diff --git a/data/schemas/definitions.jsonschema b/data/schemas/definitions.jsonschema
index a1fdd2dcc680..c0c541ebb7a4 100644
--- a/data/schemas/definitions.jsonschema
+++ b/data/schemas/definitions.jsonschema
@@ -86,6 +86,10 @@
"maxLength": 7,
"pattern": "^[A-Z][A-Zs_0-9]*$"
},
+ "snake_case": {
+ "type": "string",
+ "pattern": "^[a-z][a-z0-9_]*$"
+ },
"layout_macro": {
"oneOf": [
{
diff --git a/keyboards/acheron/themis/88htsc/keymaps/via/rules.mk b/keyboards/acheron/themis/88htsc/keymaps/via/rules.mk
index 1e5b99807cb7..f1adcab005e8 100644
--- a/keyboards/acheron/themis/88htsc/keymaps/via/rules.mk
+++ b/keyboards/acheron/themis/88htsc/keymaps/via/rules.mk
@@ -1 +1,2 @@
VIA_ENABLE = yes
+ENCODER_MAP_ENABLE = yes
diff --git a/keyboards/ai03/duet/keymaps/coordinate/keymap.c b/keyboards/ai03/duet/keymaps/coordinate/keymap.c
new file mode 100644
index 000000000000..821c0295343f
--- /dev/null
+++ b/keyboards/ai03/duet/keymaps/coordinate/keymap.c
@@ -0,0 +1,34 @@
+// Copyright 2023 QMK
+// SPDX-License-Identifier: GPL-2.0-or-later
+
+#include QMK_KEYBOARD_H
+
+
+/* Coordinate keymap - designed to send a string in the format [a-r][0-5] corresponding to the matrix position, where a0 is the top left and r5 is bottom right */
+
+const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
+
+ [0] = LAYOUT(
+ KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0,
+ KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0,
+ KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0,
+ KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0,
+ KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0,
+ KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0, KC_0
+ ),
+};
+
+
+/* String generation */
+
+bool process_record_user(uint16_t keycode, keyrecord_t *record) {
+
+ if (record->event.pressed) {
+ char col = record->event.key.col + 'a';
+ char row = record->event.key.row + '0';
+ char result[2] = {col, row};
+ send_string(result);
+ }
+
+ return false;
+}
diff --git a/keyboards/ai03/duet/keymaps/coordinate/readme.md b/keyboards/ai03/duet/keymaps/coordinate/readme.md
new file mode 100644
index 000000000000..de8245875d11
--- /dev/null
+++ b/keyboards/ai03/duet/keymaps/coordinate/readme.md
@@ -0,0 +1,4 @@
+# The coordinate keymap for Duet
+
+An example keymap which sends a string `[a-r][0-5]` corresponding to the column-row position of the pressed switch.
+Possibly useful for pairing to a kiosk device provided the display application can parse and handle this format.
diff --git a/keyboards/bestway/halconf.h b/keyboards/bestway/halconf.h
index 76f44ff900a3..5028cac1eb4b 100644
--- a/keyboards/bestway/halconf.h
+++ b/keyboards/bestway/halconf.h
@@ -13,7 +13,6 @@
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
-
#pragma once
#define HAL_USE_PWM TRUE
diff --git a/keyboards/bredworks/wyvern_hs/keymaps/regular_numpad/keymap.c b/keyboards/bredworks/wyvern_hs/keymaps/regular_numpad/keymap.c
new file mode 100644
index 000000000000..78037bac14ab
--- /dev/null
+++ b/keyboards/bredworks/wyvern_hs/keymaps/regular_numpad/keymap.c
@@ -0,0 +1,33 @@
+/* Copyright 2023 DeskDaily
+ *
+ * This program is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 2 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+ * GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ */
+#include QMK_KEYBOARD_H
+
+const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
+ [0] = LAYOUT_regular_numpad(
+ KC_NUM, KC_PSLS, KC_PAST, KC_PMNS, KC_ESC, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, KC_MINS, KC_EQL, KC_BSPC, KC_DEL,
+ KC_P7, KC_P8, KC_P9, KC_PPLS, KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_LBRC, KC_RBRC, KC_BSLS, KC_PGUP,
+ KC_P4, KC_P5, KC_P6, KC_CAPS, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT, KC_ENT, KC_PGDN,
+ KC_P1, KC_P2, KC_P3, KC_ENT, KC_LSFT, KC_BSLS, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_LSFT, MO(1), KC_UP,
+ KC_P0, KC_DEL, KC_LCTL, KC_LGUI, KC_LALT, KC_SPC, KC_SPC, KC_SPC, KC_RALT, KC_RGUI, KC_RCTL, KC_LEFT, KC_DOWN, KC_RGHT, KC_BSPC
+ ),
+ [1] = LAYOUT_regular_numpad(
+ _______, _______, _______, _______, QK_BOOT, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______,
+ _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______, _______
+ )
+};
\ No newline at end of file
diff --git a/keyboards/etiennecollin/wave/keymaps/feature/config.h b/keyboards/etiennecollin/wave/keymaps/feature/config.h
new file mode 100644
index 000000000000..42421b33d396
--- /dev/null
+++ b/keyboards/etiennecollin/wave/keymaps/feature/config.h
@@ -0,0 +1,42 @@
+/* Copyright 2023 Etienne Collin (@etiennecollin)
+ *
+ * This program is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+ * GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ */
+
+#pragma once
+
+// Activate caps word by pressing Left Shift + Right Shift
+#define BOTH_SHIFTS_TURNS_ON_CAPS_WORD
+
+// Maximum time between taps of tap dances
+#define TAPPING_TERM 175
+
+// Max time between taps to prevent hold function and hold auto-repeat
+#define QUICK_TAP_TERM 100
+
+// Perform hold action if pressing a dual-role key, tapping another key and
+// releasing the dual-role key withing tapping term
+#define PERMISSIVE_HOLD
+
+// Mouse key speed and acceleration.
+#define MOUSEKEY_DELAY 0
+#define MOUSEKEY_INTERVAL 16
+#define MOUSEKEY_WHEEL_DELAY 0
+#define MOUSEKEY_MAX_SPEED 6
+#define MOUSEKEY_TIME_TO_MAX 64
+
+// Thumb Combos
+#define COMBO_COUNT 2
+#define COMBO_TERM 200
+#define EXTRA_SHORT_COMBOS
diff --git a/keyboards/etiennecollin/wave/keymaps/feature/keymap.c b/keyboards/etiennecollin/wave/keymaps/feature/keymap.c
new file mode 100644
index 000000000000..24bc85d5b499
--- /dev/null
+++ b/keyboards/etiennecollin/wave/keymaps/feature/keymap.c
@@ -0,0 +1,191 @@
+/* Copyright 2023 Etienne Collin (@etiennecollin)
+ *
+ * This program is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 3 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+ * GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ */
+
+#include QMK_KEYBOARD_H
+
+enum custom_layers {
+ COL,
+ QWE,
+ GAM,
+ MED,
+ NAV,
+ MOS,
+ SYM,
+ NUM,
+ FUN,
+ SYS,
+};
+
+const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
+ [COL] = LAYOUT_split_3x5_3(
+ // ----------------------------------------- -----------------------------------------
+ // | q | w | f | p | b | | j | l | u | y | ' |
+ // |-------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | a | r | s | t | g | | m | n | e | i | o |
+ // |-------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | z | x | c | d | v | | k | h | , | . | / |
+ // --------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | esc | spc | tab | | ent | bsp | del |
+ // --------+-------+-------- --------+-------+--------
+ KC_Q, KC_W, KC_F, KC_P, KC_B, KC_J, KC_L, KC_U, KC_Y, KC_QUOT,
+ LGUI_T(KC_A), LALT_T(KC_R), LCTL_T(KC_S), LSFT_T(KC_T), KC_G, KC_M, LSFT_T(KC_N), LCTL_T(KC_E), LALT_T(KC_I), LGUI_T(KC_O),
+ KC_Z, KC_X, KC_C, KC_D, KC_V, KC_K, KC_H, KC_COMM, KC_DOT, KC_SLSH,
+ LT(MED, KC_ESC), LT(NAV, KC_SPC), LT(MOS, KC_TAB), LT(SYM, KC_ENT), LT(NUM, KC_BSPC), LT(FUN, KC_DEL)
+ ),
+ [QWE] = LAYOUT_split_3x5_3(
+ // ----------------------------------------- -----------------------------------------
+ // | q | w | e | r | t | | y | u | i | o | p |
+ // |-------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | a | s | d | f | g | | h | j | k | l | ' |
+ // |-------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | z | x | c | v | b | | n | m | , | . | / |
+ // --------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | esc | spc | tab | | ent | bsp | del |
+ // --------+-------+-------- --------+-------+--------
+ KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P,
+ LGUI_T(KC_A), LALT_T(KC_S), LCTL_T(KC_D), LSFT_T(KC_F), KC_G, KC_H, LSFT_T(KC_J), LCTL_T(KC_K), LALT_T(KC_L), LGUI_T(KC_QUOT),
+ KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH,
+ LT(MED, KC_ESC), LT(NAV, KC_SPC), LT(MOS, KC_TAB), LT(SYM, KC_ENT), LT(NUM, KC_BSPC), LT(FUN, KC_DEL)
+ ),
+ [GAM] = LAYOUT_split_3x5_3(
+ // ----------------------------------------- -----------------------------------------
+ // | tab | q | w | e | r | | t | y | u | i | o |
+ // |-------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | sht | a | s | d | f | | g | h | j | k | l |
+ // |-------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | ctl | z | x | c | v | | b | n | m | , | . |
+ // --------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | alt | cps | spc | | ent | bsp | esc |
+ // --------+-------+-------- --------+-------+--------
+ KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O,
+ KC_LSFT, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L,
+ KC_LCTL, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT,
+ KC_LALT, KC_CAPS, KC_SPC, LT(SYM, KC_ENT), LT(NUM, KC_BSPC), LT(FUN, KC_ESC)
+ ),
+ [MED] = LAYOUT_split_3x5_3(
+ // ----------------------------------------- -----------------------------------------
+ // | | | | | | | | | | | |
