-/is-stream-2.0.1.tgz",
+ "integrity": "sha512-hFoiJiTl63nn+kstHGBtewWSKnQLpyb155KHheA1l39uvtO9nWIop1p3udqPcUd/xbF1VLMO4n7OI6p7RbngDg==",
"engines": {
- "node": ">=18"
+ "node": ">=8"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@react-native/metro-babel-transformer": {
- "version": "0.73.10",
- "resolved": "https://registry.npmjs.org/@react-native/metro-babel-transformer/-/metro-babel-transformer-0.73.10.tgz",
- "integrity": "sha512-g0q12s1VcrhI4ab9ZQzFHmvjkDW5PYdJd3LiyhIO3OAWLD7iNLWL5tJe9iA3n8rxbanmf1oauqlNY1UmkxhAvQ==",
- "dev": true,
+ "node_modules/@react-native-community/cli-doctor/node_modules/mimic-fn": {
+ "version": "2.1.0",
+ "resolved": "https://registry.npmjs.org/mimic-fn/-/mimic-fn-2.1.0.tgz",
+ "integrity": "sha512-OqbOk5oEQeAZ8WXWydlu9HJjz9WVdEIvamMCcXmuqUYjTknH/sqsWvhQ3vgwKFRR1HpjvNBKQ37nbJgYzGqGcg==",
+ "engines": {
+ "node": ">=6"
+ }
+ },
+ "node_modules/@react-native-community/cli-doctor/node_modules/npm-run-path": {
+ "version": "4.0.1",
+ "resolved": "https://registry.npmjs.org/npm-run-path/-/npm-run-path-4.0.1.tgz",
+ "integrity": "sha512-S48WzZW777zhNIrn7gxOlISNAqi9ZC/uQFnRdbeIHhZhCA6UqpkOT8T1G7BvfdgP4Er8gF4sUbaS0i7QvIfCWw==",
"dependencies": {
- "@babel/core": "^7.20.0",
- "@react-native/babel-preset": "*",
- "babel-preset-fbjs": "^3.4.0",
- "hermes-parser": "0.15.0",
- "nullthrows": "^1.1.1"
+ "path-key": "^3.0.0"
},
"engines": {
- "node": ">=18"
- },
- "peerDependencies": {
- "@babel/core": "*"
+ "node": ">=8"
}
},
- "node_modules/@react-native/metro-config": {
- "version": "0.73.4",
- "resolved": "https://registry.npmjs.org/@react-native/metro-config/-/metro-config-0.73.4.tgz",
- "integrity": "sha512-4IpWb9InOY23ssua6z/ho2B4uRqF4QaNHGg4aV3D/og5yiVF39GEm/REHU36i+KoHRO3GcB6DrI7N9KrcvgGBw==",
- "dev": true,
+ "node_modules/@react-native-community/cli-doctor/node_modules/onetime": {
+ "version": "5.1.2",
+ "resolved": "https://registry.npmjs.org/onetime/-/onetime-5.1.2.tgz",
+ "integrity": "sha512-kbpaSSGJTWdAY5KPVeMOKXSrPtr8C8C7wodJbcsd51jRnmD+GZu8Y0VoU6Dm5Z4vWr0Ig/1NKuWRKf7j5aaYSg==",
"dependencies": {
- "@react-native/js-polyfills": "0.73.1",
- "@react-native/metro-babel-transformer": "0.73.14",
- "metro-config": "^0.80.3",
- "metro-runtime": "^0.80.3"
+ "mimic-fn": "^2.1.0"
},
"engines": {
- "node": ">=18"
+ "node": ">=6"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@react-native/metro-config/node_modules/@react-native/babel-preset": {
- "version": "0.73.20",
- "resolved": "https://registry.npmjs.org/@react-native/babel-preset/-/babel-preset-0.73.20.tgz",
- "integrity": "sha512-fU9NqkusbfFq71l4BWQfqqD/lLcLC0MZ++UYgieA3j8lIEppJTLVauv2RwtD2yltBkjebgYEC5Rwvt1l0MUBXw==",
- "dev": true,
+ "node_modules/@react-native-community/cli-doctor/node_modules/ora": {
+ "version": "5.4.1",
+ "resolved": "https://registry.npmjs.org/ora/-/ora-5.4.1.tgz",
+ "integrity": "sha512-5b6Y85tPxZZ7QytO+BQzysW31HJku27cRIlkbAXaNx+BdcVi+LlRFmVXzeF6a7JCwJpyw5c4b+YSVImQIrBpuQ==",
"dependencies": {
- "@babel/core": "^7.20.0",
- "@babel/plugin-proposal-async-generator-functions": "^7.0.0",
- "@babel/plugin-proposal-class-properties": "^7.18.0",
- "@babel/plugin-proposal-export-default-from": "^7.0.0",
- "@babel/plugin-proposal-nullish-coalescing-operator": "^7.18.0",
- "@babel/plugin-proposal-numeric-separator": "^7.0.0",
- "@babel/plugin-proposal-object-rest-spread": "^7.20.0",
- "@babel/plugin-proposal-optional-catch-binding": "^7.0.0",
- "@babel/plugin-proposal-optional-chaining": "^7.20.0",
- "@babel/plugin-syntax-dynamic-import": "^7.8.0",
- "@babel/plugin-syntax-export-default-from": "^7.0.0",
- "@babel/plugin-syntax-flow": "^7.18.0",
- "@babel/plugin-syntax-nullish-coalescing-operator": "^7.0.0",
- "@babel/plugin-syntax-optional-chaining": "^7.0.0",
- "@babel/plugin-transform-arrow-functions": "^7.0.0",
- "@babel/plugin-transform-async-to-generator": "^7.20.0",
- "@babel/plugin-transform-block-scoping": "^7.0.0",
- "@babel/plugin-transform-classes": "^7.0.0",
- "@babel/plugin-transform-computed-properties": "^7.0.0",
- "@babel/plugin-transform-destructuring": "^7.20.0",
- "@babel/plugin-transform-flow-strip-types": "^7.20.0",
- "@babel/plugin-transform-function-name": "^7.0.0",
- "@babel/plugin-transform-literals": "^7.0.0",
- "@babel/plugin-transform-modules-commonjs": "^7.0.0",
- "@babel/plugin-transform-named-capturing-groups-regex": "^7.0.0",
- "@babel/plugin-transform-parameters": "^7.0.0",
- "@babel/plugin-transform-private-methods": "^7.22.5",
- "@babel/plugin-transform-private-property-in-object": "^7.22.11",
- "@babel/plugin-transform-react-display-name": "^7.0.0",
- "@babel/plugin-transform-react-jsx": "^7.0.0",
- "@babel/plugin-transform-react-jsx-self": "^7.0.0",
- "@babel/plugin-transform-react-jsx-source": "^7.0.0",
- "@babel/plugin-transform-runtime": "^7.0.0",
- "@babel/plugin-transform-shorthand-properties": "^7.0.0",
- "@babel/plugin-transform-spread": "^7.0.0",
- "@babel/plugin-transform-sticky-regex": "^7.0.0",
- "@babel/plugin-transform-typescript": "^7.5.0",
- "@babel/plugin-transform-unicode-regex": "^7.0.0",
- "@babel/template": "^7.0.0",
- "@react-native/babel-plugin-codegen": "0.73.3",
- "babel-plugin-transform-flow-enums": "^0.0.2",
- "react-refresh": "^0.14.0"
+ "bl": "^4.1.0",
+ "chalk": "^4.1.0",
+ "cli-cursor": "^3.1.0",
+ "cli-spinners": "^2.5.0",
+ "is-interactive": "^1.0.0",
+ "is-unicode-supported": "^0.1.0",
+ "log-symbols": "^4.1.0",
+ "strip-ansi": "^6.0.0",
+ "wcwidth": "^1.0.1"
},
"engines": {
- "node": ">=18"
+ "node": ">=10"
},
- "peerDependencies": {
- "@babel/core": "*"
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@react-native/metro-config/node_modules/@react-native/metro-babel-transformer": {
- "version": "0.73.14",
- "resolved": "https://registry.npmjs.org/@react-native/metro-babel-transformer/-/metro-babel-transformer-0.73.14.tgz",
- "integrity": "sha512-5wLeYw/lormpSqYfI9H/geZ/EtPmi+x5qLkEit15Q/70hkzYo/M+aWztUtbOITfgTEOP8d6ybROzoGsqgyZLcw==",
- "dev": true,
+ "node_modules/@react-native-community/cli-doctor/node_modules/ora/node_modules/strip-ansi": {
+ "version": "6.0.1",
+ "resolved": "https://registry.npmjs.org/strip-ansi/-/strip-ansi-6.0.1.tgz",
+ "integrity": "sha512-Y38VPSHcqkFrCpFnQ9vuSXmquuv5oXOKpGeT6aGrr3o3Gc9AlVa6JBfUSOCnbxGGZF+/0ooI7KrPuUSztUdU5A==",
"dependencies": {
- "@babel/core": "^7.20.0",
- "@react-native/babel-preset": "0.73.20",
- "hermes-parser": "0.15.0",
- "nullthrows": "^1.1.1"
+ "ansi-regex": "^5.0.1"
},
"engines": {
- "node": ">=18"
+ "node": ">=8"
+ }
+ },
+ "node_modules/@react-native-community/cli-doctor/node_modules/path-key": {
+ "version": "3.1.1",
+ "resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
+ "integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
+ "engines": {
+ "node": ">=8"
+ }
+ },
+ "node_modules/@react-native-community/cli-doctor/node_modules/restore-cursor": {
+ "version": "3.1.0",
+ "resolved": "https://registry.npmjs.org/restore-cursor/-/restore-cursor-3.1.0.tgz",
+ "integrity": "sha512-l+sSefzHpj5qimhFSE5a8nufZYAM3sBSVMAPtYkmC+4EH2anSGaEMXSD0izRQbu9nfyQ9y5JrVmp7E8oZrUjvA==",
+ "dependencies": {
+ "onetime": "^5.1.0",
+ "signal-exit": "^3.0.2"
},
- "peerDependencies": {
- "@babel/core": "*"
+ "engines": {
+ "node": ">=8"
}
},
- "node_modules/@react-native/normalize-color": {
- "version": "2.1.0",
- "resolved": "https://registry.npmjs.org/@react-native/normalize-color/-/normalize-color-2.1.0.tgz",
- "integrity": "sha512-Z1jQI2NpdFJCVgpY+8Dq/Bt3d+YUi1928Q+/CZm/oh66fzM0RUl54vvuXlPJKybH4pdCZey1eDTPaLHkMPNgWA=="
+ "node_modules/@react-native-community/cli-doctor/node_modules/strip-ansi": {
+ "version": "5.2.0",
+ "resolved": "https://registry.npmjs.org/strip-ansi/-/strip-ansi-5.2.0.tgz",
+ "integrity": "sha512-DuRs1gKbBqsMKIZlrffwlug8MHkcnpjs5VPmL1PAh+mA30U0DTotfDZ0d2UUsXpPmPmMMJ6W773MaA3J+lbiWA==",
+ "dependencies": {
+ "ansi-regex": "^4.1.0"
+ },
+ "engines": {
+ "node": ">=6"
+ }
},
- "node_modules/@react-native/normalize-colors": {
- "version": "0.73.2",
- "resolved": "https://registry.npmjs.org/@react-native/normalize-colors/-/normalize-colors-0.73.2.tgz",
- "integrity": "sha512-bRBcb2T+I88aG74LMVHaKms2p/T8aQd8+BZ7LuuzXlRfog1bMWWn/C5i0HVuvW4RPtXQYgIlGiXVDy9Ir1So/w=="
+ "node_modules/@react-native-community/cli-doctor/node_modules/strip-ansi/node_modules/ansi-regex": {
+ "version": "4.1.1",
+ "resolved": "https://registry.npmjs.org/ansi-regex/-/ansi-regex-4.1.1.tgz",
+ "integrity": "sha512-ILlv4k/3f6vfQ4OoP2AGvirOktlQ98ZEL1k9FaQjxa3L1abBgbuTDAdPOpvbGncC0BTVQrl+OM8xZGK6tWXt7g==",
+ "engines": {
+ "node": ">=6"
+ }
},
- "node_modules/@react-native/virtualized-lists": {
- "version": "0.73.4",
- "resolved": "https://registry.npmjs.org/@react-native/virtualized-lists/-/virtualized-lists-0.73.4.tgz",
- "integrity": "sha512-HpmLg1FrEiDtrtAbXiwCgXFYyloK/dOIPIuWW3fsqukwJEWAiTzm1nXGJ7xPU5XTHiWZ4sKup5Ebaj8z7iyWog==",
+ "node_modules/@react-native-community/cli-doctor/node_modules/supports-color": {
+ "version": "7.2.0",
+ "resolved": "https://registry.npmjs.org/supports-color/-/supports-color-7.2.0.tgz",
+ "integrity": "sha512-qpCAvRl9stuOHveKsn7HncJRvv501qIacKzQlO/+Lwxc9+0q2wLyv4Dfvt80/DPn2pqOBsJdDiogXGR9+OvwRw==",
"dependencies": {
- "invariant": "^2.2.4",
- "nullthrows": "^1.1.1"
+ "has-flag": "^4.0.0"
},
"engines": {
- "node": ">=18"
+ "node": ">=8"
+ }
+ },
+ "node_modules/@react-native-community/cli-doctor/node_modules/yaml": {
+ "version": "2.6.0",
+ "resolved": "https://registry.npmjs.org/yaml/-/yaml-2.6.0.tgz",
+ "integrity": "sha512-a6ae//JvKDEra2kdi1qzCyrJW/WZCgFi8ydDV+eXExl95t+5R+ijnqHJbz9tmMh8FUjx3iv2fCQ4dclAQlO2UQ==",
+ "license": "ISC",
+ "bin": {
+ "yaml": "bin.mjs"
},
- "peerDependencies": {
- "react-native": "*"
+ "engines": {
+ "node": ">= 14"
}
},
- "node_modules/@react-navigation/core": {
- "version": "5.12.0",
- "resolved": "https://registry.npmjs.org/@react-navigation/core/-/core-5.12.0.tgz",
- "integrity": "sha512-CTmYrFXCZwInN40CpEzkPxhrpzujj20qvsUgpH05+oO1flwsnaJsyBfYawIcTS62/1/Z6SAM7iW5PbKk+qw9iQ==",
+ "node_modules/@react-native-community/cli-hermes": {
+ "version": "12.3.2",
+ "resolved": "https://registry.npmjs.org/@react-native-community/cli-hermes/-/cli-hermes-12.3.2.tgz",
+ "integrity": "sha512-SL6F9O8ghp4ESBFH2YAPLtIN39jdnvGBKnK4FGKpDCjtB3DnUmDsGFlH46S+GGt5M6VzfG2eeKEOKf3pZ6jUzA==",
"dependencies": {
- "@react-navigation/routers": "^5.4.9",
- "escape-string-regexp": "^4.0.0",
- "nanoid": "^3.1.9",
- "query-string": "^6.13.1",
- "react-is": "^16.13.0",
- "use-subscription": "^1.4.0"
- },
- "peerDependencies": {
- "react": "*"
+ "@react-native-community/cli-platform-android": "12.3.2",
+ "@react-native-community/cli-tools": "12.3.2",
+ "chalk": "^4.1.2",
+ "hermes-profile-transformer": "^0.0.6",
+ "ip": "^1.1.5"
}
},
- "node_modules/@react-navigation/core/node_modules/escape-string-regexp": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/escape-string-regexp/-/escape-string-regexp-4.0.0.tgz",
- "integrity": "sha512-TtpcNJ3XAzx3Gq8sWRzJaVajRs0uVxA2YAkdb1jm2YkPz4G6egUFAyA3n5vtEIZefPk5Wa4UXbKuS5fKkJWdgA==",
+ "node_modules/@react-native-community/cli-hermes/node_modules/chalk": {
+ "version": "4.1.2",
+ "resolved": "https://registry.npmjs.org/chalk/-/chalk-4.1.2.tgz",
+ "integrity": "sha512-oKnbhFyRIXpUuez8iBMmyEa4nbj4IOQyuhc/wy9kY7/WVPcwIO9VA668Pu8RkO7+0G76SLROeyw9CpQ061i4mA==",
+ "dependencies": {
+ "ansi-styles": "^4.1.0",
+ "supports-color": "^7.1.0"
+ },
"engines": {
"node": ">=10"
},
"funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "url": "https://github.com/chalk/chalk?sponsor=1"
}
},
- "node_modules/@react-navigation/elements": {
- "version": "1.3.18",
- "resolved": "https://registry.npmjs.org/@react-navigation/elements/-/elements-1.3.18.tgz",
- "integrity": "sha512-/0hwnJkrr415yP0Hf4PjUKgGyfshrvNUKFXN85Mrt1gY49hy9IwxZgrrxlh0THXkPeq8q4VWw44eHDfAcQf20Q==",
- "peerDependencies": {
- "@react-navigation/native": "^6.0.0",
- "react": "*",
- "react-native": "*",
- "react-native-safe-area-context": ">= 3.0.0"
+ "node_modules/@react-native-community/cli-hermes/node_modules/has-flag": {
+ "version": "4.0.0",
+ "resolved": "https://registry.npmjs.org/has-flag/-/has-flag-4.0.0.tgz",
+ "integrity": "sha512-EykJT/Q1KjTWctppgIAgfSO0tKVuZUjhgMr17kqTumMl6Afv3EISleU7qZUzoXDFTAHTDC4NOoG/ZxU3EvlMPQ==",
+ "engines": {
+ "node": ">=8"
}
},
- "node_modules/@react-navigation/native": {
- "version": "6.0.14",
- "resolved": "https://registry.npmjs.org/@react-navigation/native/-/native-6.0.14.tgz",
- "integrity": "sha512-Z95bJrRkZerBJq6Qc/xjA/kibPpB+UvPeMWS1CBhRF8FaX1483UdHqPqSbW879tPwjP2R4XfoA4dtoEHswrOjA==",
+ "node_modules/@react-native-community/cli-hermes/node_modules/supports-color": {
+ "version": "7.2.0",
+ "resolved": "https://registry.npmjs.org/supports-color/-/supports-color-7.2.0.tgz",
+ "integrity": "sha512-qpCAvRl9stuOHveKsn7HncJRvv501qIacKzQlO/+Lwxc9+0q2wLyv4Dfvt80/DPn2pqOBsJdDiogXGR9+OvwRw==",
"dependencies": {
- "@react-navigation/core": "^6.4.1",
- "escape-string-regexp": "^4.0.0",
- "fast-deep-equal": "^3.1.3",
- "nanoid": "^3.1.23"
+ "has-flag": "^4.0.0"
},
- "peerDependencies": {
- "react": "*",
- "react-native": "*"
+ "engines": {
+ "node": ">=8"
}
},
- "node_modules/@react-navigation/native/node_modules/@react-navigation/core": {
- "version": "6.4.17",
- "resolved": "https://registry.npmjs.org/@react-navigation/core/-/core-6.4.17.tgz",
- "integrity": "sha512-Nd76EpomzChWAosGqWOYE3ItayhDzIEzzZsT7PfGcRFDgW5miHV2t4MZcq9YIK4tzxZjVVpYbIynOOQQd1e0Cg==",
- "license": "MIT",
+ "node_modules/@react-native-community/cli-platform-android": {
+ "version": "12.3.2",
+ "resolved": "https://registry.npmjs.org/@react-native-community/cli-platform-android/-/cli-platform-android-12.3.2.tgz",
+ "integrity": "sha512-MZ5nO8yi/N+Fj2i9BJcJ9C/ez+9/Ir7lQt49DWRo9YDmzye66mYLr/P2l/qxsixllbbDi7BXrlLpxaEhMrDopg==",
"dependencies": {
- "@react-navigation/routers": "^6.1.9",
- "escape-string-regexp": "^4.0.0",
- "nanoid": "^3.1.23",
- "query-string": "^7.1.3",
- "react-is": "^16.13.0",
- "use-latest-callback": "^0.2.1"
+ "@react-native-community/cli-tools": "12.3.2",
+ "chalk": "^4.1.2",
+ "execa": "^5.0.0",
+ "fast-xml-parser": "^4.2.4",
+ "glob": "^7.1.3",
+ "logkitty": "^0.7.1"
+ }
+ },
+ "node_modules/@react-native-community/cli-platform-android/node_modules/chalk": {
+ "version": "4.1.2",
+ "resolved": "https://registry.npmjs.org/chalk/-/chalk-4.1.2.tgz",
+ "integrity": "sha512-oKnbhFyRIXpUuez8iBMmyEa4nbj4IOQyuhc/wy9kY7/WVPcwIO9VA668Pu8RkO7+0G76SLROeyw9CpQ061i4mA==",
+ "dependencies": {
+ "ansi-styles": "^4.1.0",
+ "supports-color": "^7.1.0"
},
- "peerDependencies": {
- "react": "*"
+ "engines": {
+ "node": ">=10"
+ },
+ "funding": {
+ "url": "https://github.com/chalk/chalk?sponsor=1"
}
},
- "node_modules/@react-navigation/native/node_modules/@react-navigation/routers": {
- "version": "6.1.9",
- "resolved": "https://registry.npmjs.org/@react-navigation/routers/-/routers-6.1.9.tgz",
- "integrity": "sha512-lTM8gSFHSfkJvQkxacGM6VJtBt61ip2XO54aNfswD+KMw6eeZ4oehl7m0me3CR9hnDE4+60iAZR8sAhvCiI3NA==",
+ "node_modules/@react-native-community/cli-platform-android/node_modules/execa": {
+ "version": "5.1.1",
+ "resolved": "https://registry.npmjs.org/execa/-/execa-5.1.1.tgz",
+ "integrity": "sha512-8uSpZZocAZRBAPIEINJj3Lo9HyGitllczc27Eh5YYojjMFMn8yHMDMaUHE2Jqfq05D/wucwI4JGURyXt1vchyg==",
"dependencies": {
- "nanoid": "^3.1.23"
+ "cross-spawn": "^7.0.3",
+ "get-stream": "^6.0.0",
+ "human-signals": "^2.1.0",
+ "is-stream": "^2.0.0",
+ "merge-stream": "^2.0.0",
+ "npm-run-path": "^4.0.1",
+ "onetime": "^5.1.2",
+ "signal-exit": "^3.0.3",
+ "strip-final-newline": "^2.0.0"
+ },
+ "engines": {
+ "node": ">=10"
+ },
+ "funding": {
+ "url": "https://github.com/sindresorhus/execa?sponsor=1"
}
},
- "node_modules/@react-navigation/native/node_modules/escape-string-regexp": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/escape-string-regexp/-/escape-string-regexp-4.0.0.tgz",
- "integrity": "sha512-TtpcNJ3XAzx3Gq8sWRzJaVajRs0uVxA2YAkdb1jm2YkPz4G6egUFAyA3n5vtEIZefPk5Wa4UXbKuS5fKkJWdgA==",
+ "node_modules/@react-native-community/cli-platform-android/node_modules/get-stream": {
+ "version": "6.0.1",
+ "resolved": "https://registry.npmjs.org/get-stream/-/get-stream-6.0.1.tgz",
+ "integrity": "sha512-ts6Wi+2j3jQjqi70w5AlN8DFnkSwC+MqmxEzdEALB2qXZYV3X/b1CTfgPLGJNMeAWxdPfU8FO1ms3NUfaHCPYg==",
"engines": {
"node": ">=10"
},
@@ -10536,1095 +9585,985 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@react-navigation/native/node_modules/query-string": {
- "version": "7.1.3",
- "resolved": "https://registry.npmjs.org/query-string/-/query-string-7.1.3.tgz",
- "integrity": "sha512-hh2WYhq4fi8+b+/2Kg9CEge4fDPvHS534aOOvOZeQ3+Vf2mCFsaFBYj0i+iXcAq6I9Vzp5fjMFBlONvayDC1qg==",
+ "node_modules/@react-native-community/cli-platform-android/node_modules/glob": {
+ "version": "7.2.3",
+ "resolved": "https://registry.npmjs.org/glob/-/glob-7.2.3.tgz",
+ "integrity": "sha512-nFR0zLpU2YCaRxwoCJvL6UvCH2JFyFVIvwTLsIf21AuHlMskA1hhTdk+LlYJtOlYt9v6dvszD2BGRqBL+iQK9Q==",
"dependencies": {
- "decode-uri-component": "^0.2.2",
- "filter-obj": "^1.1.0",
- "split-on-first": "^1.0.0",
- "strict-uri-encode": "^2.0.0"
+ "fs.realpath": "^1.0.0",
+ "inflight": "^1.0.4",
+ "inherits": "2",
+ "minimatch": "^3.1.1",
+ "once": "^1.3.0",
+ "path-is-absolute": "^1.0.0"
},
"engines": {
- "node": ">=6"
+ "node": "*"
},
"funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "url": "https://github.com/sponsors/isaacs"
}
},
- "node_modules/@react-navigation/routers": {
- "version": "5.4.9",
- "resolved": "https://registry.npmjs.org/@react-navigation/routers/-/routers-5.4.9.tgz",
- "integrity": "sha512-dYD5qrIKUmuBEp+O98hB0tDYpEsGQgCQFQgMEoFKBmVVhx2JnJJ1zxRjU7xWcCU4VdBA8IOowgHQHJsVNKYyrg==",
- "dependencies": {
- "nanoid": "^3.1.9"
+ "node_modules/@react-native-community/cli-platform-android/node_modules/has-flag": {
+ "version": "4.0.0",
+ "resolved": "https://registry.npmjs.org/has-flag/-/has-flag-4.0.0.tgz",
+ "integrity": "sha512-EykJT/Q1KjTWctppgIAgfSO0tKVuZUjhgMr17kqTumMl6Afv3EISleU7qZUzoXDFTAHTDC4NOoG/ZxU3EvlMPQ==",
+ "engines": {
+ "node": ">=8"
}
},
- "node_modules/@react-navigation/stack": {
- "version": "6.3.5",
- "resolved": "https://registry.npmjs.org/@react-navigation/stack/-/stack-6.3.5.tgz",
- "integrity": "sha512-G706Ow+8fhiLT5Cf48566YsjboU5h5ll9nva90xX3br7V6x0JMoGMvXjvbORZyzLuUytQO3mf0T3nVUuO52vZQ==",
- "dependencies": {
- "@react-navigation/elements": "^1.3.7",
- "color": "^4.2.3",
- "warn-once": "^0.1.0"
+ "node_modules/@react-native-community/cli-platform-android/node_modules/human-signals": {
+ "version": "2.1.0",
+ "resolved": "https://registry.npmjs.org/human-signals/-/human-signals-2.1.0.tgz",
+ "integrity": "sha512-B4FFZ6q/T2jhhksgkbEW3HBvWIfDW85snkQgawt07S7J5QXTk6BkNV+0yAeZrM5QpMAdYlocGoljn0sJ/WQkFw==",
+ "engines": {
+ "node": ">=10.17.0"
+ }
+ },
+ "node_modules/@react-native-community/cli-platform-android/node_modules/is-stream": {
+ "version": "2.0.1",
+ "resolved": "https://registry.npmjs.org/is-stream/-/is-stream-2.0.1.tgz",
+ "integrity": "sha512-hFoiJiTl63nn+kstHGBtewWSKnQLpyb155KHheA1l39uvtO9nWIop1p3udqPcUd/xbF1VLMO4n7OI6p7RbngDg==",
+ "engines": {
+ "node": ">=8"
},
- "peerDependencies": {
- "@react-navigation/native": "^6.0.0",
- "react": "*",
- "react-native": "*",
- "react-native-gesture-handler": ">= 1.0.0",
- "react-native-safe-area-context": ">= 3.0.0",
- "react-native-screens": ">= 3.0.0"
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@react-spring/animated": {
- "version": "9.5.5",
- "resolved": "https://registry.npmjs.org/@react-spring/animated/-/animated-9.5.5.tgz",
- "integrity": "sha512-glzViz7syQ3CE6BQOwAyr75cgh0qsihm5lkaf24I0DfU63cMm/3+br299UEYkuaHNmfDfM414uktiPlZCNJbQA==",
+ "node_modules/@react-native-community/cli-platform-android/node_modules/mimic-fn": {
+ "version": "2.1.0",
+ "resolved": "https://registry.npmjs.org/mimic-fn/-/mimic-fn-2.1.0.tgz",
+ "integrity": "sha512-OqbOk5oEQeAZ8WXWydlu9HJjz9WVdEIvamMCcXmuqUYjTknH/sqsWvhQ3vgwKFRR1HpjvNBKQ37nbJgYzGqGcg==",
+ "engines": {
+ "node": ">=6"
+ }
+ },
+ "node_modules/@react-native-community/cli-platform-android/node_modules/npm-run-path": {
+ "version": "4.0.1",
+ "resolved": "https://registry.npmjs.org/npm-run-path/-/npm-run-path-4.0.1.tgz",
+ "integrity": "sha512-S48WzZW777zhNIrn7gxOlISNAqi9ZC/uQFnRdbeIHhZhCA6UqpkOT8T1G7BvfdgP4Er8gF4sUbaS0i7QvIfCWw==",
"dependencies": {
- "@react-spring/shared": "~9.5.5",
- "@react-spring/types": "~9.5.5"
+ "path-key": "^3.0.0"
},
- "peerDependencies": {
- "react": "^16.8.0 || ^17.0.0 || ^18.0.0"
+ "engines": {
+ "node": ">=8"
}
},
- "node_modules/@react-spring/core": {
- "version": "9.5.5",
- "resolved": "https://registry.npmjs.org/@react-spring/core/-/core-9.5.5.tgz",
- "integrity": "sha512-shaJYb3iX18Au6gkk8ahaF0qx0LpS0Yd+ajb4asBaAQf6WPGuEdJsbsNSgei1/O13JyEATsJl20lkjeslJPMYA==",
+ "node_modules/@react-native-community/cli-platform-android/node_modules/onetime": {
+ "version": "5.1.2",
+ "resolved": "https://registry.npmjs.org/onetime/-/onetime-5.1.2.tgz",
+ "integrity": "sha512-kbpaSSGJTWdAY5KPVeMOKXSrPtr8C8C7wodJbcsd51jRnmD+GZu8Y0VoU6Dm5Z4vWr0Ig/1NKuWRKf7j5aaYSg==",
"dependencies": {
- "@react-spring/animated": "~9.5.5",
- "@react-spring/rafz": "~9.5.5",
- "@react-spring/shared": "~9.5.5",
- "@react-spring/types": "~9.5.5"
+ "mimic-fn": "^2.1.0"
},
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/react-spring/donate"
+ "engines": {
+ "node": ">=6"
},
- "peerDependencies": {
- "react": "^16.8.0 || ^17.0.0 || ^18.0.0"
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@react-spring/rafz": {
- "version": "9.5.5",
- "resolved": "https://registry.npmjs.org/@react-spring/rafz/-/rafz-9.5.5.tgz",
- "integrity": "sha512-F/CLwB0d10jL6My5vgzRQxCNY2RNyDJZedRBK7FsngdCmzoq3V4OqqNc/9voJb9qRC2wd55oGXUeXv2eIaFmsw=="
+ "node_modules/@react-native-community/cli-platform-android/node_modules/path-key": {
+ "version": "3.1.1",
+ "resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
+ "integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
+ "engines": {
+ "node": ">=8"
+ }
},
- "node_modules/@react-spring/shared": {
- "version": "9.5.5",
- "resolved": "https://registry.npmjs.org/@react-spring/shared/-/shared-9.5.5.tgz",
- "integrity": "sha512-YwW70Pa/YXPOwTutExHZmMQSHcNC90kJOnNR4G4mCDNV99hE98jWkIPDOsgqbYx3amIglcFPiYKMaQuGdr8dyQ==",
+ "node_modules/@react-native-community/cli-platform-android/node_modules/supports-color": {
+ "version": "7.2.0",
+ "resolved": "https://registry.npmjs.org/supports-color/-/supports-color-7.2.0.tgz",
+ "integrity": "sha512-qpCAvRl9stuOHveKsn7HncJRvv501qIacKzQlO/+Lwxc9+0q2wLyv4Dfvt80/DPn2pqOBsJdDiogXGR9+OvwRw==",
"dependencies": {
- "@react-spring/rafz": "~9.5.5",
- "@react-spring/types": "~9.5.5"
+ "has-flag": "^4.0.0"
},
- "peerDependencies": {
- "react": "^16.8.0 || ^17.0.0 || ^18.0.0"
+ "engines": {
+ "node": ">=8"
}
},
- "node_modules/@react-spring/types": {
- "version": "9.5.5",
- "resolved": "https://registry.npmjs.org/@react-spring/types/-/types-9.5.5.tgz",
- "integrity": "sha512-7I/qY8H7Enwasxr4jU6WmtNK+RZ4Z/XvSlDvjXFVe7ii1x0MoSlkw6pD7xuac8qrHQRm9BTcbZNyeeKApYsvCg=="
- },
- "node_modules/@react-spring/web": {
- "version": "9.5.5",
- "resolved": "https://registry.npmjs.org/@react-spring/web/-/web-9.5.5.tgz",
- "integrity": "sha512-+moT8aDX/ho/XAhU+HRY9m0LVV9y9CK6NjSRaI+30Re150pB3iEip6QfnF4qnhSCQ5drpMF0XRXHgOTY/xbtFw==",
+ "node_modules/@react-native-community/cli-platform-ios": {
+ "version": "12.3.2",
+ "resolved": "https://registry.npmjs.org/@react-native-community/cli-platform-ios/-/cli-platform-ios-12.3.2.tgz",
+ "integrity": "sha512-OcWEAbkev1IL6SUiQnM6DQdsvfsKZhRZtoBNSj9MfdmwotVZSOEZJ+IjZ1FR9ChvMWayO9ns/o8LgoQxr1ZXeg==",
"dependencies": {
- "@react-spring/animated": "~9.5.5",
- "@react-spring/core": "~9.5.5",
- "@react-spring/shared": "~9.5.5",
- "@react-spring/types": "~9.5.5"
- },
- "peerDependencies": {
- "react": "^16.8.0 || ^17.0.0 || ^18.0.0",
- "react-dom": "^16.8.0 || ^17.0.0 || ^18.0.0"
+ "@react-native-community/cli-tools": "12.3.2",
+ "chalk": "^4.1.2",
+ "execa": "^5.0.0",
+ "fast-xml-parser": "^4.0.12",
+ "glob": "^7.1.3",
+ "ora": "^5.4.1"
}
},
- "node_modules/@samverschueren/stream-to-observable": {
- "version": "0.3.1",
- "resolved": "https://registry.npmjs.org/@samverschueren/stream-to-observable/-/stream-to-observable-0.3.1.tgz",
- "integrity": "sha512-c/qwwcHyafOQuVQJj0IlBjf5yYgBI7YPJ77k4fOJYesb41jio65eaJODRUmfYKhTOFBrIZ66kgvGPlNbjuoRdQ==",
- "dev": true,
+ "node_modules/@react-native-community/cli-platform-ios/node_modules/chalk": {
+ "version": "4.1.2",
+ "resolved": "https://registry.npmjs.org/chalk/-/chalk-4.1.2.tgz",
+ "integrity": "sha512-oKnbhFyRIXpUuez8iBMmyEa4nbj4IOQyuhc/wy9kY7/WVPcwIO9VA668Pu8RkO7+0G76SLROeyw9CpQ061i4mA==",
"dependencies": {
- "any-observable": "^0.3.0"
+ "ansi-styles": "^4.1.0",
+ "supports-color": "^7.1.0"
},
"engines": {
- "node": ">=6"
+ "node": ">=10"
},
- "peerDependenciesMeta": {
- "rxjs": {
- "optional": true
- },
- "zen-observable": {
- "optional": true
- }
+ "funding": {
+ "url": "https://github.com/chalk/chalk?sponsor=1"
}
},
- "node_modules/@sentry/core": {
- "version": "6.19.7",
- "resolved": "https://registry.npmjs.org/@sentry/core/-/core-6.19.7.tgz",
- "integrity": "sha512-tOfZ/umqB2AcHPGbIrsFLcvApdTm9ggpi/kQZFkej7kMphjT+SGBiQfYtjyg9jcRW+ilAR4JXC9BGKsdEQ+8Vw==",
+ "node_modules/@react-native-community/cli-platform-ios/node_modules/cli-cursor": {
+ "version": "3.1.0",
+ "resolved": "https://registry.npmjs.org/cli-cursor/-/cli-cursor-3.1.0.tgz",
+ "integrity": "sha512-I/zHAwsKf9FqGoXM4WWRACob9+SNukZTd94DWF57E4toouRulbCxcUh6RKUEOQlYTHJnzkPMySvPNaaSLNfLZw==",
"dependencies": {
- "@sentry/hub": "6.19.7",
- "@sentry/minimal": "6.19.7",
- "@sentry/types": "6.19.7",
- "@sentry/utils": "6.19.7",
- "tslib": "^1.9.3"
+ "restore-cursor": "^3.1.0"
},
"engines": {
- "node": ">=6"
+ "node": ">=8"
}
},
- "node_modules/@sentry/core/node_modules/tslib": {
- "version": "1.14.1",
- "resolved": "https://registry.npmjs.org/tslib/-/tslib-1.14.1.tgz",
- "integrity": "sha512-Xni35NKzjgMrwevysHTCArtLDpPvye8zV/0E4EyYn43P7/7qvQwPh9BGkHewbMulVntbigmcT7rdX3BNo9wRJg=="
- },
- "node_modules/@sentry/hub": {
- "version": "6.19.7",
- "resolved": "https://registry.npmjs.org/@sentry/hub/-/hub-6.19.7.tgz",
- "integrity": "sha512-y3OtbYFAqKHCWezF0EGGr5lcyI2KbaXW2Ik7Xp8Mu9TxbSTuwTe4rTntwg8ngPjUQU3SUHzgjqVB8qjiGqFXCA==",
+ "node_modules/@react-native-community/cli-platform-ios/node_modules/execa": {
+ "version": "5.1.1",
+ "resolved": "https://registry.npmjs.org/execa/-/execa-5.1.1.tgz",
+ "integrity": "sha512-8uSpZZocAZRBAPIEINJj3Lo9HyGitllczc27Eh5YYojjMFMn8yHMDMaUHE2Jqfq05D/wucwI4JGURyXt1vchyg==",
"dependencies": {
- "@sentry/types": "6.19.7",
- "@sentry/utils": "6.19.7",
- "tslib": "^1.9.3"
+ "cross-spawn": "^7.0.3",
+ "get-stream": "^6.0.0",
+ "human-signals": "^2.1.0",
+ "is-stream": "^2.0.0",
+ "merge-stream": "^2.0.0",
+ "npm-run-path": "^4.0.1",
+ "onetime": "^5.1.2",
+ "signal-exit": "^3.0.3",
+ "strip-final-newline": "^2.0.0"
},
"engines": {
- "node": ">=6"
+ "node": ">=10"
+ },
+ "funding": {
+ "url": "https://github.com/sindresorhus/execa?sponsor=1"
}
},
- "node_modules/@sentry/hub/node_modules/tslib": {
- "version": "1.14.1",
- "resolved": "https://registry.npmjs.org/tslib/-/tslib-1.14.1.tgz",
- "integrity": "sha512-Xni35NKzjgMrwevysHTCArtLDpPvye8zV/0E4EyYn43P7/7qvQwPh9BGkHewbMulVntbigmcT7rdX3BNo9wRJg=="
- },
- "node_modules/@sentry/minimal": {
- "version": "6.19.7",
- "resolved": "https://registry.npmjs.org/@sentry/minimal/-/minimal-6.19.7.tgz",
- "integrity": "sha512-wcYmSJOdvk6VAPx8IcmZgN08XTXRwRtB1aOLZm+MVHjIZIhHoBGZJYTVQS/BWjldsamj2cX3YGbGXNunaCfYJQ==",
- "dependencies": {
- "@sentry/hub": "6.19.7",
- "@sentry/types": "6.19.7",
- "tslib": "^1.9.3"
- },
+ "node_modules/@react-native-community/cli-platform-ios/node_modules/get-stream": {
+ "version": "6.0.1",
+ "resolved": "https://registry.npmjs.org/get-stream/-/get-stream-6.0.1.tgz",
+ "integrity": "sha512-ts6Wi+2j3jQjqi70w5AlN8DFnkSwC+MqmxEzdEALB2qXZYV3X/b1CTfgPLGJNMeAWxdPfU8FO1ms3NUfaHCPYg==",
"engines": {
- "node": ">=6"
+ "node": ">=10"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@sentry/minimal/node_modules/tslib": {
- "version": "1.14.1",
- "resolved": "https://registry.npmjs.org/tslib/-/tslib-1.14.1.tgz",
- "integrity": "sha512-Xni35NKzjgMrwevysHTCArtLDpPvye8zV/0E4EyYn43P7/7qvQwPh9BGkHewbMulVntbigmcT7rdX3BNo9wRJg=="
- },
- "node_modules/@sentry/node": {
- "version": "6.19.7",
- "resolved": "https://registry.npmjs.org/@sentry/node/-/node-6.19.7.tgz",
- "integrity": "sha512-gtmRC4dAXKODMpHXKfrkfvyBL3cI8y64vEi3fDD046uqYcrWdgoQsffuBbxMAizc6Ez1ia+f0Flue6p15Qaltg==",
+ "node_modules/@react-native-community/cli-platform-ios/node_modules/glob": {
+ "version": "7.2.3",
+ "resolved": "https://registry.npmjs.org/glob/-/glob-7.2.3.tgz",
+ "integrity": "sha512-nFR0zLpU2YCaRxwoCJvL6UvCH2JFyFVIvwTLsIf21AuHlMskA1hhTdk+LlYJtOlYt9v6dvszD2BGRqBL+iQK9Q==",
"dependencies": {
- "@sentry/core": "6.19.7",
- "@sentry/hub": "6.19.7",
- "@sentry/types": "6.19.7",
- "@sentry/utils": "6.19.7",
- "cookie": "^0.4.1",
- "https-proxy-agent": "^5.0.0",
- "lru_map": "^0.3.3",
- "tslib": "^1.9.3"
+ "fs.realpath": "^1.0.0",
+ "inflight": "^1.0.4",
+ "inherits": "2",
+ "minimatch": "^3.1.1",
+ "once": "^1.3.0",
+ "path-is-absolute": "^1.0.0"
},
"engines": {
- "node": ">=6"
+ "node": "*"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
}
},
- "node_modules/@sentry/node/node_modules/cookie": {
- "version": "0.4.2",
- "resolved": "https://registry.npmjs.org/cookie/-/cookie-0.4.2.tgz",
- "integrity": "sha512-aSWTXFzaKWkvHO1Ny/s+ePFpvKsPnjc551iI41v3ny/ow6tBG5Vd+FuqGNhh1LxOmVzOlGUriIlOaokOvhaStA==",
+ "node_modules/@react-native-community/cli-platform-ios/node_modules/has-flag": {
+ "version": "4.0.0",
+ "resolved": "https://registry.npmjs.org/has-flag/-/has-flag-4.0.0.tgz",
+ "integrity": "sha512-EykJT/Q1KjTWctppgIAgfSO0tKVuZUjhgMr17kqTumMl6Afv3EISleU7qZUzoXDFTAHTDC4NOoG/ZxU3EvlMPQ==",
"engines": {
- "node": ">= 0.6"
+ "node": ">=8"
}
},
- "node_modules/@sentry/node/node_modules/tslib": {
- "version": "1.14.1",
- "resolved": "https://registry.npmjs.org/tslib/-/tslib-1.14.1.tgz",
- "integrity": "sha512-Xni35NKzjgMrwevysHTCArtLDpPvye8zV/0E4EyYn43P7/7qvQwPh9BGkHewbMulVntbigmcT7rdX3BNo9wRJg=="
- },
- "node_modules/@sentry/types": {
- "version": "6.19.7",
- "resolved": "https://registry.npmjs.org/@sentry/types/-/types-6.19.7.tgz",
- "integrity": "sha512-jH84pDYE+hHIbVnab3Hr+ZXr1v8QABfhx39KknxqKWr2l0oEItzepV0URvbEhB446lk/S/59230dlUUIBGsXbg==",
+ "node_modules/@react-native-community/cli-platform-ios/node_modules/human-signals": {
+ "version": "2.1.0",
+ "resolved": "https://registry.npmjs.org/human-signals/-/human-signals-2.1.0.tgz",
+ "integrity": "sha512-B4FFZ6q/T2jhhksgkbEW3HBvWIfDW85snkQgawt07S7J5QXTk6BkNV+0yAeZrM5QpMAdYlocGoljn0sJ/WQkFw==",
"engines": {
- "node": ">=6"
+ "node": ">=10.17.0"
}
},
- "node_modules/@sentry/utils": {
- "version": "6.19.7",
- "resolved": "https://registry.npmjs.org/@sentry/utils/-/utils-6.19.7.tgz",
- "integrity": "sha512-z95ECmE3i9pbWoXQrD/7PgkBAzJYR+iXtPuTkpBjDKs86O3mT+PXOT3BAn79w2wkn7/i3vOGD2xVr1uiMl26dA==",
- "dependencies": {
- "@sentry/types": "6.19.7",
- "tslib": "^1.9.3"
+ "node_modules/@react-native-community/cli-platform-ios/node_modules/is-stream": {
+ "version": "2.0.1",
+ "resolved": "https://registry.npmjs.org/is-stream/-/is-stream-2.0.1.tgz",
+ "integrity": "sha512-hFoiJiTl63nn+kstHGBtewWSKnQLpyb155KHheA1l39uvtO9nWIop1p3udqPcUd/xbF1VLMO4n7OI6p7RbngDg==",
+ "engines": {
+ "node": ">=8"
},
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
+ }
+ },
+ "node_modules/@react-native-community/cli-platform-ios/node_modules/mimic-fn": {
+ "version": "2.1.0",
+ "resolved": "https://registry.npmjs.org/mimic-fn/-/mimic-fn-2.1.0.tgz",
+ "integrity": "sha512-OqbOk5oEQeAZ8WXWydlu9HJjz9WVdEIvamMCcXmuqUYjTknH/sqsWvhQ3vgwKFRR1HpjvNBKQ37nbJgYzGqGcg==",
"engines": {
"node": ">=6"
}
},
- "node_modules/@sentry/utils/node_modules/tslib": {
- "version": "1.14.1",
- "resolved": "https://registry.npmjs.org/tslib/-/tslib-1.14.1.tgz",
- "integrity": "sha512-Xni35NKzjgMrwevysHTCArtLDpPvye8zV/0E4EyYn43P7/7qvQwPh9BGkHewbMulVntbigmcT7rdX3BNo9wRJg=="
- },
- "node_modules/@sideway/address": {
- "version": "4.1.5",
- "resolved": "https://registry.npmjs.org/@sideway/address/-/address-4.1.5.tgz",
- "integrity": "sha512-IqO/DUQHUkPeixNQ8n0JA6102hT9CmaljNTPmQ1u8MEhBo/R4Q8eKLN/vGZxuebwOroDB4cbpjheD4+/sKFK4Q==",
+ "node_modules/@react-native-community/cli-platform-ios/node_modules/npm-run-path": {
+ "version": "4.0.1",
+ "resolved": "https://registry.npmjs.org/npm-run-path/-/npm-run-path-4.0.1.tgz",
+ "integrity": "sha512-S48WzZW777zhNIrn7gxOlISNAqi9ZC/uQFnRdbeIHhZhCA6UqpkOT8T1G7BvfdgP4Er8gF4sUbaS0i7QvIfCWw==",
"dependencies": {
- "@hapi/hoek": "^9.0.0"
+ "path-key": "^3.0.0"
+ },
+ "engines": {
+ "node": ">=8"
}
},
- "node_modules/@sideway/formula": {
- "version": "3.0.1",
- "resolved": "https://registry.npmjs.org/@sideway/formula/-/formula-3.0.1.tgz",
- "integrity": "sha512-/poHZJJVjx3L+zVD6g9KgHfYnb443oi7wLu/XKojDviHy6HOEOA6z1Trk5aR1dGcmPenJEgb2sK2I80LeS3MIg=="
- },
- "node_modules/@sideway/pinpoint": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/@sideway/pinpoint/-/pinpoint-2.0.0.tgz",
- "integrity": "sha512-RNiOoTPkptFtSVzQevY/yWtZwf/RxyVnPy/OcA9HBM3MlGDnBEYL5B41H0MTn0Uec8Hi+2qUtTfG2WWZBmMejQ=="
- },
- "node_modules/@sidvind/better-ajv-errors": {
- "version": "2.1.0",
- "resolved": "https://registry.npmjs.org/@sidvind/better-ajv-errors/-/better-ajv-errors-2.1.0.tgz",
- "integrity": "sha512-JuIb009FhHuL9priFBho2kv7QmZOydj0LgYvj+h1t0mMCmhM/YmQNRlJR5wVtBZya6wrVFK5Hi5TIbv5BKEx7w==",
- "dev": true,
+ "node_modules/@react-native-community/cli-platform-ios/node_modules/onetime": {
+ "version": "5.1.2",
+ "resolved": "https://registry.npmjs.org/onetime/-/onetime-5.1.2.tgz",
+ "integrity": "sha512-kbpaSSGJTWdAY5KPVeMOKXSrPtr8C8C7wodJbcsd51jRnmD+GZu8Y0VoU6Dm5Z4vWr0Ig/1NKuWRKf7j5aaYSg==",
"dependencies": {
- "@babel/code-frame": "^7.16.0",
- "chalk": "^4.1.0"
+ "mimic-fn": "^2.1.0"
},
"engines": {
- "node": ">= 14.0.0"
+ "node": ">=6"
},
- "peerDependencies": {
- "ajv": "4.11.8 - 8"
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@sigstore/bundle": {
- "version": "1.0.0",
- "resolved": "https://registry.npmjs.org/@sigstore/bundle/-/bundle-1.0.0.tgz",
- "integrity": "sha512-yLvrWDOh6uMOUlFCTJIZEnwOT9Xte7NPXUqVexEKGSF5XtBAuSg5du0kn3dRR0p47a4ah10Y0mNt8+uyeQXrBQ==",
- "dev": true,
+ "node_modules/@react-native-community/cli-platform-ios/node_modules/ora": {
+ "version": "5.4.1",
+ "resolved": "https://registry.npmjs.org/ora/-/ora-5.4.1.tgz",
+ "integrity": "sha512-5b6Y85tPxZZ7QytO+BQzysW31HJku27cRIlkbAXaNx+BdcVi+LlRFmVXzeF6a7JCwJpyw5c4b+YSVImQIrBpuQ==",
"dependencies": {
- "@sigstore/protobuf-specs": "^0.2.0"
+ "bl": "^4.1.0",
+ "chalk": "^4.1.0",
+ "cli-cursor": "^3.1.0",
+ "cli-spinners": "^2.5.0",
+ "is-interactive": "^1.0.0",
+ "is-unicode-supported": "^0.1.0",
+ "log-symbols": "^4.1.0",
+ "strip-ansi": "^6.0.0",
+ "wcwidth": "^1.0.1"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": ">=10"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@sigstore/protobuf-specs": {
- "version": "0.2.0",
- "resolved": "https://registry.npmjs.org/@sigstore/protobuf-specs/-/protobuf-specs-0.2.0.tgz",
- "integrity": "sha512-8ZhZKAVfXjIspDWwm3D3Kvj0ddbJ0HqDZ/pOs5cx88HpT8mVsotFrg7H1UMnXOuDHz6Zykwxn4mxG3QLuN+RUg==",
- "dev": true,
+ "node_modules/@react-native-community/cli-platform-ios/node_modules/path-key": {
+ "version": "3.1.1",
+ "resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
+ "integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": ">=8"
}
},
- "node_modules/@sigstore/tuf": {
- "version": "1.0.3",
- "resolved": "https://registry.npmjs.org/@sigstore/tuf/-/tuf-1.0.3.tgz",
- "integrity": "sha512-2bRovzs0nJZFlCN3rXirE4gwxCn97JNjMmwpecqlbgV9WcxX7WRuIrgzx/X7Ib7MYRbyUTpBYE0s2x6AmZXnlg==",
- "dev": true,
+ "node_modules/@react-native-community/cli-platform-ios/node_modules/restore-cursor": {
+ "version": "3.1.0",
+ "resolved": "https://registry.npmjs.org/restore-cursor/-/restore-cursor-3.1.0.tgz",
+ "integrity": "sha512-l+sSefzHpj5qimhFSE5a8nufZYAM3sBSVMAPtYkmC+4EH2anSGaEMXSD0izRQbu9nfyQ9y5JrVmp7E8oZrUjvA==",
"dependencies": {
- "@sigstore/protobuf-specs": "^0.2.0",
- "tuf-js": "^1.1.7"
+ "onetime": "^5.1.0",
+ "signal-exit": "^3.0.2"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
- }
- },
- "node_modules/@sinclair/typebox": {
- "version": "0.27.8",
- "resolved": "https://registry.npmjs.org/@sinclair/typebox/-/typebox-0.27.8.tgz",
- "integrity": "sha512-+Fj43pSMwJs4KRrH/938Uf+uAELIgVBmQzg/q1YG10djyfA3TnrU8N8XzqCh/okZdszqBQTZf96idMfE5lnwTA=="
- },
- "node_modules/@sindresorhus/is": {
- "version": "4.6.0",
- "resolved": "https://registry.npmjs.org/@sindresorhus/is/-/is-4.6.0.tgz",
- "integrity": "sha512-t09vSN3MdfsyCHoFcTRCH/iUtG7OJ0CsjzB8cjAmKc/va/kIgeDI/TxsigdncE/4be734m0cvIYwNaV4i2XqAw==",
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/sindresorhus/is?sponsor=1"
+ "node": ">=8"
}
},
- "node_modules/@sinonjs/commons": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/@sinonjs/commons/-/commons-3.0.0.tgz",
- "integrity": "sha512-jXBtWAF4vmdNmZgD5FoKsVLv3rPgDnLgPbU84LIJ3otV44vJlDRokVng5v8NFJdCf/da9legHcKaRuZs4L7faA==",
+ "node_modules/@react-native-community/cli-platform-ios/node_modules/supports-color": {
+ "version": "7.2.0",
+ "resolved": "https://registry.npmjs.org/supports-color/-/supports-color-7.2.0.tgz",
+ "integrity": "sha512-qpCAvRl9stuOHveKsn7HncJRvv501qIacKzQlO/+Lwxc9+0q2wLyv4Dfvt80/DPn2pqOBsJdDiogXGR9+OvwRw==",
"dependencies": {
- "type-detect": "4.0.8"
+ "has-flag": "^4.0.0"
+ },
+ "engines": {
+ "node": ">=8"
}
},
- "node_modules/@sinonjs/fake-timers": {
- "version": "10.3.0",
- "resolved": "https://registry.npmjs.org/@sinonjs/fake-timers/-/fake-timers-10.3.0.tgz",
- "integrity": "sha512-V4BG07kuYSUkTCSBHG8G8TNhM+F19jXFWnQtzj+we8DrkpSBCee9Z3Ms8yiGer/dlmhe35/Xdgyo3/0rQKg7YA==",
+ "node_modules/@react-native-community/cli-plugin-metro": {
+ "version": "12.3.2",
+ "resolved": "https://registry.npmjs.org/@react-native-community/cli-plugin-metro/-/cli-plugin-metro-12.3.2.tgz",
+ "integrity": "sha512-FpFBwu+d2E7KRhYPTkKvQsWb2/JKsJv+t1tcqgQkn+oByhp+qGyXBobFB8/R3yYvRRDCSDhS+atWTJzk9TjM8g=="
+ },
+ "node_modules/@react-native-community/cli-server-api": {
+ "version": "12.3.2",
+ "resolved": "https://registry.npmjs.org/@react-native-community/cli-server-api/-/cli-server-api-12.3.2.tgz",
+ "integrity": "sha512-iwa7EO9XFA/OjI5pPLLpI/6mFVqv8L73kNck3CNOJIUCCveGXBKK0VMyOkXaf/BYnihgQrXh+x5cxbDbggr7+Q==",
"dependencies": {
- "@sinonjs/commons": "^3.0.0"
+ "@react-native-community/cli-debugger-ui": "12.3.2",
+ "@react-native-community/cli-tools": "12.3.2",
+ "compression": "^1.7.1",
+ "connect": "^3.6.5",
+ "errorhandler": "^1.5.1",
+ "nocache": "^3.0.1",
+ "pretty-format": "^26.6.2",
+ "serve-static": "^1.13.1",
+ "ws": "^7.5.1"
}
},
- "node_modules/@sliphua/lilconfig-ts-loader": {
- "version": "3.2.2",
- "resolved": "https://registry.npmjs.org/@sliphua/lilconfig-ts-loader/-/lilconfig-ts-loader-3.2.2.tgz",
- "integrity": "sha512-nX2aBwAykiG50fSUzK9eyA5UvWcrEKzA0ZzCq9mLwHMwpKxM+U05YH8PHba1LJrbeZ7R1HSjJagWKMqFyq8cxw==",
- "dev": true,
+ "node_modules/@react-native-community/cli-server-api/node_modules/@jest/types": {
+ "version": "26.6.2",
+ "resolved": "https://registry.npmjs.org/@jest/types/-/types-26.6.2.tgz",
+ "integrity": "sha512-fC6QCp7Sc5sX6g8Tvbmj4XUTbyrik0akgRy03yjXbQaBWWNWGE7SGtJk98m0N8nzegD/7SggrUlivxo5ax4KWQ==",
"dependencies": {
- "lodash.get": "^4",
- "make-error": "^1",
- "ts-node": "^9",
- "tslib": "^2"
+ "@types/istanbul-lib-coverage": "^2.0.0",
+ "@types/istanbul-reports": "^3.0.0",
+ "@types/node": "*",
+ "@types/yargs": "^15.0.0",
+ "chalk": "^4.0.0"
},
"engines": {
- "node": ">=10.0.0"
- },
- "peerDependencies": {
- "lilconfig": ">=2"
+ "node": ">= 10.14.2"
}
},
- "node_modules/@storybook/addon-a11y": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/addon-a11y/-/addon-a11y-7.6.15.tgz",
- "integrity": "sha512-8PxRMBJUSxNoceo2IYXFyZp3VU+/ONK/DsD0dj/fVrv7izFrS8aw2GWSsSMK8xAbEUpANXWMKGaSyvrRFVgsVQ==",
- "dev": true,
+ "node_modules/@react-native-community/cli-server-api/node_modules/@types/yargs": {
+ "version": "15.0.19",
+ "resolved": "https://registry.npmjs.org/@types/yargs/-/yargs-15.0.19.tgz",
+ "integrity": "sha512-2XUaGVmyQjgyAZldf0D0c14vvo/yv0MhQBSTJcejMMaitsn3nxCB6TmH4G0ZQf+uxROOa9mpanoSm8h6SG/1ZA==",
"dependencies": {
- "@storybook/addon-highlight": "7.6.15",
- "axe-core": "^4.2.0"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/storybook"
+ "@types/yargs-parser": "*"
}
},
- "node_modules/@storybook/addon-actions": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/addon-actions/-/addon-actions-7.6.15.tgz",
- "integrity": "sha512-2Jfvbahe/tmq1iNnNxmcP0JnX0rqCuijjXXai9yMDV3koIMawn6t88MPVrdcso5ch/fxE45522nZqA3SZJbM4g==",
- "dev": true,
+ "node_modules/@react-native-community/cli-server-api/node_modules/pretty-format": {
+ "version": "26.6.2",
+ "resolved": "https://registry.npmjs.org/pretty-format/-/pretty-format-26.6.2.tgz",
+ "integrity": "sha512-7AeGuCYNGmycyQbCqd/3PWH4eOoX/OiCa0uphp57NVTeAGdJGaAliecxwBDHYQCIvrW7aDBZCYeNTP/WX69mkg==",
"dependencies": {
- "@storybook/core-events": "7.6.15",
- "@storybook/global": "^5.0.0",
- "@types/uuid": "^9.0.1",
- "dequal": "^2.0.2",
- "polished": "^4.2.2",
- "uuid": "^9.0.0"
+ "@jest/types": "^26.6.2",
+ "ansi-regex": "^5.0.0",
+ "ansi-styles": "^4.0.0",
+ "react-is": "^17.0.1"
},
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/storybook"
+ "engines": {
+ "node": ">= 10"
}
},
- "node_modules/@storybook/addon-actions/node_modules/@types/uuid": {
- "version": "9.0.8",
- "resolved": "https://registry.npmjs.org/@types/uuid/-/uuid-9.0.8.tgz",
- "integrity": "sha512-jg+97EGIcY9AGHJJRaaPVgetKDsrTgbRjQ5Msgjh/DQKEFl0DtyRr/VCOyD1T2R1MNeWPK/u7JoGhlDZnKBAfA==",
- "dev": true
+ "node_modules/@react-native-community/cli-server-api/node_modules/react-is": {
+ "version": "17.0.2",
+ "resolved": "https://registry.npmjs.org/react-is/-/react-is-17.0.2.tgz",
+ "integrity": "sha512-w2GsyukL62IJnlaff/nRegPQR94C/XXamvMWmSHRJ4y7Ts/4ocGRmTHvOs8PSE6pB3dWOrD/nueuU5sduBsQ4w=="
},
- "node_modules/@storybook/addon-controls": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/addon-controls/-/addon-controls-7.6.15.tgz",
- "integrity": "sha512-HXcG/Lr4ri7WUFz14Y5lEBTA1XmKy0E/DepW88XVy6YNsTpERVWEBcvjKoLAU1smKrfhVto96hK2AVFL3A8EBQ==",
- "dev": true,
+ "node_modules/@react-native-community/cli-tools": {
+ "version": "12.3.2",
+ "resolved": "https://registry.npmjs.org/@react-native-community/cli-tools/-/cli-tools-12.3.2.tgz",
+ "integrity": "sha512-nDH7vuEicHI2TI0jac/DjT3fr977iWXRdgVAqPZFFczlbs7A8GQvEdGnZ1G8dqRUmg+kptw0e4hwczAOG89JzQ==",
"dependencies": {
- "@storybook/blocks": "7.6.15",
- "lodash": "^4.17.21",
- "ts-dedent": "^2.0.0"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/storybook"
+ "appdirsjs": "^1.2.4",
+ "chalk": "^4.1.2",
+ "find-up": "^5.0.0",
+ "mime": "^2.4.1",
+ "node-fetch": "^2.6.0",
+ "open": "^6.2.0",
+ "ora": "^5.4.1",
+ "semver": "^7.5.2",
+ "shell-quote": "^1.7.3",
+ "sudo-prompt": "^9.0.0"
}
},
- "node_modules/@storybook/addon-docs": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/addon-docs/-/addon-docs-7.6.15.tgz",
- "integrity": "sha512-UPODqO+mrYaKyTSAtfRslxOFgSP/v/5vfDx896pbNTC4Sf8xLytoudw4I14hzkHmRdXiOnd21FqXJfmF/Onsvw==",
- "dev": true,
- "dependencies": {
- "@jest/transform": "^29.3.1",
- "@mdx-js/react": "^2.1.5",
- "@storybook/blocks": "7.6.15",
- "@storybook/client-logger": "7.6.15",
- "@storybook/components": "7.6.15",
- "@storybook/csf-plugin": "7.6.15",
- "@storybook/csf-tools": "7.6.15",
- "@storybook/global": "^5.0.0",
- "@storybook/mdx2-csf": "^1.0.0",
- "@storybook/node-logger": "7.6.15",
- "@storybook/postinstall": "7.6.15",
- "@storybook/preview-api": "7.6.15",
- "@storybook/react-dom-shim": "7.6.15",
- "@storybook/theming": "7.6.15",
- "@storybook/types": "7.6.15",
- "fs-extra": "^11.1.0",
- "remark-external-links": "^8.0.0",
- "remark-slug": "^6.0.0",
- "ts-dedent": "^2.0.0"
+ "node_modules/@react-native-community/cli-tools/node_modules/chalk": {
+ "version": "4.1.2",
+ "resolved": "https://registry.npmjs.org/chalk/-/chalk-4.1.2.tgz",
+ "integrity": "sha512-oKnbhFyRIXpUuez8iBMmyEa4nbj4IOQyuhc/wy9kY7/WVPcwIO9VA668Pu8RkO7+0G76SLROeyw9CpQ061i4mA==",
+ "dependencies": {
+ "ansi-styles": "^4.1.0",
+ "supports-color": "^7.1.0"
},
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/storybook"
+ "engines": {
+ "node": ">=10"
},
- "peerDependencies": {
- "react": "^16.8.0 || ^17.0.0 || ^18.0.0",
- "react-dom": "^16.8.0 || ^17.0.0 || ^18.0.0"
+ "funding": {
+ "url": "https://github.com/chalk/chalk?sponsor=1"
}
},
- "node_modules/@storybook/addon-docs/node_modules/fs-extra": {
- "version": "11.2.0",
- "resolved": "https://registry.npmjs.org/fs-extra/-/fs-extra-11.2.0.tgz",
- "integrity": "sha512-PmDi3uwK5nFuXh7XDTlVnS17xJS7vW36is2+w3xcv8SVxiB4NyATf4ctkVY5bkSjX0Y4nbvZCq1/EjtEyr9ktw==",
- "dev": true,
+ "node_modules/@react-native-community/cli-tools/node_modules/cli-cursor": {
+ "version": "3.1.0",
+ "resolved": "https://registry.npmjs.org/cli-cursor/-/cli-cursor-3.1.0.tgz",
+ "integrity": "sha512-I/zHAwsKf9FqGoXM4WWRACob9+SNukZTd94DWF57E4toouRulbCxcUh6RKUEOQlYTHJnzkPMySvPNaaSLNfLZw==",
"dependencies": {
- "graceful-fs": "^4.2.0",
- "jsonfile": "^6.0.1",
- "universalify": "^2.0.0"
+ "restore-cursor": "^3.1.0"
},
"engines": {
- "node": ">=14.14"
+ "node": ">=8"
}
},
- "node_modules/@storybook/addon-docs/node_modules/jsonfile": {
- "version": "6.1.0",
- "resolved": "https://registry.npmjs.org/jsonfile/-/jsonfile-6.1.0.tgz",
- "integrity": "sha512-5dgndWOriYSm5cnYaJNhalLNDKOqFwyDB/rr1E9ZsGciGvKPs8R2xYGCacuf3z6K1YKDz182fd+fY3cn3pMqXQ==",
- "dev": true,
+ "node_modules/@react-native-community/cli-tools/node_modules/find-up": {
+ "version": "5.0.0",
+ "resolved": "https://registry.npmjs.org/find-up/-/find-up-5.0.0.tgz",
+ "integrity": "sha512-78/PXT1wlLLDgTzDs7sjq9hzz0vXD+zn+7wypEe4fXQxCmdmqfGsEPQxmiCSQI3ajFV91bVSsvNtrJRiW6nGng==",
"dependencies": {
- "universalify": "^2.0.0"
+ "locate-path": "^6.0.0",
+ "path-exists": "^4.0.0"
},
- "optionalDependencies": {
- "graceful-fs": "^4.1.6"
+ "engines": {
+ "node": ">=10"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@storybook/addon-docs/node_modules/universalify": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/universalify/-/universalify-2.0.1.tgz",
- "integrity": "sha512-gptHNQghINnc/vTGIk0SOFGFNXw7JVrlRUtConJRlvaw6DuX0wO5Jeko9sWrMBhh+PsYAZ7oXAiOnf/UKogyiw==",
- "dev": true,
+ "node_modules/@react-native-community/cli-tools/node_modules/has-flag": {
+ "version": "4.0.0",
+ "resolved": "https://registry.npmjs.org/has-flag/-/has-flag-4.0.0.tgz",
+ "integrity": "sha512-EykJT/Q1KjTWctppgIAgfSO0tKVuZUjhgMr17kqTumMl6Afv3EISleU7qZUzoXDFTAHTDC4NOoG/ZxU3EvlMPQ==",
"engines": {
- "node": ">= 10.0.0"
+ "node": ">=8"
}
},
- "node_modules/@storybook/addon-highlight": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/addon-highlight/-/addon-highlight-7.6.15.tgz",
- "integrity": "sha512-ptidWZJJcEM83YsxCjf+m1q8Rr9sN8piJ4PJlM2vyc4MLZY4q6htb1JJFeq3ov1Iz6SY9KjKc/zOkWo4L54nxw==",
- "dev": true,
+ "node_modules/@react-native-community/cli-tools/node_modules/locate-path": {
+ "version": "6.0.0",
+ "resolved": "https://registry.npmjs.org/locate-path/-/locate-path-6.0.0.tgz",
+ "integrity": "sha512-iPZK6eYjbxRu3uB4/WZ3EsEIMJFMqAoopl3R+zuq0UjcAm/MO6KCweDgPfP3elTztoKP3KtnVHxTn2NHBSDVUw==",
"dependencies": {
- "@storybook/global": "^5.0.0"
+ "p-locate": "^5.0.0"
+ },
+ "engines": {
+ "node": ">=10"
},
"funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/storybook"
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@storybook/addon-toolbars": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/addon-toolbars/-/addon-toolbars-7.6.15.tgz",
- "integrity": "sha512-QougKS2eABB5Jd332i9tBpKgh2lN4aaqXkvmVC5egT5dOuJ9IeuZbGwiALef/uf1f3IuyUP41So9l2dI4u19aw==",
- "dev": true,
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/storybook"
+ "node_modules/@react-native-community/cli-tools/node_modules/mime": {
+ "version": "2.6.0",
+ "resolved": "https://registry.npmjs.org/mime/-/mime-2.6.0.tgz",
+ "integrity": "sha512-USPkMeET31rOMiarsBNIHZKLGgvKc/LrjofAnBlOttf5ajRvqiRA8QsenbcooctK6d6Ts6aqZXBA+XbkKthiQg==",
+ "bin": {
+ "mime": "cli.js"
+ },
+ "engines": {
+ "node": ">=4.0.0"
}
},
- "node_modules/@storybook/addon-viewport": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/addon-viewport/-/addon-viewport-7.6.15.tgz",
- "integrity": "sha512-0esg0+onJftU2prD3n/sbxBTrTOIGQnZhbrKPP+/S26dVHuYaR/65XdwpRgXNY5PHK2yjU78HxiJP+Kyu75ntw==",
- "dev": true,
- "dependencies": {
- "memoizerific": "^1.11.3"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/storybook"
+ "node_modules/@react-native-community/cli-tools/node_modules/mimic-fn": {
+ "version": "2.1.0",
+ "resolved": "https://registry.npmjs.org/mimic-fn/-/mimic-fn-2.1.0.tgz",
+ "integrity": "sha512-OqbOk5oEQeAZ8WXWydlu9HJjz9WVdEIvamMCcXmuqUYjTknH/sqsWvhQ3vgwKFRR1HpjvNBKQ37nbJgYzGqGcg==",
+ "engines": {
+ "node": ">=6"
}
},
- "node_modules/@storybook/blocks": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/blocks/-/blocks-7.6.15.tgz",
- "integrity": "sha512-ODP7AVh2iIGblI5WKGokWSHbp9YQHc+Uce7JCGcnDbNavoy64Z6R6G+wXzF5jfl7xQlbhQ8yQCuSSL4GNdYTeA==",
- "dev": true,
+ "node_modules/@react-native-community/cli-tools/node_modules/onetime": {
+ "version": "5.1.2",
+ "resolved": "https://registry.npmjs.org/onetime/-/onetime-5.1.2.tgz",
+ "integrity": "sha512-kbpaSSGJTWdAY5KPVeMOKXSrPtr8C8C7wodJbcsd51jRnmD+GZu8Y0VoU6Dm5Z4vWr0Ig/1NKuWRKf7j5aaYSg==",
"dependencies": {
- "@storybook/channels": "7.6.15",
- "@storybook/client-logger": "7.6.15",
- "@storybook/components": "7.6.15",
- "@storybook/core-events": "7.6.15",
- "@storybook/csf": "^0.1.2",
- "@storybook/docs-tools": "7.6.15",
- "@storybook/global": "^5.0.0",
- "@storybook/manager-api": "7.6.15",
- "@storybook/preview-api": "7.6.15",
- "@storybook/theming": "7.6.15",
- "@storybook/types": "7.6.15",
- "@types/lodash": "^4.14.167",
- "color-convert": "^2.0.1",
- "dequal": "^2.0.2",
- "lodash": "^4.17.21",
- "markdown-to-jsx": "^7.1.8",
- "memoizerific": "^1.11.3",
- "polished": "^4.2.2",
- "react-colorful": "^5.1.2",
- "telejson": "^7.2.0",
- "tocbot": "^4.20.1",
- "ts-dedent": "^2.0.0",
- "util-deprecate": "^1.0.2"
+ "mimic-fn": "^2.1.0"
},
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/storybook"
+ "engines": {
+ "node": ">=6"
},
- "peerDependencies": {
- "react": "^16.8.0 || ^17.0.0 || ^18.0.0",
- "react-dom": "^16.8.0 || ^17.0.0 || ^18.0.0"
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@storybook/blocks/node_modules/color-convert": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/color-convert/-/color-convert-2.0.1.tgz",
- "integrity": "sha512-RRECPsj7iu/xb5oKYcsFHSppFNnsj/52OVTRKb4zP5onXwVF3zVmmToNcOfGC+CRDpfK/U584fMg38ZHCaElKQ==",
- "dev": true,
+ "node_modules/@react-native-community/cli-tools/node_modules/ora": {
+ "version": "5.4.1",
+ "resolved": "https://registry.npmjs.org/ora/-/ora-5.4.1.tgz",
+ "integrity": "sha512-5b6Y85tPxZZ7QytO+BQzysW31HJku27cRIlkbAXaNx+BdcVi+LlRFmVXzeF6a7JCwJpyw5c4b+YSVImQIrBpuQ==",
"dependencies": {
- "color-name": "~1.1.4"
+ "bl": "^4.1.0",
+ "chalk": "^4.1.0",
+ "cli-cursor": "^3.1.0",
+ "cli-spinners": "^2.5.0",
+ "is-interactive": "^1.0.0",
+ "is-unicode-supported": "^0.1.0",
+ "log-symbols": "^4.1.0",
+ "strip-ansi": "^6.0.0",
+ "wcwidth": "^1.0.1"
},
"engines": {
- "node": ">=7.0.0"
- }
- },
- "node_modules/@storybook/blocks/node_modules/color-name": {
- "version": "1.1.4",
- "resolved": "https://registry.npmjs.org/color-name/-/color-name-1.1.4.tgz",
- "integrity": "sha512-dOy+3AuW3a2wNbZHIuMZpTcgjGuLU/uBL/ubcZF9OXbDo8ff4O8yVp5Bf0efS8uEoYo5q4Fx7dY9OgQGXgAsQA==",
- "dev": true
- },
- "node_modules/@storybook/builder-manager": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/builder-manager/-/builder-manager-7.6.15.tgz",
- "integrity": "sha512-vfpfCywiasyP7vtbgLJhjssBEwUjZhBsRsubDAzumgOochPiKKPNwsSc5NU/4ZIGaC5zRO26kUaUqFIbJdTEUQ==",
- "dev": true,
- "dependencies": {
- "@fal-works/esbuild-plugin-global-externals": "^2.1.2",
- "@storybook/core-common": "7.6.15",
- "@storybook/manager": "7.6.15",
- "@storybook/node-logger": "7.6.15",
- "@types/ejs": "^3.1.1",
- "@types/find-cache-dir": "^3.2.1",
- "@yarnpkg/esbuild-plugin-pnp": "^3.0.0-rc.10",
- "browser-assert": "^1.2.1",
- "ejs": "^3.1.8",
- "esbuild": "^0.18.0",
- "esbuild-plugin-alias": "^0.2.1",
- "express": "^4.17.3",
- "find-cache-dir": "^3.0.0",
- "fs-extra": "^11.1.0",
- "process": "^0.11.10",
- "util": "^0.12.4"
+ "node": ">=10"
},
"funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/storybook"
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@storybook/builder-manager/node_modules/find-cache-dir": {
- "version": "3.3.2",
- "resolved": "https://registry.npmjs.org/find-cache-dir/-/find-cache-dir-3.3.2.tgz",
- "integrity": "sha512-wXZV5emFEjrridIgED11OoUKLxiYjAcqot/NJdAkOhlJ+vGzwhOAfcG5OX1jP+S0PcjEn8bdMJv+g2jwQ3Onig==",
- "dev": true,
+ "node_modules/@react-native-community/cli-tools/node_modules/p-locate": {
+ "version": "5.0.0",
+ "resolved": "https://registry.npmjs.org/p-locate/-/p-locate-5.0.0.tgz",
+ "integrity": "sha512-LaNjtRWUBY++zB5nE/NwcaoMylSPk+S+ZHNB1TzdbMJMny6dynpAGt7X/tl/QYq3TIeE6nxHppbo2LGymrG5Pw==",
"dependencies": {
- "commondir": "^1.0.1",
- "make-dir": "^3.0.2",
- "pkg-dir": "^4.1.0"
+ "p-limit": "^3.0.2"
},
"engines": {
- "node": ">=8"
+ "node": ">=10"
},
"funding": {
- "url": "https://github.com/avajs/find-cache-dir?sponsor=1"
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@storybook/builder-manager/node_modules/find-up": {
- "version": "4.1.0",
- "resolved": "https://registry.npmjs.org/find-up/-/find-up-4.1.0.tgz",
- "integrity": "sha512-PpOwAdQ/YlXQ2vj8a3h8IipDuYRi3wceVQQGYWxNINccq40Anw7BlsEXCMbt1Zt+OLA6Fq9suIpIWD0OsnISlw==",
- "dev": true,
- "dependencies": {
- "locate-path": "^5.0.0",
- "path-exists": "^4.0.0"
- },
+ "node_modules/@react-native-community/cli-tools/node_modules/path-exists": {
+ "version": "4.0.0",
+ "resolved": "https://registry.npmjs.org/path-exists/-/path-exists-4.0.0.tgz",
+ "integrity": "sha512-ak9Qy5Q7jYb2Wwcey5Fpvg2KoAc/ZIhLSLOSBmRmygPsGwkVVt0fZa0qrtMz+m6tJTAHfZQ8FnmB4MG4LWy7/w==",
"engines": {
"node": ">=8"
}
},
- "node_modules/@storybook/builder-manager/node_modules/fs-extra": {
- "version": "11.2.0",
- "resolved": "https://registry.npmjs.org/fs-extra/-/fs-extra-11.2.0.tgz",
- "integrity": "sha512-PmDi3uwK5nFuXh7XDTlVnS17xJS7vW36is2+w3xcv8SVxiB4NyATf4ctkVY5bkSjX0Y4nbvZCq1/EjtEyr9ktw==",
- "dev": true,
+ "node_modules/@react-native-community/cli-tools/node_modules/restore-cursor": {
+ "version": "3.1.0",
+ "resolved": "https://registry.npmjs.org/restore-cursor/-/restore-cursor-3.1.0.tgz",
+ "integrity": "sha512-l+sSefzHpj5qimhFSE5a8nufZYAM3sBSVMAPtYkmC+4EH2anSGaEMXSD0izRQbu9nfyQ9y5JrVmp7E8oZrUjvA==",
"dependencies": {
- "graceful-fs": "^4.2.0",
- "jsonfile": "^6.0.1",
- "universalify": "^2.0.0"
+ "onetime": "^5.1.0",
+ "signal-exit": "^3.0.2"
},
"engines": {
- "node": ">=14.14"
+ "node": ">=8"
}
},
- "node_modules/@storybook/builder-manager/node_modules/jsonfile": {
- "version": "6.1.0",
- "resolved": "https://registry.npmjs.org/jsonfile/-/jsonfile-6.1.0.tgz",
- "integrity": "sha512-5dgndWOriYSm5cnYaJNhalLNDKOqFwyDB/rr1E9ZsGciGvKPs8R2xYGCacuf3z6K1YKDz182fd+fY3cn3pMqXQ==",
- "dev": true,
+ "node_modules/@react-native-community/cli-tools/node_modules/supports-color": {
+ "version": "7.2.0",
+ "resolved": "https://registry.npmjs.org/supports-color/-/supports-color-7.2.0.tgz",
+ "integrity": "sha512-qpCAvRl9stuOHveKsn7HncJRvv501qIacKzQlO/+Lwxc9+0q2wLyv4Dfvt80/DPn2pqOBsJdDiogXGR9+OvwRw==",
"dependencies": {
- "universalify": "^2.0.0"
+ "has-flag": "^4.0.0"
},
- "optionalDependencies": {
- "graceful-fs": "^4.1.6"
+ "engines": {
+ "node": ">=8"
}
},
- "node_modules/@storybook/builder-manager/node_modules/locate-path": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/locate-path/-/locate-path-5.0.0.tgz",
- "integrity": "sha512-t7hw9pI+WvuwNJXwk5zVHpyhIqzg2qTlklJOf0mVxGSbe3Fp2VieZcduNYjaLDoy6p9uGpQEGWG87WpMKlNq8g==",
- "dev": true,
+ "node_modules/@react-native-community/cli-types": {
+ "version": "12.3.2",
+ "resolved": "https://registry.npmjs.org/@react-native-community/cli-types/-/cli-types-12.3.2.tgz",
+ "integrity": "sha512-9D0UEFqLW8JmS16mjHJxUJWX8E+zJddrHILSH8AJHZ0NNHv4u2DXKdb0wFLMobFxGNxPT+VSOjc60fGvXzWHog==",
"dependencies": {
- "p-locate": "^4.1.0"
- },
- "engines": {
- "node": ">=8"
+ "joi": "^17.2.1"
}
},
- "node_modules/@storybook/builder-manager/node_modules/make-dir": {
- "version": "3.1.0",
- "resolved": "https://registry.npmjs.org/make-dir/-/make-dir-3.1.0.tgz",
- "integrity": "sha512-g3FeP20LNwhALb/6Cz6Dd4F2ngze0jz7tbzrD2wAV+o9FeNHe4rL+yK2md0J/fiSf1sa1ADhXqi5+oVwOM/eGw==",
- "dev": true,
+ "node_modules/@react-native-community/cli/node_modules/chalk": {
+ "version": "4.1.2",
+ "resolved": "https://registry.npmjs.org/chalk/-/chalk-4.1.2.tgz",
+ "integrity": "sha512-oKnbhFyRIXpUuez8iBMmyEa4nbj4IOQyuhc/wy9kY7/WVPcwIO9VA668Pu8RkO7+0G76SLROeyw9CpQ061i4mA==",
"dependencies": {
- "semver": "^6.0.0"
+ "ansi-styles": "^4.1.0",
+ "supports-color": "^7.1.0"
},
"engines": {
- "node": ">=8"
+ "node": ">=10"
},
"funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "url": "https://github.com/chalk/chalk?sponsor=1"
}
},
- "node_modules/@storybook/builder-manager/node_modules/p-limit": {
- "version": "2.3.0",
- "resolved": "https://registry.npmjs.org/p-limit/-/p-limit-2.3.0.tgz",
- "integrity": "sha512-//88mFWSJx8lxCzwdAABTJL2MyWB12+eIY7MDL2SqLmAkeKU9qxRvWuSyTjm3FUmpBEMuFfckAIqEaVGUDxb6w==",
- "dev": true,
+ "node_modules/@react-native-community/cli/node_modules/commander": {
+ "version": "9.5.0",
+ "resolved": "https://registry.npmjs.org/commander/-/commander-9.5.0.tgz",
+ "integrity": "sha512-KRs7WVDKg86PWiuAqhDrAQnTXZKraVcCc6vFdL14qrZ/DcWwuRo7VoiYXalXO7S5GKpqYiVEwCbgFDfxNHKJBQ==",
+ "engines": {
+ "node": "^12.20.0 || >=14"
+ }
+ },
+ "node_modules/@react-native-community/cli/node_modules/execa": {
+ "version": "5.1.1",
+ "resolved": "https://registry.npmjs.org/execa/-/execa-5.1.1.tgz",
+ "integrity": "sha512-8uSpZZocAZRBAPIEINJj3Lo9HyGitllczc27Eh5YYojjMFMn8yHMDMaUHE2Jqfq05D/wucwI4JGURyXt1vchyg==",
"dependencies": {
- "p-try": "^2.0.0"
+ "cross-spawn": "^7.0.3",
+ "get-stream": "^6.0.0",
+ "human-signals": "^2.1.0",
+ "is-stream": "^2.0.0",
+ "merge-stream": "^2.0.0",
+ "npm-run-path": "^4.0.1",
+ "onetime": "^5.1.2",
+ "signal-exit": "^3.0.3",
+ "strip-final-newline": "^2.0.0"
},
"engines": {
- "node": ">=6"
+ "node": ">=10"
},
"funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "url": "https://github.com/sindresorhus/execa?sponsor=1"
}
},
- "node_modules/@storybook/builder-manager/node_modules/p-locate": {
+ "node_modules/@react-native-community/cli/node_modules/find-up": {
"version": "4.1.0",
- "resolved": "https://registry.npmjs.org/p-locate/-/p-locate-4.1.0.tgz",
- "integrity": "sha512-R79ZZ/0wAxKGu3oYMlz8jy/kbhsNrS7SKZ7PxEHBgJ5+F2mtFW2fK2cOtBh1cHYkQsbzFV7I+EoRKe6Yt0oK7A==",
- "dev": true,
+ "resolved": "https://registry.npmjs.org/find-up/-/find-up-4.1.0.tgz",
+ "integrity": "sha512-PpOwAdQ/YlXQ2vj8a3h8IipDuYRi3wceVQQGYWxNINccq40Anw7BlsEXCMbt1Zt+OLA6Fq9suIpIWD0OsnISlw==",
"dependencies": {
- "p-limit": "^2.2.0"
+ "locate-path": "^5.0.0",
+ "path-exists": "^4.0.0"
},
"engines": {
"node": ">=8"
}
},
- "node_modules/@storybook/builder-manager/node_modules/p-try": {
- "version": "2.2.0",
- "resolved": "https://registry.npmjs.org/p-try/-/p-try-2.2.0.tgz",
- "integrity": "sha512-R4nPAVTAU0B9D35/Gk3uJf/7XYbQcyohSKdvAxIRSNghFl4e71hVoGnBNQz9cWaXxO2I10KTC+3jMdvvoKw6dQ==",
- "dev": true,
+ "node_modules/@react-native-community/cli/node_modules/get-stream": {
+ "version": "6.0.1",
+ "resolved": "https://registry.npmjs.org/get-stream/-/get-stream-6.0.1.tgz",
+ "integrity": "sha512-ts6Wi+2j3jQjqi70w5AlN8DFnkSwC+MqmxEzdEALB2qXZYV3X/b1CTfgPLGJNMeAWxdPfU8FO1ms3NUfaHCPYg==",
"engines": {
- "node": ">=6"
+ "node": ">=10"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@storybook/builder-manager/node_modules/path-exists": {
+ "node_modules/@react-native-community/cli/node_modules/has-flag": {
"version": "4.0.0",
- "resolved": "https://registry.npmjs.org/path-exists/-/path-exists-4.0.0.tgz",
- "integrity": "sha512-ak9Qy5Q7jYb2Wwcey5Fpvg2KoAc/ZIhLSLOSBmRmygPsGwkVVt0fZa0qrtMz+m6tJTAHfZQ8FnmB4MG4LWy7/w==",
- "dev": true,
+ "resolved": "https://registry.npmjs.org/has-flag/-/has-flag-4.0.0.tgz",
+ "integrity": "sha512-EykJT/Q1KjTWctppgIAgfSO0tKVuZUjhgMr17kqTumMl6Afv3EISleU7qZUzoXDFTAHTDC4NOoG/ZxU3EvlMPQ==",
"engines": {
"node": ">=8"
}
},
- "node_modules/@storybook/builder-manager/node_modules/pkg-dir": {
- "version": "4.2.0",
- "resolved": "https://registry.npmjs.org/pkg-dir/-/pkg-dir-4.2.0.tgz",
- "integrity": "sha512-HRDzbaKjC+AOWVXxAU/x54COGeIv9eb+6CkDSQoNTt4XyWoIJvuPsXizxu/Fr23EiekbtZwmh1IcIG/l/a10GQ==",
- "dev": true,
- "dependencies": {
- "find-up": "^4.0.0"
- },
+ "node_modules/@react-native-community/cli/node_modules/human-signals": {
+ "version": "2.1.0",
+ "resolved": "https://registry.npmjs.org/human-signals/-/human-signals-2.1.0.tgz",
+ "integrity": "sha512-B4FFZ6q/T2jhhksgkbEW3HBvWIfDW85snkQgawt07S7J5QXTk6BkNV+0yAeZrM5QpMAdYlocGoljn0sJ/WQkFw==",
"engines": {
- "node": ">=8"
- }
- },
- "node_modules/@storybook/builder-manager/node_modules/semver": {
- "version": "6.3.1",
- "resolved": "https://registry.npmjs.org/semver/-/semver-6.3.1.tgz",
- "integrity": "sha512-BR7VvDCVHO+q2xBEWskxS6DJE1qRnb7DxzUrogb71CWoSficBxYsiAGd+Kl0mmq/MprG9yArRkyrQxTO6XjMzA==",
- "dev": true,
- "bin": {
- "semver": "bin/semver.js"
+ "node": ">=10.17.0"
}
},
- "node_modules/@storybook/builder-manager/node_modules/universalify": {
+ "node_modules/@react-native-community/cli/node_modules/is-stream": {
"version": "2.0.1",
- "resolved": "https://registry.npmjs.org/universalify/-/universalify-2.0.1.tgz",
- "integrity": "sha512-gptHNQghINnc/vTGIk0SOFGFNXw7JVrlRUtConJRlvaw6DuX0wO5Jeko9sWrMBhh+PsYAZ7oXAiOnf/UKogyiw==",
- "dev": true,
+ "resolved": "https://registry.npmjs.org/is-stream/-/is-stream-2.0.1.tgz",
+ "integrity": "sha512-hFoiJiTl63nn+kstHGBtewWSKnQLpyb155KHheA1l39uvtO9nWIop1p3udqPcUd/xbF1VLMO4n7OI6p7RbngDg==",
"engines": {
- "node": ">= 10.0.0"
+ "node": ">=8"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@storybook/builder-manager/node_modules/util": {
- "version": "0.12.5",
- "resolved": "https://registry.npmjs.org/util/-/util-0.12.5.tgz",
- "integrity": "sha512-kZf/K6hEIrWHI6XqOFUiiMa+79wE/D8Q+NCNAWclkyg3b4d2k7s0QGepNjiABc+aR3N1PAyHL7p6UcLY6LmrnA==",
- "dev": true,
+ "node_modules/@react-native-community/cli/node_modules/locate-path": {
+ "version": "5.0.0",
+ "resolved": "https://registry.npmjs.org/locate-path/-/locate-path-5.0.0.tgz",
+ "integrity": "sha512-t7hw9pI+WvuwNJXwk5zVHpyhIqzg2qTlklJOf0mVxGSbe3Fp2VieZcduNYjaLDoy6p9uGpQEGWG87WpMKlNq8g==",
"dependencies": {
- "inherits": "^2.0.3",
- "is-arguments": "^1.0.4",
- "is-generator-function": "^1.0.7",
- "is-typed-array": "^1.1.3",
- "which-typed-array": "^1.1.2"
+ "p-locate": "^4.1.0"
+ },
+ "engines": {
+ "node": ">=8"
}
},
- "node_modules/@storybook/builder-webpack5": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/builder-webpack5/-/builder-webpack5-7.6.15.tgz",
- "integrity": "sha512-HF+TSK/eU2ld8uQ8VWgcAIzOQ2hjnEkzup363vGZkYUfsHsVbjMpZgf+foDjI4LZNfQ/RjcVEZxqJqIbpM0Sjg==",
- "dev": true,
- "dependencies": {
- "@babel/core": "^7.23.2",
- "@storybook/channels": "7.6.15",
- "@storybook/client-logger": "7.6.15",
- "@storybook/core-common": "7.6.15",
- "@storybook/core-events": "7.6.15",
- "@storybook/core-webpack": "7.6.15",
- "@storybook/node-logger": "7.6.15",
- "@storybook/preview": "7.6.15",
- "@storybook/preview-api": "7.6.15",
- "@swc/core": "^1.3.82",
- "@types/node": "^18.0.0",
- "@types/semver": "^7.3.4",
- "babel-loader": "^9.0.0",
- "browser-assert": "^1.2.1",
- "case-sensitive-paths-webpack-plugin": "^2.4.0",
- "cjs-module-lexer": "^1.2.3",
- "constants-browserify": "^1.0.0",
- "css-loader": "^6.7.1",
- "es-module-lexer": "^1.4.1",
- "express": "^4.17.3",
- "fork-ts-checker-webpack-plugin": "^8.0.0",
- "fs-extra": "^11.1.0",
- "html-webpack-plugin": "^5.5.0",
- "magic-string": "^0.30.5",
- "path-browserify": "^1.0.1",
- "process": "^0.11.10",
- "semver": "^7.3.7",
- "style-loader": "^3.3.1",
- "swc-loader": "^0.2.3",
- "terser-webpack-plugin": "^5.3.1",
- "ts-dedent": "^2.0.0",
- "url": "^0.11.0",
- "util": "^0.12.4",
- "util-deprecate": "^1.0.2",
- "webpack": "5",
- "webpack-dev-middleware": "^6.1.1",
- "webpack-hot-middleware": "^2.25.1",
- "webpack-virtual-modules": "^0.5.0"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/storybook"
+ "node_modules/@react-native-community/cli/node_modules/mimic-fn": {
+ "version": "2.1.0",
+ "resolved": "https://registry.npmjs.org/mimic-fn/-/mimic-fn-2.1.0.tgz",
+ "integrity": "sha512-OqbOk5oEQeAZ8WXWydlu9HJjz9WVdEIvamMCcXmuqUYjTknH/sqsWvhQ3vgwKFRR1HpjvNBKQ37nbJgYzGqGcg==",
+ "engines": {
+ "node": ">=6"
+ }
+ },
+ "node_modules/@react-native-community/cli/node_modules/npm-run-path": {
+ "version": "4.0.1",
+ "resolved": "https://registry.npmjs.org/npm-run-path/-/npm-run-path-4.0.1.tgz",
+ "integrity": "sha512-S48WzZW777zhNIrn7gxOlISNAqi9ZC/uQFnRdbeIHhZhCA6UqpkOT8T1G7BvfdgP4Er8gF4sUbaS0i7QvIfCWw==",
+ "dependencies": {
+ "path-key": "^3.0.0"
},
- "peerDependenciesMeta": {
- "typescript": {
- "optional": true
- }
+ "engines": {
+ "node": ">=8"
}
},
- "node_modules/@storybook/builder-webpack5/node_modules/css-loader": {
- "version": "6.11.0",
- "resolved": "https://registry.npmjs.org/css-loader/-/css-loader-6.11.0.tgz",
- "integrity": "sha512-CTJ+AEQJjq5NzLga5pE39qdiSV56F8ywCIsqNIRF0r7BDgWsN25aazToqAFg7ZrtA/U016xudB3ffgweORxX7g==",
- "dev": true,
+ "node_modules/@react-native-community/cli/node_modules/onetime": {
+ "version": "5.1.2",
+ "resolved": "https://registry.npmjs.org/onetime/-/onetime-5.1.2.tgz",
+ "integrity": "sha512-kbpaSSGJTWdAY5KPVeMOKXSrPtr8C8C7wodJbcsd51jRnmD+GZu8Y0VoU6Dm5Z4vWr0Ig/1NKuWRKf7j5aaYSg==",
"dependencies": {
- "icss-utils": "^5.1.0",
- "postcss": "^8.4.33",
- "postcss-modules-extract-imports": "^3.1.0",
- "postcss-modules-local-by-default": "^4.0.5",
- "postcss-modules-scope": "^3.2.0",
- "postcss-modules-values": "^4.0.0",
- "postcss-value-parser": "^4.2.0",
- "semver": "^7.5.4"
+ "mimic-fn": "^2.1.0"
},
"engines": {
- "node": ">= 12.13.0"
+ "node": ">=6"
},
"funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/webpack"
- },
- "peerDependencies": {
- "@rspack/core": "0.x || 1.x",
- "webpack": "^5.0.0"
- },
- "peerDependenciesMeta": {
- "@rspack/core": {
- "optional": true
- },
- "webpack": {
- "optional": true
- }
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@storybook/builder-webpack5/node_modules/fs-extra": {
- "version": "11.2.0",
- "resolved": "https://registry.npmjs.org/fs-extra/-/fs-extra-11.2.0.tgz",
- "integrity": "sha512-PmDi3uwK5nFuXh7XDTlVnS17xJS7vW36is2+w3xcv8SVxiB4NyATf4ctkVY5bkSjX0Y4nbvZCq1/EjtEyr9ktw==",
- "dev": true,
+ "node_modules/@react-native-community/cli/node_modules/p-limit": {
+ "version": "2.3.0",
+ "resolved": "https://registry.npmjs.org/p-limit/-/p-limit-2.3.0.tgz",
+ "integrity": "sha512-//88mFWSJx8lxCzwdAABTJL2MyWB12+eIY7MDL2SqLmAkeKU9qxRvWuSyTjm3FUmpBEMuFfckAIqEaVGUDxb6w==",
"dependencies": {
- "graceful-fs": "^4.2.0",
- "jsonfile": "^6.0.1",
- "universalify": "^2.0.0"
+ "p-try": "^2.0.0"
},
"engines": {
- "node": ">=14.14"
+ "node": ">=6"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@storybook/builder-webpack5/node_modules/jsonfile": {
- "version": "6.1.0",
- "resolved": "https://registry.npmjs.org/jsonfile/-/jsonfile-6.1.0.tgz",
- "integrity": "sha512-5dgndWOriYSm5cnYaJNhalLNDKOqFwyDB/rr1E9ZsGciGvKPs8R2xYGCacuf3z6K1YKDz182fd+fY3cn3pMqXQ==",
- "dev": true,
+ "node_modules/@react-native-community/cli/node_modules/p-locate": {
+ "version": "4.1.0",
+ "resolved": "https://registry.npmjs.org/p-locate/-/p-locate-4.1.0.tgz",
+ "integrity": "sha512-R79ZZ/0wAxKGu3oYMlz8jy/kbhsNrS7SKZ7PxEHBgJ5+F2mtFW2fK2cOtBh1cHYkQsbzFV7I+EoRKe6Yt0oK7A==",
"dependencies": {
- "universalify": "^2.0.0"
+ "p-limit": "^2.2.0"
},
- "optionalDependencies": {
- "graceful-fs": "^4.1.6"
+ "engines": {
+ "node": ">=8"
}
},
- "node_modules/@storybook/builder-webpack5/node_modules/path-browserify": {
- "version": "1.0.1",
- "resolved": "https://registry.npmjs.org/path-browserify/-/path-browserify-1.0.1.tgz",
- "integrity": "sha512-b7uo2UCUOYZcnF/3ID0lulOJi/bafxa1xPe7ZPsammBSpjSWQkjNxlt635YGS2MiR9GjvuXCtz2emr3jbsz98g==",
- "dev": true
+ "node_modules/@react-native-community/cli/node_modules/p-try": {
+ "version": "2.2.0",
+ "resolved": "https://registry.npmjs.org/p-try/-/p-try-2.2.0.tgz",
+ "integrity": "sha512-R4nPAVTAU0B9D35/Gk3uJf/7XYbQcyohSKdvAxIRSNghFl4e71hVoGnBNQz9cWaXxO2I10KTC+3jMdvvoKw6dQ==",
+ "engines": {
+ "node": ">=6"
+ }
},
- "node_modules/@storybook/builder-webpack5/node_modules/postcss-value-parser": {
- "version": "4.2.0",
- "resolved": "https://registry.npmjs.org/postcss-value-parser/-/postcss-value-parser-4.2.0.tgz",
- "integrity": "sha512-1NNCs6uurfkVbeXG4S8JFT9t19m45ICnif8zWLd5oPSZ50QnwMfK+H3jv408d4jw/7Bttv5axS5IiHoLaVNHeQ==",
- "dev": true
+ "node_modules/@react-native-community/cli/node_modules/path-exists": {
+ "version": "4.0.0",
+ "resolved": "https://registry.npmjs.org/path-exists/-/path-exists-4.0.0.tgz",
+ "integrity": "sha512-ak9Qy5Q7jYb2Wwcey5Fpvg2KoAc/ZIhLSLOSBmRmygPsGwkVVt0fZa0qrtMz+m6tJTAHfZQ8FnmB4MG4LWy7/w==",
+ "engines": {
+ "node": ">=8"
+ }
},
- "node_modules/@storybook/builder-webpack5/node_modules/style-loader": {
- "version": "3.3.4",
- "resolved": "https://registry.npmjs.org/style-loader/-/style-loader-3.3.4.tgz",
- "integrity": "sha512-0WqXzrsMTyb8yjZJHDqwmnwRJvhALK9LfRtRc6B4UTWe8AijYLZYZ9thuJTZc2VfQWINADW/j+LiJnfy2RoC1w==",
- "dev": true,
+ "node_modules/@react-native-community/cli/node_modules/path-key": {
+ "version": "3.1.1",
+ "resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
+ "integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
"engines": {
- "node": ">= 12.13.0"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/webpack"
+ "node": ">=8"
+ }
+ },
+ "node_modules/@react-native-community/cli/node_modules/supports-color": {
+ "version": "7.2.0",
+ "resolved": "https://registry.npmjs.org/supports-color/-/supports-color-7.2.0.tgz",
+ "integrity": "sha512-qpCAvRl9stuOHveKsn7HncJRvv501qIacKzQlO/+Lwxc9+0q2wLyv4Dfvt80/DPn2pqOBsJdDiogXGR9+OvwRw==",
+ "dependencies": {
+ "has-flag": "^4.0.0"
},
+ "engines": {
+ "node": ">=8"
+ }
+ },
+ "node_modules/@react-native-community/slider": {
+ "version": "3.0.2-wp-5",
+ "resolved": "https://raw.githubusercontent.com/wordpress-mobile/react-native-slider/v3.0.2-wp-5/react-native-community-slider-3.0.2-wp-5.tgz",
+ "integrity": "sha512-19yl9Em1mFKFLB9o3IO3VpBFw+U7cswjRk6cZ7kkhDCpDD3u0cpzkaIZK6NuFIPCLqOwU0MPtP+gonanN1JQKw==",
+ "workspaces": [
+ "src",
+ "example"
+ ]
+ },
+ "node_modules/@react-native-masked-view/masked-view": {
+ "version": "0.3.0",
+ "resolved": "https://registry.npmjs.org/@react-native-masked-view/masked-view/-/masked-view-0.3.0.tgz",
+ "integrity": "sha512-qLyoObcjzrkpNcoJjXquUePXfL1dXjHtuv+yX0zZ0Q4kG5yvVqd620+tSh7WbRoHkjpXhFBfLwvGhcWB2I0Lpw==",
"peerDependencies": {
- "webpack": "^5.0.0"
+ "react": ">=16",
+ "react-native": ">=0.57"
}
},
- "node_modules/@storybook/builder-webpack5/node_modules/universalify": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/universalify/-/universalify-2.0.1.tgz",
- "integrity": "sha512-gptHNQghINnc/vTGIk0SOFGFNXw7JVrlRUtConJRlvaw6DuX0wO5Jeko9sWrMBhh+PsYAZ7oXAiOnf/UKogyiw==",
- "dev": true,
+ "node_modules/@react-native/assets-registry": {
+ "version": "0.73.1",
+ "resolved": "https://registry.npmjs.org/@react-native/assets-registry/-/assets-registry-0.73.1.tgz",
+ "integrity": "sha512-2FgAbU7uKM5SbbW9QptPPZx8N9Ke2L7bsHb+EhAanZjFZunA9PaYtyjUQ1s7HD+zDVqOQIvjkpXSv7Kejd2tqg==",
"engines": {
- "node": ">= 10.0.0"
+ "node": ">=18"
}
},
- "node_modules/@storybook/builder-webpack5/node_modules/util": {
- "version": "0.12.5",
- "resolved": "https://registry.npmjs.org/util/-/util-0.12.5.tgz",
- "integrity": "sha512-kZf/K6hEIrWHI6XqOFUiiMa+79wE/D8Q+NCNAWclkyg3b4d2k7s0QGepNjiABc+aR3N1PAyHL7p6UcLY6LmrnA==",
- "dev": true,
+ "node_modules/@react-native/babel-plugin-codegen": {
+ "version": "0.73.3",
+ "resolved": "https://registry.npmjs.org/@react-native/babel-plugin-codegen/-/babel-plugin-codegen-0.73.3.tgz",
+ "integrity": "sha512-+zQrDDbz6lB48LyzFHxNCgXDCBHH+oTRdXAjikRcBUdeG9St9ABbYFLtb799zSxLOrCqFVyXqhJR2vlgLLEbcg==",
"dependencies": {
- "inherits": "^2.0.3",
- "is-arguments": "^1.0.4",
- "is-generator-function": "^1.0.7",
- "is-typed-array": "^1.1.3",
- "which-typed-array": "^1.1.2"
+ "@react-native/codegen": "0.73.2"
+ },
+ "engines": {
+ "node": ">=18"
}
},
- "node_modules/@storybook/channels": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/channels/-/channels-7.6.15.tgz",
- "integrity": "sha512-UPDYRzGkygYFa8QUpEiumWrvZm4u4RKVzgiBt9C4RmHORqkkZzL9LXhaZJp2SmIz1ND5gx6KR5ze8ZnAdwxxoQ==",
+ "node_modules/@react-native/babel-preset": {
+ "version": "0.73.10",
+ "resolved": "https://registry.npmjs.org/@react-native/babel-preset/-/babel-preset-0.73.10.tgz",
+ "integrity": "sha512-LPxR9DZK1EtVSEm2+fpei/AWwzgyHfaY7fuX8wDiy2THRYc/56+v28IihN8g0IAbQH+gdjXVcshJRYfV6bh2gg==",
"dev": true,
"dependencies": {
- "@storybook/client-logger": "7.6.15",
- "@storybook/core-events": "7.6.15",
- "@storybook/global": "^5.0.0",
- "qs": "^6.10.0",
- "telejson": "^7.2.0",
- "tiny-invariant": "^1.3.1"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/storybook"
- }
- },
- "node_modules/@storybook/cli": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/cli/-/cli-7.6.15.tgz",
- "integrity": "sha512-2QRqCyVGDSkraHxX2JPYkkFccbu5Uo+JYFaFJo4vmMXzDurjWON+Ga2B8FCTd4A8P4C02Ca/79jgQoyBB3xoew==",
- "dev": true,
- "dependencies": {
- "@babel/core": "^7.23.2",
- "@babel/preset-env": "^7.23.2",
- "@babel/types": "^7.23.0",
- "@ndelangen/get-tarball": "^3.0.7",
- "@storybook/codemod": "7.6.15",
- "@storybook/core-common": "7.6.15",
- "@storybook/core-events": "7.6.15",
- "@storybook/core-server": "7.6.15",
- "@storybook/csf-tools": "7.6.15",
- "@storybook/node-logger": "7.6.15",
- "@storybook/telemetry": "7.6.15",
- "@storybook/types": "7.6.15",
- "@types/semver": "^7.3.4",
- "@yarnpkg/fslib": "2.10.3",
- "@yarnpkg/libzip": "2.3.0",
- "chalk": "^4.1.0",
- "commander": "^6.2.1",
- "cross-spawn": "^7.0.3",
- "detect-indent": "^6.1.0",
- "envinfo": "^7.7.3",
- "execa": "^5.0.0",
- "express": "^4.17.3",
- "find-up": "^5.0.0",
- "fs-extra": "^11.1.0",
- "get-npm-tarball-url": "^2.0.3",
- "get-port": "^5.1.1",
- "giget": "^1.0.0",
- "globby": "^11.0.2",
- "jscodeshift": "^0.15.1",
- "leven": "^3.1.0",
- "ora": "^5.4.1",
- "prettier": "^2.8.0",
- "prompts": "^2.4.0",
- "puppeteer-core": "^2.1.1",
- "read-pkg-up": "^7.0.1",
- "semver": "^7.3.7",
- "strip-json-comments": "^3.0.1",
- "tempy": "^1.0.1",
- "ts-dedent": "^2.0.0",
- "util-deprecate": "^1.0.2"
+ "@babel/core": "^7.20.0",
+ "@babel/plugin-proposal-async-generator-functions": "^7.0.0",
+ "@babel/plugin-proposal-class-properties": "^7.18.0",
+ "@babel/plugin-proposal-export-default-from": "^7.0.0",
+ "@babel/plugin-proposal-nullish-coalescing-operator": "^7.18.0",
+ "@babel/plugin-proposal-numeric-separator": "^7.0.0",
+ "@babel/plugin-proposal-object-rest-spread": "^7.20.0",
+ "@babel/plugin-proposal-optional-catch-binding": "^7.0.0",
+ "@babel/plugin-proposal-optional-chaining": "^7.20.0",
+ "@babel/plugin-syntax-dynamic-import": "^7.8.0",
+ "@babel/plugin-syntax-export-default-from": "^7.0.0",
+ "@babel/plugin-syntax-flow": "^7.18.0",
+ "@babel/plugin-syntax-nullish-coalescing-operator": "^7.0.0",
+ "@babel/plugin-syntax-optional-chaining": "^7.0.0",
+ "@babel/plugin-transform-arrow-functions": "^7.0.0",
+ "@babel/plugin-transform-async-to-generator": "^7.20.0",
+ "@babel/plugin-transform-block-scoping": "^7.0.0",
+ "@babel/plugin-transform-classes": "^7.0.0",
+ "@babel/plugin-transform-computed-properties": "^7.0.0",
+ "@babel/plugin-transform-destructuring": "^7.20.0",
+ "@babel/plugin-transform-flow-strip-types": "^7.20.0",
+ "@babel/plugin-transform-function-name": "^7.0.0",
+ "@babel/plugin-transform-literals": "^7.0.0",
+ "@babel/plugin-transform-modules-commonjs": "^7.0.0",
+ "@babel/plugin-transform-named-capturing-groups-regex": "^7.0.0",
+ "@babel/plugin-transform-parameters": "^7.0.0",
+ "@babel/plugin-transform-react-display-name": "^7.0.0",
+ "@babel/plugin-transform-react-jsx": "^7.0.0",
+ "@babel/plugin-transform-react-jsx-self": "^7.0.0",
+ "@babel/plugin-transform-react-jsx-source": "^7.0.0",
+ "@babel/plugin-transform-runtime": "^7.0.0",
+ "@babel/plugin-transform-shorthand-properties": "^7.0.0",
+ "@babel/plugin-transform-spread": "^7.0.0",
+ "@babel/plugin-transform-sticky-regex": "^7.0.0",
+ "@babel/plugin-transform-typescript": "^7.5.0",
+ "@babel/plugin-transform-unicode-regex": "^7.0.0",
+ "@babel/template": "^7.0.0",
+ "babel-plugin-transform-flow-enums": "^0.0.2",
+ "react-refresh": "^0.4.0"
},
- "bin": {
- "getstorybook": "bin/index.js",
- "sb": "bin/index.js"
+ "engines": {
+ "node": ">=18"
},
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/storybook"
+ "peerDependencies": {
+ "@babel/core": "*"
}
},
- "node_modules/@storybook/cli/node_modules/agent-base": {
- "version": "5.1.1",
- "resolved": "https://registry.npmjs.org/agent-base/-/agent-base-5.1.1.tgz",
- "integrity": "sha512-TMeqbNl2fMW0nMjTEPOwe3J/PRFP4vqeoNuQMG0HlMrtm5QxKqdvAkZ1pRBQ/ulIyDD5Yq0nJ7YbdD8ey0TO3g==",
+ "node_modules/@react-native/babel-preset/node_modules/react-refresh": {
+ "version": "0.4.3",
+ "resolved": "https://registry.npmjs.org/react-refresh/-/react-refresh-0.4.3.tgz",
+ "integrity": "sha512-Hwln1VNuGl/6bVwnd0Xdn1e84gT/8T9aYNL+HAKDArLCS7LWjwr7StE30IEYbIkx0Vi3vs+coQxe+SQDbGbbpA==",
"dev": true,
"engines": {
- "node": ">= 6.0.0"
+ "node": ">=0.10.0"
}
},
- "node_modules/@storybook/cli/node_modules/chalk": {
- "version": "4.1.2",
- "resolved": "https://registry.npmjs.org/chalk/-/chalk-4.1.2.tgz",
- "integrity": "sha512-oKnbhFyRIXpUuez8iBMmyEa4nbj4IOQyuhc/wy9kY7/WVPcwIO9VA668Pu8RkO7+0G76SLROeyw9CpQ061i4mA==",
- "dev": true,
+ "node_modules/@react-native/codegen": {
+ "version": "0.73.2",
+ "resolved": "https://registry.npmjs.org/@react-native/codegen/-/codegen-0.73.2.tgz",
+ "integrity": "sha512-lfy8S7umhE3QLQG5ViC4wg5N1Z+E6RnaeIw8w1voroQsXXGPB72IBozh8dAHR3+ceTxIU0KX3A8OpJI8e1+HpQ==",
"dependencies": {
- "ansi-styles": "^4.1.0",
- "supports-color": "^7.1.0"
+ "@babel/parser": "^7.20.0",
+ "flow-parser": "^0.206.0",
+ "glob": "^7.1.1",
+ "invariant": "^2.2.4",
+ "jscodeshift": "^0.14.0",
+ "mkdirp": "^0.5.1",
+ "nullthrows": "^1.1.1"
},
"engines": {
- "node": ">=10"
+ "node": ">=18"
},
- "funding": {
- "url": "https://github.com/chalk/chalk?sponsor=1"
+ "peerDependencies": {
+ "@babel/preset-env": "^7.1.6"
}
},
- "node_modules/@storybook/cli/node_modules/cli-cursor": {
- "version": "3.1.0",
- "resolved": "https://registry.npmjs.org/cli-cursor/-/cli-cursor-3.1.0.tgz",
- "integrity": "sha512-I/zHAwsKf9FqGoXM4WWRACob9+SNukZTd94DWF57E4toouRulbCxcUh6RKUEOQlYTHJnzkPMySvPNaaSLNfLZw==",
- "dev": true,
+ "node_modules/@react-native/codegen/node_modules/mkdirp": {
+ "version": "0.5.6",
+ "resolved": "https://registry.npmjs.org/mkdirp/-/mkdirp-0.5.6.tgz",
+ "integrity": "sha512-FP+p8RB8OWpF3YZBCrP5gtADmtXApB5AMLn+vdyA+PyxCjrCs00mjyUozssO33cwDeT3wNGdLxJ5M//YqtHAJw==",
"dependencies": {
- "restore-cursor": "^3.1.0"
+ "minimist": "^1.2.6"
},
- "engines": {
- "node": ">=8"
+ "bin": {
+ "mkdirp": "bin/cmd.js"
}
},
- "node_modules/@storybook/cli/node_modules/commander": {
- "version": "6.2.1",
- "resolved": "https://registry.npmjs.org/commander/-/commander-6.2.1.tgz",
- "integrity": "sha512-U7VdrJFnJgo4xjrHpTzu0yrHPGImdsmD95ZlgYSEajAn2JKzDhDTPG9kBTefmObL2w/ngeZnilk+OV9CG3d7UA==",
- "dev": true,
+ "node_modules/@react-native/community-cli-plugin": {
+ "version": "0.73.14",
+ "resolved": "https://registry.npmjs.org/@react-native/community-cli-plugin/-/community-cli-plugin-0.73.14.tgz",
+ "integrity": "sha512-KzIwsTvAJrXPtwhGOSm+OcJH1B8TpY8cS4xxzu/e2qv3a2n4VLePHTPAfco1tmvekV8OHWvvD9JSIX7i2fB1gg==",
+ "dependencies": {
+ "@react-native-community/cli-server-api": "12.3.2",
+ "@react-native-community/cli-tools": "12.3.2",
+ "@react-native/dev-middleware": "0.73.7",
+ "@react-native/metro-babel-transformer": "0.73.14",
+ "chalk": "^4.0.0",
+ "execa": "^5.1.1",
+ "metro": "^0.80.3",
+ "metro-config": "^0.80.3",
+ "metro-core": "^0.80.3",
+ "node-fetch": "^2.2.0",
+ "readline": "^1.3.0"
+ },
"engines": {
- "node": ">= 6"
+ "node": ">=18"
}
},
- "node_modules/@storybook/cli/node_modules/cross-spawn": {
- "version": "7.0.3",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-7.0.3.tgz",
- "integrity": "sha512-iRDPJKUPVEND7dHPO8rkbOnPpyDygcDFtWjpeWNCgy8WP2rXcxXL8TskReQl6OrB2G7+UJrags1q15Fudc7G6w==",
- "dev": true,
+ "node_modules/@react-native/community-cli-plugin/node_modules/@react-native/babel-preset": {
+ "version": "0.73.20",
+ "resolved": "https://registry.npmjs.org/@react-native/babel-preset/-/babel-preset-0.73.20.tgz",
+ "integrity": "sha512-fU9NqkusbfFq71l4BWQfqqD/lLcLC0MZ++UYgieA3j8lIEppJTLVauv2RwtD2yltBkjebgYEC5Rwvt1l0MUBXw==",
"dependencies": {
- "path-key": "^3.1.0",
- "shebang-command": "^2.0.0",
- "which": "^2.0.1"
+ "@babel/core": "^7.20.0",
+ "@babel/plugin-proposal-async-generator-functions": "^7.0.0",
+ "@babel/plugin-proposal-class-properties": "^7.18.0",
+ "@babel/plugin-proposal-export-default-from": "^7.0.0",
+ "@babel/plugin-proposal-nullish-coalescing-operator": "^7.18.0",
+ "@babel/plugin-proposal-numeric-separator": "^7.0.0",
+ "@babel/plugin-proposal-object-rest-spread": "^7.20.0",
+ "@babel/plugin-proposal-optional-catch-binding": "^7.0.0",
+ "@babel/plugin-proposal-optional-chaining": "^7.20.0",
+ "@babel/plugin-syntax-dynamic-import": "^7.8.0",
+ "@babel/plugin-syntax-export-default-from": "^7.0.0",
+ "@babel/plugin-syntax-flow": "^7.18.0",
+ "@babel/plugin-syntax-nullish-coalescing-operator": "^7.0.0",
+ "@babel/plugin-syntax-optional-chaining": "^7.0.0",
+ "@babel/plugin-transform-arrow-functions": "^7.0.0",
+ "@babel/plugin-transform-async-to-generator": "^7.20.0",
+ "@babel/plugin-transform-block-scoping": "^7.0.0",
+ "@babel/plugin-transform-classes": "^7.0.0",
+ "@babel/plugin-transform-computed-properties": "^7.0.0",
+ "@babel/plugin-transform-destructuring": "^7.20.0",
+ "@babel/plugin-transform-flow-strip-types": "^7.20.0",
+ "@babel/plugin-transform-function-name": "^7.0.0",
+ "@babel/plugin-transform-literals": "^7.0.0",
+ "@babel/plugin-transform-modules-commonjs": "^7.0.0",
+ "@babel/plugin-transform-named-capturing-groups-regex": "^7.0.0",
+ "@babel/plugin-transform-parameters": "^7.0.0",
+ "@babel/plugin-transform-private-methods": "^7.22.5",
+ "@babel/plugin-transform-private-property-in-object": "^7.22.11",
+ "@babel/plugin-transform-react-display-name": "^7.0.0",
+ "@babel/plugin-transform-react-jsx": "^7.0.0",
+ "@babel/plugin-transform-react-jsx-self": "^7.0.0",
+ "@babel/plugin-transform-react-jsx-source": "^7.0.0",
+ "@babel/plugin-transform-runtime": "^7.0.0",
+ "@babel/plugin-transform-shorthand-properties": "^7.0.0",
+ "@babel/plugin-transform-spread": "^7.0.0",
+ "@babel/plugin-transform-sticky-regex": "^7.0.0",
+ "@babel/plugin-transform-typescript": "^7.5.0",
+ "@babel/plugin-transform-unicode-regex": "^7.0.0",
+ "@babel/template": "^7.0.0",
+ "@react-native/babel-plugin-codegen": "0.73.3",
+ "babel-plugin-transform-flow-enums": "^0.0.2",
+ "react-refresh": "^0.14.0"
},
"engines": {
- "node": ">= 8"
+ "node": ">=18"
+ },
+ "peerDependencies": {
+ "@babel/core": "*"
}
},
- "node_modules/@storybook/cli/node_modules/detect-indent": {
- "version": "6.1.0",
- "resolved": "https://registry.npmjs.org/detect-indent/-/detect-indent-6.1.0.tgz",
- "integrity": "sha512-reYkTUJAZb9gUuZ2RvVCNhVHdg62RHnJ7WJl8ftMi4diZ6NWlciOzQN88pUhSELEwflJht4oQDv0F0BMlwaYtA==",
- "dev": true,
+ "node_modules/@react-native/community-cli-plugin/node_modules/@react-native/metro-babel-transformer": {
+ "version": "0.73.14",
+ "resolved": "https://registry.npmjs.org/@react-native/metro-babel-transformer/-/metro-babel-transformer-0.73.14.tgz",
+ "integrity": "sha512-5wLeYw/lormpSqYfI9H/geZ/EtPmi+x5qLkEit15Q/70hkzYo/M+aWztUtbOITfgTEOP8d6ybROzoGsqgyZLcw==",
+ "dependencies": {
+ "@babel/core": "^7.20.0",
+ "@react-native/babel-preset": "0.73.20",
+ "hermes-parser": "0.15.0",
+ "nullthrows": "^1.1.1"
+ },
"engines": {
- "node": ">=8"
+ "node": ">=18"
+ },
+ "peerDependencies": {
+ "@babel/core": "*"
}
},
- "node_modules/@storybook/cli/node_modules/execa": {
+ "node_modules/@react-native/community-cli-plugin/node_modules/execa": {
"version": "5.1.1",
"resolved": "https://registry.npmjs.org/execa/-/execa-5.1.1.tgz",
"integrity": "sha512-8uSpZZocAZRBAPIEINJj3Lo9HyGitllczc27Eh5YYojjMFMn8yHMDMaUHE2Jqfq05D/wucwI4JGURyXt1vchyg==",
- "dev": true,
"dependencies": {
"cross-spawn": "^7.0.3",
"get-stream": "^6.0.0",
@@ -11643,41 +10582,10 @@
"url": "https://github.com/sindresorhus/execa?sponsor=1"
}
},
- "node_modules/@storybook/cli/node_modules/find-up": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/find-up/-/find-up-5.0.0.tgz",
- "integrity": "sha512-78/PXT1wlLLDgTzDs7sjq9hzz0vXD+zn+7wypEe4fXQxCmdmqfGsEPQxmiCSQI3ajFV91bVSsvNtrJRiW6nGng==",
- "dev": true,
- "dependencies": {
- "locate-path": "^6.0.0",
- "path-exists": "^4.0.0"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/@storybook/cli/node_modules/fs-extra": {
- "version": "11.2.0",
- "resolved": "https://registry.npmjs.org/fs-extra/-/fs-extra-11.2.0.tgz",
- "integrity": "sha512-PmDi3uwK5nFuXh7XDTlVnS17xJS7vW36is2+w3xcv8SVxiB4NyATf4ctkVY5bkSjX0Y4nbvZCq1/EjtEyr9ktw==",
- "dev": true,
- "dependencies": {
- "graceful-fs": "^4.2.0",
- "jsonfile": "^6.0.1",
- "universalify": "^2.0.0"
- },
- "engines": {
- "node": ">=14.14"
- }
- },
- "node_modules/@storybook/cli/node_modules/get-stream": {
+ "node_modules/@react-native/community-cli-plugin/node_modules/get-stream": {
"version": "6.0.1",
"resolved": "https://registry.npmjs.org/get-stream/-/get-stream-6.0.1.tgz",
"integrity": "sha512-ts6Wi+2j3jQjqi70w5AlN8DFnkSwC+MqmxEzdEALB2qXZYV3X/b1CTfgPLGJNMeAWxdPfU8FO1ms3NUfaHCPYg==",
- "dev": true,
"engines": {
"node": ">=10"
},
@@ -11685,62 +10593,18 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@storybook/cli/node_modules/glob": {
- "version": "7.2.3",
- "resolved": "https://registry.npmjs.org/glob/-/glob-7.2.3.tgz",
- "integrity": "sha512-nFR0zLpU2YCaRxwoCJvL6UvCH2JFyFVIvwTLsIf21AuHlMskA1hhTdk+LlYJtOlYt9v6dvszD2BGRqBL+iQK9Q==",
- "dev": true,
- "dependencies": {
- "fs.realpath": "^1.0.0",
- "inflight": "^1.0.4",
- "inherits": "2",
- "minimatch": "^3.1.1",
- "once": "^1.3.0",
- "path-is-absolute": "^1.0.0"
- },
- "engines": {
- "node": "*"
- },
- "funding": {
- "url": "https://github.com/sponsors/isaacs"
- }
- },
- "node_modules/@storybook/cli/node_modules/has-flag": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/has-flag/-/has-flag-4.0.0.tgz",
- "integrity": "sha512-EykJT/Q1KjTWctppgIAgfSO0tKVuZUjhgMr17kqTumMl6Afv3EISleU7qZUzoXDFTAHTDC4NOoG/ZxU3EvlMPQ==",
- "dev": true,
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@storybook/cli/node_modules/https-proxy-agent": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/https-proxy-agent/-/https-proxy-agent-4.0.0.tgz",
- "integrity": "sha512-zoDhWrkR3of1l9QAL8/scJZyLu8j/gBkcwcaQOZh7Gyh/+uJQzGVETdgT30akuwkpL8HTRfssqI3BZuV18teDg==",
- "dev": true,
- "dependencies": {
- "agent-base": "5",
- "debug": "4"
- },
- "engines": {
- "node": ">= 6.0.0"
- }
- },
- "node_modules/@storybook/cli/node_modules/human-signals": {
+ "node_modules/@react-native/community-cli-plugin/node_modules/human-signals": {
"version": "2.1.0",
"resolved": "https://registry.npmjs.org/human-signals/-/human-signals-2.1.0.tgz",
"integrity": "sha512-B4FFZ6q/T2jhhksgkbEW3HBvWIfDW85snkQgawt07S7J5QXTk6BkNV+0yAeZrM5QpMAdYlocGoljn0sJ/WQkFw==",
- "dev": true,
"engines": {
"node": ">=10.17.0"
}
},
- "node_modules/@storybook/cli/node_modules/is-stream": {
+ "node_modules/@react-native/community-cli-plugin/node_modules/is-stream": {
"version": "2.0.1",
"resolved": "https://registry.npmjs.org/is-stream/-/is-stream-2.0.1.tgz",
"integrity": "sha512-hFoiJiTl63nn+kstHGBtewWSKnQLpyb155KHheA1l39uvtO9nWIop1p3udqPcUd/xbF1VLMO4n7OI6p7RbngDg==",
- "dev": true,
"engines": {
"node": ">=8"
},
@@ -11748,1048 +10612,1142 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@storybook/cli/node_modules/jscodeshift": {
- "version": "0.15.2",
- "resolved": "https://registry.npmjs.org/jscodeshift/-/jscodeshift-0.15.2.tgz",
- "integrity": "sha512-FquR7Okgmc4Sd0aEDwqho3rEiKR3BdvuG9jfdHjLJ6JQoWSMpavug3AoIfnfWhxFlf+5pzQh8qjqz0DWFrNQzA==",
- "dev": true,
- "dependencies": {
- "@babel/core": "^7.23.0",
- "@babel/parser": "^7.23.0",
- "@babel/plugin-transform-class-properties": "^7.22.5",
- "@babel/plugin-transform-modules-commonjs": "^7.23.0",
- "@babel/plugin-transform-nullish-coalescing-operator": "^7.22.11",
- "@babel/plugin-transform-optional-chaining": "^7.23.0",
- "@babel/plugin-transform-private-methods": "^7.22.5",
- "@babel/preset-flow": "^7.22.15",
- "@babel/preset-typescript": "^7.23.0",
- "@babel/register": "^7.22.15",
- "babel-core": "^7.0.0-bridge.0",
- "chalk": "^4.1.2",
- "flow-parser": "0.*",
- "graceful-fs": "^4.2.4",
- "micromatch": "^4.0.4",
- "neo-async": "^2.5.0",
- "node-dir": "^0.1.17",
- "recast": "^0.23.3",
- "temp": "^0.8.4",
- "write-file-atomic": "^2.3.0"
- },
- "bin": {
- "jscodeshift": "bin/jscodeshift.js"
- },
- "peerDependencies": {
- "@babel/preset-env": "^7.1.6"
- },
- "peerDependenciesMeta": {
- "@babel/preset-env": {
- "optional": true
- }
+ "node_modules/@react-native/community-cli-plugin/node_modules/mimic-fn": {
+ "version": "2.1.0",
+ "resolved": "https://registry.npmjs.org/mimic-fn/-/mimic-fn-2.1.0.tgz",
+ "integrity": "sha512-OqbOk5oEQeAZ8WXWydlu9HJjz9WVdEIvamMCcXmuqUYjTknH/sqsWvhQ3vgwKFRR1HpjvNBKQ37nbJgYzGqGcg==",
+ "engines": {
+ "node": ">=6"
}
},
- "node_modules/@storybook/cli/node_modules/jsonfile": {
- "version": "6.1.0",
- "resolved": "https://registry.npmjs.org/jsonfile/-/jsonfile-6.1.0.tgz",
- "integrity": "sha512-5dgndWOriYSm5cnYaJNhalLNDKOqFwyDB/rr1E9ZsGciGvKPs8R2xYGCacuf3z6K1YKDz182fd+fY3cn3pMqXQ==",
- "dev": true,
+ "node_modules/@react-native/community-cli-plugin/node_modules/npm-run-path": {
+ "version": "4.0.1",
+ "resolved": "https://registry.npmjs.org/npm-run-path/-/npm-run-path-4.0.1.tgz",
+ "integrity": "sha512-S48WzZW777zhNIrn7gxOlISNAqi9ZC/uQFnRdbeIHhZhCA6UqpkOT8T1G7BvfdgP4Er8gF4sUbaS0i7QvIfCWw==",
"dependencies": {
- "universalify": "^2.0.0"
+ "path-key": "^3.0.0"
},
- "optionalDependencies": {
- "graceful-fs": "^4.1.6"
+ "engines": {
+ "node": ">=8"
}
},
- "node_modules/@storybook/cli/node_modules/locate-path": {
- "version": "6.0.0",
- "resolved": "https://registry.npmjs.org/locate-path/-/locate-path-6.0.0.tgz",
- "integrity": "sha512-iPZK6eYjbxRu3uB4/WZ3EsEIMJFMqAoopl3R+zuq0UjcAm/MO6KCweDgPfP3elTztoKP3KtnVHxTn2NHBSDVUw==",
- "dev": true,
+ "node_modules/@react-native/community-cli-plugin/node_modules/onetime": {
+ "version": "5.1.2",
+ "resolved": "https://registry.npmjs.org/onetime/-/onetime-5.1.2.tgz",
+ "integrity": "sha512-kbpaSSGJTWdAY5KPVeMOKXSrPtr8C8C7wodJbcsd51jRnmD+GZu8Y0VoU6Dm5Z4vWr0Ig/1NKuWRKf7j5aaYSg==",
"dependencies": {
- "p-locate": "^5.0.0"
+ "mimic-fn": "^2.1.0"
},
"engines": {
- "node": ">=10"
+ "node": ">=6"
},
"funding": {
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@storybook/cli/node_modules/mime": {
- "version": "2.6.0",
- "resolved": "https://registry.npmjs.org/mime/-/mime-2.6.0.tgz",
- "integrity": "sha512-USPkMeET31rOMiarsBNIHZKLGgvKc/LrjofAnBlOttf5ajRvqiRA8QsenbcooctK6d6Ts6aqZXBA+XbkKthiQg==",
- "dev": true,
- "bin": {
- "mime": "cli.js"
- },
+ "node_modules/@react-native/community-cli-plugin/node_modules/path-key": {
+ "version": "3.1.1",
+ "resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
+ "integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
"engines": {
- "node": ">=4.0.0"
+ "node": ">=8"
}
},
- "node_modules/@storybook/cli/node_modules/mimic-fn": {
- "version": "2.1.0",
- "resolved": "https://registry.npmjs.org/mimic-fn/-/mimic-fn-2.1.0.tgz",
- "integrity": "sha512-OqbOk5oEQeAZ8WXWydlu9HJjz9WVdEIvamMCcXmuqUYjTknH/sqsWvhQ3vgwKFRR1HpjvNBKQ37nbJgYzGqGcg==",
- "dev": true,
+ "node_modules/@react-native/debugger-frontend": {
+ "version": "0.73.3",
+ "resolved": "https://registry.npmjs.org/@react-native/debugger-frontend/-/debugger-frontend-0.73.3.tgz",
+ "integrity": "sha512-RgEKnWuoo54dh7gQhV7kvzKhXZEhpF9LlMdZolyhGxHsBqZ2gXdibfDlfcARFFifPIiaZ3lXuOVVa4ei+uPgTw==",
"engines": {
- "node": ">=6"
+ "node": ">=18"
}
},
- "node_modules/@storybook/cli/node_modules/npm-run-path": {
- "version": "4.0.1",
- "resolved": "https://registry.npmjs.org/npm-run-path/-/npm-run-path-4.0.1.tgz",
- "integrity": "sha512-S48WzZW777zhNIrn7gxOlISNAqi9ZC/uQFnRdbeIHhZhCA6UqpkOT8T1G7BvfdgP4Er8gF4sUbaS0i7QvIfCWw==",
- "dev": true,
+ "node_modules/@react-native/dev-middleware": {
+ "version": "0.73.7",
+ "resolved": "https://registry.npmjs.org/@react-native/dev-middleware/-/dev-middleware-0.73.7.tgz",
+ "integrity": "sha512-BZXpn+qKp/dNdr4+TkZxXDttfx8YobDh8MFHsMk9usouLm22pKgFIPkGBV0X8Do4LBkFNPGtrnsKkWk/yuUXKg==",
"dependencies": {
- "path-key": "^3.0.0"
+ "@isaacs/ttlcache": "^1.4.1",
+ "@react-native/debugger-frontend": "0.73.3",
+ "chrome-launcher": "^0.15.2",
+ "chromium-edge-launcher": "^1.0.0",
+ "connect": "^3.6.5",
+ "debug": "^2.2.0",
+ "node-fetch": "^2.2.0",
+ "open": "^7.0.3",
+ "serve-static": "^1.13.1",
+ "temp-dir": "^2.0.0"
},
"engines": {
- "node": ">=8"
+ "node": ">=18"
}
},
- "node_modules/@storybook/cli/node_modules/onetime": {
- "version": "5.1.2",
- "resolved": "https://registry.npmjs.org/onetime/-/onetime-5.1.2.tgz",
- "integrity": "sha512-kbpaSSGJTWdAY5KPVeMOKXSrPtr8C8C7wodJbcsd51jRnmD+GZu8Y0VoU6Dm5Z4vWr0Ig/1NKuWRKf7j5aaYSg==",
- "dev": true,
+ "node_modules/@react-native/dev-middleware/node_modules/debug": {
+ "version": "2.6.9",
+ "resolved": "https://registry.npmjs.org/debug/-/debug-2.6.9.tgz",
+ "integrity": "sha512-bC7ElrdJaJnPbAP+1EotYvqZsb3ecl5wi6Bfi6BJTUcNowp6cvspg0jXznRTKDjm/E7AdgFBVeAPVMNcKGsHMA==",
"dependencies": {
- "mimic-fn": "^2.1.0"
- },
- "engines": {
- "node": ">=6"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "ms": "2.0.0"
}
},
- "node_modules/@storybook/cli/node_modules/ora": {
- "version": "5.4.1",
- "resolved": "https://registry.npmjs.org/ora/-/ora-5.4.1.tgz",
- "integrity": "sha512-5b6Y85tPxZZ7QytO+BQzysW31HJku27cRIlkbAXaNx+BdcVi+LlRFmVXzeF6a7JCwJpyw5c4b+YSVImQIrBpuQ==",
- "dev": true,
+ "node_modules/@react-native/dev-middleware/node_modules/is-wsl": {
+ "version": "2.2.0",
+ "resolved": "https://registry.npmjs.org/is-wsl/-/is-wsl-2.2.0.tgz",
+ "integrity": "sha512-fKzAra0rGJUUBwGBgNkHZuToZcn+TtXHpeCgmkMJMMYx1sQDYaCSyjJBSCa2nH1DGm7s3n1oBnohoVTBaN7Lww==",
"dependencies": {
- "bl": "^4.1.0",
- "chalk": "^4.1.0",
- "cli-cursor": "^3.1.0",
- "cli-spinners": "^2.5.0",
- "is-interactive": "^1.0.0",
- "is-unicode-supported": "^0.1.0",
- "log-symbols": "^4.1.0",
- "strip-ansi": "^6.0.0",
- "wcwidth": "^1.0.1"
+ "is-docker": "^2.0.0"
},
"engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "node": ">=8"
}
},
- "node_modules/@storybook/cli/node_modules/p-locate": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/p-locate/-/p-locate-5.0.0.tgz",
- "integrity": "sha512-LaNjtRWUBY++zB5nE/NwcaoMylSPk+S+ZHNB1TzdbMJMny6dynpAGt7X/tl/QYq3TIeE6nxHppbo2LGymrG5Pw==",
- "dev": true,
+ "node_modules/@react-native/dev-middleware/node_modules/open": {
+ "version": "7.4.2",
+ "resolved": "https://registry.npmjs.org/open/-/open-7.4.2.tgz",
+ "integrity": "sha512-MVHddDVweXZF3awtlAS+6pgKLlm/JgxZ90+/NBurBoQctVOOB/zDdVjcyPzQ+0laDGbsWgrRkflI65sQeOgT9Q==",
"dependencies": {
- "p-limit": "^3.0.2"
+ "is-docker": "^2.0.0",
+ "is-wsl": "^2.1.1"
},
"engines": {
- "node": ">=10"
+ "node": ">=8"
},
"funding": {
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@storybook/cli/node_modules/path-exists": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/path-exists/-/path-exists-4.0.0.tgz",
- "integrity": "sha512-ak9Qy5Q7jYb2Wwcey5Fpvg2KoAc/ZIhLSLOSBmRmygPsGwkVVt0fZa0qrtMz+m6tJTAHfZQ8FnmB4MG4LWy7/w==",
- "dev": true,
+ "node_modules/@react-native/dev-middleware/node_modules/temp-dir": {
+ "version": "2.0.0",
+ "resolved": "https://registry.npmjs.org/temp-dir/-/temp-dir-2.0.0.tgz",
+ "integrity": "sha512-aoBAniQmmwtcKp/7BzsH8Cxzv8OL736p7v1ihGb5e9DJ9kTwGWHrQrVB5+lfVDzfGrdRzXch+ig7LHaY1JTOrg==",
"engines": {
"node": ">=8"
}
},
- "node_modules/@storybook/cli/node_modules/path-key": {
- "version": "3.1.1",
- "resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
- "integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
- "dev": true,
+ "node_modules/@react-native/gradle-plugin": {
+ "version": "0.73.4",
+ "resolved": "https://registry.npmjs.org/@react-native/gradle-plugin/-/gradle-plugin-0.73.4.tgz",
+ "integrity": "sha512-PMDnbsZa+tD55Ug+W8CfqXiGoGneSSyrBZCMb5JfiB3AFST3Uj5e6lw8SgI/B6SKZF7lG0BhZ6YHZsRZ5MlXmg==",
"engines": {
- "node": ">=8"
+ "node": ">=18"
}
},
- "node_modules/@storybook/cli/node_modules/prettier": {
- "version": "2.8.8",
- "resolved": "https://registry.npmjs.org/prettier/-/prettier-2.8.8.tgz",
- "integrity": "sha512-tdN8qQGvNjw4CHbY+XXk0JgCXn9QiF21a55rBe5LJAU+kDyC4WQn4+awm2Xfk2lQMk5fKup9XgzTZtGkjBdP9Q==",
- "dev": true,
- "bin": {
- "prettier": "bin-prettier.js"
- },
+ "node_modules/@react-native/js-polyfills": {
+ "version": "0.73.1",
+ "resolved": "https://registry.npmjs.org/@react-native/js-polyfills/-/js-polyfills-0.73.1.tgz",
+ "integrity": "sha512-ewMwGcumrilnF87H4jjrnvGZEaPFCAC4ebraEK+CurDDmwST/bIicI4hrOAv+0Z0F7DEK4O4H7r8q9vH7IbN4g==",
"engines": {
- "node": ">=10.13.0"
- },
- "funding": {
- "url": "https://github.com/prettier/prettier?sponsor=1"
+ "node": ">=18"
}
},
- "node_modules/@storybook/cli/node_modules/puppeteer-core": {
- "version": "2.1.1",
- "resolved": "https://registry.npmjs.org/puppeteer-core/-/puppeteer-core-2.1.1.tgz",
- "integrity": "sha512-n13AWriBMPYxnpbb6bnaY5YoY6rGj8vPLrz6CZF3o0qJNEwlcfJVxBzYZ0NJsQ21UbdJoijPCDrM++SUVEz7+w==",
+ "node_modules/@react-native/metro-babel-transformer": {
+ "version": "0.73.10",
+ "resolved": "https://registry.npmjs.org/@react-native/metro-babel-transformer/-/metro-babel-transformer-0.73.10.tgz",
+ "integrity": "sha512-g0q12s1VcrhI4ab9ZQzFHmvjkDW5PYdJd3LiyhIO3OAWLD7iNLWL5tJe9iA3n8rxbanmf1oauqlNY1UmkxhAvQ==",
"dev": true,
"dependencies": {
- "@types/mime-types": "^2.1.0",
- "debug": "^4.1.0",
- "extract-zip": "^1.6.6",
- "https-proxy-agent": "^4.0.0",
- "mime": "^2.0.3",
- "mime-types": "^2.1.25",
- "progress": "^2.0.1",
- "proxy-from-env": "^1.0.0",
- "rimraf": "^2.6.1",
- "ws": "^6.1.0"
+ "@babel/core": "^7.20.0",
+ "@react-native/babel-preset": "*",
+ "babel-preset-fbjs": "^3.4.0",
+ "hermes-parser": "0.15.0",
+ "nullthrows": "^1.1.1"
},
"engines": {
- "node": ">=8.16.0"
+ "node": ">=18"
+ },
+ "peerDependencies": {
+ "@babel/core": "*"
}
},
- "node_modules/@storybook/cli/node_modules/recast": {
- "version": "0.23.9",
- "resolved": "https://registry.npmjs.org/recast/-/recast-0.23.9.tgz",
- "integrity": "sha512-Hx/BGIbwj+Des3+xy5uAtAbdCyqK9y9wbBcDFDYanLS9JnMqf7OeF87HQwUimE87OEc72mr6tkKUKMBBL+hF9Q==",
+ "node_modules/@react-native/metro-config": {
+ "version": "0.73.4",
+ "resolved": "https://registry.npmjs.org/@react-native/metro-config/-/metro-config-0.73.4.tgz",
+ "integrity": "sha512-4IpWb9InOY23ssua6z/ho2B4uRqF4QaNHGg4aV3D/og5yiVF39GEm/REHU36i+KoHRO3GcB6DrI7N9KrcvgGBw==",
"dev": true,
- "license": "MIT",
"dependencies": {
- "ast-types": "^0.16.1",
- "esprima": "~4.0.0",
- "source-map": "~0.6.1",
- "tiny-invariant": "^1.3.3",
- "tslib": "^2.0.1"
+ "@react-native/js-polyfills": "0.73.1",
+ "@react-native/metro-babel-transformer": "0.73.14",
+ "metro-config": "^0.80.3",
+ "metro-runtime": "^0.80.3"
},
"engines": {
- "node": ">= 4"
+ "node": ">=18"
}
},
- "node_modules/@storybook/cli/node_modules/restore-cursor": {
- "version": "3.1.0",
- "resolved": "https://registry.npmjs.org/restore-cursor/-/restore-cursor-3.1.0.tgz",
- "integrity": "sha512-l+sSefzHpj5qimhFSE5a8nufZYAM3sBSVMAPtYkmC+4EH2anSGaEMXSD0izRQbu9nfyQ9y5JrVmp7E8oZrUjvA==",
+ "node_modules/@react-native/metro-config/node_modules/@react-native/babel-preset": {
+ "version": "0.73.20",
+ "resolved": "https://registry.npmjs.org/@react-native/babel-preset/-/babel-preset-0.73.20.tgz",
+ "integrity": "sha512-fU9NqkusbfFq71l4BWQfqqD/lLcLC0MZ++UYgieA3j8lIEppJTLVauv2RwtD2yltBkjebgYEC5Rwvt1l0MUBXw==",
"dev": true,
"dependencies": {
- "onetime": "^5.1.0",
- "signal-exit": "^3.0.2"
+ "@babel/core": "^7.20.0",
+ "@babel/plugin-proposal-async-generator-functions": "^7.0.0",
+ "@babel/plugin-proposal-class-properties": "^7.18.0",
+ "@babel/plugin-proposal-export-default-from": "^7.0.0",
+ "@babel/plugin-proposal-nullish-coalescing-operator": "^7.18.0",
+ "@babel/plugin-proposal-numeric-separator": "^7.0.0",
+ "@babel/plugin-proposal-object-rest-spread": "^7.20.0",
+ "@babel/plugin-proposal-optional-catch-binding": "^7.0.0",
+ "@babel/plugin-proposal-optional-chaining": "^7.20.0",
+ "@babel/plugin-syntax-dynamic-import": "^7.8.0",
+ "@babel/plugin-syntax-export-default-from": "^7.0.0",
+ "@babel/plugin-syntax-flow": "^7.18.0",
+ "@babel/plugin-syntax-nullish-coalescing-operator": "^7.0.0",
+ "@babel/plugin-syntax-optional-chaining": "^7.0.0",
+ "@babel/plugin-transform-arrow-functions": "^7.0.0",
+ "@babel/plugin-transform-async-to-generator": "^7.20.0",
+ "@babel/plugin-transform-block-scoping": "^7.0.0",
+ "@babel/plugin-transform-classes": "^7.0.0",
+ "@babel/plugin-transform-computed-properties": "^7.0.0",
+ "@babel/plugin-transform-destructuring": "^7.20.0",
+ "@babel/plugin-transform-flow-strip-types": "^7.20.0",
+ "@babel/plugin-transform-function-name": "^7.0.0",
+ "@babel/plugin-transform-literals": "^7.0.0",
+ "@babel/plugin-transform-modules-commonjs": "^7.0.0",
+ "@babel/plugin-transform-named-capturing-groups-regex": "^7.0.0",
+ "@babel/plugin-transform-parameters": "^7.0.0",
+ "@babel/plugin-transform-private-methods": "^7.22.5",
+ "@babel/plugin-transform-private-property-in-object": "^7.22.11",
+ "@babel/plugin-transform-react-display-name": "^7.0.0",
+ "@babel/plugin-transform-react-jsx": "^7.0.0",
+ "@babel/plugin-transform-react-jsx-self": "^7.0.0",
+ "@babel/plugin-transform-react-jsx-source": "^7.0.0",
+ "@babel/plugin-transform-runtime": "^7.0.0",
+ "@babel/plugin-transform-shorthand-properties": "^7.0.0",
+ "@babel/plugin-transform-spread": "^7.0.0",
+ "@babel/plugin-transform-sticky-regex": "^7.0.0",
+ "@babel/plugin-transform-typescript": "^7.5.0",
+ "@babel/plugin-transform-unicode-regex": "^7.0.0",
+ "@babel/template": "^7.0.0",
+ "@react-native/babel-plugin-codegen": "0.73.3",
+ "babel-plugin-transform-flow-enums": "^0.0.2",
+ "react-refresh": "^0.14.0"
},
"engines": {
- "node": ">=8"
+ "node": ">=18"
+ },
+ "peerDependencies": {
+ "@babel/core": "*"
}
},
- "node_modules/@storybook/cli/node_modules/rimraf": {
- "version": "2.7.1",
- "resolved": "https://registry.npmjs.org/rimraf/-/rimraf-2.7.1.tgz",
- "integrity": "sha512-uWjbaKIK3T1OSVptzX7Nl6PvQ3qAGtKEtVRjRuazjfL3Bx5eI409VZSqgND+4UNnmzLVdPj9FqFJNPqBZFve4w==",
+ "node_modules/@react-native/metro-config/node_modules/@react-native/metro-babel-transformer": {
+ "version": "0.73.14",
+ "resolved": "https://registry.npmjs.org/@react-native/metro-babel-transformer/-/metro-babel-transformer-0.73.14.tgz",
+ "integrity": "sha512-5wLeYw/lormpSqYfI9H/geZ/EtPmi+x5qLkEit15Q/70hkzYo/M+aWztUtbOITfgTEOP8d6ybROzoGsqgyZLcw==",
"dev": true,
"dependencies": {
- "glob": "^7.1.3"
+ "@babel/core": "^7.20.0",
+ "@react-native/babel-preset": "0.73.20",
+ "hermes-parser": "0.15.0",
+ "nullthrows": "^1.1.1"
},
- "bin": {
- "rimraf": "bin.js"
+ "engines": {
+ "node": ">=18"
+ },
+ "peerDependencies": {
+ "@babel/core": "*"
}
},
- "node_modules/@storybook/cli/node_modules/shebang-command": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/shebang-command/-/shebang-command-2.0.0.tgz",
- "integrity": "sha512-kHxr2zZpYtdmrN1qDjrrX/Z1rR1kG8Dx+gkpK1G4eXmvXswmcE1hTWBWYUzlraYw1/yZp6YuDY77YtvbN0dmDA==",
- "dev": true,
+ "node_modules/@react-native/normalize-color": {
+ "version": "2.1.0",
+ "resolved": "https://registry.npmjs.org/@react-native/normalize-color/-/normalize-color-2.1.0.tgz",
+ "integrity": "sha512-Z1jQI2NpdFJCVgpY+8Dq/Bt3d+YUi1928Q+/CZm/oh66fzM0RUl54vvuXlPJKybH4pdCZey1eDTPaLHkMPNgWA=="
+ },
+ "node_modules/@react-native/normalize-colors": {
+ "version": "0.73.2",
+ "resolved": "https://registry.npmjs.org/@react-native/normalize-colors/-/normalize-colors-0.73.2.tgz",
+ "integrity": "sha512-bRBcb2T+I88aG74LMVHaKms2p/T8aQd8+BZ7LuuzXlRfog1bMWWn/C5i0HVuvW4RPtXQYgIlGiXVDy9Ir1So/w=="
+ },
+ "node_modules/@react-native/virtualized-lists": {
+ "version": "0.73.4",
+ "resolved": "https://registry.npmjs.org/@react-native/virtualized-lists/-/virtualized-lists-0.73.4.tgz",
+ "integrity": "sha512-HpmLg1FrEiDtrtAbXiwCgXFYyloK/dOIPIuWW3fsqukwJEWAiTzm1nXGJ7xPU5XTHiWZ4sKup5Ebaj8z7iyWog==",
"dependencies": {
- "shebang-regex": "^3.0.0"
+ "invariant": "^2.2.4",
+ "nullthrows": "^1.1.1"
},
"engines": {
- "node": ">=8"
+ "node": ">=18"
+ },
+ "peerDependencies": {
+ "react-native": "*"
}
},
- "node_modules/@storybook/cli/node_modules/shebang-regex": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/shebang-regex/-/shebang-regex-3.0.0.tgz",
- "integrity": "sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==",
- "dev": true,
- "engines": {
- "node": ">=8"
+ "node_modules/@react-navigation/core": {
+ "version": "5.12.0",
+ "resolved": "https://registry.npmjs.org/@react-navigation/core/-/core-5.12.0.tgz",
+ "integrity": "sha512-CTmYrFXCZwInN40CpEzkPxhrpzujj20qvsUgpH05+oO1flwsnaJsyBfYawIcTS62/1/Z6SAM7iW5PbKk+qw9iQ==",
+ "dependencies": {
+ "@react-navigation/routers": "^5.4.9",
+ "escape-string-regexp": "^4.0.0",
+ "nanoid": "^3.1.9",
+ "query-string": "^6.13.1",
+ "react-is": "^16.13.0",
+ "use-subscription": "^1.4.0"
+ },
+ "peerDependencies": {
+ "react": "*"
}
},
- "node_modules/@storybook/cli/node_modules/strip-json-comments": {
- "version": "3.1.1",
- "resolved": "https://registry.npmjs.org/strip-json-comments/-/strip-json-comments-3.1.1.tgz",
- "integrity": "sha512-6fPc+R4ihwqP6N/aIv2f1gMH8lOVtWQHoqC4yK6oSDVVocumAsfCqjkXnqiYMhmMwS/mEHLp7Vehlt3ql6lEig==",
- "dev": true,
+ "node_modules/@react-navigation/core/node_modules/escape-string-regexp": {
+ "version": "4.0.0",
+ "resolved": "https://registry.npmjs.org/escape-string-regexp/-/escape-string-regexp-4.0.0.tgz",
+ "integrity": "sha512-TtpcNJ3XAzx3Gq8sWRzJaVajRs0uVxA2YAkdb1jm2YkPz4G6egUFAyA3n5vtEIZefPk5Wa4UXbKuS5fKkJWdgA==",
"engines": {
- "node": ">=8"
+ "node": ">=10"
},
"funding": {
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@storybook/cli/node_modules/supports-color": {
- "version": "7.2.0",
- "resolved": "https://registry.npmjs.org/supports-color/-/supports-color-7.2.0.tgz",
- "integrity": "sha512-qpCAvRl9stuOHveKsn7HncJRvv501qIacKzQlO/+Lwxc9+0q2wLyv4Dfvt80/DPn2pqOBsJdDiogXGR9+OvwRw==",
- "dev": true,
- "dependencies": {
- "has-flag": "^4.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@storybook/cli/node_modules/universalify": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/universalify/-/universalify-2.0.1.tgz",
- "integrity": "sha512-gptHNQghINnc/vTGIk0SOFGFNXw7JVrlRUtConJRlvaw6DuX0wO5Jeko9sWrMBhh+PsYAZ7oXAiOnf/UKogyiw==",
- "dev": true,
- "engines": {
- "node": ">= 10.0.0"
+ "node_modules/@react-navigation/elements": {
+ "version": "1.3.18",
+ "resolved": "https://registry.npmjs.org/@react-navigation/elements/-/elements-1.3.18.tgz",
+ "integrity": "sha512-/0hwnJkrr415yP0Hf4PjUKgGyfshrvNUKFXN85Mrt1gY49hy9IwxZgrrxlh0THXkPeq8q4VWw44eHDfAcQf20Q==",
+ "peerDependencies": {
+ "@react-navigation/native": "^6.0.0",
+ "react": "*",
+ "react-native": "*",
+ "react-native-safe-area-context": ">= 3.0.0"
}
},
- "node_modules/@storybook/cli/node_modules/which": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
- "integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
- "dev": true,
+ "node_modules/@react-navigation/native": {
+ "version": "6.0.14",
+ "resolved": "https://registry.npmjs.org/@react-navigation/native/-/native-6.0.14.tgz",
+ "integrity": "sha512-Z95bJrRkZerBJq6Qc/xjA/kibPpB+UvPeMWS1CBhRF8FaX1483UdHqPqSbW879tPwjP2R4XfoA4dtoEHswrOjA==",
"dependencies": {
- "isexe": "^2.0.0"
- },
- "bin": {
- "node-which": "bin/node-which"
+ "@react-navigation/core": "^6.4.1",
+ "escape-string-regexp": "^4.0.0",
+ "fast-deep-equal": "^3.1.3",
+ "nanoid": "^3.1.23"
},
- "engines": {
- "node": ">= 8"
+ "peerDependencies": {
+ "react": "*",
+ "react-native": "*"
}
},
- "node_modules/@storybook/cli/node_modules/ws": {
- "version": "6.2.3",
- "resolved": "https://registry.npmjs.org/ws/-/ws-6.2.3.tgz",
- "integrity": "sha512-jmTjYU0j60B+vHey6TfR3Z7RD61z/hmxBS3VMSGIrroOWXQEneK1zNuotOUrGyBHQj0yrpsLHPWtigEFd13ndA==",
- "dev": true,
+ "node_modules/@react-navigation/native/node_modules/@react-navigation/core": {
+ "version": "6.4.17",
+ "resolved": "https://registry.npmjs.org/@react-navigation/core/-/core-6.4.17.tgz",
+ "integrity": "sha512-Nd76EpomzChWAosGqWOYE3ItayhDzIEzzZsT7PfGcRFDgW5miHV2t4MZcq9YIK4tzxZjVVpYbIynOOQQd1e0Cg==",
"license": "MIT",
"dependencies": {
- "async-limiter": "~1.0.0"
+ "@react-navigation/routers": "^6.1.9",
+ "escape-string-regexp": "^4.0.0",
+ "nanoid": "^3.1.23",
+ "query-string": "^7.1.3",
+ "react-is": "^16.13.0",
+ "use-latest-callback": "^0.2.1"
+ },
+ "peerDependencies": {
+ "react": "*"
}
},
- "node_modules/@storybook/client-logger": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/client-logger/-/client-logger-7.6.15.tgz",
- "integrity": "sha512-n+K8IqnombqiQNnywVovS+lK61tvv/XSfgPt0cgvoF/hJZB0VDOMRjWsV+v9qQpj1TQEl1lLWeJwZMthTWupJA==",
- "dev": true,
+ "node_modules/@react-navigation/native/node_modules/@react-navigation/routers": {
+ "version": "6.1.9",
+ "resolved": "https://registry.npmjs.org/@react-navigation/routers/-/routers-6.1.9.tgz",
+ "integrity": "sha512-lTM8gSFHSfkJvQkxacGM6VJtBt61ip2XO54aNfswD+KMw6eeZ4oehl7m0me3CR9hnDE4+60iAZR8sAhvCiI3NA==",
"dependencies": {
- "@storybook/global": "^5.0.0"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/storybook"
+ "nanoid": "^3.1.23"
}
},
- "node_modules/@storybook/codemod": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/codemod/-/codemod-7.6.15.tgz",
- "integrity": "sha512-NiEbTLCdacj6TMxC7G49IImXeMzkG8wpPr8Ayxm9HeG6q5UkiF5/DiZdqbJm2zaosOsOKWwvXg1t6Pq6Nivytg==",
- "dev": true,
- "dependencies": {
- "@babel/core": "^7.23.2",
- "@babel/preset-env": "^7.23.2",
- "@babel/types": "^7.23.0",
- "@storybook/csf": "^0.1.2",
- "@storybook/csf-tools": "7.6.15",
- "@storybook/node-logger": "7.6.15",
- "@storybook/types": "7.6.15",
- "@types/cross-spawn": "^6.0.2",
- "cross-spawn": "^7.0.3",
- "globby": "^11.0.2",
- "jscodeshift": "^0.15.1",
- "lodash": "^4.17.21",
- "prettier": "^2.8.0",
- "recast": "^0.23.1"
+ "node_modules/@react-navigation/native/node_modules/escape-string-regexp": {
+ "version": "4.0.0",
+ "resolved": "https://registry.npmjs.org/escape-string-regexp/-/escape-string-regexp-4.0.0.tgz",
+ "integrity": "sha512-TtpcNJ3XAzx3Gq8sWRzJaVajRs0uVxA2YAkdb1jm2YkPz4G6egUFAyA3n5vtEIZefPk5Wa4UXbKuS5fKkJWdgA==",
+ "engines": {
+ "node": ">=10"
},
"funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/storybook"
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@storybook/codemod/node_modules/chalk": {
- "version": "4.1.2",
- "resolved": "https://registry.npmjs.org/chalk/-/chalk-4.1.2.tgz",
- "integrity": "sha512-oKnbhFyRIXpUuez8iBMmyEa4nbj4IOQyuhc/wy9kY7/WVPcwIO9VA668Pu8RkO7+0G76SLROeyw9CpQ061i4mA==",
- "dev": true,
+ "node_modules/@react-navigation/native/node_modules/query-string": {
+ "version": "7.1.3",
+ "resolved": "https://registry.npmjs.org/query-string/-/query-string-7.1.3.tgz",
+ "integrity": "sha512-hh2WYhq4fi8+b+/2Kg9CEge4fDPvHS534aOOvOZeQ3+Vf2mCFsaFBYj0i+iXcAq6I9Vzp5fjMFBlONvayDC1qg==",
"dependencies": {
- "ansi-styles": "^4.1.0",
- "supports-color": "^7.1.0"
+ "decode-uri-component": "^0.2.2",
+ "filter-obj": "^1.1.0",
+ "split-on-first": "^1.0.0",
+ "strict-uri-encode": "^2.0.0"
},
"engines": {
- "node": ">=10"
+ "node": ">=6"
},
"funding": {
- "url": "https://github.com/chalk/chalk?sponsor=1"
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@storybook/codemod/node_modules/cross-spawn": {
- "version": "7.0.3",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-7.0.3.tgz",
- "integrity": "sha512-iRDPJKUPVEND7dHPO8rkbOnPpyDygcDFtWjpeWNCgy8WP2rXcxXL8TskReQl6OrB2G7+UJrags1q15Fudc7G6w==",
- "dev": true,
+ "node_modules/@react-navigation/routers": {
+ "version": "5.4.9",
+ "resolved": "https://registry.npmjs.org/@react-navigation/routers/-/routers-5.4.9.tgz",
+ "integrity": "sha512-dYD5qrIKUmuBEp+O98hB0tDYpEsGQgCQFQgMEoFKBmVVhx2JnJJ1zxRjU7xWcCU4VdBA8IOowgHQHJsVNKYyrg==",
"dependencies": {
- "path-key": "^3.1.0",
- "shebang-command": "^2.0.0",
- "which": "^2.0.1"
+ "nanoid": "^3.1.9"
+ }
+ },
+ "node_modules/@react-navigation/stack": {
+ "version": "6.3.5",
+ "resolved": "https://registry.npmjs.org/@react-navigation/stack/-/stack-6.3.5.tgz",
+ "integrity": "sha512-G706Ow+8fhiLT5Cf48566YsjboU5h5ll9nva90xX3br7V6x0JMoGMvXjvbORZyzLuUytQO3mf0T3nVUuO52vZQ==",
+ "dependencies": {
+ "@react-navigation/elements": "^1.3.7",
+ "color": "^4.2.3",
+ "warn-once": "^0.1.0"
},
- "engines": {
- "node": ">= 8"
+ "peerDependencies": {
+ "@react-navigation/native": "^6.0.0",
+ "react": "*",
+ "react-native": "*",
+ "react-native-gesture-handler": ">= 1.0.0",
+ "react-native-safe-area-context": ">= 3.0.0",
+ "react-native-screens": ">= 3.0.0"
}
},
- "node_modules/@storybook/codemod/node_modules/has-flag": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/has-flag/-/has-flag-4.0.0.tgz",
- "integrity": "sha512-EykJT/Q1KjTWctppgIAgfSO0tKVuZUjhgMr17kqTumMl6Afv3EISleU7qZUzoXDFTAHTDC4NOoG/ZxU3EvlMPQ==",
- "dev": true,
- "engines": {
- "node": ">=8"
+ "node_modules/@react-spring/animated": {
+ "version": "9.5.5",
+ "resolved": "https://registry.npmjs.org/@react-spring/animated/-/animated-9.5.5.tgz",
+ "integrity": "sha512-glzViz7syQ3CE6BQOwAyr75cgh0qsihm5lkaf24I0DfU63cMm/3+br299UEYkuaHNmfDfM414uktiPlZCNJbQA==",
+ "dependencies": {
+ "@react-spring/shared": "~9.5.5",
+ "@react-spring/types": "~9.5.5"
+ },
+ "peerDependencies": {
+ "react": "^16.8.0 || ^17.0.0 || ^18.0.0"
}
},
- "node_modules/@storybook/codemod/node_modules/jscodeshift": {
- "version": "0.15.2",
- "resolved": "https://registry.npmjs.org/jscodeshift/-/jscodeshift-0.15.2.tgz",
- "integrity": "sha512-FquR7Okgmc4Sd0aEDwqho3rEiKR3BdvuG9jfdHjLJ6JQoWSMpavug3AoIfnfWhxFlf+5pzQh8qjqz0DWFrNQzA==",
- "dev": true,
+ "node_modules/@react-spring/core": {
+ "version": "9.5.5",
+ "resolved": "https://registry.npmjs.org/@react-spring/core/-/core-9.5.5.tgz",
+ "integrity": "sha512-shaJYb3iX18Au6gkk8ahaF0qx0LpS0Yd+ajb4asBaAQf6WPGuEdJsbsNSgei1/O13JyEATsJl20lkjeslJPMYA==",
"dependencies": {
- "@babel/core": "^7.23.0",
- "@babel/parser": "^7.23.0",
- "@babel/plugin-transform-class-properties": "^7.22.5",
- "@babel/plugin-transform-modules-commonjs": "^7.23.0",
- "@babel/plugin-transform-nullish-coalescing-operator": "^7.22.11",
- "@babel/plugin-transform-optional-chaining": "^7.23.0",
- "@babel/plugin-transform-private-methods": "^7.22.5",
- "@babel/preset-flow": "^7.22.15",
- "@babel/preset-typescript": "^7.23.0",
- "@babel/register": "^7.22.15",
- "babel-core": "^7.0.0-bridge.0",
- "chalk": "^4.1.2",
- "flow-parser": "0.*",
- "graceful-fs": "^4.2.4",
- "micromatch": "^4.0.4",
- "neo-async": "^2.5.0",
- "node-dir": "^0.1.17",
- "recast": "^0.23.3",
- "temp": "^0.8.4",
- "write-file-atomic": "^2.3.0"
+ "@react-spring/animated": "~9.5.5",
+ "@react-spring/rafz": "~9.5.5",
+ "@react-spring/shared": "~9.5.5",
+ "@react-spring/types": "~9.5.5"
},
- "bin": {
- "jscodeshift": "bin/jscodeshift.js"
+ "funding": {
+ "type": "opencollective",
+ "url": "https://opencollective.com/react-spring/donate"
},
"peerDependencies": {
- "@babel/preset-env": "^7.1.6"
+ "react": "^16.8.0 || ^17.0.0 || ^18.0.0"
+ }
+ },
+ "node_modules/@react-spring/rafz": {
+ "version": "9.5.5",
+ "resolved": "https://registry.npmjs.org/@react-spring/rafz/-/rafz-9.5.5.tgz",
+ "integrity": "sha512-F/CLwB0d10jL6My5vgzRQxCNY2RNyDJZedRBK7FsngdCmzoq3V4OqqNc/9voJb9qRC2wd55oGXUeXv2eIaFmsw=="
+ },
+ "node_modules/@react-spring/shared": {
+ "version": "9.5.5",
+ "resolved": "https://registry.npmjs.org/@react-spring/shared/-/shared-9.5.5.tgz",
+ "integrity": "sha512-YwW70Pa/YXPOwTutExHZmMQSHcNC90kJOnNR4G4mCDNV99hE98jWkIPDOsgqbYx3amIglcFPiYKMaQuGdr8dyQ==",
+ "dependencies": {
+ "@react-spring/rafz": "~9.5.5",
+ "@react-spring/types": "~9.5.5"
},
- "peerDependenciesMeta": {
- "@babel/preset-env": {
- "optional": true
- }
+ "peerDependencies": {
+ "react": "^16.8.0 || ^17.0.0 || ^18.0.0"
}
},
- "node_modules/@storybook/codemod/node_modules/path-key": {
- "version": "3.1.1",
- "resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
- "integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
- "dev": true,
- "engines": {
- "node": ">=8"
+ "node_modules/@react-spring/types": {
+ "version": "9.5.5",
+ "resolved": "https://registry.npmjs.org/@react-spring/types/-/types-9.5.5.tgz",
+ "integrity": "sha512-7I/qY8H7Enwasxr4jU6WmtNK+RZ4Z/XvSlDvjXFVe7ii1x0MoSlkw6pD7xuac8qrHQRm9BTcbZNyeeKApYsvCg=="
+ },
+ "node_modules/@react-spring/web": {
+ "version": "9.5.5",
+ "resolved": "https://registry.npmjs.org/@react-spring/web/-/web-9.5.5.tgz",
+ "integrity": "sha512-+moT8aDX/ho/XAhU+HRY9m0LVV9y9CK6NjSRaI+30Re150pB3iEip6QfnF4qnhSCQ5drpMF0XRXHgOTY/xbtFw==",
+ "dependencies": {
+ "@react-spring/animated": "~9.5.5",
+ "@react-spring/core": "~9.5.5",
+ "@react-spring/shared": "~9.5.5",
+ "@react-spring/types": "~9.5.5"
+ },
+ "peerDependencies": {
+ "react": "^16.8.0 || ^17.0.0 || ^18.0.0",
+ "react-dom": "^16.8.0 || ^17.0.0 || ^18.0.0"
}
},
- "node_modules/@storybook/codemod/node_modules/prettier": {
- "version": "2.8.8",
- "resolved": "https://registry.npmjs.org/prettier/-/prettier-2.8.8.tgz",
- "integrity": "sha512-tdN8qQGvNjw4CHbY+XXk0JgCXn9QiF21a55rBe5LJAU+kDyC4WQn4+awm2Xfk2lQMk5fKup9XgzTZtGkjBdP9Q==",
+ "node_modules/@remote-ui/rpc": {
+ "version": "1.4.5",
+ "resolved": "https://registry.npmjs.org/@remote-ui/rpc/-/rpc-1.4.5.tgz",
+ "integrity": "sha512-Cr+06niG/vmE4A9YsmaKngRuuVSWKMY42NMwtZfy+gctRWGu6Wj9BWuMJg5CEp+JTkRBPToqT5rqnrg1G/Wvow==",
+ "license": "MIT"
+ },
+ "node_modules/@samverschueren/stream-to-observable": {
+ "version": "0.3.1",
+ "resolved": "https://registry.npmjs.org/@samverschueren/stream-to-observable/-/stream-to-observable-0.3.1.tgz",
+ "integrity": "sha512-c/qwwcHyafOQuVQJj0IlBjf5yYgBI7YPJ77k4fOJYesb41jio65eaJODRUmfYKhTOFBrIZ66kgvGPlNbjuoRdQ==",
"dev": true,
- "bin": {
- "prettier": "bin-prettier.js"
+ "dependencies": {
+ "any-observable": "^0.3.0"
},
"engines": {
- "node": ">=10.13.0"
+ "node": ">=6"
},
- "funding": {
- "url": "https://github.com/prettier/prettier?sponsor=1"
+ "peerDependenciesMeta": {
+ "rxjs": {
+ "optional": true
+ },
+ "zen-observable": {
+ "optional": true
+ }
}
},
- "node_modules/@storybook/codemod/node_modules/recast": {
- "version": "0.23.9",
- "resolved": "https://registry.npmjs.org/recast/-/recast-0.23.9.tgz",
- "integrity": "sha512-Hx/BGIbwj+Des3+xy5uAtAbdCyqK9y9wbBcDFDYanLS9JnMqf7OeF87HQwUimE87OEc72mr6tkKUKMBBL+hF9Q==",
- "dev": true,
+ "node_modules/@sentry-internal/tracing": {
+ "version": "7.120.1",
+ "resolved": "https://registry.npmjs.org/@sentry-internal/tracing/-/tracing-7.120.1.tgz",
+ "integrity": "sha512-MwZlhQY27oM4V05m2Q46WB2F7jqFu8fewg14yRcjCuK3tdxvQoLsXOEPMZxLxpoXPTqPCm3Ig7mA4GwdlCL41w==",
"license": "MIT",
"dependencies": {
- "ast-types": "^0.16.1",
- "esprima": "~4.0.0",
- "source-map": "~0.6.1",
- "tiny-invariant": "^1.3.3",
- "tslib": "^2.0.1"
+ "@sentry/core": "7.120.1",
+ "@sentry/types": "7.120.1",
+ "@sentry/utils": "7.120.1"
},
"engines": {
- "node": ">= 4"
+ "node": ">=8"
}
},
- "node_modules/@storybook/codemod/node_modules/shebang-command": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/shebang-command/-/shebang-command-2.0.0.tgz",
- "integrity": "sha512-kHxr2zZpYtdmrN1qDjrrX/Z1rR1kG8Dx+gkpK1G4eXmvXswmcE1hTWBWYUzlraYw1/yZp6YuDY77YtvbN0dmDA==",
- "dev": true,
+ "node_modules/@sentry/core": {
+ "version": "7.120.1",
+ "resolved": "https://registry.npmjs.org/@sentry/core/-/core-7.120.1.tgz",
+ "integrity": "sha512-tXpJlf/8ngsSCpcRD+4DDvh4TqUbY0MlvE9Mpc/jO5GgYl/goAH2H1COw6W/UNfkr/l80P2jejS0HLPk0moi0A==",
+ "license": "MIT",
"dependencies": {
- "shebang-regex": "^3.0.0"
+ "@sentry/types": "7.120.1",
+ "@sentry/utils": "7.120.1"
},
"engines": {
"node": ">=8"
}
},
- "node_modules/@storybook/codemod/node_modules/shebang-regex": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/shebang-regex/-/shebang-regex-3.0.0.tgz",
- "integrity": "sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==",
- "dev": true,
+ "node_modules/@sentry/integrations": {
+ "version": "7.120.1",
+ "resolved": "https://registry.npmjs.org/@sentry/integrations/-/integrations-7.120.1.tgz",
+ "integrity": "sha512-dshhLZUN+pYpyZiS5QRYKaYSqvWYtmsbwmBlH4SCGOnN9sbY4nZn0h8njr+xKT8UFnPxoTlbZmkcrVY3qPVMfg==",
+ "license": "MIT",
+ "dependencies": {
+ "@sentry/core": "7.120.1",
+ "@sentry/types": "7.120.1",
+ "@sentry/utils": "7.120.1",
+ "localforage": "^1.8.1"
+ },
"engines": {
"node": ">=8"
}
},
- "node_modules/@storybook/codemod/node_modules/supports-color": {
- "version": "7.2.0",
- "resolved": "https://registry.npmjs.org/supports-color/-/supports-color-7.2.0.tgz",
- "integrity": "sha512-qpCAvRl9stuOHveKsn7HncJRvv501qIacKzQlO/+Lwxc9+0q2wLyv4Dfvt80/DPn2pqOBsJdDiogXGR9+OvwRw==",
- "dev": true,
+ "node_modules/@sentry/node": {
+ "version": "7.120.1",
+ "resolved": "https://registry.npmjs.org/@sentry/node/-/node-7.120.1.tgz",
+ "integrity": "sha512-YF/TDUCtUOQeUMwL4vcUWGNv/8Qz9624xBnaL8nXW888xNBoSRr2vH/zMrmTup5zfmWAh9lVbp98BZFF6F0WJg==",
+ "license": "MIT",
"dependencies": {
- "has-flag": "^4.0.0"
+ "@sentry-internal/tracing": "7.120.1",
+ "@sentry/core": "7.120.1",
+ "@sentry/integrations": "7.120.1",
+ "@sentry/types": "7.120.1",
+ "@sentry/utils": "7.120.1"
},
"engines": {
"node": ">=8"
}
},
- "node_modules/@storybook/codemod/node_modules/which": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
- "integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
- "dev": true,
+ "node_modules/@sentry/types": {
+ "version": "7.120.1",
+ "resolved": "https://registry.npmjs.org/@sentry/types/-/types-7.120.1.tgz",
+ "integrity": "sha512-f/WT7YUH8SA2Jhez/hYz/dA351AJqr1Eht/URUdYsqMFecXr/blAcNKRVFccSsvQeTqWVV9HVQ9BXUSjPJOvFA==",
+ "license": "MIT",
+ "engines": {
+ "node": ">=8"
+ }
+ },
+ "node_modules/@sentry/utils": {
+ "version": "7.120.1",
+ "resolved": "https://registry.npmjs.org/@sentry/utils/-/utils-7.120.1.tgz",
+ "integrity": "sha512-4boeo5Y3zw3gFrWZmPHsYOIlTh//eBaGBgWL25FqLbLObO23gFE86G6O6knP1Gamm1DGX2IWH7w4MChYuBm6tA==",
+ "license": "MIT",
"dependencies": {
- "isexe": "^2.0.0"
- },
- "bin": {
- "node-which": "bin/node-which"
+ "@sentry/types": "7.120.1"
},
"engines": {
- "node": ">= 8"
+ "node": ">=8"
}
},
- "node_modules/@storybook/components": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/components/-/components-7.6.15.tgz",
- "integrity": "sha512-xD+maP7+C9HeZXi2vJ+uK9hXN4S4spP4uDj9pyZ9yViKb+ztEO6WpovUMT8WRQ0mMegWyLXkx3zqu43hZvXM1g==",
- "dev": true,
+ "node_modules/@shopify/web-worker": {
+ "version": "6.4.0",
+ "resolved": "https://registry.npmjs.org/@shopify/web-worker/-/web-worker-6.4.0.tgz",
+ "integrity": "sha512-RvY1mgRyAqawFiYBvsBkek2pVK4GVpV9mmhWFCZXwx01usxXd2HMhKNTFeRYhSp29uoUcfBlKZAwCwQzt826tg==",
+ "license": "MIT",
"dependencies": {
- "@radix-ui/react-select": "^1.2.2",
- "@radix-ui/react-toolbar": "^1.0.4",
- "@storybook/client-logger": "7.6.15",
- "@storybook/csf": "^0.1.2",
- "@storybook/global": "^5.0.0",
- "@storybook/theming": "7.6.15",
- "@storybook/types": "7.6.15",
- "memoizerific": "^1.11.3",
- "use-resize-observer": "^9.1.0",
- "util-deprecate": "^1.0.2"
+ "@remote-ui/rpc": "^1.2.5"
},
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/storybook"
+ "engines": {
+ "node": ">=18.12.0"
},
"peerDependencies": {
- "react": "^16.8.0 || ^17.0.0 || ^18.0.0",
- "react-dom": "^16.8.0 || ^17.0.0 || ^18.0.0"
- }
- },
- "node_modules/@storybook/core-client": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/core-client/-/core-client-7.6.15.tgz",
- "integrity": "sha512-jwWol+zo+ItKBzPm9i80bEL6seHMsV0wKSaViVMQ4TqHtEbNeFE8sFEc2NTr18VNBnQOdlQPnEWmdboXBUrGcA==",
- "dev": true,
- "dependencies": {
- "@storybook/client-logger": "7.6.15",
- "@storybook/preview-api": "7.6.15"
+ "@babel/core": "^7.0.0",
+ "webpack": "^5.38.0",
+ "webpack-virtual-modules": "^0.4.3 || ^0.5.0 || ^0.6.0"
},
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/storybook"
+ "peerDependenciesMeta": {
+ "@babel/core": {
+ "optional": true
+ },
+ "webpack": {
+ "optional": true
+ },
+ "webpack-virtual-modules": {
+ "optional": true
+ }
}
},
- "node_modules/@storybook/core-common": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/core-common/-/core-common-7.6.15.tgz",
- "integrity": "sha512-VGmcLJ5U1r1s8/YnLbKcyB4GnNL+/sZIPqwlcSKzDXO76HoVFv1kywf7PbASote7P3gdhLSxBdg95LH2bdIbmw==",
- "dev": true,
+ "node_modules/@sideway/address": {
+ "version": "4.1.5",
+ "resolved": "https://registry.npmjs.org/@sideway/address/-/address-4.1.5.tgz",
+ "integrity": "sha512-IqO/DUQHUkPeixNQ8n0JA6102hT9CmaljNTPmQ1u8MEhBo/R4Q8eKLN/vGZxuebwOroDB4cbpjheD4+/sKFK4Q==",
"dependencies": {
- "@storybook/core-events": "7.6.15",
- "@storybook/node-logger": "7.6.15",
- "@storybook/types": "7.6.15",
- "@types/find-cache-dir": "^3.2.1",
- "@types/node": "^18.0.0",
- "@types/node-fetch": "^2.6.4",
- "@types/pretty-hrtime": "^1.0.0",
- "chalk": "^4.1.0",
- "esbuild": "^0.18.0",
- "esbuild-register": "^3.5.0",
- "file-system-cache": "2.3.0",
- "find-cache-dir": "^3.0.0",
- "find-up": "^5.0.0",
- "fs-extra": "^11.1.0",
- "glob": "^10.0.0",
- "handlebars": "^4.7.7",
- "lazy-universal-dotenv": "^4.0.0",
- "node-fetch": "^2.0.0",
- "picomatch": "^2.3.0",
- "pkg-dir": "^5.0.0",
- "pretty-hrtime": "^1.0.3",
- "resolve-from": "^5.0.0",
- "ts-dedent": "^2.0.0"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/storybook"
+ "@hapi/hoek": "^9.0.0"
}
},
- "node_modules/@storybook/core-common/node_modules/brace-expansion": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/brace-expansion/-/brace-expansion-2.0.1.tgz",
- "integrity": "sha512-XnAIvQ8eM+kC6aULx6wuQiwVsnzsi9d3WxzV3FpWTGA19F621kwdbsAcFKXgKUHZWsy+mY6iL1sHTxWEFCytDA==",
- "dev": true,
- "dependencies": {
- "balanced-match": "^1.0.0"
- }
+ "node_modules/@sideway/formula": {
+ "version": "3.0.1",
+ "resolved": "https://registry.npmjs.org/@sideway/formula/-/formula-3.0.1.tgz",
+ "integrity": "sha512-/poHZJJVjx3L+zVD6g9KgHfYnb443oi7wLu/XKojDviHy6HOEOA6z1Trk5aR1dGcmPenJEgb2sK2I80LeS3MIg=="
},
- "node_modules/@storybook/core-common/node_modules/find-cache-dir": {
- "version": "3.3.2",
- "resolved": "https://registry.npmjs.org/find-cache-dir/-/find-cache-dir-3.3.2.tgz",
- "integrity": "sha512-wXZV5emFEjrridIgED11OoUKLxiYjAcqot/NJdAkOhlJ+vGzwhOAfcG5OX1jP+S0PcjEn8bdMJv+g2jwQ3Onig==",
+ "node_modules/@sideway/pinpoint": {
+ "version": "2.0.0",
+ "resolved": "https://registry.npmjs.org/@sideway/pinpoint/-/pinpoint-2.0.0.tgz",
+ "integrity": "sha512-RNiOoTPkptFtSVzQevY/yWtZwf/RxyVnPy/OcA9HBM3MlGDnBEYL5B41H0MTn0Uec8Hi+2qUtTfG2WWZBmMejQ=="
+ },
+ "node_modules/@sidvind/better-ajv-errors": {
+ "version": "2.1.0",
+ "resolved": "https://registry.npmjs.org/@sidvind/better-ajv-errors/-/better-ajv-errors-2.1.0.tgz",
+ "integrity": "sha512-JuIb009FhHuL9priFBho2kv7QmZOydj0LgYvj+h1t0mMCmhM/YmQNRlJR5wVtBZya6wrVFK5Hi5TIbv5BKEx7w==",
"dev": true,
"dependencies": {
- "commondir": "^1.0.1",
- "make-dir": "^3.0.2",
- "pkg-dir": "^4.1.0"
+ "@babel/code-frame": "^7.16.0",
+ "chalk": "^4.1.0"
},
"engines": {
- "node": ">=8"
+ "node": ">= 14.0.0"
},
- "funding": {
- "url": "https://github.com/avajs/find-cache-dir?sponsor=1"
+ "peerDependencies": {
+ "ajv": "4.11.8 - 8"
}
},
- "node_modules/@storybook/core-common/node_modules/find-cache-dir/node_modules/find-up": {
- "version": "4.1.0",
- "resolved": "https://registry.npmjs.org/find-up/-/find-up-4.1.0.tgz",
- "integrity": "sha512-PpOwAdQ/YlXQ2vj8a3h8IipDuYRi3wceVQQGYWxNINccq40Anw7BlsEXCMbt1Zt+OLA6Fq9suIpIWD0OsnISlw==",
+ "node_modules/@sigstore/bundle": {
+ "version": "2.3.2",
+ "resolved": "https://registry.npmjs.org/@sigstore/bundle/-/bundle-2.3.2.tgz",
+ "integrity": "sha512-wueKWDk70QixNLB363yHc2D2ItTgYiMTdPwK8D9dKQMR3ZQ0c35IxP5xnwQ8cNLoCgCRcHf14kE+CLIvNX1zmA==",
"dev": true,
+ "license": "Apache-2.0",
"dependencies": {
- "locate-path": "^5.0.0",
- "path-exists": "^4.0.0"
+ "@sigstore/protobuf-specs": "^0.3.2"
},
"engines": {
- "node": ">=8"
+ "node": "^16.14.0 || >=18.0.0"
}
},
- "node_modules/@storybook/core-common/node_modules/find-cache-dir/node_modules/locate-path": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/locate-path/-/locate-path-5.0.0.tgz",
- "integrity": "sha512-t7hw9pI+WvuwNJXwk5zVHpyhIqzg2qTlklJOf0mVxGSbe3Fp2VieZcduNYjaLDoy6p9uGpQEGWG87WpMKlNq8g==",
+ "node_modules/@sigstore/core": {
+ "version": "1.1.0",
+ "resolved": "https://registry.npmjs.org/@sigstore/core/-/core-1.1.0.tgz",
+ "integrity": "sha512-JzBqdVIyqm2FRQCulY6nbQzMpJJpSiJ8XXWMhtOX9eKgaXXpfNOF53lzQEjIydlStnd/eFtuC1dW4VYdD93oRg==",
"dev": true,
- "dependencies": {
- "p-locate": "^4.1.0"
- },
+ "license": "Apache-2.0",
"engines": {
- "node": ">=8"
+ "node": "^16.14.0 || >=18.0.0"
}
},
- "node_modules/@storybook/core-common/node_modules/find-cache-dir/node_modules/p-limit": {
- "version": "2.3.0",
- "resolved": "https://registry.npmjs.org/p-limit/-/p-limit-2.3.0.tgz",
- "integrity": "sha512-//88mFWSJx8lxCzwdAABTJL2MyWB12+eIY7MDL2SqLmAkeKU9qxRvWuSyTjm3FUmpBEMuFfckAIqEaVGUDxb6w==",
+ "node_modules/@sigstore/protobuf-specs": {
+ "version": "0.3.2",
+ "resolved": "https://registry.npmjs.org/@sigstore/protobuf-specs/-/protobuf-specs-0.3.2.tgz",
+ "integrity": "sha512-c6B0ehIWxMI8wiS/bj6rHMPqeFvngFV7cDU/MY+B16P9Z3Mp9k8L93eYZ7BYzSickzuqAQqAq0V956b3Ju6mLw==",
"dev": true,
- "dependencies": {
- "p-try": "^2.0.0"
- },
+ "license": "Apache-2.0",
"engines": {
- "node": ">=6"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "node": "^16.14.0 || >=18.0.0"
}
},
- "node_modules/@storybook/core-common/node_modules/find-cache-dir/node_modules/p-locate": {
- "version": "4.1.0",
- "resolved": "https://registry.npmjs.org/p-locate/-/p-locate-4.1.0.tgz",
- "integrity": "sha512-R79ZZ/0wAxKGu3oYMlz8jy/kbhsNrS7SKZ7PxEHBgJ5+F2mtFW2fK2cOtBh1cHYkQsbzFV7I+EoRKe6Yt0oK7A==",
+ "node_modules/@sigstore/sign": {
+ "version": "2.3.2",
+ "resolved": "https://registry.npmjs.org/@sigstore/sign/-/sign-2.3.2.tgz",
+ "integrity": "sha512-5Vz5dPVuunIIvC5vBb0APwo7qKA4G9yM48kPWJT+OEERs40md5GoUR1yedwpekWZ4m0Hhw44m6zU+ObsON+iDA==",
"dev": true,
+ "license": "Apache-2.0",
"dependencies": {
- "p-limit": "^2.2.0"
+ "@sigstore/bundle": "^2.3.2",
+ "@sigstore/core": "^1.0.0",
+ "@sigstore/protobuf-specs": "^0.3.2",
+ "make-fetch-happen": "^13.0.1",
+ "proc-log": "^4.2.0",
+ "promise-retry": "^2.0.1"
},
"engines": {
- "node": ">=8"
+ "node": "^16.14.0 || >=18.0.0"
}
},
- "node_modules/@storybook/core-common/node_modules/find-cache-dir/node_modules/pkg-dir": {
- "version": "4.2.0",
- "resolved": "https://registry.npmjs.org/pkg-dir/-/pkg-dir-4.2.0.tgz",
- "integrity": "sha512-HRDzbaKjC+AOWVXxAU/x54COGeIv9eb+6CkDSQoNTt4XyWoIJvuPsXizxu/Fr23EiekbtZwmh1IcIG/l/a10GQ==",
+ "node_modules/@sigstore/tuf": {
+ "version": "2.3.4",
+ "resolved": "https://registry.npmjs.org/@sigstore/tuf/-/tuf-2.3.4.tgz",
+ "integrity": "sha512-44vtsveTPUpqhm9NCrbU8CWLe3Vck2HO1PNLw7RIajbB7xhtn5RBPm1VNSCMwqGYHhDsBJG8gDF0q4lgydsJvw==",
"dev": true,
+ "license": "Apache-2.0",
"dependencies": {
- "find-up": "^4.0.0"
+ "@sigstore/protobuf-specs": "^0.3.2",
+ "tuf-js": "^2.2.1"
},
"engines": {
- "node": ">=8"
+ "node": "^16.14.0 || >=18.0.0"
}
},
- "node_modules/@storybook/core-common/node_modules/find-up": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/find-up/-/find-up-5.0.0.tgz",
- "integrity": "sha512-78/PXT1wlLLDgTzDs7sjq9hzz0vXD+zn+7wypEe4fXQxCmdmqfGsEPQxmiCSQI3ajFV91bVSsvNtrJRiW6nGng==",
+ "node_modules/@sigstore/verify": {
+ "version": "1.2.1",
+ "resolved": "https://registry.npmjs.org/@sigstore/verify/-/verify-1.2.1.tgz",
+ "integrity": "sha512-8iKx79/F73DKbGfRf7+t4dqrc0bRr0thdPrxAtCKWRm/F0tG71i6O1rvlnScncJLLBZHn3h8M3c1BSUAb9yu8g==",
"dev": true,
+ "license": "Apache-2.0",
"dependencies": {
- "locate-path": "^6.0.0",
- "path-exists": "^4.0.0"
+ "@sigstore/bundle": "^2.3.2",
+ "@sigstore/core": "^1.1.0",
+ "@sigstore/protobuf-specs": "^0.3.2"
},
+ "engines": {
+ "node": "^16.14.0 || >=18.0.0"
+ }
+ },
+ "node_modules/@sinclair/typebox": {
+ "version": "0.27.8",
+ "resolved": "https://registry.npmjs.org/@sinclair/typebox/-/typebox-0.27.8.tgz",
+ "integrity": "sha512-+Fj43pSMwJs4KRrH/938Uf+uAELIgVBmQzg/q1YG10djyfA3TnrU8N8XzqCh/okZdszqBQTZf96idMfE5lnwTA=="
+ },
+ "node_modules/@sindresorhus/is": {
+ "version": "4.6.0",
+ "resolved": "https://registry.npmjs.org/@sindresorhus/is/-/is-4.6.0.tgz",
+ "integrity": "sha512-t09vSN3MdfsyCHoFcTRCH/iUtG7OJ0CsjzB8cjAmKc/va/kIgeDI/TxsigdncE/4be734m0cvIYwNaV4i2XqAw==",
"engines": {
"node": ">=10"
},
"funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "url": "https://github.com/sindresorhus/is?sponsor=1"
}
},
- "node_modules/@storybook/core-common/node_modules/fs-extra": {
- "version": "11.2.0",
- "resolved": "https://registry.npmjs.org/fs-extra/-/fs-extra-11.2.0.tgz",
- "integrity": "sha512-PmDi3uwK5nFuXh7XDTlVnS17xJS7vW36is2+w3xcv8SVxiB4NyATf4ctkVY5bkSjX0Y4nbvZCq1/EjtEyr9ktw==",
- "dev": true,
+ "node_modules/@sinonjs/commons": {
+ "version": "3.0.0",
+ "resolved": "https://registry.npmjs.org/@sinonjs/commons/-/commons-3.0.0.tgz",
+ "integrity": "sha512-jXBtWAF4vmdNmZgD5FoKsVLv3rPgDnLgPbU84LIJ3otV44vJlDRokVng5v8NFJdCf/da9legHcKaRuZs4L7faA==",
"dependencies": {
- "graceful-fs": "^4.2.0",
- "jsonfile": "^6.0.1",
- "universalify": "^2.0.0"
- },
- "engines": {
- "node": ">=14.14"
+ "type-detect": "4.0.8"
}
},
- "node_modules/@storybook/core-common/node_modules/glob": {
- "version": "10.4.5",
- "resolved": "https://registry.npmjs.org/glob/-/glob-10.4.5.tgz",
- "integrity": "sha512-7Bv8RF0k6xjo7d4A/PxYLbUCfb6c+Vpd2/mB2yRDlew7Jb5hEXiCD9ibfO7wpk8i4sevK6DFny9h7EYbM3/sHg==",
+ "node_modules/@sinonjs/fake-timers": {
+ "version": "10.3.0",
+ "resolved": "https://registry.npmjs.org/@sinonjs/fake-timers/-/fake-timers-10.3.0.tgz",
+ "integrity": "sha512-V4BG07kuYSUkTCSBHG8G8TNhM+F19jXFWnQtzj+we8DrkpSBCee9Z3Ms8yiGer/dlmhe35/Xdgyo3/0rQKg7YA==",
+ "dependencies": {
+ "@sinonjs/commons": "^3.0.0"
+ }
+ },
+ "node_modules/@sliphua/lilconfig-ts-loader": {
+ "version": "3.2.2",
+ "resolved": "https://registry.npmjs.org/@sliphua/lilconfig-ts-loader/-/lilconfig-ts-loader-3.2.2.tgz",
+ "integrity": "sha512-nX2aBwAykiG50fSUzK9eyA5UvWcrEKzA0ZzCq9mLwHMwpKxM+U05YH8PHba1LJrbeZ7R1HSjJagWKMqFyq8cxw==",
"dev": true,
- "license": "ISC",
"dependencies": {
- "foreground-child": "^3.1.0",
- "jackspeak": "^3.1.2",
- "minimatch": "^9.0.4",
- "minipass": "^7.1.2",
- "package-json-from-dist": "^1.0.0",
- "path-scurry": "^1.11.1"
+ "lodash.get": "^4",
+ "make-error": "^1",
+ "ts-node": "^9",
+ "tslib": "^2"
},
- "bin": {
- "glob": "dist/esm/bin.mjs"
+ "engines": {
+ "node": ">=10.0.0"
},
- "funding": {
- "url": "https://github.com/sponsors/isaacs"
+ "peerDependencies": {
+ "lilconfig": ">=2"
}
},
- "node_modules/@storybook/core-common/node_modules/jackspeak": {
- "version": "3.4.3",
- "resolved": "https://registry.npmjs.org/jackspeak/-/jackspeak-3.4.3.tgz",
- "integrity": "sha512-OGlZQpz2yfahA/Rd1Y8Cd9SIEsqvXkLVoSw/cgwhnhFMDbsQFeZYoJJ7bIZBS9BcamUW96asq/npPWugM+RQBw==",
+ "node_modules/@storybook/addon-a11y": {
+ "version": "8.4.7",
+ "resolved": "https://registry.npmjs.org/@storybook/addon-a11y/-/addon-a11y-8.4.7.tgz",
+ "integrity": "sha512-GpUvXp6n25U1ZSv+hmDC+05BEqxWdlWjQTb/GaboRXZQeMBlze6zckpVb66spjmmtQAIISo0eZxX1+mGcVR7lA==",
"dev": true,
- "license": "BlueOak-1.0.0",
"dependencies": {
- "@isaacs/cliui": "^8.0.2"
+ "@storybook/addon-highlight": "8.4.7",
+ "axe-core": "^4.2.0"
},
"funding": {
- "url": "https://github.com/sponsors/isaacs"
+ "type": "opencollective",
+ "url": "https://opencollective.com/storybook"
},
- "optionalDependencies": {
- "@pkgjs/parseargs": "^0.11.0"
+ "peerDependencies": {
+ "storybook": "^8.4.7"
}
},
- "node_modules/@storybook/core-common/node_modules/jsonfile": {
- "version": "6.1.0",
- "resolved": "https://registry.npmjs.org/jsonfile/-/jsonfile-6.1.0.tgz",
- "integrity": "sha512-5dgndWOriYSm5cnYaJNhalLNDKOqFwyDB/rr1E9ZsGciGvKPs8R2xYGCacuf3z6K1YKDz182fd+fY3cn3pMqXQ==",
+ "node_modules/@storybook/addon-actions": {
+ "version": "8.4.7",
+ "resolved": "https://registry.npmjs.org/@storybook/addon-actions/-/addon-actions-8.4.7.tgz",
+ "integrity": "sha512-mjtD5JxcPuW74T6h7nqMxWTvDneFtokg88p6kQ5OnC1M259iAXb//yiSZgu/quunMHPCXSiqn4FNOSgASTSbsA==",
"dev": true,
"dependencies": {
- "universalify": "^2.0.0"
+ "@storybook/global": "^5.0.0",
+ "@types/uuid": "^9.0.1",
+ "dequal": "^2.0.2",
+ "polished": "^4.2.2",
+ "uuid": "^9.0.0"
},
- "optionalDependencies": {
- "graceful-fs": "^4.1.6"
+ "funding": {
+ "type": "opencollective",
+ "url": "https://opencollective.com/storybook"
+ },
+ "peerDependencies": {
+ "storybook": "^8.4.7"
}
},
- "node_modules/@storybook/core-common/node_modules/locate-path": {
- "version": "6.0.0",
- "resolved": "https://registry.npmjs.org/locate-path/-/locate-path-6.0.0.tgz",
- "integrity": "sha512-iPZK6eYjbxRu3uB4/WZ3EsEIMJFMqAoopl3R+zuq0UjcAm/MO6KCweDgPfP3elTztoKP3KtnVHxTn2NHBSDVUw==",
+ "node_modules/@storybook/addon-actions/node_modules/@types/uuid": {
+ "version": "9.0.8",
+ "resolved": "https://registry.npmjs.org/@types/uuid/-/uuid-9.0.8.tgz",
+ "integrity": "sha512-jg+97EGIcY9AGHJJRaaPVgetKDsrTgbRjQ5Msgjh/DQKEFl0DtyRr/VCOyD1T2R1MNeWPK/u7JoGhlDZnKBAfA==",
+ "dev": true
+ },
+ "node_modules/@storybook/addon-controls": {
+ "version": "8.4.7",
+ "resolved": "https://registry.npmjs.org/@storybook/addon-controls/-/addon-controls-8.4.7.tgz",
+ "integrity": "sha512-377uo5IsJgXLnQLJixa47+11V+7Wn9KcDEw+96aGCBCfLbWNH8S08tJHHnSu+jXg9zoqCAC23MetntVp6LetHA==",
"dev": true,
"dependencies": {
- "p-locate": "^5.0.0"
- },
- "engines": {
- "node": ">=10"
+ "@storybook/global": "^5.0.0",
+ "dequal": "^2.0.2",
+ "ts-dedent": "^2.0.0"
},
"funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "type": "opencollective",
+ "url": "https://opencollective.com/storybook"
+ },
+ "peerDependencies": {
+ "storybook": "^8.4.7"
}
},
- "node_modules/@storybook/core-common/node_modules/make-dir": {
- "version": "3.1.0",
- "resolved": "https://registry.npmjs.org/make-dir/-/make-dir-3.1.0.tgz",
- "integrity": "sha512-g3FeP20LNwhALb/6Cz6Dd4F2ngze0jz7tbzrD2wAV+o9FeNHe4rL+yK2md0J/fiSf1sa1ADhXqi5+oVwOM/eGw==",
+ "node_modules/@storybook/addon-docs": {
+ "version": "8.4.7",
+ "resolved": "https://registry.npmjs.org/@storybook/addon-docs/-/addon-docs-8.4.7.tgz",
+ "integrity": "sha512-NwWaiTDT5puCBSUOVuf6ME7Zsbwz7Y79WF5tMZBx/sLQ60vpmJVQsap6NSjvK1Ravhc21EsIXqemAcBjAWu80w==",
"dev": true,
"dependencies": {
- "semver": "^6.0.0"
- },
- "engines": {
- "node": ">=8"
+ "@mdx-js/react": "^3.0.0",
+ "@storybook/blocks": "8.4.7",
+ "@storybook/csf-plugin": "8.4.7",
+ "@storybook/react-dom-shim": "8.4.7",
+ "react": "^16.8.0 || ^17.0.0 || ^18.0.0",
+ "react-dom": "^16.8.0 || ^17.0.0 || ^18.0.0",
+ "ts-dedent": "^2.0.0"
},
"funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "type": "opencollective",
+ "url": "https://opencollective.com/storybook"
+ },
+ "peerDependencies": {
+ "storybook": "^8.4.7"
}
},
- "node_modules/@storybook/core-common/node_modules/minimatch": {
- "version": "9.0.5",
- "resolved": "https://registry.npmjs.org/minimatch/-/minimatch-9.0.5.tgz",
- "integrity": "sha512-G6T0ZX48xgozx7587koeX9Ys2NYy6Gmv//P89sEte9V9whIapMNF4idKxnW2QtCcLiTWlb/wfCabAtAFWhhBow==",
+ "node_modules/@storybook/addon-highlight": {
+ "version": "8.4.7",
+ "resolved": "https://registry.npmjs.org/@storybook/addon-highlight/-/addon-highlight-8.4.7.tgz",
+ "integrity": "sha512-whQIDBd3PfVwcUCrRXvCUHWClXe9mQ7XkTPCdPo4B/tZ6Z9c6zD8JUHT76ddyHivixFLowMnA8PxMU6kCMAiNw==",
"dev": true,
- "license": "ISC",
"dependencies": {
- "brace-expansion": "^2.0.1"
- },
- "engines": {
- "node": ">=16 || 14 >=14.17"
+ "@storybook/global": "^5.0.0"
},
"funding": {
- "url": "https://github.com/sponsors/isaacs"
+ "type": "opencollective",
+ "url": "https://opencollective.com/storybook"
+ },
+ "peerDependencies": {
+ "storybook": "^8.4.7"
}
},
- "node_modules/@storybook/core-common/node_modules/minipass": {
- "version": "7.1.2",
- "resolved": "https://registry.npmjs.org/minipass/-/minipass-7.1.2.tgz",
- "integrity": "sha512-qOOzS1cBTWYF4BH8fVePDBOO9iptMnGUEZwNc/cMWnTV2nVLZ7VoNWEPHkYczZA0pdoA7dl6e7FL659nX9S2aw==",
+ "node_modules/@storybook/addon-toolbars": {
+ "version": "8.4.7",
+ "resolved": "https://registry.npmjs.org/@storybook/addon-toolbars/-/addon-toolbars-8.4.7.tgz",
+ "integrity": "sha512-OSfdv5UZs+NdGB+nZmbafGUWimiweJ/56gShlw8Neo/4jOJl1R3rnRqqY7MYx8E4GwoX+i3GF5C3iWFNQqlDcw==",
"dev": true,
- "license": "ISC",
- "engines": {
- "node": ">=16 || 14 >=14.17"
+ "funding": {
+ "type": "opencollective",
+ "url": "https://opencollective.com/storybook"
+ },
+ "peerDependencies": {
+ "storybook": "^8.4.7"
}
},
- "node_modules/@storybook/core-common/node_modules/p-locate": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/p-locate/-/p-locate-5.0.0.tgz",
- "integrity": "sha512-LaNjtRWUBY++zB5nE/NwcaoMylSPk+S+ZHNB1TzdbMJMny6dynpAGt7X/tl/QYq3TIeE6nxHppbo2LGymrG5Pw==",
+ "node_modules/@storybook/addon-viewport": {
+ "version": "8.4.7",
+ "resolved": "https://registry.npmjs.org/@storybook/addon-viewport/-/addon-viewport-8.4.7.tgz",
+ "integrity": "sha512-hvczh/jjuXXcOogih09a663sRDDSATXwbE866al1DXgbDFraYD/LxX/QDb38W9hdjU9+Qhx8VFIcNWoMQns5HQ==",
"dev": true,
"dependencies": {
- "p-limit": "^3.0.2"
- },
- "engines": {
- "node": ">=10"
+ "memoizerific": "^1.11.3"
},
"funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "type": "opencollective",
+ "url": "https://opencollective.com/storybook"
+ },
+ "peerDependencies": {
+ "storybook": "^8.4.7"
}
},
- "node_modules/@storybook/core-common/node_modules/p-try": {
- "version": "2.2.0",
- "resolved": "https://registry.npmjs.org/p-try/-/p-try-2.2.0.tgz",
- "integrity": "sha512-R4nPAVTAU0B9D35/Gk3uJf/7XYbQcyohSKdvAxIRSNghFl4e71hVoGnBNQz9cWaXxO2I10KTC+3jMdvvoKw6dQ==",
+ "node_modules/@storybook/addon-webpack5-compiler-babel": {
+ "version": "3.0.3",
+ "resolved": "https://registry.npmjs.org/@storybook/addon-webpack5-compiler-babel/-/addon-webpack5-compiler-babel-3.0.3.tgz",
+ "integrity": "sha512-rVQTTw+oxJltbVKaejIWSHwVKOBJs3au21f/pYXhV0aiNgNhxEa3vr79t/j0j8ox8uJtzM8XYOb7FlkvGfHlwQ==",
"dev": true,
+ "dependencies": {
+ "@babel/core": "^7.23.7",
+ "babel-loader": "^9.1.3"
+ },
"engines": {
- "node": ">=6"
+ "node": ">=18"
}
},
- "node_modules/@storybook/core-common/node_modules/path-exists": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/path-exists/-/path-exists-4.0.0.tgz",
- "integrity": "sha512-ak9Qy5Q7jYb2Wwcey5Fpvg2KoAc/ZIhLSLOSBmRmygPsGwkVVt0fZa0qrtMz+m6tJTAHfZQ8FnmB4MG4LWy7/w==",
+ "node_modules/@storybook/blocks": {
+ "version": "8.4.7",
+ "resolved": "https://registry.npmjs.org/@storybook/blocks/-/blocks-8.4.7.tgz",
+ "integrity": "sha512-+QH7+JwXXXIyP3fRCxz/7E2VZepAanXJM7G8nbR3wWsqWgrRp4Wra6MvybxAYCxU7aNfJX5c+RW84SNikFpcIA==",
"dev": true,
- "engines": {
- "node": ">=8"
+ "dependencies": {
+ "@storybook/csf": "^0.1.11",
+ "@storybook/icons": "^1.2.12",
+ "ts-dedent": "^2.0.0"
+ },
+ "funding": {
+ "type": "opencollective",
+ "url": "https://opencollective.com/storybook"
+ },
+ "peerDependencies": {
+ "react": "^16.8.0 || ^17.0.0 || ^18.0.0 || ^19.0.0-beta",
+ "react-dom": "^16.8.0 || ^17.0.0 || ^18.0.0 || ^19.0.0-beta",
+ "storybook": "^8.4.7"
+ },
+ "peerDependenciesMeta": {
+ "react": {
+ "optional": true
+ },
+ "react-dom": {
+ "optional": true
+ }
}
},
- "node_modules/@storybook/core-common/node_modules/pkg-dir": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/pkg-dir/-/pkg-dir-5.0.0.tgz",
- "integrity": "sha512-NPE8TDbzl/3YQYY7CSS228s3g2ollTFnc+Qi3tqmqJp9Vg2ovUpixcJEo2HJScN2Ez+kEaal6y70c0ehqJBJeA==",
+ "node_modules/@storybook/builder-webpack5": {
+ "version": "8.4.7",
+ "resolved": "https://registry.npmjs.org/@storybook/builder-webpack5/-/builder-webpack5-8.4.7.tgz",
+ "integrity": "sha512-O8LpsQ+4g2x5kh7rI9+jEUdX8k1a5egBQU1lbudmHchqsV0IKiVqBD9LL5Gj3wpit4vB8coSW4ZWTFBw8FQb4Q==",
"dev": true,
"dependencies": {
- "find-up": "^5.0.0"
+ "@storybook/core-webpack": "8.4.7",
+ "@types/node": "^22.0.0",
+ "@types/semver": "^7.3.4",
+ "browser-assert": "^1.2.1",
+ "case-sensitive-paths-webpack-plugin": "^2.4.0",
+ "cjs-module-lexer": "^1.2.3",
+ "constants-browserify": "^1.0.0",
+ "css-loader": "^6.7.1",
+ "es-module-lexer": "^1.5.0",
+ "fork-ts-checker-webpack-plugin": "^8.0.0",
+ "html-webpack-plugin": "^5.5.0",
+ "magic-string": "^0.30.5",
+ "path-browserify": "^1.0.1",
+ "process": "^0.11.10",
+ "semver": "^7.3.7",
+ "style-loader": "^3.3.1",
+ "terser-webpack-plugin": "^5.3.1",
+ "ts-dedent": "^2.0.0",
+ "url": "^0.11.0",
+ "util": "^0.12.4",
+ "util-deprecate": "^1.0.2",
+ "webpack": "5",
+ "webpack-dev-middleware": "^6.1.2",
+ "webpack-hot-middleware": "^2.25.1",
+ "webpack-virtual-modules": "^0.6.0"
},
- "engines": {
- "node": ">=10"
+ "funding": {
+ "type": "opencollective",
+ "url": "https://opencollective.com/storybook"
+ },
+ "peerDependencies": {
+ "storybook": "^8.4.7"
+ },
+ "peerDependenciesMeta": {
+ "typescript": {
+ "optional": true
+ }
}
},
- "node_modules/@storybook/core-common/node_modules/resolve-from": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/resolve-from/-/resolve-from-5.0.0.tgz",
- "integrity": "sha512-qYg9KP24dD5qka9J47d0aVky0N+b4fTU89LN9iDnjB5waksiC49rvMB0PrUJQGoTmH50XPiqOvAjDfaijGxYZw==",
+ "node_modules/@storybook/builder-webpack5/node_modules/css-loader": {
+ "version": "6.11.0",
+ "resolved": "https://registry.npmjs.org/css-loader/-/css-loader-6.11.0.tgz",
+ "integrity": "sha512-CTJ+AEQJjq5NzLga5pE39qdiSV56F8ywCIsqNIRF0r7BDgWsN25aazToqAFg7ZrtA/U016xudB3ffgweORxX7g==",
"dev": true,
+ "dependencies": {
+ "icss-utils": "^5.1.0",
+ "postcss": "^8.4.33",
+ "postcss-modules-extract-imports": "^3.1.0",
+ "postcss-modules-local-by-default": "^4.0.5",
+ "postcss-modules-scope": "^3.2.0",
+ "postcss-modules-values": "^4.0.0",
+ "postcss-value-parser": "^4.2.0",
+ "semver": "^7.5.4"
+ },
"engines": {
- "node": ">=8"
+ "node": ">= 12.13.0"
+ },
+ "funding": {
+ "type": "opencollective",
+ "url": "https://opencollective.com/webpack"
+ },
+ "peerDependencies": {
+ "@rspack/core": "0.x || 1.x",
+ "webpack": "^5.0.0"
+ },
+ "peerDependenciesMeta": {
+ "@rspack/core": {
+ "optional": true
+ },
+ "webpack": {
+ "optional": true
+ }
}
},
- "node_modules/@storybook/core-common/node_modules/semver": {
- "version": "6.3.1",
- "resolved": "https://registry.npmjs.org/semver/-/semver-6.3.1.tgz",
- "integrity": "sha512-BR7VvDCVHO+q2xBEWskxS6DJE1qRnb7DxzUrogb71CWoSficBxYsiAGd+Kl0mmq/MprG9yArRkyrQxTO6XjMzA==",
- "dev": true,
- "bin": {
- "semver": "bin/semver.js"
- }
+ "node_modules/@storybook/builder-webpack5/node_modules/path-browserify": {
+ "version": "1.0.1",
+ "resolved": "https://registry.npmjs.org/path-browserify/-/path-browserify-1.0.1.tgz",
+ "integrity": "sha512-b7uo2UCUOYZcnF/3ID0lulOJi/bafxa1xPe7ZPsammBSpjSWQkjNxlt635YGS2MiR9GjvuXCtz2emr3jbsz98g==",
+ "dev": true
},
- "node_modules/@storybook/core-common/node_modules/universalify": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/universalify/-/universalify-2.0.1.tgz",
- "integrity": "sha512-gptHNQghINnc/vTGIk0SOFGFNXw7JVrlRUtConJRlvaw6DuX0wO5Jeko9sWrMBhh+PsYAZ7oXAiOnf/UKogyiw==",
+ "node_modules/@storybook/builder-webpack5/node_modules/postcss-value-parser": {
+ "version": "4.2.0",
+ "resolved": "https://registry.npmjs.org/postcss-value-parser/-/postcss-value-parser-4.2.0.tgz",
+ "integrity": "sha512-1NNCs6uurfkVbeXG4S8JFT9t19m45ICnif8zWLd5oPSZ50QnwMfK+H3jv408d4jw/7Bttv5axS5IiHoLaVNHeQ==",
+ "dev": true
+ },
+ "node_modules/@storybook/builder-webpack5/node_modules/style-loader": {
+ "version": "3.3.4",
+ "resolved": "https://registry.npmjs.org/style-loader/-/style-loader-3.3.4.tgz",
+ "integrity": "sha512-0WqXzrsMTyb8yjZJHDqwmnwRJvhALK9LfRtRc6B4UTWe8AijYLZYZ9thuJTZc2VfQWINADW/j+LiJnfy2RoC1w==",
"dev": true,
"engines": {
- "node": ">= 10.0.0"
+ "node": ">= 12.13.0"
+ },
+ "funding": {
+ "type": "opencollective",
+ "url": "https://opencollective.com/webpack"
+ },
+ "peerDependencies": {
+ "webpack": "^5.0.0"
}
},
- "node_modules/@storybook/core-events": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/core-events/-/core-events-7.6.15.tgz",
- "integrity": "sha512-i4YnjGecbpGyrFe0340sPhQ9QjZZEBqvMy6kF4XWt6DYLHxZmsTj1HEdvxVl4Ej7V49Vw0Dm8MepJ1d4Y8MKrQ==",
+ "node_modules/@storybook/builder-webpack5/node_modules/util": {
+ "version": "0.12.5",
+ "resolved": "https://registry.npmjs.org/util/-/util-0.12.5.tgz",
+ "integrity": "sha512-kZf/K6hEIrWHI6XqOFUiiMa+79wE/D8Q+NCNAWclkyg3b4d2k7s0QGepNjiABc+aR3N1PAyHL7p6UcLY6LmrnA==",
"dev": true,
"dependencies": {
- "ts-dedent": "^2.0.0"
- },
+ "inherits": "^2.0.3",
+ "is-arguments": "^1.0.4",
+ "is-generator-function": "^1.0.7",
+ "is-typed-array": "^1.1.3",
+ "which-typed-array": "^1.1.2"
+ }
+ },
+ "node_modules/@storybook/components": {
+ "version": "8.4.7",
+ "resolved": "https://registry.npmjs.org/@storybook/components/-/components-8.4.7.tgz",
+ "integrity": "sha512-uyJIcoyeMWKAvjrG9tJBUCKxr2WZk+PomgrgrUwejkIfXMO76i6jw9BwLa0NZjYdlthDv30r9FfbYZyeNPmF0g==",
+ "dev": true,
"funding": {
"type": "opencollective",
"url": "https://opencollective.com/storybook"
+ },
+ "peerDependencies": {
+ "storybook": "^8.2.0 || ^8.3.0-0 || ^8.4.0-0 || ^8.5.0-0 || ^8.6.0-0"
}
},
- "node_modules/@storybook/core-server": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/core-server/-/core-server-7.6.15.tgz",
- "integrity": "sha512-iIlxEAkrmKTSA3iGNqt/4QG7hf5suxBGYIB3DZAOfBo8EdZogMYaEmuCm5dbuaJr0mcVwlqwdhQiWb1VsR/NhA==",
+ "node_modules/@storybook/core": {
+ "version": "8.4.7",
+ "resolved": "https://registry.npmjs.org/@storybook/core/-/core-8.4.7.tgz",
+ "integrity": "sha512-7Z8Z0A+1YnhrrSXoKKwFFI4gnsLbWzr8fnDCU6+6HlDukFYh8GHRcZ9zKfqmy6U3hw2h8H5DrHsxWfyaYUUOoA==",
"dev": true,
"dependencies": {
- "@aw-web-design/x-default-browser": "1.4.126",
- "@discoveryjs/json-ext": "^0.5.3",
- "@storybook/builder-manager": "7.6.15",
- "@storybook/channels": "7.6.15",
- "@storybook/core-common": "7.6.15",
- "@storybook/core-events": "7.6.15",
- "@storybook/csf": "^0.1.2",
- "@storybook/csf-tools": "7.6.15",
- "@storybook/docs-mdx": "^0.1.0",
- "@storybook/global": "^5.0.0",
- "@storybook/manager": "7.6.15",
- "@storybook/node-logger": "7.6.15",
- "@storybook/preview-api": "7.6.15",
- "@storybook/telemetry": "7.6.15",
- "@storybook/types": "7.6.15",
- "@types/detect-port": "^1.3.0",
- "@types/node": "^18.0.0",
- "@types/pretty-hrtime": "^1.0.0",
- "@types/semver": "^7.3.4",
+ "@storybook/csf": "^0.1.11",
"better-opn": "^3.0.2",
- "chalk": "^4.1.0",
- "cli-table3": "^0.6.1",
- "compression": "^1.7.4",
- "detect-port": "^1.3.0",
- "express": "^4.17.3",
- "fs-extra": "^11.1.0",
- "globby": "^11.0.2",
- "ip": "^2.0.0",
- "lodash": "^4.17.21",
- "open": "^8.4.0",
- "pretty-hrtime": "^1.0.3",
- "prompts": "^2.4.0",
- "read-pkg-up": "^7.0.1",
- "semver": "^7.3.7",
- "telejson": "^7.2.0",
- "tiny-invariant": "^1.3.1",
- "ts-dedent": "^2.0.0",
- "util": "^0.12.4",
- "util-deprecate": "^1.0.2",
- "watchpack": "^2.2.0",
+ "browser-assert": "^1.2.1",
+ "esbuild": "^0.18.0 || ^0.19.0 || ^0.20.0 || ^0.21.0 || ^0.22.0 || ^0.23.0 || ^0.24.0",
+ "esbuild-register": "^3.5.0",
+ "jsdoc-type-pratt-parser": "^4.0.0",
+ "process": "^0.11.10",
+ "recast": "^0.23.5",
+ "semver": "^7.6.2",
+ "util": "^0.12.5",
"ws": "^8.2.3"
},
"funding": {
"type": "opencollective",
"url": "https://opencollective.com/storybook"
- }
- },
- "node_modules/@storybook/core-server/node_modules/fs-extra": {
- "version": "11.2.0",
- "resolved": "https://registry.npmjs.org/fs-extra/-/fs-extra-11.2.0.tgz",
- "integrity": "sha512-PmDi3uwK5nFuXh7XDTlVnS17xJS7vW36is2+w3xcv8SVxiB4NyATf4ctkVY5bkSjX0Y4nbvZCq1/EjtEyr9ktw==",
- "dev": true,
- "dependencies": {
- "graceful-fs": "^4.2.0",
- "jsonfile": "^6.0.1",
- "universalify": "^2.0.0"
},
- "engines": {
- "node": ">=14.14"
- }
- },
- "node_modules/@storybook/core-server/node_modules/ip": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/ip/-/ip-2.0.1.tgz",
- "integrity": "sha512-lJUL9imLTNi1ZfXT+DU6rBBdbiKGBuay9B6xGSPVjUeQwaH1RIGqef8RZkUtHioLmSNpPR5M4HVKJGm1j8FWVQ==",
- "dev": true
- },
- "node_modules/@storybook/core-server/node_modules/is-wsl": {
- "version": "2.2.0",
- "resolved": "https://registry.npmjs.org/is-wsl/-/is-wsl-2.2.0.tgz",
- "integrity": "sha512-fKzAra0rGJUUBwGBgNkHZuToZcn+TtXHpeCgmkMJMMYx1sQDYaCSyjJBSCa2nH1DGm7s3n1oBnohoVTBaN7Lww==",
- "dev": true,
- "dependencies": {
- "is-docker": "^2.0.0"
+ "peerDependencies": {
+ "prettier": "^2 || ^3"
},
- "engines": {
- "node": ">=8"
+ "peerDependenciesMeta": {
+ "prettier": {
+ "optional": true
+ }
}
},
- "node_modules/@storybook/core-server/node_modules/jsonfile": {
- "version": "6.1.0",
- "resolved": "https://registry.npmjs.org/jsonfile/-/jsonfile-6.1.0.tgz",
- "integrity": "sha512-5dgndWOriYSm5cnYaJNhalLNDKOqFwyDB/rr1E9ZsGciGvKPs8R2xYGCacuf3z6K1YKDz182fd+fY3cn3pMqXQ==",
+ "node_modules/@storybook/core-webpack": {
+ "version": "8.4.7",
+ "resolved": "https://registry.npmjs.org/@storybook/core-webpack/-/core-webpack-8.4.7.tgz",
+ "integrity": "sha512-Tj+CjQLpFyBJxhhMms+vbPT3+gTRAiQlrhY3L1IEVwBa3wtRMS0qjozH26d1hK4G6mUIEdwu13L54HMU/w33Sg==",
"dev": true,
"dependencies": {
- "universalify": "^2.0.0"
+ "@types/node": "^22.0.0",
+ "ts-dedent": "^2.0.0"
},
- "optionalDependencies": {
- "graceful-fs": "^4.1.6"
+ "funding": {
+ "type": "opencollective",
+ "url": "https://opencollective.com/storybook"
+ },
+ "peerDependencies": {
+ "storybook": "^8.4.7"
}
},
- "node_modules/@storybook/core-server/node_modules/open": {
- "version": "8.4.2",
- "resolved": "https://registry.npmjs.org/open/-/open-8.4.2.tgz",
- "integrity": "sha512-7x81NCL719oNbsq/3mh+hVrAWmFuEYUqrq/Iw3kUzH8ReypT9QQ0BLoJS7/G9k6N81XjW4qHWtjWwe/9eLy1EQ==",
+ "node_modules/@storybook/core/node_modules/recast": {
+ "version": "0.23.9",
+ "resolved": "https://registry.npmjs.org/recast/-/recast-0.23.9.tgz",
+ "integrity": "sha512-Hx/BGIbwj+Des3+xy5uAtAbdCyqK9y9wbBcDFDYanLS9JnMqf7OeF87HQwUimE87OEc72mr6tkKUKMBBL+hF9Q==",
"dev": true,
"dependencies": {
- "define-lazy-prop": "^2.0.0",
- "is-docker": "^2.1.1",
- "is-wsl": "^2.2.0"
+ "ast-types": "^0.16.1",
+ "esprima": "~4.0.0",
+ "source-map": "~0.6.1",
+ "tiny-invariant": "^1.3.3",
+ "tslib": "^2.0.1"
},
"engines": {
- "node": ">=12"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
+ "node": ">= 4"
}
},
- "node_modules/@storybook/core-server/node_modules/universalify": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/universalify/-/universalify-2.0.1.tgz",
- "integrity": "sha512-gptHNQghINnc/vTGIk0SOFGFNXw7JVrlRUtConJRlvaw6DuX0wO5Jeko9sWrMBhh+PsYAZ7oXAiOnf/UKogyiw==",
+ "node_modules/@storybook/core/node_modules/semver": {
+ "version": "7.6.3",
+ "resolved": "https://registry.npmjs.org/semver/-/semver-7.6.3.tgz",
+ "integrity": "sha512-oVekP1cKtI+CTDvHWYFUcMtsK/00wmAEfyqKfNdARm8u1wNVhSgaX7A8d4UuIlUI5e84iEwOhs7ZPYRmzU9U6A==",
"dev": true,
+ "bin": {
+ "semver": "bin/semver.js"
+ },
"engines": {
- "node": ">= 10.0.0"
+ "node": ">=10"
}
},
- "node_modules/@storybook/core-server/node_modules/util": {
+ "node_modules/@storybook/core/node_modules/util": {
"version": "0.12.5",
"resolved": "https://registry.npmjs.org/util/-/util-0.12.5.tgz",
"integrity": "sha512-kZf/K6hEIrWHI6XqOFUiiMa+79wE/D8Q+NCNAWclkyg3b4d2k7s0QGepNjiABc+aR3N1PAyHL7p6UcLY6LmrnA==",
@@ -12802,26 +11760,11 @@
"which-typed-array": "^1.1.2"
}
},
- "node_modules/@storybook/core-server/node_modules/watchpack": {
- "version": "2.4.2",
- "resolved": "https://registry.npmjs.org/watchpack/-/watchpack-2.4.2.tgz",
- "integrity": "sha512-TnbFSbcOCcDgjZ4piURLCbJ3nJhznVh9kw6F6iokjiFPl8ONxe9A6nMDVXDiNbrSfLILs6vB07F7wLBrwPYzJw==",
- "dev": true,
- "license": "MIT",
- "dependencies": {
- "glob-to-regexp": "^0.4.1",
- "graceful-fs": "^4.1.2"
- },
- "engines": {
- "node": ">=10.13.0"
- }
- },
- "node_modules/@storybook/core-server/node_modules/ws": {
+ "node_modules/@storybook/core/node_modules/ws": {
"version": "8.18.0",
"resolved": "https://registry.npmjs.org/ws/-/ws-8.18.0.tgz",
"integrity": "sha512-8VbfWfHLbbwu3+N6OKsOMpBdT4kXPDDB9cJk2bJ6mh9ucxdlnNvH1e+roYkKmN9Nxw2yjz7VzeO9oOz2zJ04Pw==",
"dev": true,
- "license": "MIT",
"engines": {
"node": ">=10.0.0"
},
@@ -12838,23 +11781,6 @@
}
}
},
- "node_modules/@storybook/core-webpack": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/core-webpack/-/core-webpack-7.6.15.tgz",
- "integrity": "sha512-6Qk/kc7OKcy4jNowQFz6TFLWM2NYeLoJ73dIbFnN2o8DYS5WwmQLZhZ+MRvr92M+w1nlnc268kaqooYmAj8Mnw==",
- "dev": true,
- "dependencies": {
- "@storybook/core-common": "7.6.15",
- "@storybook/node-logger": "7.6.15",
- "@storybook/types": "7.6.15",
- "@types/node": "^18.0.0",
- "ts-dedent": "^2.0.0"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/storybook"
- }
- },
"node_modules/@storybook/csf": {
"version": "0.1.11",
"resolved": "https://registry.npmjs.org/@storybook/csf/-/csf-0.1.11.tgz",
@@ -12866,90 +11792,19 @@
}
},
"node_modules/@storybook/csf-plugin": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/csf-plugin/-/csf-plugin-7.6.15.tgz",
- "integrity": "sha512-5Pm2B8XKNdG3fHyItWKbWnXHSRDFSvetlML+sMWGWYIjwOsnvPqt+gAvLksWhv/uJgDujGxNcPEh+/Y5C8ZAjQ==",
+ "version": "8.4.7",
+ "resolved": "https://registry.npmjs.org/@storybook/csf-plugin/-/csf-plugin-8.4.7.tgz",
+ "integrity": "sha512-Fgogplu4HImgC+AYDcdGm1rmL6OR1rVdNX1Be9C/NEXwOCpbbBwi0BxTf/2ZxHRk9fCeaPEcOdP5S8QHfltc1g==",
"dev": true,
"dependencies": {
- "@storybook/csf-tools": "7.6.15",
"unplugin": "^1.3.1"
},
"funding": {
"type": "opencollective",
"url": "https://opencollective.com/storybook"
- }
- },
- "node_modules/@storybook/csf-tools": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/csf-tools/-/csf-tools-7.6.15.tgz",
- "integrity": "sha512-8iKgg2cmbFTpVhRRJOqouhPcEh0c8ywabG4S8ICZvnJooSXUI9mD9p3tYCS7MYuSiHj0epa1Kkn9DtXJRo9o6g==",
- "dev": true,
- "dependencies": {
- "@babel/generator": "^7.23.0",
- "@babel/parser": "^7.23.0",
- "@babel/traverse": "^7.23.2",
- "@babel/types": "^7.23.0",
- "@storybook/csf": "^0.1.2",
- "@storybook/types": "7.6.15",
- "fs-extra": "^11.1.0",
- "recast": "^0.23.1",