+ // |-------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | gui | alt | ctl | sht | | | | prev | vol - | vol + | next |
+ // |-------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | | | | | | | | | | | |
+ // --------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | ___ | | | | stop | pause | mute |
+ // --------+-------+-------- --------+-------+--------
+ XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
+ KC_LGUI, KC_LALT, KC_LCTL, KC_LSFT, XXXXXXX, XXXXXXX, KC_MPRV, KC_VOLD, KC_VOLU, KC_MNXT,
+ XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, KC_MRWD, XXXXXXX, XXXXXXX, KC_MFFD,
+ _______, XXXXXXX, XXXXXXX, KC_MSTP, KC_MPLY, KC_MUTE
+ ),
+ [NAV] = LAYOUT_split_3x5_3(
+ // ----------------------------------------- -----------------------------------------
+ // | | | | | | | | | | | |
+ // |-------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | gui | alt | ctl | sht | | | cps | ← | ↓ | ↑ | → |
+ // |-------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | | | | | | | insrt | home | pageu | paged | end |
+ // --------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | | ___ | | | ent | bsp | del |
+ // --------+-------+-------- --------+-------+--------
+ XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
+ KC_LGUI, KC_LALT, KC_LCTL, KC_LSFT, XXXXXXX, KC_CAPS, KC_LEFT, KC_DOWN, KC_UP, KC_RGHT,
+ XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, KC_INS, KC_HOME, KC_PGDN, KC_PGUP, KC_END,
+ XXXXXXX, _______, XXXXXXX, KC_ENT, KC_BSPC, KC_DEL
+ ),
+ [MOS] = LAYOUT_split_3x5_3(
+ // ----------------------------------------- -----------------------------------------
+ // | | | | | | | | acc0 | acc1 | acc2 | |
+ // |-------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | gui | alt | ctl | sht | | | | ← | ↓ | ↑ | → |
+ // |-------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | | | | | | | | w← | w↓ | w↑ | w→ |
+ // --------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | | | ___ | | left | right | mid |
+ // --------+-------+-------- --------+-------+--------
+ XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, KC_ACL0, KC_ACL1, KC_ACL2, XXXXXXX,
+ KC_LGUI, KC_LALT, KC_LCTL, KC_LSFT, XXXXXXX, XXXXXXX, KC_MS_L, KC_MS_D, KC_MS_U, KC_MS_R,
+ XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, KC_WH_L, KC_WH_D, KC_WH_U, KC_WH_R,
+ XXXXXXX, XXXXXXX, _______, KC_BTN1, KC_BTN2, KC_BTN3
+ ),
+ [SYM] = LAYOUT_split_3x5_3(
+ // ----------------------------------------- -----------------------------------------
+ // | { | & | * | ( | } | | | | | | |
+ // |-------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | : | $ | % | ^ | + | | | sht | ctl | alt | gui |
+ // |-------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | ~ | ! | @ | # | | | | | | | | |
+ // --------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | ( | ) | _ | | ___ | | |
+ // --------+-------+-------- --------+-------+--------
+ KC_LCBR, KC_AMPR, KC_ASTR, KC_LPRN, KC_RCBR, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
+ KC_COLN, KC_DLR, KC_PERC, KC_CIRC, KC_PLUS, XXXXXXX, KC_LSFT, KC_LCTL, KC_LALT, KC_LGUI,
+ KC_TILD, KC_EXLM, KC_AT, KC_HASH, KC_PIPE, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
+ KC_LPRN, KC_RPRN, KC_UNDS, _______, XXXXXXX, XXXXXXX
+ ),
+ [NUM] = LAYOUT_split_3x5_3(
+ // ----------------------------------------- -----------------------------------------
+ // | [ { | 7 & | 8 * | 9 ( | ] } | | | | | | |
+ // |-------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | ; : | 4 $ | 5 % | 6 ^ | = + | | | sht | ctl | alt | gui |
+ // |-------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | ` ~ | 1 ! | 2 @ | 3 # | \ | | | | | | | |
+ // |-------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | . > | 0 ) | - _ | | | ___ | |
+ // --------+-------+-------- --------+-------+--------
+ KC_LBRC, KC_7, KC_8, KC_9, KC_RBRC, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
+ KC_SCLN, KC_4, KC_5, KC_6, KC_EQL, XXXXXXX, KC_LSFT, KC_LCTL, KC_LALT, KC_LGUI,
+ KC_GRV, KC_1, KC_2, KC_3, KC_BSLS, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
+ KC_DOT, KC_0, KC_MINS, XXXXXXX, _______, XXXXXXX
+ ),
+ [FUN] = LAYOUT_split_3x5_3(
+ // ----------------------------------------- -----------------------------------------
+ // | F12 | F 7 | F 8 | F 9 | PrScr | | | | | | |
+ // |-------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | F11 | F 4 | F 5 | F 6 | pause | | | sht | ctl | alt | gui |
+ // |-------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | F10 | F 1 | F 2 | F 3 | scrlk | | | | | | |
+ // --------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | app | spc | tab | | | | ___ |
+ // --------+-------+-------- --------+-------+--------
+ KC_F12, KC_F7, KC_F8, KC_F9, KC_PSCR, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
+ KC_F11, KC_F4, KC_F5, KC_F6, KC_PAUS, XXXXXXX, KC_LSFT, KC_LCTL, KC_LALT, KC_LGUI,
+ KC_F10, KC_F1, KC_F2, KC_F3, KC_SCRL, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
+ KC_APP, KC_SPC, KC_TAB, XXXXXXX, XXXXXXX, _______
+ ),
+ [SYS] = LAYOUT_split_3x5_3(
+ // ----------------------------------------- -----------------------------------------
+ // | BOOT | | GAME | QWERT | COLMK | | COLMK | QWERT | GAME | | BOOT |
+ // |-------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | | | | | | | | | | | |
+ // |-------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | | | | | | | | | | | |
+ // --------+-------+-------+-------+-------| |-------+-------+-------+-------+-------|
+ // | | | | | | | |
+ // --------+-------+-------- --------+-------+--------
+ QK_BOOT, XXXXXXX, DF(GAM), DF(QWE), DF(COL), DF(COL), DF(QWE), DF(GAM), XXXXXXX, QK_BOOT,
+ XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
+ XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
+ _______, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, _______
+ )
+};
+
+const uint16_t PROGMEM combo_sys[] = {LT(MED, KC_ESC), LT(FUN, KC_DEL), COMBO_END};
+const uint16_t PROGMEM combo_sys_gam[] = {KC_LALT, LT(FUN, KC_ESC), COMBO_END};
+
+combo_t key_combos[] = {
+ COMBO(combo_sys, MO(SYS)),
+ COMBO(combo_sys_gam, MO(SYS))
+};
diff --git a/keyboards/etiennecollin/wave/keymaps/feature/readme.md b/keyboards/etiennecollin/wave/keymaps/feature/readme.md
new file mode 100644
index 000000000000..d9971b352b14
--- /dev/null
+++ b/keyboards/etiennecollin/wave/keymaps/feature/readme.md
@@ -0,0 +1,11 @@
+# Feature keymap
+
+This keymap is heavily inspired by the [miryoku](https://github.com/manna-harbour/miryoku) layout.
+
+It defaults to a [COLEMAK mod dh](https://colemakmods.github.io/mod-dh/) layer, but a QWERTY and a gaming layer are available from the `sys` layer.
+
+It uses the following features:
+
+- Home row modifiers
+- Usual special layers (`media`, `navigation`, `mouse`, `symbols`, `numbers`, `functions`, `system`)
+- Combos (to access the `sys` layer by pressing both external thumb keys)
diff --git a/keyboards/etiennecollin/wave/keymaps/feature/rules.mk b/keyboards/etiennecollin/wave/keymaps/feature/rules.mk
new file mode 100644
index 000000000000..96093b82013e
--- /dev/null
+++ b/keyboards/etiennecollin/wave/keymaps/feature/rules.mk
@@ -0,0 +1,2 @@
+CAPS_WORD_ENABLE = yes
+COMBO_ENABLE = yes
diff --git a/keyboards/eyeohdesigns/humble40/config.h b/keyboards/eyeohdesigns/humble40/config.h
index 2458474199aa..39ceae137a18 100644
--- a/keyboards/eyeohdesigns/humble40/config.h
+++ b/keyboards/eyeohdesigns/humble40/config.h
@@ -5,7 +5,6 @@ This program is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 2 of the License, or
(at your option) any later version.
-
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
diff --git a/keyboards/gmmk/pro/config.h b/keyboards/gmmk/pro/config.h
index 995dd95ec811..b8d4788fc3eb 100644
--- a/keyboards/gmmk/pro/config.h
+++ b/keyboards/gmmk/pro/config.h
@@ -33,6 +33,8 @@
#define RGB_DISABLE_WHEN_USB_SUSPENDED
+#define RGB_DISABLE_WHEN_USB_SUSPENDED
+
// RGB Matrix Animation modes. Explicitly enabled
// For full list of effects, see:
// https://docs.qmk.fm/#/feature_rgb_matrix?id=rgb-matrix-effects
diff --git a/keyboards/handwired/dmote/info.json b/keyboards/handwired/dmote/info.json
index fc52fee11e91..317720d3a135 100644
--- a/keyboards/handwired/dmote/info.json
+++ b/keyboards/handwired/dmote/info.json
@@ -26,6 +26,14 @@
"knight": true
}
},
+ "rgblight": {
+ "led_count": 6,
+ "split_count": [3, 3],
+ "animations": {
+ "knight": true,
+ "christmas": true
+ }
+ },
"ws2812": {
"pin": "D1"
},
diff --git a/keyboards/handwired/scottokeebs/scottomouse/keymaps/scotto/config.h b/keyboards/handwired/scottokeebs/scottomouse/keymaps/scotto/config.h
new file mode 100644
index 000000000000..4b0d2cd2fb00
--- /dev/null
+++ b/keyboards/handwired/scottokeebs/scottomouse/keymaps/scotto/config.h
@@ -0,0 +1,26 @@
+/*
+Copyright 2023 Joe Scotto
+
+This program is free software: you can redistribute it and/or modify
+it under the terms of the GNU General Public License as published by
+the Free Software Foundation, either version 2 of the License, or
+(at your option) any later version.
+
+This program is distributed in the hope that it will be useful,
+but WITHOUT ANY WARRANTY; without even the implied warranty of
+MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+GNU General Public License for more details.
+
+You should have received a copy of the GNU General Public License
+along with this program. If not, see .
+*/
+
+#pragma once
+
+// Mouse key speed and acceleration.
+#define MOUSEKEY_DELAY 0
+#define MOUSEKEY_INTERVAL 16
+#define MOUSEKEY_WHEEL_DELAY 0
+#define MOUSEKEY_MAX_SPEED 6
+#define MOUSEKEY_TIME_TO_MAX 64
+
diff --git a/keyboards/handwired/scottokeebs/scottomouse/keymaps/scotto/rules.mk b/keyboards/handwired/scottokeebs/scottomouse/keymaps/scotto/rules.mk
new file mode 100644
index 000000000000..ab1e438182a3
--- /dev/null
+++ b/keyboards/handwired/scottokeebs/scottomouse/keymaps/scotto/rules.mk
@@ -0,0 +1 @@
+COMBO_ENABLE = yes
diff --git a/keyboards/handwired/scottokeebs/scottoslant/keymaps/scotto/keymap.c b/keyboards/handwired/scottokeebs/scottoslant/keymaps/scotto/keymap.c
new file mode 100644
index 000000000000..dc2d08d91053
--- /dev/null
+++ b/keyboards/handwired/scottokeebs/scottoslant/keymaps/scotto/keymap.c
@@ -0,0 +1,69 @@
+/*
+Copyright 2022 Joe Scotto
+
+This program is free software: you can redistribute it and/or modify
+it under the terms of the GNU General Public License as published by
+the Free Software Foundation, either version 2 of the License, or
+(at your option) any later version.
+
+This program is distributed in the hope that it will be useful,
+but WITHOUT ANY WARRANTY; without even the implied warranty of
+MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+GNU General Public License for more details.
+
+You should have received a copy of the GNU General Public License
+along with this program. If not, see .
+*/
+
+#include QMK_KEYBOARD_H
+
+const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
+ [0] = LAYOUT(
+ KC_Q, KC_W, KC_F, KC_P, KC_G, KC_J, KC_L, KC_U, KC_Y, KC_BSPC,
+ KC_A, KC_R, KC_S, KC_T, KC_D, KC_H, KC_N, KC_E, KC_I, KC_O,
+ LSFT_T(KC_Z), KC_X, KC_C, KC_V, KC_B, KC_K, KC_M, KC_COMMA, KC_DOT, RSFT_T(KC_SLSH),
+ KC_ESC, KC_LCTL, KC_LALT, LGUI_T(KC_SPC), LT(1, KC_TAB), LT(2, KC_ENT), KC_ESC
+ ),
+ [1] = LAYOUT(
+ KC_UNDS, KC_MINS, KC_PLUS, KC_EQL, KC_COLN, KC_GRV, KC_MRWD, KC_MPLY, KC_MFFD, KC_DEL,
+ KC_LCBR, KC_LPRN, KC_RPRN, KC_RCBR, KC_PIPE, KC_ESC, KC_LEFT, KC_UP, KC_DOWN, KC_RGHT,
+ LSFT_T(KC_LBRC), KC_QUOT, KC_DQUO, KC_RBRC, KC_SCLN, KC_TILDE, KC_VOLD, KC_MUTE, KC_VOLU, RSFT_T(KC_BSLS),
+ KC_ESC, KC_LCTL, KC_LALT, LGUI_T(KC_SPC), KC_TRNS, KC_TRNS, KC_ESC
+ ),
+ [2] = LAYOUT(
+ KC_EXLM, KC_AT, KC_HASH, KC_DLR, KC_PERC, KC_CIRC, KC_AMPR, KC_ASTR, KC_CAPS, KC_BSPC,
+ KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0,
+ KC_LSFT, KC_NO, KC_NO, KC_NO, MO(3), KC_NO, KC_NO, KC_COMM, KC_DOT, RSFT_T(KC_SLSH),
+ KC_ESC, KC_LCTL, KC_LALT, LGUI_T(KC_SPC), KC_TRNS, KC_TRNS, KC_ESC
+ ),
+ [3] = LAYOUT(
+ KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, TO(4),
+ KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10,
+ KC_F11, KC_NO, KC_NO, QK_BOOT, KC_TRNS, KC_NO, KC_NO, KC_NO, KC_NO, KC_F12,
+ KC_ESC, KC_LCTL, KC_LALT, LGUI_T(KC_SPC), KC_TRNS, KC_TRNS, KC_ESC
+ ),
+ [4] = LAYOUT(
+ KC_Q, KC_W, KC_F, KC_P, KC_G, KC_J, KC_L, KC_U, KC_Y, KC_BSPC,
+ KC_A, KC_R, KC_S, KC_T, KC_D, KC_H, KC_N, KC_E, KC_I, KC_O,
+ LSFT_T(KC_Z), KC_X, KC_C, KC_V, KC_B, KC_K, KC_M, KC_COMMA, KC_DOT, RSFT_T(KC_SLSH),
+ KC_ESC, KC_LALT, KC_LCTL, KC_SPC, LT(5, KC_TAB), LT(6, KC_ENT), KC_ESC
+ ),
+ [5] = LAYOUT(
+ KC_UNDS, KC_MINS, KC_PLUS, KC_EQL, KC_COLN, KC_GRV, KC_MRWD, KC_MPLY, KC_MFFD, KC_DEL,
+ KC_LCBR, KC_LPRN, KC_RPRN, KC_RCBR, KC_PIPE, KC_ESC, KC_LEFT, KC_UP, KC_DOWN, KC_RGHT,
+ LSFT_T(KC_LBRC), KC_QUOT, KC_DQUO, KC_RBRC, KC_SCLN, KC_TILDE, KC_VOLD, KC_MUTE, KC_VOLU, RSFT_T(KC_BSLS),
+ KC_ESC, KC_LALT, KC_LCTL, KC_SPC, LT(5, KC_TAB), LT(6, KC_ENT), KC_ESC
+ ),
+ [6] = LAYOUT(
+ KC_EXLM, KC_AT, KC_HASH, KC_DLR, KC_PERC, KC_CIRC, KC_AMPR, KC_ASTR, KC_CAPS, KC_BSPC,
+ KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0,
+ KC_LSFT, KC_NO, KC_NO, KC_NO, MO(7), KC_NO, KC_NO, KC_COMM, KC_DOT, RSFT_T(KC_SLSH),
+ KC_ESC, KC_LALT, KC_LCTL, KC_SPC, LT(5, KC_TAB), LT(6, KC_ENT), KC_ESC
+ ),
+ [7] = LAYOUT(
+ KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, KC_NO, TO(0),
+ KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10,
+ KC_F11, KC_NO, KC_NO, QK_BOOT, KC_TRNS, KC_NO, KC_NO, KC_NO, KC_NO, KC_F12,
+ KC_ESC, KC_LALT, KC_LCTL, KC_SPC, LT(5, KC_TAB), LT(6, KC_ENT), KC_ESC
+ )
+};
diff --git a/keyboards/helix/rev2/info.json b/keyboards/helix/rev2/info.json
index aac3cc9dbb15..60f452562249 100644
--- a/keyboards/helix/rev2/info.json
+++ b/keyboards/helix/rev2/info.json
@@ -37,6 +37,21 @@
"static_gradient": true
}
},
+ "tapping": {
+ "term": 100
+ },
+ "rgblight": {
+ "hue_steps": 10,
+ "animations": {
+ "breathing": true,
+ "rainbow_mood": true,
+ "rainbow_swirl": true,
+ "snake": true,
+ "knight": true,
+ "christmas": true,
+ "static_gradient": true
+ }
+ },
"ws2812": {
"pin": "D3"
},
diff --git a/keyboards/jorne/rev1/info.json b/keyboards/jorne/rev1/info.json
index f76b9c0e7d9f..7433b1c33444 100644
--- a/keyboards/jorne/rev1/info.json
+++ b/keyboards/jorne/rev1/info.json
@@ -26,6 +26,10 @@
"led_count": 56,
"split_count": [28, 28]
},
+ "rgblight": {
+ "led_count": 56,
+ "split_count": [28, 28]
+ },
"ws2812": {
"pin": "D3"
},
diff --git a/keyboards/kbdfans/odin75/lib/bongocat.h b/keyboards/kbdfans/odin75/lib/bongocat.h
index 2ffeca199353..1ba068a486cd 100644
--- a/keyboards/kbdfans/odin75/lib/bongocat.h
+++ b/keyboards/kbdfans/odin75/lib/bongocat.h
@@ -1,4 +1,4 @@
-/* Copyright 2022 HorrorTroll
+/* Copyright 2023 eerraa
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
diff --git a/keyboards/lfkeyboards/smk65/revf/info.json b/keyboards/lfkeyboards/smk65/revf/info.json
index 63e9d0abc752..50c039b76cb2 100644
--- a/keyboards/lfkeyboards/smk65/revf/info.json
+++ b/keyboards/lfkeyboards/smk65/revf/info.json
@@ -4,6 +4,10 @@
},
"processor": "atmega32u4",
"bootloader": "halfkay",
+ "rgblight": {
+ "driver": "custom",
+ "led_count": 28
+ },
"layouts": {
"LAYOUT_65_ansi": {
"layout": [
diff --git a/keyboards/mechwild/mokulua/mirrored/info.json b/keyboards/mechwild/mokulua/mirrored/info.json