- "ts-dedent": "^2.0.0"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/storybook"
- }
- },
- "node_modules/@storybook/csf-tools/node_modules/fs-extra": {
- "version": "11.2.0",
- "resolved": "https://registry.npmjs.org/fs-extra/-/fs-extra-11.2.0.tgz",
- "integrity": "sha512-PmDi3uwK5nFuXh7XDTlVnS17xJS7vW36is2+w3xcv8SVxiB4NyATf4ctkVY5bkSjX0Y4nbvZCq1/EjtEyr9ktw==",
- "dev": true,
- "dependencies": {
- "graceful-fs": "^4.2.0",
- "jsonfile": "^6.0.1",
- "universalify": "^2.0.0"
- },
- "engines": {
- "node": ">=14.14"
- }
- },
- "node_modules/@storybook/csf-tools/node_modules/jsonfile": {
- "version": "6.1.0",
- "resolved": "https://registry.npmjs.org/jsonfile/-/jsonfile-6.1.0.tgz",
- "integrity": "sha512-5dgndWOriYSm5cnYaJNhalLNDKOqFwyDB/rr1E9ZsGciGvKPs8R2xYGCacuf3z6K1YKDz182fd+fY3cn3pMqXQ==",
- "dev": true,
- "dependencies": {
- "universalify": "^2.0.0"
- },
- "optionalDependencies": {
- "graceful-fs": "^4.1.6"
- }
- },
- "node_modules/@storybook/csf-tools/node_modules/recast": {
- "version": "0.23.9",
- "resolved": "https://registry.npmjs.org/recast/-/recast-0.23.9.tgz",
- "integrity": "sha512-Hx/BGIbwj+Des3+xy5uAtAbdCyqK9y9wbBcDFDYanLS9JnMqf7OeF87HQwUimE87OEc72mr6tkKUKMBBL+hF9Q==",
- "dev": true,
- "license": "MIT",
- "dependencies": {
- "ast-types": "^0.16.1",
- "esprima": "~4.0.0",
- "source-map": "~0.6.1",
- "tiny-invariant": "^1.3.3",
- "tslib": "^2.0.1"
},
- "engines": {
- "node": ">= 4"
- }
- },
- "node_modules/@storybook/csf-tools/node_modules/universalify": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/universalify/-/universalify-2.0.1.tgz",
- "integrity": "sha512-gptHNQghINnc/vTGIk0SOFGFNXw7JVrlRUtConJRlvaw6DuX0wO5Jeko9sWrMBhh+PsYAZ7oXAiOnf/UKogyiw==",
- "dev": true,
- "engines": {
- "node": ">= 10.0.0"
+ "peerDependencies": {
+ "storybook": "^8.4.7"
}
},
"node_modules/@storybook/csf/node_modules/type-fest": {
@@ -12964,294 +11819,240 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@storybook/docs-mdx": {
- "version": "0.1.0",
- "resolved": "https://registry.npmjs.org/@storybook/docs-mdx/-/docs-mdx-0.1.0.tgz",
- "integrity": "sha512-JDaBR9lwVY4eSH5W8EGHrhODjygPd6QImRbwjAuJNEnY0Vw4ie3bPkeGfnacB3OBW6u/agqPv2aRlR46JcAQLg==",
+ "node_modules/@storybook/global": {
+ "version": "5.0.0",
+ "resolved": "https://registry.npmjs.org/@storybook/global/-/global-5.0.0.tgz",
+ "integrity": "sha512-FcOqPAXACP0I3oJ/ws6/rrPT9WGhu915Cg8D02a9YxLo0DE9zI+a9A5gRGvmQ09fiWPukqI8ZAEoQEdWUKMQdQ==",
"dev": true
},
- "node_modules/@storybook/docs-tools": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/docs-tools/-/docs-tools-7.6.15.tgz",
- "integrity": "sha512-npZEaI9Wpn9uJcRXFElqyiRw8bSxt95mLywPiEEGMT2kE5FfXM8d5Uj5O64kzoXdRI9IhRPEEZZidOtA/UInfQ==",
+ "node_modules/@storybook/icons": {
+ "version": "1.3.0",
+ "resolved": "https://registry.npmjs.org/@storybook/icons/-/icons-1.3.0.tgz",
+ "integrity": "sha512-Nz/UzeYQdUZUhacrPyfkiiysSjydyjgg/p0P9HxB4p/WaJUUjMAcaoaLgy3EXx61zZJ3iD36WPuDkZs5QYrA0A==",
"dev": true,
- "dependencies": {
- "@storybook/core-common": "7.6.15",
- "@storybook/preview-api": "7.6.15",
- "@storybook/types": "7.6.15",
- "@types/doctrine": "^0.0.3",
- "assert": "^2.1.0",
- "doctrine": "^3.0.0",
- "lodash": "^4.17.21"
+ "engines": {
+ "node": ">=14.0.0"
},
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/storybook"
- }
- },
- "node_modules/@storybook/docs-tools/node_modules/assert": {
- "version": "2.1.0",
- "resolved": "https://registry.npmjs.org/assert/-/assert-2.1.0.tgz",
- "integrity": "sha512-eLHpSK/Y4nhMJ07gDaAzoX/XAKS8PSaojml3M0DM4JpV1LAi5JOJ/p6H/XWrl8L+DzVEvVCW1z3vWAaB9oTsQw==",
- "dev": true,
- "dependencies": {
- "call-bind": "^1.0.2",
- "is-nan": "^1.3.2",
- "object-is": "^1.1.5",
- "object.assign": "^4.1.4",
- "util": "^0.12.5"
+ "peerDependencies": {
+ "react": "^16.8.0 || ^17.0.0 || ^18.0.0 || ^19.0.0-beta",
+ "react-dom": "^16.8.0 || ^17.0.0 || ^18.0.0 || ^19.0.0-beta"
}
},
- "node_modules/@storybook/docs-tools/node_modules/doctrine": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/doctrine/-/doctrine-3.0.0.tgz",
- "integrity": "sha512-yS+Q5i3hBf7GBkd4KG8a7eBNNWNGLTaEwwYWUijIYM7zrlYDM0BFXHjjPWlWZ1Rg7UaddZeIDmi9jF3HmqiQ2w==",
+ "node_modules/@storybook/instrumenter": {
+ "version": "8.4.7",
+ "resolved": "https://registry.npmjs.org/@storybook/instrumenter/-/instrumenter-8.4.7.tgz",
+ "integrity": "sha512-k6NSD3jaRCCHAFtqXZ7tw8jAzD/yTEWXGya+REgZqq5RCkmJ+9S4Ytp/6OhQMPtPFX23gAuJJzTQVLcCr+gjRg==",
"dev": true,
"dependencies": {
- "esutils": "^2.0.2"
+ "@storybook/global": "^5.0.0",
+ "@vitest/utils": "^2.1.1"
},
- "engines": {
- "node": ">=6.0.0"
- }
- },
- "node_modules/@storybook/docs-tools/node_modules/util": {
- "version": "0.12.5",
- "resolved": "https://registry.npmjs.org/util/-/util-0.12.5.tgz",
- "integrity": "sha512-kZf/K6hEIrWHI6XqOFUiiMa+79wE/D8Q+NCNAWclkyg3b4d2k7s0QGepNjiABc+aR3N1PAyHL7p6UcLY6LmrnA==",
- "dev": true,
- "dependencies": {
- "inherits": "^2.0.3",
- "is-arguments": "^1.0.4",
- "is-generator-function": "^1.0.7",
- "is-typed-array": "^1.1.3",
- "which-typed-array": "^1.1.2"
- }
- },
- "node_modules/@storybook/global": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/@storybook/global/-/global-5.0.0.tgz",
- "integrity": "sha512-FcOqPAXACP0I3oJ/ws6/rrPT9WGhu915Cg8D02a9YxLo0DE9zI+a9A5gRGvmQ09fiWPukqI8ZAEoQEdWUKMQdQ==",
- "dev": true
- },
- "node_modules/@storybook/manager": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/manager/-/manager-7.6.15.tgz",
- "integrity": "sha512-GGV2ElV5AOIApy/FSDzoSlLUbyd2VhQVD3TdNGRxNauYRjEO8ulXHw2tNbT6ludtpYpDTAILzI6zT/iag8hmPQ==",
- "dev": true,
"funding": {
"type": "opencollective",
"url": "https://opencollective.com/storybook"
+ },
+ "peerDependencies": {
+ "storybook": "^8.4.7"
}
},
- "node_modules/@storybook/manager-api": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/manager-api/-/manager-api-7.6.15.tgz",
- "integrity": "sha512-cPBsXcnJiaO3QyaEum2JgdihYea3cI03FeV35JdrBYLIelT4oqbYFnzjznsFg9+Ia9iAbz7aOBNyyRsWnC/UKw==",
+ "node_modules/@storybook/instrumenter/node_modules/@vitest/pretty-format": {
+ "version": "2.1.8",
+ "resolved": "https://registry.npmjs.org/@vitest/pretty-format/-/pretty-format-2.1.8.tgz",
+ "integrity": "sha512-9HiSZ9zpqNLKlbIDRWOnAWqgcA7xu+8YxXSekhr0Ykab7PAYFkhkwoqVArPOtJhPmYeE2YHgKZlj3CP36z2AJQ==",
"dev": true,
"dependencies": {
- "@storybook/channels": "7.6.15",
- "@storybook/client-logger": "7.6.15",
- "@storybook/core-events": "7.6.15",
- "@storybook/csf": "^0.1.2",
- "@storybook/global": "^5.0.0",
- "@storybook/router": "7.6.15",
- "@storybook/theming": "7.6.15",
- "@storybook/types": "7.6.15",
- "dequal": "^2.0.2",
- "lodash": "^4.17.21",
- "memoizerific": "^1.11.3",
- "store2": "^2.14.2",
- "telejson": "^7.2.0",
- "ts-dedent": "^2.0.0"
+ "tinyrainbow": "^1.2.0"
},
"funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/storybook"
+ "url": "https://opencollective.com/vitest"
}
},
- "node_modules/@storybook/mdx2-csf": {
- "version": "1.1.0",
- "resolved": "https://registry.npmjs.org/@storybook/mdx2-csf/-/mdx2-csf-1.1.0.tgz",
- "integrity": "sha512-TXJJd5RAKakWx4BtpwvSNdgTDkKM6RkXU8GK34S/LhidQ5Pjz3wcnqb0TxEkfhK/ztbP8nKHqXFwLfa2CYkvQw==",
- "dev": true
- },
- "node_modules/@storybook/node-logger": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/node-logger/-/node-logger-7.6.15.tgz",
- "integrity": "sha512-C+sCvRjR+5uVU3VTrfyv7/RlPBxesAjIucUAK0keGyIZ7sFQYCPdkm4m/C4s+TcubgAzVvuoUHlRrSppdA7WzQ==",
+ "node_modules/@storybook/instrumenter/node_modules/@vitest/utils": {
+ "version": "2.1.8",
+ "resolved": "https://registry.npmjs.org/@vitest/utils/-/utils-2.1.8.tgz",
+ "integrity": "sha512-dwSoui6djdwbfFmIgbIjX2ZhIoG7Ex/+xpxyiEgIGzjliY8xGkcpITKTlp6B4MgtGkF2ilvm97cPM96XZaAgcA==",
"dev": true,
+ "dependencies": {
+ "@vitest/pretty-format": "2.1.8",
+ "loupe": "^3.1.2",
+ "tinyrainbow": "^1.2.0"
+ },
"funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/storybook"
+ "url": "https://opencollective.com/vitest"
}
},
- "node_modules/@storybook/postinstall": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/postinstall/-/postinstall-7.6.15.tgz",
- "integrity": "sha512-DXQQ4kjAbQ7BSd9M4lDI/12vEEciYMP8uYFDlrPFjwD9LezsxtRiORkazjNRRX4730faO5zZsnWhXxCVkxck0g==",
+ "node_modules/@storybook/manager-api": {
+ "version": "8.4.7",
+ "resolved": "https://registry.npmjs.org/@storybook/manager-api/-/manager-api-8.4.7.tgz",
+ "integrity": "sha512-ELqemTviCxAsZ5tqUz39sDmQkvhVAvAgiplYy9Uf15kO0SP2+HKsCMzlrm2ue2FfkUNyqbDayCPPCB0Cdn/mpQ==",
"dev": true,
"funding": {
"type": "opencollective",
"url": "https://opencollective.com/storybook"
+ },
+ "peerDependencies": {
+ "storybook": "^8.2.0 || ^8.3.0-0 || ^8.4.0-0 || ^8.5.0-0 || ^8.6.0-0"
}
},
"node_modules/@storybook/preset-react-webpack": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/preset-react-webpack/-/preset-react-webpack-7.6.15.tgz",
- "integrity": "sha512-Oo3J7RKO/tFUVnRXs16tZGcX6n90gTpHdlT2Z1fZ+y8wEd9o+VvvKFEIIeMcRxf3hHa49R6Kbc4AQaE9FAuDlw==",
+ "version": "8.4.7",
+ "resolved": "https://registry.npmjs.org/@storybook/preset-react-webpack/-/preset-react-webpack-8.4.7.tgz",
+ "integrity": "sha512-geTSBKyrBagVihil5MF7LkVFynbfHhCinvnbCZZqXW7M1vgcxvatunUENB+iV8eWg/0EJ+8O7scZL+BAxQ/2qg==",
"dev": true,
"dependencies": {
- "@babel/preset-flow": "^7.22.15",
- "@babel/preset-react": "^7.22.15",
- "@pmmmwh/react-refresh-webpack-plugin": "^0.5.11",
- "@storybook/core-webpack": "7.6.15",
- "@storybook/docs-tools": "7.6.15",
- "@storybook/node-logger": "7.6.15",
- "@storybook/react": "7.6.15",
+ "@storybook/core-webpack": "8.4.7",
+ "@storybook/react": "8.4.7",
"@storybook/react-docgen-typescript-plugin": "1.0.6--canary.9.0c3f3b7.0",
- "@types/node": "^18.0.0",
+ "@types/node": "^22.0.0",
"@types/semver": "^7.3.4",
- "babel-plugin-add-react-displayname": "^0.0.5",
- "fs-extra": "^11.1.0",
+ "find-up": "^5.0.0",
"magic-string": "^0.30.5",
"react-docgen": "^7.0.0",
- "react-refresh": "^0.14.0",
+ "resolve": "^1.22.8",
"semver": "^7.3.7",
+ "tsconfig-paths": "^4.2.0",
"webpack": "5"
},
"engines": {
- "node": ">=16.0.0"
+ "node": ">=18.0.0"
},
"funding": {
"type": "opencollective",
"url": "https://opencollective.com/storybook"
},
"peerDependencies": {
- "@babel/core": "^7.22.0",
- "react": "^16.8.0 || ^17.0.0 || ^18.0.0",
- "react-dom": "^16.8.0 || ^17.0.0 || ^18.0.0"
+ "react": "^16.8.0 || ^17.0.0 || ^18.0.0 || ^19.0.0-beta",
+ "react-dom": "^16.8.0 || ^17.0.0 || ^18.0.0 || ^19.0.0-beta",
+ "storybook": "^8.4.7"
},
"peerDependenciesMeta": {
- "@babel/core": {
- "optional": true
- },
"typescript": {
"optional": true
}
}
},
- "node_modules/@storybook/preset-react-webpack/node_modules/fs-extra": {
- "version": "11.2.0",
- "resolved": "https://registry.npmjs.org/fs-extra/-/fs-extra-11.2.0.tgz",
- "integrity": "sha512-PmDi3uwK5nFuXh7XDTlVnS17xJS7vW36is2+w3xcv8SVxiB4NyATf4ctkVY5bkSjX0Y4nbvZCq1/EjtEyr9ktw==",
+ "node_modules/@storybook/preset-react-webpack/node_modules/find-up": {
+ "version": "5.0.0",
+ "resolved": "https://registry.npmjs.org/find-up/-/find-up-5.0.0.tgz",
+ "integrity": "sha512-78/PXT1wlLLDgTzDs7sjq9hzz0vXD+zn+7wypEe4fXQxCmdmqfGsEPQxmiCSQI3ajFV91bVSsvNtrJRiW6nGng==",
"dev": true,
"dependencies": {
- "graceful-fs": "^4.2.0",
- "jsonfile": "^6.0.1",
- "universalify": "^2.0.0"
+ "locate-path": "^6.0.0",
+ "path-exists": "^4.0.0"
},
"engines": {
- "node": ">=14.14"
+ "node": ">=10"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@storybook/preset-react-webpack/node_modules/jsonfile": {
- "version": "6.1.0",
- "resolved": "https://registry.npmjs.org/jsonfile/-/jsonfile-6.1.0.tgz",
- "integrity": "sha512-5dgndWOriYSm5cnYaJNhalLNDKOqFwyDB/rr1E9ZsGciGvKPs8R2xYGCacuf3z6K1YKDz182fd+fY3cn3pMqXQ==",
+ "node_modules/@storybook/preset-react-webpack/node_modules/locate-path": {
+ "version": "6.0.0",
+ "resolved": "https://registry.npmjs.org/locate-path/-/locate-path-6.0.0.tgz",
+ "integrity": "sha512-iPZK6eYjbxRu3uB4/WZ3EsEIMJFMqAoopl3R+zuq0UjcAm/MO6KCweDgPfP3elTztoKP3KtnVHxTn2NHBSDVUw==",
"dev": true,
"dependencies": {
- "universalify": "^2.0.0"
+ "p-locate": "^5.0.0"
},
- "optionalDependencies": {
- "graceful-fs": "^4.1.6"
+ "engines": {
+ "node": ">=10"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@storybook/preset-react-webpack/node_modules/universalify": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/universalify/-/universalify-2.0.1.tgz",
- "integrity": "sha512-gptHNQghINnc/vTGIk0SOFGFNXw7JVrlRUtConJRlvaw6DuX0wO5Jeko9sWrMBhh+PsYAZ7oXAiOnf/UKogyiw==",
+ "node_modules/@storybook/preset-react-webpack/node_modules/p-locate": {
+ "version": "5.0.0",
+ "resolved": "https://registry.npmjs.org/p-locate/-/p-locate-5.0.0.tgz",
+ "integrity": "sha512-LaNjtRWUBY++zB5nE/NwcaoMylSPk+S+ZHNB1TzdbMJMny6dynpAGt7X/tl/QYq3TIeE6nxHppbo2LGymrG5Pw==",
"dev": true,
+ "dependencies": {
+ "p-limit": "^3.0.2"
+ },
"engines": {
- "node": ">= 10.0.0"
+ "node": ">=10"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@storybook/preview": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/preview/-/preview-7.6.15.tgz",
- "integrity": "sha512-q8d9v0+Bo/DHLV68OyV3Klep4knf2GAbrlHhLW1X4jlPccuEDUojIfqfK7m48ayeIxJzO48fcO0JdKM1XABx7g==",
+ "node_modules/@storybook/preset-react-webpack/node_modules/path-exists": {
+ "version": "4.0.0",
+ "resolved": "https://registry.npmjs.org/path-exists/-/path-exists-4.0.0.tgz",
+ "integrity": "sha512-ak9Qy5Q7jYb2Wwcey5Fpvg2KoAc/ZIhLSLOSBmRmygPsGwkVVt0fZa0qrtMz+m6tJTAHfZQ8FnmB4MG4LWy7/w==",
"dev": true,
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/storybook"
+ "engines": {
+ "node": ">=8"
}
},
- "node_modules/@storybook/preview-api": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/preview-api/-/preview-api-7.6.15.tgz",
- "integrity": "sha512-2KN9vlizF6sFlYsJEGnFqcQaJXs4TTdawC1VazVdtaMSHANDxxDu8F1cP+u7lpPH3DkNZUmTGQDBYfYY9xR0eQ==",
+ "node_modules/@storybook/preset-react-webpack/node_modules/strip-bom": {
+ "version": "3.0.0",
+ "resolved": "https://registry.npmjs.org/strip-bom/-/strip-bom-3.0.0.tgz",
+ "integrity": "sha512-vavAMRXOgBVNF6nyEEmL3DBK19iRpDcoIwW+swQ+CbGiu7lju6t+JklA1MHweoWtadgt4ISVUsXLyDq34ddcwA==",
+ "dev": true,
+ "engines": {
+ "node": ">=4"
+ }
+ },
+ "node_modules/@storybook/preset-react-webpack/node_modules/tsconfig-paths": {
+ "version": "4.2.0",
+ "resolved": "https://registry.npmjs.org/tsconfig-paths/-/tsconfig-paths-4.2.0.tgz",
+ "integrity": "sha512-NoZ4roiN7LnbKn9QqE1amc9DJfzvZXxF4xDavcOWt1BPkdx+m+0gJuPM+S0vCe7zTJMYUP0R8pO2XMr+Y8oLIg==",
"dev": true,
"dependencies": {
- "@storybook/channels": "7.6.15",
- "@storybook/client-logger": "7.6.15",
- "@storybook/core-events": "7.6.15",
- "@storybook/csf": "^0.1.2",
- "@storybook/global": "^5.0.0",
- "@storybook/types": "7.6.15",
- "@types/qs": "^6.9.5",
- "dequal": "^2.0.2",
- "lodash": "^4.17.21",
- "memoizerific": "^1.11.3",
- "qs": "^6.10.0",
- "synchronous-promise": "^2.0.15",
- "ts-dedent": "^2.0.0",
- "util-deprecate": "^1.0.2"
+ "json5": "^2.2.2",
+ "minimist": "^1.2.6",
+ "strip-bom": "^3.0.0"
},
+ "engines": {
+ "node": ">=6"
+ }
+ },
+ "node_modules/@storybook/preview-api": {
+ "version": "8.4.7",
+ "resolved": "https://registry.npmjs.org/@storybook/preview-api/-/preview-api-8.4.7.tgz",
+ "integrity": "sha512-0QVQwHw+OyZGHAJEXo6Knx+6/4er7n2rTDE5RYJ9F2E2Lg42E19pfdLlq2Jhoods2Xrclo3wj6GWR//Ahi39Eg==",
+ "dev": true,
"funding": {
"type": "opencollective",
"url": "https://opencollective.com/storybook"
+ },
+ "peerDependencies": {
+ "storybook": "^8.2.0 || ^8.3.0-0 || ^8.4.0-0 || ^8.5.0-0 || ^8.6.0-0"
}
},
"node_modules/@storybook/react": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/react/-/react-7.6.15.tgz",
- "integrity": "sha512-oJMSh4iTGu6OqCmj0LhkuPyMkxGMTCoohN4HcDpXd96jCSyWotVebRsg9xm5ddB7f54e6DY4XDoGH0WnVoR23g==",
+ "version": "8.4.7",
+ "resolved": "https://registry.npmjs.org/@storybook/react/-/react-8.4.7.tgz",
+ "integrity": "sha512-nQ0/7i2DkaCb7dy0NaT95llRVNYWQiPIVuhNfjr1mVhEP7XD090p0g7eqUmsx8vfdHh2BzWEo6CoBFRd3+EXxw==",
"dev": true,
"dependencies": {
- "@storybook/client-logger": "7.6.15",
- "@storybook/core-client": "7.6.15",
- "@storybook/docs-tools": "7.6.15",
+ "@storybook/components": "8.4.7",
"@storybook/global": "^5.0.0",
- "@storybook/preview-api": "7.6.15",
- "@storybook/react-dom-shim": "7.6.15",
- "@storybook/types": "7.6.15",
- "@types/escodegen": "^0.0.6",
- "@types/estree": "^0.0.51",
- "@types/node": "^18.0.0",
- "acorn": "^7.4.1",
- "acorn-jsx": "^5.3.1",
- "acorn-walk": "^7.2.0",
- "escodegen": "^2.1.0",
- "html-tags": "^3.1.0",
- "lodash": "^4.17.21",
- "prop-types": "^15.7.2",
- "react-element-to-jsx-string": "^15.0.0",
- "ts-dedent": "^2.0.0",
- "type-fest": "~2.19",
- "util-deprecate": "^1.0.2"
+ "@storybook/manager-api": "8.4.7",
+ "@storybook/preview-api": "8.4.7",
+ "@storybook/react-dom-shim": "8.4.7",
+ "@storybook/theming": "8.4.7"
},
"engines": {
- "node": ">=16.0.0"
+ "node": ">=18.0.0"
},
"funding": {
"type": "opencollective",
"url": "https://opencollective.com/storybook"
},
"peerDependencies": {
- "react": "^16.8.0 || ^17.0.0 || ^18.0.0",
- "react-dom": "^16.8.0 || ^17.0.0 || ^18.0.0",
- "typescript": "*"
+ "@storybook/test": "8.4.7",
+ "react": "^16.8.0 || ^17.0.0 || ^18.0.0 || ^19.0.0-beta",
+ "react-dom": "^16.8.0 || ^17.0.0 || ^18.0.0 || ^19.0.0-beta",
+ "storybook": "^8.4.7",
+ "typescript": ">= 4.2.x"
},
"peerDependenciesMeta": {
+ "@storybook/test": {
+ "optional": true
+ },
"typescript": {
"optional": true
}
@@ -13400,112 +12201,67 @@
}
},
"node_modules/@storybook/react-dom-shim": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/react-dom-shim/-/react-dom-shim-7.6.15.tgz",
- "integrity": "sha512-2+X0HIxIyvjfSKVyGGjSJJLEFJ2ox7Rr8FjlMiRo5QfoOJhohZuWH7p4Lw7JMwm5PotnjrwlfsZI3cCilYJeYA==",
+ "version": "8.4.7",
+ "resolved": "https://registry.npmjs.org/@storybook/react-dom-shim/-/react-dom-shim-8.4.7.tgz",
+ "integrity": "sha512-6bkG2jvKTmWrmVzCgwpTxwIugd7Lu+2btsLAqhQSzDyIj2/uhMNp8xIMr/NBDtLgq3nomt9gefNa9xxLwk/OMg==",
"dev": true,
"funding": {
"type": "opencollective",
"url": "https://opencollective.com/storybook"
},
"peerDependencies": {
- "react": "^16.8.0 || ^17.0.0 || ^18.0.0",
- "react-dom": "^16.8.0 || ^17.0.0 || ^18.0.0"
+ "react": "^16.8.0 || ^17.0.0 || ^18.0.0 || ^19.0.0-beta",
+ "react-dom": "^16.8.0 || ^17.0.0 || ^18.0.0 || ^19.0.0-beta",
+ "storybook": "^8.4.7"
}
},
"node_modules/@storybook/react-webpack5": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/react-webpack5/-/react-webpack5-7.6.15.tgz",
- "integrity": "sha512-TyYYSDho+4cQRBCVMKu7XDTCrAsLWaeldCoZm910e4DTXZUV3NDG8hVJIXzweaCu1o7JtDOelxsA6iizR/22GQ==",
+ "version": "8.4.7",
+ "resolved": "https://registry.npmjs.org/@storybook/react-webpack5/-/react-webpack5-8.4.7.tgz",
+ "integrity": "sha512-T9GLqlsP4It4El7cC8rSkBPRWvORAsTDULeWlO36RST2TrYnmBOUytsi22mk7cAAAVhhD6rTrs1YdqWRMpfa1w==",
"dev": true,
"dependencies": {
- "@storybook/builder-webpack5": "7.6.15",
- "@storybook/preset-react-webpack": "7.6.15",
- "@storybook/react": "7.6.15",
- "@types/node": "^18.0.0"
+ "@storybook/builder-webpack5": "8.4.7",
+ "@storybook/preset-react-webpack": "8.4.7",
+ "@storybook/react": "8.4.7",
+ "@types/node": "^22.0.0"
},
"engines": {
- "node": ">=16.0.0"
+ "node": ">=18.0.0"
},
"funding": {
"type": "opencollective",
"url": "https://opencollective.com/storybook"
},
"peerDependencies": {
- "@babel/core": "^7.22.0",
- "react": "^16.8.0 || ^17.0.0 || ^18.0.0",
- "react-dom": "^16.8.0 || ^17.0.0 || ^18.0.0",
- "typescript": "*"
+ "react": "^16.8.0 || ^17.0.0 || ^18.0.0 || ^19.0.0-beta",
+ "react-dom": "^16.8.0 || ^17.0.0 || ^18.0.0 || ^19.0.0-beta",
+ "storybook": "^8.4.7",
+ "typescript": ">= 4.2.x"
},
"peerDependenciesMeta": {
- "@babel/core": {
- "optional": true
- },
"typescript": {
"optional": true
}
}
},
- "node_modules/@storybook/react/node_modules/@types/estree": {
- "version": "0.0.51",
- "resolved": "https://registry.npmjs.org/@types/estree/-/estree-0.0.51.tgz",
- "integrity": "sha512-CuPgU6f3eT/XgKKPqKd/gLZV1Xmvf1a2R5POBOGQa6uv82xpls89HU5zKeVoyR8XzHd1RGNOlQlvUe3CFkjWNQ==",
- "dev": true
- },
- "node_modules/@storybook/react/node_modules/acorn": {
- "version": "7.4.1",
- "resolved": "https://registry.npmjs.org/acorn/-/acorn-7.4.1.tgz",
- "integrity": "sha512-nQyp0o1/mNdbTO1PO6kHkwSrmgZ0MT/jCCpNiwbUjGoRN4dlBhqJtoQuCnEOKzgTVwg0ZWiCoQy6SxMebQVh8A==",
- "dev": true,
- "bin": {
- "acorn": "bin/acorn"
- },
- "engines": {
- "node": ">=0.4.0"
- }
- },
- "node_modules/@storybook/react/node_modules/type-fest": {
- "version": "2.19.0",
- "resolved": "https://registry.npmjs.org/type-fest/-/type-fest-2.19.0.tgz",
- "integrity": "sha512-RAH822pAdBgcNMAfWnCBU3CFZcfZ/i1eZjwFU/dsLKumyuuP3niueg2UAukXYF0E2AAoc82ZSSf9J0WQBinzHA==",
- "dev": true,
- "engines": {
- "node": ">=12.20"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/@storybook/router": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/router/-/router-7.6.15.tgz",
- "integrity": "sha512-5yhXXoVZ1iKUgeZoO8PGqBclrLgoJisxIYVK/Y1iJMXZ2ZvwUiTswLALT6lu97tSrcoBVxmqSghg0+U0YEU4Fg==",
- "dev": true,
- "dependencies": {
- "@storybook/client-logger": "7.6.15",
- "memoizerific": "^1.11.3",
- "qs": "^6.10.0"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/storybook"
- }
- },
"node_modules/@storybook/source-loader": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/source-loader/-/source-loader-7.6.15.tgz",
- "integrity": "sha512-E7LqjfvEUs2dn8ZWc1OfqzXU3vyi2/yP7rPHPRFjDUIpz1QI4IUCUIFY+n3YWkbk8wlmf6dV/2QYzYZPp6RD0g==",
+ "version": "8.4.7",
+ "resolved": "https://registry.npmjs.org/@storybook/source-loader/-/source-loader-8.4.7.tgz",
+ "integrity": "sha512-DrsYGGfNbbqlMzkhbLoNyNqrPa4QIkZ6O7FJ8Z/8jWb0cerQH2N6JW6k12ZnXgs8dO2Z33+iSEDIV8odh0E0PA==",
"dev": true,
"dependencies": {
- "@storybook/csf": "^0.1.2",
- "@storybook/types": "7.6.15",
+ "@storybook/csf": "^0.1.11",
+ "es-toolkit": "^1.22.0",
"estraverse": "^5.2.0",
- "lodash": "^4.17.21",
- "prettier": "^2.8.0"
+ "prettier": "^3.1.1"
},
"funding": {
"type": "opencollective",
"url": "https://opencollective.com/storybook"
+ },
+ "peerDependencies": {
+ "storybook": "^8.4.7"
}
},
"node_modules/@storybook/source-loader/node_modules/estraverse": {
@@ -13518,109 +12274,200 @@
}
},
"node_modules/@storybook/source-loader/node_modules/prettier": {
- "version": "2.8.8",
- "resolved": "https://registry.npmjs.org/prettier/-/prettier-2.8.8.tgz",
- "integrity": "sha512-tdN8qQGvNjw4CHbY+XXk0JgCXn9QiF21a55rBe5LJAU+kDyC4WQn4+awm2Xfk2lQMk5fKup9XgzTZtGkjBdP9Q==",
+ "version": "3.4.2",
+ "resolved": "https://registry.npmjs.org/prettier/-/prettier-3.4.2.tgz",
+ "integrity": "sha512-e9MewbtFo+Fevyuxn/4rrcDAaq0IYxPGLvObpQjiZBMAzB9IGmzlnG9RZy3FFas+eBMu2vA0CszMeduow5dIuQ==",
"dev": true,
"bin": {
- "prettier": "bin-prettier.js"
+ "prettier": "bin/prettier.cjs"
},
"engines": {
- "node": ">=10.13.0"
+ "node": ">=14"
},
"funding": {
"url": "https://github.com/prettier/prettier?sponsor=1"
}
},
- "node_modules/@storybook/telemetry": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/telemetry/-/telemetry-7.6.15.tgz",
- "integrity": "sha512-klhKXLUS3OXozGEtMbbhKZLDfm+m3nNk2jvGwD6kkBenzFUzb0P2m8awxU7h1pBcKZKH/27U9t3KVzNFzWoWPw==",
+ "node_modules/@storybook/test": {
+ "version": "8.4.7",
+ "resolved": "https://registry.npmjs.org/@storybook/test/-/test-8.4.7.tgz",
+ "integrity": "sha512-AhvJsu5zl3uG40itSQVuSy5WByp3UVhS6xAnme4FWRwgSxhvZjATJ3AZkkHWOYjnnk+P2/sbz/XuPli1FVCWoQ==",
"dev": true,
"dependencies": {
- "@storybook/client-logger": "7.6.15",
- "@storybook/core-common": "7.6.15",
- "@storybook/csf-tools": "7.6.15",
- "chalk": "^4.1.0",
- "detect-package-manager": "^2.0.1",
- "fetch-retry": "^5.0.2",
- "fs-extra": "^11.1.0",
- "read-pkg-up": "^7.0.1"
+ "@storybook/csf": "^0.1.11",
+ "@storybook/global": "^5.0.0",
+ "@storybook/instrumenter": "8.4.7",
+ "@testing-library/dom": "10.4.0",
+ "@testing-library/jest-dom": "6.5.0",
+ "@testing-library/user-event": "14.5.2",
+ "@vitest/expect": "2.0.5",
+ "@vitest/spy": "2.0.5"
},
"funding": {
"type": "opencollective",
"url": "https://opencollective.com/storybook"
+ },
+ "peerDependencies": {
+ "storybook": "^8.4.7"
}
},
- "node_modules/@storybook/telemetry/node_modules/fs-extra": {
- "version": "11.2.0",
- "resolved": "https://registry.npmjs.org/fs-extra/-/fs-extra-11.2.0.tgz",
- "integrity": "sha512-PmDi3uwK5nFuXh7XDTlVnS17xJS7vW36is2+w3xcv8SVxiB4NyATf4ctkVY5bkSjX0Y4nbvZCq1/EjtEyr9ktw==",
+ "node_modules/@storybook/test/node_modules/@testing-library/dom": {
+ "version": "10.4.0",
+ "resolved": "https://registry.npmjs.org/@testing-library/dom/-/dom-10.4.0.tgz",
+ "integrity": "sha512-pemlzrSESWbdAloYml3bAJMEfNh1Z7EduzqPKprCH5S341frlpYnUEW0H72dLxa6IsYr+mPno20GiSm+h9dEdQ==",
"dev": true,
"dependencies": {
- "graceful-fs": "^4.2.0",
- "jsonfile": "^6.0.1",
- "universalify": "^2.0.0"
+ "@babel/code-frame": "^7.10.4",
+ "@babel/runtime": "^7.12.5",
+ "@types/aria-query": "^5.0.1",
+ "aria-query": "5.3.0",
+ "chalk": "^4.1.0",
+ "dom-accessibility-api": "^0.5.9",
+ "lz-string": "^1.5.0",
+ "pretty-format": "^27.0.2"
},
"engines": {
- "node": ">=14.14"
+ "node": ">=18"
}
},
- "node_modules/@storybook/telemetry/node_modules/jsonfile": {
- "version": "6.1.0",
- "resolved": "https://registry.npmjs.org/jsonfile/-/jsonfile-6.1.0.tgz",
- "integrity": "sha512-5dgndWOriYSm5cnYaJNhalLNDKOqFwyDB/rr1E9ZsGciGvKPs8R2xYGCacuf3z6K1YKDz182fd+fY3cn3pMqXQ==",
+ "node_modules/@storybook/test/node_modules/@testing-library/jest-dom": {
+ "version": "6.5.0",
+ "resolved": "https://registry.npmjs.org/@testing-library/jest-dom/-/jest-dom-6.5.0.tgz",
+ "integrity": "sha512-xGGHpBXYSHUUr6XsKBfs85TWlYKpTc37cSBBVrXcib2MkHLboWlkClhWF37JKlDb9KEq3dHs+f2xR7XJEWGBxA==",
"dev": true,
"dependencies": {
- "universalify": "^2.0.0"
+ "@adobe/css-tools": "^4.4.0",
+ "aria-query": "^5.0.0",
+ "chalk": "^3.0.0",
+ "css.escape": "^1.5.1",
+ "dom-accessibility-api": "^0.6.3",
+ "lodash": "^4.17.21",
+ "redent": "^3.0.0"
},
- "optionalDependencies": {
- "graceful-fs": "^4.1.6"
+ "engines": {
+ "node": ">=14",
+ "npm": ">=6",
+ "yarn": ">=1"
}
},
- "node_modules/@storybook/telemetry/node_modules/universalify": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/universalify/-/universalify-2.0.1.tgz",
- "integrity": "sha512-gptHNQghINnc/vTGIk0SOFGFNXw7JVrlRUtConJRlvaw6DuX0wO5Jeko9sWrMBhh+PsYAZ7oXAiOnf/UKogyiw==",
+ "node_modules/@storybook/test/node_modules/@testing-library/jest-dom/node_modules/chalk": {
+ "version": "3.0.0",
+ "resolved": "https://registry.npmjs.org/chalk/-/chalk-3.0.0.tgz",
+ "integrity": "sha512-4D3B6Wf41KOYRFdszmDqMCGq5VV/uMAB273JILmO+3jAlh8X4qDtdtgCR3fxtbLEMzSx22QdhnDcJvu2u1fVwg==",
"dev": true,
+ "dependencies": {
+ "ansi-styles": "^4.1.0",
+ "supports-color": "^7.1.0"
+ },
"engines": {
- "node": ">= 10.0.0"
+ "node": ">=8"
}
},
- "node_modules/@storybook/theming": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/theming/-/theming-7.6.15.tgz",
- "integrity": "sha512-9PpsHAbUf6o0w33/P3mnb7QheTmfGlTYCismj5HMM1O2/zY0kQK9XcG9W+Cyvu56D/lFC19fz9YHQY8W4AbfnQ==",
+ "node_modules/@storybook/test/node_modules/@testing-library/jest-dom/node_modules/dom-accessibility-api": {
+ "version": "0.6.3",
+ "resolved": "https://registry.npmjs.org/dom-accessibility-api/-/dom-accessibility-api-0.6.3.tgz",
+ "integrity": "sha512-7ZgogeTnjuHbo+ct10G9Ffp0mif17idi0IyWNVA/wcwcm7NPOD/WEHVP3n7n3MhXqxoIYm8d6MuZohYWIZ4T3w==",
+ "dev": true
+ },
+ "node_modules/@storybook/test/node_modules/@testing-library/user-event": {
+ "version": "14.5.2",
+ "resolved": "https://registry.npmjs.org/@testing-library/user-event/-/user-event-14.5.2.tgz",
+ "integrity": "sha512-YAh82Wh4TIrxYLmfGcixwD18oIjyC1pFQC2Y01F2lzV2HTMiYrI0nze0FD0ocB//CKS/7jIUgae+adPqxK5yCQ==",
+ "dev": true,
+ "engines": {
+ "node": ">=12",
+ "npm": ">=6"
+ },
+ "peerDependencies": {
+ "@testing-library/dom": ">=7.21.4"
+ }
+ },
+ "node_modules/@storybook/test/node_modules/aria-query": {
+ "version": "5.3.0",
+ "resolved": "https://registry.npmjs.org/aria-query/-/aria-query-5.3.0.tgz",
+ "integrity": "sha512-b0P0sZPKtyu8HkeRAfCq0IfURZK+SuwMjY1UXGBU27wpAiTwQAIlq56IbIO+ytk/JjS1fMR14ee5WBBfKi5J6A==",
"dev": true,
"dependencies": {
- "@emotion/use-insertion-effect-with-fallbacks": "^1.0.0",
- "@storybook/client-logger": "7.6.15",
- "@storybook/global": "^5.0.0",
- "memoizerific": "^1.11.3"
+ "dequal": "^2.0.3"
+ }
+ },
+ "node_modules/@storybook/test/node_modules/has-flag": {
+ "version": "4.0.0",
+ "resolved": "https://registry.npmjs.org/has-flag/-/has-flag-4.0.0.tgz",
+ "integrity": "sha512-EykJT/Q1KjTWctppgIAgfSO0tKVuZUjhgMr17kqTumMl6Afv3EISleU7qZUzoXDFTAHTDC4NOoG/ZxU3EvlMPQ==",
+ "dev": true,
+ "engines": {
+ "node": ">=8"
+ }
+ },
+ "node_modules/@storybook/test/node_modules/pretty-format": {
+ "version": "27.5.1",
+ "resolved": "https://registry.npmjs.org/pretty-format/-/pretty-format-27.5.1.tgz",
+ "integrity": "sha512-Qb1gy5OrP5+zDf2Bvnzdl3jsTf1qXVMazbvCoKhtKqVs4/YK4ozX4gKQJJVyNe+cajNPn0KoC0MC3FUmaHWEmQ==",
+ "dev": true,
+ "dependencies": {
+ "ansi-regex": "^5.0.1",
+ "ansi-styles": "^5.0.0",
+ "react-is": "^17.0.1"
+ },
+ "engines": {
+ "node": "^10.13.0 || ^12.13.0 || ^14.15.0 || >=15.0.0"
+ }
+ },
+ "node_modules/@storybook/test/node_modules/pretty-format/node_modules/ansi-styles": {
+ "version": "5.2.0",
+ "resolved": "https://registry.npmjs.org/ansi-styles/-/ansi-styles-5.2.0.tgz",
+ "integrity": "sha512-Cxwpt2SfTzTtXcfOlzGEee8O+c+MmUgGrNiBcXnuWxuFJHe6a5Hz7qwhwe5OgaSYI0IJvkLqWX1ASG+cJOkEiA==",
+ "dev": true,
+ "engines": {
+ "node": ">=10"
+ },
+ "funding": {
+ "url": "https://github.com/chalk/ansi-styles?sponsor=1"
+ }
+ },
+ "node_modules/@storybook/test/node_modules/react-is": {
+ "version": "17.0.2",
+ "resolved": "https://registry.npmjs.org/react-is/-/react-is-17.0.2.tgz",
+ "integrity": "sha512-w2GsyukL62IJnlaff/nRegPQR94C/XXamvMWmSHRJ4y7Ts/4ocGRmTHvOs8PSE6pB3dWOrD/nueuU5sduBsQ4w==",
+ "dev": true
+ },
+ "node_modules/@storybook/test/node_modules/supports-color": {
+ "version": "7.2.0",
+ "resolved": "https://registry.npmjs.org/supports-color/-/supports-color-7.2.0.tgz",
+ "integrity": "sha512-qpCAvRl9stuOHveKsn7HncJRvv501qIacKzQlO/+Lwxc9+0q2wLyv4Dfvt80/DPn2pqOBsJdDiogXGR9+OvwRw==",
+ "dev": true,
+ "dependencies": {
+ "has-flag": "^4.0.0"
},
+ "engines": {
+ "node": ">=8"
+ }
+ },
+ "node_modules/@storybook/theming": {
+ "version": "8.4.7",
+ "resolved": "https://registry.npmjs.org/@storybook/theming/-/theming-8.4.7.tgz",
+ "integrity": "sha512-99rgLEjf7iwfSEmdqlHkSG3AyLcK0sfExcr0jnc6rLiAkBhzuIsvcHjjUwkR210SOCgXqBPW0ZA6uhnuyppHLw==",
+ "dev": true,
"funding": {
"type": "opencollective",
"url": "https://opencollective.com/storybook"
},
"peerDependencies": {
- "react": "^16.8.0 || ^17.0.0 || ^18.0.0",
- "react-dom": "^16.8.0 || ^17.0.0 || ^18.0.0"
+ "storybook": "^8.2.0 || ^8.3.0-0 || ^8.4.0-0 || ^8.5.0-0 || ^8.6.0-0"
}
},
"node_modules/@storybook/types": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/@storybook/types/-/types-7.6.15.tgz",
- "integrity": "sha512-tLH0lK6SXECSfMpKin9bge+7XiHZII17n6jc9ZI1TfSBZJyq3M6VzWh2r1C2lC97FlkcKXjIwM3n8h1xNjnI+A==",
+ "version": "8.4.7",
+ "resolved": "https://registry.npmjs.org/@storybook/types/-/types-8.4.7.tgz",
+ "integrity": "sha512-zuf0uPFjODB9Ls9/lqXnb1YsDKFuaASLOpTzpRlz9amFtTepo1dB0nVF9ZWcseTgGs7UxA4+ZR2SZrduXw/ihw==",
"dev": true,
- "dependencies": {
- "@storybook/channels": "7.6.15",
- "@types/babel__core": "^7.0.0",
- "@types/express": "^4.7.0",
- "file-system-cache": "2.3.0"
- },
"funding": {
"type": "opencollective",
"url": "https://opencollective.com/storybook"
+ },
+ "peerDependencies": {
+ "storybook": "^8.2.0 || ^8.3.0-0 || ^8.4.0-0 || ^8.5.0-0 || ^8.6.0-0"
}
},
"node_modules/@stylistic/stylelint-plugin": {
@@ -14012,216 +12859,6 @@
"url": "https://github.com/sponsors/gregberge"
}
},
- "node_modules/@swc/core": {
- "version": "1.4.1",
- "resolved": "https://registry.npmjs.org/@swc/core/-/core-1.4.1.tgz",
- "integrity": "sha512-3y+Y8js+e7BbM16iND+6Rcs3jdiL28q3iVtYsCviYSSpP2uUVKkp5sJnCY4pg8AaVvyN7CGQHO7gLEZQ5ByozQ==",
- "dev": true,
- "hasInstallScript": true,
- "dependencies": {
- "@swc/counter": "^0.1.2",
- "@swc/types": "^0.1.5"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/swc"
- },
- "optionalDependencies": {
- "@swc/core-darwin-arm64": "1.4.1",
- "@swc/core-darwin-x64": "1.4.1",
- "@swc/core-linux-arm-gnueabihf": "1.4.1",
- "@swc/core-linux-arm64-gnu": "1.4.1",
- "@swc/core-linux-arm64-musl": "1.4.1",
- "@swc/core-linux-x64-gnu": "1.4.1",
- "@swc/core-linux-x64-musl": "1.4.1",
- "@swc/core-win32-arm64-msvc": "1.4.1",
- "@swc/core-win32-ia32-msvc": "1.4.1",
- "@swc/core-win32-x64-msvc": "1.4.1"
- },
- "peerDependencies": {
- "@swc/helpers": "^0.5.0"
- },
- "peerDependenciesMeta": {
- "@swc/helpers": {
- "optional": true
- }
- }
- },
- "node_modules/@swc/core-darwin-arm64": {
- "version": "1.4.1",
- "resolved": "https://registry.npmjs.org/@swc/core-darwin-arm64/-/core-darwin-arm64-1.4.1.tgz",
- "integrity": "sha512-ePyfx0348UbR4DOAW24TedeJbafnzha8liXFGuQ4bdXtEVXhLfPngprrxKrAddCuv42F9aTxydlF6+adD3FBhA==",
- "cpu": [
- "arm64"
- ],
- "dev": true,
- "optional": true,
- "os": [
- "darwin"
- ],
- "engines": {
- "node": ">=10"
- }
- },
- "node_modules/@swc/core-darwin-x64": {
- "version": "1.4.1",
- "resolved": "https://registry.npmjs.org/@swc/core-darwin-x64/-/core-darwin-x64-1.4.1.tgz",
- "integrity": "sha512-eLf4JSe6VkCMdDowjM8XNC5rO+BrgfbluEzAVtKR8L2HacNYukieumN7EzpYCi0uF1BYwu1ku6tLyG2r0VcGxA==",
- "cpu": [
- "x64"
- ],
- "dev": true,
- "optional": true,
- "os": [
- "darwin"
- ],
- "engines": {
- "node": ">=10"
- }
- },
- "node_modules/@swc/core-linux-arm-gnueabihf": {
- "version": "1.4.1",
- "resolved": "https://registry.npmjs.org/@swc/core-linux-arm-gnueabihf/-/core-linux-arm-gnueabihf-1.4.1.tgz",
- "integrity": "sha512-K8VtTLWMw+rkN/jDC9o/Q9SMmzdiHwYo2CfgkwVT29NsGccwmNhCQx6XoYiPKyKGIFKt4tdQnJHKUFzxUqQVtQ==",
- "cpu": [
- "arm"
- ],
- "dev": true,
- "optional": true,
- "os": [
- "linux"
- ],
- "engines": {
- "node": ">=10"
- }
- },
- "node_modules/@swc/core-linux-arm64-gnu": {
- "version": "1.4.1",
- "resolved": "https://registry.npmjs.org/@swc/core-linux-arm64-gnu/-/core-linux-arm64-gnu-1.4.1.tgz",
- "integrity": "sha512-0e8p4g0Bfkt8lkiWgcdiENH3RzkcqKtpRXIVNGOmVc0OBkvc2tpm2WTx/eoCnes2HpTT4CTtR3Zljj4knQ4Fvw==",
- "cpu": [
- "arm64"
- ],
- "dev": true,
- "optional": true,
- "os": [
- "linux"
- ],
- "engines": {
- "node": ">=10"
- }
- },
- "node_modules/@swc/core-linux-arm64-musl": {
- "version": "1.4.1",
- "resolved": "https://registry.npmjs.org/@swc/core-linux-arm64-musl/-/core-linux-arm64-musl-1.4.1.tgz",
- "integrity": "sha512-b/vWGQo2n7lZVUnSQ7NBq3Qrj85GrAPPiRbpqaIGwOytiFSk8VULFihbEUwDe0rXgY4LDm8z8wkgADZcLnmdUA==",
- "cpu": [
- "arm64"
- ],
- "dev": true,
- "optional": true,
- "os": [
- "linux"
- ],
- "engines": {
- "node": ">=10"
- }
- },
- "node_modules/@swc/core-linux-x64-gnu": {
- "version": "1.4.1",
- "resolved": "https://registry.npmjs.org/@swc/core-linux-x64-gnu/-/core-linux-x64-gnu-1.4.1.tgz",
- "integrity": "sha512-AFMQlvkKEdNi1Vk2GFTxxJzbICttBsOQaXa98kFTeWTnFFIyiIj2w7Sk8XRTEJ/AjF8ia8JPKb1zddBWr9+bEQ==",
- "cpu": [
- "x64"
- ],
- "dev": true,
- "optional": true,
- "os": [
- "linux"
- ],
- "engines": {
- "node": ">=10"
- }
- },
- "node_modules/@swc/core-linux-x64-musl": {
- "version": "1.4.1",
- "resolved": "https://registry.npmjs.org/@swc/core-linux-x64-musl/-/core-linux-x64-musl-1.4.1.tgz",
- "integrity": "sha512-QX2MxIECX1gfvUVZY+jk528/oFkS9MAl76e3ZRvG2KC/aKlCQL0KSzcTSm13mOxkDKS30EaGRDRQWNukGpMeRg==",
- "cpu": [
- "x64"
- ],
- "dev": true,
- "optional": true,
- "os": [
- "linux"
- ],
- "engines": {
- "node": ">=10"
- }
- },
- "node_modules/@swc/core-win32-arm64-msvc": {
- "version": "1.4.1",
- "resolved": "https://registry.npmjs.org/@swc/core-win32-arm64-msvc/-/core-win32-arm64-msvc-1.4.1.tgz",
- "integrity": "sha512-OklkJYXXI/tntD2zaY8i3iZldpyDw5q+NAP3k9OlQ7wXXf37djRsHLV0NW4+ZNHBjE9xp2RsXJ0jlOJhfgGoFA==",
- "cpu": [
- "arm64"
- ],
- "dev": true,
- "optional": true,
- "os": [
- "win32"
- ],
- "engines": {
- "node": ">=10"
- }
- },
- "node_modules/@swc/core-win32-ia32-msvc": {
- "version": "1.4.1",
- "resolved": "https://registry.npmjs.org/@swc/core-win32-ia32-msvc/-/core-win32-ia32-msvc-1.4.1.tgz",
- "integrity": "sha512-MBuc3/QfKX9FnLOU7iGN+6yHRTQaPQ9WskiC8s8JFiKQ+7I2p25tay2RplR9dIEEGgVAu6L7auv96LbNTh+FaA==",
- "cpu": [
- "ia32"
- ],
- "dev": true,
- "optional": true,
- "os": [
- "win32"
- ],
- "engines": {
- "node": ">=10"
- }
- },
- "node_modules/@swc/core-win32-x64-msvc": {
- "version": "1.4.1",
- "resolved": "https://registry.npmjs.org/@swc/core-win32-x64-msvc/-/core-win32-x64-msvc-1.4.1.tgz",
- "integrity": "sha512-lu4h4wFBb/bOK6N2MuZwg7TrEpwYXgpQf5R7ObNSXL65BwZ9BG8XRzD+dLJmALu8l5N08rP/TrpoKRoGT4WSxw==",
- "cpu": [
- "x64"
- ],
- "dev": true,
- "optional": true,
- "os": [
- "win32"
- ],
- "engines": {
- "node": ">=10"
- }
- },
- "node_modules/@swc/counter": {
- "version": "0.1.3",
- "resolved": "https://registry.npmjs.org/@swc/counter/-/counter-0.1.3.tgz",
- "integrity": "sha512-e2BR4lsJkkRlKZ/qCHPw9ZaSxc0MVUd7gtbtaB7aMvHeJVYe8sOB8DBZkP2DtISHGSku9sCK6T6cnY0CtXrOCQ==",
- "dev": true
- },
- "node_modules/@swc/types": {
- "version": "0.1.5",
- "resolved": "https://registry.npmjs.org/@swc/types/-/types-0.1.5.tgz",
- "integrity": "sha512-myfUej5naTBWnqOCc/MdVOLVjXUXtIA+NpDrDBKJtLLg2shUjBu3cZmB/85RyitKc55+lUUyl7oRfLOvkr2hsw==",
- "dev": true
- },
"node_modules/@szmarczak/http-timer": {
"version": "4.0.6",
"resolved": "https://registry.npmjs.org/@szmarczak/http-timer/-/http-timer-4.0.6.tgz",
@@ -14261,9 +12898,9 @@
"integrity": "sha512-oocsqY7g0cR+Gur5jRQLSrX2OtpMLMse1I10JQBm8CdGMrDkh1Mg2gjsiquMHRtBs4Qwu5wgEp5GgIYHk4SNPw=="
},
"node_modules/@testing-library/dom": {
- "version": "9.3.1",
- "resolved": "https://registry.npmjs.org/@testing-library/dom/-/dom-9.3.1.tgz",
- "integrity": "sha512-0DGPd9AR3+iDTjGoMpxIkAsUihHZ3Ai6CneU6bRRrffXMgzCdlNk43jTrD2/5LT6CBb3MWTP8v510JzYtahD2w==",
+ "version": "9.3.4",
+ "resolved": "https://registry.npmjs.org/@testing-library/dom/-/dom-9.3.4.tgz",
+ "integrity": "sha512-FlS4ZWlp97iiNWig0Muq8p+3rVDjRiYE+YKGbAqXOu9nwJFFOdL00kFpz42M+4huzYi86vAK1sOOfyOG45muIQ==",
"dev": true,
"dependencies": {
"@babel/code-frame": "^7.10.4",
@@ -14491,28 +13128,31 @@
"version": "14.1.0",
"resolved": "https://registry.npmjs.org/@tsconfig/node14/-/node14-14.1.0.tgz",
"integrity": "sha512-VmsCG04YR58ciHBeJKBDNMWWfYbyP8FekWVuTlpstaUPlat1D0x/tXzkWP7yCMU0eSz9V4OZU0LBWTFJ3xZf6w==",
- "dev": true
+ "dev": true,
+ "license": "MIT"
},
"node_modules/@tufjs/canonical-json": {
- "version": "1.0.0",
- "resolved": "https://registry.npmjs.org/@tufjs/canonical-json/-/canonical-json-1.0.0.tgz",
- "integrity": "sha512-QTnf++uxunWvG2z3UFNzAoQPHxnSXOwtaI3iJ+AohhV+5vONuArPjJE7aPXPVXfXJsqrVbZBu9b81AJoSd09IQ==",
+ "version": "2.0.0",
+ "resolved": "https://registry.npmjs.org/@tufjs/canonical-json/-/canonical-json-2.0.0.tgz",
+ "integrity": "sha512-yVtV8zsdo8qFHe+/3kw81dSLyF7D576A5cCFCi4X7B39tWT7SekaEFUnvnWJHz+9qO7qJTah1JbrDjWKqFtdWA==",
"dev": true,
+ "license": "MIT",
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/@tufjs/models": {
- "version": "1.0.4",
- "resolved": "https://registry.npmjs.org/@tufjs/models/-/models-1.0.4.tgz",
- "integrity": "sha512-qaGV9ltJP0EO25YfFUPhxRVK0evXFIAGicsVXuRim4Ed9cjPxYhNnNJ49SFmbeLgtxpslIkX317IgpfcHPVj/A==",
+ "version": "2.0.1",
+ "resolved": "https://registry.npmjs.org/@tufjs/models/-/models-2.0.1.tgz",
+ "integrity": "sha512-92F7/SFyufn4DXsha9+QfKnN03JGqtMFMXgSHbZOo8JG59WkTni7UzAouNQDf7AuP9OAMxVOPQcqG3sB7w+kkg==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "@tufjs/canonical-json": "1.0.0",
- "minimatch": "^9.0.0"
+ "@tufjs/canonical-json": "2.0.0",
+ "minimatch": "^9.0.4"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/@tufjs/models/node_modules/brace-expansion": {
@@ -14520,6 +13160,7 @@
"resolved": "https://registry.npmjs.org/brace-expansion/-/brace-expansion-2.0.1.tgz",
"integrity": "sha512-XnAIvQ8eM+kC6aULx6wuQiwVsnzsi9d3WxzV3FpWTGA19F621kwdbsAcFKXgKUHZWsy+mY6iL1sHTxWEFCytDA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"balanced-match": "^1.0.0"
}
@@ -14540,11 +13181,22 @@
"url": "https://github.com/sponsors/isaacs"
}
},
+ "node_modules/@tybys/wasm-util": {
+ "version": "0.9.0",
+ "resolved": "https://registry.npmjs.org/@tybys/wasm-util/-/wasm-util-0.9.0.tgz",
+ "integrity": "sha512-6+7nlbMVX/PVDCwaIQ8nTOPveOcFLSt8GcXdx8hD0bt39uWxYT88uXzqTd4fTvqta7oeUJqudepapKNt2DYJFw==",
+ "dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "tslib": "^2.4.0"
+ }
+ },
"node_modules/@types/archiver": {
"version": "5.3.3",
"resolved": "https://registry.npmjs.org/@types/archiver/-/archiver-5.3.3.tgz",
"integrity": "sha512-0ABdVcXL6jOwNGY+hjWPqrxUvKelBEwNLcuv/SV2vZ4YCH8w9NttFCt+/QqI5zgMX+iX/XqVy89/r7EmLJmMpQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"@types/readdir-glob": "*"
}
@@ -14565,7 +13217,8 @@
"version": "1.4.0",
"resolved": "https://registry.npmjs.org/@types/async-lock/-/async-lock-1.4.0.tgz",
"integrity": "sha512-2+rYSaWrpdbQG3SA0LmMT6YxWLrI81AqpMlSkw3QtFc2HGDufkweQSn30Eiev7x9LL0oyFrBqk1PXOnB9IEgKg==",
- "dev": true
+ "dev": true,
+ "license": "MIT"
},
"node_modules/@types/babel__core": {
"version": "7.20.0",
@@ -14609,6 +13262,7 @@
"resolved": "https://registry.npmjs.org/@types/base64-stream/-/base64-stream-1.0.3.tgz",
"integrity": "sha512-cbLPBuRLRq7l2+syfKT3byML5C/UNK/6hkcDDMxwttE+NtpXvQxhgXj0PNbcLCwUCmfEWMm3eRBpDmpieLegDQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"@types/node": "*"
}
@@ -14629,9 +13283,10 @@
}
},
"node_modules/@types/bonjour": {
- "version": "3.5.11",
- "resolved": "https://registry.npmjs.org/@types/bonjour/-/bonjour-3.5.11.tgz",
- "integrity": "sha512-isGhjmBtLIxdHBDl2xGwUzEM8AOyOvWsADWq7rqirdi/ZQoHnLWErHvsThcEzTX8juDRiZtzp2Qkv5bgNh6mAg==",
+ "version": "3.5.13",
+ "resolved": "https://registry.npmjs.org/@types/bonjour/-/bonjour-3.5.13.tgz",
+ "integrity": "sha512-z9fJ5Im06zvUL548KvYNecEVlA7cVDkGUi6kZusb04mpyEFKCIZJvloCcmpmLaIahDpOQGHaHmG6imtPMmPXGQ==",
+ "license": "MIT",
"dependencies": {
"@types/node": "*"
}
@@ -14656,63 +13311,40 @@
}
},
"node_modules/@types/connect-history-api-fallback": {
- "version": "1.5.1",
- "resolved": "https://registry.npmjs.org/@types/connect-history-api-fallback/-/connect-history-api-fallback-1.5.1.tgz",
- "integrity": "sha512-iaQslNbARe8fctL5Lk+DsmgWOM83lM+7FzP0eQUJs1jd3kBE8NWqBTIT2S8SqQOJjxvt2eyIjpOuYeRXq2AdMw==",
+ "version": "1.5.4",
+ "resolved": "https://registry.npmjs.org/@types/connect-history-api-fallback/-/connect-history-api-fallback-1.5.4.tgz",
+ "integrity": "sha512-n6Cr2xS1h4uAulPRdlw6Jl6s1oG8KrVilPN2yUITEs+K48EzMJJ3W1xy8K5eWuFvjp3R74AOIGSmp2UfBJ8HFw==",
+ "license": "MIT",
"dependencies": {
"@types/express-serve-static-core": "*",
"@types/node": "*"
}
},
- "node_modules/@types/cross-spawn": {
- "version": "6.0.6",
- "resolved": "https://registry.npmjs.org/@types/cross-spawn/-/cross-spawn-6.0.6.tgz",
- "integrity": "sha512-fXRhhUkG4H3TQk5dBhQ7m/JDdSNHKwR2BBia62lhwEIq9xGiQKLxd6LymNhn47SjXhsUEPmxi+PKw2OkW4LLjA==",
- "dev": true,
- "dependencies": {
- "@types/node": "*"
- }
- },
- "node_modules/@types/detect-port": {
- "version": "1.3.5",
- "resolved": "https://registry.npmjs.org/@types/detect-port/-/detect-port-1.3.5.tgz",
- "integrity": "sha512-Rf3/lB9WkDfIL9eEKaSYKc+1L/rNVYBjThk22JTqQw0YozXarX8YljFAz+HCoC6h4B4KwCMsBPZHaFezwT4BNA==",
- "dev": true
- },
"node_modules/@types/doctrine": {
- "version": "0.0.3",
- "resolved": "https://registry.npmjs.org/@types/doctrine/-/doctrine-0.0.3.tgz",
- "integrity": "sha512-w5jZ0ee+HaPOaX25X2/2oGR/7rgAQSYII7X7pp0m9KgBfMP7uKfMfTvcpl5Dj+eDBbpxKGiqE+flqDr6XTd2RA==",
- "dev": true
- },
- "node_modules/@types/ejs": {
- "version": "3.1.5",
- "resolved": "https://registry.npmjs.org/@types/ejs/-/ejs-3.1.5.tgz",
- "integrity": "sha512-nv+GSx77ZtXiJzwKdsASqi+YQ5Z7vwHsTP0JY2SiQgjGckkBRKZnk8nIM+7oUZ1VCtuTz0+By4qVR7fqzp/Dfg==",
- "dev": true
- },
- "node_modules/@types/emscripten": {
- "version": "1.39.10",
- "resolved": "https://registry.npmjs.org/@types/emscripten/-/emscripten-1.39.10.tgz",
- "integrity": "sha512-TB/6hBkYQJxsZHSqyeuO1Jt0AB/bW6G7rHt9g7lML7SOF6lbgcHvw/Lr+69iqN0qxgXLhWKScAon73JNnptuDw==",
- "dev": true
- },
- "node_modules/@types/escodegen": {
- "version": "0.0.6",
- "resolved": "https://registry.npmjs.org/@types/escodegen/-/escodegen-0.0.6.tgz",
- "integrity": "sha512-AjwI4MvWx3HAOaZqYsjKWyEObT9lcVV0Y0V8nXo6cXzN8ZiMxVhf6F3d/UNvXVGKrEzL/Dluc5p+y9GkzlTWig==",
+ "version": "0.0.9",
+ "resolved": "https://registry.npmjs.org/@types/doctrine/-/doctrine-0.0.9.tgz",
+ "integrity": "sha512-eOIHzCUSH7SMfonMG1LsC2f8vxBFtho6NGBznK41R84YzPuvSBzrhEps33IsQiOW9+VL6NQ9DbjQJznk/S4uRA==",
"dev": true
},
"node_modules/@types/eslint": {
"version": "8.56.9",
"resolved": "https://registry.npmjs.org/@types/eslint/-/eslint-8.56.9.tgz",
"integrity": "sha512-W4W3KcqzjJ0sHg2vAq9vfml6OhsJ53TcUjUqfzzZf/EChUtwspszj/S0pzMxnfRcO55/iGq47dscXw71Fxc4Zg==",
- "dev": true,
"dependencies": {
"@types/estree": "*",
"@types/json-schema": "*"
}
},
+ "node_modules/@types/eslint-scope": {
+ "version": "3.7.7",
+ "resolved": "https://registry.npmjs.org/@types/eslint-scope/-/eslint-scope-3.7.7.tgz",
+ "integrity": "sha512-MzMFlSLBqNF2gcHWO0G1vP/YQyfvrxZ0bF+u7mzUdZ1/xK4A4sru+nraZz5i3iEIk1l1uyicaDVTB4QbbEkAYg==",
+ "license": "MIT",
+ "dependencies": {
+ "@types/eslint": "*",
+ "@types/estree": "*"
+ }
+ },
"node_modules/@types/estree": {
"version": "1.0.5",
"resolved": "https://registry.npmjs.org/@types/estree/-/estree-1.0.5.tgz",
@@ -14751,17 +13383,12 @@
"integrity": "sha512-g39Vp8ZJ3D0gXhhkhDidVvdy4QajkF7/PV6HGn23FMaMqE/tLC1JNHUeQ7SshKLsBjucakZsXBLkWULbGLdL5g==",
"dev": true
},
- "node_modules/@types/find-cache-dir": {
- "version": "3.2.1",
- "resolved": "https://registry.npmjs.org/@types/find-cache-dir/-/find-cache-dir-3.2.1.tgz",
- "integrity": "sha512-frsJrz2t/CeGifcu/6uRo4b+SzAwT4NYCVPu1GN8IB9XTzrpPkGuV0tmh9mN+/L0PklAlsC3u5Fxt0ju00LXIw==",
- "dev": true
- },
"node_modules/@types/find-root": {
"version": "1.1.2",
"resolved": "https://registry.npmjs.org/@types/find-root/-/find-root-1.1.2.tgz",
"integrity": "sha512-lGuMq71TL466jtCpvh7orGd+mrdBmo2h8ozvtOOTbq3ByfWpuN+UVxv4sOv3YpsD4NhW2k6ESGhnT/FIg4Ouzw==",
- "dev": true
+ "dev": true,
+ "license": "MIT"
},
"node_modules/@types/glob": {
"version": "7.1.1",
@@ -14861,6 +13488,7 @@
"resolved": "https://registry.npmjs.org/@types/jsftp/-/jsftp-2.1.3.tgz",
"integrity": "sha512-R5rP70tkPnH+rhz0s6VuMKqMrkA7ET1AfU3FDGKNhYBgU/XTIbzsYNCgTByo60eitl0mI5OoEA9+MW/t/5iVzA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"@types/node": "*"
}
@@ -14888,6 +13516,7 @@
"resolved": "https://registry.npmjs.org/@types/klaw/-/klaw-3.0.4.tgz",
"integrity": "sha512-0M5F/WMU9yu2MyRued1VTQvUSwZ3siqYsX6MU7JF7VXRF5RzL0FXWFUrmdrWuGDWmuN6W+SyLhhg1Wp/sXkjtg==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"@types/node": "*"
}
@@ -14896,18 +13525,20 @@
"version": "1.0.2",
"resolved": "https://registry.npmjs.org/@types/lockfile/-/lockfile-1.0.2.tgz",
"integrity": "sha512-jD5VbvhfMhaYN4M3qPJuhMVUg3Dfc4tvPvLEAXn6GXbs/ajDFtCQahX37GIE65ipTI3I+hEvNaXS3MYAn9Ce3Q==",
- "dev": true
+ "dev": true,
+ "license": "MIT"
},
"node_modules/@types/lodash": {
"version": "4.14.199",
"resolved": "https://registry.npmjs.org/@types/lodash/-/lodash-4.14.199.tgz",
"integrity": "sha512-Vrjz5N5Ia4SEzWWgIVwnHNEnb1UE1XMkvY5DGXrAeOGE9imk0hgTHh5GyDjLDJi9OTCn9oo9dXH1uToK1VRfrg==",
- "dev": true
+ "dev": true,
+ "license": "MIT"
},
"node_modules/@types/mdx": {
- "version": "2.0.6",
- "resolved": "https://registry.npmjs.org/@types/mdx/-/mdx-2.0.6.tgz",
- "integrity": "sha512-sVcwEG10aFU2KcM7cIA0M410UPv/DesOPyG8zMVk0QUDexHA3lYmGucpEpZ2dtWWhi2ip3CG+5g/iH0PwoW4Fw==",
+ "version": "2.0.13",
+ "resolved": "https://registry.npmjs.org/@types/mdx/-/mdx-2.0.13.tgz",
+ "integrity": "sha512-+OWZQfAYyio6YkJb3HLxDrvnx6SWWDbC0zVPfBRzUk0/nqoDyf6dNxQi3eArPe8rJ473nobTMQ/8Zk+LxJ+Yuw==",
"dev": true
},
"node_modules/@types/method-override": {
@@ -14915,6 +13546,7 @@
"resolved": "https://registry.npmjs.org/@types/method-override/-/method-override-0.0.33.tgz",
"integrity": "sha512-H6hK7AZdUOCmboTTUlhfDG3uT0XDljjrk3vIb+GJ3ylkogXu5s/NncGB85r3rtCz6sxZBWF62dlf7I04sIQA5A==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"@types/express": "*"
}
@@ -14922,12 +13554,7 @@
"node_modules/@types/mime": {
"version": "2.0.3",
"resolved": "https://registry.npmjs.org/@types/mime/-/mime-2.0.3.tgz",
- "integrity": "sha512-Jus9s4CDbqwocc5pOAnh8ShfrnMcPHuJYzVcSUU7lrh8Ni5HuIqX3oilL86p3dlTrk0LzHRCgA/GQ7uNCw6l2Q=="
- },
- "node_modules/@types/mime-types": {
- "version": "2.1.4",
- "resolved": "https://registry.npmjs.org/@types/mime-types/-/mime-types-2.1.4.tgz",
- "integrity": "sha512-lfU4b34HOri+kAY5UheuFMWPDOI+OPceBSHZKp69gEyTL/mmJ4cnU6Y/rlme3UL3GyOn6Y42hyIEw0/q8sWx5w==",
+ "integrity": "sha512-Jus9s4CDbqwocc5pOAnh8ShfrnMcPHuJYzVcSUU7lrh8Ni5HuIqX3oilL86p3dlTrk0LzHRCgA/GQ7uNCw6l2Q==",
"dev": true
},
"node_modules/@types/minimatch": {
@@ -14949,34 +13576,34 @@
"version": "2.1.2",
"resolved": "https://registry.npmjs.org/@types/mv/-/mv-2.1.2.tgz",
"integrity": "sha512-IvAjPuiQ2exDicnTrMidt1m+tj3gZ60BM0PaoRsU0m9Cn+lrOyemuO9Tf8CvHFmXlxMjr1TVCfadi9sfwbSuKg==",
- "dev": true
+ "dev": true,
+ "license": "MIT"
},
"node_modules/@types/ncp": {
"version": "2.0.6",
"resolved": "https://registry.npmjs.org/@types/ncp/-/ncp-2.0.6.tgz",
"integrity": "sha512-A5CcYVkrcbRD+5ghRzn+LjEYUNT+YpjcPfnpYte6fBNfq3rXJRYA03cATV0XfVbSnOZa2Yp5sXYI1fIkjAF/UA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"@types/node": "*"
}
},
"node_modules/@types/node": {
- "version": "18.19.59",
- "resolved": "https://registry.npmjs.org/@types/node/-/node-18.19.59.tgz",
- "integrity": "sha512-vizm2EqwV/7Zay+A6J3tGl9Lhr7CjZe2HmWS988sefiEmsyP9CeXEleho6i4hJk/8UtZAo0bWN4QPZZr83RxvQ==",
- "license": "MIT",
+ "version": "22.10.2",
+ "resolved": "https://registry.npmjs.org/@types/node/-/node-22.10.2.tgz",
+ "integrity": "sha512-Xxr6BBRCAOQixvonOye19wnzyDiUtTeqldOOmj3CkeblonbccA12PFwlufvRdrpjXxqnmUaeiU5EOA+7s5diUQ==",
"dependencies": {
- "undici-types": "~5.26.4"
+ "undici-types": "~6.20.0"
}
},
- "node_modules/@types/node-fetch": {
- "version": "2.6.11",
- "resolved": "https://registry.npmjs.org/@types/node-fetch/-/node-fetch-2.6.11.tgz",
- "integrity": "sha512-24xFj9R5+rfQJLRyM56qh+wnVSYhyXC2tkoBndtY0U+vubqNsYXGjufB2nn8Q6gt0LrARwL6UBtMCSVCwl4B1g==",
- "dev": true,
+ "node_modules/@types/node-forge": {
+ "version": "1.3.11",
+ "resolved": "https://registry.npmjs.org/@types/node-forge/-/node-forge-1.3.11.tgz",
+ "integrity": "sha512-FQx220y22OKNTqaByeBGqHWYz4cl94tpcxeFdvBo3wjG6XPBuZ0BNgNZRV5J5TFmmcsJ4IzsLkmGRiQbnYsBEQ==",
+ "license": "MIT",
"dependencies": {
- "@types/node": "*",
- "form-data": "^4.0.0"
+ "@types/node": "*"
}
},
"node_modules/@types/normalize-package-data": {
@@ -15010,7 +13637,8 @@
"version": "0.0.31",
"resolved": "https://registry.npmjs.org/@types/pluralize/-/pluralize-0.0.31.tgz",
"integrity": "sha512-MQh69PPwFlYAL2qz/Mw5Zc34VTdt7pTck0Xbb6pbPSzdt5oaLB87iyJJxEMS5Dco/s7lXHunEezAvQurZZdrsQ==",
- "dev": true
+ "dev": true,
+ "license": "MIT"
},
"node_modules/@types/prettier": {
"version": "2.4.4",
@@ -15018,12 +13646,6 @@
"integrity": "sha512-ReVR2rLTV1kvtlWFyuot+d1pkpG2Fw/XKE3PDAdj57rbM97ttSp9JZ2UsP+2EHTylra9cUf6JA7tGwW1INzUrA==",
"dev": true
},
- "node_modules/@types/pretty-hrtime": {
- "version": "1.0.3",
- "resolved": "https://registry.npmjs.org/@types/pretty-hrtime/-/pretty-hrtime-1.0.3.tgz",
- "integrity": "sha512-nj39q0wAIdhwn7DGUyT9irmsKK1tV0bd5WFEhgpqNTMFZ8cE+jieuTphCW0tfdm47S2zVT5mr09B28b1chmQMA==",
- "dev": true
- },
"node_modules/@types/prop-types": {
"version": "15.7.4",
"resolved": "https://registry.npmjs.org/@types/prop-types/-/prop-types-15.7.4.tgz",
@@ -15077,10 +13699,11 @@
}
},
"node_modules/@types/readdir-glob": {
- "version": "1.1.2",
- "resolved": "https://registry.npmjs.org/@types/readdir-glob/-/readdir-glob-1.1.2.tgz",
- "integrity": "sha512-vwAYrNN/8yhp/FJRU6HUSD0yk6xfoOS8HrZa8ZL7j+X8hJpaC1hTcAiXX2IxaAkkvrz9mLyoEhYZTE3cEYvA9Q==",
+ "version": "1.1.5",
+ "resolved": "https://registry.npmjs.org/@types/readdir-glob/-/readdir-glob-1.1.5.tgz",
+ "integrity": "sha512-raiuEPUYqXu+nvtY2Pe8s8FEmZ3x5yAH4VkLdihcPdalvsHltomrRC9BzuStrJ9yk06470hS0Crw0f1pXqD+Hg==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"@types/node": "*"
}
@@ -15106,9 +13729,10 @@
}
},
"node_modules/@types/retry": {
- "version": "0.12.1",
- "resolved": "https://registry.npmjs.org/@types/retry/-/retry-0.12.1.tgz",
- "integrity": "sha512-xoDlM2S4ortawSWORYqsdU+2rxdh4LRW9ytc3zmT37RIKQh6IHyKwwtKhKis9ah8ol07DCkZxPt8BBvPjC6v4g=="
+ "version": "0.12.0",
+ "resolved": "https://registry.npmjs.org/@types/retry/-/retry-0.12.0.tgz",
+ "integrity": "sha512-wWKOClTTiizcZhXnPY4wikVAwmdYHp8q6DmC+EJUzAMsycb7HB32Kh9RN4+0gExjmPmZSAQjgURXIGATPegAvA==",
+ "license": "MIT"
},
"node_modules/@types/scheduler": {
"version": "0.16.2",
@@ -15140,33 +13764,37 @@
"resolved": "https://registry.npmjs.org/@types/serve-favicon/-/serve-favicon-2.5.5.tgz",
"integrity": "sha512-E/P1MhsGcalASnOVUPr9QQ4BIXyqQoGtLscG4fcMcEpZ7Z7tl6S4uSJnBJzWj7bj6rRZLIFOv0dR1YcepLNFFA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"@types/express": "*"
}
},
"node_modules/@types/serve-index": {
- "version": "1.9.1",
- "resolved": "https://registry.npmjs.org/@types/serve-index/-/serve-index-1.9.1.tgz",
- "integrity": "sha512-d/Hs3nWDxNL2xAczmOVZNj92YZCS6RGxfBPjKzuu/XirCgXdpKEb88dYNbrYGint6IVWLNP+yonwVAuRC0T2Dg==",
+ "version": "1.9.4",
+ "resolved": "https://registry.npmjs.org/@types/serve-index/-/serve-index-1.9.4.tgz",
+ "integrity": "sha512-qLpGZ/c2fhSs5gnYsQxtDEq3Oy8SXPClIXkW5ghvAvsNuVSA8k+gCONcUCS/UjLEYvYps+e8uBtfgXgvhwfNug==",
+ "license": "MIT",
"dependencies": {
"@types/express": "*"
}
},
"node_modules/@types/serve-static": {
- "version": "1.15.2",
- "resolved": "https://registry.npmjs.org/@types/serve-static/-/serve-static-1.15.2.tgz",
- "integrity": "sha512-J2LqtvFYCzaj8pVYKw8klQXrLLk7TBZmQ4ShlcdkELFKGwGMfevMLneMMRkMgZxotOD9wg497LpC7O8PcvAmfw==",
+ "version": "1.15.7",
+ "resolved": "https://registry.npmjs.org/@types/serve-static/-/serve-static-1.15.7.tgz",
+ "integrity": "sha512-W8Ym+h8nhuRwaKPaDw34QUkwsGi6Rc4yYqvKFo5rm2FUEhCFbzVWrxXUxuKK8TASjWsysJY0nsmNCGhCOIsrOw==",
+ "license": "MIT",
"dependencies": {
"@types/http-errors": "*",
- "@types/mime": "*",
- "@types/node": "*"
+ "@types/node": "*",
+ "@types/send": "*"
}
},
"node_modules/@types/shell-quote": {
"version": "1.7.2",
"resolved": "https://registry.npmjs.org/@types/shell-quote/-/shell-quote-1.7.2.tgz",
"integrity": "sha512-p3SZxGp6LXB6RPdMpJmquKjaxQlN/ijyBLTKGPg9IJK6J2g2sJsMmtXP9kNR+Axxi6MDKN2e/76HCOUmvkIpcw==",
- "dev": true
+ "dev": true,
+ "license": "MIT"
},
"node_modules/@types/simple-peer": {
"version": "9.11.6",
@@ -15177,9 +13805,10 @@
}
},
"node_modules/@types/sockjs": {
- "version": "0.3.33",
- "resolved": "https://registry.npmjs.org/@types/sockjs/-/sockjs-0.3.33.tgz",
- "integrity": "sha512-f0KEEe05NvUnat+boPTZ0dgaLZ4SfSouXUgv5noUiefG2ajgKjmETo9ZJyuqsl7dfl2aHlLJUiki6B4ZYldiiw==",
+ "version": "0.3.36",
+ "resolved": "https://registry.npmjs.org/@types/sockjs/-/sockjs-0.3.36.tgz",
+ "integrity": "sha512-MK9V6NzAS1+Ud7JV9lJLFqW85VbC9dq3LmwZCuBe4wBDgKC0Kj/jd8Xl+nSviU+Qc3+m7umHHyHg//2KSa0a0Q==",
+ "license": "MIT",
"dependencies": {
"@types/node": "*"
}
@@ -15204,7 +13833,8 @@
"version": "8.1.1",
"resolved": "https://registry.npmjs.org/@types/supports-color/-/supports-color-8.1.1.tgz",
"integrity": "sha512-dPWnWsf+kzIG140B8z2w3fr5D03TLWbOAFQl45xUpI3vcizeXriNR5VYkWZ+WTMsUHqZ9Xlt3hrxGNANFyNQfw==",
- "dev": true
+ "dev": true,
+ "license": "MIT"
},
"node_modules/@types/tapable": {
"version": "1.0.5",
@@ -15305,7 +13935,8 @@
"version": "3.0.0",
"resolved": "https://registry.npmjs.org/@types/which/-/which-3.0.0.tgz",
"integrity": "sha512-ASCxdbsrwNfSMXALlC3Decif9rwDMu+80KGp5zI2RLRotfMsTv7fHL8W8VDp24wymzDyIFudhUeSCugrgRFfHQ==",
- "dev": true
+ "dev": true,
+ "license": "MIT"
},
"node_modules/@types/wrap-ansi": {
"version": "3.0.0",
@@ -15871,11 +14502,66 @@
"react": ">= 16.8.0"
}
},
+ "node_modules/@vitest/expect": {
+ "version": "2.0.5",
+ "resolved": "https://registry.npmjs.org/@vitest/expect/-/expect-2.0.5.tgz",
+ "integrity": "sha512-yHZtwuP7JZivj65Gxoi8upUN2OzHTi3zVfjwdpu2WrvCZPLwsJ2Ey5ILIPccoW23dd/zQBlJ4/dhi7DWNyXCpA==",
+ "dev": true,
+ "dependencies": {
+ "@vitest/spy": "2.0.5",
+ "@vitest/utils": "2.0.5",
+ "chai": "^5.1.1",
+ "tinyrainbow": "^1.2.0"
+ },
+ "funding": {
+ "url": "https://opencollective.com/vitest"
+ }
+ },
+ "node_modules/@vitest/pretty-format": {
+ "version": "2.0.5",
+ "resolved": "https://registry.npmjs.org/@vitest/pretty-format/-/pretty-format-2.0.5.tgz",
+ "integrity": "sha512-h8k+1oWHfwTkyTkb9egzwNMfJAEx4veaPSnMeKbVSjp4euqGSbQlm5+6VHwTr7u4FJslVVsUG5nopCaAYdOmSQ==",
+ "dev": true,
+ "dependencies": {
+ "tinyrainbow": "^1.2.0"
+ },
+ "funding": {
+ "url": "https://opencollective.com/vitest"
+ }
+ },
+ "node_modules/@vitest/spy": {
+ "version": "2.0.5",
+ "resolved": "https://registry.npmjs.org/@vitest/spy/-/spy-2.0.5.tgz",
+ "integrity": "sha512-c/jdthAhvJdpfVuaexSrnawxZz6pywlTPe84LUB2m/4t3rl2fTo9NFGBG4oWgaD+FTgDDV8hJ/nibT7IfH3JfA==",
+ "dev": true,
+ "dependencies": {
+ "tinyspy": "^3.0.0"
+ },
+ "funding": {
+ "url": "https://opencollective.com/vitest"
+ }
+ },
+ "node_modules/@vitest/utils": {
+ "version": "2.0.5",
+ "resolved": "https://registry.npmjs.org/@vitest/utils/-/utils-2.0.5.tgz",
+ "integrity": "sha512-d8HKbqIcya+GR67mkZbrzhS5kKhtp8dQLcmRZLGTscGVg7yImT82cIrhtn2L8+VujWcy6KZweApgNmPsTAO/UQ==",
+ "dev": true,
+ "dependencies": {
+ "@vitest/pretty-format": "2.0.5",
+ "estree-walker": "^3.0.3",
+ "loupe": "^3.1.1",
+ "tinyrainbow": "^1.2.0"
+ },
+ "funding": {
+ "url": "https://opencollective.com/vitest"
+ }
+ },
"node_modules/@wdio/config": {
"version": "8.16.20",
"resolved": "https://registry.npmjs.org/@wdio/config/-/config-8.16.20.tgz",
"integrity": "sha512-JFD7aYs3nGF2kNhc0eV03mWFQMJku42NCBl+aedb1jzP3z6tBWV3n1a0ETS4MTLps8lFXBDZWvWEnl+ZvVrHZw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"@wdio/logger": "8.16.17",
"@wdio/types": "8.16.12",
@@ -15895,6 +14581,7 @@
"resolved": "https://registry.npmjs.org/brace-expansion/-/brace-expansion-2.0.1.tgz",
"integrity": "sha512-XnAIvQ8eM+kC6aULx6wuQiwVsnzsi9d3WxzV3FpWTGA19F621kwdbsAcFKXgKUHZWsy+mY6iL1sHTxWEFCytDA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"balanced-match": "^1.0.0"
}
@@ -15904,6 +14591,7 @@
"resolved": "https://registry.npmjs.org/decamelize/-/decamelize-6.0.0.tgz",
"integrity": "sha512-Fv96DCsdOgB6mdGl67MT5JaTNKRzrzill5OH5s8bjYJXVlcXyPYGyPsUkWyGV5p1TXI5esYIYMMeDJL0hEIwaA==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": "^12.20.0 || ^14.13.1 || >=16.0.0"
},
@@ -15916,6 +14604,7 @@
"resolved": "https://registry.npmjs.org/find-up/-/find-up-6.3.0.tgz",
"integrity": "sha512-v2ZsoEuVHYy8ZIlYqwPe/39Cy+cFDzp4dXPaxNvkEuouymu+2Jbz0PxpKarJHYJTmv2HWT3O382qY8l4jMWthw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"locate-path": "^7.1.0",
"path-exists": "^5.0.0"
@@ -15953,6 +14642,7 @@
"resolved": "https://registry.npmjs.org/hosted-git-info/-/hosted-git-info-7.0.2.tgz",
"integrity": "sha512-puUZAUKT5m8Zzvs72XWy3HtvVbTWljRE66cP60bxJzAqf2DgICo7lYTY2IHUmLnNpjYvw5bvmoHvPc0QO2a62w==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"lru-cache": "^10.0.1"
},
@@ -15960,27 +14650,12 @@
"node": "^16.14.0 || >=18.0.0"
}
},
- "node_modules/@wdio/config/node_modules/jackspeak": {
- "version": "3.4.3",
- "resolved": "https://registry.npmjs.org/jackspeak/-/jackspeak-3.4.3.tgz",
- "integrity": "sha512-OGlZQpz2yfahA/Rd1Y8Cd9SIEsqvXkLVoSw/cgwhnhFMDbsQFeZYoJJ7bIZBS9BcamUW96asq/npPWugM+RQBw==",
- "dev": true,
- "license": "BlueOak-1.0.0",
- "dependencies": {
- "@isaacs/cliui": "^8.0.2"
- },
- "funding": {
- "url": "https://github.com/sponsors/isaacs"
- },
- "optionalDependencies": {
- "@pkgjs/parseargs": "^0.11.0"
- }
- },
"node_modules/@wdio/config/node_modules/json-parse-even-better-errors": {
"version": "3.0.2",
"resolved": "https://registry.npmjs.org/json-parse-even-better-errors/-/json-parse-even-better-errors-3.0.2.tgz",
"integrity": "sha512-fi0NG4bPjCHunUJffmLd0gxssIgkNmArMvis4iNah6Owg1MCJjWhEcDLmsK6iGkJq3tHwbDkTlce70/tmXN4cQ==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
@@ -15990,6 +14665,7 @@
"resolved": "https://registry.npmjs.org/lines-and-columns/-/lines-and-columns-2.0.4.tgz",
"integrity": "sha512-wM1+Z03eypVAVUCE7QdSqpVIvelbOakn1M0bPDoA4SGWPx3sNDVUiMo3L6To6WWGClB7VyXnhQ4Sn7gxiJbE6A==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": "^12.20.0 || ^14.13.1 || >=16.0.0"
}
@@ -15999,6 +14675,7 @@
"resolved": "https://registry.npmjs.org/locate-path/-/locate-path-7.2.0.tgz",
"integrity": "sha512-gvVijfZvn7R+2qyPX8mAuKcFGDf6Nc61GdvGafQsHL0sBIxfKzA+usWn4GFC/bk+QdwPUD4kWFJLhElipq+0VA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"p-locate": "^6.0.0"
},
@@ -16009,13 +14686,6 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@wdio/config/node_modules/lru-cache": {
- "version": "10.4.3",
- "resolved": "https://registry.npmjs.org/lru-cache/-/lru-cache-10.4.3.tgz",
- "integrity": "sha512-JNAzZcXrCt42VGLuYz0zfAzDfAvJWW6AfYlDBQyDV5DClI2m5sAmK+OIO7s59XfsRsWHp02jAJrRadPRGTt6SQ==",
- "dev": true,
- "license": "ISC"
- },
"node_modules/@wdio/config/node_modules/minimatch": {
"version": "9.0.5",
"resolved": "https://registry.npmjs.org/minimatch/-/minimatch-9.0.5.tgz",
@@ -16062,6 +14732,7 @@
"resolved": "https://registry.npmjs.org/p-limit/-/p-limit-4.0.0.tgz",
"integrity": "sha512-5b0R4txpzjPWVw/cXXUResoD4hb6U/x9BH08L7nw+GN1sezDzPdxeRvpc9c433fZhBan/wusjbCsqwqm4EIBIQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"yocto-queue": "^1.0.0"
},
@@ -16077,6 +14748,7 @@
"resolved": "https://registry.npmjs.org/p-locate/-/p-locate-6.0.0.tgz",
"integrity": "sha512-wPrq66Llhl7/4AGC6I+cqxT07LhXvWL08LNXz1fENOw0Ap4sRZZ/gZpTTJ5jpurzzzfS2W/Ge9BY3LgLjCShcw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"p-limit": "^4.0.0"
},
@@ -16092,6 +14764,7 @@
"resolved": "https://registry.npmjs.org/parse-json/-/parse-json-7.1.1.tgz",
"integrity": "sha512-SgOTCX/EZXtZxBE5eJ97P4yGM5n37BwRU+YMsH4vNzFqJV/oWFXXCmwFlgWUM4PrakybVOueJJ6pwHqSVhTFDw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"@babel/code-frame": "^7.21.4",
"error-ex": "^1.3.2",
@@ -16111,6 +14784,7 @@
"resolved": "https://registry.npmjs.org/type-fest/-/type-fest-3.13.1.tgz",
"integrity": "sha512-tLq3bSNx+xSpwvAJnzrK0Ep5CLNWjvFTOp71URMaAEWBfRb9nnJiBoUe0tF8bI4ZFO3omgBR6NvnbzVUT3Ly4g==",
"dev": true,
+ "license": "(MIT OR CC0-1.0)",
"engines": {
"node": ">=14.16"
},
@@ -16123,6 +14797,7 @@
"resolved": "https://registry.npmjs.org/path-exists/-/path-exists-5.0.0.tgz",
"integrity": "sha512-RjhtfwJOxzcFmNOi6ltcbcu4Iu+FL3zEj83dk4kAS+fVpTxXLO1b38RvJgT/0QwvV/L3aY9TAnyv0EOqW4GoMQ==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": "^12.20.0 || ^14.13.1 || >=16.0.0"
}
@@ -16132,6 +14807,7 @@
"resolved": "https://registry.npmjs.org/read-pkg/-/read-pkg-8.1.0.tgz",
"integrity": "sha512-PORM8AgzXeskHO/WEv312k9U03B8K9JSiWF/8N9sUuFjBa+9SF2u6K7VClzXwDXab51jCd8Nd36CNM+zR97ScQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"@types/normalize-package-data": "^2.4.1",
"normalize-package-data": "^6.0.0",
@@ -16150,6 +14826,7 @@
"resolved": "https://registry.npmjs.org/read-pkg-up/-/read-pkg-up-10.1.0.tgz",
"integrity": "sha512-aNtBq4jR8NawpKJQldrQcSW9y/d+KWH4v24HWkHljOZ7H0av+YTGANBzRh9A5pw7v/bLVsLVPpOhJ7gHNVy8lA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"find-up": "^6.3.0",
"read-pkg": "^8.1.0",
@@ -16163,9 +14840,9 @@
}
},
"node_modules/@wdio/config/node_modules/type-fest": {
- "version": "4.26.1",
- "resolved": "https://registry.npmjs.org/type-fest/-/type-fest-4.26.1.tgz",
- "integrity": "sha512-yOGpmOAL7CkKe/91I5O3gPICmJNLJ1G4zFYVAsRHg7M64biSnPtRj0WNQt++bRkjYOqjWXrhnUw1utzmVErAdg==",
+ "version": "4.30.0",
+ "resolved": "https://registry.npmjs.org/type-fest/-/type-fest-4.30.0.tgz",
+ "integrity": "sha512-G6zXWS1dLj6eagy6sVhOMQiLtJdxQBHIA9Z6HFUNLOlr6MFOgzV8wvmidtPONfPtEUv0uZsy77XJNzTAfwPDaA==",
"dev": true,
"license": "(MIT OR CC0-1.0)",
"engines": {
@@ -16193,6 +14870,7 @@
"resolved": "https://registry.npmjs.org/@wdio/logger/-/logger-8.16.17.tgz",
"integrity": "sha512-zeQ41z3T+b4IsrriZZipayXxLNDuGsm7TdExaviNGojPVrIsQUCSd/FvlLHM32b7ZrMyInHenu/zx1cjAZO71g==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"chalk": "^5.1.2",
"loglevel": "^1.6.0",
@@ -16221,6 +14899,7 @@
"resolved": "https://registry.npmjs.org/chalk/-/chalk-5.3.0.tgz",
"integrity": "sha512-dLitG79d+GV1Nb/VYcCDFivJeK1hiukt9QjRNVOsUtTy1rR1YJsmpGGTZ3qJos+uw7WmWF4wUwBd9jxjocFC2w==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": "^12.17.0 || ^14.13 || >=16.0.0"
},
@@ -16233,6 +14912,7 @@
"resolved": "https://registry.npmjs.org/strip-ansi/-/strip-ansi-7.1.0.tgz",
"integrity": "sha512-iq6eVVI64nQQTRYq2KtEg2d2uU7LElhTJwsH4YzIHZshxlgZms/wIc4VoDQTlG/IvVIrBKG06CrZnp0qv7hkcQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"ansi-regex": "^6.0.1"
},
@@ -16247,13 +14927,15 @@
"version": "8.16.5",
"resolved": "https://registry.npmjs.org/@wdio/protocols/-/protocols-8.16.5.tgz",
"integrity": "sha512-u9I57hIqmcOgrDH327ZCc2GTXv2YFN5bg6UaA3OUoJU7eJgGYHFB6RrjiNjLXer68iIx07wwVM70V/1xzijd3Q==",
- "dev": true
+ "dev": true,
+ "license": "MIT"
},
"node_modules/@wdio/repl": {
"version": "8.10.1",
"resolved": "https://registry.npmjs.org/@wdio/repl/-/repl-8.10.1.tgz",
"integrity": "sha512-VZ1WFHTNKjR8Ga97TtV2SZM6fvRjWbYI2i/f4pJB4PtusorKvONAMJf2LQcUBIyzbVobqr7KSrcjmSwRolI+yw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"@types/node": "^20.1.0"
},
@@ -16262,9 +14944,9 @@
}
},
"node_modules/@wdio/repl/node_modules/@types/node": {
- "version": "20.16.15",
- "resolved": "https://registry.npmjs.org/@types/node/-/node-20.16.15.tgz",
- "integrity": "sha512-DV58qQz9dBMqVVn+qnKwGa51QzCD4YM/tQM16qLKxdf5tqz5W4QwtrMzjSTbabN1cFTSuyxVYBy+QWHjWW8X/g==",
+ "version": "20.17.9",
+ "resolved": "https://registry.npmjs.org/@types/node/-/node-20.17.9.tgz",
+ "integrity": "sha512-0JOXkRyLanfGPE2QRCwgxhzlBAvaRdCNMcvbd7jFfpmD4eEXll7LRwy5ymJmyeZqk7Nh7eD2LeUyQ68BbndmXw==",
"dev": true,
"license": "MIT",
"dependencies": {
@@ -16283,6 +14965,7 @@
"resolved": "https://registry.npmjs.org/@wdio/types/-/types-8.16.12.tgz",
"integrity": "sha512-TjCZJ3P9ual21G0dRv0lC9QgHGd3Igv+guEINevBKf/oD4/N84PvQ2eZG1nSbZ3xh8X/dvi+O64A6VEv43gx2w==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"@types/node": "^20.1.0"
},
@@ -16291,9 +14974,9 @@
}
},
"node_modules/@wdio/types/node_modules/@types/node": {
- "version": "20.16.15",
- "resolved": "https://registry.npmjs.org/@types/node/-/node-20.16.15.tgz",
- "integrity": "sha512-DV58qQz9dBMqVVn+qnKwGa51QzCD4YM/tQM16qLKxdf5tqz5W4QwtrMzjSTbabN1cFTSuyxVYBy+QWHjWW8X/g==",
+ "version": "20.17.9",
+ "resolved": "https://registry.npmjs.org/@types/node/-/node-20.17.9.tgz",
+ "integrity": "sha512-0JOXkRyLanfGPE2QRCwgxhzlBAvaRdCNMcvbd7jFfpmD4eEXll7LRwy5ymJmyeZqk7Nh7eD2LeUyQ68BbndmXw==",
"dev": true,
"license": "MIT",
"dependencies": {
@@ -16312,6 +14995,7 @@
"resolved": "https://registry.npmjs.org/@wdio/utils/-/utils-8.16.17.tgz",
"integrity": "sha512-jDyOrxbQRDJO0OPt9UBgnwpUIKqtRn4+R0gR5VSDrIG/in5ZZg28yer8urrIVY4yY9ut5r/22VaMHZI9LEXF5w==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"@puppeteer/browsers": "^1.6.0",
"@wdio/logger": "8.16.17",
@@ -16332,32 +15016,12 @@
"node": "^16.13 || >=18"
}
},
- "node_modules/@wdio/utils/node_modules/@puppeteer/browsers": {
- "version": "1.9.1",
- "resolved": "https://registry.npmjs.org/@puppeteer/browsers/-/browsers-1.9.1.tgz",
- "integrity": "sha512-PuvK6xZzGhKPvlx3fpfdM2kYY3P/hB1URtK8wA7XUJ6prn6pp22zvJHu48th0SGcHL9SutbPHrFuQgfXTFobWA==",
- "dev": true,
- "dependencies": {
- "debug": "4.3.4",
- "extract-zip": "2.0.1",
- "progress": "2.0.3",
- "proxy-agent": "6.3.1",
- "tar-fs": "3.0.4",
- "unbzip2-stream": "1.4.3",
- "yargs": "17.7.2"
- },
- "bin": {
- "browsers": "lib/cjs/main-cli.js"
- },
- "engines": {
- "node": ">=16.3.0"
- }
- },
"node_modules/@wdio/utils/node_modules/@sindresorhus/is": {
"version": "5.6.0",
"resolved": "https://registry.npmjs.org/@sindresorhus/is/-/is-5.6.0.tgz",
"integrity": "sha512-TV7t8GKYaJWsn00tFDqBw8+Uqmr8A0fRU1tvTQhyZzGv0sJCGRQL3JGMI3ucuKo3XIZdUP+Lx7/gh2t3lewy7g==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=14.16"
},
@@ -16370,6 +15034,7 @@
"resolved": "https://registry.npmjs.org/@szmarczak/http-timer/-/http-timer-5.0.1.tgz",
"integrity": "sha512-+PmQX0PiAYPMeVYe237LJAYvOMYW1j2rH5YROyS3b4CTVJum34HfRvKvAzozHAQG0TnHNdUfY9nCeUyRAs//cw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"defer-to-connect": "^2.0.1"
},
@@ -16377,23 +15042,12 @@
"node": ">=14.16"
}
},
- "node_modules/@wdio/utils/node_modules/agent-base": {
- "version": "7.1.1",
- "resolved": "https://registry.npmjs.org/agent-base/-/agent-base-7.1.1.tgz",
- "integrity": "sha512-H0TSyFNDMomMNJQBn8wFV5YC/2eJ+VXECwOadZJT554xP6cODZHPX3H9QMQECxvrgiSOP1pHjy1sMWQVYJOUOA==",
- "dev": true,
- "dependencies": {
- "debug": "^4.3.4"
- },
- "engines": {
- "node": ">= 14"
- }
- },
"node_modules/@wdio/utils/node_modules/cacheable-lookup": {
"version": "7.0.0",
"resolved": "https://registry.npmjs.org/cacheable-lookup/-/cacheable-lookup-7.0.0.tgz",
"integrity": "sha512-+qJyx4xiKra8mZrcwhjMRMUhD5NR1R8esPkzIYxX96JiecFoxAXFuz/GpR3+ev4PE1WamHip78wV0vcmPQtp8w==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=14.16"
}
@@ -16403,6 +15057,7 @@
"resolved": "https://registry.npmjs.org/cacheable-request/-/cacheable-request-10.2.14.tgz",
"integrity": "sha512-zkDT5WAF4hSSoUgyfg5tFIxz8XQK+25W/TLVojJTMKBaxevLBBtLxgqguAuVQB8PVW79FVjHcU+GJ9tVbDZ9mQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"@types/http-cache-semantics": "^4.0.2",
"get-stream": "^6.0.1",
@@ -16416,37 +15071,12 @@
"node": ">=14.16"
}
},
- "node_modules/@wdio/utils/node_modules/cacheable-request/node_modules/get-stream": {
- "version": "6.0.1",
- "resolved": "https://registry.npmjs.org/get-stream/-/get-stream-6.0.1.tgz",
- "integrity": "sha512-ts6Wi+2j3jQjqi70w5AlN8DFnkSwC+MqmxEzdEALB2qXZYV3X/b1CTfgPLGJNMeAWxdPfU8FO1ms3NUfaHCPYg==",
- "dev": true,
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/@wdio/utils/node_modules/cliui": {
- "version": "8.0.1",
- "resolved": "https://registry.npmjs.org/cliui/-/cliui-8.0.1.tgz",
- "integrity": "sha512-BSeNnyus75C4//NQ9gQt1/csTXyo/8Sb+afLAkzAptFuMsod9HFokGNudZpi/oQV73hnVK+sR+5PVRMd+Dr7YQ==",
- "dev": true,
- "dependencies": {
- "string-width": "^4.2.0",
- "strip-ansi": "^6.0.1",
- "wrap-ansi": "^7.0.0"
- },
- "engines": {
- "node": ">=12"
- }
- },
"node_modules/@wdio/utils/node_modules/decamelize": {
"version": "6.0.0",
"resolved": "https://registry.npmjs.org/decamelize/-/decamelize-6.0.0.tgz",
"integrity": "sha512-Fv96DCsdOgB6mdGl67MT5JaTNKRzrzill5OH5s8bjYJXVlcXyPYGyPsUkWyGV5p1TXI5esYIYMMeDJL0hEIwaA==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": "^12.20.0 || ^14.13.1 || >=16.0.0"
},
@@ -16454,37 +15084,12 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@wdio/utils/node_modules/emoji-regex": {
- "version": "8.0.0",
- "resolved": "https://registry.npmjs.org/emoji-regex/-/emoji-regex-8.0.0.tgz",
- "integrity": "sha512-MSjYzcWNOA0ewAHpz0MxpYFvwg6yjy1NG3xteoqz644VCo/RPgnr1/GGt+ic3iJTzQ8Eu3TdM14SawnVUmGE6A==",
- "dev": true
- },
- "node_modules/@wdio/utils/node_modules/extract-zip": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/extract-zip/-/extract-zip-2.0.1.tgz",
- "integrity": "sha512-GDhU9ntwuKyGXdZBUgTIe+vXnWj0fppUEtMDL0+idd5Sta8TGpHssn/eusA9mrPr9qNDym6SxAYZjNvCn/9RBg==",
- "dev": true,
- "dependencies": {
- "debug": "^4.1.1",
- "get-stream": "^5.1.0",
- "yauzl": "^2.10.0"
- },
- "bin": {
- "extract-zip": "cli.js"
- },
- "engines": {
- "node": ">= 10.17.0"
- },
- "optionalDependencies": {
- "@types/yauzl": "^2.9.1"
- }
- },
"node_modules/@wdio/utils/node_modules/get-port": {
"version": "7.1.0",
"resolved": "https://registry.npmjs.org/get-port/-/get-port-7.1.0.tgz",
"integrity": "sha512-QB9NKEeDg3xxVwCCwJQ9+xycaz6pBB6iQ76wiWMl1927n0Kir6alPiP+yuiICLLU4jpMe08dXfpebuQppFA2zw==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=16"
},
@@ -16492,11 +15097,25 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
+ "node_modules/@wdio/utils/node_modules/get-stream": {
+ "version": "6.0.1",
+ "resolved": "https://registry.npmjs.org/get-stream/-/get-stream-6.0.1.tgz",
+ "integrity": "sha512-ts6Wi+2j3jQjqi70w5AlN8DFnkSwC+MqmxEzdEALB2qXZYV3X/b1CTfgPLGJNMeAWxdPfU8FO1ms3NUfaHCPYg==",
+ "dev": true,
+ "license": "MIT",
+ "engines": {
+ "node": ">=10"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
+ }
+ },
"node_modules/@wdio/utils/node_modules/got": {
"version": "13.0.0",
"resolved": "https://registry.npmjs.org/got/-/got-13.0.0.tgz",
"integrity": "sha512-XfBk1CxOOScDcMr9O1yKkNaQyy865NbYs+F7dr4H0LZMVgCj2Le59k6PqbNHoL5ToeaEQUYh6c6yMfVcc6SJxA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"@sindresorhus/is": "^5.2.0",
"@szmarczak/http-timer": "^5.0.1",
@@ -16517,36 +15136,12 @@
"url": "https://github.com/sindresorhus/got?sponsor=1"
}
},
- "node_modules/@wdio/utils/node_modules/got/node_modules/get-stream": {
- "version": "6.0.1",
- "resolved": "https://registry.npmjs.org/get-stream/-/get-stream-6.0.1.tgz",
- "integrity": "sha512-ts6Wi+2j3jQjqi70w5AlN8DFnkSwC+MqmxEzdEALB2qXZYV3X/b1CTfgPLGJNMeAWxdPfU8FO1ms3NUfaHCPYg==",
- "dev": true,
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/@wdio/utils/node_modules/http-proxy-agent": {
- "version": "7.0.2",
- "resolved": "https://registry.npmjs.org/http-proxy-agent/-/http-proxy-agent-7.0.2.tgz",
- "integrity": "sha512-T1gkAiYYDWYx3V5Bmyu7HcfcvL7mUrTWiM6yOfa3PIphViJ/gFPbvidQ+veqSOHci/PxBcDabeUNCzpOODJZig==",
- "dev": true,
- "dependencies": {
- "agent-base": "^7.1.0",
- "debug": "^4.3.4"
- },
- "engines": {
- "node": ">= 14"
- }
- },
"node_modules/@wdio/utils/node_modules/http2-wrapper": {
"version": "2.2.1",
"resolved": "https://registry.npmjs.org/http2-wrapper/-/http2-wrapper-2.2.1.tgz",
"integrity": "sha512-V5nVw1PAOgfI3Lmeaj2Exmeg7fenjhRUgz1lPSezy1CuhPYbgQtbQj4jZfEAEMlaL+vupsvhjqCyjzob0yxsmQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"quick-lru": "^5.1.1",
"resolve-alpn": "^1.2.0"
@@ -16555,34 +15150,12 @@
"node": ">=10.19.0"
}
},
- "node_modules/@wdio/utils/node_modules/https-proxy-agent": {
- "version": "7.0.5",
- "resolved": "https://registry.npmjs.org/https-proxy-agent/-/https-proxy-agent-7.0.5.tgz",
- "integrity": "sha512-1e4Wqeblerz+tMKPIq2EMGiiWW1dIjZOksyHWSUm1rmuvw/how9hBHZ38lAGj5ID4Ik6EdkOw7NmWPy6LAwalw==",
- "dev": true,
- "license": "MIT",
- "dependencies": {
- "agent-base": "^7.0.2",
- "debug": "4"
- },
- "engines": {
- "node": ">= 14"
- }
- },
- "node_modules/@wdio/utils/node_modules/is-fullwidth-code-point": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/is-fullwidth-code-point/-/is-fullwidth-code-point-3.0.0.tgz",
- "integrity": "sha512-zymm5+u+sCsSWyD9qNaejV3DFvhCKclKdizYaJUuHA83RLjb7nSuGnddCHGv0hk+KY7BMAlsWeK4Ueg6EV6XQg==",
- "dev": true,
- "engines": {
- "node": ">=8"
- }
- },
"node_modules/@wdio/utils/node_modules/lowercase-keys": {
"version": "3.0.0",
"resolved": "https://registry.npmjs.org/lowercase-keys/-/lowercase-keys-3.0.0.tgz",
"integrity": "sha512-ozCC6gdQ+glXOQsveKD0YsDy8DSQFjDTz4zyzEHNV5+JP5D62LmfDZ6o1cycFx9ouG940M5dE8C8CTewdj2YWQ==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": "^12.20.0 || ^14.13.1 || >=16.0.0"
},
@@ -16590,20 +15163,12 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@wdio/utils/node_modules/lru-cache": {
- "version": "7.18.3",
- "resolved": "https://registry.npmjs.org/lru-cache/-/lru-cache-7.18.3.tgz",
- "integrity": "sha512-jumlc0BIUrS3qJGgIkWZsyfAM7NCWiBcCDhnd+3NNM5KbBmLTgHVfWBcg6W+rLUsIpzpERPsvwUP7CckAQSOoA==",
- "dev": true,
- "engines": {
- "node": ">=12"
- }
- },
"node_modules/@wdio/utils/node_modules/mimic-response": {
"version": "4.0.0",
"resolved": "https://registry.npmjs.org/mimic-response/-/mimic-response-4.0.0.tgz",
"integrity": "sha512-e5ISH9xMYU0DzrT+jl8q2ze9D6eWBto+I8CNpe+VI+K2J/F/k3PdkdTdz4wvGVH4NTpo+NRYTVIuMQEMMcsLqg==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": "^12.20.0 || ^14.13.1 || >=16.0.0"
},
@@ -16616,6 +15181,7 @@
"resolved": "https://registry.npmjs.org/normalize-url/-/normalize-url-8.0.1.tgz",
"integrity": "sha512-IO9QvjUMWxPQQhs60oOu10CRkWCiZzSUkzbXGGV9pviYl1fXYcvkzQ5jV9z8Y6un8ARoVRl4EtC6v6jNqbaJ/w==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=14.16"
},
@@ -16628,45 +15194,17 @@
"resolved": "https://registry.npmjs.org/p-cancelable/-/p-cancelable-3.0.0.tgz",
"integrity": "sha512-mlVgR3PGuzlo0MmTdk4cXqXWlwQDLnONTAg6sm62XkMJEiRxN3GL3SffkYvqwonbkJBcrI7Uvv5Zh9yjvn2iUw==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=12.20"
}
},
- "node_modules/@wdio/utils/node_modules/proxy-agent": {
- "version": "6.3.1",
- "resolved": "https://registry.npmjs.org/proxy-agent/-/proxy-agent-6.3.1.tgz",
- "integrity": "sha512-Rb5RVBy1iyqOtNl15Cw/llpeLH8bsb37gM1FUfKQ+Wck6xHlbAhWGUFiTRHtkjqGTA5pSHz6+0hrPW/oECihPQ==",
- "dev": true,
- "dependencies": {
- "agent-base": "^7.0.2",
- "debug": "^4.3.4",
- "http-proxy-agent": "^7.0.0",
- "https-proxy-agent": "^7.0.2",
- "lru-cache": "^7.14.1",
- "pac-proxy-agent": "^7.0.1",
- "proxy-from-env": "^1.1.0",
- "socks-proxy-agent": "^8.0.2"
- },
- "engines": {
- "node": ">= 14"
- }
- },
- "node_modules/@wdio/utils/node_modules/pump": {
- "version": "3.0.2",
- "resolved": "https://registry.npmjs.org/pump/-/pump-3.0.2.tgz",
- "integrity": "sha512-tUPXtzlGM8FE3P0ZL6DVs/3P58k9nk8/jZeQCurTJylQA8qFYzHFfhBJkuqyE0FifOsQ0uKWekiZ5g8wtr28cw==",
- "dev": true,
- "license": "MIT",
- "dependencies": {
- "end-of-stream": "^1.1.0",
- "once": "^1.3.1"
- }
- },
"node_modules/@wdio/utils/node_modules/quick-lru": {
"version": "5.1.1",
"resolved": "https://registry.npmjs.org/quick-lru/-/quick-lru-5.1.1.tgz",
"integrity": "sha512-WuyALRjWPDGtt/wzJiadO5AXY+8hZ80hVpe6MyivgraREW751X3SbhRvG3eLKOYN+8VEvqLcf3wdnt44Z4S4SA==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=10"
},
@@ -16679,6 +15217,7 @@
"resolved": "https://registry.npmjs.org/responselike/-/responselike-3.0.0.tgz",
"integrity": "sha512-40yHxbNcl2+rzXvZuVkrYohathsSJlMTXKryG5y8uciHv1+xDLHQpgjG64JUO9nrEq2jGLH6IZ8BcZyw3wrweg==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"lowercase-keys": "^3.0.0"
},
@@ -16689,119 +15228,16 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/@wdio/utils/node_modules/socks-proxy-agent": {
- "version": "8.0.4",
- "resolved": "https://registry.npmjs.org/socks-proxy-agent/-/socks-proxy-agent-8.0.4.tgz",
- "integrity": "sha512-GNAq/eg8Udq2x0eNiFkr9gRg5bA7PXEWagQdeRX4cPSG+X/8V38v637gim9bjFptMk1QWsCTr0ttrJEiXbNnRw==",
- "dev": true,
- "license": "MIT",
- "dependencies": {
- "agent-base": "^7.1.1",
- "debug": "^4.3.4",
- "socks": "^2.8.3"
- },
- "engines": {
- "node": ">= 14"
- }
- },
"node_modules/@wdio/utils/node_modules/split2": {
"version": "4.2.0",
"resolved": "https://registry.npmjs.org/split2/-/split2-4.2.0.tgz",
"integrity": "sha512-UcjcJOWknrNkF6PLX83qcHM6KHgVKNkV62Y8a5uYDVv9ydGQVwAHMKqHdJje1VTWpljG0WYpCDhrCdAOYH4TWg==",
"dev": true,
+ "license": "ISC",
"engines": {
"node": ">= 10.x"
}
},
- "node_modules/@wdio/utils/node_modules/string-width": {
- "version": "4.2.3",
- "resolved": "https://registry.npmjs.org/string-width/-/string-width-4.2.3.tgz",
- "integrity": "sha512-wKyQRQpjJ0sIp62ErSZdGsjMJWsap5oRNihHhu6G7JVO/9jIB6UyevL+tXuOqrng8j/cxKTWyWUwvSTriiZz/g==",
- "dev": true,
- "dependencies": {
- "emoji-regex": "^8.0.0",
- "is-fullwidth-code-point": "^3.0.0",
- "strip-ansi": "^6.0.1"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/@wdio/utils/node_modules/tar-fs": {
- "version": "3.0.4",
- "resolved": "https://registry.npmjs.org/tar-fs/-/tar-fs-3.0.4.tgz",
- "integrity": "sha512-5AFQU8b9qLfZCX9zp2duONhPmZv0hGYiBPJsyUdqMjzq/mqVpy/rEUSeHk1+YitmxugaptgBh5oDGU3VsAJq4w==",
- "dev": true,
- "dependencies": {
- "mkdirp-classic": "^0.5.2",
- "pump": "^3.0.0",
- "tar-stream": "^3.1.5"
- }
- },
- "node_modules/@wdio/utils/node_modules/tar-stream": {
- "version": "3.1.7",
- "resolved": "https://registry.npmjs.org/tar-stream/-/tar-stream-3.1.7.tgz",
- "integrity": "sha512-qJj60CXt7IU1Ffyc3NJMjh6EkuCFej46zUqJ4J7pqYlThyd9bO0XBTmcOIhSzZJVWfsLks0+nle/j538YAW9RQ==",
- "dev": true,
- "dependencies": {
- "b4a": "^1.6.4",
- "fast-fifo": "^1.2.0",
- "streamx": "^2.15.0"
- }
- },
- "node_modules/@wdio/utils/node_modules/wrap-ansi": {
- "version": "7.0.0",
- "resolved": "https://registry.npmjs.org/wrap-ansi/-/wrap-ansi-7.0.0.tgz",
- "integrity": "sha512-YVGIj2kamLSTxw6NsZjoBxfSwsn0ycdesmc4p+Q21c5zPuZ1pl+NfxVdxPtdHvmNVOQ6XSYG4AUtyt/Fi7D16Q==",
- "dev": true,
- "dependencies": {
- "ansi-styles": "^4.0.0",
- "string-width": "^4.1.0",
- "strip-ansi": "^6.0.0"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/chalk/wrap-ansi?sponsor=1"
- }
- },
- "node_modules/@wdio/utils/node_modules/y18n": {
- "version": "5.0.8",
- "resolved": "https://registry.npmjs.org/y18n/-/y18n-5.0.8.tgz",
- "integrity": "sha512-0pfFzegeDWJHJIAmTLRP2DwHjdF5s7jo9tuztdQxAhINCdvS+3nGINqPd00AphqJR/0LhANUS6/+7SCb98YOfA==",
- "dev": true,
- "engines": {
- "node": ">=10"
- }
- },
- "node_modules/@wdio/utils/node_modules/yargs": {
- "version": "17.7.2",
- "resolved": "https://registry.npmjs.org/yargs/-/yargs-17.7.2.tgz",
- "integrity": "sha512-7dSzzRQ++CKnNI/krKnYRV7JKKPUXMEh61soaHKg9mrWEhzFWhFnxPxGl+69cD1Ou63C13NUPCnmIcrvqCuM6w==",
- "dev": true,
- "dependencies": {
- "cliui": "^8.0.1",
- "escalade": "^3.1.1",
- "get-caller-file": "^2.0.5",
- "require-directory": "^2.1.1",
- "string-width": "^4.2.3",
- "y18n": "^5.0.5",
- "yargs-parser": "^21.1.1"
- },
- "engines": {
- "node": ">=12"
- }
- },
- "node_modules/@wdio/utils/node_modules/yargs-parser": {
- "version": "21.1.1",
- "resolved": "https://registry.npmjs.org/yargs-parser/-/yargs-parser-21.1.1.tgz",
- "integrity": "sha512-tVpsJW7DdjecAiFpbIB1e3qxIQsE6NoPc5/eTdrbbIC4h0LVsWhnoa3g+m2HclBIujHzsxZ4VJVA+GUuc2/LBw==",
- "dev": true,
- "engines": {
- "node": ">=12"
- }
- },
"node_modules/@webassemblyjs/ast": {
"version": "1.9.0",
"resolved": "https://registry.npmjs.org/@webassemblyjs/ast/-/ast-1.9.0.tgz",
@@ -16856,24 +15292,27 @@
}
},
"node_modules/@webassemblyjs/helper-numbers": {
- "version": "1.11.6",
- "resolved": "https://registry.npmjs.org/@webassemblyjs/helper-numbers/-/helper-numbers-1.11.6.tgz",
- "integrity": "sha512-vUIhZ8LZoIWHBohiEObxVm6hwP034jwmc9kuq5GdHZH0wiLVLIPcMCdpJzG4C11cHoQ25TFIQj9kaVADVX7N3g==",
+ "version": "1.13.2",
+ "resolved": "https://registry.npmjs.org/@webassemblyjs/helper-numbers/-/helper-numbers-1.13.2.tgz",
+ "integrity": "sha512-FE8aCmS5Q6eQYcV3gI35O4J789wlQA+7JrqTTpJqn5emA4U2hvwJmvFRC0HODS+3Ye6WioDklgd6scJ3+PLnEA==",
+ "license": "MIT",
"dependencies": {
- "@webassemblyjs/floating-point-hex-parser": "1.11.6",
- "@webassemblyjs/helper-api-error": "1.11.6",
+ "@webassemblyjs/floating-point-hex-parser": "1.13.2",
+ "@webassemblyjs/helper-api-error": "1.13.2",
"@xtuc/long": "4.2.2"
}
},
"node_modules/@webassemblyjs/helper-numbers/node_modules/@webassemblyjs/floating-point-hex-parser": {
- "version": "1.11.6",
- "resolved": "https://registry.npmjs.org/@webassemblyjs/floating-point-hex-parser/-/floating-point-hex-parser-1.11.6.tgz",
- "integrity": "sha512-ejAj9hfRJ2XMsNHk/v6Fu2dGS+i4UaXBXGemOfQ/JfQ6mdQg/WXtwleQRLLS4OvfDhv8rYnVwH27YJLMyYsxhw=="
+ "version": "1.13.2",
+ "resolved": "https://registry.npmjs.org/@webassemblyjs/floating-point-hex-parser/-/floating-point-hex-parser-1.13.2.tgz",
+ "integrity": "sha512-6oXyTOzbKxGH4steLbLNOu71Oj+C8Lg34n6CqRvqfS2O71BxY6ByfMDRhBytzknj9yGUPVJ1qIKhRlAwO1AovA==",
+ "license": "MIT"
},
"node_modules/@webassemblyjs/helper-numbers/node_modules/@webassemblyjs/helper-api-error": {
- "version": "1.11.6",
- "resolved": "https://registry.npmjs.org/@webassemblyjs/helper-api-error/-/helper-api-error-1.11.6.tgz",
- "integrity": "sha512-o0YkoP4pVu4rN8aTJgAyj9hC2Sv5UlkzCHhxqWj8butaLvnpdc2jOwh4ewE6CX0txSfLn/UYaV/pheS2Txg//Q=="
+ "version": "1.13.2",
+ "resolved": "https://registry.npmjs.org/@webassemblyjs/helper-api-error/-/helper-api-error-1.13.2.tgz",
+ "integrity": "sha512-U56GMYxy4ZQCbDZd6JuvvNV/WFildOjsaWD3Tzzvmw/mas3cXzRJPMjP83JqEsgSbyrmaGjBfDtV7KDXV9UzFQ==",
+ "license": "MIT"
},
"node_modules/@webassemblyjs/helper-wasm-bytecode": {
"version": "1.9.0",
@@ -17418,6 +15857,10 @@
"resolved": "packages/undo-manager",
"link": true
},
+ "node_modules/@wordpress/upload-media": {
+ "resolved": "packages/upload-media",
+ "link": true
+ },
"node_modules/@wordpress/url": {
"resolved": "packages/url",
"link": true
@@ -17461,59 +15904,6 @@
"resolved": "https://registry.npmjs.org/@xtuc/long/-/long-4.2.2.tgz",
"integrity": "sha512-NuHqBY1PB/D8xU6s/thBgOAiAP7HOYDQ32+BFZILJ8ivkUkAHQnWfn6WhL79Owj1qmUnoN/YPhktdIoucipkAQ=="
},
- "node_modules/@yarnpkg/esbuild-plugin-pnp": {
- "version": "3.0.0-rc.15",
- "resolved": "https://registry.npmjs.org/@yarnpkg/esbuild-plugin-pnp/-/esbuild-plugin-pnp-3.0.0-rc.15.tgz",
- "integrity": "sha512-kYzDJO5CA9sy+on/s2aIW0411AklfCi8Ck/4QDivOqsMKpStZA2SsR+X27VTggGwpStWaLrjJcDcdDMowtG8MA==",
- "dev": true,
- "dependencies": {
- "tslib": "^2.4.0"
- },
- "engines": {
- "node": ">=14.15.0"
- },
- "peerDependencies": {
- "esbuild": ">=0.10.0"
- }
- },
- "node_modules/@yarnpkg/fslib": {
- "version": "2.10.3",
- "resolved": "https://registry.npmjs.org/@yarnpkg/fslib/-/fslib-2.10.3.tgz",
- "integrity": "sha512-41H+Ga78xT9sHvWLlFOZLIhtU6mTGZ20pZ29EiZa97vnxdohJD2AF42rCoAoWfqUz486xY6fhjMH+DYEM9r14A==",
- "dev": true,
- "dependencies": {
- "@yarnpkg/libzip": "^2.3.0",
- "tslib": "^1.13.0"
- },
- "engines": {
- "node": ">=12 <14 || 14.2 - 14.9 || >14.10.0"
- }
- },
- "node_modules/@yarnpkg/fslib/node_modules/tslib": {
- "version": "1.14.1",
- "resolved": "https://registry.npmjs.org/tslib/-/tslib-1.14.1.tgz",
- "integrity": "sha512-Xni35NKzjgMrwevysHTCArtLDpPvye8zV/0E4EyYn43P7/7qvQwPh9BGkHewbMulVntbigmcT7rdX3BNo9wRJg==",
- "dev": true
- },
- "node_modules/@yarnpkg/libzip": {
- "version": "2.3.0",
- "resolved": "https://registry.npmjs.org/@yarnpkg/libzip/-/libzip-2.3.0.tgz",
- "integrity": "sha512-6xm38yGVIa6mKm/DUCF2zFFJhERh/QWp1ufm4cNUvxsONBmfPg8uZ9pZBdOmF6qFGr/HlT6ABBkCSx/dlEtvWg==",
- "dev": true,
- "dependencies": {
- "@types/emscripten": "^1.39.6",
- "tslib": "^1.13.0"
- },
- "engines": {
- "node": ">=12 <14 || 14.2 - 14.9 || >14.10.0"
- }
- },
- "node_modules/@yarnpkg/libzip/node_modules/tslib": {
- "version": "1.14.1",
- "resolved": "https://registry.npmjs.org/tslib/-/tslib-1.14.1.tgz",
- "integrity": "sha512-Xni35NKzjgMrwevysHTCArtLDpPvye8zV/0E4EyYn43P7/7qvQwPh9BGkHewbMulVntbigmcT7rdX3BNo9wRJg==",
- "dev": true
- },
"node_modules/@yarnpkg/lockfile": {
"version": "1.1.0",
"resolved": "https://registry.npmjs.org/@yarnpkg/lockfile/-/lockfile-1.1.0.tgz",
@@ -17521,23 +15911,37 @@
"dev": true
},
"node_modules/@yarnpkg/parsers": {
- "version": "3.0.0-rc.46",
- "resolved": "https://registry.npmjs.org/@yarnpkg/parsers/-/parsers-3.0.0-rc.46.tgz",
- "integrity": "sha512-aiATs7pSutzda/rq8fnuPwTglyVwjM22bNnK2ZgjrpAjQHSSl3lztd2f9evst1W/qnC58DRz7T7QndUDumAR4Q==",
+ "version": "3.0.2",
+ "resolved": "https://registry.npmjs.org/@yarnpkg/parsers/-/parsers-3.0.2.tgz",
+ "integrity": "sha512-/HcYgtUSiJiot/XWGLOlGxPYUG65+/31V8oqk17vZLW1xlCoR4PampyePljOxY2n8/3jz9+tIFzICsyGujJZoA==",
"dev": true,
+ "license": "BSD-2-Clause",
"dependencies": {
"js-yaml": "^3.10.0",
"tslib": "^2.4.0"
},
"engines": {
- "node": ">=14.15.0"
+ "node": ">=18.12.0"
+ }
+ },
+ "node_modules/@zip.js/zip.js": {
+ "version": "2.7.54",
+ "resolved": "https://registry.npmjs.org/@zip.js/zip.js/-/zip.js-2.7.54.tgz",
+ "integrity": "sha512-qMrJVg2hoEsZJjMJez9yI2+nZlBUxgYzGV3mqcb2B/6T1ihXp0fWBDYlVHlHquuorgNUQP5a8qSmX6HF5rFJNg==",
+ "dev": true,
+ "license": "BSD-3-Clause",
+ "engines": {
+ "bun": ">=0.7.0",
+ "deno": ">=1.0.0",
+ "node": ">=16.5.0"
}
},
"node_modules/@zkochan/js-yaml": {
- "version": "0.0.6",
- "resolved": "https://registry.npmjs.org/@zkochan/js-yaml/-/js-yaml-0.0.6.tgz",
- "integrity": "sha512-nzvgl3VfhcELQ8LyVrYOru+UtAy1nrygk2+AGbTm8a5YcO6o8lSjAT+pfg3vJWxIoZKOUhrK6UU7xW/+00kQrg==",
+ "version": "0.0.7",
+ "resolved": "https://registry.npmjs.org/@zkochan/js-yaml/-/js-yaml-0.0.7.tgz",
+ "integrity": "sha512-nrUSn7hzt7J6JWgWGz78ZYI8wj+gdIJdk0Ynjpp8l+trkn58Uqsf6RYrYkEK+3X18EX+TNdtJI0WxAtc+L84SQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"argparse": "^2.0.1"
},
@@ -17549,7 +15953,8 @@
"version": "2.0.1",
"resolved": "https://registry.npmjs.org/argparse/-/argparse-2.0.1.tgz",
"integrity": "sha512-8+9WqebbFzpX9OR+Wa6O29asIogeRMzcGtAINdpMHHyAg10f05aSFVBbcEqGf/PXw1EjAZ+q2/bEBg3DvurK3Q==",
- "dev": true
+ "dev": true,
+ "license": "Python-2.0"
},
"node_modules/abab": {
"version": "2.0.6",
@@ -17557,10 +15962,14 @@
"integrity": "sha512-j2afSsaIENvHZN2B8GOpF566vZ5WVk5opAiMTvWgaQT8DkbOqsTfvNAvHoRGU2zzP8cPoqys+xHTRDWW8L+/BA=="
},
"node_modules/abbrev": {
- "version": "1.1.1",
- "resolved": "https://registry.npmjs.org/abbrev/-/abbrev-1.1.1.tgz",
- "integrity": "sha512-nne9/IiQ/hzIhY6pdDnbBtz7DjPTKrY00P/zvPSm5pOFkl6xuGrGnXn/VtTNNfNtAfZ9/1RtehkszU9qcTii0Q==",
- "dev": true
+ "version": "2.0.0",
+ "resolved": "https://registry.npmjs.org/abbrev/-/abbrev-2.0.0.tgz",
+ "integrity": "sha512-6/mh1E2u2YgEsCHdY0Yx5oW+61gZU+1vXaoiHHrpKeuRNNgFvS+/jrwHiQhB5apAf5oB7UB7E19ol2R2LKH8hQ==",
+ "dev": true,
+ "license": "ISC",
+ "engines": {
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ }
},
"node_modules/abort-controller": {
"version": "3.0.0",
@@ -17597,14 +16006,6 @@
"node": ">=0.4.0"
}
},
- "node_modules/acorn-import-attributes": {
- "version": "1.9.5",
- "resolved": "https://registry.npmjs.org/acorn-import-attributes/-/acorn-import-attributes-1.9.5.tgz",
- "integrity": "sha512-n02Vykv5uA3eHGM/Z2dQrcD56kL8TyDb2p1+0P83PClMnC/nc+anbQRhIOWnSq4Ke/KvDPrY3C9hDtC/A3eHnQ==",
- "peerDependencies": {
- "acorn": "^8"
- }
- },
"node_modules/acorn-jsx": {
"version": "5.3.1",
"resolved": "https://registry.npmjs.org/acorn-jsx/-/acorn-jsx-5.3.1.tgz",
@@ -17613,29 +16014,12 @@
"acorn": "^6.0.0 || ^7.0.0 || ^8.0.0"
}
},
- "node_modules/acorn-walk": {
- "version": "7.2.0",
- "resolved": "https://registry.npmjs.org/acorn-walk/-/acorn-walk-7.2.0.tgz",
- "integrity": "sha512-OPdCF6GsMIP+Az+aWfAAOEt2/+iVDKE7oy6lJ098aoe59oAmK76qV6Gw60SbZ8jHuG2wH058GF4pLFbYamYrVA==",
- "dev": true,
- "engines": {
- "node": ">=0.4.0"
- }
- },
"node_modules/add-stream": {
"version": "1.0.0",
"resolved": "https://registry.npmjs.org/add-stream/-/add-stream-1.0.0.tgz",
"integrity": "sha512-qQLMr+8o0WC4FZGQTcJiKBVC59JylcPSrTtk6usvmIDFUOCKegapy1VHQwRbFMOFyb/inzUVqHs+eMYKDM1YeQ==",
- "dev": true
- },
- "node_modules/address": {
- "version": "1.2.2",
- "resolved": "https://registry.npmjs.org/address/-/address-1.2.2.tgz",
- "integrity": "sha512-4B/qKCfeE/ODUaAUpSwfzazo5x29WD4r3vXiWsB7I2mSDAihwEqKO+g8GELZUQSSAo5e1XTYh3ZVfLyxBc12nA==",
"dev": true,
- "engines": {
- "node": ">= 10.0.0"
- }
+ "license": "MIT"
},
"node_modules/adm-zip": {
"version": "0.5.9",
@@ -17646,26 +16030,15 @@
}
},
"node_modules/agent-base": {
- "version": "6.0.2",
- "resolved": "https://registry.npmjs.org/agent-base/-/agent-base-6.0.2.tgz",
- "integrity": "sha512-RZNwNclF7+MS/8bDg70amg32dyeZGZxiDuQmZxKLAlQjr3jGyLx+4Kkk58UO7D2QdgFIQCovuSuZESne6RG6XQ==",
- "dependencies": {
- "debug": "4"
- },
- "engines": {
- "node": ">= 6.0.0"
- }
- },
- "node_modules/agentkeepalive": {
- "version": "4.5.0",
- "resolved": "https://registry.npmjs.org/agentkeepalive/-/agentkeepalive-4.5.0.tgz",
- "integrity": "sha512-5GG/5IbQQpC9FpkRGsSvZI5QYeSCzlJHdpBQntCsuTOxhKD8lqKhrleg2Yi7yvMIf82Ycmmqln9U8V9qwEiJew==",
- "dev": true,
+ "version": "7.1.2",
+ "resolved": "https://registry.npmjs.org/agent-base/-/agent-base-7.1.2.tgz",
+ "integrity": "sha512-JVzqkCNRT+VfqzzgPWDPnwvDheSAUdiMUn3NoLXpDJF5lRqeJqyC9iGsAxIOAW+mzIdq+uP1TvcX6bMtrH0agg==",
+ "license": "MIT",
"dependencies": {
- "humanize-ms": "^1.2.1"
+ "debug": "^4.3.4"
},
"engines": {
- "node": ">= 8.0.0"
+ "node": ">= 14"
}
},
"node_modules/aggregate-error": {
@@ -17909,12 +16282,6 @@
"node": ">= 8"
}
},
- "node_modules/app-root-dir": {
- "version": "1.0.2",
- "resolved": "https://registry.npmjs.org/app-root-dir/-/app-root-dir-1.0.2.tgz",
- "integrity": "sha512-jlpIfsOoNoafl92Sz//64uQHGSyMrD2vYG5d8o2a4qGvyNCvXur7bzIsWtAC/6flI2RYAp3kv8rsfBtaLm7w0g==",
- "dev": true
- },
"node_modules/app-root-path": {
"version": "2.2.1",
"resolved": "https://registry.npmjs.org/app-root-path/-/app-root-path-2.2.1.tgz",
@@ -18049,38 +16416,6 @@
"node": ">=8"
}
},
- "node_modules/appium/node_modules/cross-env": {
- "version": "7.0.3",
- "resolved": "https://registry.npmjs.org/cross-env/-/cross-env-7.0.3.tgz",
- "integrity": "sha512-+/HKd6EgcQCJGh2PSjZuUitQBQynKor4wrFbRg4DtAgS1aWO+gU52xpH7M9ScGgXSYmAVS9bIJ8EzuaGw0oNAw==",
- "dev": true,
- "dependencies": {
- "cross-spawn": "^7.0.1"
- },
- "bin": {
- "cross-env": "src/bin/cross-env.js",
- "cross-env-shell": "src/bin/cross-env-shell.js"
- },
- "engines": {
- "node": ">=10.14",
- "npm": ">=6",
- "yarn": ">=1"
- }
- },
- "node_modules/appium/node_modules/cross-spawn": {
- "version": "7.0.3",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-7.0.3.tgz",
- "integrity": "sha512-iRDPJKUPVEND7dHPO8rkbOnPpyDygcDFtWjpeWNCgy8WP2rXcxXL8TskReQl6OrB2G7+UJrags1q15Fudc7G6w==",
- "dev": true,
- "dependencies": {
- "path-key": "^3.1.0",
- "shebang-command": "^2.0.0",
- "which": "^2.0.1"
- },
- "engines": {
- "node": ">= 8"
- }
- },
"node_modules/appium/node_modules/emoji-regex": {
"version": "8.0.0",
"resolved": "https://registry.npmjs.org/emoji-regex/-/emoji-regex-8.0.0.tgz",
@@ -18281,15 +16616,6 @@
"node": ">=8"
}
},
- "node_modules/appium/node_modules/path-key": {
- "version": "3.1.1",
- "resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
- "integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
- "dev": true,
- "engines": {
- "node": ">=8"
- }
- },
"node_modules/appium/node_modules/resolve-from": {
"version": "5.0.0",
"resolved": "https://registry.npmjs.org/resolve-from/-/resolve-from-5.0.0.tgz",
@@ -18333,27 +16659,6 @@
"node": ">=10"
}
},
- "node_modules/appium/node_modules/shebang-command": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/shebang-command/-/shebang-command-2.0.0.tgz",
- "integrity": "sha512-kHxr2zZpYtdmrN1qDjrrX/Z1rR1kG8Dx+gkpK1G4eXmvXswmcE1hTWBWYUzlraYw1/yZp6YuDY77YtvbN0dmDA==",
- "dev": true,
- "dependencies": {
- "shebang-regex": "^3.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/appium/node_modules/shebang-regex": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/shebang-regex/-/shebang-regex-3.0.0.tgz",
- "integrity": "sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==",
- "dev": true,
- "engines": {
- "node": ">=8"
- }
- },
"node_modules/appium/node_modules/signal-exit": {
"version": "4.1.0",
"resolved": "https://registry.npmjs.org/signal-exit/-/signal-exit-4.1.0.tgz",
@@ -18392,21 +16697,6 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/appium/node_modules/which": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
- "integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
- "dev": true,
- "dependencies": {
- "isexe": "^2.0.0"
- },
- "bin": {
- "node-which": "bin/node-which"
- },
- "engines": {
- "node": ">= 8"
- }
- },
"node_modules/appium/node_modules/wrap-ansi": {
"version": "7.0.0",
"resolved": "https://registry.npmjs.org/wrap-ansi/-/wrap-ansi-7.0.0.tgz",
@@ -18424,12 +16714,6 @@
"url": "https://github.com/chalk/wrap-ansi?sponsor=1"
}
},
- "node_modules/appium/node_modules/yallist": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/yallist/-/yallist-4.0.0.tgz",
- "integrity": "sha512-3wdGidZyq5PB084XLES5TpOSRA3wjXAlIWMhum2kRcv/41Sn2emQ0dycQW4uZXLejwKvg6EsvbdlVL+FYEct7A==",
- "dev": true
- },
"node_modules/appium/node_modules/yaml": {
"version": "2.3.1",
"resolved": "https://registry.npmjs.org/yaml/-/yaml-2.3.1.tgz",
@@ -18450,6 +16734,7 @@
"resolved": "https://registry.npmjs.org/archiver/-/archiver-6.0.1.tgz",
"integrity": "sha512-CXGy4poOLBKptiZH//VlWdFuUC1RESbdZjGjILwBuZ73P7WkAUN0htfSfBq/7k6FRFlpu7bg4JOkj1vU9G6jcQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"archiver-utils": "^4.0.1",
"async": "^3.2.4",
@@ -18468,6 +16753,7 @@
"resolved": "https://registry.npmjs.org/archiver-utils/-/archiver-utils-4.0.1.tgz",
"integrity": "sha512-Q4Q99idbvzmgCTEAAhi32BkOyq8iVI5EwdO0PmBDSGIzzjYNdcFn7Q7k3OzbLy4kLUPXfJtG6fO2RjftXbobBg==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"glob": "^8.0.0",
"graceful-fs": "^4.2.0",
@@ -18485,6 +16771,7 @@
"resolved": "https://registry.npmjs.org/brace-expansion/-/brace-expansion-2.0.1.tgz",
"integrity": "sha512-XnAIvQ8eM+kC6aULx6wuQiwVsnzsi9d3WxzV3FpWTGA19F621kwdbsAcFKXgKUHZWsy+mY6iL1sHTxWEFCytDA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"balanced-match": "^1.0.0"
}
@@ -18493,7 +16780,9 @@
"version": "8.1.0",
"resolved": "https://registry.npmjs.org/glob/-/glob-8.1.0.tgz",
"integrity": "sha512-r8hpEjiQEYlF2QU0df3dS+nxxSIreXQS1qRhMJM0Q5NDdR386C7jb7Hwwod8Fgiuex+k0GFjgft18yvxm5XoCQ==",
+ "deprecated": "Glob versions prior to v9 are no longer supported",
"dev": true,
+ "license": "ISC",
"dependencies": {
"fs.realpath": "^1.0.0",
"inflight": "^1.0.4",
@@ -18513,6 +16802,7 @@
"resolved": "https://registry.npmjs.org/minimatch/-/minimatch-5.1.6.tgz",
"integrity": "sha512-lKwV/1brpG6mBUFHtb7NUmtABCb2WZZmm2wNiOA5hAb8VdCS4B3dtMWyvcoViccwAW/COERjXLt0zP1zXUN26g==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"brace-expansion": "^2.0.1"
},
@@ -18525,6 +16815,7 @@
"resolved": "https://registry.npmjs.org/readable-stream/-/readable-stream-3.6.2.tgz",
"integrity": "sha512-9u/sniCrY3D5WdsERHzHE4G2YCXqoG5FTHUiCC4SIbr6XcLZBY05ya9EKjYek9O5xOAwjGq+1JdGBAS7Q9ScoA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"inherits": "^2.0.3",
"string_decoder": "^1.1.1",
@@ -18539,6 +16830,7 @@
"resolved": "https://registry.npmjs.org/readable-stream/-/readable-stream-3.6.2.tgz",
"integrity": "sha512-9u/sniCrY3D5WdsERHzHE4G2YCXqoG5FTHUiCC4SIbr6XcLZBY05ya9EKjYek9O5xOAwjGq+1JdGBAS7Q9ScoA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"inherits": "^2.0.3",
"string_decoder": "^1.1.1",
@@ -18553,6 +16845,7 @@
"resolved": "https://registry.npmjs.org/tar-stream/-/tar-stream-3.1.7.tgz",
"integrity": "sha512-qJj60CXt7IU1Ffyc3NJMjh6EkuCFej46zUqJ4J7pqYlThyd9bO0XBTmcOIhSzZJVWfsLks0+nle/j538YAW9RQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"b4a": "^1.6.4",
"fast-fifo": "^1.2.0",
@@ -18567,33 +16860,6 @@
"node": ">=14"
}
},
- "node_modules/are-we-there-yet": {
- "version": "3.0.1",
- "resolved": "https://registry.npmjs.org/are-we-there-yet/-/are-we-there-yet-3.0.1.tgz",
- "integrity": "sha512-QZW4EDmGwlYur0Yyf/b2uGucHQMa8aFUP7eu9ddR73vvhFyt4V0Vl3QHPcTNJ8l6qYOBdxgXdnBXQrHilfRQBg==",
- "dev": true,
- "dependencies": {
- "delegates": "^1.0.0",
- "readable-stream": "^3.6.0"
- },
- "engines": {
- "node": "^12.13.0 || ^14.15.0 || >=16.0.0"
- }
- },
- "node_modules/are-we-there-yet/node_modules/readable-stream": {
- "version": "3.6.2",
- "resolved": "https://registry.npmjs.org/readable-stream/-/readable-stream-3.6.2.tgz",
- "integrity": "sha512-9u/sniCrY3D5WdsERHzHE4G2YCXqoG5FTHUiCC4SIbr6XcLZBY05ya9EKjYek9O5xOAwjGq+1JdGBAS7Q9ScoA==",
- "dev": true,
- "dependencies": {
- "inherits": "^2.0.3",
- "string_decoder": "^1.1.1",
- "util-deprecate": "^1.0.1"
- },
- "engines": {
- "node": ">= 6"
- }
- },
"node_modules/arg": {
"version": "4.1.3",
"resolved": "https://registry.npmjs.org/arg/-/arg-4.1.3.tgz",
@@ -18679,6 +16945,7 @@
"resolved": "https://registry.npmjs.org/array-differ/-/array-differ-3.0.0.tgz",
"integrity": "sha512-THtfYS6KtME/yIAhKjZ2ul7XI96lQGHRputJQHO80LAWQnuGP4iCIN8vdMRboGbIEYBwU33q8Tch1os2+X0kMg==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=8"
}
@@ -18692,7 +16959,8 @@
"version": "1.0.0",
"resolved": "https://registry.npmjs.org/array-ify/-/array-ify-1.0.0.tgz",
"integrity": "sha512-c5AMf34bKdvPhQ7tBGhqkgKNUzMr4WUs+WDtC2ZUGOUncbxKMTvqxYctiseW3+L4bA8ec+GcZ6/A/FW4m8ukng==",
- "dev": true
+ "dev": true,
+ "license": "MIT"
},
"node_modules/array-includes": {
"version": "3.1.6",
@@ -18848,6 +17116,15 @@
"inherits": "2.0.3"
}
},
+ "node_modules/assertion-error": {
+ "version": "2.0.1",
+ "resolved": "https://registry.npmjs.org/assertion-error/-/assertion-error-2.0.1.tgz",
+ "integrity": "sha512-Izi8RQcffqCeNVgFigKli1ssklIbpHnCYc6AknXGYoB6grJqyeby7jv12JUQgmTAnIDnbck1uxksT4dzN3PWBA==",
+ "dev": true,
+ "engines": {
+ "node": ">=12"
+ }
+ },
"node_modules/assign-symbols": {
"version": "1.0.0",
"resolved": "https://registry.npmjs.org/assign-symbols/-/assign-symbols-1.0.0.tgz",
@@ -19032,9 +17309,10 @@
}
},
"node_modules/axe-core": {
- "version": "4.7.2",
- "resolved": "https://registry.npmjs.org/axe-core/-/axe-core-4.7.2.tgz",
- "integrity": "sha512-zIURGIS1E1Q4pcrMjp+nnEh+16G56eG/MUllJH8yEvw7asDo7Ac9uhC9KIH5jzpITueEZolfYglnCGIuSBz39g==",
+ "version": "4.10.2",
+ "resolved": "https://registry.npmjs.org/axe-core/-/axe-core-4.10.2.tgz",
+ "integrity": "sha512-RE3mdQ7P3FRSe7eqCWoeQ/Z9QXrtniSjp1wUjt5nRC3WIpz5rSCve6o3fsZ2aCpJtrZjSZgjwXAoTO5k4tEI0w==",
+ "license": "MPL-2.0",
"engines": {
"node": ">=4"
}
@@ -19044,6 +17322,7 @@
"resolved": "https://registry.npmjs.org/axios/-/axios-1.5.1.tgz",
"integrity": "sha512-Q28iYCWzNHjAm+yEAot5QaAMxhMghWLFVf7rRdwhUI+c2jix2DUXjAHXVi+s1ibs3mjPO/cCgbA++3BjD0vP/A==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"follow-redirects": "^1.15.0",
"form-data": "^4.0.0",
@@ -19092,7 +17371,6 @@
"version": "9.2.1",
"resolved": "https://registry.npmjs.org/babel-loader/-/babel-loader-9.2.1.tgz",
"integrity": "sha512-fqe8naHt46e0yIdkjUZYqddSXfej3AHajX+CSO5X7oy0EmPc6o5Xh+RClNoHjnieWz9AW4kZxW9yyFMhVB1QLA==",
- "dev": true,
"license": "MIT",
"dependencies": {
"find-cache-dir": "^4.0.0",
@@ -19110,7 +17388,6 @@
"version": "8.17.1",
"resolved": "https://registry.npmjs.org/ajv/-/ajv-8.17.1.tgz",
"integrity": "sha512-B/gBuNg5SiMTrPkC+A2+cW0RszwxYmn6VYxB/inlBStS5nx6xHIt/ehKRhIMhqusl7a8LjQoZnjCs5vhwxOQ1g==",
- "dev": true,
"license": "MIT",
"dependencies": {
"fast-deep-equal": "^3.1.3",
@@ -19127,7 +17404,6 @@
"version": "5.1.0",
"resolved": "https://registry.npmjs.org/ajv-keywords/-/ajv-keywords-5.1.0.tgz",
"integrity": "sha512-YCS/JNFAUyr5vAuhk1DWm1CBxRHW9LbJ2ozWeemrIqpbsqKjHVxYPyi5GC0rjZIT5JxJ3virVTS8wk4i/Z+krw==",
- "dev": true,
"dependencies": {
"fast-deep-equal": "^3.1.3"
},
@@ -19139,7 +17415,6 @@
"version": "4.0.0",
"resolved": "https://registry.npmjs.org/find-cache-dir/-/find-cache-dir-4.0.0.tgz",
"integrity": "sha512-9ZonPT4ZAK4a+1pUPVPZJapbi7O5qbbJPdYw/NOQWZZbVLdDTYM3A4R9z/DpAM08IDaFGsvPgiGZ82WEwUDWjg==",
- "dev": true,
"dependencies": {
"common-path-prefix": "^3.0.0",
"pkg-dir": "^7.0.0"
@@ -19155,7 +17430,6 @@
"version": "6.3.0",
"resolved": "https://registry.npmjs.org/find-up/-/find-up-6.3.0.tgz",
"integrity": "sha512-v2ZsoEuVHYy8ZIlYqwPe/39Cy+cFDzp4dXPaxNvkEuouymu+2Jbz0PxpKarJHYJTmv2HWT3O382qY8l4jMWthw==",
- "dev": true,
"dependencies": {
"locate-path": "^7.1.0",
"path-exists": "^5.0.0"
@@ -19170,14 +17444,12 @@
"node_modules/babel-loader/node_modules/json-schema-traverse": {
"version": "1.0.0",
"resolved": "https://registry.npmjs.org/json-schema-traverse/-/json-schema-traverse-1.0.0.tgz",
- "integrity": "sha512-NM8/P9n3XjXhIZn1lLhkFaACTOURQXjWhV4BA/RnOv8xvgqtqpAX9IO4mRQxSx1Rlo4tqzeqb0sOlruaOy3dug==",
- "dev": true
+ "integrity": "sha512-NM8/P9n3XjXhIZn1lLhkFaACTOURQXjWhV4BA/RnOv8xvgqtqpAX9IO4mRQxSx1Rlo4tqzeqb0sOlruaOy3dug=="
},
"node_modules/babel-loader/node_modules/locate-path": {
"version": "7.2.0",
"resolved": "https://registry.npmjs.org/locate-path/-/locate-path-7.2.0.tgz",
"integrity": "sha512-gvVijfZvn7R+2qyPX8mAuKcFGDf6Nc61GdvGafQsHL0sBIxfKzA+usWn4GFC/bk+QdwPUD4kWFJLhElipq+0VA==",
- "dev": true,
"dependencies": {
"p-locate": "^6.0.0"
},
@@ -19192,7 +17464,6 @@
"version": "4.0.0",
"resolved": "https://registry.npmjs.org/p-limit/-/p-limit-4.0.0.tgz",
"integrity": "sha512-5b0R4txpzjPWVw/cXXUResoD4hb6U/x9BH08L7nw+GN1sezDzPdxeRvpc9c433fZhBan/wusjbCsqwqm4EIBIQ==",
- "dev": true,
"dependencies": {
"yocto-queue": "^1.0.0"
},
@@ -19207,7 +17478,6 @@
"version": "6.0.0",
"resolved": "https://registry.npmjs.org/p-locate/-/p-locate-6.0.0.tgz",
"integrity": "sha512-wPrq66Llhl7/4AGC6I+cqxT07LhXvWL08LNXz1fENOw0Ap4sRZZ/gZpTTJ5jpurzzzfS2W/Ge9BY3LgLjCShcw==",
- "dev": true,
"dependencies": {
"p-limit": "^4.0.0"
},
@@ -19222,7 +17492,6 @@
"version": "5.0.0",
"resolved": "https://registry.npmjs.org/path-exists/-/path-exists-5.0.0.tgz",
"integrity": "sha512-RjhtfwJOxzcFmNOi6ltcbcu4Iu+FL3zEj83dk4kAS+fVpTxXLO1b38RvJgT/0QwvV/L3aY9TAnyv0EOqW4GoMQ==",
- "dev": true,
"engines": {
"node": "^12.20.0 || ^14.13.1 || >=16.0.0"
}
@@ -19231,7 +17500,6 @@
"version": "7.0.0",
"resolved": "https://registry.npmjs.org/pkg-dir/-/pkg-dir-7.0.0.tgz",
"integrity": "sha512-Ie9z/WINcxxLp27BKOCHGde4ITq9UklYKDzVo1nhk5sqGEXU3FpkwP5GM2voTGJkGd9B3Otl+Q4uwSOeSUtOBA==",
- "dev": true,
"dependencies": {
"find-up": "^6.3.0"
},
@@ -19246,7 +17514,6 @@
"version": "4.2.0",
"resolved": "https://registry.npmjs.org/schema-utils/-/schema-utils-4.2.0.tgz",
"integrity": "sha512-L0jRsrPpjdckP3oPug3/VxNKt2trR8TcabrM6FOAAlvC/9Phcmm+cuAgTlxBqdBR1WJx7Naj9WHw+aOmheSVbw==",
- "dev": true,
"dependencies": {
"@types/json-schema": "^7.0.9",
"ajv": "^8.9.0",
@@ -19265,7 +17532,6 @@
"version": "1.1.1",
"resolved": "https://registry.npmjs.org/yocto-queue/-/yocto-queue-1.1.1.tgz",
"integrity": "sha512-b4JR1PFR10y1mKjhHY9LaGo6tmrgjit7hxVIeAmyMw3jegXR4dhYqLaQF5zMXZxY7tLpMyJeLjr1C4rLmkVe8g==",
- "dev": true,
"license": "MIT",
"engines": {
"node": ">=12.20"
@@ -19274,12 +17540,6 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/babel-plugin-add-react-displayname": {
- "version": "0.0.5",
- "resolved": "https://registry.npmjs.org/babel-plugin-add-react-displayname/-/babel-plugin-add-react-displayname-0.0.5.tgz",
- "integrity": "sha512-LY3+Y0XVDYcShHHorshrDbt4KFWL4bSeniCtl4SYZbask+Syngk1uMPCeN9+nSiZo6zX5s0RTq/J9Pnaaf/KHw==",
- "dev": true
- },
"node_modules/babel-plugin-inline-json-import": {
"version": "0.3.2",
"resolved": "https://registry.npmjs.org/babel-plugin-inline-json-import/-/babel-plugin-inline-json-import-0.3.2.tgz",
@@ -19720,17 +17980,57 @@
"node": "*"
}
},
- "node_modules/binary": {
- "version": "0.3.0",
- "resolved": "https://registry.npmjs.org/binary/-/binary-0.3.0.tgz",
- "integrity": "sha512-D4H1y5KYwpJgK8wk1Cue5LLPgmwHKYSChkbspQg5JtVuR5ulGckxfR62H3AE9UDkdMC8yyXlqYihuz3Aqg2XZg==",
+ "node_modules/bin-links": {
+ "version": "4.0.4",
+ "resolved": "https://registry.npmjs.org/bin-links/-/bin-links-4.0.4.tgz",
+ "integrity": "sha512-cMtq4W5ZsEwcutJrVId+a/tjt8GSbS+h0oNkdl6+6rBuEv8Ot33Bevj5KPm40t309zuhVic8NjpuL42QCiJWWA==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "buffers": "~0.1.1",
- "chainsaw": "~0.1.0"
+ "cmd-shim": "^6.0.0",
+ "npm-normalize-package-bin": "^3.0.0",
+ "read-cmd-shim": "^4.0.0",
+ "write-file-atomic": "^5.0.0"
},
"engines": {
- "node": "*"
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ }
+ },
+ "node_modules/bin-links/node_modules/npm-normalize-package-bin": {
+ "version": "3.0.1",
+ "resolved": "https://registry.npmjs.org/npm-normalize-package-bin/-/npm-normalize-package-bin-3.0.1.tgz",
+ "integrity": "sha512-dMxCf+zZ+3zeQZXKxmyuCKlIDPGuv8EF940xbkC4kQVDTtqoh6rJFO+JTKSA6/Rwi0getWmtuy4Itup0AMcaDQ==",
+ "dev": true,
+ "license": "ISC",
+ "engines": {
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ }
+ },
+ "node_modules/bin-links/node_modules/signal-exit": {
+ "version": "4.1.0",
+ "resolved": "https://registry.npmjs.org/signal-exit/-/signal-exit-4.1.0.tgz",
+ "integrity": "sha512-bzyZ1e88w9O1iNJbKnOlvYTrWPDl46O1bG0D3XInv+9tkPrxrN8jUUTiFlDkkmKWgn1M6CfIA13SuGqOa9Korw==",
+ "dev": true,
+ "license": "ISC",
+ "engines": {
+ "node": ">=14"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
+ }
+ },
+ "node_modules/bin-links/node_modules/write-file-atomic": {
+ "version": "5.0.1",
+ "resolved": "https://registry.npmjs.org/write-file-atomic/-/write-file-atomic-5.0.1.tgz",
+ "integrity": "sha512-+QU2zd6OTD8XWIJCbffaiQeH9U73qIqafo1x6V1snCWYGJf6cVE0cDR4D8xRzcEnfI21IFrUPzPGtcPf8AC+Rw==",
+ "dev": true,
+ "license": "ISC",
+ "dependencies": {
+ "imurmurhash": "^0.1.4",
+ "signal-exit": "^4.0.1"
+ },
+ "engines": {
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
"node_modules/binary-extensions": {
@@ -19821,6 +18121,7 @@
"version": "1.20.1",
"resolved": "https://registry.npmjs.org/body-parser/-/body-parser-1.20.1.tgz",
"integrity": "sha512-jWi7abTbYwajOytWCQc37VulmWiRae5RyTpaCyDcS5/lMdtwSz5lOpDE67srw/HYe35f1z3fDQw+3txg7gNtWw==",
+ "license": "MIT",
"dependencies": {
"bytes": "3.1.2",
"content-type": "~1.0.4",
@@ -19844,6 +18145,7 @@
"version": "3.1.2",
"resolved": "https://registry.npmjs.org/bytes/-/bytes-3.1.2.tgz",
"integrity": "sha512-/Nf7TyzTx6S3yRJObOAV7956r8cr2+Oj8AC5dt8wSP3BQAoeX58NoHyCU8P8zGkNXStjTSi6fzO6F0pBdcYbEg==",
+ "license": "MIT",
"engines": {
"node": ">= 0.8"
}
@@ -19852,6 +18154,7 @@
"version": "2.6.9",
"resolved": "https://registry.npmjs.org/debug/-/debug-2.6.9.tgz",
"integrity": "sha512-bC7ElrdJaJnPbAP+1EotYvqZsb3ecl5wi6Bfi6BJTUcNowp6cvspg0jXznRTKDjm/E7AdgFBVeAPVMNcKGsHMA==",
+ "license": "MIT",
"dependencies": {
"ms": "2.0.0"
}
@@ -19860,6 +18163,7 @@
"version": "2.0.0",
"resolved": "https://registry.npmjs.org/depd/-/depd-2.0.0.tgz",
"integrity": "sha512-g7nH6P6dyDioJogAAGprGpCtVImJhpPk/roCzdb3fIh61/s/nPsfR6onyMwkCAR/OlC3yBC0lESvUoQEAssIrw==",
+ "license": "MIT",
"engines": {
"node": ">= 0.8"
}
@@ -19868,6 +18172,7 @@
"version": "0.4.24",
"resolved": "https://registry.npmjs.org/iconv-lite/-/iconv-lite-0.4.24.tgz",
"integrity": "sha512-v3MXnZAcvnywkTUEZomIActle7RXXeedOR31wwl7VlyoXO4Qi9arvSenNQWne1TcRwhCL1HwLI21bEqdpj8/rA==",
+ "license": "MIT",
"dependencies": {
"safer-buffer": ">= 2.1.2 < 3"
},
@@ -19879,6 +18184,7 @@
"version": "2.4.1",
"resolved": "https://registry.npmjs.org/on-finished/-/on-finished-2.4.1.tgz",
"integrity": "sha512-oVlzkg3ENAhCk2zdv7IJwd/QUD4z2RxRwpkcGY8psCVcCYZNq4wYnVWALHM+brtuJjePWiYF/ClmuDr8Ch5+kg==",
+ "license": "MIT",
"dependencies": {
"ee-first": "1.1.1"
},
@@ -19886,22 +18192,31 @@
"node": ">= 0.8"
}
},
+ "node_modules/body-parser/node_modules/qs": {
+ "version": "6.11.0",
+ "resolved": "https://registry.npmjs.org/qs/-/qs-6.11.0.tgz",
+ "integrity": "sha512-MvjoMCJwEarSbUYk5O+nmoSzSutSsTwF85zcHPQ9OrlFoZOYIjaqBAJIqIXjptyD5vThxGq52Xu/MaJzRkIk4Q==",
+ "license": "BSD-3-Clause",
+ "dependencies": {
+ "side-channel": "^1.0.4"
+ },
+ "engines": {
+ "node": ">=0.6"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/ljharb"
+ }
+ },
"node_modules/bonjour-service": {
- "version": "1.1.1",
- "resolved": "https://registry.npmjs.org/bonjour-service/-/bonjour-service-1.1.1.tgz",
- "integrity": "sha512-Z/5lQRMOG9k7W+FkeGTNjh7htqn/2LMnfOvBZ8pynNZCM9MwkQkI3zeI4oz09uWdcgmgHugVvBqxGg4VQJ5PCg==",
+ "version": "1.3.0",
+ "resolved": "https://registry.npmjs.org/bonjour-service/-/bonjour-service-1.3.0.tgz",
+ "integrity": "sha512-3YuAUiSkWykd+2Azjgyxei8OWf8thdn8AITIog2M4UICzoqfjlqr64WIjEXZllf/W6vK1goqleSR6brGomxQqA==",
+ "license": "MIT",
"dependencies": {
- "array-flatten": "^2.1.2",
- "dns-equal": "^1.0.0",
"fast-deep-equal": "^3.1.3",
"multicast-dns": "^7.2.5"
}
},
- "node_modules/bonjour-service/node_modules/array-flatten": {
- "version": "2.1.2",
- "resolved": "https://registry.npmjs.org/array-flatten/-/array-flatten-2.1.2.tgz",
- "integrity": "sha512-hNfzcOV8W4NdualtqBFPyVO+54DSJuZGY9qT4pRroB6S9e3iiido2ISIC5h9R2sPJ8H3FHCIiEnsv1lPXO3KtQ=="
- },
"node_modules/boolbase": {
"version": "1.0.0",
"resolved": "https://registry.npmjs.org/boolbase/-/boolbase-1.0.0.tgz",
@@ -20145,30 +18460,12 @@
"resolved": "https://registry.npmjs.org/buffer-from/-/buffer-from-1.1.2.tgz",
"integrity": "sha512-E+XQCRwSbaaiChtv6k6Dwgc+bx+Bs6vuKJHHl5kox/BaKbhiXzqQOwK4cO22yElGp2OCmjwVhT3HmxgyPGnJfQ=="
},
- "node_modules/buffer-indexof-polyfill": {
- "version": "1.0.2",
- "resolved": "https://registry.npmjs.org/buffer-indexof-polyfill/-/buffer-indexof-polyfill-1.0.2.tgz",
- "integrity": "sha512-I7wzHwA3t1/lwXQh+A5PbNvJxgfo5r3xulgpYDB5zckTu/Z9oUK9biouBKQUjEqzaz3HnAT6TYoovmE+GqSf7A==",
- "dev": true,
- "engines": {
- "node": ">=0.10"
- }
- },
"node_modules/buffer-xor": {
"version": "1.0.3",
"resolved": "https://registry.npmjs.org/buffer-xor/-/buffer-xor-1.0.3.tgz",
"integrity": "sha512-571s0T7nZWK6vB67HI5dyUF7wXiNcfaPPPTl6zYCNApANjIvYJTg7hlud/+cJpdAhS7dVzqMLmfhfHR3rAcOjQ==",
"dev": true
},
- "node_modules/buffers": {
- "version": "0.1.1",
- "resolved": "https://registry.npmjs.org/buffers/-/buffers-0.1.1.tgz",
- "integrity": "sha512-9q/rDEGSb/Qsvv2qvzIzdluL5k7AaJOTrw23z9reQthrbF7is4CtlT0DXyO1oei2DCp4uojjzQ7igaSHp1kAEQ==",
- "dev": true,
- "engines": {
- "node": ">=0.2.0"
- }
- },
"node_modules/builtin-modules": {
"version": "3.3.0",
"resolved": "https://registry.npmjs.org/builtin-modules/-/builtin-modules-3.3.0.tgz",
@@ -20210,6 +18507,7 @@
"resolved": "https://registry.npmjs.org/byte-size/-/byte-size-8.1.1.tgz",
"integrity": "sha512-tUkzZWK0M/qdoLEqikxBWe4kumyuwjl3HO6zHTr4yEI23EojPtLYXdG1+AQY7MN0cGyNDvEaJ8wiYQm6P2bPxg==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=12.17"
}
@@ -20517,25 +18815,20 @@
"resolved": "https://registry.npmjs.org/ccount/-/ccount-1.0.3.tgz",
"integrity": "sha512-Jt9tIBkRc9POUof7QA/VwWd+58fKkEEfI+/t1/eOlxKM7ZhrczNzMFefge7Ai+39y1pR/pP6cI19guHy3FSLmw=="
},
- "node_modules/chainsaw": {
- "version": "0.1.0",
- "resolved": "https://registry.npmjs.org/chainsaw/-/chainsaw-0.1.0.tgz",
- "integrity": "sha512-75kWfWt6MEKNC8xYXIdRpDehRYY/tNSgwKaJq+dbbDcxORuVrrQ+SEHoWsniVn9XPYfP4gmdWIeDk/4YNp1rNQ==",
+ "node_modules/chai": {
+ "version": "5.1.2",
+ "resolved": "https://registry.npmjs.org/chai/-/chai-5.1.2.tgz",
+ "integrity": "sha512-aGtmf24DW6MLHHG5gCx4zaI3uBq3KRtxeVs0DjFH6Z0rDNbsvTxFASFvdj79pxjxZ8/5u3PIiN3IwEIQkiiuPw==",
"dev": true,
"dependencies": {
- "traverse": ">=0.3.0 <0.4"
+ "assertion-error": "^2.0.1",
+ "check-error": "^2.1.1",
+ "deep-eql": "^5.0.1",
+ "loupe": "^3.1.0",
+ "pathval": "^2.0.0"
},
"engines": {
- "node": "*"
- }
- },
- "node_modules/chainsaw/node_modules/traverse": {
- "version": "0.3.9",
- "resolved": "https://registry.npmjs.org/traverse/-/traverse-0.3.9.tgz",
- "integrity": "sha512-iawgk0hLP3SxGKDfnDJf8wTz4p2qImnyihM5Hh/sGvQ3K37dPi/w8sRhdNIxYA1TwFwc5mDhIJq+O0RsvXBKdQ==",
- "dev": true,
- "engines": {
- "node": "*"
+ "node": ">=12"
}
},
"node_modules/chalk": {
@@ -20624,6 +18917,15 @@
"resolved": "https://registry.npmjs.org/chardet/-/chardet-0.7.0.tgz",
"integrity": "sha512-mT8iDcrh03qDGRRmoA2hmBJnxpllMR+0/0qlzjqZES6NdiWDcZkCNAk4rPFZ9Q85r27unkiNNg8ZOiwZXBHwcA=="
},
+ "node_modules/check-error": {
+ "version": "2.1.1",
+ "resolved": "https://registry.npmjs.org/check-error/-/check-error-2.1.1.tgz",
+ "integrity": "sha512-OAlb+T7V4Op9OwdkjmguYRqncdlx5JiofwOAUkmTF+jNdHwzTaTs4sRAGpzLF3oOz5xAyDGrPgeIDFQmDOTiJw==",
+ "dev": true,
+ "engines": {
+ "node": ">= 16"
+ }
+ },
"node_modules/check-node-version": {
"version": "4.1.0",
"resolved": "https://registry.npmjs.org/check-node-version/-/check-node-version-4.1.0.tgz",
@@ -20773,6 +19075,8 @@
"version": "0.4.16",
"resolved": "https://registry.npmjs.org/chromium-bidi/-/chromium-bidi-0.4.16.tgz",
"integrity": "sha512-7ZbXdWERxRxSwo3txsBjjmc/NLxqb1Bk30mRb0BMS4YIaiV6zvKZqL/UAH+DdqcDYayDWk2n/y8klkBDODrPvA==",
+ "dev": true,
+ "license": "Apache-2.0",
"dependencies": {
"mitt": "3.0.0"
},
@@ -20780,6 +19084,13 @@
"devtools-protocol": "*"
}
},
+ "node_modules/chromium-bidi/node_modules/mitt": {
+ "version": "3.0.0",
+ "resolved": "https://registry.npmjs.org/mitt/-/mitt-3.0.0.tgz",
+ "integrity": "sha512-7dX2/10ITVyqh4aOSVI9gdape+t9l2/8QxHrFmUXu4EEUpdlxl6RudZUPZoc+zuY2hk1j7XxVroIVIan/pD/SQ==",
+ "dev": true,
+ "license": "MIT"
+ },
"node_modules/chromium-edge-launcher": {
"version": "1.0.0",
"resolved": "https://registry.npmjs.org/chromium-edge-launcher/-/chromium-edge-launcher-1.0.0.tgz",
@@ -20804,6 +19115,27 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
+ "node_modules/chromium-edge-launcher/node_modules/glob": {
+ "version": "7.2.3",
+ "resolved": "https://registry.npmjs.org/glob/-/glob-7.2.3.tgz",
+ "integrity": "sha512-nFR0zLpU2YCaRxwoCJvL6UvCH2JFyFVIvwTLsIf21AuHlMskA1hhTdk+LlYJtOlYt9v6dvszD2BGRqBL+iQK9Q==",
+ "deprecated": "Glob versions prior to v9 are no longer supported",
+ "license": "ISC",
+ "dependencies": {
+ "fs.realpath": "^1.0.0",
+ "inflight": "^1.0.4",
+ "inherits": "2",
+ "minimatch": "^3.1.1",
+ "once": "^1.3.0",
+ "path-is-absolute": "^1.0.0"
+ },
+ "engines": {
+ "node": "*"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
+ }
+ },
"node_modules/chromium-edge-launcher/node_modules/is-wsl": {
"version": "2.2.0",
"resolved": "https://registry.npmjs.org/is-wsl/-/is-wsl-2.2.0.tgz",
@@ -20826,6 +19158,22 @@
"node": ">=10"
}
},
+ "node_modules/chromium-edge-launcher/node_modules/rimraf": {
+ "version": "3.0.2",
+ "resolved": "https://registry.npmjs.org/rimraf/-/rimraf-3.0.2.tgz",
+ "integrity": "sha512-JZkJMZkAGFFPP2YqXZXPbMlMBgsxzE8ILs4lMIX/2o0L9UBw9O/Y3o6wFw/i9YLapcUJWwqbi3kdxIPdC62TIA==",
+ "deprecated": "Rimraf versions prior to v4 are no longer supported",
+ "license": "ISC",
+ "dependencies": {
+ "glob": "^7.1.3"
+ },
+ "bin": {
+ "rimraf": "bin.js"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
+ }
+ },
"node_modules/ci-info": {
"version": "3.9.0",
"resolved": "https://registry.npmjs.org/ci-info/-/ci-info-3.9.0.tgz",
@@ -20850,24 +19198,6 @@
"safe-buffer": "^5.0.1"
}
},
- "node_modules/citty": {
- "version": "0.1.6",
- "resolved": "https://registry.npmjs.org/citty/-/citty-0.1.6.tgz",
- "integrity": "sha512-tskPPKEs8D2KPafUypv2gxwJP8h/OaJmC82QQGGDQcHvXX43xF2VDACcJVmZ0EuSxkpO9Kc4MlrA3q0+FG58AQ==",
- "dev": true,
- "dependencies": {
- "consola": "^3.2.3"
- }
- },
- "node_modules/citty/node_modules/consola": {
- "version": "3.2.3",
- "resolved": "https://registry.npmjs.org/consola/-/consola-3.2.3.tgz",
- "integrity": "sha512-I5qxpzLv+sJhTVEoLYNcTW+bThDCPsit0vLNKShZx6rLtpilNpmmeTPaeqJb9ZE9dV3DGaeby6Vuhrw38WjeyQ==",
- "dev": true,
- "engines": {
- "node": "^14.18.0 || >=16.10.0"
- }
- },
"node_modules/cjs-module-lexer": {
"version": "1.2.3",
"resolved": "https://registry.npmjs.org/cjs-module-lexer/-/cjs-module-lexer-1.2.3.tgz",
@@ -20958,50 +19288,6 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/cli-table3": {
- "version": "0.6.3",
- "resolved": "https://registry.npmjs.org/cli-table3/-/cli-table3-0.6.3.tgz",
- "integrity": "sha512-w5Jac5SykAeZJKntOxJCrm63Eg5/4dhMWIcuTbo9rpE+brgaSZo0RuNJZeOyMgsUdhDeojvgyQLmjI+K50ZGyg==",
- "dev": true,
- "dependencies": {
- "string-width": "^4.2.0"
- },
- "engines": {
- "node": "10.* || >= 12.*"
- },
- "optionalDependencies": {
- "@colors/colors": "1.5.0"
- }
- },
- "node_modules/cli-table3/node_modules/emoji-regex": {
- "version": "8.0.0",
- "resolved": "https://registry.npmjs.org/emoji-regex/-/emoji-regex-8.0.0.tgz",
- "integrity": "sha512-MSjYzcWNOA0ewAHpz0MxpYFvwg6yjy1NG3xteoqz644VCo/RPgnr1/GGt+ic3iJTzQ8Eu3TdM14SawnVUmGE6A==",
- "dev": true
- },
- "node_modules/cli-table3/node_modules/is-fullwidth-code-point": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/is-fullwidth-code-point/-/is-fullwidth-code-point-3.0.0.tgz",
- "integrity": "sha512-zymm5+u+sCsSWyD9qNaejV3DFvhCKclKdizYaJUuHA83RLjb7nSuGnddCHGv0hk+KY7BMAlsWeK4Ueg6EV6XQg==",
- "dev": true,
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/cli-table3/node_modules/string-width": {
- "version": "4.2.3",
- "resolved": "https://registry.npmjs.org/string-width/-/string-width-4.2.3.tgz",
- "integrity": "sha512-wKyQRQpjJ0sIp62ErSZdGsjMJWsap5oRNihHhu6G7JVO/9jIB6UyevL+tXuOqrng8j/cxKTWyWUwvSTriiZz/g==",
- "dev": true,
- "dependencies": {
- "emoji-regex": "^8.0.0",
- "is-fullwidth-code-point": "^3.0.0",
- "strip-ansi": "^6.0.1"
- },
- "engines": {
- "node": ">=8"
- }
- },
"node_modules/cli-truncate": {
"version": "0.2.1",
"resolved": "https://registry.npmjs.org/cli-truncate/-/cli-truncate-0.2.1.tgz",
@@ -21161,10 +19447,11 @@
}
},
"node_modules/cmd-shim": {
- "version": "6.0.1",
- "resolved": "https://registry.npmjs.org/cmd-shim/-/cmd-shim-6.0.1.tgz",
- "integrity": "sha512-S9iI9y0nKR4hwEQsVWpyxld/6kRfGepGfzff83FcaiEBpmvlbA2nnGe7Cylgrx2f/p1P5S5wpRm9oL8z1PbS3Q==",
+ "version": "6.0.3",
+ "resolved": "https://registry.npmjs.org/cmd-shim/-/cmd-shim-6.0.3.tgz",
+ "integrity": "sha512-FMabTRlc5t5zjdenF6mS0MBeFZm0XqHqeOkcskKFb/LYCcRQ5fVgLOHVc4Lq9CqABd9zhjwPjMBCJvMCziSVtA==",
"dev": true,
+ "license": "ISC",
"engines": {
"node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
@@ -21304,6 +19591,7 @@
"resolved": "https://registry.npmjs.org/columnify/-/columnify-1.6.0.tgz",
"integrity": "sha512-lomjuFZKfM6MSAnV9aCZC9sc0qGbmZdfygNv+nCpqVkSKdCxCklLtd16O0EILGkImHw9ZpHkAnHaB+8Zxq5W6Q==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"strip-ansi": "^6.0.1",
"wcwidth": "^1.0.0"
@@ -21344,6 +19632,13 @@
"node": ">= 12.0.0"
}
},
+ "node_modules/common-ancestor-path": {
+ "version": "1.0.1",
+ "resolved": "https://registry.npmjs.org/common-ancestor-path/-/common-ancestor-path-1.0.1.tgz",
+ "integrity": "sha512-L3sHRo1pXXEqX8VU28kfgUY+YGsk09hPqZiZmLacNib6XNTCM8ubYeT7ryXQw8asB1sKgcU5lkB7ONug08aB8w==",
+ "dev": true,
+ "license": "ISC"
+ },
"node_modules/common-path-prefix": {
"version": "3.0.0",
"resolved": "https://registry.npmjs.org/common-path-prefix/-/common-path-prefix-3.0.0.tgz",
@@ -21359,6 +19654,7 @@
"resolved": "https://registry.npmjs.org/compare-func/-/compare-func-2.0.0.tgz",
"integrity": "sha512-zHig5N+tPWARooBnb0Zx1MFcdfpyJrfTJ3Y5L+IFvUm8rM74hHz66z0gw0x4tijh5CorKkKUCnW82R2vmpeCRA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"array-ify": "^1.0.0",
"dot-prop": "^5.1.0"
@@ -21384,10 +19680,11 @@
"dev": true
},
"node_modules/compress-commons": {
- "version": "5.0.1",
- "resolved": "https://registry.npmjs.org/compress-commons/-/compress-commons-5.0.1.tgz",
- "integrity": "sha512-MPh//1cERdLtqwO3pOFLeXtpuai0Y2WCd5AhtKxznqM7WtaMYaOEMSgn45d9D10sIHSfIKE603HlOp8OPGrvag==",
+ "version": "5.0.3",
+ "resolved": "https://registry.npmjs.org/compress-commons/-/compress-commons-5.0.3.tgz",
+ "integrity": "sha512-/UIcLWvwAQyVibgpQDPtfNM3SvqN7G9elAPAV7GM0L53EbNWwWiCsWtK8Fwed/APEbptPHXs5PuW+y8Bq8lFTA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"crc-32": "^1.2.0",
"crc32-stream": "^5.0.0",
@@ -21403,6 +19700,7 @@
"resolved": "https://registry.npmjs.org/readable-stream/-/readable-stream-3.6.2.tgz",
"integrity": "sha512-9u/sniCrY3D5WdsERHzHE4G2YCXqoG5FTHUiCC4SIbr6XcLZBY05ya9EKjYek9O5xOAwjGq+1JdGBAS7Q9ScoA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"inherits": "^2.0.3",
"string_decoder": "^1.1.1",
@@ -21737,15 +20035,16 @@
}
},
"node_modules/conventional-changelog-angular": {
- "version": "6.0.0",
- "resolved": "https://registry.npmjs.org/conventional-changelog-angular/-/conventional-changelog-angular-6.0.0.tgz",
- "integrity": "sha512-6qLgrBF4gueoC7AFVHu51nHL9pF9FRjXrH+ceVf7WmAfH3gs+gEYOkvxhjMPjZu57I4AGUGoNTY8V7Hrgf1uqg==",
+ "version": "7.0.0",
+ "resolved": "https://registry.npmjs.org/conventional-changelog-angular/-/conventional-changelog-angular-7.0.0.tgz",
+ "integrity": "sha512-ROjNchA9LgfNMTTFSIWPzebCwOGFdgkEq45EnvvrmSLvCtAw0HSmrCs7/ty+wAeYUZyNay0YMUNYFTRL72PkBQ==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"compare-func": "^2.0.0"
},
"engines": {
- "node": ">=14"
+ "node": ">=16"
}
},
"node_modules/conventional-changelog-core": {
@@ -21753,6 +20052,7 @@
"resolved": "https://registry.npmjs.org/conventional-changelog-core/-/conventional-changelog-core-5.0.1.tgz",
"integrity": "sha512-Rvi5pH+LvgsqGwZPZ3Cq/tz4ty7mjijhr3qR4m9IBXNbxGGYgTVVO+duXzz9aArmHxFtwZ+LRkrNIMDQzgoY4A==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"add-stream": "^1.0.0",
"conventional-changelog-writer": "^6.0.0",
@@ -21775,6 +20075,7 @@
"resolved": "https://registry.npmjs.org/load-json-file/-/load-json-file-4.0.0.tgz",
"integrity": "sha512-Kx8hMakjX03tiGTLAIdJ+lL0htKnXjEZN6hk/tozf/WOuYGdZBJrZ+rCJRbVCugsjB3jMLn9746NsQIf5VjBMw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"graceful-fs": "^4.1.2",
"parse-json": "^4.0.0",
@@ -21790,6 +20091,7 @@
"resolved": "https://registry.npmjs.org/normalize-package-data/-/normalize-package-data-3.0.3.tgz",
"integrity": "sha512-p2W1sgqij3zMMyRC067Dg16bfzVH+w7hyegmpIvZ4JNjqtGOVAIvLmjBx3yP7YTe9vKJgkoNOPjwQGogDoMXFA==",
"dev": true,
+ "license": "BSD-2-Clause",
"dependencies": {
"hosted-git-info": "^4.0.1",
"is-core-module": "^2.5.0",
@@ -21805,6 +20107,7 @@
"resolved": "https://registry.npmjs.org/parse-json/-/parse-json-4.0.0.tgz",
"integrity": "sha512-aOIos8bujGN93/8Ox/jPLh7RwVnPEysynVFE+fQZyg6jKELEHwzgKdLRFHUgXJL6kylijVSBC4BvN9OmsB48Rw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"error-ex": "^1.3.1",
"json-parse-better-errors": "^1.0.1"
@@ -21818,6 +20121,7 @@
"resolved": "https://registry.npmjs.org/pify/-/pify-3.0.0.tgz",
"integrity": "sha512-C3FsVNH1udSEX48gGX1xfvwTWfsYWj5U+8/uK15BGzIGrKoUpghX8hWZwa/OFnakBiiVNmBvemTJR5mcy7iPcg==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=4"
}
@@ -21827,6 +20131,7 @@
"resolved": "https://registry.npmjs.org/read-pkg/-/read-pkg-3.0.0.tgz",
"integrity": "sha512-BLq/cCO9two+lBgiTYNqD6GdtK8s4NpaWrl6/rCO9w0TUS8oJl7cmToOZfRYllKTISY6nt1U7jQ53brmKqY6BA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"load-json-file": "^4.0.0",
"normalize-package-data": "^2.3.2",
@@ -21841,6 +20146,7 @@
"resolved": "https://registry.npmjs.org/read-pkg-up/-/read-pkg-up-3.0.0.tgz",
"integrity": "sha512-YFzFrVvpC6frF1sz8psoHDBGF7fLPc+llq/8NB43oagqWkx8ar5zYtsTORtOjw9W2RHLpWP+zTWwBvf1bCmcSw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"find-up": "^2.0.0",
"read-pkg": "^3.0.0"
@@ -21853,13 +20159,15 @@
"version": "2.8.9",
"resolved": "https://registry.npmjs.org/hosted-git-info/-/hosted-git-info-2.8.9.tgz",
"integrity": "sha512-mxIDAb9Lsm6DoOJ7xH+5+X4y1LU/4Hi50L9C5sIswK3JzULS4bwk1FvjdBgvYR4bzT4tuUQiC15FE2f5HbLvYw==",
- "dev": true
+ "dev": true,
+ "license": "ISC"
},
"node_modules/conventional-changelog-core/node_modules/read-pkg/node_modules/normalize-package-data": {
"version": "2.5.0",
"resolved": "https://registry.npmjs.org/normalize-package-data/-/normalize-package-data-2.5.0.tgz",
"integrity": "sha512-/5CMN3T0R4XTj4DcGaexo+roZSdSFW/0AOOTROrjxzCG1wrWXEsGbRKevjlIL+ZDE4sZlJr5ED4YW0yqmkK+eA==",
"dev": true,
+ "license": "BSD-2-Clause",
"dependencies": {
"hosted-git-info": "^2.1.4",
"resolve": "^1.10.0",
@@ -21872,6 +20180,7 @@
"resolved": "https://registry.npmjs.org/semver/-/semver-5.7.2.tgz",
"integrity": "sha512-cBznnQ9KjJqU67B52RMC65CMarK2600WFnbkcaiwWq3xy/5haFJlshgnpjovMVJ+Hff49d8GEn0b87C5pDQ10g==",
"dev": true,
+ "license": "ISC",
"bin": {
"semver": "bin/semver"
}
@@ -21881,6 +20190,7 @@
"resolved": "https://registry.npmjs.org/strip-bom/-/strip-bom-3.0.0.tgz",
"integrity": "sha512-vavAMRXOgBVNF6nyEEmL3DBK19iRpDcoIwW+swQ+CbGiu7lju6t+JklA1MHweoWtadgt4ISVUsXLyDq34ddcwA==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=4"
}
@@ -21890,6 +20200,7 @@
"resolved": "https://registry.npmjs.org/conventional-changelog-preset-loader/-/conventional-changelog-preset-loader-3.0.0.tgz",
"integrity": "sha512-qy9XbdSLmVnwnvzEisjxdDiLA4OmV3o8db+Zdg4WiFw14fP3B6XNz98X0swPPpkTd/pc1K7+adKgEDM1JCUMiA==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=14"
}
@@ -21899,6 +20210,7 @@
"resolved": "https://registry.npmjs.org/conventional-changelog-writer/-/conventional-changelog-writer-6.0.1.tgz",
"integrity": "sha512-359t9aHorPw+U+nHzUXHS5ZnPBOizRxfQsWT5ZDHBfvfxQOAik+yfuhKXG66CN5LEWPpMNnIMHUTCKeYNprvHQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"conventional-commits-filter": "^3.0.0",
"dateformat": "^3.0.3",
@@ -21920,6 +20232,7 @@
"resolved": "https://registry.npmjs.org/conventional-commits-filter/-/conventional-commits-filter-3.0.0.tgz",
"integrity": "sha512-1ymej8b5LouPx9Ox0Dw/qAO2dVdfpRFq28e5Y0jJEU8ZrLdy0vOSkkIInwmxErFGhg6SALro60ZrwYFVTUDo4Q==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"lodash.ismatch": "^4.4.0",
"modify-values": "^1.0.1"
@@ -21933,6 +20246,7 @@
"resolved": "https://registry.npmjs.org/conventional-commits-parser/-/conventional-commits-parser-4.0.0.tgz",
"integrity": "sha512-WRv5j1FsVM5FISJkoYMR6tPk07fkKT0UodruX4je86V4owk451yjXAKzKAPOs9l7y59E2viHUS9eQ+dfUA9NSg==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"is-text-path": "^1.0.1",
"JSONStream": "^1.3.5",
@@ -21951,6 +20265,7 @@
"resolved": "https://registry.npmjs.org/conventional-recommended-bump/-/conventional-recommended-bump-7.0.1.tgz",
"integrity": "sha512-Ft79FF4SlOFvX4PkwFDRnaNiIVX7YbmqGU0RwccUaiGvgp3S0a8ipR2/Qxk31vclDNM+GSdJOVs2KrsUCjblVA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"concat-stream": "^2.0.0",
"conventional-changelog-preset-loader": "^3.0.0",
@@ -21975,6 +20290,7 @@
"engines": [
"node >= 6.0"
],
+ "license": "MIT",
"dependencies": {
"buffer-from": "^1.0.0",
"inherits": "^2.0.3",
@@ -21987,6 +20303,7 @@
"resolved": "https://registry.npmjs.org/readable-stream/-/readable-stream-3.6.2.tgz",
"integrity": "sha512-9u/sniCrY3D5WdsERHzHE4G2YCXqoG5FTHUiCC4SIbr6XcLZBY05ya9EKjYek9O5xOAwjGq+1JdGBAS7Q9ScoA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"inherits": "^2.0.3",
"string_decoder": "^1.1.1",
@@ -22005,6 +20322,7 @@
"version": "0.5.0",
"resolved": "https://registry.npmjs.org/cookie/-/cookie-0.5.0.tgz",
"integrity": "sha512-YZ3GUyn/o8gfKJlnlX7g7xq4gyO6OSuhGPKaaGssGB2qgDUS0gPgtTvoyZLTt9Ab6dC4hfc9dV5arkvc/OCmrw==",
+ "license": "MIT",
"engines": {
"node": ">= 0.6"
}
@@ -22220,23 +20538,25 @@
}
},
"node_modules/core-js": {
- "version": "3.38.1",
- "resolved": "https://registry.npmjs.org/core-js/-/core-js-3.38.1.tgz",
- "integrity": "sha512-OP35aUorbU3Zvlx7pjsFdu1rGNnD4pgw/CWoYzRY3t2EzoVT7shKHY1dlAy3f41cGIO7ZDPQimhGFTlEYkG/Hw==",
+ "version": "3.39.0",
+ "resolved": "https://registry.npmjs.org/core-js/-/core-js-3.39.0.tgz",
+ "integrity": "sha512-raM0ew0/jJUqkJ0E6e8UDtl+y/7ktFivgWvqw8dNSQeNWoSDLvQ1H/RN3aPXB9tBd4/FhyR4RDPGhsNIMsAn7g==",
"hasInstallScript": true,
+ "license": "MIT",
"funding": {
"type": "opencollective",
"url": "https://opencollective.com/core-js"
}
},
"node_modules/core-js-builder": {
- "version": "3.38.1",
- "resolved": "https://registry.npmjs.org/core-js-builder/-/core-js-builder-3.38.1.tgz",
- "integrity": "sha512-SxxFz0xLPX+MMCC3QtRavu5rzhFe28QqiaQKko+25DrUEsPYbNasZACUkKDDGfInxJiUk/9/PhmHwIdyN4vSKA==",
+ "version": "3.39.0",
+ "resolved": "https://registry.npmjs.org/core-js-builder/-/core-js-builder-3.39.0.tgz",
+ "integrity": "sha512-XyqDy/xNDx3B+fSQmkBYmYlCYheI7YjxadD5Bje5MbrfjcZ/VMAQfKPPUBohEUUuLR/bdyYTjyXtbICkQeDHcQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "core-js": "3.38.1",
- "core-js-compat": "3.38.1",
+ "core-js": "3.39.0",
+ "core-js-compat": "3.39.0",
"mkdirp": ">=0.5.6 <1",
"webpack": ">=4.47.0 <5"
},
@@ -22493,17 +20813,70 @@
}
},
"node_modules/core-js-compat": {
- "version": "3.38.1",
- "resolved": "https://registry.npmjs.org/core-js-compat/-/core-js-compat-3.38.1.tgz",
- "integrity": "sha512-JRH6gfXxGmrzF3tZ57lFx97YARxCXPaMzPo6jELZhv88pBH5VXpQ+y0znKGlFnzuaihqhLbefxSJxWJMPtfDzw==",
+ "version": "3.39.0",
+ "resolved": "https://registry.npmjs.org/core-js-compat/-/core-js-compat-3.39.0.tgz",
+ "integrity": "sha512-VgEUx3VwlExr5no0tXlBt+silBvhTryPwCXRI2Id1PN8WTKu7MreethvddqOubrYxkFdv/RnYrqlv1sFNAUelw==",
+ "license": "MIT",
"dependencies": {
- "browserslist": "^4.23.3"
+ "browserslist": "^4.24.2"
},
"funding": {
"type": "opencollective",
"url": "https://opencollective.com/core-js"
}
},
+ "node_modules/core-js-compat/node_modules/browserslist": {
+ "version": "4.24.2",
+ "resolved": "https://registry.npmjs.org/browserslist/-/browserslist-4.24.2.tgz",
+ "integrity": "sha512-ZIc+Q62revdMcqC6aChtW4jz3My3klmCO1fEmINZY/8J3EpBg5/A/D0AKmBveUh6pgoeycoMkVMko84tuYS+Gg==",
+ "funding": [
+ {
+ "type": "opencollective",
+ "url": "https://opencollective.com/browserslist"
+ },
+ {
+ "type": "tidelift",
+ "url": "https://tidelift.com/funding/github/npm/browserslist"
+ },
+ {
+ "type": "github",
+ "url": "https://github.com/sponsors/ai"
+ }
+ ],
+ "license": "MIT",
+ "dependencies": {
+ "caniuse-lite": "^1.0.30001669",
+ "electron-to-chromium": "^1.5.41",
+ "node-releases": "^2.0.18",
+ "update-browserslist-db": "^1.1.1"
+ },
+ "bin": {
+ "browserslist": "cli.js"
+ },
+ "engines": {
+ "node": "^6 || ^7 || ^8 || ^9 || ^10 || ^11 || ^12 || >=13.7"
+ }
+ },
+ "node_modules/core-js-compat/node_modules/caniuse-lite": {
+ "version": "1.0.30001687",
+ "resolved": "https://registry.npmjs.org/caniuse-lite/-/caniuse-lite-1.0.30001687.tgz",
+ "integrity": "sha512-0S/FDhf4ZiqrTUiQ39dKeUjYRjkv7lOZU1Dgif2rIqrTzX/1wV2hfKu9TOm1IHkdSijfLswxTFzl/cvir+SLSQ==",
+ "funding": [
+ {
+ "type": "opencollective",
+ "url": "https://opencollective.com/browserslist"
+ },
+ {
+ "type": "tidelift",
+ "url": "https://tidelift.com/funding/github/npm/caniuse-lite"
+ },
+ {
+ "type": "github",
+ "url": "https://github.com/sponsors/ai"
+ }
+ ],
+ "license": "CC-BY-4.0"
+ },
"node_modules/core-js-pure": {
"version": "3.31.0",
"resolved": "https://registry.npmjs.org/core-js-pure/-/core-js-pure-3.31.0.tgz",
@@ -22547,6 +20920,7 @@
"resolved": "https://registry.npmjs.org/crc-32/-/crc-32-1.2.2.tgz",
"integrity": "sha512-ROmzCKrTnOwybPcJApAA6WBWij23HVfGVNKqqrZpuyZOHqK2CwHSvpGuyt/UNNvaIjEd8X5IFGp4Mh+Ie1IHJQ==",
"dev": true,
+ "license": "Apache-2.0",
"bin": {
"crc32": "bin/crc32.njs"
},
@@ -22555,10 +20929,11 @@
}
},
"node_modules/crc32-stream": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/crc32-stream/-/crc32-stream-5.0.0.tgz",
- "integrity": "sha512-B0EPa1UK+qnpBZpG+7FgPCu0J2ETLpXq09o9BkLkEAhdB6Z61Qo4pJ3JYu0c+Qi+/SAL7QThqnzS06pmSSyZaw==",
+ "version": "5.0.1",
+ "resolved": "https://registry.npmjs.org/crc32-stream/-/crc32-stream-5.0.1.tgz",
+ "integrity": "sha512-lO1dFui+CEUh/ztYIpgpKItKW9Bb4NWakCRJrnqAbFIYD+OZAwb2VfD5T5eXMw2FNcsDHkQcNl/Wh3iVXYwU6g==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"crc-32": "^1.2.0",
"readable-stream": "^3.4.0"
@@ -22572,6 +20947,7 @@
"resolved": "https://registry.npmjs.org/readable-stream/-/readable-stream-3.6.2.tgz",
"integrity": "sha512-9u/sniCrY3D5WdsERHzHE4G2YCXqoG5FTHUiCC4SIbr6XcLZBY05ya9EKjYek9O5xOAwjGq+1JdGBAS7Q9ScoA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"inherits": "^2.0.3",
"string_decoder": "^1.1.1",
@@ -22631,38 +21007,91 @@
"dev": true
},
"node_modules/cross-env": {
- "version": "3.2.4",
- "resolved": "https://registry.npmjs.org/cross-env/-/cross-env-3.2.4.tgz",
- "integrity": "sha512-T8AFEAiuJ0w53ou6rnu3Fipaiu1W6ZO9GYfd33uxe1kAIiXM0fD8QnIm7orcJBOt7WQC5Ply63E7WZW/jSM+FA==",
+ "version": "7.0.3",
+ "resolved": "https://registry.npmjs.org/cross-env/-/cross-env-7.0.3.tgz",
+ "integrity": "sha512-+/HKd6EgcQCJGh2PSjZuUitQBQynKor4wrFbRg4DtAgS1aWO+gU52xpH7M9ScGgXSYmAVS9bIJ8EzuaGw0oNAw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "cross-spawn": "^5.1.0",
- "is-windows": "^1.0.0"
+ "cross-spawn": "^7.0.1"
},
"bin": {
- "cross-env": "dist/bin/cross-env.js"
+ "cross-env": "src/bin/cross-env.js",
+ "cross-env-shell": "src/bin/cross-env-shell.js"
},
"engines": {
- "node": ">=4.0"
+ "node": ">=10.14",
+ "npm": ">=6",
+ "yarn": ">=1"
}
},
"node_modules/cross-fetch": {
"version": "4.0.0",
"resolved": "https://registry.npmjs.org/cross-fetch/-/cross-fetch-4.0.0.tgz",
"integrity": "sha512-e4a5N8lVvuLgAWgnCrLr2PP0YyDOTHa9H/Rj54dirp61qXnNq46m82bRhNqIA5VccJtWBvPTFRV3TtvHUKPB1g==",
+ "dev": true,
"license": "MIT",
"dependencies": {
"node-fetch": "^2.6.12"
}
},
"node_modules/cross-spawn": {
- "version": "5.1.0",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-5.1.0.tgz",
- "integrity": "sha512-pTgQJ5KC0d2hcY8eyL1IzlBPYjTkyH72XRZPnLyKus2mBfNjQs3klqbJU2VILqZryAZUt9JOb3h/mWMy23/f5A==",
+ "version": "7.0.6",
+ "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-7.0.6.tgz",
+ "integrity": "sha512-uV2QOWP2nWzsy2aMp8aRibhi9dlzF5Hgh5SHaB9OiTGEyDTiJJyx0uy51QXdyWbtAHNua4XJzUKca3OzKUd3vA==",
+ "license": "MIT",
"dependencies": {
- "lru-cache": "^4.0.1",
- "shebang-command": "^1.2.0",
- "which": "^1.2.9"
+ "path-key": "^3.1.0",
+ "shebang-command": "^2.0.0",
+ "which": "^2.0.1"
+ },
+ "engines": {
+ "node": ">= 8"
+ }
+ },
+ "node_modules/cross-spawn/node_modules/path-key": {
+ "version": "3.1.1",
+ "resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
+ "integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
+ "license": "MIT",
+ "engines": {
+ "node": ">=8"
+ }
+ },
+ "node_modules/cross-spawn/node_modules/shebang-command": {
+ "version": "2.0.0",
+ "resolved": "https://registry.npmjs.org/shebang-command/-/shebang-command-2.0.0.tgz",
+ "integrity": "sha512-kHxr2zZpYtdmrN1qDjrrX/Z1rR1kG8Dx+gkpK1G4eXmvXswmcE1hTWBWYUzlraYw1/yZp6YuDY77YtvbN0dmDA==",
+ "license": "MIT",
+ "dependencies": {
+ "shebang-regex": "^3.0.0"
+ },
+ "engines": {
+ "node": ">=8"
+ }
+ },
+ "node_modules/cross-spawn/node_modules/shebang-regex": {
+ "version": "3.0.0",
+ "resolved": "https://registry.npmjs.org/shebang-regex/-/shebang-regex-3.0.0.tgz",
+ "integrity": "sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==",
+ "license": "MIT",
+ "engines": {
+ "node": ">=8"
+ }
+ },
+ "node_modules/cross-spawn/node_modules/which": {
+ "version": "2.0.2",
+ "resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
+ "integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
+ "license": "ISC",
+ "dependencies": {
+ "isexe": "^2.0.0"
+ },
+ "bin": {
+ "node-which": "bin/node-which"
+ },
+ "engines": {
+ "node": ">= 8"
}
},
"node_modules/crypto-browserify": {
@@ -23077,6 +21506,7 @@
"resolved": "https://registry.npmjs.org/dargs/-/dargs-7.0.0.tgz",
"integrity": "sha512-2iy1EkLdlBzQGvbweYRFxmFath8+K7+AKB0TlhHWkNuH+TmovaMH/Wp7V7R4u7f4SnX3OgLsU9t1NI9ioDnUpg==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=8"
}
@@ -23146,6 +21576,7 @@
"resolved": "https://registry.npmjs.org/dateformat/-/dateformat-3.0.3.tgz",
"integrity": "sha512-jyCETtSl3VMZMWeRo7iY1FL19ges1t55hMo5yaam4Jrsm5EPL89UQkoQRyiI+Yf4k8r2ZpdngkV8hr1lIdjb3Q==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": "*"
}
@@ -23249,6 +21680,15 @@
"integrity": "sha512-Q6fKUPqnAHAyhiUgFU7BUzLiv0kd8saH9al7tnu5Q/okj6dnupxyTgFIBjVzJATdfIAm9NAsvXNzjaKa+bxVyA==",
"dev": true
},
+ "node_modules/deep-eql": {
+ "version": "5.0.2",
+ "resolved": "https://registry.npmjs.org/deep-eql/-/deep-eql-5.0.2.tgz",
+ "integrity": "sha512-h5k/5U50IJJFpzfL6nO9jaaumfjO/f2NjK/oYB2Djzm4p9L+3T9qWpZqZ2hAbLPuuYq9wrU08WQyBTL5GbPk5Q==",
+ "dev": true,
+ "engines": {
+ "node": ">=6"
+ }
+ },
"node_modules/deep-extend": {
"version": "0.6.0",
"resolved": "https://registry.npmjs.org/deep-extend/-/deep-extend-0.6.0.tgz",
@@ -23281,6 +21721,7 @@
"resolved": "https://registry.npmjs.org/deepmerge-ts/-/deepmerge-ts-5.1.0.tgz",
"integrity": "sha512-eS8dRJOckyo9maw9Tu5O5RUi/4inFLrnoLkBe3cPfDMx3WZioXtmOew4TXQaxq7Rhl4xjDtR7c6x8nNTxOvbFw==",
"dev": true,
+ "license": "BSD-3-Clause",
"engines": {
"node": ">=16.0.0"
}
@@ -23317,19 +21758,6 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/default-browser/node_modules/cross-spawn": {
- "version": "7.0.3",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-7.0.3.tgz",
- "integrity": "sha512-iRDPJKUPVEND7dHPO8rkbOnPpyDygcDFtWjpeWNCgy8WP2rXcxXL8TskReQl6OrB2G7+UJrags1q15Fudc7G6w==",
- "dependencies": {
- "path-key": "^3.1.0",
- "shebang-command": "^2.0.0",
- "which": "^2.0.1"
- },
- "engines": {
- "node": ">= 8"
- }
- },
"node_modules/default-browser/node_modules/execa": {
"version": "7.2.0",
"resolved": "https://registry.npmjs.org/execa/-/execa-7.2.0.tgz",
@@ -23432,33 +21860,6 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/default-browser/node_modules/path-key": {
- "version": "3.1.1",
- "resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
- "integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/default-browser/node_modules/shebang-command": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/shebang-command/-/shebang-command-2.0.0.tgz",
- "integrity": "sha512-kHxr2zZpYtdmrN1qDjrrX/Z1rR1kG8Dx+gkpK1G4eXmvXswmcE1hTWBWYUzlraYw1/yZp6YuDY77YtvbN0dmDA==",
- "dependencies": {
- "shebang-regex": "^3.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/default-browser/node_modules/shebang-regex": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/shebang-regex/-/shebang-regex-3.0.0.tgz",
- "integrity": "sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==",
- "engines": {
- "node": ">=8"
- }
- },
"node_modules/default-browser/node_modules/strip-final-newline": {
"version": "3.0.0",
"resolved": "https://registry.npmjs.org/strip-final-newline/-/strip-final-newline-3.0.0.tgz",
@@ -23470,24 +21871,11 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/default-browser/node_modules/which": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
- "integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
- "dependencies": {
- "isexe": "^2.0.0"
- },
- "bin": {
- "node-which": "bin/node-which"
- },
- "engines": {
- "node": ">= 8"
- }
- },
"node_modules/default-gateway": {
"version": "6.0.3",
"resolved": "https://registry.npmjs.org/default-gateway/-/default-gateway-6.0.3.tgz",
"integrity": "sha512-fwSOJsbbNzZ/CUFpqFBqYfYNLj1NbMPm8MMCIzHjC83iSJRBEGmDUxU+WP661BaBQImeC2yHwXtz+P/O9o+XEg==",
+ "license": "BSD-2-Clause",
"dependencies": {
"execa": "^5.0.0"
},
@@ -23495,23 +21883,11 @@
"node": ">= 10"
}
},
- "node_modules/default-gateway/node_modules/cross-spawn": {
- "version": "7.0.3",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-7.0.3.tgz",
- "integrity": "sha512-iRDPJKUPVEND7dHPO8rkbOnPpyDygcDFtWjpeWNCgy8WP2rXcxXL8TskReQl6OrB2G7+UJrags1q15Fudc7G6w==",
- "dependencies": {
- "path-key": "^3.1.0",
- "shebang-command": "^2.0.0",
- "which": "^2.0.1"
- },
- "engines": {
- "node": ">= 8"
- }
- },
"node_modules/default-gateway/node_modules/execa": {
"version": "5.1.1",
"resolved": "https://registry.npmjs.org/execa/-/execa-5.1.1.tgz",
"integrity": "sha512-8uSpZZocAZRBAPIEINJj3Lo9HyGitllczc27Eh5YYojjMFMn8yHMDMaUHE2Jqfq05D/wucwI4JGURyXt1vchyg==",
+ "license": "MIT",
"dependencies": {
"cross-spawn": "^7.0.3",
"get-stream": "^6.0.0",
@@ -23534,6 +21910,7 @@
"version": "6.0.1",
"resolved": "https://registry.npmjs.org/get-stream/-/get-stream-6.0.1.tgz",
"integrity": "sha512-ts6Wi+2j3jQjqi70w5AlN8DFnkSwC+MqmxEzdEALB2qXZYV3X/b1CTfgPLGJNMeAWxdPfU8FO1ms3NUfaHCPYg==",
+ "license": "MIT",
"engines": {
"node": ">=10"
},
@@ -23545,6 +21922,7 @@
"version": "2.1.0",
"resolved": "https://registry.npmjs.org/human-signals/-/human-signals-2.1.0.tgz",
"integrity": "sha512-B4FFZ6q/T2jhhksgkbEW3HBvWIfDW85snkQgawt07S7J5QXTk6BkNV+0yAeZrM5QpMAdYlocGoljn0sJ/WQkFw==",
+ "license": "Apache-2.0",
"engines": {
"node": ">=10.17.0"
}
@@ -23553,6 +21931,7 @@
"version": "2.0.1",
"resolved": "https://registry.npmjs.org/is-stream/-/is-stream-2.0.1.tgz",
"integrity": "sha512-hFoiJiTl63nn+kstHGBtewWSKnQLpyb155KHheA1l39uvtO9nWIop1p3udqPcUd/xbF1VLMO4n7OI6p7RbngDg==",
+ "license": "MIT",
"engines": {
"node": ">=8"
},
@@ -23564,6 +21943,7 @@
"version": "2.1.0",
"resolved": "https://registry.npmjs.org/mimic-fn/-/mimic-fn-2.1.0.tgz",
"integrity": "sha512-OqbOk5oEQeAZ8WXWydlu9HJjz9WVdEIvamMCcXmuqUYjTknH/sqsWvhQ3vgwKFRR1HpjvNBKQ37nbJgYzGqGcg==",
+ "license": "MIT",
"engines": {
"node": ">=6"
}
@@ -23572,6 +21952,7 @@
"version": "4.0.1",
"resolved": "https://registry.npmjs.org/npm-run-path/-/npm-run-path-4.0.1.tgz",
"integrity": "sha512-S48WzZW777zhNIrn7gxOlISNAqi9ZC/uQFnRdbeIHhZhCA6UqpkOT8T1G7BvfdgP4Er8gF4sUbaS0i7QvIfCWw==",
+ "license": "MIT",
"dependencies": {
"path-key": "^3.0.0"
},
@@ -23583,6 +21964,7 @@
"version": "5.1.2",
"resolved": "https://registry.npmjs.org/onetime/-/onetime-5.1.2.tgz",
"integrity": "sha512-kbpaSSGJTWdAY5KPVeMOKXSrPtr8C8C7wodJbcsd51jRnmD+GZu8Y0VoU6Dm5Z4vWr0Ig/1NKuWRKf7j5aaYSg==",
+ "license": "MIT",
"dependencies": {
"mimic-fn": "^2.1.0"
},
@@ -23597,43 +21979,11 @@
"version": "3.1.1",
"resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
"integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
+ "license": "MIT",
"engines": {
"node": ">=8"
}
},
- "node_modules/default-gateway/node_modules/shebang-command": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/shebang-command/-/shebang-command-2.0.0.tgz",
- "integrity": "sha512-kHxr2zZpYtdmrN1qDjrrX/Z1rR1kG8Dx+gkpK1G4eXmvXswmcE1hTWBWYUzlraYw1/yZp6YuDY77YtvbN0dmDA==",
- "dependencies": {
- "shebang-regex": "^3.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/default-gateway/node_modules/shebang-regex": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/shebang-regex/-/shebang-regex-3.0.0.tgz",
- "integrity": "sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==",
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/default-gateway/node_modules/which": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
- "integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
- "dependencies": {
- "isexe": "^2.0.0"
- },
- "bin": {
- "node-which": "bin/node-which"
- },
- "engines": {
- "node": ">= 8"
- }
- },
"node_modules/defaults": {
"version": "1.0.3",
"resolved": "https://registry.npmjs.org/defaults/-/defaults-1.0.3.tgz",
@@ -23715,16 +22065,11 @@
"node": ">= 0.4"
}
},
- "node_modules/defu": {
- "version": "6.1.4",
- "resolved": "https://registry.npmjs.org/defu/-/defu-6.1.4.tgz",
- "integrity": "sha512-mEQCMmwJu317oSz8CwdIOdwf3xMif1ttiM8LTufzc3g6kR+9Pe236twL8j3IYT1F7GfRgGcW6MWxzZjLIkuHIg==",
- "dev": true
- },
"node_modules/degenerator": {
"version": "5.0.1",
"resolved": "https://registry.npmjs.org/degenerator/-/degenerator-5.0.1.tgz",
"integrity": "sha512-TllpMR/t0M5sqCXfj85i4XaAzxmS5tVA16dqvdkMwGmzI+dXLXnw3J+3Vdv7VKw+ThlTMboK6i9rnZ6Nntj5CQ==",
+ "license": "MIT",
"dependencies": {
"ast-types": "^0.13.4",
"escodegen": "^2.1.0",
@@ -23738,6 +22083,7 @@
"version": "0.13.4",
"resolved": "https://registry.npmjs.org/ast-types/-/ast-types-0.13.4.tgz",
"integrity": "sha512-x1FCFnFifvYDDzTaLII71vG5uvDwgtmDTEVWAxrgeiR8VjMONcCXJx7E+USjDtHlwFmt9MysbqgF9b9Vjr6w+w==",
+ "license": "MIT",
"dependencies": {
"tslib": "^2.0.1"
},
@@ -23844,12 +22190,6 @@
"resolved": "https://registry.npmjs.org/delegate/-/delegate-3.2.0.tgz",
"integrity": "sha512-IofjkYBZaZivn0V8nnsMJGBr4jVLxHDheKSW88PyxS5QC4Vo9ZbZVvhzlSxY87fVq3STR6r+4cGepyHkcWOQSw=="
},
- "node_modules/delegates": {
- "version": "1.0.0",
- "resolved": "https://registry.npmjs.org/delegates/-/delegates-1.0.0.tgz",
- "integrity": "sha512-bd2L678uiWATM6m5Z1VzNCErI3jiGzt6HGY8OVICs40JQq/HALfbyNJmp0UDakEY4pMMaN0Ly5om/B1VI/+xfQ==",
- "dev": true
- },
"node_modules/denodeify": {
"version": "1.2.1",
"resolved": "https://registry.npmjs.org/denodeify/-/denodeify-1.2.1.tgz",
@@ -23915,10 +22255,11 @@
}
},
"node_modules/detect-libc": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/detect-libc/-/detect-libc-2.0.2.tgz",
- "integrity": "sha512-UX6sGumvvqSaXgdKGUsgZWqcUyIXZ/vZTrlRT/iobiKhGL0zL4d3osHj3uqllWJK+i+sixDS/3COVEOFbupFyw==",
+ "version": "2.0.3",
+ "resolved": "https://registry.npmjs.org/detect-libc/-/detect-libc-2.0.3.tgz",
+ "integrity": "sha512-bwy0MGW55bG41VqxxypOsdSdGqLwXPI/focwgTYCFMbdUiBAxLg9CFzG08sz2aqzknwiX7Hkl0bQENjg8iLByw==",
"dev": true,
+ "license": "Apache-2.0",
"optional": true,
"engines": {
"node": ">=8"
@@ -23942,187 +22283,10 @@
"resolved": "https://registry.npmjs.org/detect-node-es/-/detect-node-es-1.1.0.tgz",
"integrity": "sha512-ypdmJU/TbBby2Dxibuv7ZLW3Bs1QEmM7nHjEANfohJLvE0XVujisn1qPJcZxg+qDucsr+bP6fLD1rPS3AhJ7EQ=="
},
- "node_modules/detect-package-manager": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/detect-package-manager/-/detect-package-manager-2.0.1.tgz",
- "integrity": "sha512-j/lJHyoLlWi6G1LDdLgvUtz60Zo5GEj+sVYtTVXnYLDPuzgC3llMxonXym9zIwhhUII8vjdw0LXxavpLqTbl1A==",
- "dev": true,
- "dependencies": {
- "execa": "^5.1.1"
- },
- "engines": {
- "node": ">=12"
- }
- },
- "node_modules/detect-package-manager/node_modules/cross-spawn": {
- "version": "7.0.3",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-7.0.3.tgz",
- "integrity": "sha512-iRDPJKUPVEND7dHPO8rkbOnPpyDygcDFtWjpeWNCgy8WP2rXcxXL8TskReQl6OrB2G7+UJrags1q15Fudc7G6w==",
- "dev": true,
- "dependencies": {
- "path-key": "^3.1.0",
- "shebang-command": "^2.0.0",
- "which": "^2.0.1"
- },
- "engines": {
- "node": ">= 8"
- }
- },
- "node_modules/detect-package-manager/node_modules/execa": {
- "version": "5.1.1",
- "resolved": "https://registry.npmjs.org/execa/-/execa-5.1.1.tgz",
- "integrity": "sha512-8uSpZZocAZRBAPIEINJj3Lo9HyGitllczc27Eh5YYojjMFMn8yHMDMaUHE2Jqfq05D/wucwI4JGURyXt1vchyg==",
- "dev": true,
- "dependencies": {
- "cross-spawn": "^7.0.3",
- "get-stream": "^6.0.0",
- "human-signals": "^2.1.0",
- "is-stream": "^2.0.0",
- "merge-stream": "^2.0.0",
- "npm-run-path": "^4.0.1",
- "onetime": "^5.1.2",
- "signal-exit": "^3.0.3",
- "strip-final-newline": "^2.0.0"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/sindresorhus/execa?sponsor=1"
- }
- },
- "node_modules/detect-package-manager/node_modules/get-stream": {
- "version": "6.0.1",
- "resolved": "https://registry.npmjs.org/get-stream/-/get-stream-6.0.1.tgz",
- "integrity": "sha512-ts6Wi+2j3jQjqi70w5AlN8DFnkSwC+MqmxEzdEALB2qXZYV3X/b1CTfgPLGJNMeAWxdPfU8FO1ms3NUfaHCPYg==",
- "dev": true,
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/detect-package-manager/node_modules/human-signals": {
- "version": "2.1.0",
- "resolved": "https://registry.npmjs.org/human-signals/-/human-signals-2.1.0.tgz",
- "integrity": "sha512-B4FFZ6q/T2jhhksgkbEW3HBvWIfDW85snkQgawt07S7J5QXTk6BkNV+0yAeZrM5QpMAdYlocGoljn0sJ/WQkFw==",
- "dev": true,
- "engines": {
- "node": ">=10.17.0"
- }
- },
- "node_modules/detect-package-manager/node_modules/is-stream": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/is-stream/-/is-stream-2.0.1.tgz",
- "integrity": "sha512-hFoiJiTl63nn+kstHGBtewWSKnQLpyb155KHheA1l39uvtO9nWIop1p3udqPcUd/xbF1VLMO4n7OI6p7RbngDg==",
- "dev": true,
- "engines": {
- "node": ">=8"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/detect-package-manager/node_modules/mimic-fn": {
- "version": "2.1.0",
- "resolved": "https://registry.npmjs.org/mimic-fn/-/mimic-fn-2.1.0.tgz",
- "integrity": "sha512-OqbOk5oEQeAZ8WXWydlu9HJjz9WVdEIvamMCcXmuqUYjTknH/sqsWvhQ3vgwKFRR1HpjvNBKQ37nbJgYzGqGcg==",
- "dev": true,
- "engines": {
- "node": ">=6"
- }
- },
- "node_modules/detect-package-manager/node_modules/npm-run-path": {
- "version": "4.0.1",
- "resolved": "https://registry.npmjs.org/npm-run-path/-/npm-run-path-4.0.1.tgz",
- "integrity": "sha512-S48WzZW777zhNIrn7gxOlISNAqi9ZC/uQFnRdbeIHhZhCA6UqpkOT8T1G7BvfdgP4Er8gF4sUbaS0i7QvIfCWw==",
- "dev": true,
- "dependencies": {
- "path-key": "^3.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/detect-package-manager/node_modules/onetime": {
- "version": "5.1.2",
- "resolved": "https://registry.npmjs.org/onetime/-/onetime-5.1.2.tgz",
- "integrity": "sha512-kbpaSSGJTWdAY5KPVeMOKXSrPtr8C8C7wodJbcsd51jRnmD+GZu8Y0VoU6Dm5Z4vWr0Ig/1NKuWRKf7j5aaYSg==",
- "dev": true,
- "dependencies": {
- "mimic-fn": "^2.1.0"
- },
- "engines": {
- "node": ">=6"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/detect-package-manager/node_modules/path-key": {
- "version": "3.1.1",
- "resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
- "integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
- "dev": true,
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/detect-package-manager/node_modules/shebang-command": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/shebang-command/-/shebang-command-2.0.0.tgz",
- "integrity": "sha512-kHxr2zZpYtdmrN1qDjrrX/Z1rR1kG8Dx+gkpK1G4eXmvXswmcE1hTWBWYUzlraYw1/yZp6YuDY77YtvbN0dmDA==",
- "dev": true,
- "dependencies": {
- "shebang-regex": "^3.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/detect-package-manager/node_modules/shebang-regex": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/shebang-regex/-/shebang-regex-3.0.0.tgz",
- "integrity": "sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==",
- "dev": true,
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/detect-package-manager/node_modules/which": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
- "integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
- "dev": true,
- "dependencies": {
- "isexe": "^2.0.0"
- },
- "bin": {
- "node-which": "bin/node-which"
- },
- "engines": {
- "node": ">= 8"
- }
- },
- "node_modules/detect-port": {
- "version": "1.5.1",
- "resolved": "https://registry.npmjs.org/detect-port/-/detect-port-1.5.1.tgz",
- "integrity": "sha512-aBzdj76lueB6uUst5iAs7+0H/oOjqI5D16XUWxlWMIMROhcM0rfsNVk93zTngq1dDNpoXRr++Sus7ETAExppAQ==",
- "dev": true,
- "dependencies": {
- "address": "^1.0.1",
- "debug": "4"
- },
- "bin": {
- "detect": "bin/detect-port.js",
- "detect-port": "bin/detect-port.js"
- }
- },
"node_modules/devtools-protocol": {
- "version": "0.0.1312386",
- "resolved": "https://registry.npmjs.org/devtools-protocol/-/devtools-protocol-0.0.1312386.tgz",
- "integrity": "sha512-DPnhUXvmvKT2dFA/j7B+riVLUt9Q6RKJlcppojL5CoRywJJKLDYnRlw0gTFKfgDPHP5E04UoB71SxoJlVZy8FA==",
+ "version": "0.0.1367902",
+ "resolved": "https://registry.npmjs.org/devtools-protocol/-/devtools-protocol-0.0.1367902.tgz",
+ "integrity": "sha512-XxtPuC3PGakY6PD7dG66/o8KwJ/LkH2/EKe19Dcw58w53dv4/vSQEkn/SzuyhHE2q4zPgCkxQBxus3VV4ql+Pg==",
"license": "BSD-3-Clause"
},
"node_modules/diff": {
@@ -24183,11 +22347,6 @@
"integrity": "sha512-+HlytyjlPKnIG8XuRG8WvmBP8xs8P71y+SKKS6ZXWoEgLuePxtDoUEiH7WkdePWrQ5JBpE6aoVqfZfJUQkjXwA==",
"dev": true
},
- "node_modules/dns-equal": {
- "version": "1.0.0",
- "resolved": "https://registry.npmjs.org/dns-equal/-/dns-equal-1.0.0.tgz",
- "integrity": "sha512-z+paD6YUQsk+AbGCEM4PrOXSss5gd66QfcVBFTKR/HpFL9jCqikS94HYwKww6fQyO7IxrIIyUu+g0Ka9tUS2Cg=="
- },
"node_modules/dns-packet": {
"version": "5.6.1",
"resolved": "https://registry.npmjs.org/dns-packet/-/dns-packet-5.6.1.tgz",
@@ -24325,15 +22484,6 @@
"url": "https://dotenvx.com"
}
},
- "node_modules/dotenv-expand": {
- "version": "10.0.0",
- "resolved": "https://registry.npmjs.org/dotenv-expand/-/dotenv-expand-10.0.0.tgz",
- "integrity": "sha512-GopVGCpVS1UKH75VKHGuQFqS1Gusej0z4FyQkPdwjil2gNIv+LNsqBlboOzpJFZKVT95GkCyWJbBSdFEFUWI2A==",
- "dev": true,
- "engines": {
- "node": ">=12"
- }
- },
"node_modules/dset": {
"version": "3.1.4",
"resolved": "https://registry.npmjs.org/dset/-/dset-3.1.4.tgz",
@@ -24348,15 +22498,6 @@
"resolved": "https://registry.npmjs.org/duplexer/-/duplexer-0.1.2.tgz",
"integrity": "sha512-jtD6YG370ZCIi/9GTaJKQxWTZD045+4R4hTk/x1UyoqadyJ9x9CgSi1RlVDQF8U2sxLLSnFkCaMihqljHIWgMg=="
},
- "node_modules/duplexer2": {
- "version": "0.1.4",
- "resolved": "https://registry.npmjs.org/duplexer2/-/duplexer2-0.1.4.tgz",
- "integrity": "sha512-asLFVfWWtJ90ZyOUHMqk7/S2w2guQKxUI2itj3d92ADHhxUSbCMGi1f1cBcJ7xM1To+pE/Khbwo1yuNbMEPKeA==",
- "dev": true,
- "dependencies": {
- "readable-stream": "^2.0.2"
- }
- },
"node_modules/duplexify": {
"version": "3.6.0",
"resolved": "https://registry.npmjs.org/duplexify/-/duplexify-3.6.0.tgz",
@@ -24372,14 +22513,14 @@
"node_modules/eastasianwidth": {
"version": "0.2.0",
"resolved": "https://registry.npmjs.org/eastasianwidth/-/eastasianwidth-0.2.0.tgz",
- "integrity": "sha512-I88TYZWc9XiYHRQ4/3c5rjjfgkjhLyW2luGIheGERbNQ6OY7yTybanSpDXZa8y7VUP9YmDcYa+eyq4ca7iLqWA==",
- "dev": true
+ "integrity": "sha512-I88TYZWc9XiYHRQ4/3c5rjjfgkjhLyW2luGIheGERbNQ6OY7yTybanSpDXZa8y7VUP9YmDcYa+eyq4ca7iLqWA=="
},
"node_modules/edge-paths": {
"version": "3.0.5",
"resolved": "https://registry.npmjs.org/edge-paths/-/edge-paths-3.0.5.tgz",
"integrity": "sha512-sB7vSrDnFa4ezWQk9nZ/n0FdpdUuC6R1EOrlU3DL+bovcNFK28rqu2emmAUjujYEJTWIgQGqgVVWUZXMnc8iWg==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"@types/which": "^2.0.1",
"which": "^2.0.2"
@@ -24395,13 +22536,15 @@
"version": "2.0.2",
"resolved": "https://registry.npmjs.org/@types/which/-/which-2.0.2.tgz",
"integrity": "sha512-113D3mDkZDjo+EeUEHCFy0qniNc1ZpecGiAU7WSo7YDoSzolZIQKpYFHrPpjkB2nuyahcKfrmLXeQlh7gqJYdw==",
- "dev": true
+ "dev": true,
+ "license": "MIT"
},
"node_modules/edge-paths/node_modules/which": {
"version": "2.0.2",
"resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
"integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"isexe": "^2.0.0"
},
@@ -24413,28 +22556,73 @@
}
},
"node_modules/edgedriver": {
- "version": "5.3.7",
- "resolved": "https://registry.npmjs.org/edgedriver/-/edgedriver-5.3.7.tgz",
- "integrity": "sha512-E1qNFEA9NbaCPSvGaeZhyd7mEZLar+oFS0NRAe5TehJcQ3cayoUdJE5uOFrbxdv/rM4NEPH7aK9a9kgG09rszA==",
+ "version": "5.6.1",
+ "resolved": "https://registry.npmjs.org/edgedriver/-/edgedriver-5.6.1.tgz",
+ "integrity": "sha512-3Ve9cd5ziLByUdigw6zovVeWJjVs8QHVmqOB0sJ0WNeVPcwf4p18GnxMmVvlFmYRloUwf5suNuorea4QzwBIOA==",
"dev": true,
"hasInstallScript": true,
+ "license": "MIT",
"dependencies": {
- "@wdio/logger": "^8.11.0",
+ "@wdio/logger": "^8.38.0",
+ "@zip.js/zip.js": "^2.7.48",
"decamelize": "^6.0.0",
"edge-paths": "^3.0.5",
+ "fast-xml-parser": "^4.4.1",
"node-fetch": "^3.3.2",
- "unzipper": "^0.10.14",
"which": "^4.0.0"
},
"bin": {
"edgedriver": "bin/edgedriver.js"
}
},
+ "node_modules/edgedriver/node_modules/@wdio/logger": {
+ "version": "8.38.0",
+ "resolved": "https://registry.npmjs.org/@wdio/logger/-/logger-8.38.0.tgz",
+ "integrity": "sha512-kcHL86RmNbcQP+Gq/vQUGlArfU6IIcbbnNp32rRIraitomZow+iEoc519rdQmSVusDozMS5DZthkgDdxK+vz6Q==",
+ "dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "chalk": "^5.1.2",
+ "loglevel": "^1.6.0",
+ "loglevel-plugin-prefix": "^0.8.4",
+ "strip-ansi": "^7.1.0"
+ },
+ "engines": {
+ "node": "^16.13 || >=18"
+ }
+ },
+ "node_modules/edgedriver/node_modules/ansi-regex": {
+ "version": "6.1.0",
+ "resolved": "https://registry.npmjs.org/ansi-regex/-/ansi-regex-6.1.0.tgz",
+ "integrity": "sha512-7HSX4QQb4CspciLpVFwyRe79O3xsIZDDLER21kERQ71oaPodF8jL725AgJMFAYbooIqolJoRLuM81SpeUkpkvA==",
+ "dev": true,
+ "license": "MIT",
+ "engines": {
+ "node": ">=12"
+ },
+ "funding": {
+ "url": "https://github.com/chalk/ansi-regex?sponsor=1"
+ }
+ },
+ "node_modules/edgedriver/node_modules/chalk": {
+ "version": "5.3.0",
+ "resolved": "https://registry.npmjs.org/chalk/-/chalk-5.3.0.tgz",
+ "integrity": "sha512-dLitG79d+GV1Nb/VYcCDFivJeK1hiukt9QjRNVOsUtTy1rR1YJsmpGGTZ3qJos+uw7WmWF4wUwBd9jxjocFC2w==",
+ "dev": true,
+ "license": "MIT",
+ "engines": {
+ "node": "^12.17.0 || ^14.13 || >=16.0.0"
+ },
+ "funding": {
+ "url": "https://github.com/chalk/chalk?sponsor=1"
+ }
+ },
"node_modules/edgedriver/node_modules/data-uri-to-buffer": {
"version": "4.0.1",
"resolved": "https://registry.npmjs.org/data-uri-to-buffer/-/data-uri-to-buffer-4.0.1.tgz",
"integrity": "sha512-0R9ikRb668HB7QDxT1vkpuUBtqc53YyAwMwGeUFKRojY/NWKvdZ+9UYtRfGmhqNbRkTSVpMbmyhXipFFv2cb/A==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">= 12"
}
@@ -24444,6 +22632,7 @@
"resolved": "https://registry.npmjs.org/decamelize/-/decamelize-6.0.0.tgz",
"integrity": "sha512-Fv96DCsdOgB6mdGl67MT5JaTNKRzrzill5OH5s8bjYJXVlcXyPYGyPsUkWyGV5p1TXI5esYIYMMeDJL0hEIwaA==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": "^12.20.0 || ^14.13.1 || >=16.0.0"
},
@@ -24456,6 +22645,7 @@
"resolved": "https://registry.npmjs.org/isexe/-/isexe-3.1.1.tgz",
"integrity": "sha512-LpB/54B+/2J5hqQ7imZHfdU31OlgQqx7ZicVlkm9kzg9/w8GKLEcFfJl/t7DCEDueOyBAD6zCCwTO6Fzs0NoEQ==",
"dev": true,
+ "license": "ISC",
"engines": {
"node": ">=16"
}
@@ -24465,6 +22655,7 @@
"resolved": "https://registry.npmjs.org/node-fetch/-/node-fetch-3.3.2.tgz",
"integrity": "sha512-dRB78srN/l6gqWulah9SrxeYnxeddIG30+GOqK/9OlLVyLg3HPnr6SqOWTWOXKRwC2eGYCkZ59NNuSgvSrpgOA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"data-uri-to-buffer": "^4.0.0",
"fetch-blob": "^3.1.4",
@@ -24478,11 +22669,28 @@
"url": "https://opencollective.com/node-fetch"
}
},
+ "node_modules/edgedriver/node_modules/strip-ansi": {
+ "version": "7.1.0",
+ "resolved": "https://registry.npmjs.org/strip-ansi/-/strip-ansi-7.1.0.tgz",