index 7289147e6b5f..cdb20188abc1 100644
--- a/keyboards/mechwild/mokulua/mirrored/info.json
+++ b/keyboards/mechwild/mokulua/mirrored/info.json
@@ -43,6 +43,17 @@
"rainbow_swirl": true
}
},
+ "rgblight": {
+ "saturation_steps": 8,
+ "brightness_steps": 8,
+ "led_count": 16,
+ "sleep": true,
+ "split_count": [8, 8],
+ "animations": {
+ "rainbow_mood": true,
+ "rainbow_swirl": true
+ }
+ },
"ws2812": {
"pin": "B6"
},
diff --git a/keyboards/mechwild/mokulua/standard/info.json b/keyboards/mechwild/mokulua/standard/info.json
index da82447980fb..c3397f9f5015 100644
--- a/keyboards/mechwild/mokulua/standard/info.json
+++ b/keyboards/mechwild/mokulua/standard/info.json
@@ -43,6 +43,17 @@
"rainbow_swirl": true
}
},
+ "rgblight": {
+ "saturation_steps": 8,
+ "brightness_steps": 8,
+ "led_count": 16,
+ "sleep": true,
+ "split_count": [8, 8],
+ "animations": {
+ "rainbow_mood": true,
+ "rainbow_swirl": true
+ }
+ },
"ws2812": {
"pin": "B6"
},
diff --git a/keyboards/mlego/m65/rev1/info.json b/keyboards/mlego/m65/rev1/info.json
index d8bb91de3009..30d6075233a9 100644
--- a/keyboards/mlego/m65/rev1/info.json
+++ b/keyboards/mlego/m65/rev1/info.json
@@ -57,6 +57,22 @@
"pid": "0x6061",
"vid": "0xBABA"
},
+ "rgblight": {
+ "saturation_steps": 8,
+ "led_count": 20,
+ "animations": {
+ "breathing": true,
+ "rainbow_mood": true,
+ "rainbow_swirl": true,
+ "snake": true,
+ "knight": true,
+ "christmas": true,
+ "static_gradient": true,
+ "rgb_test": true,
+ "alternating": true,
+ "twinkle": true
+ }
+ },
"ws2812": {
"pin": "B15"
},
diff --git a/keyboards/mlego/m65/rev2/info.json b/keyboards/mlego/m65/rev2/info.json
index e8575e23e85b..b96ca466e318 100644
--- a/keyboards/mlego/m65/rev2/info.json
+++ b/keyboards/mlego/m65/rev2/info.json
@@ -56,6 +56,22 @@
"pid": "0x6061",
"vid": "0xBABA"
},
+ "rgblight": {
+ "saturation_steps": 8,
+ "led_count": 20,
+ "animations": {
+ "breathing": true,
+ "rainbow_mood": true,
+ "rainbow_swirl": true,
+ "snake": true,
+ "knight": true,
+ "christmas": true,
+ "static_gradient": true,
+ "rgb_test": true,
+ "alternating": true,
+ "twinkle": true
+ }
+ },
"ws2812": {
"pin": "B15"
},
diff --git a/keyboards/mlego/m65/rev3/info.json b/keyboards/mlego/m65/rev3/info.json
index d48d52d9d97c..94de105ec1ee 100644
--- a/keyboards/mlego/m65/rev3/info.json
+++ b/keyboards/mlego/m65/rev3/info.json
@@ -57,6 +57,22 @@
"pid": "0x6062",
"vid": "0xBABA"
},
+ "rgblight": {
+ "saturation_steps": 8,
+ "led_count": 20,
+ "animations": {
+ "breathing": true,
+ "rainbow_mood": true,
+ "rainbow_swirl": true,
+ "snake": true,
+ "knight": true,
+ "christmas": true,
+ "static_gradient": true,
+ "rgb_test": true,
+ "alternating": true,
+ "twinkle": true
+ }
+ },
"ws2812": {
"pin": "B15"
},
diff --git a/keyboards/mlego/m65/rev4/info.json b/keyboards/mlego/m65/rev4/info.json
index 56fed15317fe..bc6c83083d4f 100644
--- a/keyboards/mlego/m65/rev4/info.json
+++ b/keyboards/mlego/m65/rev4/info.json
@@ -59,6 +59,22 @@
"pid": "0x6062",
"vid": "0xBABA"
},
+ "rgblight": {
+ "saturation_steps": 8,
+ "led_count": 20,
+ "animations": {
+ "breathing": true,
+ "rainbow_mood": true,
+ "rainbow_swirl": true,
+ "snake": true,
+ "knight": true,
+ "christmas": true,
+ "static_gradient": true,
+ "rgb_test": true,
+ "alternating": true,
+ "twinkle": true
+ }
+ },
"ws2812": {
"pin": "B15"
},
diff --git a/keyboards/mode/m60s/halconf.h b/keyboards/mode/m60s/halconf.h
old mode 100644
new mode 100755
diff --git a/keyboards/rastersoft/minitkl/keymaps/hybrid/keymap.c b/keyboards/rastersoft/minitkl/keymaps/hybrid/keymap.c
new file mode 100644
index 000000000000..a65df705739c
--- /dev/null
+++ b/keyboards/rastersoft/minitkl/keymaps/hybrid/keymap.c
@@ -0,0 +1,37 @@
+/*
+ * Copyright 2023 Raster Software Vigo
+ *
+ * This program is free software: you can redistribute it and/or modify
+ * it under the terms of the GNU General Public License as published by
+ * the Free Software Foundation, either version 2 of the License, or
+ * (at your option) any later version.
+ *
+ * This program is distributed in the hope that it will be useful,
+ * but WITHOUT ANY WARRANTY; without even the implied warranty of
+ * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
+ * GNU General Public License for more details.
+ *
+ * You should have received a copy of the GNU General Public License
+ * along with this program. If not, see .
+ */
+
+#include QMK_KEYBOARD_H
+
+const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
+ [0] = LAYOUT_hybrid(
+ KC_ESC, KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10, KC_F11, KC_F12, KC_PSCR, KC_SCRL, KC_PAUS,
+ KC_GRV, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, KC_MINS, KC_EQL, KC_BSPC, KC_HOME, KC_END,
+ KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_LBRC, KC_RBRC, KC_NUHS, KC_INS, KC_PGUP,
+ KC_CAPS, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT, KC_ENT, KC_DEL, KC_PGDN,
+ KC_LSFT, KC_NUBS, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_RSFT, KC_UP, LT(1, KC_APP),
+ KC_LCTL, KC_LGUI, KC_LALT, KC_SPC, KC_RALT, KC_APP, KC_RGUI, KC_LEFT, KC_DOWN, KC_RGHT
+ ),
+ [1] = LAYOUT_hybrid(
+ XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
+ XXXXXXX, RGB_TOG, RGB_MODE_FORWARD, RGB_MODE_REVERSE, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
+ XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX,
+ XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, KC_MUTE, XXXXXXX,
+ XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, KC_VOLU, XXXXXXX,
+ XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, XXXXXXX, KC_VOLD, KC_VOLD, KC_VOLU
+ ),
+};
diff --git a/keyboards/rastersoft/minitkl/keymaps/hybrid/readme.md b/keyboards/rastersoft/minitkl/keymaps/hybrid/readme.md
new file mode 100644
index 000000000000..121f6277f2c3
--- /dev/null
+++ b/keyboards/rastersoft/minitkl/keymaps/hybrid/readme.md
@@ -0,0 +1,11 @@
+# The HYBRID keymap for MiniTKL
+
+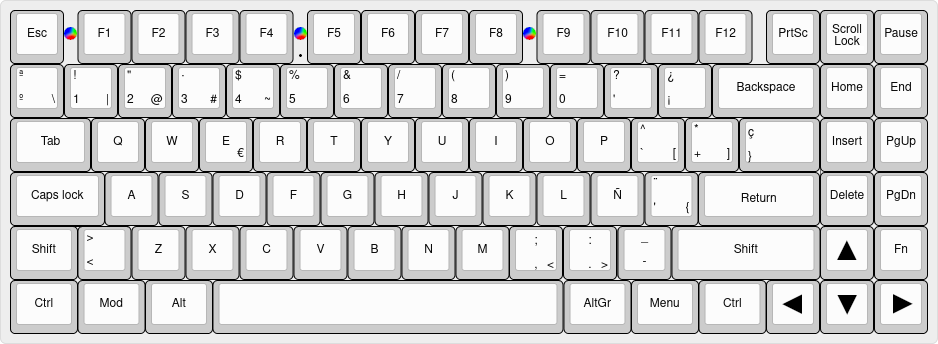
+
+This keymap is designed to be able to have an ISO keyboard with the ANSI Return
+key. This is useful in, at least, two cases:
+
+* some programmers prefer the ANSI key, but want to use European languages.
+* some keycaps come without ISO return key, even for European languages layouts.
+
+The `Fn` key trigger `KC_APP` when tapped, and activate Layer 1 when held.
diff --git a/keyboards/rgbkb/mun/keymaps/peott-fr/rules.mk b/keyboards/rgbkb/mun/keymaps/peott-fr/rules.mk
new file mode 100644
index 000000000000..ee325681483f
--- /dev/null
+++ b/keyboards/rgbkb/mun/keymaps/peott-fr/rules.mk
@@ -0,0 +1 @@
+ENCODER_MAP_ENABLE = yes
diff --git a/keyboards/splitkb/aurora/helix/keymaps/debug/config.h b/keyboards/splitkb/aurora/helix/keymaps/debug/config.h
index a15def3fb913..e6231cabf8b8 100644
--- a/keyboards/splitkb/aurora/helix/keymaps/debug/config.h
+++ b/keyboards/splitkb/aurora/helix/keymaps/debug/config.h
@@ -13,7 +13,6 @@
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
-
#pragma once
#define RGBLIGHT_EFFECT_BREATHING
\ No newline at end of file
diff --git a/keyboards/studiokestra/line_tkl/config.h b/keyboards/studiokestra/line_tkl/config.h
new file mode 100644
index 000000000000..c37d8a5ad28d
--- /dev/null
+++ b/keyboards/studiokestra/line_tkl/config.h
@@ -0,0 +1,9 @@
+// Copyright 2023 studiokestra (@studiokestra)
+// SPDX-License-Identifier: GPL-2.0-or-later
+
+#pragma once
+
+/* Mechanical locking support. Use KC_LCAP, KC_LNUM or KC_LSCR instead in keymap */
+#define LOCKING_SUPPORT_ENABLE
+/* Locking resynchronize hack */
+#define LOCKING_RESYNC_ENABLE
diff --git a/keyboards/studiokestra/line_tkl/info.json b/keyboards/studiokestra/line_tkl/info.json
new file mode 100644
index 000000000000..ba6601a3304d
--- /dev/null
+++ b/keyboards/studiokestra/line_tkl/info.json
@@ -0,0 +1,216 @@
+{
+ "manufacturer": "Studio Kestra",
+ "keyboard_name": "Line TKL",
+ "maintainer": "studiokestra",
+ "bootloader": "atmel-dfu",
+ "diode_direction": "COL2ROW",
+ "features": {
+ "bootmagic": true,
+ "command": false,
+ "console": false,
+ "extrakey": true,
+ "mousekey": true,
+ "nkro": true
+ },
+ "indicators": {
+ "caps_lock": "B6",
+ "on_state": 1,
+ "scroll_lock": "D2"
+ },
+ "matrix_pins": {
+ "cols": ["F0", "F1", "F4", "F5", "F6", "F7", "B5", "D5", "D3"],
+ "rows": ["D0", "D1", "B0", "B7", "B3", "E6", "C7", "C6", "B4", "D7", "D6", "D4"]
+ },
+ "processor": "atmega32u4",
+ "url": "https://minokeys.com/collections/line-friends",
+ "usb": {
+ "device_version": "1.0.0",
+ "pid": "0x8704",
+ "vid": "0x7C10"
+ },
+ "community_layouts": ["tkl_ansi_tsangan", "tkl_ansi_tsangan_split_bs_rshift"],
+ "layouts": {
+ "LAYOUT_tkl_ansi_tsangan": {
+ "layout": [
+ {"matrix": [0, 0], "x": 0, "y": 0},
+ {"matrix": [0, 1], "x": 2, "y": 0},
+ {"matrix": [0, 2], "x": 3, "y": 0},
+ {"matrix": [1, 2], "x": 4, "y": 0},
+ {"matrix": [0, 3], "x": 5, "y": 0},
+ {"matrix": [1, 3], "x": 6.5, "y": 0},
+ {"matrix": [0, 4], "x": 7.5, "y": 0},
+ {"matrix": [1, 4], "x": 8.5, "y": 0},
+ {"matrix": [0, 5], "x": 9.5, "y": 0},
+ {"matrix": [1, 5], "x": 11, "y": 0},
+ {"matrix": [0, 6], "x": 12, "y": 0},
+ {"matrix": [1, 6], "x": 13, "y": 0},
+ {"matrix": [0, 7], "x": 14, "y": 0},
+ {"matrix": [1, 7], "x": 15.25, "y": 0},
+ {"matrix": [0, 8], "x": 16.25, "y": 0},
+ {"matrix": [1, 8], "x": 17.25, "y": 0},
+ {"matrix": [2, 0], "x": 0, "y": 1.25},
+ {"matrix": [3, 0], "x": 1, "y": 1.25},
+ {"matrix": [2, 1], "x": 2, "y": 1.25},
+ {"matrix": [3, 1], "x": 3, "y": 1.25},
+ {"matrix": [2, 2], "x": 4, "y": 1.25},
+ {"matrix": [3, 2], "x": 5, "y": 1.25},
+ {"matrix": [2, 3], "x": 6, "y": 1.25},
+ {"matrix": [3, 3], "x": 7, "y": 1.25},
+ {"matrix": [2, 4], "x": 8, "y": 1.25},
+ {"matrix": [3, 4], "x": 9, "y": 1.25},
+ {"matrix": [2, 5], "x": 10, "y": 1.25},
+ {"matrix": [3, 5], "x": 11, "y": 1.25},
+ {"matrix": [2, 6], "x": 12, "y": 1.25},
+ {"matrix": [3, 6], "x": 13, "y": 1.25, "w": 2},
+ {"matrix": [3, 7], "x": 15.25, "y": 1.25},
+ {"matrix": [2, 8], "x": 16.25, "y": 1.25},
+ {"matrix": [3, 8], "x": 17.25, "y": 1.25},
+ {"matrix": [4, 0], "x": 0, "y": 2.25, "w": 1.5},
+ {"matrix": [5, 0], "x": 1.5, "y": 2.25},
+ {"matrix": [4, 1], "x": 2.5, "y": 2.25},
+ {"matrix": [5, 1], "x": 3.5, "y": 2.25},
+ {"matrix": [4, 2], "x": 4.5, "y": 2.25},
+ {"matrix": [5, 2], "x": 5.5, "y": 2.25},
+ {"matrix": [4, 3], "x": 6.5, "y": 2.25},
+ {"matrix": [5, 3], "x": 7.5, "y": 2.25},
+ {"matrix": [4, 4], "x": 8.5, "y": 2.25},
+ {"matrix": [5, 4], "x": 9.5, "y": 2.25},
+ {"matrix": [4, 5], "x": 10.5, "y": 2.25},
+ {"matrix": [5, 5], "x": 11.5, "y": 2.25},
+ {"matrix": [4, 6], "x": 12.5, "y": 2.25},
+ {"matrix": [4, 7], "x": 13.5, "y": 2.25, "w": 1.5},
+ {"matrix": [5, 7], "x": 15.25, "y": 2.25},
+ {"matrix": [4, 8], "x": 16.25, "y": 2.25},
+ {"matrix": [5, 8], "x": 17.25, "y": 2.25},
+ {"matrix": [6, 0], "x": 0, "y": 3.25, "w": 1.75},
+ {"matrix": [7, 0], "x": 1.75, "y": 3.25},
+ {"matrix": [6, 1], "x": 2.75, "y": 3.25},
+ {"matrix": [7, 1], "x": 3.75, "y": 3.25},
+ {"matrix": [6, 2], "x": 4.75, "y": 3.25},
+ {"matrix": [7, 2], "x": 5.75, "y": 3.25},
+ {"matrix": [6, 3], "x": 6.75, "y": 3.25},
+ {"matrix": [7, 3], "x": 7.75, "y": 3.25},
+ {"matrix": [6, 4], "x": 8.75, "y": 3.25},
+ {"matrix": [7, 4], "x": 9.75, "y": 3.25},
+ {"matrix": [6, 5], "x": 10.75, "y": 3.25},
+ {"matrix": [7, 5], "x": 11.75, "y": 3.25},
+ {"matrix": [6, 6], "x": 12.75, "y": 3.25, "w": 2.25},
+ {"matrix": [8, 0], "x": 0, "y": 4.25, "w": 2.25},
+ {"matrix": [8, 1], "x": 2.25, "y": 4.25},
+ {"matrix": [9, 1], "x": 3.25, "y": 4.25},
+ {"matrix": [8, 2], "x": 4.25, "y": 4.25},
+ {"matrix": [9, 2], "x": 5.25, "y": 4.25},
+ {"matrix": [8, 3], "x": 6.25, "y": 4.25},
+ {"matrix": [9, 3], "x": 7.25, "y": 4.25},
+ {"matrix": [8, 4], "x": 8.25, "y": 4.25},
+ {"matrix": [9, 4], "x": 9.25, "y": 4.25},
+ {"matrix": [8, 5], "x": 10.25, "y": 4.25},
+ {"matrix": [9, 5], "x": 11.25, "y": 4.25},
+ {"matrix": [8, 6], "x": 12.25, "y": 4.25, "w": 2.75},
+ {"matrix": [9, 8], "x": 16.25, "y": 4.25},
+ {"matrix": [10, 0], "x": 0, "y": 5.25, "w": 1.5},
+ {"matrix": [11, 0], "x": 1.5, "y": 5.25},
+ {"matrix": [10, 1], "x": 2.5, "y": 5.25, "w": 1.5},
+ {"matrix": [10, 3], "x": 4, "y": 5.25, "w": 7},
+ {"matrix": [11, 5], "x": 11, "y": 5.25, "w": 1.5},
+ {"matrix": [10, 6], "x": 12.5, "y": 5.25},
+ {"matrix": [10, 7], "x": 13.5, "y": 5.25, "w": 1.5},
+ {"matrix": [11, 7], "x": 15.25, "y": 5.25},
+ {"matrix": [10, 8], "x": 16.25, "y": 5.25},
+ {"matrix": [11, 8], "x": 17.25, "y": 5.25}
+ ]
+ },
+ "LAYOUT_tkl_ansi_tsangan_split_bs_rshift": {
+ "layout": [
+ {"matrix": [0, 0], "x": 0, "y": 0},
+ {"matrix": [0, 1], "x": 2, "y": 0},
+ {"matrix": [0, 2], "x": 3, "y": 0},
+ {"matrix": [1, 2], "x": 4, "y": 0},