+ "integrity": "sha512-iq6eVVI64nQQTRYq2KtEg2d2uU7LElhTJwsH4YzIHZshxlgZms/wIc4VoDQTlG/IvVIrBKG06CrZnp0qv7hkcQ==",
+ "dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "ansi-regex": "^6.0.1"
+ },
+ "engines": {
+ "node": ">=12"
+ },
+ "funding": {
+ "url": "https://github.com/chalk/strip-ansi?sponsor=1"
+ }
+ },
"node_modules/edgedriver/node_modules/which": {
"version": "4.0.0",
"resolved": "https://registry.npmjs.org/which/-/which-4.0.0.tgz",
"integrity": "sha512-GlaYyEb07DPxYCKhKzplCWBJtvxZcZMrL+4UkrTSJHHPyZU4mYYTv3qaOe77H7EODLSSopAUFAc6W8U4yqvscg==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"isexe": "^3.1.1"
},
@@ -24515,9 +22723,10 @@
}
},
"node_modules/electron-to-chromium": {
- "version": "1.5.33",
- "resolved": "https://registry.npmjs.org/electron-to-chromium/-/electron-to-chromium-1.5.33.tgz",
- "integrity": "sha512-+cYTcFB1QqD4j4LegwLfpCNxifb6dDFUAwk6RsLusCwIaZI6or2f+q8rs5tTB2YC53HhOlIbEaqHMAAC8IOIwA=="
+ "version": "1.5.71",
+ "resolved": "https://registry.npmjs.org/electron-to-chromium/-/electron-to-chromium-1.5.71.tgz",
+ "integrity": "sha512-dB68l59BI75W1BUGVTAEJy45CEVuEGy9qPVVQ8pnHyHMn36PLPPoE1mjLH+lo9rKulO3HC2OhbACI/8tCqJBcA==",
+ "license": "ISC"
},
"node_modules/elegant-spinner": {
"version": "1.0.1",
@@ -24529,10 +22738,11 @@
}
},
"node_modules/elliptic": {
- "version": "6.5.4",
- "resolved": "https://registry.npmjs.org/elliptic/-/elliptic-6.5.4.tgz",
- "integrity": "sha512-iLhC6ULemrljPZb+QutR5TQGB+pdW6KGD5RSegS+8sorOZT+rdQFbsQFJgvN3eRqNALqJer4oQ16YvJHlU8hzQ==",
+ "version": "6.6.1",
+ "resolved": "https://registry.npmjs.org/elliptic/-/elliptic-6.6.1.tgz",
+ "integrity": "sha512-RaddvvMatK2LJHqFJ+YA4WysVN5Ita9E35botqIYspQ4TkRAlCicdzKOjlyv/1Za5RyTNn7di//eEV0uTAfe3g==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"bn.js": "^4.11.9",
"brorand": "^1.1.0",
@@ -24672,9 +22882,9 @@
}
},
"node_modules/envinfo": {
- "version": "7.14.0",
- "resolved": "https://registry.npmjs.org/envinfo/-/envinfo-7.14.0.tgz",
- "integrity": "sha512-CO40UI41xDQzhLB1hWyqUKgFhs250pNcGbyGKe1l/e4FSaI/+YE4IMG76GDt0In67WLPACIITC+sOi08x4wIvg==",
+ "version": "7.13.0",
+ "resolved": "https://registry.npmjs.org/envinfo/-/envinfo-7.13.0.tgz",
+ "integrity": "sha512-cvcaMr7KqXVh4nyzGTVqTum+gAiL265x5jUWQIDLq//zOGbW+gSW/C+OWLleY/rs9Qole6AZLMXPbtIFQbqu+Q==",
"license": "MIT",
"bin": {
"envinfo": "dist/cli.js"
@@ -24692,7 +22902,8 @@
"version": "2.0.3",
"resolved": "https://registry.npmjs.org/err-code/-/err-code-2.0.3.tgz",
"integrity": "sha512-2bmlRpNKBxT/CRmPOlyISQpNj+qSeYvcym/uT0Jx2bMOlKLtSy1ZmLuVxSEKKyor/N5yhvp/ZiG1oE3DEYMSFA==",
- "dev": true
+ "dev": true,
+ "license": "MIT"
},
"node_modules/errno": {
"version": "0.1.7",
@@ -24832,9 +23043,9 @@
"dev": true
},
"node_modules/es-module-lexer": {
- "version": "1.4.1",
- "resolved": "https://registry.npmjs.org/es-module-lexer/-/es-module-lexer-1.4.1.tgz",
- "integrity": "sha512-cXLGjP0c4T3flZJKQSuziYoq7MlT+rnvfZjfp7h+I7K9BNX54kP9nyWvdbwjQ4u1iWbOL4u96fgeZLToQlZC7w=="
+ "version": "1.5.4",
+ "resolved": "https://registry.npmjs.org/es-module-lexer/-/es-module-lexer-1.5.4.tgz",
+ "integrity": "sha512-MVNK56NiMrOwitFB7cqDwq0CQutbw+0BvLshJSse0MUNU+y1FC3bUS/AQg7oUng+/wKrrki7JfmwtVHkVfPLlw=="
},
"node_modules/es-set-tostringtag": {
"version": "2.0.1",
@@ -24873,11 +23084,18 @@
"url": "https://github.com/sponsors/ljharb"
}
},
+ "node_modules/es-toolkit": {
+ "version": "1.29.0",
+ "resolved": "https://registry.npmjs.org/es-toolkit/-/es-toolkit-1.29.0.tgz",
+ "integrity": "sha512-GjTll+E6APcfAQA09D89HdT8Qn2Yb+TeDSDBTMcxAo+V+w1amAtCI15LJu4YPH/UCPoSo/F47Gr1LIM0TE0lZA==",
+ "dev": true
+ },
"node_modules/es6-error": {
"version": "4.1.1",
"resolved": "https://registry.npmjs.org/es6-error/-/es6-error-4.1.1.tgz",
"integrity": "sha512-Um/+FxMr9CISWh0bi5Zv0iOD+4cFh5qLeks1qhAopKVAJw3drgKbKySikp7wGhDL0HPeaja0P5ULZrxLkniUVg==",
- "dev": true
+ "dev": true,
+ "license": "MIT"
},
"node_modules/esbuild": {
"version": "0.18.20",
@@ -24916,16 +23134,10 @@
"@esbuild/win32-x64": "0.18.20"
}
},
- "node_modules/esbuild-plugin-alias": {
- "version": "0.2.1",
- "resolved": "https://registry.npmjs.org/esbuild-plugin-alias/-/esbuild-plugin-alias-0.2.1.tgz",
- "integrity": "sha512-jyfL/pwPqaFXyKnj8lP8iLk6Z0m099uXR45aSN8Av1XD4vhvQutxxPzgA2bTcAwQpa1zCXDcWOlhFgyP3GKqhQ==",
- "dev": true
- },
"node_modules/esbuild-register": {
- "version": "3.5.0",
- "resolved": "https://registry.npmjs.org/esbuild-register/-/esbuild-register-3.5.0.tgz",
- "integrity": "sha512-+4G/XmakeBAsvJuDugJvtyF1x+XJT4FMocynNpxrvEBViirpfUn2PgNpCHedfWhF4WokNsO/OvMKrmJOIJsI5A==",
+ "version": "3.6.0",
+ "resolved": "https://registry.npmjs.org/esbuild-register/-/esbuild-register-3.6.0.tgz",
+ "integrity": "sha512-H2/S7Pm8a9CL1uhp9OvjwrBh5Pvx0H8qVOxNu8Wed9Y7qv56MPtq+GGM8RJpq6glYJn9Wspr8uw7l55uyinNeg==",
"dev": true,
"dependencies": {
"debug": "^4.3.4"
@@ -25173,9 +23385,10 @@
"integrity": "sha512-6FlzubTLZG3J2a/NVCAleEhjzq5oxgHyaCU9yYXvcLsvoVaHJq/s5xXI6/XXP6tz7R9xAOtHnSO/tXtF3WRTlA=="
},
"node_modules/eslint-plugin-jest": {
- "version": "27.2.3",
- "resolved": "https://registry.npmjs.org/eslint-plugin-jest/-/eslint-plugin-jest-27.2.3.tgz",
- "integrity": "sha512-sRLlSCpICzWuje66Gl9zvdF6mwD5X86I4u55hJyFBsxYOsBCmT5+kSUjf+fkFWVMMgpzNEupjW8WzUqi83hJAQ==",
+ "version": "27.4.3",
+ "resolved": "https://registry.npmjs.org/eslint-plugin-jest/-/eslint-plugin-jest-27.4.3.tgz",
+ "integrity": "sha512-7S6SmmsHsgIm06BAGCAxL+ABd9/IB3MWkz2pudj6Qqor2y1qQpWPfuFU4SG9pWj4xDjF0e+D7Llh5useuSzAZw==",
+ "license": "MIT",
"dependencies": {
"@typescript-eslint/utils": "^5.10.0"
},
@@ -25347,6 +23560,41 @@
"eslint": "^3 || ^4 || ^5 || ^6 || ^7 || ^8"
}
},
+ "node_modules/eslint-plugin-react-compiler": {
+ "version": "19.0.0-beta-0dec889-20241115",
+ "resolved": "https://registry.npmjs.org/eslint-plugin-react-compiler/-/eslint-plugin-react-compiler-19.0.0-beta-0dec889-20241115.tgz",
+ "integrity": "sha512-jTjEHuE8/R6qD/CD2d+5YvWMy1q9/tX3kft4WDyg42/HktjHtHXrEToyZ6THEQf8t/YWMY1RGeCkykePbACtFA==",
+ "dev": true,
+ "dependencies": {
+ "@babel/core": "^7.24.4",
+ "@babel/parser": "^7.24.4",
+ "@babel/plugin-proposal-private-methods": "^7.18.6",
+ "hermes-parser": "^0.20.1",
+ "zod": "^3.22.4",
+ "zod-validation-error": "^3.0.3"
+ },
+ "engines": {
+ "node": "^14.17.0 || ^16.0.0 || >= 18.0.0"
+ },
+ "peerDependencies": {
+ "eslint": ">=7"
+ }
+ },
+ "node_modules/eslint-plugin-react-compiler/node_modules/hermes-estree": {
+ "version": "0.20.1",
+ "resolved": "https://registry.npmjs.org/hermes-estree/-/hermes-estree-0.20.1.tgz",
+ "integrity": "sha512-SQpZK4BzR48kuOg0v4pb3EAGNclzIlqMj3Opu/mu7bbAoFw6oig6cEt/RAi0zTFW/iW6Iz9X9ggGuZTAZ/yZHg==",
+ "dev": true
+ },
+ "node_modules/eslint-plugin-react-compiler/node_modules/hermes-parser": {
+ "version": "0.20.1",
+ "resolved": "https://registry.npmjs.org/hermes-parser/-/hermes-parser-0.20.1.tgz",
+ "integrity": "sha512-BL5P83cwCogI8D7rrDCgsFY0tdYUtmFP9XaXtl2IQjC+2Xo+4okjfXintlTxcIwl4qeGddEl28Z11kbVIw0aNA==",
+ "dev": true,
+ "dependencies": {
+ "hermes-estree": "0.20.1"
+ }
+ },
"node_modules/eslint-plugin-react-hooks": {
"version": "4.3.0",
"resolved": "https://registry.npmjs.org/eslint-plugin-react-hooks/-/eslint-plugin-react-hooks-4.3.0.tgz",
@@ -25503,19 +23751,6 @@
"resolved": "https://registry.npmjs.org/argparse/-/argparse-2.0.1.tgz",
"integrity": "sha512-8+9WqebbFzpX9OR+Wa6O29asIogeRMzcGtAINdpMHHyAg10f05aSFVBbcEqGf/PXw1EjAZ+q2/bEBg3DvurK3Q=="
},
- "node_modules/eslint/node_modules/cross-spawn": {
- "version": "7.0.3",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-7.0.3.tgz",
- "integrity": "sha512-iRDPJKUPVEND7dHPO8rkbOnPpyDygcDFtWjpeWNCgy8WP2rXcxXL8TskReQl6OrB2G7+UJrags1q15Fudc7G6w==",
- "dependencies": {
- "path-key": "^3.1.0",
- "shebang-command": "^2.0.0",
- "which": "^2.0.1"
- },
- "engines": {
- "node": ">= 8"
- }
- },
"node_modules/eslint/node_modules/doctrine": {
"version": "3.0.0",
"resolved": "https://registry.npmjs.org/doctrine/-/doctrine-3.0.0.tgz",
@@ -25630,14 +23865,6 @@
"node": ">= 0.8.0"
}
},
- "node_modules/eslint/node_modules/path-key": {
- "version": "3.1.1",
- "resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
- "integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
- "engines": {
- "node": ">=8"
- }
- },
"node_modules/eslint/node_modules/prelude-ls": {
"version": "1.2.1",
"resolved": "https://registry.npmjs.org/prelude-ls/-/prelude-ls-1.2.1.tgz",
@@ -25646,25 +23873,6 @@
"node": ">= 0.8.0"
}
},
- "node_modules/eslint/node_modules/shebang-command": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/shebang-command/-/shebang-command-2.0.0.tgz",
- "integrity": "sha512-kHxr2zZpYtdmrN1qDjrrX/Z1rR1kG8Dx+gkpK1G4eXmvXswmcE1hTWBWYUzlraYw1/yZp6YuDY77YtvbN0dmDA==",
- "dependencies": {
- "shebang-regex": "^3.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/eslint/node_modules/shebang-regex": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/shebang-regex/-/shebang-regex-3.0.0.tgz",
- "integrity": "sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==",
- "engines": {
- "node": ">=8"
- }
- },
"node_modules/eslint/node_modules/strip-json-comments": {
"version": "3.1.1",
"resolved": "https://registry.npmjs.org/strip-json-comments/-/strip-json-comments-3.1.1.tgz",
@@ -25687,20 +23895,6 @@
"node": ">= 0.8.0"
}
},
- "node_modules/eslint/node_modules/which": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
- "integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
- "dependencies": {
- "isexe": "^2.0.0"
- },
- "bin": {
- "node-which": "bin/node-which"
- },
- "engines": {
- "node": ">= 8"
- }
- },
"node_modules/espree": {
"version": "9.1.0",
"resolved": "https://registry.npmjs.org/espree/-/espree-9.1.0.tgz",
@@ -25795,6 +23989,15 @@
"node": ">=4.0"
}
},
+ "node_modules/estree-walker": {
+ "version": "3.0.3",
+ "resolved": "https://registry.npmjs.org/estree-walker/-/estree-walker-3.0.3.tgz",
+ "integrity": "sha512-7RUKfXgSMMkzt6ZuXmqapOurLGPPfgj6l9uRZ7lRGolvk0y2yocc35LdcxKC5PQZdn2DMqioAQ2NoWcrTKmm6g==",
+ "dev": true,
+ "dependencies": {
+ "@types/estree": "^1.0.0"
+ }
+ },
"node_modules/esutils": {
"version": "2.0.2",
"resolved": "https://registry.npmjs.org/esutils/-/esutils-2.0.2.tgz",
@@ -25869,19 +24072,6 @@
"url": "https://github.com/sindresorhus/execa?sponsor=1"
}
},
- "node_modules/execa/node_modules/cross-spawn": {
- "version": "7.0.3",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-7.0.3.tgz",
- "integrity": "sha512-iRDPJKUPVEND7dHPO8rkbOnPpyDygcDFtWjpeWNCgy8WP2rXcxXL8TskReQl6OrB2G7+UJrags1q15Fudc7G6w==",
- "dependencies": {
- "path-key": "^3.1.0",
- "shebang-command": "^2.0.0",
- "which": "^2.0.1"
- },
- "engines": {
- "node": ">= 8"
- }
- },
"node_modules/execa/node_modules/is-stream": {
"version": "2.0.1",
"resolved": "https://registry.npmjs.org/is-stream/-/is-stream-2.0.1.tgz",
@@ -25934,39 +24124,6 @@
"node": ">=8"
}
},
- "node_modules/execa/node_modules/shebang-command": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/shebang-command/-/shebang-command-2.0.0.tgz",
- "integrity": "sha512-kHxr2zZpYtdmrN1qDjrrX/Z1rR1kG8Dx+gkpK1G4eXmvXswmcE1hTWBWYUzlraYw1/yZp6YuDY77YtvbN0dmDA==",
- "dependencies": {
- "shebang-regex": "^3.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/execa/node_modules/shebang-regex": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/shebang-regex/-/shebang-regex-3.0.0.tgz",
- "integrity": "sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==",
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/execa/node_modules/which": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
- "integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
- "dependencies": {
- "isexe": "^2.0.0"
- },
- "bin": {
- "node-which": "bin/node-which"
- },
- "engines": {
- "node": ">= 8"
- }
- },
"node_modules/exit": {
"version": "0.1.2",
"resolved": "https://registry.npmjs.org/exit/-/exit-0.1.2.tgz",
@@ -26031,6 +24188,7 @@
"resolved": "https://registry.npmjs.org/expand-template/-/expand-template-2.0.3.tgz",
"integrity": "sha512-XYfuKMvj4O35f/pOXLObndIRvyQ+/+6AhODh+OKWj9S9498pHHn/IMszH+gt0fBCRWMNfk1ZSp5x3AifmnI2vg==",
"dev": true,
+ "license": "(MIT OR WTFPL)",
"optional": true,
"engines": {
"node": ">=6"
@@ -26072,12 +24230,14 @@
"version": "3.1.1",
"resolved": "https://registry.npmjs.org/exponential-backoff/-/exponential-backoff-3.1.1.tgz",
"integrity": "sha512-dX7e/LHVJ6W3DE1MHWi9S1EYzDESENfLrYohG2G++ovZrYOkm4Knwa0mc1cn84xJOR4KEU0WSchhLbd0UklbHw==",
- "dev": true
+ "dev": true,
+ "license": "Apache-2.0"
},
"node_modules/express": {
"version": "4.18.2",
"resolved": "https://registry.npmjs.org/express/-/express-4.18.2.tgz",
"integrity": "sha512-5/PsL6iGPdfQ/lKM1UuielYgv3BUoJfz1aUwU9vHZ+J7gyvwdQXFEBIEIaxeGf0GIcreATNyBExtalisDbuMqQ==",
+ "license": "MIT",
"dependencies": {
"accepts": "~1.3.8",
"array-flatten": "1.1.1",
@@ -26119,6 +24279,7 @@
"version": "2.6.9",
"resolved": "https://registry.npmjs.org/debug/-/debug-2.6.9.tgz",
"integrity": "sha512-bC7ElrdJaJnPbAP+1EotYvqZsb3ecl5wi6Bfi6BJTUcNowp6cvspg0jXznRTKDjm/E7AdgFBVeAPVMNcKGsHMA==",
+ "license": "MIT",
"dependencies": {
"ms": "2.0.0"
}
@@ -26135,6 +24296,7 @@
"version": "1.2.0",
"resolved": "https://registry.npmjs.org/finalhandler/-/finalhandler-1.2.0.tgz",
"integrity": "sha512-5uXcUVftlQMFnWC9qu/svkWv3GTd2PfUhK/3PLkYNAe7FbqJMt3515HaxE6eRL74GdsriiwujiawdaB1BpEISg==",
+ "license": "MIT",
"dependencies": {
"debug": "2.6.9",
"encodeurl": "~1.0.2",
@@ -26152,6 +24314,7 @@
"version": "2.4.1",
"resolved": "https://registry.npmjs.org/on-finished/-/on-finished-2.4.1.tgz",
"integrity": "sha512-oVlzkg3ENAhCk2zdv7IJwd/QUD4z2RxRwpkcGY8psCVcCYZNq4wYnVWALHM+brtuJjePWiYF/ClmuDr8Ch5+kg==",
+ "license": "MIT",
"dependencies": {
"ee-first": "1.1.1"
},
@@ -26159,6 +24322,21 @@
"node": ">= 0.8"
}
},
+ "node_modules/express/node_modules/qs": {
+ "version": "6.11.0",
+ "resolved": "https://registry.npmjs.org/qs/-/qs-6.11.0.tgz",
+ "integrity": "sha512-MvjoMCJwEarSbUYk5O+nmoSzSutSsTwF85zcHPQ9OrlFoZOYIjaqBAJIqIXjptyD5vThxGq52Xu/MaJzRkIk4Q==",
+ "license": "BSD-3-Clause",
+ "dependencies": {
+ "side-channel": "^1.0.4"
+ },
+ "engines": {
+ "node": ">=0.6"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/ljharb"
+ }
+ },
"node_modules/express/node_modules/safe-buffer": {
"version": "5.2.1",
"resolved": "https://registry.npmjs.org/safe-buffer/-/safe-buffer-5.2.1.tgz",
@@ -26182,6 +24360,7 @@
"version": "2.0.1",
"resolved": "https://registry.npmjs.org/statuses/-/statuses-2.0.1.tgz",
"integrity": "sha512-RwNA9Z/7PrK06rYLIzFMlaF+l73iwpzsqRIFgbMLbTcLD6cOao82TaWefPXQvB2fOC4AjuYSEndS7N/mTCbkdQ==",
+ "license": "MIT",
"engines": {
"node": ">= 0.8"
}
@@ -26426,9 +24605,9 @@
"license": "MIT"
},
"node_modules/fast-xml-parser": {
- "version": "4.3.4",
- "resolved": "https://registry.npmjs.org/fast-xml-parser/-/fast-xml-parser-4.3.4.tgz",
- "integrity": "sha512-utnwm92SyozgA3hhH2I8qldf2lBqm6qHOICawRNRFu1qMe3+oqr+GcXjGqTmXTMGE5T4eC03kr/rlh5C1IRdZA==",
+ "version": "4.5.0",
+ "resolved": "https://registry.npmjs.org/fast-xml-parser/-/fast-xml-parser-4.5.0.tgz",
+ "integrity": "sha512-/PlTQCI96+fZMAOLMZK4CWG1ItCbfZ/0jx7UIJFChPNrx7tcEgerUgWbeieCM9MfHInUDyK8DWYZ+YrywDJuTg==",
"funding": [
{
"type": "github",
@@ -26439,6 +24618,7 @@
"url": "https://paypal.me/naturalintelligence"
}
],
+ "license": "MIT",
"dependencies": {
"strnum": "^1.0.5"
},
@@ -26516,6 +24696,7 @@
"url": "https://paypal.me/jimmywarting"
}
],
+ "license": "MIT",
"dependencies": {
"node-domexception": "^1.0.0",
"web-streams-polyfill": "^3.0.3"
@@ -26524,12 +24705,6 @@
"node": "^12.20 || >= 14.13"
}
},
- "node_modules/fetch-retry": {
- "version": "5.0.6",
- "resolved": "https://registry.npmjs.org/fetch-retry/-/fetch-retry-5.0.6.tgz",
- "integrity": "sha512-3yurQZ2hD9VISAhJJP9bpYFNQrHHBXE2JxxjY5aLEcDi46RmAzJE2OC9FAde0yis5ElW0jTTzs0zfg/Cca4XqQ==",
- "dev": true
- },
"node_modules/figgy-pudding": {
"version": "3.5.2",
"resolved": "https://registry.npmjs.org/figgy-pudding/-/figgy-pudding-3.5.2.tgz",
@@ -26560,51 +24735,6 @@
"node": "^10.12.0 || >=12.0.0"
}
},
- "node_modules/file-system-cache": {
- "version": "2.3.0",
- "resolved": "https://registry.npmjs.org/file-system-cache/-/file-system-cache-2.3.0.tgz",
- "integrity": "sha512-l4DMNdsIPsVnKrgEXbJwDJsA5mB8rGwHYERMgqQx/xAUtChPJMre1bXBzDEqqVbWv9AIbFezXMxeEkZDSrXUOQ==",
- "dev": true,
- "dependencies": {
- "fs-extra": "11.1.1",
- "ramda": "0.29.0"
- }
- },
- "node_modules/file-system-cache/node_modules/fs-extra": {
- "version": "11.1.1",
- "resolved": "https://registry.npmjs.org/fs-extra/-/fs-extra-11.1.1.tgz",
- "integrity": "sha512-MGIE4HOvQCeUCzmlHs0vXpih4ysz4wg9qiSAu6cd42lVwPbTM1TjV7RusoyQqMmk/95gdQZX72u+YW+c3eEpFQ==",
- "dev": true,
- "dependencies": {
- "graceful-fs": "^4.2.0",
- "jsonfile": "^6.0.1",
- "universalify": "^2.0.0"
- },
- "engines": {
- "node": ">=14.14"
- }
- },
- "node_modules/file-system-cache/node_modules/jsonfile": {
- "version": "6.1.0",
- "resolved": "https://registry.npmjs.org/jsonfile/-/jsonfile-6.1.0.tgz",
- "integrity": "sha512-5dgndWOriYSm5cnYaJNhalLNDKOqFwyDB/rr1E9ZsGciGvKPs8R2xYGCacuf3z6K1YKDz182fd+fY3cn3pMqXQ==",
- "dev": true,
- "dependencies": {
- "universalify": "^2.0.0"
- },
- "optionalDependencies": {
- "graceful-fs": "^4.1.6"
- }
- },
- "node_modules/file-system-cache/node_modules/universalify": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/universalify/-/universalify-2.0.1.tgz",
- "integrity": "sha512-gptHNQghINnc/vTGIk0SOFGFNXw7JVrlRUtConJRlvaw6DuX0wO5Jeko9sWrMBhh+PsYAZ7oXAiOnf/UKogyiw==",
- "dev": true,
- "engines": {
- "node": ">= 10.0.0"
- }
- },
"node_modules/file-uri-to-path": {
"version": "1.0.0",
"resolved": "https://registry.npmjs.org/file-uri-to-path/-/file-uri-to-path-1.0.0.tgz",
@@ -26919,6 +25049,7 @@
"resolved": "https://registry.npmjs.org/flat/-/flat-5.0.2.tgz",
"integrity": "sha512-b6suED+5/3rTpUBdG1gupIl8MPFCAMA0QXwmljLhvCUKcUvdE4gWky9zpuGCcXHOsz4J9wPGNWq6OKpmIzz3hQ==",
"dev": true,
+ "license": "BSD-3-Clause",
"bin": {
"flat": "cli.js"
}
@@ -26937,6 +25068,43 @@
"node": "^10.12.0 || >=12.0.0"
}
},
+ "node_modules/flat-cache/node_modules/glob": {
+ "version": "7.2.3",
+ "resolved": "https://registry.npmjs.org/glob/-/glob-7.2.3.tgz",
+ "integrity": "sha512-nFR0zLpU2YCaRxwoCJvL6UvCH2JFyFVIvwTLsIf21AuHlMskA1hhTdk+LlYJtOlYt9v6dvszD2BGRqBL+iQK9Q==",
+ "deprecated": "Glob versions prior to v9 are no longer supported",
+ "license": "ISC",
+ "dependencies": {
+ "fs.realpath": "^1.0.0",
+ "inflight": "^1.0.4",
+ "inherits": "2",
+ "minimatch": "^3.1.1",
+ "once": "^1.3.0",
+ "path-is-absolute": "^1.0.0"
+ },
+ "engines": {
+ "node": "*"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
+ }
+ },
+ "node_modules/flat-cache/node_modules/rimraf": {
+ "version": "3.0.2",
+ "resolved": "https://registry.npmjs.org/rimraf/-/rimraf-3.0.2.tgz",
+ "integrity": "sha512-JZkJMZkAGFFPP2YqXZXPbMlMBgsxzE8ILs4lMIX/2o0L9UBw9O/Y3o6wFw/i9YLapcUJWwqbi3kdxIPdC62TIA==",
+ "deprecated": "Rimraf versions prior to v4 are no longer supported",
+ "license": "ISC",
+ "dependencies": {
+ "glob": "^7.1.3"
+ },
+ "bin": {
+ "rimraf": "bin.js"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
+ }
+ },
"node_modules/flatted": {
"version": "3.3.1",
"resolved": "https://registry.npmjs.org/flatted/-/flatted-3.3.1.tgz",
@@ -27021,7 +25189,6 @@
"version": "3.1.1",
"resolved": "https://registry.npmjs.org/foreground-child/-/foreground-child-3.1.1.tgz",
"integrity": "sha512-TMKDUnIte6bfb5nWv7V/caI169OHgvwjb7V4WkeUvbQQdjr5rWKqHFiKWb/fcOwB+CzBT+qbWjvj+DVwRskpIg==",
- "dev": true,
"dependencies": {
"cross-spawn": "^7.0.0",
"signal-exit": "^4.0.1"
@@ -27033,55 +25200,10 @@
"url": "https://github.com/sponsors/isaacs"
}
},
- "node_modules/foreground-child/node_modules/cross-spawn": {
- "version": "7.0.3",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-7.0.3.tgz",
- "integrity": "sha512-iRDPJKUPVEND7dHPO8rkbOnPpyDygcDFtWjpeWNCgy8WP2rXcxXL8TskReQl6OrB2G7+UJrags1q15Fudc7G6w==",
- "dev": true,
- "dependencies": {
- "path-key": "^3.1.0",
- "shebang-command": "^2.0.0",
- "which": "^2.0.1"
- },
- "engines": {
- "node": ">= 8"
- }
- },
- "node_modules/foreground-child/node_modules/path-key": {
- "version": "3.1.1",
- "resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
- "integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
- "dev": true,
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/foreground-child/node_modules/shebang-command": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/shebang-command/-/shebang-command-2.0.0.tgz",
- "integrity": "sha512-kHxr2zZpYtdmrN1qDjrrX/Z1rR1kG8Dx+gkpK1G4eXmvXswmcE1hTWBWYUzlraYw1/yZp6YuDY77YtvbN0dmDA==",
- "dev": true,
- "dependencies": {
- "shebang-regex": "^3.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/foreground-child/node_modules/shebang-regex": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/shebang-regex/-/shebang-regex-3.0.0.tgz",
- "integrity": "sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==",
- "dev": true,
- "engines": {
- "node": ">=8"
- }
- },
"node_modules/foreground-child/node_modules/signal-exit": {
"version": "4.1.0",
"resolved": "https://registry.npmjs.org/signal-exit/-/signal-exit-4.1.0.tgz",
"integrity": "sha512-bzyZ1e88w9O1iNJbKnOlvYTrWPDl46O1bG0D3XInv+9tkPrxrN8jUUTiFlDkkmKWgn1M6CfIA13SuGqOa9Korw==",
- "dev": true,
"engines": {
"node": ">=14"
},
@@ -27089,21 +25211,6 @@
"url": "https://github.com/sponsors/isaacs"
}
},
- "node_modules/foreground-child/node_modules/which": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
- "integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
- "dev": true,
- "dependencies": {
- "isexe": "^2.0.0"
- },
- "bin": {
- "node-which": "bin/node-which"
- },
- "engines": {
- "node": ">= 8"
- }
- },
"node_modules/fork-ts-checker-webpack-plugin": {
"version": "8.0.0",
"resolved": "https://registry.npmjs.org/fork-ts-checker-webpack-plugin/-/fork-ts-checker-webpack-plugin-8.0.0.tgz",
@@ -27265,6 +25372,7 @@
"resolved": "https://registry.npmjs.org/form-data-encoder/-/form-data-encoder-2.1.4.tgz",
"integrity": "sha512-yDYSgNMraqvnxiEXO4hi88+YZxaHC6QKzb5N84iRCTDeRO7ZALpir/lVmf/uXUhnwUr2O4HU8s/n6x+yNjQkHw==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">= 14.17"
}
@@ -27274,6 +25382,7 @@
"resolved": "https://registry.npmjs.org/formdata-polyfill/-/formdata-polyfill-4.0.10.tgz",
"integrity": "sha512-buewHzMvYL29jdeQTVILecSaZKnt/RJWjoZCF5OW60Z67/GmSLBkOFM7qh1PI3zFNtJbaZL5eQu1vLfazOwj4g==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"fetch-blob": "^3.1.2"
},
@@ -27355,6 +25464,16 @@
"readable-stream": "^2.0.0"
}
},
+ "node_modules/front-matter": {
+ "version": "4.0.2",
+ "resolved": "https://registry.npmjs.org/front-matter/-/front-matter-4.0.2.tgz",
+ "integrity": "sha512-I8ZuJ/qG92NWX8i5x1Y8qyj3vizhXS31OxjKDu3LKP+7/qBgfIKValiZIEwoVoJKUHlhWtYrktkxV1XsX+pPlg==",
+ "dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "js-yaml": "^3.13.1"
+ }
+ },
"node_modules/fs-constants": {
"version": "1.0.0",
"resolved": "https://registry.npmjs.org/fs-constants/-/fs-constants-1.0.0.tgz",
@@ -27429,65 +25548,6 @@
"node": "^8.16.0 || ^10.6.0 || >=11.0.0"
}
},
- "node_modules/fstream": {
- "version": "1.0.12",
- "resolved": "https://registry.npmjs.org/fstream/-/fstream-1.0.12.tgz",
- "integrity": "sha512-WvJ193OHa0GHPEL+AycEJgxvBEwyfRkN1vhjca23OaPVMCaLCXTd5qAu82AjTcgP1UJmytkOKb63Ypde7raDIg==",
- "dev": true,
- "dependencies": {
- "graceful-fs": "^4.1.2",
- "inherits": "~2.0.0",
- "mkdirp": ">=0.5 0",
- "rimraf": "2"
- },
- "engines": {
- "node": ">=0.6"
- }
- },
- "node_modules/fstream/node_modules/glob": {
- "version": "7.2.3",
- "resolved": "https://registry.npmjs.org/glob/-/glob-7.2.3.tgz",
- "integrity": "sha512-nFR0zLpU2YCaRxwoCJvL6UvCH2JFyFVIvwTLsIf21AuHlMskA1hhTdk+LlYJtOlYt9v6dvszD2BGRqBL+iQK9Q==",
- "dev": true,
- "dependencies": {
- "fs.realpath": "^1.0.0",
- "inflight": "^1.0.4",
- "inherits": "2",
- "minimatch": "^3.1.1",
- "once": "^1.3.0",
- "path-is-absolute": "^1.0.0"
- },
- "engines": {
- "node": "*"
- },
- "funding": {
- "url": "https://github.com/sponsors/isaacs"
- }
- },
- "node_modules/fstream/node_modules/mkdirp": {
- "version": "0.5.6",
- "resolved": "https://registry.npmjs.org/mkdirp/-/mkdirp-0.5.6.tgz",
- "integrity": "sha512-FP+p8RB8OWpF3YZBCrP5gtADmtXApB5AMLn+vdyA+PyxCjrCs00mjyUozssO33cwDeT3wNGdLxJ5M//YqtHAJw==",
- "dev": true,
- "dependencies": {
- "minimist": "^1.2.6"
- },
- "bin": {
- "mkdirp": "bin/cmd.js"
- }
- },
- "node_modules/fstream/node_modules/rimraf": {
- "version": "2.7.1",
- "resolved": "https://registry.npmjs.org/rimraf/-/rimraf-2.7.1.tgz",
- "integrity": "sha512-uWjbaKIK3T1OSVptzX7Nl6PvQ3qAGtKEtVRjRuazjfL3Bx5eI409VZSqgND+4UNnmzLVdPj9FqFJNPqBZFve4w==",
- "dev": true,
- "dependencies": {
- "glob": "^7.1.3"
- },
- "bin": {
- "rimraf": "bin.js"
- }
- },
"node_modules/ftp-response-parser": {
"version": "1.0.1",
"resolved": "https://registry.npmjs.org/ftp-response-parser/-/ftp-response-parser-1.0.1.tgz",
@@ -27562,68 +25622,21 @@
"url": "https://github.com/sponsors/ljharb"
}
},
- "node_modules/gauge": {
- "version": "4.0.4",
- "resolved": "https://registry.npmjs.org/gauge/-/gauge-4.0.4.tgz",
- "integrity": "sha512-f9m+BEN5jkg6a0fZjleidjN51VE1X+mPFQ2DJ0uv1V39oCLCbsGe6yjbBnp7eK7z/+GAon99a3nHuqbuuthyPg==",
- "dev": true,
- "dependencies": {
- "aproba": "^1.0.3 || ^2.0.0",
- "color-support": "^1.1.3",
- "console-control-strings": "^1.1.0",
- "has-unicode": "^2.0.1",
- "signal-exit": "^3.0.7",
- "string-width": "^4.2.3",
- "strip-ansi": "^6.0.1",
- "wide-align": "^1.1.5"
- },
- "engines": {
- "node": "^12.13.0 || ^14.15.0 || >=16.0.0"
- }
- },
- "node_modules/gauge/node_modules/emoji-regex": {
- "version": "8.0.0",
- "resolved": "https://registry.npmjs.org/emoji-regex/-/emoji-regex-8.0.0.tgz",
- "integrity": "sha512-MSjYzcWNOA0ewAHpz0MxpYFvwg6yjy1NG3xteoqz644VCo/RPgnr1/GGt+ic3iJTzQ8Eu3TdM14SawnVUmGE6A==",
- "dev": true
- },
- "node_modules/gauge/node_modules/is-fullwidth-code-point": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/is-fullwidth-code-point/-/is-fullwidth-code-point-3.0.0.tgz",
- "integrity": "sha512-zymm5+u+sCsSWyD9qNaejV3DFvhCKclKdizYaJUuHA83RLjb7nSuGnddCHGv0hk+KY7BMAlsWeK4Ueg6EV6XQg==",
- "dev": true,
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/gauge/node_modules/string-width": {
- "version": "4.2.3",
- "resolved": "https://registry.npmjs.org/string-width/-/string-width-4.2.3.tgz",
- "integrity": "sha512-wKyQRQpjJ0sIp62ErSZdGsjMJWsap5oRNihHhu6G7JVO/9jIB6UyevL+tXuOqrng8j/cxKTWyWUwvSTriiZz/g==",
- "dev": true,
- "dependencies": {
- "emoji-regex": "^8.0.0",
- "is-fullwidth-code-point": "^3.0.0",
- "strip-ansi": "^6.0.1"
- },
- "engines": {
- "node": ">=8"
- }
- },
"node_modules/geckodriver": {
- "version": "4.2.1",
- "resolved": "https://registry.npmjs.org/geckodriver/-/geckodriver-4.2.1.tgz",
- "integrity": "sha512-4m/CRk0OI8MaANRuFIahvOxYTSjlNAO2p9JmE14zxueknq6cdtB5M9UGRQ8R9aMV0bLGNVHHDnDXmoXdOwJfWg==",
+ "version": "4.5.1",
+ "resolved": "https://registry.npmjs.org/geckodriver/-/geckodriver-4.5.1.tgz",
+ "integrity": "sha512-lGCRqPMuzbRNDWJOQcUqhNqPvNsIFu6yzXF8J/6K3WCYFd2r5ckbeF7h1cxsnjA7YLSEiWzERCt6/gjZ3tW0ug==",
"dev": true,
"hasInstallScript": true,
+ "license": "MPL-2.0",
"dependencies": {
- "@wdio/logger": "^8.11.0",
+ "@wdio/logger": "^9.0.0",
+ "@zip.js/zip.js": "^2.7.48",
"decamelize": "^6.0.0",
- "http-proxy-agent": "^7.0.0",
- "https-proxy-agent": "^7.0.1",
- "node-fetch": "^3.3.1",
- "tar-fs": "^3.0.4",
- "unzipper": "^0.10.14",
+ "http-proxy-agent": "^7.0.2",
+ "https-proxy-agent": "^7.0.5",
+ "node-fetch": "^3.3.2",
+ "tar-fs": "^3.0.6",
"which": "^4.0.0"
},
"bin": {
@@ -27633,16 +25646,46 @@
"node": "^16.13 || >=18 || >=20"
}
},
- "node_modules/geckodriver/node_modules/agent-base": {
- "version": "7.1.1",
- "resolved": "https://registry.npmjs.org/agent-base/-/agent-base-7.1.1.tgz",
- "integrity": "sha512-H0TSyFNDMomMNJQBn8wFV5YC/2eJ+VXECwOadZJT554xP6cODZHPX3H9QMQECxvrgiSOP1pHjy1sMWQVYJOUOA==",
+ "node_modules/geckodriver/node_modules/@wdio/logger": {
+ "version": "9.1.3",
+ "resolved": "https://registry.npmjs.org/@wdio/logger/-/logger-9.1.3.tgz",
+ "integrity": "sha512-cumRMK/gE1uedBUw3WmWXOQ7HtB6DR8EyKQioUz2P0IJtRRpglMBdZV7Svr3b++WWawOuzZHMfbTkJQmaVt8Gw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "debug": "^4.3.4"
+ "chalk": "^5.1.2",
+ "loglevel": "^1.6.0",
+ "loglevel-plugin-prefix": "^0.8.4",
+ "strip-ansi": "^7.1.0"
},
"engines": {
- "node": ">= 14"
+ "node": ">=18.20.0"
+ }
+ },
+ "node_modules/geckodriver/node_modules/ansi-regex": {
+ "version": "6.1.0",
+ "resolved": "https://registry.npmjs.org/ansi-regex/-/ansi-regex-6.1.0.tgz",
+ "integrity": "sha512-7HSX4QQb4CspciLpVFwyRe79O3xsIZDDLER21kERQ71oaPodF8jL725AgJMFAYbooIqolJoRLuM81SpeUkpkvA==",
+ "dev": true,
+ "license": "MIT",
+ "engines": {
+ "node": ">=12"
+ },
+ "funding": {
+ "url": "https://github.com/chalk/ansi-regex?sponsor=1"
+ }
+ },
+ "node_modules/geckodriver/node_modules/chalk": {
+ "version": "5.3.0",
+ "resolved": "https://registry.npmjs.org/chalk/-/chalk-5.3.0.tgz",
+ "integrity": "sha512-dLitG79d+GV1Nb/VYcCDFivJeK1hiukt9QjRNVOsUtTy1rR1YJsmpGGTZ3qJos+uw7WmWF4wUwBd9jxjocFC2w==",
+ "dev": true,
+ "license": "MIT",
+ "engines": {
+ "node": "^12.17.0 || ^14.13 || >=16.0.0"
+ },
+ "funding": {
+ "url": "https://github.com/chalk/chalk?sponsor=1"
}
},
"node_modules/geckodriver/node_modules/data-uri-to-buffer": {
@@ -27650,6 +25693,7 @@
"resolved": "https://registry.npmjs.org/data-uri-to-buffer/-/data-uri-to-buffer-4.0.1.tgz",
"integrity": "sha512-0R9ikRb668HB7QDxT1vkpuUBtqc53YyAwMwGeUFKRojY/NWKvdZ+9UYtRfGmhqNbRkTSVpMbmyhXipFFv2cb/A==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">= 12"
}
@@ -27659,6 +25703,7 @@
"resolved": "https://registry.npmjs.org/decamelize/-/decamelize-6.0.0.tgz",
"integrity": "sha512-Fv96DCsdOgB6mdGl67MT5JaTNKRzrzill5OH5s8bjYJXVlcXyPYGyPsUkWyGV5p1TXI5esYIYMMeDJL0hEIwaA==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": "^12.20.0 || ^14.13.1 || >=16.0.0"
},
@@ -27666,38 +25711,12 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/geckodriver/node_modules/http-proxy-agent": {
- "version": "7.0.2",
- "resolved": "https://registry.npmjs.org/http-proxy-agent/-/http-proxy-agent-7.0.2.tgz",
- "integrity": "sha512-T1gkAiYYDWYx3V5Bmyu7HcfcvL7mUrTWiM6yOfa3PIphViJ/gFPbvidQ+veqSOHci/PxBcDabeUNCzpOODJZig==",
- "dev": true,
- "dependencies": {
- "agent-base": "^7.1.0",
- "debug": "^4.3.4"
- },
- "engines": {
- "node": ">= 14"
- }
- },
- "node_modules/geckodriver/node_modules/https-proxy-agent": {
- "version": "7.0.5",
- "resolved": "https://registry.npmjs.org/https-proxy-agent/-/https-proxy-agent-7.0.5.tgz",
- "integrity": "sha512-1e4Wqeblerz+tMKPIq2EMGiiWW1dIjZOksyHWSUm1rmuvw/how9hBHZ38lAGj5ID4Ik6EdkOw7NmWPy6LAwalw==",
- "dev": true,
- "license": "MIT",
- "dependencies": {
- "agent-base": "^7.0.2",
- "debug": "4"
- },
- "engines": {
- "node": ">= 14"
- }
- },
"node_modules/geckodriver/node_modules/isexe": {
"version": "3.1.1",
"resolved": "https://registry.npmjs.org/isexe/-/isexe-3.1.1.tgz",
"integrity": "sha512-LpB/54B+/2J5hqQ7imZHfdU31OlgQqx7ZicVlkm9kzg9/w8GKLEcFfJl/t7DCEDueOyBAD6zCCwTO6Fzs0NoEQ==",
"dev": true,
+ "license": "ISC",
"engines": {
"node": ">=16"
}
@@ -27707,6 +25726,7 @@
"resolved": "https://registry.npmjs.org/node-fetch/-/node-fetch-3.3.2.tgz",
"integrity": "sha512-dRB78srN/l6gqWulah9SrxeYnxeddIG30+GOqK/9OlLVyLg3HPnr6SqOWTWOXKRwC2eGYCkZ59NNuSgvSrpgOA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"data-uri-to-buffer": "^4.0.0",
"fetch-blob": "^3.1.4",
@@ -27731,11 +25751,28 @@
"once": "^1.3.1"
}
},
+ "node_modules/geckodriver/node_modules/strip-ansi": {
+ "version": "7.1.0",
+ "resolved": "https://registry.npmjs.org/strip-ansi/-/strip-ansi-7.1.0.tgz",
+ "integrity": "sha512-iq6eVVI64nQQTRYq2KtEg2d2uU7LElhTJwsH4YzIHZshxlgZms/wIc4VoDQTlG/IvVIrBKG06CrZnp0qv7hkcQ==",
+ "dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "ansi-regex": "^6.0.1"
+ },
+ "engines": {
+ "node": ">=12"
+ },
+ "funding": {
+ "url": "https://github.com/chalk/strip-ansi?sponsor=1"
+ }
+ },
"node_modules/geckodriver/node_modules/tar-fs": {
"version": "3.0.6",
"resolved": "https://registry.npmjs.org/tar-fs/-/tar-fs-3.0.6.tgz",
"integrity": "sha512-iokBDQQkUyeXhgPYaZxmczGPhnhXZ0CmrqI+MOb/WFGS9DW5wnfrLgtjUJBvz50vQ3qfRwJ62QVoCFu8mPVu5w==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"pump": "^3.0.0",
"tar-stream": "^3.1.5"
@@ -27750,6 +25787,7 @@
"resolved": "https://registry.npmjs.org/tar-stream/-/tar-stream-3.1.7.tgz",
"integrity": "sha512-qJj60CXt7IU1Ffyc3NJMjh6EkuCFej46zUqJ4J7pqYlThyd9bO0XBTmcOIhSzZJVWfsLks0+nle/j538YAW9RQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"b4a": "^1.6.4",
"fast-fifo": "^1.2.0",
@@ -27761,6 +25799,7 @@
"resolved": "https://registry.npmjs.org/which/-/which-4.0.0.tgz",
"integrity": "sha512-GlaYyEb07DPxYCKhKzplCWBJtvxZcZMrL+4UkrTSJHHPyZU4mYYTv3qaOe77H7EODLSSopAUFAc6W8U4yqvscg==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"isexe": "^3.1.1"
},
@@ -27818,15 +25857,6 @@
"node": ">=6"
}
},
- "node_modules/get-npm-tarball-url": {
- "version": "2.1.0",
- "resolved": "https://registry.npmjs.org/get-npm-tarball-url/-/get-npm-tarball-url-2.1.0.tgz",
- "integrity": "sha512-ro+DiMu5DXgRBabqXupW38h7WPZ9+Ad8UjwhvsmmN8w1sU7ab0nzAXvVZ4kqYg57OrqomRtJvepX5/xvFKNtjA==",
- "dev": true,
- "engines": {
- "node": ">=12.17"
- }
- },
"node_modules/get-own-enumerable-property-symbols": {
"version": "3.0.2",
"resolved": "https://registry.npmjs.org/get-own-enumerable-property-symbols/-/get-own-enumerable-property-symbols-3.0.2.tgz",
@@ -27838,6 +25868,7 @@
"resolved": "https://registry.npmjs.org/get-pkg-repo/-/get-pkg-repo-4.2.1.tgz",
"integrity": "sha512-2+QbHjFRfGB74v/pYWjd5OhU3TDIC2Gv/YKUTk/tCvAz0pkn/Mz6P3uByuBimLOcPvN2jYdScl3xGFSrx0jEcA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"@hutson/parse-repository-url": "^3.0.0",
"hosted-git-info": "^4.0.0",
@@ -27851,6 +25882,97 @@
"node": ">=6.9.0"
}
},
+ "node_modules/get-pkg-repo/node_modules/cliui": {
+ "version": "7.0.4",
+ "resolved": "https://registry.npmjs.org/cliui/-/cliui-7.0.4.tgz",
+ "integrity": "sha512-OcRE68cOsVMXp1Yvonl/fzkQOyjLSu/8bhPDfQt0e0/Eb283TKP20Fs2MqoPsr9SwA595rRCA+QMzYc9nBP+JQ==",
+ "dev": true,
+ "license": "ISC",
+ "dependencies": {
+ "string-width": "^4.2.0",
+ "strip-ansi": "^6.0.0",
+ "wrap-ansi": "^7.0.0"
+ }
+ },
+ "node_modules/get-pkg-repo/node_modules/emoji-regex": {
+ "version": "8.0.0",
+ "resolved": "https://registry.npmjs.org/emoji-regex/-/emoji-regex-8.0.0.tgz",
+ "integrity": "sha512-MSjYzcWNOA0ewAHpz0MxpYFvwg6yjy1NG3xteoqz644VCo/RPgnr1/GGt+ic3iJTzQ8Eu3TdM14SawnVUmGE6A==",
+ "dev": true,
+ "license": "MIT"
+ },
+ "node_modules/get-pkg-repo/node_modules/is-fullwidth-code-point": {
+ "version": "3.0.0",
+ "resolved": "https://registry.npmjs.org/is-fullwidth-code-point/-/is-fullwidth-code-point-3.0.0.tgz",
+ "integrity": "sha512-zymm5+u+sCsSWyD9qNaejV3DFvhCKclKdizYaJUuHA83RLjb7nSuGnddCHGv0hk+KY7BMAlsWeK4Ueg6EV6XQg==",
+ "dev": true,
+ "license": "MIT",
+ "engines": {
+ "node": ">=8"
+ }
+ },
+ "node_modules/get-pkg-repo/node_modules/string-width": {
+ "version": "4.2.3",
+ "resolved": "https://registry.npmjs.org/string-width/-/string-width-4.2.3.tgz",
+ "integrity": "sha512-wKyQRQpjJ0sIp62ErSZdGsjMJWsap5oRNihHhu6G7JVO/9jIB6UyevL+tXuOqrng8j/cxKTWyWUwvSTriiZz/g==",
+ "dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "emoji-regex": "^8.0.0",
+ "is-fullwidth-code-point": "^3.0.0",
+ "strip-ansi": "^6.0.1"
+ },
+ "engines": {
+ "node": ">=8"
+ }
+ },
+ "node_modules/get-pkg-repo/node_modules/wrap-ansi": {
+ "version": "7.0.0",
+ "resolved": "https://registry.npmjs.org/wrap-ansi/-/wrap-ansi-7.0.0.tgz",
+ "integrity": "sha512-YVGIj2kamLSTxw6NsZjoBxfSwsn0ycdesmc4p+Q21c5zPuZ1pl+NfxVdxPtdHvmNVOQ6XSYG4AUtyt/Fi7D16Q==",
+ "dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "ansi-styles": "^4.0.0",
+ "string-width": "^4.1.0",
+ "strip-ansi": "^6.0.0"
+ },
+ "engines": {
+ "node": ">=10"
+ },
+ "funding": {
+ "url": "https://github.com/chalk/wrap-ansi?sponsor=1"
+ }
+ },
+ "node_modules/get-pkg-repo/node_modules/y18n": {
+ "version": "5.0.8",
+ "resolved": "https://registry.npmjs.org/y18n/-/y18n-5.0.8.tgz",
+ "integrity": "sha512-0pfFzegeDWJHJIAmTLRP2DwHjdF5s7jo9tuztdQxAhINCdvS+3nGINqPd00AphqJR/0LhANUS6/+7SCb98YOfA==",
+ "dev": true,
+ "license": "ISC",
+ "engines": {
+ "node": ">=10"
+ }
+ },
+ "node_modules/get-pkg-repo/node_modules/yargs": {
+ "version": "16.2.0",
+ "resolved": "https://registry.npmjs.org/yargs/-/yargs-16.2.0.tgz",
+ "integrity": "sha512-D1mvvtDG0L5ft/jGWkLpG1+m0eQxOfaBvTNELraWj22wSVUMWxZUvYgJYcKh6jGGIkJFhH4IZPQhR4TKpc8mBw==",
+ "dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "cliui": "^7.0.2",
+ "escalade": "^3.1.1",
+ "get-caller-file": "^2.0.5",
+ "require-directory": "^2.1.1",
+ "string-width": "^4.2.0",
+ "y18n": "^5.0.5",
+ "yargs-parser": "^20.2.2"
+ },
+ "engines": {
+ "node": ">=10"
+ }
+ },
"node_modules/get-port": {
"version": "5.1.1",
"resolved": "https://registry.npmjs.org/get-port/-/get-port-5.1.1.tgz",
@@ -27944,92 +26066,12 @@
"safe-buffer": "^5.1.1"
}
},
- "node_modules/giget": {
- "version": "1.2.1",
- "resolved": "https://registry.npmjs.org/giget/-/giget-1.2.1.tgz",
- "integrity": "sha512-4VG22mopWtIeHwogGSy1FViXVo0YT+m6BrqZfz0JJFwbSsePsCdOzdLIIli5BtMp7Xe8f/o2OmBpQX2NBOC24g==",
- "dev": true,
- "dependencies": {
- "citty": "^0.1.5",
- "consola": "^3.2.3",
- "defu": "^6.1.3",
- "node-fetch-native": "^1.6.1",
- "nypm": "^0.3.3",
- "ohash": "^1.1.3",
- "pathe": "^1.1.1",
- "tar": "^6.2.0"
- },
- "bin": {
- "giget": "dist/cli.mjs"
- }
- },
- "node_modules/giget/node_modules/chownr": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/chownr/-/chownr-2.0.0.tgz",
- "integrity": "sha512-bIomtDF5KGpdogkLd9VspvFzk9KfpyyGlS8YFVZl7TGPBHL5snIOnxeshwVgPteQ9b4Eydl+pVbIyE1DcvCWgQ==",
- "dev": true,
- "engines": {
- "node": ">=10"
- }
- },
- "node_modules/giget/node_modules/consola": {
- "version": "3.2.3",
- "resolved": "https://registry.npmjs.org/consola/-/consola-3.2.3.tgz",
- "integrity": "sha512-I5qxpzLv+sJhTVEoLYNcTW+bThDCPsit0vLNKShZx6rLtpilNpmmeTPaeqJb9ZE9dV3DGaeby6Vuhrw38WjeyQ==",
- "dev": true,
- "engines": {
- "node": "^14.18.0 || >=16.10.0"
- }
- },
- "node_modules/giget/node_modules/minipass": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/minipass/-/minipass-5.0.0.tgz",
- "integrity": "sha512-3FnjYuehv9k6ovOEbyOswadCDPX1piCfhV8ncmYtHOjuPwylVWsghTLo7rabjC3Rx5xD4HDx8Wm1xnMF7S5qFQ==",
- "dev": true,
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/giget/node_modules/mkdirp": {
- "version": "1.0.4",
- "resolved": "https://registry.npmjs.org/mkdirp/-/mkdirp-1.0.4.tgz",
- "integrity": "sha512-vVqVZQyf3WLx2Shd0qJ9xuvqgAyKPLAiqITEtqW0oIUjzo3PePDd6fW9iFz30ef7Ysp/oiWqbhszeGWW2T6Gzw==",
- "dev": true,
- "bin": {
- "mkdirp": "bin/cmd.js"
- },
- "engines": {
- "node": ">=10"
- }
- },
- "node_modules/giget/node_modules/tar": {
- "version": "6.2.1",
- "resolved": "https://registry.npmjs.org/tar/-/tar-6.2.1.tgz",
- "integrity": "sha512-DZ4yORTwrbTj/7MZYq2w+/ZFdI6OZ/f9SFHR+71gIVUZhOQPHzVCLpvRnPgyaMpfWxxk/4ONva3GQSyNIKRv6A==",
- "dev": true,
- "dependencies": {
- "chownr": "^2.0.0",
- "fs-minipass": "^2.0.0",
- "minipass": "^5.0.0",
- "minizlib": "^2.1.1",
- "mkdirp": "^1.0.3",
- "yallist": "^4.0.0"
- },
- "engines": {
- "node": ">=10"
- }
- },
- "node_modules/giget/node_modules/yallist": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/yallist/-/yallist-4.0.0.tgz",
- "integrity": "sha512-3wdGidZyq5PB084XLES5TpOSRA3wjXAlIWMhum2kRcv/41Sn2emQ0dycQW4uZXLejwKvg6EsvbdlVL+FYEct7A==",
- "dev": true
- },
"node_modules/git-raw-commits": {
"version": "3.0.0",
"resolved": "https://registry.npmjs.org/git-raw-commits/-/git-raw-commits-3.0.0.tgz",
"integrity": "sha512-b5OHmZ3vAgGrDn/X0kS+9qCfNKWe4K/jFnhwzVWWg0/k5eLa3060tZShrRg8Dja5kPc+YjS0Gc6y7cRr44Lpjw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"dargs": "^7.0.0",
"meow": "^8.1.2",
@@ -28047,6 +26089,7 @@
"resolved": "https://registry.npmjs.org/git-remote-origin-url/-/git-remote-origin-url-2.0.0.tgz",
"integrity": "sha512-eU+GGrZgccNJcsDH5LkXR3PB9M958hxc7sbA8DFJjrv9j4L2P/eZfKhM+QD6wyzpiv+b1BpK0XrYCxkovtjSLw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"gitconfiglocal": "^1.0.0",
"pify": "^2.3.0"
@@ -28055,11 +26098,22 @@
"node": ">=4"
}
},
+ "node_modules/git-remote-origin-url/node_modules/pify": {
+ "version": "2.3.0",
+ "resolved": "https://registry.npmjs.org/pify/-/pify-2.3.0.tgz",
+ "integrity": "sha512-udgsAY+fTnvv7kI7aaxbqwWNb0AHiB0qBO89PZKPkoTmGOgdbrHDKD+0B2X4uTfJ/FT1R09r9gTsjUjNJotuog==",
+ "dev": true,
+ "license": "MIT",
+ "engines": {
+ "node": ">=0.10.0"
+ }
+ },
"node_modules/git-semver-tags": {
"version": "5.0.1",
"resolved": "https://registry.npmjs.org/git-semver-tags/-/git-semver-tags-5.0.1.tgz",
"integrity": "sha512-hIvOeZwRbQ+7YEUmCkHqo8FOLQZCEn18yevLHADlFPZY02KJGsu5FZt9YW/lybfK2uhWFI7Qg/07LekJiTv7iA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"meow": "^8.1.2",
"semver": "^7.0.0"
@@ -28076,16 +26130,18 @@
"resolved": "https://registry.npmjs.org/git-up/-/git-up-7.0.0.tgz",
"integrity": "sha512-ONdIrbBCFusq1Oy0sC71F5azx8bVkvtZtMJAsv+a6lz5YAmbNnLD6HAB4gptHZVLPR8S2/kVN6Gab7lryq5+lQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"is-ssh": "^1.4.0",
"parse-url": "^8.1.0"
}
},
"node_modules/git-url-parse": {
- "version": "13.1.0",
- "resolved": "https://registry.npmjs.org/git-url-parse/-/git-url-parse-13.1.0.tgz",
- "integrity": "sha512-5FvPJP/70WkIprlUZ33bm4UAaFdjcLkJLpWft1BeZKqwR0uhhNGoKwlUaPtVb4LxCSQ++erHapRak9kWGj+FCA==",
+ "version": "14.0.0",
+ "resolved": "https://registry.npmjs.org/git-url-parse/-/git-url-parse-14.0.0.tgz",
+ "integrity": "sha512-NnLweV+2A4nCvn4U/m2AoYu0pPKlsmhK9cknG7IMwsjFY1S2jxM+mAhsDxyxfCIGfGaD+dozsyX4b6vkYc83yQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"git-up": "^7.0.0"
}
@@ -28095,6 +26151,7 @@
"resolved": "https://registry.npmjs.org/gitconfiglocal/-/gitconfiglocal-1.0.0.tgz",
"integrity": "sha512-spLUXeTAVHxDtKsJc8FkFVgFtMdEN9qPGpL23VfSHx4fP4+Ds097IXLvymbnDH8FnmxX5Nr9bPw3A+AQ6mWEaQ==",
"dev": true,
+ "license": "BSD",
"dependencies": {
"ini": "^1.3.2"
}
@@ -28104,14 +26161,9 @@
"resolved": "https://registry.npmjs.org/github-from-package/-/github-from-package-0.0.0.tgz",
"integrity": "sha512-SyHy3T1v2NUXn29OsWdxmK6RwHD+vkj3v8en8AOBZ1wBQ/hCAQ5bAQTD02kW4W9tUp/3Qh6J8r9EvntiyCmOOw==",
"dev": true,
+ "license": "MIT",
"optional": true
},
- "node_modules/github-slugger": {
- "version": "1.4.0",
- "resolved": "https://registry.npmjs.org/github-slugger/-/github-slugger-1.4.0.tgz",
- "integrity": "sha512-w0dzqw/nt51xMVmlaV1+JRzN+oCa1KfcgGEWhxUG16wbdA+Xnt/yoFO8Z8x/V82ZcZ0wy6ln9QDup5avbhiDhQ==",
- "dev": true
- },
"node_modules/glob": {
"version": "7.1.2",
"resolved": "https://registry.npmjs.org/glob/-/glob-7.1.2.tgz",
@@ -28345,38 +26397,6 @@
"resolved": "https://registry.npmjs.org/graphemer/-/graphemer-1.4.0.tgz",
"integrity": "sha512-EtKwoO6kxCL9WO5xipiHTZlSzBm7WLT627TqC/uVRd0HKmq8NXyebnNYxDoBi7wt8eTWrUrKXCOVaFq9x1kgag=="
},
- "node_modules/gunzip-maybe": {
- "version": "1.4.2",
- "resolved": "https://registry.npmjs.org/gunzip-maybe/-/gunzip-maybe-1.4.2.tgz",
- "integrity": "sha512-4haO1M4mLO91PW57BMsDFf75UmwoRX0GkdD+Faw+Lr+r/OZrOCS0pIBwOL1xCKQqnQzbNFGgK2V2CpBUPeFNTw==",
- "dev": true,
- "dependencies": {
- "browserify-zlib": "^0.1.4",
- "is-deflate": "^1.0.0",
- "is-gzip": "^1.0.0",
- "peek-stream": "^1.1.0",
- "pumpify": "^1.3.3",
- "through2": "^2.0.3"
- },
- "bin": {
- "gunzip-maybe": "bin.js"
- }
- },
- "node_modules/gunzip-maybe/node_modules/browserify-zlib": {
- "version": "0.1.4",
- "resolved": "https://registry.npmjs.org/browserify-zlib/-/browserify-zlib-0.1.4.tgz",
- "integrity": "sha512-19OEpq7vWgsH6WkvkBJQDFvJS1uPcbFOQ4v9CU839dO+ZZXUZO6XpE6hNCqvlIIj+4fZvRiJ6DsAQ382GwiyTQ==",
- "dev": true,
- "dependencies": {
- "pako": "~0.2.0"
- }
- },
- "node_modules/gunzip-maybe/node_modules/pako": {
- "version": "0.2.9",
- "resolved": "https://registry.npmjs.org/pako/-/pako-0.2.9.tgz",
- "integrity": "sha512-NUcwaKxUxWrZLpDG+z/xZaCgQITkA/Dv4V/T6bw7VON6l1Xz/VnrBqrYjZQ12TamKHzITTfOEIYUj48y2KXImA==",
- "dev": true
- },
"node_modules/handle-thing": {
"version": "2.0.1",
"resolved": "https://registry.npmjs.org/handle-thing/-/handle-thing-2.0.1.tgz",
@@ -28741,11 +26761,6 @@
"node": ">=10"
}
},
- "node_modules/hosted-git-info/node_modules/yallist": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/yallist/-/yallist-4.0.0.tgz",
- "integrity": "sha512-3wdGidZyq5PB084XLES5TpOSRA3wjXAlIWMhum2kRcv/41Sn2emQ0dycQW4uZXLejwKvg6EsvbdlVL+FYEct7A=="
- },
"node_modules/hpack.js": {
"version": "2.1.6",
"resolved": "https://registry.npmjs.org/hpack.js/-/hpack.js-2.1.6.tgz",
@@ -28835,9 +26850,9 @@
}
},
"node_modules/html-webpack-plugin": {
- "version": "5.6.0",
- "resolved": "https://registry.npmjs.org/html-webpack-plugin/-/html-webpack-plugin-5.6.0.tgz",
- "integrity": "sha512-iwaY4wzbe48AfKLZ/Cc8k0L+FKG6oSNRaZ8x5A/T/IVDGyXcbHncM9TdDa93wn0FsSm82FhTKW7f3vS61thXAw==",
+ "version": "5.6.3",
+ "resolved": "https://registry.npmjs.org/html-webpack-plugin/-/html-webpack-plugin-5.6.3.tgz",
+ "integrity": "sha512-QSf1yjtSAsmf7rYBV7XX86uua4W/vkhIt0xNXKbsi2foEeW7vjJQz4bhnpL3xH+l1ryl1680uNv968Z+X6jSYg==",
"dev": true,
"dependencies": {
"@types/html-minifier-terser": "^6.0.0",
@@ -29045,32 +27060,23 @@
}
},
"node_modules/http-proxy-agent": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/http-proxy-agent/-/http-proxy-agent-5.0.0.tgz",
- "integrity": "sha512-n2hY8YdoRE1i7r6M0w9DIw5GgZN0G25P8zLCRQ8rjXtTU3vsNFBI/vWK/UIeE6g5MUUz6avwAPXmL6Fy9D/90w==",
- "dev": true,
+ "version": "7.0.2",
+ "resolved": "https://registry.npmjs.org/http-proxy-agent/-/http-proxy-agent-7.0.2.tgz",
+ "integrity": "sha512-T1gkAiYYDWYx3V5Bmyu7HcfcvL7mUrTWiM6yOfa3PIphViJ/gFPbvidQ+veqSOHci/PxBcDabeUNCzpOODJZig==",
+ "license": "MIT",
"dependencies": {
- "@tootallnate/once": "2",
- "agent-base": "6",
- "debug": "4"
+ "agent-base": "^7.1.0",
+ "debug": "^4.3.4"
},
"engines": {
- "node": ">= 6"
- }
- },
- "node_modules/http-proxy-agent/node_modules/@tootallnate/once": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/@tootallnate/once/-/once-2.0.0.tgz",
- "integrity": "sha512-XCuKFP5PS55gnMVu3dty8KPatLqUoy/ZYzDzAGCQ8JNFCkLXzmI7vNHCR+XpbZaMWQK/vQubr7PkYq8g470J/A==",
- "dev": true,
- "engines": {
- "node": ">= 10"
+ "node": ">= 14"
}
},
"node_modules/http-proxy-middleware": {
- "version": "2.0.6",
- "resolved": "https://registry.npmjs.org/http-proxy-middleware/-/http-proxy-middleware-2.0.6.tgz",
- "integrity": "sha512-ya/UeJ6HVBYxrgYotAZo1KvPWlgB48kUJLDePFeneHsVujFaW5WNj2NgWCAE//B1Dl02BIfYlpNgBy8Kf8Rjmw==",
+ "version": "2.0.7",
+ "resolved": "https://registry.npmjs.org/http-proxy-middleware/-/http-proxy-middleware-2.0.7.tgz",
+ "integrity": "sha512-fgVY8AV7qU7z/MmXJ/rxwbrtQH4jBQ9m7kp3llF0liB7glmFeVZFBepQb32T3y8n8k2+AEYuMPCpinYW+/CuRA==",
+ "license": "MIT",
"dependencies": {
"@types/http-proxy": "^1.17.8",
"http-proxy": "^1.18.1",
@@ -29126,15 +27132,16 @@
"dev": true
},
"node_modules/https-proxy-agent": {
- "version": "5.0.1",
- "resolved": "https://registry.npmjs.org/https-proxy-agent/-/https-proxy-agent-5.0.1.tgz",
- "integrity": "sha512-dFcAjpTQFgoLMzC2VwU+C/CbS7uRL0lWmxDITmqm7C+7F0Odmj6s9l6alZc6AELXhrnggM2CeWSXHGOdX2YtwA==",
+ "version": "7.0.6",
+ "resolved": "https://registry.npmjs.org/https-proxy-agent/-/https-proxy-agent-7.0.6.tgz",
+ "integrity": "sha512-vK9P5/iUfdl95AI+JVyUuIcVtd4ofvtrOr3HNtM2yxC9bnMbEdp3x01OhQNnjb8IJYi38VlTE3mBXwcfvywuSw==",
+ "license": "MIT",
"dependencies": {
- "agent-base": "6",
+ "agent-base": "^7.1.2",
"debug": "4"
},
"engines": {
- "node": ">= 6"
+ "node": ">= 14"
}
},
"node_modules/human-signals": {
@@ -29145,15 +27152,6 @@
"node": ">=8.12.0"
}
},
- "node_modules/humanize-ms": {
- "version": "1.2.1",
- "resolved": "https://registry.npmjs.org/humanize-ms/-/humanize-ms-1.2.1.tgz",
- "integrity": "sha512-Fl70vYtsAFb/C06PTS9dZBo7ihau+Tu/DNCk/OyHhea07S+aeMWpFFkUaXRa8fI+ScZbEI8dfSxwY7gxZ9SAVQ==",
- "dev": true,
- "dependencies": {
- "ms": "^2.0.0"
- }
- },
"node_modules/husky": {
"version": "7.0.0",
"resolved": "https://registry.npmjs.org/husky/-/husky-7.0.0.tgz",
@@ -29254,6 +27252,18 @@
"resolved": "https://registry.npmjs.org/image-ssim/-/image-ssim-0.2.0.tgz",
"integrity": "sha512-W7+sO6/yhxy83L0G7xR8YAc5Z5QFtYEXXRV6EaE8tuYBZJnA3gVgp3q7X7muhLZVodeb9UfvjSbwt9VJwjIYAg=="
},
+ "node_modules/immediate": {
+ "version": "3.0.6",
+ "resolved": "https://registry.npmjs.org/immediate/-/immediate-3.0.6.tgz",
+ "integrity": "sha512-XXOFtyqDjNDAQxVfYxuF7g9Il/IbWmmlQg2MYKOH8ExIT1qg6xc4zyS3HaEEATgs1btfzxq15ciUiY7gjSXRGQ==",
+ "license": "MIT"
+ },
+ "node_modules/immutable": {
+ "version": "4.3.7",
+ "resolved": "https://registry.npmjs.org/immutable/-/immutable-4.3.7.tgz",
+ "integrity": "sha512-1hqclzwYwjRDFLjcFxOM5AYkkG0rpFPpr1RLPMEuGczoS7YA8gLhy8SWXYRAA/XwfEHpfo3cw5JGioS32fnMRw==",
+ "license": "MIT"
+ },
"node_modules/import-fresh": {
"version": "3.3.0",
"resolved": "https://registry.npmjs.org/import-fresh/-/import-fresh-3.3.0.tgz",
@@ -29376,10 +27386,11 @@
"integrity": "sha512-1/bPE89IZhyf7dr5Pkz7b4UyVXy5pEt7PTEfye15UEn3AK8+2zwcDCfKk9Pwun4ltfhOSszOrReSsFcDKw/yoA=="
},
"node_modules/import-meta-resolve": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/import-meta-resolve/-/import-meta-resolve-3.0.0.tgz",
- "integrity": "sha512-4IwhLhNNA8yy445rPjD/lWh++7hMDOml2eHtd58eG7h+qK3EryMuuRbsHGPikCoAgIkkDnckKfWSk2iDla/ejg==",
+ "version": "3.1.1",
+ "resolved": "https://registry.npmjs.org/import-meta-resolve/-/import-meta-resolve-3.1.1.tgz",
+ "integrity": "sha512-qeywsE/KC3w9Fd2ORrRDUw6nS/nLwZpXgfrOc2IILvZYnCaEMd+D56Vfg9k4G29gIeVi3XKql1RQatME8iYsiw==",
"dev": true,
+ "license": "MIT",
"funding": {
"type": "github",
"url": "https://github.com/sponsors/wooorm"
@@ -29435,57 +27446,51 @@
"integrity": "sha512-JV/yugV2uzW5iMRSiZAyDtQd+nxtUnjeLt0acNdw98kKLrvuRVyB80tsREOE7yvGVgalhZ6RNXCmEHkUKBKxew=="
},
"node_modules/init-package-json": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/init-package-json/-/init-package-json-5.0.0.tgz",
- "integrity": "sha512-kBhlSheBfYmq3e0L1ii+VKe3zBTLL5lDCDWR+f9dLmEGSB3MqLlMlsolubSsyI88Bg6EA+BIMlomAnQ1SwgQBw==",
+ "version": "6.0.3",
+ "resolved": "https://registry.npmjs.org/init-package-json/-/init-package-json-6.0.3.tgz",
+ "integrity": "sha512-Zfeb5ol+H+eqJWHTaGca9BovufyGeIfr4zaaBorPmJBMrJ+KBnN+kQx2ZtXdsotUTgldHmHQV44xvUWOUA7E2w==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "npm-package-arg": "^10.0.0",
+ "@npmcli/package-json": "^5.0.0",
+ "npm-package-arg": "^11.0.0",
"promzard": "^1.0.0",
- "read": "^2.0.0",
- "read-package-json": "^6.0.0",
+ "read": "^3.0.1",
"semver": "^7.3.5",
"validate-npm-package-license": "^3.0.4",
"validate-npm-package-name": "^5.0.0"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/init-package-json/node_modules/hosted-git-info": {
- "version": "6.1.1",
- "resolved": "https://registry.npmjs.org/hosted-git-info/-/hosted-git-info-6.1.1.tgz",
- "integrity": "sha512-r0EI+HBMcXadMrugk0GCQ+6BQV39PiWAZVfq7oIckeGiN7sjRGyQxPdft3nQekFTCQbYxLBH+/axZMeH8UX6+w==",
+ "version": "7.0.2",
+ "resolved": "https://registry.npmjs.org/hosted-git-info/-/hosted-git-info-7.0.2.tgz",
+ "integrity": "sha512-puUZAUKT5m8Zzvs72XWy3HtvVbTWljRE66cP60bxJzAqf2DgICo7lYTY2IHUmLnNpjYvw5bvmoHvPc0QO2a62w==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "lru-cache": "^7.5.1"
+ "lru-cache": "^10.0.1"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
- }
- },
- "node_modules/init-package-json/node_modules/lru-cache": {
- "version": "7.18.3",
- "resolved": "https://registry.npmjs.org/lru-cache/-/lru-cache-7.18.3.tgz",
- "integrity": "sha512-jumlc0BIUrS3qJGgIkWZsyfAM7NCWiBcCDhnd+3NNM5KbBmLTgHVfWBcg6W+rLUsIpzpERPsvwUP7CckAQSOoA==",
- "dev": true,
- "engines": {
- "node": ">=12"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/init-package-json/node_modules/npm-package-arg": {
- "version": "10.1.0",
- "resolved": "https://registry.npmjs.org/npm-package-arg/-/npm-package-arg-10.1.0.tgz",
- "integrity": "sha512-uFyyCEmgBfZTtrKk/5xDfHp6+MdrqGotX/VoOyEEl3mBwiEE5FlBaePanazJSVMPT7vKepcjYBY2ztg9A3yPIA==",
+ "version": "11.0.3",
+ "resolved": "https://registry.npmjs.org/npm-package-arg/-/npm-package-arg-11.0.3.tgz",
+ "integrity": "sha512-sHGJy8sOC1YraBywpzQlIKBE4pBbGbiF95U6Auspzyem956E0+FtDtsx1ZxlOJkQCZ1AFXAY/yuvtFYrOxF+Bw==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "hosted-git-info": "^6.0.0",
- "proc-log": "^3.0.0",
+ "hosted-git-info": "^7.0.0",
+ "proc-log": "^4.0.0",
"semver": "^7.3.5",
"validate-npm-package-name": "^5.0.0"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/init-package-json/node_modules/validate-npm-package-name": {
@@ -29493,6 +27498,7 @@
"resolved": "https://registry.npmjs.org/validate-npm-package-name/-/validate-npm-package-name-5.0.1.tgz",
"integrity": "sha512-OljLrQ9SQdOUqTaQxqL5dEfZWrXExyyWsozYlAWFawPVNuD83igl7uJD2RTkNMbniIYgt8l81eCJGIdQF7avLQ==",
"dev": true,
+ "license": "ISC",
"engines": {
"node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
@@ -29658,19 +27664,17 @@
}
},
"node_modules/intl-messageformat": {
- "version": "4.4.0",
- "resolved": "https://registry.npmjs.org/intl-messageformat/-/intl-messageformat-4.4.0.tgz",
- "integrity": "sha512-z+Bj2rS3LZSYU4+sNitdHrwnBhr0wO80ZJSW8EzKDBowwUe3Q/UsvgCGjrwa+HPzoGCLEb9HAjfJgo4j2Sac8w==",
+ "version": "10.7.7",
+ "resolved": "https://registry.npmjs.org/intl-messageformat/-/intl-messageformat-10.7.7.tgz",
+ "integrity": "sha512-F134jIoeYMro/3I0h08D0Yt4N9o9pjddU/4IIxMMURqbAtI2wu70X8hvG1V48W49zXHXv3RKSF/po+0fDfsGjA==",
+ "license": "BSD-3-Clause",
"dependencies": {
- "intl-messageformat-parser": "^1.8.1"
+ "@formatjs/ecma402-abstract": "2.2.4",
+ "@formatjs/fast-memoize": "2.2.3",
+ "@formatjs/icu-messageformat-parser": "2.9.4",
+ "tslib": "2"
}
},
- "node_modules/intl-messageformat-parser": {
- "version": "1.8.1",
- "resolved": "https://registry.npmjs.org/intl-messageformat-parser/-/intl-messageformat-parser-1.8.1.tgz",
- "integrity": "sha512-IMSCKVf0USrM/959vj3xac7s8f87sc+80Y/ipBzdKy4ifBv5Gsj2tZ41EAaURVg01QU71fYr77uA8Meh6kELbg==",
- "deprecated": "We've written a new parser that's 6x faster and is backwards compatible. Please use @formatjs/icu-messageformat-parser"
- },
"node_modules/invariant": {
"version": "2.2.4",
"resolved": "https://registry.npmjs.org/invariant/-/invariant-2.2.4.tgz",
@@ -29719,15 +27723,6 @@
"node": ">=8"
}
},
- "node_modules/is-absolute-url": {
- "version": "3.0.3",
- "resolved": "https://registry.npmjs.org/is-absolute-url/-/is-absolute-url-3.0.3.tgz",
- "integrity": "sha512-opmNIX7uFnS96NtPmhWQgQx6/NYFgsUXYMllcfzwWKUMwfo8kku1TvE6hkNcH+Q1ts5cMVrsY7j0bxXQDciu9Q==",
- "dev": true,
- "engines": {
- "node": ">=8"
- }
- },
"node_modules/is-accessor-descriptor": {
"version": "1.0.1",
"resolved": "https://registry.npmjs.org/is-accessor-descriptor/-/is-accessor-descriptor-1.0.1.tgz",
@@ -29927,12 +27922,6 @@
"resolved": "https://registry.npmjs.org/is-decimal/-/is-decimal-1.0.2.tgz",
"integrity": "sha512-TRzl7mOCchnhchN+f3ICUCzYvL9ul7R+TYOsZ8xia++knyZAJfv/uA1FvQXsAnYIl1T3B2X5E/J7Wb1QXiIBXg=="
},
- "node_modules/is-deflate": {
- "version": "1.0.0",
- "resolved": "https://registry.npmjs.org/is-deflate/-/is-deflate-1.0.0.tgz",
- "integrity": "sha512-YDoFpuZWu1VRXlsnlYMzKyVRITXj7Ej/V9gXQ2/pAe7X1J7M/RNOqaIYi6qUn+B7nGyB9pDXrv02dsB58d2ZAQ==",
- "dev": true
- },
"node_modules/is-descriptor": {
"version": "0.1.7",
"resolved": "https://registry.npmjs.org/is-descriptor/-/is-descriptor-0.1.7.tgz",
@@ -30026,15 +28015,6 @@
"node": ">=0.10.0"
}
},
- "node_modules/is-gzip": {
- "version": "1.0.0",
- "resolved": "https://registry.npmjs.org/is-gzip/-/is-gzip-1.0.0.tgz",
- "integrity": "sha512-rcfALRIb1YewtnksfRIHGcIY93QnK8BIQ/2c9yDYcG/Y6+vRoJuTWBmmSEbyLLYtXm7q35pHOHbZFQBaLrhlWQ==",
- "dev": true,
- "engines": {
- "node": ">=0.10.0"
- }
- },
"node_modules/is-hexadecimal": {
"version": "1.0.2",
"resolved": "https://registry.npmjs.org/is-hexadecimal/-/is-hexadecimal-1.0.2.tgz",
@@ -30083,7 +28063,8 @@
"version": "1.0.1",
"resolved": "https://registry.npmjs.org/is-lambda/-/is-lambda-1.0.1.tgz",
"integrity": "sha512-z7CMFGNrENq5iFB9Bqo64Xk6Y9sg+epq1myIcdHaGnbMTYOxvzsEtdYqQUylB7LxfkvgrrjP32T6Ywciio9UIQ==",
- "dev": true
+ "dev": true,
+ "license": "MIT"
},
"node_modules/is-map": {
"version": "2.0.2",
@@ -30094,22 +28075,6 @@
"url": "https://github.com/sponsors/ljharb"
}
},
- "node_modules/is-nan": {
- "version": "1.3.2",
- "resolved": "https://registry.npmjs.org/is-nan/-/is-nan-1.3.2.tgz",
- "integrity": "sha512-E+zBKpQ2t6MEo1VsonYmluk9NxGrbzpeeLC2xIViuO2EjU2xsXsBPwTr3Ykv9l08UYEVEdWeRZNouaZqF6RN0w==",
- "dev": true,
- "dependencies": {
- "call-bind": "^1.0.0",
- "define-properties": "^1.1.3"
- },
- "engines": {
- "node": ">= 0.4"
- },
- "funding": {
- "url": "https://github.com/sponsors/ljharb"
- }
- },
"node_modules/is-negative-zero": {
"version": "2.0.2",
"resolved": "https://registry.npmjs.org/is-negative-zero/-/is-negative-zero-2.0.2.tgz",
@@ -30288,6 +28253,7 @@
"resolved": "https://registry.npmjs.org/is-ssh/-/is-ssh-1.4.0.tgz",
"integrity": "sha512-x7+VxdxOdlV3CYpjvRLBv5Lo9OJerlYanjwFrPR9fuGPjCiNiCzFgAWpiLAohSbsnH4ZAys3SBh+hq5rJosxUQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"protocols": "^2.0.1"
}
@@ -30334,6 +28300,7 @@
"resolved": "https://registry.npmjs.org/is-text-path/-/is-text-path-1.0.1.tgz",
"integrity": "sha512-xFuJpne9oFz5qDaodwmmG08e3CawH/2ZV8Qqza1Ko7Sk8POWbkRdwIoAWVhqvq0XeUzANEhKo2n0IXUGBm7A/w==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"text-extensions": "^1.0.0"
},
@@ -30555,16 +28522,13 @@
}
},
"node_modules/jackspeak": {
- "version": "2.3.6",
- "resolved": "https://registry.npmjs.org/jackspeak/-/jackspeak-2.3.6.tgz",
- "integrity": "sha512-N3yCS/NegsOBokc8GAdM8UcmfsKiSS8cipheD/nivzr700H+nsMOxJjQnvwOcRYVuFkdH0wGUvW2WbXGmrZGbQ==",
- "dev": true,
+ "version": "3.4.3",
+ "resolved": "https://registry.npmjs.org/jackspeak/-/jackspeak-3.4.3.tgz",
+ "integrity": "sha512-OGlZQpz2yfahA/Rd1Y8Cd9SIEsqvXkLVoSw/cgwhnhFMDbsQFeZYoJJ7bIZBS9BcamUW96asq/npPWugM+RQBw==",
+ "license": "BlueOak-1.0.0",
"dependencies": {
"@isaacs/cliui": "^8.0.2"
},
- "engines": {
- "node": ">=14"
- },
"funding": {
"url": "https://github.com/sponsors/isaacs"
},
@@ -30638,19 +28602,6 @@
"node": "^14.15.0 || ^16.10.0 || >=18.0.0"
}
},
- "node_modules/jest-changed-files/node_modules/cross-spawn": {
- "version": "7.0.3",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-7.0.3.tgz",
- "integrity": "sha512-iRDPJKUPVEND7dHPO8rkbOnPpyDygcDFtWjpeWNCgy8WP2rXcxXL8TskReQl6OrB2G7+UJrags1q15Fudc7G6w==",
- "dependencies": {
- "path-key": "^3.1.0",
- "shebang-command": "^2.0.0",
- "which": "^2.0.1"
- },
- "engines": {
- "node": ">= 8"
- }
- },
"node_modules/jest-changed-files/node_modules/execa": {
"version": "5.1.1",
"resolved": "https://registry.npmjs.org/execa/-/execa-5.1.1.tgz",
@@ -30744,39 +28695,6 @@
"node": ">=8"
}
},
- "node_modules/jest-changed-files/node_modules/shebang-command": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/shebang-command/-/shebang-command-2.0.0.tgz",
- "integrity": "sha512-kHxr2zZpYtdmrN1qDjrrX/Z1rR1kG8Dx+gkpK1G4eXmvXswmcE1hTWBWYUzlraYw1/yZp6YuDY77YtvbN0dmDA==",
- "dependencies": {
- "shebang-regex": "^3.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/jest-changed-files/node_modules/shebang-regex": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/shebang-regex/-/shebang-regex-3.0.0.tgz",
- "integrity": "sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==",
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/jest-changed-files/node_modules/which": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
- "integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
- "dependencies": {
- "isexe": "^2.0.0"
- },
- "bin": {
- "node-which": "bin/node-which"
- },
- "engines": {
- "node": ">= 8"
- }
- },
"node_modules/jest-circus": {
"version": "29.6.2",
"resolved": "https://registry.npmjs.org/jest-circus/-/jest-circus-29.6.2.tgz",
@@ -30853,94 +28771,6 @@
}
}
},
- "node_modules/jest-cli/node_modules/cliui": {
- "version": "8.0.1",
- "resolved": "https://registry.npmjs.org/cliui/-/cliui-8.0.1.tgz",
- "integrity": "sha512-BSeNnyus75C4//NQ9gQt1/csTXyo/8Sb+afLAkzAptFuMsod9HFokGNudZpi/oQV73hnVK+sR+5PVRMd+Dr7YQ==",
- "dependencies": {
- "string-width": "^4.2.0",
- "strip-ansi": "^6.0.1",
- "wrap-ansi": "^7.0.0"
- },
- "engines": {
- "node": ">=12"
- }
- },
- "node_modules/jest-cli/node_modules/emoji-regex": {
- "version": "8.0.0",
- "resolved": "https://registry.npmjs.org/emoji-regex/-/emoji-regex-8.0.0.tgz",
- "integrity": "sha512-MSjYzcWNOA0ewAHpz0MxpYFvwg6yjy1NG3xteoqz644VCo/RPgnr1/GGt+ic3iJTzQ8Eu3TdM14SawnVUmGE6A=="
- },
- "node_modules/jest-cli/node_modules/is-fullwidth-code-point": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/is-fullwidth-code-point/-/is-fullwidth-code-point-3.0.0.tgz",
- "integrity": "sha512-zymm5+u+sCsSWyD9qNaejV3DFvhCKclKdizYaJUuHA83RLjb7nSuGnddCHGv0hk+KY7BMAlsWeK4Ueg6EV6XQg==",
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/jest-cli/node_modules/string-width": {
- "version": "4.2.3",
- "resolved": "https://registry.npmjs.org/string-width/-/string-width-4.2.3.tgz",
- "integrity": "sha512-wKyQRQpjJ0sIp62ErSZdGsjMJWsap5oRNihHhu6G7JVO/9jIB6UyevL+tXuOqrng8j/cxKTWyWUwvSTriiZz/g==",
- "dependencies": {
- "emoji-regex": "^8.0.0",
- "is-fullwidth-code-point": "^3.0.0",
- "strip-ansi": "^6.0.1"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/jest-cli/node_modules/wrap-ansi": {
- "version": "7.0.0",
- "resolved": "https://registry.npmjs.org/wrap-ansi/-/wrap-ansi-7.0.0.tgz",
- "integrity": "sha512-YVGIj2kamLSTxw6NsZjoBxfSwsn0ycdesmc4p+Q21c5zPuZ1pl+NfxVdxPtdHvmNVOQ6XSYG4AUtyt/Fi7D16Q==",
- "dependencies": {
- "ansi-styles": "^4.0.0",
- "string-width": "^4.1.0",
- "strip-ansi": "^6.0.0"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/chalk/wrap-ansi?sponsor=1"
- }
- },
- "node_modules/jest-cli/node_modules/y18n": {
- "version": "5.0.8",
- "resolved": "https://registry.npmjs.org/y18n/-/y18n-5.0.8.tgz",
- "integrity": "sha512-0pfFzegeDWJHJIAmTLRP2DwHjdF5s7jo9tuztdQxAhINCdvS+3nGINqPd00AphqJR/0LhANUS6/+7SCb98YOfA==",
- "engines": {
- "node": ">=10"
- }
- },
- "node_modules/jest-cli/node_modules/yargs": {
- "version": "17.7.2",
- "resolved": "https://registry.npmjs.org/yargs/-/yargs-17.7.2.tgz",
- "integrity": "sha512-7dSzzRQ++CKnNI/krKnYRV7JKKPUXMEh61soaHKg9mrWEhzFWhFnxPxGl+69cD1Ou63C13NUPCnmIcrvqCuM6w==",
- "dependencies": {
- "cliui": "^8.0.1",
- "escalade": "^3.1.1",
- "get-caller-file": "^2.0.5",
- "require-directory": "^2.1.1",
- "string-width": "^4.2.3",
- "y18n": "^5.0.5",
- "yargs-parser": "^21.1.1"
- },
- "engines": {
- "node": ">=12"
- }
- },
- "node_modules/jest-cli/node_modules/yargs-parser": {
- "version": "21.1.1",
- "resolved": "https://registry.npmjs.org/yargs-parser/-/yargs-parser-21.1.1.tgz",
- "integrity": "sha512-tVpsJW7DdjecAiFpbIB1e3qxIQsE6NoPc5/eTdrbbIC4h0LVsWhnoa3g+m2HclBIujHzsxZ4VJVA+GUuc2/LBw==",
- "engines": {
- "node": ">=12"
- }
- },
"node_modules/jest-config": {
"version": "29.6.2",
"resolved": "https://registry.npmjs.org/jest-config/-/jest-config-29.6.2.tgz",
@@ -31015,6 +28845,61 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
+ "node_modules/jest-dev-server": {
+ "version": "10.1.4",
+ "resolved": "https://registry.npmjs.org/jest-dev-server/-/jest-dev-server-10.1.4.tgz",
+ "integrity": "sha512-bGQ6sedNGtT6AFHhCVqGTXMPz7UyJi/ZrhNBgyqsP0XU9N8acCEIfqZEA22rOaZ+NdEVsaltk6tL7UT6aXfI7w==",
+ "license": "MIT",
+ "dependencies": {
+ "chalk": "^4.1.2",
+ "cwd": "^0.10.0",
+ "find-process": "^1.4.7",
+ "prompts": "^2.4.2",
+ "spawnd": "^10.1.4",
+ "tree-kill": "^1.2.2",
+ "wait-on": "^8.0.1"
+ },
+ "engines": {
+ "node": ">=16"
+ }
+ },
+ "node_modules/jest-dev-server/node_modules/chalk": {
+ "version": "4.1.2",
+ "resolved": "https://registry.npmjs.org/chalk/-/chalk-4.1.2.tgz",
+ "integrity": "sha512-oKnbhFyRIXpUuez8iBMmyEa4nbj4IOQyuhc/wy9kY7/WVPcwIO9VA668Pu8RkO7+0G76SLROeyw9CpQ061i4mA==",
+ "license": "MIT",
+ "dependencies": {
+ "ansi-styles": "^4.1.0",
+ "supports-color": "^7.1.0"
+ },
+ "engines": {
+ "node": ">=10"
+ },
+ "funding": {
+ "url": "https://github.com/chalk/chalk?sponsor=1"
+ }
+ },
+ "node_modules/jest-dev-server/node_modules/has-flag": {
+ "version": "4.0.0",
+ "resolved": "https://registry.npmjs.org/has-flag/-/has-flag-4.0.0.tgz",
+ "integrity": "sha512-EykJT/Q1KjTWctppgIAgfSO0tKVuZUjhgMr17kqTumMl6Afv3EISleU7qZUzoXDFTAHTDC4NOoG/ZxU3EvlMPQ==",
+ "license": "MIT",
+ "engines": {
+ "node": ">=8"
+ }
+ },
+ "node_modules/jest-dev-server/node_modules/supports-color": {
+ "version": "7.2.0",
+ "resolved": "https://registry.npmjs.org/supports-color/-/supports-color-7.2.0.tgz",
+ "integrity": "sha512-qpCAvRl9stuOHveKsn7HncJRvv501qIacKzQlO/+Lwxc9+0q2wLyv4Dfvt80/DPn2pqOBsJdDiogXGR9+OvwRw==",
+ "license": "MIT",
+ "dependencies": {
+ "has-flag": "^4.0.0"
+ },
+ "engines": {
+ "node": ">=8"
+ }
+ },
"node_modules/jest-diff": {
"version": "29.7.0",
"resolved": "https://registry.npmjs.org/jest-diff/-/jest-diff-29.7.0.tgz",
@@ -31826,18 +29711,6 @@
"node": ">= 14"
}
},
- "node_modules/jsdom/node_modules/http-proxy-agent": {
- "version": "7.0.2",
- "resolved": "https://registry.npmjs.org/http-proxy-agent/-/http-proxy-agent-7.0.2.tgz",
- "integrity": "sha512-T1gkAiYYDWYx3V5Bmyu7HcfcvL7mUrTWiM6yOfa3PIphViJ/gFPbvidQ+veqSOHci/PxBcDabeUNCzpOODJZig==",
- "dependencies": {
- "agent-base": "^7.1.0",
- "debug": "^4.3.4"
- },
- "engines": {
- "node": ">= 14"
- }
- },
"node_modules/jsdom/node_modules/https-proxy-agent": {
"version": "7.0.5",
"resolved": "https://registry.npmjs.org/https-proxy-agent/-/https-proxy-agent-7.0.5.tgz",
@@ -32001,6 +29874,16 @@
"integrity": "sha512-xHjhDr3cNBK0BzdUJSPXZntQUx/mwMS5Rw4A7lPJ90XGAO6ISP/ePDNuo0vhqOZU+UD5JoodwCAAoZQd3FeAKw==",
"dev": true
},
+ "node_modules/json-stringify-nice": {
+ "version": "1.1.4",
+ "resolved": "https://registry.npmjs.org/json-stringify-nice/-/json-stringify-nice-1.1.4.tgz",
+ "integrity": "sha512-5Z5RFW63yxReJ7vANgW6eZFGWaQvnPE3WNmZoOJrSkGju2etKA2L5rrOa1sm877TVTFt57A80BH1bArcmlLfPw==",
+ "dev": true,
+ "license": "ISC",
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
+ }
+ },
"node_modules/json-stringify-safe": {
"version": "5.0.1",
"resolved": "https://registry.npmjs.org/json-stringify-safe/-/json-stringify-safe-5.0.1.tgz",
@@ -32062,13 +29945,15 @@
"dev": true,
"engines": [
"node >= 0.2.0"
- ]
+ ],
+ "license": "MIT"
},
"node_modules/JSONStream": {
"version": "1.3.5",
"resolved": "https://registry.npmjs.org/JSONStream/-/JSONStream-1.3.5.tgz",
"integrity": "sha512-E+iruNOY8VV9s4JEbe1aNEm6MiszPRr/UfcHMz0TQh1BXSxHK+ASV1R6W4HpjBhSeS+54PIsAMCBmwD06LLsqQ==",
"dev": true,
+ "license": "(MIT OR Apache-2.0)",
"dependencies": {
"jsonparse": "^1.2.0",
"through": ">=2.2.7 <3"
@@ -32094,6 +29979,20 @@
"node": ">=4.0"
}
},
+ "node_modules/just-diff": {
+ "version": "6.0.2",
+ "resolved": "https://registry.npmjs.org/just-diff/-/just-diff-6.0.2.tgz",
+ "integrity": "sha512-S59eriX5u3/QhMNq3v/gm8Kd0w8OS6Tz2FS1NG4blv+z0MuQcBRJyFWjdovM0Rad4/P4aUPFtnkNjMjyMlMSYA==",
+ "dev": true,
+ "license": "MIT"
+ },
+ "node_modules/just-diff-apply": {
+ "version": "5.5.0",
+ "resolved": "https://registry.npmjs.org/just-diff-apply/-/just-diff-apply-5.5.0.tgz",
+ "integrity": "sha512-OYTthRfSh55WOItVqwpefPtNt2VdKsq5AnAK6apdtR6yCH8pr0CmSr710J0Mf+WdQy7K/OzMy7K2MgAfdQURDw==",
+ "dev": true,
+ "license": "MIT"
+ },
"node_modules/keyv": {
"version": "4.5.4",
"resolved": "https://registry.npmjs.org/keyv/-/keyv-4.5.4.tgz",
@@ -32160,6 +30059,7 @@
"resolved": "https://registry.npmjs.org/ky/-/ky-0.33.3.tgz",
"integrity": "sha512-CasD9OCEQSFIam2U8efFK81Yeg8vNMTBUqtMOHlrcWQHqUX3HeCl9Dr31u4toV7emlH8Mymk5+9p0lL6mKb/Xw==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=14.16"
},
@@ -32181,12 +30081,13 @@
}
},
"node_modules/launch-editor": {
- "version": "2.6.0",
- "resolved": "https://registry.npmjs.org/launch-editor/-/launch-editor-2.6.0.tgz",
- "integrity": "sha512-JpDCcQnyAAzZZaZ7vEiSqL690w7dAEyLao+KC96zBplnYbJS7TYNjvM3M7y3dGz+v7aIsJk3hllWuc0kWAjyRQ==",
+ "version": "2.9.1",
+ "resolved": "https://registry.npmjs.org/launch-editor/-/launch-editor-2.9.1.tgz",
+ "integrity": "sha512-Gcnl4Bd+hRO9P9icCP/RVVT2o8SFlPXofuCxvA2SaZuH45whSvf5p8x5oih5ftLiVhEI4sp5xDY+R+b3zJBh5w==",
+ "license": "MIT",
"dependencies": {
"picocolors": "^1.0.0",
- "shell-quote": "^1.7.3"
+ "shell-quote": "^1.8.1"
}
},
"node_modules/lazy-cache": {
@@ -32197,25 +30098,12 @@
"node": ">=0.10.0"
}
},
- "node_modules/lazy-universal-dotenv": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/lazy-universal-dotenv/-/lazy-universal-dotenv-4.0.0.tgz",
- "integrity": "sha512-aXpZJRnTkpK6gQ/z4nk+ZBLd/Qdp118cvPruLSIQzQNRhKwEcdXCOzXuF55VDqIiuAaY3UGZ10DJtvZzDcvsxg==",
- "dev": true,
- "dependencies": {
- "app-root-dir": "^1.0.2",
- "dotenv": "^16.0.0",
- "dotenv-expand": "^10.0.0"
- },
- "engines": {
- "node": ">=14.0.0"
- }
- },
"node_modules/lazystream": {
"version": "1.0.1",
"resolved": "https://registry.npmjs.org/lazystream/-/lazystream-1.0.1.tgz",
"integrity": "sha512-b94GiNHQNy6JNTrt5w6zNyffMrNkXZb3KTkCZJb2V1xaEGCk093vkZ2jk3tpaeP33/OiXC+WvK9AxUebnf5nbw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"readable-stream": "^2.0.5"
},
@@ -32224,92 +30112,99 @@
}
},
"node_modules/lerna": {
- "version": "7.1.4",
- "resolved": "https://registry.npmjs.org/lerna/-/lerna-7.1.4.tgz",
- "integrity": "sha512-/cabvmTTkmayyALIZx7OpHRex72i8xSOkiJchEkrKxAZHoLNaGSwqwKkj+x6WtmchhWl/gLlqwQXGRuxrJKiBw==",
+ "version": "8.1.9",
+ "resolved": "https://registry.npmjs.org/lerna/-/lerna-8.1.9.tgz",
+ "integrity": "sha512-ZRFlRUBB2obm+GkbTR7EbgTMuAdni6iwtTQTMy7LIrQ4UInG44LyfRepljtgUxh4HA0ltzsvWfPkd5J1DKGCeQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "@lerna/child-process": "7.1.4",
- "@lerna/create": "7.1.4",
- "@npmcli/run-script": "6.0.2",
- "@nx/devkit": ">=16.5.1 < 17",
+ "@lerna/create": "8.1.9",
+ "@npmcli/arborist": "7.5.4",
+ "@npmcli/package-json": "5.2.0",
+ "@npmcli/run-script": "8.1.0",
+ "@nx/devkit": ">=17.1.2 < 21",
"@octokit/plugin-enterprise-rest": "6.0.1",
"@octokit/rest": "19.0.11",
+ "aproba": "2.0.0",
"byte-size": "8.1.1",
"chalk": "4.1.0",
"clone-deep": "4.0.1",
- "cmd-shim": "6.0.1",
+ "cmd-shim": "6.0.3",
+ "color-support": "1.1.3",
"columnify": "1.6.0",
- "conventional-changelog-angular": "6.0.0",
+ "console-control-strings": "^1.1.0",
+ "conventional-changelog-angular": "7.0.0",
"conventional-changelog-core": "5.0.1",
"conventional-recommended-bump": "7.0.1",
- "cosmiconfig": "^8.2.0",
- "dedent": "0.7.0",
- "envinfo": "7.8.1",
+ "cosmiconfig": "9.0.0",
+ "dedent": "1.5.3",
+ "envinfo": "7.13.0",
"execa": "5.0.0",
- "fs-extra": "^11.1.1",
+ "fs-extra": "^11.2.0",
"get-port": "5.1.1",
"get-stream": "6.0.0",
- "git-url-parse": "13.1.0",
- "glob-parent": "5.1.2",
+ "git-url-parse": "14.0.0",
+ "glob-parent": "6.0.2",
"globby": "11.1.0",
"graceful-fs": "4.2.11",
"has-unicode": "2.0.1",
"import-local": "3.1.0",
"ini": "^1.3.8",
- "init-package-json": "5.0.0",
+ "init-package-json": "6.0.3",
"inquirer": "^8.2.4",
"is-ci": "3.0.1",
"is-stream": "2.0.0",
"jest-diff": ">=29.4.3 < 30",
"js-yaml": "4.1.0",
- "libnpmaccess": "7.0.2",
- "libnpmpublish": "7.3.0",
+ "libnpmaccess": "8.0.6",
+ "libnpmpublish": "9.0.9",
"load-json-file": "6.2.0",
"lodash": "^4.17.21",
- "make-dir": "3.1.0",
+ "make-dir": "4.0.0",
"minimatch": "3.0.5",
"multimatch": "5.0.0",
"node-fetch": "2.6.7",
- "npm-package-arg": "8.1.1",
- "npm-packlist": "5.1.1",
- "npm-registry-fetch": "^14.0.5",
- "npmlog": "^6.0.2",
- "nx": ">=16.5.1 < 17",
+ "npm-package-arg": "11.0.2",
+ "npm-packlist": "8.0.2",
+ "npm-registry-fetch": "^17.1.0",
+ "nx": ">=17.1.2 < 21",
"p-map": "4.0.0",
"p-map-series": "2.1.0",
"p-pipe": "3.1.0",
"p-queue": "6.6.2",
"p-reduce": "2.1.0",
"p-waterfall": "2.1.1",
- "pacote": "^15.2.0",
+ "pacote": "^18.0.6",
"pify": "5.0.0",
"read-cmd-shim": "4.0.0",
- "read-package-json": "6.0.4",
"resolve-from": "5.0.0",
"rimraf": "^4.4.1",
"semver": "^7.3.8",
+ "set-blocking": "^2.0.0",
"signal-exit": "3.0.7",
"slash": "3.0.0",
- "ssri": "^9.0.1",
+ "ssri": "^10.0.6",
+ "string-width": "^4.2.3",
+ "strip-ansi": "^6.0.1",
"strong-log-transformer": "2.1.0",
- "tar": "6.1.11",
+ "tar": "6.2.1",
"temp-dir": "1.0.0",
"typescript": ">=3 < 6",
"upath": "2.0.1",
- "uuid": "^9.0.0",
+ "uuid": "^10.0.0",
"validate-npm-package-license": "3.0.4",
- "validate-npm-package-name": "5.0.0",
+ "validate-npm-package-name": "5.0.1",
+ "wide-align": "1.1.5",
"write-file-atomic": "5.0.1",
"write-pkg": "4.0.0",
- "yargs": "16.2.0",
- "yargs-parser": "20.2.4"
+ "yargs": "17.7.2",
+ "yargs-parser": "21.1.1"
},
"bin": {
"lerna": "dist/cli.js"
},
"engines": {
- "node": "^14.17.0 || >=16.0.0"
+ "node": ">=18.0.0"
}
},
"node_modules/lerna/node_modules/@octokit/auth-token": {
@@ -32468,11 +30363,19 @@
"@octokit/openapi-types": "^18.0.0"
}
},
+ "node_modules/lerna/node_modules/aproba": {
+ "version": "2.0.0",
+ "resolved": "https://registry.npmjs.org/aproba/-/aproba-2.0.0.tgz",
+ "integrity": "sha512-lYe4Gx7QT+MKGbDsA+Z+he/Wtef0BiwDOlK/XkBrdfsh9J/jPPXbX0tE9x9cl27Tmu5gg3QUbUrQYa/y+KOHPQ==",
+ "dev": true,
+ "license": "ISC"
+ },
"node_modules/lerna/node_modules/argparse": {
"version": "2.0.1",
"resolved": "https://registry.npmjs.org/argparse/-/argparse-2.0.1.tgz",
"integrity": "sha512-8+9WqebbFzpX9OR+Wa6O29asIogeRMzcGtAINdpMHHyAg10f05aSFVBbcEqGf/PXw1EjAZ+q2/bEBg3DvurK3Q==",
- "dev": true
+ "dev": true,
+ "license": "Python-2.0"
},
"node_modules/lerna/node_modules/before-after-hook": {
"version": "2.2.3",
@@ -32518,15 +30421,16 @@
}
},
"node_modules/lerna/node_modules/cosmiconfig": {
- "version": "8.3.6",
- "resolved": "https://registry.npmjs.org/cosmiconfig/-/cosmiconfig-8.3.6.tgz",
- "integrity": "sha512-kcZ6+W5QzcJ3P1Mt+83OUv/oHFqZHIx8DuxG6eZ5RGMERoLqp4BuGjhHLYGK+Kf5XVkQvqBSmAy/nGWN3qDgEA==",
+ "version": "9.0.0",
+ "resolved": "https://registry.npmjs.org/cosmiconfig/-/cosmiconfig-9.0.0.tgz",
+ "integrity": "sha512-itvL5h8RETACmOTFc4UfIyB2RfEHi71Ax6E/PivVxq9NseKbOWpeyHEOIbmAw1rs8Ak0VursQNww7lf7YtUwzg==",
"dev": true,
+ "license": "MIT",
"dependencies": {
+ "env-paths": "^2.2.1",
"import-fresh": "^3.3.0",
"js-yaml": "^4.1.0",
- "parse-json": "^5.2.0",
- "path-type": "^4.0.0"
+ "parse-json": "^5.2.0"
},
"engines": {
"node": ">=14"
@@ -32543,18 +30447,19 @@
}
}
},
- "node_modules/lerna/node_modules/cross-spawn": {
- "version": "7.0.3",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-7.0.3.tgz",
- "integrity": "sha512-iRDPJKUPVEND7dHPO8rkbOnPpyDygcDFtWjpeWNCgy8WP2rXcxXL8TskReQl6OrB2G7+UJrags1q15Fudc7G6w==",
+ "node_modules/lerna/node_modules/dedent": {
+ "version": "1.5.3",
+ "resolved": "https://registry.npmjs.org/dedent/-/dedent-1.5.3.tgz",
+ "integrity": "sha512-NHQtfOOW68WD8lgypbLA5oT+Bt0xXJhiYvoR6SmmNXZfpzOGXwdKWmcwG8N7PwVVWV3eF/68nmD9BaJSsTBhyQ==",
"dev": true,
- "dependencies": {
- "path-key": "^3.1.0",
- "shebang-command": "^2.0.0",
- "which": "^2.0.1"
+ "license": "MIT",
+ "peerDependencies": {
+ "babel-plugin-macros": "^3.1.0"
},
- "engines": {
- "node": ">= 8"
+ "peerDependenciesMeta": {
+ "babel-plugin-macros": {
+ "optional": true
+ }
}
},
"node_modules/lerna/node_modules/emoji-regex": {
@@ -32563,18 +30468,6 @@
"integrity": "sha512-MSjYzcWNOA0ewAHpz0MxpYFvwg6yjy1NG3xteoqz644VCo/RPgnr1/GGt+ic3iJTzQ8Eu3TdM14SawnVUmGE6A==",
"dev": true
},
- "node_modules/lerna/node_modules/envinfo": {
- "version": "7.8.1",
- "resolved": "https://registry.npmjs.org/envinfo/-/envinfo-7.8.1.tgz",
- "integrity": "sha512-/o+BXHmB7ocbHEAs6F2EnG0ogybVVUdkRunTT2glZU9XAaGmhqskrvKwqXuDfNjEO0LZKWdejEEpnq8aM0tOaw==",
- "dev": true,
- "bin": {
- "envinfo": "dist/cli.js"
- },
- "engines": {
- "node": ">=4"
- }
- },
"node_modules/lerna/node_modules/execa": {
"version": "5.0.0",
"resolved": "https://registry.npmjs.org/execa/-/execa-5.0.0.tgz",
@@ -32640,34 +30533,35 @@
}
},
"node_modules/lerna/node_modules/glob": {
- "version": "8.1.0",
- "resolved": "https://registry.npmjs.org/glob/-/glob-8.1.0.tgz",
- "integrity": "sha512-r8hpEjiQEYlF2QU0df3dS+nxxSIreXQS1qRhMJM0Q5NDdR386C7jb7Hwwod8Fgiuex+k0GFjgft18yvxm5XoCQ==",
+ "version": "9.3.5",
+ "resolved": "https://registry.npmjs.org/glob/-/glob-9.3.5.tgz",
+ "integrity": "sha512-e1LleDykUz2Iu+MTYdkSsuWX8lvAjAcs0Xef0lNIu0S2wOAzuTxCJtcd9S3cijlwYF18EsU3rzb8jPVobxDh9Q==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"fs.realpath": "^1.0.0",
- "inflight": "^1.0.4",
- "inherits": "2",
- "minimatch": "^5.0.1",
- "once": "^1.3.0"
+ "minimatch": "^8.0.2",
+ "minipass": "^4.2.4",
+ "path-scurry": "^1.6.1"
},
"engines": {
- "node": ">=12"
+ "node": ">=16 || 14 >=14.17"
},
"funding": {
"url": "https://github.com/sponsors/isaacs"
}
},
"node_modules/lerna/node_modules/glob-parent": {
- "version": "5.1.2",
- "resolved": "https://registry.npmjs.org/glob-parent/-/glob-parent-5.1.2.tgz",
- "integrity": "sha512-AOIgSQCepiJYwP3ARnGx+5VnTu2HBYdzbGP45eLw1vr3zB3vZLeyed1sC9hnbcOc9/SrMyM5RPQrkGz4aS9Zow==",
+ "version": "6.0.2",
+ "resolved": "https://registry.npmjs.org/glob-parent/-/glob-parent-6.0.2.tgz",
+ "integrity": "sha512-XxwI8EOhVQgWp6iDL+3b0r86f4d6AX6zSU55HfB4ydCEuXLXc5FcYeOu+nnGftS4TEju/11rt4KJPTMgbfmv4A==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "is-glob": "^4.0.1"
+ "is-glob": "^4.0.3"
},
"engines": {
- "node": ">= 6"
+ "node": ">=10.13.0"
}
},
"node_modules/lerna/node_modules/glob/node_modules/brace-expansion": {
@@ -32675,20 +30569,35 @@
"resolved": "https://registry.npmjs.org/brace-expansion/-/brace-expansion-2.0.1.tgz",
"integrity": "sha512-XnAIvQ8eM+kC6aULx6wuQiwVsnzsi9d3WxzV3FpWTGA19F621kwdbsAcFKXgKUHZWsy+mY6iL1sHTxWEFCytDA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"balanced-match": "^1.0.0"
}
},
"node_modules/lerna/node_modules/glob/node_modules/minimatch": {
- "version": "5.1.6",
- "resolved": "https://registry.npmjs.org/minimatch/-/minimatch-5.1.6.tgz",
- "integrity": "sha512-lKwV/1brpG6mBUFHtb7NUmtABCb2WZZmm2wNiOA5hAb8VdCS4B3dtMWyvcoViccwAW/COERjXLt0zP1zXUN26g==",
+ "version": "8.0.4",
+ "resolved": "https://registry.npmjs.org/minimatch/-/minimatch-8.0.4.tgz",
+ "integrity": "sha512-W0Wvr9HyFXZRGIDgCicunpQ299OKXs9RgZfaukz4qAW/pJhcpUfupc9c+OObPOFueNy8VSrZgEmDtk6Kh4WzDA==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"brace-expansion": "^2.0.1"
},
"engines": {
- "node": ">=10"
+ "node": ">=16 || 14 >=14.17"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
+ }
+ },
+ "node_modules/lerna/node_modules/glob/node_modules/minipass": {
+ "version": "4.2.8",
+ "resolved": "https://registry.npmjs.org/minipass/-/minipass-4.2.8.tgz",
+ "integrity": "sha512-fNzuVyifolSLFL4NzpF+wEF4qrgqaaKX0haXPQEdQ7NKAN+WecoKMHV09YcuL/DHxrUsYQOK3MiuDf7Ip2OXfQ==",
+ "dev": true,
+ "license": "ISC",
+ "engines": {
+ "node": ">=8"
}
},
"node_modules/lerna/node_modules/has-flag": {
@@ -32701,15 +30610,16 @@
}
},
"node_modules/lerna/node_modules/hosted-git-info": {
- "version": "3.0.8",
- "resolved": "https://registry.npmjs.org/hosted-git-info/-/hosted-git-info-3.0.8.tgz",
- "integrity": "sha512-aXpmwoOhRBrw6X3j0h5RloK4x1OzsxMPyxqIHyNfSe2pypkVTZFpEiRoSipPEPlMrh0HW/XsjkJ5WgnCirpNUw==",
+ "version": "7.0.2",
+ "resolved": "https://registry.npmjs.org/hosted-git-info/-/hosted-git-info-7.0.2.tgz",
+ "integrity": "sha512-puUZAUKT5m8Zzvs72XWy3HtvVbTWljRE66cP60bxJzAqf2DgICo7lYTY2IHUmLnNpjYvw5bvmoHvPc0QO2a62w==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "lru-cache": "^6.0.0"
+ "lru-cache": "^10.0.1"
},
"engines": {
- "node": ">=10"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/lerna/node_modules/human-signals": {
@@ -32722,15 +30632,16 @@
}
},
"node_modules/lerna/node_modules/ignore-walk": {
- "version": "5.0.1",
- "resolved": "https://registry.npmjs.org/ignore-walk/-/ignore-walk-5.0.1.tgz",
- "integrity": "sha512-yemi4pMf51WKT7khInJqAvsIGzoqYXblnsz0ql8tM+yi1EKYTY1evX4NAbJrLL/Aanr2HyZeluqU+Oi7MGHokw==",
+ "version": "6.0.5",
+ "resolved": "https://registry.npmjs.org/ignore-walk/-/ignore-walk-6.0.5.tgz",
+ "integrity": "sha512-VuuG0wCnjhnylG1ABXT3dAuIpTNDs/G8jlpmwXY03fXoXy/8ZK8/T+hMzt8L4WnrLCJgdybqgPagnF/f97cg3A==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "minimatch": "^5.0.1"
+ "minimatch": "^9.0.0"
},
"engines": {
- "node": "^12.13.0 || ^14.15.0 || >=16.0.0"
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
"node_modules/lerna/node_modules/ignore-walk/node_modules/brace-expansion": {
@@ -32738,20 +30649,25 @@
"resolved": "https://registry.npmjs.org/brace-expansion/-/brace-expansion-2.0.1.tgz",
"integrity": "sha512-XnAIvQ8eM+kC6aULx6wuQiwVsnzsi9d3WxzV3FpWTGA19F621kwdbsAcFKXgKUHZWsy+mY6iL1sHTxWEFCytDA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"balanced-match": "^1.0.0"
}
},
"node_modules/lerna/node_modules/ignore-walk/node_modules/minimatch": {
- "version": "5.1.6",
- "resolved": "https://registry.npmjs.org/minimatch/-/minimatch-5.1.6.tgz",
- "integrity": "sha512-lKwV/1brpG6mBUFHtb7NUmtABCb2WZZmm2wNiOA5hAb8VdCS4B3dtMWyvcoViccwAW/COERjXLt0zP1zXUN26g==",
+ "version": "9.0.5",
+ "resolved": "https://registry.npmjs.org/minimatch/-/minimatch-9.0.5.tgz",
+ "integrity": "sha512-G6T0ZX48xgozx7587koeX9Ys2NYy6Gmv//P89sEte9V9whIapMNF4idKxnW2QtCcLiTWlb/wfCabAtAFWhhBow==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"brace-expansion": "^2.0.1"
},
"engines": {
- "node": ">=10"
+ "node": ">=16 || 14 >=14.17"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
}
},
"node_modules/lerna/node_modules/inquirer": {
@@ -32831,6 +30747,7 @@
"resolved": "https://registry.npmjs.org/js-yaml/-/js-yaml-4.1.0.tgz",
"integrity": "sha512-wpxZs9NoxZaJESJGIZTyDEaYpl0FKSA+FB9aJiyemKhMwkxQg63h4T1KJgUGHpTqPDNRcmmYLugrRjJlBtWvRA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"argparse": "^2.0.1"
},
@@ -32850,42 +30767,22 @@
"graceful-fs": "^4.1.6"
}
},
- "node_modules/lerna/node_modules/lru-cache": {
- "version": "6.0.0",
- "resolved": "https://registry.npmjs.org/lru-cache/-/lru-cache-6.0.0.tgz",
- "integrity": "sha512-Jo6dJ04CmSjuznwJSS3pUeWmd/H0ffTlkXXgwZi+eq1UCmqQwCh+eLsYOYCwY991i2Fah4h1BEMCx4qThGbsiA==",
- "dev": true,
- "dependencies": {
- "yallist": "^4.0.0"
- },
- "engines": {
- "node": ">=10"
- }
- },
"node_modules/lerna/node_modules/make-dir": {
- "version": "3.1.0",
- "resolved": "https://registry.npmjs.org/make-dir/-/make-dir-3.1.0.tgz",
- "integrity": "sha512-g3FeP20LNwhALb/6Cz6Dd4F2ngze0jz7tbzrD2wAV+o9FeNHe4rL+yK2md0J/fiSf1sa1ADhXqi5+oVwOM/eGw==",
+ "version": "4.0.0",
+ "resolved": "https://registry.npmjs.org/make-dir/-/make-dir-4.0.0.tgz",
+ "integrity": "sha512-hXdUTZYIVOt1Ex//jAQi+wTZZpUpwBj/0QsOzqegb3rGMMeJiSEu5xLHnYfBrRV4RH2+OCSOO95Is/7x1WJ4bw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "semver": "^6.0.0"
+ "semver": "^7.5.3"
},
"engines": {
- "node": ">=8"
+ "node": ">=10"
},
"funding": {
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/lerna/node_modules/make-dir/node_modules/semver": {
- "version": "6.3.1",
- "resolved": "https://registry.npmjs.org/semver/-/semver-6.3.1.tgz",
- "integrity": "sha512-BR7VvDCVHO+q2xBEWskxS6DJE1qRnb7DxzUrogb71CWoSficBxYsiAGd+Kl0mmq/MprG9yArRkyrQxTO6XjMzA==",
- "dev": true,
- "bin": {
- "semver": "bin/semver.js"
- }
- },
"node_modules/lerna/node_modules/mimic-fn": {
"version": "2.1.0",
"resolved": "https://registry.npmjs.org/mimic-fn/-/mimic-fn-2.1.0.tgz",
@@ -32907,6 +30804,16 @@
"node": "*"
}
},
+ "node_modules/lerna/node_modules/minipass": {
+ "version": "7.1.2",
+ "resolved": "https://registry.npmjs.org/minipass/-/minipass-7.1.2.tgz",
+ "integrity": "sha512-qOOzS1cBTWYF4BH8fVePDBOO9iptMnGUEZwNc/cMWnTV2nVLZ7VoNWEPHkYczZA0pdoA7dl6e7FL659nX9S2aw==",
+ "dev": true,
+ "license": "ISC",
+ "engines": {
+ "node": ">=16 || 14 >=14.17"
+ }
+ },
"node_modules/lerna/node_modules/node-fetch": {
"version": "2.6.7",
"resolved": "https://registry.npmjs.org/node-fetch/-/node-fetch-2.6.7.tgz",
@@ -32929,44 +30836,32 @@
}
},
"node_modules/lerna/node_modules/npm-package-arg": {
- "version": "8.1.1",
- "resolved": "https://registry.npmjs.org/npm-package-arg/-/npm-package-arg-8.1.1.tgz",
- "integrity": "sha512-CsP95FhWQDwNqiYS+Q0mZ7FAEDytDZAkNxQqea6IaAFJTAY9Lhhqyl0irU/6PMc7BGfUmnsbHcqxJD7XuVM/rg==",
+ "version": "11.0.2",
+ "resolved": "https://registry.npmjs.org/npm-package-arg/-/npm-package-arg-11.0.2.tgz",
+ "integrity": "sha512-IGN0IAwmhDJwy13Wc8k+4PEbTPhpJnMtfR53ZbOyjkvmEcLS4nCwp6mvMWjS5sUjeiW3mpx6cHmuhKEu9XmcQw==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "hosted-git-info": "^3.0.6",
- "semver": "^7.0.0",
- "validate-npm-package-name": "^3.0.0"
+ "hosted-git-info": "^7.0.0",
+ "proc-log": "^4.0.0",
+ "semver": "^7.3.5",
+ "validate-npm-package-name": "^5.0.0"
},
"engines": {
- "node": ">=10"
- }
- },
- "node_modules/lerna/node_modules/npm-package-arg/node_modules/validate-npm-package-name": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/validate-npm-package-name/-/validate-npm-package-name-3.0.0.tgz",
- "integrity": "sha512-M6w37eVCMMouJ9V/sdPGnC5H4uDr73/+xdq0FBLO3TFFX1+7wiUY6Es328NN+y43tmY+doUdN9g9J21vqB7iLw==",
- "dev": true,
- "dependencies": {
- "builtins": "^1.0.3"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/lerna/node_modules/npm-packlist": {
- "version": "5.1.1",
- "resolved": "https://registry.npmjs.org/npm-packlist/-/npm-packlist-5.1.1.tgz",
- "integrity": "sha512-UfpSvQ5YKwctmodvPPkK6Fwk603aoVsf8AEbmVKAEECrfvL8SSe1A2YIwrJ6xmTHAITKPwwZsWo7WwEbNk0kxw==",
+ "version": "8.0.2",
+ "resolved": "https://registry.npmjs.org/npm-packlist/-/npm-packlist-8.0.2.tgz",
+ "integrity": "sha512-shYrPFIS/JLP4oQmAwDyk5HcyysKW8/JLTEA32S0Z5TzvpaeeX2yMFfoK1fjEBnCBvVyIB/Jj/GBFdm0wsgzbA==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "glob": "^8.0.1",
- "ignore-walk": "^5.0.1",
- "npm-bundled": "^1.1.2",
- "npm-normalize-package-bin": "^1.0.1"
- },
- "bin": {
- "npm-packlist": "bin/index.js"
+ "ignore-walk": "^6.0.4"
},
"engines": {
- "node": "^12.13.0 || ^14.15.0 || >=16.0.0"
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
"node_modules/lerna/node_modules/npm-run-path": {
@@ -33028,27 +30923,6 @@
"node": ">=8"
}
},
- "node_modules/lerna/node_modules/path-type": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/path-type/-/path-type-4.0.0.tgz",
- "integrity": "sha512-gDKb8aZMDeD/tZWs9P6+q0J9Mwkdl6xMV8TjnGP3qJVJ06bdMgkbBlLU8IdfOsIsFz2BW1rNVT3XuNEl8zPAvw==",
- "dev": true,
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/lerna/node_modules/pify": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/pify/-/pify-5.0.0.tgz",
- "integrity": "sha512-eW/gHNMlxdSP6dmG6uJip6FXN0EQBwm2clYYd8Wul42Cwu/DK8HEftzsapcNdYe2MfLiIwZqsDk2RDEsTE79hA==",
- "dev": true,
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
"node_modules/lerna/node_modules/resolve-from": {
"version": "5.0.0",
"resolved": "https://registry.npmjs.org/resolve-from/-/resolve-from-5.0.0.tgz",
@@ -33076,6 +30950,7 @@
"resolved": "https://registry.npmjs.org/rimraf/-/rimraf-4.4.1.tgz",
"integrity": "sha512-Gk8NlF062+T9CqNGn6h4tls3k6T1+/nXdOcSZVikNVtlRdYpA7wRJJMoXmuvOnLW844rPjdQ7JgXCYM6PPC/og==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"glob": "^9.2.0"
},
@@ -33089,57 +30964,6 @@
"url": "https://github.com/sponsors/isaacs"
}
},
- "node_modules/lerna/node_modules/rimraf/node_modules/brace-expansion": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/brace-expansion/-/brace-expansion-2.0.1.tgz",
- "integrity": "sha512-XnAIvQ8eM+kC6aULx6wuQiwVsnzsi9d3WxzV3FpWTGA19F621kwdbsAcFKXgKUHZWsy+mY6iL1sHTxWEFCytDA==",
- "dev": true,
- "dependencies": {
- "balanced-match": "^1.0.0"
- }
- },
- "node_modules/lerna/node_modules/rimraf/node_modules/glob": {
- "version": "9.3.5",
- "resolved": "https://registry.npmjs.org/glob/-/glob-9.3.5.tgz",
- "integrity": "sha512-e1LleDykUz2Iu+MTYdkSsuWX8lvAjAcs0Xef0lNIu0S2wOAzuTxCJtcd9S3cijlwYF18EsU3rzb8jPVobxDh9Q==",
- "dev": true,
- "dependencies": {
- "fs.realpath": "^1.0.0",
- "minimatch": "^8.0.2",
- "minipass": "^4.2.4",
- "path-scurry": "^1.6.1"
- },
- "engines": {
- "node": ">=16 || 14 >=14.17"
- },
- "funding": {
- "url": "https://github.com/sponsors/isaacs"
- }
- },
- "node_modules/lerna/node_modules/rimraf/node_modules/minimatch": {
- "version": "8.0.4",
- "resolved": "https://registry.npmjs.org/minimatch/-/minimatch-8.0.4.tgz",
- "integrity": "sha512-W0Wvr9HyFXZRGIDgCicunpQ299OKXs9RgZfaukz4qAW/pJhcpUfupc9c+OObPOFueNy8VSrZgEmDtk6Kh4WzDA==",
- "dev": true,
- "dependencies": {
- "brace-expansion": "^2.0.1"
- },
- "engines": {
- "node": ">=16 || 14 >=14.17"
- },
- "funding": {
- "url": "https://github.com/sponsors/isaacs"
- }
- },
- "node_modules/lerna/node_modules/rimraf/node_modules/minipass": {
- "version": "4.2.8",
- "resolved": "https://registry.npmjs.org/minipass/-/minipass-4.2.8.tgz",
- "integrity": "sha512-fNzuVyifolSLFL4NzpF+wEF4qrgqaaKX0haXPQEdQ7NKAN+WecoKMHV09YcuL/DHxrUsYQOK3MiuDf7Ip2OXfQ==",
- "dev": true,
- "engines": {
- "node": ">=8"
- }
- },
"node_modules/lerna/node_modules/rxjs": {
"version": "7.8.1",
"resolved": "https://registry.npmjs.org/rxjs/-/rxjs-7.8.1.tgz",
@@ -33149,37 +30973,17 @@
"tslib": "^2.1.0"
}
},
- "node_modules/lerna/node_modules/shebang-command": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/shebang-command/-/shebang-command-2.0.0.tgz",
- "integrity": "sha512-kHxr2zZpYtdmrN1qDjrrX/Z1rR1kG8Dx+gkpK1G4eXmvXswmcE1hTWBWYUzlraYw1/yZp6YuDY77YtvbN0dmDA==",
- "dev": true,
- "dependencies": {
- "shebang-regex": "^3.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/lerna/node_modules/shebang-regex": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/shebang-regex/-/shebang-regex-3.0.0.tgz",
- "integrity": "sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==",
- "dev": true,
- "engines": {
- "node": ">=8"
- }
- },
"node_modules/lerna/node_modules/ssri": {
- "version": "9.0.1",
- "resolved": "https://registry.npmjs.org/ssri/-/ssri-9.0.1.tgz",
- "integrity": "sha512-o57Wcn66jMQvfHG1FlYbWeZWW/dHZhJXjpIcTfXldXEk5nz5lStPo3mK0OJQfGR3RbZUlbISexbljkJzuEj/8Q==",
+ "version": "10.0.6",
+ "resolved": "https://registry.npmjs.org/ssri/-/ssri-10.0.6.tgz",
+ "integrity": "sha512-MGrFH9Z4NP9Iyhqn16sDtBpRRNJ0Y2hNa6D65h736fVSaPCHr4DM4sWUNvVaSuC+0OBGhwsrydQwmgfg5LncqQ==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "minipass": "^3.1.1"
+ "minipass": "^7.0.3"
},
"engines": {
- "node": "^12.13.0 || ^14.15.0 || >=16.0.0"
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
"node_modules/lerna/node_modules/string-width": {
@@ -33233,40 +31037,28 @@
"yarn": "*"
}
},
- "node_modules/lerna/node_modules/validate-npm-package-name": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/validate-npm-package-name/-/validate-npm-package-name-5.0.0.tgz",
- "integrity": "sha512-YuKoXDAhBYxY7SfOKxHBDoSyENFeW5VvIIQp2TGQuit8gpK6MnWaQelBKxso72DoxTZfZdcP3W90LqpSkgPzLQ==",
- "dev": true,
- "dependencies": {
- "builtins": "^5.0.0"
- },
- "engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
- }
- },
- "node_modules/lerna/node_modules/validate-npm-package-name/node_modules/builtins": {
- "version": "5.1.0",
- "resolved": "https://registry.npmjs.org/builtins/-/builtins-5.1.0.tgz",
- "integrity": "sha512-SW9lzGTLvWTP1AY8xeAMZimqDrIaSdLQUcVr9DMef51niJ022Ri87SwRRKYm4A6iHfkPaiVUu/Duw2Wc4J7kKg==",
+ "node_modules/lerna/node_modules/uuid": {
+ "version": "10.0.0",
+ "resolved": "https://registry.npmjs.org/uuid/-/uuid-10.0.0.tgz",
+ "integrity": "sha512-8XkAphELsDnEGrDxUOHB3RGvXz6TeuYSGEZBOjtTtPm2lwhGBjLgOzLHB63IUWfBpNucQjND6d3AOudO+H3RWQ==",
"dev": true,
- "dependencies": {
- "semver": "^7.0.0"
+ "funding": [
+ "https://github.com/sponsors/broofa",
+ "https://github.com/sponsors/ctavan"
+ ],
+ "license": "MIT",
+ "bin": {
+ "uuid": "dist/bin/uuid"
}
},
- "node_modules/lerna/node_modules/which": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
- "integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
+ "node_modules/lerna/node_modules/validate-npm-package-name": {
+ "version": "5.0.1",
+ "resolved": "https://registry.npmjs.org/validate-npm-package-name/-/validate-npm-package-name-5.0.1.tgz",
+ "integrity": "sha512-OljLrQ9SQdOUqTaQxqL5dEfZWrXExyyWsozYlAWFawPVNuD83igl7uJD2RTkNMbniIYgt8l81eCJGIdQF7avLQ==",
"dev": true,
- "dependencies": {
- "isexe": "^2.0.0"
- },
- "bin": {
- "node-which": "bin/node-which"
- },
+ "license": "ISC",
"engines": {
- "node": ">= 8"
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
"node_modules/lerna/node_modules/write-file-atomic": {
@@ -33294,11 +31086,15 @@
"url": "https://github.com/sponsors/isaacs"
}
},
- "node_modules/lerna/node_modules/yallist": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/yallist/-/yallist-4.0.0.tgz",
- "integrity": "sha512-3wdGidZyq5PB084XLES5TpOSRA3wjXAlIWMhum2kRcv/41Sn2emQ0dycQW4uZXLejwKvg6EsvbdlVL+FYEct7A==",
- "dev": true
+ "node_modules/lerna/node_modules/yargs-parser": {
+ "version": "21.1.1",
+ "resolved": "https://registry.npmjs.org/yargs-parser/-/yargs-parser-21.1.1.tgz",
+ "integrity": "sha512-tVpsJW7DdjecAiFpbIB1e3qxIQsE6NoPc5/eTdrbbIC4h0LVsWhnoa3g+m2HclBIujHzsxZ4VJVA+GUuc2/LBw==",
+ "dev": true,
+ "license": "ISC",
+ "engines": {
+ "node": ">=12"
+ }
},
"node_modules/leven": {
"version": "3.1.0",
@@ -33340,52 +31136,46 @@
}
},
"node_modules/libnpmaccess": {
- "version": "7.0.2",
- "resolved": "https://registry.npmjs.org/libnpmaccess/-/libnpmaccess-7.0.2.tgz",
- "integrity": "sha512-vHBVMw1JFMTgEk15zRsJuSAg7QtGGHpUSEfnbcRL1/gTBag9iEfJbyjpDmdJmwMhvpoLoNBtdAUCdGnaP32hhw==",
+ "version": "8.0.6",
+ "resolved": "https://registry.npmjs.org/libnpmaccess/-/libnpmaccess-8.0.6.tgz",
+ "integrity": "sha512-uM8DHDEfYG6G5gVivVl+yQd4pH3uRclHC59lzIbSvy7b5FEwR+mU49Zq1jEyRtRFv7+M99mUW9S0wL/4laT4lw==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "npm-package-arg": "^10.1.0",
- "npm-registry-fetch": "^14.0.3"
+ "npm-package-arg": "^11.0.2",
+ "npm-registry-fetch": "^17.0.1"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/libnpmaccess/node_modules/hosted-git-info": {
- "version": "6.1.1",
- "resolved": "https://registry.npmjs.org/hosted-git-info/-/hosted-git-info-6.1.1.tgz",
- "integrity": "sha512-r0EI+HBMcXadMrugk0GCQ+6BQV39PiWAZVfq7oIckeGiN7sjRGyQxPdft3nQekFTCQbYxLBH+/axZMeH8UX6+w==",
+ "version": "7.0.2",
+ "resolved": "https://registry.npmjs.org/hosted-git-info/-/hosted-git-info-7.0.2.tgz",
+ "integrity": "sha512-puUZAUKT5m8Zzvs72XWy3HtvVbTWljRE66cP60bxJzAqf2DgICo7lYTY2IHUmLnNpjYvw5bvmoHvPc0QO2a62w==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "lru-cache": "^7.5.1"
+ "lru-cache": "^10.0.1"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
- }
- },
- "node_modules/libnpmaccess/node_modules/lru-cache": {
- "version": "7.18.3",
- "resolved": "https://registry.npmjs.org/lru-cache/-/lru-cache-7.18.3.tgz",
- "integrity": "sha512-jumlc0BIUrS3qJGgIkWZsyfAM7NCWiBcCDhnd+3NNM5KbBmLTgHVfWBcg6W+rLUsIpzpERPsvwUP7CckAQSOoA==",
- "dev": true,
- "engines": {
- "node": ">=12"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/libnpmaccess/node_modules/npm-package-arg": {
- "version": "10.1.0",
- "resolved": "https://registry.npmjs.org/npm-package-arg/-/npm-package-arg-10.1.0.tgz",
- "integrity": "sha512-uFyyCEmgBfZTtrKk/5xDfHp6+MdrqGotX/VoOyEEl3mBwiEE5FlBaePanazJSVMPT7vKepcjYBY2ztg9A3yPIA==",
+ "version": "11.0.3",
+ "resolved": "https://registry.npmjs.org/npm-package-arg/-/npm-package-arg-11.0.3.tgz",
+ "integrity": "sha512-sHGJy8sOC1YraBywpzQlIKBE4pBbGbiF95U6Auspzyem956E0+FtDtsx1ZxlOJkQCZ1AFXAY/yuvtFYrOxF+Bw==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "hosted-git-info": "^6.0.0",
- "proc-log": "^3.0.0",
+ "hosted-git-info": "^7.0.0",
+ "proc-log": "^4.0.0",
"semver": "^7.3.5",
"validate-npm-package-name": "^5.0.0"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/libnpmaccess/node_modules/validate-npm-package-name": {
@@ -33393,48 +31183,58 @@
"resolved": "https://registry.npmjs.org/validate-npm-package-name/-/validate-npm-package-name-5.0.1.tgz",
"integrity": "sha512-OljLrQ9SQdOUqTaQxqL5dEfZWrXExyyWsozYlAWFawPVNuD83igl7uJD2RTkNMbniIYgt8l81eCJGIdQF7avLQ==",
"dev": true,
+ "license": "ISC",
"engines": {
"node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
"node_modules/libnpmpublish": {
- "version": "7.3.0",
- "resolved": "https://registry.npmjs.org/libnpmpublish/-/libnpmpublish-7.3.0.tgz",
- "integrity": "sha512-fHUxw5VJhZCNSls0KLNEG0mCD2PN1i14gH5elGOgiVnU3VgTcRahagYP2LKI1m0tFCJ+XrAm0zVYyF5RCbXzcg==",
+ "version": "9.0.9",
+ "resolved": "https://registry.npmjs.org/libnpmpublish/-/libnpmpublish-9.0.9.tgz",
+ "integrity": "sha512-26zzwoBNAvX9AWOPiqqF6FG4HrSCPsHFkQm7nT+xU1ggAujL/eae81RnCv4CJ2In9q9fh10B88sYSzKCUh/Ghg==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "ci-info": "^3.6.1",
- "normalize-package-data": "^5.0.0",
- "npm-package-arg": "^10.1.0",
- "npm-registry-fetch": "^14.0.3",
- "proc-log": "^3.0.0",
+ "ci-info": "^4.0.0",
+ "normalize-package-data": "^6.0.1",
+ "npm-package-arg": "^11.0.2",
+ "npm-registry-fetch": "^17.0.1",
+ "proc-log": "^4.2.0",
"semver": "^7.3.7",
- "sigstore": "^1.4.0",
- "ssri": "^10.0.1"
+ "sigstore": "^2.2.0",
+ "ssri": "^10.0.6"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": "^16.14.0 || >=18.0.0"
}
},
- "node_modules/libnpmpublish/node_modules/hosted-git-info": {
- "version": "6.1.1",
- "resolved": "https://registry.npmjs.org/hosted-git-info/-/hosted-git-info-6.1.1.tgz",
- "integrity": "sha512-r0EI+HBMcXadMrugk0GCQ+6BQV39PiWAZVfq7oIckeGiN7sjRGyQxPdft3nQekFTCQbYxLBH+/axZMeH8UX6+w==",
+ "node_modules/libnpmpublish/node_modules/ci-info": {
+ "version": "4.1.0",
+ "resolved": "https://registry.npmjs.org/ci-info/-/ci-info-4.1.0.tgz",
+ "integrity": "sha512-HutrvTNsF48wnxkzERIXOe5/mlcfFcbfCmwcg6CJnizbSue78AbDt+1cgl26zwn61WFxhcPykPfZrbqjGmBb4A==",
"dev": true,
- "dependencies": {
- "lru-cache": "^7.5.1"
- },
+ "funding": [
+ {
+ "type": "github",
+ "url": "https://github.com/sponsors/sibiraj-s"
+ }
+ ],
+ "license": "MIT",
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": ">=8"
}
},
- "node_modules/libnpmpublish/node_modules/lru-cache": {
- "version": "7.18.3",
- "resolved": "https://registry.npmjs.org/lru-cache/-/lru-cache-7.18.3.tgz",
- "integrity": "sha512-jumlc0BIUrS3qJGgIkWZsyfAM7NCWiBcCDhnd+3NNM5KbBmLTgHVfWBcg6W+rLUsIpzpERPsvwUP7CckAQSOoA==",
+ "node_modules/libnpmpublish/node_modules/hosted-git-info": {
+ "version": "7.0.2",
+ "resolved": "https://registry.npmjs.org/hosted-git-info/-/hosted-git-info-7.0.2.tgz",
+ "integrity": "sha512-puUZAUKT5m8Zzvs72XWy3HtvVbTWljRE66cP60bxJzAqf2DgICo7lYTY2IHUmLnNpjYvw5bvmoHvPc0QO2a62w==",
"dev": true,
+ "license": "ISC",
+ "dependencies": {
+ "lru-cache": "^10.0.1"
+ },
"engines": {
- "node": ">=12"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/libnpmpublish/node_modules/minipass": {
@@ -33448,33 +31248,34 @@
}
},
"node_modules/libnpmpublish/node_modules/normalize-package-data": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/normalize-package-data/-/normalize-package-data-5.0.0.tgz",
- "integrity": "sha512-h9iPVIfrVZ9wVYQnxFgtw1ugSvGEMOlyPWWtm8BMJhnwyEL/FLbYbTY3V3PpjI/BUK67n9PEWDu6eHzu1fB15Q==",
+ "version": "6.0.2",
+ "resolved": "https://registry.npmjs.org/normalize-package-data/-/normalize-package-data-6.0.2.tgz",
+ "integrity": "sha512-V6gygoYb/5EmNI+MEGrWkC+e6+Rr7mTmfHrxDbLzxQogBkgzo76rkok0Am6thgSF7Mv2nLOajAJj5vDJZEFn7g==",
"dev": true,
+ "license": "BSD-2-Clause",
"dependencies": {
- "hosted-git-info": "^6.0.0",
- "is-core-module": "^2.8.1",
+ "hosted-git-info": "^7.0.0",
"semver": "^7.3.5",
"validate-npm-package-license": "^3.0.4"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/libnpmpublish/node_modules/npm-package-arg": {
- "version": "10.1.0",
- "resolved": "https://registry.npmjs.org/npm-package-arg/-/npm-package-arg-10.1.0.tgz",
- "integrity": "sha512-uFyyCEmgBfZTtrKk/5xDfHp6+MdrqGotX/VoOyEEl3mBwiEE5FlBaePanazJSVMPT7vKepcjYBY2ztg9A3yPIA==",
+ "version": "11.0.3",
+ "resolved": "https://registry.npmjs.org/npm-package-arg/-/npm-package-arg-11.0.3.tgz",
+ "integrity": "sha512-sHGJy8sOC1YraBywpzQlIKBE4pBbGbiF95U6Auspzyem956E0+FtDtsx1ZxlOJkQCZ1AFXAY/yuvtFYrOxF+Bw==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "hosted-git-info": "^6.0.0",
- "proc-log": "^3.0.0",
+ "hosted-git-info": "^7.0.0",
+ "proc-log": "^4.0.0",
"semver": "^7.3.5",
"validate-npm-package-name": "^5.0.0"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/libnpmpublish/node_modules/ssri": {
@@ -33482,6 +31283,7 @@
"resolved": "https://registry.npmjs.org/ssri/-/ssri-10.0.6.tgz",
"integrity": "sha512-MGrFH9Z4NP9Iyhqn16sDtBpRRNJ0Y2hNa6D65h736fVSaPCHr4DM4sWUNvVaSuC+0OBGhwsrydQwmgfg5LncqQ==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"minipass": "^7.0.3"
},
@@ -33494,39 +31296,51 @@
"resolved": "https://registry.npmjs.org/validate-npm-package-name/-/validate-npm-package-name-5.0.1.tgz",
"integrity": "sha512-OljLrQ9SQdOUqTaQxqL5dEfZWrXExyyWsozYlAWFawPVNuD83igl7uJD2RTkNMbniIYgt8l81eCJGIdQF7avLQ==",
"dev": true,
+ "license": "ISC",
"engines": {
"node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
+ "node_modules/lie": {
+ "version": "3.1.1",
+ "resolved": "https://registry.npmjs.org/lie/-/lie-3.1.1.tgz",
+ "integrity": "sha512-RiNhHysUjhrDQntfYSfY4MU24coXXdEOgw9WGcKHNeEwffDYbF//u87M1EWaMGzuFoSbqW0C9C6lEEhDOAswfw==",
+ "license": "MIT",
+ "dependencies": {
+ "immediate": "~3.0.5"
+ }
+ },
"node_modules/lighthouse": {
- "version": "10.4.0",
- "resolved": "https://registry.npmjs.org/lighthouse/-/lighthouse-10.4.0.tgz",
- "integrity": "sha512-XQWHEWkJ8YxSPsxttBJORy5+hQrzbvGkYfeP3fJjyYKioWkF2MXfFqNK4ZuV4jL8pBu7Z91qnQP6In0bq1yXww==",
+ "version": "12.2.2",
+ "resolved": "https://registry.npmjs.org/lighthouse/-/lighthouse-12.2.2.tgz",
+ "integrity": "sha512-avoiiFeGN1gkWhp/W1schJoXOsTPxRKWV3+uW/rGHuov2g/HGB+4SN9J/av1GNSh13sEYgkHL3iJOp1+mBVKYQ==",
+ "license": "Apache-2.0",
"dependencies": {
- "@sentry/node": "^6.17.4",
- "axe-core": "4.7.2",
- "chrome-launcher": "^0.15.2",
+ "@paulirish/trace_engine": "0.0.32",
+ "@sentry/node": "^7.0.0",
+ "axe-core": "^4.10.2",
+ "chrome-launcher": "^1.1.2",
"configstore": "^5.0.1",
"csp_evaluator": "1.1.1",
- "devtools-protocol": "0.0.1155343",
+ "devtools-protocol": "0.0.1312386",
"enquirer": "^2.3.6",
"http-link-header": "^1.1.1",
- "intl-messageformat": "^4.4.0",
+ "intl-messageformat": "^10.5.3",
"jpeg-js": "^0.4.4",
- "js-library-detector": "^6.6.0",
- "lighthouse-logger": "^1.4.1",
- "lighthouse-stack-packs": "1.11.0",
- "lodash": "^4.17.21",
+ "js-library-detector": "^6.7.0",
+ "lighthouse-logger": "^2.0.1",
+ "lighthouse-stack-packs": "1.12.2",
+ "lodash-es": "^4.17.21",
"lookup-closest-locale": "6.2.0",
"metaviewport-parser": "0.3.0",
"open": "^8.4.0",
"parse-cache-control": "1.0.1",
- "ps-list": "^8.0.0",
- "puppeteer-core": "^20.8.0",
- "robots-parser": "^3.0.0",
+ "puppeteer-core": "^23.8.0",
+ "robots-parser": "^3.0.1",
"semver": "^5.3.0",
"speedline-core": "^1.4.3",
- "third-party-web": "^0.23.3",
+ "third-party-web": "^0.26.1",
+ "tldts-icann": "^6.1.16",
"ws": "^7.0.0",
"yargs": "^17.3.1",
"yargs-parser": "^21.0.0"
@@ -33537,7 +31351,7 @@
"smokehouse": "cli/test/smokehouse/frontends/smokehouse-bin.js"
},
"engines": {
- "node": ">=16.16"
+ "node": ">=18.16"
}
},
"node_modules/lighthouse-logger": {
@@ -33558,39 +31372,54 @@
}
},
"node_modules/lighthouse-stack-packs": {
- "version": "1.11.0",
- "resolved": "https://registry.npmjs.org/lighthouse-stack-packs/-/lighthouse-stack-packs-1.11.0.tgz",
- "integrity": "sha512-sRr0z1S/I26VffRLq9KJsKtLk856YrJlNGmcJmbLX8dFn3MuzVPUbstuChEhqnSxZb8TZmVfthuXuwhG9vRoSw=="
+ "version": "1.12.2",
+ "resolved": "https://registry.npmjs.org/lighthouse-stack-packs/-/lighthouse-stack-packs-1.12.2.tgz",
+ "integrity": "sha512-Ug8feS/A+92TMTCK6yHYLwaFMuelK/hAKRMdldYkMNwv+d9PtWxjXEg6rwKtsUXTADajhdrhXyuNCJ5/sfmPFw==",
+ "license": "Apache-2.0"
},
- "node_modules/lighthouse/node_modules/cliui": {
- "version": "8.0.1",
- "resolved": "https://registry.npmjs.org/cliui/-/cliui-8.0.1.tgz",
- "integrity": "sha512-BSeNnyus75C4//NQ9gQt1/csTXyo/8Sb+afLAkzAptFuMsod9HFokGNudZpi/oQV73hnVK+sR+5PVRMd+Dr7YQ==",
+ "node_modules/lighthouse/node_modules/chrome-launcher": {
+ "version": "1.1.2",
+ "resolved": "https://registry.npmjs.org/chrome-launcher/-/chrome-launcher-1.1.2.tgz",
+ "integrity": "sha512-YclTJey34KUm5jB1aEJCq807bSievi7Nb/TU4Gu504fUYi3jw3KCIaH6L7nFWQhdEgH3V+wCh+kKD1P5cXnfxw==",
+ "license": "Apache-2.0",
"dependencies": {
- "string-width": "^4.2.0",
- "strip-ansi": "^6.0.1",
- "wrap-ansi": "^7.0.0"
+ "@types/node": "*",
+ "escape-string-regexp": "^4.0.0",
+ "is-wsl": "^2.2.0",
+ "lighthouse-logger": "^2.0.1"
+ },
+ "bin": {
+ "print-chrome-path": "bin/print-chrome-path.js"
},
"engines": {
- "node": ">=12"
+ "node": ">=12.13.0"
}
},
- "node_modules/lighthouse/node_modules/devtools-protocol": {
- "version": "0.0.1155343",
- "resolved": "https://registry.npmjs.org/devtools-protocol/-/devtools-protocol-0.0.1155343.tgz",
- "integrity": "sha512-oD9vGBV2wTc7fAzAM6KC0chSgs234V8+qDEeK+mcbRj2UvcuA7lgBztGi/opj/iahcXD3BSj8Ymvib628yy9FA=="
+ "node_modules/lighthouse/node_modules/debug": {
+ "version": "2.6.9",
+ "resolved": "https://registry.npmjs.org/debug/-/debug-2.6.9.tgz",
+ "integrity": "sha512-bC7ElrdJaJnPbAP+1EotYvqZsb3ecl5wi6Bfi6BJTUcNowp6cvspg0jXznRTKDjm/E7AdgFBVeAPVMNcKGsHMA==",
+ "license": "MIT",
+ "dependencies": {
+ "ms": "2.0.0"
+ }
},
- "node_modules/lighthouse/node_modules/emoji-regex": {
- "version": "8.0.0",
- "resolved": "https://registry.npmjs.org/emoji-regex/-/emoji-regex-8.0.0.tgz",
- "integrity": "sha512-MSjYzcWNOA0ewAHpz0MxpYFvwg6yjy1NG3xteoqz644VCo/RPgnr1/GGt+ic3iJTzQ8Eu3TdM14SawnVUmGE6A=="
+ "node_modules/lighthouse/node_modules/devtools-protocol": {
+ "version": "0.0.1312386",
+ "resolved": "https://registry.npmjs.org/devtools-protocol/-/devtools-protocol-0.0.1312386.tgz",
+ "integrity": "sha512-DPnhUXvmvKT2dFA/j7B+riVLUt9Q6RKJlcppojL5CoRywJJKLDYnRlw0gTFKfgDPHP5E04UoB71SxoJlVZy8FA==",
+ "license": "BSD-3-Clause"
},
- "node_modules/lighthouse/node_modules/is-fullwidth-code-point": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/is-fullwidth-code-point/-/is-fullwidth-code-point-3.0.0.tgz",
- "integrity": "sha512-zymm5+u+sCsSWyD9qNaejV3DFvhCKclKdizYaJUuHA83RLjb7nSuGnddCHGv0hk+KY7BMAlsWeK4Ueg6EV6XQg==",
+ "node_modules/lighthouse/node_modules/escape-string-regexp": {
+ "version": "4.0.0",
+ "resolved": "https://registry.npmjs.org/escape-string-regexp/-/escape-string-regexp-4.0.0.tgz",
+ "integrity": "sha512-TtpcNJ3XAzx3Gq8sWRzJaVajRs0uVxA2YAkdb1jm2YkPz4G6egUFAyA3n5vtEIZefPk5Wa4UXbKuS5fKkJWdgA==",
+ "license": "MIT",
"engines": {
- "node": ">=8"
+ "node": ">=10"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
"node_modules/lighthouse/node_modules/is-wsl": {
@@ -33604,6 +31433,16 @@
"node": ">=8"
}
},
+ "node_modules/lighthouse/node_modules/lighthouse-logger": {
+ "version": "2.0.1",
+ "resolved": "https://registry.npmjs.org/lighthouse-logger/-/lighthouse-logger-2.0.1.tgz",
+ "integrity": "sha512-ioBrW3s2i97noEmnXxmUq7cjIcVRjT5HBpAYy8zE11CxU9HqlWHHeRxfeN1tn8F7OEMVPIC9x1f8t3Z7US9ehQ==",
+ "license": "Apache-2.0",
+ "dependencies": {
+ "debug": "^2.6.9",
+ "marky": "^1.2.2"
+ }
+ },
"node_modules/lighthouse/node_modules/open": {
"version": "8.4.2",
"resolved": "https://registry.npmjs.org/open/-/open-8.4.2.tgz",
@@ -33620,55 +31459,6 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/lighthouse/node_modules/puppeteer-core": {
- "version": "20.9.0",
- "resolved": "https://registry.npmjs.org/puppeteer-core/-/puppeteer-core-20.9.0.tgz",
- "integrity": "sha512-H9fYZQzMTRrkboEfPmf7m3CLDN6JvbxXA3qTtS+dFt27tR+CsFHzPsT6pzp6lYL6bJbAPaR0HaPO6uSi+F94Pg==",
- "dependencies": {
- "@puppeteer/browsers": "1.4.6",
- "chromium-bidi": "0.4.16",
- "cross-fetch": "4.0.0",
- "debug": "4.3.4",
- "devtools-protocol": "0.0.1147663",
- "ws": "8.13.0"
- },
- "engines": {
- "node": ">=16.3.0"
- },
- "peerDependencies": {
- "typescript": ">= 4.7.4"
- },
- "peerDependenciesMeta": {
- "typescript": {
- "optional": true
- }
- }
- },
- "node_modules/lighthouse/node_modules/puppeteer-core/node_modules/devtools-protocol": {
- "version": "0.0.1147663",
- "resolved": "https://registry.npmjs.org/devtools-protocol/-/devtools-protocol-0.0.1147663.tgz",
- "integrity": "sha512-hyWmRrexdhbZ1tcJUGpO95ivbRhWXz++F4Ko+n21AY5PNln2ovoJw+8ZMNDTtip+CNFQfrtLVh/w4009dXO/eQ=="
- },
- "node_modules/lighthouse/node_modules/puppeteer-core/node_modules/ws": {
- "version": "8.13.0",
- "resolved": "https://registry.npmjs.org/ws/-/ws-8.13.0.tgz",
- "integrity": "sha512-x9vcZYTrFPC7aSIbj7sRCYo7L/Xb8Iy+pW0ng0wt2vCJv7M9HOMy0UoN3rr+IFC7hb7vXoqS+P9ktyLLLhO+LA==",
- "engines": {
- "node": ">=10.0.0"
- },
- "peerDependencies": {
- "bufferutil": "^4.0.1",
- "utf-8-validate": ">=5.0.2"
- },
- "peerDependenciesMeta": {
- "bufferutil": {
- "optional": true
- },
- "utf-8-validate": {
- "optional": true
- }
- }
- },
"node_modules/lighthouse/node_modules/semver": {
"version": "5.7.2",
"resolved": "https://registry.npmjs.org/semver/-/semver-5.7.2.tgz",
@@ -33677,60 +31467,6 @@
"semver": "bin/semver"
}
},
- "node_modules/lighthouse/node_modules/string-width": {
- "version": "4.2.3",
- "resolved": "https://registry.npmjs.org/string-width/-/string-width-4.2.3.tgz",
- "integrity": "sha512-wKyQRQpjJ0sIp62ErSZdGsjMJWsap5oRNihHhu6G7JVO/9jIB6UyevL+tXuOqrng8j/cxKTWyWUwvSTriiZz/g==",
- "dependencies": {
- "emoji-regex": "^8.0.0",
- "is-fullwidth-code-point": "^3.0.0",
- "strip-ansi": "^6.0.1"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/lighthouse/node_modules/wrap-ansi": {
- "version": "7.0.0",
- "resolved": "https://registry.npmjs.org/wrap-ansi/-/wrap-ansi-7.0.0.tgz",
- "integrity": "sha512-YVGIj2kamLSTxw6NsZjoBxfSwsn0ycdesmc4p+Q21c5zPuZ1pl+NfxVdxPtdHvmNVOQ6XSYG4AUtyt/Fi7D16Q==",
- "dependencies": {
- "ansi-styles": "^4.0.0",
- "string-width": "^4.1.0",
- "strip-ansi": "^6.0.0"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/chalk/wrap-ansi?sponsor=1"
- }
- },
- "node_modules/lighthouse/node_modules/y18n": {
- "version": "5.0.8",
- "resolved": "https://registry.npmjs.org/y18n/-/y18n-5.0.8.tgz",
- "integrity": "sha512-0pfFzegeDWJHJIAmTLRP2DwHjdF5s7jo9tuztdQxAhINCdvS+3nGINqPd00AphqJR/0LhANUS6/+7SCb98YOfA==",
- "engines": {
- "node": ">=10"
- }
- },
- "node_modules/lighthouse/node_modules/yargs": {
- "version": "17.7.2",
- "resolved": "https://registry.npmjs.org/yargs/-/yargs-17.7.2.tgz",
- "integrity": "sha512-7dSzzRQ++CKnNI/krKnYRV7JKKPUXMEh61soaHKg9mrWEhzFWhFnxPxGl+69cD1Ou63C13NUPCnmIcrvqCuM6w==",
- "dependencies": {
- "cliui": "^8.0.1",
- "escalade": "^3.1.1",
- "get-caller-file": "^2.0.5",
- "require-directory": "^2.1.1",
- "string-width": "^4.2.3",
- "y18n": "^5.0.5",
- "yargs-parser": "^21.1.1"
- },
- "engines": {
- "node": ">=12"
- }
- },
"node_modules/lighthouse/node_modules/yargs-parser": {
"version": "21.1.1",
"resolved": "https://registry.npmjs.org/yargs-parser/-/yargs-parser-21.1.1.tgz",
@@ -33836,20 +31572,6 @@
"node": ">=8"
}
},
- "node_modules/lint-staged/node_modules/cross-spawn": {
- "version": "7.0.3",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-7.0.3.tgz",
- "integrity": "sha512-iRDPJKUPVEND7dHPO8rkbOnPpyDygcDFtWjpeWNCgy8WP2rXcxXL8TskReQl6OrB2G7+UJrags1q15Fudc7G6w==",
- "dev": true,
- "dependencies": {
- "path-key": "^3.1.0",
- "shebang-command": "^2.0.0",
- "which": "^2.0.1"
- },
- "engines": {
- "node": ">= 8"
- }
- },
"node_modules/lint-staged/node_modules/execa": {
"version": "3.4.0",
"resolved": "https://registry.npmjs.org/execa/-/execa-3.4.0.tgz",
@@ -34014,27 +31736,6 @@
"node": ">=8"
}
},
- "node_modules/lint-staged/node_modules/shebang-command": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/shebang-command/-/shebang-command-2.0.0.tgz",
- "integrity": "sha512-kHxr2zZpYtdmrN1qDjrrX/Z1rR1kG8Dx+gkpK1G4eXmvXswmcE1hTWBWYUzlraYw1/yZp6YuDY77YtvbN0dmDA==",
- "dev": true,
- "dependencies": {
- "shebang-regex": "^3.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/lint-staged/node_modules/shebang-regex": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/shebang-regex/-/shebang-regex-3.0.0.tgz",
- "integrity": "sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==",
- "dev": true,
- "engines": {
- "node": ">=8"
- }
- },
"node_modules/lint-staged/node_modules/supports-color": {
"version": "7.2.0",
"resolved": "https://registry.npmjs.org/supports-color/-/supports-color-7.2.0.tgz",
@@ -34047,27 +31748,6 @@
"node": ">=8"
}
},
- "node_modules/lint-staged/node_modules/which": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
- "integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
- "dev": true,
- "dependencies": {
- "isexe": "^2.0.0"
- },
- "bin": {
- "node-which": "bin/node-which"
- },
- "engines": {
- "node": ">= 8"
- }
- },
- "node_modules/listenercount": {
- "version": "1.0.1",
- "resolved": "https://registry.npmjs.org/listenercount/-/listenercount-1.0.1.tgz",
- "integrity": "sha512-3mk/Zag0+IJxeDrxSgaDPy4zZ3w05PRZeJNnlWhzFz5OkX49J4krc+A8X2d2M69vGMBEX0uyl8M+W+8gH+kBqQ==",
- "dev": true
- },
"node_modules/listr": {
"version": "0.14.3",
"resolved": "https://registry.npmjs.org/listr/-/listr-0.14.3.tgz",
@@ -34266,6 +31946,7 @@
"resolved": "https://registry.npmjs.org/load-json-file/-/load-json-file-6.2.0.tgz",
"integrity": "sha512-gUD/epcRms75Cw8RT1pUdHugZYM5ce64ucs2GEISABwkRsOQr0q2wm/MV2TKThycIe5e0ytRweW2RZxclogCdQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"graceful-fs": "^4.1.15",
"parse-json": "^5.0.0",
@@ -34298,24 +31979,45 @@
"node": ">=8.9.0"
}
},
+ "node_modules/localforage": {
+ "version": "1.10.0",
+ "resolved": "https://registry.npmjs.org/localforage/-/localforage-1.10.0.tgz",
+ "integrity": "sha512-14/H1aX7hzBBmmh7sGPd+AOMkkIrHM3Z1PAyGgZigA1H1p5O5ANnMyWzvpAETtG68/dC4pC0ncy3+PPGzXZHPg==",
+ "license": "Apache-2.0",
+ "dependencies": {
+ "lie": "3.1.1"
+ }
+ },
"node_modules/locate-app": {
- "version": "2.1.0",
- "resolved": "https://registry.npmjs.org/locate-app/-/locate-app-2.1.0.tgz",
- "integrity": "sha512-rcVo/iLUxrd9d0lrmregK/Z5Y5NCpSwf9KlMbPpOHmKmdxdQY1Fj8NDQ5QymJTryCsBLqwmniFv2f3JKbk9Bvg==",
+ "version": "2.5.0",
+ "resolved": "https://registry.npmjs.org/locate-app/-/locate-app-2.5.0.tgz",
+ "integrity": "sha512-xIqbzPMBYArJRmPGUZD9CzV9wOqmVtQnaAn3wrj3s6WYW0bQvPI7x+sPYUGmDTYMHefVK//zc6HEYZ1qnxIK+Q==",
"dev": true,
+ "funding": [
+ {
+ "type": "individual",
+ "url": "https://buymeacoffee.com/hejny"
+ },
+ {
+ "type": "github",
+ "url": "https://github.com/hejny/locate-app/blob/main/README.md#%EF%B8%8F-contributing"
+ }
+ ],
+ "license": "Apache-2.0",
"dependencies": {
- "n12": "0.4.0",
- "type-fest": "2.13.0",
- "userhome": "1.0.0"
+ "@promptbook/utils": "0.69.5",
+ "type-fest": "4.26.0",
+ "userhome": "1.0.1"
}
},
"node_modules/locate-app/node_modules/type-fest": {
- "version": "2.13.0",
- "resolved": "https://registry.npmjs.org/type-fest/-/type-fest-2.13.0.tgz",
- "integrity": "sha512-lPfAm42MxE4/456+QyIaaVBAwgpJb6xZ8PRu09utnhPdWwcyj9vgy6Sq0Z5yNbJ21EdxB5dRU/Qg8bsyAMtlcw==",
+ "version": "4.26.0",
+ "resolved": "https://registry.npmjs.org/type-fest/-/type-fest-4.26.0.tgz",
+ "integrity": "sha512-OduNjVJsFbifKb57UqZ2EMP1i4u64Xwow3NYXUtBbD4vIwJdQd4+xl8YDou1dlm4DVrtwT/7Ky8z8WyCULVfxw==",
"dev": true,
+ "license": "(MIT OR CC0-1.0)",
"engines": {
- "node": ">=12.20"
+ "node": ">=16"
},
"funding": {
"url": "https://github.com/sponsors/sindresorhus"
@@ -34347,6 +32049,12 @@
"resolved": "https://registry.npmjs.org/lodash/-/lodash-4.17.21.tgz",
"integrity": "sha512-v2kDEe57lecTulaDIuNTPy3Ry4gLGJ6Z1O3vE1krgXZNrsQ+LFTGHVxVjcXPs17LhbZVGedAJv8XZ1tvj5FvSg=="
},
+ "node_modules/lodash-es": {
+ "version": "4.17.21",
+ "resolved": "https://registry.npmjs.org/lodash-es/-/lodash-es-4.17.21.tgz",
+ "integrity": "sha512-mKnC+QJ9pWVzv+C4/U3rRsHapFfHvQFoFB92e52xeyGMcX6/OlIl78je1u8vePzYZSkkogMPJ2yjxxsb89cxyw==",
+ "license": "MIT"
+ },
"node_modules/lodash.clonedeep": {
"version": "4.5.0",
"resolved": "https://registry.npmjs.org/lodash.clonedeep/-/lodash.clonedeep-4.5.0.tgz",
@@ -34383,7 +32091,8 @@
"version": "4.4.0",
"resolved": "https://registry.npmjs.org/lodash.ismatch/-/lodash.ismatch-4.4.0.tgz",
"integrity": "sha512-fPMfXjGQEV9Xsq/8MTSgUf255gawYRbjwMyDbcvDhXgV7enSZA0hynz6vMPnpAb5iONEzBHBPsT+0zes5Z301g==",
- "dev": true
+ "dev": true,
+ "license": "MIT"
},
"node_modules/lodash.memoize": {
"version": "4.1.2",
@@ -34661,10 +32370,11 @@
}
},
"node_modules/loglevel": {
- "version": "1.8.1",
- "resolved": "https://registry.npmjs.org/loglevel/-/loglevel-1.8.1.tgz",
- "integrity": "sha512-tCRIJM51SHjAayKwC+QAg8hT8vg6z7GSgLJKGvzuPb1Wc+hLzqtuVLxp6/HzSPOozuK+8ErAhy7U/sVzw8Dgfg==",
+ "version": "1.9.2",
+ "resolved": "https://registry.npmjs.org/loglevel/-/loglevel-1.9.2.tgz",
+ "integrity": "sha512-HgMmCqIJSAKqo68l0rS2AanEWfkxaZ5wNiEFb5ggm08lDs9Xl2KxBlX3PTcaD2chBM1gXAYf491/M2Rv8Jwayg==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">= 0.6.0"
},
@@ -34677,7 +32387,8 @@
"version": "0.8.4",
"resolved": "https://registry.npmjs.org/loglevel-plugin-prefix/-/loglevel-plugin-prefix-0.8.4.tgz",
"integrity": "sha512-WpG9CcFAOjz/FtNht+QJeGpvVl/cdR6P0z6OcXSkr8wFJOsV2GRj2j10JLfjuA4aYkcKCNIEqRGCyTife9R8/g==",
- "dev": true
+ "dev": true,
+ "license": "MIT"
},
"node_modules/longest-streak": {
"version": "2.0.2",
@@ -34700,6 +32411,12 @@
"loose-envify": "cli.js"
}
},
+ "node_modules/loupe": {
+ "version": "3.1.2",
+ "resolved": "https://registry.npmjs.org/loupe/-/loupe-3.1.2.tgz",
+ "integrity": "sha512-23I4pFZHmAemUnz8WZXbYRSKYj801VDaNv9ETuMh7IrMc7VuVVSo+Z9iLE3ni30+U48iDWfi30d3twAXBYmnCg==",
+ "dev": true
+ },
"node_modules/lower-case": {
"version": "2.0.2",
"resolved": "https://registry.npmjs.org/lower-case/-/lower-case-2.0.2.tgz",
@@ -34716,19 +32433,11 @@
"node": ">=8"
}
},
- "node_modules/lru_map": {
- "version": "0.3.3",
- "resolved": "https://registry.npmjs.org/lru_map/-/lru_map-0.3.3.tgz",
- "integrity": "sha512-Pn9cox5CsMYngeDbmChANltQl+5pi6XmTrraMSzhPmMBbmgcxmqWry0U3PGapCU1yB4/LqCcom7qhHZiF/jGfQ=="
- },
"node_modules/lru-cache": {
- "version": "4.1.5",
- "resolved": "https://registry.npmjs.org/lru-cache/-/lru-cache-4.1.5.tgz",
- "integrity": "sha512-sWZlbEP2OsHNkXrMl5GYk/jKk70MBng6UU4YI/qGDYbgf6YbP4EvmqISbXCoJiRKs+1bSpFHVgQxvJ17F2li5g==",
- "dependencies": {
- "pseudomap": "^1.0.2",
- "yallist": "^2.1.2"
- }
+ "version": "10.4.3",
+ "resolved": "https://registry.npmjs.org/lru-cache/-/lru-cache-10.4.3.tgz",
+ "integrity": "sha512-JNAzZcXrCt42VGLuYz0zfAzDfAvJWW6AfYlDBQyDV5DClI2m5sAmK+OIO7s59XfsRsWHp02jAJrRadPRGTt6SQ==",
+ "license": "ISC"
},
"node_modules/lunr": {
"version": "2.3.9",
@@ -34755,15 +32464,12 @@
}
},
"node_modules/magic-string": {
- "version": "0.30.7",
- "resolved": "https://registry.npmjs.org/magic-string/-/magic-string-0.30.7.tgz",
- "integrity": "sha512-8vBuFF/I/+OSLRmdf2wwFCJCz+nSn0m6DPvGH1fS/KiQoSaR+sETbov0eIk9KhEKy8CYqIkIAnbohxT/4H0kuA==",
+ "version": "0.30.15",
+ "resolved": "https://registry.npmjs.org/magic-string/-/magic-string-0.30.15.tgz",
+ "integrity": "sha512-zXeaYRgZ6ldS1RJJUrMrYgNJ4fdwnyI6tVqoiIhyCyv5IVTK9BU8Ic2l253GGETQHxI4HNUwhJ3fjDhKqEoaAw==",
"dev": true,
"dependencies": {
- "@jridgewell/sourcemap-codec": "^1.4.15"
- },
- "engines": {
- "node": ">=12"
+ "@jridgewell/sourcemap-codec": "^1.5.0"
}
},
"node_modules/make-dir": {
@@ -34792,29 +32498,27 @@
"dev": true
},
"node_modules/make-fetch-happen": {
- "version": "11.1.1",
- "resolved": "https://registry.npmjs.org/make-fetch-happen/-/make-fetch-happen-11.1.1.tgz",
- "integrity": "sha512-rLWS7GCSTcEujjVBs2YqG7Y4643u8ucvCJeSRqiLYhesrDuzeuFIk37xREzAsfQaqzl8b9rNCE4m6J8tvX4Q8w==",
+ "version": "13.0.1",
+ "resolved": "https://registry.npmjs.org/make-fetch-happen/-/make-fetch-happen-13.0.1.tgz",
+ "integrity": "sha512-cKTUFc/rbKUd/9meOvgrpJ2WrNzymt6jfRDdwg5UCnVzv9dTpEj9JS5m3wtziXVCjluIXyL8pcaukYqezIzZQA==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "agentkeepalive": "^4.2.1",
- "cacache": "^17.0.0",
+ "@npmcli/agent": "^2.0.0",
+ "cacache": "^18.0.0",
"http-cache-semantics": "^4.1.1",
- "http-proxy-agent": "^5.0.0",
- "https-proxy-agent": "^5.0.0",
"is-lambda": "^1.0.1",
- "lru-cache": "^7.7.1",
- "minipass": "^5.0.0",
+ "minipass": "^7.0.2",
"minipass-fetch": "^3.0.0",
"minipass-flush": "^1.0.5",
"minipass-pipeline": "^1.2.4",
"negotiator": "^0.6.3",
+ "proc-log": "^4.2.0",
"promise-retry": "^2.0.1",
- "socks-proxy-agent": "^7.0.0",
"ssri": "^10.0.0"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/make-fetch-happen/node_modules/brace-expansion": {
@@ -34822,22 +32526,24 @@
"resolved": "https://registry.npmjs.org/brace-expansion/-/brace-expansion-2.0.1.tgz",
"integrity": "sha512-XnAIvQ8eM+kC6aULx6wuQiwVsnzsi9d3WxzV3FpWTGA19F621kwdbsAcFKXgKUHZWsy+mY6iL1sHTxWEFCytDA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"balanced-match": "^1.0.0"
}
},
"node_modules/make-fetch-happen/node_modules/cacache": {
- "version": "17.1.4",
- "resolved": "https://registry.npmjs.org/cacache/-/cacache-17.1.4.tgz",
- "integrity": "sha512-/aJwG2l3ZMJ1xNAnqbMpA40of9dj/pIH3QfiuQSqjfPJF747VR0J/bHn+/KdNnHKc6XQcWt/AfRSBft82W1d2A==",
+ "version": "18.0.4",
+ "resolved": "https://registry.npmjs.org/cacache/-/cacache-18.0.4.tgz",
+ "integrity": "sha512-B+L5iIa9mgcjLbliir2th36yEwPftrzteHYujzsx3dFP/31GCHcIeS8f5MGd80odLOjaOvSpU3EEAmRQptkxLQ==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"@npmcli/fs": "^3.1.0",
"fs-minipass": "^3.0.0",
"glob": "^10.2.2",
- "lru-cache": "^7.7.1",
+ "lru-cache": "^10.0.1",
"minipass": "^7.0.3",
- "minipass-collect": "^1.0.2",
+ "minipass-collect": "^2.0.1",
"minipass-flush": "^1.0.5",
"minipass-pipeline": "^1.2.4",
"p-map": "^4.0.0",
@@ -34846,17 +32552,7 @@
"unique-filename": "^3.0.0"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
- }
- },
- "node_modules/make-fetch-happen/node_modules/cacache/node_modules/minipass": {
- "version": "7.1.2",
- "resolved": "https://registry.npmjs.org/minipass/-/minipass-7.1.2.tgz",
- "integrity": "sha512-qOOzS1cBTWYF4BH8fVePDBOO9iptMnGUEZwNc/cMWnTV2nVLZ7VoNWEPHkYczZA0pdoA7dl6e7FL659nX9S2aw==",
- "dev": true,
- "license": "ISC",
- "engines": {
- "node": ">=16 || 14 >=14.17"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/make-fetch-happen/node_modules/fs-minipass": {
@@ -34864,6 +32560,7 @@
"resolved": "https://registry.npmjs.org/fs-minipass/-/fs-minipass-3.0.3.tgz",
"integrity": "sha512-XUBA9XClHbnJWSfBzjkm6RvPsyg3sryZt06BEQoXcF7EK/xpGaQYJgQKDJSUH5SGZ76Y7pFx1QBnXz09rU5Fbw==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"minipass": "^7.0.3"
},
@@ -34871,16 +32568,6 @@
"node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/make-fetch-happen/node_modules/fs-minipass/node_modules/minipass": {
- "version": "7.1.2",
- "resolved": "https://registry.npmjs.org/minipass/-/minipass-7.1.2.tgz",
- "integrity": "sha512-qOOzS1cBTWYF4BH8fVePDBOO9iptMnGUEZwNc/cMWnTV2nVLZ7VoNWEPHkYczZA0pdoA7dl6e7FL659nX9S2aw==",
- "dev": true,
- "license": "ISC",
- "engines": {
- "node": ">=16 || 14 >=14.17"
- }
- },
"node_modules/make-fetch-happen/node_modules/glob": {
"version": "10.4.5",
"resolved": "https://registry.npmjs.org/glob/-/glob-10.4.5.tgz",
@@ -34902,41 +32589,6 @@
"url": "https://github.com/sponsors/isaacs"
}
},
- "node_modules/make-fetch-happen/node_modules/glob/node_modules/minipass": {
- "version": "7.1.2",
- "resolved": "https://registry.npmjs.org/minipass/-/minipass-7.1.2.tgz",
- "integrity": "sha512-qOOzS1cBTWYF4BH8fVePDBOO9iptMnGUEZwNc/cMWnTV2nVLZ7VoNWEPHkYczZA0pdoA7dl6e7FL659nX9S2aw==",
- "dev": true,
- "license": "ISC",
- "engines": {
- "node": ">=16 || 14 >=14.17"
- }
- },
- "node_modules/make-fetch-happen/node_modules/jackspeak": {
- "version": "3.4.3",
- "resolved": "https://registry.npmjs.org/jackspeak/-/jackspeak-3.4.3.tgz",
- "integrity": "sha512-OGlZQpz2yfahA/Rd1Y8Cd9SIEsqvXkLVoSw/cgwhnhFMDbsQFeZYoJJ7bIZBS9BcamUW96asq/npPWugM+RQBw==",
- "dev": true,
- "license": "BlueOak-1.0.0",
- "dependencies": {
- "@isaacs/cliui": "^8.0.2"
- },
- "funding": {
- "url": "https://github.com/sponsors/isaacs"
- },
- "optionalDependencies": {
- "@pkgjs/parseargs": "^0.11.0"
- }
- },
- "node_modules/make-fetch-happen/node_modules/lru-cache": {
- "version": "7.18.3",
- "resolved": "https://registry.npmjs.org/lru-cache/-/lru-cache-7.18.3.tgz",
- "integrity": "sha512-jumlc0BIUrS3qJGgIkWZsyfAM7NCWiBcCDhnd+3NNM5KbBmLTgHVfWBcg6W+rLUsIpzpERPsvwUP7CckAQSOoA==",
- "dev": true,
- "engines": {
- "node": ">=12"
- }
- },
"node_modules/make-fetch-happen/node_modules/minimatch": {
"version": "9.0.5",
"resolved": "https://registry.npmjs.org/minimatch/-/minimatch-9.0.5.tgz",
@@ -34954,12 +32606,13 @@
}
},
"node_modules/make-fetch-happen/node_modules/minipass": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/minipass/-/minipass-5.0.0.tgz",
- "integrity": "sha512-3FnjYuehv9k6ovOEbyOswadCDPX1piCfhV8ncmYtHOjuPwylVWsghTLo7rabjC3Rx5xD4HDx8Wm1xnMF7S5qFQ==",
+ "version": "7.1.2",
+ "resolved": "https://registry.npmjs.org/minipass/-/minipass-7.1.2.tgz",
+ "integrity": "sha512-qOOzS1cBTWYF4BH8fVePDBOO9iptMnGUEZwNc/cMWnTV2nVLZ7VoNWEPHkYczZA0pdoA7dl6e7FL659nX9S2aw==",
"dev": true,
+ "license": "ISC",
"engines": {
- "node": ">=8"
+ "node": ">=16 || 14 >=14.17"
}
},
"node_modules/make-fetch-happen/node_modules/ssri": {
@@ -34967,6 +32620,7 @@
"resolved": "https://registry.npmjs.org/ssri/-/ssri-10.0.6.tgz",
"integrity": "sha512-MGrFH9Z4NP9Iyhqn16sDtBpRRNJ0Y2hNa6D65h736fVSaPCHr4DM4sWUNvVaSuC+0OBGhwsrydQwmgfg5LncqQ==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"minipass": "^7.0.3"
},
@@ -34974,21 +32628,12 @@
"node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/make-fetch-happen/node_modules/ssri/node_modules/minipass": {
- "version": "7.1.2",
- "resolved": "https://registry.npmjs.org/minipass/-/minipass-7.1.2.tgz",
- "integrity": "sha512-qOOzS1cBTWYF4BH8fVePDBOO9iptMnGUEZwNc/cMWnTV2nVLZ7VoNWEPHkYczZA0pdoA7dl6e7FL659nX9S2aw==",
- "dev": true,
- "license": "ISC",
- "engines": {
- "node": ">=16 || 14 >=14.17"
- }
- },
"node_modules/make-fetch-happen/node_modules/unique-filename": {
"version": "3.0.0",
"resolved": "https://registry.npmjs.org/unique-filename/-/unique-filename-3.0.0.tgz",
"integrity": "sha512-afXhuC55wkAmZ0P18QsVE6kp8JaxrEokN2HGIoIVv2ijHQd419H0+6EigAFcIzXeMIkcIkNBpB3L/DXB3cTS/g==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"unique-slug": "^4.0.0"
},
@@ -35001,6 +32646,7 @@
"resolved": "https://registry.npmjs.org/unique-slug/-/unique-slug-4.0.0.tgz",
"integrity": "sha512-WrcA6AyEfqDX5bWige/4NQfPZMtASNVxdmWR76WESYQVAACSgWcR6e9i0mofqqBxYFtL4oAxPIptY73/0YE1DQ==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"imurmurhash": "^0.1.4"
},
@@ -35081,10 +32727,11 @@
}
},
"node_modules/markdown-builder/node_modules/cross-spawn": {
- "version": "6.0.5",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-6.0.5.tgz",
- "integrity": "sha512-eTVLrBSt7fjbDygz805pMnstIs2VTBNkRm0qxZd+M7A5XDdxVRWO5MxGBXZhjY4cqLYLdtrGqRf8mBPmzwSpWQ==",
+ "version": "6.0.6",
+ "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-6.0.6.tgz",
+ "integrity": "sha512-VqCUuhcd1iB+dsv8gxPttb5iZh/D0iubSP21g36KXdEuf6I5JiioesUVjpCdHV9MZRUfVFlvwtIUyPfxo5trtw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"nice-try": "^1.0.4",
"path-key": "^2.0.1",
@@ -35349,18 +32996,6 @@
"resolved": "https://registry.npmjs.org/markdown-table/-/markdown-table-1.1.3.tgz",
"integrity": "sha512-1RUZVgQlpJSPWYbFSpmudq5nHY1doEIv89gBtF0s4gW1GF2XorxcA/70M5vq7rLv0a6mhOUccRsqkwhwLCIQ2Q=="
},
- "node_modules/markdown-to-jsx": {
- "version": "7.4.1",
- "resolved": "https://registry.npmjs.org/markdown-to-jsx/-/markdown-to-jsx-7.4.1.tgz",
- "integrity": "sha512-GbrbkTnHp9u6+HqbPRFJbObi369AgJNXi/sGqq5HRsoZW063xR1XDCaConqq+whfEIAlzB1YPnOgsPc7B7bc/A==",
- "dev": true,
- "engines": {
- "node": ">= 10"
- },
- "peerDependencies": {
- "react": ">= 0.14.0"
- }
- },
"node_modules/markdownlint": {
"version": "0.25.1",
"resolved": "https://registry.npmjs.org/markdownlint/-/markdownlint-0.25.1.tgz",
@@ -35534,58 +33169,6 @@
"unist-util-visit": "^1.1.0"
}
},
- "node_modules/mdast-util-definitions": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/mdast-util-definitions/-/mdast-util-definitions-4.0.0.tgz",
- "integrity": "sha512-k8AJ6aNnUkB7IE+5azR9h81O5EQ/cTDXtWdMq9Kk5KcEW/8ritU5CeLg/9HhOC++nALHBlaogJ5jz0Ybk3kPMQ==",
- "dev": true,
- "dependencies": {
- "unist-util-visit": "^2.0.0"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/unified"
- }
- },
- "node_modules/mdast-util-definitions/node_modules/unist-util-is": {
- "version": "4.1.0",
- "resolved": "https://registry.npmjs.org/unist-util-is/-/unist-util-is-4.1.0.tgz",
- "integrity": "sha512-ZOQSsnce92GrxSqlnEEseX0gi7GH9zTJZ0p9dtu87WRb/37mMPO2Ilx1s/t9vBHrFhbgweUwb+t7cIn5dxPhZg==",
- "dev": true,
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/unified"
- }
- },
- "node_modules/mdast-util-definitions/node_modules/unist-util-visit": {
- "version": "2.0.3",
- "resolved": "https://registry.npmjs.org/unist-util-visit/-/unist-util-visit-2.0.3.tgz",
- "integrity": "sha512-iJ4/RczbJMkD0712mGktuGpm/U4By4FfDonL7N/9tATGIF4imikjOuagyMY53tnZq3NP6BcmlrHhEKAfGWjh7Q==",
- "dev": true,
- "dependencies": {
- "@types/unist": "^2.0.0",
- "unist-util-is": "^4.0.0",
- "unist-util-visit-parents": "^3.0.0"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/unified"
- }
- },
- "node_modules/mdast-util-definitions/node_modules/unist-util-visit-parents": {
- "version": "3.1.1",
- "resolved": "https://registry.npmjs.org/unist-util-visit-parents/-/unist-util-visit-parents-3.1.1.tgz",
- "integrity": "sha512-1KROIZWo6bcMrZEwiH2UrXDyalAa0uqzWCxCJj6lPOvTve2WkfgCytoDTPaMnodXh1WrXOq0haVYHj99ynJlsg==",
- "dev": true,
- "dependencies": {
- "@types/unist": "^2.0.0",
- "unist-util-is": "^4.0.0"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/unified"
- }
- },
"node_modules/mdast-util-inject": {
"version": "1.1.0",
"resolved": "https://registry.npmjs.org/mdast-util-inject/-/mdast-util-inject-1.1.0.tgz",
@@ -35671,6 +33254,7 @@
"resolved": "https://registry.npmjs.org/meow/-/meow-8.1.2.tgz",
"integrity": "sha512-r85E3NdZ+mpYk1C6RjPFEMSE+s1iZMuHtsHAqY0DT3jZczl0diWUZ8g6oU7h0M9cD2EL+PzaYghhCLzR0ZNn5Q==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"@types/minimist": "^1.2.0",
"camelcase-keys": "^6.2.2",
@@ -35696,6 +33280,7 @@
"resolved": "https://registry.npmjs.org/normalize-package-data/-/normalize-package-data-3.0.3.tgz",
"integrity": "sha512-p2W1sgqij3zMMyRC067Dg16bfzVH+w7hyegmpIvZ4JNjqtGOVAIvLmjBx3yP7YTe9vKJgkoNOPjwQGogDoMXFA==",
"dev": true,
+ "license": "BSD-2-Clause",
"dependencies": {
"hosted-git-info": "^4.0.1",
"is-core-module": "^2.5.0",
@@ -35711,6 +33296,7 @@
"resolved": "https://registry.npmjs.org/type-fest/-/type-fest-0.18.1.tgz",
"integrity": "sha512-OIAYXk8+ISY+qTOwkHtKqzAuxchoMiD9Udx+FSGQDuiRR+PJKJHc2NJAXlbhkGwTt/4/nKZxELY1w3ReWOL8mw==",
"dev": true,
+ "license": "(MIT OR CC0-1.0)",
"engines": {
"node": ">=10"
},
@@ -35804,7 +33390,8 @@
"node_modules/merge-descriptors": {
"version": "1.0.1",
"resolved": "https://registry.npmjs.org/merge-descriptors/-/merge-descriptors-1.0.1.tgz",
- "integrity": "sha512-cCi6g3/Zr1iqQi6ySbseM1Xvooa98N0w31jzUYrXPX2xqObmFGHJ0tQ5u74H3mVh7wLouTseZyYIq39g8cNp1w=="
+ "integrity": "sha512-cCi6g3/Zr1iqQi6ySbseM1Xvooa98N0w31jzUYrXPX2xqObmFGHJ0tQ5u74H3mVh7wLouTseZyYIq39g8cNp1w==",
+ "license": "MIT"
},
"node_modules/merge-stream": {
"version": "2.0.0",
@@ -35958,6 +33545,43 @@
"node": ">=18"
}
},
+ "node_modules/metro-cache/node_modules/glob": {
+ "version": "7.2.3",
+ "resolved": "https://registry.npmjs.org/glob/-/glob-7.2.3.tgz",
+ "integrity": "sha512-nFR0zLpU2YCaRxwoCJvL6UvCH2JFyFVIvwTLsIf21AuHlMskA1hhTdk+LlYJtOlYt9v6dvszD2BGRqBL+iQK9Q==",
+ "deprecated": "Glob versions prior to v9 are no longer supported",
+ "license": "ISC",
+ "dependencies": {
+ "fs.realpath": "^1.0.0",
+ "inflight": "^1.0.4",
+ "inherits": "2",
+ "minimatch": "^3.1.1",
+ "once": "^1.3.0",
+ "path-is-absolute": "^1.0.0"
+ },
+ "engines": {
+ "node": "*"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
+ }
+ },
+ "node_modules/metro-cache/node_modules/rimraf": {
+ "version": "3.0.2",
+ "resolved": "https://registry.npmjs.org/rimraf/-/rimraf-3.0.2.tgz",
+ "integrity": "sha512-JZkJMZkAGFFPP2YqXZXPbMlMBgsxzE8ILs4lMIX/2o0L9UBw9O/Y3o6wFw/i9YLapcUJWwqbi3kdxIPdC62TIA==",
+ "deprecated": "Rimraf versions prior to v4 are no longer supported",
+ "license": "ISC",
+ "dependencies": {
+ "glob": "^7.1.3"
+ },
+ "bin": {
+ "rimraf": "bin.js"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
+ }
+ },
"node_modules/metro-config": {
"version": "0.80.5",
"resolved": "https://registry.npmjs.org/metro-config/-/metro-config-0.80.5.tgz",
@@ -36181,19 +33805,6 @@
"resolved": "https://registry.npmjs.org/ci-info/-/ci-info-2.0.0.tgz",
"integrity": "sha512-5tK7EtrZ0N+OLFMthtqOj4fI2Jeb88C4CAZPu25LDVUgXJ0A3Js4PMGqrn0JU1W0Mh1/Z8wZzYPxqUrXeBboCQ=="
},
- "node_modules/metro/node_modules/cliui": {
- "version": "8.0.1",
- "resolved": "https://registry.npmjs.org/cliui/-/cliui-8.0.1.tgz",
- "integrity": "sha512-BSeNnyus75C4//NQ9gQt1/csTXyo/8Sb+afLAkzAptFuMsod9HFokGNudZpi/oQV73hnVK+sR+5PVRMd+Dr7YQ==",
- "dependencies": {
- "string-width": "^4.2.0",
- "strip-ansi": "^6.0.1",
- "wrap-ansi": "^7.0.0"
- },
- "engines": {
- "node": ">=12"
- }
- },
"node_modules/metro/node_modules/debug": {
"version": "2.6.9",
"resolved": "https://registry.npmjs.org/debug/-/debug-2.6.9.tgz",
@@ -36202,10 +33813,26 @@
"ms": "2.0.0"
}
},
- "node_modules/metro/node_modules/emoji-regex": {
- "version": "8.0.0",
- "resolved": "https://registry.npmjs.org/emoji-regex/-/emoji-regex-8.0.0.tgz",
- "integrity": "sha512-MSjYzcWNOA0ewAHpz0MxpYFvwg6yjy1NG3xteoqz644VCo/RPgnr1/GGt+ic3iJTzQ8Eu3TdM14SawnVUmGE6A=="
+ "node_modules/metro/node_modules/glob": {
+ "version": "7.2.3",
+ "resolved": "https://registry.npmjs.org/glob/-/glob-7.2.3.tgz",
+ "integrity": "sha512-nFR0zLpU2YCaRxwoCJvL6UvCH2JFyFVIvwTLsIf21AuHlMskA1hhTdk+LlYJtOlYt9v6dvszD2BGRqBL+iQK9Q==",
+ "deprecated": "Glob versions prior to v9 are no longer supported",
+ "license": "ISC",
+ "dependencies": {
+ "fs.realpath": "^1.0.0",
+ "inflight": "^1.0.4",
+ "inherits": "2",
+ "minimatch": "^3.1.1",
+ "once": "^1.3.0",
+ "path-is-absolute": "^1.0.0"
+ },
+ "engines": {
+ "node": "*"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
+ }
},
"node_modules/metro/node_modules/hermes-estree": {
"version": "0.18.2",
@@ -36220,12 +33847,20 @@
"hermes-estree": "0.18.2"
}
},
- "node_modules/metro/node_modules/is-fullwidth-code-point": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/is-fullwidth-code-point/-/is-fullwidth-code-point-3.0.0.tgz",
- "integrity": "sha512-zymm5+u+sCsSWyD9qNaejV3DFvhCKclKdizYaJUuHA83RLjb7nSuGnddCHGv0hk+KY7BMAlsWeK4Ueg6EV6XQg==",
- "engines": {
- "node": ">=8"
+ "node_modules/metro/node_modules/rimraf": {
+ "version": "3.0.2",
+ "resolved": "https://registry.npmjs.org/rimraf/-/rimraf-3.0.2.tgz",
+ "integrity": "sha512-JZkJMZkAGFFPP2YqXZXPbMlMBgsxzE8ILs4lMIX/2o0L9UBw9O/Y3o6wFw/i9YLapcUJWwqbi3kdxIPdC62TIA==",
+ "deprecated": "Rimraf versions prior to v4 are no longer supported",
+ "license": "ISC",
+ "dependencies": {
+ "glob": "^7.1.3"
+ },
+ "bin": {
+ "rimraf": "bin.js"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
}
},
"node_modules/metro/node_modules/source-map": {
@@ -36236,68 +33871,6 @@
"node": ">=0.10.0"
}
},
- "node_modules/metro/node_modules/string-width": {
- "version": "4.2.3",
- "resolved": "https://registry.npmjs.org/string-width/-/string-width-4.2.3.tgz",
- "integrity": "sha512-wKyQRQpjJ0sIp62ErSZdGsjMJWsap5oRNihHhu6G7JVO/9jIB6UyevL+tXuOqrng8j/cxKTWyWUwvSTriiZz/g==",
- "dependencies": {
- "emoji-regex": "^8.0.0",
- "is-fullwidth-code-point": "^3.0.0",
- "strip-ansi": "^6.0.1"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/metro/node_modules/wrap-ansi": {
- "version": "7.0.0",
- "resolved": "https://registry.npmjs.org/wrap-ansi/-/wrap-ansi-7.0.0.tgz",
- "integrity": "sha512-YVGIj2kamLSTxw6NsZjoBxfSwsn0ycdesmc4p+Q21c5zPuZ1pl+NfxVdxPtdHvmNVOQ6XSYG4AUtyt/Fi7D16Q==",
- "dependencies": {
- "ansi-styles": "^4.0.0",
- "string-width": "^4.1.0",
- "strip-ansi": "^6.0.0"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/chalk/wrap-ansi?sponsor=1"
- }
- },
- "node_modules/metro/node_modules/y18n": {
- "version": "5.0.8",
- "resolved": "https://registry.npmjs.org/y18n/-/y18n-5.0.8.tgz",
- "integrity": "sha512-0pfFzegeDWJHJIAmTLRP2DwHjdF5s7jo9tuztdQxAhINCdvS+3nGINqPd00AphqJR/0LhANUS6/+7SCb98YOfA==",
- "engines": {
- "node": ">=10"
- }
- },
- "node_modules/metro/node_modules/yargs": {
- "version": "17.7.2",
- "resolved": "https://registry.npmjs.org/yargs/-/yargs-17.7.2.tgz",
- "integrity": "sha512-7dSzzRQ++CKnNI/krKnYRV7JKKPUXMEh61soaHKg9mrWEhzFWhFnxPxGl+69cD1Ou63C13NUPCnmIcrvqCuM6w==",
- "dependencies": {
- "cliui": "^8.0.1",
- "escalade": "^3.1.1",
- "get-caller-file": "^2.0.5",
- "require-directory": "^2.1.1",
- "string-width": "^4.2.3",
- "y18n": "^5.0.5",
- "yargs-parser": "^21.1.1"
- },
- "engines": {
- "node": ">=12"
- }
- },
- "node_modules/metro/node_modules/yargs-parser": {
- "version": "21.1.1",
- "resolved": "https://registry.npmjs.org/yargs-parser/-/yargs-parser-21.1.1.tgz",
- "integrity": "sha512-tVpsJW7DdjecAiFpbIB1e3qxIQsE6NoPc5/eTdrbbIC4h0LVsWhnoa3g+m2HclBIujHzsxZ4VJVA+GUuc2/LBw==",
- "engines": {
- "node": ">=12"
- }
- },
"node_modules/micromatch": {
"version": "4.0.8",
"resolved": "https://registry.npmjs.org/micromatch/-/micromatch-4.0.8.tgz",
@@ -36386,11 +33959,13 @@
}
},
"node_modules/mini-css-extract-plugin": {
- "version": "2.5.1",
- "resolved": "https://registry.npmjs.org/mini-css-extract-plugin/-/mini-css-extract-plugin-2.5.1.tgz",
- "integrity": "sha512-CRC6E2yedNjfOA3nQjpqAkpnranxhxmilhBPYtldnXcPT/QZb3aJFzvt0pp8W1jhuLR/E0zDa+QEHuC/HhhaLQ==",
+ "version": "2.9.2",
+ "resolved": "https://registry.npmjs.org/mini-css-extract-plugin/-/mini-css-extract-plugin-2.9.2.tgz",
+ "integrity": "sha512-GJuACcS//jtq4kCtd5ii/M0SZf7OZRH+BxdqXZHaJfb8TJiVl+NgQRPwiYt2EuqeSkNydn/7vP+bcE27C5mb9w==",
+ "license": "MIT",
"dependencies": {
- "schema-utils": "^4.0.0"
+ "schema-utils": "^4.0.0",
+ "tapable": "^2.2.1"
},
"engines": {
"node": ">= 12.13.0"
@@ -36423,6 +33998,7 @@
"version": "5.1.0",
"resolved": "https://registry.npmjs.org/ajv-keywords/-/ajv-keywords-5.1.0.tgz",
"integrity": "sha512-YCS/JNFAUyr5vAuhk1DWm1CBxRHW9LbJ2ozWeemrIqpbsqKjHVxYPyi5GC0rjZIT5JxJ3virVTS8wk4i/Z+krw==",
+ "license": "MIT",
"dependencies": {
"fast-deep-equal": "^3.1.3"
},
@@ -36433,12 +34009,14 @@
"node_modules/mini-css-extract-plugin/node_modules/json-schema-traverse": {
"version": "1.0.0",
"resolved": "https://registry.npmjs.org/json-schema-traverse/-/json-schema-traverse-1.0.0.tgz",
- "integrity": "sha512-NM8/P9n3XjXhIZn1lLhkFaACTOURQXjWhV4BA/RnOv8xvgqtqpAX9IO4mRQxSx1Rlo4tqzeqb0sOlruaOy3dug=="
+ "integrity": "sha512-NM8/P9n3XjXhIZn1lLhkFaACTOURQXjWhV4BA/RnOv8xvgqtqpAX9IO4mRQxSx1Rlo4tqzeqb0sOlruaOy3dug==",
+ "license": "MIT"
},
"node_modules/mini-css-extract-plugin/node_modules/schema-utils": {
"version": "4.2.0",
"resolved": "https://registry.npmjs.org/schema-utils/-/schema-utils-4.2.0.tgz",
"integrity": "sha512-L0jRsrPpjdckP3oPug3/VxNKt2trR8TcabrM6FOAAlvC/9Phcmm+cuAgTlxBqdBR1WJx7Naj9WHw+aOmheSVbw==",
+ "license": "MIT",
"dependencies": {
"@types/json-schema": "^7.0.9",
"ajv": "^8.9.0",
@@ -36453,6 +34031,15 @@
"url": "https://opencollective.com/webpack"
}
},
+ "node_modules/mini-css-extract-plugin/node_modules/tapable": {
+ "version": "2.2.1",
+ "resolved": "https://registry.npmjs.org/tapable/-/tapable-2.2.1.tgz",
+ "integrity": "sha512-GNzQvQTOIP6RyTfE2Qxb8ZVlNmw0n88vp1szwWRimP02mnTsx3Wtn5qRdqY9w2XduFNUgvOwhNnQsjwCp+kqaQ==",
+ "license": "MIT",
+ "engines": {
+ "node": ">=6"
+ }
+ },
"node_modules/minimalistic-assert": {
"version": "1.0.1",
"resolved": "https://registry.npmjs.org/minimalistic-assert/-/minimalistic-assert-1.0.1.tgz",
@@ -36517,24 +34104,36 @@
}
},
"node_modules/minipass-collect": {
- "version": "1.0.2",
- "resolved": "https://registry.npmjs.org/minipass-collect/-/minipass-collect-1.0.2.tgz",
- "integrity": "sha512-6T6lH0H8OG9kITm/Jm6tdooIbogG9e0tLgpY6mphXSm/A9u8Nq1ryBG+Qspiub9LjWlBPsPS3tWQ/Botq4FdxA==",
+ "version": "2.0.1",
+ "resolved": "https://registry.npmjs.org/minipass-collect/-/minipass-collect-2.0.1.tgz",
+ "integrity": "sha512-D7V8PO9oaz7PWGLbCACuI1qEOsq7UKfLotx/C0Aet43fCUB/wfQ7DYeq2oR/svFJGYDHPr38SHATeaj/ZoKHKw==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "minipass": "^3.0.0"
+ "minipass": "^7.0.3"
},
"engines": {
- "node": ">= 8"
+ "node": ">=16 || 14 >=14.17"
+ }
+ },
+ "node_modules/minipass-collect/node_modules/minipass": {
+ "version": "7.1.2",
+ "resolved": "https://registry.npmjs.org/minipass/-/minipass-7.1.2.tgz",
+ "integrity": "sha512-qOOzS1cBTWYF4BH8fVePDBOO9iptMnGUEZwNc/cMWnTV2nVLZ7VoNWEPHkYczZA0pdoA7dl6e7FL659nX9S2aw==",
+ "dev": true,
+ "license": "ISC",
+ "engines": {
+ "node": ">=16 || 14 >=14.17"
}
},
"node_modules/minipass-fetch": {
- "version": "3.0.3",
- "resolved": "https://registry.npmjs.org/minipass-fetch/-/minipass-fetch-3.0.3.tgz",
- "integrity": "sha512-n5ITsTkDqYkYJZjcRWzZt9qnZKCT7nKCosJhHoj7S7zD+BP4jVbWs+odsniw5TA3E0sLomhTKOKjF86wf11PuQ==",
+ "version": "3.0.5",
+ "resolved": "https://registry.npmjs.org/minipass-fetch/-/minipass-fetch-3.0.5.tgz",
+ "integrity": "sha512-2N8elDQAtSnFV0Dk7gt15KHsS0Fyz6CbYZ360h0WTYV1Ty46li3rAXVOQj1THMNLdmrD9Vt5pBPtWtVkpwGBqg==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "minipass": "^5.0.0",
+ "minipass": "^7.0.3",
"minipass-sized": "^1.0.3",
"minizlib": "^2.1.2"
},
@@ -36546,12 +34145,13 @@
}
},
"node_modules/minipass-fetch/node_modules/minipass": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/minipass/-/minipass-5.0.0.tgz",
- "integrity": "sha512-3FnjYuehv9k6ovOEbyOswadCDPX1piCfhV8ncmYtHOjuPwylVWsghTLo7rabjC3Rx5xD4HDx8Wm1xnMF7S5qFQ==",
+ "version": "7.1.2",
+ "resolved": "https://registry.npmjs.org/minipass/-/minipass-7.1.2.tgz",
+ "integrity": "sha512-qOOzS1cBTWYF4BH8fVePDBOO9iptMnGUEZwNc/cMWnTV2nVLZ7VoNWEPHkYczZA0pdoA7dl6e7FL659nX9S2aw==",
"dev": true,
+ "license": "ISC",
"engines": {
- "node": ">=8"
+ "node": ">=16 || 14 >=14.17"
}
},
"node_modules/minipass-flush": {
@@ -36559,6 +34159,7 @@
"resolved": "https://registry.npmjs.org/minipass-flush/-/minipass-flush-1.0.5.tgz",
"integrity": "sha512-JmQSYYpPUqX5Jyn1mXaRwOda1uQ8HP5KAT/oDSLCzt1BYRhQU0/hDtsB1ufZfEEzMZ9aAVmsBw8+FWsIXlClWw==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"minipass": "^3.0.0"
},
@@ -36566,21 +34167,12 @@
"node": ">= 8"
}
},
- "node_modules/minipass-json-stream": {
- "version": "1.0.1",
- "resolved": "https://registry.npmjs.org/minipass-json-stream/-/minipass-json-stream-1.0.1.tgz",
- "integrity": "sha512-ODqY18UZt/I8k+b7rl2AENgbWE8IDYam+undIJONvigAz8KR5GWblsFTEfQs0WODsjbSXWlm+JHEv8Gr6Tfdbg==",
- "dev": true,
- "dependencies": {
- "jsonparse": "^1.3.1",
- "minipass": "^3.0.0"
- }
- },
"node_modules/minipass-pipeline": {
"version": "1.2.4",
"resolved": "https://registry.npmjs.org/minipass-pipeline/-/minipass-pipeline-1.2.4.tgz",
"integrity": "sha512-xuIq7cIOt09RPRJ19gdi4b+RiNvDFYe5JH+ggNvBqGqpQXcru3PcRmOZuHBKWK1Txf9+cQ+HMVN4d6z46LZP7A==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"minipass": "^3.0.0"
},
@@ -36593,6 +34185,7 @@
"resolved": "https://registry.npmjs.org/minipass-sized/-/minipass-sized-1.0.3.tgz",
"integrity": "sha512-MbkQQ2CTiBMlA2Dm/5cY+9SWFEN8pzzOXi6rlM5Xxq0Yqbda5ZQy9sU75a673FE9ZK0Zsbr6Y5iP6u9nktfg2g==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"minipass": "^3.0.0"
},
@@ -36600,12 +34193,6 @@
"node": ">=8"
}
},
- "node_modules/minipass/node_modules/yallist": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/yallist/-/yallist-4.0.0.tgz",
- "integrity": "sha512-3wdGidZyq5PB084XLES5TpOSRA3wjXAlIWMhum2kRcv/41Sn2emQ0dycQW4uZXLejwKvg6EsvbdlVL+FYEct7A==",
- "dev": true
- },
"node_modules/minizlib": {
"version": "2.1.2",
"resolved": "https://registry.npmjs.org/minizlib/-/minizlib-2.1.2.tgz",
@@ -36619,12 +34206,6 @@
"node": ">= 8"
}
},
- "node_modules/minizlib/node_modules/yallist": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/yallist/-/yallist-4.0.0.tgz",
- "integrity": "sha512-3wdGidZyq5PB084XLES5TpOSRA3wjXAlIWMhum2kRcv/41Sn2emQ0dycQW4uZXLejwKvg6EsvbdlVL+FYEct7A==",
- "dev": true
- },
"node_modules/mississippi": {
"version": "3.0.0",
"resolved": "https://registry.npmjs.org/mississippi/-/mississippi-3.0.0.tgz",
@@ -36658,9 +34239,10 @@
}
},
"node_modules/mitt": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/mitt/-/mitt-3.0.0.tgz",
- "integrity": "sha512-7dX2/10ITVyqh4aOSVI9gdape+t9l2/8QxHrFmUXu4EEUpdlxl6RudZUPZoc+zuY2hk1j7XxVroIVIan/pD/SQ=="
+ "version": "3.0.1",
+ "resolved": "https://registry.npmjs.org/mitt/-/mitt-3.0.1.tgz",
+ "integrity": "sha512-vKivATfr97l2/QBCYAkXYDbrIWPM2IIKEl7YPhjCvKlG3kE2gm+uBo6nEXK3M5/Ffh/FLpKExzOQ3JJoJGFKBw==",
+ "license": "MIT"
},
"node_modules/mixin-deep": {
"version": "1.3.2",
@@ -36737,7 +34319,8 @@
"node_modules/mkdirp-classic": {
"version": "0.5.3",
"resolved": "https://registry.npmjs.org/mkdirp-classic/-/mkdirp-classic-0.5.3.tgz",
- "integrity": "sha512-gKLcREMhtuZRwRAfqP3RFW+TK4JqApVBtOIftVgjuABpAtpxhPGaDcfvbhNvD0B8iD1oUr/txX35NjcaY6Ns/A=="
+ "integrity": "sha512-gKLcREMhtuZRwRAfqP3RFW+TK4JqApVBtOIftVgjuABpAtpxhPGaDcfvbhNvD0B8iD1oUr/txX35NjcaY6Ns/A==",
+ "dev": true
},
"node_modules/mock-match-media": {
"version": "0.4.2",
@@ -36753,6 +34336,7 @@
"resolved": "https://registry.npmjs.org/modify-values/-/modify-values-1.0.1.tgz",
"integrity": "sha512-xV2bxeN6F7oYjZWTe/YPAy6MN2M+sL4u/Rlm2AHCIVGfo2p1yGmBHQ6vHehl4bRTZBdHu3TSkWdYgkwpYzAGSw==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=0.10.0"
}
@@ -36761,6 +34345,7 @@
"version": "2.29.4",
"resolved": "https://registry.npmjs.org/moment/-/moment-2.29.4.tgz",
"integrity": "sha512-5LC9SOxjSc2HF6vO2CyuTDNivEdoz2IvyJJGj6X8DJ0eFyfszE0QiEd+iXmBvUP3WHxSjFH/vIsA0EN00cgr8w==",
+ "license": "MIT",
"engines": {
"node": "*"
}
@@ -37049,6 +34634,7 @@
"resolved": "https://registry.npmjs.org/multimatch/-/multimatch-5.0.0.tgz",
"integrity": "sha512-ypMKuglUrZUD99Tk2bUQ+xNQj43lPEfAeX2o9cTteAmShXy2VHDJpuwu1o0xqoKCt9jLVAvwyFKdLTPXKAfJyA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"@types/minimatch": "^3.0.3",
"array-differ": "^3.0.0",
@@ -37068,6 +34654,7 @@
"resolved": "https://registry.npmjs.org/array-union/-/array-union-2.1.0.tgz",
"integrity": "sha512-HGyxoOTYUyCM6stUe6EJgnd4EoewAI7zMdfqO+kGjnlZmBDz/cR5pf8r/cR4Wq60sL/p0IkcjUEEPwS3GFrIyw==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=8"
}
@@ -37077,6 +34664,7 @@
"resolved": "https://registry.npmjs.org/arrify/-/arrify-2.0.1.tgz",
"integrity": "sha512-3duEwti880xqi4eAMN8AyR4a0ByT90zoYdLlevfrvU43vb0YZwZVfxOgxWrLXXXpyugL0hNZc9G6BiB5B3nUug==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=8"
}
@@ -37151,12 +34739,6 @@
"rimraf": "bin.js"
}
},
- "node_modules/n12": {
- "version": "0.4.0",
- "resolved": "https://registry.npmjs.org/n12/-/n12-0.4.0.tgz",
- "integrity": "sha512-p/hj4zQ8d3pbbFLQuN1K9honUxiDDhueOWyFLw/XgBv+wZCE44bcLH4CIcsolOceJQduh4Jf7m/LfaTxyGmGtQ==",
- "dev": true
- },
"node_modules/nan": {
"version": "2.18.0",
"resolved": "https://registry.npmjs.org/nan/-/nan-2.18.0.tgz",
@@ -37165,15 +34747,16 @@
"optional": true
},
"node_modules/nanoid": {
- "version": "3.3.7",
- "resolved": "https://registry.npmjs.org/nanoid/-/nanoid-3.3.7.tgz",
- "integrity": "sha512-eSRppjcPIatRIMC1U6UngP8XFcz8MQWGQdt1MTBQ7NaAmvXDfvNxbvWV3x2y6CdEUciCSsDHDQZbhYaB8QEo2g==",
+ "version": "3.3.8",
+ "resolved": "https://registry.npmjs.org/nanoid/-/nanoid-3.3.8.tgz",
+ "integrity": "sha512-WNLf5Sd8oZxOm+TzppcYk8gVOgP+l58xNy58D0nbUnOxOWRWvlcCV4kUF7ltmI6PsrLl/BgKEyS4mqsGChFN0w==",
"funding": [
{
"type": "github",
"url": "https://github.com/sponsors/ai"
}
],
+ "license": "MIT",
"bin": {
"nanoid": "bin/nanoid.cjs"
},
@@ -37208,6 +34791,7 @@
"resolved": "https://registry.npmjs.org/napi-build-utils/-/napi-build-utils-1.0.2.tgz",
"integrity": "sha512-ONmRUqK7zj7DWX0D9ADe03wbwOBZxNAfF20PlGfCWQcD3+/MakShIHrMqx9YwPTfxDdF1zLeL+RGZiR9kGMLdg==",
"dev": true,
+ "license": "MIT",
"optional": true
},
"node_modules/natural-compare": {
@@ -37241,6 +34825,7 @@
"version": "2.0.2",
"resolved": "https://registry.npmjs.org/netmask/-/netmask-2.0.2.tgz",
"integrity": "sha512-dBpDMdxv9Irdq66304OLfEmQ9tbNRFnFTuZiLo+bD+r332bBmMJ8GBLXklIXXgxd3+v9+KUnZaUR5PJMa75Gsg==",
+ "license": "MIT",
"engines": {
"node": ">= 0.4.0"
}
@@ -37284,10 +34869,11 @@
}
},
"node_modules/node-abi": {
- "version": "3.47.0",
- "resolved": "https://registry.npmjs.org/node-abi/-/node-abi-3.47.0.tgz",
- "integrity": "sha512-2s6B2CWZM//kPgwnuI0KrYwNjfdByE25zvAaEpq9IH4zcNsarH8Ihu/UuX6XMPEogDAxkuUFeZn60pXNHAqn3A==",
+ "version": "3.71.0",
+ "resolved": "https://registry.npmjs.org/node-abi/-/node-abi-3.71.0.tgz",
+ "integrity": "sha512-SZ40vRiy/+wRTf21hxkkEjPJZpARzUMVcJoQse2EF8qkUWbbO2z7vd5oA/H6bVH6SZQ5STGcu0KRDS7biNRfxw==",
"dev": true,
+ "license": "MIT",
"optional": true,
"dependencies": {
"semver": "^7.3.5"
@@ -37302,10 +34888,12 @@
"integrity": "sha512-AGK2yQKIjRuqnc6VkX2Xj5d+QW8xZ87pa1UK6yA6ouUyuxfHuMP6umE5QK7UmTeOAymo+Zx1Fxiuw9rVx8taHQ=="
},
"node_modules/node-addon-api": {
- "version": "3.2.1",
- "resolved": "https://registry.npmjs.org/node-addon-api/-/node-addon-api-3.2.1.tgz",
- "integrity": "sha512-mmcei9JghVNDYydghQmeDX8KoAm0FAiYyIcUt/N4nhyAipB17pllZQDOJD2fotxABnt4Mdz+dKTO7eftLg4d0A==",
- "dev": true
+ "version": "6.1.0",
+ "resolved": "https://registry.npmjs.org/node-addon-api/-/node-addon-api-6.1.0.tgz",
+ "integrity": "sha512-+eawOlIgy680F0kBzPUNFhMZGtJ1YmqM6l4+Crf4IkImjYrO/mqPwRMh352g23uIaQKFItcQ64I7KMaJxHgAVA==",
+ "dev": true,
+ "license": "MIT",
+ "optional": true
},
"node_modules/node-dir": {
"version": "0.1.17",
@@ -37333,6 +34921,7 @@
"url": "https://paypal.me/jimmywarting"
}
],
+ "license": "MIT",
"engines": {
"node": ">=10.5.0"
}
@@ -37357,12 +34946,6 @@
}
}
},
- "node_modules/node-fetch-native": {
- "version": "1.6.2",
- "resolved": "https://registry.npmjs.org/node-fetch-native/-/node-fetch-native-1.6.2.tgz",
- "integrity": "sha512-69mtXOFZ6hSkYiXAVB5SqaRvrbITC/NPyqv7yuu/qw0nmgPyYbIMYYNIDhNtwPrzk0ptrimrLz/hhjvm4w5Z+w==",
- "dev": true
- },
"node_modules/node-forge": {
"version": "1.3.1",
"resolved": "https://registry.npmjs.org/node-forge/-/node-forge-1.3.1.tgz",
@@ -37372,74 +34955,111 @@
}
},
"node_modules/node-gyp": {
- "version": "9.4.0",
- "resolved": "https://registry.npmjs.org/node-gyp/-/node-gyp-9.4.0.tgz",
- "integrity": "sha512-dMXsYP6gc9rRbejLXmTbVRYjAHw7ppswsKyMxuxJxxOHzluIO1rGp9TOQgjFJ+2MCqcOcQTOPB/8Xwhr+7s4Eg==",
+ "version": "10.3.1",
+ "resolved": "https://registry.npmjs.org/node-gyp/-/node-gyp-10.3.1.tgz",
+ "integrity": "sha512-Pp3nFHBThHzVtNY7U6JfPjvT/DTE8+o/4xKsLQtBoU+j2HLsGlhcfzflAoUreaJbNmYnX+LlLi0qjV8kpyO6xQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"env-paths": "^2.2.0",
"exponential-backoff": "^3.1.1",
- "glob": "^7.1.4",
+ "glob": "^10.3.10",
"graceful-fs": "^4.2.6",
- "make-fetch-happen": "^11.0.3",
- "nopt": "^6.0.0",
- "npmlog": "^6.0.0",
- "rimraf": "^3.0.2",
+ "make-fetch-happen": "^13.0.0",
+ "nopt": "^7.0.0",
+ "proc-log": "^4.1.0",
"semver": "^7.3.5",
- "tar": "^6.1.2",
- "which": "^2.0.2"
+ "tar": "^6.2.1",
+ "which": "^4.0.0"
},
"bin": {
"node-gyp": "bin/node-gyp.js"
},
"engines": {
- "node": "^12.13 || ^14.13 || >=16"
+ "node": "^16.14.0 || >=18.0.0"
}
},
- "node_modules/node-gyp-build": {
- "version": "4.6.0",
- "resolved": "https://registry.npmjs.org/node-gyp-build/-/node-gyp-build-4.6.0.tgz",
- "integrity": "sha512-NTZVKn9IylLwUzaKjkas1e4u2DLNcV4rdYagA4PWdPwW87Bi7z+BznyKSRwS/761tV/lzCGXplWsiaMjLqP2zQ==",
+ "node_modules/node-gyp/node_modules/brace-expansion": {
+ "version": "2.0.1",
+ "resolved": "https://registry.npmjs.org/brace-expansion/-/brace-expansion-2.0.1.tgz",
+ "integrity": "sha512-XnAIvQ8eM+kC6aULx6wuQiwVsnzsi9d3WxzV3FpWTGA19F621kwdbsAcFKXgKUHZWsy+mY6iL1sHTxWEFCytDA==",
"dev": true,
- "bin": {
- "node-gyp-build": "bin.js",
- "node-gyp-build-optional": "optional.js",
- "node-gyp-build-test": "build-test.js"
+ "license": "MIT",
+ "dependencies": {
+ "balanced-match": "^1.0.0"
}
},
"node_modules/node-gyp/node_modules/glob": {
- "version": "7.2.3",
- "resolved": "https://registry.npmjs.org/glob/-/glob-7.2.3.tgz",
- "integrity": "sha512-nFR0zLpU2YCaRxwoCJvL6UvCH2JFyFVIvwTLsIf21AuHlMskA1hhTdk+LlYJtOlYt9v6dvszD2BGRqBL+iQK9Q==",
+ "version": "10.4.5",
+ "resolved": "https://registry.npmjs.org/glob/-/glob-10.4.5.tgz",
+ "integrity": "sha512-7Bv8RF0k6xjo7d4A/PxYLbUCfb6c+Vpd2/mB2yRDlew7Jb5hEXiCD9ibfO7wpk8i4sevK6DFny9h7EYbM3/sHg==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "fs.realpath": "^1.0.0",
- "inflight": "^1.0.4",
- "inherits": "2",
- "minimatch": "^3.1.1",
- "once": "^1.3.0",
- "path-is-absolute": "^1.0.0"
+ "foreground-child": "^3.1.0",
+ "jackspeak": "^3.1.2",
+ "minimatch": "^9.0.4",
+ "minipass": "^7.1.2",
+ "package-json-from-dist": "^1.0.0",
+ "path-scurry": "^1.11.1"
},
+ "bin": {
+ "glob": "dist/esm/bin.mjs"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
+ }
+ },
+ "node_modules/node-gyp/node_modules/isexe": {
+ "version": "3.1.1",
+ "resolved": "https://registry.npmjs.org/isexe/-/isexe-3.1.1.tgz",
+ "integrity": "sha512-LpB/54B+/2J5hqQ7imZHfdU31OlgQqx7ZicVlkm9kzg9/w8GKLEcFfJl/t7DCEDueOyBAD6zCCwTO6Fzs0NoEQ==",
+ "dev": true,
+ "license": "ISC",
"engines": {
- "node": "*"
+ "node": ">=16"
+ }
+ },
+ "node_modules/node-gyp/node_modules/minimatch": {
+ "version": "9.0.5",
+ "resolved": "https://registry.npmjs.org/minimatch/-/minimatch-9.0.5.tgz",
+ "integrity": "sha512-G6T0ZX48xgozx7587koeX9Ys2NYy6Gmv//P89sEte9V9whIapMNF4idKxnW2QtCcLiTWlb/wfCabAtAFWhhBow==",
+ "dev": true,
+ "license": "ISC",
+ "dependencies": {
+ "brace-expansion": "^2.0.1"
+ },
+ "engines": {
+ "node": ">=16 || 14 >=14.17"
},
"funding": {
"url": "https://github.com/sponsors/isaacs"
}
},
+ "node_modules/node-gyp/node_modules/minipass": {
+ "version": "7.1.2",
+ "resolved": "https://registry.npmjs.org/minipass/-/minipass-7.1.2.tgz",
+ "integrity": "sha512-qOOzS1cBTWYF4BH8fVePDBOO9iptMnGUEZwNc/cMWnTV2nVLZ7VoNWEPHkYczZA0pdoA7dl6e7FL659nX9S2aw==",
+ "dev": true,
+ "license": "ISC",
+ "engines": {
+ "node": ">=16 || 14 >=14.17"
+ }
+ },
"node_modules/node-gyp/node_modules/which": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
- "integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
+ "version": "4.0.0",
+ "resolved": "https://registry.npmjs.org/which/-/which-4.0.0.tgz",
+ "integrity": "sha512-GlaYyEb07DPxYCKhKzplCWBJtvxZcZMrL+4UkrTSJHHPyZU4mYYTv3qaOe77H7EODLSSopAUFAc6W8U4yqvscg==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "isexe": "^2.0.0"
+ "isexe": "^3.1.1"
},
"bin": {
- "node-which": "bin/node-which"
+ "node-which": "bin/which.js"
},
"engines": {
- "node": ">= 8"
+ "node": "^16.13.0 || >=18.0.0"
}
},
"node_modules/node-int64": {
@@ -37488,7 +35108,8 @@
"version": "1.1.12",
"resolved": "https://registry.npmjs.org/node-machine-id/-/node-machine-id-1.1.12.tgz",
"integrity": "sha512-QNABxbrPa3qEIfrE6GOJ7BYIuignnJw7iQ2YPbc3Nla1HzRJjXzZOiikfF8m7eAMfichLt3M4VgLOetqgDmgGQ==",
- "dev": true
+ "dev": true,
+ "license": "MIT"
},
"node_modules/node-releases": {
"version": "2.0.18",
@@ -37517,18 +35138,19 @@
}
},
"node_modules/nopt": {
- "version": "6.0.0",
- "resolved": "https://registry.npmjs.org/nopt/-/nopt-6.0.0.tgz",
- "integrity": "sha512-ZwLpbTgdhuZUnZzjd7nb1ZV+4DoiC6/sfiVKok72ym/4Tlf+DFdlHYmT2JPmcNNWV6Pi3SDf1kT+A4r9RTuT9g==",
+ "version": "7.2.1",
+ "resolved": "https://registry.npmjs.org/nopt/-/nopt-7.2.1.tgz",
+ "integrity": "sha512-taM24ViiimT/XntxbPyJQzCG+p4EKOpgD3mxFwW38mGjVUrfERQOeY4EDHjdnptttfHuHQXFx+lTP08Q+mLa/w==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "abbrev": "^1.0.0"
+ "abbrev": "^2.0.0"
},
"bin": {
"nopt": "bin/nopt.js"
},
"engines": {
- "node": "^12.13.0 || ^14.15.0 || >=16.0.0"
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
"node_modules/normalize-package-data": {
@@ -37596,10 +35218,11 @@
}
},
"node_modules/npm-install-checks": {
- "version": "6.1.1",
- "resolved": "https://registry.npmjs.org/npm-install-checks/-/npm-install-checks-6.1.1.tgz",
- "integrity": "sha512-dH3GmQL4vsPtld59cOn8uY0iOqRmqKvV+DLGwNXV/Q7MDgD2QfOADWd/mFXcIE5LVhYYGjA3baz6W9JneqnuCw==",
+ "version": "6.3.0",
+ "resolved": "https://registry.npmjs.org/npm-install-checks/-/npm-install-checks-6.3.0.tgz",
+ "integrity": "sha512-W29RiK/xtpCGqn6f3ixfRYGk+zRyr+Ew9F2E20BfXxT5/euLdA/Nm7fO7OeTGuAmTs30cpgInyJ0cYe708YTZw==",
"dev": true,
+ "license": "BSD-2-Clause",
"dependencies": {
"semver": "^7.1.1"
},
@@ -37877,39 +35500,32 @@
}
},
"node_modules/npm-pick-manifest": {
- "version": "8.0.2",
- "resolved": "https://registry.npmjs.org/npm-pick-manifest/-/npm-pick-manifest-8.0.2.tgz",
- "integrity": "sha512-1dKY+86/AIiq1tkKVD3l0WI+Gd3vkknVGAggsFeBkTvbhMQ1OND/LKkYv4JtXPKUJ8bOTCyLiqEg2P6QNdK+Gg==",
+ "version": "9.1.0",
+ "resolved": "https://registry.npmjs.org/npm-pick-manifest/-/npm-pick-manifest-9.1.0.tgz",
+ "integrity": "sha512-nkc+3pIIhqHVQr085X9d2JzPzLyjzQS96zbruppqC9aZRm/x8xx6xhI98gHtsfELP2bE+loHq8ZaHFHhe+NauA==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"npm-install-checks": "^6.0.0",
"npm-normalize-package-bin": "^3.0.0",
- "npm-package-arg": "^10.0.0",
+ "npm-package-arg": "^11.0.0",
"semver": "^7.3.5"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/npm-pick-manifest/node_modules/hosted-git-info": {
- "version": "6.1.1",
- "resolved": "https://registry.npmjs.org/hosted-git-info/-/hosted-git-info-6.1.1.tgz",
- "integrity": "sha512-r0EI+HBMcXadMrugk0GCQ+6BQV39PiWAZVfq7oIckeGiN7sjRGyQxPdft3nQekFTCQbYxLBH+/axZMeH8UX6+w==",
+ "version": "7.0.2",
+ "resolved": "https://registry.npmjs.org/hosted-git-info/-/hosted-git-info-7.0.2.tgz",
+ "integrity": "sha512-puUZAUKT5m8Zzvs72XWy3HtvVbTWljRE66cP60bxJzAqf2DgICo7lYTY2IHUmLnNpjYvw5bvmoHvPc0QO2a62w==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "lru-cache": "^7.5.1"
+ "lru-cache": "^10.0.1"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
- }
- },
- "node_modules/npm-pick-manifest/node_modules/lru-cache": {
- "version": "7.18.3",
- "resolved": "https://registry.npmjs.org/lru-cache/-/lru-cache-7.18.3.tgz",
- "integrity": "sha512-jumlc0BIUrS3qJGgIkWZsyfAM7NCWiBcCDhnd+3NNM5KbBmLTgHVfWBcg6W+rLUsIpzpERPsvwUP7CckAQSOoA==",
- "dev": true,
- "engines": {
- "node": ">=12"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/npm-pick-manifest/node_modules/npm-normalize-package-bin": {
@@ -37917,23 +35533,25 @@
"resolved": "https://registry.npmjs.org/npm-normalize-package-bin/-/npm-normalize-package-bin-3.0.1.tgz",
"integrity": "sha512-dMxCf+zZ+3zeQZXKxmyuCKlIDPGuv8EF940xbkC4kQVDTtqoh6rJFO+JTKSA6/Rwi0getWmtuy4Itup0AMcaDQ==",
"dev": true,
+ "license": "ISC",
"engines": {
"node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
"node_modules/npm-pick-manifest/node_modules/npm-package-arg": {
- "version": "10.1.0",
- "resolved": "https://registry.npmjs.org/npm-package-arg/-/npm-package-arg-10.1.0.tgz",
- "integrity": "sha512-uFyyCEmgBfZTtrKk/5xDfHp6+MdrqGotX/VoOyEEl3mBwiEE5FlBaePanazJSVMPT7vKepcjYBY2ztg9A3yPIA==",
+ "version": "11.0.3",
+ "resolved": "https://registry.npmjs.org/npm-package-arg/-/npm-package-arg-11.0.3.tgz",
+ "integrity": "sha512-sHGJy8sOC1YraBywpzQlIKBE4pBbGbiF95U6Auspzyem956E0+FtDtsx1ZxlOJkQCZ1AFXAY/yuvtFYrOxF+Bw==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "hosted-git-info": "^6.0.0",
- "proc-log": "^3.0.0",
+ "hosted-git-info": "^7.0.0",
+ "proc-log": "^4.0.0",
"semver": "^7.3.5",
"validate-npm-package-name": "^5.0.0"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/npm-pick-manifest/node_modules/validate-npm-package-name": {
@@ -37941,71 +35559,68 @@
"resolved": "https://registry.npmjs.org/validate-npm-package-name/-/validate-npm-package-name-5.0.1.tgz",
"integrity": "sha512-OljLrQ9SQdOUqTaQxqL5dEfZWrXExyyWsozYlAWFawPVNuD83igl7uJD2RTkNMbniIYgt8l81eCJGIdQF7avLQ==",
"dev": true,