+ {"matrix": [0, 3], "x": 5, "y": 0},
+ {"matrix": [1, 3], "x": 6.5, "y": 0},
+ {"matrix": [0, 4], "x": 7.5, "y": 0},
+ {"matrix": [1, 4], "x": 8.5, "y": 0},
+ {"matrix": [0, 5], "x": 9.5, "y": 0},
+ {"matrix": [1, 5], "x": 11, "y": 0},
+ {"matrix": [0, 6], "x": 12, "y": 0},
+ {"matrix": [1, 6], "x": 13, "y": 0},
+ {"matrix": [0, 7], "x": 14, "y": 0},
+ {"matrix": [1, 7], "x": 15.25, "y": 0},
+ {"matrix": [0, 8], "x": 16.25, "y": 0},
+ {"matrix": [1, 8], "x": 17.25, "y": 0},
+ {"matrix": [2, 0], "x": 0, "y": 1.25},
+ {"matrix": [3, 0], "x": 1, "y": 1.25},
+ {"matrix": [2, 1], "x": 2, "y": 1.25},
+ {"matrix": [3, 1], "x": 3, "y": 1.25},
+ {"matrix": [2, 2], "x": 4, "y": 1.25},
+ {"matrix": [3, 2], "x": 5, "y": 1.25},
+ {"matrix": [2, 3], "x": 6, "y": 1.25},
+ {"matrix": [3, 3], "x": 7, "y": 1.25},
+ {"matrix": [2, 4], "x": 8, "y": 1.25},
+ {"matrix": [3, 4], "x": 9, "y": 1.25},
+ {"matrix": [2, 5], "x": 10, "y": 1.25},
+ {"matrix": [3, 5], "x": 11, "y": 1.25},
+ {"matrix": [2, 6], "x": 12, "y": 1.25},
+ {"matrix": [3, 6], "x": 13, "y": 1.25},
+ {"matrix": [2, 7], "x": 14, "y": 1.25},
+ {"matrix": [3, 7], "x": 15.25, "y": 1.25},
+ {"matrix": [2, 8], "x": 16.25, "y": 1.25},
+ {"matrix": [3, 8], "x": 17.25, "y": 1.25},
+ {"matrix": [4, 0], "x": 0, "y": 2.25, "w": 1.5},
+ {"matrix": [5, 0], "x": 1.5, "y": 2.25},
+ {"matrix": [4, 1], "x": 2.5, "y": 2.25},
+ {"matrix": [5, 1], "x": 3.5, "y": 2.25},
+ {"matrix": [4, 2], "x": 4.5, "y": 2.25},
+ {"matrix": [5, 2], "x": 5.5, "y": 2.25},
+ {"matrix": [4, 3], "x": 6.5, "y": 2.25},
+ {"matrix": [5, 3], "x": 7.5, "y": 2.25},
+ {"matrix": [4, 4], "x": 8.5, "y": 2.25},
+ {"matrix": [5, 4], "x": 9.5, "y": 2.25},
+ {"matrix": [4, 5], "x": 10.5, "y": 2.25},
+ {"matrix": [5, 5], "x": 11.5, "y": 2.25},
+ {"matrix": [4, 6], "x": 12.5, "y": 2.25},
+ {"matrix": [4, 7], "x": 13.5, "y": 2.25, "w": 1.5},
+ {"matrix": [5, 7], "x": 15.25, "y": 2.25},
+ {"matrix": [4, 8], "x": 16.25, "y": 2.25},
+ {"matrix": [5, 8], "x": 17.25, "y": 2.25},
+ {"matrix": [6, 0], "x": 0, "y": 3.25, "w": 1.75},
+ {"matrix": [7, 0], "x": 1.75, "y": 3.25},
+ {"matrix": [6, 1], "x": 2.75, "y": 3.25},
+ {"matrix": [7, 1], "x": 3.75, "y": 3.25},
+ {"matrix": [6, 2], "x": 4.75, "y": 3.25},
+ {"matrix": [7, 2], "x": 5.75, "y": 3.25},
+ {"matrix": [6, 3], "x": 6.75, "y": 3.25},
+ {"matrix": [7, 3], "x": 7.75, "y": 3.25},
+ {"matrix": [6, 4], "x": 8.75, "y": 3.25},
+ {"matrix": [7, 4], "x": 9.75, "y": 3.25},
+ {"matrix": [6, 5], "x": 10.75, "y": 3.25},
+ {"matrix": [7, 5], "x": 11.75, "y": 3.25},
+ {"matrix": [6, 6], "x": 12.75, "y": 3.25, "w": 2.25},
+ {"matrix": [8, 0], "x": 0, "y": 4.25, "w": 2.25},
+ {"matrix": [8, 1], "x": 2.25, "y": 4.25},
+ {"matrix": [9, 1], "x": 3.25, "y": 4.25},
+ {"matrix": [8, 2], "x": 4.25, "y": 4.25},
+ {"matrix": [9, 2], "x": 5.25, "y": 4.25},
+ {"matrix": [8, 3], "x": 6.25, "y": 4.25},
+ {"matrix": [9, 3], "x": 7.25, "y": 4.25},
+ {"matrix": [8, 4], "x": 8.25, "y": 4.25},
+ {"matrix": [9, 4], "x": 9.25, "y": 4.25},
+ {"matrix": [8, 5], "x": 10.25, "y": 4.25},
+ {"matrix": [9, 5], "x": 11.25, "y": 4.25},
+ {"matrix": [8, 6], "x": 12.25, "y": 4.25, "w": 1.75},
+ {"matrix": [9, 6], "x": 14, "y": 4.25},
+ {"matrix": [9, 8], "x": 16.25, "y": 4.25},
+ {"matrix": [10, 0], "x": 0, "y": 5.25, "w": 1.5},
+ {"matrix": [11, 0], "x": 1.5, "y": 5.25},
+ {"matrix": [10, 1], "x": 2.5, "y": 5.25, "w": 1.5},
+ {"matrix": [10, 3], "x": 4, "y": 5.25, "w": 7},
+ {"matrix": [11, 5], "x": 11, "y": 5.25, "w": 1.5},
+ {"matrix": [10, 6], "x": 12.5, "y": 5.25},
+ {"matrix": [10, 7], "x": 13.5, "y": 5.25, "w": 1.5},
+ {"matrix": [11, 7], "x": 15.25, "y": 5.25},
+ {"matrix": [10, 8], "x": 16.25, "y": 5.25},
+ {"matrix": [11, 8], "x": 17.25, "y": 5.25}
+ ]
+ }
+ }
+}
diff --git a/keyboards/studiokestra/line_tkl/keymaps/default/keymap.c b/keyboards/studiokestra/line_tkl/keymaps/default/keymap.c
new file mode 100644
index 000000000000..a8dc54ee8706
--- /dev/null
+++ b/keyboards/studiokestra/line_tkl/keymaps/default/keymap.c
@@ -0,0 +1,39 @@
+// Copyright 2023 studiokestra (@studiokestra)
+// SPDX-License-Identifier: GPL-2.0-or-later
+
+#include QMK_KEYBOARD_H
+
+const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
+ /*
+ * ┌───┐ ┌───┬───┬───┬───┐ ┌───┬───┬───┬───┐ ┌───┬───┬───┬───┐ ┌───┬───┬───┐
+ * │Esc│ │F1 │F2 │F3 │F4 │ │F5 │F6 │F7 │F8 │ │F9 │F10│F11│F12│ │PSc│Scr│Pse│
+ * └───┘ └───┴───┴───┴───┘ └───┴───┴───┴───┘ └───┴───┴───┴───┘ └───┴───┴───┘
+ * ┌───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───────┐ ┌───┬───┬───┐
+ * │ ` │ 1 │ 2 │ 3 │ 4 │ 5 │ 6 │ 7 │ 8 │ 9 │ 0 │ - │ = │ Bcksp │ │Ins│Hom│PgU│
+ * ├───┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─────┤ ├───┼───┼───┤
+ * │ Tab │ Q │ W │ E │ R │ T │ Y │ U │ I │ O │ P │ [ │ ] │ \ │ │Del│End│PgD│
+ * ├─────┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴─────┤ └───┴───┴───┘
+ * │ Caps │ A │ S │ D │ F │ G │ H │ J │ K │ L │ ; │ ' │ Enter │
+ * ├──────┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴────────┤ ┌───┐
+ * │ Shift │ Z │ X │ C │ V │ B │ N │ M │ , │ . │ / │ Shift │ │ ↑ │
+ * ├─────┬──┴┬──┴──┬┴───┴───┴───┴───┴───┴───┴──┬┴───┴┬───┬─────┤ ┌───┼───┼───┐
+ * │Ctrl │GUI│Alt │ │ Alt│GUI│ Ctrl│ │ ← │ ↓ │ → │
+ * └─────┴───┴─────┴───────────────────────────┴─────┴───┴─────┘ └───┴───┴───┘
+ */
+
+ [0] = LAYOUT_tkl_ansi_tsangan(
+ KC_ESC, KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10, KC_F11, KC_F12, KC_PSCR, KC_SCRL, KC_PAUS,
+ KC_GRV, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, KC_MINS, KC_EQL, KC_BSPC, KC_INS, KC_HOME, KC_PGUP,
+ KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_LBRC, KC_RBRC, KC_BSLS, KC_DEL, KC_END, KC_PGDN,
+ KC_CAPS, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT, KC_ENT,
+ KC_LSFT, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_RSFT, KC_UP,
+ KC_LCTL, KC_LGUI, KC_LALT, KC_SPC, KC_RALT, KC_RGUI, KC_RCTL, KC_LEFT, KC_DOWN, KC_RIGHT ),
+
+ [1] = LAYOUT_tkl_ansi_tsangan(
+ QK_BOOT, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
+ KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
+ KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
+ KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
+ KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
+ KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS ),
+};
diff --git a/keyboards/studiokestra/line_tkl/keymaps/split_bs_rshift/keymap.c b/keyboards/studiokestra/line_tkl/keymaps/split_bs_rshift/keymap.c