+ "license": "ISC",
"engines": {
"node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
"node_modules/npm-registry-fetch": {
- "version": "14.0.5",
- "resolved": "https://registry.npmjs.org/npm-registry-fetch/-/npm-registry-fetch-14.0.5.tgz",
- "integrity": "sha512-kIDMIo4aBm6xg7jOttupWZamsZRkAqMqwqqbVXnUqstY5+tapvv6bkH/qMR76jdgV+YljEUCyWx3hRYMrJiAgA==",
+ "version": "17.1.0",
+ "resolved": "https://registry.npmjs.org/npm-registry-fetch/-/npm-registry-fetch-17.1.0.tgz",
+ "integrity": "sha512-5+bKQRH0J1xG1uZ1zMNvxW0VEyoNWgJpY9UDuluPFLKDfJ9u2JmmjmTJV1srBGQOROfdBMiVvnH2Zvpbm+xkVA==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "make-fetch-happen": "^11.0.0",
- "minipass": "^5.0.0",
+ "@npmcli/redact": "^2.0.0",
+ "jsonparse": "^1.3.1",
+ "make-fetch-happen": "^13.0.0",
+ "minipass": "^7.0.2",
"minipass-fetch": "^3.0.0",
- "minipass-json-stream": "^1.0.1",
"minizlib": "^2.1.2",
- "npm-package-arg": "^10.0.0",
- "proc-log": "^3.0.0"
+ "npm-package-arg": "^11.0.0",
+ "proc-log": "^4.0.0"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/npm-registry-fetch/node_modules/hosted-git-info": {
- "version": "6.1.1",
- "resolved": "https://registry.npmjs.org/hosted-git-info/-/hosted-git-info-6.1.1.tgz",
- "integrity": "sha512-r0EI+HBMcXadMrugk0GCQ+6BQV39PiWAZVfq7oIckeGiN7sjRGyQxPdft3nQekFTCQbYxLBH+/axZMeH8UX6+w==",
+ "version": "7.0.2",
+ "resolved": "https://registry.npmjs.org/hosted-git-info/-/hosted-git-info-7.0.2.tgz",
+ "integrity": "sha512-puUZAUKT5m8Zzvs72XWy3HtvVbTWljRE66cP60bxJzAqf2DgICo7lYTY2IHUmLnNpjYvw5bvmoHvPc0QO2a62w==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "lru-cache": "^7.5.1"
+ "lru-cache": "^10.0.1"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
- }
- },
- "node_modules/npm-registry-fetch/node_modules/lru-cache": {
- "version": "7.18.3",
- "resolved": "https://registry.npmjs.org/lru-cache/-/lru-cache-7.18.3.tgz",
- "integrity": "sha512-jumlc0BIUrS3qJGgIkWZsyfAM7NCWiBcCDhnd+3NNM5KbBmLTgHVfWBcg6W+rLUsIpzpERPsvwUP7CckAQSOoA==",
- "dev": true,
- "engines": {
- "node": ">=12"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/npm-registry-fetch/node_modules/minipass": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/minipass/-/minipass-5.0.0.tgz",
- "integrity": "sha512-3FnjYuehv9k6ovOEbyOswadCDPX1piCfhV8ncmYtHOjuPwylVWsghTLo7rabjC3Rx5xD4HDx8Wm1xnMF7S5qFQ==",
+ "version": "7.1.2",
+ "resolved": "https://registry.npmjs.org/minipass/-/minipass-7.1.2.tgz",
+ "integrity": "sha512-qOOzS1cBTWYF4BH8fVePDBOO9iptMnGUEZwNc/cMWnTV2nVLZ7VoNWEPHkYczZA0pdoA7dl6e7FL659nX9S2aw==",
"dev": true,
+ "license": "ISC",
"engines": {
- "node": ">=8"
+ "node": ">=16 || 14 >=14.17"
}
},
"node_modules/npm-registry-fetch/node_modules/npm-package-arg": {
- "version": "10.1.0",
- "resolved": "https://registry.npmjs.org/npm-package-arg/-/npm-package-arg-10.1.0.tgz",
- "integrity": "sha512-uFyyCEmgBfZTtrKk/5xDfHp6+MdrqGotX/VoOyEEl3mBwiEE5FlBaePanazJSVMPT7vKepcjYBY2ztg9A3yPIA==",
+ "version": "11.0.3",
+ "resolved": "https://registry.npmjs.org/npm-package-arg/-/npm-package-arg-11.0.3.tgz",
+ "integrity": "sha512-sHGJy8sOC1YraBywpzQlIKBE4pBbGbiF95U6Auspzyem956E0+FtDtsx1ZxlOJkQCZ1AFXAY/yuvtFYrOxF+Bw==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "hosted-git-info": "^6.0.0",
- "proc-log": "^3.0.0",
+ "hosted-git-info": "^7.0.0",
+ "proc-log": "^4.0.0",
"semver": "^7.3.5",
"validate-npm-package-name": "^5.0.0"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/npm-registry-fetch/node_modules/validate-npm-package-name": {
@@ -38013,6 +35628,7 @@
"resolved": "https://registry.npmjs.org/validate-npm-package-name/-/validate-npm-package-name-5.0.1.tgz",
"integrity": "sha512-OljLrQ9SQdOUqTaQxqL5dEfZWrXExyyWsozYlAWFawPVNuD83igl7uJD2RTkNMbniIYgt8l81eCJGIdQF7avLQ==",
"dev": true,
+ "license": "ISC",
"engines": {
"node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
@@ -38069,10 +35685,11 @@
}
},
"node_modules/npm-run-all/node_modules/cross-spawn": {
- "version": "6.0.5",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-6.0.5.tgz",
- "integrity": "sha512-eTVLrBSt7fjbDygz805pMnstIs2VTBNkRm0qxZd+M7A5XDdxVRWO5MxGBXZhjY4cqLYLdtrGqRf8mBPmzwSpWQ==",
+ "version": "6.0.6",
+ "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-6.0.6.tgz",
+ "integrity": "sha512-VqCUuhcd1iB+dsv8gxPttb5iZh/D0iubSP21g36KXdEuf6I5JiioesUVjpCdHV9MZRUfVFlvwtIUyPfxo5trtw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"nice-try": "^1.0.4",
"path-key": "^2.0.1",
@@ -38165,21 +35782,6 @@
"node": ">=4"
}
},
- "node_modules/npmlog": {
- "version": "6.0.2",
- "resolved": "https://registry.npmjs.org/npmlog/-/npmlog-6.0.2.tgz",
- "integrity": "sha512-/vBvz5Jfr9dT/aFWd0FIRf+T/Q2WBsLENygUaFUqstqsycmZAP/t5BvFJTK0viFmSUxiUKTUplWy5vt+rvKIxg==",
- "dev": true,
- "dependencies": {
- "are-we-there-yet": "^3.0.0",
- "console-control-strings": "^1.1.0",
- "gauge": "^4.0.3",
- "set-blocking": "^2.0.0"
- },
- "engines": {
- "node": "^12.13.0 || ^14.15.0 || >=16.0.0"
- }
- },
"node_modules/nth-check": {
"version": "2.1.1",
"resolved": "https://registry.npmjs.org/nth-check/-/nth-check-2.1.1.tgz",
@@ -38211,66 +35813,66 @@
"integrity": "sha512-cTGB9ptp9dY9A5VbMSe7fQBcl/tt22Vcqdq8+eN93rblOuE0aCFu4aZ2vMwct/2t+lFnosm8RkQW1I0Omb1UtQ=="
},
"node_modules/nx": {
- "version": "16.6.0",
- "resolved": "https://registry.npmjs.org/nx/-/nx-16.6.0.tgz",
- "integrity": "sha512-4UaS9nRakpZs45VOossA7hzSQY2dsr035EoPRGOc81yoMFW6Sqn1Rgq4hiLbHZOY8MnWNsLMkgolNMz1jC8YUQ==",
+ "version": "20.2.1",
+ "resolved": "https://registry.npmjs.org/nx/-/nx-20.2.1.tgz",
+ "integrity": "sha512-zUw1DT9BW2ajbDZgcUFKfysGqrbJwsMRjPxT6GthuqcLtDc2iJi3+/UBTLsITSeiw4Za4qPVxjaK4+yma9Ju5w==",
"dev": true,
"hasInstallScript": true,
+ "license": "MIT",
"dependencies": {
- "@nrwl/tao": "16.6.0",
- "@parcel/watcher": "2.0.4",
+ "@napi-rs/wasm-runtime": "0.2.4",
"@yarnpkg/lockfile": "^1.1.0",
- "@yarnpkg/parsers": "3.0.0-rc.46",
- "@zkochan/js-yaml": "0.0.6",
- "axios": "^1.0.0",
+ "@yarnpkg/parsers": "3.0.2",
+ "@zkochan/js-yaml": "0.0.7",
+ "axios": "^1.7.4",
"chalk": "^4.1.0",
"cli-cursor": "3.1.0",
"cli-spinners": "2.6.1",
- "cliui": "^7.0.2",
- "dotenv": "~10.0.0",
+ "cliui": "^8.0.1",
+ "dotenv": "~16.4.5",
+ "dotenv-expand": "~11.0.6",
"enquirer": "~2.3.6",
- "fast-glob": "3.2.7",
"figures": "3.2.0",
"flat": "^5.0.2",
- "fs-extra": "^11.1.0",
- "glob": "7.1.4",
+ "front-matter": "^4.0.2",
"ignore": "^5.0.4",
- "js-yaml": "4.1.0",
+ "jest-diff": "^29.4.1",
"jsonc-parser": "3.2.0",
- "lines-and-columns": "~2.0.3",
- "minimatch": "3.0.5",
+ "lines-and-columns": "2.0.3",
+ "minimatch": "9.0.3",
"node-machine-id": "1.1.12",
"npm-run-path": "^4.0.1",
"open": "^8.4.0",
- "semver": "7.5.3",
+ "ora": "5.3.0",
+ "semver": "^7.5.3",
"string-width": "^4.2.3",
- "strong-log-transformer": "^2.1.0",
"tar-stream": "~2.2.0",
"tmp": "~0.2.1",
"tsconfig-paths": "^4.1.2",
"tslib": "^2.3.0",
- "v8-compile-cache": "2.3.0",
+ "yaml": "^2.6.0",
"yargs": "^17.6.2",
"yargs-parser": "21.1.1"
},
"bin": {
- "nx": "bin/nx.js"
+ "nx": "bin/nx.js",
+ "nx-cloud": "bin/nx-cloud.js"
},
"optionalDependencies": {
- "@nx/nx-darwin-arm64": "16.6.0",
- "@nx/nx-darwin-x64": "16.6.0",
- "@nx/nx-freebsd-x64": "16.6.0",
- "@nx/nx-linux-arm-gnueabihf": "16.6.0",
- "@nx/nx-linux-arm64-gnu": "16.6.0",
- "@nx/nx-linux-arm64-musl": "16.6.0",
- "@nx/nx-linux-x64-gnu": "16.6.0",
- "@nx/nx-linux-x64-musl": "16.6.0",
- "@nx/nx-win32-arm64-msvc": "16.6.0",
- "@nx/nx-win32-x64-msvc": "16.6.0"
+ "@nx/nx-darwin-arm64": "20.2.1",
+ "@nx/nx-darwin-x64": "20.2.1",
+ "@nx/nx-freebsd-x64": "20.2.1",
+ "@nx/nx-linux-arm-gnueabihf": "20.2.1",
+ "@nx/nx-linux-arm64-gnu": "20.2.1",
+ "@nx/nx-linux-arm64-musl": "20.2.1",
+ "@nx/nx-linux-x64-gnu": "20.2.1",
+ "@nx/nx-linux-x64-musl": "20.2.1",
+ "@nx/nx-win32-arm64-msvc": "20.2.1",
+ "@nx/nx-win32-x64-msvc": "20.2.1"
},
"peerDependencies": {
- "@swc-node/register": "^1.4.2",
- "@swc/core": "^1.2.173"
+ "@swc-node/register": "^1.8.0",
+ "@swc/core": "^1.3.85"
},
"peerDependenciesMeta": {
"@swc-node/register": {
@@ -38281,17 +35883,34 @@
}
}
},
- "node_modules/nx/node_modules/argparse": {
+ "node_modules/nx/node_modules/axios": {
+ "version": "1.7.9",
+ "resolved": "https://registry.npmjs.org/axios/-/axios-1.7.9.tgz",
+ "integrity": "sha512-LhLcE7Hbiryz8oMDdDptSrWowmB4Bl6RCt6sIJKpRB4XtVf0iEgewX3au/pJqm+Py1kCASkb/FFKjxQaLtxJvw==",
+ "dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "follow-redirects": "^1.15.6",
+ "form-data": "^4.0.0",
+ "proxy-from-env": "^1.1.0"
+ }
+ },
+ "node_modules/nx/node_modules/brace-expansion": {
"version": "2.0.1",
- "resolved": "https://registry.npmjs.org/argparse/-/argparse-2.0.1.tgz",
- "integrity": "sha512-8+9WqebbFzpX9OR+Wa6O29asIogeRMzcGtAINdpMHHyAg10f05aSFVBbcEqGf/PXw1EjAZ+q2/bEBg3DvurK3Q==",
- "dev": true
+ "resolved": "https://registry.npmjs.org/brace-expansion/-/brace-expansion-2.0.1.tgz",
+ "integrity": "sha512-XnAIvQ8eM+kC6aULx6wuQiwVsnzsi9d3WxzV3FpWTGA19F621kwdbsAcFKXgKUHZWsy+mY6iL1sHTxWEFCytDA==",
+ "dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "balanced-match": "^1.0.0"
+ }
},
"node_modules/nx/node_modules/cli-cursor": {
"version": "3.1.0",
"resolved": "https://registry.npmjs.org/cli-cursor/-/cli-cursor-3.1.0.tgz",
"integrity": "sha512-I/zHAwsKf9FqGoXM4WWRACob9+SNukZTd94DWF57E4toouRulbCxcUh6RKUEOQlYTHJnzkPMySvPNaaSLNfLZw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"restore-cursor": "^3.1.0"
},
@@ -38300,36 +35919,49 @@
}
},
"node_modules/nx/node_modules/cliui": {
- "version": "7.0.4",
- "resolved": "https://registry.npmjs.org/cliui/-/cliui-7.0.4.tgz",
- "integrity": "sha512-OcRE68cOsVMXp1Yvonl/fzkQOyjLSu/8bhPDfQt0e0/Eb283TKP20Fs2MqoPsr9SwA595rRCA+QMzYc9nBP+JQ==",
+ "version": "8.0.1",
+ "resolved": "https://registry.npmjs.org/cliui/-/cliui-8.0.1.tgz",
+ "integrity": "sha512-BSeNnyus75C4//NQ9gQt1/csTXyo/8Sb+afLAkzAptFuMsod9HFokGNudZpi/oQV73hnVK+sR+5PVRMd+Dr7YQ==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"string-width": "^4.2.0",
- "strip-ansi": "^6.0.0",
+ "strip-ansi": "^6.0.1",
"wrap-ansi": "^7.0.0"
+ },
+ "engines": {
+ "node": ">=12"
}
},
- "node_modules/nx/node_modules/dotenv": {
- "version": "10.0.0",
- "resolved": "https://registry.npmjs.org/dotenv/-/dotenv-10.0.0.tgz",
- "integrity": "sha512-rlBi9d8jpv9Sf1klPjNfFAuWDjKLwTIJJ/VxtoTwIR6hnZxcEOQCZg2oIL3MWBYw5GpUDKOEnND7LXTbIpQ03Q==",
+ "node_modules/nx/node_modules/dotenv-expand": {
+ "version": "11.0.7",
+ "resolved": "https://registry.npmjs.org/dotenv-expand/-/dotenv-expand-11.0.7.tgz",
+ "integrity": "sha512-zIHwmZPRshsCdpMDyVsqGmgyP0yT8GAgXUnkdAoJisxvf33k7yO6OuoKmcTGuXPWSsm8Oh88nZicRLA9Y0rUeA==",
"dev": true,
+ "license": "BSD-2-Clause",
+ "dependencies": {
+ "dotenv": "^16.4.5"
+ },
"engines": {
- "node": ">=10"
+ "node": ">=12"
+ },
+ "funding": {
+ "url": "https://dotenvx.com"
}
},
"node_modules/nx/node_modules/emoji-regex": {
"version": "8.0.0",
"resolved": "https://registry.npmjs.org/emoji-regex/-/emoji-regex-8.0.0.tgz",
"integrity": "sha512-MSjYzcWNOA0ewAHpz0MxpYFvwg6yjy1NG3xteoqz644VCo/RPgnr1/GGt+ic3iJTzQ8Eu3TdM14SawnVUmGE6A==",
- "dev": true
+ "dev": true,
+ "license": "MIT"
},
"node_modules/nx/node_modules/figures": {
"version": "3.2.0",
"resolved": "https://registry.npmjs.org/figures/-/figures-3.2.0.tgz",
"integrity": "sha512-yaduQFRKLXYOGgEn6AZau90j3ggSOyiqXU0F9JZfeXYhNa+Jk4X+s45A2zg5jns87GAFa34BBm2kXw4XpNcbdg==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"escape-string-regexp": "^1.0.5"
},
@@ -38340,42 +35972,12 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/nx/node_modules/fs-extra": {
- "version": "11.2.0",
- "resolved": "https://registry.npmjs.org/fs-extra/-/fs-extra-11.2.0.tgz",
- "integrity": "sha512-PmDi3uwK5nFuXh7XDTlVnS17xJS7vW36is2+w3xcv8SVxiB4NyATf4ctkVY5bkSjX0Y4nbvZCq1/EjtEyr9ktw==",
- "dev": true,
- "dependencies": {
- "graceful-fs": "^4.2.0",
- "jsonfile": "^6.0.1",
- "universalify": "^2.0.0"
- },
- "engines": {
- "node": ">=14.14"
- }
- },
- "node_modules/nx/node_modules/glob": {
- "version": "7.1.4",
- "resolved": "https://registry.npmjs.org/glob/-/glob-7.1.4.tgz",
- "integrity": "sha512-hkLPepehmnKk41pUGm3sYxoFs/umurYfYJCerbXEyFIWcAzvpipAgVkBqqT9RBKMGjnq6kMuyYwha6csxbiM1A==",
- "dev": true,
- "dependencies": {
- "fs.realpath": "^1.0.0",
- "inflight": "^1.0.4",
- "inherits": "2",
- "minimatch": "^3.0.4",
- "once": "^1.3.0",
- "path-is-absolute": "^1.0.0"
- },
- "engines": {
- "node": "*"
- }
- },
"node_modules/nx/node_modules/is-fullwidth-code-point": {
"version": "3.0.0",
"resolved": "https://registry.npmjs.org/is-fullwidth-code-point/-/is-fullwidth-code-point-3.0.0.tgz",
"integrity": "sha512-zymm5+u+sCsSWyD9qNaejV3DFvhCKclKdizYaJUuHA83RLjb7nSuGnddCHGv0hk+KY7BMAlsWeK4Ueg6EV6XQg==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=8"
}
@@ -38385,6 +35987,7 @@
"resolved": "https://registry.npmjs.org/is-wsl/-/is-wsl-2.2.0.tgz",
"integrity": "sha512-fKzAra0rGJUUBwGBgNkHZuToZcn+TtXHpeCgmkMJMMYx1sQDYaCSyjJBSCa2nH1DGm7s3n1oBnohoVTBaN7Lww==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"is-docker": "^2.0.0"
},
@@ -38392,76 +35995,47 @@
"node": ">=8"
}
},
- "node_modules/nx/node_modules/js-yaml": {
- "version": "4.1.0",
- "resolved": "https://registry.npmjs.org/js-yaml/-/js-yaml-4.1.0.tgz",
- "integrity": "sha512-wpxZs9NoxZaJESJGIZTyDEaYpl0FKSA+FB9aJiyemKhMwkxQg63h4T1KJgUGHpTqPDNRcmmYLugrRjJlBtWvRA==",
- "dev": true,
- "dependencies": {
- "argparse": "^2.0.1"
- },
- "bin": {
- "js-yaml": "bin/js-yaml.js"
- }
- },
"node_modules/nx/node_modules/jsonc-parser": {
"version": "3.2.0",
"resolved": "https://registry.npmjs.org/jsonc-parser/-/jsonc-parser-3.2.0.tgz",
"integrity": "sha512-gfFQZrcTc8CnKXp6Y4/CBT3fTc0OVuDofpre4aEeEpSBPV5X5v4+Vmx+8snU7RLPrNHPKSgLxGo9YuQzz20o+w==",
- "dev": true
- },
- "node_modules/nx/node_modules/jsonfile": {
- "version": "6.1.0",
- "resolved": "https://registry.npmjs.org/jsonfile/-/jsonfile-6.1.0.tgz",
- "integrity": "sha512-5dgndWOriYSm5cnYaJNhalLNDKOqFwyDB/rr1E9ZsGciGvKPs8R2xYGCacuf3z6K1YKDz182fd+fY3cn3pMqXQ==",
"dev": true,
- "dependencies": {
- "universalify": "^2.0.0"
- },
- "optionalDependencies": {
- "graceful-fs": "^4.1.6"
- }
+ "license": "MIT"
},
"node_modules/nx/node_modules/lines-and-columns": {
- "version": "2.0.4",
- "resolved": "https://registry.npmjs.org/lines-and-columns/-/lines-and-columns-2.0.4.tgz",
- "integrity": "sha512-wM1+Z03eypVAVUCE7QdSqpVIvelbOakn1M0bPDoA4SGWPx3sNDVUiMo3L6To6WWGClB7VyXnhQ4Sn7gxiJbE6A==",
+ "version": "2.0.3",
+ "resolved": "https://registry.npmjs.org/lines-and-columns/-/lines-and-columns-2.0.3.tgz",
+ "integrity": "sha512-cNOjgCnLB+FnvWWtyRTzmB3POJ+cXxTA81LoW7u8JdmhfXzriropYwpjShnz1QLLWsQwY7nIxoDmcPTwphDK9w==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": "^12.20.0 || ^14.13.1 || >=16.0.0"
}
},
- "node_modules/nx/node_modules/lru-cache": {
- "version": "6.0.0",
- "resolved": "https://registry.npmjs.org/lru-cache/-/lru-cache-6.0.0.tgz",
- "integrity": "sha512-Jo6dJ04CmSjuznwJSS3pUeWmd/H0ffTlkXXgwZi+eq1UCmqQwCh+eLsYOYCwY991i2Fah4h1BEMCx4qThGbsiA==",
- "dev": true,
- "dependencies": {
- "yallist": "^4.0.0"
- },
- "engines": {
- "node": ">=10"
- }
- },
"node_modules/nx/node_modules/mimic-fn": {
"version": "2.1.0",
"resolved": "https://registry.npmjs.org/mimic-fn/-/mimic-fn-2.1.0.tgz",
"integrity": "sha512-OqbOk5oEQeAZ8WXWydlu9HJjz9WVdEIvamMCcXmuqUYjTknH/sqsWvhQ3vgwKFRR1HpjvNBKQ37nbJgYzGqGcg==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=6"
}
},
"node_modules/nx/node_modules/minimatch": {
- "version": "3.0.5",
- "resolved": "https://registry.npmjs.org/minimatch/-/minimatch-3.0.5.tgz",
- "integrity": "sha512-tUpxzX0VAzJHjLu0xUfFv1gwVp9ba3IOuRAVH2EGuRW8a5emA2FlACLqiT/lDVtS1W+TGNwqz3sWaNyLgDJWuw==",
+ "version": "9.0.3",
+ "resolved": "https://registry.npmjs.org/minimatch/-/minimatch-9.0.3.tgz",
+ "integrity": "sha512-RHiac9mvaRw0x3AYRgDC1CxAP7HTcNrrECeA8YYJeWnpo+2Q5CegtZjaotWTWxDG3UeGA1coE05iH1mPjT/2mg==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "brace-expansion": "^1.1.7"
+ "brace-expansion": "^2.0.1"
},
"engines": {
- "node": "*"
+ "node": ">=16 || 14 >=14.17"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
}
},
"node_modules/nx/node_modules/npm-run-path": {
@@ -38469,6 +36043,7 @@
"resolved": "https://registry.npmjs.org/npm-run-path/-/npm-run-path-4.0.1.tgz",
"integrity": "sha512-S48WzZW777zhNIrn7gxOlISNAqi9ZC/uQFnRdbeIHhZhCA6UqpkOT8T1G7BvfdgP4Er8gF4sUbaS0i7QvIfCWw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"path-key": "^3.0.0"
},
@@ -38481,6 +36056,7 @@
"resolved": "https://registry.npmjs.org/onetime/-/onetime-5.1.2.tgz",
"integrity": "sha512-kbpaSSGJTWdAY5KPVeMOKXSrPtr8C8C7wodJbcsd51jRnmD+GZu8Y0VoU6Dm5Z4vWr0Ig/1NKuWRKf7j5aaYSg==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"mimic-fn": "^2.1.0"
},
@@ -38496,6 +36072,7 @@
"resolved": "https://registry.npmjs.org/open/-/open-8.4.2.tgz",
"integrity": "sha512-7x81NCL719oNbsq/3mh+hVrAWmFuEYUqrq/Iw3kUzH8ReypT9QQ0BLoJS7/G9k6N81XjW4qHWtjWwe/9eLy1EQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"define-lazy-prop": "^2.0.0",
"is-docker": "^2.1.1",
@@ -38508,11 +36085,35 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
+ "node_modules/nx/node_modules/ora": {
+ "version": "5.3.0",
+ "resolved": "https://registry.npmjs.org/ora/-/ora-5.3.0.tgz",
+ "integrity": "sha512-zAKMgGXUim0Jyd6CXK9lraBnD3H5yPGBPPOkC23a2BG6hsm4Zu6OQSjQuEtV0BHDf4aKHcUFvJiGRrFuW3MG8g==",
+ "dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "bl": "^4.0.3",
+ "chalk": "^4.1.0",
+ "cli-cursor": "^3.1.0",
+ "cli-spinners": "^2.5.0",
+ "is-interactive": "^1.0.0",
+ "log-symbols": "^4.0.0",
+ "strip-ansi": "^6.0.0",
+ "wcwidth": "^1.0.1"
+ },
+ "engines": {
+ "node": ">=10"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
+ }
+ },
"node_modules/nx/node_modules/path-key": {
"version": "3.1.1",
"resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
"integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=8"
}
@@ -38522,6 +36123,7 @@
"resolved": "https://registry.npmjs.org/restore-cursor/-/restore-cursor-3.1.0.tgz",
"integrity": "sha512-l+sSefzHpj5qimhFSE5a8nufZYAM3sBSVMAPtYkmC+4EH2anSGaEMXSD0izRQbu9nfyQ9y5JrVmp7E8oZrUjvA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"onetime": "^5.1.0",
"signal-exit": "^3.0.2"
@@ -38530,26 +36132,12 @@
"node": ">=8"
}
},
- "node_modules/nx/node_modules/semver": {
- "version": "7.5.3",
- "resolved": "https://registry.npmjs.org/semver/-/semver-7.5.3.tgz",
- "integrity": "sha512-QBlUtyVk/5EeHbi7X0fw6liDZc7BBmEaSYn01fMU1OUYbf6GPsbTtd8WmnqbI20SeycoHSeiybkE/q1Q+qlThQ==",
- "dev": true,
- "dependencies": {
- "lru-cache": "^6.0.0"
- },
- "bin": {
- "semver": "bin/semver.js"
- },
- "engines": {
- "node": ">=10"
- }
- },
"node_modules/nx/node_modules/string-width": {
"version": "4.2.3",
"resolved": "https://registry.npmjs.org/string-width/-/string-width-4.2.3.tgz",
"integrity": "sha512-wKyQRQpjJ0sIp62ErSZdGsjMJWsap5oRNihHhu6G7JVO/9jIB6UyevL+tXuOqrng8j/cxKTWyWUwvSTriiZz/g==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"emoji-regex": "^8.0.0",
"is-fullwidth-code-point": "^3.0.0",
@@ -38564,6 +36152,7 @@
"resolved": "https://registry.npmjs.org/strip-bom/-/strip-bom-3.0.0.tgz",
"integrity": "sha512-vavAMRXOgBVNF6nyEEmL3DBK19iRpDcoIwW+swQ+CbGiu7lju6t+JklA1MHweoWtadgt4ISVUsXLyDq34ddcwA==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=4"
}
@@ -38573,6 +36162,7 @@
"resolved": "https://registry.npmjs.org/tmp/-/tmp-0.2.3.tgz",
"integrity": "sha512-nZD7m9iCPC5g0pYmcaxogYKggSfLsdxl8of3Q/oIbqCqLLIO9IAF0GWjX1z9NZRHPiXv8Wex4yDCaZsgEw0Y8w==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=14.14"
}
@@ -38582,6 +36172,7 @@
"resolved": "https://registry.npmjs.org/tsconfig-paths/-/tsconfig-paths-4.2.0.tgz",
"integrity": "sha512-NoZ4roiN7LnbKn9QqE1amc9DJfzvZXxF4xDavcOWt1BPkdx+m+0gJuPM+S0vCe7zTJMYUP0R8pO2XMr+Y8oLIg==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"json5": "^2.2.2",
"minimist": "^1.2.6",
@@ -38591,20 +36182,12 @@
"node": ">=6"
}
},
- "node_modules/nx/node_modules/universalify": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/universalify/-/universalify-2.0.1.tgz",
- "integrity": "sha512-gptHNQghINnc/vTGIk0SOFGFNXw7JVrlRUtConJRlvaw6DuX0wO5Jeko9sWrMBhh+PsYAZ7oXAiOnf/UKogyiw==",
- "dev": true,
- "engines": {
- "node": ">= 10.0.0"
- }
- },
"node_modules/nx/node_modules/wrap-ansi": {
"version": "7.0.0",
"resolved": "https://registry.npmjs.org/wrap-ansi/-/wrap-ansi-7.0.0.tgz",
"integrity": "sha512-YVGIj2kamLSTxw6NsZjoBxfSwsn0ycdesmc4p+Q21c5zPuZ1pl+NfxVdxPtdHvmNVOQ6XSYG4AUtyt/Fi7D16Q==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"ansi-styles": "^4.0.0",
"string-width": "^4.1.0",
@@ -38617,37 +36200,17 @@
"url": "https://github.com/chalk/wrap-ansi?sponsor=1"
}
},
- "node_modules/nx/node_modules/y18n": {
- "version": "5.0.8",
- "resolved": "https://registry.npmjs.org/y18n/-/y18n-5.0.8.tgz",
- "integrity": "sha512-0pfFzegeDWJHJIAmTLRP2DwHjdF5s7jo9tuztdQxAhINCdvS+3nGINqPd00AphqJR/0LhANUS6/+7SCb98YOfA==",
- "dev": true,
- "engines": {
- "node": ">=10"
- }
- },
- "node_modules/nx/node_modules/yallist": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/yallist/-/yallist-4.0.0.tgz",
- "integrity": "sha512-3wdGidZyq5PB084XLES5TpOSRA3wjXAlIWMhum2kRcv/41Sn2emQ0dycQW4uZXLejwKvg6EsvbdlVL+FYEct7A==",
- "dev": true
- },
- "node_modules/nx/node_modules/yargs": {
- "version": "17.7.2",
- "resolved": "https://registry.npmjs.org/yargs/-/yargs-17.7.2.tgz",
- "integrity": "sha512-7dSzzRQ++CKnNI/krKnYRV7JKKPUXMEh61soaHKg9mrWEhzFWhFnxPxGl+69cD1Ou63C13NUPCnmIcrvqCuM6w==",
+ "node_modules/nx/node_modules/yaml": {
+ "version": "2.6.1",
+ "resolved": "https://registry.npmjs.org/yaml/-/yaml-2.6.1.tgz",
+ "integrity": "sha512-7r0XPzioN/Q9kXBro/XPnA6kznR73DHq+GXh5ON7ZozRO6aMjbmiBuKste2wslTFkC5d1dw0GooOCepZXJ2SAg==",
"dev": true,
- "dependencies": {
- "cliui": "^8.0.1",
- "escalade": "^3.1.1",
- "get-caller-file": "^2.0.5",
- "require-directory": "^2.1.1",
- "string-width": "^4.2.3",
- "y18n": "^5.0.5",
- "yargs-parser": "^21.1.1"
+ "license": "ISC",
+ "bin": {
+ "yaml": "bin.mjs"
},
"engines": {
- "node": ">=12"
+ "node": ">= 14"
}
},
"node_modules/nx/node_modules/yargs-parser": {
@@ -38655,235 +36218,11 @@
"resolved": "https://registry.npmjs.org/yargs-parser/-/yargs-parser-21.1.1.tgz",
"integrity": "sha512-tVpsJW7DdjecAiFpbIB1e3qxIQsE6NoPc5/eTdrbbIC4h0LVsWhnoa3g+m2HclBIujHzsxZ4VJVA+GUuc2/LBw==",
"dev": true,
+ "license": "ISC",
"engines": {
"node": ">=12"
}
},
- "node_modules/nx/node_modules/yargs/node_modules/cliui": {
- "version": "8.0.1",
- "resolved": "https://registry.npmjs.org/cliui/-/cliui-8.0.1.tgz",
- "integrity": "sha512-BSeNnyus75C4//NQ9gQt1/csTXyo/8Sb+afLAkzAptFuMsod9HFokGNudZpi/oQV73hnVK+sR+5PVRMd+Dr7YQ==",
- "dev": true,
- "dependencies": {
- "string-width": "^4.2.0",
- "strip-ansi": "^6.0.1",
- "wrap-ansi": "^7.0.0"
- },
- "engines": {
- "node": ">=12"
- }
- },
- "node_modules/nypm": {
- "version": "0.3.6",
- "resolved": "https://registry.npmjs.org/nypm/-/nypm-0.3.6.tgz",
- "integrity": "sha512-2CATJh3pd6CyNfU5VZM7qSwFu0ieyabkEdnogE30Obn1czrmOYiZ8DOZLe1yBdLKWoyD3Mcy2maUs+0MR3yVjQ==",
- "dev": true,
- "dependencies": {
- "citty": "^0.1.5",
- "execa": "^8.0.1",
- "pathe": "^1.1.2",
- "ufo": "^1.3.2"
- },
- "bin": {
- "nypm": "dist/cli.mjs"
- },
- "engines": {
- "node": "^14.16.0 || >=16.10.0"
- }
- },
- "node_modules/nypm/node_modules/cross-spawn": {
- "version": "7.0.3",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-7.0.3.tgz",
- "integrity": "sha512-iRDPJKUPVEND7dHPO8rkbOnPpyDygcDFtWjpeWNCgy8WP2rXcxXL8TskReQl6OrB2G7+UJrags1q15Fudc7G6w==",
- "dev": true,
- "dependencies": {
- "path-key": "^3.1.0",
- "shebang-command": "^2.0.0",
- "which": "^2.0.1"
- },
- "engines": {
- "node": ">= 8"
- }
- },
- "node_modules/nypm/node_modules/execa": {
- "version": "8.0.1",
- "resolved": "https://registry.npmjs.org/execa/-/execa-8.0.1.tgz",
- "integrity": "sha512-VyhnebXciFV2DESc+p6B+y0LjSm0krU4OgJN44qFAhBY0TJ+1V61tYD2+wHusZ6F9n5K+vl8k0sTy7PEfV4qpg==",
- "dev": true,
- "dependencies": {
- "cross-spawn": "^7.0.3",
- "get-stream": "^8.0.1",
- "human-signals": "^5.0.0",
- "is-stream": "^3.0.0",
- "merge-stream": "^2.0.0",
- "npm-run-path": "^5.1.0",
- "onetime": "^6.0.0",
- "signal-exit": "^4.1.0",
- "strip-final-newline": "^3.0.0"
- },
- "engines": {
- "node": ">=16.17"
- },
- "funding": {
- "url": "https://github.com/sindresorhus/execa?sponsor=1"
- }
- },
- "node_modules/nypm/node_modules/get-stream": {
- "version": "8.0.1",
- "resolved": "https://registry.npmjs.org/get-stream/-/get-stream-8.0.1.tgz",
- "integrity": "sha512-VaUJspBffn/LMCJVoMvSAdmscJyS1auj5Zulnn5UoYcY531UWmdwhRWkcGKnGU93m5HSXP9LP2usOryrBtQowA==",
- "dev": true,
- "engines": {
- "node": ">=16"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/nypm/node_modules/human-signals": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/human-signals/-/human-signals-5.0.0.tgz",
- "integrity": "sha512-AXcZb6vzzrFAUE61HnN4mpLqd/cSIwNQjtNWR0euPm6y0iqx3G4gOXaIDdtdDwZmhwe82LA6+zinmW4UBWVePQ==",
- "dev": true,
- "engines": {
- "node": ">=16.17.0"
- }
- },
- "node_modules/nypm/node_modules/is-stream": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/is-stream/-/is-stream-3.0.0.tgz",
- "integrity": "sha512-LnQR4bZ9IADDRSkvpqMGvt/tEJWclzklNgSw48V5EAaAeDd6qGvN8ei6k5p0tvxSR171VmGyHuTiAOfxAbr8kA==",
- "dev": true,
- "engines": {
- "node": "^12.20.0 || ^14.13.1 || >=16.0.0"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/nypm/node_modules/mimic-fn": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/mimic-fn/-/mimic-fn-4.0.0.tgz",
- "integrity": "sha512-vqiC06CuhBTUdZH+RYl8sFrL096vA45Ok5ISO6sE/Mr1jRbGH4Csnhi8f3wKVl7x8mO4Au7Ir9D3Oyv1VYMFJw==",
- "dev": true,
- "engines": {
- "node": ">=12"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/nypm/node_modules/npm-run-path": {
- "version": "5.3.0",
- "resolved": "https://registry.npmjs.org/npm-run-path/-/npm-run-path-5.3.0.tgz",
- "integrity": "sha512-ppwTtiJZq0O/ai0z7yfudtBpWIoxM8yE6nHi1X47eFR2EWORqfbu6CnPlNsjeN683eT0qG6H/Pyf9fCcvjnnnQ==",
- "dev": true,
- "dependencies": {
- "path-key": "^4.0.0"
- },
- "engines": {
- "node": "^12.20.0 || ^14.13.1 || >=16.0.0"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/nypm/node_modules/npm-run-path/node_modules/path-key": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/path-key/-/path-key-4.0.0.tgz",
- "integrity": "sha512-haREypq7xkM7ErfgIyA0z+Bj4AGKlMSdlQE2jvJo6huWD1EdkKYV+G/T4nq0YEF2vgTT8kqMFKo1uHn950r4SQ==",
- "dev": true,
- "engines": {
- "node": ">=12"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/nypm/node_modules/onetime": {
- "version": "6.0.0",
- "resolved": "https://registry.npmjs.org/onetime/-/onetime-6.0.0.tgz",
- "integrity": "sha512-1FlR+gjXK7X+AsAHso35MnyN5KqGwJRi/31ft6x0M194ht7S+rWAvd7PHss9xSKMzE0asv1pyIHaJYq+BbacAQ==",
- "dev": true,
- "dependencies": {
- "mimic-fn": "^4.0.0"
- },
- "engines": {
- "node": ">=12"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/nypm/node_modules/path-key": {
- "version": "3.1.1",
- "resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
- "integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
- "dev": true,
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/nypm/node_modules/shebang-command": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/shebang-command/-/shebang-command-2.0.0.tgz",
- "integrity": "sha512-kHxr2zZpYtdmrN1qDjrrX/Z1rR1kG8Dx+gkpK1G4eXmvXswmcE1hTWBWYUzlraYw1/yZp6YuDY77YtvbN0dmDA==",
- "dev": true,
- "dependencies": {
- "shebang-regex": "^3.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/nypm/node_modules/shebang-regex": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/shebang-regex/-/shebang-regex-3.0.0.tgz",
- "integrity": "sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==",
- "dev": true,
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/nypm/node_modules/signal-exit": {
- "version": "4.1.0",
- "resolved": "https://registry.npmjs.org/signal-exit/-/signal-exit-4.1.0.tgz",
- "integrity": "sha512-bzyZ1e88w9O1iNJbKnOlvYTrWPDl46O1bG0D3XInv+9tkPrxrN8jUUTiFlDkkmKWgn1M6CfIA13SuGqOa9Korw==",
- "dev": true,
- "engines": {
- "node": ">=14"
- },
- "funding": {
- "url": "https://github.com/sponsors/isaacs"
- }
- },
- "node_modules/nypm/node_modules/strip-final-newline": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/strip-final-newline/-/strip-final-newline-3.0.0.tgz",
- "integrity": "sha512-dOESqjYr96iWYylGObzd39EuNTa5VJxyvVAEm5Jnh7KGo75V43Hk1odPQkNDyXNmUR6k+gEiDVXnjB8HJ3crXw==",
- "dev": true,
- "engines": {
- "node": ">=12"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/nypm/node_modules/which": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
- "integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
- "dev": true,
- "dependencies": {
- "isexe": "^2.0.0"
- },
- "bin": {
- "node-which": "bin/node-which"
- },
- "engines": {
- "node": ">= 8"
- }
- },
"node_modules/ob1": {
"version": "0.80.5",
"resolved": "https://registry.npmjs.org/ob1/-/ob1-0.80.5.tgz",
@@ -39090,12 +36429,6 @@
"integrity": "sha512-fZ4qZdQ2nxJvtcasX7Ghl+WlWS/d9IgnBIwFZXVNNZUmzpno91SX5bc5vuxiuKoCtK78XxGGNuSCrDC7xYB3OQ==",
"dev": true
},
- "node_modules/ohash": {
- "version": "1.1.3",
- "resolved": "https://registry.npmjs.org/ohash/-/ohash-1.1.3.tgz",
- "integrity": "sha512-zuHHiGTYTA1sYJ/wZN+t5HKZaH23i4yI1HMwbuXm24Nid7Dv0KcuRlKoNKS9UNfAVSBlnGLcuQrnOKWOZoEGaw==",
- "dev": true
- },
"node_modules/on-finished": {
"version": "2.3.0",
"resolved": "https://registry.npmjs.org/on-finished/-/on-finished-2.3.0.tgz",
@@ -39444,6 +36777,7 @@
"resolved": "https://registry.npmjs.org/p-map-series/-/p-map-series-2.1.0.tgz",
"integrity": "sha512-RpYIIK1zXSNEOdwxcfe7FdvGcs7+y5n8rifMhMNWvaxRNMPINJHF5GDeuVxWqnfrcHPSCnp7Oo5yNXHId9Av2Q==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=8"
}
@@ -39465,6 +36799,7 @@
"resolved": "https://registry.npmjs.org/p-queue/-/p-queue-6.6.2.tgz",
"integrity": "sha512-RwFpb72c/BhQLEXIZ5K2e+AhgNVmIejGlTgiB9MzZ0e93GRvqZ7uSi0dvRF7/XIXDeNkra2fNHBxTyPDGySpjQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"eventemitter3": "^4.0.4",
"p-timeout": "^3.2.0"
@@ -39486,11 +36821,12 @@
}
},
"node_modules/p-retry": {
- "version": "4.6.1",
- "resolved": "https://registry.npmjs.org/p-retry/-/p-retry-4.6.1.tgz",
- "integrity": "sha512-e2xXGNhZOZ0lfgR9kL34iGlU8N/KO0xZnQxVEwdeOvpqNDQfdnxIYizvWtK8RglUa3bGqI8g0R/BdfzLMxRkiA==",
+ "version": "4.6.2",
+ "resolved": "https://registry.npmjs.org/p-retry/-/p-retry-4.6.2.tgz",
+ "integrity": "sha512-312Id396EbJdvRONlngUx0NydfrIQ5lsYu0znKVUzVvArzEIt08V1qhtyESbGVd1FGX7UKtiFp5uwKZdM8wIuQ==",
+ "license": "MIT",
"dependencies": {
- "@types/retry": "^0.12.0",
+ "@types/retry": "0.12.0",
"retry": "^0.13.1"
},
"engines": {
@@ -39501,6 +36837,7 @@
"version": "0.13.1",
"resolved": "https://registry.npmjs.org/retry/-/retry-0.13.1.tgz",
"integrity": "sha512-XQBQ3I8W1Cge0Seh+6gjj03LbmRFWuoszgK9ooCpwYIrhhoO80pfq4cUkU5DkknwfOfFteRwlZ56PYOGYyFWdg==",
+ "license": "MIT",
"engines": {
"node": ">= 4"
}
@@ -39510,6 +36847,7 @@
"resolved": "https://registry.npmjs.org/p-timeout/-/p-timeout-3.2.0.tgz",
"integrity": "sha512-rhIwUycgwwKcP9yTOOFK/AKsAopjjCakVqLHePO3CC6Mir1Z99xT+R63jZxAT5lFZLa2inS5h+ZS2GvR99/FBg==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"p-finally": "^1.0.0"
},
@@ -39541,80 +36879,31 @@
}
},
"node_modules/pac-proxy-agent": {
- "version": "7.0.1",
- "resolved": "https://registry.npmjs.org/pac-proxy-agent/-/pac-proxy-agent-7.0.1.tgz",
- "integrity": "sha512-ASV8yU4LLKBAjqIPMbrgtaKIvxQri/yh2OpI+S6hVa9JRkUI3Y3NPFbfngDtY7oFtSMD3w31Xns89mDa3Feo5A==",
+ "version": "7.1.0",
+ "resolved": "https://registry.npmjs.org/pac-proxy-agent/-/pac-proxy-agent-7.1.0.tgz",
+ "integrity": "sha512-Z5FnLVVZSnX7WjBg0mhDtydeRZ1xMcATZThjySQUHqr+0ksP8kqaw23fNKkaaN/Z8gwLUs/W7xdl0I75eP2Xyw==",
+ "license": "MIT",
"dependencies": {
"@tootallnate/quickjs-emscripten": "^0.23.0",
- "agent-base": "^7.0.2",
+ "agent-base": "^7.1.2",
"debug": "^4.3.4",
"get-uri": "^6.0.1",
"http-proxy-agent": "^7.0.0",
- "https-proxy-agent": "^7.0.2",
- "pac-resolver": "^7.0.0",
- "socks-proxy-agent": "^8.0.2"
- },
- "engines": {
- "node": ">= 14"
- }
- },
- "node_modules/pac-proxy-agent/node_modules/agent-base": {
- "version": "7.1.1",
- "resolved": "https://registry.npmjs.org/agent-base/-/agent-base-7.1.1.tgz",
- "integrity": "sha512-H0TSyFNDMomMNJQBn8wFV5YC/2eJ+VXECwOadZJT554xP6cODZHPX3H9QMQECxvrgiSOP1pHjy1sMWQVYJOUOA==",
- "dependencies": {
- "debug": "^4.3.4"
- },
- "engines": {
- "node": ">= 14"
- }
- },
- "node_modules/pac-proxy-agent/node_modules/http-proxy-agent": {
- "version": "7.0.2",
- "resolved": "https://registry.npmjs.org/http-proxy-agent/-/http-proxy-agent-7.0.2.tgz",
- "integrity": "sha512-T1gkAiYYDWYx3V5Bmyu7HcfcvL7mUrTWiM6yOfa3PIphViJ/gFPbvidQ+veqSOHci/PxBcDabeUNCzpOODJZig==",
- "dependencies": {
- "agent-base": "^7.1.0",
- "debug": "^4.3.4"
- },
- "engines": {
- "node": ">= 14"
- }
- },
- "node_modules/pac-proxy-agent/node_modules/https-proxy-agent": {
- "version": "7.0.5",
- "resolved": "https://registry.npmjs.org/https-proxy-agent/-/https-proxy-agent-7.0.5.tgz",
- "integrity": "sha512-1e4Wqeblerz+tMKPIq2EMGiiWW1dIjZOksyHWSUm1rmuvw/how9hBHZ38lAGj5ID4Ik6EdkOw7NmWPy6LAwalw==",
- "license": "MIT",
- "dependencies": {
- "agent-base": "^7.0.2",
- "debug": "4"
- },
- "engines": {
- "node": ">= 14"
- }
- },
- "node_modules/pac-proxy-agent/node_modules/socks-proxy-agent": {
- "version": "8.0.4",
- "resolved": "https://registry.npmjs.org/socks-proxy-agent/-/socks-proxy-agent-8.0.4.tgz",
- "integrity": "sha512-GNAq/eg8Udq2x0eNiFkr9gRg5bA7PXEWagQdeRX4cPSG+X/8V38v637gim9bjFptMk1QWsCTr0ttrJEiXbNnRw==",
- "license": "MIT",
- "dependencies": {
- "agent-base": "^7.1.1",
- "debug": "^4.3.4",
- "socks": "^2.8.3"
+ "https-proxy-agent": "^7.0.6",
+ "pac-resolver": "^7.0.1",
+ "socks-proxy-agent": "^8.0.5"
},
"engines": {
"node": ">= 14"
}
},
"node_modules/pac-resolver": {
- "version": "7.0.0",
- "resolved": "https://registry.npmjs.org/pac-resolver/-/pac-resolver-7.0.0.tgz",
- "integrity": "sha512-Fd9lT9vJbHYRACT8OhCbZBbxr6KRSawSovFpy8nDGshaK99S/EBhVIHp9+crhxrsZOuvLpgL1n23iyPg6Rl2hg==",
+ "version": "7.0.1",
+ "resolved": "https://registry.npmjs.org/pac-resolver/-/pac-resolver-7.0.1.tgz",
+ "integrity": "sha512-5NPgf87AT2STgwa2ntRMr45jTKrYBGkVU36yT0ig/n/GMAa3oPqhZfIQ2kMEimReg0+t9kZViDVZ83qfVUlckg==",
+ "license": "MIT",
"dependencies": {
"degenerator": "^5.0.0",
- "ip": "^1.1.8",
"netmask": "^2.0.2"
},
"engines": {
@@ -39646,39 +36935,38 @@
"version": "1.0.0",
"resolved": "https://registry.npmjs.org/package-json-from-dist/-/package-json-from-dist-1.0.0.tgz",
"integrity": "sha512-dATvCeZN/8wQsGywez1mzHtTlP22H8OEfPrVMLNr4/eGa+ijtLn/6M5f0dY8UKNrC2O9UCU6SSoG3qRKnt7STw==",
- "dev": true,
"license": "BlueOak-1.0.0"
},
"node_modules/pacote": {
- "version": "15.2.0",
- "resolved": "https://registry.npmjs.org/pacote/-/pacote-15.2.0.tgz",
- "integrity": "sha512-rJVZeIwHTUta23sIZgEIM62WYwbmGbThdbnkt81ravBplQv+HjyroqnLRNH2+sLJHcGZmLRmhPwACqhfTcOmnA==",
+ "version": "18.0.6",
+ "resolved": "https://registry.npmjs.org/pacote/-/pacote-18.0.6.tgz",
+ "integrity": "sha512-+eK3G27SMwsB8kLIuj4h1FUhHtwiEUo21Tw8wNjmvdlpOEr613edv+8FUsTj/4F/VN5ywGE19X18N7CC2EJk6A==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "@npmcli/git": "^4.0.0",
+ "@npmcli/git": "^5.0.0",
"@npmcli/installed-package-contents": "^2.0.1",
- "@npmcli/promise-spawn": "^6.0.1",
- "@npmcli/run-script": "^6.0.0",
- "cacache": "^17.0.0",
+ "@npmcli/package-json": "^5.1.0",
+ "@npmcli/promise-spawn": "^7.0.0",
+ "@npmcli/run-script": "^8.0.0",
+ "cacache": "^18.0.0",
"fs-minipass": "^3.0.0",
- "minipass": "^5.0.0",
- "npm-package-arg": "^10.0.0",
- "npm-packlist": "^7.0.0",
- "npm-pick-manifest": "^8.0.0",
- "npm-registry-fetch": "^14.0.0",
- "proc-log": "^3.0.0",
+ "minipass": "^7.0.2",
+ "npm-package-arg": "^11.0.0",
+ "npm-packlist": "^8.0.0",
+ "npm-pick-manifest": "^9.0.0",
+ "npm-registry-fetch": "^17.0.0",
+ "proc-log": "^4.0.0",
"promise-retry": "^2.0.1",
- "read-package-json": "^6.0.0",
- "read-package-json-fast": "^3.0.0",
- "sigstore": "^1.3.0",
+ "sigstore": "^2.2.0",
"ssri": "^10.0.0",
"tar": "^6.1.11"
},
"bin": {
- "pacote": "lib/bin.js"
+ "pacote": "bin/index.js"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/pacote/node_modules/brace-expansion": {
@@ -39686,22 +36974,24 @@
"resolved": "https://registry.npmjs.org/brace-expansion/-/brace-expansion-2.0.1.tgz",
"integrity": "sha512-XnAIvQ8eM+kC6aULx6wuQiwVsnzsi9d3WxzV3FpWTGA19F621kwdbsAcFKXgKUHZWsy+mY6iL1sHTxWEFCytDA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"balanced-match": "^1.0.0"
}
},
"node_modules/pacote/node_modules/cacache": {
- "version": "17.1.4",
- "resolved": "https://registry.npmjs.org/cacache/-/cacache-17.1.4.tgz",
- "integrity": "sha512-/aJwG2l3ZMJ1xNAnqbMpA40of9dj/pIH3QfiuQSqjfPJF747VR0J/bHn+/KdNnHKc6XQcWt/AfRSBft82W1d2A==",
+ "version": "18.0.4",
+ "resolved": "https://registry.npmjs.org/cacache/-/cacache-18.0.4.tgz",
+ "integrity": "sha512-B+L5iIa9mgcjLbliir2th36yEwPftrzteHYujzsx3dFP/31GCHcIeS8f5MGd80odLOjaOvSpU3EEAmRQptkxLQ==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"@npmcli/fs": "^3.1.0",
"fs-minipass": "^3.0.0",
"glob": "^10.2.2",
- "lru-cache": "^7.7.1",
+ "lru-cache": "^10.0.1",
"minipass": "^7.0.3",
- "minipass-collect": "^1.0.2",
+ "minipass-collect": "^2.0.1",
"minipass-flush": "^1.0.5",
"minipass-pipeline": "^1.2.4",
"p-map": "^4.0.0",
@@ -39710,17 +37000,7 @@
"unique-filename": "^3.0.0"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
- }
- },
- "node_modules/pacote/node_modules/cacache/node_modules/minipass": {
- "version": "7.1.2",
- "resolved": "https://registry.npmjs.org/minipass/-/minipass-7.1.2.tgz",
- "integrity": "sha512-qOOzS1cBTWYF4BH8fVePDBOO9iptMnGUEZwNc/cMWnTV2nVLZ7VoNWEPHkYczZA0pdoA7dl6e7FL659nX9S2aw==",
- "dev": true,
- "license": "ISC",
- "engines": {
- "node": ">=16 || 14 >=14.17"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/pacote/node_modules/fs-minipass": {
@@ -39728,6 +37008,7 @@
"resolved": "https://registry.npmjs.org/fs-minipass/-/fs-minipass-3.0.3.tgz",
"integrity": "sha512-XUBA9XClHbnJWSfBzjkm6RvPsyg3sryZt06BEQoXcF7EK/xpGaQYJgQKDJSUH5SGZ76Y7pFx1QBnXz09rU5Fbw==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"minipass": "^7.0.3"
},
@@ -39735,16 +37016,6 @@
"node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/pacote/node_modules/fs-minipass/node_modules/minipass": {
- "version": "7.1.2",
- "resolved": "https://registry.npmjs.org/minipass/-/minipass-7.1.2.tgz",
- "integrity": "sha512-qOOzS1cBTWYF4BH8fVePDBOO9iptMnGUEZwNc/cMWnTV2nVLZ7VoNWEPHkYczZA0pdoA7dl6e7FL659nX9S2aw==",
- "dev": true,
- "license": "ISC",
- "engines": {
- "node": ">=16 || 14 >=14.17"
- }
- },
"node_modules/pacote/node_modules/glob": {
"version": "10.4.5",
"resolved": "https://registry.npmjs.org/glob/-/glob-10.4.5.tgz",
@@ -39766,26 +37037,17 @@
"url": "https://github.com/sponsors/isaacs"
}
},
- "node_modules/pacote/node_modules/glob/node_modules/minipass": {
- "version": "7.1.2",
- "resolved": "https://registry.npmjs.org/minipass/-/minipass-7.1.2.tgz",
- "integrity": "sha512-qOOzS1cBTWYF4BH8fVePDBOO9iptMnGUEZwNc/cMWnTV2nVLZ7VoNWEPHkYczZA0pdoA7dl6e7FL659nX9S2aw==",
- "dev": true,
- "license": "ISC",
- "engines": {
- "node": ">=16 || 14 >=14.17"
- }
- },
"node_modules/pacote/node_modules/hosted-git-info": {
- "version": "6.1.1",
- "resolved": "https://registry.npmjs.org/hosted-git-info/-/hosted-git-info-6.1.1.tgz",
- "integrity": "sha512-r0EI+HBMcXadMrugk0GCQ+6BQV39PiWAZVfq7oIckeGiN7sjRGyQxPdft3nQekFTCQbYxLBH+/axZMeH8UX6+w==",
+ "version": "7.0.2",
+ "resolved": "https://registry.npmjs.org/hosted-git-info/-/hosted-git-info-7.0.2.tgz",
+ "integrity": "sha512-puUZAUKT5m8Zzvs72XWy3HtvVbTWljRE66cP60bxJzAqf2DgICo7lYTY2IHUmLnNpjYvw5bvmoHvPc0QO2a62w==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "lru-cache": "^7.5.1"
+ "lru-cache": "^10.0.1"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/pacote/node_modules/ignore-walk": {
@@ -39793,6 +37055,7 @@
"resolved": "https://registry.npmjs.org/ignore-walk/-/ignore-walk-6.0.5.tgz",
"integrity": "sha512-VuuG0wCnjhnylG1ABXT3dAuIpTNDs/G8jlpmwXY03fXoXy/8ZK8/T+hMzt8L4WnrLCJgdybqgPagnF/f97cg3A==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"minimatch": "^9.0.0"
},
@@ -39800,31 +37063,6 @@
"node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/pacote/node_modules/jackspeak": {
- "version": "3.4.3",
- "resolved": "https://registry.npmjs.org/jackspeak/-/jackspeak-3.4.3.tgz",
- "integrity": "sha512-OGlZQpz2yfahA/Rd1Y8Cd9SIEsqvXkLVoSw/cgwhnhFMDbsQFeZYoJJ7bIZBS9BcamUW96asq/npPWugM+RQBw==",
- "dev": true,
- "license": "BlueOak-1.0.0",
- "dependencies": {
- "@isaacs/cliui": "^8.0.2"
- },
- "funding": {
- "url": "https://github.com/sponsors/isaacs"
- },
- "optionalDependencies": {
- "@pkgjs/parseargs": "^0.11.0"
- }
- },
- "node_modules/pacote/node_modules/lru-cache": {
- "version": "7.18.3",
- "resolved": "https://registry.npmjs.org/lru-cache/-/lru-cache-7.18.3.tgz",
- "integrity": "sha512-jumlc0BIUrS3qJGgIkWZsyfAM7NCWiBcCDhnd+3NNM5KbBmLTgHVfWBcg6W+rLUsIpzpERPsvwUP7CckAQSOoA==",
- "dev": true,
- "engines": {
- "node": ">=12"
- }
- },
"node_modules/pacote/node_modules/minimatch": {
"version": "9.0.5",
"resolved": "https://registry.npmjs.org/minimatch/-/minimatch-9.0.5.tgz",
@@ -39842,36 +37080,39 @@
}
},
"node_modules/pacote/node_modules/minipass": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/minipass/-/minipass-5.0.0.tgz",
- "integrity": "sha512-3FnjYuehv9k6ovOEbyOswadCDPX1piCfhV8ncmYtHOjuPwylVWsghTLo7rabjC3Rx5xD4HDx8Wm1xnMF7S5qFQ==",
+ "version": "7.1.2",
+ "resolved": "https://registry.npmjs.org/minipass/-/minipass-7.1.2.tgz",
+ "integrity": "sha512-qOOzS1cBTWYF4BH8fVePDBOO9iptMnGUEZwNc/cMWnTV2nVLZ7VoNWEPHkYczZA0pdoA7dl6e7FL659nX9S2aw==",
"dev": true,
+ "license": "ISC",
"engines": {
- "node": ">=8"
+ "node": ">=16 || 14 >=14.17"
}
},
"node_modules/pacote/node_modules/npm-package-arg": {
- "version": "10.1.0",
- "resolved": "https://registry.npmjs.org/npm-package-arg/-/npm-package-arg-10.1.0.tgz",
- "integrity": "sha512-uFyyCEmgBfZTtrKk/5xDfHp6+MdrqGotX/VoOyEEl3mBwiEE5FlBaePanazJSVMPT7vKepcjYBY2ztg9A3yPIA==",
+ "version": "11.0.3",
+ "resolved": "https://registry.npmjs.org/npm-package-arg/-/npm-package-arg-11.0.3.tgz",
+ "integrity": "sha512-sHGJy8sOC1YraBywpzQlIKBE4pBbGbiF95U6Auspzyem956E0+FtDtsx1ZxlOJkQCZ1AFXAY/yuvtFYrOxF+Bw==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "hosted-git-info": "^6.0.0",
- "proc-log": "^3.0.0",
+ "hosted-git-info": "^7.0.0",
+ "proc-log": "^4.0.0",
"semver": "^7.3.5",
"validate-npm-package-name": "^5.0.0"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/pacote/node_modules/npm-packlist": {
- "version": "7.0.4",
- "resolved": "https://registry.npmjs.org/npm-packlist/-/npm-packlist-7.0.4.tgz",
- "integrity": "sha512-d6RGEuRrNS5/N84iglPivjaJPxhDbZmlbTwTDX2IbcRHG5bZCdtysYMhwiPvcF4GisXHGn7xsxv+GQ7T/02M5Q==",
+ "version": "8.0.2",
+ "resolved": "https://registry.npmjs.org/npm-packlist/-/npm-packlist-8.0.2.tgz",
+ "integrity": "sha512-shYrPFIS/JLP4oQmAwDyk5HcyysKW8/JLTEA32S0Z5TzvpaeeX2yMFfoK1fjEBnCBvVyIB/Jj/GBFdm0wsgzbA==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "ignore-walk": "^6.0.0"
+ "ignore-walk": "^6.0.4"
},
"engines": {
"node": "^14.17.0 || ^16.13.0 || >=18.0.0"
@@ -39882,6 +37123,7 @@
"resolved": "https://registry.npmjs.org/ssri/-/ssri-10.0.6.tgz",
"integrity": "sha512-MGrFH9Z4NP9Iyhqn16sDtBpRRNJ0Y2hNa6D65h736fVSaPCHr4DM4sWUNvVaSuC+0OBGhwsrydQwmgfg5LncqQ==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"minipass": "^7.0.3"
},
@@ -39889,21 +37131,12 @@
"node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/pacote/node_modules/ssri/node_modules/minipass": {
- "version": "7.1.2",
- "resolved": "https://registry.npmjs.org/minipass/-/minipass-7.1.2.tgz",
- "integrity": "sha512-qOOzS1cBTWYF4BH8fVePDBOO9iptMnGUEZwNc/cMWnTV2nVLZ7VoNWEPHkYczZA0pdoA7dl6e7FL659nX9S2aw==",
- "dev": true,
- "license": "ISC",
- "engines": {
- "node": ">=16 || 14 >=14.17"
- }
- },
"node_modules/pacote/node_modules/unique-filename": {
"version": "3.0.0",
"resolved": "https://registry.npmjs.org/unique-filename/-/unique-filename-3.0.0.tgz",
"integrity": "sha512-afXhuC55wkAmZ0P18QsVE6kp8JaxrEokN2HGIoIVv2ijHQd419H0+6EigAFcIzXeMIkcIkNBpB3L/DXB3cTS/g==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"unique-slug": "^4.0.0"
},
@@ -39916,6 +37149,7 @@
"resolved": "https://registry.npmjs.org/unique-slug/-/unique-slug-4.0.0.tgz",
"integrity": "sha512-WrcA6AyEfqDX5bWige/4NQfPZMtASNVxdmWR76WESYQVAACSgWcR6e9i0mofqqBxYFtL4oAxPIptY73/0YE1DQ==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"imurmurhash": "^0.1.4"
},
@@ -39928,6 +37162,7 @@
"resolved": "https://registry.npmjs.org/validate-npm-package-name/-/validate-npm-package-name-5.0.1.tgz",
"integrity": "sha512-OljLrQ9SQdOUqTaQxqL5dEfZWrXExyyWsozYlAWFawPVNuD83igl7uJD2RTkNMbniIYgt8l81eCJGIdQF7avLQ==",
"dev": true,
+ "license": "ISC",
"engines": {
"node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
@@ -39987,6 +37222,31 @@
"resolved": "https://registry.npmjs.org/parse-cache-control/-/parse-cache-control-1.0.1.tgz",
"integrity": "sha512-60zvsJReQPX5/QP0Kzfd/VrpjScIQ7SHBW6bFCYfEP+fp0Eppr1SHhIO5nd1PjZtvclzSzES9D/p5nFJurwfWg=="
},
+ "node_modules/parse-conflict-json": {
+ "version": "3.0.1",
+ "resolved": "https://registry.npmjs.org/parse-conflict-json/-/parse-conflict-json-3.0.1.tgz",
+ "integrity": "sha512-01TvEktc68vwbJOtWZluyWeVGWjP+bZwXtPDMQVbBKzbJ/vZBif0L69KH1+cHv1SZ6e0FKLvjyHe8mqsIqYOmw==",
+ "dev": true,
+ "license": "ISC",
+ "dependencies": {
+ "json-parse-even-better-errors": "^3.0.0",
+ "just-diff": "^6.0.0",
+ "just-diff-apply": "^5.2.0"
+ },
+ "engines": {
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ }
+ },
+ "node_modules/parse-conflict-json/node_modules/json-parse-even-better-errors": {
+ "version": "3.0.2",
+ "resolved": "https://registry.npmjs.org/json-parse-even-better-errors/-/json-parse-even-better-errors-3.0.2.tgz",
+ "integrity": "sha512-fi0NG4bPjCHunUJffmLd0gxssIgkNmArMvis4iNah6Owg1MCJjWhEcDLmsK6iGkJq3tHwbDkTlce70/tmXN4cQ==",
+ "dev": true,
+ "license": "MIT",
+ "engines": {
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ }
+ },
"node_modules/parse-entities": {
"version": "1.2.2",
"resolved": "https://registry.npmjs.org/parse-entities/-/parse-entities-1.2.2.tgz",
@@ -40039,6 +37299,7 @@
"resolved": "https://registry.npmjs.org/parse-path/-/parse-path-7.0.0.tgz",
"integrity": "sha512-Euf9GG8WT9CdqwuWJGdf3RkUcTBArppHABkO7Lm8IzRQp0e2r/kkFnmhu4TSK30Wcu5rVAZLmfPKSBBi9tWFog==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"protocols": "^2.0.0"
}
@@ -40048,6 +37309,7 @@
"resolved": "https://registry.npmjs.org/parse-url/-/parse-url-8.1.0.tgz",
"integrity": "sha512-xDvOoLU5XRrcOZvnI6b8zA6n9O9ejNk/GExuz1yBuWUGn9KA97GI6HTs6u02wKara1CeVmZhH+0TZFdWScR89w==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"parse-path": "^7.0.0"
}
@@ -40152,20 +37414,6 @@
"url": "https://github.com/chalk/chalk?sponsor=1"
}
},
- "node_modules/patch-package/node_modules/cross-spawn": {
- "version": "7.0.3",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-7.0.3.tgz",
- "integrity": "sha512-iRDPJKUPVEND7dHPO8rkbOnPpyDygcDFtWjpeWNCgy8WP2rXcxXL8TskReQl6OrB2G7+UJrags1q15Fudc7G6w==",
- "dev": true,
- "dependencies": {
- "path-key": "^3.1.0",
- "shebang-command": "^2.0.0",
- "which": "^2.0.1"
- },
- "engines": {
- "node": ">= 8"
- }
- },
"node_modules/patch-package/node_modules/fs-extra": {
"version": "9.1.0",
"resolved": "https://registry.npmjs.org/fs-extra/-/fs-extra-9.1.0.tgz",
@@ -40250,15 +37498,6 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/patch-package/node_modules/path-key": {
- "version": "3.1.1",
- "resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
- "integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
- "dev": true,
- "engines": {
- "node": ">=8"
- }
- },
"node_modules/patch-package/node_modules/rimraf": {
"version": "2.7.1",
"resolved": "https://registry.npmjs.org/rimraf/-/rimraf-2.7.1.tgz",
@@ -40271,27 +37510,6 @@
"rimraf": "bin.js"
}
},
- "node_modules/patch-package/node_modules/shebang-command": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/shebang-command/-/shebang-command-2.0.0.tgz",
- "integrity": "sha512-kHxr2zZpYtdmrN1qDjrrX/Z1rR1kG8Dx+gkpK1G4eXmvXswmcE1hTWBWYUzlraYw1/yZp6YuDY77YtvbN0dmDA==",
- "dev": true,
- "dependencies": {
- "shebang-regex": "^3.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/patch-package/node_modules/shebang-regex": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/shebang-regex/-/shebang-regex-3.0.0.tgz",
- "integrity": "sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==",
- "dev": true,
- "engines": {
- "node": ">=8"
- }
- },
"node_modules/patch-package/node_modules/slash": {
"version": "2.0.0",
"resolved": "https://registry.npmjs.org/slash/-/slash-2.0.0.tgz",
@@ -40322,21 +37540,6 @@
"node": ">= 10.0.0"
}
},
- "node_modules/patch-package/node_modules/which": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
- "integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
- "dev": true,
- "dependencies": {
- "isexe": "^2.0.0"
- },
- "bin": {
- "node-which": "bin/node-which"
- },
- "engines": {
- "node": ">= 8"
- }
- },
"node_modules/patch-package/node_modules/yaml": {
"version": "2.6.0",
"resolved": "https://registry.npmjs.org/yaml/-/yaml-2.6.0.tgz",
@@ -40411,7 +37614,6 @@
"version": "1.11.1",
"resolved": "https://registry.npmjs.org/path-scurry/-/path-scurry-1.11.1.tgz",
"integrity": "sha512-Xa4Nw17FS9ApQFJ9umLiJS4orGjm7ZzwUrwamcGQuHSzDyth9boKDaycYdDcZDuqYATXw4HFXgaqWTctW/v1HA==",
- "dev": true,
"license": "BlueOak-1.0.0",
"dependencies": {
"lru-cache": "^10.2.0",
@@ -40424,18 +37626,10 @@
"url": "https://github.com/sponsors/isaacs"
}
},
- "node_modules/path-scurry/node_modules/lru-cache": {
- "version": "10.4.3",
- "resolved": "https://registry.npmjs.org/lru-cache/-/lru-cache-10.4.3.tgz",
- "integrity": "sha512-JNAzZcXrCt42VGLuYz0zfAzDfAvJWW6AfYlDBQyDV5DClI2m5sAmK+OIO7s59XfsRsWHp02jAJrRadPRGTt6SQ==",
- "dev": true,
- "license": "ISC"
- },
"node_modules/path-scurry/node_modules/minipass": {
"version": "7.1.2",
"resolved": "https://registry.npmjs.org/minipass/-/minipass-7.1.2.tgz",
"integrity": "sha512-qOOzS1cBTWYF4BH8fVePDBOO9iptMnGUEZwNc/cMWnTV2nVLZ7VoNWEPHkYczZA0pdoA7dl6e7FL659nX9S2aw==",
- "dev": true,
"license": "ISC",
"engines": {
"node": ">=16 || 14 >=14.17"
@@ -40444,7 +37638,8 @@
"node_modules/path-to-regexp": {
"version": "0.1.7",
"resolved": "https://registry.npmjs.org/path-to-regexp/-/path-to-regexp-0.1.7.tgz",
- "integrity": "sha512-5DFkuoqlv1uYQKxy8omFBeJPQcdoE07Kv2sferDCrAq1ohOU+MSDswDIbnx3YAM60qIOnYa53wBhXW0EbMonrQ=="
+ "integrity": "sha512-5DFkuoqlv1uYQKxy8omFBeJPQcdoE07Kv2sferDCrAq1ohOU+MSDswDIbnx3YAM60qIOnYa53wBhXW0EbMonrQ==",
+ "license": "MIT"
},
"node_modules/path-type": {
"version": "3.0.0",
@@ -40467,11 +37662,14 @@
"node": ">=4"
}
},
- "node_modules/pathe": {
- "version": "1.1.2",
- "resolved": "https://registry.npmjs.org/pathe/-/pathe-1.1.2.tgz",
- "integrity": "sha512-whLdWMYL2TwI08hn8/ZqAbrVemu0LNaNNJZX73O6qaIdCTfXutsLhMkjdENX0qhsQ9uIimo4/aQOmXkoon2nDQ==",
- "dev": true
+ "node_modules/pathval": {
+ "version": "2.0.0",
+ "resolved": "https://registry.npmjs.org/pathval/-/pathval-2.0.0.tgz",
+ "integrity": "sha512-vE7JKRyES09KiunauX7nd2Q9/L7lhok4smP9RZTDeD4MVs72Dp2qNFVz39Nz5a0FVEW0BJR6C0DYrq6unoziZA==",
+ "dev": true,
+ "engines": {
+ "node": ">= 14.16"
+ }
},
"node_modules/pbkdf2": {
"version": "3.1.2",
@@ -40489,17 +37687,6 @@
"node": ">=0.12"
}
},
- "node_modules/peek-stream": {
- "version": "1.1.3",
- "resolved": "https://registry.npmjs.org/peek-stream/-/peek-stream-1.1.3.tgz",
- "integrity": "sha512-FhJ+YbOSBb9/rIl2ZeE/QHEsWn7PqNYt8ARAY3kIgNGOk13g9FGyIY6JIl/xB/3TFRVoTv5as0l11weORrTekA==",
- "dev": true,
- "dependencies": {
- "buffer-from": "^1.0.0",
- "duplexify": "^3.5.0",
- "through2": "^2.0.3"
- }
- },
"node_modules/pegjs": {
"version": "0.10.0",
"resolved": "https://registry.npmjs.org/pegjs/-/pegjs-0.10.0.tgz",
@@ -40554,11 +37741,16 @@
}
},
"node_modules/pify": {
- "version": "2.3.0",
- "resolved": "https://registry.npmjs.org/pify/-/pify-2.3.0.tgz",
- "integrity": "sha512-udgsAY+fTnvv7kI7aaxbqwWNb0AHiB0qBO89PZKPkoTmGOgdbrHDKD+0B2X4uTfJ/FT1R09r9gTsjUjNJotuog==",
+ "version": "5.0.0",
+ "resolved": "https://registry.npmjs.org/pify/-/pify-5.0.0.tgz",
+ "integrity": "sha512-eW/gHNMlxdSP6dmG6uJip6FXN0EQBwm2clYYd8Wul42Cwu/DK8HEftzsapcNdYe2MfLiIwZqsDk2RDEsTE79hA==",
+ "dev": true,
+ "license": "MIT",
"engines": {
- "node": ">=0.10.0"
+ "node": ">=10"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/sindresorhus"
}
},
"node_modules/pinkie": {
@@ -40836,27 +38028,6 @@
"postcss": "^8.2.15"
}
},
- "node_modules/postcss-import": {
- "version": "16.1.0",
- "resolved": "https://registry.npmjs.org/postcss-import/-/postcss-import-16.1.0.tgz",
- "integrity": "sha512-7hsAZ4xGXl4MW+OKEWCnF6T5jqBw80/EE9aXg1r2yyn1RsVEU8EtKXbijEODa+rg7iih4bKf7vlvTGYR4CnPNg==",
- "dependencies": {
- "postcss-value-parser": "^4.0.0",
- "read-cache": "^1.0.0",
- "resolve": "^1.1.7"
- },
- "engines": {
- "node": ">=18.0.0"
- },
- "peerDependencies": {
- "postcss": "^8.0.0"
- }
- },
- "node_modules/postcss-import/node_modules/postcss-value-parser": {
- "version": "4.2.0",
- "resolved": "https://registry.npmjs.org/postcss-value-parser/-/postcss-value-parser-4.2.0.tgz",
- "integrity": "sha512-1NNCs6uurfkVbeXG4S8JFT9t19m45ICnif8zWLd5oPSZ50QnwMfK+H3jv408d4jw/7Bttv5axS5IiHoLaVNHeQ=="
- },
"node_modules/postcss-loader": {
"version": "6.2.1",
"resolved": "https://registry.npmjs.org/postcss-loader/-/postcss-loader-6.2.1.tgz",
@@ -41420,10 +38591,11 @@
}
},
"node_modules/prebuild-install": {
- "version": "7.1.1",
- "resolved": "https://registry.npmjs.org/prebuild-install/-/prebuild-install-7.1.1.tgz",
- "integrity": "sha512-jAXscXWMcCK8GgCoHOfIr0ODh5ai8mj63L2nWrjuAgXE6tDyYGnx4/8o/rCgU+B4JSyZBKbeZqzhtwtC3ovxjw==",
+ "version": "7.1.2",
+ "resolved": "https://registry.npmjs.org/prebuild-install/-/prebuild-install-7.1.2.tgz",
+ "integrity": "sha512-UnNke3IQb6sgarcZIDU3gbMeTp/9SSU1DAIkil7PrqG1vZlBtY5msYccSKSHDqa3hNg436IXK+SNImReuA1wEQ==",
"dev": true,
+ "license": "MIT",
"optional": true,
"dependencies": {
"detect-libc": "^2.0.0",
@@ -41531,20 +38703,12 @@
"resolved": "https://registry.npmjs.org/react-is/-/react-is-18.3.1.tgz",
"integrity": "sha512-/LLMVyas0ljjAtoYiPqYiL8VWXzUUdThrmU5+n20DZv+a+ClRoevUzw5JxU+Ieh5/c87ytoTBV9G1FiKfNJdmg=="
},
- "node_modules/pretty-hrtime": {
- "version": "1.0.3",
- "resolved": "https://registry.npmjs.org/pretty-hrtime/-/pretty-hrtime-1.0.3.tgz",
- "integrity": "sha512-66hKPCr+72mlfiSjlEB1+45IjXSqvVAIy6mocupoww4tBFE9R9IhwwUGoI4G++Tc9Aq+2rxOt0RFU6gPcrte0A==",
- "dev": true,
- "engines": {
- "node": ">= 0.8"
- }
- },
"node_modules/proc-log": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/proc-log/-/proc-log-3.0.0.tgz",
- "integrity": "sha512-++Vn7NS4Xf9NacaU9Xq3URUuqZETPsf8L4j5/ckhaRYsfPeRyzGw+iDjFhV/Jr3uNmTvvddEJFWh5R1gRgUH8A==",
+ "version": "4.2.0",
+ "resolved": "https://registry.npmjs.org/proc-log/-/proc-log-4.2.0.tgz",
+ "integrity": "sha512-g8+OnU/L2v+wyiVK+D5fA34J7EH8jZ8DDlvwhRCMxmMj7UCBvxiO1mGeN+36JXIKF4zevU4kRBd8lVgG9vLelA==",
"dev": true,
+ "license": "ISC",
"engines": {
"node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
@@ -41563,6 +38727,16 @@
"resolved": "https://registry.npmjs.org/process-nextick-args/-/process-nextick-args-2.0.1.tgz",
"integrity": "sha512-3ouUOpQhtgrbOa17J7+uxOTpITYWaGP7/AhoR3+A+/1e9skrzelGi/dXzEYyvbxubEF6Wn2ypscTKiKJFFn1ag=="
},
+ "node_modules/proggy": {
+ "version": "2.0.0",
+ "resolved": "https://registry.npmjs.org/proggy/-/proggy-2.0.0.tgz",
+ "integrity": "sha512-69agxLtnI8xBs9gUGqEnK26UfiexpHy+KUpBQWabiytQjnn5wFY8rklAi7GRfABIuPNnQ/ik48+LGLkYYJcy4A==",
+ "dev": true,
+ "license": "ISC",
+ "engines": {
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ }
+ },
"node_modules/progress": {
"version": "2.0.3",
"resolved": "https://registry.npmjs.org/progress/-/progress-2.0.3.tgz",
@@ -41579,6 +38753,26 @@
"asap": "~2.0.6"
}
},
+ "node_modules/promise-all-reject-late": {
+ "version": "1.0.1",
+ "resolved": "https://registry.npmjs.org/promise-all-reject-late/-/promise-all-reject-late-1.0.1.tgz",
+ "integrity": "sha512-vuf0Lf0lOxyQREH7GDIOUMLS7kz+gs8i6B+Yi8dC68a2sychGrHTJYghMBD6k7eUcH0H5P73EckCA48xijWqXw==",
+ "dev": true,
+ "license": "ISC",
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
+ }
+ },
+ "node_modules/promise-call-limit": {
+ "version": "3.0.2",
+ "resolved": "https://registry.npmjs.org/promise-call-limit/-/promise-call-limit-3.0.2.tgz",
+ "integrity": "sha512-mRPQO2T1QQVw11E7+UdCJu7S61eJVWknzml9sC1heAdj1jxl0fWMBypIt9ZOcLFf8FkG995ZD7RnVk7HH72fZw==",
+ "dev": true,
+ "license": "ISC",
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
+ }
+ },
"node_modules/promise-inflight": {
"version": "1.0.1",
"resolved": "https://registry.npmjs.org/promise-inflight/-/promise-inflight-1.0.1.tgz",
@@ -41590,6 +38784,7 @@
"resolved": "https://registry.npmjs.org/promise-retry/-/promise-retry-2.0.1.tgz",
"integrity": "sha512-y+WKFlBR8BGXnsNlIHFGPZmyDf3DFMoLhaflAnyZgV6rG6xu+JwesTo2Q9R6XwYmtmwAFCkAk3e35jEdoeh/3g==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"err-code": "^2.0.2",
"retry": "^0.12.0"
@@ -41611,12 +38806,13 @@
}
},
"node_modules/promzard": {
- "version": "1.0.0",
- "resolved": "https://registry.npmjs.org/promzard/-/promzard-1.0.0.tgz",
- "integrity": "sha512-KQVDEubSUHGSt5xLakaToDFrSoZhStB8dXLzk2xvwR67gJktrHFvpR63oZgHyK19WKbHFLXJqCPXdVR3aBP8Ig==",
+ "version": "1.0.2",
+ "resolved": "https://registry.npmjs.org/promzard/-/promzard-1.0.2.tgz",
+ "integrity": "sha512-2FPputGL+mP3jJ3UZg/Dl9YOkovB7DX0oOr+ck5QbZ5MtORtds8k/BZdn+02peDLI8/YWbmzx34k5fA+fHvCVQ==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "read": "^2.0.0"
+ "read": "^3.0.1"
},
"engines": {
"node": "^14.17.0 || ^16.13.0 || >=18.0.0"
@@ -41645,7 +38841,8 @@
"version": "2.0.1",
"resolved": "https://registry.npmjs.org/protocols/-/protocols-2.0.1.tgz",
"integrity": "sha512-/XJ368cyBJ7fzLMwLKv1e4vLxOju2MNAIokcr7meSaNcVbWz/CPcW22cP04mwxOErdA5mwjA8Q6w/cdAQxVn7Q==",
- "dev": true
+ "dev": true,
+ "license": "MIT"
},
"node_modules/proxy-addr": {
"version": "2.0.7",
@@ -41660,54 +38857,20 @@
}
},
"node_modules/proxy-agent": {
- "version": "6.3.0",
- "resolved": "https://registry.npmjs.org/proxy-agent/-/proxy-agent-6.3.0.tgz",
- "integrity": "sha512-0LdR757eTj/JfuU7TL2YCuAZnxWXu3tkJbg4Oq3geW/qFNT/32T0sp2HnZ9O0lMR4q3vwAt0+xCA8SR0WAD0og==",
+ "version": "6.3.1",
+ "resolved": "https://registry.npmjs.org/proxy-agent/-/proxy-agent-6.3.1.tgz",
+ "integrity": "sha512-Rb5RVBy1iyqOtNl15Cw/llpeLH8bsb37gM1FUfKQ+Wck6xHlbAhWGUFiTRHtkjqGTA5pSHz6+0hrPW/oECihPQ==",
+ "dev": true,
+ "license": "MIT",
"dependencies": {
"agent-base": "^7.0.2",
"debug": "^4.3.4",
"http-proxy-agent": "^7.0.0",
- "https-proxy-agent": "^7.0.0",
+ "https-proxy-agent": "^7.0.2",
"lru-cache": "^7.14.1",
- "pac-proxy-agent": "^7.0.0",
+ "pac-proxy-agent": "^7.0.1",
"proxy-from-env": "^1.1.0",
- "socks-proxy-agent": "^8.0.1"
- },
- "engines": {
- "node": ">= 14"
- }
- },
- "node_modules/proxy-agent/node_modules/agent-base": {
- "version": "7.1.1",
- "resolved": "https://registry.npmjs.org/agent-base/-/agent-base-7.1.1.tgz",
- "integrity": "sha512-H0TSyFNDMomMNJQBn8wFV5YC/2eJ+VXECwOadZJT554xP6cODZHPX3H9QMQECxvrgiSOP1pHjy1sMWQVYJOUOA==",
- "dependencies": {
- "debug": "^4.3.4"
- },
- "engines": {
- "node": ">= 14"
- }
- },
- "node_modules/proxy-agent/node_modules/http-proxy-agent": {
- "version": "7.0.2",
- "resolved": "https://registry.npmjs.org/http-proxy-agent/-/http-proxy-agent-7.0.2.tgz",
- "integrity": "sha512-T1gkAiYYDWYx3V5Bmyu7HcfcvL7mUrTWiM6yOfa3PIphViJ/gFPbvidQ+veqSOHci/PxBcDabeUNCzpOODJZig==",
- "dependencies": {
- "agent-base": "^7.1.0",
- "debug": "^4.3.4"
- },
- "engines": {
- "node": ">= 14"
- }
- },
- "node_modules/proxy-agent/node_modules/https-proxy-agent": {
- "version": "7.0.5",
- "resolved": "https://registry.npmjs.org/https-proxy-agent/-/https-proxy-agent-7.0.5.tgz",
- "integrity": "sha512-1e4Wqeblerz+tMKPIq2EMGiiWW1dIjZOksyHWSUm1rmuvw/how9hBHZ38lAGj5ID4Ik6EdkOw7NmWPy6LAwalw==",
- "license": "MIT",
- "dependencies": {
- "agent-base": "^7.0.2",
- "debug": "4"
+ "socks-proxy-agent": "^8.0.2"
},
"engines": {
"node": ">= 14"
@@ -41717,24 +38880,12 @@
"version": "7.18.3",
"resolved": "https://registry.npmjs.org/lru-cache/-/lru-cache-7.18.3.tgz",
"integrity": "sha512-jumlc0BIUrS3qJGgIkWZsyfAM7NCWiBcCDhnd+3NNM5KbBmLTgHVfWBcg6W+rLUsIpzpERPsvwUP7CckAQSOoA==",
+ "dev": true,
+ "license": "ISC",
"engines": {
"node": ">=12"
}
},
- "node_modules/proxy-agent/node_modules/socks-proxy-agent": {
- "version": "8.0.4",
- "resolved": "https://registry.npmjs.org/socks-proxy-agent/-/socks-proxy-agent-8.0.4.tgz",
- "integrity": "sha512-GNAq/eg8Udq2x0eNiFkr9gRg5bA7PXEWagQdeRX4cPSG+X/8V38v637gim9bjFptMk1QWsCTr0ttrJEiXbNnRw==",
- "license": "MIT",
- "dependencies": {
- "agent-base": "^7.1.1",
- "debug": "^4.3.4",
- "socks": "^2.8.3"
- },
- "engines": {
- "node": ">= 14"
- }
- },
"node_modules/proxy-from-env": {
"version": "1.1.0",
"resolved": "https://registry.npmjs.org/proxy-from-env/-/proxy-from-env-1.1.0.tgz",
@@ -41746,22 +38897,6 @@
"integrity": "sha512-yPw4Sng1gWghHQWj0B3ZggWUm4qVbPwPFcRG8KyxiU7J2OHFSoEHKS+EZ3fv5l1t9CyCiop6l/ZYeWbrgoQejw==",
"dev": true
},
- "node_modules/ps-list": {
- "version": "8.1.1",
- "resolved": "https://registry.npmjs.org/ps-list/-/ps-list-8.1.1.tgz",
- "integrity": "sha512-OPS9kEJYVmiO48u/B9qneqhkMvgCxT+Tm28VCEJpheTpl8cJ0ffZRRNgS5mrQRTrX5yRTpaJ+hRDeefXYmmorQ==",
- "engines": {
- "node": "^12.20.0 || ^14.13.1 || >=16.0.0"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/pseudomap": {
- "version": "1.0.2",
- "resolved": "https://registry.npmjs.org/pseudomap/-/pseudomap-1.0.2.tgz",
- "integrity": "sha512-b/YwNhb8lk1Zz2+bXXpS/LK9OisiZZ1SNsSLxN1x2OXVEhW2Ckr/7mWE5vrC1ZTiJlD9g19jWszTmJsB+oEpFQ=="
- },
"node_modules/public-encrypt": {
"version": "4.0.3",
"resolved": "https://registry.npmjs.org/public-encrypt/-/public-encrypt-4.0.3.tgz",
@@ -41812,15 +38947,15 @@
}
},
"node_modules/puppeteer-core": {
- "version": "23.1.0",
- "resolved": "https://registry.npmjs.org/puppeteer-core/-/puppeteer-core-23.1.0.tgz",
- "integrity": "sha512-SvAsu+xnLN2FMXE/59bp3s3WXp8ewqUGzVV4AQtml/2xmsciZnU/bXcCW+eETHPWQ6Agg2vTI7QzWXPpEARK2g==",
+ "version": "23.10.1",
+ "resolved": "https://registry.npmjs.org/puppeteer-core/-/puppeteer-core-23.10.1.tgz",
+ "integrity": "sha512-ey6NwixHYEUnhCA/uYi7uQQ4a0CZw4k+MatbHXGl5GEzaiRQziYUxc2HGpdQZ/gnh4KQWAKkocyIg1/dIm5d0g==",
"license": "Apache-2.0",
"dependencies": {
- "@puppeteer/browsers": "2.3.1",
- "chromium-bidi": "0.6.4",
- "debug": "^4.3.6",
- "devtools-protocol": "0.0.1312386",
+ "@puppeteer/browsers": "2.5.0",
+ "chromium-bidi": "0.8.0",
+ "debug": "^4.3.7",
+ "devtools-protocol": "0.0.1367902",
"typed-query-selector": "^2.12.0",
"ws": "^8.18.0"
},
@@ -41829,12 +38964,12 @@
}
},
"node_modules/puppeteer-core/node_modules/@puppeteer/browsers": {
- "version": "2.3.1",
- "resolved": "https://registry.npmjs.org/@puppeteer/browsers/-/browsers-2.3.1.tgz",
- "integrity": "sha512-uK7o3hHkK+naEobMSJ+2ySYyXtQkBxIH8Gn4MK9ciePjNV+Pf+PgY/W7iPzn2MTjl3stcYB5AlcTmPYw7AXDwA==",
+ "version": "2.5.0",
+ "resolved": "https://registry.npmjs.org/@puppeteer/browsers/-/browsers-2.5.0.tgz",
+ "integrity": "sha512-6TQAc/5uRILE6deixJ1CR8rXyTbzXIXNgO1D0Woi9Bqicz2FV5iKP3BHYEg6o4UATCMcbQQ0jbmeaOkn/HQk2w==",
"license": "Apache-2.0",
"dependencies": {
- "debug": "^4.3.6",
+ "debug": "^4.3.7",
"extract-zip": "^2.0.1",
"progress": "^2.0.3",
"proxy-agent": "^6.4.0",
@@ -41850,22 +38985,10 @@
"node": ">=18"
}
},
- "node_modules/puppeteer-core/node_modules/agent-base": {
- "version": "7.1.1",
- "resolved": "https://registry.npmjs.org/agent-base/-/agent-base-7.1.1.tgz",
- "integrity": "sha512-H0TSyFNDMomMNJQBn8wFV5YC/2eJ+VXECwOadZJT554xP6cODZHPX3H9QMQECxvrgiSOP1pHjy1sMWQVYJOUOA==",
- "license": "MIT",
- "dependencies": {
- "debug": "^4.3.4"
- },
- "engines": {
- "node": ">= 14"
- }
- },
"node_modules/puppeteer-core/node_modules/chromium-bidi": {
- "version": "0.6.4",
- "resolved": "https://registry.npmjs.org/chromium-bidi/-/chromium-bidi-0.6.4.tgz",
- "integrity": "sha512-8zoq6ogmhQQkAKZVKO2ObFTl4uOkqoX1PlKQX3hZQ5E9cbUotcAb7h4pTNVAGGv8Z36PF3CtdOriEp/Rz82JqQ==",
+ "version": "0.8.0",
+ "resolved": "https://registry.npmjs.org/chromium-bidi/-/chromium-bidi-0.8.0.tgz",
+ "integrity": "sha512-uJydbGdTw0DEUjhoogGveneJVWX/9YuqkWePzMmkBYwtdAqo5d3J/ovNKFr+/2hWXYmYCr6it8mSSTIj6SS6Ug==",
"license": "Apache-2.0",
"dependencies": {
"mitt": "3.0.1",
@@ -41876,24 +38999,10 @@
"devtools-protocol": "*"
}
},
- "node_modules/puppeteer-core/node_modules/cliui": {
- "version": "8.0.1",
- "resolved": "https://registry.npmjs.org/cliui/-/cliui-8.0.1.tgz",
- "integrity": "sha512-BSeNnyus75C4//NQ9gQt1/csTXyo/8Sb+afLAkzAptFuMsod9HFokGNudZpi/oQV73hnVK+sR+5PVRMd+Dr7YQ==",
- "license": "ISC",
- "dependencies": {
- "string-width": "^4.2.0",
- "strip-ansi": "^6.0.1",
- "wrap-ansi": "^7.0.0"
- },
- "engines": {
- "node": ">=12"
- }
- },
"node_modules/puppeteer-core/node_modules/debug": {
- "version": "4.3.7",
- "resolved": "https://registry.npmjs.org/debug/-/debug-4.3.7.tgz",
- "integrity": "sha512-Er2nc/H7RrMXZBFCEim6TCmMk02Z8vLC2Rbi1KEBggpo0fS6l0S1nnapwmIi3yW/+GOJap1Krg4w0Hg80oCqgQ==",
+ "version": "4.4.0",
+ "resolved": "https://registry.npmjs.org/debug/-/debug-4.4.0.tgz",
+ "integrity": "sha512-6WTZ/IxCY/T6BALoZHaE4ctp9xm+Z5kY/pzYaCHRFeyVhojxlrm+46y68HA6hr0TcwEssoxNiDEUJQjfPZ/RYA==",
"license": "MIT",
"dependencies": {
"ms": "^2.1.3"
@@ -41907,12 +39016,6 @@
}
}
},
- "node_modules/puppeteer-core/node_modules/emoji-regex": {
- "version": "8.0.0",
- "resolved": "https://registry.npmjs.org/emoji-regex/-/emoji-regex-8.0.0.tgz",
- "integrity": "sha512-MSjYzcWNOA0ewAHpz0MxpYFvwg6yjy1NG3xteoqz644VCo/RPgnr1/GGt+ic3iJTzQ8Eu3TdM14SawnVUmGE6A==",
- "license": "MIT"
- },
"node_modules/puppeteer-core/node_modules/extract-zip": {
"version": "2.0.1",
"resolved": "https://registry.npmjs.org/extract-zip/-/extract-zip-2.0.1.tgz",
@@ -41933,41 +39036,6 @@
"@types/yauzl": "^2.9.1"
}
},
- "node_modules/puppeteer-core/node_modules/http-proxy-agent": {
- "version": "7.0.2",
- "resolved": "https://registry.npmjs.org/http-proxy-agent/-/http-proxy-agent-7.0.2.tgz",
- "integrity": "sha512-T1gkAiYYDWYx3V5Bmyu7HcfcvL7mUrTWiM6yOfa3PIphViJ/gFPbvidQ+veqSOHci/PxBcDabeUNCzpOODJZig==",
- "license": "MIT",
- "dependencies": {
- "agent-base": "^7.1.0",
- "debug": "^4.3.4"
- },
- "engines": {
- "node": ">= 14"
- }
- },
- "node_modules/puppeteer-core/node_modules/https-proxy-agent": {
- "version": "7.0.5",
- "resolved": "https://registry.npmjs.org/https-proxy-agent/-/https-proxy-agent-7.0.5.tgz",
- "integrity": "sha512-1e4Wqeblerz+tMKPIq2EMGiiWW1dIjZOksyHWSUm1rmuvw/how9hBHZ38lAGj5ID4Ik6EdkOw7NmWPy6LAwalw==",
- "license": "MIT",
- "dependencies": {
- "agent-base": "^7.0.2",
- "debug": "4"
- },
- "engines": {
- "node": ">= 14"
- }
- },
- "node_modules/puppeteer-core/node_modules/is-fullwidth-code-point": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/is-fullwidth-code-point/-/is-fullwidth-code-point-3.0.0.tgz",
- "integrity": "sha512-zymm5+u+sCsSWyD9qNaejV3DFvhCKclKdizYaJUuHA83RLjb7nSuGnddCHGv0hk+KY7BMAlsWeK4Ueg6EV6XQg==",
- "license": "MIT",
- "engines": {
- "node": ">=8"
- }
- },
"node_modules/puppeteer-core/node_modules/lru-cache": {
"version": "7.18.3",
"resolved": "https://registry.npmjs.org/lru-cache/-/lru-cache-7.18.3.tgz",
@@ -41977,12 +39045,6 @@
"node": ">=12"
}
},
- "node_modules/puppeteer-core/node_modules/mitt": {
- "version": "3.0.1",
- "resolved": "https://registry.npmjs.org/mitt/-/mitt-3.0.1.tgz",
- "integrity": "sha512-vKivATfr97l2/QBCYAkXYDbrIWPM2IIKEl7YPhjCvKlG3kE2gm+uBo6nEXK3M5/Ffh/FLpKExzOQ3JJoJGFKBw==",
- "license": "MIT"
- },
"node_modules/puppeteer-core/node_modules/ms": {
"version": "2.1.3",
"resolved": "https://registry.npmjs.org/ms/-/ms-2.1.3.tgz",
@@ -41990,19 +39052,19 @@
"license": "MIT"
},
"node_modules/puppeteer-core/node_modules/proxy-agent": {
- "version": "6.4.0",
- "resolved": "https://registry.npmjs.org/proxy-agent/-/proxy-agent-6.4.0.tgz",
- "integrity": "sha512-u0piLU+nCOHMgGjRbimiXmA9kM/L9EHh3zL81xCdp7m+Y2pHIsnmbdDoEDoAz5geaonNR6q6+yOPQs6n4T6sBQ==",
+ "version": "6.5.0",
+ "resolved": "https://registry.npmjs.org/proxy-agent/-/proxy-agent-6.5.0.tgz",
+ "integrity": "sha512-TmatMXdr2KlRiA2CyDu8GqR8EjahTG3aY3nXjdzFyoZbmB8hrBsTyMezhULIXKnC0jpfjlmiZ3+EaCzoInSu/A==",
"license": "MIT",
"dependencies": {
- "agent-base": "^7.0.2",
+ "agent-base": "^7.1.2",
"debug": "^4.3.4",
"http-proxy-agent": "^7.0.1",
- "https-proxy-agent": "^7.0.3",
+ "https-proxy-agent": "^7.0.6",
"lru-cache": "^7.14.1",
- "pac-proxy-agent": "^7.0.1",
+ "pac-proxy-agent": "^7.1.0",
"proxy-from-env": "^1.1.0",
- "socks-proxy-agent": "^8.0.2"
+ "socks-proxy-agent": "^8.0.5"
},
"engines": {
"node": ">= 14"
@@ -42030,34 +39092,6 @@
"node": ">=10"
}
},
- "node_modules/puppeteer-core/node_modules/socks-proxy-agent": {
- "version": "8.0.4",
- "resolved": "https://registry.npmjs.org/socks-proxy-agent/-/socks-proxy-agent-8.0.4.tgz",
- "integrity": "sha512-GNAq/eg8Udq2x0eNiFkr9gRg5bA7PXEWagQdeRX4cPSG+X/8V38v637gim9bjFptMk1QWsCTr0ttrJEiXbNnRw==",
- "license": "MIT",
- "dependencies": {
- "agent-base": "^7.1.1",
- "debug": "^4.3.4",
- "socks": "^2.8.3"
- },
- "engines": {
- "node": ">= 14"
- }
- },
- "node_modules/puppeteer-core/node_modules/string-width": {
- "version": "4.2.3",
- "resolved": "https://registry.npmjs.org/string-width/-/string-width-4.2.3.tgz",
- "integrity": "sha512-wKyQRQpjJ0sIp62ErSZdGsjMJWsap5oRNihHhu6G7JVO/9jIB6UyevL+tXuOqrng8j/cxKTWyWUwvSTriiZz/g==",
- "license": "MIT",
- "dependencies": {
- "emoji-regex": "^8.0.0",
- "is-fullwidth-code-point": "^3.0.0",
- "strip-ansi": "^6.0.1"
- },
- "engines": {
- "node": ">=8"
- }
- },
"node_modules/puppeteer-core/node_modules/tar-fs": {
"version": "3.0.6",
"resolved": "https://registry.npmjs.org/tar-fs/-/tar-fs-3.0.6.tgz",
@@ -42083,23 +39117,6 @@
"streamx": "^2.15.0"
}
},
- "node_modules/puppeteer-core/node_modules/wrap-ansi": {
- "version": "7.0.0",
- "resolved": "https://registry.npmjs.org/wrap-ansi/-/wrap-ansi-7.0.0.tgz",
- "integrity": "sha512-YVGIj2kamLSTxw6NsZjoBxfSwsn0ycdesmc4p+Q21c5zPuZ1pl+NfxVdxPtdHvmNVOQ6XSYG4AUtyt/Fi7D16Q==",
- "license": "MIT",
- "dependencies": {
- "ansi-styles": "^4.0.0",
- "string-width": "^4.1.0",
- "strip-ansi": "^6.0.0"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/chalk/wrap-ansi?sponsor=1"
- }
- },
"node_modules/puppeteer-core/node_modules/ws": {
"version": "8.18.0",
"resolved": "https://registry.npmjs.org/ws/-/ws-8.18.0.tgz",
@@ -42121,42 +39138,6 @@
}
}
},
- "node_modules/puppeteer-core/node_modules/y18n": {
- "version": "5.0.8",
- "resolved": "https://registry.npmjs.org/y18n/-/y18n-5.0.8.tgz",
- "integrity": "sha512-0pfFzegeDWJHJIAmTLRP2DwHjdF5s7jo9tuztdQxAhINCdvS+3nGINqPd00AphqJR/0LhANUS6/+7SCb98YOfA==",
- "license": "ISC",
- "engines": {
- "node": ">=10"
- }
- },
- "node_modules/puppeteer-core/node_modules/yargs": {
- "version": "17.7.2",
- "resolved": "https://registry.npmjs.org/yargs/-/yargs-17.7.2.tgz",
- "integrity": "sha512-7dSzzRQ++CKnNI/krKnYRV7JKKPUXMEh61soaHKg9mrWEhzFWhFnxPxGl+69cD1Ou63C13NUPCnmIcrvqCuM6w==",
- "license": "MIT",
- "dependencies": {
- "cliui": "^8.0.1",
- "escalade": "^3.1.1",
- "get-caller-file": "^2.0.5",
- "require-directory": "^2.1.1",
- "string-width": "^4.2.3",
- "y18n": "^5.0.5",
- "yargs-parser": "^21.1.1"
- },
- "engines": {
- "node": ">=12"
- }
- },
- "node_modules/puppeteer-core/node_modules/yargs-parser": {
- "version": "21.1.1",
- "resolved": "https://registry.npmjs.org/yargs-parser/-/yargs-parser-21.1.1.tgz",
- "integrity": "sha512-tVpsJW7DdjecAiFpbIB1e3qxIQsE6NoPc5/eTdrbbIC4h0LVsWhnoa3g+m2HclBIujHzsxZ4VJVA+GUuc2/LBw==",
- "license": "ISC",
- "engines": {
- "node": ">=12"
- }
- },
"node_modules/pure-rand": {
"version": "6.0.2",
"resolved": "https://registry.npmjs.org/pure-rand/-/pure-rand-6.0.2.tgz",
@@ -42173,11 +39154,13 @@
]
},
"node_modules/qs": {
- "version": "6.11.0",
- "resolved": "https://registry.npmjs.org/qs/-/qs-6.11.0.tgz",
- "integrity": "sha512-MvjoMCJwEarSbUYk5O+nmoSzSutSsTwF85zcHPQ9OrlFoZOYIjaqBAJIqIXjptyD5vThxGq52Xu/MaJzRkIk4Q==",
+ "version": "6.13.0",
+ "resolved": "https://registry.npmjs.org/qs/-/qs-6.13.0.tgz",
+ "integrity": "sha512-+38qI9SOr8tfZ4QmJNplMUxqjbe7LKvvZgWdExBOmd+egZTtjLB67Gu0HRX3u/XOq7UU2Nx6nsjvS16Z9uwfpg==",
+ "dev": true,
+ "license": "BSD-3-Clause",
"dependencies": {
- "side-channel": "^1.0.4"
+ "side-channel": "^1.0.6"
},
"engines": {
"node": ">=0.6"
@@ -42267,16 +39250,6 @@
"node": ">=8"
}
},
- "node_modules/ramda": {
- "version": "0.29.0",
- "resolved": "https://registry.npmjs.org/ramda/-/ramda-0.29.0.tgz",
- "integrity": "sha512-BBea6L67bYLtdbOqfp8f58fPMqEwx0doL+pAi8TZyp2YWz8R9G8z9x75CZI8W+ftqhFHCpEX2cRnUUXK130iKA==",
- "dev": true,
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/ramda"
- }
- },
"node_modules/randombytes": {
"version": "2.1.0",
"resolved": "https://registry.npmjs.org/randombytes/-/randombytes-2.1.0.tgz",
@@ -42307,6 +39280,7 @@
"version": "2.5.1",
"resolved": "https://registry.npmjs.org/raw-body/-/raw-body-2.5.1.tgz",
"integrity": "sha512-qqJBtEyVgS0ZmPGdCFPWJ3FreoqvG4MVQln/kCgF7Olq95IbOp0/BWyMwbdtn4VTvkM8Y7khCQ2Xgk/tcrCXig==",
+ "license": "MIT",
"dependencies": {
"bytes": "3.1.2",
"http-errors": "2.0.0",
@@ -42321,6 +39295,7 @@
"version": "3.1.2",
"resolved": "https://registry.npmjs.org/bytes/-/bytes-3.1.2.tgz",
"integrity": "sha512-/Nf7TyzTx6S3yRJObOAV7956r8cr2+Oj8AC5dt8wSP3BQAoeX58NoHyCU8P8zGkNXStjTSi6fzO6F0pBdcYbEg==",
+ "license": "MIT",
"engines": {
"node": ">= 0.8"
}
@@ -42329,6 +39304,7 @@
"version": "0.4.24",
"resolved": "https://registry.npmjs.org/iconv-lite/-/iconv-lite-0.4.24.tgz",
"integrity": "sha512-v3MXnZAcvnywkTUEZomIActle7RXXeedOR31wwl7VlyoXO4Qi9arvSenNQWne1TcRwhCL1HwLI21bEqdpj8/rA==",
+ "license": "MIT",
"dependencies": {
"safer-buffer": ">= 2.1.2 < 3"
},
@@ -42398,6 +39374,7 @@
"resolved": "https://registry.npmjs.org/rc/-/rc-1.2.8.tgz",
"integrity": "sha512-y3bGgqKj3QBdxLbLkomlohkvsA8gdAiUQlSBJnBhfn+BPxg4bc62d8TcBW15wavDfgexCgccckhcZvywyQYPOw==",
"dev": true,
+ "license": "(BSD-2-Clause OR MIT OR Apache-2.0)",
"optional": true,
"dependencies": {
"deep-extend": "^0.6.0",
@@ -42414,6 +39391,7 @@
"resolved": "https://registry.npmjs.org/strip-json-comments/-/strip-json-comments-2.0.1.tgz",
"integrity": "sha512-4gB8na07fecVVkOI6Rs4e7T6NOTki5EmL7TUduTs6bu3EdnSycntVJ4re8kgZA+wx9IueI2Y11bfbgwtzuE0KQ==",
"dev": true,
+ "license": "MIT",
"optional": true,
"engines": {
"node": ">=0.10.0"
@@ -42475,9 +39453,9 @@
}
},
"node_modules/react-docgen": {
- "version": "7.0.3",
- "resolved": "https://registry.npmjs.org/react-docgen/-/react-docgen-7.0.3.tgz",
- "integrity": "sha512-i8aF1nyKInZnANZ4uZrH49qn1paRgBZ7wZiCNBMnenlPzEv0mRl+ShpTVEI6wZNl8sSc79xZkivtgLKQArcanQ==",
+ "version": "7.1.0",
+ "resolved": "https://registry.npmjs.org/react-docgen/-/react-docgen-7.1.0.tgz",
+ "integrity": "sha512-APPU8HB2uZnpl6Vt/+0AFoVYgSRtfiP6FLrZgPPTDmqSb2R4qZRbgd0A3VzIFxDt5e+Fozjx79WjLWnF69DK8g==",
"dev": true,
"dependencies": {
"@babel/core": "^7.18.9",
@@ -42505,12 +39483,6 @@
"typescript": ">= 4.3.x"
}
},
- "node_modules/react-docgen/node_modules/@types/doctrine": {
- "version": "0.0.9",
- "resolved": "https://registry.npmjs.org/@types/doctrine/-/doctrine-0.0.9.tgz",
- "integrity": "sha512-eOIHzCUSH7SMfonMG1LsC2f8vxBFtho6NGBznK41R84YzPuvSBzrhEps33IsQiOW9+VL6NQ9DbjQJznk/S4uRA==",
- "dev": true
- },
"node_modules/react-docgen/node_modules/doctrine": {
"version": "3.0.0",
"resolved": "https://registry.npmjs.org/doctrine/-/doctrine-3.0.0.tgz",
@@ -42550,27 +39522,6 @@
"react": "^18.3.1"
}
},
- "node_modules/react-element-to-jsx-string": {
- "version": "15.0.0",
- "resolved": "https://registry.npmjs.org/react-element-to-jsx-string/-/react-element-to-jsx-string-15.0.0.tgz",
- "integrity": "sha512-UDg4lXB6BzlobN60P8fHWVPX3Kyw8ORrTeBtClmIlGdkOOE+GYQSFvmEU5iLLpwp/6v42DINwNcwOhOLfQ//FQ==",
- "dev": true,
- "dependencies": {
- "@base2/pretty-print-object": "1.0.1",
- "is-plain-object": "5.0.0",
- "react-is": "18.1.0"
- },
- "peerDependencies": {
- "react": "^0.14.8 || ^15.0.1 || ^16.0.0 || ^17.0.1 || ^18.0.0",
- "react-dom": "^0.14.8 || ^15.0.1 || ^16.0.0 || ^17.0.1 || ^18.0.0"
- }
- },
- "node_modules/react-element-to-jsx-string/node_modules/react-is": {
- "version": "18.1.0",
- "resolved": "https://registry.npmjs.org/react-is/-/react-is-18.1.0.tgz",
- "integrity": "sha512-Fl7FuabXsJnV5Q1qIOQwx/sagGF18kogb4gpfcG4gjLBWO0WDiiz1ko/ExayuxE7InyQkBLkxRFG5oxY6Uu3Kg==",
- "dev": true
- },
"node_modules/react-freeze": {
"version": "1.0.3",
"resolved": "https://registry.npmjs.org/react-freeze/-/react-freeze-1.0.3.tgz",
@@ -42899,19 +39850,6 @@
"@types/yargs-parser": "*"
}
},
- "node_modules/react-native/node_modules/cliui": {
- "version": "8.0.1",
- "resolved": "https://registry.npmjs.org/cliui/-/cliui-8.0.1.tgz",
- "integrity": "sha512-BSeNnyus75C4//NQ9gQt1/csTXyo/8Sb+afLAkzAptFuMsod9HFokGNudZpi/oQV73hnVK+sR+5PVRMd+Dr7YQ==",
- "dependencies": {
- "string-width": "^4.2.0",
- "strip-ansi": "^6.0.1",
- "wrap-ansi": "^7.0.0"
- },
- "engines": {
- "node": ">=12"
- }
- },
"node_modules/react-native/node_modules/deprecated-react-native-prop-types": {
"version": "5.0.0",
"resolved": "https://registry.npmjs.org/deprecated-react-native-prop-types/-/deprecated-react-native-prop-types-5.0.0.tgz",
@@ -42925,19 +39863,6 @@
"node": ">=18"
}
},
- "node_modules/react-native/node_modules/emoji-regex": {
- "version": "8.0.0",
- "resolved": "https://registry.npmjs.org/emoji-regex/-/emoji-regex-8.0.0.tgz",
- "integrity": "sha512-MSjYzcWNOA0ewAHpz0MxpYFvwg6yjy1NG3xteoqz644VCo/RPgnr1/GGt+ic3iJTzQ8Eu3TdM14SawnVUmGE6A=="
- },
- "node_modules/react-native/node_modules/is-fullwidth-code-point": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/is-fullwidth-code-point/-/is-fullwidth-code-point-3.0.0.tgz",
- "integrity": "sha512-zymm5+u+sCsSWyD9qNaejV3DFvhCKclKdizYaJUuHA83RLjb7nSuGnddCHGv0hk+KY7BMAlsWeK4Ueg6EV6XQg==",
- "engines": {
- "node": ">=8"
- }
- },
"node_modules/react-native/node_modules/mkdirp": {
"version": "0.5.6",
"resolved": "https://registry.npmjs.org/mkdirp/-/mkdirp-0.5.6.tgz",
@@ -42976,35 +39901,6 @@
"loose-envify": "^1.1.0"
}
},
- "node_modules/react-native/node_modules/string-width": {
- "version": "4.2.3",
- "resolved": "https://registry.npmjs.org/string-width/-/string-width-4.2.3.tgz",
- "integrity": "sha512-wKyQRQpjJ0sIp62ErSZdGsjMJWsap5oRNihHhu6G7JVO/9jIB6UyevL+tXuOqrng8j/cxKTWyWUwvSTriiZz/g==",
- "dependencies": {
- "emoji-regex": "^8.0.0",
- "is-fullwidth-code-point": "^3.0.0",
- "strip-ansi": "^6.0.1"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/react-native/node_modules/wrap-ansi": {
- "version": "7.0.0",
- "resolved": "https://registry.npmjs.org/wrap-ansi/-/wrap-ansi-7.0.0.tgz",
- "integrity": "sha512-YVGIj2kamLSTxw6NsZjoBxfSwsn0ycdesmc4p+Q21c5zPuZ1pl+NfxVdxPtdHvmNVOQ6XSYG4AUtyt/Fi7D16Q==",
- "dependencies": {
- "ansi-styles": "^4.0.0",
- "string-width": "^4.1.0",
- "strip-ansi": "^6.0.0"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/chalk/wrap-ansi?sponsor=1"
- }
- },
"node_modules/react-native/node_modules/ws": {
"version": "6.2.3",
"resolved": "https://registry.npmjs.org/ws/-/ws-6.2.3.tgz",
@@ -43014,39 +39910,6 @@
"async-limiter": "~1.0.0"
}
},
- "node_modules/react-native/node_modules/y18n": {
- "version": "5.0.8",
- "resolved": "https://registry.npmjs.org/y18n/-/y18n-5.0.8.tgz",
- "integrity": "sha512-0pfFzegeDWJHJIAmTLRP2DwHjdF5s7jo9tuztdQxAhINCdvS+3nGINqPd00AphqJR/0LhANUS6/+7SCb98YOfA==",
- "engines": {
- "node": ">=10"
- }
- },
- "node_modules/react-native/node_modules/yargs": {
- "version": "17.7.2",
- "resolved": "https://registry.npmjs.org/yargs/-/yargs-17.7.2.tgz",
- "integrity": "sha512-7dSzzRQ++CKnNI/krKnYRV7JKKPUXMEh61soaHKg9mrWEhzFWhFnxPxGl+69cD1Ou63C13NUPCnmIcrvqCuM6w==",
- "dependencies": {
- "cliui": "^8.0.1",
- "escalade": "^3.1.1",
- "get-caller-file": "^2.0.5",
- "require-directory": "^2.1.1",
- "string-width": "^4.2.3",
- "y18n": "^5.0.5",
- "yargs-parser": "^21.1.1"
- },
- "engines": {
- "node": ">=12"
- }
- },
- "node_modules/react-native/node_modules/yargs-parser": {
- "version": "21.1.1",
- "resolved": "https://registry.npmjs.org/yargs-parser/-/yargs-parser-21.1.1.tgz",
- "integrity": "sha512-tVpsJW7DdjecAiFpbIB1e3qxIQsE6NoPc5/eTdrbbIC4h0LVsWhnoa3g+m2HclBIujHzsxZ4VJVA+GUuc2/LBw==",
- "engines": {
- "node": ">=12"
- }
- },
"node_modules/react-refresh": {
"version": "0.14.0",
"resolved": "https://registry.npmjs.org/react-refresh/-/react-refresh-0.14.0.tgz",
@@ -43081,9 +39944,9 @@
}
},
"node_modules/react-remove-scroll-bar": {
- "version": "2.3.4",
- "resolved": "https://registry.npmjs.org/react-remove-scroll-bar/-/react-remove-scroll-bar-2.3.4.tgz",
- "integrity": "sha512-63C4YQBUt0m6ALadE9XV56hV8BgJWDmmTPY758iIJjfQKt2nYwoUrPk0LXRXcB/yIj82T1/Ixfdpdk68LwIB0A==",
+ "version": "2.3.6",
+ "resolved": "https://registry.npmjs.org/react-remove-scroll-bar/-/react-remove-scroll-bar-2.3.6.tgz",
+ "integrity": "sha512-DtSYaao4mBmX+HDo5YWYdBWQwYIQQshUV/dVxFxK+KM26Wjwp1gZ6rv6OC3oujI6Bfu6Xyg3TwK533AQutsn/g==",
"dependencies": {
"react-style-singleton": "^2.2.1",
"tslib": "^2.0.0"
@@ -43326,45 +40189,24 @@
"dev": true
},
"node_modules/read": {
- "version": "2.1.0",
- "resolved": "https://registry.npmjs.org/read/-/read-2.1.0.tgz",
- "integrity": "sha512-bvxi1QLJHcaywCAEsAk4DG3nVoqiY2Csps3qzWalhj5hFqRn1d/OixkFXtLO1PrgHUcAP0FNaSY/5GYNfENFFQ==",
+ "version": "3.0.1",
+ "resolved": "https://registry.npmjs.org/read/-/read-3.0.1.tgz",
+ "integrity": "sha512-SLBrDU/Srs/9EoWhU5GdbAoxG1GzpQHo/6qiGItaoLJ1thmYpcNIM1qISEUvyHBzfGlWIyd6p2DNi1oV1VmAuw==",
"dev": true,
+ "license": "ISC",
"dependencies": {
- "mute-stream": "~1.0.0"
+ "mute-stream": "^1.0.0"
},
"engines": {
"node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
},
- "node_modules/read-cache": {
- "version": "1.0.0",
- "resolved": "https://registry.npmjs.org/read-cache/-/read-cache-1.0.0.tgz",
- "integrity": "sha512-Owdv/Ft7IjOgm/i0xvNDZ1LrRANRfew4b2prF3OWMQLxLfu3bS8FVhCsrSCMK4lR56Y9ya+AThoTpDCTxCmpRA==",
- "dependencies": {
- "pify": "^2.3.0"
- }
- },
"node_modules/read-cmd-shim": {
"version": "4.0.0",
"resolved": "https://registry.npmjs.org/read-cmd-shim/-/read-cmd-shim-4.0.0.tgz",
"integrity": "sha512-yILWifhaSEEytfXI76kB9xEEiG1AiozaCJZ83A87ytjRiN+jVibXjedjCRNjoZviinhG+4UkalO3mWTd8u5O0Q==",
"dev": true,
- "engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
- }
- },
- "node_modules/read-package-json": {
- "version": "6.0.4",
- "resolved": "https://registry.npmjs.org/read-package-json/-/read-package-json-6.0.4.tgz",
- "integrity": "sha512-AEtWXYfopBj2z5N5PbkAOeNHRPUg5q+Nen7QLxV8M2zJq1ym6/lCz3fYNTCXe19puu2d06jfHhrP7v/S2PtMMw==",
- "dev": true,
- "dependencies": {
- "glob": "^10.2.2",
- "json-parse-even-better-errors": "^3.0.0",
- "normalize-package-data": "^5.0.0",
- "npm-normalize-package-bin": "^3.0.0"
- },
+ "license": "ISC",
"engines": {
"node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
@@ -43374,6 +40216,7 @@
"resolved": "https://registry.npmjs.org/read-package-json-fast/-/read-package-json-fast-3.0.2.tgz",
"integrity": "sha512-0J+Msgym3vrLOUB3hzQCuZHII0xkNGCtz/HJH9xZshwv9DbDwkw1KaE3gx/e2J5rpEY5rtOy6cyhKOPrkP7FZw==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"json-parse-even-better-errors": "^3.0.0",
"npm-normalize-package-bin": "^3.0.0"
@@ -43387,6 +40230,7 @@
"resolved": "https://registry.npmjs.org/json-parse-even-better-errors/-/json-parse-even-better-errors-3.0.2.tgz",
"integrity": "sha512-fi0NG4bPjCHunUJffmLd0gxssIgkNmArMvis4iNah6Owg1MCJjWhEcDLmsK6iGkJq3tHwbDkTlce70/tmXN4cQ==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
@@ -43396,132 +40240,7 @@
"resolved": "https://registry.npmjs.org/npm-normalize-package-bin/-/npm-normalize-package-bin-3.0.1.tgz",
"integrity": "sha512-dMxCf+zZ+3zeQZXKxmyuCKlIDPGuv8EF940xbkC4kQVDTtqoh6rJFO+JTKSA6/Rwi0getWmtuy4Itup0AMcaDQ==",
"dev": true,
- "engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
- }
- },
- "node_modules/read-package-json/node_modules/brace-expansion": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/brace-expansion/-/brace-expansion-2.0.1.tgz",
- "integrity": "sha512-XnAIvQ8eM+kC6aULx6wuQiwVsnzsi9d3WxzV3FpWTGA19F621kwdbsAcFKXgKUHZWsy+mY6iL1sHTxWEFCytDA==",
- "dev": true,
- "dependencies": {
- "balanced-match": "^1.0.0"
- }
- },
- "node_modules/read-package-json/node_modules/glob": {
- "version": "10.4.5",
- "resolved": "https://registry.npmjs.org/glob/-/glob-10.4.5.tgz",
- "integrity": "sha512-7Bv8RF0k6xjo7d4A/PxYLbUCfb6c+Vpd2/mB2yRDlew7Jb5hEXiCD9ibfO7wpk8i4sevK6DFny9h7EYbM3/sHg==",
- "dev": true,
- "license": "ISC",
- "dependencies": {
- "foreground-child": "^3.1.0",
- "jackspeak": "^3.1.2",
- "minimatch": "^9.0.4",
- "minipass": "^7.1.2",
- "package-json-from-dist": "^1.0.0",
- "path-scurry": "^1.11.1"
- },
- "bin": {
- "glob": "dist/esm/bin.mjs"
- },
- "funding": {
- "url": "https://github.com/sponsors/isaacs"
- }
- },
- "node_modules/read-package-json/node_modules/hosted-git-info": {
- "version": "6.1.1",
- "resolved": "https://registry.npmjs.org/hosted-git-info/-/hosted-git-info-6.1.1.tgz",
- "integrity": "sha512-r0EI+HBMcXadMrugk0GCQ+6BQV39PiWAZVfq7oIckeGiN7sjRGyQxPdft3nQekFTCQbYxLBH+/axZMeH8UX6+w==",
- "dev": true,
- "dependencies": {
- "lru-cache": "^7.5.1"
- },
- "engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
- }
- },
- "node_modules/read-package-json/node_modules/jackspeak": {
- "version": "3.4.3",
- "resolved": "https://registry.npmjs.org/jackspeak/-/jackspeak-3.4.3.tgz",
- "integrity": "sha512-OGlZQpz2yfahA/Rd1Y8Cd9SIEsqvXkLVoSw/cgwhnhFMDbsQFeZYoJJ7bIZBS9BcamUW96asq/npPWugM+RQBw==",
- "dev": true,
- "license": "BlueOak-1.0.0",
- "dependencies": {
- "@isaacs/cliui": "^8.0.2"
- },
- "funding": {
- "url": "https://github.com/sponsors/isaacs"
- },
- "optionalDependencies": {
- "@pkgjs/parseargs": "^0.11.0"
- }
- },
- "node_modules/read-package-json/node_modules/json-parse-even-better-errors": {
- "version": "3.0.2",
- "resolved": "https://registry.npmjs.org/json-parse-even-better-errors/-/json-parse-even-better-errors-3.0.2.tgz",
- "integrity": "sha512-fi0NG4bPjCHunUJffmLd0gxssIgkNmArMvis4iNah6Owg1MCJjWhEcDLmsK6iGkJq3tHwbDkTlce70/tmXN4cQ==",
- "dev": true,
- "engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
- }
- },
- "node_modules/read-package-json/node_modules/lru-cache": {
- "version": "7.18.3",
- "resolved": "https://registry.npmjs.org/lru-cache/-/lru-cache-7.18.3.tgz",
- "integrity": "sha512-jumlc0BIUrS3qJGgIkWZsyfAM7NCWiBcCDhnd+3NNM5KbBmLTgHVfWBcg6W+rLUsIpzpERPsvwUP7CckAQSOoA==",
- "dev": true,
- "engines": {
- "node": ">=12"
- }
- },
- "node_modules/read-package-json/node_modules/minimatch": {
- "version": "9.0.5",
- "resolved": "https://registry.npmjs.org/minimatch/-/minimatch-9.0.5.tgz",
- "integrity": "sha512-G6T0ZX48xgozx7587koeX9Ys2NYy6Gmv//P89sEte9V9whIapMNF4idKxnW2QtCcLiTWlb/wfCabAtAFWhhBow==",
- "dev": true,
- "license": "ISC",
- "dependencies": {
- "brace-expansion": "^2.0.1"
- },
- "engines": {
- "node": ">=16 || 14 >=14.17"
- },
- "funding": {
- "url": "https://github.com/sponsors/isaacs"
- }
- },
- "node_modules/read-package-json/node_modules/minipass": {
- "version": "7.1.2",
- "resolved": "https://registry.npmjs.org/minipass/-/minipass-7.1.2.tgz",
- "integrity": "sha512-qOOzS1cBTWYF4BH8fVePDBOO9iptMnGUEZwNc/cMWnTV2nVLZ7VoNWEPHkYczZA0pdoA7dl6e7FL659nX9S2aw==",
- "dev": true,
"license": "ISC",
- "engines": {
- "node": ">=16 || 14 >=14.17"
- }
- },
- "node_modules/read-package-json/node_modules/normalize-package-data": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/normalize-package-data/-/normalize-package-data-5.0.0.tgz",
- "integrity": "sha512-h9iPVIfrVZ9wVYQnxFgtw1ugSvGEMOlyPWWtm8BMJhnwyEL/FLbYbTY3V3PpjI/BUK67n9PEWDu6eHzu1fB15Q==",
- "dev": true,
- "dependencies": {
- "hosted-git-info": "^6.0.0",
- "is-core-module": "^2.8.1",
- "semver": "^7.3.5",
- "validate-npm-package-license": "^3.0.4"
- },
- "engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
- }
- },
- "node_modules/read-package-json/node_modules/npm-normalize-package-bin": {
- "version": "3.0.1",
- "resolved": "https://registry.npmjs.org/npm-normalize-package-bin/-/npm-normalize-package-bin-3.0.1.tgz",
- "integrity": "sha512-dMxCf+zZ+3zeQZXKxmyuCKlIDPGuv8EF940xbkC4kQVDTtqoh6rJFO+JTKSA6/Rwi0getWmtuy4Itup0AMcaDQ==",
- "dev": true,
"engines": {
"node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
@@ -43633,6 +40352,7 @@
"resolved": "https://registry.npmjs.org/mute-stream/-/mute-stream-1.0.0.tgz",
"integrity": "sha512-avsJQhyd+680gKXyG/sQc0nXaC6rBkPOfyHYcFb9+hdkqQkR9bdnkJ0AMZhke0oesPqIO+mFFJ+IdBc7mst4IA==",
"dev": true,
+ "license": "ISC",
"engines": {
"node": "^14.17.0 || ^16.13.0 || >=18.0.0"
}
@@ -43894,62 +40614,6 @@
"unified": "^7.0.0"
}
},
- "node_modules/remark-external-links": {
- "version": "8.0.0",
- "resolved": "https://registry.npmjs.org/remark-external-links/-/remark-external-links-8.0.0.tgz",
- "integrity": "sha512-5vPSX0kHoSsqtdftSHhIYofVINC8qmp0nctkeU9YoJwV3YfiBRiI6cbFRJ0oI/1F9xS+bopXG0m2KS8VFscuKA==",
- "dev": true,
- "dependencies": {
- "extend": "^3.0.0",
- "is-absolute-url": "^3.0.0",
- "mdast-util-definitions": "^4.0.0",
- "space-separated-tokens": "^1.0.0",
- "unist-util-visit": "^2.0.0"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/unified"
- }
- },
- "node_modules/remark-external-links/node_modules/unist-util-is": {
- "version": "4.1.0",
- "resolved": "https://registry.npmjs.org/unist-util-is/-/unist-util-is-4.1.0.tgz",
- "integrity": "sha512-ZOQSsnce92GrxSqlnEEseX0gi7GH9zTJZ0p9dtu87WRb/37mMPO2Ilx1s/t9vBHrFhbgweUwb+t7cIn5dxPhZg==",
- "dev": true,
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/unified"
- }
- },
- "node_modules/remark-external-links/node_modules/unist-util-visit": {
- "version": "2.0.3",
- "resolved": "https://registry.npmjs.org/unist-util-visit/-/unist-util-visit-2.0.3.tgz",
- "integrity": "sha512-iJ4/RczbJMkD0712mGktuGpm/U4By4FfDonL7N/9tATGIF4imikjOuagyMY53tnZq3NP6BcmlrHhEKAfGWjh7Q==",
- "dev": true,
- "dependencies": {
- "@types/unist": "^2.0.0",
- "unist-util-is": "^4.0.0",
- "unist-util-visit-parents": "^3.0.0"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/unified"
- }
- },
- "node_modules/remark-external-links/node_modules/unist-util-visit-parents": {
- "version": "3.1.1",
- "resolved": "https://registry.npmjs.org/unist-util-visit-parents/-/unist-util-visit-parents-3.1.1.tgz",
- "integrity": "sha512-1KROIZWo6bcMrZEwiH2UrXDyalAa0uqzWCxCJj6lPOvTve2WkfgCytoDTPaMnodXh1WrXOq0haVYHj99ynJlsg==",
- "dev": true,
- "dependencies": {
- "@types/unist": "^2.0.0",
- "unist-util-is": "^4.0.0"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/unified"
- }
- },
"node_modules/remark-parse": {
"version": "6.0.3",
"resolved": "https://registry.npmjs.org/remark-parse/-/remark-parse-6.0.3.tgz",
@@ -43972,60 +40636,6 @@
"xtend": "^4.0.1"
}
},
- "node_modules/remark-slug": {
- "version": "6.1.0",
- "resolved": "https://registry.npmjs.org/remark-slug/-/remark-slug-6.1.0.tgz",
- "integrity": "sha512-oGCxDF9deA8phWvxFuyr3oSJsdyUAxMFbA0mZ7Y1Sas+emILtO+e5WutF9564gDsEN4IXaQXm5pFo6MLH+YmwQ==",
- "dev": true,
- "dependencies": {
- "github-slugger": "^1.0.0",
- "mdast-util-to-string": "^1.0.0",
- "unist-util-visit": "^2.0.0"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/unified"
- }
- },
- "node_modules/remark-slug/node_modules/unist-util-is": {
- "version": "4.1.0",
- "resolved": "https://registry.npmjs.org/unist-util-is/-/unist-util-is-4.1.0.tgz",
- "integrity": "sha512-ZOQSsnce92GrxSqlnEEseX0gi7GH9zTJZ0p9dtu87WRb/37mMPO2Ilx1s/t9vBHrFhbgweUwb+t7cIn5dxPhZg==",
- "dev": true,
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/unified"
- }
- },
- "node_modules/remark-slug/node_modules/unist-util-visit": {
- "version": "2.0.3",
- "resolved": "https://registry.npmjs.org/unist-util-visit/-/unist-util-visit-2.0.3.tgz",
- "integrity": "sha512-iJ4/RczbJMkD0712mGktuGpm/U4By4FfDonL7N/9tATGIF4imikjOuagyMY53tnZq3NP6BcmlrHhEKAfGWjh7Q==",
- "dev": true,
- "dependencies": {
- "@types/unist": "^2.0.0",
- "unist-util-is": "^4.0.0",
- "unist-util-visit-parents": "^3.0.0"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/unified"
- }
- },
- "node_modules/remark-slug/node_modules/unist-util-visit-parents": {
- "version": "3.1.1",
- "resolved": "https://registry.npmjs.org/unist-util-visit-parents/-/unist-util-visit-parents-3.1.1.tgz",
- "integrity": "sha512-1KROIZWo6bcMrZEwiH2UrXDyalAa0uqzWCxCJj6lPOvTve2WkfgCytoDTPaMnodXh1WrXOq0haVYHj99ynJlsg==",
- "dev": true,
- "dependencies": {
- "@types/unist": "^2.0.0",
- "unist-util-is": "^4.0.0"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/unified"
- }
- },
"node_modules/remark-stringify": {
"version": "6.0.4",
"resolved": "https://registry.npmjs.org/remark-stringify/-/remark-stringify-6.0.4.tgz",
@@ -44396,6 +41006,7 @@
"resolved": "https://registry.npmjs.org/retry/-/retry-0.12.0.tgz",
"integrity": "sha512-9LkiTwjUh6rT555DtE9rTX+BKByPfrMzEAtnlEtdEwr3Nkffwiihqe2bWADg+OQRjt9gl6ICdmB/ZFDCGAtSow==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">= 4"
}
@@ -44416,38 +41027,73 @@
"dev": true
},
"node_modules/rimraf": {
- "version": "3.0.2",
- "resolved": "https://registry.npmjs.org/rimraf/-/rimraf-3.0.2.tgz",
- "integrity": "sha512-JZkJMZkAGFFPP2YqXZXPbMlMBgsxzE8ILs4lMIX/2o0L9UBw9O/Y3o6wFw/i9YLapcUJWwqbi3kdxIPdC62TIA==",
+ "version": "5.0.10",
+ "resolved": "https://registry.npmjs.org/rimraf/-/rimraf-5.0.10.tgz",
+ "integrity": "sha512-l0OE8wL34P4nJH/H2ffoaniAokM2qSmrtXHmlpvYr5AVVX8msAyW0l8NVJFDxlSK4u3Uh/f41cQheDVdnYijwQ==",
+ "license": "ISC",
"dependencies": {
- "glob": "^7.1.3"
+ "glob": "^10.3.7"
},
"bin": {
- "rimraf": "bin.js"
+ "rimraf": "dist/esm/bin.mjs"
},
"funding": {
"url": "https://github.com/sponsors/isaacs"
}
},
+ "node_modules/rimraf/node_modules/brace-expansion": {
+ "version": "2.0.1",
+ "resolved": "https://registry.npmjs.org/brace-expansion/-/brace-expansion-2.0.1.tgz",
+ "integrity": "sha512-XnAIvQ8eM+kC6aULx6wuQiwVsnzsi9d3WxzV3FpWTGA19F621kwdbsAcFKXgKUHZWsy+mY6iL1sHTxWEFCytDA==",
+ "license": "MIT",
+ "dependencies": {
+ "balanced-match": "^1.0.0"
+ }
+ },
"node_modules/rimraf/node_modules/glob": {
- "version": "7.2.3",
- "resolved": "https://registry.npmjs.org/glob/-/glob-7.2.3.tgz",
- "integrity": "sha512-nFR0zLpU2YCaRxwoCJvL6UvCH2JFyFVIvwTLsIf21AuHlMskA1hhTdk+LlYJtOlYt9v6dvszD2BGRqBL+iQK9Q==",
+ "version": "10.4.5",
+ "resolved": "https://registry.npmjs.org/glob/-/glob-10.4.5.tgz",
+ "integrity": "sha512-7Bv8RF0k6xjo7d4A/PxYLbUCfb6c+Vpd2/mB2yRDlew7Jb5hEXiCD9ibfO7wpk8i4sevK6DFny9h7EYbM3/sHg==",
+ "license": "ISC",
"dependencies": {
- "fs.realpath": "^1.0.0",
- "inflight": "^1.0.4",
- "inherits": "2",
- "minimatch": "^3.1.1",
- "once": "^1.3.0",
- "path-is-absolute": "^1.0.0"
+ "foreground-child": "^3.1.0",
+ "jackspeak": "^3.1.2",
+ "minimatch": "^9.0.4",
+ "minipass": "^7.1.2",
+ "package-json-from-dist": "^1.0.0",
+ "path-scurry": "^1.11.1"
+ },
+ "bin": {
+ "glob": "dist/esm/bin.mjs"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
+ }
+ },
+ "node_modules/rimraf/node_modules/minimatch": {
+ "version": "9.0.5",
+ "resolved": "https://registry.npmjs.org/minimatch/-/minimatch-9.0.5.tgz",
+ "integrity": "sha512-G6T0ZX48xgozx7587koeX9Ys2NYy6Gmv//P89sEte9V9whIapMNF4idKxnW2QtCcLiTWlb/wfCabAtAFWhhBow==",
+ "license": "ISC",
+ "dependencies": {
+ "brace-expansion": "^2.0.1"
},
"engines": {
- "node": "*"
+ "node": ">=16 || 14 >=14.17"
},
"funding": {
"url": "https://github.com/sponsors/isaacs"
}
},
+ "node_modules/rimraf/node_modules/minipass": {
+ "version": "7.1.2",
+ "resolved": "https://registry.npmjs.org/minipass/-/minipass-7.1.2.tgz",
+ "integrity": "sha512-qOOzS1cBTWYF4BH8fVePDBOO9iptMnGUEZwNc/cMWnTV2nVLZ7VoNWEPHkYczZA0pdoA7dl6e7FL659nX9S2aw==",
+ "license": "ISC",
+ "engines": {
+ "node": ">=16 || 14 >=14.17"
+ }
+ },
"node_modules/ripemd160": {
"version": "2.0.2",
"resolved": "https://registry.npmjs.org/ripemd160/-/ripemd160-2.0.2.tgz",
@@ -44466,6 +41112,12 @@
"node": ">=10.0.0"
}
},
+ "node_modules/route-recognizer": {
+ "version": "0.3.4",
+ "resolved": "https://registry.npmjs.org/route-recognizer/-/route-recognizer-0.3.4.tgz",
+ "integrity": "sha512-2+MhsfPhvauN1O8KaXpXAOfR/fwe8dnUXVM+xw7yt40lJRfPVQxV6yryZm0cgRvAj5fMF/mdRZbL2ptwbs5i2g==",
+ "license": "MIT"
+ },
"node_modules/rrweb-cssom": {
"version": "0.7.1",
"resolved": "https://registry.npmjs.org/rrweb-cssom/-/rrweb-cssom-0.7.1.tgz",
@@ -44600,19 +41252,6 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
- "node_modules/run-applescript/node_modules/cross-spawn": {
- "version": "7.0.3",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-7.0.3.tgz",
- "integrity": "sha512-iRDPJKUPVEND7dHPO8rkbOnPpyDygcDFtWjpeWNCgy8WP2rXcxXL8TskReQl6OrB2G7+UJrags1q15Fudc7G6w==",
- "dependencies": {
- "path-key": "^3.1.0",
- "shebang-command": "^2.0.0",
- "which": "^2.0.1"
- },
- "engines": {
- "node": ">= 8"
- }
- },
"node_modules/run-applescript/node_modules/execa": {
"version": "5.1.1",
"resolved": "https://registry.npmjs.org/execa/-/execa-5.1.1.tgz",
@@ -44706,39 +41345,6 @@
"node": ">=8"
}
},
- "node_modules/run-applescript/node_modules/shebang-command": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/shebang-command/-/shebang-command-2.0.0.tgz",
- "integrity": "sha512-kHxr2zZpYtdmrN1qDjrrX/Z1rR1kG8Dx+gkpK1G4eXmvXswmcE1hTWBWYUzlraYw1/yZp6YuDY77YtvbN0dmDA==",
- "dependencies": {
- "shebang-regex": "^3.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/run-applescript/node_modules/shebang-regex": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/shebang-regex/-/shebang-regex-3.0.0.tgz",
- "integrity": "sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==",
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/run-applescript/node_modules/which": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
- "integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
- "dependencies": {
- "isexe": "^2.0.0"
- },
- "bin": {
- "node-which": "bin/node-which"
- },
- "engines": {
- "node": ">= 8"
- }
- },
"node_modules/run-async": {
"version": "2.4.1",
"resolved": "https://registry.npmjs.org/run-async/-/run-async-2.4.1.tgz",
@@ -44846,10 +41452,11 @@
}
},
"node_modules/safaridriver": {
- "version": "0.1.0",
- "resolved": "https://registry.npmjs.org/safaridriver/-/safaridriver-0.1.0.tgz",
- "integrity": "sha512-azzzIP3gR1TB9bVPv7QO4Zjw0rR1BWEU/s2aFdUMN48gxDjxEB13grAEuXDmkKPgE74cObymDxmAmZnL3clj4w==",
- "dev": true
+ "version": "0.1.2",
+ "resolved": "https://registry.npmjs.org/safaridriver/-/safaridriver-0.1.2.tgz",
+ "integrity": "sha512-4R309+gWflJktzPXBQCobbWEHlzC4aK3a+Ov3tz2Ib2aBxiwd11phkdIBH1l0EO22x24CJMUQkpKFumRriCSRg==",
+ "dev": true,
+ "license": "MIT"
},
"node_modules/safe-array-concat": {
"version": "1.0.0",
@@ -44924,42 +41531,46 @@
}
},
"node_modules/sass": {
- "version": "1.35.2",
- "resolved": "https://registry.npmjs.org/sass/-/sass-1.35.2.tgz",
- "integrity": "sha512-jhO5KAR+AMxCEwIH3v+4zbB2WB0z67V1X0jbapfVwQQdjHZUGUyukpnoM6+iCMfsIUC016w9OPKQ5jrNOS9uXw==",
+ "version": "1.50.1",
+ "resolved": "https://registry.npmjs.org/sass/-/sass-1.50.1.tgz",
+ "integrity": "sha512-noTnY41KnlW2A9P8sdwESpDmo+KBNkukI1i8+hOK3footBUcohNHtdOJbckp46XO95nuvcHDDZ+4tmOnpK3hjw==",
+ "license": "MIT",
"dependencies": {
- "chokidar": ">=3.0.0 <4.0.0"
+ "chokidar": ">=3.0.0 <4.0.0",
+ "immutable": "^4.0.0",
+ "source-map-js": ">=0.6.2 <2.0.0"
},
"bin": {
"sass": "sass.js"
},
"engines": {
- "node": ">=8.9.0"
+ "node": ">=12.0.0"
}
},
"node_modules/sass-loader": {
- "version": "12.1.0",
- "resolved": "https://registry.npmjs.org/sass-loader/-/sass-loader-12.1.0.tgz",
- "integrity": "sha512-FVJZ9kxVRYNZTIe2xhw93n3xJNYZADr+q69/s98l9nTCrWASo+DR2Ot0s5xTKQDDEosUkatsGeHxcH4QBp5bSg==",
+ "version": "16.0.3",
+ "resolved": "https://registry.npmjs.org/sass-loader/-/sass-loader-16.0.3.tgz",
+ "integrity": "sha512-gosNorT1RCkuCMyihv6FBRR7BMV06oKRAs+l4UMp1mlcVg9rWN6KMmUj3igjQwmYys4mDP3etEYJgiHRbgHCHA==",
+ "license": "MIT",
"dependencies": {
- "klona": "^2.0.4",
"neo-async": "^2.6.2"
},
"engines": {
- "node": ">= 12.13.0"
+ "node": ">= 18.12.0"
},
"funding": {
"type": "opencollective",
"url": "https://opencollective.com/webpack"
},
"peerDependencies": {
- "fibers": ">= 3.1.0",
- "node-sass": "^4.0.0 || ^5.0.0 || ^6.0.0",
+ "@rspack/core": "0.x || 1.x",
+ "node-sass": "^4.0.0 || ^5.0.0 || ^6.0.0 || ^7.0.0 || ^8.0.0 || ^9.0.0",
"sass": "^1.3.0",
+ "sass-embedded": "*",
"webpack": "^5.0.0"
},
"peerDependenciesMeta": {
- "fibers": {
+ "@rspack/core": {
"optional": true
},
"node-sass": {
@@ -44967,6 +41578,12 @@
},
"sass": {
"optional": true
+ },
+ "sass-embedded": {
+ "optional": true
+ },
+ "webpack": {
+ "optional": true
}
}
},
@@ -45036,10 +41653,12 @@
"integrity": "sha512-mEugaLK+YfkijB4fx0e6kImuJdCIt2LxCRcbEYPqRGCs4F2ogyfZU5IAZRdjCP8JPq2AtdNoC/Dux63d9Kiryg=="
},
"node_modules/selfsigned": {
- "version": "2.1.1",
- "resolved": "https://registry.npmjs.org/selfsigned/-/selfsigned-2.1.1.tgz",
- "integrity": "sha512-GSL3aowiF7wa/WtSFwnUrludWFoNhftq8bUkH9pkzjpN2XSPOAYEgg6e0sS9s0rZwgJzJiQRPU18A6clnoW5wQ==",
+ "version": "2.4.1",
+ "resolved": "https://registry.npmjs.org/selfsigned/-/selfsigned-2.4.1.tgz",
+ "integrity": "sha512-th5B4L2U+eGLq1TVh7zNRGBapioSORUeymIydxgFpwww9d2qyKvtuPU2jJuHvYAwwqi2Y596QBL3eEqcPEYL8Q==",
+ "license": "MIT",
"dependencies": {
+ "@types/node-forge": "^1.3.0",
"node-forge": "^1"
},
"engines": {
@@ -45077,15 +41696,11 @@
"node": ">=10"
}
},
- "node_modules/semver/node_modules/yallist": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/yallist/-/yallist-4.0.0.tgz",
- "integrity": "sha512-3wdGidZyq5PB084XLES5TpOSRA3wjXAlIWMhum2kRcv/41Sn2emQ0dycQW4uZXLejwKvg6EsvbdlVL+FYEct7A=="
- },
"node_modules/send": {
"version": "0.18.0",
"resolved": "https://registry.npmjs.org/send/-/send-0.18.0.tgz",
"integrity": "sha512-qqWzuOjSFOuqPjFe4NOsMLafToQQwBSOEpS+FwEt3A2V3vKubTquT3vmLTQpFgMXp8AlFWFuP1qKaJZOtPpVXg==",
+ "license": "MIT",
"dependencies": {
"debug": "2.6.9",
"depd": "2.0.0",
@@ -45109,6 +41724,7 @@
"version": "2.6.9",
"resolved": "https://registry.npmjs.org/debug/-/debug-2.6.9.tgz",
"integrity": "sha512-bC7ElrdJaJnPbAP+1EotYvqZsb3ecl5wi6Bfi6BJTUcNowp6cvspg0jXznRTKDjm/E7AdgFBVeAPVMNcKGsHMA==",
+ "license": "MIT",
"dependencies": {
"ms": "2.0.0"
}
@@ -45116,12 +41732,14 @@
"node_modules/send/node_modules/debug/node_modules/ms": {
"version": "2.0.0",
"resolved": "https://registry.npmjs.org/ms/-/ms-2.0.0.tgz",
- "integrity": "sha512-Tpp60P6IUJDTuOq/5Z8cdskzJujfwqfOTkrwIwj7IRISpnkJnT6SyJ4PCPnGMoFjC9ddhal5KVIYtAt97ix05A=="
+ "integrity": "sha512-Tpp60P6IUJDTuOq/5Z8cdskzJujfwqfOTkrwIwj7IRISpnkJnT6SyJ4PCPnGMoFjC9ddhal5KVIYtAt97ix05A==",
+ "license": "MIT"
},
"node_modules/send/node_modules/depd": {
"version": "2.0.0",
"resolved": "https://registry.npmjs.org/depd/-/depd-2.0.0.tgz",
"integrity": "sha512-g7nH6P6dyDioJogAAGprGpCtVImJhpPk/roCzdb3fIh61/s/nPsfR6onyMwkCAR/OlC3yBC0lESvUoQEAssIrw==",
+ "license": "MIT",
"engines": {
"node": ">= 0.8"
}
@@ -45130,6 +41748,7 @@
"version": "1.6.0",
"resolved": "https://registry.npmjs.org/mime/-/mime-1.6.0.tgz",
"integrity": "sha512-x0Vn8spI+wuJ1O6S7gnbaQg8Pxh4NNHb7KSINmEWKiPE4RKOplvijn+NkmYmmRgP68mc70j2EbeTFRsrswaQeg==",
+ "license": "MIT",
"bin": {
"mime": "cli.js"
},
@@ -45140,12 +41759,14 @@
"node_modules/send/node_modules/ms": {
"version": "2.1.3",
"resolved": "https://registry.npmjs.org/ms/-/ms-2.1.3.tgz",
- "integrity": "sha512-6FlzubTLZG3J2a/NVCAleEhjzq5oxgHyaCU9yYXvcLsvoVaHJq/s5xXI6/XXP6tz7R9xAOtHnSO/tXtF3WRTlA=="
+ "integrity": "sha512-6FlzubTLZG3J2a/NVCAleEhjzq5oxgHyaCU9yYXvcLsvoVaHJq/s5xXI6/XXP6tz7R9xAOtHnSO/tXtF3WRTlA==",
+ "license": "MIT"
},
"node_modules/send/node_modules/on-finished": {
"version": "2.4.1",
"resolved": "https://registry.npmjs.org/on-finished/-/on-finished-2.4.1.tgz",
"integrity": "sha512-oVlzkg3ENAhCk2zdv7IJwd/QUD4z2RxRwpkcGY8psCVcCYZNq4wYnVWALHM+brtuJjePWiYF/ClmuDr8Ch5+kg==",
+ "license": "MIT",
"dependencies": {
"ee-first": "1.1.1"
},
@@ -45157,6 +41778,7 @@
"version": "2.0.1",
"resolved": "https://registry.npmjs.org/statuses/-/statuses-2.0.1.tgz",
"integrity": "sha512-RwNA9Z/7PrK06rYLIzFMlaF+l73iwpzsqRIFgbMLbTcLD6cOao82TaWefPXQvB2fOC4AjuYSEndS7N/mTCbkdQ==",
+ "license": "MIT",
"engines": {
"node": ">= 0.8"
}
@@ -45264,6 +41886,7 @@
"version": "1.15.0",
"resolved": "https://registry.npmjs.org/serve-static/-/serve-static-1.15.0.tgz",
"integrity": "sha512-XGuRDNjXUijsUL0vl6nSD7cwURuzEgglbOaFuZM9g3kwDXOWVTck0jLzjPzGD+TazWbboZYu52/9/XPdUgne9g==",
+ "license": "MIT",
"dependencies": {
"encodeurl": "~1.0.2",
"escape-html": "~1.0.3",
@@ -45375,6 +41998,7 @@
"integrity": "sha512-KyLTWwgcR9Oe4d9HwCwNM2l7+J0dUQwn/yf7S0EnTtb0eVS4RxO0eUSvxPtzT4F3SY+C4K6fqdv/DO27sJ/v/w==",
"dev": true,
"hasInstallScript": true,
+ "license": "Apache-2.0",
"optional": true,
"dependencies": {
"color": "^4.2.3",
@@ -45393,13 +42017,6 @@
"url": "https://opencollective.com/libvips"
}
},
- "node_modules/sharp/node_modules/node-addon-api": {
- "version": "6.1.0",
- "resolved": "https://registry.npmjs.org/node-addon-api/-/node-addon-api-6.1.0.tgz",
- "integrity": "sha512-+eawOlIgy680F0kBzPUNFhMZGtJ1YmqM6l4+Crf4IkImjYrO/mqPwRMh352g23uIaQKFItcQ64I7KMaJxHgAVA==",
- "dev": true,
- "optional": true
- },
"node_modules/sharp/node_modules/pump": {
"version": "3.0.2",
"resolved": "https://registry.npmjs.org/pump/-/pump-3.0.2.tgz",
@@ -45417,6 +42034,7 @@
"resolved": "https://registry.npmjs.org/tar-fs/-/tar-fs-3.0.6.tgz",
"integrity": "sha512-iokBDQQkUyeXhgPYaZxmczGPhnhXZ0CmrqI+MOb/WFGS9DW5wnfrLgtjUJBvz50vQ3qfRwJ62QVoCFu8mPVu5w==",
"dev": true,
+ "license": "MIT",
"optional": true,
"dependencies": {
"pump": "^3.0.0",
@@ -45432,6 +42050,7 @@
"resolved": "https://registry.npmjs.org/tar-stream/-/tar-stream-3.1.7.tgz",
"integrity": "sha512-qJj60CXt7IU1Ffyc3NJMjh6EkuCFej46zUqJ4J7pqYlThyd9bO0XBTmcOIhSzZJVWfsLks0+nle/j538YAW9RQ==",
"dev": true,
+ "license": "MIT",
"optional": true,
"dependencies": {
"b4a": "^1.6.4",
@@ -45443,6 +42062,7 @@
"version": "1.2.0",
"resolved": "https://registry.npmjs.org/shebang-command/-/shebang-command-1.2.0.tgz",
"integrity": "sha512-EV3L1+UQWGor21OmnvojK36mhg+TyIKDh3iFBKBohr5xeXIhNBcx8oWdgkTEEQ+BEFFYdLRuqMfd5L84N1V5Vg==",
+ "dev": true,
"dependencies": {
"shebang-regex": "^1.0.0"
},
@@ -45454,6 +42074,7 @@
"version": "1.0.0",
"resolved": "https://registry.npmjs.org/shebang-regex/-/shebang-regex-1.0.0.tgz",
"integrity": "sha512-wpoSFAxys6b2a2wHZ1XpDSgD7N9iVjg29Ph9uV/uaP9Ex/KXlkTZTeddxDPSYQpgvzKLGJke2UU0AzoGCjNIvQ==",
+ "dev": true,
"engines": {
"node": ">=0.10.0"
}
@@ -45668,21 +42289,21 @@
"integrity": "sha512-wnD2ZE+l+SPC/uoS0vXeE9L1+0wuaMqKlfz9AMUo38JsyLSBWSFcHR1Rri62LZc12vLr1gb3jl7iwQhgwpAbGQ=="
},
"node_modules/sigstore": {
- "version": "1.8.0",
- "resolved": "https://registry.npmjs.org/sigstore/-/sigstore-1.8.0.tgz",
- "integrity": "sha512-ogU8qtQ3VFBawRJ8wjsBEX/vIFeHuGs1fm4jZtjWQwjo8pfAt7T/rh+udlAN4+QUe0IzA8qRSc/YZ7dHP6kh+w==",
+ "version": "2.3.1",
+ "resolved": "https://registry.npmjs.org/sigstore/-/sigstore-2.3.1.tgz",
+ "integrity": "sha512-8G+/XDU8wNsJOQS5ysDVO0Etg9/2uA5gR9l4ZwijjlwxBcrU6RPfwi2+jJmbP+Ap1Hlp/nVAaEO4Fj22/SL2gQ==",
"dev": true,
+ "license": "Apache-2.0",
"dependencies": {
- "@sigstore/bundle": "^1.0.0",
- "@sigstore/protobuf-specs": "^0.2.0",
- "@sigstore/tuf": "^1.0.3",
- "make-fetch-happen": "^11.0.1"
- },
- "bin": {
- "sigstore": "bin/sigstore.js"
+ "@sigstore/bundle": "^2.3.2",
+ "@sigstore/core": "^1.0.0",
+ "@sigstore/protobuf-specs": "^0.3.2",
+ "@sigstore/sign": "^2.3.2",
+ "@sigstore/tuf": "^2.3.4",
+ "@sigstore/verify": "^1.2.1"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/simple-concat": {
@@ -45704,6 +42325,7 @@
"url": "https://feross.org/support"
}
],
+ "license": "MIT",
"optional": true
},
"node_modules/simple-get": {
@@ -45725,6 +42347,7 @@
"url": "https://feross.org/support"
}
],
+ "license": "MIT",
"optional": true,
"dependencies": {
"decompress-response": "^6.0.0",
@@ -46060,17 +42683,17 @@
}
},
"node_modules/socks-proxy-agent": {
- "version": "7.0.0",
- "resolved": "https://registry.npmjs.org/socks-proxy-agent/-/socks-proxy-agent-7.0.0.tgz",
- "integrity": "sha512-Fgl0YPZ902wEsAyiQ+idGd1A7rSFx/ayC1CQVMw5P+EQx2V0SgpGtf6OKFhVjPflPUl9YMmEOnmfjCdMUsygww==",
- "dev": true,
+ "version": "8.0.5",
+ "resolved": "https://registry.npmjs.org/socks-proxy-agent/-/socks-proxy-agent-8.0.5.tgz",
+ "integrity": "sha512-HehCEsotFqbPW9sJ8WVYB6UbmIMv7kUUORIF2Nncq4VQvBfNBLibW9YZR5dlYCSUhwcD628pRllm7n+E+YTzJw==",
+ "license": "MIT",
"dependencies": {
- "agent-base": "^6.0.2",
- "debug": "^4.3.3",
- "socks": "^2.6.2"
+ "agent-base": "^7.1.2",
+ "debug": "^4.3.4",
+ "socks": "^2.8.3"
},
"engines": {
- "node": ">= 10"
+ "node": ">= 14"
}
},
"node_modules/sort-keys": {
@@ -46162,15 +42785,22 @@
"integrity": "sha1-PpNdfd1zYxuXZZlW1VEo6HtQhKM=",
"deprecated": "See https://github.com/lydell/source-map-url#deprecated"
},
- "node_modules/space-separated-tokens": {
- "version": "1.1.5",
- "resolved": "https://registry.npmjs.org/space-separated-tokens/-/space-separated-tokens-1.1.5.tgz",
- "integrity": "sha512-q/JSVd1Lptzhf5bkYm4ob4iWPjx0KiRe3sRFBNrVqbJkFaBm5vbbowy1mymoPNLRa52+oadOhJ+K49wsSeSjTA==",
+ "node_modules/spacetrim": {
+ "version": "0.11.59",
+ "resolved": "https://registry.npmjs.org/spacetrim/-/spacetrim-0.11.59.tgz",
+ "integrity": "sha512-lLYsktklSRKprreOm7NXReW8YiX2VBjbgmXYEziOoGf/qsJqAEACaDvoTtUOycwjpaSh+bT8eu0KrJn7UNxiCg==",
"dev": true,
- "funding": {
- "type": "github",
- "url": "https://github.com/sponsors/wooorm"
- }
+ "funding": [
+ {
+ "type": "individual",
+ "url": "https://buymeacoffee.com/hejny"
+ },
+ {
+ "type": "github",
+ "url": "https://github.com/hejny/spacetrim/blob/main/README.md#%EF%B8%8F-contributing"
+ }
+ ],
+ "license": "Apache-2.0"
},
"node_modules/spawn-command": {
"version": "0.0.2-1",
@@ -46178,6 +42808,31 @@
"integrity": "sha512-n98l9E2RMSJ9ON1AKisHzz7V42VDiBQGY6PB1BwRglz99wpVsSuGzQ+jOi6lFXBGVTCrRpltvjm+/XA+tpeJrg==",
"dev": true
},
+ "node_modules/spawnd": {
+ "version": "10.1.4",
+ "resolved": "https://registry.npmjs.org/spawnd/-/spawnd-10.1.4.tgz",
+ "integrity": "sha512-drqHc0mKJmtMsiGMOCwzlc5eZ0RPtRvT7tQAluW2A0qUc0G7TQ8KLcn3E6K5qzkLkH2UkS3nYQiVGULvvsD9dw==",
+ "license": "MIT",
+ "dependencies": {
+ "signal-exit": "^4.1.0",
+ "tree-kill": "^1.2.2"
+ },
+ "engines": {
+ "node": ">=16"
+ }
+ },
+ "node_modules/spawnd/node_modules/signal-exit": {
+ "version": "4.1.0",
+ "resolved": "https://registry.npmjs.org/signal-exit/-/signal-exit-4.1.0.tgz",
+ "integrity": "sha512-bzyZ1e88w9O1iNJbKnOlvYTrWPDl46O1bG0D3XInv+9tkPrxrN8jUUTiFlDkkmKWgn1M6CfIA13SuGqOa9Korw==",
+ "license": "ISC",
+ "engines": {
+ "node": ">=14"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
+ }
+ },
"node_modules/spdx-correct": {
"version": "3.0.0",
"resolved": "https://registry.npmjs.org/spdx-correct/-/spdx-correct-3.0.0.tgz",
@@ -46274,6 +42929,7 @@
"resolved": "https://registry.npmjs.org/split/-/split-1.0.1.tgz",
"integrity": "sha512-mTyOoPbrivtXnwnIxZRFYRrPNtEFKlpB2fvjSnCQUiAA6qAZzqwna5envK4uk6OIeP17CsdF3rSBGYVBsU0Tkg==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"through": "2"
},
@@ -46306,6 +42962,7 @@
"resolved": "https://registry.npmjs.org/split2/-/split2-3.2.2.tgz",
"integrity": "sha512-9NThjpgZnifTkJpzTZ7Eue85S49QwpNhZTq6GRJwObb6jnLFNGB7Qm73V5HewTROPyxD0C29xqmaI68bQtV+hg==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"readable-stream": "^3.0.0"
}
@@ -46315,6 +42972,7 @@
"resolved": "https://registry.npmjs.org/readable-stream/-/readable-stream-3.6.2.tgz",
"integrity": "sha512-9u/sniCrY3D5WdsERHzHE4G2YCXqoG5FTHUiCC4SIbr6XcLZBY05ya9EKjYek9O5xOAwjGq+1JdGBAS7Q9ScoA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"inherits": "^2.0.3",
"string_decoder": "^1.1.1",
@@ -46440,27 +43098,30 @@
"node": ">= 0.4"
}
},
- "node_modules/store2": {
- "version": "2.14.2",
- "resolved": "https://registry.npmjs.org/store2/-/store2-2.14.2.tgz",
- "integrity": "sha512-siT1RiqlfQnGqgT/YzXVUNsom9S0H1OX+dpdGN1xkyYATo4I6sep5NmsRD/40s3IIOvlCq6akxkqG82urIZW1w==",
- "dev": true
- },
"node_modules/storybook": {
- "version": "7.6.15",
- "resolved": "https://registry.npmjs.org/storybook/-/storybook-7.6.15.tgz",
- "integrity": "sha512-Ybezq9JRk5CBhzjgzZ/oT7mnU45UwhyVSGKW+PUKZGGUG9VH2hCrTEES9f/zEF82kj/5COVPyqR/5vlXuuS39A==",
+ "version": "8.4.7",
+ "resolved": "https://registry.npmjs.org/storybook/-/storybook-8.4.7.tgz",
+ "integrity": "sha512-RP/nMJxiWyFc8EVMH5gp20ID032Wvk+Yr3lmKidoegto5Iy+2dVQnUoElZb2zpbVXNHWakGuAkfI0dY1Hfp/vw==",
"dev": true,
"dependencies": {
- "@storybook/cli": "7.6.15"
+ "@storybook/core": "8.4.7"
},
"bin": {
- "sb": "index.js",
- "storybook": "index.js"
+ "getstorybook": "bin/index.cjs",
+ "sb": "bin/index.cjs",
+ "storybook": "bin/index.cjs"
},
"funding": {
"type": "opencollective",
"url": "https://opencollective.com/storybook"
+ },
+ "peerDependencies": {
+ "prettier": "^2 || ^3"
+ },
+ "peerDependenciesMeta": {
+ "prettier": {
+ "optional": true
+ }
}
},
"node_modules/storybook-source-link": {
@@ -46608,7 +43269,6 @@
"version": "4.2.3",
"resolved": "https://registry.npmjs.org/string-width/-/string-width-4.2.3.tgz",
"integrity": "sha512-wKyQRQpjJ0sIp62ErSZdGsjMJWsap5oRNihHhu6G7JVO/9jIB6UyevL+tXuOqrng8j/cxKTWyWUwvSTriiZz/g==",
- "dev": true,
"dependencies": {
"emoji-regex": "^8.0.0",
"is-fullwidth-code-point": "^3.0.0",
@@ -46621,14 +43281,12 @@
"node_modules/string-width-cjs/node_modules/emoji-regex": {
"version": "8.0.0",
"resolved": "https://registry.npmjs.org/emoji-regex/-/emoji-regex-8.0.0.tgz",
- "integrity": "sha512-MSjYzcWNOA0ewAHpz0MxpYFvwg6yjy1NG3xteoqz644VCo/RPgnr1/GGt+ic3iJTzQ8Eu3TdM14SawnVUmGE6A==",
- "dev": true
+ "integrity": "sha512-MSjYzcWNOA0ewAHpz0MxpYFvwg6yjy1NG3xteoqz644VCo/RPgnr1/GGt+ic3iJTzQ8Eu3TdM14SawnVUmGE6A=="
},
"node_modules/string-width-cjs/node_modules/is-fullwidth-code-point": {
"version": "3.0.0",
"resolved": "https://registry.npmjs.org/is-fullwidth-code-point/-/is-fullwidth-code-point-3.0.0.tgz",
"integrity": "sha512-zymm5+u+sCsSWyD9qNaejV3DFvhCKclKdizYaJUuHA83RLjb7nSuGnddCHGv0hk+KY7BMAlsWeK4Ueg6EV6XQg==",
- "dev": true,
"engines": {
"node": ">=8"
}
@@ -46772,7 +43430,6 @@
"version": "6.0.1",
"resolved": "https://registry.npmjs.org/strip-ansi/-/strip-ansi-6.0.1.tgz",
"integrity": "sha512-Y38VPSHcqkFrCpFnQ9vuSXmquuv5oXOKpGeT6aGrr3o3Gc9AlVa6JBfUSOCnbxGGZF+/0ooI7KrPuUSztUdU5A==",
- "dev": true,
"dependencies": {
"ansi-regex": "^5.0.1"
},
@@ -46849,6 +43506,7 @@
"resolved": "https://registry.npmjs.org/strong-log-transformer/-/strong-log-transformer-2.1.0.tgz",
"integrity": "sha512-B3Hgul+z0L9a236FAUC9iZsL+nVHgoCJnqCbN588DjYxvGXaXaaFbfmQ/JhvKjZwsOukuR72XbHv71Qkug0HxA==",
"dev": true,
+ "license": "Apache-2.0",
"dependencies": {
"duplexer": "^0.1.1",
"minimist": "^1.2.0",
@@ -47415,19 +44073,6 @@
"node": ">=0.10.0"
}
},
- "node_modules/swc-loader": {
- "version": "0.2.6",
- "resolved": "https://registry.npmjs.org/swc-loader/-/swc-loader-0.2.6.tgz",
- "integrity": "sha512-9Zi9UP2YmDpgmQVbyOPJClY0dwf58JDyDMQ7uRc4krmc72twNI2fvlBWHLqVekBpPc7h5NJkGVT1zNDxFrqhvg==",
- "dev": true,
- "dependencies": {
- "@swc/counter": "^0.1.3"
- },
- "peerDependencies": {
- "@swc/core": "^1.2.147",
- "webpack": ">=2"
- }
- },
"node_modules/symbol-observable": {
"version": "1.2.0",
"resolved": "https://registry.npmjs.org/symbol-observable/-/symbol-observable-1.2.0.tgz",
@@ -47442,12 +44087,6 @@
"resolved": "https://registry.npmjs.org/symbol-tree/-/symbol-tree-3.2.4.tgz",
"integrity": "sha512-9QNk5KwDF+Bvz+PyObkmSYjI5ksVUYtjW7AU22r2NKcfLJcXp96hkDWU3+XndOsUb+AQ9QhfzfCT2O+CNWT5Tw=="
},
- "node_modules/synchronous-promise": {
- "version": "2.0.17",
- "resolved": "https://registry.npmjs.org/synchronous-promise/-/synchronous-promise-2.0.17.tgz",
- "integrity": "sha512-AsS729u2RHUfEra9xJrE39peJcc2stq2+poBXX8bcM08Y6g9j/i/PUzwNQqkaJde7Ntg1TO7bSREbR5sdosQ+g==",
- "dev": true
- },
"node_modules/synckit": {
"version": "0.8.5",
"resolved": "https://registry.npmjs.org/synckit/-/synckit-0.8.5.tgz",
@@ -47546,20 +44185,21 @@
}
},
"node_modules/tar": {
- "version": "6.1.11",
- "resolved": "https://registry.npmjs.org/tar/-/tar-6.1.11.tgz",
- "integrity": "sha512-an/KZQzQUkZCkuoAA64hM92X0Urb6VpRhAFllDzz44U2mcD5scmT3zBc4VgVpkugF580+DQn8eAFSyoQt0tznA==",
+ "version": "6.2.1",
+ "resolved": "https://registry.npmjs.org/tar/-/tar-6.2.1.tgz",
+ "integrity": "sha512-DZ4yORTwrbTj/7MZYq2w+/ZFdI6OZ/f9SFHR+71gIVUZhOQPHzVCLpvRnPgyaMpfWxxk/4ONva3GQSyNIKRv6A==",
"dev": true,
+ "license": "ISC",
"dependencies": {
"chownr": "^2.0.0",
"fs-minipass": "^2.0.0",
- "minipass": "^3.0.0",
+ "minipass": "^5.0.0",
"minizlib": "^2.1.1",
"mkdirp": "^1.0.3",
"yallist": "^4.0.0"
},
"engines": {
- "node": ">= 10"
+ "node": ">=10"
}
},
"node_modules/tar-fs": {
@@ -47567,6 +44207,7 @@
"resolved": "https://registry.npmjs.org/tar-fs/-/tar-fs-2.1.1.tgz",
"integrity": "sha512-V0r2Y9scmbDRLCNex/+hYzvp/zyYjvFbHPNgVTKfQvVrb6guiE/fxP+XblDNR011utopbkex2nM4dHNV6GDsng==",
"dev": true,
+ "optional": true,
"dependencies": {
"chownr": "^1.1.1",
"mkdirp-classic": "^0.5.2",
@@ -47580,6 +44221,7 @@
"integrity": "sha512-tUPXtzlGM8FE3P0ZL6DVs/3P58k9nk8/jZeQCurTJylQA8qFYzHFfhBJkuqyE0FifOsQ0uKWekiZ5g8wtr28cw==",
"dev": true,
"license": "MIT",
+ "optional": true,
"dependencies": {
"end-of-stream": "^1.1.0",
"once": "^1.3.1"
@@ -47620,15 +44262,27 @@
"resolved": "https://registry.npmjs.org/chownr/-/chownr-2.0.0.tgz",
"integrity": "sha512-bIomtDF5KGpdogkLd9VspvFzk9KfpyyGlS8YFVZl7TGPBHL5snIOnxeshwVgPteQ9b4Eydl+pVbIyE1DcvCWgQ==",
"dev": true,
+ "license": "ISC",
"engines": {
"node": ">=10"
}
},
+ "node_modules/tar/node_modules/minipass": {
+ "version": "5.0.0",
+ "resolved": "https://registry.npmjs.org/minipass/-/minipass-5.0.0.tgz",
+ "integrity": "sha512-3FnjYuehv9k6ovOEbyOswadCDPX1piCfhV8ncmYtHOjuPwylVWsghTLo7rabjC3Rx5xD4HDx8Wm1xnMF7S5qFQ==",
+ "dev": true,
+ "license": "ISC",
+ "engines": {
+ "node": ">=8"
+ }
+ },
"node_modules/tar/node_modules/mkdirp": {
"version": "1.0.4",
"resolved": "https://registry.npmjs.org/mkdirp/-/mkdirp-1.0.4.tgz",
"integrity": "sha512-vVqVZQyf3WLx2Shd0qJ9xuvqgAyKPLAiqITEtqW0oIUjzo3PePDd6fW9iFz30ef7Ysp/oiWqbhszeGWW2T6Gzw==",
"dev": true,
+ "license": "MIT",
"bin": {
"mkdirp": "bin/cmd.js"
},
@@ -47636,12 +44290,6 @@
"node": ">=10"
}
},
- "node_modules/tar/node_modules/yallist": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/yallist/-/yallist-4.0.0.tgz",
- "integrity": "sha512-3wdGidZyq5PB084XLES5TpOSRA3wjXAlIWMhum2kRcv/41Sn2emQ0dycQW4uZXLejwKvg6EsvbdlVL+FYEct7A==",
- "dev": true
- },
"node_modules/teen_process": {
"version": "2.0.2",
"resolved": "https://registry.npmjs.org/teen_process/-/teen_process-2.0.2.tgz",
@@ -47693,15 +44341,6 @@
"node": ">= 8"
}
},
- "node_modules/telejson": {
- "version": "7.2.0",
- "resolved": "https://registry.npmjs.org/telejson/-/telejson-7.2.0.tgz",
- "integrity": "sha512-1QTEcJkJEhc8OnStBx/ILRu5J2p0GjvWsBx56bmZRqnrkdBMUe+nX92jxV+p3dB4CP6PZCdJMQJwCggkNBMzkQ==",
- "dev": true,
- "dependencies": {
- "memoizerific": "^1.11.3"
- }
- },
"node_modules/temp": {
"version": "0.8.4",
"resolved": "https://registry.npmjs.org/temp/-/temp-0.8.4.tgz",
@@ -47718,6 +44357,7 @@
"resolved": "https://registry.npmjs.org/temp-dir/-/temp-dir-1.0.0.tgz",
"integrity": "sha512-xZFXEGbG7SNC3itwBzI3RYjq/cEhBkx2hJuKGIUOcEULmkQExXiHat2z/qkISYsuR+IKumhEfKKbV5qXmhICFQ==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=4"
}
@@ -47752,89 +44392,6 @@
"rimraf": "bin.js"
}
},
- "node_modules/tempy": {
- "version": "1.0.1",
- "resolved": "https://registry.npmjs.org/tempy/-/tempy-1.0.1.tgz",
- "integrity": "sha512-biM9brNqxSc04Ee71hzFbryD11nX7VPhQQY32AdDmjFvodsRFz/3ufeoTZ6uYkRFfGo188tENcASNs3vTdsM0w==",
- "dev": true,
- "dependencies": {
- "del": "^6.0.0",
- "is-stream": "^2.0.0",
- "temp-dir": "^2.0.0",
- "type-fest": "^0.16.0",
- "unique-string": "^2.0.0"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/tempy/node_modules/del": {
- "version": "6.1.1",
- "resolved": "https://registry.npmjs.org/del/-/del-6.1.1.tgz",
- "integrity": "sha512-ua8BhapfP0JUJKC/zV9yHHDW/rDoDxP4Zhn3AkA6/xT6gY7jYXJiaeyBZznYVujhZZET+UgcbZiQ7sN3WqcImg==",
- "dev": true,
- "dependencies": {
- "globby": "^11.0.1",
- "graceful-fs": "^4.2.4",
- "is-glob": "^4.0.1",
- "is-path-cwd": "^2.2.0",
- "is-path-inside": "^3.0.2",
- "p-map": "^4.0.0",
- "rimraf": "^3.0.2",
- "slash": "^3.0.0"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/tempy/node_modules/is-path-inside": {
- "version": "3.0.3",
- "resolved": "https://registry.npmjs.org/is-path-inside/-/is-path-inside-3.0.3.tgz",
- "integrity": "sha512-Fd4gABb+ycGAmKou8eMftCupSir5lRxqf4aD/vd0cD2qc4HL07OjCeuHMr8Ro4CoMaeCKDB0/ECBOVWjTwUvPQ==",
- "dev": true,
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/tempy/node_modules/is-stream": {
- "version": "2.0.1",
- "resolved": "https://registry.npmjs.org/is-stream/-/is-stream-2.0.1.tgz",
- "integrity": "sha512-hFoiJiTl63nn+kstHGBtewWSKnQLpyb155KHheA1l39uvtO9nWIop1p3udqPcUd/xbF1VLMO4n7OI6p7RbngDg==",
- "dev": true,
- "engines": {
- "node": ">=8"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "node_modules/tempy/node_modules/temp-dir": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/temp-dir/-/temp-dir-2.0.0.tgz",
- "integrity": "sha512-aoBAniQmmwtcKp/7BzsH8Cxzv8OL736p7v1ihGb5e9DJ9kTwGWHrQrVB5+lfVDzfGrdRzXch+ig7LHaY1JTOrg==",
- "dev": true,
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/tempy/node_modules/type-fest": {
- "version": "0.16.0",
- "resolved": "https://registry.npmjs.org/type-fest/-/type-fest-0.16.0.tgz",
- "integrity": "sha512-eaBzG6MxNzEn9kiwvtre90cXaNLkmadMWa1zQMs3XORCXNbsH/OewwbxC5ia9dCxIxnTAsSxXJaa/p5y8DlvJg==",
- "dev": true,
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
"node_modules/terminal-link": {
"version": "2.0.0",
"resolved": "https://registry.npmjs.org/terminal-link/-/terminal-link-2.0.0.tgz",
@@ -48026,6 +44583,7 @@
"resolved": "https://registry.npmjs.org/text-extensions/-/text-extensions-1.9.0.tgz",
"integrity": "sha512-wiBrwC1EhBelW12Zy26JeOUkQ5mRu+5o8rpsJk5+2t+Y5vE7e842qtZDQ2g1NpX/29HdyFeJ4nSIhI47ENSxlQ==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=0.10"
}
@@ -48042,9 +44600,10 @@
"integrity": "sha512-N+8UisAXDGk8PFXP4HAzVR9nbfmVJ3zYLAWiTIoqC5v5isinhr+r5uaO8+7r3BMfuNIufIsA7RdpVgacC2cSpw=="
},
"node_modules/third-party-web": {
- "version": "0.23.4",
- "resolved": "https://registry.npmjs.org/third-party-web/-/third-party-web-0.23.4.tgz",
- "integrity": "sha512-kwYnSZRhEvv0SBW2fp8SBBKRglMoBjV8xz6C31m0ewqOtknB5UL+Ihg+M81hyFY5ldkZuGWPb+e4GVDkzf/gYg=="
+ "version": "0.26.2",
+ "resolved": "https://registry.npmjs.org/third-party-web/-/third-party-web-0.26.2.tgz",
+ "integrity": "sha512-taJ0Us0lKoYBqcbccMuDElSUPOxmBfwlHe1OkHQ3KFf+RwovvBHdXhbFk9XJVQE2vHzxbTwvwg5GFsT9hbDokQ==",
+ "license": "MIT"
},
"node_modules/throat": {
"version": "5.0.0",
@@ -48093,6 +44652,24 @@
"integrity": "sha512-+FbBPE1o9QAYvviau/qC5SE3caw21q3xkvWKBtja5vgqOWIHHJ3ioaq1VPfn/Szqctz2bU/oYeKd9/z5BL+PVg==",
"dev": true
},
+ "node_modules/tinyrainbow": {
+ "version": "1.2.0",
+ "resolved": "https://registry.npmjs.org/tinyrainbow/-/tinyrainbow-1.2.0.tgz",
+ "integrity": "sha512-weEDEq7Z5eTHPDh4xjX789+fHfF+P8boiFB+0vbWzpbnbsEr/GRaohi/uMKxg8RZMXnl1ItAi/IUHWMsjDV7kQ==",
+ "dev": true,
+ "engines": {
+ "node": ">=14.0.0"
+ }
+ },
+ "node_modules/tinyspy": {
+ "version": "3.0.2",
+ "resolved": "https://registry.npmjs.org/tinyspy/-/tinyspy-3.0.2.tgz",
+ "integrity": "sha512-n1cw8k1k0x4pgA2+9XrOkFydTerNcJ1zWCO5Nn9scWHTD+5tp8dghT2x1uduQePZTZgd3Tupf+x9BxJjeJi77Q==",
+ "dev": true,
+ "engines": {
+ "node": ">=14.0.0"
+ }
+ },
"node_modules/titleize": {
"version": "3.0.0",
"resolved": "https://registry.npmjs.org/titleize/-/titleize-3.0.0.tgz",
@@ -48116,9 +44693,19 @@
}
},
"node_modules/tldts-core": {
- "version": "6.1.50",
- "resolved": "https://registry.npmjs.org/tldts-core/-/tldts-core-6.1.50.tgz",
- "integrity": "sha512-na2EcZqmdA2iV9zHV7OHQDxxdciEpxrjbkp+aHmZgnZKHzoElLajP59np5/4+sare9fQBfixgvXKx8ev1d7ytw=="
+ "version": "6.1.66",
+ "resolved": "https://registry.npmjs.org/tldts-core/-/tldts-core-6.1.66.tgz",
+ "integrity": "sha512-s07jJruSwndD2X8bVjwioPfqpIc1pDTzszPe9pL1Skbh4bjytL85KNQ3tolqLbCvpQHawIsGfFi9dgerWjqW4g==",
+ "license": "MIT"
+ },
+ "node_modules/tldts-icann": {
+ "version": "6.1.66",
+ "resolved": "https://registry.npmjs.org/tldts-icann/-/tldts-icann-6.1.66.tgz",
+ "integrity": "sha512-f4AgNXjymBX3/EXYrnvjyBhXVQ+NWyPzXjqRb17vr0b6SprZKVNnsWNFJAPI6JkPHCm7dHhFDgyneHQEq5uJRA==",
+ "license": "MIT",
+ "dependencies": {
+ "tldts-core": "^6.1.66"
+ }
},
"node_modules/tmp": {
"version": "0.0.33",
@@ -48194,12 +44781,6 @@
"node": ">=0.10.0"
}
},
- "node_modules/tocbot": {
- "version": "4.25.0",
- "resolved": "https://registry.npmjs.org/tocbot/-/tocbot-4.25.0.tgz",
- "integrity": "sha512-kE5wyCQJ40hqUaRVkyQ4z5+4juzYsv/eK+aqD97N62YH0TxFhzJvo22RUQQZdO3YnXAk42ZOfOpjVdy+Z0YokA==",
- "dev": true
- },
"node_modules/toidentifier": {
"version": "1.0.1",
"resolved": "https://registry.npmjs.org/toidentifier/-/toidentifier-1.0.1.tgz",
@@ -48240,6 +44821,16 @@
"tree-kill": "cli.js"
}
},
+ "node_modules/treeverse": {
+ "version": "3.0.0",
+ "resolved": "https://registry.npmjs.org/treeverse/-/treeverse-3.0.0.tgz",
+ "integrity": "sha512-gcANaAnd2QDZFmHFEOF4k7uc1J/6a6z3DJMd/QwEyxLoKGiptJRwid582r7QIsFlFMIZ3SnxfS52S4hm2DHkuQ==",
+ "dev": true,
+ "license": "ISC",
+ "engines": {
+ "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ }
+ },
"node_modules/trim": {
"version": "0.0.1",
"resolved": "https://registry.npmjs.org/trim/-/trim-0.0.1.tgz",
@@ -48401,17 +44992,18 @@
"dev": true
},
"node_modules/tuf-js": {
- "version": "1.1.7",
- "resolved": "https://registry.npmjs.org/tuf-js/-/tuf-js-1.1.7.tgz",
- "integrity": "sha512-i3P9Kgw3ytjELUfpuKVDNBJvk4u5bXL6gskv572mcevPbSKCV3zt3djhmlEQ65yERjIbOSncy7U4cQJaB1CBCg==",
+ "version": "2.2.1",
+ "resolved": "https://registry.npmjs.org/tuf-js/-/tuf-js-2.2.1.tgz",
+ "integrity": "sha512-GwIJau9XaA8nLVbUXsN3IlFi7WmQ48gBUrl3FTkkL/XLu/POhBzfmX9hd33FNMX1qAsfl6ozO1iMmW9NC8YniA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
- "@tufjs/models": "1.0.4",
+ "@tufjs/models": "2.0.1",
"debug": "^4.3.4",
- "make-fetch-happen": "^11.1.1"
+ "make-fetch-happen": "^13.0.1"
},
"engines": {
- "node": "^14.17.0 || ^16.13.0 || >=18.0.0"
+ "node": "^16.14.0 || >=18.0.0"
}
},
"node_modules/tunnel": {
@@ -48427,6 +45019,7 @@
"resolved": "https://registry.npmjs.org/tunnel-agent/-/tunnel-agent-0.6.0.tgz",
"integrity": "sha512-McnNiV1l8RYeY8tBgEpuodCC1mLUdbSN+CYBL7kJsJNInOP8UjDDEwdk6Mw60vdLLrr5NHKZhMAOSrR2NZuQ+w==",
"dev": true,
+ "license": "Apache-2.0",
"optional": true,
"dependencies": {
"safe-buffer": "^5.0.1"
@@ -48633,9 +45226,9 @@
}
},
"node_modules/typescript": {
- "version": "5.5.3",
- "resolved": "https://registry.npmjs.org/typescript/-/typescript-5.5.3.tgz",
- "integrity": "sha512-/hreyEujaB0w76zKo6717l3L0o/qEUtRgdvUBvlkhoWeOVMjMuHNHk0BRBzikzuGDqNmPQbg5ifMEqsHLiIUcQ==",
+ "version": "5.7.2",
+ "resolved": "https://registry.npmjs.org/typescript/-/typescript-5.7.2.tgz",
+ "integrity": "sha512-i5t66RHxDvVN40HfDd1PsEThGNnlMCMT3jMUuoh9/0TaqWevNontacunWyN02LA9/fIbEWlcHZcgTKb9QoaLfg==",
"dev": true,
"license": "Apache-2.0",
"bin": {
@@ -48651,12 +45244,6 @@
"resolved": "https://registry.npmjs.org/uc.micro/-/uc.micro-1.0.6.tgz",
"integrity": "sha512-8Y75pvTYkLJW2hWQHXxoqRgV7qb9B+9vFEtidML+7koHUFapnVJAZ6cKs+Qjz5Aw3aZWHMC6u0wJE3At+nSGwA=="
},
- "node_modules/ufo": {
- "version": "1.4.0",
- "resolved": "https://registry.npmjs.org/ufo/-/ufo-1.4.0.tgz",
- "integrity": "sha512-Hhy+BhRBleFjpJ2vchUNN40qgkh0366FWJGqVLYBHev0vpHTrXSA0ryT+74UiW6KWsldNurQMKGqCm1M2zBciQ==",
- "dev": true
- },
"node_modules/uglify-js": {
"version": "3.13.7",
"resolved": "https://registry.npmjs.org/uglify-js/-/uglify-js-3.13.7.tgz",
@@ -48729,9 +45316,9 @@
}
},
"node_modules/undici-types": {
- "version": "5.26.5",
- "resolved": "https://registry.npmjs.org/undici-types/-/undici-types-5.26.5.tgz",
- "integrity": "sha512-JlCMO+ehdEIKqlFxk6IfVoAUVmgz7cU7zD/h9XZ0qzeosSHmUJVOzSQvvYSYWXkFXC+IfLKSIffhv0sVZup6pA=="
+ "version": "6.20.0",
+ "resolved": "https://registry.npmjs.org/undici-types/-/undici-types-6.20.0.tgz",
+ "integrity": "sha512-Ny6QZ2Nju20vw1SRHe3d9jVu6gJ+4e3+MMpqu7pqE5HT6WsTSlce++GQmK5UXS8mzV8DSYHrQH+Xrf2jVcuKNg=="
},
"node_modules/unherit": {
"version": "1.1.1",
@@ -48930,23 +45517,23 @@
}
},
"node_modules/unplugin": {
- "version": "1.7.1",
- "resolved": "https://registry.npmjs.org/unplugin/-/unplugin-1.7.1.tgz",
- "integrity": "sha512-JqzORDAPxxs8ErLV4x+LL7bk5pk3YlcWqpSNsIkAZj972KzFZLClc/ekppahKkOczGkwIG6ElFgdOgOlK4tXZw==",
+ "version": "1.16.0",
+ "resolved": "https://registry.npmjs.org/unplugin/-/unplugin-1.16.0.tgz",
+ "integrity": "sha512-5liCNPuJW8dqh3+DM6uNM2EI3MLLpCKp/KY+9pB5M2S2SR2qvvDHhKgBOaTWEbZTAws3CXfB0rKTIolWKL05VQ==",
"dev": true,
"dependencies": {
- "acorn": "^8.11.3",
- "chokidar": "^3.5.3",
- "webpack-sources": "^3.2.3",
- "webpack-virtual-modules": "^0.6.1"
+ "acorn": "^8.14.0",
+ "webpack-virtual-modules": "^0.6.2"
+ },
+ "engines": {
+ "node": ">=14.0.0"
}
},
"node_modules/unplugin/node_modules/acorn": {
- "version": "8.13.0",
- "resolved": "https://registry.npmjs.org/acorn/-/acorn-8.13.0.tgz",
- "integrity": "sha512-8zSiw54Oxrdym50NlZ9sUusyO1Z1ZchgRLWRaK6c86XJFClyCgFKetdowBg5bKxyp/u+CDBJG4Mpp0m3HLZl9w==",
+ "version": "8.14.0",
+ "resolved": "https://registry.npmjs.org/acorn/-/acorn-8.14.0.tgz",
+ "integrity": "sha512-cl669nCJTZBsL97OF4kUQm5g5hC2uihk0NxY3WENAC0TYdILVkAyHymAntgxGkl7K+t0cXIrH5siy5S4XkFycA==",
"dev": true,
- "license": "MIT",
"bin": {
"acorn": "bin/acorn"
},
@@ -48954,13 +45541,6 @@
"node": ">=0.4.0"
}
},
- "node_modules/unplugin/node_modules/webpack-virtual-modules": {
- "version": "0.6.2",
- "resolved": "https://registry.npmjs.org/webpack-virtual-modules/-/webpack-virtual-modules-0.6.2.tgz",
- "integrity": "sha512-66/V2i5hQanC51vBQKPH4aI8NMAcBW59FVBs+rC7eGHupMyfn34q7rZIE+ETlJ+XTevqfUhVVBgSUNSW2flEUQ==",
- "dev": true,
- "license": "MIT"
- },
"node_modules/unset-value": {
"version": "1.0.0",
"resolved": "https://registry.npmjs.org/unset-value/-/unset-value-1.0.0.tgz",
@@ -49017,30 +45597,6 @@
"node": ">=8"
}
},
- "node_modules/unzipper": {
- "version": "0.10.14",
- "resolved": "https://registry.npmjs.org/unzipper/-/unzipper-0.10.14.tgz",
- "integrity": "sha512-ti4wZj+0bQTiX2KmKWuwj7lhV+2n//uXEotUmGuQqrbVZSEGFMbI68+c6JCQ8aAmUWYvtHEz2A8K6wXvueR/6g==",
- "dev": true,
- "dependencies": {
- "big-integer": "^1.6.17",
- "binary": "~0.3.0",
- "bluebird": "~3.4.1",
- "buffer-indexof-polyfill": "~1.0.0",
- "duplexer2": "~0.1.4",
- "fstream": "^1.0.12",
- "graceful-fs": "^4.2.2",
- "listenercount": "~1.0.1",
- "readable-stream": "~2.3.6",
- "setimmediate": "~1.0.4"
- }
- },
- "node_modules/unzipper/node_modules/bluebird": {
- "version": "3.4.7",
- "resolved": "https://registry.npmjs.org/bluebird/-/bluebird-3.4.7.tgz",
- "integrity": "sha512-iD3898SR7sWVRHbiQv+sHUtHnMvC1o3nW5rAcqnq3uOn07DSAppZYUkIGslDz6gXC7HfunPe7YVBgoEJASPcHA==",
- "dev": true
- },
"node_modules/upath": {
"version": "1.2.0",
"resolved": "https://registry.npmjs.org/upath/-/upath-1.2.0.tgz",
@@ -49195,26 +45751,11 @@
"integrity": "sha512-jmYNElW7yvO7TV33CjSmvSiE2yco3bV2czu/OzDKdMNVZQWfxCblURLhf+47syQRBntjfLdd/H0egrzIG+oaFQ==",
"dev": true
},
- "node_modules/url/node_modules/qs": {
- "version": "6.13.0",
- "resolved": "https://registry.npmjs.org/qs/-/qs-6.13.0.tgz",
- "integrity": "sha512-+38qI9SOr8tfZ4QmJNplMUxqjbe7LKvvZgWdExBOmd+egZTtjLB67Gu0HRX3u/XOq7UU2Nx6nsjvS16Z9uwfpg==",
- "dev": true,
- "license": "BSD-3-Clause",
- "dependencies": {
- "side-channel": "^1.0.6"
- },
- "engines": {
- "node": ">=0.6"
- },
- "funding": {
- "url": "https://github.com/sponsors/ljharb"
- }
- },
"node_modules/urlpattern-polyfill": {
"version": "10.0.0",
"resolved": "https://registry.npmjs.org/urlpattern-polyfill/-/urlpattern-polyfill-10.0.0.tgz",
- "integrity": "sha512-H/A06tKD7sS1O1X2SshBVeA5FLycRpjqiBeqGKmBwBDBy28EnRjORxTNe269KSSr5un5qyWi1iL61wLxpd+ZOg=="
+ "integrity": "sha512-H/A06tKD7sS1O1X2SshBVeA5FLycRpjqiBeqGKmBwBDBy28EnRjORxTNe269KSSr5un5qyWi1iL61wLxpd+ZOg==",
+ "license": "MIT"
},
"node_modules/use": {
"version": "3.1.1",
@@ -49262,19 +45803,6 @@
"react": "^16.8.0"
}
},
- "node_modules/use-resize-observer": {
- "version": "9.1.0",
- "resolved": "https://registry.npmjs.org/use-resize-observer/-/use-resize-observer-9.1.0.tgz",
- "integrity": "sha512-R25VqO9Wb3asSD4eqtcxk8sJalvIOYBqS8MNZlpDSQ4l4xMQxC/J7Id9HoTqPq8FwULIn0PVW+OAqF2dyYbjow==",
- "dev": true,
- "dependencies": {
- "@juggle/resize-observer": "^3.3.1"
- },
- "peerDependencies": {
- "react": "16.8.0 - 18",
- "react-dom": "16.8.0 - 18"
- }
- },
"node_modules/use-sidecar": {
"version": "1.1.2",
"resolved": "https://registry.npmjs.org/use-sidecar/-/use-sidecar-1.1.2.tgz",
@@ -49308,18 +45836,20 @@
}
},
"node_modules/use-sync-external-store": {
- "version": "1.2.0",
- "resolved": "https://registry.npmjs.org/use-sync-external-store/-/use-sync-external-store-1.2.0.tgz",
- "integrity": "sha512-eEgnFxGQ1Ife9bzYs6VLi8/4X6CObHMw9Qr9tPY43iKwsPw8xE8+EFsf/2cFZ5S3esXgpWgtSCtLNS41F+sKPA==",
+ "version": "1.2.2",
+ "resolved": "https://registry.npmjs.org/use-sync-external-store/-/use-sync-external-store-1.2.2.tgz",
+ "integrity": "sha512-PElTlVMwpblvbNqQ82d2n6RjStvdSoNe9FG28kNfz3WiXilJm4DdNkEzRhCZuIDwY8U08WVihhGR5iRqAwfDiw==",
+ "license": "MIT",
"peerDependencies": {
"react": "^16.8.0 || ^17.0.0 || ^18.0.0"
}
},
"node_modules/userhome": {
- "version": "1.0.0",
- "resolved": "https://registry.npmjs.org/userhome/-/userhome-1.0.0.tgz",
- "integrity": "sha512-ayFKY3H+Pwfy4W98yPdtH1VqH4psDeyW8lYYFzfecR9d6hqLpqhecktvYR3SEEXt7vG0S1JEpciI3g94pMErig==",
+ "version": "1.0.1",
+ "resolved": "https://registry.npmjs.org/userhome/-/userhome-1.0.1.tgz",
+ "integrity": "sha512-5cnLm4gseXjAclKowC4IjByaGsjtAoV6PrOQOljplNB54ReUYJP8HdAFq2muHinSDAh09PPX/uXDPfdxRHvuSA==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">= 0.8.0"
}
@@ -49511,11 +46041,51 @@
"node": ">=18"
}
},
+ "node_modules/wait-on": {
+ "version": "8.0.1",
+ "resolved": "https://registry.npmjs.org/wait-on/-/wait-on-8.0.1.tgz",
+ "integrity": "sha512-1wWQOyR2LVVtaqrcIL2+OM+x7bkpmzVROa0Nf6FryXkS+er5Sa1kzFGjzZRqLnHa3n1rACFLeTwUqE1ETL9Mig==",
+ "license": "MIT",
+ "dependencies": {
+ "axios": "^1.7.7",
+ "joi": "^17.13.3",
+ "lodash": "^4.17.21",
+ "minimist": "^1.2.8",
+ "rxjs": "^7.8.1"
+ },
+ "bin": {
+ "wait-on": "bin/wait-on"
+ },
+ "engines": {
+ "node": ">=12.0.0"
+ }
+ },
+ "node_modules/wait-on/node_modules/axios": {
+ "version": "1.7.9",
+ "resolved": "https://registry.npmjs.org/axios/-/axios-1.7.9.tgz",
+ "integrity": "sha512-LhLcE7Hbiryz8oMDdDptSrWowmB4Bl6RCt6sIJKpRB4XtVf0iEgewX3au/pJqm+Py1kCASkb/FFKjxQaLtxJvw==",
+ "license": "MIT",
+ "dependencies": {
+ "follow-redirects": "^1.15.6",
+ "form-data": "^4.0.0",
+ "proxy-from-env": "^1.1.0"
+ }
+ },
+ "node_modules/wait-on/node_modules/rxjs": {
+ "version": "7.8.1",
+ "resolved": "https://registry.npmjs.org/rxjs/-/rxjs-7.8.1.tgz",
+ "integrity": "sha512-AA3TVj+0A2iuIoQkWEK/tqFjBq2j+6PO6Y0zJcvzLAFhEFIO3HL0vls9hWLncZbAAbK0mar7oZ4V079I/qPMxg==",
+ "license": "Apache-2.0",
+ "dependencies": {
+ "tslib": "^2.1.0"
+ }
+ },
"node_modules/wait-port": {
"version": "1.1.0",
"resolved": "https://registry.npmjs.org/wait-port/-/wait-port-1.1.0.tgz",
"integrity": "sha512-3e04qkoN3LxTMLakdqeWth8nih8usyg+sf1Bgdf9wwUkp05iuK1eSY/QpLvscT/+F/gA89+LpUmmgBtesbqI2Q==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"chalk": "^4.1.2",
"commander": "^9.3.0",
@@ -49533,6 +46103,7 @@
"resolved": "https://registry.npmjs.org/chalk/-/chalk-4.1.2.tgz",
"integrity": "sha512-oKnbhFyRIXpUuez8iBMmyEa4nbj4IOQyuhc/wy9kY7/WVPcwIO9VA668Pu8RkO7+0G76SLROeyw9CpQ061i4mA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"ansi-styles": "^4.1.0",
"supports-color": "^7.1.0"
@@ -49549,6 +46120,7 @@
"resolved": "https://registry.npmjs.org/commander/-/commander-9.5.0.tgz",
"integrity": "sha512-KRs7WVDKg86PWiuAqhDrAQnTXZKraVcCc6vFdL14qrZ/DcWwuRo7VoiYXalXO7S5GKpqYiVEwCbgFDfxNHKJBQ==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": "^12.20.0 || >=14"
}
@@ -49558,6 +46130,7 @@
"resolved": "https://registry.npmjs.org/has-flag/-/has-flag-4.0.0.tgz",
"integrity": "sha512-EykJT/Q1KjTWctppgIAgfSO0tKVuZUjhgMr17kqTumMl6Afv3EISleU7qZUzoXDFTAHTDC4NOoG/ZxU3EvlMPQ==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=8"
}
@@ -49567,6 +46140,7 @@
"resolved": "https://registry.npmjs.org/supports-color/-/supports-color-7.2.0.tgz",
"integrity": "sha512-qpCAvRl9stuOHveKsn7HncJRvv501qIacKzQlO/+Lwxc9+0q2wLyv4Dfvt80/DPn2pqOBsJdDiogXGR9+OvwRw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"has-flag": "^4.0.0"
},
@@ -49574,6 +46148,13 @@
"node": ">=8"
}
},
+ "node_modules/walk-up-path": {
+ "version": "3.0.1",
+ "resolved": "https://registry.npmjs.org/walk-up-path/-/walk-up-path-3.0.1.tgz",
+ "integrity": "sha512-9YlCL/ynK3CTlrSRrDxZvUauLzAswPCrsaCgilqFevUYpeEW0/3ScEjaa3kbW/T0ghhkEr7mv+fpjqn1Y1YuTA==",
+ "dev": true,
+ "license": "ISC"
+ },
"node_modules/walker": {
"version": "1.0.8",
"resolved": "https://registry.npmjs.org/walker/-/walker-1.0.8.tgz",
@@ -49831,10 +46412,11 @@
}
},
"node_modules/web-streams-polyfill": {
- "version": "3.2.1",
- "resolved": "https://registry.npmjs.org/web-streams-polyfill/-/web-streams-polyfill-3.2.1.tgz",
- "integrity": "sha512-e0MO3wdXWKrLbL0DgGnUV7WHVuw9OUvL4hjgnPkIeEvESk74gAITi5G606JtZPp39cd8HA9VQzCIvA49LpPN5Q==",
+ "version": "3.3.3",
+ "resolved": "https://registry.npmjs.org/web-streams-polyfill/-/web-streams-polyfill-3.3.3.tgz",
+ "integrity": "sha512-d2JWLCivmZYTSIoge9MsgFCZrt571BikcWGYkjC1khllbTeDlGqZ2D8vD8E/lJa8WGWbb7Plm8/XJYV7IJHZZw==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">= 8"
}
@@ -49844,6 +46426,7 @@
"resolved": "https://registry.npmjs.org/webdriver/-/webdriver-8.16.20.tgz",
"integrity": "sha512-3Dynj9pfTqmbDadqmMmD/sQgGFwho92zQPGgpAqLUMebE/qEkraoIfRWdbi2tw1ityiThOJVPTXfwsY/bpvknw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"@types/node": "^20.1.0",
"@types/ws": "^8.5.3",
@@ -49866,6 +46449,7 @@
"resolved": "https://registry.npmjs.org/@sindresorhus/is/-/is-5.6.0.tgz",
"integrity": "sha512-TV7t8GKYaJWsn00tFDqBw8+Uqmr8A0fRU1tvTQhyZzGv0sJCGRQL3JGMI3ucuKo3XIZdUP+Lx7/gh2t3lewy7g==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=14.16"
},
@@ -49878,6 +46462,7 @@
"resolved": "https://registry.npmjs.org/@szmarczak/http-timer/-/http-timer-5.0.1.tgz",
"integrity": "sha512-+PmQX0PiAYPMeVYe237LJAYvOMYW1j2rH5YROyS3b4CTVJum34HfRvKvAzozHAQG0TnHNdUfY9nCeUyRAs//cw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"defer-to-connect": "^2.0.1"
},
@@ -49886,9 +46471,9 @@
}
},
"node_modules/webdriver/node_modules/@types/node": {
- "version": "20.16.15",
- "resolved": "https://registry.npmjs.org/@types/node/-/node-20.16.15.tgz",
- "integrity": "sha512-DV58qQz9dBMqVVn+qnKwGa51QzCD4YM/tQM16qLKxdf5tqz5W4QwtrMzjSTbabN1cFTSuyxVYBy+QWHjWW8X/g==",
+ "version": "20.17.9",
+ "resolved": "https://registry.npmjs.org/@types/node/-/node-20.17.9.tgz",
+ "integrity": "sha512-0JOXkRyLanfGPE2QRCwgxhzlBAvaRdCNMcvbd7jFfpmD4eEXll7LRwy5ymJmyeZqk7Nh7eD2LeUyQ68BbndmXw==",
"dev": true,
"license": "MIT",
"dependencies": {
@@ -49900,6 +46485,7 @@
"resolved": "https://registry.npmjs.org/cacheable-lookup/-/cacheable-lookup-7.0.0.tgz",
"integrity": "sha512-+qJyx4xiKra8mZrcwhjMRMUhD5NR1R8esPkzIYxX96JiecFoxAXFuz/GpR3+ev4PE1WamHip78wV0vcmPQtp8w==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=14.16"
}
@@ -49909,6 +46495,7 @@
"resolved": "https://registry.npmjs.org/cacheable-request/-/cacheable-request-10.2.14.tgz",
"integrity": "sha512-zkDT5WAF4hSSoUgyfg5tFIxz8XQK+25W/TLVojJTMKBaxevLBBtLxgqguAuVQB8PVW79FVjHcU+GJ9tVbDZ9mQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"@types/http-cache-semantics": "^4.0.2",
"get-stream": "^6.0.1",
@@ -49927,6 +46514,7 @@
"resolved": "https://registry.npmjs.org/get-stream/-/get-stream-6.0.1.tgz",
"integrity": "sha512-ts6Wi+2j3jQjqi70w5AlN8DFnkSwC+MqmxEzdEALB2qXZYV3X/b1CTfgPLGJNMeAWxdPfU8FO1ms3NUfaHCPYg==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=10"
},
@@ -49939,6 +46527,7 @@
"resolved": "https://registry.npmjs.org/got/-/got-12.6.1.tgz",
"integrity": "sha512-mThBblvlAF1d4O5oqyvN+ZxLAYwIJK7bpMxgYqPD9okW0C3qm5FFn7k811QrcuEBwaogR3ngOFoCfs6mRv7teQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"@sindresorhus/is": "^5.2.0",
"@szmarczak/http-timer": "^5.0.1",
@@ -49964,6 +46553,7 @@
"resolved": "https://registry.npmjs.org/http2-wrapper/-/http2-wrapper-2.2.1.tgz",
"integrity": "sha512-V5nVw1PAOgfI3Lmeaj2Exmeg7fenjhRUgz1lPSezy1CuhPYbgQtbQj4jZfEAEMlaL+vupsvhjqCyjzob0yxsmQ==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"quick-lru": "^5.1.1",
"resolve-alpn": "^1.2.0"
@@ -49977,6 +46567,7 @@
"resolved": "https://registry.npmjs.org/lowercase-keys/-/lowercase-keys-3.0.0.tgz",
"integrity": "sha512-ozCC6gdQ+glXOQsveKD0YsDy8DSQFjDTz4zyzEHNV5+JP5D62LmfDZ6o1cycFx9ouG940M5dE8C8CTewdj2YWQ==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": "^12.20.0 || ^14.13.1 || >=16.0.0"
},
@@ -49989,6 +46580,7 @@
"resolved": "https://registry.npmjs.org/mimic-response/-/mimic-response-4.0.0.tgz",
"integrity": "sha512-e5ISH9xMYU0DzrT+jl8q2ze9D6eWBto+I8CNpe+VI+K2J/F/k3PdkdTdz4wvGVH4NTpo+NRYTVIuMQEMMcsLqg==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": "^12.20.0 || ^14.13.1 || >=16.0.0"
},
@@ -50001,6 +46593,7 @@
"resolved": "https://registry.npmjs.org/normalize-url/-/normalize-url-8.0.1.tgz",
"integrity": "sha512-IO9QvjUMWxPQQhs60oOu10CRkWCiZzSUkzbXGGV9pviYl1fXYcvkzQ5jV9z8Y6un8ARoVRl4EtC6v6jNqbaJ/w==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=14.16"
},
@@ -50013,6 +46606,7 @@
"resolved": "https://registry.npmjs.org/p-cancelable/-/p-cancelable-3.0.0.tgz",
"integrity": "sha512-mlVgR3PGuzlo0MmTdk4cXqXWlwQDLnONTAg6sm62XkMJEiRxN3GL3SffkYvqwonbkJBcrI7Uvv5Zh9yjvn2iUw==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=12.20"
}
@@ -50022,6 +46616,7 @@
"resolved": "https://registry.npmjs.org/quick-lru/-/quick-lru-5.1.1.tgz",
"integrity": "sha512-WuyALRjWPDGtt/wzJiadO5AXY+8hZ80hVpe6MyivgraREW751X3SbhRvG3eLKOYN+8VEvqLcf3wdnt44Z4S4SA==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=10"
},
@@ -50034,6 +46629,7 @@
"resolved": "https://registry.npmjs.org/responselike/-/responselike-3.0.0.tgz",
"integrity": "sha512-40yHxbNcl2+rzXvZuVkrYohathsSJlMTXKryG5y8uciHv1+xDLHQpgjG64JUO9nrEq2jGLH6IZ8BcZyw3wrweg==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"lowercase-keys": "^3.0.0"
},
@@ -50078,6 +46674,7 @@
"resolved": "https://registry.npmjs.org/webdriverio/-/webdriverio-8.16.20.tgz",
"integrity": "sha512-2xSJDrMxwPF1kucB/r7Wc8yF689GGi7iSKrog7vkkoIiRY25vd3U129iN2mTYgNDyM6SM0kw+GP5W1s73khpYw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"@types/node": "^20.1.0",
"@wdio/config": "8.16.20",
@@ -50116,10 +46713,40 @@
}
}
},
+ "node_modules/webdriverio/node_modules/@puppeteer/browsers": {
+ "version": "1.4.6",
+ "resolved": "https://registry.npmjs.org/@puppeteer/browsers/-/browsers-1.4.6.tgz",
+ "integrity": "sha512-x4BEjr2SjOPowNeiguzjozQbsc6h437ovD/wu+JpaenxVLm3jkgzHY2xOslMTp50HoTvQreMjiexiGQw1sqZlQ==",
+ "dev": true,
+ "license": "Apache-2.0",
+ "dependencies": {
+ "debug": "4.3.4",
+ "extract-zip": "2.0.1",
+ "progress": "2.0.3",
+ "proxy-agent": "6.3.0",
+ "tar-fs": "3.0.4",
+ "unbzip2-stream": "1.4.3",
+ "yargs": "17.7.1"
+ },
+ "bin": {
+ "browsers": "lib/cjs/main-cli.js"
+ },
+ "engines": {
+ "node": ">=16.3.0"
+ },
+ "peerDependencies": {
+ "typescript": ">= 4.7.4"
+ },
+ "peerDependenciesMeta": {
+ "typescript": {
+ "optional": true
+ }
+ }
+ },
"node_modules/webdriverio/node_modules/@types/node": {
- "version": "20.16.15",
- "resolved": "https://registry.npmjs.org/@types/node/-/node-20.16.15.tgz",
- "integrity": "sha512-DV58qQz9dBMqVVn+qnKwGa51QzCD4YM/tQM16qLKxdf5tqz5W4QwtrMzjSTbabN1cFTSuyxVYBy+QWHjWW8X/g==",
+ "version": "20.17.9",
+ "resolved": "https://registry.npmjs.org/@types/node/-/node-20.17.9.tgz",
+ "integrity": "sha512-0JOXkRyLanfGPE2QRCwgxhzlBAvaRdCNMcvbd7jFfpmD4eEXll7LRwy5ymJmyeZqk7Nh7eD2LeUyQ68BbndmXw==",
"dev": true,
"license": "MIT",
"dependencies": {
@@ -50145,11 +46772,65 @@
"balanced-match": "^1.0.0"
}
},
+ "node_modules/webdriverio/node_modules/cliui": {
+ "version": "8.0.1",
+ "resolved": "https://registry.npmjs.org/cliui/-/cliui-8.0.1.tgz",
+ "integrity": "sha512-BSeNnyus75C4//NQ9gQt1/csTXyo/8Sb+afLAkzAptFuMsod9HFokGNudZpi/oQV73hnVK+sR+5PVRMd+Dr7YQ==",
+ "dev": true,
+ "license": "ISC",
+ "dependencies": {
+ "string-width": "^4.2.0",
+ "strip-ansi": "^6.0.1",
+ "wrap-ansi": "^7.0.0"
+ },
+ "engines": {
+ "node": ">=12"
+ }
+ },
"node_modules/webdriverio/node_modules/devtools-protocol": {
"version": "0.0.1203626",
"resolved": "https://registry.npmjs.org/devtools-protocol/-/devtools-protocol-0.0.1203626.tgz",
"integrity": "sha512-nEzHZteIUZfGCZtTiS1fRpC8UZmsfD1SiyPvaUNvS13dvKf666OAm8YTi0+Ca3n1nLEyu49Cy4+dPWpaHFJk9g==",
- "dev": true
+ "dev": true,
+ "license": "BSD-3-Clause"
+ },
+ "node_modules/webdriverio/node_modules/emoji-regex": {
+ "version": "8.0.0",
+ "resolved": "https://registry.npmjs.org/emoji-regex/-/emoji-regex-8.0.0.tgz",
+ "integrity": "sha512-MSjYzcWNOA0ewAHpz0MxpYFvwg6yjy1NG3xteoqz644VCo/RPgnr1/GGt+ic3iJTzQ8Eu3TdM14SawnVUmGE6A==",
+ "dev": true,
+ "license": "MIT"
+ },
+ "node_modules/webdriverio/node_modules/extract-zip": {
+ "version": "2.0.1",
+ "resolved": "https://registry.npmjs.org/extract-zip/-/extract-zip-2.0.1.tgz",
+ "integrity": "sha512-GDhU9ntwuKyGXdZBUgTIe+vXnWj0fppUEtMDL0+idd5Sta8TGpHssn/eusA9mrPr9qNDym6SxAYZjNvCn/9RBg==",
+ "dev": true,
+ "license": "BSD-2-Clause",
+ "dependencies": {
+ "debug": "^4.1.1",
+ "get-stream": "^5.1.0",
+ "yauzl": "^2.10.0"
+ },
+ "bin": {
+ "extract-zip": "cli.js"
+ },
+ "engines": {
+ "node": ">= 10.17.0"
+ },
+ "optionalDependencies": {
+ "@types/yauzl": "^2.9.1"
+ }
+ },
+ "node_modules/webdriverio/node_modules/is-fullwidth-code-point": {
+ "version": "3.0.0",
+ "resolved": "https://registry.npmjs.org/is-fullwidth-code-point/-/is-fullwidth-code-point-3.0.0.tgz",
+ "integrity": "sha512-zymm5+u+sCsSWyD9qNaejV3DFvhCKclKdizYaJUuHA83RLjb7nSuGnddCHGv0hk+KY7BMAlsWeK4Ueg6EV6XQg==",
+ "dev": true,
+ "license": "MIT",
+ "engines": {
+ "node": ">=8"
+ }
},
"node_modules/webdriverio/node_modules/is-plain-obj": {
"version": "4.1.0",
@@ -50163,6 +46844,16 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
+ "node_modules/webdriverio/node_modules/lru-cache": {
+ "version": "7.18.3",
+ "resolved": "https://registry.npmjs.org/lru-cache/-/lru-cache-7.18.3.tgz",
+ "integrity": "sha512-jumlc0BIUrS3qJGgIkWZsyfAM7NCWiBcCDhnd+3NNM5KbBmLTgHVfWBcg6W+rLUsIpzpERPsvwUP7CckAQSOoA==",
+ "dev": true,
+ "license": "ISC",
+ "engines": {
+ "node": ">=12"
+ }
+ },
"node_modules/webdriverio/node_modules/minimatch": {
"version": "9.0.5",
"resolved": "https://registry.npmjs.org/minimatch/-/minimatch-9.0.5.tgz",
@@ -50179,11 +46870,43 @@
"url": "https://github.com/sponsors/isaacs"
}
},
+ "node_modules/webdriverio/node_modules/proxy-agent": {
+ "version": "6.3.0",
+ "resolved": "https://registry.npmjs.org/proxy-agent/-/proxy-agent-6.3.0.tgz",
+ "integrity": "sha512-0LdR757eTj/JfuU7TL2YCuAZnxWXu3tkJbg4Oq3geW/qFNT/32T0sp2HnZ9O0lMR4q3vwAt0+xCA8SR0WAD0og==",
+ "dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "agent-base": "^7.0.2",
+ "debug": "^4.3.4",
+ "http-proxy-agent": "^7.0.0",
+ "https-proxy-agent": "^7.0.0",
+ "lru-cache": "^7.14.1",
+ "pac-proxy-agent": "^7.0.0",
+ "proxy-from-env": "^1.1.0",
+ "socks-proxy-agent": "^8.0.1"
+ },
+ "engines": {
+ "node": ">= 14"
+ }
+ },
+ "node_modules/webdriverio/node_modules/pump": {
+ "version": "3.0.2",
+ "resolved": "https://registry.npmjs.org/pump/-/pump-3.0.2.tgz",
+ "integrity": "sha512-tUPXtzlGM8FE3P0ZL6DVs/3P58k9nk8/jZeQCurTJylQA8qFYzHFfhBJkuqyE0FifOsQ0uKWekiZ5g8wtr28cw==",
+ "dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "end-of-stream": "^1.1.0",
+ "once": "^1.3.1"
+ }
+ },
"node_modules/webdriverio/node_modules/puppeteer-core": {
"version": "20.9.0",
"resolved": "https://registry.npmjs.org/puppeteer-core/-/puppeteer-core-20.9.0.tgz",
"integrity": "sha512-H9fYZQzMTRrkboEfPmf7m3CLDN6JvbxXA3qTtS+dFt27tR+CsFHzPsT6pzp6lYL6bJbAPaR0HaPO6uSi+F94Pg==",
"dev": true,
+ "license": "Apache-2.0",
"dependencies": {
"@puppeteer/browsers": "1.4.6",
"chromium-bidi": "0.4.16",
@@ -50208,7 +46931,8 @@
"version": "0.0.1147663",
"resolved": "https://registry.npmjs.org/devtools-protocol/-/devtools-protocol-0.0.1147663.tgz",
"integrity": "sha512-hyWmRrexdhbZ1tcJUGpO95ivbRhWXz++F4Ko+n21AY5PNln2ovoJw+8ZMNDTtip+CNFQfrtLVh/w4009dXO/eQ==",
- "dev": true
+ "dev": true,
+ "license": "BSD-3-Clause"
},
"node_modules/webdriverio/node_modules/serialize-error": {
"version": "11.0.3",
@@ -50225,6 +46949,45 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
+ "node_modules/webdriverio/node_modules/string-width": {
+ "version": "4.2.3",
+ "resolved": "https://registry.npmjs.org/string-width/-/string-width-4.2.3.tgz",
+ "integrity": "sha512-wKyQRQpjJ0sIp62ErSZdGsjMJWsap5oRNihHhu6G7JVO/9jIB6UyevL+tXuOqrng8j/cxKTWyWUwvSTriiZz/g==",
+ "dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "emoji-regex": "^8.0.0",
+ "is-fullwidth-code-point": "^3.0.0",
+ "strip-ansi": "^6.0.1"
+ },
+ "engines": {
+ "node": ">=8"
+ }
+ },
+ "node_modules/webdriverio/node_modules/tar-fs": {
+ "version": "3.0.4",
+ "resolved": "https://registry.npmjs.org/tar-fs/-/tar-fs-3.0.4.tgz",
+ "integrity": "sha512-5AFQU8b9qLfZCX9zp2duONhPmZv0hGYiBPJsyUdqMjzq/mqVpy/rEUSeHk1+YitmxugaptgBh5oDGU3VsAJq4w==",
+ "dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "mkdirp-classic": "^0.5.2",
+ "pump": "^3.0.0",
+ "tar-stream": "^3.1.5"
+ }
+ },
+ "node_modules/webdriverio/node_modules/tar-stream": {
+ "version": "3.1.7",
+ "resolved": "https://registry.npmjs.org/tar-stream/-/tar-stream-3.1.7.tgz",
+ "integrity": "sha512-qJj60CXt7IU1Ffyc3NJMjh6EkuCFej46zUqJ4J7pqYlThyd9bO0XBTmcOIhSzZJVWfsLks0+nle/j538YAW9RQ==",
+ "dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "b4a": "^1.6.4",
+ "fast-fifo": "^1.2.0",
+ "streamx": "^2.15.0"
+ }
+ },
"node_modules/webdriverio/node_modules/type-fest": {
"version": "2.19.0",
"resolved": "https://registry.npmjs.org/type-fest/-/type-fest-2.19.0.tgz",
@@ -50244,11 +47007,30 @@
"dev": true,
"license": "MIT"
},
+ "node_modules/webdriverio/node_modules/wrap-ansi": {
+ "version": "7.0.0",
+ "resolved": "https://registry.npmjs.org/wrap-ansi/-/wrap-ansi-7.0.0.tgz",
+ "integrity": "sha512-YVGIj2kamLSTxw6NsZjoBxfSwsn0ycdesmc4p+Q21c5zPuZ1pl+NfxVdxPtdHvmNVOQ6XSYG4AUtyt/Fi7D16Q==",
+ "dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "ansi-styles": "^4.0.0",
+ "string-width": "^4.1.0",
+ "strip-ansi": "^6.0.0"
+ },
+ "engines": {
+ "node": ">=10"
+ },
+ "funding": {
+ "url": "https://github.com/chalk/wrap-ansi?sponsor=1"
+ }
+ },
"node_modules/webdriverio/node_modules/ws": {
"version": "8.13.0",
"resolved": "https://registry.npmjs.org/ws/-/ws-8.13.0.tgz",
"integrity": "sha512-x9vcZYTrFPC7aSIbj7sRCYo7L/Xb8Iy+pW0ng0wt2vCJv7M9HOMy0UoN3rr+IFC7hb7vXoqS+P9ktyLLLhO+LA==",
"dev": true,
+ "license": "MIT",
"engines": {
"node": ">=10.0.0"
},
@@ -50265,23 +47047,63 @@
}
}
},
+ "node_modules/webdriverio/node_modules/y18n": {
+ "version": "5.0.8",
+ "resolved": "https://registry.npmjs.org/y18n/-/y18n-5.0.8.tgz",
+ "integrity": "sha512-0pfFzegeDWJHJIAmTLRP2DwHjdF5s7jo9tuztdQxAhINCdvS+3nGINqPd00AphqJR/0LhANUS6/+7SCb98YOfA==",
+ "dev": true,
+ "license": "ISC",
+ "engines": {
+ "node": ">=10"
+ }
+ },
+ "node_modules/webdriverio/node_modules/yargs": {
+ "version": "17.7.1",
+ "resolved": "https://registry.npmjs.org/yargs/-/yargs-17.7.1.tgz",
+ "integrity": "sha512-cwiTb08Xuv5fqF4AovYacTFNxk62th7LKJ6BL9IGUpTJrWoU7/7WdQGTP2SjKf1dUNBGzDd28p/Yfs/GI6JrLw==",
+ "dev": true,
+ "license": "MIT",
+ "dependencies": {
+ "cliui": "^8.0.1",
+ "escalade": "^3.1.1",
+ "get-caller-file": "^2.0.5",
+ "require-directory": "^2.1.1",
+ "string-width": "^4.2.3",
+ "y18n": "^5.0.5",
+ "yargs-parser": "^21.1.1"
+ },
+ "engines": {
+ "node": ">=12"
+ }
+ },
+ "node_modules/webdriverio/node_modules/yargs-parser": {
+ "version": "21.1.1",
+ "resolved": "https://registry.npmjs.org/yargs-parser/-/yargs-parser-21.1.1.tgz",
+ "integrity": "sha512-tVpsJW7DdjecAiFpbIB1e3qxIQsE6NoPc5/eTdrbbIC4h0LVsWhnoa3g+m2HclBIujHzsxZ4VJVA+GUuc2/LBw==",
+ "dev": true,
+ "license": "ISC",
+ "engines": {
+ "node": ">=12"
+ }
+ },
"node_modules/webidl-conversions": {
"version": "3.0.1",
"resolved": "https://registry.npmjs.org/webidl-conversions/-/webidl-conversions-3.0.1.tgz",
"integrity": "sha512-2JAn3z8AR6rjK8Sm8orRC0h/bcl/DqL7tRPdGZ4I1CjdF+EaMLmYxBHyXuKL849eucPFhvBoxMsflfOb8kxaeQ=="
},
"node_modules/webpack": {
- "version": "5.95.0",
- "resolved": "https://registry.npmjs.org/webpack/-/webpack-5.95.0.tgz",
- "integrity": "sha512-2t3XstrKULz41MNMBF+cJ97TyHdyQ8HCt//pqErqDvNjU9YQBnZxIHa11VXsi7F3mb5/aO2tuDxdeTPdU7xu9Q==",
- "dependencies": {
- "@types/estree": "^1.0.5",
- "@webassemblyjs/ast": "^1.12.1",
- "@webassemblyjs/wasm-edit": "^1.12.1",
- "@webassemblyjs/wasm-parser": "^1.12.1",
- "acorn": "^8.7.1",
- "acorn-import-attributes": "^1.9.5",
- "browserslist": "^4.21.10",
+ "version": "5.97.0",
+ "resolved": "https://registry.npmjs.org/webpack/-/webpack-5.97.0.tgz",
+ "integrity": "sha512-CWT8v7ShSfj7tGs4TLRtaOLmOCPWhoKEvp+eA7FVx8Xrjb3XfT0aXdxDItnRZmE8sHcH+a8ayDrJCOjXKxVFfQ==",
+ "license": "MIT",
+ "dependencies": {
+ "@types/eslint-scope": "^3.7.7",
+ "@types/estree": "^1.0.6",
+ "@webassemblyjs/ast": "^1.14.1",
+ "@webassemblyjs/wasm-edit": "^1.14.1",
+ "@webassemblyjs/wasm-parser": "^1.14.1",
+ "acorn": "^8.14.0",
+ "browserslist": "^4.24.0",
"chrome-trace-event": "^1.0.2",
"enhanced-resolve": "^5.17.1",
"es-module-lexer": "^1.2.1",
@@ -50459,60 +47281,6 @@
"node": ">=14"
}
},
- "node_modules/webpack-cli/node_modules/cross-spawn": {
- "version": "7.0.3",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-7.0.3.tgz",
- "integrity": "sha512-iRDPJKUPVEND7dHPO8rkbOnPpyDygcDFtWjpeWNCgy8WP2rXcxXL8TskReQl6OrB2G7+UJrags1q15Fudc7G6w==",
- "dependencies": {
- "path-key": "^3.1.0",
- "shebang-command": "^2.0.0",
- "which": "^2.0.1"
- },
- "engines": {
- "node": ">= 8"
- }
- },
- "node_modules/webpack-cli/node_modules/path-key": {
- "version": "3.1.1",
- "resolved": "https://registry.npmjs.org/path-key/-/path-key-3.1.1.tgz",
- "integrity": "sha512-ojmeN0qd+y0jszEtoY48r0Peq5dwMEkIlCOu6Q5f41lfkswXuKtYrhgoTpLnyIcHm24Uhqx+5Tqm2InSwLhE6Q==",
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/webpack-cli/node_modules/shebang-command": {
- "version": "2.0.0",
- "resolved": "https://registry.npmjs.org/shebang-command/-/shebang-command-2.0.0.tgz",
- "integrity": "sha512-kHxr2zZpYtdmrN1qDjrrX/Z1rR1kG8Dx+gkpK1G4eXmvXswmcE1hTWBWYUzlraYw1/yZp6YuDY77YtvbN0dmDA==",
- "dependencies": {
- "shebang-regex": "^3.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/webpack-cli/node_modules/shebang-regex": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/shebang-regex/-/shebang-regex-3.0.0.tgz",
- "integrity": "sha512-7++dFhtcx3353uBaq8DDR4NuxBetBzC7ZQOhmTQInHEd6bSrXdiEyzCvG07Z44UYdLShWUyXt5M/yhz8ekcb1A==",
- "engines": {
- "node": ">=8"
- }
- },
- "node_modules/webpack-cli/node_modules/which": {
- "version": "2.0.2",
- "resolved": "https://registry.npmjs.org/which/-/which-2.0.2.tgz",
- "integrity": "sha512-BLI3Tl1TW3Pvl70l3yq3Y64i+awpwXqsGBYWkkqMtnbXgrMD+yj7rhW0kuEDxzJaYXGjEW5ogapKNMEKNMjibA==",
- "dependencies": {
- "isexe": "^2.0.0"
- },
- "bin": {
- "node-which": "bin/node-which"
- },
- "engines": {
- "node": ">= 8"
- }
- },
"node_modules/webpack-dev-middleware": {
"version": "6.1.3",
"resolved": "https://registry.npmjs.org/webpack-dev-middleware/-/webpack-dev-middleware-6.1.3.tgz",
@@ -50546,7 +47314,6 @@
"resolved": "https://registry.npmjs.org/ajv/-/ajv-8.17.1.tgz",
"integrity": "sha512-B/gBuNg5SiMTrPkC+A2+cW0RszwxYmn6VYxB/inlBStS5nx6xHIt/ehKRhIMhqusl7a8LjQoZnjCs5vhwxOQ1g==",
"dev": true,
- "license": "MIT",
"dependencies": {
"fast-deep-equal": "^3.1.3",
"fast-uri": "^3.0.1",
@@ -50583,9 +47350,9 @@
"dev": true
},
"node_modules/webpack-dev-middleware/node_modules/schema-utils": {
- "version": "4.2.0",
- "resolved": "https://registry.npmjs.org/schema-utils/-/schema-utils-4.2.0.tgz",
- "integrity": "sha512-L0jRsrPpjdckP3oPug3/VxNKt2trR8TcabrM6FOAAlvC/9Phcmm+cuAgTlxBqdBR1WJx7Naj9WHw+aOmheSVbw==",
+ "version": "4.3.0",
+ "resolved": "https://registry.npmjs.org/schema-utils/-/schema-utils-4.3.0.tgz",
+ "integrity": "sha512-Gf9qqc58SpCA/xdziiHz35F4GNIWYWZrEshUc/G/r5BnLph6xpKuLeoJoQuj5WfBIx/eQLf+hmVPYHaxJu7V2g==",
"dev": true,
"dependencies": {
"@types/json-schema": "^7.0.9",
@@ -50594,7 +47361,7 @@
"ajv-keywords": "^5.1.0"
},
"engines": {
- "node": ">= 12.13.0"
+ "node": ">= 10.13.0"
},
"funding": {
"type": "opencollective",
@@ -50602,9 +47369,10 @@
}
},
"node_modules/webpack-dev-server": {
- "version": "4.15.1",
- "resolved": "https://registry.npmjs.org/webpack-dev-server/-/webpack-dev-server-4.15.1.tgz",
- "integrity": "sha512-5hbAst3h3C3L8w6W4P96L5vaV0PxSmJhxZvWKYIdgxOQm8pNZ5dEOmmSLBVpP85ReeyRt6AS1QJNyo/oFFPeVA==",
+ "version": "4.15.2",
+ "resolved": "https://registry.npmjs.org/webpack-dev-server/-/webpack-dev-server-4.15.2.tgz",
+ "integrity": "sha512-0XavAZbNJ5sDrCbkpWL8mia0o5WPOd2YGtxrEiZkBK9FjLppIUK2TgxK6qGD2P3hUXTJNNPVibrerKcx5WkR1g==",
+ "license": "MIT",
"dependencies": {
"@types/bonjour": "^3.5.9",
"@types/connect-history-api-fallback": "^1.3.5",
@@ -50634,7 +47402,7 @@
"serve-index": "^1.9.1",
"sockjs": "^0.3.24",
"spdy": "^4.0.2",
- "webpack-dev-middleware": "^5.3.1",
+ "webpack-dev-middleware": "^5.3.4",
"ws": "^8.13.0"
},
"bin": {
@@ -50679,6 +47447,7 @@
"version": "5.1.0",
"resolved": "https://registry.npmjs.org/ajv-keywords/-/ajv-keywords-5.1.0.tgz",
"integrity": "sha512-YCS/JNFAUyr5vAuhk1DWm1CBxRHW9LbJ2ozWeemrIqpbsqKjHVxYPyi5GC0rjZIT5JxJ3virVTS8wk4i/Z+krw==",
+ "license": "MIT",
"dependencies": {
"fast-deep-equal": "^3.1.3"
},
@@ -50689,12 +47458,35 @@
"node_modules/webpack-dev-server/node_modules/colorette": {
"version": "2.0.20",
"resolved": "https://registry.npmjs.org/colorette/-/colorette-2.0.20.tgz",
- "integrity": "sha512-IfEDxwoWIjkeXL1eXcDiow4UbKjhLdq6/EuSVR9GMN7KVH3r9gQ83e73hsz1Nd1T3ijd5xv1wcWRYO+D6kCI2w=="
+ "integrity": "sha512-IfEDxwoWIjkeXL1eXcDiow4UbKjhLdq6/EuSVR9GMN7KVH3r9gQ83e73hsz1Nd1T3ijd5xv1wcWRYO+D6kCI2w==",
+ "license": "MIT"
+ },
+ "node_modules/webpack-dev-server/node_modules/glob": {
+ "version": "7.2.3",
+ "resolved": "https://registry.npmjs.org/glob/-/glob-7.2.3.tgz",
+ "integrity": "sha512-nFR0zLpU2YCaRxwoCJvL6UvCH2JFyFVIvwTLsIf21AuHlMskA1hhTdk+LlYJtOlYt9v6dvszD2BGRqBL+iQK9Q==",
+ "deprecated": "Glob versions prior to v9 are no longer supported",
+ "license": "ISC",
+ "dependencies": {
+ "fs.realpath": "^1.0.0",
+ "inflight": "^1.0.4",
+ "inherits": "2",
+ "minimatch": "^3.1.1",
+ "once": "^1.3.0",
+ "path-is-absolute": "^1.0.0"
+ },
+ "engines": {
+ "node": "*"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
+ }
},
"node_modules/webpack-dev-server/node_modules/ipaddr.js": {
"version": "2.2.0",
"resolved": "https://registry.npmjs.org/ipaddr.js/-/ipaddr.js-2.2.0.tgz",
"integrity": "sha512-Ag3wB2o37wslZS19hZqorUnrnzSkpOVy+IiiDEiTqNubEYpYuHWIf6K4psgN2ZWKExS4xhVCrRVfb/wfW8fWJA==",
+ "license": "MIT",
"engines": {
"node": ">= 10"
}
@@ -50703,6 +47495,7 @@
"version": "2.2.0",
"resolved": "https://registry.npmjs.org/is-wsl/-/is-wsl-2.2.0.tgz",
"integrity": "sha512-fKzAra0rGJUUBwGBgNkHZuToZcn+TtXHpeCgmkMJMMYx1sQDYaCSyjJBSCa2nH1DGm7s3n1oBnohoVTBaN7Lww==",
+ "license": "MIT",
"dependencies": {
"is-docker": "^2.0.0"
},
@@ -50713,12 +47506,14 @@
"node_modules/webpack-dev-server/node_modules/json-schema-traverse": {
"version": "1.0.0",
"resolved": "https://registry.npmjs.org/json-schema-traverse/-/json-schema-traverse-1.0.0.tgz",
- "integrity": "sha512-NM8/P9n3XjXhIZn1lLhkFaACTOURQXjWhV4BA/RnOv8xvgqtqpAX9IO4mRQxSx1Rlo4tqzeqb0sOlruaOy3dug=="
+ "integrity": "sha512-NM8/P9n3XjXhIZn1lLhkFaACTOURQXjWhV4BA/RnOv8xvgqtqpAX9IO4mRQxSx1Rlo4tqzeqb0sOlruaOy3dug==",
+ "license": "MIT"
},
"node_modules/webpack-dev-server/node_modules/open": {
"version": "8.4.2",
"resolved": "https://registry.npmjs.org/open/-/open-8.4.2.tgz",
"integrity": "sha512-7x81NCL719oNbsq/3mh+hVrAWmFuEYUqrq/Iw3kUzH8ReypT9QQ0BLoJS7/G9k6N81XjW4qHWtjWwe/9eLy1EQ==",
+ "license": "MIT",
"dependencies": {
"define-lazy-prop": "^2.0.0",
"is-docker": "^2.1.1",
@@ -50731,10 +47526,27 @@
"url": "https://github.com/sponsors/sindresorhus"
}
},
+ "node_modules/webpack-dev-server/node_modules/rimraf": {
+ "version": "3.0.2",
+ "resolved": "https://registry.npmjs.org/rimraf/-/rimraf-3.0.2.tgz",
+ "integrity": "sha512-JZkJMZkAGFFPP2YqXZXPbMlMBgsxzE8ILs4lMIX/2o0L9UBw9O/Y3o6wFw/i9YLapcUJWwqbi3kdxIPdC62TIA==",
+ "deprecated": "Rimraf versions prior to v4 are no longer supported",
+ "license": "ISC",
+ "dependencies": {
+ "glob": "^7.1.3"
+ },
+ "bin": {
+ "rimraf": "bin.js"
+ },
+ "funding": {
+ "url": "https://github.com/sponsors/isaacs"
+ }
+ },
"node_modules/webpack-dev-server/node_modules/schema-utils": {
"version": "4.2.0",
"resolved": "https://registry.npmjs.org/schema-utils/-/schema-utils-4.2.0.tgz",
"integrity": "sha512-L0jRsrPpjdckP3oPug3/VxNKt2trR8TcabrM6FOAAlvC/9Phcmm+cuAgTlxBqdBR1WJx7Naj9WHw+aOmheSVbw==",
+ "license": "MIT",
"dependencies": {
"@types/json-schema": "^7.0.9",
"ajv": "^8.9.0",
@@ -50753,6 +47565,7 @@
"version": "5.3.4",
"resolved": "https://registry.npmjs.org/webpack-dev-middleware/-/webpack-dev-middleware-5.3.4.tgz",
"integrity": "sha512-BVdTqhhs+0IfoeAf7EoH5WE+exCmqGerHfDM0IL096Px60Tq2Mn9MAbnaGUe6HiMa41KMCYF19gyzZmBcq/o4Q==",
+ "license": "MIT",
"dependencies": {
"colorette": "^2.0.10",
"memfs": "^3.4.3",
@@ -50824,131 +47637,150 @@
}
},
"node_modules/webpack-virtual-modules": {
- "version": "0.5.0",
- "resolved": "https://registry.npmjs.org/webpack-virtual-modules/-/webpack-virtual-modules-0.5.0.tgz",
- "integrity": "sha512-kyDivFZ7ZM0BVOUteVbDFhlRt7Ah/CSPwJdi8hBpkK7QLumUqdLtVfm/PX/hkcnrvr0i77fO5+TjZ94Pe+C9iw==",
+ "version": "0.6.2",
+ "resolved": "https://registry.npmjs.org/webpack-virtual-modules/-/webpack-virtual-modules-0.6.2.tgz",
+ "integrity": "sha512-66/V2i5hQanC51vBQKPH4aI8NMAcBW59FVBs+rC7eGHupMyfn34q7rZIE+ETlJ+XTevqfUhVVBgSUNSW2flEUQ==",
"dev": true
},
+ "node_modules/webpack/node_modules/@types/estree": {
+ "version": "1.0.6",
+ "resolved": "https://registry.npmjs.org/@types/estree/-/estree-1.0.6.tgz",
+ "integrity": "sha512-AYnb1nQyY49te+VRAVgmzfcgjYS91mY5P0TKUDCLEM+gNnA+3T6rWITXRLYCpahpqSQbN5cE+gHpnPyXjHWxcw==",
+ "license": "MIT"
+ },
"node_modules/webpack/node_modules/@webassemblyjs/ast": {
- "version": "1.12.1",
- "resolved": "https://registry.npmjs.org/@webassemblyjs/ast/-/ast-1.12.1.tgz",
- "integrity": "sha512-EKfMUOPRRUTy5UII4qJDGPpqfwjOmZ5jeGFwid9mnoqIFK+e0vqoi1qH56JpmZSzEL53jKnNzScdmftJyG5xWg==",
+ "version": "1.14.1",
+ "resolved": "https://registry.npmjs.org/@webassemblyjs/ast/-/ast-1.14.1.tgz",
+ "integrity": "sha512-nuBEDgQfm1ccRp/8bCQrx1frohyufl4JlbMMZ4P1wpeOfDhF6FQkxZJ1b/e+PLwr6X1Nhw6OLme5usuBWYBvuQ==",
+ "license": "MIT",
"dependencies": {
- "@webassemblyjs/helper-numbers": "1.11.6",
- "@webassemblyjs/helper-wasm-bytecode": "1.11.6"
+ "@webassemblyjs/helper-numbers": "1.13.2",
+ "@webassemblyjs/helper-wasm-bytecode": "1.13.2"
}
},
"node_modules/webpack/node_modules/@webassemblyjs/helper-api-error": {
- "version": "1.11.6",
- "resolved": "https://registry.npmjs.org/@webassemblyjs/helper-api-error/-/helper-api-error-1.11.6.tgz",
- "integrity": "sha512-o0YkoP4pVu4rN8aTJgAyj9hC2Sv5UlkzCHhxqWj8butaLvnpdc2jOwh4ewE6CX0txSfLn/UYaV/pheS2Txg//Q=="
+ "version": "1.13.2",
+ "resolved": "https://registry.npmjs.org/@webassemblyjs/helper-api-error/-/helper-api-error-1.13.2.tgz",
+ "integrity": "sha512-U56GMYxy4ZQCbDZd6JuvvNV/WFildOjsaWD3Tzzvmw/mas3cXzRJPMjP83JqEsgSbyrmaGjBfDtV7KDXV9UzFQ==",
+ "license": "MIT"
},
"node_modules/webpack/node_modules/@webassemblyjs/helper-buffer": {
- "version": "1.12.1",
- "resolved": "https://registry.npmjs.org/@webassemblyjs/helper-buffer/-/helper-buffer-1.12.1.tgz",
- "integrity": "sha512-nzJwQw99DNDKr9BVCOZcLuJJUlqkJh+kVzVl6Fmq/tI5ZtEyWT1KZMyOXltXLZJmDtvLCDgwsyrkohEtopTXCw=="
+ "version": "1.14.1",
+ "resolved": "https://registry.npmjs.org/@webassemblyjs/helper-buffer/-/helper-buffer-1.14.1.tgz",
+ "integrity": "sha512-jyH7wtcHiKssDtFPRB+iQdxlDf96m0E39yb0k5uJVhFGleZFoNw1c4aeIcVUPPbXUVJ94wwnMOAqUHyzoEPVMA==",
+ "license": "MIT"
},
"node_modules/webpack/node_modules/@webassemblyjs/helper-wasm-bytecode": {
- "version": "1.11.6",
- "resolved": "https://registry.npmjs.org/@webassemblyjs/helper-wasm-bytecode/-/helper-wasm-bytecode-1.11.6.tgz",
- "integrity": "sha512-sFFHKwcmBprO9e7Icf0+gddyWYDViL8bpPjJJl0WHxCdETktXdmtWLGVzoHbqUcY4Be1LkNfwTmXOJUFZYSJdA=="
+ "version": "1.13.2",
+ "resolved": "https://registry.npmjs.org/@webassemblyjs/helper-wasm-bytecode/-/helper-wasm-bytecode-1.13.2.tgz",
+ "integrity": "sha512-3QbLKy93F0EAIXLh0ogEVR6rOubA9AoZ+WRYhNbFyuB70j3dRdwH9g+qXhLAO0kiYGlg3TxDV+I4rQTr/YNXkA==",
+ "license": "MIT"
},
"node_modules/webpack/node_modules/@webassemblyjs/helper-wasm-section": {
- "version": "1.12.1",
- "resolved": "https://registry.npmjs.org/@webassemblyjs/helper-wasm-section/-/helper-wasm-section-1.12.1.tgz",
- "integrity": "sha512-Jif4vfB6FJlUlSbgEMHUyk1j234GTNG9dBJ4XJdOySoj518Xj0oGsNi59cUQF4RRMS9ouBUxDDdyBVfPTypa5g==",
+ "version": "1.14.1",
+ "resolved": "https://registry.npmjs.org/@webassemblyjs/helper-wasm-section/-/helper-wasm-section-1.14.1.tgz",
+ "integrity": "sha512-ds5mXEqTJ6oxRoqjhWDU83OgzAYjwsCV8Lo/N+oRsNDmx/ZDpqalmrtgOMkHwxsG0iI//3BwWAErYRHtgn0dZw==",
+ "license": "MIT",
"dependencies": {
- "@webassemblyjs/ast": "1.12.1",
- "@webassemblyjs/helper-buffer": "1.12.1",
- "@webassemblyjs/helper-wasm-bytecode": "1.11.6",
- "@webassemblyjs/wasm-gen": "1.12.1"
+ "@webassemblyjs/ast": "1.14.1",
+ "@webassemblyjs/helper-buffer": "1.14.1",
+ "@webassemblyjs/helper-wasm-bytecode": "1.13.2",
+ "@webassemblyjs/wasm-gen": "1.14.1"
}
},
"node_modules/webpack/node_modules/@webassemblyjs/ieee754": {
- "version": "1.11.6",
- "resolved": "https://registry.npmjs.org/@webassemblyjs/ieee754/-/ieee754-1.11.6.tgz",
- "integrity": "sha512-LM4p2csPNvbij6U1f19v6WR56QZ8JcHg3QIJTlSwzFcmx6WSORicYj6I63f9yU1kEUtrpG+kjkiIAkevHpDXrg==",
+ "version": "1.13.2",
+ "resolved": "https://registry.npmjs.org/@webassemblyjs/ieee754/-/ieee754-1.13.2.tgz",
+ "integrity": "sha512-4LtOzh58S/5lX4ITKxnAK2USuNEvpdVV9AlgGQb8rJDHaLeHciwG4zlGr0j/SNWlr7x3vO1lDEsuePvtcDNCkw==",
+ "license": "MIT",
"dependencies": {
"@xtuc/ieee754": "^1.2.0"
}
},
"node_modules/webpack/node_modules/@webassemblyjs/leb128": {
- "version": "1.11.6",
- "resolved": "https://registry.npmjs.org/@webassemblyjs/leb128/-/leb128-1.11.6.tgz",
- "integrity": "sha512-m7a0FhE67DQXgouf1tbN5XQcdWoNgaAuoULHIfGFIEVKA6tu/edls6XnIlkmS6FrXAquJRPni3ZZKjw6FSPjPQ==",
+ "version": "1.13.2",
+ "resolved": "https://registry.npmjs.org/@webassemblyjs/leb128/-/leb128-1.13.2.tgz",
+ "integrity": "sha512-Lde1oNoIdzVzdkNEAWZ1dZ5orIbff80YPdHx20mrHwHrVNNTjNr8E3xz9BdpcGqRQbAEa+fkrCb+fRFTl/6sQw==",
+ "license": "Apache-2.0",
"dependencies": {
"@xtuc/long": "4.2.2"
}
},
"node_modules/webpack/node_modules/@webassemblyjs/utf8": {
- "version": "1.11.6",
- "resolved": "https://registry.npmjs.org/@webassemblyjs/utf8/-/utf8-1.11.6.tgz",
- "integrity": "sha512-vtXf2wTQ3+up9Zsg8sa2yWiQpzSsMyXj0qViVP6xKGCUT8p8YJ6HqI7l5eCnWx1T/FYdsv07HQs2wTFbbof/RA=="
+ "version": "1.13.2",
+ "resolved": "https://registry.npmjs.org/@webassemblyjs/utf8/-/utf8-1.13.2.tgz",
+ "integrity": "sha512-3NQWGjKTASY1xV5m7Hr0iPeXD9+RDobLll3T9d2AO+g3my8xy5peVyjSag4I50mR1bBSN/Ct12lo+R9tJk0NZQ==",
+ "license": "MIT"
},
"node_modules/webpack/node_modules/@webassemblyjs/wasm-edit": {
- "version": "1.12.1",
- "resolved": "https://registry.npmjs.org/@webassemblyjs/wasm-edit/-/wasm-edit-1.12.1.tgz",
- "integrity": "sha512-1DuwbVvADvS5mGnXbE+c9NfA8QRcZ6iKquqjjmR10k6o+zzsRVesil54DKexiowcFCPdr/Q0qaMgB01+SQ1u6g==",
+ "version": "1.14.1",
+ "resolved": "https://registry.npmjs.org/@webassemblyjs/wasm-edit/-/wasm-edit-1.14.1.tgz",
+ "integrity": "sha512-RNJUIQH/J8iA/1NzlE4N7KtyZNHi3w7at7hDjvRNm5rcUXa00z1vRz3glZoULfJ5mpvYhLybmVcwcjGrC1pRrQ==",
+ "license": "MIT",
"dependencies": {
- "@webassemblyjs/ast": "1.12.1",
- "@webassemblyjs/helper-buffer": "1.12.1",
- "@webassemblyjs/helper-wasm-bytecode": "1.11.6",
- "@webassemblyjs/helper-wasm-section": "1.12.1",
- "@webassemblyjs/wasm-gen": "1.12.1",
- "@webassemblyjs/wasm-opt": "1.12.1",
- "@webassemblyjs/wasm-parser": "1.12.1",
- "@webassemblyjs/wast-printer": "1.12.1"
+ "@webassemblyjs/ast": "1.14.1",
+ "@webassemblyjs/helper-buffer": "1.14.1",
+ "@webassemblyjs/helper-wasm-bytecode": "1.13.2",
+ "@webassemblyjs/helper-wasm-section": "1.14.1",
+ "@webassemblyjs/wasm-gen": "1.14.1",
+ "@webassemblyjs/wasm-opt": "1.14.1",
+ "@webassemblyjs/wasm-parser": "1.14.1",
+ "@webassemblyjs/wast-printer": "1.14.1"
}
},
"node_modules/webpack/node_modules/@webassemblyjs/wasm-gen": {
- "version": "1.12.1",
- "resolved": "https://registry.npmjs.org/@webassemblyjs/wasm-gen/-/wasm-gen-1.12.1.tgz",
- "integrity": "sha512-TDq4Ojh9fcohAw6OIMXqiIcTq5KUXTGRkVxbSo1hQnSy6lAM5GSdfwWeSxpAo0YzgsgF182E/U0mDNhuA0tW7w==",
+ "version": "1.14.1",
+ "resolved": "https://registry.npmjs.org/@webassemblyjs/wasm-gen/-/wasm-gen-1.14.1.tgz",
+ "integrity": "sha512-AmomSIjP8ZbfGQhumkNvgC33AY7qtMCXnN6bL2u2Js4gVCg8fp735aEiMSBbDR7UQIj90n4wKAFUSEd0QN2Ukg==",
+ "license": "MIT",
"dependencies": {
- "@webassemblyjs/ast": "1.12.1",
- "@webassemblyjs/helper-wasm-bytecode": "1.11.6",
- "@webassemblyjs/ieee754": "1.11.6",
- "@webassemblyjs/leb128": "1.11.6",
- "@webassemblyjs/utf8": "1.11.6"
+ "@webassemblyjs/ast": "1.14.1",
+ "@webassemblyjs/helper-wasm-bytecode": "1.13.2",
+ "@webassemblyjs/ieee754": "1.13.2",
+ "@webassemblyjs/leb128": "1.13.2",
+ "@webassemblyjs/utf8": "1.13.2"
}
},
"node_modules/webpack/node_modules/@webassemblyjs/wasm-opt": {
- "version": "1.12.1",
- "resolved": "https://registry.npmjs.org/@webassemblyjs/wasm-opt/-/wasm-opt-1.12.1.tgz",
- "integrity": "sha512-Jg99j/2gG2iaz3hijw857AVYekZe2SAskcqlWIZXjji5WStnOpVoat3gQfT/Q5tb2djnCjBtMocY/Su1GfxPBg==",
+ "version": "1.14.1",
+ "resolved": "https://registry.npmjs.org/@webassemblyjs/wasm-opt/-/wasm-opt-1.14.1.tgz",
+ "integrity": "sha512-PTcKLUNvBqnY2U6E5bdOQcSM+oVP/PmrDY9NzowJjislEjwP/C4an2303MCVS2Mg9d3AJpIGdUFIQQWbPds0Sw==",
+ "license": "MIT",
"dependencies": {
- "@webassemblyjs/ast": "1.12.1",
- "@webassemblyjs/helper-buffer": "1.12.1",
- "@webassemblyjs/wasm-gen": "1.12.1",
- "@webassemblyjs/wasm-parser": "1.12.1"
+ "@webassemblyjs/ast": "1.14.1",
+ "@webassemblyjs/helper-buffer": "1.14.1",
+ "@webassemblyjs/wasm-gen": "1.14.1",
+ "@webassemblyjs/wasm-parser": "1.14.1"
}
},
"node_modules/webpack/node_modules/@webassemblyjs/wasm-parser": {
- "version": "1.12.1",
- "resolved": "https://registry.npmjs.org/@webassemblyjs/wasm-parser/-/wasm-parser-1.12.1.tgz",
- "integrity": "sha512-xikIi7c2FHXysxXe3COrVUPSheuBtpcfhbpFj4gmu7KRLYOzANztwUU0IbsqvMqzuNK2+glRGWCEqZo1WCLyAQ==",
+ "version": "1.14.1",
+ "resolved": "https://registry.npmjs.org/@webassemblyjs/wasm-parser/-/wasm-parser-1.14.1.tgz",
+ "integrity": "sha512-JLBl+KZ0R5qB7mCnud/yyX08jWFw5MsoalJ1pQ4EdFlgj9VdXKGuENGsiCIjegI1W7p91rUlcB/LB5yRJKNTcQ==",
+ "license": "MIT",
"dependencies": {
- "@webassemblyjs/ast": "1.12.1",
- "@webassemblyjs/helper-api-error": "1.11.6",
- "@webassemblyjs/helper-wasm-bytecode": "1.11.6",
- "@webassemblyjs/ieee754": "1.11.6",
- "@webassemblyjs/leb128": "1.11.6",
- "@webassemblyjs/utf8": "1.11.6"
+ "@webassemblyjs/ast": "1.14.1",
+ "@webassemblyjs/helper-api-error": "1.13.2",
+ "@webassemblyjs/helper-wasm-bytecode": "1.13.2",
+ "@webassemblyjs/ieee754": "1.13.2",
+ "@webassemblyjs/leb128": "1.13.2",
+ "@webassemblyjs/utf8": "1.13.2"
}
},
"node_modules/webpack/node_modules/@webassemblyjs/wast-printer": {
- "version": "1.12.1",
- "resolved": "https://registry.npmjs.org/@webassemblyjs/wast-printer/-/wast-printer-1.12.1.tgz",
- "integrity": "sha512-+X4WAlOisVWQMikjbcvY2e0rwPsKQ9F688lksZhBcPycBBuii3O7m8FACbDMWDojpAqvjIncrG8J0XHKyQfVeA==",
+ "version": "1.14.1",
+ "resolved": "https://registry.npmjs.org/@webassemblyjs/wast-printer/-/wast-printer-1.14.1.tgz",
+ "integrity": "sha512-kPSSXE6De1XOR820C90RIo2ogvZG+c3KiHzqUoO/F34Y2shGzesfqv7o57xrxovZJH/MetF5UjroJ/R/3isoiw==",
+ "license": "MIT",
"dependencies": {
- "@webassemblyjs/ast": "1.12.1",
+ "@webassemblyjs/ast": "1.14.1",
"@xtuc/long": "4.2.2"
}
},
"node_modules/webpack/node_modules/acorn": {
- "version": "8.13.0",
- "resolved": "https://registry.npmjs.org/acorn/-/acorn-8.13.0.tgz",
- "integrity": "sha512-8zSiw54Oxrdym50NlZ9sUusyO1Z1ZchgRLWRaK6c86XJFClyCgFKetdowBg5bKxyp/u+CDBJG4Mpp0m3HLZl9w==",
+ "version": "8.14.0",
+ "resolved": "https://registry.npmjs.org/acorn/-/acorn-8.14.0.tgz",
+ "integrity": "sha512-cl669nCJTZBsL97OF4kUQm5g5hC2uihk0NxY3WENAC0TYdILVkAyHymAntgxGkl7K+t0cXIrH5siy5S4XkFycA==",
"license": "MIT",
"bin": {
"acorn": "bin/acorn"
@@ -51209,10 +48041,11 @@
}
},
"node_modules/windows-release/node_modules/cross-spawn": {
- "version": "6.0.5",
- "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-6.0.5.tgz",
- "integrity": "sha512-eTVLrBSt7fjbDygz805pMnstIs2VTBNkRm0qxZd+M7A5XDdxVRWO5MxGBXZhjY4cqLYLdtrGqRf8mBPmzwSpWQ==",
+ "version": "6.0.6",
+ "resolved": "https://registry.npmjs.org/cross-spawn/-/cross-spawn-6.0.6.tgz",
+ "integrity": "sha512-VqCUuhcd1iB+dsv8gxPttb5iZh/D0iubSP21g36KXdEuf6I5JiioesUVjpCdHV9MZRUfVFlvwtIUyPfxo5trtw==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"nice-try": "^1.0.4",
"path-key": "^2.0.1",
@@ -51390,7 +48223,6 @@
"version": "7.0.0",
"resolved": "https://registry.npmjs.org/wrap-ansi/-/wrap-ansi-7.0.0.tgz",
"integrity": "sha512-YVGIj2kamLSTxw6NsZjoBxfSwsn0ycdesmc4p+Q21c5zPuZ1pl+NfxVdxPtdHvmNVOQ6XSYG4AUtyt/Fi7D16Q==",
- "dev": true,
"dependencies": {
"ansi-styles": "^4.0.0",
"string-width": "^4.1.0",
@@ -51406,14 +48238,12 @@
"node_modules/wrap-ansi-cjs/node_modules/emoji-regex": {
"version": "8.0.0",
"resolved": "https://registry.npmjs.org/emoji-regex/-/emoji-regex-8.0.0.tgz",
- "integrity": "sha512-MSjYzcWNOA0ewAHpz0MxpYFvwg6yjy1NG3xteoqz644VCo/RPgnr1/GGt+ic3iJTzQ8Eu3TdM14SawnVUmGE6A==",
- "dev": true
+ "integrity": "sha512-MSjYzcWNOA0ewAHpz0MxpYFvwg6yjy1NG3xteoqz644VCo/RPgnr1/GGt+ic3iJTzQ8Eu3TdM14SawnVUmGE6A=="
},
"node_modules/wrap-ansi-cjs/node_modules/is-fullwidth-code-point": {
"version": "3.0.0",
"resolved": "https://registry.npmjs.org/is-fullwidth-code-point/-/is-fullwidth-code-point-3.0.0.tgz",
"integrity": "sha512-zymm5+u+sCsSWyD9qNaejV3DFvhCKclKdizYaJUuHA83RLjb7nSuGnddCHGv0hk+KY7BMAlsWeK4Ueg6EV6XQg==",
- "dev": true,
"engines": {
"node": ">=8"
}
@@ -51422,7 +48252,6 @@
"version": "4.2.3",
"resolved": "https://registry.npmjs.org/string-width/-/string-width-4.2.3.tgz",
"integrity": "sha512-wKyQRQpjJ0sIp62ErSZdGsjMJWsap5oRNihHhu6G7JVO/9jIB6UyevL+tXuOqrng8j/cxKTWyWUwvSTriiZz/g==",
- "dev": true,
"dependencies": {
"emoji-regex": "^8.0.0",
"is-fullwidth-code-point": "^3.0.0",
@@ -51664,9 +48493,10 @@
"integrity": "sha512-JKhqTOwSrqNA1NY5lSztJ1GrBiUodLMmIZuLiDaMRJ+itFd+ABVE8XBjOvIWL+rSqNDC74LCSFmlb/U4UZ4hJQ=="
},
"node_modules/yallist": {
- "version": "2.1.2",
- "resolved": "https://registry.npmjs.org/yallist/-/yallist-2.1.2.tgz",
- "integrity": "sha512-ncTzHV7NvsQZkYe1DW7cbDLm0YpzHmZF5r/iyP3ZnQtMiJ+pjzisCiMNI+Sj+xQF5pXhSHxSB3uDbsBTzY/c2A=="
+ "version": "4.0.0",
+ "resolved": "https://registry.npmjs.org/yallist/-/yallist-4.0.0.tgz",
+ "integrity": "sha512-3wdGidZyq5PB084XLES5TpOSRA3wjXAlIWMhum2kRcv/41Sn2emQ0dycQW4uZXLejwKvg6EsvbdlVL+FYEct7A==",
+ "license": "ISC"
},
"node_modules/yaml": {
"version": "1.10.2",
@@ -51677,21 +48507,21 @@
}
},
"node_modules/yargs": {
- "version": "16.2.0",
- "resolved": "https://registry.npmjs.org/yargs/-/yargs-16.2.0.tgz",
- "integrity": "sha512-D1mvvtDG0L5ft/jGWkLpG1+m0eQxOfaBvTNELraWj22wSVUMWxZUvYgJYcKh6jGGIkJFhH4IZPQhR4TKpc8mBw==",
- "dev": true,
+ "version": "17.7.2",
+ "resolved": "https://registry.npmjs.org/yargs/-/yargs-17.7.2.tgz",
+ "integrity": "sha512-7dSzzRQ++CKnNI/krKnYRV7JKKPUXMEh61soaHKg9mrWEhzFWhFnxPxGl+69cD1Ou63C13NUPCnmIcrvqCuM6w==",
+ "license": "MIT",
"dependencies": {
- "cliui": "^7.0.2",
+ "cliui": "^8.0.1",
"escalade": "^3.1.1",
"get-caller-file": "^2.0.5",
"require-directory": "^2.1.1",
- "string-width": "^4.2.0",
+ "string-width": "^4.2.3",
"y18n": "^5.0.5",
- "yargs-parser": "^20.2.2"
+ "yargs-parser": "^21.1.1"
},
"engines": {
- "node": ">=10"
+ "node": ">=12"
}
},
"node_modules/yargs-parser": {
@@ -51703,27 +48533,30 @@
}
},
"node_modules/yargs/node_modules/cliui": {
- "version": "7.0.4",
- "resolved": "https://registry.npmjs.org/cliui/-/cliui-7.0.4.tgz",
- "integrity": "sha512-OcRE68cOsVMXp1Yvonl/fzkQOyjLSu/8bhPDfQt0e0/Eb283TKP20Fs2MqoPsr9SwA595rRCA+QMzYc9nBP+JQ==",
- "dev": true,
+ "version": "8.0.1",
+ "resolved": "https://registry.npmjs.org/cliui/-/cliui-8.0.1.tgz",
+ "integrity": "sha512-BSeNnyus75C4//NQ9gQt1/csTXyo/8Sb+afLAkzAptFuMsod9HFokGNudZpi/oQV73hnVK+sR+5PVRMd+Dr7YQ==",
+ "license": "ISC",
"dependencies": {
"string-width": "^4.2.0",
- "strip-ansi": "^6.0.0",
+ "strip-ansi": "^6.0.1",
"wrap-ansi": "^7.0.0"
+ },
+ "engines": {
+ "node": ">=12"
}
},
"node_modules/yargs/node_modules/emoji-regex": {
"version": "8.0.0",
"resolved": "https://registry.npmjs.org/emoji-regex/-/emoji-regex-8.0.0.tgz",
"integrity": "sha512-MSjYzcWNOA0ewAHpz0MxpYFvwg6yjy1NG3xteoqz644VCo/RPgnr1/GGt+ic3iJTzQ8Eu3TdM14SawnVUmGE6A==",
- "dev": true
+ "license": "MIT"
},
"node_modules/yargs/node_modules/is-fullwidth-code-point": {
"version": "3.0.0",
"resolved": "https://registry.npmjs.org/is-fullwidth-code-point/-/is-fullwidth-code-point-3.0.0.tgz",
"integrity": "sha512-zymm5+u+sCsSWyD9qNaejV3DFvhCKclKdizYaJUuHA83RLjb7nSuGnddCHGv0hk+KY7BMAlsWeK4Ueg6EV6XQg==",
- "dev": true,
+ "license": "MIT",
"engines": {
"node": ">=8"
}
@@ -51732,7 +48565,7 @@
"version": "4.2.3",
"resolved": "https://registry.npmjs.org/string-width/-/string-width-4.2.3.tgz",
"integrity": "sha512-wKyQRQpjJ0sIp62ErSZdGsjMJWsap5oRNihHhu6G7JVO/9jIB6UyevL+tXuOqrng8j/cxKTWyWUwvSTriiZz/g==",
- "dev": true,
+ "license": "MIT",
"dependencies": {
"emoji-regex": "^8.0.0",
"is-fullwidth-code-point": "^3.0.0",
@@ -51746,7 +48579,7 @@
"version": "7.0.0",
"resolved": "https://registry.npmjs.org/wrap-ansi/-/wrap-ansi-7.0.0.tgz",
"integrity": "sha512-YVGIj2kamLSTxw6NsZjoBxfSwsn0ycdesmc4p+Q21c5zPuZ1pl+NfxVdxPtdHvmNVOQ6XSYG4AUtyt/Fi7D16Q==",
- "dev": true,
+ "license": "MIT",
"dependencies": {
"ansi-styles": "^4.0.0",
"string-width": "^4.1.0",
@@ -51763,11 +48596,20 @@
"version": "5.0.8",
"resolved": "https://registry.npmjs.org/y18n/-/y18n-5.0.8.tgz",
"integrity": "sha512-0pfFzegeDWJHJIAmTLRP2DwHjdF5s7jo9tuztdQxAhINCdvS+3nGINqPd00AphqJR/0LhANUS6/+7SCb98YOfA==",
- "dev": true,
+ "license": "ISC",
"engines": {
"node": ">=10"
}
},
+ "node_modules/yargs/node_modules/yargs-parser": {
+ "version": "21.1.1",
+ "resolved": "https://registry.npmjs.org/yargs-parser/-/yargs-parser-21.1.1.tgz",
+ "integrity": "sha512-tVpsJW7DdjecAiFpbIB1e3qxIQsE6NoPc5/eTdrbbIC4h0LVsWhnoa3g+m2HclBIujHzsxZ4VJVA+GUuc2/LBw==",
+ "license": "ISC",
+ "engines": {
+ "node": ">=12"
+ }
+ },
"node_modules/yauzl": {
"version": "2.10.0",
"resolved": "https://registry.npmjs.org/yauzl/-/yauzl-2.10.0.tgz",
@@ -51814,10 +48656,11 @@
}
},
"node_modules/zip-stream": {
- "version": "5.0.1",
- "resolved": "https://registry.npmjs.org/zip-stream/-/zip-stream-5.0.1.tgz",
- "integrity": "sha512-UfZ0oa0C8LI58wJ+moL46BDIMgCQbnsb+2PoiJYtonhBsMh2bq1eRBVkvjfVsqbEHd9/EgKPUuL9saSSsec8OA==",
+ "version": "5.0.2",
+ "resolved": "https://registry.npmjs.org/zip-stream/-/zip-stream-5.0.2.tgz",
+ "integrity": "sha512-LfOdrUvPB8ZoXtvOBz6DlNClfvi//b5d56mSWyJi7XbH/HfhOHfUhOqxhT/rUiR7yiktlunqRo+jY6y/cWC/5g==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"archiver-utils": "^4.0.1",
"compress-commons": "^5.0.1",
@@ -51832,6 +48675,7 @@
"resolved": "https://registry.npmjs.org/readable-stream/-/readable-stream-3.6.2.tgz",
"integrity": "sha512-9u/sniCrY3D5WdsERHzHE4G2YCXqoG5FTHUiCC4SIbr6XcLZBY05ya9EKjYek9O5xOAwjGq+1JdGBAS7Q9ScoA==",
"dev": true,
+ "license": "MIT",
"dependencies": {
"inherits": "^2.0.3",
"string_decoder": "^1.1.1",
@@ -51850,9 +48694,21 @@
"url": "https://github.com/sponsors/colinhacks"
}
},
+ "node_modules/zod-validation-error": {
+ "version": "3.4.0",
+ "resolved": "https://registry.npmjs.org/zod-validation-error/-/zod-validation-error-3.4.0.tgz",
+ "integrity": "sha512-ZOPR9SVY6Pb2qqO5XHt+MkkTRxGXb4EVtnjc9JpXUOtUB1T9Ru7mZOT361AN3MsetVe7R0a1KZshJDZdgp9miQ==",
+ "dev": true,
+ "engines": {
+ "node": ">=18.0.0"
+ },
+ "peerDependencies": {
+ "zod": "^3.18.0"
+ }
+ },
"packages/a11y": {
"name": "@wordpress/a11y",
- "version": "4.11.0",
+ "version": "4.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -51866,7 +48722,7 @@
},
"packages/annotations": {
"name": "@wordpress/annotations",
- "version": "3.11.0",
+ "version": "3.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -51894,7 +48750,7 @@
},
"packages/api-fetch": {
"name": "@wordpress/api-fetch",
- "version": "7.11.0",
+ "version": "7.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -51908,7 +48764,7 @@
},
"packages/autop": {
"name": "@wordpress/autop",
- "version": "4.11.0",
+ "version": "4.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7"
@@ -51920,7 +48776,7 @@
},
"packages/babel-plugin-import-jsx-pragma": {
"name": "@wordpress/babel-plugin-import-jsx-pragma",
- "version": "5.11.0",
+ "version": "5.14.0",
"license": "GPL-2.0-or-later",
"engines": {
"node": ">=18.12.0",
@@ -51932,7 +48788,7 @@
},
"packages/babel-plugin-makepot": {
"name": "@wordpress/babel-plugin-makepot",
- "version": "6.11.0",
+ "version": "6.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"deepmerge": "^4.3.0",
@@ -51949,7 +48805,7 @@
},
"packages/babel-preset-default": {
"name": "@wordpress/babel-preset-default",
- "version": "8.11.0",
+ "version": "8.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/core": "7.25.7",
@@ -53080,7 +49936,7 @@
},
"packages/base-styles": {
"name": "@wordpress/base-styles",
- "version": "5.11.0",
+ "version": "5.14.0",
"license": "GPL-2.0-or-later",
"engines": {
"node": ">=18.12.0",
@@ -53089,7 +49945,7 @@
},
"packages/blob": {
"name": "@wordpress/blob",
- "version": "4.11.0",
+ "version": "4.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7"
@@ -53101,7 +49957,7 @@
},
"packages/block-directory": {
"name": "@wordpress/block-directory",
- "version": "5.11.0",
+ "version": "5.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -53137,7 +49993,7 @@
},
"packages/block-editor": {
"name": "@wordpress/block-editor",
- "version": "14.6.0",
+ "version": "14.9.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -53237,7 +50093,7 @@
},
"packages/block-library": {
"name": "@wordpress/block-library",
- "version": "9.11.0",
+ "version": "9.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -53303,7 +50159,7 @@
},
"packages/block-serialization-default-parser": {
"name": "@wordpress/block-serialization-default-parser",
- "version": "5.11.0",
+ "version": "5.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7"
@@ -53315,7 +50171,7 @@
},
"packages/block-serialization-spec-parser": {
"name": "@wordpress/block-serialization-spec-parser",
- "version": "5.11.0",
+ "version": "5.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"pegjs": "^0.10.0",
@@ -53328,7 +50184,7 @@
},
"packages/blocks": {
"name": "@wordpress/blocks",
- "version": "14.0.0",
+ "version": "14.3.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -53382,7 +50238,7 @@
},
"packages/browserslist-config": {
"name": "@wordpress/browserslist-config",
- "version": "6.11.0",
+ "version": "6.14.0",
"license": "GPL-2.0-or-later",
"engines": {
"node": ">=18.12.0",
@@ -53391,7 +50247,7 @@
},
"packages/commands": {
"name": "@wordpress/commands",
- "version": "1.11.0",
+ "version": "1.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -53630,10 +50486,10 @@
},
"packages/components": {
"name": "@wordpress/components",
- "version": "28.11.0",
+ "version": "29.0.0",
"license": "GPL-2.0-or-later",
"dependencies": {
- "@ariakit/react": "^0.4.10",
+ "@ariakit/react": "^0.4.15",
"@babel/runtime": "7.25.7",
"@emotion/cache": "^11.7.1",
"@emotion/css": "^11.7.1",
@@ -53688,36 +50544,6 @@
"react-dom": "^18.0.0"
}
},
- "packages/components/node_modules/@ariakit/react": {
- "version": "0.4.10",
- "resolved": "https://registry.npmjs.org/@ariakit/react/-/react-0.4.10.tgz",
- "integrity": "sha512-c1+6sNLj57aAXrBZMCVGG+OXeFrPAG0TV1jT7oPJcN/KLRs3aCuO3CCJVep/eKepFzzK01kNRGYX3wPT1TXPNw==",
- "dependencies": {
- "@ariakit/react-core": "0.4.10"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/ariakit"
- },
- "peerDependencies": {
- "react": "^17.0.0 || ^18.0.0 || ^19.0.0",
- "react-dom": "^17.0.0 || ^18.0.0 || ^19.0.0"
- }
- },
- "packages/components/node_modules/@ariakit/react-core": {
- "version": "0.4.10",
- "resolved": "https://registry.npmjs.org/@ariakit/react-core/-/react-core-0.4.10.tgz",
- "integrity": "sha512-r6DZmtHBmSoOj848+RpBwdZy/55YxPhMhfH14JIO2OLn1F6iSFkQwR7AAGpIrlYycWJFSF7KrQu50O+SSfFJdQ==",
- "dependencies": {
- "@ariakit/core": "0.4.9",
- "@floating-ui/dom": "^1.0.0",
- "use-sync-external-store": "^1.2.0"
- },
- "peerDependencies": {
- "react": "^17.0.0 || ^18.0.0 || ^19.0.0",
- "react-dom": "^17.0.0 || ^18.0.0 || ^19.0.0"
- }
- },
"packages/components/node_modules/@floating-ui/react-dom": {
"version": "2.0.1",
"resolved": "https://registry.npmjs.org/@floating-ui/react-dom/-/react-dom-2.0.1.tgz",
@@ -53751,7 +50577,7 @@
},
"packages/compose": {
"name": "@wordpress/compose",
- "version": "7.11.0",
+ "version": "7.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -53788,7 +50614,7 @@
},
"packages/core-commands": {
"name": "@wordpress/core-commands",
- "version": "1.11.0",
+ "version": "1.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -53817,7 +50643,7 @@
},
"packages/core-data": {
"name": "@wordpress/core-data",
- "version": "7.11.0",
+ "version": "7.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -53862,7 +50688,7 @@
},
"packages/create-block": {
"name": "@wordpress/create-block",
- "version": "4.54.0",
+ "version": "4.57.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@wordpress/lazy-import": "*",
@@ -53876,7 +50702,7 @@
"make-dir": "^3.0.0",
"mustache": "^4.0.0",
"npm-package-arg": "^8.1.5",
- "rimraf": "^3.0.2",
+ "rimraf": "^5.0.10",
"write-pkg": "^4.0.0"
},
"bin": {
@@ -53889,7 +50715,7 @@
},
"packages/create-block-interactive-template": {
"name": "@wordpress/create-block-interactive-template",
- "version": "2.11.0",
+ "version": "2.14.0",
"license": "GPL-2.0-or-later",
"engines": {
"node": ">=18.12.0",
@@ -53898,7 +50724,7 @@
},
"packages/create-block-tutorial-template": {
"name": "@wordpress/create-block-tutorial-template",
- "version": "4.11.0",
+ "version": "4.14.0",
"license": "GPL-2.0-or-later",
"engines": {
"node": ">=18.12.0",
@@ -53907,7 +50733,7 @@
},
"packages/customize-widgets": {
"name": "@wordpress/customize-widgets",
- "version": "5.11.0",
+ "version": "5.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -53919,7 +50745,6 @@
"@wordpress/core-data": "*",
"@wordpress/data": "*",
"@wordpress/dom": "*",
- "@wordpress/editor": "*",
"@wordpress/element": "*",
"@wordpress/hooks": "*",
"@wordpress/i18n": "*",
@@ -53946,7 +50771,7 @@
},
"packages/data": {
"name": "@wordpress/data",
- "version": "10.11.0",
+ "version": "10.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -53975,7 +50800,7 @@
},
"packages/data-controls": {
"name": "@wordpress/data-controls",
- "version": "4.11.0",
+ "version": "4.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -53993,10 +50818,10 @@
},
"packages/dataviews": {
"name": "@wordpress/dataviews",
- "version": "4.7.0",
+ "version": "4.10.0",
"license": "GPL-2.0-or-later",
"dependencies": {
- "@ariakit/react": "^0.4.10",
+ "@ariakit/react": "^0.4.15",
"@babel/runtime": "7.25.7",
"@wordpress/components": "*",
"@wordpress/compose": "*",
@@ -54018,39 +50843,9 @@
"react": "^18.0.0"
}
},
- "packages/dataviews/node_modules/@ariakit/react": {
- "version": "0.4.10",
- "resolved": "https://registry.npmjs.org/@ariakit/react/-/react-0.4.10.tgz",
- "integrity": "sha512-c1+6sNLj57aAXrBZMCVGG+OXeFrPAG0TV1jT7oPJcN/KLRs3aCuO3CCJVep/eKepFzzK01kNRGYX3wPT1TXPNw==",
- "dependencies": {
- "@ariakit/react-core": "0.4.10"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/ariakit"
- },
- "peerDependencies": {
- "react": "^17.0.0 || ^18.0.0 || ^19.0.0",
- "react-dom": "^17.0.0 || ^18.0.0 || ^19.0.0"
- }
- },
- "packages/dataviews/node_modules/@ariakit/react-core": {
- "version": "0.4.10",
- "resolved": "https://registry.npmjs.org/@ariakit/react-core/-/react-core-0.4.10.tgz",
- "integrity": "sha512-r6DZmtHBmSoOj848+RpBwdZy/55YxPhMhfH14JIO2OLn1F6iSFkQwR7AAGpIrlYycWJFSF7KrQu50O+SSfFJdQ==",
- "dependencies": {
- "@ariakit/core": "0.4.9",
- "@floating-ui/dom": "^1.0.0",
- "use-sync-external-store": "^1.2.0"
- },
- "peerDependencies": {
- "react": "^17.0.0 || ^18.0.0 || ^19.0.0",
- "react-dom": "^17.0.0 || ^18.0.0 || ^19.0.0"
- }
- },
"packages/date": {
"name": "@wordpress/date",
- "version": "5.11.0",
+ "version": "5.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -54065,7 +50860,7 @@
},
"packages/dependency-extraction-webpack-plugin": {
"name": "@wordpress/dependency-extraction-webpack-plugin",
- "version": "6.11.0",
+ "version": "6.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"json2php": "^0.0.7"
@@ -54080,7 +50875,7 @@
},
"packages/deprecated": {
"name": "@wordpress/deprecated",
- "version": "4.11.0",
+ "version": "4.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -54093,7 +50888,7 @@
},
"packages/docgen": {
"name": "@wordpress/docgen",
- "version": "2.11.0",
+ "version": "2.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/core": "7.25.7",
@@ -54114,7 +50909,7 @@
},
"packages/dom": {
"name": "@wordpress/dom",
- "version": "4.11.0",
+ "version": "4.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -54127,7 +50922,7 @@
},
"packages/dom-ready": {
"name": "@wordpress/dom-ready",
- "version": "4.11.0",
+ "version": "4.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7"
@@ -54139,7 +50934,7 @@
},
"packages/e2e-test-utils": {
"name": "@wordpress/e2e-test-utils",
- "version": "11.11.0",
+ "version": "11.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -54156,18 +50951,18 @@
},
"peerDependencies": {
"jest": ">=29",
- "puppeteer-core": ">=11"
+ "puppeteer-core": ">=23"
}
},
"packages/e2e-test-utils-playwright": {
"name": "@wordpress/e2e-test-utils-playwright",
- "version": "1.11.0",
+ "version": "1.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"change-case": "^4.1.2",
"form-data": "^4.0.0",
"get-port": "^5.1.1",
- "lighthouse": "^10.4.0",
+ "lighthouse": "^12.2.2",
"mime": "^3.0.0",
"web-vitals": "^4.2.1"
},
@@ -54187,7 +50982,7 @@
},
"packages/e2e-tests": {
"name": "@wordpress/e2e-tests",
- "version": "8.11.0",
+ "version": "8.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@wordpress/e2e-test-utils": "*",
@@ -54210,7 +51005,7 @@
},
"peerDependencies": {
"jest": ">=29",
- "puppeteer-core": ">=11",
+ "puppeteer-core": ">=23",
"react": "^18.0.0",
"react-dom": "^18.0.0"
}
@@ -54225,7 +51020,7 @@
},
"packages/edit-post": {
"name": "@wordpress/edit-post",
- "version": "8.11.0",
+ "version": "8.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -54272,7 +51067,7 @@
},
"packages/edit-site": {
"name": "@wordpress/edit-site",
- "version": "6.11.0",
+ "version": "6.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -54334,7 +51129,7 @@
},
"packages/edit-widgets": {
"name": "@wordpress/edit-widgets",
- "version": "6.11.0",
+ "version": "6.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -54348,7 +51143,6 @@
"@wordpress/data": "*",
"@wordpress/deprecated": "*",
"@wordpress/dom": "*",
- "@wordpress/editor": "*",
"@wordpress/element": "*",
"@wordpress/hooks": "*",
"@wordpress/i18n": "*",
@@ -54378,7 +51172,7 @@
},
"packages/editor": {
"name": "@wordpress/editor",
- "version": "14.11.0",
+ "version": "14.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -54440,7 +51234,7 @@
},
"packages/element": {
"name": "@wordpress/element",
- "version": "6.11.0",
+ "version": "6.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -54459,7 +51253,7 @@
},
"packages/env": {
"name": "@wordpress/env",
- "version": "10.11.0",
+ "version": "10.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"chalk": "^4.0.0",
@@ -54470,7 +51264,7 @@
"inquirer": "^7.1.0",
"js-yaml": "^3.13.1",
"ora": "^4.0.2",
- "rimraf": "^3.0.2",
+ "rimraf": "^5.0.10",
"simple-git": "^3.5.0",
"terminal-link": "^2.0.0",
"yargs": "^17.3.0"
@@ -54483,19 +51277,6 @@
"npm": ">=8.19.2"
}
},
- "packages/env/node_modules/cliui": {
- "version": "8.0.1",
- "resolved": "https://registry.npmjs.org/cliui/-/cliui-8.0.1.tgz",
- "integrity": "sha512-BSeNnyus75C4//NQ9gQt1/csTXyo/8Sb+afLAkzAptFuMsod9HFokGNudZpi/oQV73hnVK+sR+5PVRMd+Dr7YQ==",
- "dependencies": {
- "string-width": "^4.2.0",
- "strip-ansi": "^6.0.1",
- "wrap-ansi": "^7.0.0"
- },
- "engines": {
- "node": ">=12"
- }
- },
"packages/env/node_modules/docker-compose": {
"version": "0.24.2",
"resolved": "https://registry.npmjs.org/docker-compose/-/docker-compose-0.24.2.tgz",
@@ -54507,53 +51288,6 @@
"node": ">= 6.0.0"
}
},
- "packages/env/node_modules/emoji-regex": {
- "version": "8.0.0",
- "resolved": "https://registry.npmjs.org/emoji-regex/-/emoji-regex-8.0.0.tgz",
- "integrity": "sha512-MSjYzcWNOA0ewAHpz0MxpYFvwg6yjy1NG3xteoqz644VCo/RPgnr1/GGt+ic3iJTzQ8Eu3TdM14SawnVUmGE6A=="
- },
- "packages/env/node_modules/is-fullwidth-code-point": {
- "version": "3.0.0",
- "resolved": "https://registry.npmjs.org/is-fullwidth-code-point/-/is-fullwidth-code-point-3.0.0.tgz",
- "integrity": "sha512-zymm5+u+sCsSWyD9qNaejV3DFvhCKclKdizYaJUuHA83RLjb7nSuGnddCHGv0hk+KY7BMAlsWeK4Ueg6EV6XQg==",
- "engines": {
- "node": ">=8"
- }
- },
- "packages/env/node_modules/string-width": {
- "version": "4.2.3",
- "resolved": "https://registry.npmjs.org/string-width/-/string-width-4.2.3.tgz",
- "integrity": "sha512-wKyQRQpjJ0sIp62ErSZdGsjMJWsap5oRNihHhu6G7JVO/9jIB6UyevL+tXuOqrng8j/cxKTWyWUwvSTriiZz/g==",
- "dependencies": {
- "emoji-regex": "^8.0.0",
- "is-fullwidth-code-point": "^3.0.0",
- "strip-ansi": "^6.0.1"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "packages/env/node_modules/wrap-ansi": {
- "version": "7.0.0",
- "resolved": "https://registry.npmjs.org/wrap-ansi/-/wrap-ansi-7.0.0.tgz",
- "integrity": "sha512-YVGIj2kamLSTxw6NsZjoBxfSwsn0ycdesmc4p+Q21c5zPuZ1pl+NfxVdxPtdHvmNVOQ6XSYG4AUtyt/Fi7D16Q==",
- "dependencies": {
- "ansi-styles": "^4.0.0",
- "string-width": "^4.1.0",
- "strip-ansi": "^6.0.0"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/chalk/wrap-ansi?sponsor=1"
- }
- },
- "packages/env/node_modules/y18n": {
- "version": "5.0.8",
- "resolved": "https://registry.npmjs.org/y18n/-/y18n-5.0.8.tgz",
- "integrity": "sha512-0pfFzegeDWJHJIAmTLRP2DwHjdF5s7jo9tuztdQxAhINCdvS+3nGINqPd00AphqJR/0LhANUS6/+7SCb98YOfA=="
- },
"packages/env/node_modules/yaml": {
"version": "2.6.0",
"resolved": "https://registry.npmjs.org/yaml/-/yaml-2.6.0.tgz",
@@ -54566,31 +51300,9 @@
"node": ">= 14"
}
},
- "packages/env/node_modules/yargs": {
- "version": "17.7.2",
- "resolved": "https://registry.npmjs.org/yargs/-/yargs-17.7.2.tgz",
- "integrity": "sha512-7dSzzRQ++CKnNI/krKnYRV7JKKPUXMEh61soaHKg9mrWEhzFWhFnxPxGl+69cD1Ou63C13NUPCnmIcrvqCuM6w==",
- "dependencies": {
- "cliui": "^8.0.1",
- "escalade": "^3.1.1",
- "get-caller-file": "^2.0.5",
- "require-directory": "^2.1.1",
- "string-width": "^4.2.3",
- "y18n": "^5.0.5",
- "yargs-parser": "^21.1.1"
- },
- "engines": {
- "node": ">=12"
- }
- },
- "packages/env/node_modules/yargs-parser": {
- "version": "21.1.1",
- "resolved": "https://registry.npmjs.org/yargs-parser/-/yargs-parser-21.1.1.tgz",
- "integrity": "sha512-tVpsJW7DdjecAiFpbIB1e3qxIQsE6NoPc5/eTdrbbIC4h0LVsWhnoa3g+m2HclBIujHzsxZ4VJVA+GUuc2/LBw=="
- },
"packages/escape-html": {
"name": "@wordpress/escape-html",
- "version": "3.11.0",
+ "version": "3.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7"
@@ -54602,7 +51314,7 @@
},
"packages/eslint-plugin": {
"name": "@wordpress/eslint-plugin",
- "version": "21.4.0",
+ "version": "22.0.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/eslint-parser": "7.25.7",
@@ -54613,7 +51325,7 @@
"cosmiconfig": "^7.0.0",
"eslint-config-prettier": "^8.3.0",
"eslint-plugin-import": "^2.25.2",
- "eslint-plugin-jest": "^27.2.3",
+ "eslint-plugin-jest": "^27.4.3",
"eslint-plugin-jsdoc": "^46.4.6",
"eslint-plugin-jsx-a11y": "^6.5.1",
"eslint-plugin-playwright": "^0.15.3",
@@ -54631,7 +51343,7 @@
"@babel/core": ">=7",
"eslint": ">=8",
"prettier": ">=3",
- "typescript": ">=4"
+ "typescript": ">=5"
},
"peerDependenciesMeta": {
"prettier": {
@@ -54671,12 +51383,13 @@
},
"packages/fields": {
"name": "@wordpress/fields",
- "version": "0.3.0",
+ "version": "0.6.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
"@wordpress/api-fetch": "*",
"@wordpress/blob": "*",
+ "@wordpress/block-editor": "*",
"@wordpress/blocks": "*",
"@wordpress/components": "*",
"@wordpress/compose": "*",
@@ -54712,7 +51425,7 @@
},
"packages/format-library": {
"name": "@wordpress/format-library",
- "version": "5.11.0",
+ "version": "5.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -54740,7 +51453,7 @@
},
"packages/hooks": {
"name": "@wordpress/hooks",
- "version": "4.11.0",
+ "version": "4.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7"
@@ -54752,7 +51465,7 @@
},
"packages/html-entities": {
"name": "@wordpress/html-entities",
- "version": "4.11.0",
+ "version": "4.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7"
@@ -54764,7 +51477,7 @@
},
"packages/i18n": {
"name": "@wordpress/i18n",
- "version": "5.11.0",
+ "version": "5.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -54784,7 +51497,7 @@
},
"packages/icons": {
"name": "@wordpress/icons",
- "version": "10.11.0",
+ "version": "10.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -54798,7 +51511,7 @@
},
"packages/interactivity": {
"name": "@wordpress/interactivity",
- "version": "6.11.0",
+ "version": "6.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@preact/signals": "^1.3.0",
@@ -54811,7 +51524,7 @@
},
"packages/interactivity-router": {
"name": "@wordpress/interactivity-router",
- "version": "2.11.0",
+ "version": "2.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@wordpress/a11y": "*",
@@ -54824,7 +51537,7 @@
},
"packages/interface": {
"name": "@wordpress/interface",
- "version": "8.0.0",
+ "version": "8.3.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -54852,7 +51565,7 @@
},
"packages/is-shallow-equal": {
"name": "@wordpress/is-shallow-equal",
- "version": "5.11.0",
+ "version": "5.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7"
@@ -54864,7 +51577,7 @@
},
"packages/jest-console": {
"name": "@wordpress/jest-console",
- "version": "8.11.0",
+ "version": "8.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -54880,7 +51593,7 @@
},
"packages/jest-preset-default": {
"name": "@wordpress/jest-preset-default",
- "version": "12.11.0",
+ "version": "12.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@wordpress/jest-console": "*",
@@ -54897,7 +51610,7 @@
},
"packages/jest-puppeteer-axe": {
"name": "@wordpress/jest-puppeteer-axe",
- "version": "7.11.0",
+ "version": "7.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@axe-core/puppeteer": "^4.0.0",
@@ -54919,7 +51632,7 @@
},
"packages/keyboard-shortcuts": {
"name": "@wordpress/keyboard-shortcuts",
- "version": "5.11.0",
+ "version": "5.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -54937,7 +51650,7 @@
},
"packages/keycodes": {
"name": "@wordpress/keycodes",
- "version": "4.11.0",
+ "version": "4.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -54950,7 +51663,7 @@
},
"packages/lazy-import": {
"name": "@wordpress/lazy-import",
- "version": "2.11.0",
+ "version": "2.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"execa": "^4.0.2",
@@ -54964,7 +51677,7 @@
},
"packages/list-reusable-blocks": {
"name": "@wordpress/list-reusable-blocks",
- "version": "5.11.0",
+ "version": "5.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -54987,7 +51700,7 @@
},
"packages/media-utils": {
"name": "@wordpress/media-utils",
- "version": "5.11.0",
+ "version": "5.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -55004,7 +51717,7 @@
},
"packages/notices": {
"name": "@wordpress/notices",
- "version": "5.11.0",
+ "version": "5.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -55021,7 +51734,7 @@
},
"packages/npm-package-json-lint-config": {
"name": "@wordpress/npm-package-json-lint-config",
- "version": "5.11.0",
+ "version": "5.14.0",
"license": "GPL-2.0-or-later",
"engines": {
"node": ">=18.12.0",
@@ -55033,7 +51746,7 @@
},
"packages/nux": {
"name": "@wordpress/nux",
- "version": "9.11.0",
+ "version": "9.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -55056,7 +51769,7 @@
},
"packages/patterns": {
"name": "@wordpress/patterns",
- "version": "2.11.0",
+ "version": "2.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -55086,7 +51799,7 @@
},
"packages/plugins": {
"name": "@wordpress/plugins",
- "version": "7.11.0",
+ "version": "7.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -55110,7 +51823,7 @@
},
"packages/postcss-plugins-preset": {
"name": "@wordpress/postcss-plugins-preset",
- "version": "5.11.0",
+ "version": "5.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@wordpress/base-styles": "*",
@@ -55126,7 +51839,7 @@
},
"packages/postcss-themes": {
"name": "@wordpress/postcss-themes",
- "version": "6.11.0",
+ "version": "6.14.0",
"license": "GPL-2.0-or-later",
"engines": {
"node": ">=18.12.0",
@@ -55138,7 +51851,7 @@
},
"packages/preferences": {
"name": "@wordpress/preferences",
- "version": "4.11.0",
+ "version": "4.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -55164,7 +51877,7 @@
},
"packages/preferences-persistence": {
"name": "@wordpress/preferences-persistence",
- "version": "2.11.0",
+ "version": "2.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -55177,7 +51890,7 @@
},
"packages/prettier-config": {
"name": "@wordpress/prettier-config",
- "version": "4.11.0",
+ "version": "4.14.0",
"license": "GPL-2.0-or-later",
"engines": {
"node": ">=18.12.0",
@@ -55189,7 +51902,7 @@
},
"packages/primitives": {
"name": "@wordpress/primitives",
- "version": "4.11.0",
+ "version": "4.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -55206,7 +51919,7 @@
},
"packages/priority-queue": {
"name": "@wordpress/priority-queue",
- "version": "3.11.0",
+ "version": "3.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -55219,7 +51932,7 @@
},
"packages/private-apis": {
"name": "@wordpress/private-apis",
- "version": "1.11.0",
+ "version": "1.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7"
@@ -55231,7 +51944,7 @@
},
"packages/project-management-automation": {
"name": "@wordpress/project-management-automation",
- "version": "2.11.0",
+ "version": "2.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@actions/core": "1.9.1",
@@ -55259,7 +51972,7 @@
},
"packages/react-i18n": {
"name": "@wordpress/react-i18n",
- "version": "4.11.0",
+ "version": "4.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -55415,7 +52128,7 @@
},
"packages/readable-js-assets-webpack-plugin": {
"name": "@wordpress/readable-js-assets-webpack-plugin",
- "version": "3.11.0",
+ "version": "3.14.0",
"license": "GPL-2.0-or-later",
"engines": {
"node": ">=18.12.0",
@@ -55427,7 +52140,7 @@
},
"packages/redux-routine": {
"name": "@wordpress/redux-routine",
- "version": "5.11.0",
+ "version": "5.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -55470,7 +52183,7 @@
},
"packages/reusable-blocks": {
"name": "@wordpress/reusable-blocks",
- "version": "5.11.0",
+ "version": "5.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -55497,7 +52210,7 @@
},
"packages/rich-text": {
"name": "@wordpress/rich-text",
- "version": "7.11.0",
+ "version": "7.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -55521,14 +52234,16 @@
},
"packages/router": {
"name": "@wordpress/router",
- "version": "1.11.0",
+ "version": "1.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
+ "@wordpress/compose": "*",
"@wordpress/element": "*",
"@wordpress/private-apis": "*",
"@wordpress/url": "*",
- "history": "^5.3.0"
+ "history": "^5.3.0",
+ "route-recognizer": "^0.3.4"
},
"engines": {
"node": ">=18.12.0",
@@ -55540,7 +52255,7 @@
},
"packages/scripts": {
"name": "@wordpress/scripts",
- "version": "30.4.0",
+ "version": "30.7.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/core": "7.25.7",
@@ -55564,7 +52279,7 @@
"check-node-version": "^4.1.0",
"clean-webpack-plugin": "^3.0.0",
"copy-webpack-plugin": "^10.2.0",
- "cross-spawn": "^5.1.0",
+ "cross-spawn": "^7.0.6",
"css-loader": "^6.2.0",
"cssnano": "^6.0.1",
"cwd": "^0.10.0",
@@ -55574,33 +52289,32 @@
"fast-glob": "^3.2.7",
"filenamify": "^4.2.0",
"jest": "^29.6.2",
- "jest-dev-server": "^9.0.1",
+ "jest-dev-server": "^10.1.4",
"jest-environment-jsdom": "^29.6.2",
"jest-environment-node": "^29.6.2",
"json2php": "^0.0.9",
"markdownlint-cli": "^0.31.1",
"merge-deep": "^3.0.3",
- "mini-css-extract-plugin": "^2.5.1",
+ "mini-css-extract-plugin": "^2.9.2",
"minimist": "^1.2.0",
"npm-package-json-lint": "^6.4.0",
"npm-packlist": "^3.0.0",
"postcss": "^8.4.5",
- "postcss-import": "^16.1.0",
"postcss-loader": "^6.2.1",
"prettier": "npm:wp-prettier@3.0.3",
- "puppeteer-core": "^23.1.0",
+ "puppeteer-core": "^23.10.1",
"react-refresh": "^0.14.0",
"read-pkg-up": "^7.0.1",
"resolve-bin": "^0.4.0",
"rtlcss-webpack-plugin": "^4.0.7",
- "sass": "^1.35.2",
- "sass-loader": "^12.1.0",
+ "sass": "^1.50.1",
+ "sass-loader": "^16.0.3",
"schema-utils": "^4.2.0",
"source-map-loader": "^3.0.0",
"stylelint": "^16.8.2",
"terser-webpack-plugin": "^5.3.10",
"url-loader": "^4.1.1",
- "webpack": "^5.95.0",
+ "webpack": "^5.97.0",
"webpack-bundle-analyzer": "^4.9.1",
"webpack-cli": "^5.1.4",
"webpack-dev-server": "^4.15.1"
@@ -55634,154 +52348,16 @@
"url": "https://github.com/sponsors/epoberezkin"
}
},
- "packages/scripts/node_modules/axios": {
- "version": "1.7.7",
- "resolved": "https://registry.npmjs.org/axios/-/axios-1.7.7.tgz",
- "integrity": "sha512-S4kL7XrjgBmvdGut0sN3yJxqYzrDOnivkBiN0OFs6hLiUam3UPvswUo0kqGyhqUZGEOytHyumEdXsAkgCOUf3Q==",
- "license": "MIT",
- "dependencies": {
- "follow-redirects": "^1.15.6",
- "form-data": "^4.0.0",
- "proxy-from-env": "^1.1.0"
- }
- },
- "packages/scripts/node_modules/babel-loader": {
- "version": "8.4.1",
- "resolved": "https://registry.npmjs.org/babel-loader/-/babel-loader-8.4.1.tgz",
- "integrity": "sha512-nXzRChX+Z1GoE6yWavBQg6jDslyFF3SDjl2paADuoQtQW10JqShJt62R6eJQ5m/pjJFDT8xgKIWSP85OY8eXeA==",
+ "packages/scripts/node_modules/ajv-keywords": {
+ "version": "5.1.0",
+ "resolved": "https://registry.npmjs.org/ajv-keywords/-/ajv-keywords-5.1.0.tgz",
+ "integrity": "sha512-YCS/JNFAUyr5vAuhk1DWm1CBxRHW9LbJ2ozWeemrIqpbsqKjHVxYPyi5GC0rjZIT5JxJ3virVTS8wk4i/Z+krw==",
"license": "MIT",
"dependencies": {
- "find-cache-dir": "^3.3.1",
- "loader-utils": "^2.0.4",
- "make-dir": "^3.1.0",
- "schema-utils": "^2.6.5"
- },
- "engines": {
- "node": ">= 8.9"
+ "fast-deep-equal": "^3.1.3"
},
"peerDependencies": {
- "@babel/core": "^7.0.0",
- "webpack": ">=2"
- }
- },
- "packages/scripts/node_modules/babel-loader/node_modules/ajv": {
- "version": "6.12.6",
- "resolved": "https://registry.npmjs.org/ajv/-/ajv-6.12.6.tgz",
- "integrity": "sha512-j3fVLgvTo527anyYyJOGTYJbG+vnnQYvE0m5mmkc1TK+nxAppkCLMIL0aZ4dblVCNoGShhm+kzE4ZUykBoMg4g==",
- "license": "MIT",
- "dependencies": {
- "fast-deep-equal": "^3.1.1",
- "fast-json-stable-stringify": "^2.0.0",
- "json-schema-traverse": "^0.4.1",
- "uri-js": "^4.2.2"
- },
- "funding": {
- "type": "github",
- "url": "https://github.com/sponsors/epoberezkin"
- }
- },
- "packages/scripts/node_modules/babel-loader/node_modules/json-schema-traverse": {
- "version": "0.4.1",
- "resolved": "https://registry.npmjs.org/json-schema-traverse/-/json-schema-traverse-0.4.1.tgz",
- "integrity": "sha512-xbbCH5dCYU5T8LcEhhuh7HJ88HXuW3qsI3Y0zOZFKfZEHcpWiHU/Jxzk629Brsab/mMiHQti9wMP+845RPe3Vg==",
- "license": "MIT"
- },
- "packages/scripts/node_modules/babel-loader/node_modules/schema-utils": {
- "version": "2.7.1",
- "resolved": "https://registry.npmjs.org/schema-utils/-/schema-utils-2.7.1.tgz",
- "integrity": "sha512-SHiNtMOUGWBQJwzISiVYKu82GiV4QYGePp3odlY1tuKO7gPtphAT5R/py0fA6xtbgLL/RvtJZnU9b8s0F1q0Xg==",
- "license": "MIT",
- "dependencies": {
- "@types/json-schema": "^7.0.5",
- "ajv": "^6.12.4",
- "ajv-keywords": "^3.5.2"
- },
- "engines": {
- "node": ">= 8.9.0"
- },
- "funding": {
- "type": "opencollective",
- "url": "https://opencollective.com/webpack"
- }
- },
- "packages/scripts/node_modules/chalk": {
- "version": "4.1.2",
- "resolved": "https://registry.npmjs.org/chalk/-/chalk-4.1.2.tgz",
- "integrity": "sha512-oKnbhFyRIXpUuez8iBMmyEa4nbj4IOQyuhc/wy9kY7/WVPcwIO9VA668Pu8RkO7+0G76SLROeyw9CpQ061i4mA==",
- "dependencies": {
- "ansi-styles": "^4.1.0",
- "supports-color": "^7.1.0"
- },
- "engines": {
- "node": ">=10"
- },
- "funding": {
- "url": "https://github.com/chalk/chalk?sponsor=1"
- }
- },
- "packages/scripts/node_modules/find-cache-dir": {
- "version": "3.3.2",
- "resolved": "https://registry.npmjs.org/find-cache-dir/-/find-cache-dir-3.3.2.tgz",
- "integrity": "sha512-wXZV5emFEjrridIgED11OoUKLxiYjAcqot/NJdAkOhlJ+vGzwhOAfcG5OX1jP+S0PcjEn8bdMJv+g2jwQ3Onig==",
- "dependencies": {
- "commondir": "^1.0.1",
- "make-dir": "^3.0.2",
- "pkg-dir": "^4.1.0"
- },
- "engines": {
- "node": ">=8"
- },
- "funding": {
- "url": "https://github.com/avajs/find-cache-dir?sponsor=1"
- }
- },
- "packages/scripts/node_modules/find-up": {
- "version": "4.1.0",
- "resolved": "https://registry.npmjs.org/find-up/-/find-up-4.1.0.tgz",
- "integrity": "sha512-PpOwAdQ/YlXQ2vj8a3h8IipDuYRi3wceVQQGYWxNINccq40Anw7BlsEXCMbt1Zt+OLA6Fq9suIpIWD0OsnISlw==",
- "dependencies": {
- "locate-path": "^5.0.0",
- "path-exists": "^4.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "packages/scripts/node_modules/has-flag": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/has-flag/-/has-flag-4.0.0.tgz",
- "integrity": "sha512-EykJT/Q1KjTWctppgIAgfSO0tKVuZUjhgMr17kqTumMl6Afv3EISleU7qZUzoXDFTAHTDC4NOoG/ZxU3EvlMPQ==",
- "engines": {
- "node": ">=8"
- }
- },
- "packages/scripts/node_modules/jest-dev-server": {
- "version": "9.0.1",
- "resolved": "https://registry.npmjs.org/jest-dev-server/-/jest-dev-server-9.0.1.tgz",
- "integrity": "sha512-eqpJKSvVl4M0ojHZUPNbka8yEzLNbIMiINXDsuMF3lYfIdRO2iPqy+ASR4wBQ6nUyR3OT24oKPWhpsfLhgAVyg==",
- "dependencies": {
- "chalk": "^4.1.2",
- "cwd": "^0.10.0",
- "find-process": "^1.4.7",
- "prompts": "^2.4.2",
- "spawnd": "^9.0.1",
- "tree-kill": "^1.2.2",
- "wait-on": "^7.0.1"
- },
- "engines": {
- "node": ">=16"
- }
- },
- "packages/scripts/node_modules/joi": {
- "version": "17.11.0",
- "resolved": "https://registry.npmjs.org/joi/-/joi-17.11.0.tgz",
- "integrity": "sha512-NgB+lZLNoqISVy1rZocE9PZI36bL/77ie924Ri43yEvi9GUUMPeyVIr8KdFTMUlby1p0PBYMk9spIxEUQYqrJQ==",
- "dependencies": {
- "@hapi/hoek": "^9.0.0",
- "@hapi/topo": "^5.0.0",
- "@sideway/address": "^4.1.3",
- "@sideway/formula": "^3.0.1",
- "@sideway/pinpoint": "^2.0.0"
+ "ajv": "^8.8.2"
}
},
"packages/scripts/node_modules/json-schema-traverse": {
@@ -55796,91 +52372,6 @@
"integrity": "sha512-fQMYwvPsQt8hxRnCGyg1r2JVi6yL8Um0DIIawiKiMK9yhAAkcRNj5UsBWoaFvFzPpcWbgw9L6wzj+UMYA702Mw==",
"license": "BSD"
},
- "packages/scripts/node_modules/locate-path": {
- "version": "5.0.0",
- "resolved": "https://registry.npmjs.org/locate-path/-/locate-path-5.0.0.tgz",
- "integrity": "sha512-t7hw9pI+WvuwNJXwk5zVHpyhIqzg2qTlklJOf0mVxGSbe3Fp2VieZcduNYjaLDoy6p9uGpQEGWG87WpMKlNq8g==",
- "dependencies": {
- "p-locate": "^4.1.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "packages/scripts/node_modules/make-dir": {
- "version": "3.1.0",
- "resolved": "https://registry.npmjs.org/make-dir/-/make-dir-3.1.0.tgz",
- "integrity": "sha512-g3FeP20LNwhALb/6Cz6Dd4F2ngze0jz7tbzrD2wAV+o9FeNHe4rL+yK2md0J/fiSf1sa1ADhXqi5+oVwOM/eGw==",
- "dependencies": {
- "semver": "^6.0.0"
- },
- "engines": {
- "node": ">=8"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "packages/scripts/node_modules/p-limit": {
- "version": "2.3.0",
- "resolved": "https://registry.npmjs.org/p-limit/-/p-limit-2.3.0.tgz",
- "integrity": "sha512-//88mFWSJx8lxCzwdAABTJL2MyWB12+eIY7MDL2SqLmAkeKU9qxRvWuSyTjm3FUmpBEMuFfckAIqEaVGUDxb6w==",
- "dependencies": {
- "p-try": "^2.0.0"
- },
- "engines": {
- "node": ">=6"
- },
- "funding": {
- "url": "https://github.com/sponsors/sindresorhus"
- }
- },
- "packages/scripts/node_modules/p-locate": {
- "version": "4.1.0",
- "resolved": "https://registry.npmjs.org/p-locate/-/p-locate-4.1.0.tgz",
- "integrity": "sha512-R79ZZ/0wAxKGu3oYMlz8jy/kbhsNrS7SKZ7PxEHBgJ5+F2mtFW2fK2cOtBh1cHYkQsbzFV7I+EoRKe6Yt0oK7A==",
- "dependencies": {
- "p-limit": "^2.2.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "packages/scripts/node_modules/p-try": {
- "version": "2.2.0",
- "resolved": "https://registry.npmjs.org/p-try/-/p-try-2.2.0.tgz",
- "integrity": "sha512-R4nPAVTAU0B9D35/Gk3uJf/7XYbQcyohSKdvAxIRSNghFl4e71hVoGnBNQz9cWaXxO2I10KTC+3jMdvvoKw6dQ==",
- "engines": {
- "node": ">=6"
- }
- },
- "packages/scripts/node_modules/path-exists": {
- "version": "4.0.0",
- "resolved": "https://registry.npmjs.org/path-exists/-/path-exists-4.0.0.tgz",
- "integrity": "sha512-ak9Qy5Q7jYb2Wwcey5Fpvg2KoAc/ZIhLSLOSBmRmygPsGwkVVt0fZa0qrtMz+m6tJTAHfZQ8FnmB4MG4LWy7/w==",
- "engines": {
- "node": ">=8"
- }
- },
- "packages/scripts/node_modules/pkg-dir": {
- "version": "4.2.0",
- "resolved": "https://registry.npmjs.org/pkg-dir/-/pkg-dir-4.2.0.tgz",
- "integrity": "sha512-HRDzbaKjC+AOWVXxAU/x54COGeIv9eb+6CkDSQoNTt4XyWoIJvuPsXizxu/Fr23EiekbtZwmh1IcIG/l/a10GQ==",
- "dependencies": {
- "find-up": "^4.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "packages/scripts/node_modules/rxjs": {
- "version": "7.8.1",
- "resolved": "https://registry.npmjs.org/rxjs/-/rxjs-7.8.1.tgz",
- "integrity": "sha512-AA3TVj+0A2iuIoQkWEK/tqFjBq2j+6PO6Y0zJcvzLAFhEFIO3HL0vls9hWLncZbAAbK0mar7oZ4V079I/qPMxg==",
- "dependencies": {
- "tslib": "^2.1.0"
- }
- },
"packages/scripts/node_modules/schema-utils": {
"version": "4.2.0",
"resolved": "https://registry.npmjs.org/schema-utils/-/schema-utils-4.2.0.tgz",
@@ -55900,81 +52391,9 @@
"url": "https://opencollective.com/webpack"
}
},
- "packages/scripts/node_modules/schema-utils/node_modules/ajv-keywords": {
- "version": "5.1.0",
- "resolved": "https://registry.npmjs.org/ajv-keywords/-/ajv-keywords-5.1.0.tgz",
- "integrity": "sha512-YCS/JNFAUyr5vAuhk1DWm1CBxRHW9LbJ2ozWeemrIqpbsqKjHVxYPyi5GC0rjZIT5JxJ3virVTS8wk4i/Z+krw==",
- "license": "MIT",
- "dependencies": {
- "fast-deep-equal": "^3.1.3"
- },
- "peerDependencies": {
- "ajv": "^8.8.2"
- }
- },
- "packages/scripts/node_modules/semver": {
- "version": "6.3.1",
- "resolved": "https://registry.npmjs.org/semver/-/semver-6.3.1.tgz",
- "integrity": "sha512-BR7VvDCVHO+q2xBEWskxS6DJE1qRnb7DxzUrogb71CWoSficBxYsiAGd+Kl0mmq/MprG9yArRkyrQxTO6XjMzA==",
- "bin": {
- "semver": "bin/semver.js"
- }
- },
- "packages/scripts/node_modules/signal-exit": {
- "version": "4.1.0",
- "resolved": "https://registry.npmjs.org/signal-exit/-/signal-exit-4.1.0.tgz",
- "integrity": "sha512-bzyZ1e88w9O1iNJbKnOlvYTrWPDl46O1bG0D3XInv+9tkPrxrN8jUUTiFlDkkmKWgn1M6CfIA13SuGqOa9Korw==",
- "engines": {
- "node": ">=14"
- },
- "funding": {
- "url": "https://github.com/sponsors/isaacs"
- }
- },
- "packages/scripts/node_modules/spawnd": {
- "version": "9.0.1",
- "resolved": "https://registry.npmjs.org/spawnd/-/spawnd-9.0.1.tgz",
- "integrity": "sha512-vaMk8E9CpbjTYToBxLXowDeArGf1+yI7A6PU6Nr57b2g8BVY8nRi5vTBj3bMF8UkCrMdTMyf/Lh+lrcrW2z7pw==",
- "dependencies": {
- "signal-exit": "^4.1.0",
- "tree-kill": "^1.2.2"
- },
- "engines": {
- "node": ">=16"
- }
- },
- "packages/scripts/node_modules/supports-color": {
- "version": "7.2.0",
- "resolved": "https://registry.npmjs.org/supports-color/-/supports-color-7.2.0.tgz",
- "integrity": "sha512-qpCAvRl9stuOHveKsn7HncJRvv501qIacKzQlO/+Lwxc9+0q2wLyv4Dfvt80/DPn2pqOBsJdDiogXGR9+OvwRw==",
- "dependencies": {
- "has-flag": "^4.0.0"
- },
- "engines": {
- "node": ">=8"
- }
- },
- "packages/scripts/node_modules/wait-on": {
- "version": "7.2.0",
- "resolved": "https://registry.npmjs.org/wait-on/-/wait-on-7.2.0.tgz",
- "integrity": "sha512-wCQcHkRazgjG5XoAq9jbTMLpNIjoSlZslrJ2+N9MxDsGEv1HnFoVjOCexL0ESva7Y9cu350j+DWADdk54s4AFQ==",
- "dependencies": {
- "axios": "^1.6.1",
- "joi": "^17.11.0",
- "lodash": "^4.17.21",
- "minimist": "^1.2.8",
- "rxjs": "^7.8.1"
- },
- "bin": {
- "wait-on": "bin/wait-on"
- },
- "engines": {
- "node": ">=12.0.0"
- }
- },
"packages/server-side-render": {
"name": "@wordpress/server-side-render",
- "version": "5.11.0",
+ "version": "5.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -56000,7 +52419,7 @@
},
"packages/shortcode": {
"name": "@wordpress/shortcode",
- "version": "4.11.0",
+ "version": "4.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -56013,7 +52432,7 @@
},
"packages/style-engine": {
"name": "@wordpress/style-engine",
- "version": "2.11.0",
+ "version": "2.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -56026,7 +52445,7 @@
},
"packages/stylelint-config": {
"name": "@wordpress/stylelint-config",
- "version": "23.3.0",
+ "version": "23.6.0",
"license": "MIT",
"dependencies": {
"@stylistic/stylelint-plugin": "^3.0.1",
@@ -56137,7 +52556,7 @@
},
"packages/sync": {
"name": "@wordpress/sync",
- "version": "1.11.0",
+ "version": "1.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -56158,7 +52577,7 @@
},
"packages/token-list": {
"name": "@wordpress/token-list",
- "version": "3.11.0",
+ "version": "3.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7"
@@ -56170,7 +52589,7 @@
},
"packages/undo-manager": {
"name": "@wordpress/undo-manager",
- "version": "1.11.0",
+ "version": "1.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -56181,9 +52600,31 @@
"npm": ">=8.19.2"
}
},
+ "packages/upload-media": {
+ "name": "@wordpress/upload-media",
+ "version": "1.0.0-prerelease",
+ "license": "GPL-2.0-or-later",
+ "dependencies": {
+ "@shopify/web-worker": "^6.4.0",
+ "@wordpress/api-fetch": "file:../api-fetch",
+ "@wordpress/blob": "file:../blob",
+ "@wordpress/compose": "file:../compose",
+ "@wordpress/data": "file:../data",
+ "@wordpress/element": "file:../element",
+ "@wordpress/i18n": "file:../i18n",
+ "@wordpress/preferences": "file:../preferences",
+ "@wordpress/private-apis": "file:../private-apis",
+ "@wordpress/url": "file:../url",
+ "uuid": "^9.0.1"
+ },
+ "engines": {
+ "node": ">=18.12.0",
+ "npm": ">=8.19.2"
+ }
+ },
"packages/url": {
"name": "@wordpress/url",
- "version": "4.11.0",
+ "version": "4.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -56196,7 +52637,7 @@
},
"packages/viewport": {
"name": "@wordpress/viewport",
- "version": "6.11.0",
+ "version": "6.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -56226,7 +52667,7 @@
},
"packages/warning": {
"name": "@wordpress/warning",
- "version": "3.11.0",
+ "version": "3.14.0",
"license": "GPL-2.0-or-later",
"engines": {
"node": ">=18.12.0",
@@ -56235,7 +52676,7 @@
},
"packages/widgets": {
"name": "@wordpress/widgets",
- "version": "4.11.0",
+ "version": "4.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7",
@@ -56263,7 +52704,7 @@
},
"packages/wordcount": {
"name": "@wordpress/wordcount",
- "version": "4.11.0",
+ "version": "4.14.0",
"license": "GPL-2.0-or-later",
"dependencies": {
"@babel/runtime": "7.25.7"
diff --git a/package.json b/package.json
index a2059f7d53a65..07d725c4a3edc 100644
--- a/package.json
+++ b/package.json
@@ -1,6 +1,6 @@
{
"name": "gutenberg",
- "version": "19.7.0-rc.1",
+ "version": "19.9.0-rc.1",
"private": true,
"description": "A new WordPress editor experience.",
"author": "The WordPress Contributors",
@@ -25,7 +25,7 @@
"@actions/core": "1.9.1",
"@actions/github": "5.0.0",
"@apidevtools/json-schema-ref-parser": "11.6.4",
- "@ariakit/test": "^0.4.2",
+ "@ariakit/test": "^0.4.7",
"@babel/core": "7.25.7",
"@babel/plugin-syntax-jsx": "7.25.7",
"@babel/runtime-corejs3": "7.25.7",
@@ -33,7 +33,7 @@
"@emotion/babel-plugin": "11.11.0",
"@emotion/jest": "11.7.1",
"@emotion/native": "11.0.0",
- "@geometricpanda/storybook-addon-badges": "2.0.1",
+ "@geometricpanda/storybook-addon-badges": "2.0.5",
"@octokit/rest": "16.26.0",
"@octokit/types": "6.34.0",
"@octokit/webhooks-types": "5.8.0",
@@ -42,16 +42,19 @@
"@react-native/babel-preset": "0.73.10",
"@react-native/metro-babel-transformer": "0.73.10",
"@react-native/metro-config": "0.73.4",
- "@storybook/addon-a11y": "7.6.15",
- "@storybook/addon-actions": "7.6.15",
- "@storybook/addon-controls": "7.6.15",
- "@storybook/addon-docs": "7.6.15",
- "@storybook/addon-toolbars": "7.6.15",
- "@storybook/addon-viewport": "7.6.15",
- "@storybook/react": "7.6.15",
- "@storybook/react-webpack5": "7.6.15",
- "@storybook/source-loader": "7.6.15",
- "@storybook/theming": "7.6.15",
+ "@storybook/addon-a11y": "8.4.7",
+ "@storybook/addon-actions": "8.4.7",
+ "@storybook/addon-controls": "8.4.7",
+ "@storybook/addon-docs": "8.4.7",
+ "@storybook/addon-toolbars": "8.4.7",
+ "@storybook/addon-viewport": "8.4.7",
+ "@storybook/addon-webpack5-compiler-babel": "3.0.3",
+ "@storybook/react": "8.4.7",
+ "@storybook/react-webpack5": "8.4.7",
+ "@storybook/source-loader": "8.4.7",
+ "@storybook/test": "8.4.7",
+ "@storybook/theming": "8.4.7",
+ "@storybook/types": "8.4.7",
"@testing-library/jest-dom": "5.16.5",
"@testing-library/react": "14.3.0",
"@testing-library/react-native": "12.4.3",
@@ -87,19 +90,21 @@
"commander": "9.2.0",
"concurrently": "3.5.0",
"copy-webpack-plugin": "10.2.0",
- "core-js-builder": "3.38.1",
- "cross-env": "3.2.4",
+ "core-js-builder": "3.39.0",
+ "cross-env": "7.0.3",
"css-loader": "6.2.0",
"cssnano": "6.0.1",
"deep-freeze": "0.0.1",
"equivalent-key-map": "0.2.2",
+ "esbuild": "0.18.20",
"escape-html": "1.0.3",
"eslint-import-resolver-node": "0.3.4",
"eslint-plugin-eslint-comments": "3.1.2",
"eslint-plugin-import": "2.25.2",
- "eslint-plugin-jest": "27.2.3",
+ "eslint-plugin-jest": "27.4.3",
"eslint-plugin-jest-dom": "5.0.2",
"eslint-plugin-prettier": "5.0.0",
+ "eslint-plugin-react-compiler": "19.0.0-beta-0dec889-20241115",
"eslint-plugin-ssr-friendly": "1.0.6",
"eslint-plugin-storybook": "0.6.13",
"eslint-plugin-testing-library": "6.0.2",
@@ -116,7 +121,7 @@
"jest-message-util": "29.6.2",
"jest-watch-typeahead": "2.2.2",
"json2md": "2.0.1",
- "lerna": "7.1.4",
+ "lerna": "8.1.9",
"lint-staged": "10.0.2",
"make-dir": "3.0.0",
"mkdirp": "3.0.1",
@@ -128,12 +133,11 @@
"npm-run-all": "4.1.5",
"patch-package": "8.0.0",
"postcss": "8.4.38",
- "postcss-import": "16.1.0",
"postcss-loader": "6.2.1",
"postcss-local-keyframes": "^0.0.2",
"prettier": "npm:wp-prettier@3.0.3",
"progress": "2.0.3",
- "puppeteer-core": "23.1.0",
+ "puppeteer-core": "23.10.1",
"raw-loader": "4.0.2",
"react": "18.3.1",
"react-docgen-typescript": "2.2.2",
@@ -146,25 +150,25 @@
"reassure": "0.7.1",
"redux": "5.0.1",
"resize-observer-polyfill": "1.5.1",
- "rimraf": "3.0.2",
+ "rimraf": "5.0.10",
"rtlcss": "4.0.0",
- "sass": "1.35.2",
- "sass-loader": "12.1.0",
+ "sass": "1.50.1",
+ "sass-loader": "16.0.3",
"semver": "7.5.4",
"simple-git": "3.24.0",
"snapshot-diff": "0.10.0",
"source-map-loader": "3.0.0",
"sprintf-js": "1.1.1",
- "storybook": "7.6.15",
+ "storybook": "8.4.7",
"storybook-source-link": "2.0.9",
"strip-json-comments": "5.0.0",
"style-loader": "3.2.1",
"terser": "5.32.0",
"terser-webpack-plugin": "5.3.10",
- "typescript": "5.5.3",
+ "typescript": "5.7.2",
"uuid": "9.0.1",
"webdriverio": "8.16.20",
- "webpack": "5.95.0",
+ "webpack": "5.97.0",
"webpack-bundle-analyzer": "4.9.1",
"worker-farm": "1.7.0"
},
@@ -177,9 +181,10 @@
"build:package-types": "node ./bin/packages/validate-typescript-version.js && ( tsc --build || ( echo 'tsc failed. Try cleaning up first: `npm run clean:package-types`'; exit 1 ) ) && node ./bin/packages/check-build-type-declaration-files.js",
"prebuild:packages": "npm run clean:packages && npm run --if-present --workspaces build",
"build:packages": "npm run --silent build:package-types && node ./bin/packages/build.js",
+ "postbuild:packages": " npm run --if-present --workspaces build:wp",
"build:plugin-zip": "bash ./bin/build-plugin-zip.sh",
- "clean:package-types": "tsc --build --clean && rimraf \"./packages/*/build-types\"",
- "clean:packages": "rimraf \"./packages/*/@(build|build-module|build-style)\"",
+ "clean:package-types": "tsc --build --clean && rimraf --glob \"./packages/*/build-types\"",
+ "clean:packages": "rimraf --glob \"./packages/*/{build,build-module,build-wp,build-style}\"",
"component-usage-stats": "node ./node_modules/react-scanner/bin/react-scanner -c ./react-scanner.config.js",
"dev": "cross-env NODE_ENV=development npm run build:packages && concurrently \"wp-scripts start\" \"npm run dev:packages\"",
"dev:packages": "cross-env NODE_ENV=development concurrently \"node ./bin/packages/watch.js\" \"tsc --build --watch\"",
@@ -191,7 +196,7 @@
"docs:gen": "node ./docs/tool/index.js",
"docs:theme-ref": "node ./bin/api-docs/gen-theme-reference.mjs",
"env": "wp-env",
- "fixtures:clean": "rimraf \"test/integration/fixtures/blocks/*.+(json|serialized.html)\"",
+ "fixtures:clean": "rimraf --glob \"test/integration/fixtures/blocks/*.{json,serialized.html}\"",
"fixtures:generate": "cross-env GENERATE_MISSING_FIXTURES=y npm run test:unit test/integration/full-content/ && npm run format test/integration/fixtures/blocks/*.json",
"fixtures:regenerate": "npm-run-all fixtures:clean fixtures:generate",
"format": "wp-scripts format",
diff --git a/packages/README.md b/packages/README.md
index f73aca35786f5..79f07af29382c 100644
--- a/packages/README.md
+++ b/packages/README.md
@@ -25,19 +25,25 @@ When creating a new package, you need to provide at least the following. Package
"bugs": {
"url": "https://github.com/WordPress/gutenberg/issues"
},
+ "engines": {
+ "node": ">=18.12.0",
+ "npm": ">=8.19.2"
+ },
"main": "build/index.js",
"module": "build-module/index.js",
"react-native": "src/index",
+ // Include this line to include the package as a WordPress script.
+ "wpScript": true,
+ // Include this line to include the package as a WordPress script module.
+ "wpScriptModuleExports": "./build-module/index.js",
+ "types": "build-types",
+ "sideEffects": false,
"dependencies": {
"@babel/runtime": "7.25.7"
},
"publishConfig": {
"access": "public"
- },
- // Include this line to include the package as a WordPress script.
- "wpScript": true,
- // Include this line to include the package as a WordPress script module.
- "wpScriptModuleExports": "./build-module/index.js"
+ }
}
```
@@ -84,7 +90,7 @@ When creating a new package, you need to provide at least the following. Package
Initial release.
```
-To ensure your package is recognized, you should also _manually_ add your new package to the root `package.json` file and then run `npm install` to update the dependencies.
+To ensure your package is recognized in npm workspaces, you should run `npm install` to update the package lock file.
## Managing Dependencies
@@ -255,13 +261,17 @@ For consumers to use the published type declarations, we'll set the `types` fiel
```json
{
"main": "build/index.js",
- "main-module": "build-module/index.js",
+ "module": "build-module/index.js",
"types": "build-types"
}
```
Ensure that the `build-types` directory will be included in the published package, for example if a `files` field is declared.
+## Supported Node.js and npm versions
+
+WordPress packages adhere the [Node.js Release Schedule](https://nodejs.org/en/about/previous-releases/). Consequently, the minimum required versions of Node.js and npm are specified using the `engines` field in `package.json` for all packages. This ensures that production applications run only on Active LTS or Maintenance LTS releases on Node.js. LTS release status is "long-term support", which typically guarantees that critical bugs will be fixed for a total of 30 months.
+
## Optimizing for bundlers
In order for bundlers to tree-shake packages effectively, they often need to know whether a package includes side effects in its code. This is done through the `sideEffects` field in the package's `package.json`.
diff --git a/packages/a11y/CHANGELOG.md b/packages/a11y/CHANGELOG.md
index 5e90577e9155a..d61f28833d3f6 100644
--- a/packages/a11y/CHANGELOG.md
+++ b/packages/a11y/CHANGELOG.md
@@ -2,6 +2,12 @@
## Unreleased
+## 4.14.0 (2024-12-11)
+
+## 4.13.0 (2024-11-27)
+
+## 4.12.0 (2024-11-16)
+
## 4.11.0 (2024-10-30)
## 4.10.0 (2024-10-16)
diff --git a/packages/a11y/package.json b/packages/a11y/package.json
index 008bd9088e077..6ad4f8acb9bd8 100644
--- a/packages/a11y/package.json
+++ b/packages/a11y/package.json
@@ -1,6 +1,6 @@
{
"name": "@wordpress/a11y",
- "version": "4.11.0",
+ "version": "4.14.0",
"description": "Accessibility (a11y) utilities for WordPress.",
"author": "The WordPress Contributors",
"license": "GPL-2.0-or-later",
diff --git a/packages/annotations/CHANGELOG.md b/packages/annotations/CHANGELOG.md
index 0618f4962f5eb..66433930b6375 100644
--- a/packages/annotations/CHANGELOG.md
+++ b/packages/annotations/CHANGELOG.md
@@ -2,6 +2,12 @@
## Unreleased
+## 3.14.0 (2024-12-11)
+
+## 3.13.0 (2024-11-27)
+
+## 3.12.0 (2024-11-16)
+
## 3.11.0 (2024-10-30)
## 3.10.0 (2024-10-16)
diff --git a/packages/annotations/package.json b/packages/annotations/package.json
index b1d6d210807a8..e10ece8600e81 100644
--- a/packages/annotations/package.json
+++ b/packages/annotations/package.json
@@ -1,6 +1,6 @@
{
"name": "@wordpress/annotations",
- "version": "3.11.0",
+ "version": "3.14.0",
"description": "Annotate content in the Gutenberg editor.",
"author": "The WordPress Contributors",
"license": "GPL-2.0-or-later",
diff --git a/packages/api-fetch/CHANGELOG.md b/packages/api-fetch/CHANGELOG.md
index 8d3faea535faf..d1d481d6ff9fc 100644
--- a/packages/api-fetch/CHANGELOG.md
+++ b/packages/api-fetch/CHANGELOG.md
@@ -2,6 +2,12 @@
## Unreleased
+## 7.14.0 (2024-12-11)
+
+## 7.13.0 (2024-11-27)
+
+## 7.12.0 (2024-11-16)
+
## 7.11.0 (2024-10-30)
## 7.10.0 (2024-10-16)
diff --git a/packages/api-fetch/package.json b/packages/api-fetch/package.json
index 7d5b8dfd58889..fd2430dfc7760 100644
--- a/packages/api-fetch/package.json
+++ b/packages/api-fetch/package.json
@@ -1,6 +1,6 @@
{
"name": "@wordpress/api-fetch",
- "version": "7.11.0",
+ "version": "7.14.0",
"description": "Utility to make WordPress REST API requests.",
"author": "The WordPress Contributors",
"license": "GPL-2.0-or-later",
diff --git a/packages/autop/CHANGELOG.md b/packages/autop/CHANGELOG.md
index 9534b183d6ebb..fc054bb8c14e0 100644
--- a/packages/autop/CHANGELOG.md
+++ b/packages/autop/CHANGELOG.md
@@ -2,6 +2,12 @@
## Unreleased
+## 4.14.0 (2024-12-11)
+
+## 4.13.0 (2024-11-27)
+
+## 4.12.0 (2024-11-16)
+
## 4.11.0 (2024-10-30)
## 4.10.0 (2024-10-16)
diff --git a/packages/autop/package.json b/packages/autop/package.json
index 336dda06edfe2..cdffb6175b31e 100644
--- a/packages/autop/package.json
+++ b/packages/autop/package.json
@@ -1,6 +1,6 @@
{
"name": "@wordpress/autop",
- "version": "4.11.0",
+ "version": "4.14.0",
"description": "WordPress's automatic paragraph functions `autop` and `removep`.",
"author": "The WordPress Contributors",
"license": "GPL-2.0-or-later",
diff --git a/packages/babel-plugin-import-jsx-pragma/CHANGELOG.md b/packages/babel-plugin-import-jsx-pragma/CHANGELOG.md
index 87bc412fd90eb..d372ad314b1b6 100644
--- a/packages/babel-plugin-import-jsx-pragma/CHANGELOG.md
+++ b/packages/babel-plugin-import-jsx-pragma/CHANGELOG.md
@@ -2,6 +2,12 @@
## Unreleased
+## 5.14.0 (2024-12-11)
+
+## 5.13.0 (2024-11-27)
+
+## 5.12.0 (2024-11-16)
+
## 5.11.0 (2024-10-30)
## 5.10.0 (2024-10-16)
diff --git a/packages/babel-plugin-import-jsx-pragma/package.json b/packages/babel-plugin-import-jsx-pragma/package.json
index d9b565b4e27ec..d7ebed0e46f28 100644
--- a/packages/babel-plugin-import-jsx-pragma/package.json
+++ b/packages/babel-plugin-import-jsx-pragma/package.json
@@ -1,6 +1,6 @@
{
"name": "@wordpress/babel-plugin-import-jsx-pragma",
- "version": "5.11.0",
+ "version": "5.14.0",
"description": "Babel transform plugin for automatically injecting an import to be used as the pragma for the React JSX Transform plugin.",
"author": "The WordPress Contributors",
"license": "GPL-2.0-or-later",
diff --git a/packages/babel-plugin-makepot/CHANGELOG.md b/packages/babel-plugin-makepot/CHANGELOG.md
index f643a9304c68a..7f608c6704635 100644
--- a/packages/babel-plugin-makepot/CHANGELOG.md
+++ b/packages/babel-plugin-makepot/CHANGELOG.md
@@ -2,6 +2,12 @@
## Unreleased
+## 6.14.0 (2024-12-11)
+
+## 6.13.0 (2024-11-27)
+
+## 6.12.0 (2024-11-16)
+
## 6.11.0 (2024-10-30)
## 6.10.0 (2024-10-16)
diff --git a/packages/babel-plugin-makepot/package.json b/packages/babel-plugin-makepot/package.json
index cf04d3dc72e40..352da89d84b37 100644
--- a/packages/babel-plugin-makepot/package.json
+++ b/packages/babel-plugin-makepot/package.json
@@ -1,6 +1,6 @@
{
"name": "@wordpress/babel-plugin-makepot",
- "version": "6.11.0",
+ "version": "6.14.0",
"description": "WordPress Babel internationalization (i18n) plugin.",
"author": "The WordPress Contributors",
"license": "GPL-2.0-or-later",
diff --git a/packages/babel-preset-default/CHANGELOG.md b/packages/babel-preset-default/CHANGELOG.md
index d6eef13b90d45..1401fc5d1452b 100644
--- a/packages/babel-preset-default/CHANGELOG.md
+++ b/packages/babel-preset-default/CHANGELOG.md
@@ -2,6 +2,12 @@
## Unreleased
+## 8.14.0 (2024-12-11)
+
+## 8.13.0 (2024-11-27)
+
+## 8.12.0 (2024-11-16)
+
## 8.11.0 (2024-10-30)
## 8.10.0 (2024-10-16)
diff --git a/packages/babel-preset-default/package.json b/packages/babel-preset-default/package.json
index 2f727d1886425..b983a198f42f9 100644
--- a/packages/babel-preset-default/package.json
+++ b/packages/babel-preset-default/package.json
@@ -1,6 +1,6 @@
{
"name": "@wordpress/babel-preset-default",
- "version": "8.11.0",
+ "version": "8.14.0",
"description": "Default Babel preset for WordPress development.",
"author": "The WordPress Contributors",
"license": "GPL-2.0-or-later",
diff --git a/packages/babel-preset-default/polyfill-exclusions.js b/packages/babel-preset-default/polyfill-exclusions.js
index 507396c930b99..ca8c045d12414 100644
--- a/packages/babel-preset-default/polyfill-exclusions.js
+++ b/packages/babel-preset-default/polyfill-exclusions.js
@@ -7,4 +7,25 @@ module.exports = [
// This is an IE-only feature which we don't use, and don't want to polyfill.
// @see https://github.com/WordPress/gutenberg/pull/49234
'web.immediate',
+ // Remove Set feature polyfills.
+ //
+ // The Babel/core-js integration has a severe limitation, in that any Set
+ // objects (e.g. `new Set()`) are assumed to need all instance methods, and
+ // get them all polyfilled. There is no validation as to whether those
+ // methods are actually in use.
+ //
+ // This limitation causes a number of packages to unnecessarily get a
+ // dependency on `wp-polyfill`, which in most cases gets loaded as part of
+ // the critical path and can thus have an impact on performance.
+ //
+ // There is no good solution to this, and the one we've opted for here is
+ // to disable polyfilling these features entirely. Developers will need to
+ // take care not to use them in scenarios where the code may be running in
+ // older browsers without native support for them.
+ //
+ // These need to be specified as both `es.` and `esnext.` due to the way
+ // internal dependencies are set up in Babel / core-js.
+ //
+ // @see https://github.com/WordPress/gutenberg/pull/67230
+ /^es(next)?\.set\./,
];
diff --git a/packages/base-styles/CHANGELOG.md b/packages/base-styles/CHANGELOG.md
index 61ff1100ac645..ccdb7976cd0c2 100644
--- a/packages/base-styles/CHANGELOG.md
+++ b/packages/base-styles/CHANGELOG.md
@@ -2,6 +2,12 @@
## Unreleased
+## 5.14.0 (2024-12-11)
+
+## 5.13.0 (2024-11-27)
+
+## 5.12.0 (2024-11-16)
+
## 5.11.0 (2024-10-30)
## 5.10.0 (2024-10-16)
diff --git a/packages/base-styles/_animations.scss b/packages/base-styles/_animations.scss
index ae5de9a803008..e5bbf86375735 100644
--- a/packages/base-styles/_animations.scss
+++ b/packages/base-styles/_animations.scss
@@ -41,8 +41,3 @@
@warn "The `edit-post__fade-in-animation` mixin is deprecated. Use `animation__fade-in` instead.";
@include animation__fade-in($speed, $delay);
}
-
-@mixin editor-canvas-resize-animation($additional-transition-rules...) {
- transition: all 400ms cubic-bezier(0.46, 0.03, 0.52, 0.96), $additional-transition-rules;
- @include reduce-motion("transition");
-}
diff --git a/packages/base-styles/_mixins.scss b/packages/base-styles/_mixins.scss
index 65f98bf6f15bf..e2f953e578781 100644
--- a/packages/base-styles/_mixins.scss
+++ b/packages/base-styles/_mixins.scss
@@ -18,25 +18,25 @@
@mixin heading-small() {
@include _text-heading();
font-size: $font-size-x-small;
- line-height: $line-height-x-small;
+ line-height: $font-line-height-x-small;
}
@mixin heading-medium() {
@include _text-heading();
font-size: $font-size-medium;
- line-height: $line-height-small;
+ line-height: $font-line-height-small;
}
@mixin heading-large() {
@include _text-heading();
font-size: $font-size-large;
- line-height: $line-height-small;
+ line-height: $font-line-height-small;
}
@mixin heading-x-large() {
@include _text-heading();
font-size: $font-size-x-large;
- line-height: $line-height-medium;
+ line-height: $font-line-height-medium;
}
@mixin heading-2x-large() {
@@ -48,25 +48,25 @@
@mixin body-small() {
@include _text-body();
font-size: $font-size-small;
- line-height: $line-height-x-small;
+ line-height: $font-line-height-x-small;
}
@mixin body-medium() {
@include _text-body();
font-size: $font-size-medium;
- line-height: $line-height-small;
+ line-height: $font-line-height-small;
}
@mixin body-large() {
@include _text-body();
font-size: $font-size-large;
- line-height: $line-height-medium;
+ line-height: $font-line-height-medium;
}
@mixin body-x-large() {
@include _text-body();
font-size: $font-size-x-large;
- line-height: $line-height-x-large;
+ line-height: $font-line-height-x-large;
}
/**
diff --git a/packages/base-styles/_z-index.scss b/packages/base-styles/_z-index.scss
index e4d6ce4ce1b1c..af679edb91064 100644
--- a/packages/base-styles/_z-index.scss
+++ b/packages/base-styles/_z-index.scss
@@ -123,7 +123,7 @@ $z-layers: (
// Should be above the popover (dropdown)
".reusable-blocks-menu-items__convert-modal": 1000001,
".patterns-menu-items__convert-modal": 1000001,
- ".editor-create-template-part-modal": 1000001,
+ ".fields-create-template-part-modal": 1000001,
".block-editor-block-lock-modal": 1000001,
".block-editor-template-part__selection-modal": 1000001,
".block-editor-block-rename-modal": 1000001,
@@ -132,6 +132,7 @@ $z-layers: (
".editor-action-modal": 1000001,
".editor-post-template__swap-template-modal": 1000001,
".edit-site-template-panel__replace-template-modal": 1000001,
+ ".fields-controls__template-modal": 1000001,
// Note: The ConfirmDialog component's z-index is being set to 1000001 in packages/components/src/confirm-dialog/styles.ts
// because it uses emotion and not sass. We need it to render on top its parent popover.
@@ -165,9 +166,9 @@ $z-layers: (
".components-resizable-box__corner-handle": 2,
// Make sure block manager sticky category titles appear above the options
- ".editor-block-manager__category-title": 1,
+ ".block-editor-block-manager__category-title": 1,
// And block manager sticky disabled block count is higher still
- ".editor-block-manager__disabled-blocks-count": 2,
+ ".block-editor-block-manager__disabled-blocks-count": 2,
// Needs to appear below other color circular picker related UI elements.
".components-circular-option-picker__option-wrapper::before": -1,
diff --git a/packages/base-styles/package.json b/packages/base-styles/package.json
index 7246dc83eaf4c..0677b61ca0bfd 100644
--- a/packages/base-styles/package.json
+++ b/packages/base-styles/package.json
@@ -1,6 +1,6 @@
{
"name": "@wordpress/base-styles",
- "version": "5.11.0",
+ "version": "5.14.0",
"description": "Base SCSS utilities and variables for WordPress.",
"author": "The WordPress Contributors",
"license": "GPL-2.0-or-later",
diff --git a/packages/blob/CHANGELOG.md b/packages/blob/CHANGELOG.md
index 660c60776c7bd..03c4724426eb6 100644
--- a/packages/blob/CHANGELOG.md
+++ b/packages/blob/CHANGELOG.md
@@ -2,6 +2,12 @@
## Unreleased
+## 4.14.0 (2024-12-11)
+
+## 4.13.0 (2024-11-27)
+
+## 4.12.0 (2024-11-16)
+
## 4.11.0 (2024-10-30)
## 4.10.0 (2024-10-16)
diff --git a/packages/blob/package.json b/packages/blob/package.json
index 0dc01ac7198f5..b69a5c2a5d913 100644
--- a/packages/blob/package.json
+++ b/packages/blob/package.json
@@ -1,6 +1,6 @@
{
"name": "@wordpress/blob",
- "version": "4.11.0",
+ "version": "4.14.0",
"description": "Blob utilities for WordPress.",
"author": "The WordPress Contributors",
"license": "GPL-2.0-or-later",
diff --git a/packages/block-directory/CHANGELOG.md b/packages/block-directory/CHANGELOG.md
index 53636f34dad58..eb6b832b407e1 100644
--- a/packages/block-directory/CHANGELOG.md
+++ b/packages/block-directory/CHANGELOG.md
@@ -2,6 +2,12 @@
## Unreleased
+## 5.14.0 (2024-12-11)
+
+## 5.13.0 (2024-11-27)
+
+## 5.12.0 (2024-11-16)
+
## 5.11.0 (2024-10-30)
## 5.10.0 (2024-10-16)
diff --git a/packages/block-directory/package.json b/packages/block-directory/package.json
index 18a40824aa475..fc1176b98fa3a 100644
--- a/packages/block-directory/package.json
+++ b/packages/block-directory/package.json
@@ -1,6 +1,6 @@
{
"name": "@wordpress/block-directory",
- "version": "5.11.0",
+ "version": "5.14.0",
"description": "Extend editor with block directory features to search, download and install blocks.",
"author": "The WordPress Contributors",
"license": "GPL-2.0-or-later",
diff --git a/packages/block-editor/CHANGELOG.md b/packages/block-editor/CHANGELOG.md
index 6755c4ec83f21..06e1c9a4a746e 100644
--- a/packages/block-editor/CHANGELOG.md
+++ b/packages/block-editor/CHANGELOG.md
@@ -2,6 +2,12 @@
## Unreleased
+## 14.9.0 (2024-12-11)
+
+## 14.8.0 (2024-11-27)
+
+## 14.7.0 (2024-11-16)
+
## 14.6.0 (2024-10-30)
## 14.5.0 (2024-10-16)
diff --git a/packages/block-editor/README.md b/packages/block-editor/README.md
index a0f7568391440..13dffce114f59 100644
--- a/packages/block-editor/README.md
+++ b/packages/block-editor/README.md
@@ -659,6 +659,14 @@ _Related_
-
+### LinkControl
+
+Renders a link control. A link control is a controlled input which maintains a value associated with a link (HTML anchor element) and relevant settings for how that link is expected to behave.
+
+_Parameters_
+
+- _props_ `WPLinkControlProps`: Component props.
+
### MediaPlaceholder
_Related_
diff --git a/packages/block-editor/package.json b/packages/block-editor/package.json
index b4672bc57690e..c5e82b5924585 100644
--- a/packages/block-editor/package.json
+++ b/packages/block-editor/package.json
@@ -1,6 +1,6 @@
{
"name": "@wordpress/block-editor",
- "version": "14.6.0",
+ "version": "14.9.0",
"description": "Generic block editor.",
"author": "The WordPress Contributors",
"license": "GPL-2.0-or-later",
diff --git a/packages/block-editor/src/autocompleters/block.js b/packages/block-editor/src/autocompleters/block.js
index 5fc107c4d3d69..eedd5e102db2c 100644
--- a/packages/block-editor/src/autocompleters/block.js
+++ b/packages/block-editor/src/autocompleters/block.js
@@ -23,12 +23,10 @@ import { orderInserterBlockItems } from '../utils/order-inserter-block-items';
const noop = () => {};
const SHOWN_BLOCK_TYPES = 9;
-/** @typedef {import('@wordpress/components').WPCompleter} WPCompleter */
-
/**
* Creates a blocks repeater for replacing the current block with a selected block type.
*
- * @return {WPCompleter} A blocks completer.
+ * @return {Object} A blocks completer.
*/
function createBlockCompleter() {
return {
@@ -157,6 +155,6 @@ function createBlockCompleter() {
/**
* Creates a blocks repeater for replacing the current block with a selected block type.
*
- * @return {WPCompleter} A blocks completer.
+ * @return {Object} A blocks completer.
*/
export default createBlockCompleter();
diff --git a/packages/block-editor/src/autocompleters/link.js b/packages/block-editor/src/autocompleters/link.js
index fb64cb151294d..9088b65b4abc8 100644
--- a/packages/block-editor/src/autocompleters/link.js
+++ b/packages/block-editor/src/autocompleters/link.js
@@ -10,12 +10,10 @@ import { decodeEntities } from '@wordpress/html-entities';
const SHOWN_SUGGESTIONS = 10;
-/** @typedef {import('@wordpress/components').WPCompleter} WPCompleter */
-
/**
* Creates a suggestion list for links to posts or pages.
*
- * @return {WPCompleter} A links completer.
+ * @return {Object} A links completer.
*/
function createLinkCompleter() {
return {
@@ -60,6 +58,6 @@ function createLinkCompleter() {
/**
* Creates a suggestion list for links to posts or pages..
*
- * @return {WPCompleter} A link completer.
+ * @return {Object} A link completer.
*/
export default createLinkCompleter();
diff --git a/packages/block-editor/src/components/alignment-control/stories/aliginment-toolbar.story.js b/packages/block-editor/src/components/alignment-control/stories/aliginment-toolbar.story.js
new file mode 100644
index 0000000000000..9d029c30b4846
--- /dev/null
+++ b/packages/block-editor/src/components/alignment-control/stories/aliginment-toolbar.story.js
@@ -0,0 +1,47 @@
+/**
+ * WordPress dependencies
+ */
+import { useState } from '@wordpress/element';
+
+/**
+ * Internal dependencies
+ */
+import { AlignmentToolbar } from '..';
+
+/**
+ * The `AlignmentToolbar` component renders a dropdown menu that displays alignment options for the selected block in `Toolbar`.
+ */
+const meta = {
+ title: 'BlockEditor/AlignmentToolbar',
+ component: AlignmentToolbar,
+ argTypes: {
+ value: {
+ control: false,
+ defaultValue: 'undefined',
+ description: 'The current value of the alignment setting.',
+ },
+ onChange: {
+ action: 'onChange',
+ control: false,
+ description:
+ "A callback function invoked when the toolbar's alignment value is changed via an interaction with any of the toolbar's buttons. Called with the new alignment value (ie: `left`, `center`, `right`, `undefined`) as the only argument.",
+ },
+ },
+};
+export default meta;
+
+export const Default = {
+ render: function Template( { onChange, ...args } ) {
+ const [ value, setValue ] = useState();
+ return (
+ {
+ onChange( ...changeArgs );
+ setValue( ...changeArgs );
+ } }
+ value={ value }
+ />
+ );
+ },
+};
diff --git a/packages/block-editor/src/components/alignment-control/stories/index.story.js b/packages/block-editor/src/components/alignment-control/stories/index.story.js
new file mode 100644
index 0000000000000..165f9343e1710
--- /dev/null
+++ b/packages/block-editor/src/components/alignment-control/stories/index.story.js
@@ -0,0 +1,51 @@
+/**
+ * WordPress dependencies
+ */
+import { useState } from '@wordpress/element';
+
+/**
+ * Internal dependencies
+ */
+import { AlignmentControl } from '../';
+
+/**
+ * The `AlignmentControl` component renders a dropdown menu that displays alignment options for the selected block.
+ *
+ * This component is mostly used for blocks that display text, such as Heading, Paragraph, Post Author, Post Comments, Verse, Quote, Post Title, etc... And the available alignment options are `left`, `center` or `right` alignment.
+ *
+ * If you want to use the alignment control in a toolbar, you should use the `AlignmentToolbar` component instead.
+ */
+const meta = {
+ title: 'BlockEditor/AlignmentControl',
+ component: AlignmentControl,
+ argTypes: {
+ value: {
+ control: false,
+ defaultValue: 'undefined',
+ description: 'The current value of the alignment setting.',
+ },
+ onChange: {
+ action: 'onChange',
+ control: false,
+ description:
+ "A callback function invoked when the toolbar's alignment value is changed via an interaction with any of the toolbar's buttons. Called with the new alignment value (ie: `left`, `center`, `right`, `undefined`) as the only argument.",
+ },
+ },
+};
+export default meta;
+
+export const Default = {
+ render: function Template( { onChange, ...args } ) {
+ const [ value, setValue ] = useState();
+ return (
+ {
+ onChange( ...changeArgs );
+ setValue( ...changeArgs );
+ } }
+ value={ value }
+ />
+ );
+ },
+};
diff --git a/packages/block-editor/src/components/alignment-control/test/__snapshots__/index.js.snap b/packages/block-editor/src/components/alignment-control/test/__snapshots__/index.js.snap
index f2915ead7417b..c1383ae8ecc44 100644
--- a/packages/block-editor/src/components/alignment-control/test/__snapshots__/index.js.snap
+++ b/packages/block-editor/src/components/alignment-control/test/__snapshots__/index.js.snap
@@ -12,7 +12,7 @@ exports[`AlignmentUI should allow custom alignment controls to be specified 1`]
align="custom-left"
aria-label="My custom left"
aria-pressed="false"
- class="components-button components-toolbar__control has-icon"
+ class="components-button components-toolbar__control is-compact has-icon"
data-toolbar-item="true"
type="button"
>
@@ -35,7 +35,7 @@ exports[`AlignmentUI should allow custom alignment controls to be specified 1`]
align="custom-right"
aria-label="My custom right"
aria-pressed="true"
- class="components-button components-toolbar__control is-pressed has-icon"
+ class="components-button components-toolbar__control is-compact is-pressed has-icon"
data-toolbar-item="true"
type="button"
>
@@ -100,7 +100,7 @@ exports[`AlignmentUI should match snapshot when controls are visible 1`] = `
align="left"
aria-label="Align text left"
aria-pressed="true"
- class="components-button components-toolbar__control is-pressed has-icon"
+ class="components-button components-toolbar__control is-compact is-pressed has-icon"
data-toolbar-item="true"
type="button"
>
@@ -123,7 +123,7 @@ exports[`AlignmentUI should match snapshot when controls are visible 1`] = `
align="center"
aria-label="Align text center"
aria-pressed="false"
- class="components-button components-toolbar__control has-icon"
+ class="components-button components-toolbar__control is-compact has-icon"
data-toolbar-item="true"
type="button"
>
@@ -146,7 +146,7 @@ exports[`AlignmentUI should match snapshot when controls are visible 1`] = `
align="right"
aria-label="Align text right"
aria-pressed="false"
- class="components-button components-toolbar__control has-icon"
+ class="components-button components-toolbar__control is-compact has-icon"
data-toolbar-item="true"
type="button"
>
diff --git a/packages/block-editor/src/components/audio-player/index.native.js b/packages/block-editor/src/components/audio-player/index.native.js
index bee31ea5872ef..734226408cb92 100644
--- a/packages/block-editor/src/components/audio-player/index.native.js
+++ b/packages/block-editor/src/components/audio-player/index.native.js
@@ -17,7 +17,7 @@ import { View } from '@wordpress/primitives';
import { Icon } from '@wordpress/components';
import { withPreferredColorScheme } from '@wordpress/compose';
import { __ } from '@wordpress/i18n';
-import { audio, warning } from '@wordpress/icons';
+import { audio, cautionFilled } from '@wordpress/icons';
import {
requestImageFailedRetryDialog,
requestImageUploadCancelDialog,
@@ -167,7 +167,7 @@ function Player( {
{ isUploadFailed && (
@@ -64,7 +64,7 @@ exports[`BlockAlignmentUI should match snapshot when controls are visible 1`] =
@@ -86,7 +86,7 @@ exports[`BlockAlignmentUI should match snapshot when controls are visible 1`] =
@@ -108,7 +108,7 @@ exports[`BlockAlignmentUI should match snapshot when controls are visible 1`] =
diff --git a/packages/block-editor/src/components/block-canvas/index.js b/packages/block-editor/src/components/block-canvas/index.js
index c399f38054ed4..36aca7fa1c722 100644
--- a/packages/block-editor/src/components/block-canvas/index.js
+++ b/packages/block-editor/src/components/block-canvas/index.js
@@ -56,7 +56,8 @@ export function ExperimentalBlockCanvas( {
return (
{ children }
@@ -81,6 +78,7 @@ export function ExperimentalBlockCanvas( {
return (
;
if ( group === 'default' ) {
diff --git a/packages/block-editor/src/components/block-draggable/content.scss b/packages/block-editor/src/components/block-draggable/content.scss
index 102230168e213..25a0f5c256595 100644
--- a/packages/block-editor/src/components/block-draggable/content.scss
+++ b/packages/block-editor/src/components/block-draggable/content.scss
@@ -1,13 +1,12 @@
// This creates a "slot" where the block you're dragging appeared.
// We use !important as one of the rules are meant to be overridden.
.block-editor-block-list__layout .is-dragging {
- background-color: currentColor !important;
- opacity: 0.05 !important;
+ opacity: 0.1 !important;
border-radius: $radius-small !important;
- // Disabling pointer events during the drag event is necessary,
- // lest the block might affect your drag operation.
- pointer-events: none !important;
+ iframe {
+ pointer-events: none;
+ }
// Hide the multi selection indicator when dragging.
&::selection {
@@ -18,3 +17,10 @@
content: none !important;
}
}
+
+// Images are draggable by default, so disable drag for them if not explicitly
+// set. This is done so that the block can capture the drag event instead.
+.wp-block img:not([draggable]),
+.wp-block svg:not([draggable]) {
+ pointer-events: none;
+}
diff --git a/packages/block-editor/src/components/block-edit/edit.js b/packages/block-editor/src/components/block-edit/edit.js
index 83d0e3f406f82..27d3650f3a090 100644
--- a/packages/block-editor/src/components/block-edit/edit.js
+++ b/packages/block-editor/src/components/block-edit/edit.js
@@ -6,18 +6,27 @@ import clsx from 'clsx';
/**
* WordPress dependencies
*/
-import { withFilters } from '@wordpress/components';
import {
getBlockDefaultClassName,
- hasBlockSupport,
getBlockType,
+ hasBlockSupport,
+ store as blocksStore,
} from '@wordpress/blocks';
-import { useContext, useMemo } from '@wordpress/element';
+import { withFilters } from '@wordpress/components';
+import { useRegistry, useSelect } from '@wordpress/data';
+import { useCallback, useContext, useMemo } from '@wordpress/element';
/**
* Internal dependencies
*/
import BlockContext from '../block-context';
+import isURLLike from '../link-control/is-url-like';
+import {
+ canBindAttribute,
+ hasPatternOverridesDefaultBinding,
+ replacePatternOverridesDefaultBinding,
+} from '../../utils/block-bindings';
+import { unlock } from '../../lock-unlock';
/**
* Default value used for blocks which do not define their own context needs,
@@ -48,27 +57,223 @@ const Edit = ( props ) => {
const EditWithFilters = withFilters( 'editor.BlockEdit' )( Edit );
const EditWithGeneratedProps = ( props ) => {
- const { attributes = {}, name } = props;
+ const { name, clientId, attributes, setAttributes } = props;
+ const registry = useRegistry();
const blockType = getBlockType( name );
const blockContext = useContext( BlockContext );
+ const registeredSources = useSelect(
+ ( select ) =>
+ unlock( select( blocksStore ) ).getAllBlockBindingsSources(),
+ []
+ );
- // Assign context values using the block type's declared context needs.
- const context = useMemo( () => {
- return blockType && blockType.usesContext
+ const { blockBindings, context, hasPatternOverrides } = useMemo( () => {
+ // Assign context values using the block type's declared context needs.
+ const computedContext = blockType?.usesContext
? Object.fromEntries(
Object.entries( blockContext ).filter( ( [ key ] ) =>
blockType.usesContext.includes( key )
)
)
: DEFAULT_BLOCK_CONTEXT;
- }, [ blockType, blockContext ] );
+ // Add context requested by Block Bindings sources.
+ if ( attributes?.metadata?.bindings ) {
+ Object.values( attributes?.metadata?.bindings || {} ).forEach(
+ ( binding ) => {
+ registeredSources[ binding?.source ]?.usesContext?.forEach(
+ ( key ) => {
+ computedContext[ key ] = blockContext[ key ];
+ }
+ );
+ }
+ );
+ }
+ return {
+ blockBindings: replacePatternOverridesDefaultBinding(
+ name,
+ attributes?.metadata?.bindings
+ ),
+ context: computedContext,
+ hasPatternOverrides: hasPatternOverridesDefaultBinding(
+ attributes?.metadata?.bindings
+ ),
+ };
+ }, [
+ name,
+ blockType?.usesContext,
+ blockContext,
+ attributes?.metadata?.bindings,
+ registeredSources,
+ ] );
+
+ const computedAttributes = useSelect(
+ ( select ) => {
+ if ( ! blockBindings ) {
+ return attributes;
+ }
+
+ const attributesFromSources = {};
+ const blockBindingsBySource = new Map();
+
+ for ( const [ attributeName, binding ] of Object.entries(
+ blockBindings
+ ) ) {
+ const { source: sourceName, args: sourceArgs } = binding;
+ const source = registeredSources[ sourceName ];
+ if ( ! source || ! canBindAttribute( name, attributeName ) ) {
+ continue;
+ }
+
+ blockBindingsBySource.set( source, {
+ ...blockBindingsBySource.get( source ),
+ [ attributeName ]: {
+ args: sourceArgs,
+ },
+ } );
+ }
+
+ if ( blockBindingsBySource.size ) {
+ for ( const [ source, bindings ] of blockBindingsBySource ) {
+ // Get values in batch if the source supports it.
+ let values = {};
+ if ( ! source.getValues ) {
+ Object.keys( bindings ).forEach( ( attr ) => {
+ // Default to the the source label when `getValues` doesn't exist.
+ values[ attr ] = source.label;
+ } );
+ } else {
+ values = source.getValues( {
+ select,
+ context,
+ clientId,
+ bindings,
+ } );
+ }
+ for ( const [ attributeName, value ] of Object.entries(
+ values
+ ) ) {
+ if (
+ attributeName === 'url' &&
+ ( ! value || ! isURLLike( value ) )
+ ) {
+ // Return null if value is not a valid URL.
+ attributesFromSources[ attributeName ] = null;
+ } else {
+ attributesFromSources[ attributeName ] = value;
+ }
+ }
+ }
+ }
+
+ return {
+ ...attributes,
+ ...attributesFromSources,
+ };
+ },
+ [
+ attributes,
+ blockBindings,
+ clientId,
+ context,
+ name,
+ registeredSources,
+ ]
+ );
+
+ const setBoundAttributes = useCallback(
+ ( nextAttributes ) => {
+ if ( ! blockBindings ) {
+ setAttributes( nextAttributes );
+ return;
+ }
+
+ registry.batch( () => {
+ const keptAttributes = { ...nextAttributes };
+ const blockBindingsBySource = new Map();
+
+ // Loop only over the updated attributes to avoid modifying the bound ones that haven't changed.
+ for ( const [ attributeName, newValue ] of Object.entries(
+ keptAttributes
+ ) ) {
+ if (
+ ! blockBindings[ attributeName ] ||
+ ! canBindAttribute( name, attributeName )
+ ) {
+ continue;
+ }
+
+ const binding = blockBindings[ attributeName ];
+ const source = registeredSources[ binding?.source ];
+ if ( ! source?.setValues ) {
+ continue;
+ }
+ blockBindingsBySource.set( source, {
+ ...blockBindingsBySource.get( source ),
+ [ attributeName ]: {
+ args: binding.args,
+ newValue,
+ },
+ } );
+ delete keptAttributes[ attributeName ];
+ }
+
+ if ( blockBindingsBySource.size ) {
+ for ( const [
+ source,
+ bindings,
+ ] of blockBindingsBySource ) {
+ source.setValues( {
+ select: registry.select,
+ dispatch: registry.dispatch,
+ context,
+ clientId,
+ bindings,
+ } );
+ }
+ }
+
+ const hasParentPattern = !! context[ 'pattern/overrides' ];
+
+ if (
+ // Don't update non-connected attributes if the block is using pattern overrides
+ // and the editing is happening while overriding the pattern (not editing the original).
+ ! ( hasPatternOverrides && hasParentPattern ) &&
+ Object.keys( keptAttributes ).length
+ ) {
+ // Don't update caption and href until they are supported.
+ if ( hasPatternOverrides ) {
+ delete keptAttributes.caption;
+ delete keptAttributes.href;
+ }
+ setAttributes( keptAttributes );
+ }
+ } );
+ },
+ [
+ blockBindings,
+ clientId,
+ context,
+ hasPatternOverrides,
+ setAttributes,
+ registeredSources,
+ name,
+ registry,
+ ]
+ );
if ( ! blockType ) {
return null;
}
if ( blockType.apiVersion > 1 ) {
- return ;
+ return (
+
+ );
}
// Generate a class name for the block's editable form.
@@ -77,15 +282,17 @@ const EditWithGeneratedProps = ( props ) => {
: null;
const className = clsx(
generatedClassName,
- attributes.className,
+ attributes?.className,
props.className
);
return (
);
};
diff --git a/packages/block-editor/src/components/block-heading-level-dropdown/stories/index.story.js b/packages/block-editor/src/components/block-heading-level-dropdown/stories/index.story.js
new file mode 100644
index 0000000000000..a8293258f7a00
--- /dev/null
+++ b/packages/block-editor/src/components/block-heading-level-dropdown/stories/index.story.js
@@ -0,0 +1,61 @@
+/**
+ * WordPress dependencies
+ */
+import { useState } from '@wordpress/element';
+
+/**
+ * Internal dependencies
+ */
+import HeadingLevelDropdown from '../';
+
+const meta = {
+ title: 'BlockEditor/HeadingLevelDropdown',
+ component: HeadingLevelDropdown,
+ parameters: {
+ docs: {
+ canvas: { sourceState: 'shown' },
+ description: {
+ component:
+ 'Dropdown for selecting a heading level (1 through 6) or paragraph (0).',
+ },
+ },
+ },
+ argTypes: {
+ value: {
+ control: { type: null },
+ description: 'The chosen heading level.',
+ },
+ options: {
+ control: 'check',
+ options: [ 1, 2, 3, 4, 5, 6 ],
+ description: 'An array of supported heading levels.',
+ },
+ onChange: {
+ action: 'onChange',
+ control: { type: null },
+ description: 'Function called with the selected value changes.',
+ },
+ },
+};
+
+export default meta;
+
+export const Default = {
+ render: function Template( { onChange, ...args } ) {
+ const [ value, setValue ] = useState( args.value );
+
+ return (
+ {
+ setValue( ...changeArgs );
+ onChange( ...changeArgs );
+ } }
+ />
+ );
+ },
+ args: {
+ value: 2,
+ },
+};
diff --git a/packages/block-editor/src/components/block-info-slot-fill/index.js b/packages/block-editor/src/components/block-info-slot-fill/index.js
deleted file mode 100644
index 8c9503313d754..0000000000000
--- a/packages/block-editor/src/components/block-info-slot-fill/index.js
+++ /dev/null
@@ -1,27 +0,0 @@
-/**
- * WordPress dependencies
- */
-import { privateApis as componentsPrivateApis } from '@wordpress/components';
-
-/**
- * Internal dependencies
- */
-import { unlock } from '../../lock-unlock';
-import {
- useBlockEditContext,
- mayDisplayControlsKey,
-} from '../block-edit/context';
-
-const { createPrivateSlotFill } = unlock( componentsPrivateApis );
-const { Fill, Slot } = createPrivateSlotFill( 'BlockInformation' );
-
-const BlockInfo = ( props ) => {
- const context = useBlockEditContext();
- if ( ! context[ mayDisplayControlsKey ] ) {
- return null;
- }
- return ;
-};
-BlockInfo.Slot = ( props ) => ;
-
-export default BlockInfo;
diff --git a/packages/block-editor/src/components/block-inspector/index.js b/packages/block-editor/src/components/block-inspector/index.js
index 475d4f6a4b8c2..450a370b5c212 100644
--- a/packages/block-editor/src/components/block-inspector/index.js
+++ b/packages/block-editor/src/components/block-inspector/index.js
@@ -26,7 +26,6 @@ import useInspectorControlsTabs from '../inspector-controls-tabs/use-inspector-c
import AdvancedControls from '../inspector-controls-tabs/advanced-controls-panel';
import PositionControls from '../inspector-controls-tabs/position-controls-panel';
import useBlockInspectorAnimationSettings from './useBlockInspectorAnimationSettings';
-import BlockInfo from '../block-info-slot-fill';
import BlockQuickNavigation from '../block-quick-navigation';
import { useBorderPanelLabel } from '../../hooks/border';
@@ -253,7 +252,6 @@ const BlockInspectorSingleBlock = ( {
className={ blockInformation.isSynced && 'is-synced' }
/>
-
{ showTabs && (
removeBlock( clientId ),
- [ clientId, removeBlock ]
- );
const parentLayout = useLayout() || {};
@@ -537,6 +527,9 @@ const applyWithDispatch = withDispatch( ( dispatch, ownProps, registry ) => {
initialPosition
);
},
+ onRemove() {
+ removeBlock( ownProps.clientId );
+ },
toggleSelection( selectionEnabled ) {
toggleSelection( selectionEnabled );
},
@@ -797,6 +790,7 @@ function BlockListBlockProvider( props ) {
mayDisplayParentControls,
originalBlockClientId,
themeSupportsLayout,
+ canMove,
};
// Here we separate between the props passed to BlockListBlock and any other
diff --git a/packages/block-editor/src/components/block-list/content.scss b/packages/block-editor/src/components/block-list/content.scss
index 3d3b8517ca09c..cd517fced833e 100644
--- a/packages/block-editor/src/components/block-list/content.scss
+++ b/packages/block-editor/src/components/block-list/content.scss
@@ -427,3 +427,9 @@ _::-webkit-full-page-media, _:future, :root [data-has-multi-selection="true"] .b
// Additional -1px is required to avoid sub pixel rounding errors allowing background to show.
margin: 0 calc(-1 * var(--wp--style--root--padding-right) - 1px) 0 calc(-1 * var(--wp--style--root--padding-left) - 1px) !important;
}
+
+// This only works in Firefox, Chrome and Safari don't accept a custom cursor
+// during drag.
+.is-dragging {
+ cursor: grabbing;
+}
diff --git a/packages/block-editor/src/components/block-list/use-block-props/index.js b/packages/block-editor/src/components/block-list/use-block-props/index.js
index 25b9a21f0d286..14cda82fe7cd2 100644
--- a/packages/block-editor/src/components/block-list/use-block-props/index.js
+++ b/packages/block-editor/src/components/block-list/use-block-props/index.js
@@ -29,7 +29,8 @@ import { useBlockRefProvider } from './use-block-refs';
import { useIntersectionObserver } from './use-intersection-observer';
import { useScrollIntoView } from './use-scroll-into-view';
import { useFlashEditableBlocks } from '../../use-flash-editable-blocks';
-import { canBindBlock } from '../../../hooks/use-bindings-attributes';
+import { canBindBlock } from '../../../utils/block-bindings';
+import { useFirefoxDraggableCompatibility } from './use-firefox-draggable-compatibility';
/**
* This hook is used to lightly mark an element as a block element. The element
@@ -100,11 +101,13 @@ export function useBlockProps( props = {}, { __unstableIsHtml } = {} ) {
isTemporarilyEditingAsBlocks,
defaultClassName,
isSectionBlock,
+ canMove,
} = useContext( PrivateBlockContext );
// translators: %s: Type of block (i.e. Text, Image etc)
const blockLabel = sprintf( __( 'Block: %s' ), blockTitle );
const htmlSuffix = mode === 'html' && ! __unstableIsHtml ? '-visual' : '';
+ const ffDragRef = useFirefoxDraggableCompatibility();
const mergedRefs = useMergeRefs( [
props.ref,
useFocusFirstElement( { clientId, initialPosition } ),
@@ -120,6 +123,7 @@ export function useBlockProps( props = {}, { __unstableIsHtml } = {} ) {
isEnabled: isSectionBlock,
} ),
useScrollIntoView( { isSelected } ),
+ canMove ? ffDragRef : undefined,
] );
const blockEditContext = useBlockEditContext();
@@ -152,6 +156,7 @@ export function useBlockProps( props = {}, { __unstableIsHtml } = {} ) {
return {
tabIndex: blockEditingMode === 'disabled' ? -1 : 0,
+ draggable: canMove && ! hasChildSelected ? true : undefined,
...wrapperProps,
...props,
ref: mergedRefs,
diff --git a/packages/block-editor/src/components/block-list/use-block-props/use-firefox-draggable-compatibility.js b/packages/block-editor/src/components/block-list/use-block-props/use-firefox-draggable-compatibility.js
new file mode 100644
index 0000000000000..a53983b95954a
--- /dev/null
+++ b/packages/block-editor/src/components/block-list/use-block-props/use-firefox-draggable-compatibility.js
@@ -0,0 +1,83 @@
+/**
+ * WordPress dependencies
+ */
+import { useRefEffect } from '@wordpress/compose';
+
+const nodesByDocument = new Map();
+
+function add( doc, node ) {
+ let set = nodesByDocument.get( doc );
+ if ( ! set ) {
+ set = new Set();
+ nodesByDocument.set( doc, set );
+ doc.addEventListener( 'pointerdown', down );
+ }
+ set.add( node );
+}
+
+function remove( doc, node ) {
+ const set = nodesByDocument.get( doc );
+ if ( set ) {
+ set.delete( node );
+ restore( node );
+ if ( set.size === 0 ) {
+ nodesByDocument.delete( doc );
+ doc.removeEventListener( 'pointerdown', down );
+ }
+ }
+}
+
+function restore( node ) {
+ const prevDraggable = node.getAttribute( 'data-draggable' );
+ if ( prevDraggable ) {
+ node.removeAttribute( 'data-draggable' );
+ // Only restore if `draggable` is still removed. It could have been
+ // changed by React in the meantime.
+ if ( prevDraggable === 'true' && ! node.getAttribute( 'draggable' ) ) {
+ node.setAttribute( 'draggable', 'true' );
+ }
+ }
+}
+
+function down( event ) {
+ const { target } = event;
+ const { ownerDocument, isContentEditable } = target;
+ const nodes = nodesByDocument.get( ownerDocument );
+
+ if ( isContentEditable ) {
+ // Whenever an editable element is clicked, check which draggable
+ // blocks contain this element, and temporarily disable draggability.
+ for ( const node of nodes ) {
+ if (
+ node.getAttribute( 'draggable' ) === 'true' &&
+ node.contains( target )
+ ) {
+ node.removeAttribute( 'draggable' );
+ node.setAttribute( 'data-draggable', 'true' );
+ }
+ }
+ } else {
+ // Whenever a non-editable element is clicked, re-enable draggability
+ // for any blocks that were previously disabled.
+ for ( const node of nodes ) {
+ restore( node );
+ }
+ }
+}
+
+/**
+ * In Firefox, the `draggable` and `contenteditable` attributes don't play well
+ * together. When `contenteditable` is within a `draggable` element, selection
+ * doesn't get set in the right place. The only solution is to temporarily
+ * remove the `draggable` attribute clicking inside `contenteditable` elements.
+ *
+ * @return {Function} Cleanup function.
+ */
+export function useFirefoxDraggableCompatibility() {
+ return useRefEffect( ( node ) => {
+ add( node.ownerDocument, node );
+ return () => {
+ remove( node.ownerDocument, node );
+ };
+ }, [] );
+}
diff --git a/packages/block-editor/src/components/block-list/use-block-props/use-selected-block-event-handlers.js b/packages/block-editor/src/components/block-list/use-block-props/use-selected-block-event-handlers.js
index 68f8a671adbe9..0a13ce6700b8e 100644
--- a/packages/block-editor/src/components/block-list/use-block-props/use-selected-block-event-handlers.js
+++ b/packages/block-editor/src/components/block-list/use-block-props/use-selected-block-event-handlers.js
@@ -5,12 +5,15 @@ import { isTextField } from '@wordpress/dom';
import { ENTER, BACKSPACE, DELETE } from '@wordpress/keycodes';
import { useSelect, useDispatch } from '@wordpress/data';
import { useRefEffect } from '@wordpress/compose';
+import { createRoot } from '@wordpress/element';
+import { store as blocksStore } from '@wordpress/blocks';
/**
* Internal dependencies
*/
import { store as blockEditorStore } from '../../../store';
import { unlock } from '../../../lock-unlock';
+import BlockDraggableChip from '../../../components/block-draggable/draggable-chip';
/**
* Adds block behaviour:
@@ -21,12 +24,16 @@ import { unlock } from '../../../lock-unlock';
* @param {string} clientId Block client ID.
*/
export function useEventHandlers( { clientId, isSelected } ) {
- const { getBlockRootClientId, getBlockIndex, isZoomOut } = unlock(
- useSelect( blockEditorStore )
- );
- const { insertAfterBlock, removeBlock, resetZoomLevel } = unlock(
- useDispatch( blockEditorStore )
- );
+ const { getBlockType } = useSelect( blocksStore );
+ const { getBlockRootClientId, isZoomOut, hasMultiSelection, getBlockName } =
+ unlock( useSelect( blockEditorStore ) );
+ const {
+ insertAfterBlock,
+ removeBlock,
+ resetZoomLevel,
+ startDraggingBlocks,
+ stopDraggingBlocks,
+ } = unlock( useDispatch( blockEditorStore ) );
return useRefEffect(
( node ) => {
@@ -76,7 +83,102 @@ export function useEventHandlers( { clientId, isSelected } ) {
* @param {DragEvent} event Drag event.
*/
function onDragStart( event ) {
- event.preventDefault();
+ if (
+ node !== event.target ||
+ node.isContentEditable ||
+ node.ownerDocument.activeElement !== node ||
+ hasMultiSelection()
+ ) {
+ event.preventDefault();
+ return;
+ }
+ const data = JSON.stringify( {
+ type: 'block',
+ srcClientIds: [ clientId ],
+ srcRootClientId: getBlockRootClientId( clientId ),
+ } );
+ event.dataTransfer.effectAllowed = 'move'; // remove "+" cursor
+ event.dataTransfer.clearData();
+ event.dataTransfer.setData( 'wp-blocks', data );
+ const { ownerDocument } = node;
+ const { defaultView } = ownerDocument;
+ const selection = defaultView.getSelection();
+ selection.removeAllRanges();
+
+ const domNode = document.createElement( 'div' );
+ const root = createRoot( domNode );
+ root.render(
+
+ );
+ document.body.appendChild( domNode );
+ domNode.style.position = 'absolute';
+ domNode.style.top = '0';
+ domNode.style.left = '0';
+ domNode.style.zIndex = '1000';
+ domNode.style.pointerEvents = 'none';
+
+ // Setting the drag chip as the drag image actually works, but
+ // the behaviour is slightly different in every browser. In
+ // Safari, it animates, in Firefox it's slightly transparent...
+ // So we set a fake drag image and have to reposition it
+ // ourselves.
+ const dragElement = ownerDocument.createElement( 'div' );
+ // Chrome will show a globe icon if the drag element does not
+ // have dimensions.
+ dragElement.style.width = '1px';
+ dragElement.style.height = '1px';
+ dragElement.style.position = 'fixed';
+ dragElement.style.visibility = 'hidden';
+ ownerDocument.body.appendChild( dragElement );
+ event.dataTransfer.setDragImage( dragElement, 0, 0 );
+
+ let offset = { x: 0, y: 0 };
+
+ if ( document !== ownerDocument ) {
+ const frame = defaultView.frameElement;
+ if ( frame ) {
+ const rect = frame.getBoundingClientRect();
+ offset = { x: rect.left, y: rect.top };
+ }
+ }
+
+ // chip handle offset
+ offset.x -= 58;
+
+ function over( e ) {
+ domNode.style.transform = `translate( ${
+ e.clientX + offset.x
+ }px, ${ e.clientY + offset.y }px )`;
+ }
+
+ over( event );
+
+ function end() {
+ ownerDocument.removeEventListener( 'dragover', over );
+ ownerDocument.removeEventListener( 'dragend', end );
+ domNode.remove();
+ dragElement.remove();
+ stopDraggingBlocks();
+ document.body.classList.remove(
+ 'is-dragging-components-draggable'
+ );
+ ownerDocument.documentElement.classList.remove(
+ 'is-dragging'
+ );
+ }
+
+ ownerDocument.addEventListener( 'dragover', over );
+ ownerDocument.addEventListener( 'dragend', end );
+ ownerDocument.addEventListener( 'drop', end );
+
+ startDraggingBlocks( [ clientId ] );
+ // Important because it hides the block toolbar.
+ document.body.classList.add(
+ 'is-dragging-components-draggable'
+ );
+ ownerDocument.documentElement.classList.add( 'is-dragging' );
}
node.addEventListener( 'keydown', onKeyDown );
@@ -91,11 +193,13 @@ export function useEventHandlers( { clientId, isSelected } ) {
clientId,
isSelected,
getBlockRootClientId,
- getBlockIndex,
insertAfterBlock,
removeBlock,
isZoomOut,
resetZoomLevel,
+ hasMultiSelection,
+ startDraggingBlocks,
+ stopDraggingBlocks,
]
);
}
diff --git a/packages/block-editor/src/components/block-list/zoom-out-separator.js b/packages/block-editor/src/components/block-list/zoom-out-separator.js
index f2e6d050141fb..86191c1e4ce32 100644
--- a/packages/block-editor/src/components/block-list/zoom-out-separator.js
+++ b/packages/block-editor/src/components/block-list/zoom-out-separator.js
@@ -33,6 +33,7 @@ export function ZoomOutSeparator( {
insertionPoint,
blockInsertionPointVisible,
blockInsertionPoint,
+ blocksBeingDragged,
} = useSelect( ( select ) => {
const {
getInsertionPoint,
@@ -40,6 +41,7 @@ export function ZoomOutSeparator( {
getSectionRootClientId,
isBlockInsertionPointVisible,
getBlockInsertionPoint,
+ getDraggedBlockClientIds,
} = unlock( select( blockEditorStore ) );
const root = getSectionRootClientId();
@@ -51,6 +53,7 @@ export function ZoomOutSeparator( {
insertionPoint: getInsertionPoint(),
blockInsertionPoint: getBlockInsertionPoint(),
blockInsertionPointVisible: isBlockInsertionPointVisible(),
+ blocksBeingDragged: getDraggedBlockClientIds(),
};
}, [] );
@@ -78,6 +81,7 @@ export function ZoomOutSeparator( {
insertionPoint &&
insertionPoint.hasOwnProperty( 'index' ) &&
clientId === sectionClientIds[ insertionPoint.index - 1 ];
+
// We want to show the zoom out separator in either of these conditions:
// 1. If the inserter has an insertion index set
// 2. We are dragging a pattern over an insertion point
@@ -97,6 +101,32 @@ export function ZoomOutSeparator( {
sectionClientIds[ blockInsertionPoint.index - 1 ] );
}
+ const blockBeingDraggedClientId = blocksBeingDragged[ 0 ];
+
+ const isCurrentBlockBeingDragged = blocksBeingDragged.includes( clientId );
+
+ const blockBeingDraggedIndex = sectionClientIds.indexOf(
+ blockBeingDraggedClientId
+ );
+ const blockBeingDraggedPreviousSiblingClientId =
+ blockBeingDraggedIndex > 0
+ ? sectionClientIds[ blockBeingDraggedIndex - 1 ]
+ : null;
+
+ const isCurrentBlockPreviousSiblingOfBlockBeingDragged =
+ blockBeingDraggedPreviousSiblingClientId === clientId;
+
+ // The separators are visually top/bottom of the block, but in actual fact
+ // the "top" separator is the "bottom" separator of the previous block.
+ // Therefore, this logic hides the separator if the current block is being dragged
+ // or if the current block is the previous sibling of the block being dragged.
+ if (
+ isCurrentBlockBeingDragged ||
+ isCurrentBlockPreviousSiblingOfBlockBeingDragged
+ ) {
+ isVisible = false;
+ }
+
return (
{ isVisible && (
diff --git a/packages/block-editor/src/components/block-lock/modal.js b/packages/block-editor/src/components/block-lock/modal.js
index 7d09f7b63f8cd..df267e97165e3 100644
--- a/packages/block-editor/src/components/block-lock/modal.js
+++ b/packages/block-editor/src/components/block-lock/modal.js
@@ -24,7 +24,7 @@ import useBlockDisplayInformation from '../use-block-display-information';
import { store as blockEditorStore } from '../../store';
// Entity based blocks which allow edit locking
-const ALLOWS_EDIT_LOCKING = [ 'core/block', 'core/navigation' ];
+const ALLOWS_EDIT_LOCKING = [ 'core/navigation' ];
function getTemplateLockValue( lock ) {
// Prevents all operations.
@@ -99,9 +99,7 @@ export default function BlockLockModal( { clientId, onClose } ) {
>
- { __(
- 'Choose specific attributes to restrict or lock all available options.'
- ) }
+ { __( 'Select the features you want to lock' ) }
{ /*
* Disable reason: The `list` ARIA role is redundant but
@@ -137,7 +135,7 @@ export default function BlockLockModal( { clientId, onClose } ) {
setLock( ( prevLock ) => ( {
@@ -159,7 +157,7 @@ export default function BlockLockModal( { clientId, onClose } ) {
setLock( ( prevLock ) => ( {
@@ -178,7 +176,7 @@ export default function BlockLockModal( { clientId, onClose } ) {
setLock( ( prevLock ) => ( {
diff --git a/packages/block-editor/src/components/block-manager/category.js b/packages/block-editor/src/components/block-manager/category.js
new file mode 100644
index 0000000000000..79d5896b4502e
--- /dev/null
+++ b/packages/block-editor/src/components/block-manager/category.js
@@ -0,0 +1,102 @@
+/**
+ * WordPress dependencies
+ */
+import { useCallback } from '@wordpress/element';
+import { useInstanceId } from '@wordpress/compose';
+import { CheckboxControl } from '@wordpress/components';
+
+/**
+ * Internal dependencies
+ */
+import BlockTypesChecklist from './checklist';
+
+function BlockManagerCategory( {
+ title,
+ blockTypes,
+ selectedBlockTypes,
+ onChange,
+} ) {
+ const instanceId = useInstanceId( BlockManagerCategory );
+
+ const toggleVisible = useCallback(
+ ( blockType, nextIsChecked ) => {
+ if ( nextIsChecked ) {
+ onChange( [ ...selectedBlockTypes, blockType ] );
+ } else {
+ onChange(
+ selectedBlockTypes.filter(
+ ( { name } ) => name !== blockType.name
+ )
+ );
+ }
+ },
+ [ selectedBlockTypes, onChange ]
+ );
+
+ const toggleAllVisible = useCallback(
+ ( nextIsChecked ) => {
+ if ( nextIsChecked ) {
+ onChange( [
+ ...selectedBlockTypes,
+ ...blockTypes.filter(
+ ( blockType ) =>
+ ! selectedBlockTypes.find(
+ ( { name } ) => name === blockType.name
+ )
+ ),
+ ] );
+ } else {
+ onChange(
+ selectedBlockTypes.filter(
+ ( selectedBlockType ) =>
+ ! blockTypes.find(
+ ( { name } ) => name === selectedBlockType.name
+ )
+ )
+ );
+ }
+ },
+ [ blockTypes, selectedBlockTypes, onChange ]
+ );
+
+ if ( ! blockTypes.length ) {
+ return null;
+ }
+
+ const checkedBlockNames = blockTypes
+ .map( ( { name } ) => name )
+ .filter( ( type ) =>
+ ( selectedBlockTypes ?? [] ).some(
+ ( selectedBlockType ) => selectedBlockType.name === type
+ )
+ );
+
+ const titleId = 'block-editor-block-manager__category-title-' + instanceId;
+
+ const isAllChecked = checkedBlockNames.length === blockTypes.length;
+ const isIndeterminate = ! isAllChecked && checkedBlockNames.length > 0;
+
+ return (
+
+ { title } }
+ />
+
+
+ );
+}
+
+export default BlockManagerCategory;
diff --git a/packages/editor/src/components/block-manager/checklist.js b/packages/block-editor/src/components/block-manager/checklist.js
similarity index 70%
rename from packages/editor/src/components/block-manager/checklist.js
rename to packages/block-editor/src/components/block-manager/checklist.js
index 01bd06abdeba8..d5456a14355ef 100644
--- a/packages/editor/src/components/block-manager/checklist.js
+++ b/packages/block-editor/src/components/block-manager/checklist.js
@@ -1,23 +1,27 @@
/**
* WordPress dependencies
*/
-import { BlockIcon } from '@wordpress/block-editor';
import { CheckboxControl } from '@wordpress/components';
+/**
+ * Internal dependencies
+ */
+import BlockIcon from '../block-icon';
+
function BlockTypesChecklist( { blockTypes, value, onItemChange } ) {
return (
-
+
{ blockTypes.map( ( blockType ) => (
- onItemChange( blockType.name, ...args )
+ onItemChange( blockType, ...args )
}
/>
diff --git a/packages/editor/src/components/block-manager/index.js b/packages/block-editor/src/components/block-manager/index.js
similarity index 54%
rename from packages/editor/src/components/block-manager/index.js
rename to packages/block-editor/src/components/block-manager/index.js
index 4a1145839976f..30d10e67040c7 100644
--- a/packages/editor/src/components/block-manager/index.js
+++ b/packages/block-editor/src/components/block-manager/index.js
@@ -2,69 +2,49 @@
* WordPress dependencies
*/
import { store as blocksStore } from '@wordpress/blocks';
-import { useDispatch, useSelect } from '@wordpress/data';
+import { useSelect } from '@wordpress/data';
import { SearchControl, Button } from '@wordpress/components';
import { __, _n, sprintf } from '@wordpress/i18n';
import { useEffect, useState } from '@wordpress/element';
import { useDebounce } from '@wordpress/compose';
import { speak } from '@wordpress/a11y';
-import { store as preferencesStore } from '@wordpress/preferences';
/**
* Internal dependencies
*/
-import { unlock } from '../../lock-unlock';
-import { store as editorStore } from '../../store';
import BlockManagerCategory from './category';
-export default function BlockManager() {
+/**
+ * Provides a list of blocks with checkboxes.
+ *
+ * @param {Object} props Props.
+ * @param {Array} props.blockTypes An array of blocks.
+ * @param {Array} props.selectedBlockTypes An array of selected blocks.
+ * @param {Function} props.onChange Function to be called when the selected blocks change.
+ */
+export default function BlockManager( {
+ blockTypes,
+ selectedBlockTypes,
+ onChange,
+} ) {
const debouncedSpeak = useDebounce( speak, 500 );
const [ search, setSearch ] = useState( '' );
- const { showBlockTypes } = unlock( useDispatch( editorStore ) );
-
- const {
- blockTypes,
- categories,
- hasBlockSupport,
- isMatchingSearchTerm,
- numberOfHiddenBlocks,
- } = useSelect( ( select ) => {
- // Some hidden blocks become unregistered
- // by removing for instance the plugin that registered them, yet
- // they're still remain as hidden by the user's action.
- // We consider "hidden", blocks which were hidden and
- // are still registered.
- const _blockTypes = select( blocksStore ).getBlockTypes();
- const hiddenBlockTypes = (
- select( preferencesStore ).get( 'core', 'hiddenBlockTypes' ) ?? []
- ).filter( ( hiddenBlock ) => {
- return _blockTypes.some(
- ( registeredBlock ) => registeredBlock.name === hiddenBlock
- );
- } );
-
+ const { categories, isMatchingSearchTerm } = useSelect( ( select ) => {
return {
- blockTypes: _blockTypes,
categories: select( blocksStore ).getCategories(),
- hasBlockSupport: select( blocksStore ).hasBlockSupport,
isMatchingSearchTerm: select( blocksStore ).isMatchingSearchTerm,
- numberOfHiddenBlocks:
- Array.isArray( hiddenBlockTypes ) && hiddenBlockTypes.length,
};
}, [] );
- function enableAllBlockTypes( newBlockTypes ) {
- const blockNames = newBlockTypes.map( ( { name } ) => name );
- showBlockTypes( blockNames );
+ function enableAllBlockTypes() {
+ onChange( blockTypes );
}
- const filteredBlockTypes = blockTypes.filter(
- ( blockType ) =>
- hasBlockSupport( blockType, 'inserter', true ) &&
- ( ! search || isMatchingSearchTerm( blockType, search ) ) &&
- ( ! blockType.parent ||
- blockType.parent.includes( 'core/post-content' ) )
- );
+ const filteredBlockTypes = blockTypes.filter( ( blockType ) => {
+ return ! search || isMatchingSearchTerm( blockType, search );
+ } );
+
+ const numberOfHiddenBlocks = blockTypes.length - selectedBlockTypes.length;
// Announce search results on change
useEffect( () => {
@@ -81,9 +61,9 @@ export default function BlockManager() {
}, [ filteredBlockTypes?.length, search, debouncedSpeak ] );
return (
-
+
{ !! numberOfHiddenBlocks && (
-
+
{ sprintf(
/* translators: %d: number of blocks. */
_n(
@@ -96,9 +76,7 @@ export default function BlockManager() {
- enableAllBlockTypes( filteredBlockTypes )
- }
+ onClick={ enableAllBlockTypes }
>
{ __( 'Reset' ) }
@@ -110,16 +88,16 @@ export default function BlockManager() {
placeholder={ __( 'Search for a block' ) }
value={ search }
onChange={ ( nextSearch ) => setSearch( nextSearch ) }
- className="editor-block-manager__search"
+ className="block-editor-block-manager__search"
/>
{ filteredBlockTypes.length === 0 && (
-
+
{ __( 'No blocks found.' ) }
) }
@@ -131,6 +109,8 @@ export default function BlockManager() {
( blockType ) =>
blockType.category === category.slug
) }
+ selectedBlockTypes={ selectedBlockTypes }
+ onChange={ onChange }
/>
) ) }
! category
) }
+ selectedBlockTypes={ selectedBlockTypes }
+ onChange={ onChange }
/>
diff --git a/packages/editor/src/components/block-manager/style.scss b/packages/block-editor/src/components/block-manager/style.scss
similarity index 65%
rename from packages/editor/src/components/block-manager/style.scss
rename to packages/block-editor/src/components/block-manager/style.scss
index 411ee9faf34f7..d72c682dcbb5d 100644
--- a/packages/editor/src/components/block-manager/style.scss
+++ b/packages/block-editor/src/components/block-manager/style.scss
@@ -1,14 +1,14 @@
-.editor-block-manager__no-results {
+.block-editor-block-manager__no-results {
font-style: italic;
padding: $grid-unit-30 0;
text-align: center;
}
-.editor-block-manager__search {
+.block-editor-block-manager__search {
margin: $grid-unit-20 0;
}
-.editor-block-manager__disabled-blocks-count {
+.block-editor-block-manager__disabled-blocks-count {
border: $border-width solid $gray-300;
border-width: $border-width 0;
// Cover up horizontal areas off the sides of the box rectangle
@@ -19,10 +19,10 @@
position: sticky;
// When sticking, tuck the top border beneath the modal header border
top: ($grid-unit-05 + 1) * -1;
- z-index: z-index(".editor-block-manager__disabled-blocks-count");
+ z-index: z-index(".block-editor-block-manager__disabled-blocks-count");
// Stick the category titles to the bottom
- ~ .editor-block-manager__results .editor-block-manager__category-title {
+ ~ .block-editor-block-manager__results .block-editor-block-manager__category-title {
top: $grid-unit-40 - 1;
}
.is-link {
@@ -30,32 +30,32 @@
}
}
-.editor-block-manager__category {
+.block-editor-block-manager__category {
margin: 0 0 $grid-unit-30 0;
}
-.editor-block-manager__category-title {
+.block-editor-block-manager__category-title {
position: sticky;
top: - $grid-unit-05; // Offsets the top padding on the modal content container
padding: $grid-unit-20 0;
background-color: $white;
- z-index: z-index(".editor-block-manager__category-title");
+ z-index: z-index(".block-editor-block-manager__category-title");
.components-checkbox-control__label {
font-weight: 600;
}
}
-.editor-block-manager__checklist {
+.block-editor-block-manager__checklist {
margin-top: 0;
}
-.editor-block-manager__category-title,
-.editor-block-manager__checklist-item {
+.block-editor-block-manager__category-title,
+.block-editor-block-manager__checklist-item {
border-bottom: 1px solid $gray-300;
}
-.editor-block-manager__checklist-item {
+.block-editor-block-manager__checklist-item {
display: flex;
justify-content: space-between;
align-items: center;
@@ -72,11 +72,11 @@
}
}
-.editor-block-manager__results {
+.block-editor-block-manager__results {
border-top: $border-width solid $gray-300;
}
// Remove the top border from results when adjacent to the disabled block count
-.editor-block-manager__disabled-blocks-count + .editor-block-manager__results {
+.block-editor-block-manager__disabled-blocks-count + .block-editor-block-manager__results {
border-top-width: 0;
}
diff --git a/packages/block-editor/src/components/block-mover/README.md b/packages/block-editor/src/components/block-mover/README.md
index 38520072b4ac8..b781de773ef9f 100644
--- a/packages/block-editor/src/components/block-mover/README.md
+++ b/packages/block-editor/src/components/block-mover/README.md
@@ -1,12 +1,10 @@
-# Block mover
+# BlockMover
-Block movers allow moving blocks inside the editor using up and down buttons.
+BlockMover component allows moving blocks inside the editor using up and down buttons.

-## Development guidelines
-
-### Usage
+## Usage
Shows the block mover buttons in the block toolbar.
@@ -15,13 +13,22 @@ import { BlockMover } from '@wordpress/block-editor';
const MyMover = () =>
;
```
-### Props
+## Props
-#### clientIds
+### clientIds
-Blocks IDs
+The IDs of the blocks to move.
- Type: `Array`
+- Required: Yes
+
+### hideDragHandle
+
+If this property is true, the drag handle is hidden.
+
+- Type: `boolean`
+- Required: No
+- Default: `false`
## Related components
diff --git a/packages/block-editor/src/components/block-mover/stories/index.story.js b/packages/block-editor/src/components/block-mover/stories/index.story.js
index de30260563f91..de6d13c797b4d 100644
--- a/packages/block-editor/src/components/block-mover/stories/index.story.js
+++ b/packages/block-editor/src/components/block-mover/stories/index.story.js
@@ -14,6 +14,7 @@ import BlockMover from '../';
import { ExperimentalBlockEditorProvider } from '../../provider';
import { store as blockEditorStore } from '../../../store';
+// For the purpose of this story, we need to register the core blocks samples.
registerCoreBlocks();
const blocks = [
// vertical
@@ -30,81 +31,82 @@ const blocks = [
] ),
];
-function Provider( { children } ) {
- const wrapperStyle = { margin: '24px', position: 'relative' };
-
- return (
-
+/**
+ * BlockMover component allows moving blocks inside the editor using up and down buttons.
+ */
+const meta = {
+ title: 'BlockEditor/BlockMover',
+ component: BlockMover,
+ parameters: {
+ docs: { canvas: { sourceState: 'shown' } },
+ },
+ decorators: [
+ ( Story ) => (
- { children }
+
+
+
-
- );
-}
-
-function BlockMoverStory() {
- const { updateBlockListSettings } = useDispatch( blockEditorStore );
-
- useEffect( () => {
- /**
- * This shouldn't be needed but unfortunatley
- * the layout orientation is not declarative, we need
- * to render the blocks to update the block settings in the state.
- */
- updateBlockListSettings( blocks[ 1 ].clientId, {
- orientation: 'horizontal',
- } );
- }, [] );
-
- return (
-
-
The mover by default is vertical
-
-
-
-
-
- But it can also accommodate horizontal blocks.
-
-
-
-
+ ),
+ ],
+ argTypes: {
+ clientIds: {
+ control: {
+ type: 'none',
+ },
+ description: 'The client IDs of the blocks to move.',
+ },
+ hideDragHandle: {
+ control: {
+ type: 'boolean',
+ },
+ description: 'If this property is true, the drag handle is hidden.',
+ },
+ },
+};
+export default meta;
-
We can also hide the drag handle.
-
-
-
-
- );
-}
+export const Default = {
+ args: {
+ clientIds: [ blocks[ 0 ].innerBlocks[ 1 ].clientId ],
+ },
+};
-export default {
- title: 'BlockEditor/BlockMover',
+/**
+ * This story shows the block mover with horizontal orientation.
+ * It is necessary to render the blocks to update the block settings in the state.
+ */
+export const Horizontal = {
+ decorators: [
+ ( Story ) => {
+ const { updateBlockListSettings } = useDispatch( blockEditorStore );
+ useEffect( () => {
+ /**
+ * This shouldn't be needed but unfortunately
+ * the layout orientation is not declarative, we need
+ * to render the blocks to update the block settings in the state.
+ */
+ updateBlockListSettings( blocks[ 1 ].clientId, {
+ orientation: 'horizontal',
+ } );
+ }, [] );
+ return
;
+ },
+ ],
+ args: {
+ clientIds: [ blocks[ 1 ].innerBlocks[ 1 ].clientId ],
+ },
+ parameters: {
+ docs: { canvas: { sourceState: 'hidden' } },
+ },
};
-export const _default = () => {
- return (
-
-
-
- );
+/**
+ * You can hide the drag handle by `hideDragHandle` attribute.
+ */
+export const HideDragHandle = {
+ args: {
+ ...Default.args,
+ hideDragHandle: true,
+ },
};
diff --git a/packages/block-editor/src/components/block-mover/style.scss b/packages/block-editor/src/components/block-mover/style.scss
index c58ac9f19673f..7d23c0f1e5a98 100644
--- a/packages/block-editor/src/components/block-mover/style.scss
+++ b/packages/block-editor/src/components/block-mover/style.scss
@@ -71,6 +71,9 @@
// Specificity is necessary to override block toolbar button styles.
.components-button.block-editor-block-mover-button {
+ // Prevent the SVGs inside the button from overflowing the button.
+ overflow: hidden;
+
// Focus and toggle pseudo elements.
&::before {
content: "";
diff --git a/packages/block-editor/src/components/block-parent-selector/index.js b/packages/block-editor/src/components/block-parent-selector/index.js
index 9090de42f8b7d..84b5211089cd9 100644
--- a/packages/block-editor/src/components/block-parent-selector/index.js
+++ b/packages/block-editor/src/components/block-parent-selector/index.js
@@ -1,7 +1,6 @@
/**
* WordPress dependencies
*/
-import { getBlockType, store as blocksStore } from '@wordpress/blocks';
import { ToolbarButton } from '@wordpress/components';
import { useSelect, useDispatch } from '@wordpress/data';
import { __, sprintf } from '@wordpress/i18n';
@@ -24,31 +23,18 @@ import { unlock } from '../../lock-unlock';
*/
export default function BlockParentSelector() {
const { selectBlock } = useDispatch( blockEditorStore );
- const { parentClientId, isVisible } = useSelect( ( select ) => {
+ const { parentClientId } = useSelect( ( select ) => {
const {
- getBlockName,
getBlockParents,
getSelectedBlockClientId,
- getBlockEditingMode,
getParentSectionBlock,
} = unlock( select( blockEditorStore ) );
- const { hasBlockSupport } = select( blocksStore );
const selectedBlockClientId = getSelectedBlockClientId();
const parentSection = getParentSectionBlock( selectedBlockClientId );
const parents = getBlockParents( selectedBlockClientId );
const _parentClientId = parentSection ?? parents[ parents.length - 1 ];
- const parentBlockName = getBlockName( _parentClientId );
- const _parentBlockType = getBlockType( parentBlockName );
return {
parentClientId: _parentClientId,
- isVisible:
- _parentClientId &&
- getBlockEditingMode( _parentClientId ) !== 'disabled' &&
- hasBlockSupport(
- _parentBlockType,
- '__experimentalParentSelector',
- true
- ),
};
}, [] );
const blockInformation = useBlockDisplayInformation( parentClientId );
@@ -61,10 +47,6 @@ export default function BlockParentSelector() {
highlightParent: true,
} );
- if ( ! isVisible ) {
- return null;
- }
-
return (
@@ -192,6 +194,7 @@ function BlockPatternsList(
ref
) {
const [ activeCompositeId, setActiveCompositeId ] = useState( undefined );
+ const [ activePattern, setActivePattern ] = useState( null ); // State to track active pattern
useEffect( () => {
// Reset the active composite item whenever the available patterns change,
@@ -201,6 +204,11 @@ function BlockPatternsList(
setActiveCompositeId( firstCompositeItemId );
}, [ blockPatterns ] );
+ const handleClickPattern = ( pattern, blocks ) => {
+ setActivePattern( pattern.name );
+ onClickPattern( pattern, blocks );
+ };
+
return (
) ) }
{ pagingProps &&
}
diff --git a/packages/block-editor/src/components/block-patterns-list/stories/fixtures.js b/packages/block-editor/src/components/block-patterns-list/stories/fixtures.js
index 0fd895bbe1716..7825ad0d1391c 100644
--- a/packages/block-editor/src/components/block-patterns-list/stories/fixtures.js
+++ b/packages/block-editor/src/components/block-patterns-list/stories/fixtures.js
@@ -530,6 +530,7 @@ export default [
background: '#000000',
},
},
+ tagName: 'hr',
},
innerBlocks: [],
originalContent:
diff --git a/packages/block-editor/src/components/block-patterns-list/style.scss b/packages/block-editor/src/components/block-patterns-list/style.scss
index c46bb49b9a901..8b1b0b54c9b1a 100644
--- a/packages/block-editor/src/components/block-patterns-list/style.scss
+++ b/packages/block-editor/src/components/block-patterns-list/style.scss
@@ -44,19 +44,29 @@
outline: $border-width solid rgba($black, 0.1);
outline-offset: -$border-width;
border-radius: $radius-medium;
+
+ transition: outline 0.1s linear;
+ @include reduce-motion("transition");
}
}
- &:hover:not(:focus) .block-editor-block-preview__container::after {
+ // Selected
+ &.is-selected .block-editor-block-preview__container::after {
+ outline-color: $gray-900;
+ outline-width: var(--wp-admin-border-width-focus);
+ outline-offset: calc(-1 * var(--wp-admin-border-width-focus));
+ }
+
+ // Hover state
+ &:hover .block-editor-block-preview__container::after {
outline-color: rgba($black, 0.3);
}
- &:focus .block-editor-block-preview__container::after {
+ // Focused state
+ &[data-focus-visible] .block-editor-block-preview__container::after {
outline-color: var(--wp-admin-theme-color);
outline-width: var(--wp-admin-border-width-focus);
- outline-offset: calc((-1 * var(--wp-admin-border-width-focus)));
- transition: outline 0.1s linear;
- @include reduce-motion("transition");
+ outline-offset: calc(-1 * var(--wp-admin-border-width-focus));
}
.block-editor-patterns__pattern-details:not(:empty) {
@@ -68,6 +78,7 @@
.block-editor-patterns__pattern-icon-wrapper {
min-width: 24px;
height: 24px;
+
.block-editor-patterns__pattern-icon {
fill: var(--wp-block-synced-color);
}
diff --git a/packages/block-editor/src/components/block-popover/inbetween.js b/packages/block-editor/src/components/block-popover/inbetween.js
index 2ed9ee0bcb284..1d7c176673240 100644
--- a/packages/block-editor/src/components/block-popover/inbetween.js
+++ b/packages/block-editor/src/components/block-popover/inbetween.js
@@ -148,6 +148,10 @@ function BlockPopoverInbetween( {
? nextRect.left - previousRect.right
: 0;
}
+
+ // Avoid a negative width which happens when the next rect
+ // is on the next line.
+ width = Math.max( width, 0 );
}
return new window.DOMRect( left, top, width, height );
diff --git a/packages/block-editor/src/components/block-settings-menu-controls/index.js b/packages/block-editor/src/components/block-settings-menu-controls/index.js
index 4ebce4172e9b3..b0755be4c2629 100644
--- a/packages/block-editor/src/components/block-settings-menu-controls/index.js
+++ b/packages/block-editor/src/components/block-settings-menu-controls/index.js
@@ -55,7 +55,8 @@ const BlockSettingsMenuControlsSlot = ( { fillProps, clientIds = null } ) => {
const convertToGroupButtonProps =
useConvertToGroupButtonProps( selectedClientIds );
const { isGroupable, isUngroupable } = convertToGroupButtonProps;
- const showConvertToGroupButton = isGroupable || isUngroupable;
+ const showConvertToGroupButton =
+ ( isGroupable || isUngroupable ) && ! isContentOnly;
return (
) }
- { ! isContentOnly && (
-
- ) }
+
{ canDuplicate && (
>
) }
- <__unstableCommentIconFill.Slot
+
@@ -311,14 +311,16 @@ export function BlockSettingsDropdown( {
) }
-
+ { ! isContentOnly && (
+
+ ) }
{ typeof children === 'function'
? children( { onClose } )
: Children.map( ( child ) =>
diff --git a/packages/block-editor/src/components/block-settings-menu/index.js b/packages/block-editor/src/components/block-settings-menu/index.js
index 50e8abe09d018..1b96f30e13038 100644
--- a/packages/block-editor/src/components/block-settings-menu/index.js
+++ b/packages/block-editor/src/components/block-settings-menu/index.js
@@ -7,12 +7,12 @@ import { ToolbarGroup, ToolbarItem } from '@wordpress/components';
* Internal dependencies
*/
import BlockSettingsDropdown from './block-settings-dropdown';
-import __unstableCommentIconToolbarFill from '../../components/collab/block-comment-icon-toolbar-slot';
+import CommentIconToolbarSlotFill from '../../components/collab/block-comment-icon-toolbar-slot';
export function BlockSettingsMenu( { clientIds, ...props } ) {
return (
- <__unstableCommentIconToolbarFill.Slot />
+
{ ( toggleProps ) => (
diff --git a/packages/block-editor/src/components/block-switcher/index.js b/packages/block-editor/src/components/block-switcher/index.js
index 79f33bd30d753..285581578ead4 100644
--- a/packages/block-editor/src/components/block-switcher/index.js
+++ b/packages/block-editor/src/components/block-switcher/index.js
@@ -18,6 +18,7 @@ import {
} from '@wordpress/blocks';
import { useSelect, useDispatch } from '@wordpress/data';
import { copy } from '@wordpress/icons';
+import { store as preferencesStore } from '@wordpress/preferences';
/**
* Internal dependencies
@@ -185,21 +186,6 @@ function BlockSwitcherDropdownMenuContents( {
);
}
-const BlockIndicator = ( { icon, showTitle, blockTitle } ) => (
- <>
-
- { showTitle && blockTitle && (
-
- { blockTitle }
-
- ) }
- >
-);
-
export const BlockSwitcher = ( { clientIds } ) => {
const {
hasContentOnlyLocking,
@@ -272,6 +258,11 @@ export const BlockSwitcher = ( { clientIds } ) => {
clientId: clientIds?.[ 0 ],
maximumLength: 35,
} );
+ const showIconLabels = useSelect(
+ ( select ) =>
+ select( preferencesStore ).get( 'core', 'showIconLabels' ),
+ []
+ );
if ( invalidBlocks ) {
return null;
@@ -282,6 +273,11 @@ export const BlockSwitcher = ( { clientIds } ) => {
? blockTitle
: __( 'Multiple blocks selected' );
+ const blockIndicatorText =
+ ( isReusable || isTemplate ) && ! showIconLabels && blockTitle
+ ? blockTitle
+ : undefined;
+
const hideDropdown =
isDisabled ||
( ! hasBlockStyles && ! canRemove ) ||
@@ -295,12 +291,13 @@ export const BlockSwitcher = ( { clientIds } ) => {
className="block-editor-block-switcher__no-switcher-icon"
title={ blockSwitcherLabel }
icon={
-
}
+ text={ blockIndicatorText }
/>
);
@@ -329,12 +326,13 @@ export const BlockSwitcher = ( { clientIds } ) => {
className: 'block-editor-block-switcher__popover',
} }
icon={
-
}
+ text={ blockIndicatorText }
toggleProps={ {
description: blockSwitcherDescription,
...toggleProps,
diff --git a/packages/block-editor/src/components/block-switcher/style.scss b/packages/block-editor/src/components/block-switcher/style.scss
index 3dc2a7d591c92..62a7bebe95d27 100644
--- a/packages/block-editor/src/components/block-switcher/style.scss
+++ b/packages/block-editor/src/components/block-switcher/style.scss
@@ -26,15 +26,6 @@
}
}
-.block-editor-block-switcher__toggle-text {
- margin-left: $grid-unit-10;
-
- // Account for double label when show-text-buttons is set.
- .show-icon-labels & {
- display: none;
- }
-}
-
.components-button.block-editor-block-switcher__no-switcher-icon {
display: flex;
diff --git a/packages/block-editor/src/components/block-title/test/index.js b/packages/block-editor/src/components/block-title/test/index.js
index 8a4d3c2f52fd7..fc6af61bae9e7 100644
--- a/packages/block-editor/src/components/block-title/test/index.js
+++ b/packages/block-editor/src/components/block-title/test/index.js
@@ -31,7 +31,9 @@ const blockLabelMap = {
};
jest.mock( '@wordpress/blocks', () => {
+ const actualImplementation = jest.requireActual( '@wordpress/blocks' );
return {
+ ...actualImplementation,
isReusableBlock( { title } ) {
return title === 'Reusable Block';
},
diff --git a/packages/block-editor/src/components/block-toolbar/index.js b/packages/block-editor/src/components/block-toolbar/index.js
index b3bf7e94accb0..083d77a694a7b 100644
--- a/packages/block-editor/src/components/block-toolbar/index.js
+++ b/packages/block-editor/src/components/block-toolbar/index.js
@@ -36,6 +36,7 @@ import __unstableBlockNameContext from './block-name-context';
import NavigableToolbar from '../navigable-toolbar';
import { useHasBlockToolbar } from './use-has-block-toolbar';
import ChangeDesign from './change-design';
+import SwitchSectionStyle from './switch-section-style';
import { unlock } from '../../lock-unlock';
/**
@@ -72,6 +73,9 @@ export function PrivateBlockToolbar( {
showSlots,
showGroupButtons,
showLockButtons,
+ showSwitchSectionStyleButton,
+ hasFixedToolbar,
+ isNavigationMode,
} = useSelect( ( select ) => {
const {
getBlockName,
@@ -83,8 +87,10 @@ export function PrivateBlockToolbar( {
getBlockAttributes,
getBlockParentsByBlockName,
getTemplateLock,
+ getSettings,
getParentSectionBlock,
isZoomOut,
+ isNavigationMode: _isNavigationMode,
} = unlock( select( blockEditorStore ) );
const selectedBlockClientIds = getSelectedBlockClientIds();
const selectedBlockClientId = selectedBlockClientIds[ 0 ];
@@ -117,6 +123,9 @@ export function PrivateBlockToolbar( {
const _hasTemplateLock = selectedBlockClientIds.some(
( id ) => getTemplateLock( id ) === 'contentOnly'
);
+
+ const _isZoomOut = isZoomOut();
+
return {
blockClientId: selectedBlockClientId,
blockClientIds: selectedBlockClientIds,
@@ -125,8 +134,9 @@ export function PrivateBlockToolbar( {
shouldShowVisualToolbar: isValid && isVisual,
toolbarKey: `${ selectedBlockClientId }${ parentClientId }`,
showParentSelector:
- ! isZoomOut() &&
+ ! _isZoomOut &&
parentBlockType &&
+ editingMode !== 'contentOnly' &&
getBlockEditingMode( parentClientId ) !== 'disabled' &&
hasBlockSupport(
parentBlockType,
@@ -137,10 +147,13 @@ export function PrivateBlockToolbar( {
isUsingBindings: _isUsingBindings,
hasParentPattern: _hasParentPattern,
hasContentOnlyLocking: _hasTemplateLock,
- showShuffleButton: isZoomOut(),
- showSlots: ! isZoomOut(),
- showGroupButtons: ! isZoomOut(),
- showLockButtons: ! isZoomOut(),
+ showShuffleButton: _isZoomOut,
+ showSlots: ! _isZoomOut,
+ showGroupButtons: ! _isZoomOut,
+ showLockButtons: ! _isZoomOut,
+ showSwitchSectionStyleButton: _isZoomOut,
+ hasFixedToolbar: getSettings().hasFixedToolbar,
+ isNavigationMode: _isNavigationMode(),
};
}, [] );
@@ -167,6 +180,7 @@ export function PrivateBlockToolbar( {
// Shifts the toolbar to make room for the parent block selector.
const classes = clsx( 'block-editor-block-contextual-toolbar', {
'has-parent': showParentSelector,
+ 'is-inverted-toolbar': isNavigationMode && ! hasFixedToolbar,
} );
const innerClasses = clsx( 'block-editor-block-toolbar', {
@@ -222,6 +236,9 @@ export function PrivateBlockToolbar( {
{ showShuffleButton && (
) }
+ { showSwitchSectionStyleButton && (
+
+ ) }
{ shouldShowVisualToolbar && showSlots && (
<>
+
+
+
+);
+
+function SwitchSectionStyle( { clientId } ) {
+ const { stylesToRender, activeStyle, className } = useStylesForBlocks( {
+ clientId,
+ } );
+ const { updateBlockAttributes } = useDispatch( blockEditorStore );
+
+ // Get global styles data
+ const { merged: mergedConfig } = useContext( GlobalStylesContext );
+ const { globalSettings, globalStyles, blockName } = useSelect(
+ ( select ) => {
+ const settings = select( blockEditorStore ).getSettings();
+ return {
+ globalSettings: settings.__experimentalFeatures,
+ globalStyles: settings[ globalStylesDataKey ],
+ blockName: select( blockEditorStore ).getBlockName( clientId ),
+ };
+ },
+ [ clientId ]
+ );
+
+ // Get the background color for the active style
+ const activeStyleBackground = activeStyle?.name
+ ? getVariationStylesWithRefValues(
+ {
+ settings: mergedConfig?.settings ?? globalSettings,
+ styles: mergedConfig?.styles ?? globalStyles,
+ },
+ blockName,
+ activeStyle.name
+ )?.color?.background
+ : undefined;
+
+ if ( ! stylesToRender || stylesToRender.length === 0 ) {
+ return null;
+ }
+
+ const handleStyleSwitch = () => {
+ const currentIndex = stylesToRender.findIndex(
+ ( style ) => style.name === activeStyle.name
+ );
+
+ const nextIndex = ( currentIndex + 1 ) % stylesToRender.length;
+ const nextStyle = stylesToRender[ nextIndex ];
+
+ const styleClassName = replaceActiveStyle(
+ className,
+ activeStyle,
+ nextStyle
+ );
+
+ updateBlockAttributes( clientId, {
+ className: styleClassName,
+ } );
+ };
+
+ return (
+
+
+
+
+
+ );
+}
+
+export default SwitchSectionStyle;
diff --git a/packages/block-editor/src/components/block-tools/style.scss b/packages/block-editor/src/components/block-tools/style.scss
index b553d42668cf3..80fe4c420d1e1 100644
--- a/packages/block-editor/src/components/block-tools/style.scss
+++ b/packages/block-editor/src/components/block-tools/style.scss
@@ -139,6 +139,50 @@
border-right-color: $gray-900;
}
+ .is-inverted-toolbar {
+ background-color: $gray-900;
+ color: $gray-100;
+
+ &.block-editor-block-contextual-toolbar {
+ border-color: $gray-800;
+ }
+
+ button {
+ color: $gray-300;
+
+ &:hover {
+ color: $white;
+ }
+
+ &:focus::before {
+ box-shadow: inset 0 0 0 1px $gray-900, 0 0 0 var(--wp-admin-border-width-focus) var(--wp-admin-theme-color);
+ }
+
+ &:disabled,
+ &[aria-disabled="true"] {
+ color: $gray-700;
+ }
+ }
+
+ .block-editor-block-parent-selector .block-editor-block-parent-selector__button {
+ border-color: $gray-800;
+ background-color: $gray-900;
+ }
+
+ .block-editor-block-switcher__toggle {
+ color: $gray-100;
+ }
+
+ .components-toolbar-group,
+ .components-toolbar {
+ border-right-color: $gray-800 !important;
+ }
+
+ .is-pressed {
+ color: var(--wp-admin-theme-color);
+ }
+ }
+
// Hide the block toolbar if the insertion point is shown.
&.is-insertion-point-visible {
visibility: hidden;
diff --git a/packages/block-editor/src/components/block-vertical-alignment-control/test/__snapshots__/index.js.snap b/packages/block-editor/src/components/block-vertical-alignment-control/test/__snapshots__/index.js.snap
index e58d9a264310a..e8ad6cddbba56 100644
--- a/packages/block-editor/src/components/block-vertical-alignment-control/test/__snapshots__/index.js.snap
+++ b/packages/block-editor/src/components/block-vertical-alignment-control/test/__snapshots__/index.js.snap
@@ -42,7 +42,7 @@ exports[`BlockVerticalAlignmentUI should match snapshot when controls are visibl
@@ -64,7 +64,7 @@ exports[`BlockVerticalAlignmentUI should match snapshot when controls are visibl
@@ -86,7 +86,7 @@ exports[`BlockVerticalAlignmentUI should match snapshot when controls are visibl
diff --git a/packages/block-editor/src/components/child-layout-control/index.js b/packages/block-editor/src/components/child-layout-control/index.js
index 022acf2e1074a..20791d9751bcd 100644
--- a/packages/block-editor/src/components/child-layout-control/index.js
+++ b/packages/block-editor/src/components/child-layout-control/index.js
@@ -9,6 +9,7 @@ import {
__experimentalHStack as HStack,
__experimentalVStack as VStack,
__experimentalToolsPanelItem as ToolsPanelItem,
+ __experimentalUseCustomUnits as useCustomUnits,
Flex,
FlexItem,
} from '@wordpress/components';
@@ -21,6 +22,7 @@ import { useSelect, useDispatch } from '@wordpress/data';
*/
import { useGetNumberOfBlocksBeforeCell } from '../grid/use-get-number-of-blocks-before-cell';
import { store as blockEditorStore } from '../../store';
+import { useSettings } from '../use-settings';
function helpText( selfStretch, parentLayout ) {
const { orientation = 'horizontal' } = parentLayout;
@@ -98,6 +100,17 @@ function FlexControls( {
const hasFlexValue = () => !! selfStretch;
const flexResetLabel =
orientation === 'horizontal' ? __( 'Width' ) : __( 'Height' );
+ const [ availableUnits ] = useSettings( 'spacing.units' );
+ const units = useCustomUnits( {
+ availableUnits: availableUnits || [
+ '%',
+ 'px',
+ 'em',
+ 'rem',
+ 'vh',
+ 'vw',
+ ],
+ } );
const resetFlex = () => {
onChange( {
selfStretch: undefined,
@@ -167,6 +180,7 @@ function FlexControls( {
{ selfStretch === 'fixed' && (
{
onChange( {
selfStretch,
diff --git a/packages/block-editor/src/components/collab/block-comment-icon-slot.js b/packages/block-editor/src/components/collab/block-comment-icon-slot.js
index 600db904b2874..bcf8c5f5ff0c5 100644
--- a/packages/block-editor/src/components/collab/block-comment-icon-slot.js
+++ b/packages/block-editor/src/components/collab/block-comment-icon-slot.js
@@ -3,10 +3,6 @@
*/
import { createSlotFill } from '@wordpress/components';
-const { Fill: __unstableCommentIconFill, Slot } = createSlotFill(
- '__unstableCommentIconFill'
-);
+const CommentIconSlotFill = createSlotFill( Symbol( 'CommentIconSlotFill' ) );
-__unstableCommentIconFill.Slot = Slot;
-
-export default __unstableCommentIconFill;
+export default CommentIconSlotFill;
diff --git a/packages/block-editor/src/components/collab/block-comment-icon-toolbar-slot.js b/packages/block-editor/src/components/collab/block-comment-icon-toolbar-slot.js
index dd84b284f082a..056b9a2623262 100644
--- a/packages/block-editor/src/components/collab/block-comment-icon-toolbar-slot.js
+++ b/packages/block-editor/src/components/collab/block-comment-icon-toolbar-slot.js
@@ -3,10 +3,8 @@
*/
import { createSlotFill } from '@wordpress/components';
-const { Fill: __unstableCommentIconToolbarFill, Slot } = createSlotFill(
- '__unstableCommentIconToolbarFill'
+const CommentIconToolbarSlotFill = createSlotFill(
+ Symbol( 'CommentIconToolbarSlotFill' )
);
-__unstableCommentIconToolbarFill.Slot = Slot;
-
-export default __unstableCommentIconToolbarFill;
+export default CommentIconToolbarSlotFill;
diff --git a/packages/block-editor/src/components/color-palette/test/__snapshots__/control.js.snap b/packages/block-editor/src/components/color-palette/test/__snapshots__/control.js.snap
index 3c4cef664a310..14196a221304b 100644
--- a/packages/block-editor/src/components/color-palette/test/__snapshots__/control.js.snap
+++ b/packages/block-editor/src/components/color-palette/test/__snapshots__/control.js.snap
@@ -219,7 +219,7 @@ exports[`ColorPaletteControl matches the snapshot 1`] = `
Clear
diff --git a/packages/block-editor/src/components/color-palette/with-color-context.js b/packages/block-editor/src/components/color-palette/with-color-context.js
index 62b8c1bc4b618..38c90531edaac 100644
--- a/packages/block-editor/src/components/color-palette/with-color-context.js
+++ b/packages/block-editor/src/components/color-palette/with-color-context.js
@@ -10,14 +10,32 @@ import { useSettings } from '../use-settings';
export default createHigherOrderComponent( ( WrappedComponent ) => {
return ( props ) => {
- const [ colorsFeature, enableCustomColors ] = useSettings(
- 'color.palette',
- 'color.custom'
+ // Get the default colors, theme colors, and custom colors
+ const [
+ defaultColors,
+ themeColors,
+ customColors,
+ enableCustomColors,
+ enableDefaultColors,
+ ] = useSettings(
+ 'color.palette.default',
+ 'color.palette.theme',
+ 'color.palette.custom',
+ 'color.custom',
+ 'color.defaultPalette'
);
- const {
- colors = colorsFeature,
- disableCustomColors = ! enableCustomColors,
- } = props;
+
+ const _colors = enableDefaultColors
+ ? [
+ ...( themeColors || [] ),
+ ...( defaultColors || [] ),
+ ...( customColors || [] ),
+ ]
+ : [ ...( themeColors || [] ), ...( customColors || [] ) ];
+
+ const { colors = _colors, disableCustomColors = ! enableCustomColors } =
+ props;
+
const hasColorsToChoose =
( colors && colors.length > 0 ) || ! disableCustomColors;
return (
diff --git a/packages/block-editor/src/components/colors-gradients/style.scss b/packages/block-editor/src/components/colors-gradients/style.scss
index fc1b1a4d46903..222a5b239cf99 100644
--- a/packages/block-editor/src/components/colors-gradients/style.scss
+++ b/packages/block-editor/src/components/colors-gradients/style.scss
@@ -64,6 +64,7 @@ $swatch-gap: 12px;
.block-editor-tools-panel-color-gradient-settings__item {
padding: 0;
max-width: 100%;
+ position: relative;
// Border styles.
border-left: 1px solid $gray-300;
@@ -120,3 +121,23 @@ $swatch-gap: 12px;
flex-shrink: 0;
}
}
+
+.block-editor-panel-color-gradient-settings__reset {
+ position: absolute;
+ right: 0;
+ top: $grid-unit;
+ margin: auto $grid-unit auto;
+ opacity: 0;
+ transition: opacity 0.1s ease-in-out;
+ @include reduce-motion("transition");
+
+ &.block-editor-panel-color-gradient-settings__reset {
+ border-radius: $radius-small;
+ }
+
+ .block-editor-panel-color-gradient-settings__dropdown:hover + &,
+ &:focus,
+ &:hover {
+ opacity: 1;
+ }
+}
diff --git a/packages/block-editor/src/components/contrast-checker/index.native.js b/packages/block-editor/src/components/contrast-checker/index.native.js
index edd60473fcc36..c4f19857ccec7 100644
--- a/packages/block-editor/src/components/contrast-checker/index.native.js
+++ b/packages/block-editor/src/components/contrast-checker/index.native.js
@@ -13,7 +13,7 @@ import { speak } from '@wordpress/a11y';
import { __ } from '@wordpress/i18n';
import { useEffect } from '@wordpress/element';
import { usePreferredColorSchemeStyle } from '@wordpress/compose';
-import { Icon, warning } from '@wordpress/icons';
+import { Icon, cautionFilled } from '@wordpress/icons';
/**
* Internal dependencies
*/
@@ -52,7 +52,7 @@ function ContrastCheckerMessage( {
return (
-
+
{ msg }
);
diff --git a/packages/block-editor/src/components/date-format-picker/README.md b/packages/block-editor/src/components/date-format-picker/README.md
index e057bdc31a168..f6160cb90955b 100644
--- a/packages/block-editor/src/components/date-format-picker/README.md
+++ b/packages/block-editor/src/components/date-format-picker/README.md
@@ -1,17 +1,12 @@
# DateFormatPicker
-The `DateFormatPicker` component renders controls that let the user choose a
-_date format_. That is, how they want their dates to be formatted.