new file mode 100644
index 000000000000..595ed5d03cc5
--- /dev/null
+++ b/keyboards/studiokestra/line_tkl/keymaps/split_bs_rshift/keymap.c
@@ -0,0 +1,39 @@
+// Copyright 2023 studiokestra (@studiokestra)
+// SPDX-License-Identifier: GPL-2.0-or-later
+
+#include QMK_KEYBOARD_H
+
+const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
+ /*
+ * ┌───┐ ┌───┬───┬───┬───┐ ┌───┬───┬───┬───┐ ┌───┬───┬───┬───┐ ┌───┬───┬───┐
+ * │Esc│ │F1 │F2 │F3 │F4 │ │F5 │F6 │F7 │F8 │ │F9 │F10│F11│F12│ │PSc│Scr│Pse│
+ * └───┘ └───┴───┴───┴───┘ └───┴───┴───┴───┘ └───┴───┴───┴───┘ └───┴───┴───┘
+ * ┌───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┐ ┌───┬───┬───┐
+ * │ ` │ 1 │ 2 │ 3 │ 4 │ 5 │ 6 │ 7 │ 8 │ 9 │ 0 │ - │ = │LBk│RBk│ │Ins│Hom│PgU│
+ * ├───┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴───┤ ├───┼───┼───┤
+ * │ Tab │ Q │ W │ E │ R │ T │ Y │ U │ I │ O │ P │ [ │ ] │ \ │ │Del│End│PgD│
+ * ├─────┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴─────┤ └───┴───┴───┘
+ * │ Caps │ A │ S │ D │ F │ G │ H │ J │ K │ L │ ; │ ' │ Enter │
+ * ├──────┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴────┬───┤ ┌───┐
+ * │ Shift │ Z │ X │ C │ V │ B │ N │ M │ , │ . │ / │Shift │Mo1│ │ ↑ │
+ * ├─────┬──┴┬──┴──┬┴───┴───┴───┴───┴───┴───┴──┬┴───┴┬───┬─┴───┤ ┌───┼───┼───┐
+ * │Ctrl │GUI│Alt │ │ Alt│GUI│ Ctrl│ │ ← │ ↓ │ → │
+ * └─────┴───┴─────┴───────────────────────────┴─────┴───┴─────┘ └───┴───┴───┘
+ */
+
+ [0] = LAYOUT_tkl_ansi_tsangan_split_bs_rshift(
+ KC_ESC, KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10, KC_F11, KC_F12, KC_PSCR, KC_SCRL, KC_PAUS,
+ KC_GRV, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, KC_MINS, KC_EQL, KC_BSPC, KC_BSPC, KC_INS, KC_HOME, KC_PGUP,
+ KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_LBRC, KC_RBRC, KC_BSLS, KC_DEL, KC_END, KC_PGDN,
+ KC_CAPS, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT, KC_ENT,
+ KC_LSFT, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_RSFT, MO(1), KC_UP,
+ KC_LCTL, KC_LGUI, KC_LALT, KC_SPC, KC_RALT, KC_RGUI, KC_RCTL, KC_LEFT, KC_DOWN, KC_RIGHT ),
+
+ [1] = LAYOUT_tkl_ansi_tsangan_split_bs_rshift(
+ QK_BOOT, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
+ KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
+ KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
+ KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
+ KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
+ KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS ),
+};
diff --git a/keyboards/studiokestra/line_tkl/keymaps/via/keymap.c b/keyboards/studiokestra/line_tkl/keymaps/via/keymap.c
new file mode 100644
index 000000000000..595ed5d03cc5
--- /dev/null
+++ b/keyboards/studiokestra/line_tkl/keymaps/via/keymap.c
@@ -0,0 +1,39 @@
+// Copyright 2023 studiokestra (@studiokestra)
+// SPDX-License-Identifier: GPL-2.0-or-later
+
+#include QMK_KEYBOARD_H
+
+const uint16_t PROGMEM keymaps[][MATRIX_ROWS][MATRIX_COLS] = {
+ /*
+ * ┌───┐ ┌───┬───┬───┬───┐ ┌───┬───┬───┬───┐ ┌───┬───┬───┬───┐ ┌───┬───┬───┐
+ * │Esc│ │F1 │F2 │F3 │F4 │ │F5 │F6 │F7 │F8 │ │F9 │F10│F11│F12│ │PSc│Scr│Pse│
+ * └───┘ └───┴───┴───┴───┘ └───┴───┴───┴───┘ └───┴───┴───┴───┘ └───┴───┴───┘
+ * ┌───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┐ ┌───┬───┬───┐
+ * │ ` │ 1 │ 2 │ 3 │ 4 │ 5 │ 6 │ 7 │ 8 │ 9 │ 0 │ - │ = │LBk│RBk│ │Ins│Hom│PgU│
+ * ├───┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴───┤ ├───┼───┼───┤
+ * │ Tab │ Q │ W │ E │ R │ T │ Y │ U │ I │ O │ P │ [ │ ] │ \ │ │Del│End│PgD│
+ * ├─────┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴─────┤ └───┴───┴───┘
+ * │ Caps │ A │ S │ D │ F │ G │ H │ J │ K │ L │ ; │ ' │ Enter │
+ * ├──────┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴────┬───┤ ┌───┐
+ * │ Shift │ Z │ X │ C │ V │ B │ N │ M │ , │ . │ / │Shift │Mo1│ │ ↑ │
+ * ├─────┬──┴┬──┴──┬┴───┴───┴───┴───┴───┴───┴──┬┴───┴┬───┬─┴───┤ ┌───┼───┼───┐
+ * │Ctrl │GUI│Alt │ │ Alt│GUI│ Ctrl│ │ ← │ ↓ │ → │
+ * └─────┴───┴─────┴───────────────────────────┴─────┴───┴─────┘ └───┴───┴───┘
+ */
+
+ [0] = LAYOUT_tkl_ansi_tsangan_split_bs_rshift(
+ KC_ESC, KC_F1, KC_F2, KC_F3, KC_F4, KC_F5, KC_F6, KC_F7, KC_F8, KC_F9, KC_F10, KC_F11, KC_F12, KC_PSCR, KC_SCRL, KC_PAUS,
+ KC_GRV, KC_1, KC_2, KC_3, KC_4, KC_5, KC_6, KC_7, KC_8, KC_9, KC_0, KC_MINS, KC_EQL, KC_BSPC, KC_BSPC, KC_INS, KC_HOME, KC_PGUP,
+ KC_TAB, KC_Q, KC_W, KC_E, KC_R, KC_T, KC_Y, KC_U, KC_I, KC_O, KC_P, KC_LBRC, KC_RBRC, KC_BSLS, KC_DEL, KC_END, KC_PGDN,
+ KC_CAPS, KC_A, KC_S, KC_D, KC_F, KC_G, KC_H, KC_J, KC_K, KC_L, KC_SCLN, KC_QUOT, KC_ENT,
+ KC_LSFT, KC_Z, KC_X, KC_C, KC_V, KC_B, KC_N, KC_M, KC_COMM, KC_DOT, KC_SLSH, KC_RSFT, MO(1), KC_UP,
+ KC_LCTL, KC_LGUI, KC_LALT, KC_SPC, KC_RALT, KC_RGUI, KC_RCTL, KC_LEFT, KC_DOWN, KC_RIGHT ),
+
+ [1] = LAYOUT_tkl_ansi_tsangan_split_bs_rshift(
+ QK_BOOT, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
+ KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
+ KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
+ KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
+ KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS,
+ KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS, KC_TRNS ),
+};
diff --git a/keyboards/studiokestra/line_tkl/keymaps/via/rules.mk b/keyboards/studiokestra/line_tkl/keymaps/via/rules.mk
new file mode 100644
index 000000000000..1e5b99807cb7
--- /dev/null
+++ b/keyboards/studiokestra/line_tkl/keymaps/via/rules.mk
@@ -0,0 +1 @@
+VIA_ENABLE = yes
diff --git a/keyboards/studiokestra/line_tkl/readme.md b/keyboards/studiokestra/line_tkl/readme.md
new file mode 100644
index 000000000000..a5d7ae0c4143
--- /dev/null
+++ b/keyboards/studiokestra/line_tkl/readme.md
@@ -0,0 +1,27 @@
+# studiokestra/line_tkl
+
+
+
+PCB developed for Mino Keys in collaboration with Line Friends (C).
+
+* Keyboard Maintainer: [Studio Kestra](https://github.com/studiokestra/)
+* Hardware Supported: Line TKL
+* Hardware Availability: https://minokeys.com/collections/line-friends
+
+Make example for this keyboard (after setting up your build environment):
+
+ make studiokestra/line_tkl:default
+
+Flashing example for this keyboard:
+
+ make studiokestra/line_tkl:default:flash
+
+See the [build environment setup](https://docs.qmk.fm/#/getting_started_build_tools) and the [make instructions](https://docs.qmk.fm/#/getting_started_make_guide) for more information. Brand new to QMK? Start with our [Complete Newbs Guide](https://docs.qmk.fm/#/newbs).