+The `DateFormatPicker` component renders controls that let the user choose a _date format_. That is, how they want their dates to be formatted.
-A user can pick _Default_ to use the default date format (usually set at the
-site level).
+A user can pick _Default_ to use the default date format (usually set at the site level).
-Otherwise, a user may choose a suggested date format or type in their own date
-format by selecting _Custom_.
+Otherwise, a user may choose a suggested date format or type in their own date format by selecting _Custom_.
-All date format strings should be in the format accepted by by the [`dateI18n`
-function in
-`@wordpress/date`](https://github.com/WordPress/gutenberg/tree/trunk/packages/date#datei18n).
+All date format strings should be in the format accepted by by the [`dateI18n` function in `@wordpress/date`](https://github.com/WordPress/gutenberg/tree/trunk/packages/date#datei18n).
## Usage
@@ -43,16 +38,14 @@ The current date format selected by the user. If `null`, _Default_ is selected.
### `defaultFormat`
-The default format string. Used to show to the user what the date will look like
-if _Default_ is selected.
+The default format string. Used to show to the user what the date will look like if _Default_ is selected.
- Type: `string`
- Required: Yes
### `onChange`
-Called when the user makes a selection, or when the user types in a date format.
-`null` indicates that _Default_ is selected.
+Called when the user makes a selection, or when the user types in a date format. `null` indicates that _Default_ is selected.
- Type: `( format: string|null ) => void`
- Required: Yes
diff --git a/packages/block-editor/src/components/date-format-picker/index.js b/packages/block-editor/src/components/date-format-picker/index.js
index eb269e03ca5ab..719390a1d6f90 100644
--- a/packages/block-editor/src/components/date-format-picker/index.js
+++ b/packages/block-editor/src/components/date-format-picker/index.js
@@ -29,21 +29,10 @@ if ( exampleDate.getMonth() === 4 ) {
*
* @see https://github.com/WordPress/gutenberg/blob/HEAD/packages/block-editor/src/components/date-format-picker/README.md
*
- * @param {Object} props
- * @param {string|null} props.format The selected date
- * format. If
- * `null`,
- * _Default_ is
- * selected.
- * @param {string} props.defaultFormat The date format that
- * will be used if the
- * user selects
- * 'Default'.
- * @param {( format: string|null ) => void} props.onChange Called when a
- * selection is
- * made. If `null`,
- * _Default_ is
- * selected.
+ * @param {Object} props
+ * @param {string|null} props.format The selected date format. If `null`, _Default_ is selected.
+ * @param {string} props.defaultFormat The date format that will be used if the user selects 'Default'.
+ * @param {Function} props.onChange Called when a selection is made. If `null`, _Default_ is selected.
*/
export default function DateFormatPicker( {
format,
diff --git a/packages/block-editor/src/components/dimensions-tool/stories/aspect-ratio-tool.story.js b/packages/block-editor/src/components/dimensions-tool/stories/aspect-ratio-tool.story.js
index 9b82404a23c25..b853d78005294 100644
--- a/packages/block-editor/src/components/dimensions-tool/stories/aspect-ratio-tool.story.js
+++ b/packages/block-editor/src/components/dimensions-tool/stories/aspect-ratio-tool.story.js
@@ -16,7 +16,7 @@ export default {
title: 'BlockEditor (Private APIs)/DimensionsTool/AspectRatioTool',
component: AspectRatioTool,
argTypes: {
- panelId: { control: { type: null } },
+ panelId: { control: false },
onChange: { action: 'changed' },
},
};
diff --git a/packages/block-editor/src/components/dimensions-tool/stories/index.story.js b/packages/block-editor/src/components/dimensions-tool/stories/index.story.js
index d9e1a82771282..ebf08fba0c686 100644
--- a/packages/block-editor/src/components/dimensions-tool/stories/index.story.js
+++ b/packages/block-editor/src/components/dimensions-tool/stories/index.story.js
@@ -16,7 +16,7 @@ export default {
title: 'BlockEditor (Private APIs)/DimensionsTool',
component: DimensionsTool,
argTypes: {
- panelId: { control: { type: null } },
+ panelId: { control: false },
onChange: { action: 'changed' },
},
};
diff --git a/packages/block-editor/src/components/dimensions-tool/stories/scale-tool.story.js b/packages/block-editor/src/components/dimensions-tool/stories/scale-tool.story.js
index a5ff9a81b5304..b485bf68a892d 100644
--- a/packages/block-editor/src/components/dimensions-tool/stories/scale-tool.story.js
+++ b/packages/block-editor/src/components/dimensions-tool/stories/scale-tool.story.js
@@ -16,7 +16,7 @@ export default {
title: 'BlockEditor (Private APIs)/DimensionsTool/ScaleTool',
component: ScaleTool,
argTypes: {
- panelId: { control: { type: null } },
+ panelId: { control: false },
onChange: { action: 'changed' },
},
};
diff --git a/packages/block-editor/src/components/dimensions-tool/stories/width-height-tool.story.js b/packages/block-editor/src/components/dimensions-tool/stories/width-height-tool.story.js
index 4a9d9782ad16b..eed3cbc02f466 100644
--- a/packages/block-editor/src/components/dimensions-tool/stories/width-height-tool.story.js
+++ b/packages/block-editor/src/components/dimensions-tool/stories/width-height-tool.story.js
@@ -16,7 +16,7 @@ export default {
title: 'BlockEditor (Private APIs)/DimensionsTool/WidthHeightTool',
component: WidthHeightTool,
argTypes: {
- panelId: { control: { type: null } },
+ panelId: { control: false },
onChange: { action: 'changed' },
},
};
diff --git a/packages/block-editor/src/components/font-appearance-control/index.js b/packages/block-editor/src/components/font-appearance-control/index.js
index 38cb42e394a3b..62396c2dc7bd6 100644
--- a/packages/block-editor/src/components/font-appearance-control/index.js
+++ b/packages/block-editor/src/components/font-appearance-control/index.js
@@ -2,6 +2,7 @@
* WordPress dependencies
*/
import { CustomSelectControl } from '@wordpress/components';
+import deprecated from '@wordpress/deprecated';
import { useMemo } from '@wordpress/element';
import { __, sprintf } from '@wordpress/i18n';
@@ -147,12 +148,27 @@ export default function FontAppearanceControl( props ) {
);
};
+ if (
+ ! __next40pxDefaultSize &&
+ ( otherProps.size === undefined || otherProps.size === 'default' )
+ ) {
+ deprecated(
+ `36px default size for wp.blockEditor.__experimentalFontAppearanceControl`,
+ {
+ since: '6.8',
+ version: '7.1',
+ hint: 'Set the `__next40pxDefaultSize` prop to true to start opting into the new default size, which will become the default in a future version.',
+ }
+ );
+ }
+
return (
hasStylesOrWeights && (
{
setFontFamily( newFontFamily );
} }
__nextHasNoMarginBottom
+ __next40pxDefaultSize
/>
);
};
diff --git a/packages/block-editor/src/components/font-family/index.js b/packages/block-editor/src/components/font-family/index.js
index c87a52b4c676d..6a723bb24c48e 100644
--- a/packages/block-editor/src/components/font-family/index.js
+++ b/packages/block-editor/src/components/font-family/index.js
@@ -1,7 +1,12 @@
+/**
+ * External dependencies
+ */
+import clsx from 'clsx';
+
/**
* WordPress dependencies
*/
-import { SelectControl } from '@wordpress/components';
+import { CustomSelectControl } from '@wordpress/components';
import deprecated from '@wordpress/deprecated';
import { __ } from '@wordpress/i18n';
@@ -18,6 +23,7 @@ export default function FontFamilyControl( {
value = '',
onChange,
fontFamilies,
+ className,
...props
} ) {
const [ blockLevelFontFamilies ] = useSettings( 'typography.fontFamilies' );
@@ -30,13 +36,15 @@ export default function FontFamilyControl( {
}
const options = [
- { value: '', label: __( 'Default' ) },
- ...fontFamilies.map( ( { fontFamily, name } ) => {
- return {
- value: fontFamily,
- label: name || fontFamily,
- };
- } ),
+ {
+ key: '',
+ name: __( 'Default' ),
+ },
+ ...fontFamilies.map( ( { fontFamily, name } ) => ( {
+ key: fontFamily,
+ name: name || fontFamily,
+ style: { fontFamily },
+ } ) ),
];
if ( ! __nextHasNoMarginBottom ) {
@@ -50,15 +58,31 @@ export default function FontFamilyControl( {
);
}
+ if (
+ ! __next40pxDefaultSize &&
+ ( props.size === undefined || props.size === 'default' )
+ ) {
+ deprecated(
+ `36px default size for wp.blockEditor.__experimentalFontFamilyControl`,
+ {
+ since: '6.8',
+ version: '7.1',
+ hint: 'Set the `__next40pxDefaultSize` prop to true to start opting into the new default size, which will become the default in a future version.',
+ }
+ );
+ }
+
return (
- onChange( selectedItem.key ) }
+ options={ options }
+ className={ clsx( 'block-editor-font-family-control', className, {
+ 'is-next-has-no-margin-bottom': __nextHasNoMarginBottom,
+ } ) }
{ ...props }
/>
);
diff --git a/packages/block-editor/src/components/font-family/stories/index.story.js b/packages/block-editor/src/components/font-family/stories/index.story.js
index 54dadeb213f12..9077c131cbe3b 100644
--- a/packages/block-editor/src/components/font-family/stories/index.story.js
+++ b/packages/block-editor/src/components/font-family/stories/index.story.js
@@ -50,5 +50,6 @@ export const Default = {
},
],
__nextHasNoMarginBottom: true,
+ __next40pxDefaultSize: true,
},
};
diff --git a/packages/block-editor/src/components/font-family/style.scss b/packages/block-editor/src/components/font-family/style.scss
new file mode 100644
index 0000000000000..7ee181ebb7953
--- /dev/null
+++ b/packages/block-editor/src/components/font-family/style.scss
@@ -0,0 +1,5 @@
+.block-editor-font-family-control {
+ &:not(.is-next-has-no-margin-bottom) {
+ margin-bottom: $grid-unit-10;
+ }
+}
diff --git a/packages/block-editor/src/components/global-styles/color-panel.js b/packages/block-editor/src/components/global-styles/color-panel.js
index 7c5257ae93bfa..f1a1834967ed9 100644
--- a/packages/block-editor/src/components/global-styles/color-panel.js
+++ b/packages/block-editor/src/components/global-styles/color-panel.js
@@ -19,7 +19,7 @@ import {
Button,
privateApis as componentsPrivateApis,
} from '@wordpress/components';
-import { useCallback } from '@wordpress/element';
+import { useCallback, useRef } from '@wordpress/element';
import { __ } from '@wordpress/i18n';
/**
@@ -30,6 +30,7 @@ import { useColorsPerOrigin, useGradientsPerOrigin } from './hooks';
import { getValueFromVariable, useToolsPanelDropdownMenuProps } from './utils';
import { setImmutably } from '../../utils/object';
import { unlock } from '../../lock-unlock';
+import { reset as resetIcon } from '@wordpress/icons';
export function useHasColorPanel( settings ) {
const hasTextPanel = useHasTextPanel( settings );
@@ -208,6 +209,7 @@ function ColorPanelDropdown( {
} ) {
const currentTab = tabs.find( ( tab ) => tab.userValue !== undefined );
const { key: firstTabKey, ...firstTab } = tabs[ 0 ] ?? {};
+ const colorGradientDropdownButtonRef = useRef( undefined );
return (
-
-
+ <>
+
+
+
+ { hasValue() && (
+ {
+ resetValue();
+ // Return focus to parent button
+ colorGradientDropdownButtonRef.current?.focus();
+ } }
+ />
+ ) }
+ >
);
} }
renderContent={ () => (
diff --git a/packages/block-editor/src/components/global-styles/dimensions-panel.js b/packages/block-editor/src/components/global-styles/dimensions-panel.js
index c19788ebfcb58..385f28b668eda 100644
--- a/packages/block-editor/src/components/global-styles/dimensions-panel.js
+++ b/packages/block-editor/src/components/global-styles/dimensions-panel.js
@@ -444,17 +444,6 @@ export default function DimensionsPanel( {
const onMouseLeaveControls = () => onVisualize( false );
- const inputProps = {
- min: minMarginValue,
- onDragStart: () => {
- //Reset to 0 in case the value was negative.
- setMinMarginValue( 0 );
- },
- onDragEnd: () => {
- setMinMarginValue( minimumMargin );
- },
- };
-
return (
) }
{ showSpacingPresetsControl && (
@@ -581,14 +572,23 @@ export default function DimensionsPanel( {
__next40pxDefaultSize
values={ marginValues }
onChange={ setMarginValues }
- inputProps={ inputProps }
+ inputProps={ {
+ min: minMarginValue,
+ onDragStart: () => {
+ // Reset to 0 in case the value was negative.
+ setMinMarginValue( 0 );
+ },
+ onDragEnd: () => {
+ setMinMarginValue( minimumMargin );
+ },
+ onMouseOver: onMouseOverMargin,
+ onMouseOut: onMouseLeaveControls,
+ } }
label={ __( 'Margin' ) }
sides={ marginSides }
units={ units }
allowReset={ false }
splitOnAxis={ isAxialMargin }
- onMouseOver={ onMouseOverMargin }
- onMouseOut={ onMouseLeaveControls }
/>
) }
{ showSpacingPresetsControl && (
diff --git a/packages/block-editor/src/components/global-styles/filters-panel.js b/packages/block-editor/src/components/global-styles/filters-panel.js
index 581661e0c8407..c62099596f66c 100644
--- a/packages/block-editor/src/components/global-styles/filters-panel.js
+++ b/packages/block-editor/src/components/global-styles/filters-panel.js
@@ -10,10 +10,10 @@ import {
__experimentalToolsPanel as ToolsPanel,
__experimentalToolsPanelItem as ToolsPanelItem,
__experimentalItemGroup as ItemGroup,
+ __experimentalItem as Item,
__experimentalHStack as HStack,
__experimentalZStack as ZStack,
__experimentalDropdownContentWrapper as DropdownContentWrapper,
- Button,
MenuGroup,
ColorIndicator,
DuotonePicker,
@@ -189,15 +189,12 @@ export default function FiltersPanel( {
return (
-
+ -
-
+
);
} }
diff --git a/packages/block-editor/src/components/global-styles/test/use-global-styles-output.js b/packages/block-editor/src/components/global-styles/test/use-global-styles-output.js
index 5022e8ba591db..93e5cc9afdbb3 100644
--- a/packages/block-editor/src/components/global-styles/test/use-global-styles-output.js
+++ b/packages/block-editor/src/components/global-styles/test/use-global-styles-output.js
@@ -855,7 +855,7 @@ describe( 'global styles renderer', () => {
it( 'should return block selectors data with old experimental selectors', () => {
const imageSupports = {
- __experimentalBorder: {
+ border: {
radius: true,
__experimentalSelector: 'img, .crop-area',
},
diff --git a/packages/block-editor/src/components/global-styles/use-global-styles-output.js b/packages/block-editor/src/components/global-styles/use-global-styles-output.js
index cd4ad0cea50e0..fabc65d143d1a 100644
--- a/packages/block-editor/src/components/global-styles/use-global-styles-output.js
+++ b/packages/block-editor/src/components/global-styles/use-global-styles-output.js
@@ -47,7 +47,7 @@ const ELEMENT_CLASS_NAMES = {
// List of block support features that can have their related styles
// generated under their own feature level selector rather than the block's.
const BLOCK_SUPPORT_FEATURE_LEVEL_SELECTORS = {
- __experimentalBorder: 'border',
+ border: 'border',
color: 'color',
spacing: 'spacing',
typography: 'typography',
@@ -624,7 +624,7 @@ function pickStyleKeys( treeToPickFrom ) {
// clone the style objects so that `getFeatureDeclarations` can remove consumed keys from it
const clonedEntries = pickedEntries.map( ( [ key, style ] ) => [
key,
- JSON.parse( JSON.stringify( style ) ),
+ structuredClone( style ),
] );
return Object.fromEntries( clonedEntries );
}
diff --git a/packages/block-editor/src/components/iframe/content.scss b/packages/block-editor/src/components/iframe/content.scss
index 596c177eab2f3..05bbdb25c2dc6 100644
--- a/packages/block-editor/src/components/iframe/content.scss
+++ b/packages/block-editor/src/components/iframe/content.scss
@@ -4,55 +4,66 @@
.block-editor-iframe__html {
transform-origin: top center;
- // We don't want to animate the transform of the translateX because it is used
- // to "center" the canvas. Leaving it on causes the canvas to slide around in
- // odd ways.
- @include editor-canvas-resize-animation(transform 0s, scale 0s, padding 0s);
+ // Prevents a flash of background color change when entering/exiting zoom out
+ transition: background-color 400ms;
&.zoom-out-animation {
- // we only want to animate the scaling when entering zoom out. When sidebars
- // are toggled, the resizing of the iframe handles scaling the canvas as well,
- // and the doubled animations cause very odd animations.
- @include editor-canvas-resize-animation(transform 0s);
+ $scroll-top: var(--wp-block-editor-iframe-zoom-out-scroll-top, 0);
+ $scroll-top-next: var(--wp-block-editor-iframe-zoom-out-scroll-top-next, 0);
+ $overflow-behavior: var(--wp-block-editor-iframe-zoom-out-overflow-behavior, scroll);
+
+ position: fixed;
+ left: 0;
+ right: 0;
+ top: calc(-1 * #{$scroll-top});
+ bottom: 0;
+ // Force preserving a scrollbar gutter as scrollbar-gutter isn't supported in all browsers yet,
+ // and removing the scrollbar causes the content to shift.
+ overflow-y: $overflow-behavior;
}
-}
-.block-editor-iframe__html.is-zoomed-out {
- $scale: var(--wp-block-editor-iframe-zoom-out-scale);
- $frame-size: var(--wp-block-editor-iframe-zoom-out-frame-size);
- $inner-height: var(--wp-block-editor-iframe-zoom-out-inner-height);
- $content-height: var(--wp-block-editor-iframe-zoom-out-content-height);
- $scale-container-width: var(--wp-block-editor-iframe-zoom-out-scale-container-width);
- $container-width: var(--wp-block-editor-iframe-zoom-out-container-width, 100vw);
- // Apply an X translation to center the scaled content within the available space.
- transform: translateX(calc((#{$scale-container-width} - #{$container-width}) / 2 / #{$scale}));
- scale: #{$scale};
- background-color: $gray-300;
-
- // Chrome seems to respect that transform scale shouldn't affect the layout size of the element,
- // so we need to adjust the height of the content to match the scale by using negative margins.
- $extra-content-height: calc(#{$content-height} * (1 - #{$scale}));
- $total-frame-height: calc(2 * #{$frame-size} / #{$scale});
- $total-height: calc(#{$extra-content-height} + #{$total-frame-height} + 2px);
- margin-bottom: calc(-1 * #{$total-height});
- // Add the top/bottom frame size. We use scaling to account for the left/right, as
- // the padding left/right causes the contents to reflow, which breaks the 1:1 scaling
- // of the content.
- padding-top: calc(#{$frame-size} / #{$scale});
- padding-bottom: calc(#{$frame-size} / #{$scale});
-
- body {
- min-height: calc((#{$inner-height} - #{$total-frame-height}) / #{$scale});
-
- > .is-root-container:not(.wp-block-post-content) {
- flex: 1;
- display: flex;
- flex-direction: column;
- height: 100%;
-
- > main {
+ &.is-zoomed-out {
+ $scale: var(--wp-block-editor-iframe-zoom-out-scale, 1);
+ $frame-size: var(--wp-block-editor-iframe-zoom-out-frame-size, 0);
+ $inner-height: var(--wp-block-editor-iframe-zoom-out-inner-height);
+ $content-height: var(--wp-block-editor-iframe-zoom-out-content-height);
+ $scale-container-width: var(--wp-block-editor-iframe-zoom-out-scale-container-width);
+ $container-width: var(--wp-block-editor-iframe-zoom-out-container-width, 100vw);
+ // Apply an X translation to center the scaled content within the available space.
+ transform: translateX(calc((#{$scale-container-width} - #{$container-width}) / 2 / #{$scale}));
+ scale: $scale;
+ background-color: $gray-300;
+
+ // Chrome seems to respect that transform scale shouldn't affect the layout size of the element,
+ // so we need to adjust the height of the content to match the scale by using negative margins.
+ $extra-content-height: calc(#{$content-height} * (1 - #{$scale}));
+ $total-frame-height: calc(2 * #{$frame-size} / #{$scale});
+ $total-height: calc(#{$extra-content-height} + #{$total-frame-height} + 2px);
+ margin-bottom: calc(-1 * #{$total-height});
+
+ // Add the top/bottom frame size. We use scaling to account for the left/right, as
+ // the padding left/right causes the contents to reflow, which breaks the 1:1 scaling
+ // of the content.
+ padding-top: calc(#{$frame-size} / #{$scale});
+ padding-bottom: calc(#{$frame-size} / #{$scale});
+
+ body {
+ min-height: calc((#{$inner-height} - #{$total-frame-height}) / #{$scale});
+
+ > .is-root-container:not(.wp-block-post-content) {
flex: 1;
+ display: flex;
+ flex-direction: column;
+ height: 100%;
+
+ > main {
+ flex: 1;
+ }
}
}
+
+ .wp-block[draggable] {
+ cursor: grab;
+ }
}
}
diff --git a/packages/block-editor/src/components/iframe/index.js b/packages/block-editor/src/components/iframe/index.js
index 76d2e09dfb7a3..751e940dd166c 100644
--- a/packages/block-editor/src/components/iframe/index.js
+++ b/packages/block-editor/src/components/iframe/index.js
@@ -12,15 +12,9 @@ import {
forwardRef,
useMemo,
useEffect,
- useRef,
} from '@wordpress/element';
import { __ } from '@wordpress/i18n';
-import {
- useResizeObserver,
- useMergeRefs,
- useRefEffect,
- useDisabled,
-} from '@wordpress/compose';
+import { useMergeRefs, useRefEffect, useDisabled } from '@wordpress/compose';
import { __experimentalStyleProvider as StyleProvider } from '@wordpress/components';
import { useSelect } from '@wordpress/data';
@@ -30,6 +24,7 @@ import { useSelect } from '@wordpress/data';
import { useBlockSelectionClearer } from '../block-selection-clearer';
import { useWritingFlow } from '../writing-flow';
import { getCompatibilityStyles } from './get-compatibility-styles';
+import { useScaleCanvas } from './use-scale-canvas';
import { store as blockEditorStore } from '../../store';
function bubbleEvent( event, Constructor, frame ) {
@@ -121,15 +116,11 @@ function Iframe( {
};
}, [] );
const { styles = '', scripts = '' } = resolvedAssets;
+ /** @type {[Document, import('react').Dispatch]} */
const [ iframeDocument, setIframeDocument ] = useState();
- const initialContainerWidthRef = useRef( 0 );
const [ bodyClasses, setBodyClasses ] = useState( [] );
const clearerRef = useBlockSelectionClearer();
const [ before, writingFlowRef, after ] = useWritingFlow();
- const [ contentResizeListener, { height: contentHeight } ] =
- useResizeObserver();
- const [ containerResizeListener, { width: containerWidth } ] =
- useResizeObserver();
const setRef = useRefEffect( ( node ) => {
node._load = () => {
@@ -225,48 +216,16 @@ function Iframe( {
};
}, [] );
- const [ iframeWindowInnerHeight, setIframeWindowInnerHeight ] = useState();
-
- const iframeResizeRef = useRefEffect( ( node ) => {
- const nodeWindow = node.ownerDocument.defaultView;
-
- setIframeWindowInnerHeight( nodeWindow.innerHeight );
- const onResize = () => {
- setIframeWindowInnerHeight( nodeWindow.innerHeight );
- };
- nodeWindow.addEventListener( 'resize', onResize );
- return () => {
- nodeWindow.removeEventListener( 'resize', onResize );
- };
- }, [] );
-
- const [ windowInnerWidth, setWindowInnerWidth ] = useState();
-
- const windowResizeRef = useRefEffect( ( node ) => {
- const nodeWindow = node.ownerDocument.defaultView;
-
- setWindowInnerWidth( nodeWindow.innerWidth );
- const onResize = () => {
- setWindowInnerWidth( nodeWindow.innerWidth );
- };
- nodeWindow.addEventListener( 'resize', onResize );
- return () => {
- nodeWindow.removeEventListener( 'resize', onResize );
- };
- }, [] );
-
- const isZoomedOut = scale !== 1;
-
- useEffect( () => {
- if ( ! isZoomedOut ) {
- initialContainerWidthRef.current = containerWidth;
- }
- }, [ containerWidth, isZoomedOut ] );
-
- const scaleContainerWidth = Math.max(
- initialContainerWidthRef.current,
- containerWidth
- );
+ const {
+ contentResizeListener,
+ containerResizeListener,
+ isZoomedOut,
+ scaleContainerWidth,
+ } = useScaleCanvas( {
+ scale,
+ frameSize: parseInt( frameSize ),
+ iframeDocument,
+ } );
const disabledRef = useDisabled( { isDisabled: ! readonly } );
const bodyRef = useMergeRefs( [
@@ -275,10 +234,6 @@ function Iframe( {
clearerRef,
writingFlowRef,
disabledRef,
- // Avoid resize listeners when not needed, these will trigger
- // unnecessary re-renders when animating the iframe width, or when
- // expanding preview iframes.
- isZoomedOut ? iframeResizeRef : null,
] );
// Correct doctype is required to enable rendering in standards
@@ -320,118 +275,6 @@ function Iframe( {
useEffect( () => cleanup, [ cleanup ] );
- const zoomOutAnimationClassnameRef = useRef( null );
-
- // Toggle zoom out CSS Classes only when zoom out mode changes. We could add these into the useEffect
- // that controls settings the CSS variables, but then we would need to do more work to ensure we're
- // only toggling these when the zoom out mode changes, as that useEffect is also triggered by a large
- // number of dependencies.
- useEffect( () => {
- if ( ! iframeDocument || ! isZoomedOut ) {
- return;
- }
-
- const handleZoomOutAnimationClassname = () => {
- clearTimeout( zoomOutAnimationClassnameRef.current );
-
- iframeDocument.documentElement.classList.add(
- 'zoom-out-animation'
- );
-
- zoomOutAnimationClassnameRef.current = setTimeout( () => {
- iframeDocument.documentElement.classList.remove(
- 'zoom-out-animation'
- );
- }, 400 ); // 400ms should match the animation speed used in components/iframe/content.scss
- };
-
- handleZoomOutAnimationClassname();
- iframeDocument.documentElement.classList.add( 'is-zoomed-out' );
-
- return () => {
- handleZoomOutAnimationClassname();
- iframeDocument.documentElement.classList.remove( 'is-zoomed-out' );
- };
- }, [ iframeDocument, isZoomedOut ] );
-
- // Calculate the scaling and CSS variables for the zoom out canvas
- useEffect( () => {
- if ( ! iframeDocument || ! isZoomedOut ) {
- return;
- }
-
- const maxWidth = 750;
- // Note: When we initialize the zoom out when the canvas is smaller (sidebars open),
- // initialContainerWidthRef will be smaller than the full page, and reflow will happen
- // when the canvas area becomes larger due to sidebars closing. This is a known but
- // minor divergence for now.
-
- // This scaling calculation has to happen within the JS because CSS calc() can
- // only divide and multiply by a unitless value. I.e. calc( 100px / 2 ) is valid
- // but calc( 100px / 2px ) is not.
- iframeDocument.documentElement.style.setProperty(
- '--wp-block-editor-iframe-zoom-out-scale',
- scale === 'auto-scaled'
- ? ( Math.min( containerWidth, maxWidth ) -
- parseInt( frameSize ) * 2 ) /
- scaleContainerWidth
- : scale
- );
-
- // frameSize has to be a px value for the scaling and frame size to be computed correctly.
- iframeDocument.documentElement.style.setProperty(
- '--wp-block-editor-iframe-zoom-out-frame-size',
- typeof frameSize === 'number' ? `${ frameSize }px` : frameSize
- );
- iframeDocument.documentElement.style.setProperty(
- '--wp-block-editor-iframe-zoom-out-content-height',
- `${ contentHeight }px`
- );
- iframeDocument.documentElement.style.setProperty(
- '--wp-block-editor-iframe-zoom-out-inner-height',
- `${ iframeWindowInnerHeight }px`
- );
- iframeDocument.documentElement.style.setProperty(
- '--wp-block-editor-iframe-zoom-out-container-width',
- `${ containerWidth }px`
- );
- iframeDocument.documentElement.style.setProperty(
- '--wp-block-editor-iframe-zoom-out-scale-container-width',
- `${ scaleContainerWidth }px`
- );
-
- return () => {
- iframeDocument.documentElement.style.removeProperty(
- '--wp-block-editor-iframe-zoom-out-scale'
- );
- iframeDocument.documentElement.style.removeProperty(
- '--wp-block-editor-iframe-zoom-out-frame-size'
- );
- iframeDocument.documentElement.style.removeProperty(
- '--wp-block-editor-iframe-zoom-out-content-height'
- );
- iframeDocument.documentElement.style.removeProperty(
- '--wp-block-editor-iframe-zoom-out-inner-height'
- );
- iframeDocument.documentElement.style.removeProperty(
- '--wp-block-editor-iframe-zoom-out-container-width'
- );
- iframeDocument.documentElement.style.removeProperty(
- '--wp-block-editor-iframe-zoom-out-scale-container-width'
- );
- };
- }, [
- scale,
- frameSize,
- iframeDocument,
- iframeWindowInnerHeight,
- contentHeight,
- containerWidth,
- windowInnerWidth,
- isZoomedOut,
- scaleContainerWidth,
- ] );
-
// Make sure to not render the before and after focusable div elements in view
// mode. They're only needed to capture focus in edit mode.
const shouldRenderFocusCaptureElements = tabIndex >= 0 && ! isPreviewMode;
@@ -511,7 +354,7 @@ function Iframe( {
);
return (
-
+
{ containerResizeListener }
{
+ if ( ! isZoomedOut ) {
+ initialContainerWidthRef.current = containerWidth;
+ }
+ }, [ containerWidth, isZoomedOut ] );
+
+ const scaleContainerWidth = Math.max(
+ initialContainerWidthRef.current,
+ containerWidth
+ );
+
+ const scaleValue = isAutoScaled
+ ? calculateScale( {
+ frameSize,
+ containerWidth,
+ maxContainerWidth,
+ scaleContainerWidth,
+ } )
+ : scale;
+
+ /**
+ * The starting transition state for the zoom out animation.
+ * @type {import('react').RefObject
}
+ */
+ const transitionFromRef = useRef( {
+ scaleValue,
+ frameSize,
+ containerHeight: 0,
+ scrollTop: 0,
+ scrollHeight: 0,
+ } );
+
+ /**
+ * The ending transition state for the zoom out animation.
+ * @type {import('react').RefObject}
+ */
+ const transitionToRef = useRef( {
+ scaleValue,
+ frameSize,
+ containerHeight: 0,
+ scrollTop: 0,
+ scrollHeight: 0,
+ } );
+
+ /**
+ * Start the zoom out animation. This sets the necessary CSS variables
+ * for animating the canvas and returns the Animation object.
+ *
+ * @return {Animation} The animation object for the zoom out animation.
+ */
+ const startZoomOutAnimation = useCallback( () => {
+ const { scrollTop } = transitionFromRef.current;
+ const { scrollTop: scrollTopNext } = transitionToRef.current;
+
+ iframeDocument.documentElement.style.setProperty(
+ '--wp-block-editor-iframe-zoom-out-scroll-top',
+ `${ scrollTop }px`
+ );
+
+ iframeDocument.documentElement.style.setProperty(
+ '--wp-block-editor-iframe-zoom-out-scroll-top-next',
+ `${ scrollTopNext }px`
+ );
+
+ // If the container has a scrolllbar, force a scrollbar to prevent the content from shifting while animating.
+ iframeDocument.documentElement.style.setProperty(
+ '--wp-block-editor-iframe-zoom-out-overflow-behavior',
+ transitionFromRef.current.scrollHeight ===
+ transitionFromRef.current.containerHeight
+ ? 'auto'
+ : 'scroll'
+ );
+
+ iframeDocument.documentElement.classList.add( 'zoom-out-animation' );
+
+ return iframeDocument.documentElement.animate(
+ getAnimationKeyframes(
+ transitionFromRef.current,
+ transitionToRef.current
+ ),
+ {
+ easing: 'cubic-bezier(0.46, 0.03, 0.52, 0.96)',
+ duration: 400,
+ }
+ );
+ }, [ iframeDocument ] );
+
+ /**
+ * Callback when the zoom out animation is finished.
+ * - Cleans up animations refs.
+ * - Adds final CSS vars for scale and frame size to preserve the state.
+ * - Removes the 'zoom-out-animation' class (which has the fixed positioning).
+ * - Sets the final scroll position after the canvas is no longer in fixed position.
+ * - Removes CSS vars related to the animation.
+ * - Sets the transitionFrom to the transitionTo state to be ready for the next animation.
+ */
+ const finishZoomOutAnimation = useCallback( () => {
+ startAnimationRef.current = false;
+ animationRef.current = null;
+
+ // Add our final scale and frame size now that the animation is done.
+ iframeDocument.documentElement.style.setProperty(
+ '--wp-block-editor-iframe-zoom-out-scale',
+ transitionToRef.current.scaleValue
+ );
+ iframeDocument.documentElement.style.setProperty(
+ '--wp-block-editor-iframe-zoom-out-frame-size',
+ `${ transitionToRef.current.frameSize }px`
+ );
+
+ iframeDocument.documentElement.classList.remove( 'zoom-out-animation' );
+
+ // Set the final scroll position that was just animated to.
+ // Disable reason: Eslint isn't smart enough to know that this is a
+ // DOM element. https://github.com/facebook/react/issues/31483
+ // eslint-disable-next-line react-compiler/react-compiler
+ iframeDocument.documentElement.scrollTop =
+ transitionToRef.current.scrollTop;
+
+ iframeDocument.documentElement.style.removeProperty(
+ '--wp-block-editor-iframe-zoom-out-scroll-top'
+ );
+ iframeDocument.documentElement.style.removeProperty(
+ '--wp-block-editor-iframe-zoom-out-scroll-top-next'
+ );
+ iframeDocument.documentElement.style.removeProperty(
+ '--wp-block-editor-iframe-zoom-out-overflow-behavior'
+ );
+
+ // Update previous values.
+ transitionFromRef.current = transitionToRef.current;
+ }, [ iframeDocument ] );
+
+ const previousIsZoomedOut = useRef( false );
+
+ /**
+ * Runs when zoom out mode is toggled, and sets the startAnimation flag
+ * so the animation will start when the next useEffect runs. We _only_
+ * want to animate when the zoom out mode is toggled, not when the scale
+ * changes due to the container resizing.
+ */
+ useEffect( () => {
+ const trigger =
+ iframeDocument && previousIsZoomedOut.current !== isZoomedOut;
+
+ previousIsZoomedOut.current = isZoomedOut;
+
+ if ( ! trigger ) {
+ return;
+ }
+
+ startAnimationRef.current = true;
+
+ if ( ! isZoomedOut ) {
+ return;
+ }
+
+ iframeDocument.documentElement.classList.add( 'is-zoomed-out' );
+ return () => {
+ iframeDocument.documentElement.classList.remove( 'is-zoomed-out' );
+ };
+ }, [ iframeDocument, isZoomedOut ] );
+
+ /**
+ * This handles:
+ * 1. Setting the correct scale and vars of the canvas when zoomed out
+ * 2. If zoom out mode has been toggled, runs the animation of zooming in/out
+ */
+ useEffect( () => {
+ if ( ! iframeDocument ) {
+ return;
+ }
+
+ // We need to update the appropriate scale to exit from. If sidebars have been opened since setting the
+ // original scale, we will snap to a much smaller scale due to the scale container immediately changing sizes when exiting.
+ if ( isAutoScaled && transitionFromRef.current.scaleValue !== 1 ) {
+ // We use containerWidth as the divisor, as scaleContainerWidth will always match the containerWidth when
+ // exiting.
+ transitionFromRef.current.scaleValue = calculateScale( {
+ frameSize: transitionFromRef.current.frameSize,
+ containerWidth,
+ maxContainerWidth,
+ scaleContainerWidth: containerWidth,
+ } );
+ }
+
+ if ( scaleValue < 1 ) {
+ // If we are not going to animate the transition, set the scale and frame size directly.
+ // If we are animating, these values will be set when the animation is finished.
+ // Example: Opening sidebars that reduce the scale of the canvas, but we don't want to
+ // animate the transition.
+ if ( ! startAnimationRef.current ) {
+ iframeDocument.documentElement.style.setProperty(
+ '--wp-block-editor-iframe-zoom-out-scale',
+ scaleValue
+ );
+ iframeDocument.documentElement.style.setProperty(
+ '--wp-block-editor-iframe-zoom-out-frame-size',
+ `${ frameSize }px`
+ );
+ }
+
+ iframeDocument.documentElement.style.setProperty(
+ '--wp-block-editor-iframe-zoom-out-content-height',
+ `${ contentHeight }px`
+ );
+
+ iframeDocument.documentElement.style.setProperty(
+ '--wp-block-editor-iframe-zoom-out-inner-height',
+ `${ containerHeight }px`
+ );
+
+ iframeDocument.documentElement.style.setProperty(
+ '--wp-block-editor-iframe-zoom-out-container-width',
+ `${ containerWidth }px`
+ );
+ iframeDocument.documentElement.style.setProperty(
+ '--wp-block-editor-iframe-zoom-out-scale-container-width',
+ `${ scaleContainerWidth }px`
+ );
+ }
+
+ /**
+ * Handle the zoom out animation:
+ *
+ * - Get the current scrollTop position.
+ * - Calculate where the same scroll position is after scaling.
+ * - Apply fixed positioning to the canvas with a transform offset
+ * to keep the canvas centered.
+ * - Animate the scale and padding to the new scale and frame size.
+ * - After the animation is complete, remove the fixed positioning
+ * and set the scroll position that keeps everything centered.
+ */
+ if ( startAnimationRef.current ) {
+ // Don't allow a new transition to start again unless it was started by the zoom out mode changing.
+ startAnimationRef.current = false;
+
+ /**
+ * If we already have an animation running, reverse it.
+ */
+ if ( animationRef.current ) {
+ animationRef.current.reverse();
+ // Swap the transition to/from refs so that we set the correct values when
+ // finishZoomOutAnimation runs.
+ const tempTransitionFrom = transitionFromRef.current;
+ const tempTransitionTo = transitionToRef.current;
+ transitionFromRef.current = tempTransitionTo;
+ transitionToRef.current = tempTransitionFrom;
+ } else {
+ /**
+ * Start a new zoom animation.
+ */
+
+ // We can't trust the set value from contentHeight, as it was measured
+ // before the zoom out mode was changed. After zoom out mode is changed,
+ // appenders may appear or disappear, so we need to get the height from
+ // the iframe at this point when we're about to animate the zoom out.
+ // The iframe scrollTop, scrollHeight, and clientHeight will all be
+ // the most accurate.
+ transitionFromRef.current.scrollTop =
+ iframeDocument.documentElement.scrollTop;
+ transitionFromRef.current.scrollHeight =
+ iframeDocument.documentElement.scrollHeight;
+ // Use containerHeight, as it's the previous container height before the zoom out animation starts.
+ transitionFromRef.current.containerHeight = containerHeight;
+
+ transitionToRef.current = {
+ scaleValue,
+ frameSize,
+ containerHeight:
+ iframeDocument.documentElement.clientHeight, // use clientHeight to get the actual height of the new container after zoom state changes have rendered, as it will be the most up-to-date.
+ };
+
+ transitionToRef.current.scrollHeight = computeScrollHeightNext(
+ transitionFromRef.current,
+ transitionToRef.current
+ );
+ transitionToRef.current.scrollTop = computeScrollTopNext(
+ transitionFromRef.current,
+ transitionToRef.current
+ );
+
+ animationRef.current = startZoomOutAnimation();
+
+ // If the user prefers reduced motion, finish the animation immediately and set the final state.
+ if ( prefersReducedMotion ) {
+ finishZoomOutAnimation();
+ } else {
+ animationRef.current.onfinish = finishZoomOutAnimation;
+ }
+ }
+ }
+ }, [
+ startZoomOutAnimation,
+ finishZoomOutAnimation,
+ prefersReducedMotion,
+ isAutoScaled,
+ scaleValue,
+ frameSize,
+ iframeDocument,
+ contentHeight,
+ containerWidth,
+ containerHeight,
+ maxContainerWidth,
+ scaleContainerWidth,
+ ] );
+
+ return {
+ isZoomedOut,
+ scaleContainerWidth,
+ contentResizeListener,
+ containerResizeListener,
+ };
+}
diff --git a/packages/block-editor/src/components/image-editor/use-save-image.js b/packages/block-editor/src/components/image-editor/use-save-image.js
index 094ce1600545b..980ac9b8b1123 100644
--- a/packages/block-editor/src/components/image-editor/use-save-image.js
+++ b/packages/block-editor/src/components/image-editor/use-save-image.js
@@ -10,6 +10,12 @@ import { __, sprintf } from '@wordpress/i18n';
import { store as noticesStore } from '@wordpress/notices';
import { __unstableStripHTML as stripHTML } from '@wordpress/dom';
+const messages = {
+ crop: __( 'Image cropped.' ),
+ rotate: __( 'Image rotated.' ),
+ cropAndRotate: __( 'Image cropped and rotated.' ),
+};
+
export default function useSaveImage( {
crop,
rotation,
@@ -18,7 +24,8 @@ export default function useSaveImage( {
onSaveImage,
onFinishEditing,
} ) {
- const { createErrorNotice } = useDispatch( noticesStore );
+ const { createErrorNotice, createSuccessNotice } =
+ useDispatch( noticesStore );
const [ isInProgress, setIsInProgress ] = useState( false );
const cancel = useCallback( () => {
@@ -61,6 +68,9 @@ export default function useSaveImage( {
return;
}
+ const modifierType =
+ modifiers.length === 1 ? modifiers[ 0 ].type : 'cropAndRotate';
+
apiFetch( {
path: `/wp/v2/media/${ id }/edit`,
method: 'POST',
@@ -71,11 +81,25 @@ export default function useSaveImage( {
id: response.id,
url: response.source_url,
} );
+ createSuccessNotice( messages[ modifierType ], {
+ type: 'snackbar',
+ actions: [
+ {
+ label: __( 'Undo' ),
+ onClick: () => {
+ onSaveImage( {
+ id,
+ url,
+ } );
+ },
+ },
+ ],
+ } );
} )
.catch( ( error ) => {
createErrorNotice(
sprintf(
- /* translators: 1. Error message */
+ /* translators: %s: Error message. */
__( 'Could not edit image. %s' ),
stripHTML( error.message )
),
@@ -96,6 +120,7 @@ export default function useSaveImage( {
url,
onSaveImage,
createErrorNotice,
+ createSuccessNotice,
onFinishEditing,
] );
diff --git a/packages/block-editor/src/components/index.js b/packages/block-editor/src/components/index.js
index 29bb71b682e97..cf9167e478157 100644
--- a/packages/block-editor/src/components/index.js
+++ b/packages/block-editor/src/components/index.js
@@ -67,10 +67,13 @@ export {
JustifyToolbar,
JustifyContentControl,
} from './justify-content-control';
-export { default as __experimentalLinkControl } from './link-control';
-export { default as __experimentalLinkControlSearchInput } from './link-control/search-input';
-export { default as __experimentalLinkControlSearchResults } from './link-control/search-results';
-export { default as __experimentalLinkControlSearchItem } from './link-control/search-item';
+export {
+ default as LinkControl,
+ DeprecatedExperimentalLinkControl as __experimentalLinkControl,
+} from './link-control';
+export { __experimentalLinkControlSearchInput } from './link-control/search-input';
+export { __experimentalLinkControlSearchResults } from './link-control/search-results';
+export { __experimentalLinkControlSearchItem } from './link-control/search-item';
export { default as LineHeightControl } from './line-height-control';
export { default as __experimentalListView } from './list-view';
export { default as MediaReplaceFlow } from './media-replace-flow';
diff --git a/packages/block-editor/src/components/inserter-draggable-blocks/index.js b/packages/block-editor/src/components/inserter-draggable-blocks/index.js
index 5a63535be3d3c..ebef6304937aa 100644
--- a/packages/block-editor/src/components/inserter-draggable-blocks/index.js
+++ b/packages/block-editor/src/components/inserter-draggable-blocks/index.js
@@ -2,12 +2,9 @@
* WordPress dependencies
*/
import { Draggable } from '@wordpress/components';
-import {
- createBlock,
- serialize,
- store as blocksStore,
-} from '@wordpress/blocks';
+import { createBlock, store as blocksStore } from '@wordpress/blocks';
import { useDispatch, useSelect } from '@wordpress/data';
+import { useMemo } from '@wordpress/element';
/**
* Internal dependencies
@@ -24,11 +21,6 @@ const InserterDraggableBlocks = ( {
children,
pattern,
} ) => {
- const transferData = {
- type: 'inserter',
- blocks,
- };
-
const blockTypeIcon = useSelect(
( select ) => {
const { getBlockType } = select( blocksStore );
@@ -43,6 +35,13 @@ const InserterDraggableBlocks = ( {
useDispatch( blockEditorStore )
);
+ const patternBlock = useMemo( () => {
+ return pattern?.type === INSERTER_PATTERN_TYPES.user &&
+ pattern?.syncStatus !== 'unsynced'
+ ? [ createBlock( 'core/block', { ref: pattern.id } ) ]
+ : undefined;
+ }, [ pattern?.type, pattern?.syncStatus, pattern?.id ] );
+
if ( ! isEnabled ) {
return children( {
draggable: false,
@@ -51,21 +50,21 @@ const InserterDraggableBlocks = ( {
} );
}
+ const draggableBlocks = patternBlock ?? blocks;
return (
{
startDragging();
- const parsedBlocks =
- pattern?.type === INSERTER_PATTERN_TYPES.user &&
- pattern?.syncStatus !== 'unsynced'
- ? [ createBlock( 'core/block', { ref: pattern.id } ) ]
- : blocks;
- event.dataTransfer.setData(
- 'text/html',
- serialize( parsedBlocks )
- );
+ for ( const block of draggableBlocks ) {
+ const type = `wp-block:${ block.name }`;
+ // This will fill in the dataTransfer.types array so that
+ // the drop zone can check if the draggable is eligible.
+ // Unfortuantely, on drag start, we don't have access to the
+ // actual data, only the data keys/types.
+ event.dataTransfer.items.add( '', type );
+ }
} }
onDragEnd={ () => {
stopDragging();
diff --git a/packages/block-editor/src/components/inserter/block-patterns-tab/index.js b/packages/block-editor/src/components/inserter/block-patterns-tab/index.js
index 01e41111b7c89..45db4732aa9c6 100644
--- a/packages/block-editor/src/components/inserter/block-patterns-tab/index.js
+++ b/packages/block-editor/src/components/inserter/block-patterns-tab/index.js
@@ -3,9 +3,8 @@
*/
import { useState } from '@wordpress/element';
import { useViewportMatch } from '@wordpress/compose';
-import { Button, Spinner } from '@wordpress/components';
+import { Button } from '@wordpress/components';
import { __ } from '@wordpress/i18n';
-import { useSelect } from '@wordpress/data';
/**
* Internal dependencies
@@ -16,8 +15,6 @@ import { PatternCategoryPreviews } from './pattern-category-previews';
import { usePatternCategories } from './use-pattern-categories';
import CategoryTabs from '../category-tabs';
import InserterNoResults from '../no-results';
-import { store as blockEditorStore } from '../../../store';
-import { unlock } from '../../../lock-unlock';
function BlockPatternsTab( {
onSelectCategory,
@@ -31,19 +28,6 @@ function BlockPatternsTab( {
const categories = usePatternCategories( rootClientId );
const isMobile = useViewportMatch( 'medium', '<' );
- const isResolvingPatterns = useSelect(
- ( select ) =>
- unlock( select( blockEditorStore ) ).isResolvingPatterns(),
- []
- );
-
- if ( isResolvingPatterns ) {
- return (
-
-
-
- );
- }
if ( ! categories.length ) {
return ;
diff --git a/packages/block-editor/src/components/inserter/block-patterns-tab/pattern-category-previews.js b/packages/block-editor/src/components/inserter/block-patterns-tab/pattern-category-previews.js
index a19a579ae5c0c..c6ce9ba97d250 100644
--- a/packages/block-editor/src/components/inserter/block-patterns-tab/pattern-category-previews.js
+++ b/packages/block-editor/src/components/inserter/block-patterns-tab/pattern-category-previews.js
@@ -17,7 +17,6 @@ import {
__experimentalText as Text,
FlexBlock,
} from '@wordpress/components';
-import { useSelect } from '@wordpress/data';
/**
* Internal dependencies
@@ -34,8 +33,6 @@ import {
starterPatternsCategory,
INSERTER_PATTERN_TYPES,
} from './utils';
-import { store as blockEditorStore } from '../../../store';
-import { unlock } from '../../../lock-unlock';
const noop = () => {};
@@ -46,10 +43,6 @@ export function PatternCategoryPreviews( {
category,
showTitlesAsTooltip,
} ) {
- const isZoomOutMode = useSelect(
- ( select ) => unlock( select( blockEditorStore ) ).isZoomOut(),
- []
- );
const [ allPatterns, , onClickPattern ] = usePatternsState(
onInsert,
rootClientId,
@@ -179,15 +172,13 @@ export function PatternCategoryPreviews( {
{ currentCategoryPatterns.length > 0 && (
<>
- { isZoomOutMode && (
-
- { __( 'Drag and drop patterns into the canvas.' ) }
-
- ) }
+
+ { __( 'Drag and drop patterns into the canvas.' ) }
+
{
- // If there is no active tab, make the first tab the active tab, so that
- // when focus is moved to the tablist, the first tab will be focused
- // despite not being selected
- if ( selectedTabId === null && ! activeTabId && firstTabId ) {
- setActiveId( firstTabId );
- }
- }, [ selectedTabId, activeTabId, firstTabId, setActiveId ] );
+
+ // If there is no active tab, make the first tab the active tab, so that
+ // when focus is moved to the tablist, the first tab will be focused
+ // despite not being selected
+ if ( selectedTabId === null && ! activeTabId && firstTabId ) {
+ setActiveId( firstTabId );
+ }
return (
unlock( select( blockEditorStore ) ).isZoomOut(),
[]
);
+ const hasSectionRootClientId = useSelect(
+ ( select ) =>
+ !! unlock( select( blockEditorStore ) ).getSectionRootClientId(),
+ []
+ );
const [ filterValue, setFilterValue, delayedFilterValue ] =
useDebouncedInput( __experimentalFilterValue );
const [ hoveredItem, setHoveredItem ] = useState( null );
@@ -77,11 +82,15 @@ function InserterMenu(
if ( isZoomOutMode ) {
return 'patterns';
}
+
+ return 'blocks';
}
const [ selectedTab, setSelectedTab ] = useState( getInitialTab() );
const shouldUseZoomOut =
- selectedTab === 'patterns' || selectedTab === 'media';
+ hasSectionRootClientId &&
+ ( selectedTab === 'patterns' || selectedTab === 'media' );
+
useZoomOut( shouldUseZoomOut && isLargeViewport );
const [ destinationRootClientId, onInsertBlocks, onToggleInsertionPoint ] =
diff --git a/packages/block-editor/src/components/inspector-controls-tabs/position-controls-panel.js b/packages/block-editor/src/components/inspector-controls-tabs/position-controls-panel.js
index 9a3670b5deb28..42a8597227dee 100644
--- a/packages/block-editor/src/components/inspector-controls-tabs/position-controls-panel.js
+++ b/packages/block-editor/src/components/inspector-controls-tabs/position-controls-panel.js
@@ -53,9 +53,7 @@ const PositionControlsPanel = () => {
};
const PositionControls = () => {
- const fills = useSlotFills(
- InspectorControlsGroups.position.Slot.__unstableName
- );
+ const fills = useSlotFills( InspectorControlsGroups.position.name );
const hasFills = Boolean( fills && fills.length );
if ( ! hasFills ) {
diff --git a/packages/block-editor/src/components/inspector-controls-tabs/use-inspector-controls-tabs.js b/packages/block-editor/src/components/inspector-controls-tabs/use-inspector-controls-tabs.js
index 6a80d47f02481..c0655f4d85f3f 100644
--- a/packages/block-editor/src/components/inspector-controls-tabs/use-inspector-controls-tabs.js
+++ b/packages/block-editor/src/components/inspector-controls-tabs/use-inspector-controls-tabs.js
@@ -46,18 +46,18 @@ export default function useInspectorControlsTabs( blockName ) {
// List View Tab: If there are any fills for the list group add that tab.
const listViewDisabled = useIsListViewTabDisabled( blockName );
- const listFills = useSlotFills( listGroup.Slot.__unstableName );
+ const listFills = useSlotFills( listGroup.name );
const hasListFills = ! listViewDisabled && !! listFills && listFills.length;
// Styles Tab: Add this tab if there are any fills for block supports
// e.g. border, color, spacing, typography, etc.
const styleFills = [
- ...( useSlotFills( borderGroup.Slot.__unstableName ) || [] ),
- ...( useSlotFills( colorGroup.Slot.__unstableName ) || [] ),
- ...( useSlotFills( dimensionsGroup.Slot.__unstableName ) || [] ),
- ...( useSlotFills( stylesGroup.Slot.__unstableName ) || [] ),
- ...( useSlotFills( typographyGroup.Slot.__unstableName ) || [] ),
- ...( useSlotFills( effectsGroup.Slot.__unstableName ) || [] ),
+ ...( useSlotFills( borderGroup.name ) || [] ),
+ ...( useSlotFills( colorGroup.name ) || [] ),
+ ...( useSlotFills( dimensionsGroup.name ) || [] ),
+ ...( useSlotFills( stylesGroup.name ) || [] ),
+ ...( useSlotFills( typographyGroup.name ) || [] ),
+ ...( useSlotFills( effectsGroup.name ) || [] ),
];
const hasStyleFills = styleFills.length;
@@ -67,12 +67,12 @@ export default function useInspectorControlsTabs( blockName ) {
// the advanced controls slot as well to ensure they are rendered.
const advancedFills = [
...( useSlotFills( InspectorAdvancedControls.slotName ) || [] ),
- ...( useSlotFills( bindingsGroup.Slot.__unstableName ) || [] ),
+ ...( useSlotFills( bindingsGroup.name ) || [] ),
];
const settingsFills = [
- ...( useSlotFills( defaultGroup.Slot.__unstableName ) || [] ),
- ...( useSlotFills( positionGroup.Slot.__unstableName ) || [] ),
+ ...( useSlotFills( defaultGroup.name ) || [] ),
+ ...( useSlotFills( positionGroup.name ) || [] ),
...( hasListFills && hasStyleFills > 1 ? advancedFills : [] ),
];
diff --git a/packages/block-editor/src/components/inspector-controls/README.md b/packages/block-editor/src/components/inspector-controls/README.md
index fd4847f53472b..916f53b5f42d8 100644
--- a/packages/block-editor/src/components/inspector-controls/README.md
+++ b/packages/block-editor/src/components/inspector-controls/README.md
@@ -116,6 +116,7 @@ registerBlockType( 'my-plugin/inspector-controls-example', {
{
diff --git a/packages/block-editor/src/components/inspector-controls/slot.js b/packages/block-editor/src/components/inspector-controls/slot.js
index cc32b1c88480e..4957ca90b5679 100644
--- a/packages/block-editor/src/components/inspector-controls/slot.js
+++ b/packages/block-editor/src/components/inspector-controls/slot.js
@@ -1,11 +1,7 @@
/**
* WordPress dependencies
*/
-import {
- __experimentalUseSlotFills as useSlotFills,
- __unstableMotionContext as MotionContext,
-} from '@wordpress/components';
-import { useContext, useMemo } from '@wordpress/element';
+import { __experimentalUseSlotFills as useSlotFills } from '@wordpress/components';
import warning from '@wordpress/warning';
import deprecated from '@wordpress/deprecated';
@@ -34,23 +30,10 @@ export default function InspectorControlsSlot( {
);
group = __experimentalGroup;
}
- const Slot = groups[ group ]?.Slot;
- const fills = useSlotFills( Slot?.__unstableName );
+ const slotFill = groups[ group ];
+ const fills = useSlotFills( slotFill?.name );
- const motionContextValue = useContext( MotionContext );
-
- const computedFillProps = useMemo(
- () => ( {
- ...( fillProps ?? {} ),
- forwardedContext: [
- ...( fillProps?.forwardedContext ?? [] ),
- [ MotionContext.Provider, { value: motionContextValue } ],
- ],
- } ),
- [ motionContextValue, fillProps ]
- );
-
- if ( ! Slot ) {
+ if ( ! slotFill ) {
warning( `Unknown InspectorControls group "${ group }" provided.` );
return null;
}
@@ -59,19 +42,19 @@ export default function InspectorControlsSlot( {
return null;
}
+ const { Slot } = slotFill;
+
if ( label ) {
return (
);
}
- return (
-
- );
+ return ;
}
diff --git a/packages/block-editor/src/components/letter-spacing-control/README.md b/packages/block-editor/src/components/letter-spacing-control/README.md
index ef119bbc943d2..535ca2ae8cbbf 100644
--- a/packages/block-editor/src/components/letter-spacing-control/README.md
+++ b/packages/block-editor/src/components/letter-spacing-control/README.md
@@ -12,13 +12,14 @@ This component is used for blocks that display text, commonly inside a
Renders a letter spacing control.
```jsx
-import { LetterSpacingControl } from '@wordpress/block-editor';
+import { __experimentalLetterSpacingControl as LetterSpacingControl } from '@wordpress/block-editor';
const MyLetterSpacingControl = () => (
);
```
diff --git a/packages/block-editor/src/components/letter-spacing-control/index.js b/packages/block-editor/src/components/letter-spacing-control/index.js
index 1577e184c4a06..1edbe65a3737e 100644
--- a/packages/block-editor/src/components/letter-spacing-control/index.js
+++ b/packages/block-editor/src/components/letter-spacing-control/index.js
@@ -5,6 +5,7 @@ import {
__experimentalUnitControl as UnitControl,
__experimentalUseCustomUnits as useCustomUnits,
} from '@wordpress/components';
+import deprecated from '@wordpress/deprecated';
import { __ } from '@wordpress/i18n';
/**
@@ -35,9 +36,25 @@ export default function LetterSpacingControl( {
availableUnits: availableUnits || [ 'px', 'em', 'rem' ],
defaultValues: { px: 2, em: 0.2, rem: 0.2 },
} );
+
+ if (
+ ! __next40pxDefaultSize &&
+ ( otherProps.size === undefined || otherProps.size === 'default' )
+ ) {
+ deprecated(
+ `36px default size for wp.blockEditor.__experimentalLetterSpacingControl`,
+ {
+ since: '6.8',
+ version: '7.1',
+ hint: 'Set the `__next40pxDefaultSize` prop to true to start opting into the new default size, which will become the default in a future version.',
+ }
+ );
+ }
+
return (
(
);
```
diff --git a/packages/block-editor/src/components/line-height-control/index.js b/packages/block-editor/src/components/line-height-control/index.js
index b2c99c03f8784..ea692ceb452e3 100644
--- a/packages/block-editor/src/components/line-height-control/index.js
+++ b/packages/block-editor/src/components/line-height-control/index.js
@@ -3,6 +3,7 @@
*/
import { __ } from '@wordpress/i18n';
import { __experimentalNumberControl as NumberControl } from '@wordpress/components';
+import deprecated from '@wordpress/deprecated';
/**
* Internal dependencies
@@ -89,10 +90,22 @@ const LineHeightControl = ( {
onChange( `${ nextValue }` );
};
+ if (
+ ! __next40pxDefaultSize &&
+ ( otherProps.size === undefined || otherProps.size === 'default' )
+ ) {
+ deprecated( `36px default size for wp.blockEditor.LineHeightControl`, {
+ since: '6.8',
+ version: '7.1',
+ hint: 'Set the `__next40pxDefaultSize` prop to true to start opting into the new default size, which will become the default in a future version.',
+ } );
+ }
+
return (
{
export const Default = Template.bind( {} );
Default.args = {
+ __next40pxDefaultSize: true,
__unstableInputWidth: '100px',
};
diff --git a/packages/block-editor/src/components/line-height-control/test/index.js b/packages/block-editor/src/components/line-height-control/test/index.js
index b98bc93c48a83..488d22b768114 100644
--- a/packages/block-editor/src/components/line-height-control/test/index.js
+++ b/packages/block-editor/src/components/line-height-control/test/index.js
@@ -19,7 +19,13 @@ const SPIN = STEP * SPIN_FACTOR;
const ControlledLineHeightControl = () => {
const [ value, setValue ] = useState();
- return ;
+ return (
+
+ );
};
describe( 'LineHeightControl', () => {
diff --git a/packages/block-editor/src/components/link-control/index.js b/packages/block-editor/src/components/link-control/index.js
index 0f2ae4a0e05d2..74ee2dbfd9a7f 100644
--- a/packages/block-editor/src/components/link-control/index.js
+++ b/packages/block-editor/src/components/link-control/index.js
@@ -34,6 +34,7 @@ import useCreatePage from './use-create-page';
import useInternalValue from './use-internal-value';
import { ViewerFill } from './viewer-slot';
import { DEFAULT_LINK_SETTINGS } from './constants';
+import deprecated from '@wordpress/deprecated';
/**
* Default properties associated with a link control value.
@@ -500,4 +501,13 @@ function LinkControl( {
LinkControl.ViewerFill = ViewerFill;
LinkControl.DEFAULT_LINK_SETTINGS = DEFAULT_LINK_SETTINGS;
+export const DeprecatedExperimentalLinkControl = ( props ) => {
+ deprecated( 'wp.blockEditor.__experimentalLinkControl', {
+ since: '6.8',
+ alternative: 'wp.blockEditor.LinkControl',
+ } );
+
+ return ;
+};
+
export default LinkControl;
diff --git a/packages/block-editor/src/components/link-control/search-input.js b/packages/block-editor/src/components/link-control/search-input.js
index 3f109b8a37155..2debdec296d94 100644
--- a/packages/block-editor/src/components/link-control/search-input.js
+++ b/packages/block-editor/src/components/link-control/search-input.js
@@ -11,6 +11,7 @@ import { URLInput } from '../';
import LinkControlSearchResults from './search-results';
import { CREATE_TYPE } from './constants';
import useSearchHandler from './use-search-handler';
+import deprecated from '@wordpress/deprecated';
// Must be a function as otherwise URLInput will default
// to the fetchLinkSuggestions passed in block editor settings
@@ -156,3 +157,11 @@ const LinkControlSearchInput = forwardRef(
);
export default LinkControlSearchInput;
+
+export const __experimentalLinkControlSearchInput = ( props ) => {
+ deprecated( 'wp.blockEditor.__experimentalLinkControlSearchInput', {
+ since: '6.8',
+ } );
+
+ return ;
+};
diff --git a/packages/block-editor/src/components/link-control/search-item.js b/packages/block-editor/src/components/link-control/search-item.js
index fa8d1540b3dae..27084e5e77b96 100644
--- a/packages/block-editor/src/components/link-control/search-item.js
+++ b/packages/block-editor/src/components/link-control/search-item.js
@@ -17,6 +17,7 @@ import {
import { __unstableStripHTML as stripHTML } from '@wordpress/dom';
import { safeDecodeURI, filterURLForDisplay, getPath } from '@wordpress/url';
import { pipe } from '@wordpress/compose';
+import deprecated from '@wordpress/deprecated';
const ICONS_MAP = {
post: postList,
@@ -160,3 +161,11 @@ function getVisualTypeName( suggestion ) {
}
export default LinkControlSearchItem;
+
+export const __experimentalLinkControlSearchItem = ( props ) => {
+ deprecated( 'wp.blockEditor.__experimentalLinkControlSearchItem', {
+ since: '6.8',
+ } );
+
+ return ;
+};
diff --git a/packages/block-editor/src/components/link-control/search-results.js b/packages/block-editor/src/components/link-control/search-results.js
index 29558f69291c5..6b405b0df1770 100644
--- a/packages/block-editor/src/components/link-control/search-results.js
+++ b/packages/block-editor/src/components/link-control/search-results.js
@@ -15,8 +15,9 @@ import clsx from 'clsx';
import LinkControlSearchCreate from './search-create-button';
import LinkControlSearchItem from './search-item';
import { CREATE_TYPE, LINK_ENTRY_TYPES } from './constants';
+import deprecated from '@wordpress/deprecated';
-export default function LinkControlSearchResults( {
+function LinkControlSearchResults( {
withCreateSuggestion,
currentInputValue,
handleSuggestionClick,
@@ -121,3 +122,13 @@ export default function LinkControlSearchResults( {
);
}
+
+export default LinkControlSearchResults;
+
+export const __experimentalLinkControlSearchResults = ( props ) => {
+ deprecated( 'wp.blockEditor.__experimentalLinkControlSearchResults', {
+ since: '6.8',
+ } );
+
+ return ;
+};
diff --git a/packages/block-editor/src/components/media-placeholder/index.js b/packages/block-editor/src/components/media-placeholder/index.js
index 07a3f8829e71d..0cbc6c8c26203 100644
--- a/packages/block-editor/src/components/media-placeholder/index.js
+++ b/packages/block-editor/src/components/media-placeholder/index.js
@@ -19,7 +19,6 @@ import { __ } from '@wordpress/i18n';
import { useState, useEffect } from '@wordpress/element';
import { useSelect } from '@wordpress/data';
import { keyboardReturn } from '@wordpress/icons';
-import { pasteHandler } from '@wordpress/blocks';
import deprecated from '@wordpress/deprecated';
/**
@@ -29,6 +28,7 @@ import MediaUpload from '../media-upload';
import MediaUploadCheck from '../media-upload/check';
import URLPopover from '../url-popover';
import { store as blockEditorStore } from '../../store';
+import { parseDropEvent } from '../use-on-block-drop';
const noop = () => {};
@@ -229,56 +229,45 @@ export function MediaPlaceholder( {
} );
};
- async function handleBlocksDrop( blocks ) {
- if ( ! blocks || ! Array.isArray( blocks ) ) {
- return;
- }
+ async function handleBlocksDrop( event ) {
+ const { blocks } = parseDropEvent( event );
- function recursivelyFindMediaFromBlocks( _blocks ) {
- return _blocks.flatMap( ( block ) =>
- ( block.name === 'core/image' ||
- block.name === 'core/audio' ||
- block.name === 'core/video' ) &&
- block.attributes.url
- ? [ block ]
- : recursivelyFindMediaFromBlocks( block.innerBlocks )
- );
- }
-
- const mediaBlocks = recursivelyFindMediaFromBlocks( blocks );
-
- if ( ! mediaBlocks.length ) {
+ if ( ! blocks?.length ) {
return;
}
const uploadedMediaList = await Promise.all(
- mediaBlocks.map( ( block ) =>
- block.attributes.id
- ? block.attributes
- : new Promise( ( resolve, reject ) => {
- window
- .fetch( block.attributes.url )
- .then( ( response ) => response.blob() )
- .then( ( blob ) =>
- mediaUpload( {
- filesList: [ blob ],
- additionalData: {
- title: block.attributes.title,
- alt_text: block.attributes.alt,
- caption: block.attributes.caption,
- },
- onFileChange: ( [ media ] ) => {
- if ( media.id ) {
- resolve( media );
- }
- },
- allowedTypes,
- onError: reject,
- } )
- )
- .catch( () => resolve( block.attributes.url ) );
- } )
- )
+ blocks.map( ( block ) => {
+ const blockType = block.name.split( '/' )[ 1 ];
+ if ( block.attributes.id ) {
+ block.attributes.type = blockType;
+ return block.attributes;
+ }
+ return new Promise( ( resolve, reject ) => {
+ window
+ .fetch( block.attributes.url )
+ .then( ( response ) => response.blob() )
+ .then( ( blob ) =>
+ mediaUpload( {
+ filesList: [ blob ],
+ additionalData: {
+ title: block.attributes.title,
+ alt_text: block.attributes.alt,
+ caption: block.attributes.caption,
+ type: blockType,
+ },
+ onFileChange: ( [ media ] ) => {
+ if ( media.id ) {
+ resolve( media );
+ }
+ },
+ allowedTypes,
+ onError: reject,
+ } )
+ )
+ .catch( () => resolve( block.attributes.url ) );
+ } );
+ } )
).catch( ( err ) => onError( err ) );
if ( multiple ) {
@@ -288,11 +277,6 @@ export function MediaPlaceholder( {
}
}
- async function onHTMLDrop( HTML ) {
- const blocks = pasteHandler( { HTML } );
- return await handleBlocksDrop( blocks );
- }
-
const onUpload = ( event ) => {
onFilesUpload( event.target.files );
};
@@ -380,7 +364,24 @@ export function MediaPlaceholder( {
}
return (
-
+ {
+ const prefix = 'wp-block:core/';
+ const types = [];
+ for ( const type of dataTransfer.types ) {
+ if ( type.startsWith( prefix ) ) {
+ types.push( type.slice( prefix.length ) );
+ }
+ }
+ return (
+ types.every( ( type ) =>
+ allowedTypes.includes( type )
+ ) && ( multiple ? true : types.length === 1 )
+ );
+ } }
+ />
);
};
diff --git a/packages/block-editor/src/components/multi-selection-inspector/index.js b/packages/block-editor/src/components/multi-selection-inspector/index.js
index f5e7f69634768..23d890d79fff4 100644
--- a/packages/block-editor/src/components/multi-selection-inspector/index.js
+++ b/packages/block-editor/src/components/multi-selection-inspector/index.js
@@ -3,9 +3,8 @@
*/
import { sprintf, _n } from '@wordpress/i18n';
import { useSelect } from '@wordpress/data';
-import { serialize } from '@wordpress/blocks';
-import { count as wordCount } from '@wordpress/wordcount';
import { copy } from '@wordpress/icons';
+import { __experimentalHStack as HStack } from '@wordpress/components';
/**
* Internal dependencies
@@ -14,33 +13,24 @@ import BlockIcon from '../block-icon';
import { store as blockEditorStore } from '../../store';
export default function MultiSelectionInspector() {
- const { blocks } = useSelect( ( select ) => {
- const { getMultiSelectedBlocks } = select( blockEditorStore );
- return {
- blocks: getMultiSelectedBlocks(),
- };
- }, [] );
- const words = wordCount( serialize( blocks ), 'words' );
-
+ const selectedBlockCount = useSelect(
+ ( select ) => select( blockEditorStore ).getSelectedBlockCount(),
+ []
+ );
return (
-
+
-
-
- { sprintf(
- /* translators: %d: number of blocks */
- _n( '%d Block', '%d Blocks', blocks.length ),
- blocks.length
- ) }
-
-
- { sprintf(
- /* translators: %d: number of words */
- _n( '%d word selected.', '%d words selected.', words ),
- words
- ) }
-
+
+ { sprintf(
+ /* translators: %d: number of blocks */
+ _n( '%d Block', '%d Blocks', selectedBlockCount ),
+ selectedBlockCount
+ ) }
-
+
);
}
diff --git a/packages/block-editor/src/components/multi-selection-inspector/style.scss b/packages/block-editor/src/components/multi-selection-inspector/style.scss
index 61bf5f8cdb382..e37245d58f5dd 100644
--- a/packages/block-editor/src/components/multi-selection-inspector/style.scss
+++ b/packages/block-editor/src/components/multi-selection-inspector/style.scss
@@ -1,25 +1,13 @@
.block-editor-multi-selection-inspector__card {
- display: flex;
- align-items: flex-start;
padding: $grid-unit-20;
}
-.block-editor-multi-selection-inspector__card-content {
- flex-grow: 1;
-}
-
.block-editor-multi-selection-inspector__card-title {
font-weight: 500;
- margin-bottom: 5px;
-}
-
-.block-editor-multi-selection-inspector__card-description {
- font-size: $default-font-size;
}
.block-editor-multi-selection-inspector__card .block-editor-block-icon {
margin-left: -2px;
- margin-right: 10px;
padding: 0 3px;
width: $button-size;
height: $button-size-small;
diff --git a/packages/block-editor/src/components/provider/index.js b/packages/block-editor/src/components/provider/index.js
index abbb122ae3a0e..97aa0b9521687 100644
--- a/packages/block-editor/src/components/provider/index.js
+++ b/packages/block-editor/src/components/provider/index.js
@@ -2,8 +2,13 @@
* WordPress dependencies
*/
import { useDispatch } from '@wordpress/data';
-import { useEffect } from '@wordpress/element';
+import { useEffect, useMemo } from '@wordpress/element';
import { SlotFillProvider } from '@wordpress/components';
+//eslint-disable-next-line import/no-extraneous-dependencies -- Experimental package, not published.
+import {
+ MediaUploadProvider,
+ store as uploadStore,
+} from '@wordpress/upload-media';
/**
* Internal dependencies
@@ -14,12 +19,71 @@ import { store as blockEditorStore } from '../../store';
import { BlockRefsProvider } from './block-refs-provider';
import { unlock } from '../../lock-unlock';
import KeyboardShortcuts from '../keyboard-shortcuts';
+import useMediaUploadSettings from './use-media-upload-settings';
/** @typedef {import('@wordpress/data').WPDataRegistry} WPDataRegistry */
+const noop = () => {};
+
+/**
+ * Upload a media file when the file upload button is activated
+ * or when adding a file to the editor via drag & drop.
+ *
+ * @param {WPDataRegistry} registry
+ * @param {Object} $3 Parameters object passed to the function.
+ * @param {Array} $3.allowedTypes Array with the types of media that can be uploaded, if unset all types are allowed.
+ * @param {Object} $3.additionalData Additional data to include in the request.
+ * @param {Array
} $3.filesList List of files.
+ * @param {Function} $3.onError Function called when an error happens.
+ * @param {Function} $3.onFileChange Function called each time a file or a temporary representation of the file is available.
+ * @param {Function} $3.onSuccess Function called once a file has completely finished uploading, including thumbnails.
+ * @param {Function} $3.onBatchSuccess Function called once all files in a group have completely finished uploading, including thumbnails.
+ */
+function mediaUpload(
+ registry,
+ {
+ allowedTypes,
+ additionalData = {},
+ filesList,
+ onError = noop,
+ onFileChange,
+ onSuccess,
+ onBatchSuccess,
+ }
+) {
+ void registry.dispatch( uploadStore ).addItems( {
+ files: filesList,
+ onChange: onFileChange,
+ onSuccess,
+ onBatchSuccess,
+ onError: ( { message } ) => onError( message ),
+ additionalData,
+ allowedTypes,
+ } );
+}
+
export const ExperimentalBlockEditorProvider = withRegistryProvider(
( props ) => {
- const { children, settings, stripExperimentalSettings = false } = props;
+ const {
+ settings: _settings,
+ registry,
+ stripExperimentalSettings = false,
+ } = props;
+
+ const mediaUploadSettings = useMediaUploadSettings( _settings );
+
+ let settings = _settings;
+
+ if ( window.__experimentalMediaProcessing && _settings.mediaUpload ) {
+ // Create a new variable so that the original props.settings.mediaUpload is not modified.
+ settings = useMemo(
+ () => ( {
+ ..._settings,
+ mediaUpload: mediaUpload.bind( null, registry ),
+ } ),
+ [ _settings, registry ]
+ );
+ }
const { __experimentalUpdateSettings } = unlock(
useDispatch( blockEditorStore )
@@ -44,12 +108,25 @@ export const ExperimentalBlockEditorProvider = withRegistryProvider(
// Syncs the entity provider with changes in the block-editor store.
useBlockSync( props );
- return (
+ const children = (
{ ! settings?.isPreviewMode && }
- { children }
+ { props.children }
);
+
+ if ( window.__experimentalMediaProcessing ) {
+ return (
+
+ { children }
+
+ );
+ }
+
+ return children;
}
);
diff --git a/packages/block-editor/src/components/provider/test/use-block-sync.js b/packages/block-editor/src/components/provider/test/use-block-sync.js
index aae5e517c6302..b2afdb942e66f 100644
--- a/packages/block-editor/src/components/provider/test/use-block-sync.js
+++ b/packages/block-editor/src/components/provider/test/use-block-sync.js
@@ -22,7 +22,9 @@ jest.mock( '../../../store/actions', () => {
...actions,
resetBlocks: jest.fn( actions.resetBlocks ),
replaceInnerBlocks: jest.fn( actions.replaceInnerBlocks ),
- setHasControlledInnerBlocks: jest.fn( actions.replaceInnerBlocks ),
+ setHasControlledInnerBlocks: jest.fn(
+ actions.setHasControlledInnerBlocks
+ ),
};
} );
diff --git a/packages/block-editor/src/components/provider/use-media-upload-settings.js b/packages/block-editor/src/components/provider/use-media-upload-settings.js
new file mode 100644
index 0000000000000..486066c7aa730
--- /dev/null
+++ b/packages/block-editor/src/components/provider/use-media-upload-settings.js
@@ -0,0 +1,25 @@
+/**
+ * WordPress dependencies
+ */
+import { useMemo } from '@wordpress/element';
+
+/**
+ * React hook used to compute the media upload settings to use in the post editor.
+ *
+ * @param {Object} settings Media upload settings prop.
+ *
+ * @return {Object} Media upload settings.
+ */
+function useMediaUploadSettings( settings ) {
+ return useMemo(
+ () => ( {
+ mediaUpload: settings.mediaUpload,
+ mediaSideload: settings.mediaSideload,
+ maxUploadFileSize: settings.maxUploadFileSize,
+ allowedMimeTypes: settings.allowedMimeTypes,
+ } ),
+ [ settings ]
+ );
+}
+
+export default useMediaUploadSettings;
diff --git a/packages/block-editor/src/components/resolution-tool/stories/index.story.js b/packages/block-editor/src/components/resolution-tool/stories/index.story.js
index ed598acd4df98..3fedb6d6facae 100644
--- a/packages/block-editor/src/components/resolution-tool/stories/index.story.js
+++ b/packages/block-editor/src/components/resolution-tool/stories/index.story.js
@@ -16,7 +16,7 @@ export default {
title: 'BlockEditor (Private APIs)/ResolutionControl',
component: ResolutionTool,
argTypes: {
- panelId: { control: { type: null } },
+ panelId: { control: false },
onChange: { action: 'changed' },
},
};
diff --git a/packages/block-editor/src/components/rich-text/content.scss b/packages/block-editor/src/components/rich-text/content.scss
index 6f118479fc6b0..67e14b0882d7e 100644
--- a/packages/block-editor/src/components/rich-text/content.scss
+++ b/packages/block-editor/src/components/rich-text/content.scss
@@ -13,16 +13,6 @@
&:focus {
// Removes outline added by the browser.
outline: none;
-
- [data-rich-text-format-boundary] {
- border-radius: $radius-small;
- }
- }
-}
-
-.block-editor-rich-text__editable {
- > p:first-child {
- margin-top: 0;
}
}
@@ -40,3 +30,18 @@ figcaption.block-editor-rich-text__editable [data-rich-text-placeholder]::before
background: rgb(255, 255, 0);
}
}
+
+[data-rich-text-comment],
+[data-rich-text-format-boundary] {
+ border-radius: $radius-small;
+}
+
+[data-rich-text-comment] {
+ background-color: currentColor;
+
+ span {
+ filter: invert(100%);
+ color: currentColor;
+ padding: 0 2px;
+ }
+}
diff --git a/packages/block-editor/src/components/rich-text/index.js b/packages/block-editor/src/components/rich-text/index.js
index 8f179d08570ad..768ffbb0cdd2d 100644
--- a/packages/block-editor/src/components/rich-text/index.js
+++ b/packages/block-editor/src/components/rich-text/index.js
@@ -39,7 +39,7 @@ import FormatEdit from './format-edit';
import { getAllowedFormats } from './utils';
import { Content, valueToHTMLString } from './content';
import { withDeprecations } from './with-deprecations';
-import { canBindBlock } from '../../hooks/use-bindings-attributes';
+import { canBindBlock } from '../../utils/block-bindings';
import BlockContext from '../block-context';
export const keyboardShortcutContext = createContext();
@@ -431,6 +431,11 @@ export function RichTextWrapper(
aria-multiline={ ! disableLineBreaks }
aria-readonly={ shouldDisableEditing }
{ ...props }
+ // Unset draggable (coming from block props) for contentEditable
+ // elements because it will interfere with multi block selection
+ // when the contentEditable and draggable elements are the same
+ // element.
+ draggable={ undefined }
aria-label={
bindingsLabel || props[ 'aria-label' ] || placeholder
}
diff --git a/packages/block-editor/src/components/spacing-sizes-control/style.scss b/packages/block-editor/src/components/spacing-sizes-control/style.scss
index a387e5369d01e..26f3dc586bb54 100644
--- a/packages/block-editor/src/components/spacing-sizes-control/style.scss
+++ b/packages/block-editor/src/components/spacing-sizes-control/style.scss
@@ -4,40 +4,11 @@
margin-bottom: 0;
}
- .is-marked {
- .components-range-control__track {
- transition: width ease 0.1s;
- @include reduce-motion("transition");
- }
-
- .components-range-control__thumb-wrapper {
- transition: left ease 0.1s;
- @include reduce-motion("transition");
- }
- }
-
.spacing-sizes-control__range-control,
.spacing-sizes-control__custom-value-range {
flex: 1;
margin-bottom: 0; // Needed for some instances of the range control, such as the Spacer block.
}
-
- .components-range-control__mark {
- transform: translateX(-50%);
- height: $grid-unit-05;
- width: math.div($grid-unit-05, 2);
- background-color: $white;
- z-index: 1;
- top: -#{$grid-unit-05};
- }
-
- .components-range-control__marks {
- margin-top: 17px;
- }
-
- .components-range-control__thumb-wrapper {
- z-index: 3;
- }
}
.spacing-sizes-control__header {
diff --git a/packages/block-editor/src/components/text-alignment-control/stories/index.story.js b/packages/block-editor/src/components/text-alignment-control/stories/index.story.js
index b2c171497acb0..fd97f9b60e6a9 100644
--- a/packages/block-editor/src/components/text-alignment-control/stories/index.story.js
+++ b/packages/block-editor/src/components/text-alignment-control/stories/index.story.js
@@ -8,32 +8,69 @@ import { useState } from '@wordpress/element';
*/
import TextAlignmentControl from '../';
-export default {
+const meta = {
title: 'BlockEditor/TextAlignmentControl',
component: TextAlignmentControl,
+ parameters: {
+ docs: {
+ canvas: { sourceState: 'shown' },
+ description: {
+ component: 'Control to facilitate text alignment selections.',
+ },
+ },
+ },
argTypes: {
- onChange: { action: 'onChange' },
- className: { control: 'text' },
+ value: {
+ control: { type: null },
+ description: 'Currently selected text alignment value.',
+ table: {
+ type: {
+ summary: 'string',
+ },
+ },
+ },
+ onChange: {
+ action: 'onChange',
+ control: { type: null },
+ description: 'Handles change in text alignment selection.',
+ table: {
+ type: {
+ summary: 'function',
+ },
+ },
+ },
options: {
control: 'check',
+ description: 'Array of text alignment options to display.',
options: [ 'left', 'center', 'right', 'justify' ],
+ table: {
+ type: { summary: 'array' },
+ },
+ },
+ className: {
+ control: 'text',
+ description: 'Class name to add to the control.',
+ table: {
+ type: { summary: 'string' },
+ },
},
- value: { control: { type: null } },
},
};
-const Template = ( { onChange, ...args } ) => {
- const [ value, setValue ] = useState();
- return (
- {
- onChange( ...changeArgs );
- setValue( ...changeArgs );
- } }
- value={ value }
- />
- );
-};
+export default meta;
-export const Default = Template.bind( {} );
+export const Default = {
+ render: function Template( { onChange, ...args } ) {
+ const [ value, setValue ] = useState();
+ return (
+ {
+ onChange( ...changeArgs );
+ setValue( ...changeArgs );
+ } }
+ value={ value }
+ />
+ );
+ },
+};
diff --git a/packages/block-editor/src/components/use-block-drop-zone/index.js b/packages/block-editor/src/components/use-block-drop-zone/index.js
index 2a3e4948d40b3..529eb199fb76a 100644
--- a/packages/block-editor/src/components/use-block-drop-zone/index.js
+++ b/packages/block-editor/src/components/use-block-drop-zone/index.js
@@ -332,6 +332,7 @@ export default function useBlockDropZone( {
isGroupable,
isZoomOut,
getSectionRootClientId,
+ getBlockParents,
} = unlock( useSelect( blockEditorStore ) );
const {
showInsertionPoint,
@@ -358,13 +359,29 @@ export default function useBlockDropZone( {
// So, ensure that the drag state is set when the user drags over a drop zone.
startDragging();
}
+
+ const draggedBlockClientIds = getDraggedBlockClientIds();
+ const targetParents = [
+ targetRootClientId,
+ ...getBlockParents( targetRootClientId, true ),
+ ];
+
+ // Check if the target is within any of the dragged blocks.
+ const isTargetWithinDraggedBlocks = draggedBlockClientIds.some(
+ ( clientId ) => targetParents.includes( clientId )
+ );
+
+ if ( isTargetWithinDraggedBlocks ) {
+ return;
+ }
+
const allowedBlocks = getAllowedBlocks( targetRootClientId );
const targetBlockName = getBlockNamesByClientId( [
targetRootClientId,
] )[ 0 ];
const draggedBlockNames = getBlockNamesByClientId(
- getDraggedBlockClientIds()
+ draggedBlockClientIds
);
const isBlockDroppingAllowed = isDropTargetValid(
getBlockType,
@@ -439,7 +456,14 @@ export default function useBlockDropZone( {
const [ targetIndex, operation, nearestSide ] =
dropTargetPosition;
- if ( isZoomOut() && operation !== 'insert' ) {
+ const isTargetIndexEmptyDefaultBlock =
+ blocksData[ targetIndex ]?.isUnmodifiedDefaultBlock;
+
+ if (
+ isZoomOut() &&
+ ! isTargetIndexEmptyDefaultBlock &&
+ operation !== 'insert'
+ ) {
return;
}
diff --git a/packages/block-editor/src/components/use-moving-animation/index.js b/packages/block-editor/src/components/use-moving-animation/index.js
index 602b683150d0c..b11710acd2433 100644
--- a/packages/block-editor/src/components/use-moving-animation/index.js
+++ b/packages/block-editor/src/components/use-moving-animation/index.js
@@ -52,6 +52,7 @@ function useMovingAnimation( { triggerAnimationOnChange, clientId } ) {
isFirstMultiSelectedBlock,
isBlockMultiSelected,
isAncestorMultiSelected,
+ isDraggingBlocks,
} = useSelect( blockEditorStore );
// Whenever the trigger changes, we need to take a snapshot of the current
@@ -73,8 +74,14 @@ function useMovingAnimation( { triggerAnimationOnChange, clientId } ) {
const isSelected = isBlockSelected( clientId );
const adjustScrolling =
isSelected || isFirstMultiSelectedBlock( clientId );
+ const isDragging = isDraggingBlocks();
function preserveScrollPosition() {
+ // The user already scrolled when dragging blocks.
+ if ( isDragging ) {
+ return;
+ }
+
if ( adjustScrolling && prevRect ) {
const blockRect = ref.current.getBoundingClientRect();
const diff = blockRect.top - prevRect.top;
@@ -107,6 +114,13 @@ function useMovingAnimation( { triggerAnimationOnChange, clientId } ) {
isSelected ||
isBlockMultiSelected( clientId ) ||
isAncestorMultiSelected( clientId );
+
+ // The user already dragged the blocks to the new position, so don't
+ // animate the dragged blocks.
+ if ( isPartOfSelection && isDragging ) {
+ return;
+ }
+
// Make sure the other blocks move under the selected block(s).
const zIndex = isPartOfSelection ? '1' : '';
@@ -153,6 +167,7 @@ function useMovingAnimation( { triggerAnimationOnChange, clientId } ) {
isFirstMultiSelectedBlock,
isBlockMultiSelected,
isAncestorMultiSelected,
+ isDraggingBlocks,
] );
return ref;
diff --git a/packages/block-editor/src/components/use-resize-canvas/index.js b/packages/block-editor/src/components/use-resize-canvas/index.js
index 3b4d97a097964..0aa46c9c3278f 100644
--- a/packages/block-editor/src/components/use-resize-canvas/index.js
+++ b/packages/block-editor/src/components/use-resize-canvas/index.js
@@ -60,7 +60,7 @@ export default function useResizeCanvas( deviceType ) {
marginLeft: marginHorizontal,
marginRight: marginHorizontal,
height,
- overflowY: 'auto',
+ maxWidth: '100%',
};
default:
return {
diff --git a/packages/block-editor/src/components/warning/index.js b/packages/block-editor/src/components/warning/index.js
index 8b6279bef6044..17a014107b43a 100644
--- a/packages/block-editor/src/components/warning/index.js
+++ b/packages/block-editor/src/components/warning/index.js
@@ -6,7 +6,6 @@ import clsx from 'clsx';
/**
* WordPress dependencies
*/
-import { Children } from '@wordpress/element';
import { DropdownMenu, MenuGroup, MenuItem } from '@wordpress/components';
import { __ } from '@wordpress/i18n';
import { moreVertical } from '@wordpress/icons';
@@ -20,10 +19,10 @@ function Warning( { className, actions, children, secondaryActions } ) {
{ children }
- { ( Children.count( actions ) > 0 || secondaryActions ) && (
+ { ( actions?.length > 0 || secondaryActions ) && (
- { Children.count( actions ) > 0 &&
- Children.map( actions, ( action, i ) => (
+ { actions?.length > 0 &&
+ actions.map( ( action, i ) => (
{
it( 'should show primary actions', () => {
render(
- Click me }>Message
+ Click me ] }>
+ Message
+
);
expect(
diff --git a/packages/block-editor/src/components/writing-flow/use-drag-selection.js b/packages/block-editor/src/components/writing-flow/use-drag-selection.js
index 1569c45a7c676..ea4c09b3dc957 100644
--- a/packages/block-editor/src/components/writing-flow/use-drag-selection.js
+++ b/packages/block-editor/src/components/writing-flow/use-drag-selection.js
@@ -80,7 +80,17 @@ export default function useDragSelection() {
} );
}
+ let lastMouseDownTarget;
+
+ function onMouseDown( { target } ) {
+ lastMouseDownTarget = target;
+ }
+
function onMouseLeave( { buttons, target, relatedTarget } ) {
+ if ( ! target.contains( lastMouseDownTarget ) ) {
+ return;
+ }
+
// If we're moving into a child element, ignore. We're tracking
// the mouse leaving the element to a parent, no a child.
if ( target.contains( relatedTarget ) ) {
@@ -141,6 +151,7 @@ export default function useDragSelection() {
}
node.addEventListener( 'mouseout', onMouseLeave );
+ node.addEventListener( 'mousedown', onMouseDown );
return () => {
node.removeEventListener( 'mouseout', onMouseLeave );
diff --git a/packages/block-editor/src/components/writing-flow/use-tab-nav.js b/packages/block-editor/src/components/writing-flow/use-tab-nav.js
index 16a18358fb2ed..46c40d56fe96d 100644
--- a/packages/block-editor/src/components/writing-flow/use-tab-nav.js
+++ b/packages/block-editor/src/components/writing-flow/use-tab-nav.js
@@ -35,6 +35,11 @@ export default function useTabNav() {
const noCaptureRef = useRef();
function onFocusCapture( event ) {
+ const canvasElement =
+ container.current.ownerDocument === event.target.ownerDocument
+ ? container.current
+ : container.current.ownerDocument.defaultView.frameElement;
+
// Do not capture incoming focus if set by us in WritingFlow.
if ( noCaptureRef.current ) {
noCaptureRef.current = null;
@@ -64,17 +69,15 @@ export default function useTabNav() {
.focus();
}
// If we don't have any section blocks, focus the section root.
- else {
+ else if ( sectionRootClientId ) {
container.current
.querySelector( `[data-block="${ sectionRootClientId }"]` )
.focus();
+ } else {
+ // If we don't have any section root, focus the canvas.
+ canvasElement.focus();
}
} else {
- const canvasElement =
- container.current.ownerDocument === event.target.ownerDocument
- ? container.current
- : container.current.ownerDocument.defaultView.frameElement;
-
const isBefore =
// eslint-disable-next-line no-bitwise
event.target.compareDocumentPosition( canvasElement ) &
diff --git a/packages/block-editor/src/components/writing-mode-control/stories/index.story.js b/packages/block-editor/src/components/writing-mode-control/stories/index.story.js
new file mode 100644
index 0000000000000..ea4bd65a37a00
--- /dev/null
+++ b/packages/block-editor/src/components/writing-mode-control/stories/index.story.js
@@ -0,0 +1,56 @@
+/**
+ * WordPress dependencies
+ */
+import { useState } from '@wordpress/element';
+
+/**
+ * Internal dependencies
+ */
+import WritingModeControl from '../';
+
+const meta = {
+ title: 'BlockEditor/WritingModeControl',
+ component: WritingModeControl,
+ parameters: {
+ docs: {
+ canvas: { sourceState: 'shown' },
+ description: {
+ component: 'Control to facilitate writing mode selections.',
+ },
+ },
+ },
+ argTypes: {
+ value: {
+ control: { type: null },
+ description: 'Currently selected writing mode.',
+ },
+ className: {
+ control: 'text',
+ description: 'Class name to add to the control.',
+ },
+ onChange: {
+ action: 'onChange',
+ control: { type: null },
+ description: 'Handles change in the writing mode selection.',
+ },
+ },
+};
+
+export default meta;
+
+export const Default = {
+ render: function Template( { onChange, ...args } ) {
+ const [ value, setValue ] = useState();
+
+ return (
+ {
+ onChange( ...changeArgs );
+ setValue( ...changeArgs );
+ } }
+ value={ value }
+ />
+ );
+ },
+};
diff --git a/packages/block-editor/src/hooks/block-bindings.js b/packages/block-editor/src/hooks/block-bindings.js
index 615804a311c0f..11e17aba3b30d 100644
--- a/packages/block-editor/src/hooks/block-bindings.js
+++ b/packages/block-editor/src/hooks/block-bindings.js
@@ -26,12 +26,12 @@ import { useViewportMatch } from '@wordpress/compose';
import {
canBindAttribute,
getBindableAttributes,
-} from '../hooks/use-bindings-attributes';
+ useBlockBindingsUtils,
+} from '../utils/block-bindings';
import { unlock } from '../lock-unlock';
import InspectorControls from '../components/inspector-controls';
import BlockContext from '../components/block-context';
import { useBlockEditContext } from '../components/block-edit';
-import { useBlockBindingsUtils } from '../utils/block-bindings';
import { store as blockEditorStore } from '../store';
const { Menu } = unlock( componentsPrivateApis );
@@ -51,7 +51,7 @@ const useToolsPanelDropdownMenuProps = () => {
: {};
};
-function BlockBindingsPanelDropdown( { fieldsList, attribute, binding } ) {
+function BlockBindingsPanelMenuContent( { fieldsList, attribute, binding } ) {
const { clientId } = useBlockEditContext();
const registeredSources = getBlockBindingsSources();
const { updateBlockBindings } = useBlockBindingsUtils();
@@ -179,22 +179,21 @@ function EditableBlockBindingsPanelItems( {
placement={
isMobile ? 'bottom-start' : 'left-start'
}
- gutter={ isMobile ? 8 : 36 }
- trigger={
- -
-
-
- }
>
-
+ }>
+
+
+
+
+
);
@@ -300,13 +299,17 @@ export const BlockBindingsPanel = ( { name: blockName, metadata } ) => {
/>
) }
-
-
+ { /*
+ Use a div element to make the ToolsPanelHiddenInnerWrapper
+ toggle the visibility of this help text automatically.
+ */ }
+
+
{ __(
'Attributes connected to custom fields or other dynamic data.'
) }
-
-
+
+
);
diff --git a/packages/block-editor/src/hooks/border.js b/packages/block-editor/src/hooks/border.js
index 4ab4c69a41f31..14b3dbf7669b3 100644
--- a/packages/block-editor/src/hooks/border.js
+++ b/packages/block-editor/src/hooks/border.js
@@ -31,7 +31,7 @@ import {
import { store as blockEditorStore } from '../store';
import { __ } from '@wordpress/i18n';
-export const BORDER_SUPPORT_KEY = '__experimentalBorder';
+export const BORDER_SUPPORT_KEY = 'border';
export const SHADOW_SUPPORT_KEY = 'shadow';
const getColorByProperty = ( colors, property, value ) => {
@@ -161,14 +161,8 @@ export function BorderPanel( { clientId, name, setAttributes, settings } ) {
}
const defaultControls = {
- ...getBlockSupport( name, [
- BORDER_SUPPORT_KEY,
- '__experimentalDefaultControls',
- ] ),
- ...getBlockSupport( name, [
- SHADOW_SUPPORT_KEY,
- '__experimentalDefaultControls',
- ] ),
+ ...getBlockSupport( name, [ BORDER_SUPPORT_KEY, 'defaultControls' ] ),
+ ...getBlockSupport( name, [ SHADOW_SUPPORT_KEY, 'defaultControls' ] ),
};
return (
diff --git a/packages/block-editor/src/hooks/color.js b/packages/block-editor/src/hooks/color.js
index ef8984c936785..2fecc10a31198 100644
--- a/packages/block-editor/src/hooks/color.js
+++ b/packages/block-editor/src/hooks/color.js
@@ -290,7 +290,7 @@ export function ColorEdit( { clientId, name, setAttributes, settings } ) {
const defaultControls = getBlockSupport( name, [
COLOR_SUPPORT_KEY,
- '__experimentalDefaultControls',
+ 'defaultControls',
] );
const enableContrastChecking =
diff --git a/packages/block-editor/src/hooks/dimensions.js b/packages/block-editor/src/hooks/dimensions.js
index ffa4048b7740e..c98cc34e4272c 100644
--- a/packages/block-editor/src/hooks/dimensions.js
+++ b/packages/block-editor/src/hooks/dimensions.js
@@ -88,11 +88,11 @@ export function DimensionsPanel( { clientId, name, setAttributes, settings } ) {
const defaultDimensionsControls = getBlockSupport( name, [
DIMENSIONS_SUPPORT_KEY,
- '__experimentalDefaultControls',
+ 'defaultControls',
] );
const defaultSpacingControls = getBlockSupport( name, [
SPACING_SUPPORT_KEY,
- '__experimentalDefaultControls',
+ 'defaultControls',
] );
const defaultControls = {
...defaultDimensionsControls,
diff --git a/packages/block-editor/src/hooks/gap.js b/packages/block-editor/src/hooks/gap.js
index debe1ad169078..887325e6409dd 100644
--- a/packages/block-editor/src/hooks/gap.js
+++ b/packages/block-editor/src/hooks/gap.js
@@ -27,7 +27,7 @@ export function getGapBoxControlValueFromStyle( blockGapValue ) {
* Returns a CSS value for the `gap` property from a given blockGap style.
*
* @param {string? | Object?} blockGapValue A block gap string or axial object value, e.g., '10px' or { top: '10px', left: '10px'}.
- * @param {string?} defaultValue A default gap value.
+ * @param {?string} defaultValue A default gap value.
* @return {string|null} The concatenated gap value (row and column).
*/
export function getGapCSSValue( blockGapValue, defaultValue = '0' ) {
diff --git a/packages/block-editor/src/hooks/index.js b/packages/block-editor/src/hooks/index.js
index 66ff60b691b66..7f9b29376ad1f 100644
--- a/packages/block-editor/src/hooks/index.js
+++ b/packages/block-editor/src/hooks/index.js
@@ -32,7 +32,6 @@ import './metadata';
import blockHooks from './block-hooks';
import blockBindingsPanel from './block-bindings';
import './block-renaming';
-import './use-bindings-attributes';
import './grid-visualizer';
createBlockEditFilter(
diff --git a/packages/block-editor/src/hooks/style.js b/packages/block-editor/src/hooks/style.js
index 998d13cfd2224..5be2b1b3fd40a 100644
--- a/packages/block-editor/src/hooks/style.js
+++ b/packages/block-editor/src/hooks/style.js
@@ -98,22 +98,16 @@ function addAttribute( settings ) {
* @type {Record}
*/
const skipSerializationPathsEdit = {
- [ `${ BORDER_SUPPORT_KEY }.__experimentalSkipSerialization` ]: [ 'border' ],
- [ `${ COLOR_SUPPORT_KEY }.__experimentalSkipSerialization` ]: [
- COLOR_SUPPORT_KEY,
- ],
- [ `${ TYPOGRAPHY_SUPPORT_KEY }.__experimentalSkipSerialization` ]: [
+ [ `${ BORDER_SUPPORT_KEY }.skipSerialization` ]: [ 'border' ],
+ [ `${ COLOR_SUPPORT_KEY }.skipSerialization` ]: [ COLOR_SUPPORT_KEY ],
+ [ `${ TYPOGRAPHY_SUPPORT_KEY }.skipSerialization` ]: [
TYPOGRAPHY_SUPPORT_KEY,
],
- [ `${ DIMENSIONS_SUPPORT_KEY }.__experimentalSkipSerialization` ]: [
+ [ `${ DIMENSIONS_SUPPORT_KEY }.skipSerialization` ]: [
DIMENSIONS_SUPPORT_KEY,
],
- [ `${ SPACING_SUPPORT_KEY }.__experimentalSkipSerialization` ]: [
- SPACING_SUPPORT_KEY,
- ],
- [ `${ SHADOW_SUPPORT_KEY }.__experimentalSkipSerialization` ]: [
- SHADOW_SUPPORT_KEY,
- ],
+ [ `${ SPACING_SUPPORT_KEY }.skipSerialization` ]: [ SPACING_SUPPORT_KEY ],
+ [ `${ SHADOW_SUPPORT_KEY }.skipSerialization` ]: [ SHADOW_SUPPORT_KEY ],
};
/**
@@ -251,7 +245,7 @@ export function omitStyle( style, paths, preserveReference = false ) {
let newStyle = style;
if ( ! preserveReference ) {
- newStyle = JSON.parse( JSON.stringify( style ) );
+ newStyle = structuredClone( style );
}
if ( ! Array.isArray( paths ) ) {
diff --git a/packages/block-editor/src/hooks/supports.js b/packages/block-editor/src/hooks/supports.js
index c0b6bb2cc8b27..102b78bbb96e6 100644
--- a/packages/block-editor/src/hooks/supports.js
+++ b/packages/block-editor/src/hooks/supports.js
@@ -6,7 +6,7 @@ import { Platform } from '@wordpress/element';
const ALIGN_SUPPORT_KEY = 'align';
const ALIGN_WIDE_SUPPORT_KEY = 'alignWide';
-const BORDER_SUPPORT_KEY = '__experimentalBorder';
+const BORDER_SUPPORT_KEY = 'border';
const COLOR_SUPPORT_KEY = 'color';
const CUSTOM_CLASS_NAME_SUPPORT_KEY = 'customClassName';
const FONT_FAMILY_SUPPORT_KEY = 'typography.fontFamily';
diff --git a/packages/block-editor/src/hooks/test/font-size.js b/packages/block-editor/src/hooks/test/font-size.js
index 11cd024bf8a28..dc3fc9100e475 100644
--- a/packages/block-editor/src/hooks/test/font-size.js
+++ b/packages/block-editor/src/hooks/test/font-size.js
@@ -19,6 +19,15 @@ import {
import _fontSize from '../font-size';
const noop = () => {};
+const EMPTY_ARRAY = [];
+const EMPTY_OBJECT = {};
+const fontSizes = [
+ {
+ name: 'A larger font',
+ size: '32px',
+ slug: 'larger',
+ },
+];
function addUseSettingFilter( callback ) {
addFilter(
@@ -55,13 +64,7 @@ describe( 'useBlockProps', () => {
registerBlockType( blockSettings.name, blockSettings );
addUseSettingFilter( ( result, path ) => {
if ( 'typography.fontSizes' === path ) {
- return [
- {
- name: 'A larger font',
- size: '32px',
- slug: 'larger',
- },
- ];
+ return fontSizes;
}
if ( 'typography.fluid' === path ) {
@@ -69,7 +72,7 @@ describe( 'useBlockProps', () => {
}
if ( 'layout' === path ) {
- return {};
+ return EMPTY_OBJECT;
}
return result;
@@ -95,7 +98,7 @@ describe( 'useBlockProps', () => {
registerBlockType( blockSettings.name, blockSettings );
addUseSettingFilter( ( result, path ) => {
if ( 'typography.fontSizes' === path ) {
- return [];
+ return EMPTY_ARRAY;
}
if ( 'typography.fluid' === path ) {
@@ -103,7 +106,7 @@ describe( 'useBlockProps', () => {
}
if ( 'layout' === path ) {
- return {};
+ return EMPTY_OBJECT;
}
return result;
@@ -132,7 +135,7 @@ describe( 'useBlockProps', () => {
registerBlockType( blockSettings.name, blockSettings );
addUseSettingFilter( ( result, path ) => {
if ( 'typography.fontSizes' === path ) {
- return [];
+ return EMPTY_ARRAY;
}
if ( 'typography.fluid' === path ) {
@@ -140,7 +143,7 @@ describe( 'useBlockProps', () => {
}
if ( 'layout' === path ) {
- return {};
+ return EMPTY_OBJECT;
}
return result;
diff --git a/packages/block-editor/src/hooks/test/style.js b/packages/block-editor/src/hooks/test/style.js
index 2cfe299b8c8d9..40e7169194b82 100644
--- a/packages/block-editor/src/hooks/test/style.js
+++ b/packages/block-editor/src/hooks/test/style.js
@@ -133,8 +133,7 @@ describe( 'addSaveProps', () => {
const applySkipSerialization = ( features ) => {
const updatedSettings = { ...blockSettings };
Object.keys( features ).forEach( ( key ) => {
- updatedSettings.supports[ key ].__experimentalSkipSerialization =
- features[ key ];
+ updatedSettings.supports[ key ].skipSerialization = features[ key ];
} );
return updatedSettings;
};
diff --git a/packages/block-editor/src/hooks/typography.js b/packages/block-editor/src/hooks/typography.js
index ab9a464fe5efb..160894eac4e61 100644
--- a/packages/block-editor/src/hooks/typography.js
+++ b/packages/block-editor/src/hooks/typography.js
@@ -133,7 +133,7 @@ export function TypographyPanel( { clientId, name, setAttributes, settings } ) {
const defaultControls = getBlockSupport( name, [
TYPOGRAPHY_SUPPORT_KEY,
- '__experimentalDefaultControls',
+ 'defaultControls',
] );
return (
diff --git a/packages/block-editor/src/hooks/use-bindings-attributes.js b/packages/block-editor/src/hooks/use-bindings-attributes.js
deleted file mode 100644
index fdc617fda20c0..0000000000000
--- a/packages/block-editor/src/hooks/use-bindings-attributes.js
+++ /dev/null
@@ -1,322 +0,0 @@
-/**
- * WordPress dependencies
- */
-import { store as blocksStore } from '@wordpress/blocks';
-import { createHigherOrderComponent } from '@wordpress/compose';
-import { useRegistry, useSelect } from '@wordpress/data';
-import { useCallback, useMemo, useContext } from '@wordpress/element';
-import { addFilter } from '@wordpress/hooks';
-
-/**
- * Internal dependencies
- */
-import isURLLike from '../components/link-control/is-url-like';
-import { unlock } from '../lock-unlock';
-import BlockContext from '../components/block-context';
-
-/** @typedef {import('@wordpress/compose').WPHigherOrderComponent} WPHigherOrderComponent */
-/** @typedef {import('@wordpress/blocks').WPBlockSettings} WPBlockSettings */
-
-/**
- * Given a binding of block attributes, returns a higher order component that
- * overrides its `attributes` and `setAttributes` props to sync any changes needed.
- *
- * @return {WPHigherOrderComponent} Higher-order component.
- */
-
-const BLOCK_BINDINGS_ALLOWED_BLOCKS = {
- 'core/paragraph': [ 'content' ],
- 'core/heading': [ 'content' ],
- 'core/image': [ 'id', 'url', 'title', 'alt' ],
- 'core/button': [ 'url', 'text', 'linkTarget', 'rel' ],
-};
-
-const DEFAULT_ATTRIBUTE = '__default';
-
-/**
- * Returns the bindings with the `__default` binding for pattern overrides
- * replaced with the full-set of supported attributes. e.g.:
- *
- * bindings passed in: `{ __default: { source: 'core/pattern-overrides' } }`
- * bindings returned: `{ content: { source: 'core/pattern-overrides' } }`
- *
- * @param {string} blockName The block name (e.g. 'core/paragraph').
- * @param {Object} bindings A block's bindings from the metadata attribute.
- *
- * @return {Object} The bindings with default replaced for pattern overrides.
- */
-function replacePatternOverrideDefaultBindings( blockName, bindings ) {
- // The `__default` binding currently only works for pattern overrides.
- if (
- bindings?.[ DEFAULT_ATTRIBUTE ]?.source === 'core/pattern-overrides'
- ) {
- const supportedAttributes = BLOCK_BINDINGS_ALLOWED_BLOCKS[ blockName ];
- const bindingsWithDefaults = {};
- for ( const attributeName of supportedAttributes ) {
- // If the block has mixed binding sources, retain any non pattern override bindings.
- const bindingSource = bindings[ attributeName ]
- ? bindings[ attributeName ]
- : { source: 'core/pattern-overrides' };
- bindingsWithDefaults[ attributeName ] = bindingSource;
- }
-
- return bindingsWithDefaults;
- }
-
- return bindings;
-}
-
-/**
- * Based on the given block name,
- * check if it is possible to bind the block.
- *
- * @param {string} blockName - The block name.
- * @return {boolean} Whether it is possible to bind the block to sources.
- */
-export function canBindBlock( blockName ) {
- return blockName in BLOCK_BINDINGS_ALLOWED_BLOCKS;
-}
-
-/**
- * Based on the given block name and attribute name,
- * check if it is possible to bind the block attribute.
- *
- * @param {string} blockName - The block name.
- * @param {string} attributeName - The attribute name.
- * @return {boolean} Whether it is possible to bind the block attribute.
- */
-export function canBindAttribute( blockName, attributeName ) {
- return (
- canBindBlock( blockName ) &&
- BLOCK_BINDINGS_ALLOWED_BLOCKS[ blockName ].includes( attributeName )
- );
-}
-
-export function getBindableAttributes( blockName ) {
- return BLOCK_BINDINGS_ALLOWED_BLOCKS[ blockName ];
-}
-
-export const withBlockBindingSupport = createHigherOrderComponent(
- ( BlockEdit ) => ( props ) => {
- const registry = useRegistry();
- const blockContext = useContext( BlockContext );
- const sources = useSelect( ( select ) =>
- unlock( select( blocksStore ) ).getAllBlockBindingsSources()
- );
- const { name, clientId, context, setAttributes } = props;
- const blockBindings = useMemo(
- () =>
- replacePatternOverrideDefaultBindings(
- name,
- props.attributes.metadata?.bindings
- ),
- [ props.attributes.metadata?.bindings, name ]
- );
-
- // While this hook doesn't directly call any selectors, `useSelect` is
- // used purposely here to ensure `boundAttributes` is updated whenever
- // there are attribute updates.
- // `source.getValues` may also call a selector via `registry.select`.
- const updatedContext = {};
- const boundAttributes = useSelect(
- ( select ) => {
- if ( ! blockBindings ) {
- return;
- }
-
- const attributes = {};
-
- const blockBindingsBySource = new Map();
-
- for ( const [ attributeName, binding ] of Object.entries(
- blockBindings
- ) ) {
- const { source: sourceName, args: sourceArgs } = binding;
- const source = sources[ sourceName ];
- if (
- ! source ||
- ! canBindAttribute( name, attributeName )
- ) {
- continue;
- }
-
- // Populate context.
- for ( const key of source.usesContext || [] ) {
- updatedContext[ key ] = blockContext[ key ];
- }
-
- blockBindingsBySource.set( source, {
- ...blockBindingsBySource.get( source ),
- [ attributeName ]: {
- args: sourceArgs,
- },
- } );
- }
-
- if ( blockBindingsBySource.size ) {
- for ( const [
- source,
- bindings,
- ] of blockBindingsBySource ) {
- // Get values in batch if the source supports it.
- let values = {};
- if ( ! source.getValues ) {
- Object.keys( bindings ).forEach( ( attr ) => {
- // Default to the the source label when `getValues` doesn't exist.
- values[ attr ] = source.label;
- } );
- } else {
- values = source.getValues( {
- select,
- context: updatedContext,
- clientId,
- bindings,
- } );
- }
- for ( const [ attributeName, value ] of Object.entries(
- values
- ) ) {
- if (
- attributeName === 'url' &&
- ( ! value || ! isURLLike( value ) )
- ) {
- // Return null if value is not a valid URL.
- attributes[ attributeName ] = null;
- } else {
- attributes[ attributeName ] = value;
- }
- }
- }
- }
-
- return attributes;
- },
- [ blockBindings, name, clientId, updatedContext, sources ]
- );
-
- const hasParentPattern = !! updatedContext[ 'pattern/overrides' ];
- const hasPatternOverridesDefaultBinding =
- props.attributes.metadata?.bindings?.[ DEFAULT_ATTRIBUTE ]
- ?.source === 'core/pattern-overrides';
-
- const _setAttributes = useCallback(
- ( nextAttributes ) => {
- registry.batch( () => {
- if ( ! blockBindings ) {
- setAttributes( nextAttributes );
- return;
- }
-
- const keptAttributes = { ...nextAttributes };
- const blockBindingsBySource = new Map();
-
- // Loop only over the updated attributes to avoid modifying the bound ones that haven't changed.
- for ( const [ attributeName, newValue ] of Object.entries(
- keptAttributes
- ) ) {
- if (
- ! blockBindings[ attributeName ] ||
- ! canBindAttribute( name, attributeName )
- ) {
- continue;
- }
-
- const binding = blockBindings[ attributeName ];
- const source = sources[ binding?.source ];
- if ( ! source?.setValues ) {
- continue;
- }
- blockBindingsBySource.set( source, {
- ...blockBindingsBySource.get( source ),
- [ attributeName ]: {
- args: binding.args,
- newValue,
- },
- } );
- delete keptAttributes[ attributeName ];
- }
-
- if ( blockBindingsBySource.size ) {
- for ( const [
- source,
- bindings,
- ] of blockBindingsBySource ) {
- source.setValues( {
- select: registry.select,
- dispatch: registry.dispatch,
- context: updatedContext,
- clientId,
- bindings,
- } );
- }
- }
-
- if (
- // Don't update non-connected attributes if the block is using pattern overrides
- // and the editing is happening while overriding the pattern (not editing the original).
- ! (
- hasPatternOverridesDefaultBinding &&
- hasParentPattern
- ) &&
- Object.keys( keptAttributes ).length
- ) {
- // Don't update caption and href until they are supported.
- if ( hasPatternOverridesDefaultBinding ) {
- delete keptAttributes?.caption;
- delete keptAttributes?.href;
- }
- setAttributes( keptAttributes );
- }
- } );
- },
- [
- registry,
- blockBindings,
- name,
- clientId,
- updatedContext,
- setAttributes,
- sources,
- hasPatternOverridesDefaultBinding,
- hasParentPattern,
- ]
- );
-
- return (
- <>
-
- >
- );
- },
- 'withBlockBindingSupport'
-);
-
-/**
- * Filters a registered block's settings to enhance a block's `edit` component
- * to upgrade bound attributes.
- *
- * @param {WPBlockSettings} settings - Registered block settings.
- * @param {string} name - Block name.
- * @return {WPBlockSettings} Filtered block settings.
- */
-function shimAttributeSource( settings, name ) {
- if ( ! canBindBlock( name ) ) {
- return settings;
- }
-
- return {
- ...settings,
- edit: withBlockBindingSupport( settings.edit ),
- };
-}
-
-addFilter(
- 'blocks.registerBlockType',
- 'core/editor/custom-sources-backwards-compatibility/shim-attribute-source',
- shimAttributeSource
-);
diff --git a/packages/block-editor/src/hooks/use-zoom-out.js b/packages/block-editor/src/hooks/use-zoom-out.js
index bcf5d9ff882f7..adcea8b605aeb 100644
--- a/packages/block-editor/src/hooks/use-zoom-out.js
+++ b/packages/block-editor/src/hooks/use-zoom-out.js
@@ -2,7 +2,7 @@
* WordPress dependencies
*/
import { useSelect, useDispatch } from '@wordpress/data';
-import { useEffect } from '@wordpress/element';
+import { useEffect, useRef } from '@wordpress/element';
/**
* Internal dependencies
@@ -12,32 +12,64 @@ import { unlock } from '../lock-unlock';
/**
* A hook used to set the editor mode to zoomed out mode, invoking the hook sets the mode.
+ * Concepts:
+ * - If we most recently changed the zoom level for them (in or out), we always resetZoomLevel() level when unmounting.
+ * - If the user most recently changed the zoom level (manually toggling), we do nothing when unmounting.
*
- * @param {boolean} zoomOut If we should enter into zoomOut mode or not
+ * @param {boolean} enabled If we should enter into zoomOut mode or not
*/
-export function useZoomOut( zoomOut = true ) {
+export function useZoomOut( enabled = true ) {
const { setZoomLevel, resetZoomLevel } = unlock(
useDispatch( blockEditorStore )
);
- const { isZoomOut } = unlock( useSelect( blockEditorStore ) );
+ /**
+ * We need access to both the value and the function. The value is to trigger a useEffect hook
+ * and the function is to check zoom out within another hook without triggering a re-render.
+ */
+ const { isZoomedOut, isZoomOut } = useSelect( ( select ) => {
+ const { isZoomOut: _isZoomOut } = unlock( select( blockEditorStore ) );
+ return {
+ isZoomedOut: _isZoomOut(),
+ isZoomOut: _isZoomOut,
+ };
+ }, [] );
+
+ const controlZoomLevelRef = useRef( false );
+ const isEnabledRef = useRef( enabled );
+
+ /**
+ * This hook tracks if the zoom state was changed manually by the user via clicking
+ * the zoom out button. We only want this to run when isZoomedOut changes, so we use
+ * a ref to track the enabled state.
+ */
useEffect( () => {
- const isZoomOutOnMount = isZoomOut();
+ // If the zoom state changed (isZoomOut) and it does not match the requested zoom
+ // state (zoomOut), then it means the user manually changed the zoom state while
+ // this hook was mounted, and we should no longer control the zoom state.
+ if ( isZoomedOut !== isEnabledRef.current ) {
+ controlZoomLevelRef.current = false;
+ }
+ }, [ isZoomedOut ] );
- return () => {
- if ( isZoomOutOnMount ) {
+ useEffect( () => {
+ isEnabledRef.current = enabled;
+
+ if ( enabled !== isZoomOut() ) {
+ controlZoomLevelRef.current = true;
+
+ if ( enabled ) {
setZoomLevel( 'auto-scaled' );
} else {
resetZoomLevel();
}
- };
- }, [] );
-
- useEffect( () => {
- if ( zoomOut ) {
- setZoomLevel( 'auto-scaled' );
- } else {
- resetZoomLevel();
}
- }, [ zoomOut, setZoomLevel, resetZoomLevel ] );
+
+ return () => {
+ // If we are controlling zoom level and are zoomed out, reset the zoom level.
+ if ( controlZoomLevelRef.current && isZoomOut() ) {
+ resetZoomLevel();
+ }
+ };
+ }, [ enabled, isZoomOut, resetZoomLevel, setZoomLevel ] );
}
diff --git a/packages/block-editor/src/hooks/utils.js b/packages/block-editor/src/hooks/utils.js
index 4334f70b9d13b..ac6e55efe4d3b 100644
--- a/packages/block-editor/src/hooks/utils.js
+++ b/packages/block-editor/src/hooks/utils.js
@@ -124,7 +124,7 @@ export function shouldSkipSerialization(
feature
) {
const support = getBlockSupport( blockNameOrType, featureSet );
- const skipSerialization = support?.__experimentalSkipSerialization;
+ const skipSerialization = support?.skipSerialization;
if ( Array.isArray( skipSerialization ) ) {
return skipSerialization.includes( feature );
diff --git a/packages/block-editor/src/layouts/flex.js b/packages/block-editor/src/layouts/flex.js
index 8171844969565..f57ccbde46616 100644
--- a/packages/block-editor/src/layouts/flex.js
+++ b/packages/block-editor/src/layouts/flex.js
@@ -66,24 +66,27 @@ export default {
onChange,
layoutBlockSupport = {},
} ) {
- const { allowOrientation = true } = layoutBlockSupport;
+ const { allowOrientation = true, allowJustification = true } =
+ layoutBlockSupport;
return (
<>
-
-
-
-
- { allowOrientation && (
+ { allowJustification && (
+
+
+
+ ) }
+ { allowOrientation && (
+
- ) }
-
+
+ ) }
>
@@ -94,17 +97,22 @@ export default {
onChange,
layoutBlockSupport,
} ) {
- if ( layoutBlockSupport?.allowSwitching ) {
+ const { allowVerticalAlignment = true, allowJustification = true } =
+ layoutBlockSupport;
+
+ if ( ! allowJustification && ! allowVerticalAlignment ) {
return null;
}
- const { allowVerticalAlignment = true } = layoutBlockSupport;
+
return (
-
+ { allowJustification && (
+
+ ) }
{ allowVerticalAlignment && (
const privateSettings = [
'inserterMediaCategories',
'blockInspectorAnimation',
+ 'mediaSideload',
];
/**
diff --git a/packages/block-editor/src/store/private-selectors.js b/packages/block-editor/src/store/private-selectors.js
index f1b5abd7789a1..c46778d889b3e 100644
--- a/packages/block-editor/src/store/private-selectors.js
+++ b/packages/block-editor/src/store/private-selectors.js
@@ -112,12 +112,12 @@ function getEnabledClientIdsTreeUnmemoized( state, rootClientId ) {
export const getEnabledClientIdsTree = createRegistrySelector( ( select ) =>
createSelector( getEnabledClientIdsTreeUnmemoized, ( state ) => [
state.blocks.order,
+ state.derivedBlockEditingModes,
+ state.derivedNavModeBlockEditingModes,
state.blockEditingModes,
state.settings.templateLock,
state.blockListSettings,
select( STORE_NAME ).__unstableGetEditorMode( state ),
- state.zoomLevel,
- getSectionRootClientId( state ),
] )
);
@@ -330,7 +330,7 @@ function mapUserPattern(
id: userPattern.id,
type: INSERTER_PATTERN_TYPES.user,
title: userPattern.title.raw,
- categories: userPattern.wp_pattern_category.map( ( catId ) => {
+ categories: userPattern.wp_pattern_category?.map( ( catId ) => {
const category = __experimentalUserPatternCategories.find(
( { id } ) => id === catId
);
@@ -406,21 +406,6 @@ export const getAllPatterns = createRegistrySelector( ( select ) =>
}, getAllPatternsDependants( select ) )
);
-export const isResolvingPatterns = createRegistrySelector( ( select ) =>
- createSelector( ( state ) => {
- const blockPatternsSelect = state.settings[ selectBlockPatternsKey ];
- const reusableBlocksSelect = state.settings[ reusableBlocksSelectKey ];
- return (
- ( blockPatternsSelect
- ? blockPatternsSelect( select ) === undefined
- : false ) ||
- ( reusableBlocksSelect
- ? reusableBlocksSelect( select ) === undefined
- : false )
- );
- }, getAllPatternsDependants( select ) )
-);
-
const EMPTY_ARRAY = [];
export const getReusableBlocks = createRegistrySelector(
diff --git a/packages/block-editor/src/store/reducer.js b/packages/block-editor/src/store/reducer.js
index 2f0fa70d616fd..edae9c392c37d 100644
--- a/packages/block-editor/src/store/reducer.js
+++ b/packages/block-editor/src/store/reducer.js
@@ -9,12 +9,20 @@ import fastDeepEqual from 'fast-deep-equal/es6';
import { pipe } from '@wordpress/compose';
import { combineReducers, select } from '@wordpress/data';
import deprecated from '@wordpress/deprecated';
-import { store as blocksStore } from '@wordpress/blocks';
+import {
+ store as blocksStore,
+ privateApis as blocksPrivateApis,
+} from '@wordpress/blocks';
+
/**
* Internal dependencies
*/
import { PREFERENCES_DEFAULTS, SETTINGS_DEFAULTS } from './defaults';
import { insertAt, moveTo } from './array';
+import { sectionRootClientIdKey } from './private-keys';
+import { unlock } from '../lock-unlock';
+
+const { isContentBlock } = unlock( blocksPrivateApis );
const identity = ( x ) => x;
@@ -2131,6 +2139,632 @@ const combinedReducers = combineReducers( {
zoomLevel,
} );
+/**
+ * Retrieves a block's tree structure, handling both controlled and uncontrolled inner blocks.
+ *
+ * @param {Object} state The current state object.
+ * @param {string} clientId The client ID of the block to retrieve.
+ *
+ * @return {Object|undefined} The block tree object, or undefined if not found. For controlled blocks,
+ * returns a merged tree with controlled inner blocks.
+ */
+function getBlockTreeBlock( state, clientId ) {
+ if ( clientId === '' ) {
+ const rootBlock = state.blocks.tree.get( clientId );
+
+ if ( ! rootBlock ) {
+ return;
+ }
+
+ // Patch the root block to have a clientId property.
+ // TODO - consider updating the blocks reducer so that the root block has this property.
+ return {
+ clientId: '',
+ ...rootBlock,
+ };
+ }
+
+ if ( ! state.blocks.controlledInnerBlocks[ clientId ] ) {
+ return state.blocks.tree.get( clientId );
+ }
+
+ const controlledTree = state.blocks.tree.get( `controlled||${ clientId }` );
+ const regularTree = state.blocks.tree.get( clientId );
+
+ return {
+ ...regularTree,
+ innerBlocks: controlledTree?.innerBlocks,
+ };
+}
+
+/**
+ * Recursively traverses through a block tree of a given block and executes a callback for each block.
+ *
+ * @param {Object} state The store state.
+ * @param {string} clientId The clientId of the block to start traversing from.
+ * @param {Function} callback Function to execute for each block encountered during traversal.
+ * The callback receives the current block as its argument.
+ */
+function traverseBlockTree( state, clientId, callback ) {
+ const parentTree = getBlockTreeBlock( state, clientId );
+ if ( ! parentTree ) {
+ return;
+ }
+
+ callback( parentTree );
+
+ if ( ! parentTree?.innerBlocks?.length ) {
+ return;
+ }
+
+ for ( const block of parentTree?.innerBlocks ) {
+ traverseBlockTree( state, block.clientId, callback );
+ }
+}
+
+/**
+ * Checks if a block has a parent in a list of client IDs, and if so returns the client ID of the parent.
+ *
+ * @param {Object} state The current state object.
+ * @param {string} clientId The client ID of the block to search the parents of.
+ * @param {Array} clientIds The client IDs of the blocks to check.
+ *
+ * @return {string|undefined} The client ID of the parent block if found, undefined otherwise.
+ */
+function findParentInClientIdsList( state, clientId, clientIds ) {
+ let parent = state.blocks.parents.get( clientId );
+ while ( parent ) {
+ if ( clientIds.includes( parent ) ) {
+ return parent;
+ }
+ parent = state.blocks.parents.get( parent );
+ }
+}
+
+/**
+ * Checks if a block has any bindings in its metadata attributes.
+ *
+ * @param {Object} block The block object to check for bindings.
+ * @return {boolean} True if the block has bindings, false otherwise.
+ */
+function hasBindings( block ) {
+ return (
+ block?.attributes?.metadata?.bindings &&
+ Object.keys( block?.attributes?.metadata?.bindings ).length
+ );
+}
+
+/**
+ * Computes and returns derived block editing modes for a given block tree.
+ *
+ * This function calculates the editing modes for each block in the tree, taking into account
+ * various factors such as zoom level, navigation mode, sections, and synced patterns.
+ *
+ * @param {Object} state The current state object.
+ * @param {boolean} isNavMode Whether the navigation mode is active.
+ * @param {string} treeClientId The client ID of the root block for the tree. Defaults to an empty string.
+ * @return {Map} A Map containing the derived block editing modes, keyed by block client ID.
+ */
+function getDerivedBlockEditingModesForTree(
+ state,
+ isNavMode = false,
+ treeClientId = ''
+) {
+ const isZoomedOut =
+ state?.zoomLevel < 100 || state?.zoomLevel === 'auto-scaled';
+ const derivedBlockEditingModes = new Map();
+
+ // When there are sections, the majority of blocks are disabled,
+ // so the default block editing mode is set to disabled.
+ const sectionRootClientId = state.settings?.[ sectionRootClientIdKey ];
+ const sectionClientIds = state.blocks.order.get( sectionRootClientId );
+ const syncedPatternClientIds = Object.keys(
+ state.blocks.controlledInnerBlocks
+ ).filter(
+ ( clientId ) =>
+ state.blocks.byClientId?.get( clientId )?.name === 'core/block'
+ );
+
+ traverseBlockTree( state, treeClientId, ( block ) => {
+ const { clientId, name: blockName } = block;
+ if ( isZoomedOut || isNavMode ) {
+ // If the root block is the section root set its editing mode to contentOnly.
+ if ( clientId === sectionRootClientId ) {
+ derivedBlockEditingModes.set( clientId, 'contentOnly' );
+ return;
+ }
+
+ // There are no sections, so everything else is disabled.
+ if ( ! sectionClientIds?.length ) {
+ derivedBlockEditingModes.set( clientId, 'disabled' );
+ return;
+ }
+
+ if ( sectionClientIds.includes( clientId ) ) {
+ derivedBlockEditingModes.set( clientId, 'contentOnly' );
+ return;
+ }
+
+ // If zoomed out, all blocks that aren't sections or the section root are
+ // disabled.
+ // If the tree root is not in a section, set its editing mode to disabled.
+ if (
+ isZoomedOut ||
+ ! findParentInClientIdsList( state, clientId, sectionClientIds )
+ ) {
+ derivedBlockEditingModes.set( clientId, 'disabled' );
+ return;
+ }
+
+ // Handle synced pattern content so the inner blocks of a synced pattern are
+ // properly disabled.
+ if ( syncedPatternClientIds.length ) {
+ const parentPatternClientId = findParentInClientIdsList(
+ state,
+ clientId,
+ syncedPatternClientIds
+ );
+
+ if ( parentPatternClientId ) {
+ // This is a pattern nested in another pattern, it should be disabled.
+ if (
+ findParentInClientIdsList(
+ state,
+ parentPatternClientId,
+ syncedPatternClientIds
+ )
+ ) {
+ derivedBlockEditingModes.set( clientId, 'disabled' );
+ return;
+ }
+
+ if ( hasBindings( block ) ) {
+ derivedBlockEditingModes.set( clientId, 'contentOnly' );
+ return;
+ }
+
+ // Synced pattern content without a binding isn't editable
+ // from the instance, the user has to edit the pattern source,
+ // so return 'disabled'.
+ derivedBlockEditingModes.set( clientId, 'disabled' );
+ return;
+ }
+ }
+
+ if ( blockName && isContentBlock( blockName ) ) {
+ derivedBlockEditingModes.set( clientId, 'contentOnly' );
+ return;
+ }
+
+ derivedBlockEditingModes.set( clientId, 'disabled' );
+ return;
+ }
+
+ if ( syncedPatternClientIds.length ) {
+ // Synced pattern blocks (core/block).
+ if ( syncedPatternClientIds.includes( clientId ) ) {
+ // This is a pattern nested in another pattern, it should be disabled.
+ if (
+ findParentInClientIdsList(
+ state,
+ clientId,
+ syncedPatternClientIds
+ )
+ ) {
+ derivedBlockEditingModes.set( clientId, 'disabled' );
+ return;
+ }
+
+ // Else do nothing, use the default block editing mode.
+ return;
+ }
+
+ // Inner blocks of synced patterns.
+ const parentPatternClientId = findParentInClientIdsList(
+ state,
+ clientId,
+ syncedPatternClientIds
+ );
+ if ( parentPatternClientId ) {
+ // This is a pattern nested in another pattern, it should be disabled.
+ if (
+ findParentInClientIdsList(
+ state,
+ parentPatternClientId,
+ syncedPatternClientIds
+ )
+ ) {
+ derivedBlockEditingModes.set( clientId, 'disabled' );
+ return;
+ }
+
+ if ( hasBindings( block ) ) {
+ derivedBlockEditingModes.set( clientId, 'contentOnly' );
+ return;
+ }
+
+ // Synced pattern content without a binding isn't editable
+ // from the instance, the user has to edit the pattern source,
+ // so return 'disabled'.
+ derivedBlockEditingModes.set( clientId, 'disabled' );
+ }
+ }
+ } );
+
+ return derivedBlockEditingModes;
+}
+
+/**
+ * Updates the derived block editing modes based on added and removed blocks.
+ *
+ * This function handles the updating of block editing modes when blocks are added,
+ * removed, or moved within the editor.
+ *
+ * It only returns a value when modifications are made to the block editing modes.
+ *
+ * @param {Object} options The options for updating derived block editing modes.
+ * @param {Object} options.prevState The previous state object.
+ * @param {Object} options.nextState The next state object.
+ * @param {Array} [options.addedBlocks] An array of blocks that were added.
+ * @param {Array} [options.removedClientIds] An array of client IDs of blocks that were removed.
+ * @param {boolean} [options.isNavMode] Whether the navigation mode is active.
+ * @return {Map|undefined} The updated derived block editing modes, or undefined if no changes were made.
+ */
+function getDerivedBlockEditingModesUpdates( {
+ prevState,
+ nextState,
+ addedBlocks,
+ removedClientIds,
+ isNavMode = false,
+} ) {
+ const prevDerivedBlockEditingModes = isNavMode
+ ? prevState.derivedNavModeBlockEditingModes
+ : prevState.derivedBlockEditingModes;
+ let nextDerivedBlockEditingModes;
+
+ // Perform removals before additions to handle cases like the `MOVE_BLOCKS_TO_POSITION` action.
+ // That action removes a set of clientIds, but adds the same blocks back in a different location.
+ // If removals were performed after additions, those moved clientIds would be removed incorrectly.
+ removedClientIds?.forEach( ( clientId ) => {
+ // The actions only receive parent block IDs for removal.
+ // Recurse through the block tree to ensure all blocks are removed.
+ // Specifically use the previous state, before the blocks were removed.
+ traverseBlockTree( prevState, clientId, ( block ) => {
+ if ( prevDerivedBlockEditingModes.has( block.clientId ) ) {
+ if ( ! nextDerivedBlockEditingModes ) {
+ nextDerivedBlockEditingModes = new Map(
+ prevDerivedBlockEditingModes
+ );
+ }
+ nextDerivedBlockEditingModes.delete( block.clientId );
+ }
+ } );
+ } );
+
+ addedBlocks?.forEach( ( addedBlock ) => {
+ traverseBlockTree( nextState, addedBlock.clientId, ( block ) => {
+ const updates = getDerivedBlockEditingModesForTree(
+ nextState,
+ isNavMode,
+ block.clientId
+ );
+
+ if ( updates.size ) {
+ if ( ! nextDerivedBlockEditingModes ) {
+ nextDerivedBlockEditingModes = new Map( [
+ ...( prevDerivedBlockEditingModes?.size
+ ? prevDerivedBlockEditingModes
+ : [] ),
+ ...updates,
+ ] );
+ } else {
+ nextDerivedBlockEditingModes = new Map( [
+ ...( nextDerivedBlockEditingModes?.size
+ ? nextDerivedBlockEditingModes
+ : [] ),
+ ...updates,
+ ] );
+ }
+ }
+ } );
+ } );
+
+ return nextDerivedBlockEditingModes;
+}
+
+/**
+ * Higher-order reducer that adds derived block editing modes to the state.
+ *
+ * This function wraps a reducer and enhances it to handle actions that affect
+ * block editing modes. It updates the derivedBlockEditingModes in the state
+ * based on various actions such as adding, removing, or moving blocks, or changing
+ * the editor mode.
+ *
+ * @param {Function} reducer The original reducer function to be wrapped.
+ * @return {Function} A new reducer function that includes derived block editing modes handling.
+ */
+export function withDerivedBlockEditingModes( reducer ) {
+ return ( state, action ) => {
+ const nextState = reducer( state, action );
+
+ // An exception is needed here to still recompute the block editing modes when
+ // the editor mode changes since the editor mode isn't stored within the
+ // block editor state and changing it won't trigger an altered new state.
+ if ( action.type !== 'SET_EDITOR_MODE' && nextState === state ) {
+ return state;
+ }
+
+ switch ( action.type ) {
+ case 'REMOVE_BLOCKS': {
+ const nextDerivedBlockEditingModes =
+ getDerivedBlockEditingModesUpdates( {
+ prevState: state,
+ nextState,
+ removedClientIds: action.clientIds,
+ isNavMode: false,
+ } );
+ const nextDerivedNavModeBlockEditingModes =
+ getDerivedBlockEditingModesUpdates( {
+ prevState: state,
+ nextState,
+ removedClientIds: action.clientIds,
+ isNavMode: true,
+ } );
+
+ if (
+ nextDerivedBlockEditingModes ||
+ nextDerivedNavModeBlockEditingModes
+ ) {
+ return {
+ ...nextState,
+ derivedBlockEditingModes:
+ nextDerivedBlockEditingModes ??
+ state.derivedBlockEditingModes,
+ derivedNavModeBlockEditingModes:
+ nextDerivedNavModeBlockEditingModes ??
+ state.derivedNavModeBlockEditingModes,
+ };
+ }
+ break;
+ }
+ case 'RECEIVE_BLOCKS':
+ case 'INSERT_BLOCKS': {
+ const nextDerivedBlockEditingModes =
+ getDerivedBlockEditingModesUpdates( {
+ prevState: state,
+ nextState,
+ addedBlocks: action.blocks,
+ isNavMode: false,
+ } );
+ const nextDerivedNavModeBlockEditingModes =
+ getDerivedBlockEditingModesUpdates( {
+ prevState: state,
+ nextState,
+ addedBlocks: action.blocks,
+ isNavMode: true,
+ } );
+
+ if (
+ nextDerivedBlockEditingModes ||
+ nextDerivedNavModeBlockEditingModes
+ ) {
+ return {
+ ...nextState,
+ derivedBlockEditingModes:
+ nextDerivedBlockEditingModes ??
+ state.derivedBlockEditingModes,
+ derivedNavModeBlockEditingModes:
+ nextDerivedNavModeBlockEditingModes ??
+ state.derivedNavModeBlockEditingModes,
+ };
+ }
+ break;
+ }
+ case 'SET_HAS_CONTROLLED_INNER_BLOCKS': {
+ const updatedBlock = nextState.blocks.tree.get(
+ action.clientId
+ );
+ // The block might have been removed.
+ if ( ! updatedBlock ) {
+ break;
+ }
+
+ const nextDerivedBlockEditingModes =
+ getDerivedBlockEditingModesUpdates( {
+ prevState: state,
+ nextState,
+ addedBlocks: [ updatedBlock ],
+ isNavMode: false,
+ } );
+ const nextDerivedNavModeBlockEditingModes =
+ getDerivedBlockEditingModesUpdates( {
+ prevState: state,
+ nextState,
+ addedBlocks: [ updatedBlock ],
+ isNavMode: true,
+ } );
+
+ if (
+ nextDerivedBlockEditingModes ||
+ nextDerivedNavModeBlockEditingModes
+ ) {
+ return {
+ ...nextState,
+ derivedBlockEditingModes:
+ nextDerivedBlockEditingModes ??
+ state.derivedBlockEditingModes,
+ derivedNavModeBlockEditingModes:
+ nextDerivedNavModeBlockEditingModes ??
+ state.derivedNavModeBlockEditingModes,
+ };
+ }
+ break;
+ }
+ case 'REPLACE_BLOCKS': {
+ const nextDerivedBlockEditingModes =
+ getDerivedBlockEditingModesUpdates( {
+ prevState: state,
+ nextState,
+ addedBlocks: action.blocks,
+ removedClientIds: action.clientIds,
+ isNavMode: false,
+ } );
+ const nextDerivedNavModeBlockEditingModes =
+ getDerivedBlockEditingModesUpdates( {
+ prevState: state,
+ nextState,
+ addedBlocks: action.blocks,
+ removedClientIds: action.clientIds,
+ isNavMode: true,
+ } );
+
+ if (
+ nextDerivedBlockEditingModes ||
+ nextDerivedNavModeBlockEditingModes
+ ) {
+ return {
+ ...nextState,
+ derivedBlockEditingModes:
+ nextDerivedBlockEditingModes ??
+ state.derivedBlockEditingModes,
+ derivedNavModeBlockEditingModes:
+ nextDerivedNavModeBlockEditingModes ??
+ state.derivedNavModeBlockEditingModes,
+ };
+ }
+ break;
+ }
+ case 'REPLACE_INNER_BLOCKS': {
+ // Get the clientIds of the blocks that are being replaced
+ // from the old state, before they were removed.
+ const removedClientIds = state.blocks.order.get(
+ action.rootClientId
+ );
+ const nextDerivedBlockEditingModes =
+ getDerivedBlockEditingModesUpdates( {
+ prevState: state,
+ nextState,
+ addedBlocks: action.blocks,
+ removedClientIds,
+ isNavMode: false,
+ } );
+ const nextDerivedNavModeBlockEditingModes =
+ getDerivedBlockEditingModesUpdates( {
+ prevState: state,
+ nextState,
+ addedBlocks: action.blocks,
+ removedClientIds,
+ isNavMode: true,
+ } );
+
+ if (
+ nextDerivedBlockEditingModes ||
+ nextDerivedNavModeBlockEditingModes
+ ) {
+ return {
+ ...nextState,
+ derivedBlockEditingModes:
+ nextDerivedBlockEditingModes ??
+ state.derivedBlockEditingModes,
+ derivedNavModeBlockEditingModes:
+ nextDerivedNavModeBlockEditingModes ??
+ state.derivedNavModeBlockEditingModes,
+ };
+ }
+ break;
+ }
+ case 'MOVE_BLOCKS_TO_POSITION': {
+ const addedBlocks = action.clientIds.map( ( clientId ) => {
+ return nextState.blocks.byClientId.get( clientId );
+ } );
+ const nextDerivedBlockEditingModes =
+ getDerivedBlockEditingModesUpdates( {
+ prevState: state,
+ nextState,
+ addedBlocks,
+ removedClientIds: action.clientIds,
+ isNavMode: false,
+ } );
+ const nextDerivedNavModeBlockEditingModes =
+ getDerivedBlockEditingModesUpdates( {
+ prevState: state,
+ nextState,
+ addedBlocks,
+ removedClientIds: action.clientIds,
+ isNavMode: true,
+ } );
+
+ if (
+ nextDerivedBlockEditingModes ||
+ nextDerivedNavModeBlockEditingModes
+ ) {
+ return {
+ ...nextState,
+ derivedBlockEditingModes:
+ nextDerivedBlockEditingModes ??
+ state.derivedBlockEditingModes,
+ derivedNavModeBlockEditingModes:
+ nextDerivedNavModeBlockEditingModes ??
+ state.derivedNavModeBlockEditingModes,
+ };
+ }
+ break;
+ }
+ case 'UPDATE_SETTINGS': {
+ // Recompute the entire tree if the section root changes.
+ if (
+ state?.settings?.[ sectionRootClientIdKey ] !==
+ nextState?.settings?.[ sectionRootClientIdKey ]
+ ) {
+ return {
+ ...nextState,
+ derivedBlockEditingModes:
+ getDerivedBlockEditingModesForTree(
+ nextState,
+ false /* Nav mode off */
+ ),
+ derivedNavModeBlockEditingModes:
+ getDerivedBlockEditingModesForTree(
+ nextState,
+ true /* Nav mode on */
+ ),
+ };
+ }
+ break;
+ }
+ case 'RESET_BLOCKS':
+ case 'SET_EDITOR_MODE':
+ case 'RESET_ZOOM_LEVEL':
+ case 'SET_ZOOM_LEVEL': {
+ // Recompute the entire tree if the editor mode or zoom level changes,
+ // or if all the blocks are reset.
+ return {
+ ...nextState,
+ derivedBlockEditingModes:
+ getDerivedBlockEditingModesForTree(
+ nextState,
+ false /* Nav mode off */
+ ),
+ derivedNavModeBlockEditingModes:
+ getDerivedBlockEditingModesForTree(
+ nextState,
+ true /* Nav mode on */
+ ),
+ };
+ }
+ }
+
+ // If there's no change, the derivedBlockEditingModes from the previous
+ // state need to be preserved.
+ nextState.derivedBlockEditingModes =
+ state?.derivedBlockEditingModes ?? new Map();
+ nextState.derivedNavModeBlockEditingModes =
+ state?.derivedNavModeBlockEditingModes ?? new Map();
+
+ return nextState;
+ };
+}
+
function withAutomaticChangeReset( reducer ) {
return ( state, action ) => {
const nextState = reducer( state, action );
@@ -2184,4 +2818,7 @@ function withAutomaticChangeReset( reducer ) {
};
}
-export default withAutomaticChangeReset( combinedReducers );
+export default pipe(
+ withDerivedBlockEditingModes,
+ withAutomaticChangeReset
+)( combinedReducers );
diff --git a/packages/block-editor/src/store/selectors.js b/packages/block-editor/src/store/selectors.js
index 598b6b4ea480d..ed9e859f028a9 100644
--- a/packages/block-editor/src/store/selectors.js
+++ b/packages/block-editor/src/store/selectors.js
@@ -1586,14 +1586,14 @@ export function getTemplateLock( state, rootClientId ) {
* @param {string|Object} blockNameOrType The block type object, e.g., the response
* from the block directory; or a string name of
* an installed block type, e.g.' core/paragraph'.
- * @param {Set} checkedBlocks Set of block names that have already been checked.
+ * @param {?string} rootClientId Optional root client ID of block list.
*
* @return {boolean} Whether the given block type is allowed to be inserted.
*/
const isBlockVisibleInTheInserter = (
state,
blockNameOrType,
- checkedBlocks = new Set()
+ rootClientId = null
) => {
let blockType;
let blockName;
@@ -1621,26 +1621,19 @@ const isBlockVisibleInTheInserter = (
return false;
}
- if ( checkedBlocks.has( blockName ) ) {
- return false;
- }
-
- checkedBlocks.add( blockName );
-
// If parent blocks are not visible, child blocks should be hidden too.
- if ( Array.isArray( blockType.parent ) ) {
- return blockType.parent.some(
- ( name ) =>
- ( blockName !== name &&
- isBlockVisibleInTheInserter(
- state,
- name,
- checkedBlocks
- ) ) ||
- // Exception for blocks with post-content parent,
- // the root level is often consider as "core/post-content".
- // This exception should only apply to the post editor ideally though.
- name === 'core/post-content'
+ const parents = (
+ Array.isArray( blockType.parent ) ? blockType.parent : []
+ ).concat( Array.isArray( blockType.ancestor ) ? blockType.ancestor : [] );
+ if ( parents.length > 0 ) {
+ const rootBlockName = getBlockName( state, rootClientId );
+ // This is an exception to the rule that says that all blocks are visible in the inserter.
+ // Blocks that require a given parent or ancestor are only visible if we're within that parent.
+ return (
+ parents.includes( 'core/post-content' ) ||
+ parents.includes( rootBlockName ) ||
+ getBlockParentsByBlockName( state, rootClientId, parents ).length >
+ 0
);
}
@@ -1665,7 +1658,7 @@ const canInsertBlockTypeUnmemoized = (
blockName,
rootClientId = null
) => {
- if ( ! isBlockVisibleInTheInserter( state, blockName ) ) {
+ if ( ! isBlockVisibleInTheInserter( state, blockName, rootClientId ) ) {
return false;
}
@@ -2072,6 +2065,7 @@ const buildBlockTypeItem =
category: blockType.category,
keywords: blockType.keywords,
parent: blockType.parent,
+ ancestor: blockType.ancestor,
variations: inserterVariations,
example: blockType.example,
utility: 1, // Deprecated.
@@ -2169,7 +2163,11 @@ export const getInserterItems = createRegistrySelector( ( select ) =>
} else {
blockTypeInserterItems = blockTypeInserterItems
.filter( ( blockType ) =>
- isBlockVisibleInTheInserter( state, blockType )
+ isBlockVisibleInTheInserter(
+ state,
+ blockType,
+ rootClientId
+ )
)
.map( ( blockType ) => ( {
...blockType,
@@ -2501,7 +2499,11 @@ export const __experimentalGetAllowedPatterns = createRegistrySelector(
name,
rootClientId
)
- : isBlockVisibleInTheInserter( state, name )
+ : isBlockVisibleInTheInserter(
+ state,
+ name,
+ rootClientId
+ )
)
);
@@ -2774,6 +2776,9 @@ export function isNavigationMode( state ) {
*/
export const __unstableGetEditorMode = createRegistrySelector(
( select ) => ( state ) => {
+ if ( ! window?.__experimentalEditorWriteMode ) {
+ return 'edit';
+ }
return (
state.settings.editorTool ??
select( preferencesStore ).get( 'core', 'editorTool' )
@@ -2997,14 +3002,6 @@ export function __unstableIsWithinBlockOverlay( state, clientId ) {
return false;
}
-function isWithinBlock( state, clientId, parentClientId ) {
- let parent = state.blocks.parents.get( clientId );
- while ( !! parent && parent !== parentClientId ) {
- parent = state.blocks.parents.get( parent );
- }
- return parent === parentClientId;
-}
-
/**
* @typedef {import('../components/block-editing-mode').BlockEditingMode} BlockEditingMode
*/
@@ -3046,68 +3043,26 @@ export const getBlockEditingMode = createRegistrySelector(
clientId = '';
}
- // In zoom-out mode, override the behavior set by
- // __unstableSetBlockEditingMode to only allow editing the top-level
- // sections.
- if ( isZoomOut( state ) ) {
- const sectionRootClientId = getSectionRootClientId( state );
-
- if ( clientId === '' /* ROOT_CONTAINER_CLIENT_ID */ ) {
- return sectionRootClientId ? 'disabled' : 'contentOnly';
- }
- if ( clientId === sectionRootClientId ) {
- return 'contentOnly';
- }
- const sectionsClientIds = getBlockOrder(
- state,
- sectionRootClientId
- );
-
- // Sections are always contentOnly.
- if ( sectionsClientIds?.includes( clientId ) ) {
- return 'contentOnly';
- }
-
- return 'disabled';
+ const isNavMode = isNavigationMode( state );
+
+ // If the editor is currently not in navigation mode, check if the clientId
+ // has an editing mode set in the regular derived map.
+ // There may be an editing mode set here for synced patterns or in zoomed out
+ // mode.
+ if (
+ ! isNavMode &&
+ state.derivedBlockEditingModes?.has( clientId )
+ ) {
+ return state.derivedBlockEditingModes.get( clientId );
}
- const editorMode = __unstableGetEditorMode( state );
- if ( editorMode === 'navigation' ) {
- const sectionRootClientId = getSectionRootClientId( state );
-
- // The root section is "default mode"
- if ( clientId === sectionRootClientId ) {
- return 'default';
- }
-
- // Sections should always be contentOnly in navigation mode.
- const sectionsClientIds = getBlockOrder(
- state,
- sectionRootClientId
- );
- if ( sectionsClientIds.includes( clientId ) ) {
- return 'contentOnly';
- }
-
- // Blocks outside sections should be disabled.
- const isWithinSectionRoot = isWithinBlock(
- state,
- clientId,
- sectionRootClientId
- );
- if ( ! isWithinSectionRoot ) {
- return 'disabled';
- }
-
- // The rest of the blocks depend on whether they are content blocks or not.
- // This "flattens" the sections tree.
- const name = getBlockName( state, clientId );
- const { hasContentRoleAttribute } = unlock(
- select( blocksStore )
- );
- const isContent = hasContentRoleAttribute( name );
-
- return isContent ? 'contentOnly' : 'disabled';
+ // If the editor *is* in navigation mode, the block editing mode states
+ // are stored in the derivedNavModeBlockEditingModes map.
+ if (
+ isNavMode &&
+ state.derivedNavModeBlockEditingModes?.has( clientId )
+ ) {
+ return state.derivedNavModeBlockEditingModes.get( clientId );
}
// In normal mode, consider that an explicitely set editing mode takes over.
@@ -3117,7 +3072,7 @@ export const getBlockEditingMode = createRegistrySelector(
}
// In normal mode, top level is default mode.
- if ( ! clientId ) {
+ if ( clientId === '' ) {
return 'default';
}
diff --git a/packages/block-editor/src/store/test/private-selectors.js b/packages/block-editor/src/store/test/private-selectors.js
index fb1d736e175af..268d463f227d4 100644
--- a/packages/block-editor/src/store/test/private-selectors.js
+++ b/packages/block-editor/src/store/test/private-selectors.js
@@ -129,6 +129,7 @@ describe( 'private selectors', () => {
getBlockEditingMode.registry = {
select: jest.fn( () => ( {
hasContentRoleAttribute,
+ get,
} ) ),
};
__unstableGetEditorMode.registry = {
diff --git a/packages/block-editor/src/store/test/reducer.js b/packages/block-editor/src/store/test/reducer.js
index c99d639ba8a09..dd1665d6736ad 100644
--- a/packages/block-editor/src/store/test/reducer.js
+++ b/packages/block-editor/src/store/test/reducer.js
@@ -10,7 +10,10 @@ import {
registerBlockType,
unregisterBlockType,
createBlock,
+ privateApis,
} from '@wordpress/blocks';
+import { combineReducers, select } from '@wordpress/data';
+import { store as preferencesStore } from '@wordpress/preferences';
/**
* Internal dependencies
@@ -38,8 +41,30 @@ import {
blockEditingModes,
openedBlockSettingsMenu,
expandedBlock,
+ zoomLevel,
+ withDerivedBlockEditingModes,
} from '../reducer';
+import { unlock } from '../../lock-unlock';
+import { sectionRootClientIdKey } from '.././private-keys';
+
+const { isContentBlock } = unlock( privateApis );
+
+jest.mock( '@wordpress/data/src/select', () => {
+ const actualSelect = jest.requireActual( '@wordpress/data/src/select' );
+
+ return {
+ select: jest.fn( ( ...args ) => actualSelect.select( ...args ) ),
+ };
+} );
+
+jest.mock( '@wordpress/blocks/src/api/utils', () => {
+ return {
+ ...jest.requireActual( '@wordpress/blocks/src/api/utils' ),
+ isContentBlock: jest.fn(),
+ };
+} );
+
const noop = () => {};
describe( 'state', () => {
@@ -3544,4 +3569,828 @@ describe( 'state', () => {
expect( state ).toBe( null );
} );
} );
+
+ describe( 'withDerivedBlockEditingModes', () => {
+ const testReducer = withDerivedBlockEditingModes(
+ combineReducers( {
+ blocks,
+ settings,
+ zoomLevel,
+ } )
+ );
+
+ function dispatchActions( actions, reducer, initialState = {} ) {
+ return actions.reduce( ( _state, action ) => {
+ return reducer( _state, action );
+ }, initialState );
+ }
+
+ beforeEach( () => {
+ isContentBlock.mockImplementation(
+ ( blockName ) => blockName === 'core/paragraph'
+ );
+ } );
+
+ afterAll( () => {
+ isContentBlock.mockRestore();
+ } );
+
+ describe( 'edit mode', () => {
+ let initialState;
+ beforeAll( () => {
+ select.mockImplementation( ( storeName ) => {
+ if ( storeName === preferencesStore ) {
+ return {
+ get: jest.fn( () => 'edit' ),
+ };
+ }
+ return select( storeName );
+ } );
+
+ initialState = dispatchActions(
+ [
+ {
+ type: 'UPDATE_SETTINGS',
+ settings: {
+ [ sectionRootClientIdKey ]: '',
+ },
+ },
+ {
+ type: 'RESET_BLOCKS',
+ blocks: [
+ {
+ name: 'core/group',
+ clientId: 'group-1',
+ attributes: {},
+ innerBlocks: [
+ {
+ name: 'core/paragraph',
+ clientId: 'paragraph-1',
+ attributes: {},
+ innerBlocks: [],
+ },
+ {
+ name: 'core/group',
+ clientId: 'group-2',
+ attributes: {},
+ innerBlocks: [
+ {
+ name: 'core/paragraph',
+ clientId: 'paragraph-2',
+ attributes: {},
+ innerBlocks: [],
+ },
+ ],
+ },
+ ],
+ },
+ ],
+ },
+ ],
+ testReducer
+ );
+ } );
+
+ afterAll( () => {
+ select.mockRestore();
+ } );
+
+ it( 'returns no block editing modes when zoomed out / navigation mode are not active and there are no synced patterns', () => {
+ expect( initialState.derivedBlockEditingModes ).toEqual(
+ new Map()
+ );
+ } );
+ } );
+
+ describe( 'synced patterns', () => {
+ let initialState;
+ beforeAll( () => {
+ select.mockImplementation( ( storeName ) => {
+ if ( storeName === preferencesStore ) {
+ return {
+ get: jest.fn( () => 'edit' ),
+ };
+ }
+ return select( storeName );
+ } );
+
+ // Simulates how the editor typically inserts controlled blocks,
+ // - first the pattern is inserted with no inner blocks.
+ // - next the pattern is marked as a controlled block.
+ // - finally, once the inner blocks of the pattern are received, they're inserted.
+ // This process is repeated for the two patterns in this test.
+ initialState = dispatchActions(
+ [
+ {
+ type: 'UPDATE_SETTINGS',
+ settings: {
+ [ sectionRootClientIdKey ]: '',
+ },
+ },
+ {
+ type: 'RESET_BLOCKS',
+ blocks: [
+ {
+ name: 'core/group',
+ clientId: 'group-1',
+ attributes: {},
+ innerBlocks: [
+ {
+ name: 'core/paragraph',
+ clientId: 'paragraph-1',
+ attributes: {},
+ innerBlocks: [],
+ },
+ {
+ name: 'core/group',
+ clientId: 'group-2',
+ attributes: {},
+ innerBlocks: [
+ {
+ name: 'core/paragraph',
+ clientId: 'paragraph-2',
+ attributes: {},
+ innerBlocks: [],
+ },
+ ],
+ },
+ ],
+ },
+ ],
+ },
+ {
+ type: 'INSERT_BLOCKS',
+ rootClientId: '',
+ blocks: [
+ {
+ name: 'core/block',
+ clientId: 'root-pattern',
+ attributes: {},
+ innerBlocks: [],
+ },
+ ],
+ },
+ {
+ type: 'SET_HAS_CONTROLLED_INNER_BLOCKS',
+ clientId: 'root-pattern',
+ hasControlledInnerBlocks: true,
+ },
+ {
+ type: 'REPLACE_INNER_BLOCKS',
+ rootClientId: 'root-pattern',
+ blocks: [
+ {
+ name: 'core/block',
+ clientId: 'nested-pattern',
+ attributes: {},
+ innerBlocks: [],
+ },
+ {
+ name: 'core/paragraph',
+ clientId: 'pattern-paragraph',
+ attributes: {},
+ innerBlocks: [],
+ },
+ {
+ name: 'core/group',
+ clientId: 'pattern-group',
+ attributes: {},
+ innerBlocks: [
+ {
+ name: 'core/paragraph',
+ clientId:
+ 'pattern-paragraph-with-overrides',
+ attributes: {
+ metadata: {
+ bindings: {
+ __default:
+ 'core/pattern-overrides',
+ },
+ },
+ },
+ innerBlocks: [],
+ },
+ ],
+ },
+ ],
+ },
+ {
+ type: 'SET_HAS_CONTROLLED_INNER_BLOCKS',
+ clientId: 'nested-pattern',
+ hasControlledInnerBlocks: true,
+ },
+ {
+ type: 'REPLACE_INNER_BLOCKS',
+ rootClientId: 'nested-pattern',
+ blocks: [
+ {
+ name: 'core/paragraph',
+ clientId: 'nested-paragraph',
+ attributes: {},
+ innerBlocks: [],
+ },
+ {
+ name: 'core/group',
+ clientId: 'nested-group',
+ attributes: {},
+ innerBlocks: [
+ {
+ name: 'core/paragraph',
+ clientId:
+ 'nested-paragraph-with-overrides',
+ attributes: {
+ metadata: {
+ bindings: {
+ __default:
+ 'core/pattern-overrides',
+ },
+ },
+ },
+ innerBlocks: [],
+ },
+ ],
+ },
+ ],
+ },
+ ],
+ testReducer,
+ initialState
+ );
+ } );
+
+ afterAll( () => {
+ select.mockRestore();
+ } );
+
+ it( 'returns the expected block editing modes for synced patterns', () => {
+ // Only the parent pattern and its own children that have bindings
+ // are in contentOnly mode. All other blocks are disabled.
+ expect( initialState.derivedBlockEditingModes ).toEqual(
+ new Map(
+ Object.entries( {
+ 'pattern-paragraph': 'disabled',
+ 'pattern-group': 'disabled',
+ 'pattern-paragraph-with-overrides': 'contentOnly',
+ 'nested-pattern': 'disabled',
+ 'nested-paragraph': 'disabled',
+ 'nested-group': 'disabled',
+ 'nested-paragraph-with-overrides': 'disabled',
+ } )
+ )
+ );
+ } );
+
+ it( 'removes block editing modes when synced patterns are removed', () => {
+ const { derivedBlockEditingModes } = dispatchActions(
+ [
+ {
+ type: 'REMOVE_BLOCKS',
+ clientIds: [ 'root-pattern' ],
+ },
+ ],
+ testReducer,
+ initialState
+ );
+
+ expect( derivedBlockEditingModes ).toEqual( new Map() );
+ } );
+
+ it( 'returns the expected block editing modes for synced patterns when switching to navigation mode', () => {
+ select.mockImplementation( ( storeName ) => {
+ if ( storeName === preferencesStore ) {
+ return {
+ get: jest.fn( () => 'navigation' ),
+ };
+ }
+ return select( storeName );
+ } );
+
+ const {
+ derivedBlockEditingModes,
+ derivedNavModeBlockEditingModes,
+ } = dispatchActions(
+ [
+ {
+ type: 'SET_EDITOR_MODE',
+ mode: 'navigation',
+ },
+ ],
+ testReducer,
+ initialState
+ );
+
+ expect( derivedBlockEditingModes ).toEqual(
+ new Map(
+ Object.entries( {
+ 'pattern-paragraph': 'disabled',
+ 'pattern-group': 'disabled',
+ 'pattern-paragraph-with-overrides': 'contentOnly', // Pattern child with bindings.
+ 'nested-pattern': 'disabled',
+ 'nested-paragraph': 'disabled',
+ 'nested-group': 'disabled',
+ 'nested-paragraph-with-overrides': 'disabled',
+ } )
+ )
+ );
+
+ expect( derivedNavModeBlockEditingModes ).toEqual(
+ new Map(
+ Object.entries( {
+ '': 'contentOnly', // Section root.
+ 'group-1': 'contentOnly', // Section.
+ 'paragraph-1': 'contentOnly', // Content block in section.
+ 'group-2': 'disabled',
+ 'paragraph-2': 'contentOnly', // Content block in section.
+ 'root-pattern': 'contentOnly', // Section.
+ 'pattern-paragraph': 'disabled',
+ 'pattern-group': 'disabled',
+ 'pattern-paragraph-with-overrides': 'contentOnly', // Pattern child with bindings.
+ 'nested-pattern': 'disabled',
+ 'nested-paragraph': 'disabled',
+ 'nested-group': 'disabled',
+ 'nested-paragraph-with-overrides': 'disabled',
+ } )
+ )
+ );
+
+ select.mockImplementation( ( storeName ) => {
+ if ( storeName === preferencesStore ) {
+ return {
+ get: jest.fn( () => 'edit' ),
+ };
+ }
+ return select( storeName );
+ } );
+ } );
+
+ it( 'returns the expected block editing modes for synced patterns when switching to zoomed out mode', () => {
+ const { derivedBlockEditingModes } = dispatchActions(
+ [
+ {
+ type: 'SET_ZOOM_LEVEL',
+ zoom: 'auto-scaled',
+ },
+ ],
+ testReducer,
+ initialState
+ );
+
+ expect( derivedBlockEditingModes ).toEqual(
+ new Map(
+ Object.entries( {
+ '': 'contentOnly', // Section root.
+ 'group-1': 'contentOnly', // Section.
+ 'paragraph-1': 'disabled',
+ 'group-2': 'disabled',
+ 'paragraph-2': 'disabled',
+ 'root-pattern': 'contentOnly', // Pattern and section.
+ 'pattern-paragraph': 'disabled',
+ 'pattern-group': 'disabled',
+ 'pattern-paragraph-with-overrides': 'disabled',
+ 'nested-pattern': 'disabled',
+ 'nested-paragraph': 'disabled',
+ 'nested-group': 'disabled',
+ 'nested-paragraph-with-overrides': 'disabled',
+ } )
+ )
+ );
+ } );
+ } );
+
+ describe( 'navigation mode', () => {
+ let initialState;
+
+ beforeAll( () => {
+ select.mockImplementation( ( storeName ) => {
+ if ( storeName === preferencesStore ) {
+ return {
+ get: jest.fn( () => 'navigation' ),
+ };
+ }
+ return select( storeName );
+ } );
+
+ initialState = dispatchActions(
+ [
+ {
+ type: 'UPDATE_SETTINGS',
+ settings: {
+ [ sectionRootClientIdKey ]: '',
+ },
+ },
+ {
+ type: 'RESET_BLOCKS',
+ blocks: [
+ {
+ name: 'core/group',
+ clientId: 'group-1',
+ attributes: {},
+ innerBlocks: [
+ {
+ name: 'core/paragraph',
+ clientId: 'paragraph-1',
+ attributes: {},
+ innerBlocks: [],
+ },
+ {
+ name: 'core/group',
+ clientId: 'group-2',
+ attributes: {},
+ innerBlocks: [
+ {
+ name: 'core/paragraph',
+ clientId: 'paragraph-2',
+ attributes: {},
+ innerBlocks: [],
+ },
+ ],
+ },
+ ],
+ },
+ ],
+ },
+ ],
+ testReducer
+ );
+ } );
+
+ afterAll( () => {
+ select.mockRestore();
+ } );
+
+ it( 'returns the expected block editing modes', () => {
+ expect( initialState.derivedNavModeBlockEditingModes ).toEqual(
+ new Map(
+ Object.entries( {
+ '': 'contentOnly', // Section root.
+ 'group-1': 'contentOnly', // Section block.
+ 'paragraph-1': 'contentOnly', // Content block in section.
+ 'group-2': 'disabled', // Non-content block in section.
+ 'paragraph-2': 'contentOnly', // Content block in section.
+ } )
+ )
+ );
+ } );
+
+ it( 'removes block editing modes when blocks are removed', () => {
+ const { derivedNavModeBlockEditingModes } = dispatchActions(
+ [
+ {
+ type: 'REMOVE_BLOCKS',
+ clientIds: [ 'group-2' ],
+ },
+ ],
+ testReducer,
+ initialState
+ );
+
+ expect( derivedNavModeBlockEditingModes ).toEqual(
+ new Map(
+ Object.entries( {
+ '': 'contentOnly',
+ 'group-1': 'contentOnly',
+ 'paragraph-1': 'contentOnly',
+ } )
+ )
+ );
+ } );
+
+ it( 'updates block editing modes when new blocks are inserted', () => {
+ const { derivedNavModeBlockEditingModes } = dispatchActions(
+ [
+ {
+ type: 'INSERT_BLOCKS',
+ rootClientId: '',
+ blocks: [
+ {
+ name: 'core/group',
+ clientId: 'group-3',
+ attributes: {},
+ innerBlocks: [
+ {
+ name: 'core/paragraph',
+ clientId: 'paragraph-3',
+ attributes: {},
+ innerBlocks: [],
+ },
+ {
+ name: 'core/group',
+ clientId: 'group-4',
+ attributes: {},
+ innerBlocks: [],
+ },
+ ],
+ },
+ ],
+ },
+ ],
+ testReducer,
+ initialState
+ );
+
+ expect( derivedNavModeBlockEditingModes ).toEqual(
+ new Map(
+ Object.entries( {
+ '': 'contentOnly', // Section root.
+ 'group-1': 'contentOnly', // Section block.
+ 'paragraph-1': 'contentOnly', // Content block in section.
+ 'group-2': 'disabled', // Non-content block in section.
+ 'paragraph-2': 'contentOnly', // Content block in section.
+ 'group-3': 'contentOnly', // New section block.
+ 'paragraph-3': 'contentOnly', // New content block in section.
+ 'group-4': 'disabled', // Non-content block in section.
+ } )
+ )
+ );
+ } );
+
+ it( 'updates block editing modes when blocks are moved to a new position', () => {
+ const { derivedNavModeBlockEditingModes } = dispatchActions(
+ [
+ {
+ type: 'MOVE_BLOCKS_TO_POSITION',
+ clientIds: [ 'group-2' ],
+ fromRootClientId: 'group-1',
+ toRootClientId: '',
+ },
+ ],
+ testReducer,
+ initialState
+ );
+ expect( derivedNavModeBlockEditingModes ).toEqual(
+ new Map(
+ Object.entries( {
+ '': 'contentOnly', // Section root.
+ 'group-1': 'contentOnly', // Section block.
+ 'paragraph-1': 'contentOnly', // Content block in section.
+ 'group-2': 'contentOnly', // New section block.
+ 'paragraph-2': 'contentOnly', // Still a content block in a section.
+ } )
+ )
+ );
+ } );
+
+ it( 'handles changes to the section root', () => {
+ const { derivedNavModeBlockEditingModes } = dispatchActions(
+ [
+ {
+ type: 'UPDATE_SETTINGS',
+ settings: {
+ [ sectionRootClientIdKey ]: 'group-1',
+ },
+ },
+ ],
+ testReducer,
+ initialState
+ );
+
+ expect( derivedNavModeBlockEditingModes ).toEqual(
+ new Map(
+ Object.entries( {
+ '': 'disabled',
+ 'group-1': 'contentOnly',
+ 'paragraph-1': 'contentOnly',
+ 'group-2': 'contentOnly',
+ 'paragraph-2': 'contentOnly',
+ } )
+ )
+ );
+ } );
+ } );
+
+ describe( 'zoom out mode', () => {
+ let initialState;
+
+ beforeAll( () => {
+ initialState = dispatchActions(
+ [
+ {
+ type: 'UPDATE_SETTINGS',
+ settings: {
+ [ sectionRootClientIdKey ]: '',
+ },
+ },
+ {
+ type: 'SET_ZOOM_LEVEL',
+ zoom: 'auto-scaled',
+ },
+ {
+ type: 'RESET_BLOCKS',
+ blocks: [
+ {
+ name: 'core/group',
+ clientId: 'group-1',
+ attributes: {},
+ innerBlocks: [
+ {
+ name: 'core/paragraph',
+ clientId: 'paragraph-1',
+ attributes: {},
+ innerBlocks: [],
+ },
+ {
+ name: 'core/group',
+ clientId: 'group-2',
+ attributes: {},
+ innerBlocks: [
+ {
+ name: 'core/paragraph',
+ clientId: 'paragraph-2',
+ attributes: {},
+ innerBlocks: [],
+ },
+ ],
+ },
+ ],
+ },
+ ],
+ },
+ ],
+ testReducer
+ );
+ } );
+
+ it( 'returns the expected block editing modes', () => {
+ expect( initialState.derivedBlockEditingModes ).toEqual(
+ new Map(
+ Object.entries( {
+ '': 'contentOnly', // Section root.
+ 'group-1': 'contentOnly', // Section block.
+ 'paragraph-1': 'disabled',
+ 'group-2': 'disabled',
+ 'paragraph-2': 'disabled',
+ } )
+ )
+ );
+ } );
+
+ it( 'overrides navigation mode', () => {
+ select.mockImplementation( ( storeName ) => {
+ if ( storeName === preferencesStore ) {
+ return {
+ get: jest.fn( () => 'navigation' ),
+ };
+ }
+ return select( storeName );
+ } );
+
+ const { derivedBlockEditingModes } = dispatchActions(
+ [
+ {
+ type: 'SET_EDITOR_MODE',
+ mode: 'navigation',
+ },
+ ],
+ testReducer,
+ initialState
+ );
+
+ expect( derivedBlockEditingModes ).toEqual(
+ new Map(
+ Object.entries( {
+ '': 'contentOnly', // Section root.
+ 'group-1': 'contentOnly', // Section block.
+ 'paragraph-1': 'disabled',
+ 'group-2': 'disabled',
+ 'paragraph-2': 'disabled',
+ } )
+ )
+ );
+
+ select.mockImplementation( ( storeName ) => {
+ if ( storeName === preferencesStore ) {
+ return {
+ get: jest.fn( () => 'edit' ),
+ };
+ }
+ return select( storeName );
+ } );
+ } );
+
+ it( 'removes block editing modes when blocks are removed', () => {
+ const { derivedBlockEditingModes } = dispatchActions(
+ [
+ {
+ type: 'REMOVE_BLOCKS',
+ clientIds: [ 'group-2' ],
+ },
+ ],
+ testReducer,
+ initialState
+ );
+
+ expect( derivedBlockEditingModes ).toEqual(
+ new Map(
+ Object.entries( {
+ '': 'contentOnly',
+ 'group-1': 'contentOnly',
+ 'paragraph-1': 'disabled',
+ } )
+ )
+ );
+ } );
+
+ it( 'updates block editing modes when new blocks are inserted', () => {
+ const { derivedBlockEditingModes } = dispatchActions(
+ [
+ {
+ type: 'INSERT_BLOCKS',
+ rootClientId: '',
+ blocks: [
+ {
+ name: 'core/group',
+ clientId: 'group-3',
+ attributes: {},
+ innerBlocks: [
+ {
+ name: 'core/paragraph',
+ clientId: 'paragraph-3',
+ attributes: {},
+ innerBlocks: [],
+ },
+ {
+ name: 'core/group',
+ clientId: 'group-4',
+ attributes: {},
+ innerBlocks: [],
+ },
+ ],
+ },
+ ],
+ },
+ ],
+ testReducer,
+ initialState
+ );
+
+ expect( derivedBlockEditingModes ).toEqual(
+ new Map(
+ Object.entries( {
+ '': 'contentOnly', // Section root.
+ 'group-1': 'contentOnly', // Section block.
+ 'paragraph-1': 'disabled',
+ 'group-2': 'disabled',
+ 'paragraph-2': 'disabled',
+ 'group-3': 'contentOnly', // New section block.
+ 'paragraph-3': 'disabled',
+ 'group-4': 'disabled',
+ } )
+ )
+ );
+ } );
+
+ it( 'updates block editing modes when blocks are moved to a new position', () => {
+ const { derivedBlockEditingModes } = dispatchActions(
+ [
+ {
+ type: 'MOVE_BLOCKS_TO_POSITION',
+ clientIds: [ 'group-2' ],
+ fromRootClientId: 'group-1',
+ toRootClientId: '',
+ },
+ ],
+ testReducer,
+ initialState
+ );
+ expect( derivedBlockEditingModes ).toEqual(
+ new Map(
+ Object.entries( {
+ '': 'contentOnly', // Section root.
+ 'group-1': 'contentOnly', // Section block.
+ 'paragraph-1': 'disabled',
+ 'group-2': 'contentOnly', // New section block.
+ 'paragraph-2': 'disabled',
+ } )
+ )
+ );
+ } );
+
+ it( 'handles changes to the section root', () => {
+ const { derivedBlockEditingModes } = dispatchActions(
+ [
+ {
+ type: 'UPDATE_SETTINGS',
+ settings: {
+ [ sectionRootClientIdKey ]: 'group-1',
+ },
+ },
+ ],
+ testReducer,
+ initialState
+ );
+
+ expect( derivedBlockEditingModes ).toEqual(
+ new Map(
+ Object.entries( {
+ '': 'disabled',
+ 'group-1': 'contentOnly', // New section root.
+ 'paragraph-1': 'contentOnly', // Section block.
+ 'group-2': 'contentOnly', // Section block.
+ 'paragraph-2': 'disabled',
+ } )
+ )
+ );
+ } );
+ } );
+ } );
} );
diff --git a/packages/block-editor/src/store/test/selectors.js b/packages/block-editor/src/store/test/selectors.js
index 00aa085f66709..51949bfd468ca 100644
--- a/packages/block-editor/src/store/test/selectors.js
+++ b/packages/block-editor/src/store/test/selectors.js
@@ -9,14 +9,12 @@ import {
import { RawHTML } from '@wordpress/element';
import { symbol } from '@wordpress/icons';
import { select, dispatch } from '@wordpress/data';
-import { store as preferencesStore } from '@wordpress/preferences';
/**
* Internal dependencies
*/
import * as selectors from '../selectors';
import { store } from '../';
-import { sectionRootClientIdKey } from '../private-keys';
import { lock } from '../../lock-unlock';
const {
@@ -3326,7 +3324,7 @@ describe( 'selectors', () => {
settings: {},
blockEditingModes: new Map(),
};
- expect( canInsertBlocks( state, [ '2', '3' ], '1' ) ).toBe( true );
+ expect( canInsertBlocks( state, [ '2' ], '1' ) ).toBe( true );
} );
it( 'should deny blocks', () => {
@@ -4469,29 +4467,19 @@ describe( 'getBlockEditingMode', () => {
blockEditingModes: new Map( [] ),
};
- const navigationModeStateWithRootSection = {
- ...baseState,
- settings: {
- [ sectionRootClientIdKey ]: 'ef45d5fd-5234-4fd5-ac4f-c3736c7f9337', // The group is the "main" container
- },
- };
-
const hasContentRoleAttribute = jest.fn( () => false );
+ const get = jest.fn( () => 'edit' );
- const fauxPrivateAPIs = {};
+ const mockedSelectors = { get };
- lock( fauxPrivateAPIs, {
+ lock( mockedSelectors, {
hasContentRoleAttribute,
} );
getBlockEditingMode.registry = {
- select: jest.fn( () => fauxPrivateAPIs ),
+ select: jest.fn( () => mockedSelectors ),
};
- afterEach( () => {
- dispatch( preferencesStore ).set( 'core', 'editorTool', undefined );
- } );
-
it( 'should return default by default', () => {
expect(
getBlockEditingMode(
@@ -4614,69 +4602,4 @@ describe( 'getBlockEditingMode', () => {
getBlockEditingMode( state, 'b3247f75-fd94-4fef-97f9-5bfd162cc416' )
).toBe( 'contentOnly' );
} );
-
- it( 'in navigation mode, the root section container is default', () => {
- dispatch( preferencesStore ).set( 'core', 'editorTool', 'navigation' );
- expect(
- getBlockEditingMode(
- navigationModeStateWithRootSection,
- 'ef45d5fd-5234-4fd5-ac4f-c3736c7f9337'
- )
- ).toBe( 'default' );
- } );
-
- it( 'in navigation mode, anything outside the section container is disabled', () => {
- dispatch( preferencesStore ).set( 'core', 'editorTool', 'navigation' );
- expect(
- getBlockEditingMode(
- navigationModeStateWithRootSection,
- '6cf70164-9097-4460-bcbf-200560546988'
- )
- ).toBe( 'disabled' );
- } );
-
- it( 'in navigation mode, sections are contentOnly', () => {
- dispatch( preferencesStore ).set( 'core', 'editorTool', 'navigation' );
- expect(
- getBlockEditingMode(
- navigationModeStateWithRootSection,
- 'b26fc763-417d-4f01-b81c-2ec61e14a972'
- )
- ).toBe( 'contentOnly' );
- expect(
- getBlockEditingMode(
- navigationModeStateWithRootSection,
- '9b9c5c3f-2e46-4f02-9e14-9fe9515b958f'
- )
- ).toBe( 'contentOnly' );
- } );
-
- it( 'in navigation mode, blocks with content attributes within sections are contentOnly', () => {
- dispatch( preferencesStore ).set( 'core', 'editorTool', 'navigation' );
- hasContentRoleAttribute.mockReturnValueOnce( true );
- expect(
- getBlockEditingMode(
- navigationModeStateWithRootSection,
- 'b3247f75-fd94-4fef-97f9-5bfd162cc416'
- )
- ).toBe( 'contentOnly' );
-
- hasContentRoleAttribute.mockReturnValueOnce( true );
- expect(
- getBlockEditingMode(
- navigationModeStateWithRootSection,
- 'e178812d-ce5e-48c7-a945-8ae4ffcbbb7c'
- )
- ).toBe( 'contentOnly' );
- } );
-
- it( 'in navigation mode, blocks without content attributes within sections are disabled', () => {
- dispatch( preferencesStore ).set( 'core', 'editorTool', 'navigation' );
- expect(
- getBlockEditingMode(
- navigationModeStateWithRootSection,
- '9b9c5c3f-2e46-4f02-9e14-9fed515b958s'
- )
- ).toBe( 'disabled' );
- } );
} );
diff --git a/packages/block-editor/src/style.scss b/packages/block-editor/src/style.scss
index 512169351fe1f..6b2ebf5cd841f 100644
--- a/packages/block-editor/src/style.scss
+++ b/packages/block-editor/src/style.scss
@@ -10,6 +10,7 @@
@import "./components/block-card/style.scss";
@import "./components/block-compare/style.scss";
@import "./components/block-draggable/style.scss";
+@import "./components/block-manager/style.scss";
@import "./components/block-mover/style.scss";
@import "./components/block-navigation/style.scss";
@import "./components/block-patterns-list/style.scss";
@@ -27,6 +28,7 @@
@import "./components/date-format-picker/style.scss";
@import "./components/duotone-control/style.scss";
@import "./components/font-appearance-control/style.scss";
+@import "./components/font-family/style.scss";
@import "./components/global-styles/style.scss";
@import "./components/grid/style.scss";
@import "./components/height-control/style.scss";
diff --git a/packages/block-editor/src/utils/block-bindings.js b/packages/block-editor/src/utils/block-bindings.js
index dcf80d985473b..9a4c6acf9a903 100644
--- a/packages/block-editor/src/utils/block-bindings.js
+++ b/packages/block-editor/src/utils/block-bindings.js
@@ -9,10 +9,105 @@ import { useDispatch, useRegistry } from '@wordpress/data';
import { store as blockEditorStore } from '../store';
import { useBlockEditContext } from '../components/block-edit';
+const DEFAULT_ATTRIBUTE = '__default';
+const PATTERN_OVERRIDES_SOURCE = 'core/pattern-overrides';
+const BLOCK_BINDINGS_ALLOWED_BLOCKS = {
+ 'core/paragraph': [ 'content' ],
+ 'core/heading': [ 'content' ],
+ 'core/image': [ 'id', 'url', 'title', 'alt' ],
+ 'core/button': [ 'url', 'text', 'linkTarget', 'rel' ],
+};
+
+/**
+ * Checks if the given object is empty.
+ *
+ * @param {?Object} object The object to check.
+ *
+ * @return {boolean} Whether the object is empty.
+ */
function isObjectEmpty( object ) {
return ! object || Object.keys( object ).length === 0;
}
+/**
+ * Based on the given block name, checks if it is possible to bind the block.
+ *
+ * @param {string} blockName The name of the block.
+ *
+ * @return {boolean} Whether it is possible to bind the block to sources.
+ */
+export function canBindBlock( blockName ) {
+ return blockName in BLOCK_BINDINGS_ALLOWED_BLOCKS;
+}
+
+/**
+ * Based on the given block name and attribute name, checks if it is possible to bind the block attribute.
+ *
+ * @param {string} blockName The name of the block.
+ * @param {string} attributeName The name of attribute.
+ *
+ * @return {boolean} Whether it is possible to bind the block attribute.
+ */
+export function canBindAttribute( blockName, attributeName ) {
+ return (
+ canBindBlock( blockName ) &&
+ BLOCK_BINDINGS_ALLOWED_BLOCKS[ blockName ].includes( attributeName )
+ );
+}
+
+/**
+ * Gets the bindable attributes for a given block.
+ *
+ * @param {string} blockName The name of the block.
+ *
+ * @return {string[]} The bindable attributes for the block.
+ */
+export function getBindableAttributes( blockName ) {
+ return BLOCK_BINDINGS_ALLOWED_BLOCKS[ blockName ];
+}
+
+/**
+ * Checks if the block has the `__default` binding for pattern overrides.
+ *
+ * @param {?Record} bindings A block's bindings from the metadata attribute.
+ *
+ * @return {boolean} Whether the block has the `__default` binding for pattern overrides.
+ */
+export function hasPatternOverridesDefaultBinding( bindings ) {
+ return bindings?.[ DEFAULT_ATTRIBUTE ]?.source === PATTERN_OVERRIDES_SOURCE;
+}
+
+/**
+ * Returns the bindings with the `__default` binding for pattern overrides
+ * replaced with the full-set of supported attributes. e.g.:
+ *
+ * - bindings passed in: `{ __default: { source: 'core/pattern-overrides' } }`
+ * - bindings returned: `{ content: { source: 'core/pattern-overrides' } }`
+ *
+ * @param {string} blockName The block name (e.g. 'core/paragraph').
+ * @param {?Record} bindings A block's bindings from the metadata attribute.
+ *
+ * @return {Object} The bindings with default replaced for pattern overrides.
+ */
+export function replacePatternOverridesDefaultBinding( blockName, bindings ) {
+ // The `__default` binding currently only works for pattern overrides.
+ if ( hasPatternOverridesDefaultBinding( bindings ) ) {
+ const supportedAttributes = BLOCK_BINDINGS_ALLOWED_BLOCKS[ blockName ];
+ const bindingsWithDefaults = {};
+ for ( const attributeName of supportedAttributes ) {
+ // If the block has mixed binding sources, retain any non pattern override bindings.
+ const bindingSource = bindings[ attributeName ]
+ ? bindings[ attributeName ]
+ : { source: PATTERN_OVERRIDES_SOURCE };
+ bindingsWithDefaults[ attributeName ] = bindingSource;
+ }
+
+ return bindingsWithDefaults;
+ }
+
+ return bindings;
+}
+
/**
* Contains utils to update the block `bindings` metadata.
*
diff --git a/packages/block-library/CHANGELOG.md b/packages/block-library/CHANGELOG.md
index 4b5cf59f81910..823d89ecd854f 100644
--- a/packages/block-library/CHANGELOG.md
+++ b/packages/block-library/CHANGELOG.md
@@ -2,6 +2,12 @@
## Unreleased
+## 9.14.0 (2024-12-11)
+
+## 9.13.0 (2024-11-27)
+
+## 9.12.0 (2024-11-16)
+
## 9.11.0 (2024-10-30)
## 9.10.0 (2024-10-16)
diff --git a/packages/block-library/package.json b/packages/block-library/package.json
index b196e53e5cd0f..d7cc75bc17764 100644
--- a/packages/block-library/package.json
+++ b/packages/block-library/package.json
@@ -1,6 +1,6 @@
{
"name": "@wordpress/block-library",
- "version": "9.11.0",
+ "version": "9.14.0",
"description": "Block library for the WordPress editor.",
"author": "The WordPress Contributors",
"license": "GPL-2.0-or-later",
@@ -28,6 +28,7 @@
"wpScript": true,
"wpScriptModuleExports": {
"./file/view": "./build-module/file/view.js",
+ "./form/view": "./build-module/form/view.js",
"./image/view": "./build-module/image/view.js",
"./navigation/view": "./build-module/navigation/view.js",
"./query/view": "./build-module/query/view.js",
diff --git a/packages/block-library/src/archives/edit.js b/packages/block-library/src/archives/edit.js
index 60b8715988ed9..d4f25da8507f3 100644
--- a/packages/block-library/src/archives/edit.js
+++ b/packages/block-library/src/archives/edit.js
@@ -2,70 +2,128 @@
* WordPress dependencies
*/
import {
- PanelBody,
ToggleControl,
SelectControl,
Disabled,
+ __experimentalToolsPanel as ToolsPanel,
+ __experimentalToolsPanelItem as ToolsPanelItem,
} from '@wordpress/components';
import { __ } from '@wordpress/i18n';
import { InspectorControls, useBlockProps } from '@wordpress/block-editor';
import ServerSideRender from '@wordpress/server-side-render';
+/**
+ * Internal dependencies
+ */
+import { useToolsPanelDropdownMenuProps } from '../utils/hooks';
+
export default function ArchivesEdit( { attributes, setAttributes } ) {
const { showLabel, showPostCounts, displayAsDropdown, type } = attributes;
+ const dropdownMenuProps = useToolsPanelDropdownMenuProps();
+
return (
<>
-
- {
+ setAttributes( {
+ displayAsDropdown: false,
+ showLabel: false,
+ showPostCounts: false,
+ type: 'monthly',
+ } );
+ } }
+ dropdownMenuProps={ dropdownMenuProps }
+ >
+
- setAttributes( {
- displayAsDropdown: ! displayAsDropdown,
- } )
+ isShownByDefault
+ hasValue={ () => displayAsDropdown }
+ onDeselect={ () =>
+ setAttributes( { displayAsDropdown: false } )
}
- />
- { displayAsDropdown && (
+ >
setAttributes( {
- showLabel: ! showLabel,
+ displayAsDropdown: ! displayAsDropdown,
} )
}
/>
+
+
+ { displayAsDropdown && (
+ showLabel }
+ onDeselect={ () =>
+ setAttributes( { showLabel: false } )
+ }
+ >
+
+ setAttributes( {
+ showLabel: ! showLabel,
+ } )
+ }
+ />
+
) }
-
- setAttributes( {
- showPostCounts: ! showPostCounts,
- } )
+ isShownByDefault
+ hasValue={ () => showPostCounts }
+ onDeselect={ () =>
+ setAttributes( { showPostCounts: false } )
}
- />
-
+
+ setAttributes( {
+ showPostCounts: ! showPostCounts,
+ } )
+ }
+ />
+
+
+
- setAttributes( { type: value } )
+ isShownByDefault
+ hasValue={ () => !! type }
+ onDeselect={ () =>
+ setAttributes( { type: 'monthly' } )
}
- />
-
+ >
+
+ setAttributes( { type: value } )
+ }
+ />
+
+
diff --git a/packages/block-library/src/audio/test/__snapshots__/edit.native.js.snap b/packages/block-library/src/audio/test/__snapshots__/edit.native.js.snap
index 4cf28f7063ad3..9cf88d804068a 100644
--- a/packages/block-library/src/audio/test/__snapshots__/edit.native.js.snap
+++ b/packages/block-library/src/audio/test/__snapshots__/edit.native.js.snap
@@ -89,7 +89,7 @@ exports[`Audio block renders audio block error state without crashing 1`] = `
diff --git a/packages/block-library/src/block-keyboard-shortcuts/index.js b/packages/block-library/src/block-keyboard-shortcuts/index.js
index 6d9cde8364001..2ce106b8ed99d 100644
--- a/packages/block-library/src/block-keyboard-shortcuts/index.js
+++ b/packages/block-library/src/block-keyboard-shortcuts/index.js
@@ -90,22 +90,36 @@ function BlockKeyboardShortcuts() {
},
} );
} );
- }, [] );
+ }, [ registerShortcut ] );
useShortcut(
'core/block-editor/transform-heading-to-paragraph',
( event ) => handleTransformHeadingAndParagraph( event, 0 )
);
-
- [ 1, 2, 3, 4, 5, 6 ].forEach( ( level ) => {
- //the loop is based off on a constant therefore
- //the hook will execute the same way every time
- //eslint-disable-next-line react-hooks/rules-of-hooks
- useShortcut(
- `core/block-editor/transform-paragraph-to-heading-${ level }`,
- ( event ) => handleTransformHeadingAndParagraph( event, level )
- );
- } );
+ useShortcut(
+ 'core/block-editor/transform-paragraph-to-heading-1',
+ ( event ) => handleTransformHeadingAndParagraph( event, 1 )
+ );
+ useShortcut(
+ 'core/block-editor/transform-paragraph-to-heading-2',
+ ( event ) => handleTransformHeadingAndParagraph( event, 2 )
+ );
+ useShortcut(
+ 'core/block-editor/transform-paragraph-to-heading-3',
+ ( event ) => handleTransformHeadingAndParagraph( event, 3 )
+ );
+ useShortcut(
+ 'core/block-editor/transform-paragraph-to-heading-4',
+ ( event ) => handleTransformHeadingAndParagraph( event, 4 )
+ );
+ useShortcut(
+ 'core/block-editor/transform-paragraph-to-heading-5',
+ ( event ) => handleTransformHeadingAndParagraph( event, 5 )
+ );
+ useShortcut(
+ 'core/block-editor/transform-paragraph-to-heading-6',
+ ( event ) => handleTransformHeadingAndParagraph( event, 6 )
+ );
return null;
}
diff --git a/packages/block-library/src/block/edit.js b/packages/block-library/src/block/edit.js
index 104b07157cba7..3d4d07e52b386 100644
--- a/packages/block-library/src/block/edit.js
+++ b/packages/block-library/src/block/edit.js
@@ -7,7 +7,7 @@ import clsx from 'clsx';
* WordPress dependencies
*/
import { useSelect, useDispatch } from '@wordpress/data';
-import { useRef, useMemo, useEffect } from '@wordpress/element';
+import { useRef, useMemo } from '@wordpress/element';
import {
useEntityRecord,
store as coreStore,
@@ -37,12 +37,10 @@ import { getBlockBindingsSource } from '@wordpress/blocks';
/**
* Internal dependencies
*/
-import { name as patternBlockName } from './index';
import { unlock } from '../lock-unlock';
const { useLayoutClasses } = unlock( blockEditorPrivateApis );
-const { isOverridableBlock, hasOverridableBlocks } =
- unlock( patternsPrivateApis );
+const { hasOverridableBlocks } = unlock( patternsPrivateApis );
const fullAlignments = [ 'full', 'wide', 'left', 'right' ];
@@ -75,22 +73,6 @@ const useInferredLayout = ( blocks, parentLayout ) => {
}, [ blocks, parentLayout ] );
};
-function setBlockEditMode( setEditMode, blocks, mode ) {
- blocks.forEach( ( block ) => {
- const editMode =
- mode ||
- ( isOverridableBlock( block ) ? 'contentOnly' : 'disabled' );
- setEditMode( block.clientId, editMode );
-
- setBlockEditMode(
- setEditMode,
- block.innerBlocks,
- // Disable editing for nested patterns.
- block.name === patternBlockName ? 'disabled' : mode
- );
- } );
-}
-
function RecursionWarning() {
const blockProps = useBlockProps();
return (
@@ -171,7 +153,6 @@ function ReusableBlockEdit( {
name,
attributes: { ref, content },
__unstableParentLayout: parentLayout,
- clientId: patternClientId,
setAttributes,
} ) {
const { record, hasResolved } = useEntityRecord(
@@ -184,49 +165,24 @@ function ReusableBlockEdit( {
} );
const isMissing = hasResolved && ! record;
- const { setBlockEditingMode, __unstableMarkLastChangeAsPersistent } =
+ const { __unstableMarkLastChangeAsPersistent } =
useDispatch( blockEditorStore );
- const {
- innerBlocks,
- onNavigateToEntityRecord,
- editingMode,
- hasPatternOverridesSource,
- } = useSelect(
+ const { onNavigateToEntityRecord, hasPatternOverridesSource } = useSelect(
( select ) => {
- const { getBlocks, getSettings, getBlockEditingMode } =
- select( blockEditorStore );
+ const { getSettings } = select( blockEditorStore );
// For editing link to the site editor if the theme and user permissions support it.
return {
- innerBlocks: getBlocks( patternClientId ),
onNavigateToEntityRecord:
getSettings().onNavigateToEntityRecord,
- editingMode: getBlockEditingMode( patternClientId ),
hasPatternOverridesSource: !! getBlockBindingsSource(
'core/pattern-overrides'
),
};
},
- [ patternClientId ]
+ []
);
- // Sync the editing mode of the pattern block with the inner blocks.
- useEffect( () => {
- setBlockEditMode(
- setBlockEditingMode,
- innerBlocks,
- // Disable editing if the pattern itself is disabled.
- editingMode === 'disabled' || ! hasPatternOverridesSource
- ? 'disabled'
- : undefined
- );
- }, [
- editingMode,
- innerBlocks,
- setBlockEditingMode,
- hasPatternOverridesSource,
- ] );
-
const canOverrideBlocks = useMemo(
() => hasPatternOverridesSource && hasOverridableBlocks( blocks ),
[ hasPatternOverridesSource, blocks ]
@@ -244,7 +200,6 @@ function ReusableBlockEdit( {
} );
const innerBlocksProps = useInnerBlocksProps( blockProps, {
- templateLock: 'all',
layout,
value: blocks,
onInput: NOOP,
diff --git a/packages/block-library/src/button/edit.js b/packages/block-library/src/button/edit.js
index 3539fd54f4eec..520da26ef9671 100644
--- a/packages/block-library/src/button/edit.js
+++ b/packages/block-library/src/button/edit.js
@@ -16,12 +16,13 @@ import removeAnchorTag from '../utils/remove-anchor-tag';
import { __ } from '@wordpress/i18n';
import { useEffect, useState, useRef, useMemo } from '@wordpress/element';
import {
- Button,
- ButtonGroup,
- PanelBody,
TextControl,
ToolbarButton,
Popover,
+ __experimentalToolsPanel as ToolsPanel,
+ __experimentalToolsPanelItem as ToolsPanelItem,
+ __experimentalToggleGroupControl as ToggleGroupControl,
+ __experimentalToggleGroupControlOption as ToggleGroupControlOption,
} from '@wordpress/components';
import {
AlignmentControl,
@@ -29,11 +30,11 @@ import {
InspectorControls,
RichText,
useBlockProps,
+ LinkControl,
__experimentalUseBorderProps as useBorderProps,
__experimentalUseColorProps as useColorProps,
__experimentalGetSpacingClassesAndStyles as useSpacingProps,
__experimentalGetShadowClassesAndStyles as useShadowProps,
- __experimentalLinkControl as LinkControl,
__experimentalGetElementClassName,
store as blockEditorStore,
useBlockEditingMode,
@@ -114,35 +115,40 @@ function useEnter( props ) {
}
function WidthPanel( { selectedWidth, setAttributes } ) {
- function handleChange( newWidth ) {
- // Check if we are toggling the width off
- const width = selectedWidth === newWidth ? undefined : newWidth;
-
- // Update attributes.
- setAttributes( { width } );
- }
-
return (
-
-
- { [ 25, 50, 75, 100 ].map( ( widthValue ) => {
- return (
- handleChange( widthValue ) }
- >
- { widthValue }%
-
- );
- } ) }
-
-
+ setAttributes( { width: undefined } ) }
+ >
+ !! selectedWidth }
+ onDeselect={ () => setAttributes( { width: undefined } ) }
+ __nextHasNoMarginBottom
+ >
+
+ setAttributes( { width: newWidth } )
+ }
+ isBlock
+ __next40pxDefaultSize
+ __nextHasNoMarginBottom
+ >
+ { [ 25, 50, 75, 100 ].map( ( widthValue ) => {
+ return (
+
+ );
+ } ) }
+
+
+
);
}
diff --git a/packages/block-library/src/column/edit.js b/packages/block-library/src/column/edit.js
index a0f3cdcf65393..b88e72e8da699 100644
--- a/packages/block-library/src/column/edit.js
+++ b/packages/block-library/src/column/edit.js
@@ -18,8 +18,9 @@ import {
} from '@wordpress/block-editor';
import {
__experimentalUseCustomUnits as useCustomUnits,
- PanelBody,
__experimentalUnitControl as UnitControl,
+ __experimentalToolsPanel as ToolsPanel,
+ __experimentalToolsPanelItem as ToolsPanelItem,
} from '@wordpress/components';
import { useSelect, useDispatch } from '@wordpress/data';
import { sprintf, __ } from '@wordpress/i18n';
@@ -30,19 +31,32 @@ function ColumnInspectorControls( { width, setAttributes } ) {
availableUnits: availableUnits || [ '%', 'px', 'em', 'rem', 'vw' ],
} );
return (
-
- {
+ setAttributes( { width: undefined } );
+ } }
+ >
+ width !== undefined }
label={ __( 'Width' ) }
- __unstableInputWidth="calc(50% - 8px)"
- __next40pxDefaultSize
- value={ width || '' }
- onChange={ ( nextWidth ) => {
- nextWidth = 0 > parseFloat( nextWidth ) ? '0' : nextWidth;
- setAttributes( { width: nextWidth } );
- } }
- units={ units }
- />
-
+ onDeselect={ () => setAttributes( { width: undefined } ) }
+ isShownByDefault
+ >
+ {
+ nextWidth =
+ 0 > parseFloat( nextWidth ) ? '0' : nextWidth;
+ setAttributes( { width: nextWidth } );
+ } }
+ units={ units }
+ />
+
+
);
}
diff --git a/packages/block-library/src/columns/edit.js b/packages/block-library/src/columns/edit.js
index f8cf0297302cc..d79dfe4fc94a4 100644
--- a/packages/block-library/src/columns/edit.js
+++ b/packages/block-library/src/columns/edit.js
@@ -9,9 +9,10 @@ import clsx from 'clsx';
import { __ } from '@wordpress/i18n';
import {
Notice,
- PanelBody,
RangeControl,
ToggleControl,
+ __experimentalToolsPanel as ToolsPanel,
+ __experimentalToolsPanelItem as ToolsPanelItem,
} from '@wordpress/components';
import {
@@ -51,19 +52,15 @@ function ColumnInspectorControls( {
} ) {
const { count, canInsertColumnBlock, minCount } = useSelect(
( select ) => {
- const {
- canInsertBlockType,
- canRemoveBlock,
- getBlocks,
- getBlockCount,
- } = select( blockEditorStore );
- const innerBlocks = getBlocks( clientId );
+ const { canInsertBlockType, canRemoveBlock, getBlockOrder } =
+ select( blockEditorStore );
+ const blockOrder = getBlockOrder( clientId );
// Get the indexes of columns for which removal is prevented.
// The highest index will be used to determine the minimum column count.
- const preventRemovalBlockIndexes = innerBlocks.reduce(
- ( acc, block, index ) => {
- if ( ! canRemoveBlock( block.clientId ) ) {
+ const preventRemovalBlockIndexes = blockOrder.reduce(
+ ( acc, blockId, index ) => {
+ if ( ! canRemoveBlock( blockId ) ) {
acc.push( index );
}
return acc;
@@ -72,7 +69,7 @@ function ColumnInspectorControls( {
);
return {
- count: getBlockCount( clientId ),
+ count: blockOrder.length,
canInsertColumnBlock: canInsertBlockType(
'core/column',
clientId
@@ -149,9 +146,22 @@ function ColumnInspectorControls( {
}
return (
-
+ {
+ updateColumns( count, minCount );
+ setAttributes( {
+ isStackedOnMobile: true,
+ } );
+ } }
+ >
{ canInsertColumnBlock && (
- <>
+ count }
+ onDeselect={ () => updateColumns( count, minCount ) }
+ >
) }
- >
+
) }
-
+ isShownByDefault
+ hasValue={ () => isStackedOnMobile !== true }
+ onDeselect={ () =>
setAttributes( {
- isStackedOnMobile: ! isStackedOnMobile,
+ isStackedOnMobile: true,
} )
}
- />
-
+ >
+
+ setAttributes( {
+ isStackedOnMobile: ! isStackedOnMobile,
+ } )
+ }
+ />
+
+
);
}
diff --git a/packages/block-library/src/comments-pagination/editor.scss b/packages/block-library/src/comments-pagination/editor.scss
index a875c9e0ee21c..3cd99c632ee83 100644
--- a/packages/block-library/src/comments-pagination/editor.scss
+++ b/packages/block-library/src/comments-pagination/editor.scss
@@ -26,6 +26,7 @@ $pagination-margin: 0.5em;
margin-right: $pagination-margin;
margin-bottom: $pagination-margin;
+ font-size: inherit;
&:last-child {
/*rtl:ignore*/
margin-right: 0;
diff --git a/packages/block-library/src/comments-pagination/style.scss b/packages/block-library/src/comments-pagination/style.scss
index c6b5d9a0a29e9..2fb6e3dd2d48f 100644
--- a/packages/block-library/src/comments-pagination/style.scss
+++ b/packages/block-library/src/comments-pagination/style.scss
@@ -8,6 +8,7 @@ $pagination-margin: 0.5em;
margin-right: $pagination-margin;
margin-bottom: $pagination-margin;
+ font-size: inherit;
&:last-child {
/*rtl:ignore*/
margin-right: 0;
diff --git a/packages/block-library/src/cover/edit.native.js b/packages/block-library/src/cover/edit.native.js
index 99324545bf798..7f73ec85a798e 100644
--- a/packages/block-library/src/cover/edit.native.js
+++ b/packages/block-library/src/cover/edit.native.js
@@ -58,7 +58,7 @@ import {
useCallback,
useMemo,
} from '@wordpress/element';
-import { cover as icon, replace, image, warning } from '@wordpress/icons';
+import { cover as icon, replace, image, cautionFilled } from '@wordpress/icons';
import { getProtocol } from '@wordpress/url';
// eslint-disable-next-line no-restricted-imports
import { store as editPostStore } from '@wordpress/edit-post';
@@ -665,7 +665,10 @@ const Cover = ( {
style={ styles.uploadFailedContainer }
>
-
+
) }
diff --git a/packages/block-library/src/cover/edit/index.js b/packages/block-library/src/cover/edit/index.js
index 1c86d953bc0ba..ced3097320329 100644
--- a/packages/block-library/src/cover/edit/index.js
+++ b/packages/block-library/src/cover/edit/index.js
@@ -120,7 +120,9 @@ function CoverEdit( {
select( coreStore ).getMedia( featuredImage, { context: 'view' } ),
[ featuredImage ]
);
- const mediaUrl = media?.source_url;
+ const mediaUrl =
+ media?.media_details?.sizes?.[ sizeSlug ]?.source_url ??
+ media?.source_url;
// User can change the featured image outside of the block, but we still
// need to update the block when that happens. This effect should only
@@ -201,7 +203,7 @@ function CoverEdit( {
averageBackgroundColor
);
- if ( backgroundType === IMAGE_BACKGROUND_TYPE && mediaAttributes.id ) {
+ if ( backgroundType === IMAGE_BACKGROUND_TYPE && mediaAttributes?.id ) {
const { imageDefaultSize } = getSettings();
// Try to use the previous selected image size if it's available
@@ -451,6 +453,7 @@ function CoverEdit( {
toggleUseFeaturedImage={ toggleUseFeaturedImage }
updateDimRatio={ onUpdateDimRatio }
onClearMedia={ onClearMedia }
+ featuredImage={ media }
/>
);
diff --git a/packages/block-library/src/cover/edit/inspector-controls.js b/packages/block-library/src/cover/edit/inspector-controls.js
index c0807869ee1a5..b0d4b435163b7 100644
--- a/packages/block-library/src/cover/edit/inspector-controls.js
+++ b/packages/block-library/src/cover/edit/inspector-controls.js
@@ -96,6 +96,7 @@ export default function CoverInspectorControls( {
coverRef,
currentSettings,
updateDimRatio,
+ featuredImage,
} ) {
const {
useFeaturedImage,
@@ -132,8 +133,12 @@ export default function CoverInspectorControls( {
[ id, isImageBackground ]
);
+ const currentBackgroundImage = useFeaturedImage ? featuredImage : image;
+
function updateImage( newSizeSlug ) {
- const newUrl = image?.media_details?.sizes?.[ newSizeSlug ]?.source_url;
+ const newUrl =
+ currentBackgroundImage?.media_details?.sizes?.[ newSizeSlug ]
+ ?.source_url;
if ( ! newUrl ) {
return null;
}
@@ -146,7 +151,9 @@ export default function CoverInspectorControls( {
const imageSizeOptions = imageSizes
?.filter(
- ( { slug } ) => image?.media_details?.sizes?.[ slug ]?.source_url
+ ( { slug } ) =>
+ currentBackgroundImage?.media_details?.sizes?.[ slug ]
+ ?.source_url
)
?.map( ( { name, slug } ) => ( { value: slug, label: name } ) );
@@ -321,7 +328,7 @@ export default function CoverInspectorControls( {
/>
) }
- { ! useFeaturedImage && !! imageSizeOptions?.length && (
+ { !! imageSizeOptions?.length && (
Snow Patrol' ),
align: 'center',
- style: {
- typography: {
- fontSize: 48,
- },
- color: {
- text: 'white',
- },
- },
},
},
],
diff --git a/packages/block-library/src/cover/index.php b/packages/block-library/src/cover/index.php
index 1ffe7ab3f4dbc..630835a47947b 100644
--- a/packages/block-library/src/cover/index.php
+++ b/packages/block-library/src/cover/index.php
@@ -35,12 +35,12 @@ function render_block_core_cover( $attributes, $content ) {
$attr['style'] = 'object-position:' . $object_position . ';';
}
- $image = get_the_post_thumbnail( null, 'post-thumbnail', $attr );
+ $image = get_the_post_thumbnail( null, $attributes['sizeSlug'] ?? 'post-thumbnail', $attr );
} else {
if ( in_the_loop() ) {
update_post_thumbnail_cache();
}
- $current_featured_image = get_the_post_thumbnail_url();
+ $current_featured_image = get_the_post_thumbnail_url( null, $attributes['sizeSlug'] ?? null );
if ( ! $current_featured_image ) {
return $content;
}
diff --git a/packages/block-library/src/cover/shared.js b/packages/block-library/src/cover/shared.js
index 37390354a37d6..7628300cbf8cf 100644
--- a/packages/block-library/src/cover/shared.js
+++ b/packages/block-library/src/cover/shared.js
@@ -35,7 +35,7 @@ export function dimRatioToClass( ratio ) {
}
export function attributesFromMedia( media ) {
- if ( ! media || ! media.url ) {
+ if ( ! media || ( ! media.url && ! media.src ) ) {
return {
url: undefined,
id: undefined,
@@ -52,23 +52,23 @@ export function attributesFromMedia( media ) {
if ( media.media_type === IMAGE_BACKGROUND_TYPE ) {
mediaType = IMAGE_BACKGROUND_TYPE;
} else {
- // only images and videos are accepted so if the media_type is not an image we can assume it is a video.
+ // Only images and videos are accepted so if the media_type is not an image we can assume it is a video.
// Videos contain the media type of 'file' in the object returned from the rest api.
mediaType = VIDEO_BACKGROUND_TYPE;
}
- } else {
// For media selections originated from existing files in the media library.
- if (
- media.type !== IMAGE_BACKGROUND_TYPE &&
- media.type !== VIDEO_BACKGROUND_TYPE
- ) {
- return;
- }
+ } else if (
+ media.type &&
+ ( media.type === IMAGE_BACKGROUND_TYPE ||
+ media.type === VIDEO_BACKGROUND_TYPE )
+ ) {
mediaType = media.type;
+ } else {
+ return;
}
return {
- url: media.url,
+ url: media.url || media.src,
id: media.id,
alt: media?.alt,
backgroundType: mediaType,
diff --git a/packages/block-library/src/cover/test/edit.js b/packages/block-library/src/cover/test/edit.js
index 5c1a5b5e13e67..f5d6a5301ef6d 100644
--- a/packages/block-library/src/cover/test/edit.js
+++ b/packages/block-library/src/cover/test/edit.js
@@ -337,7 +337,7 @@ describe( 'Cover block', () => {
describe( 'when colors are disabled', () => {
test( 'does not render overlay control', async () => {
await setup( undefined, true, disabledColorSettings );
- await createAndSelectBlock();
+ await selectBlock( 'Block: Cover' );
await userEvent.click(
screen.getByRole( 'tab', { name: 'Styles' } )
);
@@ -350,7 +350,7 @@ describe( 'Cover block', () => {
} );
test( 'does not render opacity control', async () => {
await setup( undefined, true, disabledColorSettings );
- await createAndSelectBlock();
+ await selectBlock( 'Block: Cover' );
await userEvent.click(
screen.getByRole( 'tab', { name: 'Styles' } )
);
diff --git a/packages/block-library/src/details/edit.js b/packages/block-library/src/details/edit.js
index 314556ba6d591..14c89b7d0f9f0 100644
--- a/packages/block-library/src/details/edit.js
+++ b/packages/block-library/src/details/edit.js
@@ -9,7 +9,11 @@ import {
InspectorControls,
} from '@wordpress/block-editor';
import { useSelect } from '@wordpress/data';
-import { PanelBody, ToggleControl } from '@wordpress/components';
+import {
+ ToggleControl,
+ __experimentalToolsPanel as ToolsPanel,
+ __experimentalToolsPanelItem as ToolsPanelItem,
+} from '@wordpress/components';
import { __ } from '@wordpress/i18n';
const TEMPLATE = [
@@ -46,18 +50,36 @@ function DetailsEdit( { attributes, setAttributes, clientId } ) {
return (
<>
-
- {
+ setAttributes( {
+ showContent: false,
+ } );
+ } }
+ >
+
+ hasValue={ () => showContent }
+ onDeselect={ () => {
setAttributes( {
- showContent: ! showContent,
- } )
- }
- />
-
+ showContent: false,
+ } );
+ } }
+ >
+
+ setAttributes( {
+ showContent: ! showContent,
+ } )
+ }
+ />
+
+
0;
+
+ // In the list view, use the block's summary as the label.
+ // If the summary is empty, fall back to the default label.
+ if ( context === 'list-view' && ( customName || hasSummary ) ) {
+ return customName || summary;
+ }
+
+ if ( context === 'accessibility' ) {
+ return ! hasSummary
+ ? __( 'Details. Empty.' )
+ : sprintf(
+ /* translators: accessibility text; summary title. */
+ __( 'Details. %s' ),
+ summary
+ );
+ }
+ },
save,
edit,
transforms,
diff --git a/packages/block-library/src/file/edit.js b/packages/block-library/src/file/edit.js
index 937eb3d28eb19..838b807507d31 100644
--- a/packages/block-library/src/file/edit.js
+++ b/packages/block-library/src/file/edit.js
@@ -128,15 +128,21 @@ function FileEdit( { attributes, isSelected, setAttributes, clientId } ) {
}
const isPdf = newMedia.url.endsWith( '.pdf' );
+ const pdfAttributes = {
+ displayPreview: isPdf
+ ? attributes.displayPreview ?? true
+ : undefined,
+ previewHeight: isPdf ? attributes.previewHeight ?? 600 : undefined,
+ };
+
setAttributes( {
href: newMedia.url,
fileName: newMedia.title,
textLinkHref: newMedia.url,
id: newMedia.id,
- displayPreview: isPdf ? true : undefined,
- previewHeight: isPdf ? 600 : undefined,
fileId: `wp-block-file--media-${ clientId }`,
blob: undefined,
+ ...pdfAttributes,
} );
setTemporaryURL();
}
diff --git a/packages/block-library/src/file/test/__snapshots__/edit.native.js.snap b/packages/block-library/src/file/test/__snapshots__/edit.native.js.snap
index 5ce876137ade0..0c9d88a207401 100644
--- a/packages/block-library/src/file/test/__snapshots__/edit.native.js.snap
+++ b/packages/block-library/src/file/test/__snapshots__/edit.native.js.snap
@@ -132,7 +132,7 @@ exports[`File block renders file error state without crashing 1`] = `
diff --git a/packages/block-library/src/form/block.json b/packages/block-library/src/form/block.json
index fa5212822cc71..20f3b89dc62b0 100644
--- a/packages/block-library/src/form/block.json
+++ b/packages/block-library/src/form/block.json
@@ -64,6 +64,5 @@
}
},
"__experimentalSelector": "form"
- },
- "viewScript": "file:./view.min.js"
+ }
}
diff --git a/packages/block-library/src/form/index.php b/packages/block-library/src/form/index.php
index c887d46ad8618..d2b4942d6a50b 100644
--- a/packages/block-library/src/form/index.php
+++ b/packages/block-library/src/form/index.php
@@ -14,6 +14,7 @@
* @return string The content of the block being rendered.
*/
function render_block_core_form( $attributes, $content ) {
+ wp_enqueue_script_module( '@wordpress/block-library/form/view' );
$processed_content = new WP_HTML_Tag_Processor( $content );
$processed_content->next_tag( 'form' );
@@ -42,26 +43,6 @@ function render_block_core_form( $attributes, $content ) {
);
}
-/**
- * Additional data to add to the view.js script for this block.
- */
-function block_core_form_view_script() {
- if ( ! gutenberg_is_experiment_enabled( 'gutenberg-form-blocks' ) ) {
- return;
- }
-
- wp_localize_script(
- 'wp-block-form-view',
- 'wpBlockFormSettings',
- array(
- 'nonce' => wp_create_nonce( 'wp-block-form' ),
- 'ajaxUrl' => admin_url( 'admin-ajax.php' ),
- 'action' => 'wp_block_form_email_submit',
- )
- );
-}
-add_action( 'wp_enqueue_scripts', 'block_core_form_view_script' );
-
/**
* Adds extra fields to the form.
*
diff --git a/packages/block-library/src/form/view.js b/packages/block-library/src/form/view.js
index d162d66020f44..43e5af99e2128 100644
--- a/packages/block-library/src/form/view.js
+++ b/packages/block-library/src/form/view.js
@@ -1,8 +1,21 @@
+let formSettings;
+try {
+ formSettings = JSON.parse(
+ document.getElementById(
+ 'wp-script-module-data-@wordpress/block-library/form/view'
+ )?.textContent
+ );
+} catch {}
+
// eslint-disable-next-line eslint-comments/disable-enable-pair
/* eslint-disable no-undef */
document.querySelectorAll( 'form.wp-block-form' ).forEach( function ( form ) {
- // Bail If the form is not using the mailto: action.
- if ( ! form.action || ! form.action.startsWith( 'mailto:' ) ) {
+ // Bail If the form settings not provided or the form is not using the mailto: action.
+ if (
+ ! formSettings ||
+ ! form.action ||
+ ! form.action.startsWith( 'mailto:' )
+ ) {
return;
}
@@ -18,13 +31,13 @@ document.querySelectorAll( 'form.wp-block-form' ).forEach( function ( form ) {
// Get the form data and merge it with the form action and nonce.
const formData = Object.fromEntries( new FormData( form ).entries() );
formData.formAction = form.action;
- formData._ajax_nonce = wpBlockFormSettings.nonce;
- formData.action = wpBlockFormSettings.action;
+ formData._ajax_nonce = formSettings.nonce;
+ formData.action = formSettings.action;
formData._wp_http_referer = window.location.href;
formData.formAction = form.action;
try {
- const response = await fetch( wpBlockFormSettings.ajaxUrl, {
+ const response = await fetch( formSettings.ajaxUrl, {
method: 'POST',
headers: {
'Content-Type': 'application/x-www-form-urlencoded',
diff --git a/packages/block-library/src/freeform/modal.js b/packages/block-library/src/freeform/modal.js
index 4ed4ef4d3a07c..35652eb29948a 100644
--- a/packages/block-library/src/freeform/modal.js
+++ b/packages/block-library/src/freeform/modal.js
@@ -25,7 +25,7 @@ function ModalAuxiliaryActions( { onClick, isModalFullScreen } ) {
return (
event.preventDefault();
@@ -24,8 +19,6 @@ export default function HomeEdit( { attributes, setAttributes, context } ) {
return select( coreStore ).getEntityRecord( 'root', '__unstableBase' )
?.home;
}, [] );
- const { __unstableMarkNextChangeAsNotPersistent } =
- useDispatch( blockEditorStore );
const { textColor, backgroundColor, style } = context;
const blockProps = useBlockProps( {
@@ -41,42 +34,31 @@ export default function HomeEdit( { attributes, setAttributes, context } ) {
},
} );
- const { label } = attributes;
-
- useEffect( () => {
- if ( label === undefined ) {
- __unstableMarkNextChangeAsNotPersistent();
- setAttributes( { label: __( 'Home' ) } );
- }
- }, [ label ] );
-
return (
- <>
-
- >
+
);
}
diff --git a/packages/block-library/src/home-link/index.php b/packages/block-library/src/home-link/index.php
index fb7235834459d..d61aa0bc235e2 100644
--- a/packages/block-library/src/home-link/index.php
+++ b/packages/block-library/src/home-link/index.php
@@ -137,9 +137,6 @@ function block_core_home_link_build_li_wrapper_attributes( $context ) {
*/
function render_block_core_home_link( $attributes, $content, $block ) {
if ( empty( $attributes['label'] ) ) {
- // Using a fallback for the label attribute allows rendering the block even if no attributes have been set,
- // e.g. when using the block as a hooked block.
- // Note that the fallback value needs to be kept in sync with the one set in `edit.js` (upon first loading the block in the editor).
$attributes['label'] = __( 'Home' );
}
$aria_current = '';
diff --git a/packages/block-library/src/image/image.js b/packages/block-library/src/image/image.js
index b4d269f967822..c3783b50e0006 100644
--- a/packages/block-library/src/image/image.js
+++ b/packages/block-library/src/image/image.js
@@ -1126,7 +1126,7 @@ export default function Image( {
id &&
clientId === selectedClientIds[ 0 ] && (
- { __( 'Set featured image' ) }
+ { __( 'Set as featured image' ) }
)
}
diff --git a/packages/block-library/src/image/index.php b/packages/block-library/src/image/index.php
index 1a5fae7ce9cbb..ac03011d73f63 100644
--- a/packages/block-library/src/image/index.php
+++ b/packages/block-library/src/image/index.php
@@ -290,6 +290,7 @@ class="wp-lightbox-overlay zoom"
data-wp-on-async--click="actions.hideLightbox"
data-wp-on-async-window--resize="callbacks.setOverlayStyles"
data-wp-on-async-window--scroll="actions.handleScroll"
+ data-wp-bind--style="state.overlayStyles"
tabindex="-1"
>
@@ -306,7 +307,6 @@ class="wp-lightbox-overlay zoom"
-
HTML;
}
diff --git a/packages/block-library/src/image/style.scss b/packages/block-library/src/image/style.scss
index 1bb19bf29da69..8ca5795cfd911 100644
--- a/packages/block-library/src/image/style.scss
+++ b/packages/block-library/src/image/style.scss
@@ -1,6 +1,7 @@
.wp-block-image {
- a {
+ > a,
+ > figure > a {
display: inline-block;
}
@@ -42,8 +43,8 @@
text-align: center;
}
- &.alignfull a,
- &.alignwide a {
+ &.alignfull > a,
+ &.alignwide > a {
width: 100%;
}
diff --git a/packages/block-library/src/image/transforms.js b/packages/block-library/src/image/transforms.js
index 347d240828017..0119009b2182c 100644
--- a/packages/block-library/src/image/transforms.js
+++ b/packages/block-library/src/image/transforms.js
@@ -59,6 +59,7 @@ const schema = ( { phrasingContentSchema } ) => ( {
...imageSchema,
a: {
attributes: [ 'href', 'rel', 'target' ],
+ classes: [ '*' ],
children: imageSchema,
},
figcaption: {
diff --git a/packages/block-library/src/image/view.js b/packages/block-library/src/image/view.js
index 0bc0dfaacea1a..3c9a729538813 100644
--- a/packages/block-library/src/image/view.js
+++ b/packages/block-library/src/image/view.js
@@ -341,7 +341,6 @@ const { state, actions, callbacks } = store(
// adding 1 pixel to the container width and height solves the problem,
// though this can be removed if the issue is fixed in the future.
state.overlayStyles = `
- :root {
--wp--lightbox-initial-top-position: ${ screenPosY }px;
--wp--lightbox-initial-left-position: ${ screenPosX }px;
--wp--lightbox-container-width: ${ containerWidth + 1 }px;
@@ -352,8 +351,7 @@ const { state, actions, callbacks } = store(
--wp--lightbox-scrollbar-width: ${
window.innerWidth - document.documentElement.clientWidth
}px;
- }
- `;
+ `;
},
setButtonStyles() {
const { imageId } = getContext();
diff --git a/packages/block-library/src/index.js b/packages/block-library/src/index.js
index 56365c87a268f..262f11de6ee22 100644
--- a/packages/block-library/src/index.js
+++ b/packages/block-library/src/index.js
@@ -99,6 +99,7 @@ import * as queryPaginationNext from './query-pagination-next';
import * as queryPaginationNumbers from './query-pagination-numbers';
import * as queryPaginationPrevious from './query-pagination-previous';
import * as queryTitle from './query-title';
+import * as queryTotal from './query-total';
import * as quote from './quote';
import * as reusableBlock from './block';
import * as readMore from './read-more';
@@ -211,6 +212,7 @@ const getAllBlocks = () => {
queryPaginationNumbers,
queryPaginationPrevious,
queryNoResults,
+ queryTotal,
readMore,
comments,
commentAuthorName,
diff --git a/packages/block-library/src/latest-posts/style.scss b/packages/block-library/src/latest-posts/style.scss
index 2a01d177ef47c..69a4746b5e751 100644
--- a/packages/block-library/src/latest-posts/style.scss
+++ b/packages/block-library/src/latest-posts/style.scss
@@ -57,7 +57,8 @@
font-size: 0.8125em;
}
-.wp-block-latest-posts__post-excerpt {
+.wp-block-latest-posts__post-excerpt,
+.wp-block-latest-posts__post-full-content {
margin-top: 0.5em;
margin-bottom: 1em;
}
diff --git a/packages/block-library/src/list-item/hooks/use-merge.js b/packages/block-library/src/list-item/hooks/use-merge.js
index 9ca4d5372ee6e..3fe755868df37 100644
--- a/packages/block-library/src/list-item/hooks/use-merge.js
+++ b/packages/block-library/src/list-item/hooks/use-merge.js
@@ -49,7 +49,7 @@ export default function useMerge( clientId, onMerge ) {
* return the next list item of the parent list item if it exists.
*
* @param {string} id A list item client ID.
- * @return {string?} The client ID of the next list item.
+ * @return {?string} The client ID of the next list item.
*/
function _getNextId( id ) {
const next = getNextBlockClientId( id );
@@ -68,7 +68,7 @@ export default function useMerge( clientId, onMerge ) {
* line, regardless of indentation level.
*
* @param {string} id The client ID of the current list item.
- * @return {string?} The client ID of the next list item.
+ * @return {?string} The client ID of the next list item.
*/
function getNextId( id ) {
const order = getBlockOrder( id );
diff --git a/packages/block-library/src/loginout/edit.js b/packages/block-library/src/loginout/edit.js
index b6c2e9cf01304..9af634c87371c 100644
--- a/packages/block-library/src/loginout/edit.js
+++ b/packages/block-library/src/loginout/edit.js
@@ -1,38 +1,74 @@
/**
* WordPress dependencies
*/
-import { PanelBody, ToggleControl } from '@wordpress/components';
-import { __ } from '@wordpress/i18n';
import { InspectorControls, useBlockProps } from '@wordpress/block-editor';
+import {
+ ToggleControl,
+ __experimentalToolsPanel as ToolsPanel,
+ __experimentalToolsPanelItem as ToolsPanelItem,
+} from '@wordpress/components';
+import { __ } from '@wordpress/i18n';
+/**
+ * Internal dependencies
+ */
+import { useToolsPanelDropdownMenuProps } from '../utils/hooks';
export default function LoginOutEdit( { attributes, setAttributes } ) {
const { displayLoginAsForm, redirectToCurrent } = attributes;
+ const dropdownMenuProps = useToolsPanelDropdownMenuProps();
return (
<>
-
- {
+ setAttributes( {
+ displayLoginAsForm: false,
+ redirectToCurrent: true,
+ } );
+ } }
+ dropdownMenuProps={ dropdownMenuProps }
+ >
+
- setAttributes( {
- displayLoginAsForm: ! displayLoginAsForm,
- } )
+ isShownByDefault
+ hasValue={ () => displayLoginAsForm }
+ onDeselect={ () =>
+ setAttributes( { displayLoginAsForm: false } )
}
- />
-
+
+ setAttributes( {
+ displayLoginAsForm: ! displayLoginAsForm,
+ } )
+ }
+ />
+
+
- setAttributes( {
- redirectToCurrent: ! redirectToCurrent,
- } )
+ isShownByDefault
+ hasValue={ () => ! redirectToCurrent }
+ onDeselect={ () =>
+ setAttributes( { redirectToCurrent: true } )
}
- />
-
+ >
+
+ setAttributes( {
+ redirectToCurrent: ! redirectToCurrent,
+ } )
+ }
+ />
+
+
-
-
-
+ {
+ setAttributes( {
+ noTeaser: false,
+ } );
+ } }
+ >
+ noTeaser }
+ onDeselect={ () =>
+ setAttributes( { noTeaser: false } )
+ }
+ >
+
+
+
{ description && (
diff --git a/packages/block-library/src/navigation-link/index.php b/packages/block-library/src/navigation-link/index.php
index 5653e04fca88a..81df2099dfc18 100644
--- a/packages/block-library/src/navigation-link/index.php
+++ b/packages/block-library/src/navigation-link/index.php
@@ -177,7 +177,22 @@ function render_block_core_navigation_link( $attributes, $content, $block ) {
// Don't render the block's subtree if it is a draft or if the ID does not exist.
if ( $is_post_type && $navigation_link_has_id ) {
$post = get_post( $attributes['id'] );
- if ( ! $post || 'publish' !== $post->post_status ) {
+ /**
+ * Filter allowed post_status for navigation link block to render.
+ *
+ * @since 6.8.0
+ *
+ * @param array $post_status
+ * @param array $attributes
+ * @param WP_Block $block
+ */
+ $allowed_post_status = (array) apply_filters(
+ 'render_block_core_navigation_link_allowed_post_status',
+ array( 'publish' ),
+ $attributes,
+ $block
+ );
+ if ( ! $post || ! in_array( $post->post_status, $allowed_post_status, true ) ) {
return '';
}
}
diff --git a/packages/block-library/src/navigation-link/link-ui.js b/packages/block-library/src/navigation-link/link-ui.js
index ee238c71ed28e..52db034c6f980 100644
--- a/packages/block-library/src/navigation-link/link-ui.js
+++ b/packages/block-library/src/navigation-link/link-ui.js
@@ -10,7 +10,7 @@ import {
} from '@wordpress/components';
import { __, sprintf, isRTL } from '@wordpress/i18n';
import {
- __experimentalLinkControl as LinkControl,
+ LinkControl,
store as blockEditorStore,
privateApis as blockEditorPrivateApis,
} from '@wordpress/block-editor';
diff --git a/packages/block-library/src/navigation-submenu/edit.js b/packages/block-library/src/navigation-submenu/edit.js
index acc9510d0d3d3..dbdbd23b13b2f 100644
--- a/packages/block-library/src/navigation-submenu/edit.js
+++ b/packages/block-library/src/navigation-submenu/edit.js
@@ -8,11 +8,12 @@ import clsx from 'clsx';
*/
import { useSelect, useDispatch } from '@wordpress/data';
import {
- PanelBody,
TextControl,
TextareaControl,
ToolbarButton,
ToolbarGroup,
+ __experimentalToolsPanel as ToolsPanel,
+ __experimentalToolsPanelItem as ToolsPanelItem,
} from '@wordpress/components';
import { displayShortcut, isKeyboardEvent } from '@wordpress/keycodes';
import { __ } from '@wordpress/i18n';
@@ -382,67 +383,119 @@ export default function NavigationSubmenuEdit( {
{ /* Warning, this duplicated in packages/block-library/src/navigation-link/edit.js */ }
-
- {
- setAttributes( { label: labelValue } );
- } }
+ {
+ setAttributes( {
+ label: '',
+ url: '',
+ description: '',
+ title: '',
+ rel: '',
+ } );
+ } }
+ >
+
- {
- setAttributes( { url: urlValue } );
- } }
+ isShownByDefault
+ hasValue={ () => !! label }
+ onDeselect={ () => setAttributes( { label: '' } ) }
+ >
+ {
+ setAttributes( { label: labelValue } );
+ } }
+ label={ __( 'Text' ) }
+ autoComplete="off"
+ />
+
+
+
- {
- setAttributes( {
- description: descriptionValue,
- } );
- } }
+ isShownByDefault
+ hasValue={ () => !! url }
+ onDeselect={ () => setAttributes( { url: '' } ) }
+ >
+ {
+ setAttributes( { url: urlValue } );
+ } }
+ label={ __( 'Link' ) }
+ autoComplete="off"
+ />
+
+
+
- {
- setAttributes( { title: titleValue } );
- } }
+ isShownByDefault
+ hasValue={ () => !! description }
+ onDeselect={ () =>
+ setAttributes( { description: '' } )
+ }
+ >
+ {
+ setAttributes( {
+ description: descriptionValue,
+ } );
+ } }
+ label={ __( 'Description' ) }
+ help={ __(
+ 'The description will be displayed in the menu if the current theme supports it.'
+ ) }
+ />
+
+
+
- {
- setAttributes( { rel: relValue } );
- } }
+ isShownByDefault
+ hasValue={ () => !! title }
+ onDeselect={ () => setAttributes( { title: '' } ) }
+ >
+ {
+ setAttributes( { title: titleValue } );
+ } }
+ label={ __( 'Title attribute' ) }
+ autoComplete="off"
+ help={ __(
+ 'Additional information to help clarify the purpose of the link.'
+ ) }
+ />
+
+
+
-
+ isShownByDefault
+ hasValue={ () => !! rel }
+ onDeselect={ () => setAttributes( { rel: '' } ) }
+ >
+ {
+ setAttributes( { rel: relValue } );
+ } }
+ label={ __( 'Rel attribute' ) }
+ autoComplete="off"
+ help={ __(
+ 'The relationship of the linked URL as space-separated link types.'
+ ) }
+ />
+
+
{ /* eslint-disable jsx-a11y/anchor-is-valid */ }
@@ -461,12 +514,6 @@ export default function NavigationSubmenuEdit( {
aria-label={ __( 'Navigation link text' ) }
placeholder={ itemLabelPlaceholder }
withoutInteractiveFormatting
- allowedFormats={ [
- 'core/bold',
- 'core/italic',
- 'core/image',
- 'core/strikethrough',
- ] }
onClick={ () => {
if ( ! openSubmenusOnClick && ! url ) {
setIsLinkOpen( true );
@@ -474,6 +521,11 @@ export default function NavigationSubmenuEdit( {
}
} }
/>
+ { description && (
+
+ { description }
+
+ ) }
{ ! openSubmenusOnClick && isLinkOpen && (
';
@@ -222,7 +238,7 @@ function render_block_core_navigation_submenu( $attributes, $content, $block ) {
if ( strpos( $inner_blocks_html, 'current-menu-item' ) ) {
$tag_processor = new WP_HTML_Tag_Processor( $html );
- while ( $tag_processor->next_tag( array( 'class_name' => 'wp-block-navigation-item__content' ) ) ) {
+ while ( $tag_processor->next_tag( array( 'class_name' => 'wp-block-navigation-item' ) ) ) {
$tag_processor->add_class( 'current-menu-ancestor' );
}
$html = $tag_processor->get_updated_html();
diff --git a/packages/block-library/src/navigation/README.md b/packages/block-library/src/navigation/README.md
index b59c4b40d85e7..cbd52a8f21f0e 100644
--- a/packages/block-library/src/navigation/README.md
+++ b/packages/block-library/src/navigation/README.md
@@ -10,4 +10,5 @@ The structural CSS for the navigation block targets generic classnames across me
- `.wp-block-navigation-item` is applied to every menu item.
- `.wp-block-navigation-item__content` is applied to the link inside a menu item.
- `.wp-block-navigation-item__label` is applied to the innermost container around the menu item text label.
+- `.wp-block-navigation-item__description` is applied to the innermost container around the menu item description.
- `.wp-block-navigation__submenu-icon` is applied to the submenu indicator (chevron).
diff --git a/packages/block-library/src/navigation/edit/deleted-navigation-warning.js b/packages/block-library/src/navigation/edit/deleted-navigation-warning.js
index 22d1e339c5c00..3d40f4d031ed3 100644
--- a/packages/block-library/src/navigation/edit/deleted-navigation-warning.js
+++ b/packages/block-library/src/navigation/edit/deleted-navigation-warning.js
@@ -4,9 +4,16 @@
import { Warning } from '@wordpress/block-editor';
import { Button, Notice } from '@wordpress/components';
import { __ } from '@wordpress/i18n';
-import { createInterpolateElement } from '@wordpress/element';
+import { useState, createInterpolateElement } from '@wordpress/element';
function DeletedNavigationWarning( { onCreateNew, isNotice = false } ) {
+ const [ isButtonDisabled, setIsButtonDisabled ] = useState( false );
+
+ const handleButtonClick = () => {
+ setIsButtonDisabled( true );
+ onCreateNew();
+ };
+
const message = createInterpolateElement(
__(
'Navigation Menu has been deleted or is unavailable. Create a new Menu? '
@@ -15,8 +22,10 @@ function DeletedNavigationWarning( { onCreateNew, isNotice = false } ) {
button: (
),
}
diff --git a/packages/block-library/src/navigation/index.php b/packages/block-library/src/navigation/index.php
index dd300eb12c6fe..9a56e399fcfec 100644
--- a/packages/block-library/src/navigation/index.php
+++ b/packages/block-library/src/navigation/index.php
@@ -567,13 +567,14 @@ private static function get_nav_wrapper_attributes( $attributes, $inner_blocks )
$is_responsive_menu = static::is_responsive( $attributes );
$style = static::get_styles( $attributes );
$class = static::get_classes( $attributes );
- $wrapper_attributes = get_block_wrapper_attributes(
- array(
- 'class' => $class,
- 'style' => $style,
- 'aria-label' => $nav_menu_name,
- )
+ $extra_attributes = array(
+ 'class' => $class,
+ 'style' => $style,
);
+ if ( ! empty( $nav_menu_name ) ) {
+ $extra_attributes['aria-label'] = $nav_menu_name;
+ }
+ $wrapper_attributes = get_block_wrapper_attributes( $extra_attributes );
if ( $is_responsive_menu ) {
$nav_element_directives = static::get_nav_element_directives( $is_interactive );
@@ -1436,20 +1437,6 @@ function block_core_navigation_get_most_recently_published_navigation() {
return null;
}
-/**
- * Accepts the serialized markup of a block and its inner blocks, and returns serialized markup of the inner blocks.
- *
- * @since 6.5.0
- *
- * @param string $serialized_block The serialized markup of a block and its inner blocks.
- * @return string
- */
-function block_core_navigation_remove_serialized_parent_block( $serialized_block ) {
- $start = strpos( $serialized_block, '-->' ) + strlen( '-->' );
- $end = strrpos( $serialized_block, '
## Context
-See examples for this section for the [ItemGroup](/packages/components/src/item-group/item-group/README.md#context) and [`Card`](/packages/components/src/card/card/README.md#context) components.
+See examples for this section for the [ItemGroup](https://github.com/WordPress/gutenberg/blob/trunk/packages/components/src/item-group/item-group/README.md#context) and [`Card`](https://github.com/WordPress/gutenberg/tree/trunk/packages/components/src/card/card#context) components.
````
## Folder structure
diff --git a/packages/components/package.json b/packages/components/package.json
index 6871511cf5b1e..79df8e92d84b6 100644
--- a/packages/components/package.json
+++ b/packages/components/package.json
@@ -1,6 +1,6 @@
{
"name": "@wordpress/components",
- "version": "28.11.0",
+ "version": "29.0.0",
"description": "UI components for WordPress.",
"author": "The WordPress Contributors",
"license": "GPL-2.0-or-later",
@@ -32,7 +32,7 @@
"src/**/*.scss"
],
"dependencies": {
- "@ariakit/react": "^0.4.10",
+ "@ariakit/react": "^0.4.15",
"@babel/runtime": "7.25.7",
"@emotion/cache": "^11.7.1",
"@emotion/css": "^11.7.1",
diff --git a/packages/components/src/alignment-matrix-control/stories/index.story.tsx b/packages/components/src/alignment-matrix-control/stories/index.story.tsx
index 433d7540197da..e04d8b6690fe8 100644
--- a/packages/components/src/alignment-matrix-control/stories/index.story.tsx
+++ b/packages/components/src/alignment-matrix-control/stories/index.story.tsx
@@ -24,8 +24,8 @@ const meta: Meta< typeof AlignmentMatrixControl > = {
'AlignmentMatrixControl.Icon': AlignmentMatrixControl.Icon,
},
argTypes: {
- onChange: { control: { type: null } },
- value: { control: { type: null } },
+ onChange: { control: false },
+ value: { control: false },
},
parameters: {
actions: { argTypesRegex: '^on.*' },
diff --git a/packages/components/src/angle-picker-control/index.tsx b/packages/components/src/angle-picker-control/index.tsx
index b824660fddb13..9c436c36caf66 100644
--- a/packages/components/src/angle-picker-control/index.tsx
+++ b/packages/components/src/angle-picker-control/index.tsx
@@ -57,12 +57,12 @@ function UnforwardedAnglePickerControl(
= {
title: 'Components/AnglePickerControl',
component: AnglePickerControl,
argTypes: {
- as: { control: { type: null } },
- value: { control: { type: null } },
+ as: { control: false },
+ value: { control: false },
},
parameters: {
actions: { argTypesRegex: '^on.*' },
diff --git a/packages/components/src/animation/index.tsx b/packages/components/src/animation/index.tsx
index 6620f8d5d4eca..1b6796cd61ee9 100644
--- a/packages/components/src/animation/index.tsx
+++ b/packages/components/src/animation/index.tsx
@@ -9,5 +9,4 @@
export {
motion as __unstableMotion,
AnimatePresence as __unstableAnimatePresence,
- MotionContext as __unstableMotionContext,
} from 'framer-motion';
diff --git a/packages/components/src/autocomplete/autocompleter-ui.tsx b/packages/components/src/autocomplete/autocompleter-ui.tsx
index 69105f6c9d3b4..dbbbe724113d7 100644
--- a/packages/components/src/autocomplete/autocompleter-ui.tsx
+++ b/packages/components/src/autocomplete/autocompleter-ui.tsx
@@ -57,6 +57,7 @@ function ListBox( {
key={ option.key }
id={ `components-autocomplete-item-${ instanceId }-${ option.key }` }
role="option"
+ __next40pxDefaultSize
aria-selected={ index === selectedIndex }
accessibleWhenDisabled
disabled={ option.isDisabled }
diff --git a/packages/components/src/badge/README.md b/packages/components/src/badge/README.md
new file mode 100644
index 0000000000000..0be531ca6f2df
--- /dev/null
+++ b/packages/components/src/badge/README.md
@@ -0,0 +1,22 @@
+# Badge
+
+
+
+See the WordPress Storybook for more detailed, interactive documentation.
+
+## Props
+
+### `children`
+
+Text to display inside the badge.
+
+ - Type: `string`
+ - Required: Yes
+
+### `intent`
+
+Badge variant.
+
+ - Type: `"default" | "info" | "success" | "warning" | "error"`
+ - Required: No
+ - Default: `default`
diff --git a/packages/components/src/badge/docs-manifest.json b/packages/components/src/badge/docs-manifest.json
new file mode 100644
index 0000000000000..3b70c0ef22843
--- /dev/null
+++ b/packages/components/src/badge/docs-manifest.json
@@ -0,0 +1,5 @@
+{
+ "$schema": "../../schemas/docs-manifest.json",
+ "displayName": "Badge",
+ "filePath": "./index.tsx"
+}
diff --git a/packages/components/src/badge/index.tsx b/packages/components/src/badge/index.tsx
new file mode 100644
index 0000000000000..8a55f3881215f
--- /dev/null
+++ b/packages/components/src/badge/index.tsx
@@ -0,0 +1,66 @@
+/**
+ * External dependencies
+ */
+import clsx from 'clsx';
+
+/**
+ * WordPress dependencies
+ */
+import { info, caution, error, published } from '@wordpress/icons';
+
+/**
+ * Internal dependencies
+ */
+import type { BadgeProps } from './types';
+import type { WordPressComponentProps } from '../context';
+import Icon from '../icon';
+
+function Badge( {
+ className,
+ intent = 'default',
+ children,
+ ...props
+}: WordPressComponentProps< BadgeProps, 'span', false > ) {
+ /**
+ * Returns an icon based on the badge context.
+ *
+ * @return The corresponding icon for the provided context.
+ */
+ function contextBasedIcon() {
+ switch ( intent ) {
+ case 'info':
+ return info;
+ case 'success':
+ return published;
+ case 'warning':
+ return caution;
+ case 'error':
+ return error;
+ default:
+ return null;
+ }
+ }
+
+ return (
+
+ { intent !== 'default' && (
+
+ ) }
+ { children }
+
+ );
+}
+
+export default Badge;
diff --git a/packages/components/src/badge/stories/index.story.tsx b/packages/components/src/badge/stories/index.story.tsx
new file mode 100644
index 0000000000000..aaa4bfb3c08f6
--- /dev/null
+++ b/packages/components/src/badge/stories/index.story.tsx
@@ -0,0 +1,53 @@
+/**
+ * External dependencies
+ */
+import type { Meta, StoryObj } from '@storybook/react';
+
+/**
+ * Internal dependencies
+ */
+import Badge from '..';
+
+const meta = {
+ component: Badge,
+ title: 'Components/Containers/Badge',
+ tags: [ 'status-private' ],
+} satisfies Meta< typeof Badge >;
+
+export default meta;
+
+type Story = StoryObj< typeof meta >;
+
+export const Default: Story = {
+ args: {
+ children: 'Code is Poetry',
+ },
+};
+
+export const Info: Story = {
+ args: {
+ ...Default.args,
+ intent: 'info',
+ },
+};
+
+export const Success: Story = {
+ args: {
+ ...Default.args,
+ intent: 'success',
+ },
+};
+
+export const Warning: Story = {
+ args: {
+ ...Default.args,
+ intent: 'warning',
+ },
+};
+
+export const Error: Story = {
+ args: {
+ ...Default.args,
+ intent: 'error',
+ },
+};
diff --git a/packages/components/src/badge/styles.scss b/packages/components/src/badge/styles.scss
new file mode 100644
index 0000000000000..e1e9cd5312d11
--- /dev/null
+++ b/packages/components/src/badge/styles.scss
@@ -0,0 +1,38 @@
+$badge-colors: (
+ "info": #3858e9,
+ "warning": $alert-yellow,
+ "error": $alert-red,
+ "success": $alert-green,
+);
+
+.components-badge {
+ background-color: color-mix(in srgb, $white 90%, var(--base-color));
+ color: color-mix(in srgb, $black 50%, var(--base-color));
+ padding: 0 $grid-unit-10;
+ min-height: $grid-unit-30;
+ border-radius: $radius-small;
+ font-size: $font-size-small;
+ font-weight: 400;
+ flex-shrink: 0;
+ line-height: $font-line-height-small;
+ width: fit-content;
+ display: flex;
+ align-items: center;
+ gap: 2px;
+
+ &:where(.is-default) {
+ background-color: $gray-100;
+ color: $gray-800;
+ }
+
+ &.has-icon {
+ padding-inline-start: $grid-unit-05;
+ }
+
+ // Generate color variants
+ @each $type, $color in $badge-colors {
+ &.is-#{$type} {
+ --base-color: #{$color};
+ }
+ }
+}
diff --git a/packages/components/src/badge/test/index.tsx b/packages/components/src/badge/test/index.tsx
new file mode 100644
index 0000000000000..47c832eb3c830
--- /dev/null
+++ b/packages/components/src/badge/test/index.tsx
@@ -0,0 +1,40 @@
+/**
+ * External dependencies
+ */
+import { render, screen } from '@testing-library/react';
+
+/**
+ * Internal dependencies
+ */
+import Badge from '..';
+
+describe( 'Badge', () => {
+ it( 'should render correctly with default props', () => {
+ render( Code is Poetry );
+ const badge = screen.getByText( 'Code is Poetry' );
+ expect( badge ).toBeInTheDocument();
+ expect( badge.tagName ).toBe( 'SPAN' );
+ expect( badge ).toHaveClass( 'components-badge' );
+ } );
+
+ it( 'should render as per its intent and contain an icon', () => {
+ render( Code is Poetry );
+ const badge = screen.getByText( 'Code is Poetry' );
+ expect( badge ).toHaveClass( 'components-badge', 'is-error' );
+ expect( badge ).toHaveClass( 'has-icon' );
+ } );
+
+ it( 'should combine custom className with default class', () => {
+ render( Code is Poetry );
+ const badge = screen.getByText( 'Code is Poetry' );
+ expect( badge ).toHaveClass( 'components-badge' );
+ expect( badge ).toHaveClass( 'custom-class' );
+ } );
+
+ it( 'should pass through additional props', () => {
+ render( Code is Poetry );
+ const badge = screen.getByTestId( 'custom-badge' );
+ expect( badge ).toHaveTextContent( 'Code is Poetry' );
+ expect( badge ).toHaveClass( 'components-badge' );
+ } );
+} );
diff --git a/packages/components/src/badge/types.ts b/packages/components/src/badge/types.ts
new file mode 100644
index 0000000000000..91cd7c39b549b
--- /dev/null
+++ b/packages/components/src/badge/types.ts
@@ -0,0 +1,12 @@
+export type BadgeProps = {
+ /**
+ * Badge variant.
+ *
+ * @default 'default'
+ */
+ intent?: 'default' | 'info' | 'success' | 'warning' | 'error';
+ /**
+ * Text to display inside the badge.
+ */
+ children: string;
+};
diff --git a/packages/components/src/base-control/stories/index.story.tsx b/packages/components/src/base-control/stories/index.story.tsx
index 90517c75b5e95..69188f869656d 100644
--- a/packages/components/src/base-control/stories/index.story.tsx
+++ b/packages/components/src/base-control/stories/index.story.tsx
@@ -18,7 +18,7 @@ const meta: Meta< typeof BaseControl > = {
'BaseControl.VisualLabel': BaseControl.VisualLabel,
},
argTypes: {
- children: { control: { type: null } },
+ children: { control: false },
help: { control: { type: 'text' } },
label: { control: { type: 'text' } },
},
diff --git a/packages/components/src/border-box-control/border-box-control-split-controls/component.tsx b/packages/components/src/border-box-control/border-box-control-split-controls/component.tsx
index 8f125cdb8f926..94e1728076b18 100644
--- a/packages/components/src/border-box-control/border-box-control-split-controls/component.tsx
+++ b/packages/components/src/border-box-control/border-box-control-split-controls/component.tsx
@@ -67,12 +67,13 @@ const BorderBoxControlSplitControls = (
isCompact: true,
__experimentalIsRenderedInSidebar,
size,
+ __shouldNotWarnDeprecated36pxSize: true,
};
const mergedRef = useMergeRefs( [ setPopoverAnchor, forwardedRef ] );
return (
-
+
) : (
diff --git a/packages/components/src/border-box-control/stories/index.story.tsx b/packages/components/src/border-box-control/stories/index.story.tsx
index 0a961d34fb93d..3f118ae7cb37c 100644
--- a/packages/components/src/border-box-control/stories/index.story.tsx
+++ b/packages/components/src/border-box-control/stories/index.story.tsx
@@ -20,7 +20,7 @@ const meta: Meta< typeof BorderBoxControl > = {
component: BorderBoxControl,
argTypes: {
onChange: { action: 'onChange' },
- value: { control: { type: null } },
+ value: { control: false },
},
parameters: {
controls: { expanded: true },
diff --git a/packages/components/src/border-control/border-control/component.tsx b/packages/components/src/border-control/border-control/component.tsx
index 31eeb166a2d60..f71599b274778 100644
--- a/packages/components/src/border-control/border-control/component.tsx
+++ b/packages/components/src/border-control/border-control/component.tsx
@@ -75,6 +75,8 @@ const UnconnectedBorderControl = (
/>
) }
diff --git a/packages/components/src/border-control/border-control/hook.ts b/packages/components/src/border-control/border-control/hook.ts
index 67af7ce42416c..9b0f064c51921 100644
--- a/packages/components/src/border-control/border-control/hook.ts
+++ b/packages/components/src/border-control/border-control/hook.ts
@@ -38,6 +38,7 @@ export function useBorderControl(
width,
__experimentalIsRenderedInSidebar = false,
__next40pxDefaultSize,
+ __shouldNotWarnDeprecated36pxSize,
...otherProps
} = useContextSystem( props, 'BorderControl' );
@@ -45,6 +46,7 @@ export function useBorderControl(
componentName: 'BorderControl',
__next40pxDefaultSize,
size,
+ __shouldNotWarnDeprecated36pxSize,
} );
const computedSize =
diff --git a/packages/components/src/border-control/stories/index.story.tsx b/packages/components/src/border-control/stories/index.story.tsx
index 3b5fa740d092d..ca8505c01a0ba 100644
--- a/packages/components/src/border-control/stories/index.story.tsx
+++ b/packages/components/src/border-control/stories/index.story.tsx
@@ -23,7 +23,7 @@ const meta: Meta< typeof BorderControl > = {
action: 'onChange',
},
width: { control: { type: 'text' } },
- value: { control: { type: null } },
+ value: { control: false },
},
parameters: {
controls: { expanded: true },
diff --git a/packages/components/src/border-control/types.ts b/packages/components/src/border-control/types.ts
index 8ab614907684d..ecd3f67c9be08 100644
--- a/packages/components/src/border-control/types.ts
+++ b/packages/components/src/border-control/types.ts
@@ -116,6 +116,13 @@ export type BorderControlProps = ColorProps &
* @default false
*/
__next40pxDefaultSize?: boolean;
+ /**
+ * Do not throw a warning for the deprecated 36px default size.
+ * For internal components of other components that already throw the warning.
+ *
+ * @ignore
+ */
+ __shouldNotWarnDeprecated36pxSize?: boolean;
};
export type DropdownProps = ColorProps &
diff --git a/packages/components/src/box-control/README.md b/packages/components/src/box-control/README.md
index 77176b49eeb6d..da08cafceee42 100644
--- a/packages/components/src/box-control/README.md
+++ b/packages/components/src/box-control/README.md
@@ -1,106 +1,142 @@
# BoxControl
-A control that lets users set values for top, right, bottom, and left. Can be used as an input control for values like `padding` or `margin`.
+
-## Usage
+See the WordPress Storybook for more detailed, interactive documentation.
+
+A control that lets users set values for top, right, bottom, and left. Can be
+used as an input control for values like `padding` or `margin`.
```jsx
import { useState } from 'react';
import { BoxControl } from '@wordpress/components';
function Example() {
- const [ values, setValues ] = useState( {
- top: '50px',
- left: '10%',
- right: '10%',
- bottom: '50px',
- } );
-
- return (
- setValues( nextValues ) }
- />
- );
-}
+ const [ values, setValues ] = useState( {
+ top: '50px',
+ left: '10%',
+ right: '10%',
+ bottom: '50px',
+ } );
+
+ return (
+
+ );
+};
```
-
## Props
-### `allowReset`: `boolean`
+### `__next40pxDefaultSize`
+
+Start opting into the larger default height that will become the default size in a future version.
+
+ - Type: `boolean`
+ - Required: No
+ - Default: `false`
+
+### `allowReset`
If this property is true, a button to reset the box control is rendered.
-- Required: No
-- Default: `true`
+ - Type: `boolean`
+ - Required: No
+ - Default: `true`
-### `splitOnAxis`: `boolean`
+### `id`
-If this property is true, when the box control is unlinked, vertical and horizontal controls can be used instead of updating individual sides.
+The id to use as a base for the unique HTML id attribute of the control.
-- Required: No
-- Default: `false`
+ - Type: `string`
+ - Required: No
-### `inputProps`: `object`
+### `inputProps`
-Props for the internal [UnitControl](../unit-control) components.
+Props for the internal `UnitControl` components.
-- Required: No
-- Default: `{ min: 0 }`
+ - Type: `UnitControlPassthroughProps`
+ - Required: No
+ - Default: `{
+ min: 0,
+ }`
-### `label`: `string`
+### `label`
Heading label for the control.
-- Required: No
-- Default: `__( 'Box Control' )`
+ - Type: `string`
+ - Required: No
+ - Default: `__( 'Box Control' )`
-### `onChange`: `(next: BoxControlValue) => void`
+### `onChange`
A callback function when an input value changes.
-- Required: Yes
+ - Type: `(next: BoxControlValue) => void`
+ - Required: No
+ - Default: `() => {}`
-### `resetValues`: `object`
+### `presets`
-The `top`, `right`, `bottom`, and `left` box dimension values to use when the control is reset.
+Available presets to pick from.
-- Required: No
-- Default: `{ top: undefined, right: undefined, bottom: undefined, left: undefined }`
+ - Type: `Preset[]`
+ - Required: No
-### `sides`: `string[]`
+### `presetKey`
-Collection of sides to allow control of. If omitted or empty, all sides will be available. Allowed values are "top", "right", "bottom", "left", "vertical", and "horizontal".
+The key of the preset to apply.
+If you provide a list of presets, you must provide a preset key to use.
+The format of preset selected values is going to be `var:preset|${ presetKey }|${ presetSlug }`
-- Required: No
+ - Type: `string`
+ - Required: No
-### `units`: `WPUnitControlUnit[]`
+### `resetValues`
+
+The `top`, `right`, `bottom`, and `left` box dimension values to use when the control is reset.
-Collection of available units which are compatible with [UnitControl](../unit-control).
+ - Type: `BoxControlValue`
+ - Required: No
+ - Default: `{
+ top: undefined,
+ right: undefined,
+ bottom: undefined,
+ left: undefined,
+ }`
-- Required: No
+### `sides`
-### `values`: `object`
+Collection of sides to allow control of. If omitted or empty, all sides will be available.
-The `top`, `right`, `bottom`, and `left` box dimension values.
+Allowed values are "top", "right", "bottom", "left", "vertical", and "horizontal".
-- Required: No
+ - Type: `readonly (keyof BoxControlValue | "horizontal" | "vertical")[]`
+ - Required: No
-### `onMouseOver`: `function`
+### `splitOnAxis`
-A handler for onMouseOver events.
+If this property is true, when the box control is unlinked, vertical and horizontal controls
+can be used instead of updating individual sides.
-- Required: No
+ - Type: `boolean`
+ - Required: No
+ - Default: `false`
-### `onMouseOut`: `function`
+### `units`
-A handler for onMouseOut events.
+Available units to select from.
-- Required: No
+ - Type: `WPUnitControlUnit[]`
+ - Required: No
+ - Default: `CSS_UNITS`
-### `__next40pxDefaultSize`: `boolean`
+### `values`
-Start opting into the larger default size that will become the default size in a future version.
+The current values of the control, expressed as an object of `top`, `right`, `bottom`, and `left` values.
-- Required: No
-- Default: `false`
+ - Type: `BoxControlValue`
+ - Required: No
diff --git a/packages/components/src/box-control/all-input-control.tsx b/packages/components/src/box-control/all-input-control.tsx
deleted file mode 100644
index e9166ff7f692e..0000000000000
--- a/packages/components/src/box-control/all-input-control.tsx
+++ /dev/null
@@ -1,108 +0,0 @@
-/**
- * WordPress dependencies
- */
-import { useInstanceId } from '@wordpress/compose';
-/**
- * Internal dependencies
- */
-import type { UnitControlProps } from '../unit-control/types';
-import {
- FlexedRangeControl,
- StyledUnitControl,
-} from './styles/box-control-styles';
-import type { BoxControlInputControlProps } from './types';
-import { parseQuantityAndUnitFromRawValue } from '../unit-control';
-import {
- LABELS,
- applyValueToSides,
- getAllValue,
- isValuesMixed,
- isValuesDefined,
- CUSTOM_VALUE_SETTINGS,
-} from './utils';
-
-const noop = () => {};
-
-export default function AllInputControl( {
- __next40pxDefaultSize,
- onChange = noop,
- onFocus = noop,
- values,
- sides,
- selectedUnits,
- setSelectedUnits,
- ...props
-}: BoxControlInputControlProps ) {
- const inputId = useInstanceId( AllInputControl, 'box-control-input-all' );
-
- const allValue = getAllValue( values, selectedUnits, sides );
- const hasValues = isValuesDefined( values );
- const isMixed = hasValues && isValuesMixed( values, selectedUnits, sides );
- const allPlaceholder = isMixed ? LABELS.mixed : undefined;
-
- const [ parsedQuantity, parsedUnit ] =
- parseQuantityAndUnitFromRawValue( allValue );
-
- const handleOnFocus: React.FocusEventHandler< HTMLInputElement > = (
- event
- ) => {
- onFocus( event, { side: 'all' } );
- };
-
- const onValueChange = ( next?: string ) => {
- const isNumeric = next !== undefined && ! isNaN( parseFloat( next ) );
- const nextValue = isNumeric ? next : undefined;
- const nextValues = applyValueToSides( values, nextValue, sides );
-
- onChange( nextValues );
- };
-
- const sliderOnChange = ( next?: number ) => {
- onValueChange(
- next !== undefined ? [ next, parsedUnit ].join( '' ) : undefined
- );
- };
-
- // Set selected unit so it can be used as fallback by unlinked controls
- // when individual sides do not have a value containing a unit.
- const handleOnUnitChange: UnitControlProps[ 'onUnitChange' ] = ( unit ) => {
- const newUnits = applyValueToSides( selectedUnits, unit, sides );
- setSelectedUnits( newUnits );
- };
-
- return (
- <>
-
-
-
- >
- );
-}
diff --git a/packages/components/src/box-control/axial-input-controls.tsx b/packages/components/src/box-control/axial-input-controls.tsx
deleted file mode 100644
index c6c0181f6c871..0000000000000
--- a/packages/components/src/box-control/axial-input-controls.tsx
+++ /dev/null
@@ -1,163 +0,0 @@
-/**
- * WordPress dependencies
- */
-import { useInstanceId } from '@wordpress/compose';
-/**
- * Internal dependencies
- */
-import { parseQuantityAndUnitFromRawValue } from '../unit-control/utils';
-import Tooltip from '../tooltip';
-import { CUSTOM_VALUE_SETTINGS, LABELS } from './utils';
-import {
- FlexedBoxControlIcon,
- FlexedRangeControl,
- InputWrapper,
- StyledUnitControl,
-} from './styles/box-control-styles';
-import type { BoxControlInputControlProps } from './types';
-
-const groupedSides = [ 'vertical', 'horizontal' ] as const;
-type GroupedSide = ( typeof groupedSides )[ number ];
-
-export default function AxialInputControls( {
- __next40pxDefaultSize,
- onChange,
- onFocus,
- values,
- selectedUnits,
- setSelectedUnits,
- sides,
- ...props
-}: BoxControlInputControlProps ) {
- const generatedId = useInstanceId(
- AxialInputControls,
- `box-control-input`
- );
-
- const createHandleOnFocus =
- ( side: GroupedSide ) =>
- ( event: React.FocusEvent< HTMLInputElement > ) => {
- if ( ! onFocus ) {
- return;
- }
- onFocus( event, { side } );
- };
-
- const handleOnValueChange = ( side: GroupedSide, next?: string ) => {
- if ( ! onChange ) {
- return;
- }
- const nextValues = { ...values };
- const isNumeric = next !== undefined && ! isNaN( parseFloat( next ) );
- const nextValue = isNumeric ? next : undefined;
-
- if ( side === 'vertical' ) {
- nextValues.top = nextValue;
- nextValues.bottom = nextValue;
- }
-
- if ( side === 'horizontal' ) {
- nextValues.left = nextValue;
- nextValues.right = nextValue;
- }
-
- onChange( nextValues );
- };
-
- const createHandleOnUnitChange =
- ( side: GroupedSide ) => ( next?: string ) => {
- const newUnits = { ...selectedUnits };
-
- if ( side === 'vertical' ) {
- newUnits.top = next;
- newUnits.bottom = next;
- }
-
- if ( side === 'horizontal' ) {
- newUnits.left = next;
- newUnits.right = next;
- }
-
- setSelectedUnits( newUnits );
- };
-
- // Filter sides if custom configuration provided, maintaining default order.
- const filteredSides = sides?.length
- ? groupedSides.filter( ( side ) => sides.includes( side ) )
- : groupedSides;
-
- return (
- <>
- { filteredSides.map( ( side ) => {
- const [ parsedQuantity, parsedUnit ] =
- parseQuantityAndUnitFromRawValue(
- side === 'vertical' ? values.top : values.left
- );
- const selectedUnit =
- side === 'vertical'
- ? selectedUnits.top
- : selectedUnits.left;
-
- const inputId = [ generatedId, side ].join( '-' );
-
- return (
-
-
-
-
- handleOnValueChange( side, newValue )
- }
- onUnitChange={ createHandleOnUnitChange(
- side
- ) }
- onFocus={ createHandleOnFocus( side ) }
- label={ LABELS[ side ] }
- hideLabelFromVision
- key={ side }
- />
-
-
- handleOnValueChange(
- side,
- newValue !== undefined
- ? [
- newValue,
- selectedUnit ?? parsedUnit,
- ].join( '' )
- : undefined
- )
- }
- min={ 0 }
- max={
- CUSTOM_VALUE_SETTINGS[ selectedUnit ?? 'px' ]
- ?.max ?? 10
- }
- step={
- CUSTOM_VALUE_SETTINGS[ selectedUnit ?? 'px' ]
- ?.step ?? 0.1
- }
- value={ parsedQuantity ?? 0 }
- withInputField={ false }
- />
-
- );
- } ) }
- >
- );
-}
diff --git a/packages/components/src/box-control/docs-manifest.json b/packages/components/src/box-control/docs-manifest.json
new file mode 100644
index 0000000000000..c506e7a9e27ee
--- /dev/null
+++ b/packages/components/src/box-control/docs-manifest.json
@@ -0,0 +1,5 @@
+{
+ "$schema": "../../schemas/docs-manifest.json",
+ "displayName": "BoxControl",
+ "filePath": "./index.tsx"
+}
diff --git a/packages/components/src/box-control/index.tsx b/packages/components/src/box-control/index.tsx
index 41e95aa88bea3..d4d4b03f89303 100644
--- a/packages/components/src/box-control/index.tsx
+++ b/packages/components/src/box-control/index.tsx
@@ -9,13 +9,10 @@ import { __ } from '@wordpress/i18n';
* Internal dependencies
*/
import { BaseControl } from '../base-control';
-import AllInputControl from './all-input-control';
-import InputControls from './input-controls';
-import AxialInputControls from './axial-input-controls';
+import InputControl from './input-control';
import LinkedButton from './linked-button';
import { Grid } from '../grid';
import {
- FlexedBoxControlIcon,
InputWrapper,
ResetButton,
LinkedButtonWrapper,
@@ -24,8 +21,9 @@ import { parseQuantityAndUnitFromRawValue } from '../unit-control/utils';
import {
DEFAULT_VALUES,
getInitialSide,
- isValuesMixed,
+ isValueMixed,
isValuesDefined,
+ getAllowedSides,
} from './utils';
import { useControlledState } from '../utils/hooks';
import type {
@@ -33,6 +31,7 @@ import type {
BoxControlProps,
BoxControlValue,
} from './types';
+import { maybeWarnDeprecated36pxSize } from '../utils/deprecated-36px-size';
const defaultInputProps = {
min: 0,
@@ -51,23 +50,24 @@ function useUniqueId( idProp?: string ) {
* used as an input control for values like `padding` or `margin`.
*
* ```jsx
+ * import { useState } from 'react';
* import { BoxControl } from '@wordpress/components';
- * import { useState } from '@wordpress/element';
*
* function Example() {
- * const [ values, setValues ] = useState( {
- * top: '50px',
- * left: '10%',
- * right: '10%',
- * bottom: '50px',
- * } );
+ * const [ values, setValues ] = useState( {
+ * top: '50px',
+ * left: '10%',
+ * right: '10%',
+ * bottom: '50px',
+ * } );
*
- * return (
- * setValues( nextValues ) }
- * />
- * );
+ * return (
+ *
+ * );
* };
* ```
*/
@@ -83,6 +83,8 @@ function BoxControl( {
splitOnAxis = false,
allowReset = true,
resetValues = DEFAULT_VALUES,
+ presets,
+ presetKey,
onMouseOver,
onMouseOut,
}: BoxControlProps ) {
@@ -95,7 +97,7 @@ function BoxControl( {
const [ isDirty, setIsDirty ] = useState( hasInitialValue );
const [ isLinked, setIsLinked ] = useState(
- ! hasInitialValue || ! isValuesMixed( inputValues ) || hasOneSide
+ ! hasInitialValue || ! isValueMixed( inputValues ) || hasOneSide
);
const [ side, setSide ] = useState< BoxControlIconProps[ 'side' ] >(
@@ -141,6 +143,8 @@ function BoxControl( {
};
const inputControlProps = {
+ onMouseOver,
+ onMouseOut,
...inputProps,
onChange: handleOnChange,
onFocus: handleOnFocus,
@@ -150,11 +154,18 @@ function BoxControl( {
setSelectedUnits,
sides,
values: inputValues,
- onMouseOver,
- onMouseOut,
__next40pxDefaultSize,
+ presets,
+ presetKey,
};
+ maybeWarnDeprecated36pxSize( {
+ componentName: 'BoxControl',
+ __next40pxDefaultSize,
+ size: undefined,
+ } );
+ const sidesToRender = getAllowedSides( sides );
+
return (
{ isLinked && (
-
-
+
) }
{ ! hasOneSide && (
@@ -181,12 +191,24 @@ function BoxControl( {
) }
- { ! isLinked && splitOnAxis && (
-
- ) }
- { ! isLinked && ! splitOnAxis && (
-
- ) }
+ { ! isLinked &&
+ splitOnAxis &&
+ [ 'vertical', 'horizontal' ].map( ( axis ) => (
+
+ ) ) }
+ { ! isLinked &&
+ ! splitOnAxis &&
+ Array.from( sidesToRender ).map( ( axis ) => (
+
+ ) ) }
{ allowReset && (
{};
+
+function getSidesToModify(
+ side: BoxControlInputControlProps[ 'side' ],
+ sides: BoxControlInputControlProps[ 'sides' ],
+ isAlt?: boolean
+) {
+ const allowedSides = getAllowedSides( sides );
+
+ let modifiedSides: ( keyof BoxControlValue )[] = [];
+ switch ( side ) {
+ case 'all':
+ modifiedSides = [ 'top', 'bottom', 'left', 'right' ];
+ break;
+ case 'horizontal':
+ modifiedSides = [ 'left', 'right' ];
+ break;
+ case 'vertical':
+ modifiedSides = [ 'top', 'bottom' ];
+ break;
+ default:
+ modifiedSides = [ side ];
+ }
+
+ if ( isAlt ) {
+ switch ( side ) {
+ case 'top':
+ modifiedSides.push( 'bottom' );
+ break;
+ case 'bottom':
+ modifiedSides.push( 'top' );
+ break;
+ case 'left':
+ modifiedSides.push( 'left' );
+ break;
+ case 'right':
+ modifiedSides.push( 'right' );
+ break;
+ }
+ }
+
+ return modifiedSides.filter( ( s ) => allowedSides.has( s ) );
+}
+
+export default function BoxInputControl( {
+ __next40pxDefaultSize,
+ onChange = noop,
+ onFocus = noop,
+ values,
+ selectedUnits,
+ setSelectedUnits,
+ sides,
+ side,
+ min = 0,
+ presets,
+ presetKey,
+ ...props
+}: BoxControlInputControlProps ) {
+ const defaultValuesToModify = getSidesToModify( side, sides );
+
+ const handleOnFocus = ( event: React.FocusEvent< HTMLInputElement > ) => {
+ onFocus( event, { side } );
+ };
+
+ const handleOnChange = ( nextValues: BoxControlValue ) => {
+ onChange( nextValues );
+ };
+
+ const handleRawOnValueChange = ( next?: string ) => {
+ const nextValues = { ...values };
+ defaultValuesToModify.forEach( ( modifiedSide ) => {
+ nextValues[ modifiedSide ] = next;
+ } );
+
+ handleOnChange( nextValues );
+ };
+
+ const handleOnValueChange = (
+ next?: string,
+ extra?: { event: React.SyntheticEvent< Element, Event > }
+ ) => {
+ const nextValues = { ...values };
+ const isNumeric = next !== undefined && ! isNaN( parseFloat( next ) );
+ const nextValue = isNumeric ? next : undefined;
+ const modifiedSides = getSidesToModify(
+ side,
+ sides,
+ /**
+ * Supports changing pair sides. For example, holding the ALT key
+ * when changing the TOP will also update BOTTOM.
+ */
+ // @ts-expect-error - TODO: event.altKey is only present when the change event was
+ // triggered by a keyboard event. Should this feature be implemented differently so
+ // it also works with drag events?
+ !! extra?.event.altKey
+ );
+
+ modifiedSides.forEach( ( modifiedSide ) => {
+ nextValues[ modifiedSide ] = nextValue;
+ } );
+
+ handleOnChange( nextValues );
+ };
+
+ const handleOnUnitChange = ( next?: string ) => {
+ const newUnits = { ...selectedUnits };
+ defaultValuesToModify.forEach( ( modifiedSide ) => {
+ newUnits[ modifiedSide ] = next;
+ } );
+ setSelectedUnits( newUnits );
+ };
+
+ const mergedValue = getMergedValue( values, defaultValuesToModify );
+ const hasValues = isValuesDefined( values );
+ const isMixed =
+ hasValues &&
+ defaultValuesToModify.length > 1 &&
+ isValueMixed( values, defaultValuesToModify );
+ const [ parsedQuantity, parsedUnit ] =
+ parseQuantityAndUnitFromRawValue( mergedValue );
+ const computedUnit = hasValues
+ ? parsedUnit
+ : selectedUnits[ defaultValuesToModify[ 0 ] ];
+ const generatedId = useInstanceId( BoxInputControl, 'box-control-input' );
+ const inputId = [ generatedId, side ].join( '-' );
+ const isMixedUnit =
+ defaultValuesToModify.length > 1 &&
+ mergedValue === undefined &&
+ defaultValuesToModify.some(
+ ( s ) => selectedUnits[ s ] !== computedUnit
+ );
+ const usedValue =
+ mergedValue === undefined && computedUnit ? computedUnit : mergedValue;
+ const mixedPlaceholder = isMixed || isMixedUnit ? __( 'Mixed' ) : undefined;
+ const hasPresets = presets && presets.length > 0 && presetKey;
+ const hasPresetValue =
+ hasPresets &&
+ mergedValue !== undefined &&
+ ! isMixed &&
+ isValuePreset( mergedValue, presetKey );
+ const [ showCustomValueControl, setShowCustomValueControl ] = useState(
+ ! hasPresets ||
+ ( ! hasPresetValue && ! isMixed && mergedValue !== undefined )
+ );
+ const presetIndex = hasPresetValue
+ ? getPresetIndexFromValue( mergedValue, presetKey, presets )
+ : undefined;
+ const marks = hasPresets
+ ? [ { value: 0, label: '', tooltip: __( 'None' ) } ].concat(
+ presets.map( ( preset, index ) => ( {
+ value: index + 1,
+ label: '',
+ tooltip: preset.name ?? preset.slug,
+ } ) )
+ )
+ : [];
+
+ return (
+
+
+ { showCustomValueControl && (
+ <>
+
+
+
+
+ {
+ handleOnValueChange(
+ newValue !== undefined
+ ? [ newValue, computedUnit ].join( '' )
+ : undefined
+ );
+ } }
+ min={ isFinite( min ) ? min : 0 }
+ max={
+ CUSTOM_VALUE_SETTINGS[ computedUnit ?? 'px' ]
+ ?.max ?? 10
+ }
+ step={
+ CUSTOM_VALUE_SETTINGS[ computedUnit ?? 'px' ]
+ ?.step ?? 0.1
+ }
+ value={ parsedQuantity ?? 0 }
+ withInputField={ false }
+ />
+ >
+ ) }
+
+ { hasPresets && ! showCustomValueControl && (
+ {
+ const newValue =
+ newIndex === 0 || newIndex === undefined
+ ? undefined
+ : getPresetValueFromIndex(
+ newIndex - 1,
+ presetKey,
+ presets
+ );
+ handleRawOnValueChange( newValue );
+ } }
+ withInputField={ false }
+ aria-valuenow={
+ presetIndex !== undefined ? presetIndex + 1 : 0
+ }
+ aria-valuetext={
+ marks[ presetIndex !== undefined ? presetIndex + 1 : 0 ]
+ .label
+ }
+ renderTooltipContent={ ( index ) =>
+ marks[ ! index ? 0 : index ].tooltip
+ }
+ min={ 0 }
+ max={ marks.length - 1 }
+ marks={ marks }
+ label={ LABELS[ side ] }
+ hideLabelFromVision
+ __nextHasNoMarginBottom
+ />
+ ) }
+
+ { hasPresets && (
+ {
+ setShowCustomValueControl( ! showCustomValueControl );
+ } }
+ isPressed={ showCustomValueControl }
+ size="small"
+ iconSize={ 24 }
+ />
+ ) }
+
+ );
+}
diff --git a/packages/components/src/box-control/input-controls.tsx b/packages/components/src/box-control/input-controls.tsx
deleted file mode 100644
index 553f547abf9b0..0000000000000
--- a/packages/components/src/box-control/input-controls.tsx
+++ /dev/null
@@ -1,165 +0,0 @@
-/**
- * WordPress dependencies
- */
-import { useInstanceId } from '@wordpress/compose';
-/**
- * Internal dependencies
- */
-import Tooltip from '../tooltip';
-import { parseQuantityAndUnitFromRawValue } from '../unit-control/utils';
-import { ALL_SIDES, CUSTOM_VALUE_SETTINGS, LABELS } from './utils';
-import {
- FlexedBoxControlIcon,
- FlexedRangeControl,
- InputWrapper,
- StyledUnitControl,
-} from './styles/box-control-styles';
-import type { BoxControlInputControlProps, BoxControlValue } from './types';
-
-const noop = () => {};
-
-export default function BoxInputControls( {
- __next40pxDefaultSize,
- onChange = noop,
- onFocus = noop,
- values,
- selectedUnits,
- setSelectedUnits,
- sides,
- ...props
-}: BoxControlInputControlProps ) {
- const generatedId = useInstanceId( BoxInputControls, 'box-control-input' );
-
- const createHandleOnFocus =
- ( side: keyof BoxControlValue ) =>
- ( event: React.FocusEvent< HTMLInputElement > ) => {
- onFocus( event, { side } );
- };
-
- const handleOnChange = ( nextValues: BoxControlValue ) => {
- onChange( nextValues );
- };
-
- const handleOnValueChange = (
- side: keyof BoxControlValue,
- next?: string,
- extra?: { event: React.SyntheticEvent< Element, Event > }
- ) => {
- const nextValues = { ...values };
- const isNumeric = next !== undefined && ! isNaN( parseFloat( next ) );
- const nextValue = isNumeric ? next : undefined;
-
- nextValues[ side ] = nextValue;
-
- /**
- * Supports changing pair sides. For example, holding the ALT key
- * when changing the TOP will also update BOTTOM.
- */
- // @ts-expect-error - TODO: event.altKey is only present when the change event was
- // triggered by a keyboard event. Should this feature be implemented differently so
- // it also works with drag events?
- if ( extra?.event.altKey ) {
- switch ( side ) {
- case 'top':
- nextValues.bottom = nextValue;
- break;
- case 'bottom':
- nextValues.top = nextValue;
- break;
- case 'left':
- nextValues.right = nextValue;
- break;
- case 'right':
- nextValues.left = nextValue;
- break;
- }
- }
-
- handleOnChange( nextValues );
- };
-
- const createHandleOnUnitChange =
- ( side: keyof BoxControlValue ) => ( next?: string ) => {
- const newUnits = { ...selectedUnits };
- newUnits[ side ] = next;
- setSelectedUnits( newUnits );
- };
-
- // Filter sides if custom configuration provided, maintaining default order.
- const filteredSides = sides?.length
- ? ALL_SIDES.filter( ( side ) => sides.includes( side ) )
- : ALL_SIDES;
-
- return (
- <>
- { filteredSides.map( ( side ) => {
- const [ parsedQuantity, parsedUnit ] =
- parseQuantityAndUnitFromRawValue( values[ side ] );
-
- const computedUnit = values[ side ]
- ? parsedUnit
- : selectedUnits[ side ];
-
- const inputId = [ generatedId, side ].join( '-' );
-
- return (
-
-
-
-
- handleOnValueChange(
- side,
- nextValue,
- extra
- )
- }
- onUnitChange={ createHandleOnUnitChange(
- side
- ) }
- onFocus={ createHandleOnFocus( side ) }
- label={ LABELS[ side ] }
- hideLabelFromVision
- />
-
-
- {
- handleOnValueChange(
- side,
- newValue !== undefined
- ? [ newValue, computedUnit ].join( '' )
- : undefined
- );
- } }
- min={ 0 }
- max={
- CUSTOM_VALUE_SETTINGS[ computedUnit ?? 'px' ]
- ?.max ?? 10
- }
- step={
- CUSTOM_VALUE_SETTINGS[ computedUnit ?? 'px' ]
- ?.step ?? 0.1
- }
- value={ parsedQuantity ?? 0 }
- withInputField={ false }
- />
-
- );
- } ) }
- >
- );
-}
diff --git a/packages/components/src/box-control/stories/index.story.tsx b/packages/components/src/box-control/stories/index.story.tsx
index 783f9d047b1bb..aa16547d24ab1 100644
--- a/packages/components/src/box-control/stories/index.story.tsx
+++ b/packages/components/src/box-control/stories/index.story.tsx
@@ -17,7 +17,7 @@ const meta: Meta< typeof BoxControl > = {
title: 'Components/BoxControl',
component: BoxControl,
argTypes: {
- values: { control: { type: null } },
+ values: { control: false },
},
parameters: {
actions: { argTypesRegex: '^on.*' },
@@ -49,6 +49,7 @@ const TemplateControlled: StoryFn< typeof BoxControl > = ( props ) => {
export const Default = TemplateUncontrolled.bind( {} );
Default.args = {
label: 'Label',
+ __next40pxDefaultSize: true,
};
export const Controlled = TemplateControlled.bind( {} );
@@ -80,3 +81,15 @@ AxialControlsWithSingleSide.args = {
sides: [ 'horizontal' ],
splitOnAxis: true,
};
+
+export const ControlWithPresets = TemplateControlled.bind( {} );
+ControlWithPresets.args = {
+ ...Default.args,
+ presets: [
+ { name: 'Small', slug: 'small', value: '4px' },
+ { name: 'Medium', slug: 'medium', value: '8px' },
+ { name: 'Large', slug: 'large', value: '12px' },
+ { name: 'Extra Large', slug: 'extra-large', value: '16px' },
+ ],
+ presetKey: 'padding',
+};
diff --git a/packages/components/src/box-control/test/index.tsx b/packages/components/src/box-control/test/index.tsx
index 681e7721d0c13..185a18b258951 100644
--- a/packages/components/src/box-control/test/index.tsx
+++ b/packages/components/src/box-control/test/index.tsx
@@ -15,11 +15,14 @@ import { useState } from '@wordpress/element';
import BoxControl from '..';
import type { BoxControlProps, BoxControlValue } from '../types';
-const Example = ( extraProps: Omit< BoxControlProps, 'onChange' > ) => {
+const ControlledBoxControl = (
+ extraProps: Omit< BoxControlProps, 'onChange' >
+) => {
const [ state, setState ] = useState< BoxControlValue >();
return (
setState( next ) }
{ ...extraProps }
@@ -27,10 +30,17 @@ const Example = ( extraProps: Omit< BoxControlProps, 'onChange' > ) => {
);
};
+const UncontrolledBoxControl = ( {
+ onChange = () => {},
+ ...props
+}: Omit< BoxControlProps, 'onChange' > & {
+ onChange?: BoxControlProps[ 'onChange' ];
+} ) => ;
+
describe( 'BoxControl', () => {
describe( 'Basic rendering', () => {
it( 'should render a box control input', () => {
- render( {} } /> );
+ render( );
expect(
screen.getByRole( 'group', { name: 'Box Control' } )
@@ -43,7 +53,7 @@ describe( 'BoxControl', () => {
it( 'should update values when interacting with input', async () => {
const user = userEvent.setup();
- render( {} } /> );
+ render( );
const input = screen.getByRole( 'textbox', { name: 'All sides' } );
@@ -54,7 +64,7 @@ describe( 'BoxControl', () => {
} );
it( 'should update input values when interacting with slider', () => {
- render( {} } /> );
+ render( );
const slider = screen.getByRole( 'slider' );
@@ -68,7 +78,7 @@ describe( 'BoxControl', () => {
it( 'should update slider values when interacting with input', async () => {
const user = userEvent.setup();
- render( {} } /> );
+ render( );
const input = screen.getByRole( 'textbox', {
name: 'All sides',
@@ -82,7 +92,7 @@ describe( 'BoxControl', () => {
} );
it( 'should render the number input with a default min value of 0', () => {
- render( {} } /> );
+ render( );
const input = screen.getByRole( 'textbox', { name: 'All sides' } );
@@ -91,10 +101,7 @@ describe( 'BoxControl', () => {
it( 'should pass down `inputProps` to the underlying number input', () => {
render(
- {} }
- inputProps={ { min: 10, max: 50 } }
- />
+
);
const input = screen.getByRole( 'textbox', { name: 'All sides' } );
@@ -108,7 +115,7 @@ describe( 'BoxControl', () => {
it( 'should reset values when clicking Reset', async () => {
const user = userEvent.setup();
- render( {} } /> );
+ render( );
const input = screen.getByRole( 'textbox', {
name: 'All sides',
@@ -127,7 +134,7 @@ describe( 'BoxControl', () => {
it( 'should reset values when clicking Reset, if controlled', async () => {
const user = userEvent.setup();
- render( );
+ render( );
const input = screen.getByRole( 'textbox', {
name: 'All sides',
@@ -146,7 +153,7 @@ describe( 'BoxControl', () => {
it( 'should reset values when clicking Reset, if controlled <-> uncontrolled state changes', async () => {
const user = userEvent.setup();
- render( );
+ render( );
const input = screen.getByRole( 'textbox', {
name: 'All sides',
@@ -166,7 +173,9 @@ describe( 'BoxControl', () => {
const user = userEvent.setup();
const spyChange = jest.fn();
- render( spyChange( v ) } /> );
+ render(
+ spyChange( v ) } />
+ );
const input = screen.getByRole( 'textbox', {
name: 'All sides',
@@ -196,7 +205,7 @@ describe( 'BoxControl', () => {
it( 'should update a single side value when unlinked', async () => {
const user = userEvent.setup();
- render( );
+ render( );
await user.click(
screen.getByRole( 'button', { name: 'Unlink sides' } )
@@ -224,7 +233,7 @@ describe( 'BoxControl', () => {
it( 'should update a single side value when using slider unlinked', async () => {
const user = userEvent.setup();
- render( );
+ render( );
await user.click(
screen.getByRole( 'button', { name: 'Unlink sides' } )
@@ -252,7 +261,7 @@ describe( 'BoxControl', () => {
it( 'should update a whole axis when value is changed when unlinked', async () => {
const user = userEvent.setup();
- render( );
+ render( );
await user.click(
screen.getByRole( 'button', { name: 'Unlink sides' } )
@@ -276,7 +285,7 @@ describe( 'BoxControl', () => {
it( 'should update a whole axis using a slider when value is changed when unlinked', async () => {
const user = userEvent.setup();
- render( );
+ render( );
await user.click(
screen.getByRole( 'button', { name: 'Unlink sides' } )
@@ -300,7 +309,7 @@ describe( 'BoxControl', () => {
it( 'should show "Mixed" label when sides have different values but are linked', async () => {
const user = userEvent.setup();
- render( );
+ render( );
const unlinkButton = screen.getByRole( 'button', {
name: 'Unlink sides',
@@ -330,7 +339,7 @@ describe( 'BoxControl', () => {
const user = userEvent.setup();
// Render control.
- render( {} } /> );
+ render( );
// Make unit selection on all input control.
await user.selectOptions(
@@ -362,7 +371,7 @@ describe( 'BoxControl', () => {
const user = userEvent.setup();
// Render control.
- const { rerender } = render( {} } /> );
+ const { rerender } = render( );
// Make unit selection on all input control.
await user.selectOptions(
@@ -390,9 +399,7 @@ describe( 'BoxControl', () => {
} );
// Rerender with individual side value & confirm unit is selected.
- rerender(
- {} } />
- );
+ rerender( );
const rerenderedControls = screen.getAllByRole( 'combobox', {
name: 'Select unit',
@@ -414,7 +421,7 @@ describe( 'BoxControl', () => {
const user = userEvent.setup();
const onChangeSpy = jest.fn();
- render( );
+ render( );
const valueInput = screen.getByRole( 'textbox', {
name: 'All sides',
@@ -443,7 +450,7 @@ describe( 'BoxControl', () => {
const user = userEvent.setup();
const setState = jest.fn();
- render( );
+ render( );
await user.selectOptions(
screen.getByRole( 'combobox', {
diff --git a/packages/components/src/box-control/types.ts b/packages/components/src/box-control/types.ts
index 5f4071aeed88a..43629e09258a5 100644
--- a/packages/components/src/box-control/types.ts
+++ b/packages/components/src/box-control/types.ts
@@ -15,70 +15,103 @@ export type CustomValueUnits = {
[ key: string ]: { max: number; step: number };
};
+export interface Preset {
+ name: string;
+ slug: string;
+ value?: string;
+}
+
type UnitControlPassthroughProps = Omit<
UnitControlProps,
- 'label' | 'onChange' | 'onFocus' | 'onMouseOver' | 'onMouseOut' | 'units'
+ 'label' | 'onChange' | 'onFocus' | 'units'
>;
-export type BoxControlProps = Pick<
- UnitControlProps,
- 'onMouseOver' | 'onMouseOut' | 'units'
-> & {
- /**
- * If this property is true, a button to reset the box control is rendered.
- *
- * @default true
- */
- allowReset?: boolean;
- /**
- * The id to use as a base for the unique HTML id attribute of the control.
- */
- id?: string;
- /**
- * Props for the internal `UnitControl` components.
- *
- * @default { min: 0 }
- */
- inputProps?: UnitControlPassthroughProps;
- /**
- * Heading label for the control.
- *
- * @default __( 'Box Control' )
- */
- label?: string;
+type DeprecatedBoxControlProps = {
/**
- * A callback function when an input value changes.
+ * @deprecated Pass to the `inputProps` prop instead.
+ * @ignore
*/
- onChange: ( next: BoxControlValue ) => void;
+ onMouseOver?: UnitControlProps[ 'onMouseOver' ];
/**
- * The `top`, `right`, `bottom`, and `left` box dimension values to use when the control is reset.
- *
- * @default { top: undefined, right: undefined, bottom: undefined, left: undefined }
+ * @deprecated Pass to the `inputProps` prop instead.
+ * @ignore
*/
- resetValues?: BoxControlValue;
- /**
- * Collection of sides to allow control of. If omitted or empty, all sides will be available.
- */
- sides?: readonly ( keyof BoxControlValue | 'horizontal' | 'vertical' )[];
- /**
- * If this property is true, when the box control is unlinked, vertical and horizontal controls
- * can be used instead of updating individual sides.
- *
- * @default false
- */
- splitOnAxis?: boolean;
- /**
- * The current values of the control, expressed as an object of `top`, `right`, `bottom`, and `left` values.
- */
- values?: BoxControlValue;
- /**
- * Start opting into the larger default height that will become the default size in a future version.
- *
- * @default false
- */
- __next40pxDefaultSize?: boolean;
+ onMouseOut?: UnitControlProps[ 'onMouseOut' ];
};
+export type BoxControlProps = Pick< UnitControlProps, 'units' > &
+ DeprecatedBoxControlProps & {
+ /**
+ * If this property is true, a button to reset the box control is rendered.
+ *
+ * @default true
+ */
+ allowReset?: boolean;
+ /**
+ * The id to use as a base for the unique HTML id attribute of the control.
+ */
+ id?: string;
+ /**
+ * Props for the internal `UnitControl` components.
+ *
+ * @default { min: 0 }
+ */
+ inputProps?: UnitControlPassthroughProps;
+ /**
+ * Heading label for the control.
+ *
+ * @default __( 'Box Control' )
+ */
+ label?: string;
+ /**
+ * A callback function when an input value changes.
+ */
+ onChange: ( next: BoxControlValue ) => void;
+ /**
+ * The `top`, `right`, `bottom`, and `left` box dimension values to use when the control is reset.
+ *
+ * @default { top: undefined, right: undefined, bottom: undefined, left: undefined }
+ */
+ resetValues?: BoxControlValue;
+ /**
+ * Collection of sides to allow control of. If omitted or empty, all sides will be available.
+ *
+ * Allowed values are "top", "right", "bottom", "left", "vertical", and "horizontal".
+ */
+ sides?: readonly (
+ | keyof BoxControlValue
+ | 'horizontal'
+ | 'vertical'
+ )[];
+ /**
+ * If this property is true, when the box control is unlinked, vertical and horizontal controls
+ * can be used instead of updating individual sides.
+ *
+ * @default false
+ */
+ splitOnAxis?: boolean;
+ /**
+ * The current values of the control, expressed as an object of `top`, `right`, `bottom`, and `left` values.
+ */
+ values?: BoxControlValue;
+ /**
+ * Start opting into the larger default height that will become the default size in a future version.
+ *
+ * @default false
+ */
+ __next40pxDefaultSize?: boolean;
+ /**
+ * Available presets to pick from.
+ */
+ presets?: Preset[];
+ /**
+ * The key of the preset to apply.
+ * If you provide a list of presets, you must provide a preset key to use.
+ * The format of preset selected values is going to be `var:preset|${ presetKey }|${ presetSlug }`
+ */
+ presetKey?: string;
+ };
+
export type BoxControlInputControlProps = UnitControlPassthroughProps & {
onChange?: ( nextValues: BoxControlValue ) => void;
onFocus?: (
@@ -93,8 +126,18 @@ export type BoxControlInputControlProps = UnitControlPassthroughProps & {
) => void;
selectedUnits: BoxControlValue;
setSelectedUnits: React.Dispatch< React.SetStateAction< BoxControlValue > >;
- sides: BoxControlProps[ 'sides' ];
values: BoxControlValue;
+ /**
+ * Collection of sides to allow control of. If omitted or empty, all sides will be available.
+ */
+ sides: BoxControlProps[ 'sides' ];
+ /**
+ * Side represents the current side being rendered by the input.
+ * It can be a concrete side like: left, right, top, bottom or a combined one like: horizontal, vertical.
+ */
+ side: keyof typeof LABELS;
+ presets?: Preset[];
+ presetKey?: string;
};
export type BoxControlIconProps = {
diff --git a/packages/components/src/box-control/utils.ts b/packages/components/src/box-control/utils.ts
index 73c7f4a6a46cf..26bdae4e55951 100644
--- a/packages/components/src/box-control/utils.ts
+++ b/packages/components/src/box-control/utils.ts
@@ -6,12 +6,14 @@ import { __ } from '@wordpress/i18n';
/**
* Internal dependencies
*/
-import { parseQuantityAndUnitFromRawValue } from '../unit-control/utils';
import type {
+ BoxControlInputControlProps,
BoxControlProps,
BoxControlValue,
CustomValueUnits,
+ Preset,
} from './types';
+import deprecated from '@wordpress/deprecated';
export const CUSTOM_VALUE_SETTINGS: CustomValueUnits = {
px: { max: 300, step: 1 },
@@ -50,7 +52,6 @@ export const LABELS = {
bottom: __( 'Bottom side' ),
left: __( 'Left side' ),
right: __( 'Right side' ),
- mixed: __( 'Mixed' ),
vertical: __( 'Top and bottom sides' ),
horizontal: __( 'Left and right sides' ),
};
@@ -82,56 +83,46 @@ function mode< T >( arr: T[] ) {
}
/**
- * Gets the 'all' input value and unit from values data.
+ * Gets the merged input value and unit from values data.
*
* @param values Box values.
- * @param selectedUnits Box units.
* @param availableSides Available box sides to evaluate.
*
* @return A value + unit for the 'all' input.
*/
-export function getAllValue(
+export function getMergedValue(
values: BoxControlValue = {},
- selectedUnits?: BoxControlValue,
availableSides: BoxControlProps[ 'sides' ] = ALL_SIDES
) {
const sides = normalizeSides( availableSides );
- const parsedQuantitiesAndUnits = sides.map( ( side ) =>
- parseQuantityAndUnitFromRawValue( values[ side ] )
- );
- const allParsedQuantities = parsedQuantitiesAndUnits.map(
- ( value ) => value[ 0 ] ?? ''
- );
- const allParsedUnits = parsedQuantitiesAndUnits.map(
- ( value ) => value[ 1 ]
- );
-
- const commonQuantity = allParsedQuantities.every(
- ( v ) => v === allParsedQuantities[ 0 ]
- )
- ? allParsedQuantities[ 0 ]
- : '';
-
- /**
- * The typeof === 'number' check is important. On reset actions, the incoming value
- * may be null or an empty string.
- *
- * Also, the value may also be zero (0), which is considered a valid unit value.
- *
- * typeof === 'number' is more specific for these cases, rather than relying on a
- * simple truthy check.
- */
- let commonUnit;
- if ( typeof commonQuantity === 'number' ) {
- commonUnit = mode( allParsedUnits );
- } else {
- // Set meaningful unit selection if no commonQuantity and user has previously
- // selected units without assigning values while controls were unlinked.
- commonUnit =
- getAllUnitFallback( selectedUnits ) ?? mode( allParsedUnits );
+ if (
+ sides.every(
+ ( side: keyof BoxControlValue ) =>
+ values[ side ] === values[ sides[ 0 ] ]
+ )
+ ) {
+ return values[ sides[ 0 ] ];
}
- return [ commonQuantity, commonUnit ].join( '' );
+ return undefined;
+}
+
+/**
+ * Checks if the values are mixed.
+ *
+ * @param values Box values.
+ * @param availableSides Available box sides to evaluate.
+ * @return Whether the values are mixed.
+ */
+export function isValueMixed(
+ values: BoxControlValue = {},
+ availableSides: BoxControlProps[ 'sides' ] = ALL_SIDES
+) {
+ const sides = normalizeSides( availableSides );
+ return sides.some(
+ ( side: keyof BoxControlValue ) =>
+ values[ side ] !== values[ sides[ 0 ] ]
+ );
}
/**
@@ -150,26 +141,6 @@ export function getAllUnitFallback( selectedUnits?: BoxControlValue ) {
return mode( filteredUnits );
}
-/**
- * Checks to determine if values are mixed.
- *
- * @param values Box values.
- * @param selectedUnits Box units.
- * @param sides Available box sides to evaluate.
- *
- * @return Whether values are mixed.
- */
-export function isValuesMixed(
- values: BoxControlValue = {},
- selectedUnits?: BoxControlValue,
- sides: BoxControlProps[ 'sides' ] = ALL_SIDES
-) {
- const allValue = getAllValue( values, selectedUnits, sides );
- const isMixed = isNaN( parseFloat( allValue ) );
-
- return isMixed;
-}
-
/**
* Checks to determine if values are defined.
*
@@ -239,6 +210,8 @@ export function normalizeSides( sides: BoxControlProps[ 'sides' ] ) {
* Applies a value to an object representing top, right, bottom and left sides
* while taking into account any custom side configuration.
*
+ * @deprecated
+ *
* @param currentValues The current values for each side.
* @param newValue The value to apply to the sides object.
* @param sides Array defining valid sides.
@@ -250,6 +223,10 @@ export function applyValueToSides(
newValue?: string,
sides?: BoxControlProps[ 'sides' ]
): BoxControlValue {
+ deprecated( 'applyValueToSides', {
+ since: '6.8',
+ version: '7.0',
+ } );
const newValues = { ...currentValues };
if ( sides?.length ) {
@@ -270,3 +247,88 @@ export function applyValueToSides(
return newValues;
}
+
+/**
+ * Return the allowed sides based on the sides configuration.
+ *
+ * @param sides Sides configuration.
+ * @return Allowed sides.
+ */
+export function getAllowedSides(
+ sides: BoxControlInputControlProps[ 'sides' ]
+) {
+ const allowedSides: Set< keyof BoxControlValue > = new Set(
+ ! sides ? ALL_SIDES : []
+ );
+ sides?.forEach( ( allowedSide ) => {
+ if ( allowedSide === 'vertical' ) {
+ allowedSides.add( 'top' );
+ allowedSides.add( 'bottom' );
+ } else if ( allowedSide === 'horizontal' ) {
+ allowedSides.add( 'right' );
+ allowedSides.add( 'left' );
+ } else {
+ allowedSides.add( allowedSide );
+ }
+ } );
+ return allowedSides;
+}
+
+/**
+ * Checks if a value is a preset value.
+ *
+ * @param value The value to check.
+ * @param presetKey The preset key to check against.
+ * @return Whether the value is a preset value.
+ */
+export function isValuePreset( value: string, presetKey: string ) {
+ return value.startsWith( `var:preset|${ presetKey }|` );
+}
+
+/**
+ * Returns the index of the preset value in the presets array.
+ *
+ * @param value The value to check.
+ * @param presetKey The preset key to check against.
+ * @param presets The array of presets to search.
+ * @return The index of the preset value in the presets array.
+ */
+export function getPresetIndexFromValue(
+ value: string,
+ presetKey: string,
+ presets: Preset[]
+) {
+ if ( ! isValuePreset( value, presetKey ) ) {
+ return undefined;
+ }
+
+ const match = value.match(
+ new RegExp( `^var:preset\\|${ presetKey }\\|(.+)$` )
+ );
+ if ( ! match ) {
+ return undefined;
+ }
+ const slug = match[ 1 ];
+ const index = presets.findIndex( ( preset ) => {
+ return preset.slug === slug;
+ } );
+
+ return index !== -1 ? index : undefined;
+}
+
+/**
+ * Returns the preset value from the index.
+ *
+ * @param index The index of the preset value in the presets array.
+ * @param presetKey The preset key to check against.
+ * @param presets The array of presets to search.
+ * @return The preset value from the index.
+ */
+export function getPresetValueFromIndex(
+ index: number,
+ presetKey: string,
+ presets: Preset[]
+) {
+ const preset = presets[ index ];
+ return `var:preset|${ presetKey }|${ preset.slug }`;
+}
diff --git a/packages/components/src/button-group/stories/index.story.tsx b/packages/components/src/button-group/stories/index.story.tsx
index f6af2416977f4..4b5ab3d5dfdb6 100644
--- a/packages/components/src/button-group/stories/index.story.tsx
+++ b/packages/components/src/button-group/stories/index.story.tsx
@@ -13,7 +13,7 @@ const meta: Meta< typeof ButtonGroup > = {
title: 'Components/ButtonGroup',
component: ButtonGroup,
argTypes: {
- children: { control: { type: null } },
+ children: { control: false },
},
parameters: {
controls: { expanded: true },
diff --git a/packages/components/src/button/README.md b/packages/components/src/button/README.md
index d458771494a33..99a6d0f9c24cf 100644
--- a/packages/components/src/button/README.md
+++ b/packages/components/src/button/README.md
@@ -1,304 +1,193 @@
# Button
-Buttons let users take actions and make choices with a single click or tap.
+
-
+See the WordPress Storybook for more detailed, interactive documentation.
-## Design guidelines
-
-### Usage
-
-Buttons tell users what actions they can take and give them a way to interact with the interface. You’ll find them throughout a UI, particularly in places like:
-
-- Modals
-- Forms
-- Toolbars
-
-### Best practices
-
-Buttons should:
-
-- **Be clearly and accurately labeled.**
-- **Clearly communicate that clicking or tapping will trigger an action.**
-- **Use established colors appropriately.** For example, only use red buttons for actions that are difficult or impossible to undo.
-- **Prioritize the most important actions.** This helps users focus. Too many calls to action on one screen can be confusing, making users unsure what to do next.
-- **Have consistent locations in the interface.**
-
-### Content guidelines
-
-Buttons should be clear and predictable—users should be able to anticipate what will happen when they click a button. Never deceive a user by mislabeling a button.
-
-Buttons text should lead with a strong verb that encourages action, and add a noun that clarifies what will actually change. The only exceptions are common actions like Save, Close, Cancel, or OK. Otherwise, use the {verb}+{noun} format to ensure that your button gives the user enough information.
-
-Button text should also be quickly scannable — avoid unnecessary words and articles like the, an, or a.
-
-### Types
-
-#### Link button
-
-Link buttons have low emphasis. They don’t stand out much on the page, so they’re used for less-important actions. What’s less important can vary based on context, but it’s usually a supplementary action to the main action we want someone to take. Link buttons are also useful when you don’t want to distract from the content.
-
-
-
-#### Default button
-
-Default buttons have medium emphasis. The button appearance helps differentiate them from the page background, so they’re useful when you want more emphasis than a link button offers.
-
-
-
-#### Primary button
-
-Primary buttons have high emphasis. Their color fill and shadow means they pop off the background.
-
-Since a high-emphasis button commands the most attention, a layout should contain a single primary button. This makes it clear that other buttons have less importance and helps users understand when an action requires their attention.
-
-
-
-#### Text label
-
-All button types use text labels to describe the action that happens when a user taps a button. If there’s no text label, there needs to be a [label](#label) added and an icon to signify what the button does.
-
-
-
-**Do**
-Use color to distinguish link button labels from other text.
-
-
-
-**Don’t**
-Don’t wrap button text. For maximum legibility, keep text labels on a single line.
-
-### Hierarchy
-
-
-
-A layout should contain a single prominently-located button. If multiple buttons are required, a single high-emphasis button can be joined by medium- and low-emphasis buttons mapped to less-important actions. When using multiple buttons, make sure the available state of one button doesn’t look like the disabled state of another.
-
-
-
-A button’s level of emphasis helps determine its appearance, typography, and placement.
-
-#### Placement
-
-Use button types to express different emphasis levels for all the actions a user can perform.
-
-
-
-This screen layout uses:
-
-1. A primary button for high emphasis.
-2. A default button for medium emphasis.
-3. A link button for low emphasis.
-
-Placement best practices:
-
-- **Do**: When using multiple buttons in a row, show users which action is more important by placing it next to a button with a lower emphasis (e.g. a primary button next to a default button, or a default button next to a link button).
-- **Don’t**: Don’t place two primary buttons next to one another — they compete for focus. Only use one primary button per view.
-- **Don’t**: Don’t place a button below another button if there is space to place them side by side.
-- **Caution**: Avoid using too many buttons on a single page. When designing pages in the app or website, think about the most important actions for users to take. Too many calls to action can cause confusion and make users unsure what to do next — we always want users to feel confident and capable.
-
-## Development guidelines
-
-### Usage
-
-Renders a button with default style.
+Lets users take actions and make choices with a single click or tap.
```jsx
import { Button } from '@wordpress/components';
-
-const MyButton = () => Click me! ;
+const Mybutton = () => (
+
+ Click here
+
+);
```
+## Props
-### Props
+### `__next40pxDefaultSize`
-The presence of a `href` prop determines whether an `anchor` element is rendered instead of a `button`.
+Start opting into the larger default height that will become the
+default size in a future version.
-Props not included in this set will be applied to the `a` or `button` element.
+ - Type: `boolean`
+ - Required: No
+ - Default: `false`
-#### `accessibleWhenDisabled`: `boolean`
+### `accessibleWhenDisabled`
Whether to keep the button focusable when disabled.
-In most cases, it is recommended to set this to `true`. Disabling a control without maintaining focusability can cause accessibility issues, by hiding their presence from screen reader users, or by preventing focus from returning to a trigger element.
+In most cases, it is recommended to set this to `true`. Disabling a control without maintaining focusability
+can cause accessibility issues, by hiding their presence from screen reader users,
+or by preventing focus from returning to a trigger element.
-Learn more about the [focusability of disabled controls](https://www.w3.org/WAI/ARIA/apg/practices/keyboard-interface/#focusabilityofdisabledcontrols) in the WAI-ARIA Authoring Practices Guide.
+Learn more about the [focusability of disabled controls](https://www.w3.org/WAI/ARIA/apg/practices/keyboard-interface/#focusabilityofdisabledcontrols)
+in the WAI-ARIA Authoring Practices Guide.
-- Required: No
-- Default: `false`
+ - Type: `boolean`
+ - Required: No
+ - Default: `false`
-#### `children`: `ReactNode`
+### `children`
The button's children.
-- Required: No
+ - Type: `ReactNode`
+ - Required: No
-#### `className`: `string`
+### `description`
-An optional additional class name to apply to the rendered button.
+A visually hidden accessible description for the button.
-- Required: No
+ - Type: `string`
+ - Required: No
-#### `description`: `string`
+### `disabled`
-An accessible description for the button.
-
-- Required: No
-
-#### `disabled`: `boolean`
-
-Whether the button is disabled. If `true`, this will force a `button` element to be rendered, even when an `href` is given.
+Whether the button is disabled. If `true`, this will force a `button` element
+to be rendered, even when an `href` is given.
In most cases, it is recommended to also set the `accessibleWhenDisabled` prop to `true`.
-- Required: No
+ - Type: `boolean`
+ - Required: No
-#### `href`: `string`
+### `href`
If provided, renders `a` instead of `button`.
-- Required: No
+ - Type: `string`
+ - Required: Yes
-#### `icon`: `IconProps< unknown >[ 'icon' ]`
+### `icon`
-If provided, renders an [Icon](/packages/components/src/icon/README.md) component inside the button.
+If provided, renders an Icon component inside the button.
-- Required: No
+ - Type: `IconType`
+ - Required: No
-#### `iconPosition`: `'left' | 'right'`
+### `iconPosition`
-If provided with `icon`, sets the position of icon relative to the `text`. Available options are `left|right`.
+If provided with `icon`, sets the position of icon relative to the `text`.
-- Required: No
-- Default: `left`
+ - Type: `"left" | "right"`
+ - Required: No
+ - Default: `'left'`
-#### `iconSize`: `IconProps< unknown >[ 'size' ]`
+### `iconSize`
-If provided with `icon`, sets the icon size. Please refer to the [Icon](/packages/components/src/icon/README.md) component for more details regarding the default value of its `size` prop.
+If provided with `icon`, sets the icon size.
+Please refer to the Icon component for more details regarding
+the default value of its `size` prop.
-- Required: No
+ - Type: `number`
+ - Required: No
-#### `isBusy`: `boolean`
+### `isBusy`
Indicates activity while a action is being performed.
-- Required: No
+ - Type: `boolean`
+ - Required: No
-#### `isDestructive`: `boolean`
+### `isDestructive`
Renders a red text-based button style to indicate destructive behavior.
-- Required: No
-
-#### `isLink`: `boolean`
+ - Type: `boolean`
+ - Required: No
-Deprecated: Renders a button with an anchor style.
-Use `variant` prop with `link` value instead.
-
-- Required: No
-- Default: `false`
-
-#### `isPressed`: `boolean`
+### `isPressed`
Renders a pressed button style.
-If the native `aria-pressed` attribute is also set, it will take precedence.
-
-- Required: No
-
-#### `isPrimary`: `boolean`
+ - Type: `boolean`
+ - Required: No
-Deprecated: Renders a primary button style.
-Use `variant` prop with `primary` value instead.
+### `label`
-- Required: No
-- Default: `false`
+Sets the `aria-label` of the component, if none is provided.
+Sets the Tooltip content if `showTooltip` is provided.
-#### `isSecondary`: `boolean`
+ - Type: `string`
+ - Required: No
-Deprecated: Renders a default button style.
-Use `variant` prop with `secondary` value instead.
+### `shortcut`
-- Required: No
-- Default: `false`
+If provided with `showTooltip`, appends the Shortcut label to the tooltip content.
+If an object is provided, it should contain `display` and `ariaLabel` keys.
-#### `isSmall`: `boolean`
+ - Type: `string | { display: string; ariaLabel: string; }`
+ - Required: No
-Decreases the size of the button.
+### `showTooltip`
-Deprecated in favor of the `size` prop. If both props are defined, the `size` prop will take precedence.
+If provided, renders a Tooltip component for the button.
-- Required: No
+ - Type: `boolean`
+ - Required: No
-#### `isTertiary`: `boolean`
-
-Deprecated: Renders a text-based button style.
-Use `variant` prop with `tertiary` value instead.
-
-- Required: No
-- Default: `false`
-
-#### `label`: `string`
-
-Sets the `aria-label` of the component, if none is provided. Sets the Tooltip content if `showTooltip` is provided.
-
-- Required: No
-
-#### `shortcut`: `string | { display: string; ariaLabel: string; }`
-
-If provided with `showTooltip`, appends the Shortcut label to the tooltip content. If an object is provided, it should contain `display` and `ariaLabel` keys.
-
-- Required: No
-
-#### `showTooltip`: `boolean`
-
-If provided, renders a [Tooltip](/packages/components/src/tooltip/README.md) component for the button.
-
-- Required: No
-
-#### `size`: `'default'` | `'compact'` | `'small'`
+### `size`
The size of the button.
-- `'default'`: For normal text-label buttons, unless it is a toggle button.
-- `'compact'`: For toggle buttons, icon buttons, and buttons when used in context of either.
-- `'small'`: For icon buttons associated with more advanced or auxiliary features.
+- `'default'`: For normal text-label buttons, unless it is a toggle button.
+- `'compact'`: For toggle buttons, icon buttons, and buttons when used in context of either.
+- `'small'`: For icon buttons associated with more advanced or auxiliary features.
If the deprecated `isSmall` prop is also defined, this prop will take precedence.
-- Required: No
-- Default: `'default'`
-
-#### `target`: `string`
+ - Type: `"small" | "default" | "compact"`
+ - Required: No
+ - Default: `'default'`
-If provided with `href`, sets the `target` attribute to the `a`.
-
-- Required: No
-
-#### `text`: `string`
+### `text`
If provided, displays the given text inside the button. If the button contains children elements, the text is displayed before them.
-- Required: No
+ - Type: `string`
+ - Required: No
-#### `tooltipPosition`: `PopoverProps[ 'position' ]`
+### `tooltipPosition`
-If provided with`showTooltip`, sets the position of the tooltip. Please refer to the [Tooltip](/packages/components/src/tooltip/README.md) component for more details regarding the defaults.
+If provided with `showTooltip`, sets the position of the tooltip.
+Please refer to the Tooltip component for more details regarding the defaults.
-- Required: No
+ - Type: `"top" | "middle" | "bottom" | "top center" | "top left" | "top right" | "middle center" | "middle left" | "middle right" | "bottom center" | ...`
+ - Required: No
-#### `variant`: `'primary' | 'secondary' | 'tertiary' | 'link'`
+### `target`
-Specifies the button's style. The accepted values are `'primary'` (the primary button styles), `'secondary'` (the default button styles), `'tertiary'` (the text-based button styles), and `'link'` (the link button styles).
+If provided with `href`, sets the `target` attribute to the `a`.
-- Required: No
+ - Type: `string`
+ - Required: No
-#### `__next40pxDefaultSize`: `boolean`
+### `variant`
-Start opting into the larger default height that will become the default size in a future version.
+Specifies the button's style.
-- Required: No
-- Default: `false`
+The accepted values are:
-## Related components
+1. `'primary'` (the primary button styles)
+2. `'secondary'` (the default button styles)
+3. `'tertiary'` (the text-based button styles)
+4. `'link'` (the link button styles)
-- To group buttons together, use the [ButtonGroup](/packages/components/src/button-group/README.md) component.
+ - Type: `"link" | "primary" | "secondary" | "tertiary"`
+ - Required: No
diff --git a/packages/components/src/button/docs-manifest.json b/packages/components/src/button/docs-manifest.json
new file mode 100644
index 0000000000000..0fd0f84f44e10
--- /dev/null
+++ b/packages/components/src/button/docs-manifest.json
@@ -0,0 +1,5 @@
+{
+ "$schema": "../../schemas/docs-manifest.json",
+ "displayName": "Button",
+ "filePath": "./index.tsx"
+}
diff --git a/packages/components/src/button/stories/best-practices.mdx b/packages/components/src/button/stories/best-practices.mdx
new file mode 100644
index 0000000000000..66ec44e6738d5
--- /dev/null
+++ b/packages/components/src/button/stories/best-practices.mdx
@@ -0,0 +1,31 @@
+import { Meta } from '@storybook/blocks';
+
+
+
+# Button
+
+## Usage
+Buttons indicate available actions and allow user interaction within the interface. As key elements in the WordPress UI, they appear in toolbars, modals, and forms. Default buttons support most actions, while primary buttons emphasize the main action in a view. Secondary buttons pair as secondary actions next to a primary action.
+
+Each layout contains one prominently placed, high-emphasis button. If you need multiple buttons, use one primary button for the main action, secondary for the rest of the actions and tertiary sparingly when an action needs to not stand out at all.
+
+### Sizes
+
+- `'default'`: For normal text-label buttons, unless it is a toggle button.
+- `'compact'`: For toggle buttons, icon buttons, and buttons when used in context of either.
+- `'small'`: For icon buttons associated with more advanced or auxiliary features.
+
+## Best practices
+
+- Label buttons to show that a click or tap initiates an action.
+- Use established color conventions; for example, reserve red buttons for irreversible or dangerous actions.
+- Avoid crowding the screen with multiple calls to action, which confuses users.
+- Keep button locations consistent across the interface.
+
+## Content guidelines
+
+Buttons should be clear and predictable, showing users what will happen when clicked. Make labels reflect actions accurately to avoid confusion.
+
+Start button text with a strong action verb and include a noun to specify the change, except for common actions like Save, Close, Cancel, or OK.
+
+For other actions, use a `{verb}+{noun}` format for context. Keep button text brief and remove unnecessary words like "the," "an," or "a" for easy scanning.
diff --git a/packages/components/src/button/stories/index.story.tsx b/packages/components/src/button/stories/index.story.tsx
index 808914893de61..605b56686c702 100644
--- a/packages/components/src/button/stories/index.story.tsx
+++ b/packages/components/src/button/stories/index.story.tsx
@@ -65,30 +65,48 @@ Default.args = {
children: 'Code is poetry',
};
+/**
+ * Primary buttons stand out with bold color fills, making them distinct
+ * from the background. Since they naturally draw attention, each layout should contain
+ * only one primary button to guide users toward the most important action.
+ */
export const Primary = Template.bind( {} );
Primary.args = {
...Default.args,
variant: 'primary',
};
+/**
+ * Secondary buttons complement primary buttons. Use them for standard actions that may appear alongside a primary action.
+ */
export const Secondary = Template.bind( {} );
Secondary.args = {
...Default.args,
variant: 'secondary',
};
+/**
+ * Tertiary buttons have minimal emphasis. Use them sparingly to subtly highlight an action.
+ */
export const Tertiary = Template.bind( {} );
Tertiary.args = {
...Default.args,
variant: 'tertiary',
};
+/**
+ * Link buttons have low emphasis and blend into the page, making them suitable for supplementary actions,
+ * especially those involving navigation away from the current view.
+ */
export const Link = Template.bind( {} );
Link.args = {
...Default.args,
variant: 'link',
};
+/**
+ * Use this variant for irreversible actions. Apply sparingly and only for actions with significant impact.
+ */
export const IsDestructive = Template.bind( {} );
IsDestructive.args = {
...Default.args,
diff --git a/packages/components/src/button/style.scss b/packages/components/src/button/style.scss
index 61455a54e26f6..460aeaa2781cd 100644
--- a/packages/components/src/button/style.scss
+++ b/packages/components/src/button/style.scss
@@ -9,7 +9,6 @@
display: inline-flex;
text-decoration: none;
font-family: inherit;
- font-weight: normal;
font-size: $default-font-size;
margin: 0;
border: 0;
@@ -139,8 +138,10 @@
color: $components-color-accent;
background: transparent;
- &:hover:not(:disabled, [aria-disabled="true"]) {
- box-shadow: inset 0 0 0 $border-width $components-color-accent-darker-10;
+ &:hover:not(:disabled, [aria-disabled="true"], .is-pressed) {
+ box-shadow: inset 0 0 0 $border-width $components-color-accent-darker-20;
+ color: $components-color-accent-darker-20;
+ background: color-mix(in srgb, $components-color-accent 4%, transparent);
}
&:disabled:not(:focus),
@@ -164,15 +165,12 @@
background: transparent;
&:hover:not(:disabled, [aria-disabled="true"]) {
- // TODO: Prepare for theming (https://github.com/WordPress/gutenberg/pull/45466/files#r1030872724)
- /* stylelint-disable-next-line declaration-property-value-disallowed-list -- Allow tertiary buttons to use colors from the user admin color scheme. */
- background: rgba(var(--wp-admin-theme-color--rgb), 0.04);
+ background: color-mix(in srgb, $components-color-accent 4%, transparent);
+ color: $components-color-accent-darker-20;
}
&:active:not(:disabled, [aria-disabled="true"]) {
- // TODO: Prepare for theming (https://github.com/WordPress/gutenberg/pull/45466/files#r1030872724)
- /* stylelint-disable-next-line declaration-property-value-disallowed-list -- Allow tertiary buttons to use colors from the user admin color scheme. */
- background: rgba(var(--wp-admin-theme-color--rgb), 0.08);
+ background: color-mix(in srgb, $components-color-accent 8%, transparent);
}
// Pull left if the tertiary button stands alone after a description, so as to vertically align with items above.
@@ -220,7 +218,8 @@
}
}
- &.is-tertiary {
+ &.is-tertiary,
+ &.is-secondary {
&:hover:not(:disabled, [aria-disabled="true"]) {
background: rgba($alert-red, 0.04);
}
diff --git a/packages/components/src/button/types.ts b/packages/components/src/button/types.ts
index 7d67b721a5036..d730f49b1e813 100644
--- a/packages/components/src/button/types.ts
+++ b/packages/components/src/button/types.ts
@@ -111,11 +111,13 @@ type BaseButtonProps = {
tooltipPosition?: PopoverProps[ 'position' ];
/**
* Specifies the button's style.
+ *
* The accepted values are:
- * 'primary' (the primary button styles)
- * 'secondary' (the default button styles)
- * 'tertiary' (the text-based button styles)
- * 'link' (the link button styles)
+ *
+ * 1. `'primary'` (the primary button styles)
+ * 2. `'secondary'` (the default button styles)
+ * 3. `'tertiary'` (the text-based button styles)
+ * 4. `'link'` (the link button styles)
*/
variant?: 'primary' | 'secondary' | 'tertiary' | 'link';
};
diff --git a/packages/components/src/card/stories/index.story.tsx b/packages/components/src/card/stories/index.story.tsx
index 03726abbe754e..22fb749461785 100644
--- a/packages/components/src/card/stories/index.story.tsx
+++ b/packages/components/src/card/stories/index.story.tsx
@@ -26,10 +26,10 @@ const meta: Meta< typeof Card > = {
id: 'components-card',
argTypes: {
as: {
- control: { type: null },
+ control: false,
},
children: {
- control: { type: null },
+ control: false,
},
},
parameters: {
diff --git a/packages/components/src/checkbox-control/stories/index.story.tsx b/packages/components/src/checkbox-control/stories/index.story.tsx
index a68e380a8f733..a2936654c8629 100644
--- a/packages/components/src/checkbox-control/stories/index.story.tsx
+++ b/packages/components/src/checkbox-control/stories/index.story.tsx
@@ -24,7 +24,7 @@ const meta: Meta< typeof CheckboxControl > = {
action: 'onChange',
},
checked: {
- control: { type: null },
+ control: false,
},
help: { control: { type: 'text' } },
},
diff --git a/packages/components/src/circular-option-picker/circular-option-picker-actions.tsx b/packages/components/src/circular-option-picker/circular-option-picker-actions.tsx
index 4cf4d6f95da38..339c614e4ca45 100644
--- a/packages/components/src/circular-option-picker/circular-option-picker-actions.tsx
+++ b/packages/components/src/circular-option-picker/circular-option-picker-actions.tsx
@@ -47,6 +47,7 @@ export function ButtonAction( {
}: WordPressComponentProps< ButtonAsButtonProps, 'button', false > ) {
return (
= {
CircularOptionPicker.DropdownLinkAction,
},
argTypes: {
- actions: { control: { type: null } },
- options: { control: { type: null } },
+ actions: { control: false },
+ options: { control: false },
children: { control: { type: 'text' } },
},
parameters: {
diff --git a/packages/components/src/circular-option-picker/style.scss b/packages/components/src/circular-option-picker/style.scss
index 24b67eb7e7021..e47764a3a60d7 100644
--- a/packages/components/src/circular-option-picker/style.scss
+++ b/packages/components/src/circular-option-picker/style.scss
@@ -67,8 +67,8 @@ $color-palette-circle-spacing: 12px;
.components-circular-option-picker__option {
display: inline-block;
vertical-align: top;
- height: 100%;
- width: 100%;
+ height: 100% !important;
+ aspect-ratio: 1;
border: none;
border-radius: $radius-round;
background: transparent;
diff --git a/packages/components/src/clipboard-button/index.tsx b/packages/components/src/clipboard-button/index.tsx
index 0bf7d177e251e..492ab64b7290e 100644
--- a/packages/components/src/clipboard-button/index.tsx
+++ b/packages/components/src/clipboard-button/index.tsx
@@ -45,9 +45,11 @@ export default function ClipboardButton( {
} );
useEffect( () => {
- if ( timeoutIdRef.current ) {
- clearTimeout( timeoutIdRef.current );
- }
+ return () => {
+ if ( timeoutIdRef.current ) {
+ clearTimeout( timeoutIdRef.current );
+ }
+ };
}, [] );
const classes = clsx( 'components-clipboard-button', className );
diff --git a/packages/components/src/color-palette/index.tsx b/packages/components/src/color-palette/index.tsx
index a65508d8278c5..ed3e903970417 100644
--- a/packages/components/src/color-palette/index.tsx
+++ b/packages/components/src/color-palette/index.tsx
@@ -249,7 +249,11 @@ function UnforwardedColorPalette(
};
const actions = !! clearable && (
-
+
{ __( 'Clear' ) }
);
diff --git a/packages/components/src/color-palette/stories/index.story.tsx b/packages/components/src/color-palette/stories/index.story.tsx
index 5342fc5222be6..e4c4b89d52444 100644
--- a/packages/components/src/color-palette/stories/index.story.tsx
+++ b/packages/components/src/color-palette/stories/index.story.tsx
@@ -18,9 +18,9 @@ const meta: Meta< typeof ColorPalette > = {
id: 'components-colorpalette',
component: ColorPalette,
argTypes: {
- as: { control: { type: null } },
- onChange: { action: 'onChange', control: { type: null } },
- value: { control: { type: null } },
+ as: { control: false },
+ onChange: { action: 'onChange', control: false },
+ value: { control: false },
},
parameters: {
controls: { expanded: true },
diff --git a/packages/components/src/color-picker/color-copy-button.tsx b/packages/components/src/color-picker/color-copy-button.tsx
index b8a4822544322..6e49fa7ae85e7 100644
--- a/packages/components/src/color-picker/color-copy-button.tsx
+++ b/packages/components/src/color-picker/color-copy-button.tsx
@@ -9,7 +9,7 @@ import { __ } from '@wordpress/i18n';
/**
* Internal dependencies
*/
-import { CopyButton } from './styles';
+import { Button } from '../button';
import Tooltip from '../tooltip';
import type { ColorCopyButtonProps } from './types';
@@ -55,16 +55,14 @@ export const ColorCopyButton = ( props: ColorCopyButtonProps ) => {
};
}, [] );
+ const label =
+ copiedColor === color.toHex() ? __( 'Copied!' ) : __( 'Copy' );
+
return (
-
-
+
diff --git a/packages/components/src/color-picker/input-with-slider.tsx b/packages/components/src/color-picker/input-with-slider.tsx
index 5e08fa42daf80..221a9289f7450 100644
--- a/packages/components/src/color-picker/input-with-slider.tsx
+++ b/packages/components/src/color-picker/input-with-slider.tsx
@@ -31,6 +31,7 @@ export const InputWithSlider = ( {
return (
}
spinControls="none"
- size="__unstable-large"
/>
= {
title: 'Components/Selection & Input/Color/ColorPicker',
id: 'components-colorpicker',
argTypes: {
- as: { control: { type: null } },
- color: { control: { type: null } },
+ as: { control: false },
+ color: { control: false },
},
parameters: {
actions: { argTypesRegex: '^on.*' },
diff --git a/packages/components/src/color-picker/styles.ts b/packages/components/src/color-picker/styles.ts
index a78f10de2e4a3..50ce33da9f233 100644
--- a/packages/components/src/color-picker/styles.ts
+++ b/packages/components/src/color-picker/styles.ts
@@ -11,7 +11,6 @@ import InnerSelectControl from '../select-control';
import InnerRangeControl from '../range-control';
import { space } from '../utils/space';
import { boxSizingReset } from '../utils';
-import Button from '../button';
import { Flex } from '../flex';
import { HStack } from '../h-stack';
import CONFIG from '../utils/config-values';
@@ -22,7 +21,6 @@ export const NumberControlWrapper = styled( NumberControl )`
export const SelectControl = styled( InnerSelectControl )`
margin-left: ${ space( -2 ) };
- width: 5em;
`;
export const RangeControl = styled( InnerRangeControl )`
@@ -101,14 +99,3 @@ export const ColorfulWrapper = styled.div`
${ interactiveHueStyles }
`;
-
-export const CopyButton = styled( Button )`
- &&&&& {
- min-width: ${ space( 6 ) };
- padding: 0;
-
- > svg {
- margin-right: 0;
- }
- }
-`;
diff --git a/packages/components/src/combobox-control/README.md b/packages/components/src/combobox-control/README.md
index 5831c5ec2832c..4089cf9c56e9b 100644
--- a/packages/components/src/combobox-control/README.md
+++ b/packages/components/src/combobox-control/README.md
@@ -34,6 +34,7 @@ function MyComboboxControl() {
const [ filteredOptions, setFilteredOptions ] = useState( options );
return (
{};
@@ -92,6 +93,7 @@ const getIndexOfMatchingSuggestion = (
* const [ filteredOptions, setFilteredOptions ] = useState( options );
* return (
*
{ allowReset && (
-
-
-
+
) }
{ isExpanded && (
diff --git a/packages/components/src/combobox-control/stories/index.story.tsx b/packages/components/src/combobox-control/stories/index.story.tsx
index 954f0d96fb0d7..f033742a33662 100644
--- a/packages/components/src/combobox-control/stories/index.story.tsx
+++ b/packages/components/src/combobox-control/stories/index.story.tsx
@@ -38,7 +38,7 @@ const meta: Meta< typeof ComboboxControl > = {
id: 'components-comboboxcontrol',
component: ComboboxControl,
argTypes: {
- value: { control: { type: null } },
+ value: { control: false },
},
parameters: {
actions: { argTypesRegex: '^on.*' },
@@ -77,6 +77,7 @@ const Template: StoryFn< typeof ComboboxControl > = ( {
};
export const Default = Template.bind( {} );
Default.args = {
+ __next40pxDefaultSize: true,
__nextHasNoMarginBottom: true,
allowReset: false,
label: 'Select a country',
diff --git a/packages/components/src/combobox-control/style.scss b/packages/components/src/combobox-control/style.scss
index 8bd4c2fb156a9..c8fd8a168c0fb 100644
--- a/packages/components/src/combobox-control/style.scss
+++ b/packages/components/src/combobox-control/style.scss
@@ -38,9 +38,3 @@ input.components-combobox-control__input[type="text"] {
}
}
-.components-combobox-control__reset.components-button {
- display: flex;
- height: $grid-unit-20;
- min-width: $grid-unit-20;
- padding: 0;
-}
diff --git a/packages/components/src/combobox-control/test/index.tsx b/packages/components/src/combobox-control/test/index.tsx
index 639407ac998ed..8f569ed381a84 100644
--- a/packages/components/src/combobox-control/test/index.tsx
+++ b/packages/components/src/combobox-control/test/index.tsx
@@ -58,7 +58,13 @@ const getOptionSearchString = ( option: ComboboxControlOption ) =>
option.label.substring( 0, 11 );
const ComboboxControl = ( props: ComboboxControlProps ) => {
- return <_ComboboxControl { ...props } __nextHasNoMarginBottom />;
+ return (
+ <_ComboboxControl
+ { ...props }
+ __next40pxDefaultSize
+ __nextHasNoMarginBottom
+ />
+ );
};
const ControlledComboboxControl = ( {
diff --git a/packages/components/src/composite/hover.tsx b/packages/components/src/composite/hover.tsx
index 1507a1879cc19..a8a0ebfb4729f 100644
--- a/packages/components/src/composite/hover.tsx
+++ b/packages/components/src/composite/hover.tsx
@@ -26,5 +26,5 @@ export const CompositeHover = forwardRef<
// obfuscated to discourage its use outside of the component's internals.
const store = ( props.store ?? context.store ) as Ariakit.CompositeStore;
- return ;
+ return ;
} );
diff --git a/packages/components/src/composite/item.tsx b/packages/components/src/composite/item.tsx
index edbf0b92e039a..4a02f76039a5c 100644
--- a/packages/components/src/composite/item.tsx
+++ b/packages/components/src/composite/item.tsx
@@ -26,23 +26,5 @@ export const CompositeItem = forwardRef<
// obfuscated to discourage its use outside of the component's internals.
const store = ( props.store ?? context.store ) as Ariakit.CompositeStore;
- // If the active item is not connected, Composite may end up in a state
- // where none of the items are tabbable. In this case, we force all items to
- // be tabbable, so that as soon as an item received focus, it becomes active
- // and Composite goes back to working as expected.
- const tabbable = Ariakit.useStoreState( store, ( state ) => {
- return (
- state?.activeId !== null &&
- ! store?.item( state?.activeId )?.element?.isConnected
- );
- } );
-
- return (
-
- );
+ return ;
} );
diff --git a/packages/components/src/composite/stories/index.story.tsx b/packages/components/src/composite/stories/index.story.tsx
index eefcd59913475..63731a15a8c9c 100644
--- a/packages/components/src/composite/stories/index.story.tsx
+++ b/packages/components/src/composite/stories/index.story.tsx
@@ -36,9 +36,9 @@ const meta: Meta< typeof Composite > = {
'Composite.Context': Composite.Context,
},
argTypes: {
- children: { control: { type: null } },
- render: { control: { type: null } },
- setActiveId: { control: { type: null } },
+ children: { control: false },
+ render: { control: false },
+ setActiveId: { control: false },
focusLoop: {
control: 'select',
options: [ true, false, 'horizontal', 'vertical', 'both' ],
diff --git a/packages/components/src/composite/typeahead.tsx b/packages/components/src/composite/typeahead.tsx
index 519c59ea374e5..3a3c3875a3360 100644
--- a/packages/components/src/composite/typeahead.tsx
+++ b/packages/components/src/composite/typeahead.tsx
@@ -26,5 +26,7 @@ export const CompositeTypeahead = forwardRef<
// obfuscated to discourage its use outside of the component's internals.
const store = ( props.store ?? context.store ) as Ariakit.CompositeStore;
- return ;
+ return (
+
+ );
} );
diff --git a/packages/components/src/confirm-dialog/stories/index.story.tsx b/packages/components/src/confirm-dialog/stories/index.story.tsx
index 9496d85939edf..7c08d48369a2b 100644
--- a/packages/components/src/confirm-dialog/stories/index.story.tsx
+++ b/packages/components/src/confirm-dialog/stories/index.story.tsx
@@ -20,7 +20,7 @@ const meta: Meta< typeof ConfirmDialog > = {
id: 'components-experimental-confirmdialog',
argTypes: {
isOpen: {
- control: { type: null },
+ control: false,
},
},
parameters: {
diff --git a/packages/components/src/custom-gradient-picker/gradient-bar/control-points.tsx b/packages/components/src/custom-gradient-picker/gradient-bar/control-points.tsx
index 3911e21e0f934..d68ee7502e1f6 100644
--- a/packages/components/src/custom-gradient-picker/gradient-bar/control-points.tsx
+++ b/packages/components/src/custom-gradient-picker/gradient-bar/control-points.tsx
@@ -66,6 +66,7 @@ function ControlPointButton( {
aria-describedby={ descriptionId }
aria-haspopup="true"
aria-expanded={ isOpen }
+ __next40pxDefaultSize
className={ clsx(
'components-custom-gradient-picker__control-point-button',
{
@@ -349,6 +350,7 @@ function InsertPoint( {
} }
renderToggle={ ( { isOpen, onToggle } ) => (
{
diff --git a/packages/components/src/custom-gradient-picker/index.tsx b/packages/components/src/custom-gradient-picker/index.tsx
index dd0659515234a..8d53cd9f3d0ea 100644
--- a/packages/components/src/custom-gradient-picker/index.tsx
+++ b/packages/components/src/custom-gradient-picker/index.tsx
@@ -140,6 +140,7 @@ const GradientTypePicker = ( {
export function CustomGradientPicker( {
value,
onChange,
+ enableAlpha = true,
__experimentalIsRenderedInSidebar = false,
}: CustomGradientPickerProps ) {
const { gradientAST, hasGradient } = getGradientAstWithDefault( value );
@@ -167,6 +168,7 @@ export function CustomGradientPicker( {
__experimentalIsRenderedInSidebar={
__experimentalIsRenderedInSidebar
}
+ disableAlpha={ ! enableAlpha }
background={ background }
hasGradient={ hasGradient }
value={ controlPoints }
diff --git a/packages/components/src/custom-gradient-picker/style.scss b/packages/components/src/custom-gradient-picker/style.scss
index fea18f340951e..b9f2bee9dbe4e 100644
--- a/packages/components/src/custom-gradient-picker/style.scss
+++ b/packages/components/src/custom-gradient-picker/style.scss
@@ -47,7 +47,7 @@ $components-custom-gradient-picker__padding: $grid-unit-20; // 48px container, 1
// Same size as the .components-custom-gradient-picker__control-point-dropdown parent
height: inherit;
width: inherit;
- min-width: $grid-unit-20;
+ min-width: $grid-unit-20 !important;
border-radius: $radius-round;
background: $white;
diff --git a/packages/components/src/custom-gradient-picker/types.ts b/packages/components/src/custom-gradient-picker/types.ts
index f9efb90799daf..17702c74ef527 100644
--- a/packages/components/src/custom-gradient-picker/types.ts
+++ b/packages/components/src/custom-gradient-picker/types.ts
@@ -26,6 +26,12 @@ export type CustomGradientPickerProps = {
* the `currentGradient` as an argument.
*/
onChange: ( currentGradient: string ) => void;
+ /**
+ * Whether to enable alpha transparency options in the picker.
+ *
+ * @default true
+ */
+ enableAlpha?: boolean;
/**
* Whether this is rendered in the sidebar.
*
diff --git a/packages/components/src/custom-select-control-v2/custom-select.tsx b/packages/components/src/custom-select-control-v2/custom-select.tsx
index bb458abcc282f..9c3baf182a399 100644
--- a/packages/components/src/custom-select-control-v2/custom-select.tsx
+++ b/packages/components/src/custom-select-control-v2/custom-select.tsx
@@ -2,7 +2,6 @@
* External dependencies
*/
import * as Ariakit from '@ariakit/react';
-import { useStoreState } from '@ariakit/react';
/**
* WordPress dependencies
@@ -63,7 +62,7 @@ const CustomSelectButton = ( {
CustomSelectStore,
'onChange'
> ) => {
- const { value: currentValue } = useStoreState( store );
+ const { value: currentValue } = Ariakit.useStoreState( store );
const computedRenderSelectedValue = useMemo(
() => renderSelectedValue ?? defaultRenderSelectedValue,
diff --git a/packages/components/src/custom-select-control-v2/stories/index.story.tsx b/packages/components/src/custom-select-control-v2/stories/index.story.tsx
index 3595ee2e95199..b65c599ec9997 100644
--- a/packages/components/src/custom-select-control-v2/stories/index.story.tsx
+++ b/packages/components/src/custom-select-control-v2/stories/index.story.tsx
@@ -2,6 +2,7 @@
* External dependencies
*/
import type { Meta, StoryFn } from '@storybook/react';
+import { fn } from '@storybook/test';
/**
* WordPress dependencies
@@ -22,8 +23,8 @@ const meta: Meta< typeof CustomSelectControlV2 > = {
'CustomSelectControlV2.Item': CustomSelectControlV2.Item,
},
argTypes: {
- children: { control: { type: null } },
- value: { control: { type: null } },
+ children: { control: false },
+ value: { control: false },
},
tags: [ 'status-wip' ],
parameters: {
@@ -44,6 +45,9 @@ const meta: Meta< typeof CustomSelectControlV2 > = {
),
],
+ args: {
+ onChange: fn(),
+ },
};
export default meta;
diff --git a/packages/components/src/custom-select-control/README.md b/packages/components/src/custom-select-control/README.md
index a764a0df133ea..6c175b1fcc5d2 100644
--- a/packages/components/src/custom-select-control/README.md
+++ b/packages/components/src/custom-select-control/README.md
@@ -41,6 +41,7 @@ function MyCustomSelectControl() {
const [ , setFontSize ] = useState();
return (
setFontSize( selectedItem ) }
@@ -52,6 +53,7 @@ function MyControlledCustomSelectControl() {
const [ fontSize, setFontSize ] = useState( options[ 0 ] );
return (
setFontSize( selectedItem ) }
diff --git a/packages/components/src/custom-select-control/index.tsx b/packages/components/src/custom-select-control/index.tsx
index ecd9dc37a8f49..e014e4bc642ee 100644
--- a/packages/components/src/custom-select-control/index.tsx
+++ b/packages/components/src/custom-select-control/index.tsx
@@ -18,6 +18,7 @@ import CustomSelectItem from '../custom-select-control-v2/item';
import * as Styled from '../custom-select-control-v2/styles';
import type { CustomSelectProps, CustomSelectOption } from './types';
import { VisuallyHidden } from '../visually-hidden';
+import { maybeWarnDeprecated36pxSize } from '../utils/deprecated-36px-size';
function useDeprecatedProps< T extends CustomSelectOption >( {
__experimentalShowSelectedHint,
@@ -56,6 +57,7 @@ function CustomSelectControl< T extends CustomSelectOption >(
) {
const {
__next40pxDefaultSize = false,
+ __shouldNotWarnDeprecated36pxSize,
describedBy,
options,
onChange,
@@ -66,6 +68,13 @@ function CustomSelectControl< T extends CustomSelectOption >(
...restProps
} = useDeprecatedProps( props );
+ maybeWarnDeprecated36pxSize( {
+ componentName: 'CustomSelectControl',
+ __next40pxDefaultSize,
+ size,
+ __shouldNotWarnDeprecated36pxSize,
+ } );
+
const descriptionId = useInstanceId(
CustomSelectControl,
'custom-select-control__description'
@@ -140,7 +149,7 @@ function CustomSelectControl< T extends CustomSelectOption >(
);
} );
- const { value: currentValue } = store.getState();
+ const currentValue = Ariakit.useStoreState( store, 'value' );
const renderSelectedValueHint = () => {
const selectedOptionHint = options
diff --git a/packages/components/src/custom-select-control/stories/index.story.tsx b/packages/components/src/custom-select-control/stories/index.story.tsx
index 836fc540c6d1e..8c5e200f7532e 100644
--- a/packages/components/src/custom-select-control/stories/index.story.tsx
+++ b/packages/components/src/custom-select-control/stories/index.story.tsx
@@ -14,11 +14,12 @@ import { useState } from '@wordpress/element';
import CustomSelectControl from '..';
const meta: Meta< typeof CustomSelectControl > = {
- title: 'Components/CustomSelectControl',
+ title: 'Components/Selection & Input/Common/CustomSelectControl',
component: CustomSelectControl,
+ id: 'components-customselectcontrol',
argTypes: {
- onChange: { control: { type: null } },
- value: { control: { type: null } },
+ onChange: { control: false },
+ value: { control: false },
},
parameters: {
actions: { argTypesRegex: '^on.*' },
@@ -62,6 +63,7 @@ const Template: StoryFn< typeof CustomSelectControl > = ( props ) => {
export const Default = Template.bind( {} );
Default.args = {
+ __next40pxDefaultSize: true,
label: 'Label',
options: [
{
diff --git a/packages/components/src/custom-select-control/test/index.tsx b/packages/components/src/custom-select-control/test/index.tsx
index b2ac5c19c6ab3..61d212c26c619 100644
--- a/packages/components/src/custom-select-control/test/index.tsx
+++ b/packages/components/src/custom-select-control/test/index.tsx
@@ -13,7 +13,11 @@ import { useState } from '@wordpress/element';
/**
* Internal dependencies
*/
-import UncontrolledCustomSelectControl from '..';
+import _CustomSelectControl from '..';
+
+const UncontrolledCustomSelectControl = (
+ props: React.ComponentProps< typeof _CustomSelectControl >
+) => <_CustomSelectControl __next40pxDefaultSize { ...props } />;
const customClassName = 'amber-skies';
const customStyles = {
@@ -716,7 +720,7 @@ describe( 'Type checking', () => {
const onChange = (): void => {};
- {
onChange={ onChange }
/>;
- {
onChange={ onChange }
/>;
- {
onChange={ onChange }
/>;
- {
}
/>;
- {
onChange={ onChange }
/>;
- {
onChange={ onChange }
/>;
- {
onChange={ onChange }
/>;
- = {
* @default false
*/
__next40pxDefaultSize?: boolean;
+ /**
+ * Do not throw a warning for the deprecated 36px default size.
+ * For internal components of other components that already throw the warning.
+ *
+ * @ignore
+ */
+ __shouldNotWarnDeprecated36pxSize?: boolean;
};
diff --git a/packages/components/src/dashicon/types.ts b/packages/components/src/dashicon/types.ts
index eeee9c2d40a19..a4a4f5156aff5 100644
--- a/packages/components/src/dashicon/types.ts
+++ b/packages/components/src/dashicon/types.ts
@@ -219,7 +219,6 @@ export type IconKey =
| 'insert-before'
| 'insert'
| 'instagram'
- | 'keyboard-hide'
| 'laptop'
| 'layout'
| 'leftright'
@@ -266,7 +265,6 @@ export type IconKey =
| 'playlist-audio'
| 'playlist-video'
| 'plus-alt'
- | 'plus-light'
| 'plus'
| 'portfolio'
| 'post-status'
diff --git a/packages/components/src/date-time/stories/date-time.story.tsx b/packages/components/src/date-time/stories/date-time.story.tsx
index 7636e2fdc80a3..e240b9da47056 100644
--- a/packages/components/src/date-time/stories/date-time.story.tsx
+++ b/packages/components/src/date-time/stories/date-time.story.tsx
@@ -20,7 +20,7 @@ const meta: Meta< typeof DateTimePicker > = {
component: DateTimePicker,
argTypes: {
currentDate: { control: 'date' },
- onChange: { action: 'onChange', control: { type: null } },
+ onChange: { action: 'onChange', control: false },
},
parameters: {
controls: { expanded: true },
@@ -51,6 +51,9 @@ const Template: StoryFn< typeof DateTimePicker > = ( {
};
export const Default: StoryFn< typeof DateTimePicker > = Template.bind( {} );
+Default.args = {
+ currentDate: new Date(),
+};
export const WithEvents: StoryFn< typeof DateTimePicker > = Template.bind( {} );
WithEvents.args = {
diff --git a/packages/components/src/date-time/stories/date.story.tsx b/packages/components/src/date-time/stories/date.story.tsx
index 36fef0c5bfd19..d305edf7a29e1 100644
--- a/packages/components/src/date-time/stories/date.story.tsx
+++ b/packages/components/src/date-time/stories/date.story.tsx
@@ -20,7 +20,7 @@ const meta: Meta< typeof DatePicker > = {
component: DatePicker,
argTypes: {
currentDate: { control: 'date' },
- onChange: { action: 'onChange', control: { type: null } },
+ onChange: { action: 'onChange', control: false },
},
parameters: {
controls: { expanded: true },
@@ -51,6 +51,9 @@ const Template: StoryFn< typeof DatePicker > = ( {
};
export const Default: StoryFn< typeof DatePicker > = Template.bind( {} );
+Default.args = {
+ currentDate: new Date(),
+};
export const WithEvents: StoryFn< typeof DatePicker > = Template.bind( {} );
WithEvents.args = {
diff --git a/packages/components/src/date-time/stories/time.story.tsx b/packages/components/src/date-time/stories/time.story.tsx
index c19b5b4f48f5c..5497b1e84138c 100644
--- a/packages/components/src/date-time/stories/time.story.tsx
+++ b/packages/components/src/date-time/stories/time.story.tsx
@@ -21,7 +21,7 @@ const meta: Meta< typeof TimePicker > = {
subcomponents: { 'TimePicker.TimeInput': TimePicker.TimeInput },
argTypes: {
currentTime: { control: 'date' },
- onChange: { action: 'onChange', control: { type: null } },
+ onChange: { action: 'onChange', control: false },
},
parameters: {
controls: { expanded: true },
@@ -52,6 +52,9 @@ const Template: StoryFn< typeof TimePicker > = ( {
};
export const Default: StoryFn< typeof TimePicker > = Template.bind( {} );
+Default.args = {
+ currentTime: new Date(),
+};
const TimeInputTemplate: StoryFn< typeof TimePicker.TimeInput > = ( args ) => {
return ;
diff --git a/packages/components/src/dimension-control/README.md b/packages/components/src/dimension-control/README.md
index 78c1a60275c13..fd04d99ca4ca9 100644
--- a/packages/components/src/dimension-control/README.md
+++ b/packages/components/src/dimension-control/README.md
@@ -22,6 +22,7 @@ export default function MyCustomDimensionControl() {
return (
setPaddingSize( value ) }
diff --git a/packages/components/src/dimension-control/index.tsx b/packages/components/src/dimension-control/index.tsx
index 25880f9b4fdb3..ffdfaeb84ee51 100644
--- a/packages/components/src/dimension-control/index.tsx
+++ b/packages/components/src/dimension-control/index.tsx
@@ -18,6 +18,7 @@ import type { DimensionControlProps, Size } from './types';
import type { SelectControlSingleSelectionProps } from '../select-control/types';
import { ContextSystemProvider } from '../context';
import deprecated from '@wordpress/deprecated';
+import { maybeWarnDeprecated36pxSize } from '../utils/deprecated-36px-size';
const CONTEXT_VALUE = {
BaseControl: {
@@ -41,6 +42,7 @@ const CONTEXT_VALUE = {
*
* return (
* {
const theSize = findSizeBySlug( sizes, val );
@@ -105,6 +113,7 @@ export function DimensionControl( props: DimensionControlProps ) {
= {
id: 'components-dimensioncontrol',
argTypes: {
onChange: { action: 'onChange' },
- value: { control: { type: null } },
+ value: { control: false },
icon: {
control: { type: 'select' },
options: [ '-', 'desktop', 'tablet', 'mobile' ],
@@ -50,6 +50,7 @@ const Template: StoryFn< typeof DimensionControl > = ( args ) => (
export const Default = Template.bind( {} );
Default.args = {
__nextHasNoMarginBottom: true,
+ __next40pxDefaultSize: true,
label: 'Please select a size',
sizes,
};
diff --git a/packages/components/src/dimension-control/test/__snapshots__/index.test.js.snap b/packages/components/src/dimension-control/test/__snapshots__/index.test.js.snap
index bd2c26d641fe7..fd6cc2df3fcde 100644
--- a/packages/components/src/dimension-control/test/__snapshots__/index.test.js.snap
+++ b/packages/components/src/dimension-control/test/__snapshots__/index.test.js.snap
@@ -126,12 +126,12 @@ exports[`DimensionControl rendering renders with custom sizes 1`] = `
white-space: nowrap;
text-overflow: ellipsis;
font-size: 16px;
- height: 32px;
- min-height: 32px;
+ height: 40px;
+ min-height: 40px;
padding-top: 0;
padding-bottom: 0;
- padding-left: 8px;
- padding-right: 26px;
+ padding-left: 12px;
+ padding-right: 30px;
overflow: hidden;
}
@@ -157,8 +157,8 @@ exports[`DimensionControl rendering renders with custom sizes 1`] = `
}
.emotion-21 {
- -webkit-padding-end: 8px;
- padding-inline-end: 8px;
+ -webkit-padding-end: 12px;
+ padding-inline-end: 12px;
position: absolute;
pointer-events: none;
right: 0;
@@ -408,12 +408,12 @@ exports[`DimensionControl rendering renders with defaults 1`] = `
white-space: nowrap;
text-overflow: ellipsis;
font-size: 16px;
- height: 32px;
- min-height: 32px;
+ height: 40px;
+ min-height: 40px;
padding-top: 0;
padding-bottom: 0;
- padding-left: 8px;
- padding-right: 26px;
+ padding-left: 12px;
+ padding-right: 30px;
overflow: hidden;
}
@@ -439,8 +439,8 @@ exports[`DimensionControl rendering renders with defaults 1`] = `
}
.emotion-21 {
- -webkit-padding-end: 8px;
- padding-inline-end: 8px;
+ -webkit-padding-end: 12px;
+ padding-inline-end: 12px;
position: absolute;
pointer-events: none;
right: 0;
@@ -700,12 +700,12 @@ exports[`DimensionControl rendering renders with icon and custom icon label 1`]
white-space: nowrap;
text-overflow: ellipsis;
font-size: 16px;
- height: 32px;
- min-height: 32px;
+ height: 40px;
+ min-height: 40px;
padding-top: 0;
padding-bottom: 0;
- padding-left: 8px;
- padding-right: 26px;
+ padding-left: 12px;
+ padding-right: 30px;
overflow: hidden;
}
@@ -731,8 +731,8 @@ exports[`DimensionControl rendering renders with icon and custom icon label 1`]
}
.emotion-21 {
- -webkit-padding-end: 8px;
- padding-inline-end: 8px;
+ -webkit-padding-end: 12px;
+ padding-inline-end: 12px;
position: absolute;
pointer-events: none;
right: 0;
@@ -1004,12 +1004,12 @@ exports[`DimensionControl rendering renders with icon and default icon label 1`]
white-space: nowrap;
text-overflow: ellipsis;
font-size: 16px;
- height: 32px;
- min-height: 32px;
+ height: 40px;
+ min-height: 40px;
padding-top: 0;
padding-bottom: 0;
- padding-left: 8px;
- padding-right: 26px;
+ padding-left: 12px;
+ padding-right: 30px;
overflow: hidden;
}
@@ -1035,8 +1035,8 @@ exports[`DimensionControl rendering renders with icon and default icon label 1`]
}
.emotion-21 {
- -webkit-padding-end: 8px;
- padding-inline-end: 8px;
+ -webkit-padding-end: 12px;
+ padding-inline-end: 12px;
position: absolute;
pointer-events: none;
right: 0;
diff --git a/packages/components/src/dimension-control/test/index.test.js b/packages/components/src/dimension-control/test/index.test.js
index 14f1c509f70cf..8f3cb7ea944cd 100644
--- a/packages/components/src/dimension-control/test/index.test.js
+++ b/packages/components/src/dimension-control/test/index.test.js
@@ -15,7 +15,13 @@ import { plus } from '@wordpress/icons';
import { DimensionControl as _DimensionControl } from '../';
const DimensionControl = ( props ) => {
- return <_DimensionControl { ...props } __nextHasNoMarginBottom />;
+ return (
+ <_DimensionControl
+ { ...props }
+ __next40pxDefaultSize
+ __nextHasNoMarginBottom
+ />
+ );
};
describe( 'DimensionControl', () => {
diff --git a/packages/components/src/disabled/README.md b/packages/components/src/disabled/README.md
index 9b257acd0f737..e9eb6398554d9 100644
--- a/packages/components/src/disabled/README.md
+++ b/packages/components/src/disabled/README.md
@@ -13,7 +13,14 @@ import { Button, Disabled, TextControl } from '@wordpress/components';
const MyDisabled = () => {
const [ isDisabled, setIsDisabled ] = useState( true );
- let input = {} } />;
+ let input = (
+ {} }
+ />
+ );
if ( isDisabled ) {
input = { input } ;
}
@@ -38,12 +45,7 @@ A component can detect if it has been wrapped in a ` ` by accessing i
```jsx
function CustomButton( props ) {
const isDisabled = useContext( Disabled.Context );
- return (
-
- );
+ return ;
}
```
diff --git a/packages/components/src/disabled/index.tsx b/packages/components/src/disabled/index.tsx
index 32baac3411054..cc55a4d2e6d67 100644
--- a/packages/components/src/disabled/index.tsx
+++ b/packages/components/src/disabled/index.tsx
@@ -31,7 +31,14 @@ const { Consumer, Provider } = Context;
* const MyDisabled = () => {
* const [ isDisabled, setIsDisabled ] = useState( true );
*
- * let input = {} } />;
+ * let input = (
+ * {} }
+ * />
+ * );
* if ( isDisabled ) {
* input = { input } ;
* }
diff --git a/packages/components/src/disabled/stories/index.story.tsx b/packages/components/src/disabled/stories/index.story.tsx
index 59ff84dec43fc..591118681a82d 100644
--- a/packages/components/src/disabled/stories/index.story.tsx
+++ b/packages/components/src/disabled/stories/index.story.tsx
@@ -22,8 +22,8 @@ const meta: Meta< typeof Disabled > = {
id: 'components-disabled',
component: Disabled,
argTypes: {
- as: { control: { type: null } },
- children: { control: { type: null } },
+ as: { control: false },
+ children: { control: false },
},
parameters: {
controls: {
@@ -42,6 +42,7 @@ const Form = () => {
{
/>
{} }
options={ [
@@ -81,7 +83,7 @@ Default.args = {
export const ContentEditable: StoryFn< typeof Disabled > = ( args ) => {
return (
-
+
contentEditable
diff --git a/packages/components/src/divider/stories/index.story.tsx b/packages/components/src/divider/stories/index.story.tsx
index 4910c1b591c52..3f143fc523769 100644
--- a/packages/components/src/divider/stories/index.story.tsx
+++ b/packages/components/src/divider/stories/index.story.tsx
@@ -24,7 +24,7 @@ const meta: Meta< typeof Divider > = {
control: { type: 'text' },
},
wrapElement: {
- control: { type: null },
+ control: false,
},
ref: {
table: {
diff --git a/packages/components/src/draggable/stories/index.story.tsx b/packages/components/src/draggable/stories/index.story.tsx
index 6ecb54a07a3fb..537dd9b40d7f3 100644
--- a/packages/components/src/draggable/stories/index.story.tsx
+++ b/packages/components/src/draggable/stories/index.story.tsx
@@ -21,8 +21,8 @@ const meta: Meta< typeof Draggable > = {
title: 'Components/Utilities/Draggable',
id: 'components-draggable',
argTypes: {
- elementId: { control: { type: null } },
- __experimentalDragComponent: { control: { type: null } },
+ elementId: { control: false },
+ __experimentalDragComponent: { control: false },
},
parameters: {
actions: { argTypesRegex: '^on.*' },
diff --git a/packages/components/src/drop-zone/index.tsx b/packages/components/src/drop-zone/index.tsx
index b1bd0199e877d..dd8b97149a059 100644
--- a/packages/components/src/drop-zone/index.tsx
+++ b/packages/components/src/drop-zone/index.tsx
@@ -15,7 +15,7 @@ import { __experimentalUseDropZone as useDropZone } from '@wordpress/compose';
/**
* Internal dependencies
*/
-import type { DropType, DropZoneProps } from './types';
+import type { DropZoneProps } from './types';
import type { WordPressComponentProps } from '../context';
/**
@@ -47,19 +47,22 @@ export function DropZoneComponent( {
onFilesDrop,
onHTMLDrop,
onDrop,
+ isEligible = () => true,
...restProps
}: WordPressComponentProps< DropZoneProps, 'div', false > ) {
const [ isDraggingOverDocument, setIsDraggingOverDocument ] =
useState< boolean >();
const [ isDraggingOverElement, setIsDraggingOverElement ] =
useState< boolean >();
- const [ type, setType ] = useState< DropType >();
+ const [ isActive, setIsActive ] = useState< boolean >();
const ref = useDropZone( {
onDrop( event ) {
- const files = event.dataTransfer
- ? getFilesFromDataTransfer( event.dataTransfer )
- : [];
- const html = event.dataTransfer?.getData( 'text/html' );
+ if ( ! event.dataTransfer ) {
+ return;
+ }
+
+ const files = getFilesFromDataTransfer( event.dataTransfer );
+ const html = event.dataTransfer.getData( 'text/html' );
/**
* From Windows Chrome 96, the `event.dataTransfer` returns both file object and HTML.
@@ -76,32 +79,31 @@ export function DropZoneComponent( {
onDragStart( event ) {
setIsDraggingOverDocument( true );
- let _type: DropType = 'default';
+ if ( ! event.dataTransfer ) {
+ return;
+ }
/**
* From Windows Chrome 96, the `event.dataTransfer` returns both file object and HTML.
* The order of the checks is important to recognize the HTML drop.
*/
- if ( event.dataTransfer?.types.includes( 'text/html' ) ) {
- _type = 'html';
+ if ( event.dataTransfer.types.includes( 'text/html' ) ) {
+ setIsActive( !! onHTMLDrop );
} else if (
// Check for the types because sometimes the files themselves
// are only available on drop.
- event.dataTransfer?.types.includes( 'Files' ) ||
- ( event.dataTransfer
- ? getFilesFromDataTransfer( event.dataTransfer )
- : []
- ).length > 0
+ event.dataTransfer.types.includes( 'Files' ) ||
+ getFilesFromDataTransfer( event.dataTransfer ).length > 0
) {
- _type = 'file';
+ setIsActive( !! onFilesDrop );
+ } else {
+ setIsActive( !! onDrop && isEligible( event.dataTransfer ) );
}
-
- setType( _type );
},
onDragEnd() {
setIsDraggingOverElement( false );
setIsDraggingOverDocument( false );
- setType( undefined );
+ setIsActive( undefined );
},
onDragEnter() {
setIsDraggingOverElement( true );
@@ -112,14 +114,9 @@ export function DropZoneComponent( {
} );
const classes = clsx( 'components-drop-zone', className, {
- 'is-active':
- ( isDraggingOverDocument || isDraggingOverElement ) &&
- ( ( type === 'file' && onFilesDrop ) ||
- ( type === 'html' && onHTMLDrop ) ||
- ( type === 'default' && onDrop ) ),
+ 'is-active': isActive,
'is-dragging-over-document': isDraggingOverDocument,
'is-dragging-over-element': isDraggingOverElement,
- [ `is-dragging-${ type }` ]: !! type,
} );
return (
diff --git a/packages/components/src/drop-zone/types.ts b/packages/components/src/drop-zone/types.ts
index 3982889a4f3ea..503f400bc4be4 100644
--- a/packages/components/src/drop-zone/types.ts
+++ b/packages/components/src/drop-zone/types.ts
@@ -26,4 +26,9 @@ export type DropZoneProps = {
* It receives the HTML being dropped as an argument.
*/
onHTMLDrop?: ( html: string ) => void;
+ /**
+ * A function to determine if the drop zone is eligible to handle the drop
+ * data transfer items.
+ */
+ isEligible?: ( dataTransfer: DataTransfer ) => boolean;
};
diff --git a/packages/components/src/dropdown-menu/index.tsx b/packages/components/src/dropdown-menu/index.tsx
index 0e4501be4839c..195595fb9dc0d 100644
--- a/packages/components/src/dropdown-menu/index.tsx
+++ b/packages/components/src/dropdown-menu/index.tsx
@@ -164,11 +164,14 @@ function UnconnectedDropdownMenu( dropdownMenuProps: DropdownMenuProps ) {
{ controlSets?.flatMap( ( controlSet, indexOfSet ) =>
controlSet.map( ( control, indexOfControl ) => (
{
+ onClick={ (
+ event: React.MouseEvent< HTMLButtonElement >
+ ) => {
event.stopPropagation();
props.onClose();
if ( control.onClick ) {
diff --git a/packages/components/src/dropdown-menu/stories/index.story.tsx b/packages/components/src/dropdown-menu/stories/index.story.tsx
index dd4907bd0b96b..7b06ae979de84 100644
--- a/packages/components/src/dropdown-menu/stories/index.story.tsx
+++ b/packages/components/src/dropdown-menu/stories/index.story.tsx
@@ -23,8 +23,9 @@ import {
} from '@wordpress/icons';
const meta: Meta< typeof DropdownMenu > = {
- title: 'Components/DropdownMenu',
+ title: 'Components/Actions/DropdownMenu',
component: DropdownMenu,
+ id: 'components-dropdownmenu',
parameters: {
actions: { argTypesRegex: '^on.*' },
controls: { expanded: true },
@@ -36,9 +37,9 @@ const meta: Meta< typeof DropdownMenu > = {
mapping: { menu, chevronDown, more },
control: { type: 'select' },
},
- open: { control: { type: null } },
- defaultOpen: { control: { type: null } },
- onToggle: { control: { type: null } },
+ open: { control: false },
+ defaultOpen: { control: false },
+ onToggle: { control: false },
},
};
export default meta;
diff --git a/packages/components/src/dropdown-menu/style.scss b/packages/components/src/dropdown-menu/style.scss
index 1c716d80410e1..29fd6db18ba28 100644
--- a/packages/components/src/dropdown-menu/style.scss
+++ b/packages/components/src/dropdown-menu/style.scss
@@ -53,7 +53,7 @@
.components-menu-item__button,
.components-menu-item__button.components-button {
- min-height: $button-size;
+ min-height: $button-size-next-default-40px;
height: auto;
text-align: left;
padding-left: $grid-unit-10;
diff --git a/packages/components/src/dropdown/stories/index.story.tsx b/packages/components/src/dropdown/stories/index.story.tsx
index bfa51a07a9717..ff4d0101a377e 100644
--- a/packages/components/src/dropdown/stories/index.story.tsx
+++ b/packages/components/src/dropdown/stories/index.story.tsx
@@ -25,13 +25,13 @@ const meta: Meta< typeof Dropdown > = {
type: 'radio',
},
},
- position: { control: { type: null } },
- renderContent: { control: { type: null } },
- renderToggle: { control: { type: null } },
- open: { control: { type: null } },
- defaultOpen: { control: { type: null } },
- onToggle: { control: { type: null } },
- onClose: { control: { type: null } },
+ position: { control: false },
+ renderContent: { control: false },
+ renderToggle: { control: false },
+ open: { control: false },
+ defaultOpen: { control: false },
+ onToggle: { control: false },
+ onClose: { control: false },
},
parameters: {
actions: { argTypesRegex: '^on.*' },
diff --git a/packages/components/src/duotone-picker/color-list-picker/index.tsx b/packages/components/src/duotone-picker/color-list-picker/index.tsx
index bd009c5db1d7d..e3925b7d064fd 100644
--- a/packages/components/src/duotone-picker/color-list-picker/index.tsx
+++ b/packages/components/src/duotone-picker/color-list-picker/index.tsx
@@ -12,7 +12,6 @@ import Button from '../../button';
import ColorPalette from '../../color-palette';
import ColorIndicator from '../../color-indicator';
import Icon from '../../icon';
-import { HStack } from '../../h-stack';
import type { ColorListPickerProps, ColorOptionProps } from './types';
import { useInstanceId } from '@wordpress/compose';
@@ -32,23 +31,24 @@ function ColorOption( {
return (
<>
setIsOpen( ( prev ) => ! prev ) }
aria-expanded={ isOpen }
aria-controls={ contentId }
- >
-
- { value ? (
+ icon={
+ value ? (
) : (
- ) }
- { label }
-
-
+ )
+ }
+ text={ label }
+ />
onChange( undefined ) }
+ accessibleWhenDisabled
+ disabled={ ! value }
>
{ __( 'Clear' ) }
diff --git a/packages/components/src/duotone-picker/stories/duotone-picker.story.tsx b/packages/components/src/duotone-picker/stories/duotone-picker.story.tsx
index f06d0ee40a6ce..bf8439c38bb85 100644
--- a/packages/components/src/duotone-picker/stories/duotone-picker.story.tsx
+++ b/packages/components/src/duotone-picker/stories/duotone-picker.story.tsx
@@ -19,7 +19,7 @@ const meta: Meta< typeof DuotonePicker > = {
component: DuotonePicker,
argTypes: {
onChange: { action: 'onChange' },
- value: { control: { type: null } },
+ value: { control: false },
},
parameters: {
controls: { expanded: true },
diff --git a/packages/components/src/flex/stories/index.story.tsx b/packages/components/src/flex/stories/index.story.tsx
index 142f279665721..fac5b2e7c31e0 100644
--- a/packages/components/src/flex/stories/index.story.tsx
+++ b/packages/components/src/flex/stories/index.story.tsx
@@ -17,7 +17,7 @@ const meta: Meta< typeof Flex > = {
argTypes: {
align: { control: { type: 'text' } },
as: { control: { type: 'text' } },
- children: { control: { type: null } },
+ children: { control: false },
gap: { control: { type: 'text' } },
justify: { control: { type: 'text' } },
// Disabled isReversed because it's deprecated.
diff --git a/packages/components/src/font-size-picker/README.md b/packages/components/src/font-size-picker/README.md
index 5d7fe2b39a737..39d916c0c7b21 100644
--- a/packages/components/src/font-size-picker/README.md
+++ b/packages/components/src/font-size-picker/README.md
@@ -29,6 +29,7 @@ const MyFontSizePicker = () => {
return (
{
return (
{
{ __( 'Font size' ) }
@@ -205,6 +212,7 @@ const UnforwardedFontSizePicker = (
= {
title: 'Components/FontSizePicker',
component: FontSizePicker,
argTypes: {
- value: { control: { type: null } },
+ value: { control: false },
},
parameters: {
actions: { argTypesRegex: '^on.*' },
@@ -66,6 +66,7 @@ const TwoFontSizePickersWithState: StoryFn< typeof FontSizePicker > = ( {
export const Default: StoryFn< typeof FontSizePicker > =
FontSizePickerWithState.bind( {} );
Default.args = {
+ __next40pxDefaultSize: true,
disableCustomFontSizes: false,
fontSizes: [
{
diff --git a/packages/components/src/font-size-picker/test/index.tsx b/packages/components/src/font-size-picker/test/index.tsx
index e7205e57eefaa..34e8ce17c67fa 100644
--- a/packages/components/src/font-size-picker/test/index.tsx
+++ b/packages/components/src/font-size-picker/test/index.tsx
@@ -8,13 +8,17 @@ import { render } from '@ariakit/test/react';
/**
* Internal dependencies
*/
-import FontSizePicker from '../';
+import _FontSizePicker from '../';
import type { FontSize } from '../types';
/**
* WordPress dependencies
*/
import { useState } from '@wordpress/element';
+const FontSizePicker = (
+ props: React.ComponentProps< typeof _FontSizePicker >
+) => <_FontSizePicker __next40pxDefaultSize { ...props } />;
+
const ControlledFontSizePicker = ( {
onChange,
...props
diff --git a/packages/components/src/form-file-upload/README.md b/packages/components/src/form-file-upload/README.md
index 4dd8affc5f54a..c6a7205815de5 100644
--- a/packages/components/src/form-file-upload/README.md
+++ b/packages/components/src/form-file-upload/README.md
@@ -1,95 +1,105 @@
# FormFileUpload
-FormFileUpload is a component that allows users to select files from their local device.
+
-## Usage
+See the WordPress Storybook for more detailed, interactive documentation.
+
+FormFileUpload allows users to select files from their local device.
```jsx
import { FormFileUpload } from '@wordpress/components';
const MyFormFileUpload = () => (
- console.log( event.currentTarget.files ) }
- >
- Upload
-
+ console.log( event.currentTarget.files ) }
+ >
+ Upload
+
);
```
-
## Props
-The component accepts the following props. Props not included in this set will be passed to the `Button` component.
+### `__next40pxDefaultSize`
+
+Start opting into the larger default height that will become the default size in a future version.
+
+ - Type: `boolean`
+ - Required: No
+ - Default: `false`
-### accept
+### `accept`
-A string passed to `input` element that tells the browser which file types can be upload to the upload by the user use. e.g: `image/*,video/*`.
-More information about this string is available in https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input/file#Unique_file_type_specifiers.
+A string passed to the `input` element that tells the browser which
+[file types](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input/file#Unique_file_type_specifiers)
+can be uploaded by the user. e.g: `image/*,video/*`.
-- Type: `String`
-- Required: No
+ - Type: `string`
+ - Required: No
-### children
+### `children`
Children are passed as children of `Button`.
-- Type: `Boolean`
-- Required: No
+ - Type: `ReactNode`
+ - Required: No
-### icon
+### `icon`
-The icon to render. Supported values are: Dashicons (specified as strings), functions, Component instances and `null`.
+The icon to render in the default button.
-- Type: `String|Function|Component|null`
-- Required: No
-- Default: `null`
+See the `Icon` component docs for more information.
-### multiple
+ - Type: `IconType`
+ - Required: No
+
+### `multiple`
Whether to allow multiple selection of files or not.
-- Type: `Boolean`
-- Required: No
-- Default: `false`
+ - Type: `boolean`
+ - Required: No
+ - Default: `false`
-### onChange
+### `onChange`
Callback function passed directly to the `input` file element.
Select files will be available in `event.currentTarget.files`.
-- Type: `Function`
-- Required: Yes
+ - Type: `ChangeEventHandler`
+ - Required: Yes
-### onClick
+### `onClick`
Callback function passed directly to the `input` file element.
-This can be useful when you want to force a `change` event to fire when the user chooses the same file again. To do this, set the target value to an empty string in the `onClick` function.
+This can be useful when you want to force a `change` event to fire when
+the user chooses the same file again. To do this, set the target value to
+an empty string in the `onClick` function.
```jsx
( event.target.value = '' ) }
- onChange={ onChange }
+ __next40pxDefaultSize
+ onClick={ ( event ) => ( event.target.value = '' ) }
+ onChange={ onChange }
>
- Upload
+ Upload
```
-- Type: `Function`
-- Required: No
-
-### render
+ - Type: `MouseEventHandler`
+ - Required: No
-Optional callback function used to render the UI. If passed, the component does not render the default UI (a button) and calls this function to render it. The function receives an object with property `openFileDialog`, a function that, when called, opens the browser native file upload modal window.
+### `render`
-- Type: `Function`
-- Required: No
+Optional callback function used to render the UI.
-### __next40pxDefaultSize
-
-Start opting into the larger default height that will become the default size in a future version.
+If passed, the component does not render the default UI (a button) and
+calls this function to render it. The function receives an object with
+property `openFileDialog`, a function that, when called, opens the browser
+native file upload modal window.
-- Type: `Boolean`
-- Required: No
-- Default: `false`
+ - Type: `(arg: { openFileDialog: () => void; }) => ReactNode`
+ - Required: No
diff --git a/packages/components/src/form-file-upload/docs-manifest.json b/packages/components/src/form-file-upload/docs-manifest.json
new file mode 100644
index 0000000000000..cb000536d7356
--- /dev/null
+++ b/packages/components/src/form-file-upload/docs-manifest.json
@@ -0,0 +1,5 @@
+{
+ "$schema": "../../schemas/docs-manifest.json",
+ "displayName": "FormFileUpload",
+ "filePath": "./index.tsx"
+}
diff --git a/packages/components/src/form-file-upload/index.tsx b/packages/components/src/form-file-upload/index.tsx
index 66f0b2ea6d648..378dc144c6fe8 100644
--- a/packages/components/src/form-file-upload/index.tsx
+++ b/packages/components/src/form-file-upload/index.tsx
@@ -9,15 +9,17 @@ import { useRef } from '@wordpress/element';
import Button from '../button';
import type { WordPressComponentProps } from '../context';
import type { FormFileUploadProps } from './types';
+import { maybeWarnDeprecated36pxSize } from '../utils/deprecated-36px-size';
/**
- * FormFileUpload is a component that allows users to select files from their local device.
+ * FormFileUpload allows users to select files from their local device.
*
* ```jsx
* import { FormFileUpload } from '@wordpress/components';
*
* const MyFormFileUpload = () => (
* console.log( event.currentTarget.files ) }
* >
@@ -40,6 +42,15 @@ export function FormFileUpload( {
ref.current?.click();
};
+ if ( ! render ) {
+ maybeWarnDeprecated36pxSize( {
+ componentName: 'FormFileUpload',
+ __next40pxDefaultSize: props.__next40pxDefaultSize,
+ // @ts-expect-error - We don't "officially" support all Button props but this likely happens.
+ size: props.size,
+ } );
+ }
+
const ui = render ? (
render( { openFileDialog } )
) : (
@@ -50,9 +61,15 @@ export function FormFileUpload( {
// @todo: Temporary fix a bug that prevents Chromium browsers from selecting ".heic" files
// from the file upload. See https://core.trac.wordpress.org/ticket/62268#comment:4.
// This can be removed once the Chromium fix is in the stable channel.
- const compatAccept = !! accept?.includes( 'image/*' )
- ? `${ accept }, image/heic, image/heif`
- : accept;
+ // Prevent Safari from adding "image/heic" and "image/heif" to the accept attribute.
+ const isSafari =
+ globalThis.window?.navigator.userAgent.includes( 'Safari' ) &&
+ ! globalThis.window?.navigator.userAgent.includes( 'Chrome' ) &&
+ ! globalThis.window?.navigator.userAgent.includes( 'Chromium' );
+ const compatAccept =
+ ! isSafari && !! accept?.includes( 'image/*' )
+ ? `${ accept }, image/heic, image/heif`
+ : accept;
return (
diff --git a/packages/components/src/form-file-upload/stories/index.story.tsx b/packages/components/src/form-file-upload/stories/index.story.tsx
index 3599ccc51c22e..cec182346c0a7 100644
--- a/packages/components/src/form-file-upload/stories/index.story.tsx
+++ b/packages/components/src/form-file-upload/stories/index.story.tsx
@@ -18,9 +18,9 @@ const meta: Meta< typeof FormFileUpload > = {
id: 'components-formfileupload',
component: FormFileUpload,
argTypes: {
- icon: { control: { type: null } },
- onChange: { action: 'onChange', control: { type: null } },
- onClick: { control: { type: null } },
+ icon: { control: false },
+ onChange: { action: 'onChange', control: false },
+ onClick: { control: false },
},
parameters: {
controls: { expanded: true },
@@ -36,6 +36,7 @@ const Template: StoryFn< typeof FormFileUpload > = ( props ) => {
export const Default = Template.bind( {} );
Default.args = {
children: 'Select file',
+ __next40pxDefaultSize: true,
};
export const RestrictFileTypes = Template.bind( {} );
diff --git a/packages/components/src/form-file-upload/test/index.tsx b/packages/components/src/form-file-upload/test/index.tsx
index 3035bcaa67064..b82dcd754bcd2 100644
--- a/packages/components/src/form-file-upload/test/index.tsx
+++ b/packages/components/src/form-file-upload/test/index.tsx
@@ -7,13 +7,17 @@ import userEvent from '@testing-library/user-event';
/**
* Internal dependencies
*/
-import FormFileUpload from '..';
+import _FormFileUpload from '..';
/**
* Browser dependencies
*/
const { File } = window;
+const FormFileUpload = (
+ props: React.ComponentProps< typeof _FormFileUpload >
+) => <_FormFileUpload __next40pxDefaultSize { ...props } />;
+
// @testing-library/user-event considers changing
to a string as a change, but it do not occur on real browsers, so the comparisons will be against this result
const fakePath = expect.objectContaining( {
target: expect.objectContaining( {
diff --git a/packages/components/src/form-file-upload/types.ts b/packages/components/src/form-file-upload/types.ts
index 728ed959aba76..3bdbbf5ac2d4c 100644
--- a/packages/components/src/form-file-upload/types.ts
+++ b/packages/components/src/form-file-upload/types.ts
@@ -17,10 +17,9 @@ export type FormFileUploadProps = {
*/
__next40pxDefaultSize?: boolean;
/**
- * A string passed to `input` element that tells the browser which file types can be
- * upload to the upload by the user use. e.g: `image/*,video/*`.
- *
- * @see https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input/file#Unique_file_type_specifiers.
+ * A string passed to the `input` element that tells the browser which
+ * [file types](https://developer.mozilla.org/en-US/docs/Web/HTML/Element/input/file#Unique_file_type_specifiers)
+ * can be uploaded by the user. e.g: `image/*,video/*`.
*/
accept?: InputHTMLAttributes< HTMLInputElement >[ 'accept' ];
/**
@@ -28,7 +27,9 @@ export type FormFileUploadProps = {
*/
children?: ReactNode;
/**
- * The icon to render in the `Button`.
+ * The icon to render in the default button.
+ *
+ * See the `Icon` component docs for more information.
*/
icon?: ComponentProps< typeof Icon >[ 'icon' ];
/**
@@ -50,10 +51,11 @@ export type FormFileUploadProps = {
*
* ```jsx
*
( event.target.value = '' ) }
- * onChange={ onChange }
+ * __next40pxDefaultSize
+ * onClick={ ( event ) => ( event.target.value = '' ) }
+ * onChange={ onChange }
* >
- * Upload
+ * Upload
*
* ```
*/
diff --git a/packages/components/src/form-token-field/README.md b/packages/components/src/form-token-field/README.md
index 70e9bd09a61a3..a04ba5ec7b9d2 100644
--- a/packages/components/src/form-token-field/README.md
+++ b/packages/components/src/form-token-field/README.md
@@ -85,6 +85,7 @@ const MyFormTokenField = () => {
return (
setSelectedContinents( tokens ) }
diff --git a/packages/components/src/form-token-field/index.tsx b/packages/components/src/form-token-field/index.tsx
index 4f2f325e409a7..987c75d769b72 100644
--- a/packages/components/src/form-token-field/index.tsx
+++ b/packages/components/src/form-token-field/index.tsx
@@ -30,6 +30,7 @@ import {
import { Spacer } from '../spacer';
import { useDeprecated36pxDefaultSizeProp } from '../utils/use-deprecated-props';
import { withIgnoreIMEEvents } from '../utils/with-ignore-ime-events';
+import { maybeWarnDeprecated36pxSize } from '../utils/deprecated-36px-size';
const identity = ( value: string ) => value;
@@ -86,6 +87,12 @@ export function FormTokenField( props: FormTokenFieldProps ) {
} );
}
+ maybeWarnDeprecated36pxSize( {
+ componentName: 'FormTokenField',
+ size: undefined,
+ __next40pxDefaultSize,
+ } );
+
const instanceId = useInstanceId( FormTokenField );
// We reset to these initial values again in the onBlur
diff --git a/packages/components/src/form-token-field/stories/index.story.tsx b/packages/components/src/form-token-field/stories/index.story.tsx
index 729120ad45655..52daabe5608b0 100644
--- a/packages/components/src/form-token-field/stories/index.story.tsx
+++ b/packages/components/src/form-token-field/stories/index.story.tsx
@@ -19,10 +19,10 @@ const meta: Meta< typeof FormTokenField > = {
id: 'components-formtokenfield',
argTypes: {
value: {
- control: { type: null },
+ control: false,
},
__experimentalValidateInput: {
- control: { type: null },
+ control: false,
},
},
parameters: {
@@ -64,6 +64,7 @@ Default.args = {
label: 'Type a continent',
suggestions: continents,
__nextHasNoMarginBottom: true,
+ __next40pxDefaultSize: true,
};
export const Async: StoryFn< typeof FormTokenField > = ( {
@@ -102,6 +103,7 @@ Async.args = {
label: 'Type a continent',
suggestions: continents,
__nextHasNoMarginBottom: true,
+ __next40pxDefaultSize: true,
};
export const DropdownSelector: StoryFn< typeof FormTokenField > =
diff --git a/packages/components/src/form-token-field/test/index.tsx b/packages/components/src/form-token-field/test/index.tsx
index 961214a574c90..60c17112717bd 100644
--- a/packages/components/src/form-token-field/test/index.tsx
+++ b/packages/components/src/form-token-field/test/index.tsx
@@ -21,7 +21,11 @@ import { useState } from '@wordpress/element';
/**
* Internal dependencies
*/
-import FormTokenField from '../';
+import _FormTokenField from '../';
+
+const FormTokenField = ( props: ComponentProps< typeof _FormTokenField > ) => (
+ <_FormTokenField __next40pxDefaultSize { ...props } />
+);
const FormTokenFieldWithState = ( {
onChange,
diff --git a/packages/components/src/gradient-picker/README.md b/packages/components/src/gradient-picker/README.md
index 815b3d8eb5dd7..ec0210d03c0a4 100644
--- a/packages/components/src/gradient-picker/README.md
+++ b/packages/components/src/gradient-picker/README.md
@@ -1,110 +1,156 @@
# GradientPicker
-GradientPicker is a React component that renders a color gradient picker to define a multi step gradient. There's either a _linear_ or a _radial_ type available.
+
-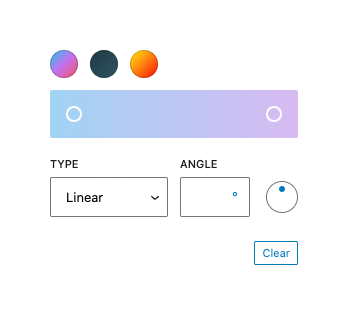
+See the WordPress Storybook for more detailed, interactive documentation.
-## Usage
-
-Render a GradientPicker.
+GradientPicker is a React component that renders a color gradient picker to
+define a multi step gradient. There's either a _linear_ or a _radial_ type
+available.
```jsx
import { useState } from 'react';
import { GradientPicker } from '@wordpress/components';
-const myGradientPicker = () => {
- const [ gradient, setGradient ] = useState( null );
-
- return (
- setGradient( currentGradient ) }
- gradients={ [
- {
- name: 'JShine',
- gradient:
- 'linear-gradient(135deg,#12c2e9 0%,#c471ed 50%,#f64f59 100%)',
- slug: 'jshine',
- },
- {
- name: 'Moonlit Asteroid',
- gradient:
- 'linear-gradient(135deg,#0F2027 0%, #203A43 0%, #2c5364 100%)',
- slug: 'moonlit-asteroid',
- },
- {
- name: 'Rastafarie',
- gradient:
- 'linear-gradient(135deg,#1E9600 0%, #FFF200 0%, #FF0000 100%)',
- slug: 'rastafari',
- },
- ] }
- />
- );
+const MyGradientPicker = () => {
+ const [ gradient, setGradient ] = useState( null );
+
+ return (
+ setGradient( currentGradient ) }
+ gradients={ [
+ {
+ name: 'JShine',
+ gradient:
+ 'linear-gradient(135deg,#12c2e9 0%,#c471ed 50%,#f64f59 100%)',
+ slug: 'jshine',
+ },
+ {
+ name: 'Moonlit Asteroid',
+ gradient:
+ 'linear-gradient(135deg,#0F2027 0%, #203A43 0%, #2c5364 100%)',
+ slug: 'moonlit-asteroid',
+ },
+ {
+ name: 'Rastafarie',
+ gradient:
+ 'linear-gradient(135deg,#1E9600 0%, #FFF200 0%, #FF0000 100%)',
+ slug: 'rastafari',
+ },
+ ] }
+ />
+ );
};
```
-
## Props
-The component accepts the following props:
+### `__experimentalIsRenderedInSidebar`
-### `className`: `string`
+Whether this is rendered in the sidebar.
-The class name added to the wrapper.
+ - Type: `boolean`
+ - Required: No
+ - Default: `false`
+
+### `asButtons`
-- Required: No
+Whether the control should present as a set of buttons,
+each with its own tab stop.
-### `value`: `string`
+ - Type: `boolean`
+ - Required: No
+ - Default: `false`
-The current value of the gradient. Pass a css gradient like `linear-gradient(90deg, rgb(6, 147, 227) 0%, rgb(155, 81, 224) 100%)`. Optionally pass in a `null` value to specify no gradient is currently selected.
+### `aria-label`
-- Required: No
-- Default: `linear-gradient(90deg, rgb(6, 147, 227) 0%, rgb(155, 81, 224) 100%)`
+A label to identify the purpose of the control.
-### `onChange`: `( currentGradient: string | undefined ) => void`
+ - Type: `string`
+ - Required: No
-The function called when a new gradient has been defined. It is passed the `currentGradient` as an argument.
+### `aria-labelledby`
-- Required: Yes
+An ID of an element to provide a label for the control.
-### `gradients`: `GradientsProp[]`
+ - Type: `string`
+ - Required: No
-An array of objects of predefined gradients displayed above the gradient selector.
+### `className`
-- Required: No
-- Default: `[]`
+The class name added to the wrapper.
+
+ - Type: `string`
+ - Required: No
-### `clearable`: `boolean`
+### `clearable`
Whether the palette should have a clearing button or not.
-- Required: No
-- Default: true
+ - Type: `boolean`
+ - Required: No
+ - Default: `true`
+
+### `disableCustomGradients`
+
+If true, the gradient picker will not be displayed and only defined
+gradients from `gradients` will be shown.
+
+ - Type: `boolean`
+ - Required: No
+ - Default: `false`
+
+### `enableAlpha`
+
+Whether to enable alpha transparency options in the picker.
+
+ - Type: `boolean`
+ - Required: No
+ - Default: `true`
+
+### `gradients`
+
+An array of objects as predefined gradients displayed above the gradient
+selector. Alternatively, if there are multiple sets (or 'origins') of
+gradients, you can pass an array of objects each with a `name` and a
+`gradients` array which will in turn contain the predefined gradient objects.
+
+ - Type: `GradientsProp`
+ - Required: No
+ - Default: `[]`
-### `disableCustomGradients`: `boolean`
+### `headingLevel`
-If true, the gradient picker will not be displayed and only defined gradients from `gradients` are available.
+The heading level. Only applies in cases where gradients are provided
+from multiple origins (i.e. when the array passed as the `gradients` prop
+contains two or more items).
-- Required: No
-- Default: false
+ - Type: `1 | 2 | 3 | 4 | 5 | 6 | "1" | "2" | "3" | "4" | ...`
+ - Required: No
+ - Default: `2`
-### `headingLevel`: `1 | 2 | 3 | 4 | 5 | 6 | '1' | '2' | '3' | '4' | '5' | '6'`
+### `loop`
-The heading level. Only applies in cases where gradients are provided from multiple origins (ie. when the array passed as the `gradients` prop contains two or more items).
+Prevents keyboard interaction from wrapping around.
+Only used when `asButtons` is not true.
-- Required: No
-- Default: `2`
+ - Type: `boolean`
+ - Required: No
+ - Default: `true`
-### `asButtons`: `boolean`
+### `onChange`
-Whether the control should present as a set of buttons, each with its own tab stop.
+The function called when a new gradient has been defined. It is passed to
+the `currentGradient` as an argument.
-- Required: No
-- Default: `false`
+ - Type: `(currentGradient: string) => void`
+ - Required: Yes
-### `loop`: `boolean`
+### `value`
-Prevents keyboard interaction from wrapping around. Only used when `asButtons` is not true.
+The current value of the gradient. Pass a css gradient string (See default value for example).
+Optionally pass in a `null` value to specify no gradient is currently selected.
-- Required: No
-- Default: `true`
+ - Type: `string`
+ - Required: No
+ - Default: `'linear-gradient(135deg,rgba(6,147,227,1) 0%,rgb(155,81,224) 100%)'`
diff --git a/packages/components/src/gradient-picker/docs-manifest.json b/packages/components/src/gradient-picker/docs-manifest.json
new file mode 100644
index 0000000000000..6bea56ccc678c
--- /dev/null
+++ b/packages/components/src/gradient-picker/docs-manifest.json
@@ -0,0 +1,5 @@
+{
+ "$schema": "../../schemas/docs-manifest.json",
+ "displayName": "GradientPicker",
+ "filePath": "./index.tsx"
+}
diff --git a/packages/components/src/gradient-picker/index.tsx b/packages/components/src/gradient-picker/index.tsx
index 8368279b8afd7..28491d8a56010 100644
--- a/packages/components/src/gradient-picker/index.tsx
+++ b/packages/components/src/gradient-picker/index.tsx
@@ -166,44 +166,44 @@ function Component( props: PickerProps< any > ) {
}
/**
- * GradientPicker is a React component that renders a color gradient picker to
+ * GradientPicker is a React component that renders a color gradient picker to
* define a multi step gradient. There's either a _linear_ or a _radial_ type
* available.
*
* ```jsx
- *import { GradientPicker } from '@wordpress/components';
- *import { useState } from '@wordpress/element';
+ * import { useState } from 'react';
+ * import { GradientPicker } from '@wordpress/components';
*
- *const myGradientPicker = () => {
- * const [ gradient, setGradient ] = useState( null );
+ * const MyGradientPicker = () => {
+ * const [ gradient, setGradient ] = useState( null );
*
- * return (
- * setGradient( currentGradient ) }
- * gradients={ [
- * {
- * name: 'JShine',
- * gradient:
- * 'linear-gradient(135deg,#12c2e9 0%,#c471ed 50%,#f64f59 100%)',
- * slug: 'jshine',
- * },
- * {
- * name: 'Moonlit Asteroid',
- * gradient:
- * 'linear-gradient(135deg,#0F2027 0%, #203A43 0%, #2c5364 100%)',
- * slug: 'moonlit-asteroid',
- * },
- * {
- * name: 'Rastafarie',
- * gradient:
- * 'linear-gradient(135deg,#1E9600 0%, #FFF200 0%, #FF0000 100%)',
- * slug: 'rastafari',
- * },
- * ] }
- * />
- * );
- *};
+ * return (
+ * setGradient( currentGradient ) }
+ * gradients={ [
+ * {
+ * name: 'JShine',
+ * gradient:
+ * 'linear-gradient(135deg,#12c2e9 0%,#c471ed 50%,#f64f59 100%)',
+ * slug: 'jshine',
+ * },
+ * {
+ * name: 'Moonlit Asteroid',
+ * gradient:
+ * 'linear-gradient(135deg,#0F2027 0%, #203A43 0%, #2c5364 100%)',
+ * slug: 'moonlit-asteroid',
+ * },
+ * {
+ * name: 'Rastafarie',
+ * gradient:
+ * 'linear-gradient(135deg,#1E9600 0%, #FFF200 0%, #FF0000 100%)',
+ * slug: 'rastafari',
+ * },
+ * ] }
+ * />
+ * );
+ * };
*```
*
*/
@@ -213,6 +213,7 @@ export function GradientPicker( {
onChange,
value,
clearable = true,
+ enableAlpha = true,
disableCustomGradients = false,
__experimentalIsRenderedInSidebar,
headingLevel = 2,
@@ -230,6 +231,7 @@ export function GradientPicker( {
__experimentalIsRenderedInSidebar={
__experimentalIsRenderedInSidebar
}
+ enableAlpha={ enableAlpha }
value={ value }
onChange={ onChange }
/>
@@ -247,6 +249,8 @@ export function GradientPicker( {
! disableCustomGradients && (
{ __( 'Clear' ) }
diff --git a/packages/components/src/gradient-picker/stories/index.story.tsx b/packages/components/src/gradient-picker/stories/index.story.tsx
index b2b73b8b60996..7dc5f62df726d 100644
--- a/packages/components/src/gradient-picker/stories/index.story.tsx
+++ b/packages/components/src/gradient-picker/stories/index.story.tsx
@@ -22,7 +22,7 @@ const meta: Meta< typeof GradientPicker > = {
actions: { argTypesRegex: '^on.*' },
},
argTypes: {
- value: { control: { type: null } },
+ value: { control: false },
},
};
export default meta;
diff --git a/packages/components/src/gradient-picker/types.ts b/packages/components/src/gradient-picker/types.ts
index b563653e33e4c..3497dd8c5ac00 100644
--- a/packages/components/src/gradient-picker/types.ts
+++ b/packages/components/src/gradient-picker/types.ts
@@ -36,7 +36,7 @@ type GradientPickerBaseProps = {
clearable?: boolean;
/**
* The heading level. Only applies in cases where gradients are provided
- * from multiple origins (ie. when the array passed as the `gradients` prop
+ * from multiple origins (i.e. when the array passed as the `gradients` prop
* contains two or more items).
*
* @default 2
@@ -56,21 +56,25 @@ type GradientPickerBaseProps = {
* @default true
*/
loop?: boolean;
+ /**
+ * Whether to enable alpha transparency options in the picker.
+ *
+ * @default true
+ */
+ enableAlpha?: boolean;
} & (
| {
+ // TODO: [#54055] Either this or `aria-labelledby` should be required
/**
* A label to identify the purpose of the control.
- *
- * @todo [#54055] Either this or `aria-labelledby` should be required
*/
'aria-label'?: string;
'aria-labelledby'?: never;
}
| {
+ // TODO: [#54055] Either this or `aria-label` should be required
/**
* An ID of an element to provide a label for the control.
- *
- * @todo [#54055] Either this or `aria-label` should be required
*/
'aria-labelledby'?: string;
'aria-label'?: never;
diff --git a/packages/components/src/grid/stories/index.story.tsx b/packages/components/src/grid/stories/index.story.tsx
index 171b324e033c0..5b2284e22d27e 100644
--- a/packages/components/src/grid/stories/index.story.tsx
+++ b/packages/components/src/grid/stories/index.story.tsx
@@ -15,7 +15,7 @@ const meta: Meta< typeof Grid > = {
argTypes: {
as: { control: { type: 'text' } },
align: { control: { type: 'text' } },
- children: { control: { type: null } },
+ children: { control: false },
columnGap: { control: { type: 'text' } },
columns: {
table: { type: { summary: 'number' } },
diff --git a/packages/components/src/h-stack/stories/index.story.tsx b/packages/components/src/h-stack/stories/index.story.tsx
index 025c3384bddce..a2e5b4fa55e9f 100644
--- a/packages/components/src/h-stack/stories/index.story.tsx
+++ b/packages/components/src/h-stack/stories/index.story.tsx
@@ -46,10 +46,10 @@ const meta: Meta< typeof HStack > = {
id: 'components-experimental-hstack',
argTypes: {
as: {
- control: { type: null },
+ control: false,
},
children: {
- control: { type: null },
+ control: false,
},
alignment: {
control: { type: 'select' },
diff --git a/packages/components/src/higher-order/with-constrained-tabbing/README.md b/packages/components/src/higher-order/with-constrained-tabbing/README.md
index 47cb8a033dfbd..417ab7c133fea 100644
--- a/packages/components/src/higher-order/with-constrained-tabbing/README.md
+++ b/packages/components/src/higher-order/with-constrained-tabbing/README.md
@@ -22,8 +22,18 @@ const MyComponentWithConstrainedTabbing = () => {
const [ isConstrainedTabbing, setIsConstrainedTabbing ] = useState( false );
let form = (
);
if ( isConstrainedTabbing ) {
@@ -43,5 +53,5 @@ const MyComponentWithConstrainedTabbing = () => {
);
-}
+};
```
diff --git a/packages/components/src/higher-order/with-focus-return/README.md b/packages/components/src/higher-order/with-focus-return/README.md
index b99d76bc6f1c9..81cecad4310f1 100644
--- a/packages/components/src/higher-order/with-focus-return/README.md
+++ b/packages/components/src/higher-order/with-focus-return/README.md
@@ -13,7 +13,12 @@ import { withFocusReturn, TextControl, Button } from '@wordpress/components';
const EnhancedComponent = withFocusReturn( () => (
Focus will return to the previous input when this component is unmounted
- {} } />
+ {} }
+ />
) );
@@ -27,6 +32,8 @@ const MyComponentWithFocusReturn = () => {
return (
setText( value ) }
@@ -39,7 +46,7 @@ const MyComponentWithFocusReturn = () => {
) }
);
-}
+};
```
`withFocusReturn` can optionally be called as a higher-order function creator. Provided an options object, a new higher-order function is returned.
diff --git a/packages/components/src/icon/README.md b/packages/components/src/icon/README.md
index 5e78f029f169f..63d52c1fd20b1 100644
--- a/packages/components/src/icon/README.md
+++ b/packages/components/src/icon/README.md
@@ -1,82 +1,39 @@
# Icon
-Allows you to render a raw icon without any initial styling or wrappers.
+
-## Usage
+See the WordPress Storybook for more detailed, interactive documentation.
-#### With a Dashicon
+Renders a raw icon without any initial styling or wrappers.
```jsx
-import { Icon } from '@wordpress/components';
+import { wordpress } from '@wordpress/icons';
-const MyIcon = () => ;
+
```
-
-#### With a function
-
-```jsx
-import { Icon } from '@wordpress/components';
-
-const MyIcon = () => (
- (
-
-
-
- ) }
- />
-);
-```
-
-#### With a Component
-
-```jsx
-import { MyIconComponent } from '../my-icon-component';
-import { Icon } from '@wordpress/components';
-
-const MyIcon = () => ;
-```
-
-#### With an SVG
-
-```jsx
-import { Icon } from '@wordpress/components';
-
-const MyIcon = () => (
-
-
-
- }
- />
-);
-```
-
-#### Specifying a className
-
-```jsx
-import { Icon } from '@wordpress/components';
-
-const MyIcon = () => ;
-```
-
## Props
-The component accepts the following props. Any additional props are passed through to the underlying icon element.
+### `icon`
-### icon
+The icon to render. In most cases, you should use an icon from
+[the `@wordpress/icons` package](https://wordpress.github.io/gutenberg/?path=/story/icons-icon--library).
-The icon to render. Supported values are: Dashicons (specified as strings), functions, Component instances and `null`.
+Other supported values are: component instances, functions,
+[Dashicons](https://developer.wordpress.org/resource/dashicons/)
+(specified as strings), and `null`.
-- Type: `String|Function|Component|null`
-- Required: No
-- Default: `null`
+The `size` value, as well as any other additional props, will be passed through.
-### size
+ - Type: `IconType`
+ - Required: No
+ - Default: `null`
+
+### `size`
The size (width and height) of the icon.
-- Type: `Number`
-- Required: No
-- Default: `20` when a Dashicon is rendered, `24` for all other icons.
+Defaults to `20` when `icon` is a string (i.e. a Dashicon id), otherwise `24`.
+
+ - Type: `number`
+ - Required: No
+ - Default: `'string' === typeof icon ? 20 : 24`
diff --git a/packages/components/src/icon/docs-manifest.json b/packages/components/src/icon/docs-manifest.json
new file mode 100644
index 0000000000000..4794049a3eb6c
--- /dev/null
+++ b/packages/components/src/icon/docs-manifest.json
@@ -0,0 +1,5 @@
+{
+ "$schema": "../../schemas/docs-manifest.json",
+ "displayName": "Icon",
+ "filePath": "./index.tsx"
+}
diff --git a/packages/components/src/icon/index.tsx b/packages/components/src/icon/index.tsx
index 3fbf4d18c5a00..283b9cd179cd1 100644
--- a/packages/components/src/icon/index.tsx
+++ b/packages/components/src/icon/index.tsx
@@ -25,10 +25,22 @@ export type IconType =
| ( ( props: { size?: number } ) => JSX.Element )
| JSX.Element;
-interface BaseProps {
+type AdditionalProps< T > = T extends ComponentType< infer U >
+ ? U
+ : T extends DashiconIconKey
+ ? SVGProps< SVGSVGElement >
+ : {};
+
+export type Props = {
/**
- * The icon to render. Supported values are: Dashicons (specified as
- * strings), functions, Component instances and `null`.
+ * The icon to render. In most cases, you should use an icon from
+ * [the `@wordpress/icons` package](https://wordpress.github.io/gutenberg/?path=/story/icons-icon--library).
+ *
+ * Other supported values are: component instances, functions,
+ * [Dashicons](https://developer.wordpress.org/resource/dashicons/)
+ * (specified as strings), and `null`.
+ *
+ * The `size` value, as well as any other additional props, will be passed through.
*
* @default null
*/
@@ -36,19 +48,22 @@ interface BaseProps {
/**
* The size (width and height) of the icon.
*
- * @default `20` when a Dashicon is rendered, `24` for all other icons.
+ * Defaults to `20` when `icon` is a string (i.e. a Dashicon id), otherwise `24`.
+ *
+ * @default `'string' === typeof icon ? 20 : 24`.
*/
size?: number;
-}
-
-type AdditionalProps< T > = T extends ComponentType< infer U >
- ? U
- : T extends DashiconIconKey
- ? SVGProps< SVGSVGElement >
- : {};
-
-export type Props = BaseProps & AdditionalProps< IconType >;
+} & AdditionalProps< IconType >;
+/**
+ * Renders a raw icon without any initial styling or wrappers.
+ *
+ * ```jsx
+ * import { wordpress } from '@wordpress/icons';
+ *
+ *
+ * ```
+ */
function Icon( {
icon = null,
size = 'string' === typeof icon ? 20 : 24,
diff --git a/packages/components/src/icon/stories/index.story.tsx b/packages/components/src/icon/stories/index.story.tsx
index 7d61be8df7f3c..d1eabf2e98b77 100644
--- a/packages/components/src/icon/stories/index.story.tsx
+++ b/packages/components/src/icon/stories/index.story.tsx
@@ -47,26 +47,68 @@ FillColor.args = {
...Default.args,
};
+/**
+ * When `icon` is a function, it will be passed the `size` prop and any other additional props.
+ */
export const WithAFunction = Template.bind( {} );
WithAFunction.args = {
...Default.args,
- icon: () => (
-
-
-
+ icon: ( { size }: { size?: number } ) => (
+
),
};
+WithAFunction.parameters = {
+ docs: {
+ source: {
+ code: `
+ (
+
+ ) }
+/>
+ `,
+ },
+ },
+};
-const MyIconComponent = () => (
-
+const MyIconComponent = ( { size }: { size?: number } ) => (
+
);
+/**
+ * When `icon` is a component, it will be passed the `size` prop and any other additional props.
+ */
export const WithAComponent = Template.bind( {} );
WithAComponent.args = {
...Default.args,
- icon: MyIconComponent,
+ icon: ,
+};
+WithAComponent.parameters = {
+ docs: {
+ source: {
+ code: `
+const MyIconComponent = ( { size } ) => (
+
+
+
+);
+
+ } />
+ `,
+ },
+ },
};
export const WithAnSVG = Template.bind( {} );
@@ -80,7 +122,7 @@ WithAnSVG.args = {
};
/**
- * Although it's preferred to use icons from the `@wordpress/icons` package, Dashicons are still supported,
+ * Although it's preferred to use icons from the `@wordpress/icons` package, [Dashicons](https://developer.wordpress.org/resource/dashicons/) are still supported,
* as long as you are in a context where the Dashicons stylesheet is loaded. To simulate that here,
* use the Global CSS Injector in the Storybook toolbar at the top and select the "WordPress" preset.
*/
diff --git a/packages/components/src/index.ts b/packages/components/src/index.ts
index e82d6da70279e..2acd609992d6a 100644
--- a/packages/components/src/index.ts
+++ b/packages/components/src/index.ts
@@ -21,11 +21,7 @@ export {
default as Animate,
getAnimateClassName as __unstableGetAnimateClassName,
} from './animate';
-export {
- __unstableMotion,
- __unstableAnimatePresence,
- __unstableMotionContext,
-} from './animation';
+export { __unstableMotion, __unstableAnimatePresence } from './animation';
export { default as AnglePickerControl } from './angle-picker-control';
export {
default as Autocomplete,
diff --git a/packages/components/src/input-control/README.md b/packages/components/src/input-control/README.md
index 58a3b4a3b1a09..ff5c70decebeb 100644
--- a/packages/components/src/input-control/README.md
+++ b/packages/components/src/input-control/README.md
@@ -17,6 +17,7 @@ const Example = () => {
return (
setValue( nextValue ?? '' ) }
/>
diff --git a/packages/components/src/input-control/index.tsx b/packages/components/src/input-control/index.tsx
index fd0fc0a5c4553..d346d1b31b111 100644
--- a/packages/components/src/input-control/index.tsx
+++ b/packages/components/src/input-control/index.tsx
@@ -20,6 +20,7 @@ import { space } from '../utils/space';
import { useDraft } from './utils';
import BaseControl from '../base-control';
import { useDeprecated36pxDefaultSizeProp } from '../utils/use-deprecated-props';
+import { maybeWarnDeprecated36pxSize } from '../utils/deprecated-36px-size';
const noop = () => {};
@@ -36,6 +37,7 @@ export function UnforwardedInputControl(
) {
const {
__next40pxDefaultSize,
+ __shouldNotWarnDeprecated36pxSize,
__unstableStateReducer: stateReducer = ( state ) => state,
__unstableInputWidth,
className,
@@ -68,6 +70,13 @@ export function UnforwardedInputControl(
const helpProp = !! help ? { 'aria-describedby': `${ id }__help` } : {};
+ maybeWarnDeprecated36pxSize( {
+ componentName: 'InputControl',
+ __next40pxDefaultSize,
+ size,
+ __shouldNotWarnDeprecated36pxSize,
+ } );
+
return (
setValue( nextValue ?? '' ) }
* />
diff --git a/packages/components/src/input-control/stories/index.story.tsx b/packages/components/src/input-control/stories/index.story.tsx
index 8cef6a5d37c81..40630938dbb37 100644
--- a/packages/components/src/input-control/stories/index.story.tsx
+++ b/packages/components/src/input-control/stories/index.story.tsx
@@ -23,10 +23,10 @@ const meta: Meta< typeof InputControl > = {
subcomponents: { InputControlPrefixWrapper, InputControlSuffixWrapper },
argTypes: {
__unstableInputWidth: { control: { type: 'text' } },
- __unstableStateReducer: { control: { type: null } },
- onChange: { control: { type: null } },
- prefix: { control: { type: null } },
- suffix: { control: { type: null } },
+ __unstableStateReducer: { control: false },
+ onChange: { control: false },
+ prefix: { control: false },
+ suffix: { control: false },
type: { control: { type: 'text' } },
value: { control: { disable: true } },
},
@@ -46,6 +46,7 @@ export const Default = Template.bind( {} );
Default.args = {
label: 'Value',
placeholder: 'Placeholder',
+ __next40pxDefaultSize: true,
};
export const WithHelpText = Template.bind( {} );
@@ -117,7 +118,6 @@ export const ShowPassword: StoryFn< typeof InputControl > = ( args ) => {
return (
= ( args ) => {
/>
);
};
+ShowPassword.args = {
+ ...Default.args,
+ label: 'Password',
+ placeholder: undefined,
+};
diff --git a/packages/components/src/input-control/test/index.js b/packages/components/src/input-control/test/index.js
index ace3086c388c8..46332eb6eea70 100644
--- a/packages/components/src/input-control/test/index.js
+++ b/packages/components/src/input-control/test/index.js
@@ -17,9 +17,15 @@ import BaseInputControl from '../';
const getInput = () => screen.getByTestId( 'input' );
describe( 'InputControl', () => {
- const InputControl = ( props ) => (
-
- );
+ const InputControl = ( props ) => {
+ return (
+
+ );
+ };
describe( 'Basic rendering', () => {
it( 'should render', () => {
diff --git a/packages/components/src/input-control/types.ts b/packages/components/src/input-control/types.ts
index 13f078cd89cc1..edb69def61905 100644
--- a/packages/components/src/input-control/types.ts
+++ b/packages/components/src/input-control/types.ts
@@ -40,6 +40,13 @@ interface BaseProps {
* @default false
*/
__next40pxDefaultSize?: boolean;
+ /**
+ * Do not throw a warning for the deprecated 36px default size.
+ * For internal components of other components that already throw the warning.
+ *
+ * @ignore
+ */
+ __shouldNotWarnDeprecated36pxSize?: boolean;
__unstableInputWidth?: CSSProperties[ 'width' ];
/**
* If true, the label will only be visible to screen readers.
@@ -129,7 +136,7 @@ export interface InputBaseProps extends BaseProps, FlexProps {
* If you want to apply standard padding in accordance with the size variant, wrap the element in
* the provided `` component.
*
- * @example
+ * ```jsx
* import {
* __experimentalInputControl as InputControl,
* __experimentalInputControlPrefixWrapper as InputControlPrefixWrapper,
@@ -138,6 +145,7 @@ export interface InputBaseProps extends BaseProps, FlexProps {
* @ }
* />
+ * ```
*/
prefix?: ReactNode;
/**
@@ -147,7 +155,7 @@ export interface InputBaseProps extends BaseProps, FlexProps {
* If you want to apply standard padding in accordance with the size variant, wrap the element in
* the provided `` component.
*
- * @example
+ * ```jsx
* import {
* __experimentalInputControl as InputControl,
* __experimentalInputControlSuffixWrapper as InputControlSuffixWrapper,
@@ -156,6 +164,7 @@ export interface InputBaseProps extends BaseProps, FlexProps {
* % }
* />
+ * ```
*/
suffix?: ReactNode;
/**
diff --git a/packages/components/src/item-group/stories/index.story.tsx b/packages/components/src/item-group/stories/index.story.tsx
index 99309b81ea314..845843d2433db 100644
--- a/packages/components/src/item-group/stories/index.story.tsx
+++ b/packages/components/src/item-group/stories/index.story.tsx
@@ -17,8 +17,8 @@ const meta: Meta< typeof ItemGroup > = {
subcomponents: { Item },
title: 'Components (Experimental)/ItemGroup',
argTypes: {
- as: { control: { type: null } },
- children: { control: { type: null } },
+ as: { control: false },
+ children: { control: false },
},
parameters: {
controls: { expanded: true },
diff --git a/packages/components/src/menu-group/stories/index.story.tsx b/packages/components/src/menu-group/stories/index.story.tsx
index 7cb9004b45a8c..c46804bc99900 100644
--- a/packages/components/src/menu-group/stories/index.story.tsx
+++ b/packages/components/src/menu-group/stories/index.story.tsx
@@ -16,10 +16,11 @@ import MenuItemsChoice from '../../menu-items-choice';
import type { Meta, StoryFn } from '@storybook/react';
const meta: Meta< typeof MenuGroup > = {
- title: 'Components/MenuGroup',
+ title: 'Components/Actions/MenuGroup',
component: MenuGroup,
+ id: 'components-menugroup',
argTypes: {
- children: { control: { type: null } },
+ children: { control: false },
},
parameters: {
controls: { expanded: true },
diff --git a/packages/components/src/menu-item/stories/index.story.tsx b/packages/components/src/menu-item/stories/index.story.tsx
index 763ee6e96be92..bef548c59d946 100644
--- a/packages/components/src/menu-item/stories/index.story.tsx
+++ b/packages/components/src/menu-item/stories/index.story.tsx
@@ -17,9 +17,10 @@ import Shortcut from '../../shortcut';
const meta: Meta< typeof MenuItem > = {
component: MenuItem,
- title: 'Components/MenuItem',
+ title: 'Components/Actions/MenuItem',
+ id: 'components-menuitem',
argTypes: {
- children: { control: { type: null } },
+ children: { control: false },
icon: {
control: { type: 'select' },
options: [ 'check', 'link', 'more' ],
diff --git a/packages/components/src/menu-items-choice/stories/index.story.tsx b/packages/components/src/menu-items-choice/stories/index.story.tsx
index 02e76158981e8..b634eb5becedb 100644
--- a/packages/components/src/menu-items-choice/stories/index.story.tsx
+++ b/packages/components/src/menu-items-choice/stories/index.story.tsx
@@ -16,11 +16,12 @@ import MenuGroup from '../../menu-group';
const meta: Meta< typeof MenuItemsChoice > = {
component: MenuItemsChoice,
- title: 'Components/MenuItemsChoice',
+ title: 'Components/Actions/MenuItemsChoice',
+ id: 'components-menuitemschoice',
argTypes: {
onHover: { action: 'onHover' },
onSelect: { action: 'onSelect' },
- value: { control: { type: null } },
+ value: { control: false },
},
parameters: {
controls: {
diff --git a/packages/components/src/menu-items-choice/style.scss b/packages/components/src/menu-items-choice/style.scss
index 383eb4066ba86..c33ce43301842 100644
--- a/packages/components/src/menu-items-choice/style.scss
+++ b/packages/components/src/menu-items-choice/style.scss
@@ -1,5 +1,6 @@
.components-menu-items-choice,
.components-menu-items-choice.components-button {
+ min-height: $button-size-next-default-40px;
height: auto;
svg {
diff --git a/packages/components/src/menu/checkbox-item.tsx b/packages/components/src/menu/checkbox-item.tsx
index b9a9b8105e517..ddb700b43324a 100644
--- a/packages/components/src/menu/checkbox-item.tsx
+++ b/packages/components/src/menu/checkbox-item.tsx
@@ -16,30 +16,32 @@ import type { WordPressComponentProps } from '../context';
import { MenuContext } from './context';
import type { MenuCheckboxItemProps } from './types';
import * as Styled from './styles';
-import { useTemporaryFocusVisibleFix } from './use-temporary-focus-visible-fix';
export const MenuCheckboxItem = forwardRef<
HTMLDivElement,
WordPressComponentProps< MenuCheckboxItemProps, 'div', false >
>( function MenuCheckboxItem(
- { suffix, children, onBlur, hideOnClick = false, ...props },
+ { suffix, children, hideOnClick = false, ...props },
ref
) {
- // TODO: Remove when https://github.com/ariakit/ariakit/issues/4083 is fixed
- const focusVisibleFixProps = useTemporaryFocusVisibleFix( { onBlur } );
const menuContext = useContext( MenuContext );
+ if ( ! menuContext?.store ) {
+ throw new Error(
+ 'Menu.CheckboxItem can only be rendered inside a Menu component'
+ );
+ }
+
return (
}
// Override some ariakit inline styles
style={ { width: 'auto', height: 'auto' } }
diff --git a/packages/components/src/menu/group-label.tsx b/packages/components/src/menu/group-label.tsx
index 71c5c7de69941..5bf081880cb1d 100644
--- a/packages/components/src/menu/group-label.tsx
+++ b/packages/components/src/menu/group-label.tsx
@@ -17,6 +17,13 @@ export const MenuGroupLabel = forwardRef<
WordPressComponentProps< MenuGroupLabelProps, 'div', false >
>( function MenuGroup( props, ref ) {
const menuContext = useContext( MenuContext );
+
+ if ( ! menuContext?.store ) {
+ throw new Error(
+ 'Menu.GroupLabel can only be rendered inside a Menu component'
+ );
+ }
+
return (
}
{ ...props }
- store={ menuContext?.store }
+ store={ menuContext.store }
/>
);
} );
diff --git a/packages/components/src/menu/group.tsx b/packages/components/src/menu/group.tsx
index f9a4138fe4358..834350955f3c5 100644
--- a/packages/components/src/menu/group.tsx
+++ b/packages/components/src/menu/group.tsx
@@ -16,11 +16,18 @@ export const MenuGroup = forwardRef<
WordPressComponentProps< MenuGroupProps, 'div', false >
>( function MenuGroup( props, ref ) {
const menuContext = useContext( MenuContext );
+
+ if ( ! menuContext?.store ) {
+ throw new Error(
+ 'Menu.Group can only be rendered inside a Menu component'
+ );
+ }
+
return (
);
} );
diff --git a/packages/components/src/menu/index.tsx b/packages/components/src/menu/index.tsx
index 6f6e89b0a1c72..2e0fc91cfbc34 100644
--- a/packages/components/src/menu/index.tsx
+++ b/packages/components/src/menu/index.tsx
@@ -2,28 +2,18 @@
* External dependencies
*/
import * as Ariakit from '@ariakit/react';
-import { useStoreState } from '@ariakit/react';
/**
* WordPress dependencies
*/
-import {
- useContext,
- useMemo,
- cloneElement,
- isValidElement,
- useCallback,
-} from '@wordpress/element';
-import { isRTL } from '@wordpress/i18n';
-import { chevronRightSmall } from '@wordpress/icons';
+import { useContext, useMemo } from '@wordpress/element';
+import { isRTL as isRTLFn } from '@wordpress/i18n';
/**
* Internal dependencies
*/
-import { useContextSystem, contextConnect } from '../context';
-import type { WordPressComponentProps } from '../context';
+import { useContextSystem, contextConnectWithoutRef } from '../context';
import type { MenuContext as MenuContextType, MenuProps } from './types';
-import * as Styled from './styles';
import { MenuContext } from './context';
import { MenuItem } from './item';
import { MenuCheckboxItem } from './checkbox-item';
@@ -33,49 +23,36 @@ import { MenuGroupLabel } from './group-label';
import { MenuSeparator } from './separator';
import { MenuItemLabel } from './item-label';
import { MenuItemHelpText } from './item-help-text';
+import { MenuTriggerButton } from './trigger-button';
+import { MenuSubmenuTriggerItem } from './submenu-trigger-item';
+import { MenuPopover } from './popover';
-const UnconnectedMenu = (
- props: WordPressComponentProps< MenuProps, 'div', false >,
- ref: React.ForwardedRef< HTMLDivElement >
-) => {
+const UnconnectedMenu = ( props: MenuProps ) => {
const {
- // Store props
- open,
+ children,
defaultOpen = false,
+ open,
onOpenChange,
placement,
- // Menu trigger props
- trigger,
-
- // Menu props
- gutter,
- children,
- shift,
- modal = true,
-
// From internal components context
variant,
-
- // Rest
- ...otherProps
- } = useContextSystem< typeof props & Pick< MenuContextType, 'variant' > >(
- props,
- 'Menu'
- );
+ } = useContextSystem<
+ // @ts-expect-error TODO: missing 'className' in MenuProps
+ typeof props & Pick< MenuContextType, 'variant' >
+ >( props, 'Menu' );
const parentContext = useContext( MenuContext );
- const computedDirection = isRTL() ? 'rtl' : 'ltr';
+ const rtl = isRTLFn();
// If an explicit value for the `placement` prop is not passed,
// apply a default placement of `bottom-start` for the root menu popover,
// and of `right-start` for nested menu popovers.
let computedPlacement =
- props.placement ??
- ( parentContext?.store ? 'right-start' : 'bottom-start' );
+ placement ?? ( parentContext?.store ? 'right-start' : 'bottom-start' );
// Swap left/right in case of RTL direction
- if ( computedDirection === 'rtl' ) {
+ if ( rtl ) {
if ( /right/.test( computedPlacement ) ) {
computedPlacement = computedPlacement.replace(
'right',
@@ -98,7 +75,7 @@ const UnconnectedMenu = (
setOpen( willBeOpen ) {
onOpenChange?.( willBeOpen );
},
- rtl: computedDirection === 'rtl',
+ rtl,
} );
const contextValue = useMemo(
@@ -106,134 +83,53 @@ const UnconnectedMenu = (
[ menuStore, variant ]
);
- // Extract the side from the applied placement — useful for animations.
- // Using `currentPlacement` instead of `placement` to make sure that we
- // use the final computed placement (including "flips" etc).
- const appliedPlacementSide = useStoreState(
- menuStore,
- 'currentPlacement'
- ).split( '-' )[ 0 ];
-
- if (
- menuStore.parent &&
- ! ( isValidElement( trigger ) && MenuItem === trigger.type )
- ) {
- // eslint-disable-next-line no-console
- console.warn(
- 'For nested Menus, the `trigger` should always be a `MenuItem`.'
- );
- }
-
- const hideOnEscape = useCallback(
- ( event: React.KeyboardEvent< Element > ) => {
- // Pressing Escape can cause unexpected consequences (ie. exiting
- // full screen mode on MacOs, close parent modals...).
- event.preventDefault();
- // Returning `true` causes the menu to hide.
- return true;
- },
- []
- );
-
- const wrapperProps = useMemo(
- () => ( {
- dir: computedDirection,
- style: {
- direction:
- computedDirection as React.CSSProperties[ 'direction' ],
- },
- } ),
- [ computedDirection ]
- );
-
return (
- <>
- { /* Menu trigger */ }
-
- { trigger.props.suffix }
-
- >
- ),
- } )
- : trigger
- }
- />
-
- { /* Menu popover */ }
- (
- // Two wrappers are needed for the entry animation, where the menu
- // container scales with a different factor than its contents.
- // The {...renderProps} are passed to the inner wrapper, so that the
- // menu element is the direct parent of the menu item elements.
-
-
-
- ) }
- >
-
- { children }
-
-
- >
+
+ { children }
+
);
};
-export const Menu = Object.assign( contextConnect( UnconnectedMenu, 'Menu' ), {
- Context: Object.assign( MenuContext, {
- displayName: 'Menu.Context',
- } ),
- Item: Object.assign( MenuItem, {
- displayName: 'Menu.Item',
- } ),
- RadioItem: Object.assign( MenuRadioItem, {
- displayName: 'Menu.RadioItem',
- } ),
- CheckboxItem: Object.assign( MenuCheckboxItem, {
- displayName: 'Menu.CheckboxItem',
- } ),
- Group: Object.assign( MenuGroup, {
- displayName: 'Menu.Group',
- } ),
- GroupLabel: Object.assign( MenuGroupLabel, {
- displayName: 'Menu.GroupLabel',
- } ),
- Separator: Object.assign( MenuSeparator, {
- displayName: 'Menu.Separator',
- } ),
- ItemLabel: Object.assign( MenuItemLabel, {
- displayName: 'Menu.ItemLabel',
- } ),
- ItemHelpText: Object.assign( MenuItemHelpText, {
- displayName: 'Menu.ItemHelpText',
- } ),
-} );
+export const Menu = Object.assign(
+ contextConnectWithoutRef( UnconnectedMenu, 'Menu' ),
+ {
+ Context: Object.assign( MenuContext, {
+ displayName: 'Menu.Context',
+ } ),
+ Item: Object.assign( MenuItem, {
+ displayName: 'Menu.Item',
+ } ),
+ RadioItem: Object.assign( MenuRadioItem, {
+ displayName: 'Menu.RadioItem',
+ } ),
+ CheckboxItem: Object.assign( MenuCheckboxItem, {
+ displayName: 'Menu.CheckboxItem',
+ } ),
+ Group: Object.assign( MenuGroup, {
+ displayName: 'Menu.Group',
+ } ),
+ GroupLabel: Object.assign( MenuGroupLabel, {
+ displayName: 'Menu.GroupLabel',
+ } ),
+ Separator: Object.assign( MenuSeparator, {
+ displayName: 'Menu.Separator',
+ } ),
+ ItemLabel: Object.assign( MenuItemLabel, {
+ displayName: 'Menu.ItemLabel',
+ } ),
+ ItemHelpText: Object.assign( MenuItemHelpText, {
+ displayName: 'Menu.ItemHelpText',
+ } ),
+ Popover: Object.assign( MenuPopover, {
+ displayName: 'Menu.Popover',
+ } ),
+ TriggerButton: Object.assign( MenuTriggerButton, {
+ displayName: 'Menu.TriggerButton',
+ } ),
+ SubmenuTriggerItem: Object.assign( MenuSubmenuTriggerItem, {
+ displayName: 'Menu.SubmenuTriggerItem',
+ } ),
+ }
+);
export default Menu;
diff --git a/packages/components/src/menu/item-help-text.tsx b/packages/components/src/menu/item-help-text.tsx
index 0ccc8f7461a8f..13d14c294125b 100644
--- a/packages/components/src/menu/item-help-text.tsx
+++ b/packages/components/src/menu/item-help-text.tsx
@@ -1,18 +1,27 @@
/**
* WordPress dependencies
*/
-import { forwardRef } from '@wordpress/element';
+import { forwardRef, useContext } from '@wordpress/element';
/**
* Internal dependencies
*/
import type { WordPressComponentProps } from '../context';
+import { MenuContext } from './context';
import * as Styled from './styles';
export const MenuItemHelpText = forwardRef<
HTMLSpanElement,
WordPressComponentProps< { children: React.ReactNode }, 'span', true >
>( function MenuItemHelpText( props, ref ) {
+ const menuContext = useContext( MenuContext );
+
+ if ( ! menuContext?.store ) {
+ throw new Error(
+ 'Menu.ItemHelpText can only be rendered inside a Menu component'
+ );
+ }
+
return (
);
diff --git a/packages/components/src/menu/item-label.tsx b/packages/components/src/menu/item-label.tsx
index 458f69558eafb..4f5f80e547861 100644
--- a/packages/components/src/menu/item-label.tsx
+++ b/packages/components/src/menu/item-label.tsx
@@ -1,18 +1,27 @@
/**
* WordPress dependencies
*/
-import { forwardRef } from '@wordpress/element';
+import { forwardRef, useContext } from '@wordpress/element';
/**
* Internal dependencies
*/
import type { WordPressComponentProps } from '../context';
+import { MenuContext } from './context';
import * as Styled from './styles';
export const MenuItemLabel = forwardRef<
HTMLSpanElement,
WordPressComponentProps< { children: React.ReactNode }, 'span', true >
>( function MenuItemLabel( props, ref ) {
+ const menuContext = useContext( MenuContext );
+
+ if ( ! menuContext?.store ) {
+ throw new Error(
+ 'Menu.ItemLabel can only be rendered inside a Menu component'
+ );
+ }
+
return (
);
diff --git a/packages/components/src/menu/item.tsx b/packages/components/src/menu/item.tsx
index f8ae670846f55..84ff050bcc223 100644
--- a/packages/components/src/menu/item.tsx
+++ b/packages/components/src/menu/item.tsx
@@ -10,27 +10,35 @@ import type { WordPressComponentProps } from '../context';
import type { MenuItemProps } from './types';
import * as Styled from './styles';
import { MenuContext } from './context';
-import { useTemporaryFocusVisibleFix } from './use-temporary-focus-visible-fix';
export const MenuItem = forwardRef<
HTMLDivElement,
WordPressComponentProps< MenuItemProps, 'div', false >
>( function MenuItem(
- { prefix, suffix, children, onBlur, hideOnClick = true, ...props },
+ { prefix, suffix, children, hideOnClick = true, store, ...props },
ref
) {
- // TODO: Remove when https://github.com/ariakit/ariakit/issues/4083 is fixed
- const focusVisibleFixProps = useTemporaryFocusVisibleFix( { onBlur } );
const menuContext = useContext( MenuContext );
+ if ( ! menuContext?.store ) {
+ throw new Error(
+ 'Menu.Item can only be rendered inside a Menu component'
+ );
+ }
+
+ // In most cases, the menu store will be retrieved from context (ie. the store
+ // created by the top-level menu component). But in rare cases (ie.
+ // `Menu.SubmenuTriggerItem`), the context store wouldn't be correct. This is
+ // why the component accepts a `store` prop to override the context store.
+ const computedStore = store ?? menuContext.store;
+
return (
{ prefix }
diff --git a/packages/components/src/menu/popover.tsx b/packages/components/src/menu/popover.tsx
new file mode 100644
index 0000000000000..19972a31027ce
--- /dev/null
+++ b/packages/components/src/menu/popover.tsx
@@ -0,0 +1,103 @@
+/**
+ * External dependencies
+ */
+import * as Ariakit from '@ariakit/react';
+
+/**
+ * WordPress dependencies
+ */
+import {
+ useContext,
+ useMemo,
+ forwardRef,
+ useCallback,
+} from '@wordpress/element';
+
+/**
+ * Internal dependencies
+ */
+import type { WordPressComponentProps } from '../context';
+import type { MenuPopoverProps } from './types';
+import * as Styled from './styles';
+import { MenuContext } from './context';
+
+export const MenuPopover = forwardRef<
+ HTMLDivElement,
+ WordPressComponentProps< MenuPopoverProps, 'div', false >
+>( function MenuPopover(
+ { gutter, children, shift, modal = true, ...otherProps },
+ ref
+) {
+ const menuContext = useContext( MenuContext );
+
+ // Extract the side from the applied placement — useful for animations.
+ // Using `currentPlacement` instead of `placement` to make sure that we
+ // use the final computed placement (including "flips" etc).
+ const appliedPlacementSide = Ariakit.useStoreState(
+ menuContext?.store,
+ 'currentPlacement'
+ )?.split( '-' )[ 0 ];
+
+ const hideOnEscape = useCallback(
+ ( event: React.KeyboardEvent< Element > ) => {
+ // Pressing Escape can cause unexpected consequences (ie. exiting
+ // full screen mode on MacOs, close parent modals...).
+ event.preventDefault();
+ // Returning `true` causes the menu to hide.
+ return true;
+ },
+ []
+ );
+
+ const computedDirection = Ariakit.useStoreState( menuContext?.store, 'rtl' )
+ ? 'rtl'
+ : 'ltr';
+
+ const wrapperProps = useMemo(
+ () => ( {
+ dir: computedDirection,
+ style: {
+ direction:
+ computedDirection as React.CSSProperties[ 'direction' ],
+ },
+ } ),
+ [ computedDirection ]
+ );
+
+ if ( ! menuContext?.store ) {
+ throw new Error(
+ 'Menu.Popover can only be rendered inside a Menu component'
+ );
+ }
+
+ return (
+ (
+ // Two wrappers are needed for the entry animation, where the menu
+ // container scales with a different factor than its contents.
+ // The {...renderProps} are passed to the inner wrapper, so that the
+ // menu element is the direct parent of the menu item elements.