+
+## Bootloader
+
+Enter the bootloader in 3 ways:
+
+* **Bootmagic reset**: Hold down the key at (0,0) in the matrix (usually the top left key or Escape) and plug in the keyboard
+* **Physical reset button**: Briefly press the button on the back of the PCB - some may have pads you must short instead
+* **Keycode in layout**: Press the key mapped to `QK_BOOT` if it is available
diff --git a/keyboards/studiokestra/line_tkl/rules.mk b/keyboards/studiokestra/line_tkl/rules.mk
new file mode 100644
index 000000000000..6e7633bfe015
--- /dev/null
+++ b/keyboards/studiokestra/line_tkl/rules.mk
@@ -0,0 +1 @@
+# This file intentionally left blank
diff --git a/keyboards/woodkeys/meira/post_rules.mk b/keyboards/woodkeys/meira/post_rules.mk
new file mode 100644
index 000000000000..5d85581c6eeb
--- /dev/null
+++ b/keyboards/woodkeys/meira/post_rules.mk
@@ -0,0 +1,9 @@
+SRC += matrix.c TWIlib.c issi.c lighting.c
+
+#ifeq ($(strip $(ISSI_ENABLE)), yes)
+# OPT_DEFS += -DISSI_ENABLE
+#endif
+
+#ifeq ($(strip $(WATCHDOG_ENABLE)), yes)
+# OPT_DEFS += -DWATCHDOG_ENABLE
+#endif
diff --git a/keyboards/ymdk/melody96/matrix_diagram.md b/keyboards/ymdk/melody96/matrix_diagram.md
new file mode 100644
index 000000000000..e589bcc469de
--- /dev/null
+++ b/keyboards/ymdk/melody96/matrix_diagram.md
@@ -0,0 +1,35 @@
+# Matrix Diagram for YMDK Melody96
+
+```
+┌───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┬───┐
+│50 │51 │52 │53 │54 │55 │56 │57 │58 │B8 │B7 │B5 │B4 │B3 │B6 │B2 │B1 │B0 │63 │
+├───┼───┼───┼───┼───┼───┼───┼───┼───┼───┼───┼───┼───┼───┼───┼───┼───┼───┼───┤ ┌───────┐
+│40 │41 │42 │43 │44 │45 │56 │47 │48 │A8 │A7 │A5 │A4 │A3 │A6 │A2 │A1 │A0 │64 │ │A6 │ 2u Backpsace
+├───┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴───┼───┼───┼───┼───┤ └─┬─────┤ ┌───┐
+│30 │31 │32 │33 │34 │35 │36 │37 │38 │98 │97 │95 │94 │84 │96 │92 │91 │90 │ │ │ │ │
+├─────┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴┬──┴─────┼───┼───┼───┼───┤ ┌──┴┐93 │ ISO Enter │80 │ Numpad "+" 2u
+│20 │21 │22 │23 │24 │25 │26 │27 │28 │88 │87 │85 │93 │86 │82 │81 │80 │ │84 │ │ │ │
+├────┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴─┬─┴────┬───┼───┼───┼───┼───┤ └───┴────┘ ├───┤
+│10 │11 │12 │13 │14 │15 │16 │17 │18 │78 │77 │75 │74 │73 │76 │72 │71 │70 │ │ │
+├────┼───┴┬──┴─┬─┴───┴───┴───┴───┴───┴──┬┴──┬┴──┬┴──┬───┼───┼───┼───┼───┼───┤ ┌───────┐ │60 │ Numpad Enter 2U
+│00 │01 │02 │06 │08 │07 │05 │04 │03 │66 │62 │61 │60 │ Numpad 0 2u │62 │ │ │
+└────┴────┴────┴────────────────────────┴───┴───┴───┴───┴───┴───┴───┴───┴───┘ └───────┘ └───┘
+┌────────┐ ┌──────────┐
+│10 │ 2.25u LShift 2.75u RShift │74 │
+└────────┘ └──────────┘
+┌────┬────┬────┬────────────────────────┬────┬────┬────┬────┐
+│00 │01 │02 │06 │08 │07 │04 │03 │ 4x1.25u Right Mod
+└────┴────┴────┴────────────────────────┴────┴────┴────┴────┘
+┌─────┬─────┬───────────────────────────┬───┬───┬───┬───┬───┐
+│00 │01 │06 │08 │07 │05 │04 │03 │ 2x1.5u Left Mod
+└─────┴─────┴───────────────────────────┴───┴───┴───┴───┴───┘
+┌─────┬───┬─────┬───────────────────────┬─────┬─────┬───┬───┐
+│00 │01 │02 │06 │08 │05 │04 │03 │ Tsangan Left Mod, 2x1.5u-2x1u Right Mod
+└─────┴───┴─────┴───────────────────────┴─────┴─────┴───┴───┘
+┌─────┬───┬─────┬───────────────────────────┬─────┬───┬─────┐
+│00 │01 │02 │06 │07 │04 │03 │ Tsangan/WKL/HHKB
+└─────┴───┴─────┴───────────────────────────┴─────┴───┴─────┘
+┌─────┬───┬─────┬───────────────────────────┬───┬───┬───┬───┐
+│00 │01 │02 │06 │07 │05 │04 │03 │ 4x1u Right Mod
+└─────┴───┴─────┴───────────────────────────┴───┴───┴───┴───┘
+```
diff --git a/lib/python/qmk/cli/compile.py b/lib/python/qmk/cli/compile.py
index 4c36dec3e792..7cbbbc1429c9 100755
--- a/lib/python/qmk/cli/compile.py
+++ b/lib/python/qmk/cli/compile.py
@@ -7,6 +7,7 @@
from milc import cli
import qmk.path
+from qmk.constants import QMK_FIRMWARE
from qmk.decorators import automagic_keyboard, automagic_keymap
from qmk.commands import build_environment
from qmk.keyboard import keyboard_completer, keyboard_folder_or_all, is_all_keyboards
@@ -60,6 +61,9 @@ def compile(cli):
# Handler for the build target
target = None
+ current_keyboard = None
+ current_keymap = None
+
if cli.args.filename:
# if we were given a filename, assume we have a json build target
target = JsonKeymapBuildTarget(cli.args.filename)
diff --git a/lib/python/qmk/keyboard.py b/lib/python/qmk/keyboard.py
index b56505d649ca..6851684ee42a 100644
--- a/lib/python/qmk/keyboard.py
+++ b/lib/python/qmk/keyboard.py
@@ -54,6 +54,20 @@
}
+class AllKeyboards:
+ """Represents all keyboards.
+ """
+ def __str__(self):
+ return 'all'
+
+ def __repr__(self):
+ return 'all'
+
+ def __eq__(self, other):
+ return isinstance(other, AllKeyboards)
+
+
+
class AllKeyboards:
"""Represents all keyboards.
"""
diff --git a/quantum/led_matrix/led_matrix.h b/quantum/led_matrix/led_matrix.h
index c903a230f470..ed3afafe8093 100644
--- a/quantum/led_matrix/led_matrix.h
+++ b/quantum/led_matrix/led_matrix.h
@@ -88,6 +88,7 @@
#ifndef LED_MATRIX_LED_PROCESS_LIMIT
# define LED_MATRIX_LED_PROCESS_LIMIT ((LED_MATRIX_LED_COUNT + 4) / 5)
#endif
+#define LED_MATRIX_LED_PROCESS_MAX_ITERATIONS ((LED_MATRIX_LED_COUNT + LED_MATRIX_LED_PROCESS_LIMIT - 1) / LED_MATRIX_LED_PROCESS_LIMIT)
struct led_matrix_limits_t {
uint8_t led_min_index;
diff --git a/quantum/painter/qp.c b/quantum/painter/qp.c
index 375986650938..cb17ba101e77 100644
--- a/quantum/painter/qp.c
+++ b/quantum/painter/qp.c
@@ -225,7 +225,7 @@ uint16_t qp_get_offset_y(painter_device_t device) {
}
void qp_get_geometry(painter_device_t device, uint16_t *width, uint16_t *height, painter_rotation_t *rotation, uint16_t *offset_x, uint16_t *offset_y) {
- qp_dprintf("qp_geometry: entry\n");
+ qp_dprintf("qp_get_geometry: entry\n");
painter_driver_t *driver = (painter_driver_t *)device;
if (!driver || !driver->validate_ok) {
diff --git a/quantum/rgb_matrix/rgb_matrix.h b/quantum/rgb_matrix/rgb_matrix.h
index 9a3ffb8ea3a2..581ccbbf424e 100644
--- a/quantum/rgb_matrix/rgb_matrix.h
+++ b/quantum/rgb_matrix/rgb_matrix.h
@@ -106,6 +106,7 @@
#ifndef RGB_MATRIX_LED_PROCESS_LIMIT
# define RGB_MATRIX_LED_PROCESS_LIMIT ((RGB_MATRIX_LED_COUNT + 4) / 5)
#endif
+#define RGB_MATRIX_LED_PROCESS_MAX_ITERATIONS ((RGB_MATRIX_LED_COUNT + RGB_MATRIX_LED_PROCESS_LIMIT - 1) / RGB_MATRIX_LED_PROCESS_LIMIT)
struct rgb_matrix_limits_t {
uint8_t led_min_index;
diff --git a/tests/auto_shift/auto_shift_repeat/auto_shift_no_auto_repeat/config.h b/tests/auto_shift/auto_shift_repeat/auto_shift_no_auto_repeat/config.h
index 973e04582fda..f6d544dd9003 100644
--- a/tests/auto_shift/auto_shift_repeat/auto_shift_no_auto_repeat/config.h
+++ b/tests/auto_shift/auto_shift_repeat/auto_shift_no_auto_repeat/config.h
@@ -14,6 +14,8 @@
* along with this program. If not, see .
*/
+
+
#pragma once
#include "test_common.h"
diff --git a/tests/auto_shift/retro_shift/tap_hold_configurations/hold_on_other_key_press/config.h b/tests/auto_shift/retro_shift/tap_hold_configurations/hold_on_other_key_press/config.h
index 396683963d36..e946a26bdce6 100644
--- a/tests/auto_shift/retro_shift/tap_hold_configurations/hold_on_other_key_press/config.h
+++ b/tests/auto_shift/retro_shift/tap_hold_configurations/hold_on_other_key_press/config.h
@@ -13,7 +13,7 @@
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
-
+
#pragma once
#include "test_common.h"