+
+
+
+ ) }
+ >
+ { children }
+
+ );
+} );
diff --git a/packages/components/src/menu/radio-item.tsx b/packages/components/src/menu/radio-item.tsx
index 3848d2062c0c2..5534a6b7f3e10 100644
--- a/packages/components/src/menu/radio-item.tsx
+++ b/packages/components/src/menu/radio-item.tsx
@@ -17,7 +17,6 @@ import { MenuContext } from './context';
import type { MenuRadioItemProps } from './types';
import * as Styled from './styles';
import { SVG, Circle } from '@wordpress/primitives';
-import { useTemporaryFocusVisibleFix } from './use-temporary-focus-visible-fix';
const radioCheck = (
@@ -29,24 +28,27 @@ export const MenuRadioItem = forwardRef<
HTMLDivElement,
WordPressComponentProps< MenuRadioItemProps, 'div', false >
>( function MenuRadioItem(
- { suffix, children, onBlur, hideOnClick = false, ...props },
+ { suffix, children, hideOnClick = false, ...props },
ref
) {
- // TODO: Remove when https://github.com/ariakit/ariakit/issues/4083 is fixed
- const focusVisibleFixProps = useTemporaryFocusVisibleFix( { onBlur } );
const menuContext = useContext( MenuContext );
+ if ( ! menuContext?.store ) {
+ throw new Error(
+ 'Menu.RadioItem can only be rendered inside a Menu component'
+ );
+ }
+
return (
}
// Override some ariakit inline styles
style={ { width: 'auto', height: 'auto' } }
diff --git a/packages/components/src/menu/separator.tsx b/packages/components/src/menu/separator.tsx
index 5d0110016d9c4..57cff572c287a 100644
--- a/packages/components/src/menu/separator.tsx
+++ b/packages/components/src/menu/separator.tsx
@@ -16,12 +16,19 @@ export const MenuSeparator = forwardRef<
WordPressComponentProps< MenuSeparatorProps, 'hr', false >
>( function MenuSeparator( props, ref ) {
const menuContext = useContext( MenuContext );
+
+ if ( ! menuContext?.store ) {
+ throw new Error(
+ 'Menu.Separator can only be rendered inside a Menu component'
+ );
+ }
+
return (
);
} );
diff --git a/packages/components/src/menu/stories/index.story.tsx b/packages/components/src/menu/stories/index.story.tsx
index 92501c3326958..dcd890370a1e0 100644
--- a/packages/components/src/menu/stories/index.story.tsx
+++ b/packages/components/src/menu/stories/index.story.tsx
@@ -20,6 +20,7 @@ import Button from '../../button';
import Modal from '../../modal';
import { createSlotFill, Provider as SlotFillProvider } from '../../slot-fill';
import { ContextSystemProvider } from '../../context';
+import type { MenuProps } from '../types';
const meta: Meta< typeof Menu > = {
id: 'components-experimental-menu',
@@ -44,10 +45,15 @@ const meta: Meta< typeof Menu > = {
ItemLabel: Menu.ItemLabel,
// @ts-expect-error - See https://github.com/storybookjs/storybook/issues/23170
ItemHelpText: Menu.ItemHelpText,
+ // @ts-expect-error - See https://github.com/storybookjs/storybook/issues/23170
+ TriggerButton: Menu.TriggerButton,
+ // @ts-expect-error - See https://github.com/storybookjs/storybook/issues/23170
+ SubmenuTriggerItem: Menu.SubmenuTriggerItem,
+ // @ts-expect-error - See https://github.com/storybookjs/storybook/issues/23170
+ Popover: Menu.Popover,
},
argTypes: {
- children: { control: { type: null } },
- trigger: { control: { type: null } },
+ children: { control: false },
},
tags: [ 'status-private' ],
parameters: {
@@ -61,95 +67,103 @@ const meta: Meta< typeof Menu > = {
};
export default meta;
-export const Default: StoryFn< typeof Menu > = ( props ) => (
+export const Default: StoryFn< typeof Menu > = ( props: MenuProps ) => (
-
- Label
-
-
- Label
- Help text
-
-
- Label
-
- The menu item help text is automatically truncated when there
- are more than two lines of text
-
-
-
- Label
-
- This item doesn't close the menu on click
-
-
- Disabled item
-
-
- Group label
- }>
- With prefix
+ }
+ >
+ Open menu
+
+
+
+ Label
- With suffix
- }
- suffix="⌥⌘T"
- >
- Disabled with prefix and suffix
- And help text
+
+ Label
+ Help text
-
+
+ Label
+
+ The menu item help text is automatically truncated when
+ there are more than two lines of text
+
+
+
+ Label
+
+ This item doesn't close the menu on click
+
+
+ Disabled item
+
+
+ Group label
+ }>
+ With prefix
+
+ With suffix
+ }
+ suffix="⌥⌘T"
+ >
+
+ Disabled with prefix and suffix
+
+ And help text
+
+
+
);
-Default.args = {
- trigger: (
-
- Open menu
-
- ),
-};
+Default.args = {};
-export const WithSubmenu: StoryFn< typeof Menu > = ( props ) => (
+export const WithSubmenu: StoryFn< typeof Menu > = ( props: MenuProps ) => (
- Level 1 item
-
+ }
+ >
+ Open menu
+
+
+ Level 1 item
+
+
Submenu trigger item with a long label
-
- }
- >
-
- Level 2 item
-
-
- Level 2 item
-
-
+
- Submenu trigger
+ Level 2 item
- }
- >
-
- Level 3 item
-
-
- Level 3 item
-
+
+ Level 2 item
+
+
+
+ Submenu trigger
+
+
+
+ Level 3 item
+
+
+ Level 3 item
+
+
+
+
-
+
);
WithSubmenu.args = {
...Default.args,
};
-export const WithCheckboxes: StoryFn< typeof Menu > = ( props ) => {
+export const WithCheckboxes: StoryFn< typeof Menu > = ( props: MenuProps ) => {
const [ isAChecked, setAChecked ] = useState( false );
const [ isBChecked, setBChecked ] = useState( true );
const [ multipleCheckboxesValue, setMultipleCheckboxesValue ] = useState<
@@ -169,94 +183,113 @@ export const WithCheckboxes: StoryFn< typeof Menu > = ( props ) => {
return (
-
-
- Single selection, uncontrolled
-
-
- Checkbox item A
- Initially unchecked
-
-
- Checkbox item B
- Initially checked
-
-
-
-
- Single selection, controlled
- setAChecked( e.target.checked ) }
- >
- Checkbox item A
- Initially unchecked
-
- setBChecked( e.target.checked ) }
- >
- Checkbox item B
- Initially checked
-
-
-
-
-
- Multiple selection, uncontrolled
-
-
- Checkbox item A
- Initially unchecked
-
-
- Checkbox item B
- Initially checked
-
-
-
-
-
- Multiple selection, controlled
-
-
- Checkbox item A
- Initially unchecked
-
-
- Checkbox item B
- Initially checked
-
-
+ }
+ >
+ Open menu
+
+
+
+
+ Single selection, uncontrolled
+
+
+ Checkbox item A
+
+ Initially unchecked
+
+
+
+ Checkbox item B
+ Initially checked
+
+
+
+
+
+ Single selection, controlled
+
+ {
+ setAChecked( e.target.checked );
+ } }
+ >
+ Checkbox item A
+
+ Initially unchecked
+
+
+ setBChecked( e.target.checked ) }
+ >
+ Checkbox item B
+ Initially checked
+
+
+
+
+
+ Multiple selection, uncontrolled
+
+
+ Checkbox item A
+
+ Initially unchecked
+
+
+
+ Checkbox item B
+ Initially checked
+
+
+
+
+
+ Multiple selection, controlled
+
+
+ Checkbox item A
+
+ Initially unchecked
+
+
+
+ Checkbox item B
+ Initially checked
+
+
+
);
};
@@ -264,7 +297,7 @@ WithCheckboxes.args = {
...Default.args,
};
-export const WithRadios: StoryFn< typeof Menu > = ( props ) => {
+export const WithRadios: StoryFn< typeof Menu > = ( props: MenuProps ) => {
const [ radioValue, setRadioValue ] = useState( 'two' );
const onRadioChange: React.ComponentProps<
typeof Menu.RadioItem
@@ -272,43 +305,54 @@ export const WithRadios: StoryFn< typeof Menu > = ( props ) => {
return (
-
- Uncontrolled
-
- Radio item 1
- Initially unchecked
-
-
- Radio item 2
- Initially checked
-
-
-
-
- Controlled
-
- Radio item 1
- Initially unchecked
-
-
- Radio item 2
- Initially checked
-
-
+ }
+ >
+ Open menu
+
+
+
+ Uncontrolled
+
+ Radio item 1
+
+ Initially unchecked
+
+
+
+ Radio item 2
+ Initially checked
+
+
+
+
+ Controlled
+
+ Radio item 1
+
+ Initially unchecked
+
+
+
+ Radio item 2
+ Initially checked
+
+
+
);
};
@@ -323,7 +367,7 @@ const modalOnTopOfMenuPopover = css`
`;
// For more examples with `Modal`, check https://ariakit.org/examples/menu-wordpress-modal
-export const WithModals: StoryFn< typeof Menu > = ( props ) => {
+export const WithModals: StoryFn< typeof Menu > = ( props: MenuProps ) => {
const [ isOuterModalOpen, setOuterModalOpen ] = useState( false );
const [ isInnerModalOpen, setInnerModalOpen ] = useState( false );
@@ -333,29 +377,40 @@ export const WithModals: StoryFn< typeof Menu > = ( props ) => {
return (
<>
- setOuterModalOpen( true ) }
- hideOnClick={ false }
- >
- Open outer modal
-
- setInnerModalOpen( true ) }
- hideOnClick={ false }
+
+ }
>
- Open inner modal
-
- { isInnerModalOpen && (
- setInnerModalOpen( false ) }
- overlayClassName={ modalOverlayClassName }
+ Open menu
+
+
+ setOuterModalOpen( true ) }
+ hideOnClick={ false }
>
- Modal's contents
- setInnerModalOpen( false ) }>
- Close
-
-
- ) }
+ Open outer modal
+
+ setInnerModalOpen( true ) }
+ hideOnClick={ false }
+ >
+ Open inner modal
+
+ { isInnerModalOpen && (
+ setInnerModalOpen( false ) }
+ overlayClassName={ modalOverlayClassName }
+ >
+ Modal's contents
+ setInnerModalOpen( false ) }
+ >
+ Close
+
+
+ ) }
+
{ isOuterModalOpen && (
{
);
};
-export const WithSlotFill: StoryFn< typeof Menu > = ( props ) => {
+export const WithSlotFill: StoryFn< typeof Menu > = ( props: MenuProps ) => {
return (
-
- Item
-
-
+
+ }
+ >
+ Open menu
+
+
+
+ Item
+
+
+
Item from fill
-
+
+ Submenu from fill
+
+
- Submenu from fill
+
+ Submenu item from fill
+
- }
- >
-
- Submenu item from fill
-
+
@@ -461,28 +526,34 @@ const toolbarVariantContextValue = {
variant: 'toolbar',
},
};
-export const ToolbarVariant: StoryFn< typeof Menu > = ( props ) => (
+export const ToolbarVariant: StoryFn< typeof Menu > = ( props: MenuProps ) => (
// TODO: add toolbar
-
- Level 1 item
-
-
- Level 1 item
-
-
-
- Submenu trigger
-
- }
+ }
>
+ Open menu
+
+
- Level 2 item
+ Level 1 item
-
+
+ Level 1 item
+
+
+
+
+ Submenu trigger
+
+
+
+ Level 2 item
+
+
+
+
);
@@ -490,7 +561,7 @@ ToolbarVariant.args = {
...Default.args,
};
-export const InsideModal: StoryFn< typeof Menu > = ( props ) => {
+export const InsideModal: StoryFn< typeof Menu > = ( props: MenuProps ) => {
const [ isModalOpen, setModalOpen ] = useState( false );
return (
<>
@@ -502,28 +573,44 @@ export const InsideModal: StoryFn< typeof Menu > = ( props ) => {
Open modal
{ isModalOpen && (
- setModalOpen( false ) }>
+ setModalOpen( false ) }
+ title="Menu inside modal"
+ >
-
- Level 1 item
-
-
- Level 1 item
-
-
-
-
- Submenu trigger
-
-
+
}
>
+ Open menu
+
+
+
+ Level 1 item
+
- Level 2 item
+ Level 1 item
-
+
+
+
+
+ Submenu trigger
+
+
+
+
+
+ Level 2 item
+
+
+
+
+
setModalOpen( false ) }>
Close modal
diff --git a/packages/components/src/menu/styles.ts b/packages/components/src/menu/styles.ts
index 3312c8cb2de16..cda5c7321f38b 100644
--- a/packages/components/src/menu/styles.ts
+++ b/packages/components/src/menu/styles.ts
@@ -201,7 +201,7 @@ const baseItem = css`
[aria-disabled='true']
) {
background-color: ${ COLORS.theme.accent };
- color: ${ COLORS.white };
+ color: ${ COLORS.theme.accentInverted };
}
/* Keyboard focus (focus-visible) */
@@ -380,7 +380,7 @@ export const MenuItemHelpText = styled( Truncate )`
font-size: ${ font( 'helpText.fontSize' ) };
line-height: 16px;
color: ${ LIGHTER_TEXT_COLOR };
- word-break: break-all;
+ overflow-wrap: anywhere;
[data-active-item]:not( [data-focus-visible] )
*:not( ${ MenuPopoverInnerWrapper } )
diff --git a/packages/components/src/menu/submenu-trigger-item.tsx b/packages/components/src/menu/submenu-trigger-item.tsx
new file mode 100644
index 0000000000000..23932a14bdaff
--- /dev/null
+++ b/packages/components/src/menu/submenu-trigger-item.tsx
@@ -0,0 +1,61 @@
+/**
+ * External dependencies
+ */
+import * as Ariakit from '@ariakit/react';
+
+/**
+ * WordPress dependencies
+ */
+import { forwardRef, useContext } from '@wordpress/element';
+import { chevronRightSmall } from '@wordpress/icons';
+
+/**
+ * Internal dependencies
+ */
+import type { WordPressComponentProps } from '../context';
+import type { MenuItemProps } from './types';
+import { MenuContext } from './context';
+import { MenuItem } from './item';
+import * as Styled from './styles';
+
+export const MenuSubmenuTriggerItem = forwardRef<
+ HTMLDivElement,
+ WordPressComponentProps< MenuItemProps, 'div', false >
+>( function MenuSubmenuTriggerItem( { suffix, ...otherProps }, ref ) {
+ const menuContext = useContext( MenuContext );
+
+ if ( ! menuContext?.store.parent ) {
+ throw new Error(
+ 'Menu.SubmenuTriggerItem can only be rendered inside a nested Menu component'
+ );
+ }
+
+ return (
+
+ { suffix }
+
+ >
+ }
+ />
+ }
+ />
+ );
+} );
diff --git a/packages/components/src/menu/test/index.tsx b/packages/components/src/menu/test/index.tsx
index 60276cdb2379a..42e1516d94bbb 100644
--- a/packages/components/src/menu/test/index.tsx
+++ b/packages/components/src/menu/test/index.tsx
@@ -18,17 +18,28 @@ const delay = ( delayInMs: number ) => {
return new Promise( ( resolve ) => setTimeout( resolve, delayInMs ) );
};
+// Open dropdown => open menu
+// Submenu trigger item => open submenu
+
describe( 'Menu', () => {
// See https://www.w3.org/WAI/ARIA/apg/patterns/menu-button/
it( 'should follow the WAI-ARIA spec', async () => {
render(
- Open dropdown }>
- Menu item
-
- Submenu trigger item }>
- Submenu item 1
- Submenu item 2
-
+
+ Open dropdown
+
+ Menu item
+
+
+
+ Submenu trigger item
+
+
+ Submenu item 1
+ Submenu item 2
+
+
+
);
@@ -84,8 +95,11 @@ describe( 'Menu', () => {
describe( 'pointer and keyboard interactions', () => {
it( 'should open and focus the menu when clicking the trigger', async () => {
render(
- Open dropdown }>
- Menu item
+
+ Open dropdown
+
+ Menu item
+
);
@@ -105,10 +119,13 @@ describe( 'Menu', () => {
it( 'should open and focus the first item when pressing the arrow down key on the trigger', async () => {
render(
- Open dropdown }>
- First item
- Second item
- Third item
+
+ Open dropdown
+
+ First item
+ Second item
+ Third item
+
);
@@ -135,10 +152,13 @@ describe( 'Menu', () => {
it( 'should open and focus the first item when pressing the space key on the trigger', async () => {
render(
- Open dropdown }>
- First item
- Second item
- Third item
+
+ Open dropdown
+
+ First item
+ Second item
+ Third item
+
);
@@ -165,8 +185,11 @@ describe( 'Menu', () => {
it( 'should close when pressing the escape key', async () => {
render(
- Open dropdown }>
- Menu item
+
+ Open dropdown
+
+ Menu item
+
);
@@ -194,8 +217,11 @@ describe( 'Menu', () => {
it( 'should close when clicking outside of the content', async () => {
render(
- Open dropdown }>
- Menu item
+
+ Open dropdown
+
+ Menu item
+
);
@@ -209,8 +235,11 @@ describe( 'Menu', () => {
it( 'should close when clicking on a menu item', async () => {
render(
- Open dropdown }>
- Menu item
+
+ Open dropdown
+
+ Menu item
+
);
@@ -224,8 +253,11 @@ describe( 'Menu', () => {
it( 'should not close when clicking on a menu item when the `hideOnClick` prop is set to `false`', async () => {
render(
- Open dropdown }>
- Menu item
+
+ Open dropdown
+
+ Menu item
+
);
@@ -239,8 +271,11 @@ describe( 'Menu', () => {
it( 'should not close when clicking on a disabled menu item', async () => {
render(
- Open dropdown }>
- Menu item
+
+ Open dropdown
+
+ Menu item
+
);
@@ -254,16 +289,22 @@ describe( 'Menu', () => {
it( 'should reveal submenu content when hovering over the submenu trigger', async () => {
render(
- Open dropdown }>
- Menu item 1
- Menu item 2
- Submenu trigger item }
- >
- Submenu item 1
- Submenu item 2
-
- Menu item 3
+
+ Open dropdown
+
+ Menu item 1
+ Menu item 2
+
+
+ Submenu trigger item
+
+
+ Submenu item 1
+ Submenu item 2
+
+
+ Menu item 3
+
);
@@ -288,16 +329,22 @@ describe( 'Menu', () => {
it( 'should navigate menu items and subitems using the arrow, spacebar and enter keys', async () => {
render(
- Open dropdown }>
- Menu item 1
- Menu item 2
- Submenu trigger item }
- >
- Submenu item 1
- Submenu item 2
-
- Menu item 3
+
+ Open dropdown
+
+ Menu item 1
+ Menu item 2
+
+
+ Submenu trigger item
+
+
+ Submenu item 1
+ Submenu item 2
+
+
+ Menu item 3
+
);
@@ -407,25 +454,28 @@ describe( 'Menu', () => {
setRadioValue( e.target.value );
};
return (
- Open dropdown }>
-
-
- Radio item one
-
-
- Radio item two
-
-
+
+ Open dropdown
+
+
+
+ Radio item one
+
+
+ Radio item two
+
+
+
);
};
@@ -484,28 +534,31 @@ describe( 'Menu', () => {
it( 'should check radio items and keep the menu open when clicking (uncontrolled)', async () => {
const onRadioValueChangeSpy = jest.fn();
render(
- Open dropdown }>
-
-
- onRadioValueChangeSpy( e.target.value )
- }
- >
- Radio item one
-
-
- onRadioValueChangeSpy( e.target.value )
- }
- >
- Radio item two
-
-
+
+ Open dropdown
+
+
+
+ onRadioValueChangeSpy( e.target.value )
+ }
+ >
+ Radio item one
+
+
+ onRadioValueChangeSpy( e.target.value )
+ }
+ >
+ Radio item two
+
+
+
);
@@ -568,38 +621,41 @@ describe( 'Menu', () => {
useState< boolean >();
return (
- Open dropdown }>
- {
- onCheckboxValueChangeSpy(
- e.target.name,
- e.target.value,
- e.target.checked
- );
- setItemOneChecked( e.target.checked );
- } }
- >
- Checkbox item one
-
-
- {
- onCheckboxValueChangeSpy(
- e.target.name,
- e.target.value,
- e.target.checked
- );
- setItemTwoChecked( e.target.checked );
- } }
- >
- Checkbox item two
-
+
+ Open dropdown
+
+ {
+ onCheckboxValueChangeSpy(
+ e.target.name,
+ e.target.value,
+ e.target.checked
+ );
+ setItemOneChecked( e.target.checked );
+ } }
+ >
+ Checkbox item one
+
+
+ {
+ onCheckboxValueChangeSpy(
+ e.target.name,
+ e.target.value,
+ e.target.checked
+ );
+ setItemTwoChecked( e.target.checked );
+ } }
+ >
+ Checkbox item two
+
+
);
};
@@ -691,35 +747,38 @@ describe( 'Menu', () => {
const onCheckboxValueChangeSpy = jest.fn();
render(
- Open dropdown }>
- {
- onCheckboxValueChangeSpy(
- e.target.name,
- e.target.value,
- e.target.checked
- );
- } }
- >
- Checkbox item one
-
-
- {
- onCheckboxValueChangeSpy(
- e.target.name,
- e.target.value,
- e.target.checked
- );
- } }
- >
- Checkbox item two
-
+
+ Open dropdown
+
+ {
+ onCheckboxValueChangeSpy(
+ e.target.name,
+ e.target.value,
+ e.target.checked
+ );
+ } }
+ >
+ Checkbox item one
+
+
+ {
+ onCheckboxValueChangeSpy(
+ e.target.name,
+ e.target.value,
+ e.target.checked
+ );
+ } }
+ >
+ Checkbox item two
+
+
);
@@ -809,8 +868,11 @@ describe( 'Menu', () => {
it( 'should be modal by default', async () => {
render(
<>
- Open dropdown }>
- Menu item
+
+ Open dropdown
+
+ Menu item
+
Button outside of dropdown
>
@@ -836,11 +898,11 @@ describe( 'Menu', () => {
it( 'should not be modal when the `modal` prop is set to `false`', async () => {
render(
<>
- Open dropdown }
- modal={ false }
- >
- Menu item
+
+ Open dropdown
+
+ Menu item
+
Button outside of dropdown
>
@@ -873,8 +935,13 @@ describe( 'Menu', () => {
describe( 'items prefix and suffix', () => {
it( 'should display a prefix on regular items', async () => {
render(
- Open dropdown }>
- Item prefix> }>Menu item
+
+ Open dropdown
+
+ Item prefix> }>
+ Menu item
+
+
);
@@ -895,8 +962,13 @@ describe( 'Menu', () => {
it( 'should display a suffix on regular items', async () => {
render(
- Open dropdown }>
- Item suffix> }>Menu item
+
+ Open dropdown
+
+ Item suffix> }>
+ Menu item
+
+
);
@@ -917,14 +989,17 @@ describe( 'Menu', () => {
it( 'should display a suffix on radio items', async () => {
render(
- Open dropdown }>
-
- Radio item one
-
+
+ Open dropdown
+
+
+ Radio item one
+
+
);
@@ -945,14 +1020,17 @@ describe( 'Menu', () => {
it( 'should display a suffix on checkbox items', async () => {
render(
- Open dropdown }>
-
- Checkbox item one
-
+
+ Open dropdown
+
+
+ Checkbox item one
+
+
);
@@ -975,9 +1053,12 @@ describe( 'Menu', () => {
describe( 'typeahead', () => {
it( 'should highlight matching item', async () => {
render(
- Open dropdown }>
- One
- Two
+
+ Open dropdown
+
+ One
+ Two
+
);
@@ -1008,9 +1089,12 @@ describe( 'Menu', () => {
it( 'should keep previous focus when no matches are found', async () => {
render(
- Open dropdown }>
- One
- Two
+
+ Open dropdown
+
+ One
+ Two
+
);
diff --git a/packages/components/src/menu/trigger-button.tsx b/packages/components/src/menu/trigger-button.tsx
new file mode 100644
index 0000000000000..b99804efef0f1
--- /dev/null
+++ b/packages/components/src/menu/trigger-button.tsx
@@ -0,0 +1,46 @@
+/**
+ * External dependencies
+ */
+import * as Ariakit from '@ariakit/react';
+
+/**
+ * WordPress dependencies
+ */
+import { forwardRef, useContext } from '@wordpress/element';
+
+/**
+ * Internal dependencies
+ */
+import type { WordPressComponentProps } from '../context';
+import type { MenuTriggerButtonProps } from './types';
+import { MenuContext } from './context';
+
+export const MenuTriggerButton = forwardRef<
+ HTMLDivElement,
+ WordPressComponentProps< MenuTriggerButtonProps, 'button', false >
+>( function MenuTriggerButton( { children, disabled = false, ...props }, ref ) {
+ const menuContext = useContext( MenuContext );
+
+ if ( ! menuContext?.store ) {
+ throw new Error(
+ 'Menu.TriggerButton can only be rendered inside a Menu component'
+ );
+ }
+
+ if ( menuContext.store.parent ) {
+ throw new Error(
+ 'Menu.TriggerButton should not be rendered inside a nested Menu component. Use Menu.SubmenuTriggerItem instead.'
+ );
+ }
+
+ return (
+
+ { children }
+
+ );
+} );
diff --git a/packages/components/src/menu/types.ts b/packages/components/src/menu/types.ts
index 7b58cef241743..f58b5bcc89b95 100644
--- a/packages/components/src/menu/types.ts
+++ b/packages/components/src/menu/types.ts
@@ -16,10 +16,6 @@ export interface MenuContext {
}
export interface MenuProps {
- /**
- * The button triggering the menu popover.
- */
- trigger: React.ReactElement;
/**
* The contents of the menu (ie. one or more menu items).
*/
@@ -40,6 +36,19 @@ export interface MenuProps {
* Event handler called when the open state of the menu popover changes.
*/
onOpenChange?: ( open: boolean ) => void;
+ /**
+ * The placement of the menu popover.
+ *
+ * @default 'bottom-start' for root-level menus, 'right-start' for nested menus
+ */
+ placement?: Placement;
+}
+
+export interface MenuPopoverProps {
+ /**
+ * The contents of the dropdown.
+ */
+ children?: React.ReactNode;
/**
* The modality of the menu popover. When set to true, interaction with
* outside elements will be disabled and only menu content will be visible to
@@ -48,12 +57,6 @@ export interface MenuProps {
* @default true
*/
modal?: boolean;
- /**
- * The placement of the menu popover.
- *
- * @default 'bottom-start' for root-level menus, 'right-start' for nested menus
- */
- placement?: Placement;
/**
* The distance between the popover and the anchor element.
*
@@ -80,6 +83,50 @@ export interface MenuProps {
) => boolean );
}
+export interface MenuTriggerButtonProps {
+ /**
+ * The contents of the menu trigger button.
+ */
+ children?: React.ReactNode;
+ /**
+ * Allows the component to be rendered as a different HTML element or React
+ * component. The value can be a React element or a function that takes in the
+ * original component props and gives back a React element with the props
+ * merged.
+ */
+ render?: Ariakit.MenuButtonProps[ 'render' ];
+ /**
+ * Determines if the element is disabled. This sets the `aria-disabled`
+ * attribute accordingly, enabling support for all elements, including those
+ * that don't support the native `disabled` attribute.
+ *
+ * This feature can be combined with the `accessibleWhenDisabled` prop to
+ * make disabled elements still accessible via keyboard.
+ *
+ * **Note**: For this prop to work, the `focusable` prop must be set to
+ * `true`, if it's not set by default.
+ *
+ * @default false
+ */
+ disabled?: Ariakit.MenuButtonProps[ 'disabled' ];
+ /**
+ * Indicates whether the element should be focusable even when it is
+ * `disabled`.
+ *
+ * This is important when discoverability is a concern. For example:
+ *
+ * > A toolbar in an editor contains a set of special smart paste functions
+ * that are disabled when the clipboard is empty or when the function is not
+ * applicable to the current content of the clipboard. It could be helpful to
+ * keep the disabled buttons focusable if the ability to discover their
+ * functionality is primarily via their presence on the toolbar.
+ *
+ * Learn more on [Focusability of disabled
+ * controls](https://www.w3.org/WAI/ARIA/apg/practices/keyboard-interface/#focusabilityofdisabledcontrols).
+ */
+ accessibleWhenDisabled?: Ariakit.MenuButtonProps[ 'accessibleWhenDisabled' ];
+}
+
export interface MenuGroupProps {
/**
* The contents of the menu group (ie. an optional menu group label and one
@@ -118,6 +165,18 @@ export interface MenuItemProps {
* Determines if the element is disabled.
*/
disabled?: boolean;
+ /**
+ * Allows the component to be rendered as a different HTML element or React
+ * component. The value can be a React element or a function that takes in the
+ * original component props and gives back a React element with the props
+ * merged.
+ */
+ render?: Ariakit.MenuItemProps[ 'render' ];
+ /**
+ * The ariakit store. This prop is only meant for internal use.
+ * @ignore
+ */
+ store?: Ariakit.MenuItemProps[ 'store' ];
}
export interface MenuCheckboxItemProps
diff --git a/packages/components/src/menu/use-temporary-focus-visible-fix.ts b/packages/components/src/menu/use-temporary-focus-visible-fix.ts
deleted file mode 100644
index 0df1313373960..0000000000000
--- a/packages/components/src/menu/use-temporary-focus-visible-fix.ts
+++ /dev/null
@@ -1,22 +0,0 @@
-/**
- * WordPress dependencies
- */
-import { useState, flushSync } from '@wordpress/element';
-
-export function useTemporaryFocusVisibleFix( {
- onBlur: onBlurProp,
-}: {
- onBlur?: React.FocusEventHandler< HTMLDivElement >;
-} ) {
- const [ focusVisible, setFocusVisible ] = useState( false );
- return {
- 'data-focus-visible': focusVisible || undefined,
- onFocusVisible: () => {
- flushSync( () => setFocusVisible( true ) );
- },
- onBlur: ( ( event ) => {
- onBlurProp?.( event );
- setFocusVisible( false );
- } ) as React.FocusEventHandler< HTMLDivElement >,
- };
-}
diff --git a/packages/components/src/modal/index.tsx b/packages/components/src/modal/index.tsx
index 7d55c9a4d5d1e..2dec0f65240f9 100644
--- a/packages/components/src/modal/index.tsx
+++ b/packages/components/src/modal/index.tsx
@@ -335,10 +335,10 @@ function UnforwardedModal(
<>
) =>
diff --git a/packages/components/src/modal/stories/index.story.tsx b/packages/components/src/modal/stories/index.story.tsx
index 92c922bcb8a97..3a7b817458ad2 100644
--- a/packages/components/src/modal/stories/index.story.tsx
+++ b/packages/components/src/modal/stories/index.story.tsx
@@ -23,10 +23,10 @@ const meta: Meta< typeof Modal > = {
id: 'components-modal',
argTypes: {
children: {
- control: { type: null },
+ control: false,
},
onKeyDown: {
- control: { type: null },
+ control: false,
},
focusOnMount: {
options: [ true, false, 'firstElement', 'firstContentElement' ],
@@ -75,7 +75,10 @@ const Template: StoryFn< typeof Modal > = ( { onRequestClose, ...args } ) => {
anim id est laborum.
-
+
Close Modal
@@ -111,7 +114,7 @@ export const WithHeaderActions: StoryFn< typeof Modal > = Template.bind( {} );
WithHeaderActions.args = {
...Default.args,
headerActions: (
-
+
),
children:
,
};
diff --git a/packages/components/src/modal/test/index.tsx b/packages/components/src/modal/test/index.tsx
index a0d0ee2653edb..05dcc35dce18d 100644
--- a/packages/components/src/modal/test/index.tsx
+++ b/packages/components/src/modal/test/index.tsx
@@ -269,7 +269,8 @@ describe( 'Modal', () => {
} );
describe( 'Focus handling', () => {
- let originalGetClientRects: () => DOMRectList;
+ const originalGetClientRects =
+ window.HTMLElement.prototype.getClientRects;
const FocusMountDemo = ( {
focusOnMount,
diff --git a/packages/components/src/navigable-container/stories/navigable-menu.story.tsx b/packages/components/src/navigable-container/stories/navigable-menu.story.tsx
index 2ad7e028d6faf..30986ff479e43 100644
--- a/packages/components/src/navigable-container/stories/navigable-menu.story.tsx
+++ b/packages/components/src/navigable-container/stories/navigable-menu.story.tsx
@@ -13,7 +13,7 @@ const meta: Meta< typeof NavigableMenu > = {
id: 'components-navigablemenu',
component: NavigableMenu,
argTypes: {
- children: { control: { type: null } },
+ children: { control: false },
},
parameters: {
actions: { argTypesRegex: '^on.*' },
diff --git a/packages/components/src/navigable-container/stories/tabbable-container.story.tsx b/packages/components/src/navigable-container/stories/tabbable-container.story.tsx
index 07c87a0c20f1b..afb4119015b52 100644
--- a/packages/components/src/navigable-container/stories/tabbable-container.story.tsx
+++ b/packages/components/src/navigable-container/stories/tabbable-container.story.tsx
@@ -13,7 +13,7 @@ const meta: Meta< typeof TabbableContainer > = {
id: 'components-tabbablecontainer',
component: TabbableContainer,
argTypes: {
- children: { control: { type: null } },
+ children: { control: false },
},
parameters: {
actions: { argTypesRegex: '^on.*' },
diff --git a/packages/components/src/navigation/stories/index.story.tsx b/packages/components/src/navigation/stories/index.story.tsx
index 2f09ace29f16e..8510b6f20b537 100644
--- a/packages/components/src/navigation/stories/index.story.tsx
+++ b/packages/components/src/navigation/stories/index.story.tsx
@@ -39,10 +39,10 @@ const meta: Meta< typeof Navigation > = {
NavigationMenu,
},
argTypes: {
- activeItem: { control: { type: null } },
- activeMenu: { control: { type: null } },
- children: { control: { type: null } },
- onActivateMenu: { control: { type: null } },
+ activeItem: { control: false },
+ activeMenu: { control: false },
+ children: { control: false },
+ onActivateMenu: { control: false },
},
parameters: {
actions: { argTypesRegex: '^on.*' },
diff --git a/packages/components/src/navigation/styles/navigation-styles.tsx b/packages/components/src/navigation/styles/navigation-styles.tsx
index 580c0eef4dba8..aa0976a9a0f27 100644
--- a/packages/components/src/navigation/styles/navigation-styles.tsx
+++ b/packages/components/src/navigation/styles/navigation-styles.tsx
@@ -134,11 +134,12 @@ export const ItemBaseUI = styled.li`
&.is-active {
background-color: ${ COLORS.theme.accent };
- color: ${ COLORS.white };
+ color: ${ COLORS.theme.accentInverted };
> button,
+ .components-button:hover,
> a {
- color: ${ COLORS.white };
+ color: ${ COLORS.theme.accentInverted };
opacity: 1;
}
}
diff --git a/packages/components/src/navigator/stories/index.story.tsx b/packages/components/src/navigator/stories/index.story.tsx
index bd2cdc17a1263..0baf6a1c9cf5b 100644
--- a/packages/components/src/navigator/stories/index.story.tsx
+++ b/packages/components/src/navigator/stories/index.story.tsx
@@ -24,9 +24,9 @@ const meta: Meta< typeof Navigator > = {
title: 'Components/Navigation/Navigator',
id: 'components-navigator',
argTypes: {
- as: { control: { type: null } },
- children: { control: { type: null } },
- initialPath: { control: { type: null } },
+ as: { control: false },
+ children: { control: false },
+ initialPath: { control: false },
},
parameters: {
controls: { expanded: true },
diff --git a/packages/components/src/number-control/README.md b/packages/components/src/number-control/README.md
index 8421691296e90..486092790548e 100644
--- a/packages/components/src/number-control/README.md
+++ b/packages/components/src/number-control/README.md
@@ -16,6 +16,7 @@ const Example = () => {
return (
{};
@@ -53,9 +54,17 @@ function UnforwardedNumberControl(
size = 'default',
suffix,
onChange = noop,
+ __shouldNotWarnDeprecated36pxSize,
...restProps
} = useDeprecated36pxDefaultSizeProp< NumberControlProps >( props );
+ maybeWarnDeprecated36pxSize( {
+ componentName: 'NumberControl',
+ size,
+ __next40pxDefaultSize: restProps.__next40pxDefaultSize,
+ __shouldNotWarnDeprecated36pxSize,
+ } );
+
if ( hideHTMLArrows ) {
deprecated( 'wp.components.NumberControl hideHTMLArrows prop ', {
alternative: 'spinControls="none"',
@@ -233,6 +242,7 @@ function UnforwardedNumberControl(
return stateReducerProp?.( baseState, action ) ?? baseState;
} }
size={ size }
+ __shouldNotWarnDeprecated36pxSize
suffix={
spinControls === 'custom' ? (
<>
diff --git a/packages/components/src/number-control/stories/index.story.tsx b/packages/components/src/number-control/stories/index.story.tsx
index 3feb0d63eadc2..e66be3490bb71 100644
--- a/packages/components/src/number-control/stories/index.story.tsx
+++ b/packages/components/src/number-control/stories/index.story.tsx
@@ -23,7 +23,7 @@ const meta: Meta< typeof NumberControl > = {
step: { control: { type: 'text' } },
suffix: { control: { type: 'text' } },
type: { control: { type: 'text' } },
- value: { control: null },
+ value: { control: false },
},
parameters: {
controls: { expanded: true },
@@ -62,4 +62,5 @@ const Template: StoryFn< typeof NumberControl > = ( {
export const Default = Template.bind( {} );
Default.args = {
label: 'Value',
+ __next40pxDefaultSize: true,
};
diff --git a/packages/components/src/number-control/test/index.tsx b/packages/components/src/number-control/test/index.tsx
index 3cf3368f1636b..bf97b520673ea 100644
--- a/packages/components/src/number-control/test/index.tsx
+++ b/packages/components/src/number-control/test/index.tsx
@@ -12,9 +12,13 @@ import { useState } from '@wordpress/element';
/**
* Internal dependencies
*/
-import NumberControl from '..';
+import _NumberControl from '..';
import type { NumberControlProps } from '../types';
+const NumberControl = (
+ props: React.ComponentProps< typeof _NumberControl >
+) => <_NumberControl __next40pxDefaultSize { ...props } />;
+
function StatefulNumberControl( props: NumberControlProps ) {
const [ value, setValue ] = useState( props.value );
const handleOnChange = ( v: string | undefined ) => setValue( v );
diff --git a/packages/components/src/number-control/types.ts b/packages/components/src/number-control/types.ts
index 8d198e777bd55..2a0fbf402d356 100644
--- a/packages/components/src/number-control/types.ts
+++ b/packages/components/src/number-control/types.ts
@@ -91,4 +91,11 @@ export type NumberControlProps = Omit<
* The value of the input.
*/
value?: number | string;
+ /**
+ * Do not throw a warning for the deprecated 36px default size.
+ * For internal components of other components that already throw the warning.
+ *
+ * @ignore
+ */
+ __shouldNotWarnDeprecated36pxSize?: boolean;
};
diff --git a/packages/components/src/palette-edit/index.tsx b/packages/components/src/palette-edit/index.tsx
index a58ecbb685e51..2eb6e3bbe3b6f 100644
--- a/packages/components/src/palette-edit/index.tsx
+++ b/packages/components/src/palette-edit/index.tsx
@@ -60,6 +60,7 @@ const DEFAULT_COLOR = '#000';
function NameInput( { value, onChange, label }: NameInputProps ) {
return (
= {
// @ts-expect-error - See https://github.com/storybookjs/storybook/issues/23170
subcomponents: { PanelRow, PanelBody },
argTypes: {
- children: { control: { type: null } },
+ children: { control: false },
},
parameters: {
controls: { expanded: true },
@@ -74,12 +74,12 @@ _PanelRow.args = {
children: (
-
-
+
+
-
+
diff --git a/packages/components/src/placeholder/stories/index.story.tsx b/packages/components/src/placeholder/stories/index.story.tsx
index 541eeceedc27d..ffe60df0b4b85 100644
--- a/packages/components/src/placeholder/stories/index.story.tsx
+++ b/packages/components/src/placeholder/stories/index.story.tsx
@@ -21,9 +21,9 @@ const meta: Meta< typeof Placeholder > = {
component: Placeholder,
title: 'Components/Placeholder',
argTypes: {
- children: { control: { type: null } },
- notices: { control: { type: null } },
- preview: { control: { type: null } },
+ children: { control: false },
+ notices: { control: false },
+ preview: { control: false },
icon: {
control: { type: 'select' },
options: Object.keys( ICONS ),
@@ -45,6 +45,7 @@ const Template: StoryFn< typeof Placeholder > = ( args ) => {
= {
id: 'components-popover',
component: Popover,
argTypes: {
- anchor: { control: { type: null } },
- anchorRef: { control: { type: null } },
- anchorRect: { control: { type: null } },
- children: { control: { type: null } },
+ anchor: { control: false },
+ anchorRef: { control: false },
+ anchorRect: { control: false },
+ children: { control: false },
focusOnMount: {
control: { type: 'select' },
options: [ 'firstElement', true, false ],
},
- getAnchorRect: { control: { type: null } },
+ getAnchorRect: { control: false },
onClose: { action: 'onClose' },
onFocusOutside: { action: 'onFocusOutside' },
- __unstableSlotName: { control: { type: null } },
+ __unstableSlotName: { control: false },
},
parameters: {
controls: { expanded: true },
@@ -58,7 +58,9 @@ const meta: Meta< typeof Popover > = {
export default meta;
const PopoverWithAnchor = ( args: PopoverProps ) => {
- const anchorRef = useRef( null );
+ const [ popoverAnchor, setPopoverAnchor ] = useState< Element | null >(
+ null
+ );
return (
);
};
diff --git a/packages/components/src/private-apis.ts b/packages/components/src/private-apis.ts
index bea16b719a463..f5a9ee90519c2 100644
--- a/packages/components/src/private-apis.ts
+++ b/packages/components/src/private-apis.ts
@@ -2,21 +2,21 @@
* Internal dependencies
*/
import { positionToPlacement as __experimentalPopoverLegacyPositionToPlacement } from './popover/utils';
-import { createPrivateSlotFill } from './slot-fill';
import { Menu } from './menu';
import { ComponentsContext } from './context/context-system-provider';
import Theme from './theme';
import { Tabs } from './tabs';
import { kebabCase } from './utils/strings';
import { lock } from './lock-unlock';
+import Badge from './badge';
export const privateApis = {};
lock( privateApis, {
__experimentalPopoverLegacyPositionToPlacement,
- createPrivateSlotFill,
ComponentsContext,
Tabs,
Theme,
Menu,
kebabCase,
+ Badge,
} );
diff --git a/packages/components/src/query-controls/stories/index.story.tsx b/packages/components/src/query-controls/stories/index.story.tsx
index 04fe185a59eac..ad28d9aed0d0d 100644
--- a/packages/components/src/query-controls/stories/index.story.tsx
+++ b/packages/components/src/query-controls/stories/index.story.tsx
@@ -22,12 +22,12 @@ const meta: Meta< typeof QueryControls > = {
title: 'Components/QueryControls',
component: QueryControls,
argTypes: {
- numberOfItems: { control: { type: null } },
- order: { control: { type: null } },
- orderBy: { control: { type: null } },
- selectedAuthorId: { control: { type: null } },
- selectedCategories: { control: { type: null } },
- selectedCategoryId: { control: { type: null } },
+ numberOfItems: { control: false },
+ order: { control: false },
+ orderBy: { control: false },
+ selectedAuthorId: { control: false },
+ selectedCategories: { control: false },
+ selectedCategoryId: { control: false },
},
parameters: {
actions: { argTypesRegex: '^on.*' },
diff --git a/packages/components/src/radio-control/stories/index.story.tsx b/packages/components/src/radio-control/stories/index.story.tsx
index 3c76f7610d0d7..7b7bc773f323a 100644
--- a/packages/components/src/radio-control/stories/index.story.tsx
+++ b/packages/components/src/radio-control/stories/index.story.tsx
@@ -22,7 +22,7 @@ const meta: Meta< typeof RadioControl > = {
action: 'onChange',
},
selected: {
- control: { type: null },
+ control: false,
},
label: {
control: { type: 'text' },
diff --git a/packages/components/src/radio-group/radio.tsx b/packages/components/src/radio-group/radio.tsx
index 782a737b6ba28..4c54e0694f4bd 100644
--- a/packages/components/src/radio-group/radio.tsx
+++ b/packages/components/src/radio-group/radio.tsx
@@ -7,7 +7,6 @@ import { forwardRef, useContext } from '@wordpress/element';
* External dependencies
*/
import * as Ariakit from '@ariakit/react';
-import { useStoreState } from '@ariakit/react';
/**
* Internal dependencies
@@ -28,7 +27,7 @@ function UnforwardedRadio(
) {
const { store, disabled } = useContext( RadioGroupContext );
- const selectedValue = useStoreState( store, 'value' );
+ const selectedValue = Ariakit.useStoreState( store, 'value' );
const isChecked = selectedValue !== undefined && selectedValue === value;
maybeWarnDeprecated36pxSize( {
diff --git a/packages/components/src/radio-group/stories/index.story.tsx b/packages/components/src/radio-group/stories/index.story.tsx
index a19fb077e7ec4..aee8610e1b700 100644
--- a/packages/components/src/radio-group/stories/index.story.tsx
+++ b/packages/components/src/radio-group/stories/index.story.tsx
@@ -21,8 +21,8 @@ const meta: Meta< typeof RadioGroup > = {
// @ts-expect-error - See https://github.com/storybookjs/storybook/issues/23170
subcomponents: { Radio },
argTypes: {
- onChange: { control: { type: null } },
- children: { control: { type: null } },
+ onChange: { control: false },
+ children: { control: false },
checked: { control: { type: 'text' } },
},
parameters: {
@@ -99,5 +99,5 @@ Controlled.args = {
id: 'controlled-radiogroup',
};
Controlled.argTypes = {
- checked: { control: { type: null } },
+ checked: { control: false },
};
diff --git a/packages/components/src/range-control/README.md b/packages/components/src/range-control/README.md
index cfa8c76740e74..f21285c5f2625 100644
--- a/packages/components/src/range-control/README.md
+++ b/packages/components/src/range-control/README.md
@@ -88,9 +88,10 @@ import { RangeControl } from '@wordpress/components';
const MyRangeControl = () => {
const [ columns, setColumns ] = useState( 2 );
- return(
+ return (
setColumns( value ) }
@@ -153,7 +154,6 @@ Disables the `input`, preventing new values from being applied.
- Required: No
- Platform: Web
-
### `help`: `string|Element`
If this property is added, a help text will be generated using help property as the content.
@@ -165,7 +165,7 @@ If this property is added, a help text will be generated using help property as
Provides control over whether the label will only be visible to screen readers.
-- Required: No
+- Required: No
### `icon`: `string`
@@ -334,6 +334,7 @@ The minimum amount by which `value` changes. It is also a factor in validation a
- Required: No
- Platform: Web
+
### `trackColor`: `CSSProperties[ 'color' ]`
CSS color string to customize the track element's background.
diff --git a/packages/components/src/range-control/index.tsx b/packages/components/src/range-control/index.tsx
index c9fbdc0055c85..89dd8248a1e61 100644
--- a/packages/components/src/range-control/index.tsx
+++ b/packages/components/src/range-control/index.tsx
@@ -38,6 +38,7 @@ import {
import type { RangeControlProps } from './types';
import type { WordPressComponentProps } from '../context';
import { space } from '../utils/space';
+import { maybeWarnDeprecated36pxSize } from '../utils/deprecated-36px-size';
const noop = () => {};
@@ -96,6 +97,7 @@ function UnforwardedRangeControl(
trackColor,
value: valueProp,
withInputField = true,
+ __shouldNotWarnDeprecated36pxSize,
...otherProps
} = props;
@@ -229,6 +231,14 @@ function UnforwardedRangeControl(
[ isRTL() ? 'right' : 'left' ]: fillValueOffset,
};
+ // Add default size deprecation warning.
+ maybeWarnDeprecated36pxSize( {
+ componentName: 'RangeControl',
+ __next40pxDefaultSize,
+ size: undefined,
+ __shouldNotWarnDeprecated36pxSize,
+ } );
+
return (
) }
{ allowReset && (
@@ -384,6 +395,7 @@ function UnforwardedRangeControl(
* return (
*
{ label && (
diff --git a/packages/components/src/range-control/stories/index.story.tsx b/packages/components/src/range-control/stories/index.story.tsx
index dcff351373352..5a4b2342a49ff 100644
--- a/packages/components/src/range-control/stories/index.story.tsx
+++ b/packages/components/src/range-control/stories/index.story.tsx
@@ -33,18 +33,18 @@ const meta: Meta< typeof RangeControl > = {
},
color: { control: { type: 'color' } },
help: { control: { type: 'text' } },
- icon: { control: { type: null } },
+ icon: { control: false },
marks: { control: { type: 'object' } },
- onBlur: { control: { type: null } },
- onChange: { control: { type: null } },
- onFocus: { control: { type: null } },
- onMouseLeave: { control: { type: null } },
- onMouseMove: { control: { type: null } },
+ onBlur: { control: false },
+ onChange: { control: false },
+ onFocus: { control: false },
+ onMouseLeave: { control: false },
+ onMouseMove: { control: false },
railColor: { control: { type: 'color' } },
step: { control: { type: 'number' } },
trackColor: { control: { type: 'color' } },
type: { control: { type: 'check' }, options: [ 'stepper' ] },
- value: { control: { type: null } },
+ value: { control: false },
},
parameters: {
actions: { argTypesRegex: '^on.*' },
@@ -72,6 +72,7 @@ const Template: StoryFn< typeof RangeControl > = ( { onChange, ...args } ) => {
export const Default: StoryFn< typeof RangeControl > = Template.bind( {} );
Default.args = {
__nextHasNoMarginBottom: true,
+ __next40pxDefaultSize: true,
help: 'Please select how transparent you would like this.',
initialPosition: 50,
label: 'Opacity',
@@ -107,6 +108,7 @@ export const WithAnyStep: StoryFn< typeof RangeControl > = ( {
};
WithAnyStep.args = {
__nextHasNoMarginBottom: true,
+ __next40pxDefaultSize: true,
label: 'Brightness',
step: 'any',
};
@@ -171,6 +173,7 @@ export const WithIntegerStepAndMarks: StoryFn< typeof RangeControl > =
WithIntegerStepAndMarks.args = {
__nextHasNoMarginBottom: true,
+ __next40pxDefaultSize: true,
label: 'Integer Step',
marks: marksBase,
max: 10,
@@ -188,6 +191,7 @@ export const WithDecimalStepAndMarks: StoryFn< typeof RangeControl > =
WithDecimalStepAndMarks.args = {
__nextHasNoMarginBottom: true,
+ __next40pxDefaultSize: true,
marks: [
...marksBase,
{ value: 3.5, label: '3.5' },
@@ -208,6 +212,7 @@ export const WithNegativeMinimumAndMarks: StoryFn< typeof RangeControl > =
WithNegativeMinimumAndMarks.args = {
__nextHasNoMarginBottom: true,
+ __next40pxDefaultSize: true,
marks: marksWithNegatives,
max: 10,
min: -10,
@@ -224,6 +229,7 @@ export const WithNegativeRangeAndMarks: StoryFn< typeof RangeControl > =
WithNegativeRangeAndMarks.args = {
__nextHasNoMarginBottom: true,
+ __next40pxDefaultSize: true,
marks: marksWithNegatives,
max: -1,
min: -10,
@@ -240,6 +246,7 @@ export const WithAnyStepAndMarks: StoryFn< typeof RangeControl > =
WithAnyStepAndMarks.args = {
__nextHasNoMarginBottom: true,
+ __next40pxDefaultSize: true,
marks: marksBase,
max: 10,
min: 0,
diff --git a/packages/components/src/range-control/styles/range-control-styles.ts b/packages/components/src/range-control/styles/range-control-styles.ts
index 6e9c68ace9753..c86c57800cac4 100644
--- a/packages/components/src/range-control/styles/range-control-styles.ts
+++ b/packages/components/src/range-control/styles/range-control-styles.ts
@@ -130,6 +130,12 @@ export const Track = styled.span`
margin-top: ${ ( rangeHeightValue - railHeight ) / 2 }px;
top: 0;
+ .is-marked & {
+ @media not ( prefers-reduced-motion ) {
+ transition: width ease 0.1s;
+ }
+ }
+
${ trackBackgroundColor };
`;
@@ -139,28 +145,18 @@ export const MarksWrapper = styled.span`
position: relative;
width: 100%;
user-select: none;
+ margin-top: 17px;
`;
-const markFill = ( { disabled, isFilled }: RangeMarkProps ) => {
- let backgroundColor = isFilled ? 'currentColor' : COLORS.gray[ 300 ];
-
- if ( disabled ) {
- backgroundColor = COLORS.gray[ 400 ];
- }
-
- return css( {
- backgroundColor,
- } );
-};
-
export const Mark = styled.span`
- height: ${ thumbSize }px;
- left: 0;
position: absolute;
- top: 9px;
- width: 1px;
-
- ${ markFill };
+ left: 0;
+ top: -4px;
+ height: 4px;
+ width: 2px;
+ transform: translateX( -50% );
+ background-color: ${ COLORS.ui.background };
+ z-index: 1;
`;
const markLabelFill = ( { isFilled }: RangeMarkProps ) => {
@@ -173,7 +169,7 @@ export const MarkLabel = styled.span`
color: ${ COLORS.gray[ 300 ] };
font-size: 11px;
position: absolute;
- top: 22px;
+ top: 8px;
white-space: nowrap;
${ rtl( { left: 0 } ) };
@@ -207,6 +203,13 @@ export const ThumbWrapper = styled.span`
user-select: none;
width: ${ thumbSize }px;
border-radius: ${ CONFIG.radiusRound };
+ z-index: 3;
+
+ .is-marked & {
+ @media not ( prefers-reduced-motion ) {
+ transition: left ease 0.1s;
+ }
+ }
${ thumbColor };
${ rtl( { marginLeft: -10 } ) };
diff --git a/packages/components/src/range-control/test/index.tsx b/packages/components/src/range-control/test/index.tsx
index 3ce741867d0db..3d2db30eea186 100644
--- a/packages/components/src/range-control/test/index.tsx
+++ b/packages/components/src/range-control/test/index.tsx
@@ -18,7 +18,13 @@ const fireChangeEvent = ( input: HTMLInputElement, value?: number | string ) =>
const RangeControl = (
props: React.ComponentProps< typeof _RangeControl >
) => {
- return <_RangeControl { ...props } __nextHasNoMarginBottom />;
+ return (
+ <_RangeControl
+ { ...props }
+ __nextHasNoMarginBottom
+ __next40pxDefaultSize
+ />
+ );
};
describe( 'RangeControl', () => {
diff --git a/packages/components/src/range-control/types.ts b/packages/components/src/range-control/types.ts
index a427ab4f942af..e4792296f8314 100644
--- a/packages/components/src/range-control/types.ts
+++ b/packages/components/src/range-control/types.ts
@@ -233,6 +233,13 @@ export type RangeControlProps = Pick<
* @default true
*/
withInputField?: boolean;
+ /**
+ * Do not throw a warning for the deprecated 36px default size.
+ * For internal components of other components that already throw the warning.
+ *
+ * @ignore
+ */
+ __shouldNotWarnDeprecated36pxSize?: boolean;
};
export type RailProps = MarksProps & {
diff --git a/packages/components/src/resizable-box/index.tsx b/packages/components/src/resizable-box/index.tsx
index 1b05270ea0bf2..3bf3d36aa0d5c 100644
--- a/packages/components/src/resizable-box/index.tsx
+++ b/packages/components/src/resizable-box/index.tsx
@@ -112,6 +112,16 @@ function UnforwardedResizableBox(
showHandle && 'has-show-handle',
className
) }
+ // Add a focusable element within the drag handle. Unfortunately,
+ // `re-resizable` does not make them properly focusable by default,
+ // causing focus to move the the block wrapper which triggers block
+ // drag.
+ handleComponent={ Object.fromEntries(
+ Object.keys( HANDLE_CLASSES ).map( ( key ) => [
+ key,
+
,
+ ] )
+ ) }
handleClasses={ HANDLE_CLASSES }
handleStyles={ HANDLE_STYLES }
ref={ ref }
diff --git a/packages/components/src/resizable-box/stories/index.story.tsx b/packages/components/src/resizable-box/stories/index.story.tsx
index 489a094c33f11..aa5b080d00b51 100644
--- a/packages/components/src/resizable-box/stories/index.story.tsx
+++ b/packages/components/src/resizable-box/stories/index.story.tsx
@@ -18,7 +18,7 @@ const meta: Meta< typeof ResizableBox > = {
id: 'components-resizablebox',
component: ResizableBox,
argTypes: {
- children: { control: { type: null } },
+ children: { control: false },
enable: { control: 'object' },
onResizeStop: { action: 'onResizeStop' },
},
diff --git a/packages/components/src/resizable-box/style.scss b/packages/components/src/resizable-box/style.scss
index 3c9efd2713646..4db3d27b5fab6 100644
--- a/packages/components/src/resizable-box/style.scss
+++ b/packages/components/src/resizable-box/style.scss
@@ -15,6 +15,14 @@ $resize-handler-container-size: $resize-handler-size + ($grid-unit-05 * 2); // M
.components-resizable-box__container.has-show-handle & {
display: block;
}
+
+ > div {
+ position: relative;
+ width: 100%;
+ height: 100%;
+ z-index: z-index(".components-resizable-box__handle");
+ outline: none;
+ }
}
// Make the image inside the resize to get the full width
diff --git a/packages/components/src/responsive-wrapper/stories/index.story.tsx b/packages/components/src/responsive-wrapper/stories/index.story.tsx
index d684a00c87002..5a834b999b715 100644
--- a/packages/components/src/responsive-wrapper/stories/index.story.tsx
+++ b/packages/components/src/responsive-wrapper/stories/index.story.tsx
@@ -13,7 +13,7 @@ const meta: Meta< typeof ResponsiveWrapper > = {
title: 'Components/Layout/ResponsiveWrapper',
id: 'components-responsivewrapper',
argTypes: {
- children: { control: { type: null } },
+ children: { control: false },
},
parameters: {
controls: { expanded: true },
diff --git a/packages/components/src/sandbox/stories/index.story.tsx b/packages/components/src/sandbox/stories/index.story.tsx
index 0d083eac3e902..6d5eaa4868e78 100644
--- a/packages/components/src/sandbox/stories/index.story.tsx
+++ b/packages/components/src/sandbox/stories/index.story.tsx
@@ -13,7 +13,7 @@ const meta: Meta< typeof SandBox > = {
title: 'Components/Utilities/SandBox',
id: 'components-sandbox',
argTypes: {
- onFocus: { control: { type: null } },
+ onFocus: { control: false },
},
parameters: {
actions: { argTypesRegex: '^on.*' },
diff --git a/packages/components/src/scrollable/stories/index.story.tsx b/packages/components/src/scrollable/stories/index.story.tsx
index 53d4919de3aab..4970b3720e8a0 100644
--- a/packages/components/src/scrollable/stories/index.story.tsx
+++ b/packages/components/src/scrollable/stories/index.story.tsx
@@ -22,7 +22,7 @@ const meta: Meta< typeof Scrollable > = {
control: { type: 'text' },
},
children: {
- control: { type: null },
+ control: false,
},
},
parameters: {
@@ -70,6 +70,7 @@ const Template: StoryFn< typeof Scrollable > = ( { ...args } ) => {
} }
type="text"
value="Focus me"
+ readOnly
/>
diff --git a/packages/components/src/search-control/stories/index.story.tsx b/packages/components/src/search-control/stories/index.story.tsx
index 5e5f6b594e73e..c3385c4eb21b4 100644
--- a/packages/components/src/search-control/stories/index.story.tsx
+++ b/packages/components/src/search-control/stories/index.story.tsx
@@ -19,7 +19,7 @@ const meta: Meta< typeof SearchControl > = {
component: SearchControl,
argTypes: {
onChange: { action: 'onChange' },
- value: { control: { type: null } },
+ value: { control: false },
},
parameters: {
controls: { expanded: true },
diff --git a/packages/components/src/select-control/README.md b/packages/components/src/select-control/README.md
index c240243408fab..d8742fce74f54 100644
--- a/packages/components/src/select-control/README.md
+++ b/packages/components/src/select-control/README.md
@@ -92,6 +92,7 @@ const MySelectControl = () => {
{ label: 'Small', value: '25%' },
] }
onChange={ ( newSize ) => setSize( newSize ) }
+ __next40pxDefaultSize
__nextHasNoMarginBottom
/>
);
@@ -114,6 +115,7 @@ Render a user interface to select multiple users from a list.
{ value: 'b', label: 'User B' },
{ value: 'c', label: 'User c' },
] }
+ __next40pxDefaultSize
__nextHasNoMarginBottom
/>
```
@@ -129,6 +131,7 @@ const [ item, setItem ] = useState( '' );
label={ __( 'My dinosaur' ) }
value={ item } // e.g: value = 'a'
onChange={ ( selection ) => { setItem( selection ) } }
+ __next40pxDefaultSize
__nextHasNoMarginBottom
>
diff --git a/packages/components/src/select-control/index.tsx b/packages/components/src/select-control/index.tsx
index 3686661b8a58d..e93e9385a9c23 100644
--- a/packages/components/src/select-control/index.tsx
+++ b/packages/components/src/select-control/index.tsx
@@ -18,6 +18,7 @@ import type { WordPressComponentProps } from '../context';
import type { SelectControlProps } from './types';
import SelectControlChevronDown from './chevron-down';
import { useDeprecated36pxDefaultSizeProp } from '../utils/use-deprecated-props';
+import { maybeWarnDeprecated36pxSize } from '../utils/deprecated-36px-size';
function useUniqueId( idProp?: string ) {
const instanceId = useInstanceId( SelectControl );
@@ -65,6 +66,7 @@ function UnforwardedSelectControl< V extends string >(
variant = 'default',
__next40pxDefaultSize = false,
__nextHasNoMarginBottom = false,
+ __shouldNotWarnDeprecated36pxSize,
...restProps
} = useDeprecated36pxDefaultSizeProp( props );
const id = useUniqueId( idProp );
@@ -94,6 +96,13 @@ function UnforwardedSelectControl< V extends string >(
const classes = clsx( 'components-select-control', className );
+ maybeWarnDeprecated36pxSize( {
+ componentName: 'SelectControl',
+ __next40pxDefaultSize,
+ size,
+ __shouldNotWarnDeprecated36pxSize,
+ } );
+
return (
(
*
* return (
* = {
label: { control: { type: 'text' } },
prefix: { control: { type: 'text' } },
suffix: { control: { type: 'text' } },
- value: { control: { type: null } },
+ value: { control: false },
},
parameters: {
actions: { argTypesRegex: '^on.*' },
@@ -65,6 +65,7 @@ const SelectControlWithState: StoryFn< typeof SelectControl > = ( props ) => {
export const Default = SelectControlWithState.bind( {} );
Default.args = {
+ __next40pxDefaultSize: true,
__nextHasNoMarginBottom: true,
label: 'Label',
options: [
@@ -87,6 +88,7 @@ WithLabelAndHelpText.args = {
*/
export const WithCustomChildren = SelectControlWithState.bind( {} );
WithCustomChildren.args = {
+ __next40pxDefaultSize: true,
__nextHasNoMarginBottom: true,
label: 'Label',
children: (
diff --git a/packages/components/src/select-control/test/select-control.tsx b/packages/components/src/select-control/test/select-control.tsx
index 47b684cd20e28..37935d60384b1 100644
--- a/packages/components/src/select-control/test/select-control.tsx
+++ b/packages/components/src/select-control/test/select-control.tsx
@@ -12,7 +12,13 @@ import _SelectControl from '..';
const SelectControl = (
props: React.ComponentProps< typeof _SelectControl >
) => {
- return <_SelectControl { ...props } __nextHasNoMarginBottom />;
+ return (
+ <_SelectControl
+ { ...props }
+ __nextHasNoMarginBottom
+ __next40pxDefaultSize
+ />
+ );
};
describe( 'SelectControl', () => {
diff --git a/packages/components/src/select-control/types.ts b/packages/components/src/select-control/types.ts
index 4e7211ab9abfb..3d9f06385c753 100644
--- a/packages/components/src/select-control/types.ts
+++ b/packages/components/src/select-control/types.ts
@@ -13,6 +13,7 @@ type SelectControlBaseProps< V extends string > = Pick<
InputBaseProps,
| '__next36pxDefaultSize'
| '__next40pxDefaultSize'
+ | '__shouldNotWarnDeprecated36pxSize'
| 'disabled'
| 'hideLabelFromVision'
| 'label'
diff --git a/packages/components/src/slot-fill/README.md b/packages/components/src/slot-fill/README.md
index 9059566deefdf..3f14b9ccde9ee 100644
--- a/packages/components/src/slot-fill/README.md
+++ b/packages/components/src/slot-fill/README.md
@@ -1,18 +1,18 @@
-# Slot Fill
+# Slot/Fill
-Slot and Fill are a pair of components which enable developers to render elsewhere in a React element tree, a pattern often referred to as "portal" rendering. It is a pattern for component extensibility, where a single Slot may be occupied by an indeterminate number of Fills elsewhere in the application.
+`Slot` and `Fill` are a pair of components which enable developers to render React UI elsewhere in a React element tree, a pattern often referred to as "portal" rendering. It is a pattern for component extensibility, where a single `Slot` may be occupied by multiple `Fill`s rendered in different parts of the application.
-Slot Fill is heavily inspired by the [`react-slot-fill` library](https://github.com/camwest/react-slot-fill), but uses React's own portal rendering API.
+Slot/Fill was originally inspired by the [`react-slot-fill` library](https://github.com/camwest/react-slot-fill).
## Usage
-At the root of your application, you must render a `SlotFillProvider` which coordinates Slot and Fill rendering.
+At the root of your application, you must render a `SlotFillProvider` which coordinates `Slot` and `Fill` rendering.
-Then, render a Slot component anywhere in your application, giving it a name.
+Then, render a `Slot` component anywhere in your application, giving it a `name`. The `name` is either a `string` or a symbol. Symbol names are useful for slots that are supposed to be private, accessible only to clients that have access to the symbol value.
-Any Fill will automatically occupy this Slot space, even if rendered elsewhere in the application.
+Any `Fill` will render its UI in this `Slot` space, even if rendered elsewhere in the application.
-You can either use the Fill component directly, or a wrapper component type as in the below example to abstract the slot name from consumer awareness.
+You can either use the `Fill` component directly, or create a wrapper component (as in the following example) to hide the slot name from the consumer.
```jsx
import {
@@ -43,7 +43,7 @@ const MySlotFillProvider = () => {
};
```
-There is also `createSlotFill` helper method which was created to simplify the process of matching the corresponding `Slot` and `Fill` components:
+There is also the `createSlotFill` helper method which was created to simplify the process of matching the corresponding `Slot` and `Fill` components:
```jsx
const { Fill, Slot } = createSlotFill( 'Toolbar' );
@@ -59,18 +59,27 @@ const Toolbar = () => (
## Props
-The `SlotFillProvider` component does not accept any props.
+The `SlotFillProvider` component does not accept any props (except `children`).
Both `Slot` and `Fill` accept a `name` string prop, where a `Slot` with a given `name` will render the `children` of any associated `Fill`s.
-`Slot` accepts a `bubblesVirtually` prop which changes the event bubbling behaviour:
+`Slot` accepts a `bubblesVirtually` prop which changes the method how the `Fill` children are rendered. With `bubblesVirtually`, the `Fill` is rendered using a React portal. That affects the event bubbling and React context propagation behaviour:
-- By default, events will bubble to their parents on the DOM hierarchy (native event bubbling)
-- If `bubblesVirtually` is set to true, events will bubble to their virtual parent in the React elements hierarchy instead.
+### `bubblesVirtually=false`
-`Slot` with `bubblesVirtually` set to true also accept optional `className` and `style` props to add to the slot container.
+- events will bubble to their parents on the DOM hierarchy (native event bubbling)
+- the React elements inside the `Fill` will be rendered with React context of the `Slot`
+- renders the `Fill` elements directly, inside a `Fragment`, with no wrapper DOM element
-`Slot` **without** `bubblesVirtually` accepts an optional `children` function prop, which takes `fills` as a param. It allows you to perform additional processing and wrap `fills` conditionally.
+### `bubblesVirtually=true`
+
+- events will bubble to their virtual (React) parent in the React elements hierarchy
+- the React elements inside the `Fill` will keep the React context of the `Fill` and its parents
+- renders a wrapper DOM element inside which the `Fill` elements are rendered (used as an argument for React `createPortal`)
+
+`Slot` with `bubblesVirtually=true` renders a wrapper DOM element (a `div` by default) and accepts additional props that customize this element, like `className` or `style`. You can also replace the `div` with another element by passing an `as` prop.
+
+`Slot` **without** `bubblesVirtually` accepts an optional `children` prop, which is a function that receives `fills` array as a param. It allows you to perform additional processing: render a placeholder when there are no fills, or render a wrapper only when there are fills.
_Example_:
@@ -90,7 +99,9 @@ const Toolbar = ( { isMobile } ) => (
);
```
-Props can also be passed from a `Slot` to a `Fill` by using the prop `fillProps` on the `Slot`:
+Additional information (props) can also be passed from a `Slot` to a `Fill` by a combination of:
+1. Adding a `fillProps` prop to the `Slot`.
+2. Passing a function as `children` to the `Fill`. This function will receive the `fillProps` as an argument.
```jsx
const { Fill, Slot } = createSlotFill( 'Toolbar' );
diff --git a/packages/components/src/slot-fill/bubbles-virtually/fill.tsx b/packages/components/src/slot-fill/bubbles-virtually/fill.tsx
index 3cfadbadc62c4..ef7bc94ff540b 100644
--- a/packages/components/src/slot-fill/bubbles-virtually/fill.tsx
+++ b/packages/components/src/slot-fill/bubbles-virtually/fill.tsx
@@ -1,50 +1,36 @@
/**
* WordPress dependencies
*/
-import { useRef, useState, useEffect, createPortal } from '@wordpress/element';
+import { useObservableValue } from '@wordpress/compose';
+import {
+ useContext,
+ useRef,
+ useEffect,
+ createPortal,
+} from '@wordpress/element';
/**
* Internal dependencies
*/
-import useSlot from './use-slot';
+import SlotFillContext from './slot-fill-context';
import StyleProvider from '../../style-provider';
import type { FillComponentProps } from '../types';
-function useForceUpdate() {
- const [ , setState ] = useState( {} );
- const mountedRef = useRef( true );
+export default function Fill( { name, children }: FillComponentProps ) {
+ const registry = useContext( SlotFillContext );
+ const slot = useObservableValue( registry.slots, name );
+ const instanceRef = useRef( {} );
+ // We register fills so we can keep track of their existence.
+ // Slots can use the `useSlotFills` hook to know if there're already fills
+ // registered so they can choose to render themselves or not.
useEffect( () => {
- mountedRef.current = true;
- return () => {
- mountedRef.current = false;
- };
- }, [] );
+ const instance = instanceRef.current;
+ registry.registerFill( name, instance );
+ return () => registry.unregisterFill( name, instance );
+ }, [ registry, name ] );
- return () => {
- if ( mountedRef.current ) {
- setState( {} );
- }
- };
-}
-
-export default function Fill( props: FillComponentProps ) {
- const { name, children } = props;
- const { registerFill, unregisterFill, ...slot } = useSlot( name );
- const rerender = useForceUpdate();
- const ref = useRef( { rerender } );
-
- useEffect( () => {
- // We register fills so we can keep track of their existence.
- // Some Slot implementations need to know if there're already fills
- // registered so they can choose to render themselves or not.
- registerFill( ref );
- return () => {
- unregisterFill( ref );
- };
- }, [ registerFill, unregisterFill ] );
-
- if ( ! slot.ref || ! slot.ref.current ) {
+ if ( ! slot || ! slot.ref.current ) {
return null;
}
diff --git a/packages/components/src/slot-fill/bubbles-virtually/slot-fill-provider.tsx b/packages/components/src/slot-fill/bubbles-virtually/slot-fill-provider.tsx
index 4d68db6fd175e..cf692700eef79 100644
--- a/packages/components/src/slot-fill/bubbles-virtually/slot-fill-provider.tsx
+++ b/packages/components/src/slot-fill/bubbles-virtually/slot-fill-provider.tsx
@@ -23,26 +23,28 @@ function createSlotRegistry(): SlotFillBubblesVirtuallyContext {
ref,
fillProps
) => {
- const slot = slots.get( name );
-
- slots.set( name, {
- ...slot,
- ref: ref || slot?.ref,
- fillProps: fillProps || slot?.fillProps || {},
- } );
+ slots.set( name, { ref, fillProps } );
};
const unregisterSlot: SlotFillBubblesVirtuallyContext[ 'unregisterSlot' ] =
( name, ref ) => {
+ const slot = slots.get( name );
+ if ( ! slot ) {
+ return;
+ }
+
// Make sure we're not unregistering a slot registered by another element
// See https://github.com/WordPress/gutenberg/pull/19242#issuecomment-590295412
- if ( slots.get( name )?.ref === ref ) {
- slots.delete( name );
+ if ( slot.ref !== ref ) {
+ return;
}
+
+ slots.delete( name );
};
const updateSlot: SlotFillBubblesVirtuallyContext[ 'updateSlot' ] = (
name,
+ ref,
fillProps
) => {
const slot = slots.get( name );
@@ -50,16 +52,15 @@ function createSlotRegistry(): SlotFillBubblesVirtuallyContext {
return;
}
- if ( isShallowEqual( slot.fillProps, fillProps ) ) {
+ if ( slot.ref !== ref ) {
return;
}
- slot.fillProps = fillProps;
- const slotFills = fills.get( name );
- if ( slotFills ) {
- // Force update fills.
- slotFills.forEach( ( fill ) => fill.current.rerender() );
+ if ( isShallowEqual( slot.fillProps, fillProps ) ) {
+ return;
}
+
+ slots.set( name, { ref, fillProps } );
};
const registerFill: SlotFillBubblesVirtuallyContext[ 'registerFill' ] = (
@@ -69,20 +70,18 @@ function createSlotRegistry(): SlotFillBubblesVirtuallyContext {
fills.set( name, [ ...( fills.get( name ) || [] ), ref ] );
};
- const unregisterFill: SlotFillBubblesVirtuallyContext[ 'registerFill' ] = (
- name,
- ref
- ) => {
- const fillsForName = fills.get( name );
- if ( ! fillsForName ) {
- return;
- }
+ const unregisterFill: SlotFillBubblesVirtuallyContext[ 'unregisterFill' ] =
+ ( name, ref ) => {
+ const fillsForName = fills.get( name );
+ if ( ! fillsForName ) {
+ return;
+ }
- fills.set(
- name,
- fillsForName.filter( ( fillRef ) => fillRef !== ref )
- );
- };
+ fills.set(
+ name,
+ fillsForName.filter( ( fillRef ) => fillRef !== ref )
+ );
+ };
return {
slots,
diff --git a/packages/components/src/slot-fill/bubbles-virtually/slot.tsx b/packages/components/src/slot-fill/bubbles-virtually/slot.tsx
index 6ac2d51e1f857..e65c055c410a6 100644
--- a/packages/components/src/slot-fill/bubbles-virtually/slot.tsx
+++ b/packages/components/src/slot-fill/bubbles-virtually/slot.tsx
@@ -35,29 +35,30 @@ function Slot(
as,
// `children` is not allowed. However, if it is passed,
// it will be displayed as is, so remove `children`.
- // @ts-ignore
children,
...restProps
} = props;
- const { registerSlot, unregisterSlot, ...registry } =
- useContext( SlotFillContext );
+ const registry = useContext( SlotFillContext );
+
const ref = useRef< HTMLElement >( null );
+ // We don't want to unregister and register the slot whenever
+ // `fillProps` change, which would cause the fill to be re-mounted. Instead,
+ // we can just update the slot (see hook below).
+ // For more context, see https://github.com/WordPress/gutenberg/pull/44403#discussion_r994415973
+ const fillPropsRef = useRef( fillProps );
+ useLayoutEffect( () => {
+ fillPropsRef.current = fillProps;
+ }, [ fillProps ] );
+
useLayoutEffect( () => {
- registerSlot( name, ref, fillProps );
- return () => {
- unregisterSlot( name, ref );
- };
- // We don't want to unregister and register the slot whenever
- // `fillProps` change, which would cause the fill to be re-mounted. Instead,
- // we can just update the slot (see hook below).
- // For more context, see https://github.com/WordPress/gutenberg/pull/44403#discussion_r994415973
- }, [ registerSlot, unregisterSlot, name ] );
- // fillProps may be an update that interacts with the layout, so we
- // useLayoutEffect.
+ registry.registerSlot( name, ref, fillPropsRef.current );
+ return () => registry.unregisterSlot( name, ref );
+ }, [ registry, name ] );
+
useLayoutEffect( () => {
- registry.updateSlot( name, fillProps );
+ registry.updateSlot( name, ref, fillPropsRef.current );
} );
return (
diff --git a/packages/components/src/slot-fill/bubbles-virtually/use-slot.ts b/packages/components/src/slot-fill/bubbles-virtually/use-slot.ts
index d1d37e1d8e541..cac57a024e4ee 100644
--- a/packages/components/src/slot-fill/bubbles-virtually/use-slot.ts
+++ b/packages/components/src/slot-fill/bubbles-virtually/use-slot.ts
@@ -1,40 +1,17 @@
/**
* WordPress dependencies
*/
-import { useMemo, useContext } from '@wordpress/element';
+import { useContext } from '@wordpress/element';
import { useObservableValue } from '@wordpress/compose';
/**
* Internal dependencies
*/
import SlotFillContext from './slot-fill-context';
-import type {
- SlotFillBubblesVirtuallyFillRef,
- SlotFillBubblesVirtuallySlotRef,
- FillProps,
- SlotKey,
-} from '../types';
+import type { SlotKey } from '../types';
export default function useSlot( name: SlotKey ) {
const registry = useContext( SlotFillContext );
const slot = useObservableValue( registry.slots, name );
-
- const api = useMemo(
- () => ( {
- updateSlot: ( fillProps: FillProps ) =>
- registry.updateSlot( name, fillProps ),
- unregisterSlot: ( ref: SlotFillBubblesVirtuallySlotRef ) =>
- registry.unregisterSlot( name, ref ),
- registerFill: ( ref: SlotFillBubblesVirtuallyFillRef ) =>
- registry.registerFill( name, ref ),
- unregisterFill: ( ref: SlotFillBubblesVirtuallyFillRef ) =>
- registry.unregisterFill( name, ref ),
- } ),
- [ name, registry ]
- );
-
- return {
- ...slot,
- ...api,
- };
+ return { ...slot };
}
diff --git a/packages/components/src/slot-fill/context.ts b/packages/components/src/slot-fill/context.ts
index c4839462fbce0..b1f0718180e9e 100644
--- a/packages/components/src/slot-fill/context.ts
+++ b/packages/components/src/slot-fill/context.ts
@@ -1,20 +1,22 @@
/**
* WordPress dependencies
*/
+import { observableMap } from '@wordpress/compose';
import { createContext } from '@wordpress/element';
+
/**
* Internal dependencies
*/
import type { BaseSlotFillContext } from './types';
const initialValue: BaseSlotFillContext = {
+ slots: observableMap(),
+ fills: observableMap(),
registerSlot: () => {},
unregisterSlot: () => {},
registerFill: () => {},
unregisterFill: () => {},
- getSlot: () => undefined,
- getFills: () => [],
- subscribe: () => () => {},
+ updateFill: () => {},
};
export const SlotFillContext = createContext( initialValue );
diff --git a/packages/components/src/slot-fill/fill.ts b/packages/components/src/slot-fill/fill.ts
index 4134af25684b0..0bd1aec8fa3e0 100644
--- a/packages/components/src/slot-fill/fill.ts
+++ b/packages/components/src/slot-fill/fill.ts
@@ -7,46 +7,26 @@ import { useContext, useLayoutEffect, useRef } from '@wordpress/element';
* Internal dependencies
*/
import SlotFillContext from './context';
-import useSlot from './use-slot';
import type { FillComponentProps } from './types';
export default function Fill( { name, children }: FillComponentProps ) {
- const { registerFill, unregisterFill } = useContext( SlotFillContext );
- const slot = useSlot( name );
-
- const ref = useRef( {
- name,
- children,
- } );
+ const registry = useContext( SlotFillContext );
+ const instanceRef = useRef( {} );
+ const childrenRef = useRef( children );
useLayoutEffect( () => {
- const refValue = ref.current;
- registerFill( name, refValue );
- return () => unregisterFill( name, refValue );
- // The useLayoutEffects here are written to fire at specific times, and introducing new dependencies could cause unexpected changes in behavior.
- // We'll leave them as-is until a more detailed investigation/refactor can be performed.
- }, [] );
+ childrenRef.current = children;
+ }, [ children ] );
useLayoutEffect( () => {
- ref.current.children = children;
- if ( slot ) {
- slot.forceUpdate();
- }
- // The useLayoutEffects here are written to fire at specific times, and introducing new dependencies could cause unexpected changes in behavior.
- // We'll leave them as-is until a more detailed investigation/refactor can be performed.
- }, [ children ] );
+ const instance = instanceRef.current;
+ registry.registerFill( name, instance, childrenRef.current );
+ return () => registry.unregisterFill( name, instance );
+ }, [ registry, name ] );
useLayoutEffect( () => {
- if ( name === ref.current.name ) {
- // Ignore initial effect.
- return;
- }
- unregisterFill( ref.current.name, ref.current );
- ref.current.name = name;
- registerFill( name, ref.current );
- // The useLayoutEffects here are written to fire at specific times, and introducing new dependencies could cause unexpected changes in behavior.
- // We'll leave them as-is until a more detailed investigation/refactor can be performed.
- }, [ name ] );
+ registry.updateFill( name, instanceRef.current, childrenRef.current );
+ } );
return null;
}
diff --git a/packages/components/src/slot-fill/index.tsx b/packages/components/src/slot-fill/index.tsx
index 03ed33a67f13b..caf97091b67ac 100644
--- a/packages/components/src/slot-fill/index.tsx
+++ b/packages/components/src/slot-fill/index.tsx
@@ -84,17 +84,15 @@ export function createSlotFill( key: SlotKey ) {
props: DistributiveOmit< SlotComponentProps, 'name' >
) => ;
SlotComponent.displayName = `${ baseName }Slot`;
+ /**
+ * @deprecated 6.8.0
+ * Please use `slotFill.name` instead of `slotFill.Slot.__unstableName`.
+ */
SlotComponent.__unstableName = key;
return {
+ name: key,
Fill: FillComponent,
Slot: SlotComponent,
};
}
-
-export const createPrivateSlotFill = ( name: string ) => {
- const privateKey = Symbol( name );
- const privateSlotFill = createSlotFill( privateKey );
-
- return { privateKey, ...privateSlotFill };
-};
diff --git a/packages/components/src/slot-fill/provider.tsx b/packages/components/src/slot-fill/provider.tsx
index 6ed624bab67a3..e5319bc7f33e4 100644
--- a/packages/components/src/slot-fill/provider.tsx
+++ b/packages/components/src/slot-fill/provider.tsx
@@ -1,7 +1,6 @@
/**
* WordPress dependencies
*/
-import type { Component } from '@wordpress/element';
import { useState } from '@wordpress/element';
/**
@@ -9,111 +8,102 @@ import { useState } from '@wordpress/element';
*/
import SlotFillContext from './context';
import type {
- FillComponentProps,
+ FillInstance,
+ FillChildren,
+ BaseSlotInstance,
BaseSlotFillContext,
- BaseSlotComponentProps,
SlotFillProviderProps,
SlotKey,
} from './types';
+import { observableMap } from '@wordpress/compose';
function createSlotRegistry(): BaseSlotFillContext {
- const slots: Record< SlotKey, Component< BaseSlotComponentProps > > = {};
- const fills: Record< SlotKey, FillComponentProps[] > = {};
- let listeners: Array< () => void > = [];
-
- function registerSlot(
- name: SlotKey,
- slot: Component< BaseSlotComponentProps >
- ) {
- const previousSlot = slots[ name ];
- slots[ name ] = slot;
- triggerListeners();
-
- // Sometimes the fills are registered after the initial render of slot
- // But before the registerSlot call, we need to rerender the slot.
- forceUpdateSlot( name );
-
- // If a new instance of a slot is being mounted while another with the
- // same name exists, force its update _after_ the new slot has been
- // assigned into the instance, such that its own rendering of children
- // will be empty (the new Slot will subsume all fills for this name).
- if ( previousSlot ) {
- previousSlot.forceUpdate();
- }
- }
-
- function registerFill( name: SlotKey, instance: FillComponentProps ) {
- fills[ name ] = [ ...( fills[ name ] || [] ), instance ];
- forceUpdateSlot( name );
+ const slots = observableMap< SlotKey, BaseSlotInstance >();
+ const fills = observableMap<
+ SlotKey,
+ { instance: FillInstance; children: FillChildren }[]
+ >();
+
+ function registerSlot( name: SlotKey, instance: BaseSlotInstance ) {
+ slots.set( name, instance );
}
- function unregisterSlot(
- name: SlotKey,
- instance: Component< BaseSlotComponentProps >
- ) {
+ function unregisterSlot( name: SlotKey, instance: BaseSlotInstance ) {
// If a previous instance of a Slot by this name unmounts, do nothing,
// as the slot and its fills should only be removed for the current
// known instance.
- if ( slots[ name ] !== instance ) {
+ if ( slots.get( name ) !== instance ) {
return;
}
- delete slots[ name ];
- triggerListeners();
+ slots.delete( name );
}
- function unregisterFill( name: SlotKey, instance: FillComponentProps ) {
- fills[ name ] =
- fills[ name ]?.filter( ( fill ) => fill !== instance ) ?? [];
- forceUpdateSlot( name );
+ function registerFill(
+ name: SlotKey,
+ instance: FillInstance,
+ children: FillChildren
+ ) {
+ fills.set( name, [
+ ...( fills.get( name ) || [] ),
+ { instance, children },
+ ] );
}
- function getSlot(
- name: SlotKey
- ): Component< BaseSlotComponentProps > | undefined {
- return slots[ name ];
+ function unregisterFill( name: SlotKey, instance: FillInstance ) {
+ const fillsForName = fills.get( name );
+ if ( ! fillsForName ) {
+ return;
+ }
+
+ fills.set(
+ name,
+ fillsForName.filter( ( fill ) => fill.instance !== instance )
+ );
}
- function getFills(
+ function updateFill(
name: SlotKey,
- slotInstance: Component< BaseSlotComponentProps >
- ): FillComponentProps[] {
- // Fills should only be returned for the current instance of the slot
- // in which they occupy.
- if ( slots[ name ] !== slotInstance ) {
- return [];
+ instance: FillInstance,
+ children: FillChildren
+ ) {
+ const fillsForName = fills.get( name );
+ if ( ! fillsForName ) {
+ return;
}
- return fills[ name ];
- }
-
- function forceUpdateSlot( name: SlotKey ) {
- const slot = getSlot( name );
- if ( slot ) {
- slot.forceUpdate();
+ const fillForInstance = fillsForName.find(
+ ( f ) => f.instance === instance
+ );
+ if ( ! fillForInstance ) {
+ return;
}
- }
-
- function triggerListeners() {
- listeners.forEach( ( listener ) => listener() );
- }
- function subscribe( listener: () => void ) {
- listeners.push( listener );
+ if ( fillForInstance.children === children ) {
+ return;
+ }
- return () => {
- listeners = listeners.filter( ( l ) => l !== listener );
- };
+ fills.set(
+ name,
+ fillsForName.map( ( f ) => {
+ if ( f.instance === instance ) {
+ // Replace with new record with updated `children`.
+ return { instance, children };
+ }
+
+ return f;
+ } )
+ );
}
return {
+ slots,
+ fills,
registerSlot,
unregisterSlot,
registerFill,
unregisterFill,
- getSlot,
- getFills,
- subscribe,
+ updateFill,
};
}
diff --git a/packages/components/src/slot-fill/slot.tsx b/packages/components/src/slot-fill/slot.tsx
index 3fe2a54935926..82feaa04199f5 100644
--- a/packages/components/src/slot-fill/slot.tsx
+++ b/packages/components/src/slot-fill/slot.tsx
@@ -6,9 +6,12 @@ import type { ReactElement, ReactNode, Key } from 'react';
/**
* WordPress dependencies
*/
+import { useObservableValue } from '@wordpress/compose';
import {
+ useContext,
+ useEffect,
+ useRef,
Children,
- Component,
cloneElement,
isEmptyElement,
} from '@wordpress/element';
@@ -17,7 +20,7 @@ import {
* Internal dependencies
*/
import SlotFillContext from './context';
-import type { BaseSlotComponentProps, SlotComponentProps } from './types';
+import type { SlotComponentProps } from './types';
/**
* Whether the argument is a function.
@@ -29,90 +32,63 @@ function isFunction( maybeFunc: any ): maybeFunc is Function {
return typeof maybeFunc === 'function';
}
-class SlotComponent extends Component< BaseSlotComponentProps > {
- private isUnmounted: boolean;
-
- constructor( props: BaseSlotComponentProps ) {
- super( props );
+function addKeysToChildren( children: ReactNode ) {
+ return Children.map( children, ( child, childIndex ) => {
+ if ( ! child || typeof child === 'string' ) {
+ return child;
+ }
+ let childKey: Key = childIndex;
+ if ( typeof child === 'object' && 'key' in child && child?.key ) {
+ childKey = child.key;
+ }
- this.isUnmounted = false;
- }
+ return cloneElement( child as ReactElement, {
+ key: childKey,
+ } );
+ } );
+}
- componentDidMount() {
- const { registerSlot } = this.props;
- this.isUnmounted = false;
- registerSlot( this.props.name, this );
- }
+function Slot( props: Omit< SlotComponentProps, 'bubblesVirtually' > ) {
+ const registry = useContext( SlotFillContext );
+ const instanceRef = useRef( {} );
- componentWillUnmount() {
- const { unregisterSlot } = this.props;
- this.isUnmounted = true;
- unregisterSlot( this.props.name, this );
- }
+ const { name, children, fillProps = {} } = props;
- componentDidUpdate( prevProps: BaseSlotComponentProps ) {
- const { name, unregisterSlot, registerSlot } = this.props;
+ useEffect( () => {
+ const instance = instanceRef.current;
+ registry.registerSlot( name, instance );
+ return () => registry.unregisterSlot( name, instance );
+ }, [ registry, name ] );
- if ( prevProps.name !== name ) {
- unregisterSlot( prevProps.name, this );
- registerSlot( name, this );
- }
- }
+ let fills = useObservableValue( registry.fills, name ) ?? [];
+ const currentSlot = useObservableValue( registry.slots, name );
- forceUpdate() {
- if ( this.isUnmounted ) {
- return;
- }
- super.forceUpdate();
+ // Fills should only be rendered in the currently registered instance of the slot.
+ if ( currentSlot !== instanceRef.current ) {
+ fills = [];
}
- render() {
- const { children, name, fillProps = {}, getFills } = this.props;
- const fills: ReactNode[] = ( getFills( name, this ) ?? [] )
- .map( ( fill ) => {
- const fillChildren = isFunction( fill.children )
- ? fill.children( fillProps )
- : fill.children;
- return Children.map( fillChildren, ( child, childIndex ) => {
- if ( ! child || typeof child === 'string' ) {
- return child;
- }
- let childKey: Key = childIndex;
- if (
- typeof child === 'object' &&
- 'key' in child &&
- child?.key
- ) {
- childKey = child.key;
- }
-
- return cloneElement( child as ReactElement, {
- key: childKey,
- } );
- } );
- } )
- .filter(
- // In some cases fills are rendered only when some conditions apply.
- // This ensures that we only use non-empty fills when rendering, i.e.,
- // it allows us to render wrappers only when the fills are actually present.
- ( element ) => ! isEmptyElement( element )
- );
-
- return <>{ isFunction( children ) ? children( fills ) : fills }>;
- }
+ const renderedFills = fills
+ .map( ( fill ) => {
+ const fillChildren = isFunction( fill.children )
+ ? fill.children( fillProps )
+ : fill.children;
+ return addKeysToChildren( fillChildren );
+ } )
+ .filter(
+ // In some cases fills are rendered only when some conditions apply.
+ // This ensures that we only use non-empty fills when rendering, i.e.,
+ // it allows us to render wrappers only when the fills are actually present.
+ ( element ) => ! isEmptyElement( element )
+ );
+
+ return (
+ <>
+ { isFunction( children )
+ ? children( renderedFills )
+ : renderedFills }
+ >
+ );
}
-const Slot = ( props: Omit< SlotComponentProps, 'bubblesVirtually' > ) => (
-
- { ( { registerSlot, unregisterSlot, getFills } ) => (
-
- ) }
-
-);
-
export default Slot;
diff --git a/packages/components/src/slot-fill/stories/index.story.tsx b/packages/components/src/slot-fill/stories/index.story.tsx
index bc6c4f57ad9ce..2c74496e1eada 100644
--- a/packages/components/src/slot-fill/stories/index.story.tsx
+++ b/packages/components/src/slot-fill/stories/index.story.tsx
@@ -20,9 +20,9 @@ const meta: Meta< typeof Slot > = {
// @ts-expect-error - See https://github.com/storybookjs/storybook/issues/23170
subcomponents: { Fill, SlotFillProvider },
argTypes: {
- name: { control: { type: null } },
+ name: { control: false },
as: { control: { type: 'text' } },
- fillProps: { control: { type: null } },
+ fillProps: { control: false },
},
parameters: {
controls: { expanded: true },
diff --git a/packages/components/src/slot-fill/types.ts b/packages/components/src/slot-fill/types.ts
index 1711e04cbb1f4..758f1c8257d54 100644
--- a/packages/components/src/slot-fill/types.ts
+++ b/packages/components/src/slot-fill/types.ts
@@ -1,7 +1,7 @@
/**
* External dependencies
*/
-import type { Component, MutableRefObject, ReactNode, RefObject } from 'react';
+import type { ReactNode, RefObject } from 'react';
/**
* WordPress dependencies
@@ -84,6 +84,10 @@ export type SlotComponentProps =
style?: never;
} );
+export type FillChildren =
+ | ReactNode
+ | ( ( fillProps: FillProps ) => ReactNode );
+
export type FillComponentProps = {
/**
* The name of the slot to fill into.
@@ -93,7 +97,7 @@ export type FillComponentProps = {
/**
* Children elements or render function.
*/
- children?: ReactNode | ( ( fillProps: FillProps ) => ReactNode );
+ children?: FillChildren;
};
export type SlotFillProviderProps = {
@@ -108,38 +112,18 @@ export type SlotFillProviderProps = {
passthrough?: boolean;
};
-export type SlotFillBubblesVirtuallySlotRef = RefObject< HTMLElement >;
-export type SlotFillBubblesVirtuallyFillRef = MutableRefObject< {
- rerender: () => void;
-} >;
+export type SlotRef = RefObject< HTMLElement >;
+export type FillInstance = {};
+export type BaseSlotInstance = {};
export type SlotFillBubblesVirtuallyContext = {
- slots: ObservableMap<
- SlotKey,
- {
- ref: SlotFillBubblesVirtuallySlotRef;
- fillProps: FillProps;
- }
- >;
- fills: ObservableMap< SlotKey, SlotFillBubblesVirtuallyFillRef[] >;
- registerSlot: (
- name: SlotKey,
- ref: SlotFillBubblesVirtuallySlotRef,
- fillProps: FillProps
- ) => void;
- unregisterSlot: (
- name: SlotKey,
- ref: SlotFillBubblesVirtuallySlotRef
- ) => void;
- updateSlot: ( name: SlotKey, fillProps: FillProps ) => void;
- registerFill: (
- name: SlotKey,
- ref: SlotFillBubblesVirtuallyFillRef
- ) => void;
- unregisterFill: (
- name: SlotKey,
- ref: SlotFillBubblesVirtuallyFillRef
- ) => void;
+ slots: ObservableMap< SlotKey, { ref: SlotRef; fillProps: FillProps } >;
+ fills: ObservableMap< SlotKey, FillInstance[] >;
+ registerSlot: ( name: SlotKey, ref: SlotRef, fillProps: FillProps ) => void;
+ unregisterSlot: ( name: SlotKey, ref: SlotRef ) => void;
+ updateSlot: ( name: SlotKey, ref: SlotRef, fillProps: FillProps ) => void;
+ registerFill: ( name: SlotKey, instance: FillInstance ) => void;
+ unregisterFill: ( name: SlotKey, instance: FillInstance ) => void;
/**
* This helps the provider know if it's using the default context value or not.
@@ -148,30 +132,22 @@ export type SlotFillBubblesVirtuallyContext = {
};
export type BaseSlotFillContext = {
- registerSlot: (
+ slots: ObservableMap< SlotKey, BaseSlotInstance >;
+ fills: ObservableMap<
+ SlotKey,
+ { instance: FillInstance; children: FillChildren }[]
+ >;
+ registerSlot: ( name: SlotKey, slot: BaseSlotInstance ) => void;
+ unregisterSlot: ( name: SlotKey, slot: BaseSlotInstance ) => void;
+ registerFill: (
name: SlotKey,
- slot: Component< BaseSlotComponentProps >
+ instance: FillInstance,
+ children: FillChildren
) => void;
- unregisterSlot: (
+ unregisterFill: ( name: SlotKey, instance: FillInstance ) => void;
+ updateFill: (
name: SlotKey,
- slot: Component< BaseSlotComponentProps >
+ instance: FillInstance,
+ children: FillChildren
) => void;
- registerFill: ( name: SlotKey, instance: FillComponentProps ) => void;
- unregisterFill: ( name: SlotKey, instance: FillComponentProps ) => void;
- getSlot: (
- name: SlotKey
- ) => Component< BaseSlotComponentProps > | undefined;
- getFills: (
- name: SlotKey,
- slotInstance: Component< BaseSlotComponentProps >
- ) => FillComponentProps[];
- subscribe: ( listener: () => void ) => () => void;
};
-
-export type BaseSlotComponentProps = Pick<
- BaseSlotFillContext,
- 'registerSlot' | 'unregisterSlot' | 'getFills'
-> &
- Omit< SlotComponentProps, 'bubblesVirtually' > & {
- children?: ( fills: ReactNode ) => ReactNode;
- };
diff --git a/packages/components/src/slot-fill/use-slot.ts b/packages/components/src/slot-fill/use-slot.ts
deleted file mode 100644
index 4ab419be1ad2b..0000000000000
--- a/packages/components/src/slot-fill/use-slot.ts
+++ /dev/null
@@ -1,27 +0,0 @@
-/**
- * WordPress dependencies
- */
-import { useContext, useSyncExternalStore } from '@wordpress/element';
-
-/**
- * Internal dependencies
- */
-import SlotFillContext from './context';
-import type { SlotKey } from './types';
-
-/**
- * React hook returning the active slot given a name.
- *
- * @param name Slot name.
- * @return Slot object.
- */
-const useSlot = ( name: SlotKey ) => {
- const { getSlot, subscribe } = useContext( SlotFillContext );
- return useSyncExternalStore(
- subscribe,
- () => getSlot( name ),
- () => getSlot( name )
- );
-};
-
-export default useSlot;
diff --git a/packages/components/src/snackbar/stories/index.story.tsx b/packages/components/src/snackbar/stories/index.story.tsx
index 9bd1dae42b71b..5c24044cc1ae6 100644
--- a/packages/components/src/snackbar/stories/index.story.tsx
+++ b/packages/components/src/snackbar/stories/index.story.tsx
@@ -19,17 +19,17 @@ const meta: Meta< typeof Snackbar > = {
id: 'components-snackbar',
component: Snackbar,
argTypes: {
- as: { control: { type: null } },
+ as: { control: false },
onRemove: {
action: 'onRemove',
- control: { type: null },
+ control: false,
},
onDismiss: {
action: 'onDismiss',
- control: { type: null },
+ control: false,
},
listRef: {
- control: { type: null },
+ control: false,
},
},
parameters: {
diff --git a/packages/components/src/snackbar/stories/list.story.tsx b/packages/components/src/snackbar/stories/list.story.tsx
index 1f93f374ec174..69a4367c48575 100644
--- a/packages/components/src/snackbar/stories/list.story.tsx
+++ b/packages/components/src/snackbar/stories/list.story.tsx
@@ -18,10 +18,10 @@ const meta: Meta< typeof SnackbarList > = {
id: 'components-snackbarlist',
component: SnackbarList,
argTypes: {
- as: { control: { type: null } },
+ as: { control: false },
onRemove: {
action: 'onRemove',
- control: { type: null },
+ control: false,
},
},
parameters: {
diff --git a/packages/components/src/style.scss b/packages/components/src/style.scss
index 70317f4a2d0e0..368dec0f5e253 100644
--- a/packages/components/src/style.scss
+++ b/packages/components/src/style.scss
@@ -10,6 +10,7 @@
// Components
@import "./animate/style.scss";
@import "./autocomplete/style.scss";
+@import "./badge/styles.scss";
@import "./button-group/style.scss";
@import "./button/style.scss";
@import "./checkbox-control/style.scss";
diff --git a/packages/components/src/surface/stories/index.story.tsx b/packages/components/src/surface/stories/index.story.tsx
index 7f6790d09c848..1ef0c0d5637cb 100644
--- a/packages/components/src/surface/stories/index.story.tsx
+++ b/packages/components/src/surface/stories/index.story.tsx
@@ -13,7 +13,7 @@ const meta: Meta< typeof Surface > = {
component: Surface,
title: 'Components (Experimental)/Surface',
argTypes: {
- children: { control: { type: null } },
+ children: { control: false },
as: { control: { type: 'text' } },
},
parameters: {
diff --git a/packages/components/src/tab-panel/index.tsx b/packages/components/src/tab-panel/index.tsx
index be06b42fcd013..ec4f33d875a38 100644
--- a/packages/components/src/tab-panel/index.tsx
+++ b/packages/components/src/tab-panel/index.tsx
@@ -2,7 +2,6 @@
* External dependencies
*/
import * as Ariakit from '@ariakit/react';
-import { useStoreState } from '@ariakit/react';
import clsx from 'clsx';
import type { ForwardedRef } from 'react';
@@ -125,7 +124,7 @@ const UnforwardedTabPanel = (
} );
const selectedTabName = extractTabName(
- useStoreState( tabStore, 'selectedId' )
+ Ariakit.useStoreState( tabStore, 'selectedId' )
);
const setTabStoreSelectedId = useCallback(
diff --git a/packages/components/src/tab-panel/stories/index.story.tsx b/packages/components/src/tab-panel/stories/index.story.tsx
index 57a3cc311f863..8f40c61beb523 100644
--- a/packages/components/src/tab-panel/stories/index.story.tsx
+++ b/packages/components/src/tab-panel/stories/index.story.tsx
@@ -2,6 +2,7 @@
* External dependencies
*/
import type { Meta, StoryFn } from '@storybook/react';
+import { fn } from '@storybook/test';
/**
* WordPress dependencies
@@ -22,6 +23,9 @@ const meta: Meta< typeof TabPanel > = {
controls: { expanded: true },
docs: { canvas: { sourceState: 'shown' } },
},
+ args: {
+ onSelect: fn(),
+ },
};
export default meta;
diff --git a/packages/components/src/tabs/stories/index.story.tsx b/packages/components/src/tabs/stories/index.story.tsx
index 5b2fd621bbb43..e434bb501d85c 100644
--- a/packages/components/src/tabs/stories/index.story.tsx
+++ b/packages/components/src/tabs/stories/index.story.tsx
@@ -2,6 +2,7 @@
* External dependencies
*/
import type { Meta, StoryFn } from '@storybook/react';
+import { fn } from '@storybook/test';
/**
* WordPress dependencies
@@ -39,6 +40,10 @@ const meta: Meta< typeof Tabs > = {
controls: { expanded: true },
docs: { canvas: { sourceState: 'shown' } },
},
+ args: {
+ onActiveTabIdChange: fn(),
+ onSelect: fn(),
+ },
};
export default meta;
diff --git a/packages/components/src/tabs/tab.tsx b/packages/components/src/tabs/tab.tsx
index 70f56e52ad262..8226d0589f08c 100644
--- a/packages/components/src/tabs/tab.tsx
+++ b/packages/components/src/tabs/tab.tsx
@@ -1,8 +1,3 @@
-/**
- * External dependencies
- */
-import * as Ariakit from '@ariakit/react';
-
/**
* WordPress dependencies
*/
@@ -29,18 +24,6 @@ export const Tab = forwardRef<
>( function Tab( { children, tabId, disabled, render, ...otherProps }, ref ) {
const { store, instanceId } = useTabsContext() ?? {};
- // If the active item is not connected, the tablist may end up in a state
- // where none of the tabs are tabbable. In this case, we force all tabs to
- // be tabbable, so that as soon as an item received focus, it becomes active
- // and Tablist goes back to working as expected.
- // eslint-disable-next-line @wordpress/no-unused-vars-before-return
- const tabbable = Ariakit.useStoreState( store, ( state ) => {
- return (
- state?.activeId !== null &&
- ! store?.item( state?.activeId )?.element?.isConnected
- );
- } );
-
if ( ! store ) {
warning( '`Tabs.Tab` must be wrapped in a `Tabs` component.' );
return null;
@@ -55,7 +38,6 @@ export const Tab = forwardRef<
id={ instancedTabId }
disabled={ disabled }
render={ render }
- tabbable={ tabbable }
{ ...otherProps }
>
{ children }
diff --git a/packages/components/src/tabs/test/index.tsx b/packages/components/src/tabs/test/index.tsx
index dcf64102c9fa6..fd9ceb38190a7 100644
--- a/packages/components/src/tabs/test/index.tsx
+++ b/packages/components/src/tabs/test/index.tsx
@@ -9,6 +9,7 @@ import { render } from '@ariakit/test/react';
* WordPress dependencies
*/
import { useEffect, useState } from '@wordpress/element';
+import { isRTL } from '@wordpress/i18n';
/**
* Internal dependencies
@@ -16,6 +17,16 @@ import { useEffect, useState } from '@wordpress/element';
import { Tabs } from '..';
import type { TabsProps } from '../types';
+// Setup mocking the `isRTL` function to test arrow key navigation behavior.
+jest.mock( '@wordpress/i18n', () => {
+ const original = jest.requireActual( '@wordpress/i18n' );
+ return {
+ ...original,
+ isRTL: jest.fn( () => false ),
+ };
+} );
+const mockedIsRTL = isRTL as jest.Mock;
+
type Tab = {
tabId: string;
title: string;
@@ -50,6 +61,30 @@ const TABS: Tab[] = [
},
];
+const TABS_WITH_ALPHA_DISABLED = TABS.map( ( tabObj ) =>
+ tabObj.tabId === 'alpha'
+ ? {
+ ...tabObj,
+ tab: {
+ ...tabObj.tab,
+ disabled: true,
+ },
+ }
+ : tabObj
+);
+
+const TABS_WITH_BETA_DISABLED = TABS.map( ( tabObj ) =>
+ tabObj.tabId === 'beta'
+ ? {
+ ...tabObj,
+ tab: {
+ ...tabObj.tab,
+ disabled: true,
+ },
+ }
+ : tabObj
+);
+
const TABS_WITH_DELTA: Tab[] = [
...TABS,
{
@@ -141,11 +176,47 @@ const ControlledTabs = ( {
);
};
-const getSelectedTab = async () =>
- await screen.findByRole( 'tab', { selected: true } );
-
let originalGetClientRects: () => DOMRectList;
+async function waitForComponentToBeInitializedWithSelectedTab(
+ selectedTabName: string | undefined
+) {
+ if ( ! selectedTabName ) {
+ // Wait for the tablist to be tabbable as a mean to know
+ // that ariakit has finished initializing.
+ await waitFor( () =>
+ expect( screen.getByRole( 'tablist' ) ).toHaveAttribute(
+ 'tabindex',
+ expect.stringMatching( /^(0|-1)$/ )
+ )
+ );
+ // No initially selected tabs or tabpanels.
+ await waitFor( () =>
+ expect(
+ screen.queryByRole( 'tab', { selected: true } )
+ ).not.toBeInTheDocument()
+ );
+ await waitFor( () =>
+ expect( screen.queryByRole( 'tabpanel' ) ).not.toBeInTheDocument()
+ );
+ } else {
+ // Waiting for a tab to be selected is a sign that the component
+ // has fully initialized.
+ expect(
+ await screen.findByRole( 'tab', {
+ selected: true,
+ name: selectedTabName,
+ } )
+ ).toBeVisible();
+ // The corresponding tabpanel is also shown.
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: selectedTabName,
+ } )
+ ).toBeVisible();
+ }
+}
+
describe( 'Tabs', () => {
beforeAll( () => {
originalGetClientRects = window.HTMLElement.prototype.getClientRects;
@@ -162,13 +233,16 @@ describe( 'Tabs', () => {
window.HTMLElement.prototype.getClientRects = originalGetClientRects;
} );
- describe( 'Accessibility and semantics', () => {
- it( 'should use the correct aria attributes', async () => {
+ describe( 'Adherence to spec and basic behavior', () => {
+ it( 'should apply the correct roles, semantics and attributes', async () => {
await render( );
+ // Alpha is automatically selected as the selected tab.
+ await waitForComponentToBeInitializedWithSelectedTab( 'Alpha' );
+
const tabList = screen.getByRole( 'tablist' );
const allTabs = screen.getAllByRole( 'tab' );
- const selectedTabPanel = await screen.findByRole( 'tabpanel' );
+ const allTabpanels = screen.getAllByRole( 'tabpanel' );
expect( tabList ).toBeVisible();
expect( tabList ).toHaveAttribute(
@@ -178,133 +252,103 @@ describe( 'Tabs', () => {
expect( allTabs ).toHaveLength( TABS.length );
- // The selected `tab` aria-controls the active `tabpanel`,
- // which is `aria-labelledby` the selected `tab`.
- expect( selectedTabPanel ).toBeVisible();
+ // Only 1 tab panel is accessible — the one associated with the
+ // selected tab. The selected `tab` aria-controls the active
+ /// `tabpanel`, which is `aria-labelledby` the selected `tab`.
+ expect( allTabpanels ).toHaveLength( 1 );
+
+ expect( allTabpanels[ 0 ] ).toBeVisible();
expect( allTabs[ 0 ] ).toHaveAttribute(
'aria-controls',
- selectedTabPanel.getAttribute( 'id' )
+ allTabpanels[ 0 ].getAttribute( 'id' )
);
- expect( selectedTabPanel ).toHaveAttribute(
+ expect( allTabpanels[ 0 ] ).toHaveAttribute(
'aria-labelledby',
allTabs[ 0 ].getAttribute( 'id' )
);
} );
- } );
- describe( 'Focus Behavior', () => {
- it( 'should focus on the related TabPanel when pressing the Tab key', async () => {
- await render( );
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
-
- const selectedTabPanel = await screen.findByRole( 'tabpanel' );
-
- // Tab should initially focus the first tab in the tablist, which
- // is Alpha.
- await press.Tab();
- expect(
- await screen.findByRole( 'tab', { name: 'Alpha' } )
- ).toHaveFocus();
-
- // By default the tabpanel should receive focus
- await press.Tab();
- expect( selectedTabPanel ).toHaveFocus();
- } );
- it( 'should not focus on the related TabPanel when pressing the Tab key if `focusable: false` is set', async () => {
- const TABS_WITH_ALPHA_FOCUSABLE_FALSE = TABS.map( ( tabObj ) =>
- tabObj.tabId === 'alpha'
- ? {
- ...tabObj,
- content: (
- <>
- Selected Tab: Alpha
- Alpha Button
- >
- ),
- tabpanel: { focusable: false },
- }
- : tabObj
- );
+ it( 'should associate each `tab` with the correct `tabpanel`, even if they are not rendered in the same order', async () => {
+ const TABS_WITH_DELTA_REVERSED = [ ...TABS_WITH_DELTA ].reverse();
await render(
-
+
+
+ { TABS_WITH_DELTA.map( ( tabObj ) => (
+
+ { tabObj.title }
+
+ ) ) }
+
+ { TABS_WITH_DELTA_REVERSED.map( ( tabObj ) => (
+
+ { tabObj.content }
+
+ ) ) }
+
);
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
-
- const alphaButton = await screen.findByRole( 'button', {
- name: /alpha button/i,
- } );
+ // Alpha is automatically selected as the selected tab.
+ await waitForComponentToBeInitializedWithSelectedTab( 'Alpha' );
- // Tab should initially focus the first tab in the tablist, which
- // is Alpha.
- await press.Tab();
+ // Select Beta, make sure the correct tabpanel is rendered
+ await click( screen.getByRole( 'tab', { name: 'Beta' } ) );
expect(
- await screen.findByRole( 'tab', { name: 'Alpha' } )
- ).toHaveFocus();
- // Because the alpha tabpanel is set to `focusable: false`, pressing
- // the Tab key should focus the button, not the tabpanel
- await press.Tab();
- expect( alphaButton ).toHaveFocus();
- } );
-
- it( "should focus the first tab, even if disabled, when the current selected tab id doesn't match an existing one", async () => {
- const TABS_WITH_ALPHA_DISABLED = TABS.map( ( tabObj ) =>
- tabObj.tabId === 'alpha'
- ? {
- ...tabObj,
- tab: {
- ...tabObj.tab,
- disabled: true,
- },
- }
- : tabObj
- );
-
- await render(
-
- );
-
- // No tab should be selected i.e. it doesn't fall back to first tab.
- await waitFor( () =>
- expect(
- screen.queryByRole( 'tab', { selected: true } )
- ).not.toBeInTheDocument()
- );
-
- // No tabpanel should be rendered either
- expect( screen.queryByRole( 'tabpanel' ) ).not.toBeInTheDocument();
-
- await press.Tab();
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Beta',
+ } )
+ ).toBeVisible();
expect(
- await screen.findByRole( 'tab', { name: 'Alpha' } )
- ).toHaveFocus();
+ screen.getByRole( 'tabpanel', {
+ name: 'Beta',
+ } )
+ ).toBeVisible();
- await press.ArrowRight();
+ // Select Gamma, make sure the correct tabpanel is rendered
+ await click( screen.getByRole( 'tab', { name: 'Gamma' } ) );
expect(
- await screen.findByRole( 'tab', { name: 'Beta' } )
- ).toHaveFocus();
-
- await press.ArrowRight();
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Gamma',
+ } )
+ ).toBeVisible();
expect(
- await screen.findByRole( 'tab', { name: 'Gamma' } )
- ).toHaveFocus();
+ screen.getByRole( 'tabpanel', {
+ name: 'Gamma',
+ } )
+ ).toBeVisible();
- await press.Tab();
- await press.ShiftTab();
+ // Select Delta, make sure the correct tabpanel is rendered
+ await click( screen.getByRole( 'tab', { name: 'Delta' } ) );
+ expect(
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Delta',
+ } )
+ ).toBeVisible();
expect(
- await screen.findByRole( 'tab', { name: 'Gamma' } )
- ).toHaveFocus();
+ screen.getByRole( 'tabpanel', {
+ name: 'Delta',
+ } )
+ ).toBeVisible();
} );
- } );
- describe( 'Tab Attributes', () => {
it( "should apply the tab's `className` to the tab button", async () => {
await render( );
+ // Alpha is automatically selected as the selected tab.
+ await waitForComponentToBeInitializedWithSelectedTab( 'Alpha' );
+
expect(
await screen.findByRole( 'tab', { name: 'Alpha' } )
).toHaveClass( 'alpha-class' );
@@ -317,908 +361,1076 @@ describe( 'Tabs', () => {
} );
} );
- describe( 'Tab Activation', () => {
- it( 'defaults to automatic tab activation (pointer clicks)', async () => {
+ describe( 'pointer interactions', () => {
+ it( 'should select a tab when clicked', async () => {
const mockOnSelect = jest.fn();
await render(
);
- // Alpha is the initially selected tab
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
- expect(
- await screen.findByRole( 'tabpanel', { name: 'Alpha' } )
- ).toBeInTheDocument();
+ // Alpha is automatically selected as the selected tab.
+ await waitForComponentToBeInitializedWithSelectedTab( 'Alpha' );
+
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
expect( mockOnSelect ).toHaveBeenLastCalledWith( 'alpha' );
// Click on Beta, make sure beta is the selected tab
await click( screen.getByRole( 'tab', { name: 'Beta' } ) );
- expect( await getSelectedTab() ).toHaveTextContent( 'Beta' );
expect(
- screen.getByRole( 'tabpanel', { name: 'Beta' } )
- ).toBeInTheDocument();
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Beta',
+ } )
+ ).toBeVisible();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Beta',
+ } )
+ ).toBeVisible();
+
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 2 );
expect( mockOnSelect ).toHaveBeenLastCalledWith( 'beta' );
- // Click on Alpha, make sure beta is the selected tab
+ // Click on Alpha, make sure alpha is the selected tab
await click( screen.getByRole( 'tab', { name: 'Alpha' } ) );
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
expect(
- screen.getByRole( 'tabpanel', { name: 'Alpha' } )
- ).toBeInTheDocument();
- expect( mockOnSelect ).toHaveBeenLastCalledWith( 'alpha' );
- } );
-
- it( 'defaults to automatic tab activation (arrow keys)', async () => {
- const mockOnSelect = jest.fn();
-
- await render(
-
- );
-
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
-
- // onSelect gets called on the initial render. It should be called
- // with the first enabled tab, which is alpha.
- expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
- expect( mockOnSelect ).toHaveBeenLastCalledWith( 'alpha' );
-
- // Tab to focus the tablist. Make sure alpha is focused.
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
- expect( await getSelectedTab() ).not.toHaveFocus();
- await press.Tab();
- expect( await getSelectedTab() ).toHaveFocus();
-
- // Navigate forward with arrow keys and make sure the Beta tab is
- // selected automatically.
- await press.ArrowRight();
- expect( await getSelectedTab() ).toHaveTextContent( 'Beta' );
- expect( await getSelectedTab() ).toHaveFocus();
- expect( mockOnSelect ).toHaveBeenCalledTimes( 2 );
- expect( mockOnSelect ).toHaveBeenLastCalledWith( 'beta' );
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Alpha',
+ } )
+ ).toBeVisible();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Alpha',
+ } )
+ ).toBeVisible();
- // Navigate backwards with arrow keys. Make sure alpha is
- // selected automatically.
- await press.ArrowLeft();
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
- expect( await getSelectedTab() ).toHaveFocus();
expect( mockOnSelect ).toHaveBeenCalledTimes( 3 );
expect( mockOnSelect ).toHaveBeenLastCalledWith( 'alpha' );
} );
- it( 'wraps around the last/first tab when using arrow keys', async () => {
+ it( 'should not select a disabled tab when clicked', async () => {
const mockOnSelect = jest.fn();
await render(
-
+
);
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
- expect( await getSelectedTab() ).not.toHaveFocus();
+ // Alpha is automatically selected as the selected tab.
+ await waitForComponentToBeInitializedWithSelectedTab( 'Alpha' );
- // onSelect gets called on the initial render.
expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
expect( mockOnSelect ).toHaveBeenLastCalledWith( 'alpha' );
- // Tab to focus the tablist. Make sure Alpha is focused.
- await press.Tab();
- expect( await getSelectedTab() ).toHaveFocus();
+ // Clicking on Beta does not result in beta being selected
+ // because the tab is disabled.
+ await click( screen.getByRole( 'tab', { name: 'Beta' } ) );
- // Navigate backwards with arrow keys and make sure that the Gamma tab
- // (the last tab) is selected automatically.
- await press.ArrowLeft();
- expect( await getSelectedTab() ).toHaveTextContent( 'Gamma' );
- expect( await getSelectedTab() ).toHaveFocus();
- expect( mockOnSelect ).toHaveBeenCalledTimes( 2 );
- expect( mockOnSelect ).toHaveBeenLastCalledWith( 'gamma' );
+ expect(
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Alpha',
+ } )
+ ).toBeVisible();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Alpha',
+ } )
+ ).toBeVisible();
- // Navigate forward with arrow keys. Make sure alpha (the first tab) is
- // selected automatically.
- await press.ArrowRight();
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
- expect( await getSelectedTab() ).toHaveFocus();
- expect( mockOnSelect ).toHaveBeenCalledTimes( 3 );
- expect( mockOnSelect ).toHaveBeenLastCalledWith( 'alpha' );
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
} );
+ } );
- it( 'should not move tab selection when pressing the up/down arrow keys, unless the orientation is changed to `vertical`', async () => {
- const mockOnSelect = jest.fn();
+ describe( 'initial tab selection', () => {
+ describe( 'when a selected tab id is not specified', () => {
+ describe( 'when left `undefined` [Uncontrolled]', () => {
+ it( 'should choose the first tab as selected', async () => {
+ await render( );
- const { rerender } = await render(
-
- );
+ // Alpha is automatically selected as the selected tab.
+ await waitForComponentToBeInitializedWithSelectedTab(
+ 'Alpha'
+ );
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
- expect( await getSelectedTab() ).not.toHaveFocus();
+ // Press tab. The selected tab (alpha) received focus.
+ await press.Tab();
+ expect(
+ await screen.findByRole( 'tab', {
+ selected: true,
+ name: 'Alpha',
+ } )
+ ).toHaveFocus();
+ } );
+
+ it( 'should choose the first non-disabled tab if the first tab is disabled', async () => {
+ await render(
+
+ );
+
+ // Beta is automatically selected as the selected tab, since alpha is
+ // disabled.
+ await waitForComponentToBeInitializedWithSelectedTab(
+ 'Beta'
+ );
+
+ // Press tab. The selected tab (beta) received focus. The corresponding
+ // tabpanel is shown.
+ await press.Tab();
+ expect(
+ await screen.findByRole( 'tab', {
+ selected: true,
+ name: 'Beta',
+ } )
+ ).toHaveFocus();
+ } );
+ } );
+ describe( 'when `null` [Controlled]', () => {
+ it( 'should not have a selected tab nor show any tabpanels, make the tablist tabbable and still allow selecting tabs', async () => {
+ await render(
+
+ );
+
+ // No initially selected tabs or tabpanels.
+ await waitForComponentToBeInitializedWithSelectedTab(
+ undefined
+ );
+
+ // Press tab. The tablist receives focus
+ await press.Tab();
+ expect(
+ await screen.findByRole( 'tablist' )
+ ).toHaveFocus();
- // onSelect gets called on the initial render.
- expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
- expect( mockOnSelect ).toHaveBeenLastCalledWith( 'alpha' );
+ // Press right arrow to select the first tab (alpha) and
+ // show the related tabpanel.
+ await press.ArrowRight();
+ expect(
+ await screen.findByRole( 'tab', {
+ selected: true,
+ name: 'Alpha',
+ } )
+ ).toHaveFocus();
+ expect(
+ await screen.findByRole( 'tabpanel', {
+ name: 'Alpha',
+ } )
+ ).toBeVisible();
+ } );
+ } );
+ } );
- // Tab to focus the tablist. Make sure Alpha is focused.
- await press.Tab();
- expect( await getSelectedTab() ).toHaveFocus();
+ describe( 'when a selected tab id is specified', () => {
+ describe( 'through the `defaultTabId` prop [Uncontrolled]', () => {
+ it( 'should select the initial tab matching the `defaultTabId` prop', async () => {
+ await render(
+
+ );
+
+ // Beta is the initially selected tab
+ await waitForComponentToBeInitializedWithSelectedTab(
+ 'Beta'
+ );
+
+ // Press tab. The selected tab (beta) received focus. The corresponding
+ // tabpanel is shown.
+ await press.Tab();
+ expect(
+ await screen.findByRole( 'tab', {
+ selected: true,
+ name: 'Beta',
+ } )
+ ).toHaveFocus();
+ } );
+
+ it( 'should select the initial tab matching the `defaultTabId` prop even if the tab is disabled', async () => {
+ await render(
+
+ );
+
+ // Beta is automatically selected as the selected tab despite being
+ // disabled, respecting the `defaultTabId` prop.
+ await waitForComponentToBeInitializedWithSelectedTab(
+ 'Beta'
+ );
+
+ // Press tab. The selected tab (beta) received focus, since it is
+ // accessible despite being disabled.
+ await press.Tab();
+ expect(
+ await screen.findByRole( 'tab', {
+ selected: true,
+ name: 'Beta',
+ } )
+ ).toHaveFocus();
+ } );
+
+ it( 'should not have a selected tab nor show any tabpanels, but allow tabbing to the first tab when `defaultTabId` prop does not match any known tab', async () => {
+ await render(
+
+ );
+
+ // No initially selected tabs or tabpanels, since the `defaultTabId`
+ // prop is not matching any known tabs.
+ await waitForComponentToBeInitializedWithSelectedTab(
+ undefined
+ );
+
+ // Press tab. The first tab receives focus, but it's
+ // not selected.
+ await press.Tab();
+ expect(
+ screen.getByRole( 'tab', { name: 'Alpha' } )
+ ).toHaveFocus();
+ await waitFor( () =>
+ expect(
+ screen.queryByRole( 'tab', { selected: true } )
+ ).not.toBeInTheDocument()
+ );
+ await waitFor( () =>
+ expect(
+ screen.queryByRole( 'tabpanel' )
+ ).not.toBeInTheDocument()
+ );
- // Press the arrow up key, nothing happens.
- await press.ArrowUp();
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
- expect( await getSelectedTab() ).toHaveFocus();
- expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
- expect( mockOnSelect ).toHaveBeenLastCalledWith( 'alpha' );
+ // Press right arrow to select the next tab (beta) and
+ // show the related tabpanel.
+ await press.ArrowRight();
+ expect(
+ await screen.findByRole( 'tab', {
+ selected: true,
+ name: 'Beta',
+ } )
+ ).toHaveFocus();
+ expect(
+ await screen.findByRole( 'tabpanel', {
+ name: 'Beta',
+ } )
+ ).toBeVisible();
+ } );
+
+ it( 'should not have a selected tab nor show any tabpanels, but allow tabbing to the first tab, even when disabled, when `defaultTabId` prop does not match any known tab', async () => {
+ await render(
+
+ );
+
+ // No initially selected tabs or tabpanels, since the `defaultTabId`
+ // prop is not matching any known tabs.
+ await waitForComponentToBeInitializedWithSelectedTab(
+ undefined
+ );
+
+ // Press tab. The first tab receives focus, but it's
+ // not selected.
+ await press.Tab();
+ expect(
+ screen.getByRole( 'tab', { name: 'Alpha' } )
+ ).toHaveFocus();
+ await waitFor( () =>
+ expect(
+ screen.queryByRole( 'tab', { selected: true } )
+ ).not.toBeInTheDocument()
+ );
+ await waitFor( () =>
+ expect(
+ screen.queryByRole( 'tabpanel' )
+ ).not.toBeInTheDocument()
+ );
- // Press the arrow down key, nothing happens
- await press.ArrowDown();
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
- expect( await getSelectedTab() ).toHaveFocus();
- expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
- expect( mockOnSelect ).toHaveBeenLastCalledWith( 'alpha' );
+ // Press right arrow to select the next tab (beta) and
+ // show the related tabpanel.
+ await press.ArrowRight();
+ expect(
+ await screen.findByRole( 'tab', {
+ selected: true,
+ name: 'Beta',
+ } )
+ ).toHaveFocus();
+ expect(
+ await screen.findByRole( 'tabpanel', {
+ name: 'Beta',
+ } )
+ ).toBeVisible();
+ } );
+
+ it( 'should ignore any changes to the `defaultTabId` prop after the first render', async () => {
+ const mockOnSelect = jest.fn();
+
+ const { rerender } = await render(
+
+ );
+
+ // Beta is the initially selected tab
+ await waitForComponentToBeInitializedWithSelectedTab(
+ 'Beta'
+ );
+
+ // Changing the defaultTabId prop to gamma should not have any effect.
+ await rerender(
+
+ );
- // Change orientation to `vertical`. When the orientation is vertical,
- // left/right arrow keys are replaced by up/down arrow keys.
- await rerender(
-
- );
+ expect(
+ await screen.findByRole( 'tab', {
+ selected: true,
+ name: 'Beta',
+ } )
+ ).toBeVisible();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Beta',
+ } )
+ ).toBeVisible();
- expect( screen.getByRole( 'tablist' ) ).toHaveAttribute(
- 'aria-orientation',
- 'vertical'
- );
+ expect( mockOnSelect ).not.toHaveBeenCalled();
+ } );
+ } );
- // Make sure alpha is still focused.
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
- expect( await getSelectedTab() ).toHaveFocus();
+ describe( 'through the `selectedTabId` prop [Controlled]', () => {
+ describe( 'when the `selectedTabId` matches an existing tab', () => {
+ it( 'should choose the initial tab matching the `selectedTabId`', async () => {
+ await render(
+
+ );
- // Navigate forward with arrow keys and make sure the Beta tab is
- // selected automatically.
- await press.ArrowDown();
- expect( await getSelectedTab() ).toHaveTextContent( 'Beta' );
- expect( await getSelectedTab() ).toHaveFocus();
- expect( mockOnSelect ).toHaveBeenCalledTimes( 2 );
- expect( mockOnSelect ).toHaveBeenLastCalledWith( 'beta' );
+ // Beta is the initially selected tab
+ await waitForComponentToBeInitializedWithSelectedTab(
+ 'Beta'
+ );
- // Navigate backwards with arrow keys. Make sure alpha is
- // selected automatically.
- await press.ArrowUp();
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
- expect( await getSelectedTab() ).toHaveFocus();
- expect( mockOnSelect ).toHaveBeenCalledTimes( 3 );
- expect( mockOnSelect ).toHaveBeenLastCalledWith( 'alpha' );
+ // Press tab. The selected tab (beta) received focus, since it is
+ // accessible despite being disabled.
+ await press.Tab();
+ expect(
+ await screen.findByRole( 'tab', {
+ selected: true,
+ name: 'Beta',
+ } )
+ ).toHaveFocus();
+ } );
- // Navigate backwards with arrow keys. Make sure alpha is
- // selected automatically.
- await press.ArrowUp();
- expect( await getSelectedTab() ).toHaveTextContent( 'Gamma' );
- expect( await getSelectedTab() ).toHaveFocus();
- expect( mockOnSelect ).toHaveBeenCalledTimes( 4 );
- expect( mockOnSelect ).toHaveBeenLastCalledWith( 'gamma' );
-
- // Navigate backwards with arrow keys. Make sure alpha is
- // selected automatically.
- await press.ArrowDown();
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
- expect( await getSelectedTab() ).toHaveFocus();
- expect( mockOnSelect ).toHaveBeenCalledTimes( 5 );
- expect( mockOnSelect ).toHaveBeenLastCalledWith( 'alpha' );
- } );
+ it( 'should choose the initial tab matching the `selectedTabId` even if a `defaultTabId` is passed', async () => {
+ await render(
+
+ );
- it( 'should move focus on a tab even if disabled with arrow key, but not with pointer clicks', async () => {
- const mockOnSelect = jest.fn();
+ // Gamma is the initially selected tab
+ await waitForComponentToBeInitializedWithSelectedTab(
+ 'Gamma'
+ );
- const TABS_WITH_DELTA_DISABLED = TABS_WITH_DELTA.map( ( tabObj ) =>
- tabObj.tabId === 'delta'
- ? {
- ...tabObj,
- tab: {
- ...tabObj.tab,
- disabled: true,
- },
- }
- : tabObj
- );
+ // Press tab. The selected tab (gamma) received focus, since it is
+ // accessible despite being disabled.
+ await press.Tab();
+ expect(
+ await screen.findByRole( 'tab', {
+ selected: true,
+ name: 'Gamma',
+ } )
+ ).toHaveFocus();
+ } );
- await render(
-
- );
+ it( 'should choose the initial tab matching the `selectedTabId` even if the tab is disabled', async () => {
+ await render(
+
+ );
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
- expect( await getSelectedTab() ).not.toHaveFocus();
+ // Beta is the initially selected tab
+ await waitForComponentToBeInitializedWithSelectedTab(
+ 'Beta'
+ );
- // onSelect gets called on the initial render.
- expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
- expect( mockOnSelect ).toHaveBeenLastCalledWith( 'alpha' );
+ // Press tab. The selected tab (beta) received focus, since it is
+ // accessible despite being disabled.
+ await press.Tab();
+ expect(
+ await screen.findByRole( 'tab', {
+ selected: true,
+ name: 'Beta',
+ } )
+ ).toHaveFocus();
+ } );
+ } );
- // Tab to focus the tablist. Make sure Alpha is focused.
- await press.Tab();
- expect( await getSelectedTab() ).toHaveFocus();
+ describe( "when the `selectedTabId` doesn't match an existing tab", () => {
+ it( 'should not have a selected tab nor show any tabpanels, but allow tabbing to the first tab', async () => {
+ await render(
+
+ );
- // Confirm onSelect has not been re-called
- expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
+ // No initially selected tabs or tabpanels, since the `selectedTabId`
+ // prop is not matching any known tabs.
+ await waitForComponentToBeInitializedWithSelectedTab(
+ undefined
+ );
- // Press the right arrow key three times. Since the delta tab is disabled:
- // - it won't be selected. The gamma tab will be selected instead, since
- // it was the tab that was last selected before delta. Therefore, the
- // `mockOnSelect` function gets called only twice (and not three times)
- // - it will receive focus, when using arrow keys
- await press.ArrowRight();
- await press.ArrowRight();
- await press.ArrowRight();
- expect( await getSelectedTab() ).toHaveTextContent( 'Gamma' );
- expect(
- screen.getByRole( 'tab', { name: 'Delta' } )
- ).toHaveFocus();
- expect( mockOnSelect ).toHaveBeenCalledTimes( 3 );
- expect( mockOnSelect ).toHaveBeenLastCalledWith( 'gamma' );
-
- // Navigate backwards with arrow keys. The gamma tab receives focus.
- // The `mockOnSelect` callback doesn't fire, since the gamma tab was
- // already selected.
- await press.ArrowLeft();
- expect( await getSelectedTab() ).toHaveTextContent( 'Gamma' );
- expect( await getSelectedTab() ).toHaveFocus();
- expect( mockOnSelect ).toHaveBeenCalledTimes( 3 );
+ // Press tab. The first tab receives focus, but it's
+ // not selected.
+ await press.Tab();
+ expect(
+ screen.getByRole( 'tab', { name: 'Alpha' } )
+ ).toHaveFocus();
+ await waitFor( () =>
+ expect(
+ screen.queryByRole( 'tab', { selected: true } )
+ ).not.toBeInTheDocument()
+ );
+ await waitFor( () =>
+ expect(
+ screen.queryByRole( 'tabpanel' )
+ ).not.toBeInTheDocument()
+ );
- // Click on the disabled tab. Compared to using arrow keys to move the
- // focus, disabled tabs ignore pointer clicks — and therefore, they don't
- // receive focus, nor they cause the `mockOnSelect` function to fire.
- await click( screen.getByRole( 'tab', { name: 'Delta' } ) );
- expect( await getSelectedTab() ).toHaveTextContent( 'Gamma' );
- expect( await getSelectedTab() ).toHaveFocus();
- expect( mockOnSelect ).toHaveBeenCalledTimes( 3 );
- } );
+ // Press right arrow to select the next tab (beta) and
+ // show the related tabpanel.
+ await press.ArrowRight();
+ expect(
+ await screen.findByRole( 'tab', {
+ selected: true,
+ name: 'Beta',
+ } )
+ ).toHaveFocus();
+ expect(
+ await screen.findByRole( 'tabpanel', {
+ name: 'Beta',
+ } )
+ ).toBeVisible();
+ } );
- it( 'should not focus the next tab when the Tab key is pressed', async () => {
- await render( );
+ it( 'should not have a selected tab nor show any tabpanels, but allow tabbing to the first tab even when disabled', async () => {
+ await render(
+
+ );
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
- expect( await getSelectedTab() ).not.toHaveFocus();
+ // No initially selected tabs or tabpanels, since the `selectedTabId`
+ // prop is not matching any known tabs.
+ await waitForComponentToBeInitializedWithSelectedTab(
+ undefined
+ );
- // Tab should initially focus the first tab in the tablist, which
- // is Alpha.
- await press.Tab();
- expect(
- await screen.findByRole( 'tab', { name: 'Alpha' } )
- ).toHaveFocus();
+ // Press tab. The first tab receives focus, but it's
+ // not selected.
+ await press.Tab();
+ expect(
+ screen.getByRole( 'tab', { name: 'Alpha' } )
+ ).toHaveFocus();
+ await waitFor( () =>
+ expect(
+ screen.queryByRole( 'tab', { selected: true } )
+ ).not.toBeInTheDocument()
+ );
+ await waitFor( () =>
+ expect(
+ screen.queryByRole( 'tabpanel' )
+ ).not.toBeInTheDocument()
+ );
- // Because all other tabs should have `tabindex=-1`, pressing Tab
- // should NOT move the focus to the next tab, which is Beta.
- // Instead, focus should go to the currently selected tabpanel (alpha).
- await press.Tab();
- expect(
- await screen.findByRole( 'tabpanel', {
- name: 'Alpha',
- } )
- ).toHaveFocus();
+ // Press right arrow to select the next tab (beta) and
+ // show the related tabpanel.
+ await press.ArrowRight();
+ expect(
+ await screen.findByRole( 'tab', {
+ selected: true,
+ name: 'Beta',
+ } )
+ ).toHaveFocus();
+ expect(
+ await screen.findByRole( 'tabpanel', {
+ name: 'Beta',
+ } )
+ ).toBeVisible();
+ } );
+ } );
+ } );
} );
+ } );
- it( 'switches to manual tab activation when the `selectOnMove` prop is set to `false`', async () => {
- const mockOnSelect = jest.fn();
+ describe( 'keyboard interactions', () => {
+ describe.each( [
+ [ 'Uncontrolled', UncontrolledTabs ],
+ [ 'Controlled', ControlledTabs ],
+ ] )( '[`%s`]', ( _mode, Component ) => {
+ it( 'should handle the tablist as one tab stop', async () => {
+ await render( );
- await render(
-
- );
+ // Alpha is automatically selected as the selected tab.
+ await waitForComponentToBeInitializedWithSelectedTab( 'Alpha' );
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
- expect( await getSelectedTab() ).not.toHaveFocus();
+ // Press tab. The selected tab (alpha) received focus.
+ await press.Tab();
+ expect(
+ await screen.findByRole( 'tab', {
+ selected: true,
+ name: 'Alpha',
+ } )
+ ).toHaveFocus();
- // onSelect gets called on the initial render.
- expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
- expect( mockOnSelect ).toHaveBeenLastCalledWith( 'alpha' );
+ // By default the tabpanel should receive focus
+ await press.Tab();
+ expect(
+ await screen.findByRole( 'tabpanel', {
+ name: 'Alpha',
+ } )
+ ).toHaveFocus();
+ } );
- // Click on Alpha and make sure it is selected.
- // onSelect shouldn't fire since the selected tab didn't change.
- await click( screen.getByRole( 'tab', { name: 'Alpha' } ) );
- expect(
- await screen.findByRole( 'tab', { name: 'Alpha' } )
- ).toHaveFocus();
- expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
- expect( mockOnSelect ).toHaveBeenLastCalledWith( 'alpha' );
-
- // Navigate forward with arrow keys. Make sure Beta is focused, but
- // that the tab selection happens only when pressing the spacebar
- // or enter key. onSelect shouldn't fire since the selected tab
- // didn't change.
- await press.ArrowRight();
- expect(
- await screen.findByRole( 'tab', { name: 'Beta' } )
- ).toHaveFocus();
- expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
-
- await press.Enter();
- expect( mockOnSelect ).toHaveBeenCalledTimes( 2 );
- expect( mockOnSelect ).toHaveBeenLastCalledWith( 'beta' );
+ it( 'should not focus the tabpanel container when its `focusable` property is set to `false`', async () => {
+ await render(
+
+ tabObj.tabId === 'alpha'
+ ? {
+ ...tabObj,
+ content: (
+ <>
+ Selected Tab: Alpha
+ Alpha Button
+ >
+ ),
+ tabpanel: { focusable: false },
+ }
+ : tabObj
+ ) }
+ />
+ );
- // Navigate forward with arrow keys. Make sure Gamma (last tab) is
- // focused, but that tab selection happens only when pressing the
- // spacebar or enter key. onSelect shouldn't fire since the selected
- // tab didn't change.
- await press.ArrowRight();
- expect(
- await screen.findByRole( 'tab', { name: 'Gamma' } )
- ).toHaveFocus();
- expect( mockOnSelect ).toHaveBeenCalledTimes( 2 );
- expect(
- screen.getByRole( 'tab', { name: 'Gamma' } )
- ).toHaveFocus();
+ // Alpha is automatically selected as the selected tab.
+ await waitForComponentToBeInitializedWithSelectedTab( 'Alpha' );
- await press.Space();
- expect( mockOnSelect ).toHaveBeenCalledTimes( 3 );
- expect( mockOnSelect ).toHaveBeenLastCalledWith( 'gamma' );
- } );
- } );
- describe( 'Uncontrolled mode', () => {
- describe( 'Without `defaultTabId` prop', () => {
- it( 'should render first tab', async () => {
- await render( );
+ // Tab should initially focus the first tab in the tablist, which
+ // is Alpha.
+ await press.Tab();
+ expect(
+ await screen.findByRole( 'tab', {
+ selected: true,
+ name: 'Alpha',
+ } )
+ ).toHaveFocus();
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
+ // In this case, the tabpanel container is skipped and focus is
+ // moved directly to its contents
+ await press.Tab();
expect(
- await screen.findByRole( 'tabpanel', { name: 'Alpha' } )
- ).toBeInTheDocument();
+ await screen.findByRole( 'button', {
+ name: 'Alpha Button',
+ } )
+ ).toHaveFocus();
} );
- it( 'should not have a selected tab if the currently selected tab is removed', async () => {
- const { rerender } = await render(
-
- );
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
- expect( await getSelectedTab() ).not.toHaveFocus();
+ it( 'should select tabs in the tablist when using the left and right arrow keys by default (automatic tab activation)', async () => {
+ const mockOnSelect = jest.fn();
- // Tab to focus the tablist. Make sure Alpha is focused.
- await press.Tab();
- expect( await getSelectedTab() ).toHaveFocus();
+ await render(
+
+ );
- // Remove first item from `TABS` array
- await rerender( );
+ // Alpha is automatically selected as the selected tab.
+ await waitForComponentToBeInitializedWithSelectedTab( 'Alpha' );
- // No tab should be selected i.e. it doesn't fall back to first tab.
- await waitFor( () =>
- expect(
- screen.queryByRole( 'tab', { selected: true } )
- ).not.toBeInTheDocument()
- );
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
+ expect( mockOnSelect ).toHaveBeenLastCalledWith( 'alpha' );
- // No tabpanel should be rendered either
+ // Focus the tablist (and the selected tab, alpha)
+ // Tab should initially focus the first tab in the tablist, which
+ // is Alpha.
+ await press.Tab();
expect(
- screen.queryByRole( 'tabpanel' )
- ).not.toBeInTheDocument();
- } );
- } );
+ await screen.findByRole( 'tab', {
+ selected: true,
+ name: 'Alpha',
+ } )
+ ).toHaveFocus();
- describe( 'With `defaultTabId`', () => {
- it( 'should render the tab set by `defaultTabId` prop', async () => {
- await render(
-
- );
+ // Press the right arrow key to select the beta tab
+ await press.ArrowRight();
- expect( await getSelectedTab() ).toHaveTextContent( 'Beta' );
- } );
+ expect(
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Beta',
+ } )
+ ).toHaveFocus();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Beta',
+ } )
+ ).toBeVisible();
- it( 'should not select a tab when `defaultTabId` does not match any known tab', async () => {
- await render(
-
- );
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 2 );
+ expect( mockOnSelect ).toHaveBeenLastCalledWith( 'beta' );
- // No tab should be selected i.e. it doesn't fall back to first tab.
- expect(
- screen.queryByRole( 'tab', { selected: true } )
- ).not.toBeInTheDocument();
+ // Press the right arrow key to select the gamma tab
+ await press.ArrowRight();
- // No tabpanel should be rendered either
expect(
- screen.queryByRole( 'tabpanel' )
- ).not.toBeInTheDocument();
- } );
- it( 'should not change tabs when defaultTabId is changed', async () => {
- const { rerender } = await render(
-
- );
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Gamma',
+ } )
+ ).toHaveFocus();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Gamma',
+ } )
+ ).toBeVisible();
- expect( await getSelectedTab() ).toHaveTextContent( 'Beta' );
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 3 );
+ expect( mockOnSelect ).toHaveBeenLastCalledWith( 'gamma' );
- await rerender(
-
- );
+ // Press the left arrow key to select the beta tab
+ await press.ArrowLeft();
- expect( await getSelectedTab() ).toHaveTextContent( 'Beta' );
+ expect(
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Beta',
+ } )
+ ).toHaveFocus();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Beta',
+ } )
+ ).toBeVisible();
+
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 4 );
+ expect( mockOnSelect ).toHaveBeenLastCalledWith( 'beta' );
} );
- it( 'should not have any selected tabs if the currently selected tab is removed, even if a tab is matching the defaultTabId', async () => {
+ it( 'should not automatically select tabs in the tablist when pressing the left and right arrow keys if the `selectOnMove` prop is set to `false` (manual tab activation)', async () => {
const mockOnSelect = jest.fn();
- const { rerender } = await render(
-
);
- expect( await getSelectedTab() ).toHaveTextContent( 'Gamma' );
+ // Alpha is automatically selected as the selected tab.
+ await waitForComponentToBeInitializedWithSelectedTab( 'Alpha' );
- await click( screen.getByRole( 'tab', { name: 'Alpha' } ) );
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
expect( mockOnSelect ).toHaveBeenLastCalledWith( 'alpha' );
- await rerender(
-
- );
+ // Focus the tablist (and the selected tab, alpha)
+ // Tab should initially focus the first tab in the tablist, which
+ // is Alpha.
+ await press.Tab();
+ expect(
+ await screen.findByRole( 'tab', {
+ selected: true,
+ name: 'Alpha',
+ } )
+ ).toHaveFocus();
- // No tab should be selected i.e. it doesn't fall back to first tab.
- await waitFor( () =>
- expect(
- screen.queryByRole( 'tab', { selected: true } )
- ).not.toBeInTheDocument()
- );
+ // Press the right arrow key to move focus to the beta tab,
+ // but without selecting it
+ await press.ArrowRight();
+
+ expect(
+ screen.getByRole( 'tab', {
+ selected: false,
+ name: 'Beta',
+ } )
+ ).toHaveFocus();
+ expect(
+ await screen.findByRole( 'tab', {
+ selected: true,
+ name: 'Alpha',
+ } )
+ ).toBeVisible();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Alpha',
+ } )
+ ).toBeVisible();
+
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
- // No tabpanel should be rendered either
+ // Press the space key to click the beta tab, and select it.
+ // The same should be true with any other mean of clicking the tab button
+ // (ie. mouse click, enter key).
+ await press.Space();
+
+ expect(
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Beta',
+ } )
+ ).toHaveFocus();
expect(
- screen.queryByRole( 'tabpanel' )
- ).not.toBeInTheDocument();
+ screen.getByRole( 'tabpanel', {
+ name: 'Beta',
+ } )
+ ).toBeVisible();
+
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 2 );
+ expect( mockOnSelect ).toHaveBeenLastCalledWith( 'beta' );
} );
- it( 'should keep the currently selected tab even if it becomes disabled', async () => {
+ it( 'should not select tabs in the tablist when using the up and down arrow keys, unless the `orientation` prop is set to `vertical`', async () => {
const mockOnSelect = jest.fn();
const { rerender } = await render(
-
+
);
- expect( await getSelectedTab() ).toHaveTextContent( 'Gamma' );
-
- await click( screen.getByRole( 'tab', { name: 'Alpha' } ) );
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
+ // Alpha is automatically selected as the selected tab.
+ await waitForComponentToBeInitializedWithSelectedTab( 'Alpha' );
expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
expect( mockOnSelect ).toHaveBeenLastCalledWith( 'alpha' );
- const TABS_WITH_ALPHA_DISABLED = TABS.map( ( tabObj ) =>
- tabObj.tabId === 'alpha'
- ? {
- ...tabObj,
- tab: {
- ...tabObj.tab,
- disabled: true,
- },
- }
- : tabObj
- );
+ // Focus the tablist (and the selected tab, alpha)
+ // Tab should initially focus the first tab in the tablist, which
+ // is Alpha.
+ await press.Tab();
+ expect(
+ await screen.findByRole( 'tab', {
+ selected: true,
+ name: 'Alpha',
+ } )
+ ).toHaveFocus();
- await rerender(
-
- );
+ // Press the up arrow key, but the focused/selected tab does not change.
+ await press.ArrowUp();
+
+ expect(
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Alpha',
+ } )
+ ).toHaveFocus();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Alpha',
+ } )
+ ).toBeVisible();
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
- } );
- it( 'should have no active tabs when the tab associated to `defaultTabId` is removed while being the active tab', async () => {
- const { rerender } = await render(
-
- );
+ // Press the down arrow key, but the focused/selected tab does not change.
+ await press.ArrowDown();
+
+ expect(
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Alpha',
+ } )
+ ).toHaveFocus();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Alpha',
+ } )
+ ).toBeVisible();
- expect( await getSelectedTab() ).toHaveTextContent( 'Gamma' );
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
- // Remove gamma
+ // Change the orientation to "vertical" and rerender the component.
await rerender(
-
);
- expect( screen.getAllByRole( 'tab' ) ).toHaveLength( 2 );
- // No tab should be selected i.e. it doesn't fall back to first tab.
+ // Pressing the down arrow key now selects the next tab (beta).
+ await press.ArrowDown();
+
expect(
- screen.queryByRole( 'tab', { selected: true } )
- ).not.toBeInTheDocument();
- // No tabpanel should be rendered either
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Beta',
+ } )
+ ).toHaveFocus();
expect(
- screen.queryByRole( 'tabpanel' )
- ).not.toBeInTheDocument();
- } );
+ screen.getByRole( 'tabpanel', {
+ name: 'Beta',
+ } )
+ ).toBeVisible();
- it( 'waits for the tab with the `defaultTabId` to be present in the `tabs` array before selecting it', async () => {
- const { rerender } = await render(
-
- );
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 2 );
+ expect( mockOnSelect ).toHaveBeenLastCalledWith( 'beta' );
- // No tab should be selected i.e. it doesn't fall back to first tab.
- await waitFor( () =>
- expect(
- screen.queryByRole( 'tab', { selected: true } )
- ).not.toBeInTheDocument()
- );
+ // Pressing the up arrow key now selects the previous tab (alpha).
+ await press.ArrowUp();
- // No tabpanel should be rendered either
expect(
- screen.queryByRole( 'tabpanel' )
- ).not.toBeInTheDocument();
-
- await rerender(
-
- );
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Alpha',
+ } )
+ ).toHaveFocus();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Alpha',
+ } )
+ ).toBeVisible();
- expect( await getSelectedTab() ).toHaveTextContent( 'Delta' );
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 3 );
+ expect( mockOnSelect ).toHaveBeenLastCalledWith( 'alpha' );
} );
- } );
- describe( 'Disabled tab', () => {
- it( 'should disable the tab when `disabled` is `true`', async () => {
+ it( 'should loop tab focus at the end of the tablist when using arrow keys', async () => {
const mockOnSelect = jest.fn();
- const TABS_WITH_DELTA_DISABLED = TABS_WITH_DELTA.map(
- ( tabObj ) =>
- tabObj.tabId === 'delta'
- ? {
- ...tabObj,
- tab: {
- ...tabObj.tab,
- disabled: true,
- },
- }
- : tabObj
- );
-
await render(
-
+
);
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
+ // Alpha is automatically selected as the selected tab.
+ await waitForComponentToBeInitializedWithSelectedTab( 'Alpha' );
- expect(
- screen.getByRole( 'tab', { name: 'Delta' } )
- ).toHaveAttribute( 'aria-disabled', 'true' );
-
- // onSelect gets called on the initial render.
expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
expect( mockOnSelect ).toHaveBeenLastCalledWith( 'alpha' );
- // Move focus to the tablist, make sure alpha is focused.
+ // Focus the tablist (and the selected tab, alpha)
+ // Tab should initially focus the first tab in the tablist, which
+ // is Alpha.
await press.Tab();
expect(
- screen.getByRole( 'tab', { name: 'Alpha' } )
+ await screen.findByRole( 'tab', {
+ selected: true,
+ name: 'Alpha',
+ } )
).toHaveFocus();
- // onSelect should not be called since the disabled tab is
- // highlighted, but not selected.
+ // Press the left arrow key to loop around and select the gamma tab
await press.ArrowLeft();
- expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
- // Delta (which is disabled) has focus
expect(
- screen.getByRole( 'tab', { name: 'Delta' } )
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Gamma',
+ } )
).toHaveFocus();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Gamma',
+ } )
+ ).toBeVisible();
- // Alpha retains the selection, even if it's not focused.
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
- } );
-
- it( 'should select first enabled tab when the initial tab is disabled', async () => {
- const TABS_WITH_ALPHA_DISABLED = TABS.map( ( tabObj ) =>
- tabObj.tabId === 'alpha'
- ? {
- ...tabObj,
- tab: {
- ...tabObj.tab,
- disabled: true,
- },
- }
- : tabObj
- );
-
- const { rerender } = await render(
-
- );
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 2 );
+ expect( mockOnSelect ).toHaveBeenLastCalledWith( 'gamma' );
- // As alpha (first tab) is disabled,
- // the first enabled tab should be beta.
- expect( await getSelectedTab() ).toHaveTextContent( 'Beta' );
+ // Press the right arrow key to loop around and select the alpha tab
+ await press.ArrowRight();
- // Re-enable all tabs
- await rerender( );
+ expect(
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Alpha',
+ } )
+ ).toHaveFocus();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Alpha',
+ } )
+ ).toBeVisible();
- // Even if the initial tab becomes enabled again, the selected
- // tab doesn't change.
- expect( await getSelectedTab() ).toHaveTextContent( 'Beta' );
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 3 );
+ expect( mockOnSelect ).toHaveBeenLastCalledWith( 'alpha' );
} );
- it( 'should select the tab associated to `defaultTabId` even if the tab is disabled', async () => {
- const TABS_ONLY_GAMMA_ENABLED = TABS.map( ( tabObj ) =>
- tabObj.tabId !== 'gamma'
- ? {
- ...tabObj,
- tab: {
- ...tabObj.tab,
- disabled: true,
- },
- }
- : tabObj
- );
- const { rerender } = await render(
-
- );
+ // TODO: mock writing direction to RTL
+ it( 'should swap the left and right arrow keys when selecting tabs if the writing direction is set to RTL', async () => {
+ // For this test only, mock the writing direction to RTL.
+ mockedIsRTL.mockImplementation( () => true );
- // As alpha (first tab), and beta (the initial tab), are both
- // disabled the first enabled tab should be gamma.
- expect( await getSelectedTab() ).toHaveTextContent( 'Beta' );
+ const mockOnSelect = jest.fn();
- // Re-enable all tabs
- await rerender(
-
+ await render(
+
);
- // Even if the initial tab becomes enabled again, the selected tab doesn't
- // change.
- expect( await getSelectedTab() ).toHaveTextContent( 'Beta' );
- } );
+ // Alpha is automatically selected as the selected tab.
+ await waitForComponentToBeInitializedWithSelectedTab( 'Alpha' );
- it( 'should keep the currently tab as selected even when it becomes disabled', async () => {
- const mockOnSelect = jest.fn();
- const { rerender } = await render(
-
- );
-
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
expect( mockOnSelect ).toHaveBeenLastCalledWith( 'alpha' );
- const TABS_WITH_ALPHA_DISABLED = TABS.map( ( tabObj ) =>
- tabObj.tabId === 'alpha'
- ? {
- ...tabObj,
- tab: {
- ...tabObj.tab,
- disabled: true,
- },
- }
- : tabObj
- );
-
- // Disable alpha
- await rerender(
-
- );
+ // Focus the tablist (and the selected tab, alpha)
+ // Tab should initially focus the first tab in the tablist, which
+ // is Alpha.
+ await press.Tab();
+ expect(
+ await screen.findByRole( 'tab', {
+ selected: true,
+ name: 'Alpha',
+ } )
+ ).toHaveFocus();
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
- expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
+ // Press the left arrow key to select the beta tab
+ await press.ArrowLeft();
- // Re-enable all tabs
- await rerender(
-
- );
+ expect(
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Beta',
+ } )
+ ).toHaveFocus();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Beta',
+ } )
+ ).toBeVisible();
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
- expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
- } );
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 2 );
+ expect( mockOnSelect ).toHaveBeenLastCalledWith( 'beta' );
- it( 'should select the tab associated to `defaultTabId` even when disabled', async () => {
- const mockOnSelect = jest.fn();
+ // Press the left arrow key to select the gamma tab
+ await press.ArrowLeft();
- const { rerender } = await render(
-
- );
+ expect(
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Gamma',
+ } )
+ ).toHaveFocus();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Gamma',
+ } )
+ ).toBeVisible();
- expect( await getSelectedTab() ).toHaveTextContent( 'Gamma' );
-
- const TABS_WITH_GAMMA_DISABLED = TABS.map( ( tabObj ) =>
- tabObj.tabId === 'gamma'
- ? {
- ...tabObj,
- tab: {
- ...tabObj.tab,
- disabled: true,
- },
- }
- : tabObj
- );
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 3 );
+ expect( mockOnSelect ).toHaveBeenLastCalledWith( 'gamma' );
- // Disable gamma
- await rerender(
-
- );
+ // Press the right arrow key to select the beta tab
+ await press.ArrowRight();
- expect( await getSelectedTab() ).toHaveTextContent( 'Gamma' );
+ expect(
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Beta',
+ } )
+ ).toHaveFocus();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Beta',
+ } )
+ ).toBeVisible();
- // Re-enable all tabs
- await rerender(
-
- );
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 4 );
+ expect( mockOnSelect ).toHaveBeenLastCalledWith( 'beta' );
- // Confirm that alpha is still selected, and that onSelect has
- // not been called again.
- expect( await getSelectedTab() ).toHaveTextContent( 'Gamma' );
- expect( mockOnSelect ).not.toHaveBeenCalled();
+ // Restore the original implementation of the isRTL function.
+ mockedIsRTL.mockRestore();
} );
- } );
- } );
-
- describe( 'Controlled mode', () => {
- it( 'should render the tab specified by the `selectedTabId` prop', async () => {
- await render(
-
- );
- expect( await getSelectedTab() ).toHaveTextContent( 'Beta' );
- expect(
- await screen.findByRole( 'tabpanel', { name: 'Beta' } )
- ).toBeInTheDocument();
- } );
- it( 'should render the specified `selectedTabId`, and ignore the `defaultTabId` prop', async () => {
- await render(
-
- );
-
- expect( await getSelectedTab() ).toHaveTextContent( 'Gamma' );
- } );
- it( 'should not have a selected tab if `selectedTabId` does not match any known tab', async () => {
- await render(
-
- );
-
- expect(
- screen.queryByRole( 'tab', { selected: true } )
- ).not.toBeInTheDocument();
+ it( 'should focus tabs in the tablist even if disabled', async () => {
+ const mockOnSelect = jest.fn();
- // No tabpanel should be rendered either
- expect( screen.queryByRole( 'tabpanel' ) ).not.toBeInTheDocument();
- } );
- it( 'should not have a selected tab if the active tab is removed, but should select a tab that gets added if it matches the selectedTabId', async () => {
- const { rerender } = await render(
-
- );
+ await render(
+
+ );
- expect( await getSelectedTab() ).toHaveTextContent( 'Beta' );
+ // Alpha is automatically selected as the selected tab.
+ await waitForComponentToBeInitializedWithSelectedTab( 'Alpha' );
- // Remove beta
- await rerender(
- tab.tabId !== 'beta' ) }
- selectedTabId="beta"
- />
- );
-
- expect( screen.getAllByRole( 'tab' ) ).toHaveLength( 2 );
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
+ expect( mockOnSelect ).toHaveBeenLastCalledWith( 'alpha' );
- // No tab should be selected i.e. it doesn't fall back to first tab.
- // `waitFor` is needed here to prevent testing library from
- // throwing a 'not wrapped in `act()`' error.
- await waitFor( () =>
+ // Focus the tablist (and the selected tab, alpha)
+ // Tab should initially focus the first tab in the tablist, which
+ // is Alpha.
+ await press.Tab();
expect(
- screen.queryByRole( 'tab', { selected: true } )
- ).not.toBeInTheDocument()
- );
-
- // No tabpanel should be rendered either
- expect( screen.queryByRole( 'tabpanel' ) ).not.toBeInTheDocument();
-
- // Restore beta
- await rerender(
-
- );
-
- expect( await getSelectedTab() ).toHaveTextContent( 'Beta' );
- } );
-
- describe( 'Disabled tab', () => {
- it( 'should `selectedTabId` refers to a disabled tab', async () => {
- const TABS_WITH_DELTA_WITH_BETA_DISABLED = TABS_WITH_DELTA.map(
- ( tabObj ) =>
- tabObj.tabId === 'beta'
- ? {
- ...tabObj,
- tab: {
- ...tabObj.tab,
- disabled: true,
- },
- }
- : tabObj
- );
-
- await render(
-
- );
+ await screen.findByRole( 'tab', {
+ selected: true,
+ name: 'Alpha',
+ } )
+ ).toHaveFocus();
- expect( await getSelectedTab() ).toHaveTextContent( 'Beta' );
- } );
- it( 'should keep the currently selected tab as selected even when it becomes disabled', async () => {
- const { rerender } = await render(
-
- );
+ // Pressing the right arrow key moves focus to the beta tab, but alpha
+ // remains the selected tab because beta is disabled.
+ await press.ArrowRight();
- expect( await getSelectedTab() ).toHaveTextContent( 'Beta' );
-
- const TABS_WITH_BETA_DISABLED = TABS.map( ( tabObj ) =>
- tabObj.tabId === 'beta'
- ? {
- ...tabObj,
- tab: {
- ...tabObj.tab,
- disabled: true,
- },
- }
- : tabObj
- );
+ expect(
+ screen.getByRole( 'tab', {
+ selected: false,
+ name: 'Beta',
+ } )
+ ).toHaveFocus();
+ expect(
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Alpha',
+ } )
+ ).toBeVisible();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Alpha',
+ } )
+ ).toBeVisible();
- await rerender(
-
- );
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
- expect( await getSelectedTab() ).toHaveTextContent( 'Beta' );
+ // Press the right arrow key to select the gamma tab
+ await press.ArrowRight();
- // re-enable all tabs
- await rerender(
-
- );
+ expect(
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Gamma',
+ } )
+ ).toHaveFocus();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Gamma',
+ } )
+ ).toBeVisible();
- expect( await getSelectedTab() ).toHaveTextContent( 'Beta' );
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 2 );
+ expect( mockOnSelect ).toHaveBeenLastCalledWith( 'gamma' );
} );
} );
- describe( 'When `selectedId` is changed by the controlling component', () => {
+
+ describe( 'When `selectedId` is changed by the controlling component [Controlled]', () => {
describe.each( [ true, false ] )(
'and `selectOnMove` is %s',
( selectOnMove ) => {
@@ -1231,17 +1443,18 @@ describe( 'Tabs', () => {
/>
);
- expect( await getSelectedTab() ).toHaveTextContent(
+ // Beta is the selected tab.
+ await waitForComponentToBeInitializedWithSelectedTab(
'Beta'
);
// Tab key should focus the currently selected tab, which is Beta.
await press.Tab();
- expect( await getSelectedTab() ).toHaveTextContent(
- 'Beta'
- );
expect(
- screen.getByRole( 'tab', { name: 'Beta' } )
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Beta',
+ } )
).toHaveFocus();
await rerender(
@@ -1253,17 +1466,28 @@ describe( 'Tabs', () => {
);
// When the selected tab is changed, focus should not be changed.
- expect( await getSelectedTab() ).toHaveTextContent(
- 'Gamma'
- );
expect(
- screen.getByRole( 'tab', { name: 'Beta' } )
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Gamma',
+ } )
+ ).toBeVisible();
+ expect(
+ screen.getByRole( 'tab', {
+ selected: false,
+ name: 'Beta',
+ } )
).toHaveFocus();
- // Arrow keys should move focus to the next tab, which is Gamma
- await press.ArrowRight();
+ // Arrow left should move focus to the previous tab (alpha).
+ // The alpha tab should be always focused, and should be selected
+ // when the `selectOnMove` prop is set to `true`.
+ await press.ArrowLeft();
expect(
- screen.getByRole( 'tab', { name: 'Gamma' } )
+ screen.getByRole( 'tab', {
+ selected: selectOnMove,
+ name: 'Alpha',
+ } )
).toHaveFocus();
} );
@@ -1279,20 +1503,22 @@ describe( 'Tabs', () => {
>
);
- expect( await getSelectedTab() ).toHaveTextContent(
+ // Beta is the selected tab.
+ await waitForComponentToBeInitializedWithSelectedTab(
'Beta'
);
// Tab key should focus the currently selected tab, which is Beta.
await press.Tab();
await press.Tab();
- expect( await getSelectedTab() ).toHaveTextContent(
- 'Beta'
- );
expect(
- screen.getByRole( 'tab', { name: 'Beta' } )
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Beta',
+ } )
).toHaveFocus();
+ // Change the selected tab to gamma via a controlled update.
await rerender(
<>
Focus me
@@ -1305,12 +1531,17 @@ describe( 'Tabs', () => {
);
// When the selected tab is changed, it should not automatically receive focus.
- expect( await getSelectedTab() ).toHaveTextContent(
- 'Gamma'
- );
-
expect(
- screen.getByRole( 'tab', { name: 'Beta' } )
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Gamma',
+ } )
+ ).toBeVisible();
+ expect(
+ screen.getByRole( 'tab', {
+ selected: false,
+ name: 'Beta',
+ } )
).toHaveFocus();
// Press shift+tab, move focus to the button before Tabs
@@ -1336,125 +1567,439 @@ describe( 'Tabs', () => {
}
);
} );
+ } );
- describe( 'When `selectOnMove` is `true`', () => {
- it( 'should automatically select a newly focused tab', async () => {
- await render(
-
- );
+ describe( 'miscellaneous runtime changes', () => {
+ describe( 'removing a tab', () => {
+ describe( 'with no explicitly set initial tab', () => {
+ it( 'should not select a new tab when the selected tab is removed', async () => {
+ const mockOnSelect = jest.fn();
- expect( await getSelectedTab() ).toHaveTextContent( 'Beta' );
+ const { rerender } = await render(
+
+ );
- await press.Tab();
+ // Alpha is automatically selected as the selected tab.
+ await waitForComponentToBeInitializedWithSelectedTab(
+ 'Alpha'
+ );
- // Tab key should focus the currently selected tab, which is Beta.
- expect( await getSelectedTab() ).toHaveTextContent( 'Beta' );
- expect( await getSelectedTab() ).toHaveFocus();
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
+ expect( mockOnSelect ).toHaveBeenLastCalledWith( 'alpha' );
- // Arrow keys should select and move focus to the next tab.
- await press.ArrowRight();
- expect( await getSelectedTab() ).toHaveTextContent( 'Gamma' );
- expect( await getSelectedTab() ).toHaveFocus();
+ // Select gamma
+ await click( screen.getByRole( 'tab', { name: 'Gamma' } ) );
+
+ expect(
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Gamma',
+ } )
+ ).toHaveFocus();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Gamma',
+ } )
+ ).toBeVisible();
+
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 2 );
+ expect( mockOnSelect ).toHaveBeenLastCalledWith( 'gamma' );
+
+ // Remove gamma
+ await rerender(
+
+ );
+
+ expect( screen.getAllByRole( 'tab' ) ).toHaveLength( 2 );
+
+ // No tab should be selected i.e. it doesn't fall back to gamma,
+ // even if it matches the `defaultTabId` prop.
+ expect(
+ screen.queryByRole( 'tab', { selected: true } )
+ ).not.toBeInTheDocument();
+ // No tabpanel should be rendered either
+ expect(
+ screen.queryByRole( 'tabpanel' )
+ ).not.toBeInTheDocument();
+
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 2 );
+ } );
} );
+
+ describe.each( [
+ [ 'defaultTabId', 'Uncontrolled', UncontrolledTabs ],
+ [ 'selectedTabId', 'Controlled', ControlledTabs ],
+ ] )(
+ 'when using the `%s` prop [%s]',
+ ( propName, _mode, Component ) => {
+ it( 'should not select a new tab when the selected tab is removed', async () => {
+ const mockOnSelect = jest.fn();
+
+ const initialComponentProps = {
+ tabs: TABS,
+ [ propName ]: 'gamma',
+ onSelect: mockOnSelect,
+ };
+
+ const { rerender } = await render(
+
+ );
+
+ // Gamma is the selected tab.
+ await waitForComponentToBeInitializedWithSelectedTab(
+ 'Gamma'
+ );
+
+ // Remove gamma
+ await rerender(
+
+ );
+
+ expect( screen.getAllByRole( 'tab' ) ).toHaveLength(
+ 2
+ );
+ // No tab should be selected i.e. it doesn't fall back to first tab.
+ expect(
+ screen.queryByRole( 'tab', { selected: true } )
+ ).not.toBeInTheDocument();
+ // No tabpanel should be rendered either
+ expect(
+ screen.queryByRole( 'tabpanel' )
+ ).not.toBeInTheDocument();
+
+ // Re-add gamma. Gamma becomes selected again.
+ await rerender(
+
+ );
+
+ expect( screen.getAllByRole( 'tab' ) ).toHaveLength(
+ TABS.length
+ );
+
+ expect(
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Gamma',
+ } )
+ ).toBeVisible();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Gamma',
+ } )
+ ).toBeVisible();
+
+ expect( mockOnSelect ).not.toHaveBeenCalled();
+ } );
+
+ it( `should not select the tab matching the \`${ propName }\` prop as a fallback when the selected tab is removed`, async () => {
+ const mockOnSelect = jest.fn();
+
+ const initialComponentProps = {
+ tabs: TABS,
+ [ propName ]: 'gamma',
+ onSelect: mockOnSelect,
+ };
+
+ const { rerender } = await render(
+
+ );
+
+ // Gamma is the selected tab.
+ await waitForComponentToBeInitializedWithSelectedTab(
+ 'Gamma'
+ );
+
+ // Select alpha
+ await click(
+ screen.getByRole( 'tab', { name: 'Alpha' } )
+ );
+
+ expect(
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Alpha',
+ } )
+ ).toHaveFocus();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Alpha',
+ } )
+ ).toBeVisible();
+
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
+ expect( mockOnSelect ).toHaveBeenLastCalledWith(
+ 'alpha'
+ );
+
+ // Remove alpha
+ await rerender(
+
+ );
+
+ expect( screen.getAllByRole( 'tab' ) ).toHaveLength(
+ 2
+ );
+
+ // No tab should be selected i.e. it doesn't fall back to gamma,
+ // even if it matches the `defaultTabId` prop.
+ expect(
+ screen.queryByRole( 'tab', { selected: true } )
+ ).not.toBeInTheDocument();
+ // No tabpanel should be rendered either
+ expect(
+ screen.queryByRole( 'tabpanel' )
+ ).not.toBeInTheDocument();
+
+ // Re-add alpha. Alpha becomes selected again.
+ await rerender(
+
+ );
+
+ expect( screen.getAllByRole( 'tab' ) ).toHaveLength(
+ TABS.length
+ );
+
+ expect(
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Alpha',
+ } )
+ ).toBeVisible();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Alpha',
+ } )
+ ).toBeVisible();
+
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
+ } );
+ }
+ );
} );
- describe( 'When `selectOnMove` is `false`', () => {
- it( 'should apply focus without automatically changing the selected tab', async () => {
- await render(
-
- );
- expect( await getSelectedTab() ).toHaveTextContent( 'Beta' );
+ describe( 'adding a tab', () => {
+ describe.each( [
+ [ 'defaultTabId', 'Uncontrolled', UncontrolledTabs ],
+ [ 'selectedTabId', 'Controlled', ControlledTabs ],
+ ] )(
+ 'when using the `%s` prop [%s]',
+ ( propName, _mode, Component ) => {
+ it( `should select a newly added tab if it matches the \`${ propName }\` prop`, async () => {
+ const mockOnSelect = jest.fn();
+
+ const initialComponentProps = {
+ tabs: TABS,
+ [ propName ]: 'delta',
+ onSelect: mockOnSelect,
+ };
- // Tab key should focus the currently selected tab, which is Beta.
- await press.Tab();
- await waitFor( async () =>
- expect(
- await screen.findByRole( 'tab', { name: 'Beta' } )
- ).toHaveFocus()
- );
+ const { rerender } = await render(
+
+ );
- // Arrow key should move focus but not automatically change the selected tab.
- await press.ArrowRight();
- expect(
- screen.getByRole( 'tab', { name: 'Gamma' } )
- ).toHaveFocus();
- expect( await getSelectedTab() ).toHaveTextContent( 'Beta' );
+ // No initially selected tabs or tabpanels, since the `defaultTabId`
+ // prop is not matching any known tabs.
+ await waitForComponentToBeInitializedWithSelectedTab(
+ undefined
+ );
- // Pressing the spacebar should select the focused tab.
- await press.Space();
- expect( await getSelectedTab() ).toHaveTextContent( 'Gamma' );
+ expect( mockOnSelect ).not.toHaveBeenCalled();
- // Arrow key should move focus but not automatically change the selected tab.
- await press.ArrowRight();
- expect(
- screen.getByRole( 'tab', { name: 'Alpha' } )
- ).toHaveFocus();
- expect( await getSelectedTab() ).toHaveTextContent( 'Gamma' );
+ // Re-render with beta disabled.
+ await rerender(
+
+ );
- // Pressing the enter/return should select the focused tab.
- await press.Enter();
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
- } );
+ // Delta becomes selected
+ expect(
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Delta',
+ } )
+ ).toBeVisible();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Delta',
+ } )
+ ).toBeVisible();
+
+ expect( mockOnSelect ).not.toHaveBeenCalled();
+ } );
+ }
+ );
} );
- } );
- it( 'should associate each `Tab` with the correct `TabPanel`, even if they are not rendered in the same order', async () => {
- const TABS_WITH_DELTA_REVERSED = [ ...TABS_WITH_DELTA ].reverse();
-
- await render(
-
-
- { TABS_WITH_DELTA.map( ( tabObj ) => (
-
- { tabObj.title }
-
- ) ) }
-
- { TABS_WITH_DELTA_REVERSED.map( ( tabObj ) => (
-
- { tabObj.content }
-
- ) ) }
-
- );
+ describe( 'a tab becomes disabled', () => {
+ describe.each( [
+ [ 'defaultTabId', 'Uncontrolled', UncontrolledTabs ],
+ [ 'selectedTabId', 'Controlled', ControlledTabs ],
+ ] )(
+ 'when using the `%s` prop [%s]',
+ ( propName, _mode, Component ) => {
+ it( `should keep the initial tab matching the \`${ propName }\` prop as selected even if it becomes disabled`, async () => {
+ const mockOnSelect = jest.fn();
+
+ const initialComponentProps = {
+ tabs: TABS,
+ [ propName ]: 'beta',
+ onSelect: mockOnSelect,
+ };
- // Alpha is the initially selected tab,and should render the correct tabpanel
- expect( await getSelectedTab() ).toHaveTextContent( 'Alpha' );
- expect( screen.getByRole( 'tabpanel' ) ).toHaveTextContent(
- 'Selected tab: Alpha'
- );
+ const { rerender } = await render(
+
+ );
- // Select Beta, make sure the correct tabpanel is rendered
- await click( screen.getByRole( 'tab', { name: 'Beta' } ) );
- expect( await getSelectedTab() ).toHaveTextContent( 'Beta' );
- expect( screen.getByRole( 'tabpanel' ) ).toHaveTextContent(
- 'Selected tab: Beta'
- );
+ // Beta is the selected tab.
+ await waitForComponentToBeInitializedWithSelectedTab(
+ 'Beta'
+ );
- // Select Gamma, make sure the correct tabpanel is rendered
- await click( screen.getByRole( 'tab', { name: 'Gamma' } ) );
- expect( await getSelectedTab() ).toHaveTextContent( 'Gamma' );
- expect( screen.getByRole( 'tabpanel' ) ).toHaveTextContent(
- 'Selected tab: Gamma'
- );
+ expect( mockOnSelect ).not.toHaveBeenCalled();
- // Select Delta, make sure the correct tabpanel is rendered
- await click( screen.getByRole( 'tab', { name: 'Delta' } ) );
- expect( await getSelectedTab() ).toHaveTextContent( 'Delta' );
- expect( screen.getByRole( 'tabpanel' ) ).toHaveTextContent(
- 'Selected tab: Delta'
- );
+ // Re-render with beta disabled.
+ await rerender(
+
+ );
+
+ // Beta continues to be selected and focused, even if it is disabled.
+ expect(
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Beta',
+ } )
+ ).toBeVisible();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Beta',
+ } )
+ ).toBeVisible();
+
+ // Re-enable beta.
+ await rerender(
+
+ );
+
+ // Beta continues to be selected and focused.
+ expect(
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Beta',
+ } )
+ ).toBeVisible();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Beta',
+ } )
+ ).toBeVisible();
+
+ expect( mockOnSelect ).not.toHaveBeenCalled();
+ } );
+
+ it( 'should keep the current tab selected by the user as selected even if it becomes disabled', async () => {
+ const mockOnSelect = jest.fn();
+
+ const { rerender } = await render(
+
+ );
+
+ // Alpha is automatically selected as the selected tab.
+ await waitForComponentToBeInitializedWithSelectedTab(
+ 'Alpha'
+ );
+
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 1 );
+ expect( mockOnSelect ).toHaveBeenLastCalledWith(
+ 'alpha'
+ );
+
+ // Click on beta tab, beta becomes selected.
+ await click(
+ screen.getByRole( 'tab', { name: 'Beta' } )
+ );
+
+ expect(
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Beta',
+ } )
+ ).toBeVisible();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Beta',
+ } )
+ ).toBeVisible();
+
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 2 );
+ expect( mockOnSelect ).toHaveBeenLastCalledWith(
+ 'beta'
+ );
+
+ // Re-render with beta disabled.
+ await rerender(
+
+ );
+
+ // Beta continues to be selected, even if it is disabled.
+ expect(
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Beta',
+ } )
+ ).toHaveFocus();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Beta',
+ } )
+ ).toBeVisible();
+
+ // Re-enable beta.
+ await rerender(
+
+ );
+
+ // Beta continues to be selected and focused.
+ expect(
+ screen.getByRole( 'tab', {
+ selected: true,
+ name: 'Beta',
+ } )
+ ).toBeVisible();
+ expect(
+ screen.getByRole( 'tabpanel', {
+ name: 'Beta',
+ } )
+ ).toBeVisible();
+
+ expect( mockOnSelect ).toHaveBeenCalledTimes( 2 );
+ } );
+ }
+ );
+ } );
} );
} );
diff --git a/packages/components/src/text-control/README.md b/packages/components/src/text-control/README.md
index 0b6e2d4ebc684..80f3145a4325b 100644
--- a/packages/components/src/text-control/README.md
+++ b/packages/components/src/text-control/README.md
@@ -63,6 +63,7 @@ const MyTextControl = () => {
return (
setClassName( value ) }
diff --git a/packages/components/src/text-control/index.tsx b/packages/components/src/text-control/index.tsx
index ea2d2c17bb9cf..83881542fe7b7 100644
--- a/packages/components/src/text-control/index.tsx
+++ b/packages/components/src/text-control/index.tsx
@@ -16,6 +16,7 @@ import { forwardRef } from '@wordpress/element';
import BaseControl from '../base-control';
import type { WordPressComponentProps } from '../context';
import type { TextControlProps } from './types';
+import { maybeWarnDeprecated36pxSize } from '../utils/deprecated-36px-size';
function UnforwardedTextControl(
props: WordPressComponentProps< TextControlProps, 'input', false >,
@@ -38,6 +39,12 @@ function UnforwardedTextControl(
const onChangeValue = ( event: ChangeEvent< HTMLInputElement > ) =>
onChange( event.target.value );
+ maybeWarnDeprecated36pxSize( {
+ componentName: 'TextControl',
+ size: undefined,
+ __next40pxDefaultSize,
+ } );
+
return (
setClassName( value ) }
diff --git a/packages/components/src/text-control/stories/index.story.tsx b/packages/components/src/text-control/stories/index.story.tsx
index bebdb2caf75f6..fe7fb538805da 100644
--- a/packages/components/src/text-control/stories/index.story.tsx
+++ b/packages/components/src/text-control/stories/index.story.tsx
@@ -15,12 +15,13 @@ import TextControl from '..';
const meta: Meta< typeof TextControl > = {
component: TextControl,
- title: 'Components/TextControl',
+ title: 'Components/Selection & Input/Common/TextControl',
+ id: 'components-textcontrol',
argTypes: {
help: { control: { type: 'text' } },
label: { control: { type: 'text' } },
onChange: { action: 'onChange' },
- value: { control: { type: null } },
+ value: { control: false },
},
parameters: {
controls: {
@@ -54,6 +55,7 @@ export const Default: StoryFn< typeof TextControl > = DefaultTemplate.bind(
);
Default.args = {
__nextHasNoMarginBottom: true,
+ __next40pxDefaultSize: true,
};
export const WithLabelAndHelpText: StoryFn< typeof TextControl > =
diff --git a/packages/components/src/text-control/test/text-control.tsx b/packages/components/src/text-control/test/text-control.tsx
index 19b17cae44361..7eb3a82d2fb5f 100644
--- a/packages/components/src/text-control/test/text-control.tsx
+++ b/packages/components/src/text-control/test/text-control.tsx
@@ -9,7 +9,13 @@ import { render, screen } from '@testing-library/react';
import _TextControl from '..';
const TextControl = ( props: React.ComponentProps< typeof _TextControl > ) => {
- return <_TextControl { ...props } __nextHasNoMarginBottom />;
+ return (
+ <_TextControl
+ { ...props }
+ __nextHasNoMarginBottom
+ __next40pxDefaultSize
+ />
+ );
};
const noop = () => {};
diff --git a/packages/components/src/textarea-control/stories/index.story.tsx b/packages/components/src/textarea-control/stories/index.story.tsx
index c303883a92c5d..3160e0bfe68f3 100644
--- a/packages/components/src/textarea-control/stories/index.story.tsx
+++ b/packages/components/src/textarea-control/stories/index.story.tsx
@@ -21,7 +21,7 @@ const meta: Meta< typeof TextareaControl > = {
onChange: { action: 'onChange' },
label: { control: { type: 'text' } },
help: { control: { type: 'text' } },
- value: { control: { type: null } },
+ value: { control: false },
},
parameters: {
controls: {
diff --git a/packages/components/src/theme/stories/index.story.tsx b/packages/components/src/theme/stories/index.story.tsx
index 67eec72533ff3..8ef87cbe8ddb4 100644
--- a/packages/components/src/theme/stories/index.story.tsx
+++ b/packages/components/src/theme/stories/index.story.tsx
@@ -37,7 +37,7 @@ export const Default = Template.bind( {} );
Default.args = {};
export const Nested: StoryFn< typeof Theme > = ( args ) => (
-
+
Outer theme (hardcoded)
diff --git a/packages/components/src/toggle-control/stories/index.story.tsx b/packages/components/src/toggle-control/stories/index.story.tsx
index b9db0474bc760..6511655810066 100644
--- a/packages/components/src/toggle-control/stories/index.story.tsx
+++ b/packages/components/src/toggle-control/stories/index.story.tsx
@@ -18,7 +18,7 @@ const meta: Meta< typeof ToggleControl > = {
id: 'components-togglecontrol',
component: ToggleControl,
argTypes: {
- checked: { control: { type: null } },
+ checked: { control: false },
help: { control: { type: 'text' } },
label: { control: { type: 'text' } },
onChange: { action: 'onChange' },
diff --git a/packages/components/src/toggle-group-control/stories/index.story.tsx b/packages/components/src/toggle-group-control/stories/index.story.tsx
index afdfa457f6634..0f3c0a299617a 100644
--- a/packages/components/src/toggle-group-control/stories/index.story.tsx
+++ b/packages/components/src/toggle-group-control/stories/index.story.tsx
@@ -32,7 +32,7 @@ const meta: Meta< typeof ToggleGroupControl > = {
argTypes: {
help: { control: { type: 'text' } },
onChange: { action: 'onChange' },
- value: { control: { type: null } },
+ value: { control: false },
},
parameters: {
controls: { expanded: true },
@@ -51,6 +51,7 @@ const Template: StoryFn< typeof ToggleGroupControl > = ( {
return (
{
setValue( ...changeArgs );
diff --git a/packages/components/src/toggle-group-control/test/__snapshots__/index.tsx.snap b/packages/components/src/toggle-group-control/test/__snapshots__/index.tsx.snap
index 832c6d7cb7a8c..91e9f291ddf01 100644
--- a/packages/components/src/toggle-group-control/test/__snapshots__/index.tsx.snap
+++ b/packages/components/src/toggle-group-control/test/__snapshots__/index.tsx.snap
@@ -44,8 +44,8 @@ exports[`ToggleGroupControl controlled should render correctly with icons 1`] =
display: inline-flex;
min-width: 0;
position: relative;
- min-height: 36px;
- padding: 2px;
+ min-height: 40px;
+ padding: 3px;
}
.emotion-8:hover {
@@ -72,7 +72,7 @@ exports[`ToggleGroupControl controlled should render correctly with icons 1`] =
content: '';
position: absolute;
pointer-events: none;
- background: #1e1e1e;
+ background: var(--wp-components-color-foreground, #1e1e1e);
outline: 2px solid transparent;
outline-offset: -3px;
--antialiasing-factor: 100;
@@ -134,7 +134,7 @@ exports[`ToggleGroupControl controlled should render correctly with icons 1`] =
background: transparent;
border: none;
border-radius: 1px;
- color: #757575;
+ color: var(--wp-components-color-gray-700, #757575);
fill: currentColor;
cursor: pointer;
display: -webkit-box;
@@ -158,12 +158,12 @@ exports[`ToggleGroupControl controlled should render correctly with icons 1`] =
user-select: none;
width: 100%;
z-index: 2;
- color: #1e1e1e;
- height: 30px;
+ color: var(--wp-components-color-foreground, #1e1e1e);
+ height: 32px;
aspect-ratio: 1;
padding-left: 0;
padding-right: 0;
- color: #fff;
+ color: var(--wp-components-color-foreground-inverted, #fff);
}
@media not ( prefers-reduced-motion ) {
@@ -183,7 +183,7 @@ exports[`ToggleGroupControl controlled should render correctly with icons 1`] =
}
.emotion-12:active {
- background: #fff;
+ background: var(--wp-components-color-background, #fff);
}
.emotion-12:active {
@@ -211,7 +211,7 @@ exports[`ToggleGroupControl controlled should render correctly with icons 1`] =
background: transparent;
border: none;
border-radius: 1px;
- color: #757575;
+ color: var(--wp-components-color-gray-700, #757575);
fill: currentColor;
cursor: pointer;
display: -webkit-box;
@@ -235,8 +235,8 @@ exports[`ToggleGroupControl controlled should render correctly with icons 1`] =
user-select: none;
width: 100%;
z-index: 2;
- color: #1e1e1e;
- height: 30px;
+ color: var(--wp-components-color-foreground, #1e1e1e);
+ height: 32px;
aspect-ratio: 1;
padding-left: 0;
padding-right: 0;
@@ -259,7 +259,7 @@ exports[`ToggleGroupControl controlled should render correctly with icons 1`] =
}
.emotion-17:active {
- background: #fff;
+ background: var(--wp-components-color-background, #fff);
}
@@ -409,8 +409,8 @@ exports[`ToggleGroupControl controlled should render correctly with text options
display: inline-flex;
min-width: 0;
position: relative;
- min-height: 36px;
- padding: 2px;
+ min-height: 40px;
+ padding: 3px;
}
.emotion-8:hover {
@@ -437,7 +437,7 @@ exports[`ToggleGroupControl controlled should render correctly with text options
content: '';
position: absolute;
pointer-events: none;
- background: #1e1e1e;
+ background: var(--wp-components-color-foreground, #1e1e1e);
outline: 2px solid transparent;
outline-offset: -3px;
--antialiasing-factor: 100;
@@ -499,7 +499,7 @@ exports[`ToggleGroupControl controlled should render correctly with text options
background: transparent;
border: none;
border-radius: 1px;
- color: #757575;
+ color: var(--wp-components-color-gray-700, #757575);
fill: currentColor;
cursor: pointer;
display: -webkit-box;
@@ -542,7 +542,7 @@ exports[`ToggleGroupControl controlled should render correctly with text options
}
.emotion-12:active {
- background: #fff;
+ background: var(--wp-components-color-background, #fff);
}
.emotion-13 {
@@ -678,8 +678,8 @@ exports[`ToggleGroupControl uncontrolled should render correctly with icons 1`]
display: inline-flex;
min-width: 0;
position: relative;
- min-height: 36px;
- padding: 2px;
+ min-height: 40px;
+ padding: 3px;
}
.emotion-8:hover {
@@ -706,7 +706,7 @@ exports[`ToggleGroupControl uncontrolled should render correctly with icons 1`]
content: '';
position: absolute;
pointer-events: none;
- background: #1e1e1e;
+ background: var(--wp-components-color-foreground, #1e1e1e);
outline: 2px solid transparent;
outline-offset: -3px;
--antialiasing-factor: 100;
@@ -768,7 +768,7 @@ exports[`ToggleGroupControl uncontrolled should render correctly with icons 1`]
background: transparent;
border: none;
border-radius: 1px;
- color: #757575;
+ color: var(--wp-components-color-gray-700, #757575);
fill: currentColor;
cursor: pointer;
display: -webkit-box;
@@ -792,12 +792,12 @@ exports[`ToggleGroupControl uncontrolled should render correctly with icons 1`]
user-select: none;
width: 100%;
z-index: 2;
- color: #1e1e1e;
- height: 30px;
+ color: var(--wp-components-color-foreground, #1e1e1e);
+ height: 32px;
aspect-ratio: 1;
padding-left: 0;
padding-right: 0;
- color: #fff;
+ color: var(--wp-components-color-foreground-inverted, #fff);
}
@media not ( prefers-reduced-motion ) {
@@ -817,7 +817,7 @@ exports[`ToggleGroupControl uncontrolled should render correctly with icons 1`]
}
.emotion-12:active {
- background: #fff;
+ background: var(--wp-components-color-background, #fff);
}
.emotion-12:active {
@@ -845,7 +845,7 @@ exports[`ToggleGroupControl uncontrolled should render correctly with icons 1`]
background: transparent;
border: none;
border-radius: 1px;
- color: #757575;
+ color: var(--wp-components-color-gray-700, #757575);
fill: currentColor;
cursor: pointer;
display: -webkit-box;
@@ -869,8 +869,8 @@ exports[`ToggleGroupControl uncontrolled should render correctly with icons 1`]
user-select: none;
width: 100%;
z-index: 2;
- color: #1e1e1e;
- height: 30px;
+ color: var(--wp-components-color-foreground, #1e1e1e);
+ height: 32px;
aspect-ratio: 1;
padding-left: 0;
padding-right: 0;
@@ -893,7 +893,7 @@ exports[`ToggleGroupControl uncontrolled should render correctly with icons 1`]
}
.emotion-17:active {
- background: #fff;
+ background: var(--wp-components-color-background, #fff);
}
@@ -1037,8 +1037,8 @@ exports[`ToggleGroupControl uncontrolled should render correctly with text optio
display: inline-flex;
min-width: 0;
position: relative;
- min-height: 36px;
- padding: 2px;
+ min-height: 40px;
+ padding: 3px;
}
.emotion-8:hover {
@@ -1065,7 +1065,7 @@ exports[`ToggleGroupControl uncontrolled should render correctly with text optio
content: '';
position: absolute;
pointer-events: none;
- background: #1e1e1e;
+ background: var(--wp-components-color-foreground, #1e1e1e);
outline: 2px solid transparent;
outline-offset: -3px;
--antialiasing-factor: 100;
@@ -1127,7 +1127,7 @@ exports[`ToggleGroupControl uncontrolled should render correctly with text optio
background: transparent;
border: none;
border-radius: 1px;
- color: #757575;
+ color: var(--wp-components-color-gray-700, #757575);
fill: currentColor;
cursor: pointer;
display: -webkit-box;
@@ -1170,7 +1170,7 @@ exports[`ToggleGroupControl uncontrolled should render correctly with text optio
}
.emotion-12:active {
- background: #fff;
+ background: var(--wp-components-color-background, #fff);
}
.emotion-13 {
diff --git a/packages/components/src/toggle-group-control/test/index.tsx b/packages/components/src/toggle-group-control/test/index.tsx
index 168e8f498958b..44cfda69c423c 100644
--- a/packages/components/src/toggle-group-control/test/index.tsx
+++ b/packages/components/src/toggle-group-control/test/index.tsx
@@ -28,7 +28,13 @@ const hoverOutside = async () => {
};
const ToggleGroupControl = ( props: ToggleGroupControlProps ) => {
- return <_ToggleGroupControl { ...props } __nextHasNoMarginBottom />;
+ return (
+ <_ToggleGroupControl
+ { ...props }
+ __nextHasNoMarginBottom
+ __next40pxDefaultSize
+ />
+ );
};
const ControlledToggleGroupControl = ( {
diff --git a/packages/components/src/toggle-group-control/toggle-group-control-option-base/styles.ts b/packages/components/src/toggle-group-control/toggle-group-control-option-base/styles.ts
index c0248f9b3f7f2..a53eced1219db 100644
--- a/packages/components/src/toggle-group-control/toggle-group-control-option-base/styles.ts
+++ b/packages/components/src/toggle-group-control/toggle-group-control-option-base/styles.ts
@@ -38,7 +38,7 @@ export const buttonView = ( {
background: transparent;
border: none;
border-radius: ${ CONFIG.radiusXSmall };
- color: ${ COLORS.gray[ 700 ] };
+ color: ${ COLORS.theme.gray[ 700 ] };
fill: currentColor;
cursor: pointer;
display: flex;
@@ -70,7 +70,7 @@ export const buttonView = ( {
}
&:active {
- background: ${ CONFIG.controlBackgroundColor };
+ background: ${ COLORS.ui.background };
}
${ isDeselectable && deselectable }
@@ -79,7 +79,7 @@ export const buttonView = ( {
`;
const pressed = css`
- color: ${ COLORS.white };
+ color: ${ COLORS.theme.foregroundInverted };
&:active {
background: transparent;
@@ -87,11 +87,11 @@ const pressed = css`
`;
const deselectable = css`
- color: ${ COLORS.gray[ 900 ] };
+ color: ${ COLORS.theme.foreground };
&:focus {
box-shadow:
- inset 0 0 0 1px ${ COLORS.white },
+ inset 0 0 0 1px ${ COLORS.ui.background },
0 0 0 ${ CONFIG.borderWidthFocus } ${ COLORS.theme.accent };
outline: 2px solid transparent;
}
@@ -112,7 +112,7 @@ const isIconStyles = ( {
};
return css`
- color: ${ COLORS.gray[ 900 ] };
+ color: ${ COLORS.theme.foreground };
height: ${ iconButtonSizes[ size ] };
aspect-ratio: 1;
padding-left: 0;
diff --git a/packages/components/src/toggle-group-control/toggle-group-control-option-icon/README.md b/packages/components/src/toggle-group-control/toggle-group-control-option-icon/README.md
index a0e3a44cf7460..1ee82b26a9199 100644
--- a/packages/components/src/toggle-group-control/toggle-group-control-option-icon/README.md
+++ b/packages/components/src/toggle-group-control/toggle-group-control-option-icon/README.md
@@ -17,7 +17,7 @@ import { formatLowercase, formatUppercase } from '@wordpress/icons';
function Example() {
return (
-
+
+ *
*
*
*
diff --git a/packages/components/src/toggle-group-control/toggle-group-control/README.md b/packages/components/src/toggle-group-control/toggle-group-control/README.md
index ca5c5d14eb6b5..841d474c148d4 100644
--- a/packages/components/src/toggle-group-control/toggle-group-control/README.md
+++ b/packages/components/src/toggle-group-control/toggle-group-control/README.md
@@ -25,6 +25,7 @@ function Example() {
value="vertical"
isBlock
__nextHasNoMarginBottom
+ __next40pxDefaultSize
>
@@ -100,4 +101,4 @@ Start opting into the larger default height that will become the default size in
Start opting into the new margin-free styles that will become the default in a future version.
- Required: No
-- Default: `false`
\ No newline at end of file
+- Default: `false`
diff --git a/packages/components/src/toggle-group-control/toggle-group-control/as-radio-group.tsx b/packages/components/src/toggle-group-control/toggle-group-control/as-radio-group.tsx
index 0166728dbafba..56fb5faca5638 100644
--- a/packages/components/src/toggle-group-control/toggle-group-control/as-radio-group.tsx
+++ b/packages/components/src/toggle-group-control/toggle-group-control/as-radio-group.tsx
@@ -3,7 +3,6 @@
*/
import type { ForwardedRef } from 'react';
import * as Ariakit from '@ariakit/react';
-import { useStoreState } from '@ariakit/react';
/**
* WordPress dependencies
@@ -70,7 +69,7 @@ function UnforwardedToggleGroupControlAsRadioGroup(
rtl: isRTL(),
} );
- const selectedValue = useStoreState( radio, 'value' );
+ const selectedValue = Ariakit.useStoreState( radio, 'value' );
const setValue = radio.setValue;
// Ensures that the active id is also reset after the value is "reset" by the consumer.
diff --git a/packages/components/src/toggle-group-control/toggle-group-control/component.tsx b/packages/components/src/toggle-group-control/toggle-group-control/component.tsx
index 0c3cadf210d84..9f3427e95a601 100644
--- a/packages/components/src/toggle-group-control/toggle-group-control/component.tsx
+++ b/packages/components/src/toggle-group-control/toggle-group-control/component.tsx
@@ -23,6 +23,7 @@ import { ToggleGroupControlAsButtonGroup } from './as-button-group';
import { useTrackElementOffsetRect } from '../../utils/element-rect';
import { useMergeRefs } from '@wordpress/compose';
import { useAnimatedOffsetRect } from '../../utils/hooks/use-animated-offset-rect';
+import { maybeWarnDeprecated36pxSize } from '../../utils/deprecated-36px-size';
function UnconnectedToggleGroupControl(
props: WordPressComponentProps< ToggleGroupControlProps, 'div', false >,
@@ -31,6 +32,7 @@ function UnconnectedToggleGroupControl(
const {
__nextHasNoMarginBottom = false,
__next40pxDefaultSize = false,
+ __shouldNotWarnDeprecated36pxSize,
className,
isAdaptiveWidth = false,
isBlock = false,
@@ -81,6 +83,13 @@ function UnconnectedToggleGroupControl(
? ToggleGroupControlAsButtonGroup
: ToggleGroupControlAsRadioGroup;
+ maybeWarnDeprecated36pxSize( {
+ componentName: 'ToggleGroupControl',
+ size,
+ __next40pxDefaultSize,
+ __shouldNotWarnDeprecated36pxSize,
+ } );
+
return (
*
*
diff --git a/packages/components/src/toggle-group-control/toggle-group-control/styles.ts b/packages/components/src/toggle-group-control/toggle-group-control/styles.ts
index bb6efe476b2b2..8376b66a5a86c 100644
--- a/packages/components/src/toggle-group-control/toggle-group-control/styles.ts
+++ b/packages/components/src/toggle-group-control/toggle-group-control/styles.ts
@@ -39,7 +39,7 @@ export const toggleGroupControl = ( {
content: '';
position: absolute;
pointer-events: none;
- background: ${ COLORS.gray[ 900 ] };
+ background: ${ COLORS.theme.foreground };
// Windows High Contrast mode will show this outline, but not the box-shadow.
outline: 2px solid transparent;
diff --git a/packages/components/src/toggle-group-control/types.ts b/packages/components/src/toggle-group-control/types.ts
index 463d8d26e6441..cfa9d00080467 100644
--- a/packages/components/src/toggle-group-control/types.ts
+++ b/packages/components/src/toggle-group-control/types.ts
@@ -128,6 +128,13 @@ export type ToggleGroupControlProps = Pick<
* @default false
*/
__next40pxDefaultSize?: boolean;
+ /**
+ * Do not throw a warning for the deprecated 36px default size.
+ * For internal components of other components that already throw the warning.
+ *
+ * @ignore
+ */
+ __shouldNotWarnDeprecated36pxSize?: boolean;
};
export type ToggleGroupControlContextProps = {
diff --git a/packages/components/src/toolbar/stories/index.story.tsx b/packages/components/src/toolbar/stories/index.story.tsx
index e4fb3b07e1c90..8590c1ec8a2c6 100644
--- a/packages/components/src/toolbar/stories/index.story.tsx
+++ b/packages/components/src/toolbar/stories/index.story.tsx
@@ -51,7 +51,7 @@ const meta: Meta< typeof Toolbar > = {
ToolbarDropdownMenu,
},
argTypes: {
- children: { control: { type: null } },
+ children: { control: false },
variant: {
options: [ undefined, 'unstyled' ],
control: { type: 'radio' },
diff --git a/packages/components/src/toolbar/toolbar-button/index.tsx b/packages/components/src/toolbar/toolbar-button/index.tsx
index bb591ff7b521c..ae1f54acbfc14 100644
--- a/packages/components/src/toolbar/toolbar-button/index.tsx
+++ b/packages/components/src/toolbar/toolbar-button/index.tsx
@@ -54,6 +54,7 @@ function UnforwardedToolbarButton(
{ ( toolbarItemProps ) => (
= {
// @ts-expect-error - See https://github.com/storybookjs/storybook/issues/23170
subcomponents: { ToolsPanelItem },
argTypes: {
- as: { control: { type: null } },
- children: { control: { type: null } },
- panelId: { control: { type: null } },
+ as: { control: false },
+ children: { control: false },
+ panelId: { control: false },
resetAll: { action: 'resetAll' },
},
parameters: {
@@ -74,6 +74,7 @@ export const Default: StoryFn< typeof ToolsPanel > = ( {
isShownByDefault
>
setWidth( next ) }
@@ -86,6 +87,7 @@ export const Default: StoryFn< typeof ToolsPanel > = ( {
isShownByDefault
>
setHeight( next ) }
@@ -98,6 +100,7 @@ export const Default: StoryFn< typeof ToolsPanel > = ( {
isShownByDefault
>
setMinHeight( next ) }
@@ -110,6 +113,7 @@ export const Default: StoryFn< typeof ToolsPanel > = ( {
>
setScale( next ) }
@@ -166,6 +170,7 @@ export const WithNonToolsPanelItems: StoryFn< typeof ToolsPanel > = ( {
isShownByDefault
>
setWidth( next ) }
@@ -178,6 +183,7 @@ export const WithNonToolsPanelItems: StoryFn< typeof ToolsPanel > = ( {
isShownByDefault
>
setHeight( next ) }
@@ -236,6 +242,7 @@ export const WithOptionalItemsPlusIcon: StoryFn< typeof ToolsPanel > = ( {
}
>
setMinWidth( next ) }
@@ -248,6 +255,7 @@ export const WithOptionalItemsPlusIcon: StoryFn< typeof ToolsPanel > = ( {
isShownByDefault={ false }
>
setWidth( next ) }
@@ -260,6 +268,7 @@ export const WithOptionalItemsPlusIcon: StoryFn< typeof ToolsPanel > = ( {
isShownByDefault={ false }
>
setHeight( next ) }
@@ -340,6 +349,7 @@ export const WithSlotFillItems: StoryFn< typeof ToolsPanel > = ( {
panelId={ panelId }
>
@@ -355,6 +365,7 @@ export const WithSlotFillItems: StoryFn< typeof ToolsPanel > = ( {
panelId={ panelId }
>
@@ -440,6 +451,7 @@ export const WithConditionalDefaultControl: StoryFn< typeof ToolsPanel > = ( {
isShownByDefault
>
@@ -457,6 +469,7 @@ export const WithConditionalDefaultControl: StoryFn< typeof ToolsPanel > = ( {
>
@@ -539,6 +552,7 @@ export const WithConditionallyRenderedControl: StoryFn<
isShownByDefault
>
@@ -559,6 +573,7 @@ export const WithConditionallyRenderedControl: StoryFn<
>
diff --git a/packages/components/src/tools-panel/test/index.tsx b/packages/components/src/tools-panel/test/index.tsx
index 8d2c4f17a8b17..68cf72233121e 100644
--- a/packages/components/src/tools-panel/test/index.tsx
+++ b/packages/components/src/tools-panel/test/index.tsx
@@ -154,7 +154,6 @@ const renderWrappedItemInPanel = () => {
const renderPanel = () => {
return render(
- { false && Hidden
}
Example control
@@ -236,22 +235,6 @@ describe( 'ToolsPanel', () => {
it( 'should not render panel menu when there are no panel items', () => {
render(
- { false && (
- false }
- >
- Should not show
-
- ) }
- { false && (
- false }
- >
- Not shown either
-
- ) }
Visible but insignificant
);
diff --git a/packages/components/src/tools-panel/tools-panel/README.md b/packages/components/src/tools-panel/tools-panel/README.md
index 1daa7537335e1..b5e6860e2bd07 100644
--- a/packages/components/src/tools-panel/tools-panel/README.md
+++ b/packages/components/src/tools-panel/tools-panel/README.md
@@ -101,6 +101,7 @@ export function DimensionPanel() {
isShownByDefault
>
setPadding( undefined ) }
>
setMargin( undefined ) }
>
setHeight() }
* >
* setWidth() }
* >
* = {
id: 'components-tooltip',
component: Tooltip,
argTypes: {
- children: { control: { type: null } },
+ children: { control: false },
position: {
control: { type: 'select' },
options: [
diff --git a/packages/components/src/tree-grid/cell.tsx b/packages/components/src/tree-grid/cell.tsx
index a9883eb051226..08261abe10313 100644
--- a/packages/components/src/tree-grid/cell.tsx
+++ b/packages/components/src/tree-grid/cell.tsx
@@ -21,7 +21,11 @@ function UnforwardedTreeGridCell(
return (
{ withoutGridItem ? (
- <>{ children }>
+ <>
+ { typeof children === 'function'
+ ? children( { ...props, ref } )
+ : children }
+ >
) : (
{ children }
) }
diff --git a/packages/components/src/tree-grid/stories/index.story.tsx b/packages/components/src/tree-grid/stories/index.story.tsx
index 5a1ed95e1fd62..e113103897dbd 100644
--- a/packages/components/src/tree-grid/stories/index.story.tsx
+++ b/packages/components/src/tree-grid/stories/index.story.tsx
@@ -22,7 +22,7 @@ const meta: Meta< typeof TreeGrid > = {
// @ts-expect-error - See https://github.com/storybookjs/storybook/issues/23170
subcomponents: { TreeGridRow, TreeGridCell },
argTypes: {
- children: { control: { type: null } },
+ children: { control: false },
},
parameters: {
actions: { argTypesRegex: '^on.*' },
@@ -111,6 +111,7 @@ const Rows = ( {
label="Description"
hideLabelFromVision
placeholder="Description"
+ __next40pxDefaultSize
{ ...props }
/>
) }
@@ -121,6 +122,7 @@ const Rows = ( {
label="Notes"
hideLabelFromVision
placeholder="Notes"
+ __next40pxDefaultSize
{ ...props }
/>
) }
diff --git a/packages/components/src/tree-grid/types.ts b/packages/components/src/tree-grid/types.ts
index 31d04882d1a81..f4946ba58ca8a 100644
--- a/packages/components/src/tree-grid/types.ts
+++ b/packages/components/src/tree-grid/types.ts
@@ -36,7 +36,7 @@ export type TreeGridRowProps = {
type RovingTabIndexItemPassThruProps = {
ref: React.ForwardedRef< any >;
tabIndex?: number;
- onFocus: React.FocusEventHandler< any >;
+ onFocus?: React.FocusEventHandler< any >;
[ key: string ]: any;
};
diff --git a/packages/components/src/tree-select/README.md b/packages/components/src/tree-select/README.md
index 3d26488478bd0..493c83bf993b0 100644
--- a/packages/components/src/tree-select/README.md
+++ b/packages/components/src/tree-select/README.md
@@ -1,10 +1,10 @@
# TreeSelect
-TreeSelect component is used to generate select input fields.
+
-## Usage
+See the WordPress Storybook for more detailed, interactive documentation.
-Render a user interface to select the parent page in a hierarchy of pages:
+Generates a hierarchical select input.
```jsx
import { useState } from 'react';
@@ -15,7 +15,8 @@ const MyTreeSelect = () => {
return (
setPage( newPage ) }
@@ -50,51 +51,165 @@ const MyTreeSelect = () => {
);
}
```
-
## Props
-The set of props accepted by the component will be specified below.
-Props not included in this set will be applied to the SelectControl component being used.
+### `__next40pxDefaultSize`
+
+Start opting into the larger default height that will become the default size in a future version.
+
+ - Type: `boolean`
+ - Required: No
+ - Default: `false`
+
+### `__nextHasNoMarginBottom`
+
+Start opting into the new margin-free styles that will become the default in a future version.
+
+ - Type: `boolean`
+ - Required: No
+ - Default: `false`
+
+### `children`
+
+As an alternative to the `options` prop, `optgroup`s and `options` can be
+passed in as `children` for more customizability.
+
+ - Type: `ReactNode`
+ - Required: No
+
+### `disabled`
-### label
+If true, the `input` will be disabled.
+
+ - Type: `boolean`
+ - Required: No
+ - Default: `false`
+
+### `hideLabelFromVision`
+
+If true, the label will only be visible to screen readers.
+
+ - Type: `boolean`
+ - Required: No
+ - Default: `false`
+
+### `help`
+
+Additional description for the control.
+
+Only use for meaningful description or instructions for the control. An element containing the description will be programmatically associated to the BaseControl by the means of an `aria-describedby` attribute.
+
+ - Type: `ReactNode`
+ - Required: No
+
+### `label`
If this property is added, a label will be generated using label property as the content.
-- Type: `String`
-- Required: No
+ - Type: `ReactNode`
+ - Required: No
+
+### `labelPosition`
+
+The position of the label.
-### noOptionLabel
+ - Type: `"top" | "bottom" | "side" | "edge"`
+ - Required: No
+ - Default: `'top'`
+
+### `noOptionLabel`
If this property is added, an option will be added with this label to represent empty selection.
-- Type: `String`
-- Required: No
+ - Type: `string`
+ - Required: No
+
+### `onChange`
+
+A function that receives the value of the new option that is being selected as input.
+
+ - Type: `(value: string, extra?: { event?: ChangeEvent; }) => void`
+ - Required: No
+
+### `options`
+
+An array of option property objects to be rendered,
+each with a `label` and `value` property, as well as any other
+`` attributes.
-### onChange
+ - Type: `readonly ({ label: string; value: string; } & Omit, "label" | "value">)[]`
+ - Required: No
-A function that receives the id of the new node element that is being selected.
+### `prefix`
+
+Renders an element on the left side of the input.
+
+By default, the prefix is aligned with the edge of the input border, with no padding.
+If you want to apply standard padding in accordance with the size variant, wrap the element in
+the provided `` component.
+
+```jsx
+import {
+ __experimentalInputControl as InputControl,
+ __experimentalInputControlPrefixWrapper as InputControlPrefixWrapper,
+} from '@wordpress/components';
+
+@ }
+/>
+```
-- Type: `function`
-- Required: Yes
+ - Type: `ReactNode`
+ - Required: No
-### selectedId
+### `selectedId`
The id of the currently selected node.
-- Type: `string` | `string[]`
-- Required: No
+ - Type: `string`
+ - Required: No
-### tree
+### `size`
+
+Adjusts the size of the input.
+
+ - Type: `"default" | "small" | "compact" | "__unstable-large"`
+ - Required: No
+ - Default: `'default'`
+
+### `suffix`
+
+Renders an element on the right side of the input.
+
+By default, the suffix is aligned with the edge of the input border, with no padding.
+If you want to apply standard padding in accordance with the size variant, wrap the element in
+the provided `` component.
+
+```jsx
+import {
+ __experimentalInputControl as InputControl,
+ __experimentalInputControlSuffixWrapper as InputControlSuffixWrapper,
+} from '@wordpress/components';
+
+% }
+/>
+```
+
+ - Type: `ReactNode`
+ - Required: No
+
+### `tree`
An array containing the tree objects with the possible nodes the user can select.
-- Type: `Object[]`
-- Required: No
+ - Type: `Tree[]`
+ - Required: No
-#### __nextHasNoMarginBottom
+### `variant`
-Start opting into the new margin-free styles that will become the default in a future version.
+The style variant of the control.
-- Type: `Boolean`
-- Required: No
-- Default: `false`
+ - Type: `"default" | "minimal"`
+ - Required: No
+ - Default: `'default'`
diff --git a/packages/components/src/tree-select/docs-manifest.json b/packages/components/src/tree-select/docs-manifest.json
new file mode 100644
index 0000000000000..0e74d71d309e1
--- /dev/null
+++ b/packages/components/src/tree-select/docs-manifest.json
@@ -0,0 +1,5 @@
+{
+ "$schema": "../../schemas/docs-manifest.json",
+ "displayName": "TreeSelect",
+ "filePath": "./index.tsx"
+}
diff --git a/packages/components/src/tree-select/index.tsx b/packages/components/src/tree-select/index.tsx
index bd92807bff4cc..6611657636162 100644
--- a/packages/components/src/tree-select/index.tsx
+++ b/packages/components/src/tree-select/index.tsx
@@ -11,6 +11,7 @@ import { SelectControl } from '../select-control';
import type { TreeSelectProps, Tree, Truthy } from './types';
import { useDeprecated36pxDefaultSizeProp } from '../utils/use-deprecated-props';
import { ContextSystemProvider } from '../context';
+import { maybeWarnDeprecated36pxSize } from '../utils/deprecated-36px-size';
const CONTEXT_VALUE = {
BaseControl: {
@@ -35,11 +36,11 @@ function getSelectOptions(
}
/**
- * TreeSelect component is used to generate select input fields.
+ * Generates a hierarchical select input.
*
* ```jsx
+ * import { useState } from 'react';
* import { TreeSelect } from '@wordpress/components';
- * import { useState } from '@wordpress/element';
*
* const MyTreeSelect = () => {
* const [ page, setPage ] = useState( 'p21' );
@@ -47,6 +48,7 @@ function getSelectOptions(
* return (
* setPage( newPage ) }
@@ -99,9 +101,16 @@ export function TreeSelect( props: TreeSelectProps ) {
].filter( < T, >( option: T ): option is Truthy< T > => !! option );
}, [ noOptionLabel, tree ] );
+ maybeWarnDeprecated36pxSize( {
+ componentName: 'TreeSelect',
+ size: restProps.size,
+ __next40pxDefaultSize: restProps.__next40pxDefaultSize,
+ } );
+
return (
= {
label: { control: { type: 'text' } },
prefix: { control: { type: 'text' } },
suffix: { control: { type: 'text' } },
- selectedId: { control: { type: null } },
+ selectedId: { control: false },
},
parameters: {
controls: {
@@ -50,6 +50,7 @@ const TreeSelectWithState: StoryFn< typeof TreeSelect > = ( props ) => {
export const Default = TreeSelectWithState.bind( {} );
Default.args = {
__nextHasNoMarginBottom: true,
+ __next40pxDefaultSize: true,
label: 'Label Text',
noOptionLabel: 'No parent page',
help: 'Help text to explain the select control.',
diff --git a/packages/components/src/tree-select/types.ts b/packages/components/src/tree-select/types.ts
index da90ece3a658e..59e8e173fab02 100644
--- a/packages/components/src/tree-select/types.ts
+++ b/packages/components/src/tree-select/types.ts
@@ -16,11 +16,18 @@ export interface Tree {
// `TreeSelect` inherits props from `SelectControl`, but only
// in single selection mode (ie. when the `multiple` prop is not defined).
export interface TreeSelectProps
- extends Omit< SelectControlSingleSelectionProps, 'value' | 'multiple' > {
+ extends Omit<
+ SelectControlSingleSelectionProps,
+ 'value' | 'multiple' | 'onChange'
+ > {
/**
* If this property is added, an option will be added with this label to represent empty selection.
*/
noOptionLabel?: string;
+ /**
+ * A function that receives the value of the new option that is being selected as input.
+ */
+ onChange?: SelectControlSingleSelectionProps[ 'onChange' ];
/**
* An array containing the tree objects with the possible nodes the user can select.
*/
diff --git a/packages/components/src/unit-control/README.md b/packages/components/src/unit-control/README.md
index dd54de80357d8..65d9e4b74062d 100644
--- a/packages/components/src/unit-control/README.md
+++ b/packages/components/src/unit-control/README.md
@@ -15,7 +15,7 @@ import { __experimentalUnitControl as UnitControl } from '@wordpress/components'
const Example = () => {
const [ value, setValue ] = useState( '10px' );
- return ;
+ return ;
};
```
@@ -128,7 +128,7 @@ const Example = () => {
];
return (
-
+
);
};
```
@@ -143,7 +143,7 @@ For example, a `value` of `50%` will set the current unit to `%`.
Example:
```jsx
-
+
```
- Required: No
diff --git a/packages/components/src/unit-control/index.tsx b/packages/components/src/unit-control/index.tsx
index 2dd08cc155225..65e1e56cda3b3 100644
--- a/packages/components/src/unit-control/index.tsx
+++ b/packages/components/src/unit-control/index.tsx
@@ -27,6 +27,7 @@ import { useControlledState } from '../utils/hooks';
import { escapeRegExp } from '../utils/strings';
import type { UnitControlProps, UnitControlOnChangeCallback } from './types';
import { useDeprecated36pxDefaultSizeProp } from '../utils/use-deprecated-props';
+import { maybeWarnDeprecated36pxSize } from '../utils/deprecated-36px-size';
function UnforwardedUnitControl(
unitControlProps: WordPressComponentProps<
@@ -55,9 +56,17 @@ function UnforwardedUnitControl(
units: unitsProp = CSS_UNITS,
value: valueProp,
onFocus: onFocusProp,
+ __shouldNotWarnDeprecated36pxSize,
...props
} = useDeprecated36pxDefaultSizeProp( unitControlProps );
+ maybeWarnDeprecated36pxSize( {
+ componentName: 'UnitControl',
+ __next40pxDefaultSize: props.__next40pxDefaultSize,
+ size,
+ __shouldNotWarnDeprecated36pxSize,
+ } );
+
if ( 'unit' in unitControlProps ) {
deprecated( 'UnitControl unit prop', {
since: '5.6',
@@ -215,6 +224,7 @@ function UnforwardedUnitControl(
return (
{
* const [ value, setValue ] = useState( '10px' );
*
- * return ;
+ * return ;
* };
* ```
*/
diff --git a/packages/components/src/unit-control/stories/index.story.tsx b/packages/components/src/unit-control/stories/index.story.tsx
index de8f476e26e5c..3a9da70ad5095 100644
--- a/packages/components/src/unit-control/stories/index.story.tsx
+++ b/packages/components/src/unit-control/stories/index.story.tsx
@@ -20,11 +20,11 @@ const meta: Meta< typeof UnitControl > = {
id: 'components-experimental-unitcontrol',
argTypes: {
__unstableInputWidth: { control: { type: 'text' } },
- __unstableStateReducer: { control: { type: null } },
- onChange: { control: { type: null } },
- onUnitChange: { control: { type: null } },
+ __unstableStateReducer: { control: false },
+ onChange: { control: false },
+ onUnitChange: { control: false },
prefix: { control: { type: 'text' } },
- value: { control: { type: null } },
+ value: { control: false },
},
parameters: {
actions: { argTypesRegex: '^on.*' },
@@ -59,6 +59,7 @@ export const Default: StoryFn< typeof UnitControl > = DefaultTemplate.bind(
);
Default.args = {
label: 'Label',
+ __next40pxDefaultSize: true,
};
/**
diff --git a/packages/components/src/unit-control/test/index.tsx b/packages/components/src/unit-control/test/index.tsx
index d91498d46478b..ad98d57cae640 100644
--- a/packages/components/src/unit-control/test/index.tsx
+++ b/packages/components/src/unit-control/test/index.tsx
@@ -12,9 +12,13 @@ import { useState } from '@wordpress/element';
/**
* Internal dependencies
*/
-import UnitControl from '..';
+import _UnitControl from '..';
import { CSS_UNITS, parseQuantityAndUnitFromRawValue } from '../utils';
+const UnitControl = ( props: React.ComponentProps< typeof _UnitControl > ) => (
+ <_UnitControl __next40pxDefaultSize { ...props } />
+);
+
const getInput = ( {
isInputTypeText = false,
}: {
diff --git a/packages/components/src/unit-control/types.ts b/packages/components/src/unit-control/types.ts
index 9164502668a2b..891945b422862 100644
--- a/packages/components/src/unit-control/types.ts
+++ b/packages/components/src/unit-control/types.ts
@@ -107,4 +107,11 @@ export type UnitControlProps = Pick< InputControlProps, 'size' > &
* Callback when either the quantity or the unit inputs gains focus.
*/
onFocus?: FocusEventHandler< HTMLInputElement | HTMLSelectElement >;
+ /**
+ * Do not throw a warning for the deprecated 36px default size.
+ * For internal components of other components that already throw the warning.
+ *
+ * @ignore
+ */
+ __shouldNotWarnDeprecated36pxSize?: boolean;
};
diff --git a/packages/components/src/utils/colors-values.js b/packages/components/src/utils/colors-values.js
index 1ad528d13f010..439d464f1460b 100644
--- a/packages/components/src/utils/colors-values.js
+++ b/packages/components/src/utils/colors-values.js
@@ -75,6 +75,9 @@ export const COLORS = Object.freeze( {
* @deprecated Use semantic aliases in `COLORS.ui` or theme-ready variables in `COLORS.theme.gray`.
*/
gray: GRAY, // TODO: Stop exporting this when everything is migrated to `theme` or `ui`
+ /**
+ * @deprecated Prefer theme-ready variables in `COLORS.theme`.
+ */
white,
alert: ALERT,
/**
diff --git a/packages/components/src/utils/config-values.js b/packages/components/src/utils/config-values.js
index 1bc3945f9b3b1..13b704540e9c4 100644
--- a/packages/components/src/utils/config-values.js
+++ b/packages/components/src/utils/config-values.js
@@ -12,7 +12,6 @@ const CONTROL_PROPS = {
controlPaddingXSmall: 8,
controlPaddingXLarge: 12 * 1.3334, // TODO: Deprecate
- controlBackgroundColor: COLORS.white,
controlBoxShadowFocus: `0 0 0 0.5px ${ COLORS.theme.accent }`,
controlHeight: CONTROL_HEIGHT,
controlHeightXSmall: `calc( ${ CONTROL_HEIGHT } * 0.6 )`,
diff --git a/packages/components/src/utils/deprecated-36px-size.ts b/packages/components/src/utils/deprecated-36px-size.ts
index be5baa2515637..0f87c2cd270c7 100644
--- a/packages/components/src/utils/deprecated-36px-size.ts
+++ b/packages/components/src/utils/deprecated-36px-size.ts
@@ -7,12 +7,15 @@ export function maybeWarnDeprecated36pxSize( {
componentName,
__next40pxDefaultSize,
size,
+ __shouldNotWarnDeprecated36pxSize,
}: {
componentName: string;
__next40pxDefaultSize: boolean | undefined;
size: string | undefined;
+ __shouldNotWarnDeprecated36pxSize?: boolean;
} ) {
if (
+ __shouldNotWarnDeprecated36pxSize ||
__next40pxDefaultSize ||
( size !== undefined && size !== 'default' )
) {
diff --git a/packages/components/src/view/stories/index.story.tsx b/packages/components/src/view/stories/index.story.tsx
index 324825059deb2..ee4bb71b8a243 100644
--- a/packages/components/src/view/stories/index.story.tsx
+++ b/packages/components/src/view/stories/index.story.tsx
@@ -12,7 +12,7 @@ const meta: Meta< typeof View > = {
component: View,
title: 'Components (Experimental)/View',
argTypes: {
- as: { control: { type: null } },
+ as: { control: false },
children: { control: { type: 'text' } },
},
parameters: {
diff --git a/packages/components/src/visually-hidden/stories/index.story.tsx b/packages/components/src/visually-hidden/stories/index.story.tsx
index 6ebcf9a2e949c..1c79718ddf6e0 100644
--- a/packages/components/src/visually-hidden/stories/index.story.tsx
+++ b/packages/components/src/visually-hidden/stories/index.story.tsx
@@ -13,7 +13,7 @@ const meta: Meta< typeof VisuallyHidden > = {
title: 'Components/Typography/VisuallyHidden',
id: 'components-visuallyhidden',
argTypes: {
- children: { control: { type: null } },
+ children: { control: false },
as: { control: { type: 'text' } },
},
parameters: {
diff --git a/packages/components/src/z-stack/stories/index.story.tsx b/packages/components/src/z-stack/stories/index.story.tsx
index 46a364bc520f3..53c7d950e75b1 100644
--- a/packages/components/src/z-stack/stories/index.story.tsx
+++ b/packages/components/src/z-stack/stories/index.story.tsx
@@ -16,7 +16,7 @@ const meta: Meta< typeof ZStack > = {
title: 'Components (Experimental)/ZStack',
argTypes: {
as: { control: { type: 'text' } },
- children: { control: { type: null } },
+ children: { control: false },
},
parameters: {
controls: {
diff --git a/packages/compose/CHANGELOG.md b/packages/compose/CHANGELOG.md
index 8f8bedfde15d4..452eacbe289ff 100644
--- a/packages/compose/CHANGELOG.md
+++ b/packages/compose/CHANGELOG.md
@@ -2,6 +2,12 @@
## Unreleased
+## 7.14.0 (2024-12-11)
+
+## 7.13.0 (2024-11-27)
+
+## 7.12.0 (2024-11-16)
+
## 7.11.0 (2024-10-30)
## 7.10.0 (2024-10-16)
diff --git a/packages/compose/package.json b/packages/compose/package.json
index 68b00b24298d8..6afbb1c954f47 100644
--- a/packages/compose/package.json
+++ b/packages/compose/package.json
@@ -1,6 +1,6 @@
{
"name": "@wordpress/compose",
- "version": "7.11.0",
+ "version": "7.14.0",
"description": "WordPress higher-order components (HOCs).",
"author": "The WordPress Contributors",
"license": "GPL-2.0-or-later",
diff --git a/packages/core-commands/CHANGELOG.md b/packages/core-commands/CHANGELOG.md
index b98e0c55e24ff..fe5f12707b086 100644
--- a/packages/core-commands/CHANGELOG.md
+++ b/packages/core-commands/CHANGELOG.md
@@ -2,6 +2,12 @@
## Unreleased
+## 1.14.0 (2024-12-11)
+
+## 1.13.0 (2024-11-27)
+
+## 1.12.0 (2024-11-16)
+
## 1.11.0 (2024-10-30)
## 1.10.0 (2024-10-16)
diff --git a/packages/core-commands/package.json b/packages/core-commands/package.json
index db2fb900865c8..32cd82f4686b0 100644
--- a/packages/core-commands/package.json
+++ b/packages/core-commands/package.json
@@ -1,6 +1,6 @@
{
"name": "@wordpress/core-commands",
- "version": "1.11.0",
+ "version": "1.14.0",
"description": "WordPress core reusable commands.",
"author": "The WordPress Contributors",
"license": "GPL-2.0-or-later",
diff --git a/packages/core-commands/src/admin-navigation-commands.js b/packages/core-commands/src/admin-navigation-commands.js
index 8a8167bb29b82..f82faa05baae4 100644
--- a/packages/core-commands/src/admin-navigation-commands.js
+++ b/packages/core-commands/src/admin-navigation-commands.js
@@ -44,11 +44,7 @@ const getAddNewPageCommand = () =>
}
);
if ( page?.id ) {
- history.push( {
- postId: page.id,
- postType: 'page',
- canvas: 'edit',
- } );
+ history.navigate( `/page/${ page.id }?canvas=edit` );
}
} catch ( error ) {
const errorMessage =
diff --git a/packages/core-commands/src/site-editor-navigation-commands.js b/packages/core-commands/src/site-editor-navigation-commands.js
index 2785d809d41e0..c1b12a84d4d61 100644
--- a/packages/core-commands/src/site-editor-navigation-commands.js
+++ b/packages/core-commands/src/site-editor-navigation-commands.js
@@ -136,19 +136,18 @@ const getNavigationCommandLoaderPerPostType = ( postType ) =>
return {
...command,
callback: ( { close } ) => {
- const args = {
- postType,
- postId: record.id,
- canvas: 'edit',
- };
- const targetUrl = addQueryArgs(
- 'site-editor.php',
- args
- );
if ( isSiteEditor ) {
- history.push( args );
+ history.navigate(
+ `/${ postType }/${ record.id }?canvas=edit`
+ );
} else {
- document.location = targetUrl;
+ document.location = addQueryArgs(
+ 'site-editor.php',
+ {
+ p: `/${ postType }/${ record.id }`,
+ canvas: 'edit',
+ }
+ );
}
close();
},
@@ -220,19 +219,18 @@ const getNavigationCommandLoaderPerTemplate = ( templateType ) =>
: __( '(no title)' ),
icon: icons[ templateType ],
callback: ( { close } ) => {
- const args = {
- postType: templateType,
- postId: record.id,
- canvas: 'edit',
- };
- const targetUrl = addQueryArgs(
- 'site-editor.php',
- args
- );
if ( isSiteEditor ) {
- history.push( args );
+ history.navigate(
+ `/${ templateType }/${ record.id }?canvas=edit`
+ );
} else {
- document.location = targetUrl;
+ document.location = addQueryArgs(
+ 'site-editor.php',
+ {
+ p: `/${ templateType }/${ record.id }`,
+ canvas: 'edit',
+ }
+ );
}
close();
},
@@ -249,18 +247,19 @@ const getNavigationCommandLoaderPerTemplate = ( templateType ) =>
label: __( 'Template parts' ),
icon: symbolFilled,
callback: ( { close } ) => {
- const args = {
- postType: 'wp_template_part',
- categoryId: 'all-parts',
- };
- const targetUrl = addQueryArgs(
- 'site-editor.php',
- args
- );
if ( isSiteEditor ) {
- history.push( args );
+ history.navigate(
+ '/pattern?postType=wp_template_part&categoryId=all-parts'
+ );
} else {
- document.location = targetUrl;
+ document.location = addQueryArgs(
+ 'site-editor.php',
+ {
+ p: '/pattern',
+ postType: 'wp_template_part',
+ categoryId: 'all-parts',
+ }
+ );
}
close();
},
@@ -303,17 +302,15 @@ const getSiteEditorBasicNavigationCommands = () =>
label: __( 'Navigation' ),
icon: navigation,
callback: ( { close } ) => {
- const args = {
- postType: 'wp_navigation',
- };
- const targetUrl = addQueryArgs(
- 'site-editor.php',
- args
- );
if ( isSiteEditor ) {
- history.push( args );
+ history.navigate( '/navigation' );
} else {
- document.location = targetUrl;
+ document.location = addQueryArgs(
+ 'site-editor.php',
+ {
+ p: '/navigation',
+ }
+ );
}
close();
},
@@ -324,17 +321,15 @@ const getSiteEditorBasicNavigationCommands = () =>
label: __( 'Styles' ),
icon: styles,
callback: ( { close } ) => {
- const args = {
- path: '/wp_global_styles',
- };
- const targetUrl = addQueryArgs(
- 'site-editor.php',
- args
- );
if ( isSiteEditor ) {
- history.push( args );
+ history.navigate( '/styles' );
} else {
- document.location = targetUrl;
+ document.location = addQueryArgs(
+ 'site-editor.php',
+ {
+ p: '/styles',
+ }
+ );
}
close();
},
@@ -345,17 +340,15 @@ const getSiteEditorBasicNavigationCommands = () =>
label: __( 'Pages' ),
icon: page,
callback: ( { close } ) => {
- const args = {
- postType: 'page',
- };
- const targetUrl = addQueryArgs(
- 'site-editor.php',
- args
- );
if ( isSiteEditor ) {
- history.push( args );
+ history.navigate( '/page' );
} else {
- document.location = targetUrl;
+ document.location = addQueryArgs(
+ 'site-editor.php',
+ {
+ p: '/page',
+ }
+ );
}
close();
},
@@ -366,17 +359,15 @@ const getSiteEditorBasicNavigationCommands = () =>
label: __( 'Templates' ),
icon: layout,
callback: ( { close } ) => {
- const args = {
- postType: 'wp_template',
- };
- const targetUrl = addQueryArgs(
- 'site-editor.php',
- args
- );
if ( isSiteEditor ) {
- history.push( args );
+ history.navigate( '/template' );
} else {
- document.location = targetUrl;
+ document.location = addQueryArgs(
+ 'site-editor.php',
+ {
+ p: '/template',
+ }
+ );
}
close();
},
@@ -389,17 +380,15 @@ const getSiteEditorBasicNavigationCommands = () =>
icon: symbol,
callback: ( { close } ) => {
if ( canCreateTemplate ) {
- const args = {
- postType: 'wp_block',
- };
- const targetUrl = addQueryArgs(
- 'site-editor.php',
- args
- );
if ( isSiteEditor ) {
- history.push( args );
+ history.navigate( '/pattern' );
} else {
- document.location = targetUrl;
+ document.location = addQueryArgs(
+ 'site-editor.php',
+ {
+ p: '/pattern',
+ }
+ );
}
close();
} else {
diff --git a/packages/core-data/CHANGELOG.md b/packages/core-data/CHANGELOG.md
index 31b44fa32dd0a..90395f58fc802 100644
--- a/packages/core-data/CHANGELOG.md
+++ b/packages/core-data/CHANGELOG.md
@@ -2,6 +2,12 @@
## Unreleased
+## 7.14.0 (2024-12-11)
+
+## 7.13.0 (2024-11-27)
+
+## 7.12.0 (2024-11-16)
+
## 7.11.0 (2024-10-30)
## 7.10.0 (2024-10-16)
diff --git a/packages/core-data/README.md b/packages/core-data/README.md
index 079f95ddbfc7a..9549e6742d8cd 100644
--- a/packages/core-data/README.md
+++ b/packages/core-data/README.md
@@ -581,7 +581,7 @@ _Parameters_
- _state_ `State`: State tree
- _kind_ `string`: Entity kind.
- _name_ `string`: Entity name.
-- _key_ `EntityRecordKey`: Record's key
+- _key_ `EntityRecordKey`: Optional record's key. If requesting a global record (e.g. site settings), the key can be omitted. If requesting a specific item, the key must always be included.
- _query_ `GetRecordsHttpQuery`: Optional query. If requesting specific fields, fields must always include the ID. For valid query parameters see the [Reference](https://developer.wordpress.org/rest-api/reference/) in the REST API Handbook and select the entity kind. Then see the arguments available "Retrieve a [Entity kind]".
_Returns_
@@ -1083,6 +1083,8 @@ function PageRenameForm( { id } ) {
return (